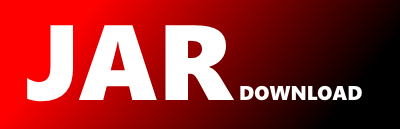
com.amazonaws.services.rds.AmazonRDSClient Maven / Gradle / Ivy
Show all versions of aws-java-sdk-rds Show documentation
/*
* Copyright 2016-2021 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.rds;
import org.w3c.dom.*;
import java.net.*;
import java.util.*;
import javax.annotation.Generated;
import org.apache.commons.logging.*;
import com.amazonaws.*;
import com.amazonaws.annotation.SdkInternalApi;
import com.amazonaws.auth.*;
import com.amazonaws.handlers.*;
import com.amazonaws.http.*;
import com.amazonaws.internal.*;
import com.amazonaws.internal.auth.*;
import com.amazonaws.metrics.*;
import com.amazonaws.regions.*;
import com.amazonaws.transform.*;
import com.amazonaws.util.*;
import com.amazonaws.protocol.json.*;
import com.amazonaws.util.AWSRequestMetrics.Field;
import com.amazonaws.annotation.ThreadSafe;
import com.amazonaws.client.AwsSyncClientParams;
import com.amazonaws.client.builder.AdvancedConfig;
import com.amazonaws.services.rds.AmazonRDSClientBuilder;
import com.amazonaws.services.rds.waiters.AmazonRDSWaiters;
import com.amazonaws.AmazonServiceException;
import com.amazonaws.services.rds.model.*;
import com.amazonaws.services.rds.model.transform.*;
/**
* Client for accessing Amazon RDS. All service calls made using this client are blocking, and will not return until the
* service call completes.
*
* Amazon Relational Database Service
*
*
*
* Amazon Relational Database Service (Amazon RDS) is a web service that makes it easier to set up, operate, and scale a
* relational database in the cloud. It provides cost-efficient, resizeable capacity for an industry-standard relational
* database and manages common database administration tasks, freeing up developers to focus on what makes their
* applications and businesses unique.
*
*
* Amazon RDS gives you access to the capabilities of a MySQL, MariaDB, PostgreSQL, Microsoft SQL Server, Oracle, or
* Amazon Aurora database server. These capabilities mean that the code, applications, and tools you already use today
* with your existing databases work with Amazon RDS without modification. Amazon RDS automatically backs up your
* database and maintains the database software that powers your DB instance. Amazon RDS is flexible: you can scale your
* DB instance's compute resources and storage capacity to meet your application's demand. As with all Amazon Web
* Services, there are no up-front investments, and you pay only for the resources you use.
*
*
* This interface reference for Amazon RDS contains documentation for a programming or command line interface you can
* use to manage Amazon RDS. Amazon RDS is asynchronous, which means that some interfaces might require techniques such
* as polling or callback functions to determine when a command has been applied. In this reference, the parameter
* descriptions indicate whether a command is applied immediately, on the next instance reboot, or during the
* maintenance window. The reference structure is as follows, and we list following some related topics from the user
* guide.
*
*
* Amazon RDS API Reference
*
*
* -
*
* For the alphabetical list of API actions, see API Actions.
*
*
* -
*
* For the alphabetical list of data types, see Data Types.
*
*
* -
*
* For a list of common query parameters, see Common Parameters.
*
*
* -
*
* For descriptions of the error codes, see Common Errors.
*
*
*
*
* Amazon RDS User Guide
*
*
* -
*
* For a summary of the Amazon RDS interfaces, see Available RDS
* Interfaces.
*
*
* -
*
* For more information about how to use the Query API, see Using the Query API.
*
*
*
*/
@ThreadSafe
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class AmazonRDSClient extends AmazonWebServiceClient implements AmazonRDS {
/** Provider for AWS credentials. */
private final AWSCredentialsProvider awsCredentialsProvider;
private static final Log log = LogFactory.getLog(AmazonRDS.class);
/** Default signing name for the service. */
private static final String DEFAULT_SIGNING_NAME = "rds";
private volatile AmazonRDSWaiters waiters;
/** Client configuration factory providing ClientConfigurations tailored to this client */
protected static final ClientConfigurationFactory configFactory = new ClientConfigurationFactory();
private final AdvancedConfig advancedConfig;
/**
* List of exception unmarshallers for all modeled exceptions
*/
protected final List> exceptionUnmarshallers = new ArrayList>();
/**
* Constructs a new client to invoke service methods on Amazon RDS. A credentials provider chain will be used that
* searches for credentials in this order:
*
* - Environment Variables - AWS_ACCESS_KEY_ID and AWS_SECRET_KEY
* - Java System Properties - aws.accessKeyId and aws.secretKey
* - Instance profile credentials delivered through the Amazon EC2 metadata service
*
*
*
* All service calls made using this new client object are blocking, and will not return until the service call
* completes.
*
* @see DefaultAWSCredentialsProviderChain
* @deprecated use {@link AmazonRDSClientBuilder#defaultClient()}
*/
@Deprecated
public AmazonRDSClient() {
this(DefaultAWSCredentialsProviderChain.getInstance(), configFactory.getConfig());
}
/**
* Constructs a new client to invoke service methods on Amazon RDS. A credentials provider chain will be used that
* searches for credentials in this order:
*
* - Environment Variables - AWS_ACCESS_KEY_ID and AWS_SECRET_KEY
* - Java System Properties - aws.accessKeyId and aws.secretKey
* - Instance profile credentials delivered through the Amazon EC2 metadata service
*
*
*
* All service calls made using this new client object are blocking, and will not return until the service call
* completes.
*
* @param clientConfiguration
* The client configuration options controlling how this client connects to Amazon RDS (ex: proxy settings,
* retry counts, etc.).
*
* @see DefaultAWSCredentialsProviderChain
* @deprecated use {@link AmazonRDSClientBuilder#withClientConfiguration(ClientConfiguration)}
*/
@Deprecated
public AmazonRDSClient(ClientConfiguration clientConfiguration) {
this(DefaultAWSCredentialsProviderChain.getInstance(), clientConfiguration);
}
/**
* Constructs a new client to invoke service methods on Amazon RDS using the specified AWS account credentials.
*
*
* All service calls made using this new client object are blocking, and will not return until the service call
* completes.
*
* @param awsCredentials
* The AWS credentials (access key ID and secret key) to use when authenticating with AWS services.
* @deprecated use {@link AmazonRDSClientBuilder#withCredentials(AWSCredentialsProvider)} for example:
* {@code AmazonRDSClientBuilder.standard().withCredentials(new AWSStaticCredentialsProvider(awsCredentials)).build();}
*/
@Deprecated
public AmazonRDSClient(AWSCredentials awsCredentials) {
this(awsCredentials, configFactory.getConfig());
}
/**
* Constructs a new client to invoke service methods on Amazon RDS using the specified AWS account credentials and
* client configuration options.
*
*
* All service calls made using this new client object are blocking, and will not return until the service call
* completes.
*
* @param awsCredentials
* The AWS credentials (access key ID and secret key) to use when authenticating with AWS services.
* @param clientConfiguration
* The client configuration options controlling how this client connects to Amazon RDS (ex: proxy settings,
* retry counts, etc.).
* @deprecated use {@link AmazonRDSClientBuilder#withCredentials(AWSCredentialsProvider)} and
* {@link AmazonRDSClientBuilder#withClientConfiguration(ClientConfiguration)}
*/
@Deprecated
public AmazonRDSClient(AWSCredentials awsCredentials, ClientConfiguration clientConfiguration) {
super(clientConfiguration);
this.awsCredentialsProvider = new StaticCredentialsProvider(awsCredentials);
this.advancedConfig = AdvancedConfig.EMPTY;
init();
}
/**
* Constructs a new client to invoke service methods on Amazon RDS using the specified AWS account credentials
* provider.
*
*
* All service calls made using this new client object are blocking, and will not return until the service call
* completes.
*
* @param awsCredentialsProvider
* The AWS credentials provider which will provide credentials to authenticate requests with AWS services.
* @deprecated use {@link AmazonRDSClientBuilder#withCredentials(AWSCredentialsProvider)}
*/
@Deprecated
public AmazonRDSClient(AWSCredentialsProvider awsCredentialsProvider) {
this(awsCredentialsProvider, configFactory.getConfig());
}
/**
* Constructs a new client to invoke service methods on Amazon RDS using the specified AWS account credentials
* provider and client configuration options.
*
*
* All service calls made using this new client object are blocking, and will not return until the service call
* completes.
*
* @param awsCredentialsProvider
* The AWS credentials provider which will provide credentials to authenticate requests with AWS services.
* @param clientConfiguration
* The client configuration options controlling how this client connects to Amazon RDS (ex: proxy settings,
* retry counts, etc.).
* @deprecated use {@link AmazonRDSClientBuilder#withCredentials(AWSCredentialsProvider)} and
* {@link AmazonRDSClientBuilder#withClientConfiguration(ClientConfiguration)}
*/
@Deprecated
public AmazonRDSClient(AWSCredentialsProvider awsCredentialsProvider, ClientConfiguration clientConfiguration) {
this(awsCredentialsProvider, clientConfiguration, null);
}
/**
* Constructs a new client to invoke service methods on Amazon RDS using the specified AWS account credentials
* provider, client configuration options, and request metric collector.
*
*
* All service calls made using this new client object are blocking, and will not return until the service call
* completes.
*
* @param awsCredentialsProvider
* The AWS credentials provider which will provide credentials to authenticate requests with AWS services.
* @param clientConfiguration
* The client configuration options controlling how this client connects to Amazon RDS (ex: proxy settings,
* retry counts, etc.).
* @param requestMetricCollector
* optional request metric collector
* @deprecated use {@link AmazonRDSClientBuilder#withCredentials(AWSCredentialsProvider)} and
* {@link AmazonRDSClientBuilder#withClientConfiguration(ClientConfiguration)} and
* {@link AmazonRDSClientBuilder#withMetricsCollector(RequestMetricCollector)}
*/
@Deprecated
public AmazonRDSClient(AWSCredentialsProvider awsCredentialsProvider, ClientConfiguration clientConfiguration, RequestMetricCollector requestMetricCollector) {
super(clientConfiguration, requestMetricCollector);
this.awsCredentialsProvider = awsCredentialsProvider;
this.advancedConfig = AdvancedConfig.EMPTY;
init();
}
public static AmazonRDSClientBuilder builder() {
return AmazonRDSClientBuilder.standard();
}
/**
* Constructs a new client to invoke service methods on Amazon RDS using the specified parameters.
*
*
* All service calls made using this new client object are blocking, and will not return until the service call
* completes.
*
* @param clientParams
* Object providing client parameters.
*/
AmazonRDSClient(AwsSyncClientParams clientParams) {
this(clientParams, false);
}
/**
* Constructs a new client to invoke service methods on Amazon RDS using the specified parameters.
*
*
* All service calls made using this new client object are blocking, and will not return until the service call
* completes.
*
* @param clientParams
* Object providing client parameters.
*/
AmazonRDSClient(AwsSyncClientParams clientParams, boolean endpointDiscoveryEnabled) {
super(clientParams);
this.awsCredentialsProvider = clientParams.getCredentialsProvider();
this.advancedConfig = clientParams.getAdvancedConfig();
init();
}
private void init() {
exceptionUnmarshallers.add(new IamRoleNotFoundExceptionUnmarshaller());
exceptionUnmarshallers.add(new DBClusterRoleNotFoundExceptionUnmarshaller());
exceptionUnmarshallers.add(new InstallationMediaAlreadyExistsExceptionUnmarshaller());
exceptionUnmarshallers.add(new ExportTaskNotFoundExceptionUnmarshaller());
exceptionUnmarshallers.add(new InvalidRestoreExceptionUnmarshaller());
exceptionUnmarshallers.add(new InvalidExportOnlyExceptionUnmarshaller());
exceptionUnmarshallers.add(new DBInstanceAlreadyExistsExceptionUnmarshaller());
exceptionUnmarshallers.add(new DBSnapshotAlreadyExistsExceptionUnmarshaller());
exceptionUnmarshallers.add(new DBParameterGroupAlreadyExistsExceptionUnmarshaller());
exceptionUnmarshallers.add(new DBClusterBacktrackNotFoundExceptionUnmarshaller());
exceptionUnmarshallers.add(new DBLogFileNotFoundExceptionUnmarshaller());
exceptionUnmarshallers.add(new InvalidOptionGroupStateExceptionUnmarshaller());
exceptionUnmarshallers.add(new DBSecurityGroupNotSupportedExceptionUnmarshaller());
exceptionUnmarshallers.add(new InvalidDBSubnetStateExceptionUnmarshaller());
exceptionUnmarshallers.add(new InvalidDBSecurityGroupStateExceptionUnmarshaller());
exceptionUnmarshallers.add(new DBSubnetQuotaExceededExceptionUnmarshaller());
exceptionUnmarshallers.add(new BackupPolicyNotFoundExceptionUnmarshaller());
exceptionUnmarshallers.add(new DBSecurityGroupNotFoundExceptionUnmarshaller());
exceptionUnmarshallers.add(new CustomDBEngineVersionNotFoundExceptionUnmarshaller());
exceptionUnmarshallers.add(new InsufficientDBInstanceCapacityExceptionUnmarshaller());
exceptionUnmarshallers.add(new DBUpgradeDependencyFailureExceptionUnmarshaller());
exceptionUnmarshallers.add(new DBClusterEndpointNotFoundExceptionUnmarshaller());
exceptionUnmarshallers.add(new InsufficientStorageClusterCapacityExceptionUnmarshaller());
exceptionUnmarshallers.add(new ProvisionedIopsNotAvailableInAZExceptionUnmarshaller());
exceptionUnmarshallers.add(new ReservedDBInstanceAlreadyExistsExceptionUnmarshaller());
exceptionUnmarshallers.add(new PointInTimeRestoreNotEnabledExceptionUnmarshaller());
exceptionUnmarshallers.add(new InstallationMediaNotFoundExceptionUnmarshaller());
exceptionUnmarshallers.add(new DBParameterGroupNotFoundExceptionUnmarshaller());
exceptionUnmarshallers.add(new InvalidDBInstanceAutomatedBackupStateExceptionUnmarshaller());
exceptionUnmarshallers.add(new CustomAvailabilityZoneNotFoundExceptionUnmarshaller());
exceptionUnmarshallers.add(new CustomDBEngineVersionAlreadyExistsExceptionUnmarshaller());
exceptionUnmarshallers.add(new DBSubnetGroupDoesNotCoverEnoughAZsExceptionUnmarshaller());
exceptionUnmarshallers.add(new DBSubnetGroupNotFoundExceptionUnmarshaller());
exceptionUnmarshallers.add(new DBProxyNotFoundExceptionUnmarshaller());
exceptionUnmarshallers.add(new DBClusterEndpointQuotaExceededExceptionUnmarshaller());
exceptionUnmarshallers.add(new AuthorizationQuotaExceededExceptionUnmarshaller());
exceptionUnmarshallers.add(new InstanceQuotaExceededExceptionUnmarshaller());
exceptionUnmarshallers.add(new DBSecurityGroupQuotaExceededExceptionUnmarshaller());
exceptionUnmarshallers.add(new DBInstanceAutomatedBackupQuotaExceededExceptionUnmarshaller());
exceptionUnmarshallers.add(new SnapshotQuotaExceededExceptionUnmarshaller());
exceptionUnmarshallers.add(new GlobalClusterQuotaExceededExceptionUnmarshaller());
exceptionUnmarshallers.add(new DBInstanceNotFoundExceptionUnmarshaller());
exceptionUnmarshallers.add(new DBProxyAlreadyExistsExceptionUnmarshaller());
exceptionUnmarshallers.add(new DBProxyTargetAlreadyRegisteredExceptionUnmarshaller());
exceptionUnmarshallers.add(new SharedSnapshotQuotaExceededExceptionUnmarshaller());
exceptionUnmarshallers.add(new DBInstanceRoleQuotaExceededExceptionUnmarshaller());
exceptionUnmarshallers.add(new DBInstanceAutomatedBackupNotFoundExceptionUnmarshaller());
exceptionUnmarshallers.add(new ReservedDBInstanceQuotaExceededExceptionUnmarshaller());
exceptionUnmarshallers.add(new DBInstanceRoleNotFoundExceptionUnmarshaller());
exceptionUnmarshallers.add(new InvalidVPCNetworkStateExceptionUnmarshaller());
exceptionUnmarshallers.add(new SNSTopicArnNotFoundExceptionUnmarshaller());
exceptionUnmarshallers.add(new InvalidDBSubnetGroupExceptionUnmarshaller());
exceptionUnmarshallers.add(new CustomAvailabilityZoneQuotaExceededExceptionUnmarshaller());
exceptionUnmarshallers.add(new OptionGroupNotFoundExceptionUnmarshaller());
exceptionUnmarshallers.add(new InvalidDBClusterSnapshotStateExceptionUnmarshaller());
exceptionUnmarshallers.add(new ReservedDBInstancesOfferingNotFoundExceptionUnmarshaller());
exceptionUnmarshallers.add(new InvalidDBParameterGroupStateExceptionUnmarshaller());
exceptionUnmarshallers.add(new DomainNotFoundExceptionUnmarshaller());
exceptionUnmarshallers.add(new StorageQuotaExceededExceptionUnmarshaller());
exceptionUnmarshallers.add(new InvalidCustomDBEngineVersionStateExceptionUnmarshaller());
exceptionUnmarshallers.add(new DBProxyEndpointAlreadyExistsExceptionUnmarshaller());
exceptionUnmarshallers.add(new AuthorizationNotFoundExceptionUnmarshaller());
exceptionUnmarshallers.add(new OptionGroupAlreadyExistsExceptionUnmarshaller());
exceptionUnmarshallers.add(new OptionGroupQuotaExceededExceptionUnmarshaller());
exceptionUnmarshallers.add(new InvalidExportSourceStateExceptionUnmarshaller());
exceptionUnmarshallers.add(new DBSnapshotNotFoundExceptionUnmarshaller());
exceptionUnmarshallers.add(new SubscriptionCategoryNotFoundExceptionUnmarshaller());
exceptionUnmarshallers.add(new DBProxyTargetGroupNotFoundExceptionUnmarshaller());
exceptionUnmarshallers.add(new InvalidDBProxyEndpointStateExceptionUnmarshaller());
exceptionUnmarshallers.add(new CustomAvailabilityZoneAlreadyExistsExceptionUnmarshaller());
exceptionUnmarshallers.add(new DBClusterRoleQuotaExceededExceptionUnmarshaller());
exceptionUnmarshallers.add(new SNSInvalidTopicExceptionUnmarshaller());
exceptionUnmarshallers.add(new InvalidDBInstanceStateExceptionUnmarshaller());
exceptionUnmarshallers.add(new InvalidDBSubnetGroupStateExceptionUnmarshaller());
exceptionUnmarshallers.add(new InsufficientDBClusterCapacityExceptionUnmarshaller());
exceptionUnmarshallers.add(new DBSubnetGroupNotAllowedExceptionUnmarshaller());
exceptionUnmarshallers.add(new CertificateNotFoundExceptionUnmarshaller());
exceptionUnmarshallers.add(new InvalidDBSnapshotStateExceptionUnmarshaller());
exceptionUnmarshallers.add(new InvalidExportTaskStateExceptionUnmarshaller());
exceptionUnmarshallers.add(new InvalidS3BucketExceptionUnmarshaller());
exceptionUnmarshallers.add(new ReservedDBInstanceNotFoundExceptionUnmarshaller());
exceptionUnmarshallers.add(new DBSubnetGroupQuotaExceededExceptionUnmarshaller());
exceptionUnmarshallers.add(new ResourceNotFoundExceptionUnmarshaller());
exceptionUnmarshallers.add(new StorageTypeNotSupportedExceptionUnmarshaller());
exceptionUnmarshallers.add(new KMSKeyNotAccessibleExceptionUnmarshaller());
exceptionUnmarshallers.add(new SubscriptionNotFoundExceptionUnmarshaller());
exceptionUnmarshallers.add(new DBClusterQuotaExceededExceptionUnmarshaller());
exceptionUnmarshallers.add(new InvalidDBClusterCapacityExceptionUnmarshaller());
exceptionUnmarshallers.add(new SubnetAlreadyInUseExceptionUnmarshaller());
exceptionUnmarshallers.add(new DBClusterSnapshotAlreadyExistsExceptionUnmarshaller());
exceptionUnmarshallers.add(new DBClusterRoleAlreadyExistsExceptionUnmarshaller());
exceptionUnmarshallers.add(new GlobalClusterNotFoundExceptionUnmarshaller());
exceptionUnmarshallers.add(new InvalidEventSubscriptionStateExceptionUnmarshaller());
exceptionUnmarshallers.add(new InsufficientAvailableIPsInSubnetExceptionUnmarshaller());
exceptionUnmarshallers.add(new InvalidSubnetExceptionUnmarshaller());
exceptionUnmarshallers.add(new DBSubnetGroupAlreadyExistsExceptionUnmarshaller());
exceptionUnmarshallers.add(new SNSNoAuthorizationExceptionUnmarshaller());
exceptionUnmarshallers.add(new DBClusterSnapshotNotFoundExceptionUnmarshaller());
exceptionUnmarshallers.add(new DBProxyEndpointQuotaExceededExceptionUnmarshaller());
exceptionUnmarshallers.add(new CustomDBEngineVersionQuotaExceededExceptionUnmarshaller());
exceptionUnmarshallers.add(new DBClusterParameterGroupNotFoundExceptionUnmarshaller());
exceptionUnmarshallers.add(new DBProxyQuotaExceededExceptionUnmarshaller());
exceptionUnmarshallers.add(new ExportTaskAlreadyExistsExceptionUnmarshaller());
exceptionUnmarshallers.add(new GlobalClusterAlreadyExistsExceptionUnmarshaller());
exceptionUnmarshallers.add(new InvalidDBClusterEndpointStateExceptionUnmarshaller());
exceptionUnmarshallers.add(new InvalidGlobalClusterStateExceptionUnmarshaller());
exceptionUnmarshallers.add(new IamRoleMissingPermissionsExceptionUnmarshaller());
exceptionUnmarshallers.add(new InvalidDBClusterStateExceptionUnmarshaller());
exceptionUnmarshallers.add(new DBClusterAlreadyExistsExceptionUnmarshaller());
exceptionUnmarshallers.add(new InvalidDBProxyStateExceptionUnmarshaller());
exceptionUnmarshallers.add(new DBProxyTargetNotFoundExceptionUnmarshaller());
exceptionUnmarshallers.add(new SubscriptionAlreadyExistExceptionUnmarshaller());
exceptionUnmarshallers.add(new DBClusterNotFoundExceptionUnmarshaller());
exceptionUnmarshallers.add(new DBParameterGroupQuotaExceededExceptionUnmarshaller());
exceptionUnmarshallers.add(new DBInstanceRoleAlreadyExistsExceptionUnmarshaller());
exceptionUnmarshallers.add(new DBProxyEndpointNotFoundExceptionUnmarshaller());
exceptionUnmarshallers.add(new DBSecurityGroupAlreadyExistsExceptionUnmarshaller());
exceptionUnmarshallers.add(new SourceNotFoundExceptionUnmarshaller());
exceptionUnmarshallers.add(new EventSubscriptionQuotaExceededExceptionUnmarshaller());
exceptionUnmarshallers.add(new AuthorizationAlreadyExistsExceptionUnmarshaller());
exceptionUnmarshallers.add(new DBClusterEndpointAlreadyExistsExceptionUnmarshaller());
exceptionUnmarshallers.add(new StandardErrorUnmarshaller(com.amazonaws.services.rds.model.AmazonRDSException.class));
setServiceNameIntern(DEFAULT_SIGNING_NAME);
setEndpointPrefix(ENDPOINT_PREFIX);
// calling this.setEndPoint(...) will also modify the signer accordingly
this.setEndpoint("rds.amazonaws.com");
HandlerChainFactory chainFactory = new HandlerChainFactory();
requestHandler2s.addAll(chainFactory.newRequestHandlerChain("/com/amazonaws/services/rds/request.handlers"));
requestHandler2s.addAll(chainFactory.newRequestHandler2Chain("/com/amazonaws/services/rds/request.handler2s"));
requestHandler2s.addAll(chainFactory.getGlobalHandlers());
}
/**
*
* Associates an Identity and Access Management (IAM) role from an Amazon Aurora DB cluster. For more information,
* see Authorizing Amazon Aurora MySQL to Access Other Amazon Web Services Services on Your Behalf in the Amazon
* Aurora User Guide.
*
*
*
* This action only applies to Aurora DB clusters.
*
*
*
* @param addRoleToDBClusterRequest
* @return Result of the AddRoleToDBCluster operation returned by the service.
* @throws DBClusterNotFoundException
* DBClusterIdentifier
doesn't refer to an existing DB cluster.
* @throws DBClusterRoleAlreadyExistsException
* The specified IAM role Amazon Resource Name (ARN) is already associated with the specified DB cluster.
* @throws InvalidDBClusterStateException
* The requested operation can't be performed while the cluster is in this state.
* @throws DBClusterRoleQuotaExceededException
* You have exceeded the maximum number of IAM roles that can be associated with the specified DB cluster.
* @sample AmazonRDS.AddRoleToDBCluster
* @see AWS API
* Documentation
*/
@Override
public AddRoleToDBClusterResult addRoleToDBCluster(AddRoleToDBClusterRequest request) {
request = beforeClientExecution(request);
return executeAddRoleToDBCluster(request);
}
@SdkInternalApi
final AddRoleToDBClusterResult executeAddRoleToDBCluster(AddRoleToDBClusterRequest addRoleToDBClusterRequest) {
ExecutionContext executionContext = createExecutionContext(addRoleToDBClusterRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new AddRoleToDBClusterRequestMarshaller().marshall(super.beforeMarshalling(addRoleToDBClusterRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "RDS");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "AddRoleToDBCluster");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
StaxResponseHandler responseHandler = new StaxResponseHandler(
new AddRoleToDBClusterResultStaxUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Associates an Amazon Web Services Identity and Access Management (IAM) role with a DB instance.
*
*
*
* To add a role to a DB instance, the status of the DB instance must be available
.
*
*
*
* This command doesn't apply to RDS Custom.
*
*
* @param addRoleToDBInstanceRequest
* @return Result of the AddRoleToDBInstance operation returned by the service.
* @throws DBInstanceNotFoundException
* DBInstanceIdentifier
doesn't refer to an existing DB instance.
* @throws DBInstanceRoleAlreadyExistsException
* The specified RoleArn
or FeatureName
value is already associated with the DB
* instance.
* @throws InvalidDBInstanceStateException
* The DB instance isn't in a valid state.
* @throws DBInstanceRoleQuotaExceededException
* You can't associate any more Amazon Web Services Identity and Access Management (IAM) roles with the DB
* instance because the quota has been reached.
* @sample AmazonRDS.AddRoleToDBInstance
* @see AWS API
* Documentation
*/
@Override
public AddRoleToDBInstanceResult addRoleToDBInstance(AddRoleToDBInstanceRequest request) {
request = beforeClientExecution(request);
return executeAddRoleToDBInstance(request);
}
@SdkInternalApi
final AddRoleToDBInstanceResult executeAddRoleToDBInstance(AddRoleToDBInstanceRequest addRoleToDBInstanceRequest) {
ExecutionContext executionContext = createExecutionContext(addRoleToDBInstanceRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new AddRoleToDBInstanceRequestMarshaller().marshall(super.beforeMarshalling(addRoleToDBInstanceRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "RDS");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "AddRoleToDBInstance");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
StaxResponseHandler responseHandler = new StaxResponseHandler(
new AddRoleToDBInstanceResultStaxUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Adds a source identifier to an existing RDS event notification subscription.
*
*
* @param addSourceIdentifierToSubscriptionRequest
* @return Result of the AddSourceIdentifierToSubscription operation returned by the service.
* @throws SubscriptionNotFoundException
* The subscription name does not exist.
* @throws SourceNotFoundException
* The requested source could not be found.
* @sample AmazonRDS.AddSourceIdentifierToSubscription
* @see AWS API Documentation
*/
@Override
public EventSubscription addSourceIdentifierToSubscription(AddSourceIdentifierToSubscriptionRequest request) {
request = beforeClientExecution(request);
return executeAddSourceIdentifierToSubscription(request);
}
@SdkInternalApi
final EventSubscription executeAddSourceIdentifierToSubscription(AddSourceIdentifierToSubscriptionRequest addSourceIdentifierToSubscriptionRequest) {
ExecutionContext executionContext = createExecutionContext(addSourceIdentifierToSubscriptionRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new AddSourceIdentifierToSubscriptionRequestMarshaller().marshall(super.beforeMarshalling(addSourceIdentifierToSubscriptionRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "RDS");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "AddSourceIdentifierToSubscription");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
StaxResponseHandler responseHandler = new StaxResponseHandler(new EventSubscriptionStaxUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Adds metadata tags to an Amazon RDS resource. These tags can also be used with cost allocation reporting to track
* cost associated with Amazon RDS resources, or used in a Condition statement in an IAM policy for Amazon RDS.
*
*
* For an overview on tagging Amazon RDS resources, see Tagging Amazon RDS
* Resources.
*
*
* @param addTagsToResourceRequest
* @return Result of the AddTagsToResource operation returned by the service.
* @throws DBInstanceNotFoundException
* DBInstanceIdentifier
doesn't refer to an existing DB instance.
* @throws DBClusterNotFoundException
* DBClusterIdentifier
doesn't refer to an existing DB cluster.
* @throws DBSnapshotNotFoundException
* DBSnapshotIdentifier
doesn't refer to an existing DB snapshot.
* @throws DBProxyNotFoundException
* The specified proxy name doesn't correspond to a proxy owned by your Amazon Web Services account in the
* specified Amazon Web Services Region.
* @throws DBProxyTargetGroupNotFoundException
* The specified target group isn't available for a proxy owned by your Amazon Web Services account in the
* specified Amazon Web Services Region.
* @sample AmazonRDS.AddTagsToResource
* @see AWS API
* Documentation
*/
@Override
public AddTagsToResourceResult addTagsToResource(AddTagsToResourceRequest request) {
request = beforeClientExecution(request);
return executeAddTagsToResource(request);
}
@SdkInternalApi
final AddTagsToResourceResult executeAddTagsToResource(AddTagsToResourceRequest addTagsToResourceRequest) {
ExecutionContext executionContext = createExecutionContext(addTagsToResourceRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new AddTagsToResourceRequestMarshaller().marshall(super.beforeMarshalling(addTagsToResourceRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "RDS");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "AddTagsToResource");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
StaxResponseHandler responseHandler = new StaxResponseHandler(
new AddTagsToResourceResultStaxUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Applies a pending maintenance action to a resource (for example, to a DB instance).
*
*
* @param applyPendingMaintenanceActionRequest
* @return Result of the ApplyPendingMaintenanceAction operation returned by the service.
* @throws ResourceNotFoundException
* The specified resource ID was not found.
* @throws InvalidDBClusterStateException
* The requested operation can't be performed while the cluster is in this state.
* @throws InvalidDBInstanceStateException
* The DB instance isn't in a valid state.
* @sample AmazonRDS.ApplyPendingMaintenanceAction
* @see AWS API Documentation
*/
@Override
public ResourcePendingMaintenanceActions applyPendingMaintenanceAction(ApplyPendingMaintenanceActionRequest request) {
request = beforeClientExecution(request);
return executeApplyPendingMaintenanceAction(request);
}
@SdkInternalApi
final ResourcePendingMaintenanceActions executeApplyPendingMaintenanceAction(ApplyPendingMaintenanceActionRequest applyPendingMaintenanceActionRequest) {
ExecutionContext executionContext = createExecutionContext(applyPendingMaintenanceActionRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new ApplyPendingMaintenanceActionRequestMarshaller().marshall(super.beforeMarshalling(applyPendingMaintenanceActionRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "RDS");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "ApplyPendingMaintenanceAction");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
StaxResponseHandler responseHandler = new StaxResponseHandler(
new ResourcePendingMaintenanceActionsStaxUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Enables ingress to a DBSecurityGroup using one of two forms of authorization. First, EC2 or VPC security groups
* can be added to the DBSecurityGroup if the application using the database is running on EC2 or VPC instances.
* Second, IP ranges are available if the application accessing your database is running on the Internet. Required
* parameters for this API are one of CIDR range, EC2SecurityGroupId for VPC, or (EC2SecurityGroupOwnerId and either
* EC2SecurityGroupName or EC2SecurityGroupId for non-VPC).
*
*
*
* You can't authorize ingress from an EC2 security group in one Amazon Web Services Region to an Amazon RDS DB
* instance in another. You can't authorize ingress from a VPC security group in one VPC to an Amazon RDS DB
* instance in another.
*
*
*
* For an overview of CIDR ranges, go to the Wikipedia Tutorial.
*
*
* @param authorizeDBSecurityGroupIngressRequest
* @return Result of the AuthorizeDBSecurityGroupIngress operation returned by the service.
* @throws DBSecurityGroupNotFoundException
* DBSecurityGroupName
doesn't refer to an existing DB security group.
* @throws InvalidDBSecurityGroupStateException
* The state of the DB security group doesn't allow deletion.
* @throws AuthorizationAlreadyExistsException
* The specified CIDR IP range or Amazon EC2 security group is already authorized for the specified DB
* security group.
* @throws AuthorizationQuotaExceededException
* The DB security group authorization quota has been reached.
* @sample AmazonRDS.AuthorizeDBSecurityGroupIngress
* @see AWS API Documentation
*/
@Override
public DBSecurityGroup authorizeDBSecurityGroupIngress(AuthorizeDBSecurityGroupIngressRequest request) {
request = beforeClientExecution(request);
return executeAuthorizeDBSecurityGroupIngress(request);
}
@SdkInternalApi
final DBSecurityGroup executeAuthorizeDBSecurityGroupIngress(AuthorizeDBSecurityGroupIngressRequest authorizeDBSecurityGroupIngressRequest) {
ExecutionContext executionContext = createExecutionContext(authorizeDBSecurityGroupIngressRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new AuthorizeDBSecurityGroupIngressRequestMarshaller().marshall(super.beforeMarshalling(authorizeDBSecurityGroupIngressRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "RDS");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "AuthorizeDBSecurityGroupIngress");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
StaxResponseHandler responseHandler = new StaxResponseHandler(new DBSecurityGroupStaxUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Backtracks a DB cluster to a specific time, without creating a new DB cluster.
*
*
* For more information on backtracking, see
* Backtracking an Aurora DB Cluster in the Amazon Aurora User Guide.
*
*
*
* This action only applies to Aurora MySQL DB clusters.
*
*
*
* @param backtrackDBClusterRequest
* @return Result of the BacktrackDBCluster operation returned by the service.
* @throws DBClusterNotFoundException
* DBClusterIdentifier
doesn't refer to an existing DB cluster.
* @throws InvalidDBClusterStateException
* The requested operation can't be performed while the cluster is in this state.
* @sample AmazonRDS.BacktrackDBCluster
* @see AWS API
* Documentation
*/
@Override
public BacktrackDBClusterResult backtrackDBCluster(BacktrackDBClusterRequest request) {
request = beforeClientExecution(request);
return executeBacktrackDBCluster(request);
}
@SdkInternalApi
final BacktrackDBClusterResult executeBacktrackDBCluster(BacktrackDBClusterRequest backtrackDBClusterRequest) {
ExecutionContext executionContext = createExecutionContext(backtrackDBClusterRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new BacktrackDBClusterRequestMarshaller().marshall(super.beforeMarshalling(backtrackDBClusterRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "RDS");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "BacktrackDBCluster");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
StaxResponseHandler responseHandler = new StaxResponseHandler(
new BacktrackDBClusterResultStaxUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Cancels an export task in progress that is exporting a snapshot to Amazon S3. Any data that has already been
* written to the S3 bucket isn't removed.
*
*
* @param cancelExportTaskRequest
* @return Result of the CancelExportTask operation returned by the service.
* @throws ExportTaskNotFoundException
* The export task doesn't exist.
* @throws InvalidExportTaskStateException
* You can't cancel an export task that has completed.
* @sample AmazonRDS.CancelExportTask
* @see AWS API
* Documentation
*/
@Override
public CancelExportTaskResult cancelExportTask(CancelExportTaskRequest request) {
request = beforeClientExecution(request);
return executeCancelExportTask(request);
}
@SdkInternalApi
final CancelExportTaskResult executeCancelExportTask(CancelExportTaskRequest cancelExportTaskRequest) {
ExecutionContext executionContext = createExecutionContext(cancelExportTaskRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new CancelExportTaskRequestMarshaller().marshall(super.beforeMarshalling(cancelExportTaskRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "RDS");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "CancelExportTask");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
StaxResponseHandler responseHandler = new StaxResponseHandler(
new CancelExportTaskResultStaxUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Copies the specified DB cluster parameter group.
*
*
*
* This action only applies to Aurora DB clusters.
*
*
*
* @param copyDBClusterParameterGroupRequest
* @return Result of the CopyDBClusterParameterGroup operation returned by the service.
* @throws DBParameterGroupNotFoundException
* DBParameterGroupName
doesn't refer to an existing DB parameter group.
* @throws DBParameterGroupQuotaExceededException
* The request would result in the user exceeding the allowed number of DB parameter groups.
* @throws DBParameterGroupAlreadyExistsException
* A DB parameter group with the same name exists.
* @sample AmazonRDS.CopyDBClusterParameterGroup
* @see AWS API Documentation
*/
@Override
public DBClusterParameterGroup copyDBClusterParameterGroup(CopyDBClusterParameterGroupRequest request) {
request = beforeClientExecution(request);
return executeCopyDBClusterParameterGroup(request);
}
@SdkInternalApi
final DBClusterParameterGroup executeCopyDBClusterParameterGroup(CopyDBClusterParameterGroupRequest copyDBClusterParameterGroupRequest) {
ExecutionContext executionContext = createExecutionContext(copyDBClusterParameterGroupRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new CopyDBClusterParameterGroupRequestMarshaller().marshall(super.beforeMarshalling(copyDBClusterParameterGroupRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "RDS");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "CopyDBClusterParameterGroup");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
StaxResponseHandler responseHandler = new StaxResponseHandler(
new DBClusterParameterGroupStaxUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Copies a snapshot of a DB cluster.
*
*
* To copy a DB cluster snapshot from a shared manual DB cluster snapshot,
* SourceDBClusterSnapshotIdentifier
must be the Amazon Resource Name (ARN) of the shared DB cluster
* snapshot.
*
*
* You can copy an encrypted DB cluster snapshot from another Amazon Web Services Region. In that case, the Amazon
* Web Services Region where you call the CopyDBClusterSnapshot
action is the destination Amazon Web
* Services Region for the encrypted DB cluster snapshot to be copied to. To copy an encrypted DB cluster snapshot
* from another Amazon Web Services Region, you must provide the following values:
*
*
* -
*
* KmsKeyId
- The Amazon Web Services Key Management System (Amazon Web Services KMS) key identifier
* for the key to use to encrypt the copy of the DB cluster snapshot in the destination Amazon Web Services Region.
*
*
* -
*
* PreSignedUrl
- A URL that contains a Signature Version 4 signed request for the
* CopyDBClusterSnapshot
action to be called in the source Amazon Web Services Region where the DB
* cluster snapshot is copied from. The pre-signed URL must be a valid request for the
* CopyDBClusterSnapshot
API action that can be executed in the source Amazon Web Services Region that
* contains the encrypted DB cluster snapshot to be copied.
*
*
* The pre-signed URL request must contain the following parameter values:
*
*
* -
*
* KmsKeyId
- The Amazon Web Services KMS key identifier for the KMS key to use to encrypt the copy of
* the DB cluster snapshot in the destination Amazon Web Services Region. This is the same identifier for both the
* CopyDBClusterSnapshot
action that is called in the destination Amazon Web Services Region, and the
* action contained in the pre-signed URL.
*
*
* -
*
* DestinationRegion
- The name of the Amazon Web Services Region that the DB cluster snapshot is to be
* created in.
*
*
* -
*
* SourceDBClusterSnapshotIdentifier
- The DB cluster snapshot identifier for the encrypted DB cluster
* snapshot to be copied. This identifier must be in the Amazon Resource Name (ARN) format for the source Amazon Web
* Services Region. For example, if you are copying an encrypted DB cluster snapshot from the us-west-2 Amazon Web
* Services Region, then your SourceDBClusterSnapshotIdentifier
looks like the following example:
* arn:aws:rds:us-west-2:123456789012:cluster-snapshot:aurora-cluster1-snapshot-20161115
.
*
*
*
*
* To learn how to generate a Signature Version 4 signed request, see Authenticating Requests:
* Using Query Parameters (Amazon Web Services Signature Version 4) and Signature Version 4 Signing
* Process.
*
*
*
* If you are using an Amazon Web Services SDK tool or the CLI, you can specify SourceRegion
(or
* --source-region
for the CLI) instead of specifying PreSignedUrl
manually. Specifying
* SourceRegion
autogenerates a pre-signed URL that is a valid request for the operation that can be
* executed in the source Amazon Web Services Region.
*
*
* -
*
* TargetDBClusterSnapshotIdentifier
- The identifier for the new copy of the DB cluster snapshot in
* the destination Amazon Web Services Region.
*
*
* -
*
* SourceDBClusterSnapshotIdentifier
- The DB cluster snapshot identifier for the encrypted DB cluster
* snapshot to be copied. This identifier must be in the ARN format for the source Amazon Web Services Region and is
* the same value as the SourceDBClusterSnapshotIdentifier
in the pre-signed URL.
*
*
*
*
* To cancel the copy operation once it is in progress, delete the target DB cluster snapshot identified by
* TargetDBClusterSnapshotIdentifier
while that DB cluster snapshot is in "copying" status.
*
*
* For more information on copying encrypted DB cluster snapshots from one Amazon Web Services Region to another,
* see Copying a
* Snapshot in the Amazon Aurora User Guide.
*
*
* For more information on Amazon Aurora, see What Is Amazon
* Aurora? in the Amazon Aurora User Guide.
*
*
*
* This action only applies to Aurora DB clusters.
*
*
*
* @param copyDBClusterSnapshotRequest
* @return Result of the CopyDBClusterSnapshot operation returned by the service.
* @throws DBClusterSnapshotAlreadyExistsException
* The user already has a DB cluster snapshot with the given identifier.
* @throws DBClusterSnapshotNotFoundException
* DBClusterSnapshotIdentifier
doesn't refer to an existing DB cluster snapshot.
* @throws InvalidDBClusterStateException
* The requested operation can't be performed while the cluster is in this state.
* @throws InvalidDBClusterSnapshotStateException
* The supplied value isn't a valid DB cluster snapshot state.
* @throws SnapshotQuotaExceededException
* The request would result in the user exceeding the allowed number of DB snapshots.
* @throws KMSKeyNotAccessibleException
* An error occurred accessing an Amazon Web Services KMS key.
* @sample AmazonRDS.CopyDBClusterSnapshot
* @see AWS API
* Documentation
*/
@Override
public DBClusterSnapshot copyDBClusterSnapshot(CopyDBClusterSnapshotRequest request) {
request = beforeClientExecution(request);
return executeCopyDBClusterSnapshot(request);
}
@SdkInternalApi
final DBClusterSnapshot executeCopyDBClusterSnapshot(CopyDBClusterSnapshotRequest copyDBClusterSnapshotRequest) {
ExecutionContext executionContext = createExecutionContext(copyDBClusterSnapshotRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new CopyDBClusterSnapshotRequestMarshaller().marshall(super.beforeMarshalling(copyDBClusterSnapshotRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "RDS");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "CopyDBClusterSnapshot");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
StaxResponseHandler responseHandler = new StaxResponseHandler(new DBClusterSnapshotStaxUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Copies the specified DB parameter group.
*
*
* @param copyDBParameterGroupRequest
* @return Result of the CopyDBParameterGroup operation returned by the service.
* @throws DBParameterGroupNotFoundException
* DBParameterGroupName
doesn't refer to an existing DB parameter group.
* @throws DBParameterGroupAlreadyExistsException
* A DB parameter group with the same name exists.
* @throws DBParameterGroupQuotaExceededException
* The request would result in the user exceeding the allowed number of DB parameter groups.
* @sample AmazonRDS.CopyDBParameterGroup
* @see AWS API
* Documentation
*/
@Override
public DBParameterGroup copyDBParameterGroup(CopyDBParameterGroupRequest request) {
request = beforeClientExecution(request);
return executeCopyDBParameterGroup(request);
}
@SdkInternalApi
final DBParameterGroup executeCopyDBParameterGroup(CopyDBParameterGroupRequest copyDBParameterGroupRequest) {
ExecutionContext executionContext = createExecutionContext(copyDBParameterGroupRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new CopyDBParameterGroupRequestMarshaller().marshall(super.beforeMarshalling(copyDBParameterGroupRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "RDS");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "CopyDBParameterGroup");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
StaxResponseHandler responseHandler = new StaxResponseHandler(new DBParameterGroupStaxUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Copies the specified DB snapshot. The source DB snapshot must be in the available
state.
*
*
* You can copy a snapshot from one Amazon Web Services Region to another. In that case, the Amazon Web Services
* Region where you call the CopyDBSnapshot
action is the destination Amazon Web Services Region for
* the DB snapshot copy.
*
*
* This command doesn't apply to RDS Custom.
*
*
* For more information about copying snapshots, see Copying
* a DB Snapshot in the Amazon RDS User Guide.
*
*
* @param copyDBSnapshotRequest
* @return Result of the CopyDBSnapshot operation returned by the service.
* @throws DBSnapshotAlreadyExistsException
* DBSnapshotIdentifier
is already used by an existing snapshot.
* @throws DBSnapshotNotFoundException
* DBSnapshotIdentifier
doesn't refer to an existing DB snapshot.
* @throws InvalidDBSnapshotStateException
* The state of the DB snapshot doesn't allow deletion.
* @throws SnapshotQuotaExceededException
* The request would result in the user exceeding the allowed number of DB snapshots.
* @throws KMSKeyNotAccessibleException
* An error occurred accessing an Amazon Web Services KMS key.
* @throws CustomAvailabilityZoneNotFoundException
* CustomAvailabilityZoneId
doesn't refer to an existing custom Availability Zone identifier.
* @sample AmazonRDS.CopyDBSnapshot
* @see AWS API
* Documentation
*/
@Override
public DBSnapshot copyDBSnapshot(CopyDBSnapshotRequest request) {
request = beforeClientExecution(request);
return executeCopyDBSnapshot(request);
}
@SdkInternalApi
final DBSnapshot executeCopyDBSnapshot(CopyDBSnapshotRequest copyDBSnapshotRequest) {
ExecutionContext executionContext = createExecutionContext(copyDBSnapshotRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new CopyDBSnapshotRequestMarshaller().marshall(super.beforeMarshalling(copyDBSnapshotRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "RDS");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "CopyDBSnapshot");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
StaxResponseHandler responseHandler = new StaxResponseHandler(new DBSnapshotStaxUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Copies the specified option group.
*
*
* @param copyOptionGroupRequest
* @return Result of the CopyOptionGroup operation returned by the service.
* @throws OptionGroupAlreadyExistsException
* The option group you are trying to create already exists.
* @throws OptionGroupNotFoundException
* The specified option group could not be found.
* @throws OptionGroupQuotaExceededException
* The quota of 20 option groups was exceeded for this Amazon Web Services account.
* @sample AmazonRDS.CopyOptionGroup
* @see AWS API
* Documentation
*/
@Override
public OptionGroup copyOptionGroup(CopyOptionGroupRequest request) {
request = beforeClientExecution(request);
return executeCopyOptionGroup(request);
}
@SdkInternalApi
final OptionGroup executeCopyOptionGroup(CopyOptionGroupRequest copyOptionGroupRequest) {
ExecutionContext executionContext = createExecutionContext(copyOptionGroupRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new CopyOptionGroupRequestMarshaller().marshall(super.beforeMarshalling(copyOptionGroupRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "RDS");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "CopyOptionGroup");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
StaxResponseHandler responseHandler = new StaxResponseHandler(new OptionGroupStaxUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Creates a custom Availability Zone (AZ).
*
*
* A custom AZ is an on-premises AZ that is integrated with a VMware vSphere cluster.
*
*
* For more information about RDS on VMware, see the RDS on VMware User
* Guide.
*
*
* @param createCustomAvailabilityZoneRequest
* @return Result of the CreateCustomAvailabilityZone operation returned by the service.
* @throws CustomAvailabilityZoneAlreadyExistsException
* CustomAvailabilityZoneName
is already used by an existing custom Availability Zone.
* @throws CustomAvailabilityZoneQuotaExceededException
* You have exceeded the maximum number of custom Availability Zones.
* @throws KMSKeyNotAccessibleException
* An error occurred accessing an Amazon Web Services KMS key.
* @sample AmazonRDS.CreateCustomAvailabilityZone
* @see AWS API Documentation
*/
@Override
public CustomAvailabilityZone createCustomAvailabilityZone(CreateCustomAvailabilityZoneRequest request) {
request = beforeClientExecution(request);
return executeCreateCustomAvailabilityZone(request);
}
@SdkInternalApi
final CustomAvailabilityZone executeCreateCustomAvailabilityZone(CreateCustomAvailabilityZoneRequest createCustomAvailabilityZoneRequest) {
ExecutionContext executionContext = createExecutionContext(createCustomAvailabilityZoneRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new CreateCustomAvailabilityZoneRequestMarshaller().marshall(super.beforeMarshalling(createCustomAvailabilityZoneRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "RDS");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "CreateCustomAvailabilityZone");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
StaxResponseHandler responseHandler = new StaxResponseHandler(
new CustomAvailabilityZoneStaxUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Creates a custom DB engine version (CEV). A CEV is a binary volume snapshot of a database engine and specific
* AMI. The only supported engine is Oracle Database 19c Enterprise Edition with the January 2021 or later RU/RUR.
* For more information, see
* Amazon RDS Custom requirements and limitations in the Amazon RDS User Guide.
*
*
* Amazon RDS, which is a fully managed service, supplies the Amazon Machine Image (AMI) and database software. The
* Amazon RDS database software is preinstalled, so you need only select a DB engine and version, and create your
* database. With Amazon RDS Custom, you upload your database installation files in Amazon S3. For more information,
* see
* Preparing to create a CEV in the Amazon RDS User Guide.
*
*
* When you create a custom engine version, you specify the files in a JSON document called a CEV manifest. This
* document describes installation .zip files stored in Amazon S3. RDS Custom creates your CEV from the installation
* files that you provided. This service model is called Bring Your Own Media (BYOM).
*
*
* Creation takes approximately two hours. If creation fails, RDS Custom issues RDS-EVENT-0196
with the
* message Creation failed for custom engine version
, and includes details about the failure. For
* example, the event prints missing files.
*
*
* After you create the CEV, it is available for use. You can create multiple CEVs, and create multiple RDS Custom
* instances from any CEV. You can also change the status of a CEV to make it available or inactive.
*
*
*
* The MediaImport service that imports files from Amazon S3 to create CEVs isn't integrated with Amazon Web
* Services CloudTrail. If you turn on data logging for Amazon RDS in CloudTrail, calls to the
* CreateCustomDbEngineVersion
event aren't logged. However, you might see calls from the API gateway
* that accesses your Amazon S3 bucket. These calls originate from the MediaImport service for the
* CreateCustomDbEngineVersion
event.
*
*
*
* For more information, see Creating a
* CEV in the Amazon RDS User Guide.
*
*
* @param createCustomDBEngineVersionRequest
* @return Result of the CreateCustomDBEngineVersion operation returned by the service.
* @throws CustomDBEngineVersionAlreadyExistsException
* A CEV with the specified name already exists.
* @throws CustomDBEngineVersionQuotaExceededException
* You have exceeded your CEV quota.
* @throws KMSKeyNotAccessibleException
* An error occurred accessing an Amazon Web Services KMS key.
* @sample AmazonRDS.CreateCustomDBEngineVersion
* @see AWS API Documentation
*/
@Override
public CreateCustomDBEngineVersionResult createCustomDBEngineVersion(CreateCustomDBEngineVersionRequest request) {
request = beforeClientExecution(request);
return executeCreateCustomDBEngineVersion(request);
}
@SdkInternalApi
final CreateCustomDBEngineVersionResult executeCreateCustomDBEngineVersion(CreateCustomDBEngineVersionRequest createCustomDBEngineVersionRequest) {
ExecutionContext executionContext = createExecutionContext(createCustomDBEngineVersionRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new CreateCustomDBEngineVersionRequestMarshaller().marshall(super.beforeMarshalling(createCustomDBEngineVersionRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "RDS");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "CreateCustomDBEngineVersion");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
StaxResponseHandler responseHandler = new StaxResponseHandler(
new CreateCustomDBEngineVersionResultStaxUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Creates a new Amazon Aurora DB cluster.
*
*
* You can use the ReplicationSourceIdentifier
parameter to create the DB cluster as a read replica of
* another DB cluster or Amazon RDS MySQL or PostgreSQL DB instance. For cross-region replication where the DB
* cluster identified by ReplicationSourceIdentifier
is encrypted, you must also specify the
* PreSignedUrl
parameter.
*
*
* For more information on Amazon Aurora, see What Is Amazon
* Aurora? in the Amazon Aurora User Guide.
*
*
*
* This action only applies to Aurora DB clusters.
*
*
*
* @param createDBClusterRequest
* @return Result of the CreateDBCluster operation returned by the service.
* @throws DBClusterAlreadyExistsException
* The user already has a DB cluster with the given identifier.
* @throws InsufficientStorageClusterCapacityException
* There is insufficient storage available for the current action. You might be able to resolve this error
* by updating your subnet group to use different Availability Zones that have more storage available.
* @throws DBClusterQuotaExceededException
* The user attempted to create a new DB cluster and the user has already reached the maximum allowed DB
* cluster quota.
* @throws StorageQuotaExceededException
* The request would result in the user exceeding the allowed amount of storage available across all DB
* instances.
* @throws DBSubnetGroupNotFoundException
* DBSubnetGroupName
doesn't refer to an existing DB subnet group.
* @throws InvalidVPCNetworkStateException
* The DB subnet group doesn't cover all Availability Zones after it's created because of users' change.
* @throws InvalidDBClusterStateException
* The requested operation can't be performed while the cluster is in this state.
* @throws InvalidDBSubnetGroupStateException
* The DB subnet group cannot be deleted because it's in use.
* @throws InvalidSubnetException
* The requested subnet is invalid, or multiple subnets were requested that are not all in a common VPC.
* @throws InvalidDBInstanceStateException
* The DB instance isn't in a valid state.
* @throws DBClusterParameterGroupNotFoundException
* DBClusterParameterGroupName
doesn't refer to an existing DB cluster parameter group.
* @throws KMSKeyNotAccessibleException
* An error occurred accessing an Amazon Web Services KMS key.
* @throws DBClusterNotFoundException
* DBClusterIdentifier
doesn't refer to an existing DB cluster.
* @throws DBInstanceNotFoundException
* DBInstanceIdentifier
doesn't refer to an existing DB instance.
* @throws DBSubnetGroupDoesNotCoverEnoughAZsException
* Subnets in the DB subnet group should cover at least two Availability Zones unless there is only one
* Availability Zone.
* @throws GlobalClusterNotFoundException
* The GlobalClusterIdentifier
doesn't refer to an existing global database cluster.
* @throws InvalidGlobalClusterStateException
* The global cluster is in an invalid state and can't perform the requested operation.
* @throws DomainNotFoundException
* Domain
doesn't refer to an existing Active Directory domain.
* @sample AmazonRDS.CreateDBCluster
* @see AWS API
* Documentation
*/
@Override
public DBCluster createDBCluster(CreateDBClusterRequest request) {
request = beforeClientExecution(request);
return executeCreateDBCluster(request);
}
@SdkInternalApi
final DBCluster executeCreateDBCluster(CreateDBClusterRequest createDBClusterRequest) {
ExecutionContext executionContext = createExecutionContext(createDBClusterRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new CreateDBClusterRequestMarshaller().marshall(super.beforeMarshalling(createDBClusterRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "RDS");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "CreateDBCluster");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
StaxResponseHandler responseHandler = new StaxResponseHandler(new DBClusterStaxUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Creates a new custom endpoint and associates it with an Amazon Aurora DB cluster.
*
*
*
* This action only applies to Aurora DB clusters.
*
*
*
* @param createDBClusterEndpointRequest
* @return Result of the CreateDBClusterEndpoint operation returned by the service.
* @throws DBClusterEndpointQuotaExceededException
* The cluster already has the maximum number of custom endpoints.
* @throws DBClusterEndpointAlreadyExistsException
* The specified custom endpoint can't be created because it already exists.
* @throws DBClusterNotFoundException
* DBClusterIdentifier
doesn't refer to an existing DB cluster.
* @throws InvalidDBClusterStateException
* The requested operation can't be performed while the cluster is in this state.
* @throws DBInstanceNotFoundException
* DBInstanceIdentifier
doesn't refer to an existing DB instance.
* @throws InvalidDBInstanceStateException
* The DB instance isn't in a valid state.
* @sample AmazonRDS.CreateDBClusterEndpoint
* @see AWS
* API Documentation
*/
@Override
public CreateDBClusterEndpointResult createDBClusterEndpoint(CreateDBClusterEndpointRequest request) {
request = beforeClientExecution(request);
return executeCreateDBClusterEndpoint(request);
}
@SdkInternalApi
final CreateDBClusterEndpointResult executeCreateDBClusterEndpoint(CreateDBClusterEndpointRequest createDBClusterEndpointRequest) {
ExecutionContext executionContext = createExecutionContext(createDBClusterEndpointRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new CreateDBClusterEndpointRequestMarshaller().marshall(super.beforeMarshalling(createDBClusterEndpointRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "RDS");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "CreateDBClusterEndpoint");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
StaxResponseHandler responseHandler = new StaxResponseHandler(
new CreateDBClusterEndpointResultStaxUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Creates a new DB cluster parameter group.
*
*
* Parameters in a DB cluster parameter group apply to all of the instances in a DB cluster.
*
*
* A DB cluster parameter group is initially created with the default parameters for the database engine used by
* instances in the DB cluster. To provide custom values for any of the parameters, you must modify the group after
* creating it using ModifyDBClusterParameterGroup
. Once you've created a DB cluster parameter group,
* you need to associate it with your DB cluster using ModifyDBCluster
. When you associate a new DB
* cluster parameter group with a running DB cluster, you need to reboot the DB instances in the DB cluster without
* failover for the new DB cluster parameter group and associated settings to take effect.
*
*
*
* After you create a DB cluster parameter group, you should wait at least 5 minutes before creating your first DB
* cluster that uses that DB cluster parameter group as the default parameter group. This allows Amazon RDS to fully
* complete the create action before the DB cluster parameter group is used as the default for a new DB cluster.
* This is especially important for parameters that are critical when creating the default database for a DB
* cluster, such as the character set for the default database defined by the character_set_database
* parameter. You can use the Parameter Groups option of the Amazon RDS console or the DescribeDBClusterParameters
* action to verify that your DB cluster parameter group has been created or modified.
*
*
*
* For more information on Amazon Aurora, see What Is Amazon
* Aurora? in the Amazon Aurora User Guide.
*
*
*
* This action only applies to Aurora DB clusters.
*
*
*
* @param createDBClusterParameterGroupRequest
* @return Result of the CreateDBClusterParameterGroup operation returned by the service.
* @throws DBParameterGroupQuotaExceededException
* The request would result in the user exceeding the allowed number of DB parameter groups.
* @throws DBParameterGroupAlreadyExistsException
* A DB parameter group with the same name exists.
* @sample AmazonRDS.CreateDBClusterParameterGroup
* @see AWS API Documentation
*/
@Override
public DBClusterParameterGroup createDBClusterParameterGroup(CreateDBClusterParameterGroupRequest request) {
request = beforeClientExecution(request);
return executeCreateDBClusterParameterGroup(request);
}
@SdkInternalApi
final DBClusterParameterGroup executeCreateDBClusterParameterGroup(CreateDBClusterParameterGroupRequest createDBClusterParameterGroupRequest) {
ExecutionContext executionContext = createExecutionContext(createDBClusterParameterGroupRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new CreateDBClusterParameterGroupRequestMarshaller().marshall(super.beforeMarshalling(createDBClusterParameterGroupRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "RDS");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "CreateDBClusterParameterGroup");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
StaxResponseHandler responseHandler = new StaxResponseHandler(
new DBClusterParameterGroupStaxUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Creates a snapshot of a DB cluster. For more information on Amazon Aurora, see What Is Amazon
* Aurora? in the Amazon Aurora User Guide.
*
*
*
* This action only applies to Aurora DB clusters.
*
*
*
* @param createDBClusterSnapshotRequest
* @return Result of the CreateDBClusterSnapshot operation returned by the service.
* @throws DBClusterSnapshotAlreadyExistsException
* The user already has a DB cluster snapshot with the given identifier.
* @throws InvalidDBClusterStateException
* The requested operation can't be performed while the cluster is in this state.
* @throws DBClusterNotFoundException
* DBClusterIdentifier
doesn't refer to an existing DB cluster.
* @throws SnapshotQuotaExceededException
* The request would result in the user exceeding the allowed number of DB snapshots.
* @throws InvalidDBClusterSnapshotStateException
* The supplied value isn't a valid DB cluster snapshot state.
* @sample AmazonRDS.CreateDBClusterSnapshot
* @see AWS
* API Documentation
*/
@Override
public DBClusterSnapshot createDBClusterSnapshot(CreateDBClusterSnapshotRequest request) {
request = beforeClientExecution(request);
return executeCreateDBClusterSnapshot(request);
}
@SdkInternalApi
final DBClusterSnapshot executeCreateDBClusterSnapshot(CreateDBClusterSnapshotRequest createDBClusterSnapshotRequest) {
ExecutionContext executionContext = createExecutionContext(createDBClusterSnapshotRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new CreateDBClusterSnapshotRequestMarshaller().marshall(super.beforeMarshalling(createDBClusterSnapshotRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "RDS");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "CreateDBClusterSnapshot");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
StaxResponseHandler responseHandler = new StaxResponseHandler(new DBClusterSnapshotStaxUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Creates a new DB instance.
*
*
* @param createDBInstanceRequest
* @return Result of the CreateDBInstance operation returned by the service.
* @throws DBInstanceAlreadyExistsException
* The user already has a DB instance with the given identifier.
* @throws InsufficientDBInstanceCapacityException
* The specified DB instance class isn't available in the specified Availability Zone.
* @throws DBParameterGroupNotFoundException
* DBParameterGroupName
doesn't refer to an existing DB parameter group.
* @throws DBSecurityGroupNotFoundException
* DBSecurityGroupName
doesn't refer to an existing DB security group.
* @throws InstanceQuotaExceededException
* The request would result in the user exceeding the allowed number of DB instances.
* @throws StorageQuotaExceededException
* The request would result in the user exceeding the allowed amount of storage available across all DB
* instances.
* @throws DBSubnetGroupNotFoundException
* DBSubnetGroupName
doesn't refer to an existing DB subnet group.
* @throws DBSubnetGroupDoesNotCoverEnoughAZsException
* Subnets in the DB subnet group should cover at least two Availability Zones unless there is only one
* Availability Zone.
* @throws InvalidDBClusterStateException
* The requested operation can't be performed while the cluster is in this state.
* @throws InvalidSubnetException
* The requested subnet is invalid, or multiple subnets were requested that are not all in a common VPC.
* @throws InvalidVPCNetworkStateException
* The DB subnet group doesn't cover all Availability Zones after it's created because of users' change.
* @throws ProvisionedIopsNotAvailableInAZException
* Provisioned IOPS not available in the specified Availability Zone.
* @throws OptionGroupNotFoundException
* The specified option group could not be found.
* @throws DBClusterNotFoundException
* DBClusterIdentifier
doesn't refer to an existing DB cluster.
* @throws StorageTypeNotSupportedException
* Storage of the StorageType
specified can't be associated with the DB instance.
* @throws AuthorizationNotFoundException
* The specified CIDR IP range or Amazon EC2 security group might not be authorized for the specified DB
* security group.
*
* Or, RDS might not be authorized to perform necessary actions using IAM on your behalf.
* @throws KMSKeyNotAccessibleException
* An error occurred accessing an Amazon Web Services KMS key.
* @throws DomainNotFoundException
* Domain
doesn't refer to an existing Active Directory domain.
* @throws BackupPolicyNotFoundException
* @sample AmazonRDS.CreateDBInstance
* @see AWS API
* Documentation
*/
@Override
public DBInstance createDBInstance(CreateDBInstanceRequest request) {
request = beforeClientExecution(request);
return executeCreateDBInstance(request);
}
@SdkInternalApi
final DBInstance executeCreateDBInstance(CreateDBInstanceRequest createDBInstanceRequest) {
ExecutionContext executionContext = createExecutionContext(createDBInstanceRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new CreateDBInstanceRequestMarshaller().marshall(super.beforeMarshalling(createDBInstanceRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "RDS");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "CreateDBInstance");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
StaxResponseHandler responseHandler = new StaxResponseHandler(new DBInstanceStaxUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Creates a new DB instance that acts as a read replica for an existing source DB instance. You can create a read
* replica for a DB instance running MySQL, MariaDB, Oracle, PostgreSQL, or SQL Server. For more information, see Working with Read Replicas
* in the Amazon RDS User Guide.
*
*
* Amazon Aurora doesn't support this action. Call the CreateDBInstance
action to create a DB instance
* for an Aurora DB cluster.
*
*
* All read replica DB instances are created with backups disabled. All other DB instance attributes (including DB
* security groups and DB parameter groups) are inherited from the source DB instance, except as specified.
*
*
*
* Your source DB instance must have backup retention enabled.
*
*
*
* @param createDBInstanceReadReplicaRequest
* @return Result of the CreateDBInstanceReadReplica operation returned by the service.
* @throws DBInstanceAlreadyExistsException
* The user already has a DB instance with the given identifier.
* @throws InsufficientDBInstanceCapacityException
* The specified DB instance class isn't available in the specified Availability Zone.
* @throws DBParameterGroupNotFoundException
* DBParameterGroupName
doesn't refer to an existing DB parameter group.
* @throws DBSecurityGroupNotFoundException
* DBSecurityGroupName
doesn't refer to an existing DB security group.
* @throws InstanceQuotaExceededException
* The request would result in the user exceeding the allowed number of DB instances.
* @throws StorageQuotaExceededException
* The request would result in the user exceeding the allowed amount of storage available across all DB
* instances.
* @throws DBInstanceNotFoundException
* DBInstanceIdentifier
doesn't refer to an existing DB instance.
* @throws InvalidDBInstanceStateException
* The DB instance isn't in a valid state.
* @throws DBSubnetGroupNotFoundException
* DBSubnetGroupName
doesn't refer to an existing DB subnet group.
* @throws DBSubnetGroupDoesNotCoverEnoughAZsException
* Subnets in the DB subnet group should cover at least two Availability Zones unless there is only one
* Availability Zone.
* @throws InvalidSubnetException
* The requested subnet is invalid, or multiple subnets were requested that are not all in a common VPC.
* @throws InvalidVPCNetworkStateException
* The DB subnet group doesn't cover all Availability Zones after it's created because of users' change.
* @throws ProvisionedIopsNotAvailableInAZException
* Provisioned IOPS not available in the specified Availability Zone.
* @throws OptionGroupNotFoundException
* The specified option group could not be found.
* @throws DBSubnetGroupNotAllowedException
* The DBSubnetGroup shouldn't be specified while creating read replicas that lie in the same region as the
* source instance.
* @throws InvalidDBSubnetGroupException
* The DBSubnetGroup doesn't belong to the same VPC as that of an existing cross-region read replica of the
* same source instance.
* @throws StorageTypeNotSupportedException
* Storage of the StorageType
specified can't be associated with the DB instance.
* @throws KMSKeyNotAccessibleException
* An error occurred accessing an Amazon Web Services KMS key.
* @throws DomainNotFoundException
* Domain
doesn't refer to an existing Active Directory domain.
* @sample AmazonRDS.CreateDBInstanceReadReplica
* @see AWS API Documentation
*/
@Override
public DBInstance createDBInstanceReadReplica(CreateDBInstanceReadReplicaRequest request) {
request = beforeClientExecution(request);
return executeCreateDBInstanceReadReplica(request);
}
@SdkInternalApi
final DBInstance executeCreateDBInstanceReadReplica(CreateDBInstanceReadReplicaRequest createDBInstanceReadReplicaRequest) {
ExecutionContext executionContext = createExecutionContext(createDBInstanceReadReplicaRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new CreateDBInstanceReadReplicaRequestMarshaller().marshall(super.beforeMarshalling(createDBInstanceReadReplicaRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "RDS");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "CreateDBInstanceReadReplica");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
StaxResponseHandler responseHandler = new StaxResponseHandler(new DBInstanceStaxUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Creates a new DB parameter group.
*
*
* A DB parameter group is initially created with the default parameters for the database engine used by the DB
* instance. To provide custom values for any of the parameters, you must modify the group after creating it using
* ModifyDBParameterGroup
. Once you've created a DB parameter group, you need to associate it with your
* DB instance using ModifyDBInstance
. When you associate a new DB parameter group with a running DB
* instance, you need to reboot the DB instance without failover for the new DB parameter group and associated
* settings to take effect.
*
*
* This command doesn't apply to RDS Custom.
*
*
*
* After you create a DB parameter group, you should wait at least 5 minutes before creating your first DB instance
* that uses that DB parameter group as the default parameter group. This allows Amazon RDS to fully complete the
* create action before the parameter group is used as the default for a new DB instance. This is especially
* important for parameters that are critical when creating the default database for a DB instance, such as the
* character set for the default database defined by the character_set_database
parameter. You can use
* the Parameter Groups option of the Amazon RDS console or
* the DescribeDBParameters command to verify that your DB parameter group has been created or modified.
*
*
*
* @param createDBParameterGroupRequest
* @return Result of the CreateDBParameterGroup operation returned by the service.
* @throws DBParameterGroupQuotaExceededException
* The request would result in the user exceeding the allowed number of DB parameter groups.
* @throws DBParameterGroupAlreadyExistsException
* A DB parameter group with the same name exists.
* @sample AmazonRDS.CreateDBParameterGroup
* @see AWS API
* Documentation
*/
@Override
public DBParameterGroup createDBParameterGroup(CreateDBParameterGroupRequest request) {
request = beforeClientExecution(request);
return executeCreateDBParameterGroup(request);
}
@SdkInternalApi
final DBParameterGroup executeCreateDBParameterGroup(CreateDBParameterGroupRequest createDBParameterGroupRequest) {
ExecutionContext executionContext = createExecutionContext(createDBParameterGroupRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new CreateDBParameterGroupRequestMarshaller().marshall(super.beforeMarshalling(createDBParameterGroupRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "RDS");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "CreateDBParameterGroup");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
StaxResponseHandler responseHandler = new StaxResponseHandler(new DBParameterGroupStaxUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Creates a new DB proxy.
*
*
* @param createDBProxyRequest
* @return Result of the CreateDBProxy operation returned by the service.
* @throws InvalidSubnetException
* The requested subnet is invalid, or multiple subnets were requested that are not all in a common VPC.
* @throws DBProxyAlreadyExistsException
* The specified proxy name must be unique for all proxies owned by your Amazon Web Services account in the
* specified Amazon Web Services Region.
* @throws DBProxyQuotaExceededException
* Your Amazon Web Services account already has the maximum number of proxies in the specified Amazon Web
* Services Region.
* @sample AmazonRDS.CreateDBProxy
* @see AWS API
* Documentation
*/
@Override
public CreateDBProxyResult createDBProxy(CreateDBProxyRequest request) {
request = beforeClientExecution(request);
return executeCreateDBProxy(request);
}
@SdkInternalApi
final CreateDBProxyResult executeCreateDBProxy(CreateDBProxyRequest createDBProxyRequest) {
ExecutionContext executionContext = createExecutionContext(createDBProxyRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new CreateDBProxyRequestMarshaller().marshall(super.beforeMarshalling(createDBProxyRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "RDS");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "CreateDBProxy");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
StaxResponseHandler responseHandler = new StaxResponseHandler(new CreateDBProxyResultStaxUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Creates a DBProxyEndpoint
. Only applies to proxies that are associated with Aurora DB clusters. You
* can use DB proxy endpoints to specify read/write or read-only access to the DB cluster. You can also use DB proxy
* endpoints to access a DB proxy through a different VPC than the proxy's default VPC.
*
*
* @param createDBProxyEndpointRequest
* @return Result of the CreateDBProxyEndpoint operation returned by the service.
* @throws InvalidSubnetException
* The requested subnet is invalid, or multiple subnets were requested that are not all in a common VPC.
* @throws DBProxyNotFoundException
* The specified proxy name doesn't correspond to a proxy owned by your Amazon Web Services account in the
* specified Amazon Web Services Region.
* @throws DBProxyEndpointAlreadyExistsException
* The specified DB proxy endpoint name must be unique for all DB proxy endpoints owned by your Amazon Web
* Services account in the specified Amazon Web Services Region.
* @throws DBProxyEndpointQuotaExceededException
* The DB proxy already has the maximum number of endpoints.
* @throws InvalidDBProxyStateException
* The requested operation can't be performed while the proxy is in this state.
* @sample AmazonRDS.CreateDBProxyEndpoint
* @see AWS API
* Documentation
*/
@Override
public CreateDBProxyEndpointResult createDBProxyEndpoint(CreateDBProxyEndpointRequest request) {
request = beforeClientExecution(request);
return executeCreateDBProxyEndpoint(request);
}
@SdkInternalApi
final CreateDBProxyEndpointResult executeCreateDBProxyEndpoint(CreateDBProxyEndpointRequest createDBProxyEndpointRequest) {
ExecutionContext executionContext = createExecutionContext(createDBProxyEndpointRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new CreateDBProxyEndpointRequestMarshaller().marshall(super.beforeMarshalling(createDBProxyEndpointRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "RDS");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "CreateDBProxyEndpoint");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
StaxResponseHandler responseHandler = new StaxResponseHandler(
new CreateDBProxyEndpointResultStaxUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Creates a new DB security group. DB security groups control access to a DB instance.
*
*
*
* A DB security group controls access to EC2-Classic DB instances that are not in a VPC.
*
*
*
* @param createDBSecurityGroupRequest
* @return Result of the CreateDBSecurityGroup operation returned by the service.
* @throws DBSecurityGroupAlreadyExistsException
* A DB security group with the name specified in DBSecurityGroupName
already exists.
* @throws DBSecurityGroupQuotaExceededException
* The request would result in the user exceeding the allowed number of DB security groups.
* @throws DBSecurityGroupNotSupportedException
* A DB security group isn't allowed for this action.
* @sample AmazonRDS.CreateDBSecurityGroup
* @see AWS API
* Documentation
*/
@Override
public DBSecurityGroup createDBSecurityGroup(CreateDBSecurityGroupRequest request) {
request = beforeClientExecution(request);
return executeCreateDBSecurityGroup(request);
}
@SdkInternalApi
final DBSecurityGroup executeCreateDBSecurityGroup(CreateDBSecurityGroupRequest createDBSecurityGroupRequest) {
ExecutionContext executionContext = createExecutionContext(createDBSecurityGroupRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new CreateDBSecurityGroupRequestMarshaller().marshall(super.beforeMarshalling(createDBSecurityGroupRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "RDS");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "CreateDBSecurityGroup");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
StaxResponseHandler responseHandler = new StaxResponseHandler(new DBSecurityGroupStaxUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Creates a snapshot of a DB instance. The source DB instance must be in the available
or
* storage-optimization
state.
*
*
* @param createDBSnapshotRequest
* @return Result of the CreateDBSnapshot operation returned by the service.
* @throws DBSnapshotAlreadyExistsException
* DBSnapshotIdentifier
is already used by an existing snapshot.
* @throws InvalidDBInstanceStateException
* The DB instance isn't in a valid state.
* @throws DBInstanceNotFoundException
* DBInstanceIdentifier
doesn't refer to an existing DB instance.
* @throws SnapshotQuotaExceededException
* The request would result in the user exceeding the allowed number of DB snapshots.
* @sample AmazonRDS.CreateDBSnapshot
* @see AWS API
* Documentation
*/
@Override
public DBSnapshot createDBSnapshot(CreateDBSnapshotRequest request) {
request = beforeClientExecution(request);
return executeCreateDBSnapshot(request);
}
@SdkInternalApi
final DBSnapshot executeCreateDBSnapshot(CreateDBSnapshotRequest createDBSnapshotRequest) {
ExecutionContext executionContext = createExecutionContext(createDBSnapshotRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new CreateDBSnapshotRequestMarshaller().marshall(super.beforeMarshalling(createDBSnapshotRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "RDS");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "CreateDBSnapshot");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
StaxResponseHandler responseHandler = new StaxResponseHandler(new DBSnapshotStaxUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Creates a new DB subnet group. DB subnet groups must contain at least one subnet in at least two AZs in the
* Amazon Web Services Region.
*
*
* @param createDBSubnetGroupRequest
* @return Result of the CreateDBSubnetGroup operation returned by the service.
* @throws DBSubnetGroupAlreadyExistsException
* DBSubnetGroupName
is already used by an existing DB subnet group.
* @throws DBSubnetGroupQuotaExceededException
* The request would result in the user exceeding the allowed number of DB subnet groups.
* @throws DBSubnetQuotaExceededException
* The request would result in the user exceeding the allowed number of subnets in a DB subnet groups.
* @throws DBSubnetGroupDoesNotCoverEnoughAZsException
* Subnets in the DB subnet group should cover at least two Availability Zones unless there is only one
* Availability Zone.
* @throws InvalidSubnetException
* The requested subnet is invalid, or multiple subnets were requested that are not all in a common VPC.
* @sample AmazonRDS.CreateDBSubnetGroup
* @see AWS API
* Documentation
*/
@Override
public DBSubnetGroup createDBSubnetGroup(CreateDBSubnetGroupRequest request) {
request = beforeClientExecution(request);
return executeCreateDBSubnetGroup(request);
}
@SdkInternalApi
final DBSubnetGroup executeCreateDBSubnetGroup(CreateDBSubnetGroupRequest createDBSubnetGroupRequest) {
ExecutionContext executionContext = createExecutionContext(createDBSubnetGroupRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new CreateDBSubnetGroupRequestMarshaller().marshall(super.beforeMarshalling(createDBSubnetGroupRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "RDS");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "CreateDBSubnetGroup");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
StaxResponseHandler responseHandler = new StaxResponseHandler(new DBSubnetGroupStaxUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Creates an RDS event notification subscription. This action requires a topic Amazon Resource Name (ARN) created
* by either the RDS console, the SNS console, or the SNS API. To obtain an ARN with SNS, you must create a topic in
* Amazon SNS and subscribe to the topic. The ARN is displayed in the SNS console.
*
*
* You can specify the type of source (SourceType
) that you want to be notified of and provide a list
* of RDS sources (SourceIds
) that triggers the events. You can also provide a list of event categories
* (EventCategories
) for events that you want to be notified of. For example, you can specify
* SourceType
= db-instance
, SourceIds
= mydbinstance1
,
* mydbinstance2
and EventCategories
= Availability
, Backup
.
*
*
* If you specify both the SourceType
and SourceIds
, such as SourceType
=
* db-instance
and SourceIdentifier
= myDBInstance1
, you are notified of all
* the db-instance
events for the specified source. If you specify a SourceType
but do not
* specify a SourceIdentifier
, you receive notice of the events for that source type for all your RDS
* sources. If you don't specify either the SourceType or the SourceIdentifier
, you are notified of
* events generated from all RDS sources belonging to your customer account.
*
*
*
* RDS event notification is only available for unencrypted SNS topics. If you specify an encrypted SNS topic, event
* notifications aren't sent for the topic.
*
*
*
* @param createEventSubscriptionRequest
* @return Result of the CreateEventSubscription operation returned by the service.
* @throws EventSubscriptionQuotaExceededException
* You have reached the maximum number of event subscriptions.
* @throws SubscriptionAlreadyExistException
* The supplied subscription name already exists.
* @throws SNSInvalidTopicException
* SNS has responded that there is a problem with the SND topic specified.
* @throws SNSNoAuthorizationException
* You do not have permission to publish to the SNS topic ARN.
* @throws SNSTopicArnNotFoundException
* The SNS topic ARN does not exist.
* @throws SubscriptionCategoryNotFoundException
* The supplied category does not exist.
* @throws SourceNotFoundException
* The requested source could not be found.
* @sample AmazonRDS.CreateEventSubscription
* @see AWS
* API Documentation
*/
@Override
public EventSubscription createEventSubscription(CreateEventSubscriptionRequest request) {
request = beforeClientExecution(request);
return executeCreateEventSubscription(request);
}
@SdkInternalApi
final EventSubscription executeCreateEventSubscription(CreateEventSubscriptionRequest createEventSubscriptionRequest) {
ExecutionContext executionContext = createExecutionContext(createEventSubscriptionRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new CreateEventSubscriptionRequestMarshaller().marshall(super.beforeMarshalling(createEventSubscriptionRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "RDS");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "CreateEventSubscription");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
StaxResponseHandler responseHandler = new StaxResponseHandler(new EventSubscriptionStaxUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Creates an Aurora global database spread across multiple Amazon Web Services Regions. The global database
* contains a single primary cluster with read-write capability, and a read-only secondary cluster that receives
* data from the primary cluster through high-speed replication performed by the Aurora storage subsystem.
*
*
* You can create a global database that is initially empty, and then add a primary cluster and a secondary cluster
* to it. Or you can specify an existing Aurora cluster during the create operation, and this cluster becomes the
* primary cluster of the global database.
*
*
*
* This action only applies to Aurora DB clusters.
*
*
*
* @param createGlobalClusterRequest
* @return Result of the CreateGlobalCluster operation returned by the service.
* @throws GlobalClusterAlreadyExistsException
* The GlobalClusterIdentifier
already exists. Choose a new global database identifier (unique
* name) to create a new global database cluster.
* @throws GlobalClusterQuotaExceededException
* The number of global database clusters for this account is already at the maximum allowed.
* @throws InvalidDBClusterStateException
* The requested operation can't be performed while the cluster is in this state.
* @throws DBClusterNotFoundException
* DBClusterIdentifier
doesn't refer to an existing DB cluster.
* @sample AmazonRDS.CreateGlobalCluster
* @see AWS API
* Documentation
*/
@Override
public GlobalCluster createGlobalCluster(CreateGlobalClusterRequest request) {
request = beforeClientExecution(request);
return executeCreateGlobalCluster(request);
}
@SdkInternalApi
final GlobalCluster executeCreateGlobalCluster(CreateGlobalClusterRequest createGlobalClusterRequest) {
ExecutionContext executionContext = createExecutionContext(createGlobalClusterRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new CreateGlobalClusterRequestMarshaller().marshall(super.beforeMarshalling(createGlobalClusterRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "RDS");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "CreateGlobalCluster");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
StaxResponseHandler responseHandler = new StaxResponseHandler(new GlobalClusterStaxUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Creates a new option group. You can create up to 20 option groups.
*
*
* This command doesn't apply to RDS Custom.
*
*
* @param createOptionGroupRequest
* @return Result of the CreateOptionGroup operation returned by the service.
* @throws OptionGroupAlreadyExistsException
* The option group you are trying to create already exists.
* @throws OptionGroupQuotaExceededException
* The quota of 20 option groups was exceeded for this Amazon Web Services account.
* @sample AmazonRDS.CreateOptionGroup
* @see AWS API
* Documentation
*/
@Override
public OptionGroup createOptionGroup(CreateOptionGroupRequest request) {
request = beforeClientExecution(request);
return executeCreateOptionGroup(request);
}
@SdkInternalApi
final OptionGroup executeCreateOptionGroup(CreateOptionGroupRequest createOptionGroupRequest) {
ExecutionContext executionContext = createExecutionContext(createOptionGroupRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new CreateOptionGroupRequestMarshaller().marshall(super.beforeMarshalling(createOptionGroupRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "RDS");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "CreateOptionGroup");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
StaxResponseHandler responseHandler = new StaxResponseHandler(new OptionGroupStaxUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Deletes a custom Availability Zone (AZ).
*
*
* A custom AZ is an on-premises AZ that is integrated with a VMware vSphere cluster.
*
*
* For more information about RDS on VMware, see the RDS on VMware User
* Guide.
*
*
* @param deleteCustomAvailabilityZoneRequest
* @return Result of the DeleteCustomAvailabilityZone operation returned by the service.
* @throws CustomAvailabilityZoneNotFoundException
* CustomAvailabilityZoneId
doesn't refer to an existing custom Availability Zone identifier.
* @throws KMSKeyNotAccessibleException
* An error occurred accessing an Amazon Web Services KMS key.
* @sample AmazonRDS.DeleteCustomAvailabilityZone
* @see AWS API Documentation
*/
@Override
public CustomAvailabilityZone deleteCustomAvailabilityZone(DeleteCustomAvailabilityZoneRequest request) {
request = beforeClientExecution(request);
return executeDeleteCustomAvailabilityZone(request);
}
@SdkInternalApi
final CustomAvailabilityZone executeDeleteCustomAvailabilityZone(DeleteCustomAvailabilityZoneRequest deleteCustomAvailabilityZoneRequest) {
ExecutionContext executionContext = createExecutionContext(deleteCustomAvailabilityZoneRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DeleteCustomAvailabilityZoneRequestMarshaller().marshall(super.beforeMarshalling(deleteCustomAvailabilityZoneRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "RDS");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DeleteCustomAvailabilityZone");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
StaxResponseHandler responseHandler = new StaxResponseHandler(
new CustomAvailabilityZoneStaxUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Deletes a custom engine version. To run this command, make sure you meet the following prerequisites:
*
*
* -
*
* The CEV must not be the default for RDS Custom. If it is, change the default before running this command.
*
*
* -
*
* The CEV must not be associated with an RDS Custom DB instance, RDS Custom instance snapshot, or automated backup
* of your RDS Custom instance.
*
*
*
*
* Typically, deletion takes a few minutes.
*
*
*
* The MediaImport service that imports files from Amazon S3 to create CEVs isn't integrated with Amazon Web
* Services CloudTrail. If you turn on data logging for Amazon RDS in CloudTrail, calls to the
* DeleteCustomDbEngineVersion
event aren't logged. However, you might see calls from the API gateway
* that accesses your Amazon S3 bucket. These calls originate from the MediaImport service for the
* DeleteCustomDbEngineVersion
event.
*
*
*
* For more information, see Deleting a
* CEV in the Amazon RDS User Guide.
*
*
* @param deleteCustomDBEngineVersionRequest
* @return Result of the DeleteCustomDBEngineVersion operation returned by the service.
* @throws CustomDBEngineVersionNotFoundException
* The specified CEV was not found.
* @throws InvalidCustomDBEngineVersionStateException
* You can't delete the CEV.
* @sample AmazonRDS.DeleteCustomDBEngineVersion
* @see AWS API Documentation
*/
@Override
public DeleteCustomDBEngineVersionResult deleteCustomDBEngineVersion(DeleteCustomDBEngineVersionRequest request) {
request = beforeClientExecution(request);
return executeDeleteCustomDBEngineVersion(request);
}
@SdkInternalApi
final DeleteCustomDBEngineVersionResult executeDeleteCustomDBEngineVersion(DeleteCustomDBEngineVersionRequest deleteCustomDBEngineVersionRequest) {
ExecutionContext executionContext = createExecutionContext(deleteCustomDBEngineVersionRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DeleteCustomDBEngineVersionRequestMarshaller().marshall(super.beforeMarshalling(deleteCustomDBEngineVersionRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "RDS");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DeleteCustomDBEngineVersion");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
StaxResponseHandler responseHandler = new StaxResponseHandler(
new DeleteCustomDBEngineVersionResultStaxUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* The DeleteDBCluster action deletes a previously provisioned DB cluster. When you delete a DB cluster, all
* automated backups for that DB cluster are deleted and can't be recovered. Manual DB cluster snapshots of the
* specified DB cluster are not deleted.
*
*
*
* For more information on Amazon Aurora, see What Is Amazon
* Aurora? in the Amazon Aurora User Guide.
*
*
*
* This action only applies to Aurora DB clusters.
*
*
*
* @param deleteDBClusterRequest
* @return Result of the DeleteDBCluster operation returned by the service.
* @throws DBClusterNotFoundException
* DBClusterIdentifier
doesn't refer to an existing DB cluster.
* @throws InvalidDBClusterStateException
* The requested operation can't be performed while the cluster is in this state.
* @throws DBClusterSnapshotAlreadyExistsException
* The user already has a DB cluster snapshot with the given identifier.
* @throws SnapshotQuotaExceededException
* The request would result in the user exceeding the allowed number of DB snapshots.
* @throws InvalidDBClusterSnapshotStateException
* The supplied value isn't a valid DB cluster snapshot state.
* @sample AmazonRDS.DeleteDBCluster
* @see AWS API
* Documentation
*/
@Override
public DBCluster deleteDBCluster(DeleteDBClusterRequest request) {
request = beforeClientExecution(request);
return executeDeleteDBCluster(request);
}
@SdkInternalApi
final DBCluster executeDeleteDBCluster(DeleteDBClusterRequest deleteDBClusterRequest) {
ExecutionContext executionContext = createExecutionContext(deleteDBClusterRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DeleteDBClusterRequestMarshaller().marshall(super.beforeMarshalling(deleteDBClusterRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "RDS");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DeleteDBCluster");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
StaxResponseHandler responseHandler = new StaxResponseHandler(new DBClusterStaxUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Deletes a custom endpoint and removes it from an Amazon Aurora DB cluster.
*
*
*
* This action only applies to Aurora DB clusters.
*
*
*
* @param deleteDBClusterEndpointRequest
* @return Result of the DeleteDBClusterEndpoint operation returned by the service.
* @throws InvalidDBClusterEndpointStateException
* The requested operation can't be performed on the endpoint while the endpoint is in this state.
* @throws DBClusterEndpointNotFoundException
* The specified custom endpoint doesn't exist.
* @throws InvalidDBClusterStateException
* The requested operation can't be performed while the cluster is in this state.
* @sample AmazonRDS.DeleteDBClusterEndpoint
* @see AWS
* API Documentation
*/
@Override
public DeleteDBClusterEndpointResult deleteDBClusterEndpoint(DeleteDBClusterEndpointRequest request) {
request = beforeClientExecution(request);
return executeDeleteDBClusterEndpoint(request);
}
@SdkInternalApi
final DeleteDBClusterEndpointResult executeDeleteDBClusterEndpoint(DeleteDBClusterEndpointRequest deleteDBClusterEndpointRequest) {
ExecutionContext executionContext = createExecutionContext(deleteDBClusterEndpointRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DeleteDBClusterEndpointRequestMarshaller().marshall(super.beforeMarshalling(deleteDBClusterEndpointRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "RDS");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DeleteDBClusterEndpoint");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
StaxResponseHandler responseHandler = new StaxResponseHandler(
new DeleteDBClusterEndpointResultStaxUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Deletes a specified DB cluster parameter group. The DB cluster parameter group to be deleted can't be associated
* with any DB clusters.
*
*
* For more information on Amazon Aurora, see What Is Amazon
* Aurora? in the Amazon Aurora User Guide.
*
*
*
* This action only applies to Aurora DB clusters.
*
*
*
* @param deleteDBClusterParameterGroupRequest
* @return Result of the DeleteDBClusterParameterGroup operation returned by the service.
* @throws InvalidDBParameterGroupStateException
* The DB parameter group is in use or is in an invalid state. If you are attempting to delete the parameter
* group, you can't delete it when the parameter group is in this state.
* @throws DBParameterGroupNotFoundException
* DBParameterGroupName
doesn't refer to an existing DB parameter group.
* @sample AmazonRDS.DeleteDBClusterParameterGroup
* @see AWS API Documentation
*/
@Override
public DeleteDBClusterParameterGroupResult deleteDBClusterParameterGroup(DeleteDBClusterParameterGroupRequest request) {
request = beforeClientExecution(request);
return executeDeleteDBClusterParameterGroup(request);
}
@SdkInternalApi
final DeleteDBClusterParameterGroupResult executeDeleteDBClusterParameterGroup(DeleteDBClusterParameterGroupRequest deleteDBClusterParameterGroupRequest) {
ExecutionContext executionContext = createExecutionContext(deleteDBClusterParameterGroupRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DeleteDBClusterParameterGroupRequestMarshaller().marshall(super.beforeMarshalling(deleteDBClusterParameterGroupRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "RDS");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DeleteDBClusterParameterGroup");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
StaxResponseHandler responseHandler = new StaxResponseHandler(
new DeleteDBClusterParameterGroupResultStaxUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Deletes a DB cluster snapshot. If the snapshot is being copied, the copy operation is terminated.
*
*
*
* The DB cluster snapshot must be in the available
state to be deleted.
*
*
*
* For more information on Amazon Aurora, see What Is Amazon
* Aurora? in the Amazon Aurora User Guide.
*
*
*
* This action only applies to Aurora DB clusters.
*
*
*
* @param deleteDBClusterSnapshotRequest
* @return Result of the DeleteDBClusterSnapshot operation returned by the service.
* @throws InvalidDBClusterSnapshotStateException
* The supplied value isn't a valid DB cluster snapshot state.
* @throws DBClusterSnapshotNotFoundException
* DBClusterSnapshotIdentifier
doesn't refer to an existing DB cluster snapshot.
* @sample AmazonRDS.DeleteDBClusterSnapshot
* @see AWS
* API Documentation
*/
@Override
public DBClusterSnapshot deleteDBClusterSnapshot(DeleteDBClusterSnapshotRequest request) {
request = beforeClientExecution(request);
return executeDeleteDBClusterSnapshot(request);
}
@SdkInternalApi
final DBClusterSnapshot executeDeleteDBClusterSnapshot(DeleteDBClusterSnapshotRequest deleteDBClusterSnapshotRequest) {
ExecutionContext executionContext = createExecutionContext(deleteDBClusterSnapshotRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DeleteDBClusterSnapshotRequestMarshaller().marshall(super.beforeMarshalling(deleteDBClusterSnapshotRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "RDS");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DeleteDBClusterSnapshot");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
StaxResponseHandler responseHandler = new StaxResponseHandler(new DBClusterSnapshotStaxUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* The DeleteDBInstance action deletes a previously provisioned DB instance. When you delete a DB instance, all
* automated backups for that instance are deleted and can't be recovered. Manual DB snapshots of the DB instance to
* be deleted by DeleteDBInstance
are not deleted.
*
*
* If you request a final DB snapshot the status of the Amazon RDS DB instance is deleting
until the DB
* snapshot is created. The API action DescribeDBInstance
is used to monitor the status of this
* operation. The action can't be canceled or reverted once submitted.
*
*
* When a DB instance is in a failure state and has a status of failed
,
* incompatible-restore
, or incompatible-network
, you can only delete it when you skip
* creation of the final snapshot with the SkipFinalSnapshot
parameter.
*
*
* If the specified DB instance is part of an Amazon Aurora DB cluster, you can't delete the DB instance if both of
* the following conditions are true:
*
*
* -
*
* The DB cluster is a read replica of another Amazon Aurora DB cluster.
*
*
* -
*
* The DB instance is the only instance in the DB cluster.
*
*
*
*
* To delete a DB instance in this case, first call the PromoteReadReplicaDBCluster
API action to
* promote the DB cluster so it's no longer a read replica. After the promotion completes, then call the
* DeleteDBInstance
API action to delete the final instance in the DB cluster.
*
*
* @param deleteDBInstanceRequest
* @return Result of the DeleteDBInstance operation returned by the service.
* @throws DBInstanceNotFoundException
* DBInstanceIdentifier
doesn't refer to an existing DB instance.
* @throws InvalidDBInstanceStateException
* The DB instance isn't in a valid state.
* @throws DBSnapshotAlreadyExistsException
* DBSnapshotIdentifier
is already used by an existing snapshot.
* @throws SnapshotQuotaExceededException
* The request would result in the user exceeding the allowed number of DB snapshots.
* @throws InvalidDBClusterStateException
* The requested operation can't be performed while the cluster is in this state.
* @throws DBInstanceAutomatedBackupQuotaExceededException
* The quota for retained automated backups was exceeded. This prevents you from retaining any additional
* automated backups. The retained automated backups quota is the same as your DB Instance quota.
* @sample AmazonRDS.DeleteDBInstance
* @see AWS API
* Documentation
*/
@Override
public DBInstance deleteDBInstance(DeleteDBInstanceRequest request) {
request = beforeClientExecution(request);
return executeDeleteDBInstance(request);
}
@SdkInternalApi
final DBInstance executeDeleteDBInstance(DeleteDBInstanceRequest deleteDBInstanceRequest) {
ExecutionContext executionContext = createExecutionContext(deleteDBInstanceRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DeleteDBInstanceRequestMarshaller().marshall(super.beforeMarshalling(deleteDBInstanceRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "RDS");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DeleteDBInstance");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
StaxResponseHandler responseHandler = new StaxResponseHandler(new DBInstanceStaxUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Deletes automated backups using the DbiResourceId
value of the source DB instance or the Amazon
* Resource Name (ARN) of the automated backups.
*
*
* @param deleteDBInstanceAutomatedBackupRequest
* Parameter input for the DeleteDBInstanceAutomatedBackup
operation.
* @return Result of the DeleteDBInstanceAutomatedBackup operation returned by the service.
* @throws InvalidDBInstanceAutomatedBackupStateException
* The automated backup is in an invalid state. For example, this automated backup is associated with an
* active instance.
* @throws DBInstanceAutomatedBackupNotFoundException
* No automated backup for this DB instance was found.
* @sample AmazonRDS.DeleteDBInstanceAutomatedBackup
* @see AWS API Documentation
*/
@Override
public DBInstanceAutomatedBackup deleteDBInstanceAutomatedBackup(DeleteDBInstanceAutomatedBackupRequest request) {
request = beforeClientExecution(request);
return executeDeleteDBInstanceAutomatedBackup(request);
}
@SdkInternalApi
final DBInstanceAutomatedBackup executeDeleteDBInstanceAutomatedBackup(DeleteDBInstanceAutomatedBackupRequest deleteDBInstanceAutomatedBackupRequest) {
ExecutionContext executionContext = createExecutionContext(deleteDBInstanceAutomatedBackupRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DeleteDBInstanceAutomatedBackupRequestMarshaller().marshall(super.beforeMarshalling(deleteDBInstanceAutomatedBackupRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "RDS");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DeleteDBInstanceAutomatedBackup");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
StaxResponseHandler responseHandler = new StaxResponseHandler(
new DBInstanceAutomatedBackupStaxUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Deletes a specified DB parameter group. The DB parameter group to be deleted can't be associated with any DB
* instances.
*
*
* @param deleteDBParameterGroupRequest
* @return Result of the DeleteDBParameterGroup operation returned by the service.
* @throws InvalidDBParameterGroupStateException
* The DB parameter group is in use or is in an invalid state. If you are attempting to delete the parameter
* group, you can't delete it when the parameter group is in this state.
* @throws DBParameterGroupNotFoundException
* DBParameterGroupName
doesn't refer to an existing DB parameter group.
* @sample AmazonRDS.DeleteDBParameterGroup
* @see AWS API
* Documentation
*/
@Override
public DeleteDBParameterGroupResult deleteDBParameterGroup(DeleteDBParameterGroupRequest request) {
request = beforeClientExecution(request);
return executeDeleteDBParameterGroup(request);
}
@SdkInternalApi
final DeleteDBParameterGroupResult executeDeleteDBParameterGroup(DeleteDBParameterGroupRequest deleteDBParameterGroupRequest) {
ExecutionContext executionContext = createExecutionContext(deleteDBParameterGroupRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DeleteDBParameterGroupRequestMarshaller().marshall(super.beforeMarshalling(deleteDBParameterGroupRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "RDS");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DeleteDBParameterGroup");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
StaxResponseHandler responseHandler = new StaxResponseHandler(
new DeleteDBParameterGroupResultStaxUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Deletes an existing DB proxy.
*
*
* @param deleteDBProxyRequest
* @return Result of the DeleteDBProxy operation returned by the service.
* @throws DBProxyNotFoundException
* The specified proxy name doesn't correspond to a proxy owned by your Amazon Web Services account in the
* specified Amazon Web Services Region.
* @throws InvalidDBProxyStateException
* The requested operation can't be performed while the proxy is in this state.
* @sample AmazonRDS.DeleteDBProxy
* @see AWS API
* Documentation
*/
@Override
public DeleteDBProxyResult deleteDBProxy(DeleteDBProxyRequest request) {
request = beforeClientExecution(request);
return executeDeleteDBProxy(request);
}
@SdkInternalApi
final DeleteDBProxyResult executeDeleteDBProxy(DeleteDBProxyRequest deleteDBProxyRequest) {
ExecutionContext executionContext = createExecutionContext(deleteDBProxyRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DeleteDBProxyRequestMarshaller().marshall(super.beforeMarshalling(deleteDBProxyRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "RDS");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DeleteDBProxy");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
StaxResponseHandler responseHandler = new StaxResponseHandler(new DeleteDBProxyResultStaxUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Deletes a DBProxyEndpoint
. Doing so removes the ability to access the DB proxy using the endpoint
* that you defined. The endpoint that you delete might have provided capabilities such as read/write or read-only
* operations, or using a different VPC than the DB proxy's default VPC.
*
*
* @param deleteDBProxyEndpointRequest
* @return Result of the DeleteDBProxyEndpoint operation returned by the service.
* @throws DBProxyEndpointNotFoundException
* The DB proxy endpoint doesn't exist.
* @throws InvalidDBProxyEndpointStateException
* You can't perform this operation while the DB proxy endpoint is in a particular state.
* @sample AmazonRDS.DeleteDBProxyEndpoint
* @see AWS API
* Documentation
*/
@Override
public DeleteDBProxyEndpointResult deleteDBProxyEndpoint(DeleteDBProxyEndpointRequest request) {
request = beforeClientExecution(request);
return executeDeleteDBProxyEndpoint(request);
}
@SdkInternalApi
final DeleteDBProxyEndpointResult executeDeleteDBProxyEndpoint(DeleteDBProxyEndpointRequest deleteDBProxyEndpointRequest) {
ExecutionContext executionContext = createExecutionContext(deleteDBProxyEndpointRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DeleteDBProxyEndpointRequestMarshaller().marshall(super.beforeMarshalling(deleteDBProxyEndpointRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "RDS");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DeleteDBProxyEndpoint");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
StaxResponseHandler responseHandler = new StaxResponseHandler(
new DeleteDBProxyEndpointResultStaxUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Deletes a DB security group.
*
*
*
* The specified DB security group must not be associated with any DB instances.
*
*
*
* @param deleteDBSecurityGroupRequest
* @return Result of the DeleteDBSecurityGroup operation returned by the service.
* @throws InvalidDBSecurityGroupStateException
* The state of the DB security group doesn't allow deletion.
* @throws DBSecurityGroupNotFoundException
* DBSecurityGroupName
doesn't refer to an existing DB security group.
* @sample AmazonRDS.DeleteDBSecurityGroup
* @see AWS API
* Documentation
*/
@Override
public DeleteDBSecurityGroupResult deleteDBSecurityGroup(DeleteDBSecurityGroupRequest request) {
request = beforeClientExecution(request);
return executeDeleteDBSecurityGroup(request);
}
@SdkInternalApi
final DeleteDBSecurityGroupResult executeDeleteDBSecurityGroup(DeleteDBSecurityGroupRequest deleteDBSecurityGroupRequest) {
ExecutionContext executionContext = createExecutionContext(deleteDBSecurityGroupRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DeleteDBSecurityGroupRequestMarshaller().marshall(super.beforeMarshalling(deleteDBSecurityGroupRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "RDS");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DeleteDBSecurityGroup");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
StaxResponseHandler responseHandler = new StaxResponseHandler(
new DeleteDBSecurityGroupResultStaxUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Deletes a DB snapshot. If the snapshot is being copied, the copy operation is terminated.
*
*
*
* The DB snapshot must be in the available
state to be deleted.
*
*
*
* @param deleteDBSnapshotRequest
* @return Result of the DeleteDBSnapshot operation returned by the service.
* @throws InvalidDBSnapshotStateException
* The state of the DB snapshot doesn't allow deletion.
* @throws DBSnapshotNotFoundException
* DBSnapshotIdentifier
doesn't refer to an existing DB snapshot.
* @sample AmazonRDS.DeleteDBSnapshot
* @see AWS API
* Documentation
*/
@Override
public DBSnapshot deleteDBSnapshot(DeleteDBSnapshotRequest request) {
request = beforeClientExecution(request);
return executeDeleteDBSnapshot(request);
}
@SdkInternalApi
final DBSnapshot executeDeleteDBSnapshot(DeleteDBSnapshotRequest deleteDBSnapshotRequest) {
ExecutionContext executionContext = createExecutionContext(deleteDBSnapshotRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DeleteDBSnapshotRequestMarshaller().marshall(super.beforeMarshalling(deleteDBSnapshotRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "RDS");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DeleteDBSnapshot");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
StaxResponseHandler responseHandler = new StaxResponseHandler(new DBSnapshotStaxUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Deletes a DB subnet group.
*
*
*
* The specified database subnet group must not be associated with any DB instances.
*
*
*
* @param deleteDBSubnetGroupRequest
* @return Result of the DeleteDBSubnetGroup operation returned by the service.
* @throws InvalidDBSubnetGroupStateException
* The DB subnet group cannot be deleted because it's in use.
* @throws InvalidDBSubnetStateException
* The DB subnet isn't in the available state.
* @throws DBSubnetGroupNotFoundException
* DBSubnetGroupName
doesn't refer to an existing DB subnet group.
* @sample AmazonRDS.DeleteDBSubnetGroup
* @see AWS API
* Documentation
*/
@Override
public DeleteDBSubnetGroupResult deleteDBSubnetGroup(DeleteDBSubnetGroupRequest request) {
request = beforeClientExecution(request);
return executeDeleteDBSubnetGroup(request);
}
@SdkInternalApi
final DeleteDBSubnetGroupResult executeDeleteDBSubnetGroup(DeleteDBSubnetGroupRequest deleteDBSubnetGroupRequest) {
ExecutionContext executionContext = createExecutionContext(deleteDBSubnetGroupRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DeleteDBSubnetGroupRequestMarshaller().marshall(super.beforeMarshalling(deleteDBSubnetGroupRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "RDS");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DeleteDBSubnetGroup");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
StaxResponseHandler responseHandler = new StaxResponseHandler(
new DeleteDBSubnetGroupResultStaxUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Deletes an RDS event notification subscription.
*
*
* @param deleteEventSubscriptionRequest
* @return Result of the DeleteEventSubscription operation returned by the service.
* @throws SubscriptionNotFoundException
* The subscription name does not exist.
* @throws InvalidEventSubscriptionStateException
* This error can occur if someone else is modifying a subscription. You should retry the action.
* @sample AmazonRDS.DeleteEventSubscription
* @see AWS
* API Documentation
*/
@Override
public EventSubscription deleteEventSubscription(DeleteEventSubscriptionRequest request) {
request = beforeClientExecution(request);
return executeDeleteEventSubscription(request);
}
@SdkInternalApi
final EventSubscription executeDeleteEventSubscription(DeleteEventSubscriptionRequest deleteEventSubscriptionRequest) {
ExecutionContext executionContext = createExecutionContext(deleteEventSubscriptionRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DeleteEventSubscriptionRequestMarshaller().marshall(super.beforeMarshalling(deleteEventSubscriptionRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "RDS");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DeleteEventSubscription");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
StaxResponseHandler responseHandler = new StaxResponseHandler(new EventSubscriptionStaxUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Deletes a global database cluster. The primary and secondary clusters must already be detached or destroyed
* first.
*
*
*
* This action only applies to Aurora DB clusters.
*
*
*
* @param deleteGlobalClusterRequest
* @return Result of the DeleteGlobalCluster operation returned by the service.
* @throws GlobalClusterNotFoundException
* The GlobalClusterIdentifier
doesn't refer to an existing global database cluster.
* @throws InvalidGlobalClusterStateException
* The global cluster is in an invalid state and can't perform the requested operation.
* @sample AmazonRDS.DeleteGlobalCluster
* @see AWS API
* Documentation
*/
@Override
public GlobalCluster deleteGlobalCluster(DeleteGlobalClusterRequest request) {
request = beforeClientExecution(request);
return executeDeleteGlobalCluster(request);
}
@SdkInternalApi
final GlobalCluster executeDeleteGlobalCluster(DeleteGlobalClusterRequest deleteGlobalClusterRequest) {
ExecutionContext executionContext = createExecutionContext(deleteGlobalClusterRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DeleteGlobalClusterRequestMarshaller().marshall(super.beforeMarshalling(deleteGlobalClusterRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "RDS");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DeleteGlobalCluster");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
StaxResponseHandler responseHandler = new StaxResponseHandler(new GlobalClusterStaxUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Deletes the installation medium for a DB engine that requires an on-premises customer provided license, such as
* Microsoft SQL Server.
*
*
* @param deleteInstallationMediaRequest
* @return Result of the DeleteInstallationMedia operation returned by the service.
* @throws InstallationMediaNotFoundException
* InstallationMediaID
doesn't refer to an existing installation medium.
* @sample AmazonRDS.DeleteInstallationMedia
* @see AWS
* API Documentation
*/
@Override
public DeleteInstallationMediaResult deleteInstallationMedia(DeleteInstallationMediaRequest request) {
request = beforeClientExecution(request);
return executeDeleteInstallationMedia(request);
}
@SdkInternalApi
final DeleteInstallationMediaResult executeDeleteInstallationMedia(DeleteInstallationMediaRequest deleteInstallationMediaRequest) {
ExecutionContext executionContext = createExecutionContext(deleteInstallationMediaRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DeleteInstallationMediaRequestMarshaller().marshall(super.beforeMarshalling(deleteInstallationMediaRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "RDS");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DeleteInstallationMedia");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
StaxResponseHandler responseHandler = new StaxResponseHandler(
new DeleteInstallationMediaResultStaxUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Deletes an existing option group.
*
*
* @param deleteOptionGroupRequest
* @return Result of the DeleteOptionGroup operation returned by the service.
* @throws OptionGroupNotFoundException
* The specified option group could not be found.
* @throws InvalidOptionGroupStateException
* The option group isn't in the available state.
* @sample AmazonRDS.DeleteOptionGroup
* @see AWS API
* Documentation
*/
@Override
public DeleteOptionGroupResult deleteOptionGroup(DeleteOptionGroupRequest request) {
request = beforeClientExecution(request);
return executeDeleteOptionGroup(request);
}
@SdkInternalApi
final DeleteOptionGroupResult executeDeleteOptionGroup(DeleteOptionGroupRequest deleteOptionGroupRequest) {
ExecutionContext executionContext = createExecutionContext(deleteOptionGroupRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DeleteOptionGroupRequestMarshaller().marshall(super.beforeMarshalling(deleteOptionGroupRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "RDS");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DeleteOptionGroup");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
StaxResponseHandler responseHandler = new StaxResponseHandler(
new DeleteOptionGroupResultStaxUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Remove the association between one or more DBProxyTarget
data structures and a
* DBProxyTargetGroup
.
*
*
* @param deregisterDBProxyTargetsRequest
* @return Result of the DeregisterDBProxyTargets operation returned by the service.
* @throws DBProxyTargetNotFoundException
* The specified RDS DB instance or Aurora DB cluster isn't available for a proxy owned by your Amazon Web
* Services account in the specified Amazon Web Services Region.
* @throws DBProxyTargetGroupNotFoundException
* The specified target group isn't available for a proxy owned by your Amazon Web Services account in the
* specified Amazon Web Services Region.
* @throws DBProxyNotFoundException
* The specified proxy name doesn't correspond to a proxy owned by your Amazon Web Services account in the
* specified Amazon Web Services Region.
* @throws InvalidDBProxyStateException
* The requested operation can't be performed while the proxy is in this state.
* @sample AmazonRDS.DeregisterDBProxyTargets
* @see AWS
* API Documentation
*/
@Override
public DeregisterDBProxyTargetsResult deregisterDBProxyTargets(DeregisterDBProxyTargetsRequest request) {
request = beforeClientExecution(request);
return executeDeregisterDBProxyTargets(request);
}
@SdkInternalApi
final DeregisterDBProxyTargetsResult executeDeregisterDBProxyTargets(DeregisterDBProxyTargetsRequest deregisterDBProxyTargetsRequest) {
ExecutionContext executionContext = createExecutionContext(deregisterDBProxyTargetsRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DeregisterDBProxyTargetsRequestMarshaller().marshall(super.beforeMarshalling(deregisterDBProxyTargetsRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "RDS");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DeregisterDBProxyTargets");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
StaxResponseHandler responseHandler = new StaxResponseHandler(
new DeregisterDBProxyTargetsResultStaxUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Lists all of the attributes for a customer account. The attributes include Amazon RDS quotas for the account,
* such as the number of DB instances allowed. The description for a quota includes the quota name, current usage
* toward that quota, and the quota's maximum value.
*
*
* This command doesn't take any parameters.
*
*
* @param describeAccountAttributesRequest
* @return Result of the DescribeAccountAttributes operation returned by the service.
* @sample AmazonRDS.DescribeAccountAttributes
* @see AWS
* API Documentation
*/
@Override
public DescribeAccountAttributesResult describeAccountAttributes(DescribeAccountAttributesRequest request) {
request = beforeClientExecution(request);
return executeDescribeAccountAttributes(request);
}
@SdkInternalApi
final DescribeAccountAttributesResult executeDescribeAccountAttributes(DescribeAccountAttributesRequest describeAccountAttributesRequest) {
ExecutionContext executionContext = createExecutionContext(describeAccountAttributesRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DescribeAccountAttributesRequestMarshaller().marshall(super.beforeMarshalling(describeAccountAttributesRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "RDS");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DescribeAccountAttributes");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
StaxResponseHandler responseHandler = new StaxResponseHandler(
new DescribeAccountAttributesResultStaxUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
@Override
public DescribeAccountAttributesResult describeAccountAttributes() {
return describeAccountAttributes(new DescribeAccountAttributesRequest());
}
/**
*
* Lists the set of CA certificates provided by Amazon RDS for this Amazon Web Services account.
*
*
* @param describeCertificatesRequest
* @return Result of the DescribeCertificates operation returned by the service.
* @throws CertificateNotFoundException
* CertificateIdentifier
doesn't refer to an existing certificate.
* @sample AmazonRDS.DescribeCertificates
* @see AWS API
* Documentation
*/
@Override
public DescribeCertificatesResult describeCertificates(DescribeCertificatesRequest request) {
request = beforeClientExecution(request);
return executeDescribeCertificates(request);
}
@SdkInternalApi
final DescribeCertificatesResult executeDescribeCertificates(DescribeCertificatesRequest describeCertificatesRequest) {
ExecutionContext executionContext = createExecutionContext(describeCertificatesRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DescribeCertificatesRequestMarshaller().marshall(super.beforeMarshalling(describeCertificatesRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "RDS");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DescribeCertificates");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
StaxResponseHandler responseHandler = new StaxResponseHandler(
new DescribeCertificatesResultStaxUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
@Override
public DescribeCertificatesResult describeCertificates() {
return describeCertificates(new DescribeCertificatesRequest());
}
/**
*
* Returns information about custom Availability Zones (AZs).
*
*
* A custom AZ is an on-premises AZ that is integrated with a VMware vSphere cluster.
*
*
* For more information about RDS on VMware, see the RDS on VMware User
* Guide.
*
*
* @param describeCustomAvailabilityZonesRequest
* @return Result of the DescribeCustomAvailabilityZones operation returned by the service.
* @throws CustomAvailabilityZoneNotFoundException
* CustomAvailabilityZoneId
doesn't refer to an existing custom Availability Zone identifier.
* @sample AmazonRDS.DescribeCustomAvailabilityZones
* @see AWS API Documentation
*/
@Override
public DescribeCustomAvailabilityZonesResult describeCustomAvailabilityZones(DescribeCustomAvailabilityZonesRequest request) {
request = beforeClientExecution(request);
return executeDescribeCustomAvailabilityZones(request);
}
@SdkInternalApi
final DescribeCustomAvailabilityZonesResult executeDescribeCustomAvailabilityZones(
DescribeCustomAvailabilityZonesRequest describeCustomAvailabilityZonesRequest) {
ExecutionContext executionContext = createExecutionContext(describeCustomAvailabilityZonesRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DescribeCustomAvailabilityZonesRequestMarshaller().marshall(super.beforeMarshalling(describeCustomAvailabilityZonesRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "RDS");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DescribeCustomAvailabilityZones");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
StaxResponseHandler responseHandler = new StaxResponseHandler(
new DescribeCustomAvailabilityZonesResultStaxUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Returns information about backtracks for a DB cluster.
*
*
* For more information on Amazon Aurora, see What Is Amazon
* Aurora? in the Amazon Aurora User Guide.
*
*
*
* This action only applies to Aurora MySQL DB clusters.
*
*
*
* @param describeDBClusterBacktracksRequest
* @return Result of the DescribeDBClusterBacktracks operation returned by the service.
* @throws DBClusterNotFoundException
* DBClusterIdentifier
doesn't refer to an existing DB cluster.
* @throws DBClusterBacktrackNotFoundException
* BacktrackIdentifier
doesn't refer to an existing backtrack.
* @sample AmazonRDS.DescribeDBClusterBacktracks
* @see AWS API Documentation
*/
@Override
public DescribeDBClusterBacktracksResult describeDBClusterBacktracks(DescribeDBClusterBacktracksRequest request) {
request = beforeClientExecution(request);
return executeDescribeDBClusterBacktracks(request);
}
@SdkInternalApi
final DescribeDBClusterBacktracksResult executeDescribeDBClusterBacktracks(DescribeDBClusterBacktracksRequest describeDBClusterBacktracksRequest) {
ExecutionContext executionContext = createExecutionContext(describeDBClusterBacktracksRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DescribeDBClusterBacktracksRequestMarshaller().marshall(super.beforeMarshalling(describeDBClusterBacktracksRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "RDS");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DescribeDBClusterBacktracks");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
StaxResponseHandler responseHandler = new StaxResponseHandler(
new DescribeDBClusterBacktracksResultStaxUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Returns information about endpoints for an Amazon Aurora DB cluster.
*
*
*
* This action only applies to Aurora DB clusters.
*
*
*
* @param describeDBClusterEndpointsRequest
* @return Result of the DescribeDBClusterEndpoints operation returned by the service.
* @throws DBClusterNotFoundException
* DBClusterIdentifier
doesn't refer to an existing DB cluster.
* @sample AmazonRDS.DescribeDBClusterEndpoints
* @see AWS
* API Documentation
*/
@Override
public DescribeDBClusterEndpointsResult describeDBClusterEndpoints(DescribeDBClusterEndpointsRequest request) {
request = beforeClientExecution(request);
return executeDescribeDBClusterEndpoints(request);
}
@SdkInternalApi
final DescribeDBClusterEndpointsResult executeDescribeDBClusterEndpoints(DescribeDBClusterEndpointsRequest describeDBClusterEndpointsRequest) {
ExecutionContext executionContext = createExecutionContext(describeDBClusterEndpointsRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DescribeDBClusterEndpointsRequestMarshaller().marshall(super.beforeMarshalling(describeDBClusterEndpointsRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "RDS");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DescribeDBClusterEndpoints");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
StaxResponseHandler responseHandler = new StaxResponseHandler(
new DescribeDBClusterEndpointsResultStaxUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Returns a list of DBClusterParameterGroup
descriptions. If a
* DBClusterParameterGroupName
parameter is specified, the list will contain only the description of
* the specified DB cluster parameter group.
*
*
* For more information on Amazon Aurora, see What Is Amazon
* Aurora? in the Amazon Aurora User Guide.
*
*
*
* This action only applies to Aurora DB clusters.
*
*
*
* @param describeDBClusterParameterGroupsRequest
* @return Result of the DescribeDBClusterParameterGroups operation returned by the service.
* @throws DBParameterGroupNotFoundException
* DBParameterGroupName
doesn't refer to an existing DB parameter group.
* @sample AmazonRDS.DescribeDBClusterParameterGroups
* @see AWS API Documentation
*/
@Override
public DescribeDBClusterParameterGroupsResult describeDBClusterParameterGroups(DescribeDBClusterParameterGroupsRequest request) {
request = beforeClientExecution(request);
return executeDescribeDBClusterParameterGroups(request);
}
@SdkInternalApi
final DescribeDBClusterParameterGroupsResult executeDescribeDBClusterParameterGroups(
DescribeDBClusterParameterGroupsRequest describeDBClusterParameterGroupsRequest) {
ExecutionContext executionContext = createExecutionContext(describeDBClusterParameterGroupsRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DescribeDBClusterParameterGroupsRequestMarshaller().marshall(super.beforeMarshalling(describeDBClusterParameterGroupsRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "RDS");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DescribeDBClusterParameterGroups");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
StaxResponseHandler responseHandler = new StaxResponseHandler(
new DescribeDBClusterParameterGroupsResultStaxUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
@Override
public DescribeDBClusterParameterGroupsResult describeDBClusterParameterGroups() {
return describeDBClusterParameterGroups(new DescribeDBClusterParameterGroupsRequest());
}
/**
*
* Returns the detailed parameter list for a particular DB cluster parameter group.
*
*
* For more information on Amazon Aurora, see What Is Amazon
* Aurora? in the Amazon Aurora User Guide.
*
*
*
* This action only applies to Aurora DB clusters.
*
*
*
* @param describeDBClusterParametersRequest
* @return Result of the DescribeDBClusterParameters operation returned by the service.
* @throws DBParameterGroupNotFoundException
* DBParameterGroupName
doesn't refer to an existing DB parameter group.
* @sample AmazonRDS.DescribeDBClusterParameters
* @see AWS API Documentation
*/
@Override
public DescribeDBClusterParametersResult describeDBClusterParameters(DescribeDBClusterParametersRequest request) {
request = beforeClientExecution(request);
return executeDescribeDBClusterParameters(request);
}
@SdkInternalApi
final DescribeDBClusterParametersResult executeDescribeDBClusterParameters(DescribeDBClusterParametersRequest describeDBClusterParametersRequest) {
ExecutionContext executionContext = createExecutionContext(describeDBClusterParametersRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DescribeDBClusterParametersRequestMarshaller().marshall(super.beforeMarshalling(describeDBClusterParametersRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "RDS");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DescribeDBClusterParameters");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
StaxResponseHandler responseHandler = new StaxResponseHandler(
new DescribeDBClusterParametersResultStaxUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Returns a list of DB cluster snapshot attribute names and values for a manual DB cluster snapshot.
*
*
* When sharing snapshots with other Amazon Web Services accounts, DescribeDBClusterSnapshotAttributes
* returns the restore
attribute and a list of IDs for the Amazon Web Services accounts that are
* authorized to copy or restore the manual DB cluster snapshot. If all
is included in the list of
* values for the restore
attribute, then the manual DB cluster snapshot is public and can be copied or
* restored by all Amazon Web Services accounts.
*
*
* To add or remove access for an Amazon Web Services account to copy or restore a manual DB cluster snapshot, or to
* make the manual DB cluster snapshot public or private, use the ModifyDBClusterSnapshotAttribute
API
* action.
*
*
*
* This action only applies to Aurora DB clusters.
*
*
*
* @param describeDBClusterSnapshotAttributesRequest
* @return Result of the DescribeDBClusterSnapshotAttributes operation returned by the service.
* @throws DBClusterSnapshotNotFoundException
* DBClusterSnapshotIdentifier
doesn't refer to an existing DB cluster snapshot.
* @sample AmazonRDS.DescribeDBClusterSnapshotAttributes
* @see AWS API Documentation
*/
@Override
public DBClusterSnapshotAttributesResult describeDBClusterSnapshotAttributes(DescribeDBClusterSnapshotAttributesRequest request) {
request = beforeClientExecution(request);
return executeDescribeDBClusterSnapshotAttributes(request);
}
@SdkInternalApi
final DBClusterSnapshotAttributesResult executeDescribeDBClusterSnapshotAttributes(
DescribeDBClusterSnapshotAttributesRequest describeDBClusterSnapshotAttributesRequest) {
ExecutionContext executionContext = createExecutionContext(describeDBClusterSnapshotAttributesRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DescribeDBClusterSnapshotAttributesRequestMarshaller().marshall(super
.beforeMarshalling(describeDBClusterSnapshotAttributesRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "RDS");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DescribeDBClusterSnapshotAttributes");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
StaxResponseHandler responseHandler = new StaxResponseHandler(
new DBClusterSnapshotAttributesResultStaxUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Returns information about DB cluster snapshots. This API action supports pagination.
*
*
* For more information on Amazon Aurora, see What Is Amazon
* Aurora? in the Amazon Aurora User Guide.
*
*
*
* This action only applies to Aurora DB clusters.
*
*
*
* @param describeDBClusterSnapshotsRequest
* @return Result of the DescribeDBClusterSnapshots operation returned by the service.
* @throws DBClusterSnapshotNotFoundException
* DBClusterSnapshotIdentifier
doesn't refer to an existing DB cluster snapshot.
* @sample AmazonRDS.DescribeDBClusterSnapshots
* @see AWS
* API Documentation
*/
@Override
public DescribeDBClusterSnapshotsResult describeDBClusterSnapshots(DescribeDBClusterSnapshotsRequest request) {
request = beforeClientExecution(request);
return executeDescribeDBClusterSnapshots(request);
}
@SdkInternalApi
final DescribeDBClusterSnapshotsResult executeDescribeDBClusterSnapshots(DescribeDBClusterSnapshotsRequest describeDBClusterSnapshotsRequest) {
ExecutionContext executionContext = createExecutionContext(describeDBClusterSnapshotsRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DescribeDBClusterSnapshotsRequestMarshaller().marshall(super.beforeMarshalling(describeDBClusterSnapshotsRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "RDS");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DescribeDBClusterSnapshots");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
StaxResponseHandler responseHandler = new StaxResponseHandler(
new DescribeDBClusterSnapshotsResultStaxUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
@Override
public DescribeDBClusterSnapshotsResult describeDBClusterSnapshots() {
return describeDBClusterSnapshots(new DescribeDBClusterSnapshotsRequest());
}
/**
*
* Returns information about provisioned Aurora DB clusters. This API supports pagination.
*
*
* For more information on Amazon Aurora, see What Is Amazon
* Aurora? in the Amazon Aurora User Guide.
*
*
*
* This operation can also return information for Amazon Neptune DB instances and Amazon DocumentDB instances.
*
*
*
* @param describeDBClustersRequest
* @return Result of the DescribeDBClusters operation returned by the service.
* @throws DBClusterNotFoundException
* DBClusterIdentifier
doesn't refer to an existing DB cluster.
* @sample AmazonRDS.DescribeDBClusters
* @see AWS API
* Documentation
*/
@Override
public DescribeDBClustersResult describeDBClusters(DescribeDBClustersRequest request) {
request = beforeClientExecution(request);
return executeDescribeDBClusters(request);
}
@SdkInternalApi
final DescribeDBClustersResult executeDescribeDBClusters(DescribeDBClustersRequest describeDBClustersRequest) {
ExecutionContext executionContext = createExecutionContext(describeDBClustersRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DescribeDBClustersRequestMarshaller().marshall(super.beforeMarshalling(describeDBClustersRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "RDS");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DescribeDBClusters");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
StaxResponseHandler responseHandler = new StaxResponseHandler(
new DescribeDBClustersResultStaxUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
@Override
public DescribeDBClustersResult describeDBClusters() {
return describeDBClusters(new DescribeDBClustersRequest());
}
/**
*
* Returns a list of the available DB engines.
*
*
* @param describeDBEngineVersionsRequest
* @return Result of the DescribeDBEngineVersions operation returned by the service.
* @sample AmazonRDS.DescribeDBEngineVersions
* @see AWS
* API Documentation
*/
@Override
public DescribeDBEngineVersionsResult describeDBEngineVersions(DescribeDBEngineVersionsRequest request) {
request = beforeClientExecution(request);
return executeDescribeDBEngineVersions(request);
}
@SdkInternalApi
final DescribeDBEngineVersionsResult executeDescribeDBEngineVersions(DescribeDBEngineVersionsRequest describeDBEngineVersionsRequest) {
ExecutionContext executionContext = createExecutionContext(describeDBEngineVersionsRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DescribeDBEngineVersionsRequestMarshaller().marshall(super.beforeMarshalling(describeDBEngineVersionsRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "RDS");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DescribeDBEngineVersions");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
StaxResponseHandler responseHandler = new StaxResponseHandler(
new DescribeDBEngineVersionsResultStaxUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
@Override
public DescribeDBEngineVersionsResult describeDBEngineVersions() {
return describeDBEngineVersions(new DescribeDBEngineVersionsRequest());
}
/**
*
* Displays backups for both current and deleted instances. For example, use this operation to find details about
* automated backups for previously deleted instances. Current instances with retention periods greater than zero
* (0) are returned for both the DescribeDBInstanceAutomatedBackups
and
* DescribeDBInstances
operations.
*
*
* All parameters are optional.
*
*
* @param describeDBInstanceAutomatedBackupsRequest
* Parameter input for DescribeDBInstanceAutomatedBackups.
* @return Result of the DescribeDBInstanceAutomatedBackups operation returned by the service.
* @throws DBInstanceAutomatedBackupNotFoundException
* No automated backup for this DB instance was found.
* @sample AmazonRDS.DescribeDBInstanceAutomatedBackups
* @see AWS API Documentation
*/
@Override
public DescribeDBInstanceAutomatedBackupsResult describeDBInstanceAutomatedBackups(DescribeDBInstanceAutomatedBackupsRequest request) {
request = beforeClientExecution(request);
return executeDescribeDBInstanceAutomatedBackups(request);
}
@SdkInternalApi
final DescribeDBInstanceAutomatedBackupsResult executeDescribeDBInstanceAutomatedBackups(
DescribeDBInstanceAutomatedBackupsRequest describeDBInstanceAutomatedBackupsRequest) {
ExecutionContext executionContext = createExecutionContext(describeDBInstanceAutomatedBackupsRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DescribeDBInstanceAutomatedBackupsRequestMarshaller()
.marshall(super.beforeMarshalling(describeDBInstanceAutomatedBackupsRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "RDS");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DescribeDBInstanceAutomatedBackups");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
StaxResponseHandler responseHandler = new StaxResponseHandler(
new DescribeDBInstanceAutomatedBackupsResultStaxUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Returns information about provisioned RDS instances. This API supports pagination.
*
*
*
* This operation can also return information for Amazon Neptune DB instances and Amazon DocumentDB instances.
*
*
*
* @param describeDBInstancesRequest
* @return Result of the DescribeDBInstances operation returned by the service.
* @throws DBInstanceNotFoundException
* DBInstanceIdentifier
doesn't refer to an existing DB instance.
* @sample AmazonRDS.DescribeDBInstances
* @see AWS API
* Documentation
*/
@Override
public DescribeDBInstancesResult describeDBInstances(DescribeDBInstancesRequest request) {
request = beforeClientExecution(request);
return executeDescribeDBInstances(request);
}
@SdkInternalApi
final DescribeDBInstancesResult executeDescribeDBInstances(DescribeDBInstancesRequest describeDBInstancesRequest) {
ExecutionContext executionContext = createExecutionContext(describeDBInstancesRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DescribeDBInstancesRequestMarshaller().marshall(super.beforeMarshalling(describeDBInstancesRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "RDS");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DescribeDBInstances");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
StaxResponseHandler responseHandler = new StaxResponseHandler