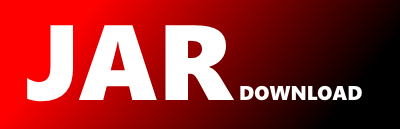
com.amazonaws.services.rds.model.DBInstance Maven / Gradle / Ivy
/*
* Copyright 2018-2023 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.rds.model;
import java.io.Serializable;
import javax.annotation.Generated;
/**
*
* Contains the details of an Amazon RDS DB instance.
*
*
* This data type is used as a response element in the operations CreateDBInstance
,
* CreateDBInstanceReadReplica
, DeleteDBInstance
, DescribeDBInstances
,
* ModifyDBInstance
, PromoteReadReplica
, RebootDBInstance
,
* RestoreDBInstanceFromDBSnapshot
, RestoreDBInstanceFromS3
,
* RestoreDBInstanceToPointInTime
, StartDBInstance
, and StopDBInstance
.
*
*
* @see AWS API
* Documentation
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class DBInstance implements Serializable, Cloneable {
/**
*
* The user-supplied database identifier. This identifier is the unique key that identifies a DB instance.
*
*/
private String dBInstanceIdentifier;
/**
*
* The name of the compute and memory capacity class of the DB instance.
*
*/
private String dBInstanceClass;
/**
*
* The database engine used for this DB instance.
*
*/
private String engine;
/**
*
* The current state of this database.
*
*
* For information about DB instance statuses, see Viewing DB instance status in the Amazon RDS User Guide.
*
*/
private String dBInstanceStatus;
/**
*
* The time when a stopped DB instance is restarted automatically.
*
*/
private java.util.Date automaticRestartTime;
/**
*
* The master username for the DB instance.
*
*/
private String masterUsername;
/**
*
* Contains the initial database name that you provided (if required) when you created the DB instance. This name is
* returned for the life of your DB instance. For an RDS for Oracle CDB instance, the name identifies the PDB rather
* than the CDB.
*
*/
private String dBName;
/**
*
* The connection endpoint for the DB instance.
*
*
*
* The endpoint might not be shown for instances with the status of creating
.
*
*
*/
private Endpoint endpoint;
/**
*
* The amount of storage in gibibytes (GiB) allocated for the DB instance.
*
*/
private Integer allocatedStorage;
/**
*
* The date and time when the DB instance was created.
*
*/
private java.util.Date instanceCreateTime;
/**
*
* The daily time range during which automated backups are created if automated backups are enabled, as determined
* by the BackupRetentionPeriod
.
*
*/
private String preferredBackupWindow;
/**
*
* The number of days for which automatic DB snapshots are retained.
*
*/
private Integer backupRetentionPeriod;
/**
*
* A list of DB security group elements containing DBSecurityGroup.Name
and
* DBSecurityGroup.Status
subelements.
*
*/
private com.amazonaws.internal.SdkInternalList dBSecurityGroups;
/**
*
* The list of Amazon EC2 VPC security groups that the DB instance belongs to.
*
*/
private com.amazonaws.internal.SdkInternalList vpcSecurityGroups;
/**
*
* The list of DB parameter groups applied to this DB instance.
*
*/
private com.amazonaws.internal.SdkInternalList dBParameterGroups;
/**
*
* The name of the Availability Zone where the DB instance is located.
*
*/
private String availabilityZone;
/**
*
* Information about the subnet group associated with the DB instance, including the name, description, and subnets
* in the subnet group.
*
*/
private DBSubnetGroup dBSubnetGroup;
/**
*
* The weekly time range during which system maintenance can occur, in Universal Coordinated Time (UTC).
*
*/
private String preferredMaintenanceWindow;
/**
*
* Information about pending changes to the DB instance. This information is returned only when there are pending
* changes. Specific changes are identified by subelements.
*
*/
private PendingModifiedValues pendingModifiedValues;
/**
*
* The latest time to which a database in this DB instance can be restored with point-in-time restore.
*
*/
private java.util.Date latestRestorableTime;
/**
*
* Indicates whether the DB instance is a Multi-AZ deployment. This setting doesn't apply to RDS Custom DB
* instances.
*
*/
private Boolean multiAZ;
/**
*
* The version of the database engine.
*
*/
private String engineVersion;
/**
*
* Indicates whether minor version patches are applied automatically.
*
*/
private Boolean autoMinorVersionUpgrade;
/**
*
* The identifier of the source DB instance if this DB instance is a read replica.
*
*/
private String readReplicaSourceDBInstanceIdentifier;
/**
*
* The identifiers of the read replicas associated with this DB instance.
*
*/
private com.amazonaws.internal.SdkInternalList readReplicaDBInstanceIdentifiers;
/**
*
* The identifiers of Aurora DB clusters to which the RDS DB instance is replicated as a read replica. For example,
* when you create an Aurora read replica of an RDS for MySQL DB instance, the Aurora MySQL DB cluster for the
* Aurora read replica is shown. This output doesn't contain information about cross-Region Aurora read replicas.
*
*
*
* Currently, each RDS DB instance can have only one Aurora read replica.
*
*
*/
private com.amazonaws.internal.SdkInternalList readReplicaDBClusterIdentifiers;
/**
*
* The open mode of an Oracle read replica. The default is open-read-only
. For more information, see Working with Oracle Read
* Replicas for Amazon RDS in the Amazon RDS User Guide.
*
*
*
* This attribute is only supported in RDS for Oracle.
*
*
*/
private String replicaMode;
/**
*
* The license model information for this DB instance. This setting doesn't apply to RDS Custom DB instances.
*
*/
private String licenseModel;
/**
*
* The Provisioned IOPS (I/O operations per second) value for the DB instance.
*
*/
private Integer iops;
/**
*
* The list of option group memberships for this DB instance.
*
*/
private com.amazonaws.internal.SdkInternalList optionGroupMemberships;
/**
*
* If present, specifies the name of the character set that this instance is associated with.
*
*/
private String characterSetName;
/**
*
* The name of the NCHAR character set for the Oracle DB instance. This character set specifies the Unicode encoding
* for data stored in table columns of type NCHAR, NCLOB, or NVARCHAR2.
*
*/
private String ncharCharacterSetName;
/**
*
* If present, specifies the name of the secondary Availability Zone for a DB instance with multi-AZ support.
*
*/
private String secondaryAvailabilityZone;
/**
*
* Indicates whether the DB instance is publicly accessible.
*
*
* When the DB cluster is publicly accessible, its Domain Name System (DNS) endpoint resolves to the private IP
* address from within the DB cluster's virtual private cloud (VPC). It resolves to the public IP address from
* outside of the DB cluster's VPC. Access to the DB cluster is ultimately controlled by the security group it uses.
* That public access isn't permitted if the security group assigned to the DB cluster doesn't permit it.
*
*
* When the DB instance isn't publicly accessible, it is an internal DB instance with a DNS name that resolves to a
* private IP address.
*
*
* For more information, see CreateDBInstance.
*
*/
private Boolean publiclyAccessible;
/**
*
* The status of a read replica. If the DB instance isn't a read replica, the value is blank.
*
*/
private com.amazonaws.internal.SdkInternalList statusInfos;
/**
*
* The storage type associated with the DB instance.
*
*/
private String storageType;
/**
*
* The ARN from the key store with which the instance is associated for TDE encryption.
*
*/
private String tdeCredentialArn;
/**
*
* The port that the DB instance listens on. If the DB instance is part of a DB cluster, this can be a different
* port than the DB cluster port.
*
*/
private Integer dbInstancePort;
/**
*
* If the DB instance is a member of a DB cluster, indicates the name of the DB cluster that the DB instance is a
* member of.
*
*/
private String dBClusterIdentifier;
/**
*
* Indicates whether the DB instance is encrypted.
*
*/
private Boolean storageEncrypted;
/**
*
* If StorageEncrypted
is enabled, the Amazon Web Services KMS key identifier for the encrypted DB
* instance.
*
*
* The Amazon Web Services KMS key identifier is the key ARN, key ID, alias ARN, or alias name for the KMS key.
*
*/
private String kmsKeyId;
/**
*
* The Amazon Web Services Region-unique, immutable identifier for the DB instance. This identifier is found in
* Amazon Web Services CloudTrail log entries whenever the Amazon Web Services KMS key for the DB instance is
* accessed.
*
*/
private String dbiResourceId;
/**
*
* The identifier of the CA certificate for this DB instance.
*
*
* For more information, see Using SSL/TLS to encrypt a
* connection to a DB instance in the Amazon RDS User Guide and Using SSL/TLS to
* encrypt a connection to a DB cluster in the Amazon Aurora User Guide.
*
*/
private String cACertificateIdentifier;
/**
*
* The Active Directory Domain membership records associated with the DB instance.
*
*/
private com.amazonaws.internal.SdkInternalList domainMemberships;
/**
*
* Indicates whether tags are copied from the DB instance to snapshots of the DB instance.
*
*
* This setting doesn't apply to Amazon Aurora DB instances. Copying tags to snapshots is managed by the DB cluster.
* Setting this value for an Aurora DB instance has no effect on the DB cluster setting. For more information, see
* DBCluster
.
*
*/
private Boolean copyTagsToSnapshot;
/**
*
* The interval, in seconds, between points when Enhanced Monitoring metrics are collected for the DB instance.
*
*/
private Integer monitoringInterval;
/**
*
* The Amazon Resource Name (ARN) of the Amazon CloudWatch Logs log stream that receives the Enhanced Monitoring
* metrics data for the DB instance.
*
*/
private String enhancedMonitoringResourceArn;
/**
*
* The ARN for the IAM role that permits RDS to send Enhanced Monitoring metrics to Amazon CloudWatch Logs.
*
*/
private String monitoringRoleArn;
/**
*
* The order of priority in which an Aurora Replica is promoted to the primary instance after a failure of the
* existing primary instance. For more information, see Fault Tolerance for an Aurora DB Cluster in the Amazon Aurora User Guide.
*
*/
private Integer promotionTier;
/**
*
* The Amazon Resource Name (ARN) for the DB instance.
*
*/
private String dBInstanceArn;
/**
*
* The time zone of the DB instance. In most cases, the Timezone
element is empty.
* Timezone
content appears only for Microsoft SQL Server DB instances that were created with a time
* zone specified.
*
*/
private String timezone;
/**
*
* Indicates whether mapping of Amazon Web Services Identity and Access Management (IAM) accounts to database
* accounts is enabled for the DB instance.
*
*
* For a list of engine versions that support IAM database authentication, see IAM database authentication in the Amazon RDS User Guide and IAM database authentication in Aurora in the Amazon Aurora User Guide.
*
*/
private Boolean iAMDatabaseAuthenticationEnabled;
/**
*
* Indicates whether Performance Insights is enabled for the DB instance.
*
*/
private Boolean performanceInsightsEnabled;
/**
*
* The Amazon Web Services KMS key identifier for encryption of Performance Insights data.
*
*
* The Amazon Web Services KMS key identifier is the key ARN, key ID, alias ARN, or alias name for the KMS key.
*
*/
private String performanceInsightsKMSKeyId;
/**
*
* The number of days to retain Performance Insights data.
*
*
* Valid Values:
*
*
* -
*
* 7
*
*
* -
*
* month * 31, where month is a number of months from 1-23. Examples: 93
(3 months * 31),
* 341
(11 months * 31), 589
(19 months * 31)
*
*
* -
*
* 731
*
*
*
*
* Default: 7
days
*
*/
private Integer performanceInsightsRetentionPeriod;
/**
*
* A list of log types that this DB instance is configured to export to CloudWatch Logs.
*
*
* Log types vary by DB engine. For information about the log types for each DB engine, see Monitoring Amazon RDS log
* files in the Amazon RDS User Guide.
*
*/
private com.amazonaws.internal.SdkInternalList enabledCloudwatchLogsExports;
/**
*
* The number of CPU cores and the number of threads per core for the DB instance class of the DB instance.
*
*/
private com.amazonaws.internal.SdkInternalList processorFeatures;
/**
*
* Indicates whether the DB instance has deletion protection enabled. The database can't be deleted when deletion
* protection is enabled. For more information, see Deleting a DB
* Instance.
*
*/
private Boolean deletionProtection;
/**
*
* The Amazon Web Services Identity and Access Management (IAM) roles associated with the DB instance.
*
*/
private com.amazonaws.internal.SdkInternalList associatedRoles;
/**
*
* The listener connection endpoint for SQL Server Always On.
*
*/
private Endpoint listenerEndpoint;
/**
*
* The upper limit in gibibytes (GiB) to which Amazon RDS can automatically scale the storage of the DB instance.
*
*/
private Integer maxAllocatedStorage;
private com.amazonaws.internal.SdkInternalList tagList;
/**
*
* The list of replicated automated backups associated with the DB instance.
*
*/
private com.amazonaws.internal.SdkInternalList dBInstanceAutomatedBackupsReplications;
/**
*
* Indicates whether a customer-owned IP address (CoIP) is enabled for an RDS on Outposts DB instance.
*
*
* A CoIP provides local or external connectivity to resources in your Outpost subnets through your
* on-premises network. For some use cases, a CoIP can provide lower latency for connections to the DB instance from
* outside of its virtual private cloud (VPC) on your local network.
*
*
* For more information about RDS on Outposts, see Working with Amazon RDS on
* Amazon Web Services Outposts in the Amazon RDS User Guide.
*
*
* For more information about CoIPs, see Customer-owned IP
* addresses in the Amazon Web Services Outposts User Guide.
*
*/
private Boolean customerOwnedIpEnabled;
/**
*
* The Amazon Resource Name (ARN) of the recovery point in Amazon Web Services Backup.
*
*/
private String awsBackupRecoveryPointArn;
/**
*
* The status of the database activity stream.
*
*/
private String activityStreamStatus;
/**
*
* The Amazon Web Services KMS key identifier used for encrypting messages in the database activity stream. The
* Amazon Web Services KMS key identifier is the key ARN, key ID, alias ARN, or alias name for the KMS key.
*
*/
private String activityStreamKmsKeyId;
/**
*
* The name of the Amazon Kinesis data stream used for the database activity stream.
*
*/
private String activityStreamKinesisStreamName;
/**
*
* The mode of the database activity stream. Database events such as a change or access generate an activity stream
* event. RDS for Oracle always handles these events asynchronously.
*
*/
private String activityStreamMode;
/**
*
* Indicates whether engine-native audit fields are included in the database activity stream.
*
*/
private Boolean activityStreamEngineNativeAuditFieldsIncluded;
/**
*
* The automation mode of the RDS Custom DB instance: full
or all paused
. If
* full
, the DB instance automates monitoring and instance recovery. If all paused
, the
* instance pauses automation for the duration set by --resume-full-automation-mode-minutes
.
*
*/
private String automationMode;
/**
*
* The number of minutes to pause the automation. When the time period ends, RDS Custom resumes full automation. The
* minimum value is 60 (default). The maximum value is 1,440.
*
*/
private java.util.Date resumeFullAutomationModeTime;
/**
*
* The instance profile associated with the underlying Amazon EC2 instance of an RDS Custom DB instance. The
* instance profile must meet the following requirements:
*
*
* -
*
* The profile must exist in your account.
*
*
* -
*
* The profile must have an IAM role that Amazon EC2 has permissions to assume.
*
*
* -
*
* The instance profile name and the associated IAM role name must start with the prefix AWSRDSCustom
.
*
*
*
*
* For the list of permissions required for the IAM role, see
* Configure IAM and your VPC in the Amazon RDS User Guide.
*
*/
private String customIamInstanceProfile;
/**
*
* The location where automated backups and manual snapshots are stored: Amazon Web Services Outposts or the Amazon
* Web Services Region.
*
*/
private String backupTarget;
/**
*
* The network type of the DB instance.
*
*
* The network type is determined by the DBSubnetGroup
specified for the DB instance. A
* DBSubnetGroup
can support only the IPv4 protocol or the IPv4 and the IPv6 protocols (
* DUAL
).
*
*
* For more information, see Working
* with a DB instance in a VPC in the Amazon RDS User Guide and
* Working with a DB instance in a VPC in the Amazon Aurora User Guide.
*
*
* Valid Values: IPV4 | DUAL
*
*/
private String networkType;
/**
*
* The status of the policy state of the activity stream.
*
*/
private String activityStreamPolicyStatus;
/**
*
* The storage throughput for the DB instance.
*
*
* This setting applies only to the gp3
storage type.
*
*/
private Integer storageThroughput;
/**
*
* The Oracle system ID (Oracle SID) for a container database (CDB). The Oracle SID is also the name of the CDB.
* This setting is only valid for RDS Custom DB instances.
*
*/
private String dBSystemId;
/**
*
* The secret managed by RDS in Amazon Web Services Secrets Manager for the master user password.
*
*
* For more information, see Password management with
* Amazon Web Services Secrets Manager in the Amazon RDS User Guide.
*
*/
private MasterUserSecret masterUserSecret;
/**
*
* The details of the DB instance's server certificate.
*
*/
private CertificateDetails certificateDetails;
/**
*
* The identifier of the source DB cluster if this DB instance is a read replica.
*
*/
private String readReplicaSourceDBClusterIdentifier;
/**
*
* The progress of the storage optimization operation as a percentage.
*
*/
private String percentProgress;
/**
*
* The user-supplied database identifier. This identifier is the unique key that identifies a DB instance.
*
*
* @param dBInstanceIdentifier
* The user-supplied database identifier. This identifier is the unique key that identifies a DB instance.
*/
public void setDBInstanceIdentifier(String dBInstanceIdentifier) {
this.dBInstanceIdentifier = dBInstanceIdentifier;
}
/**
*
* The user-supplied database identifier. This identifier is the unique key that identifies a DB instance.
*
*
* @return The user-supplied database identifier. This identifier is the unique key that identifies a DB instance.
*/
public String getDBInstanceIdentifier() {
return this.dBInstanceIdentifier;
}
/**
*
* The user-supplied database identifier. This identifier is the unique key that identifies a DB instance.
*
*
* @param dBInstanceIdentifier
* The user-supplied database identifier. This identifier is the unique key that identifies a DB instance.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DBInstance withDBInstanceIdentifier(String dBInstanceIdentifier) {
setDBInstanceIdentifier(dBInstanceIdentifier);
return this;
}
/**
*
* The name of the compute and memory capacity class of the DB instance.
*
*
* @param dBInstanceClass
* The name of the compute and memory capacity class of the DB instance.
*/
public void setDBInstanceClass(String dBInstanceClass) {
this.dBInstanceClass = dBInstanceClass;
}
/**
*
* The name of the compute and memory capacity class of the DB instance.
*
*
* @return The name of the compute and memory capacity class of the DB instance.
*/
public String getDBInstanceClass() {
return this.dBInstanceClass;
}
/**
*
* The name of the compute and memory capacity class of the DB instance.
*
*
* @param dBInstanceClass
* The name of the compute and memory capacity class of the DB instance.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DBInstance withDBInstanceClass(String dBInstanceClass) {
setDBInstanceClass(dBInstanceClass);
return this;
}
/**
*
* The database engine used for this DB instance.
*
*
* @param engine
* The database engine used for this DB instance.
*/
public void setEngine(String engine) {
this.engine = engine;
}
/**
*
* The database engine used for this DB instance.
*
*
* @return The database engine used for this DB instance.
*/
public String getEngine() {
return this.engine;
}
/**
*
* The database engine used for this DB instance.
*
*
* @param engine
* The database engine used for this DB instance.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DBInstance withEngine(String engine) {
setEngine(engine);
return this;
}
/**
*
* The current state of this database.
*
*
* For information about DB instance statuses, see Viewing DB instance status in the Amazon RDS User Guide.
*
*
* @param dBInstanceStatus
* The current state of this database.
*
* For information about DB instance statuses, see Viewing DB instance status in the Amazon RDS User Guide.
*/
public void setDBInstanceStatus(String dBInstanceStatus) {
this.dBInstanceStatus = dBInstanceStatus;
}
/**
*
* The current state of this database.
*
*
* For information about DB instance statuses, see Viewing DB instance status in the Amazon RDS User Guide.
*
*
* @return The current state of this database.
*
* For information about DB instance statuses, see Viewing DB instance status in the Amazon RDS User Guide.
*/
public String getDBInstanceStatus() {
return this.dBInstanceStatus;
}
/**
*
* The current state of this database.
*
*
* For information about DB instance statuses, see Viewing DB instance status in the Amazon RDS User Guide.
*
*
* @param dBInstanceStatus
* The current state of this database.
*
* For information about DB instance statuses, see Viewing DB instance status in the Amazon RDS User Guide.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DBInstance withDBInstanceStatus(String dBInstanceStatus) {
setDBInstanceStatus(dBInstanceStatus);
return this;
}
/**
*
* The time when a stopped DB instance is restarted automatically.
*
*
* @param automaticRestartTime
* The time when a stopped DB instance is restarted automatically.
*/
public void setAutomaticRestartTime(java.util.Date automaticRestartTime) {
this.automaticRestartTime = automaticRestartTime;
}
/**
*
* The time when a stopped DB instance is restarted automatically.
*
*
* @return The time when a stopped DB instance is restarted automatically.
*/
public java.util.Date getAutomaticRestartTime() {
return this.automaticRestartTime;
}
/**
*
* The time when a stopped DB instance is restarted automatically.
*
*
* @param automaticRestartTime
* The time when a stopped DB instance is restarted automatically.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DBInstance withAutomaticRestartTime(java.util.Date automaticRestartTime) {
setAutomaticRestartTime(automaticRestartTime);
return this;
}
/**
*
* The master username for the DB instance.
*
*
* @param masterUsername
* The master username for the DB instance.
*/
public void setMasterUsername(String masterUsername) {
this.masterUsername = masterUsername;
}
/**
*
* The master username for the DB instance.
*
*
* @return The master username for the DB instance.
*/
public String getMasterUsername() {
return this.masterUsername;
}
/**
*
* The master username for the DB instance.
*
*
* @param masterUsername
* The master username for the DB instance.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DBInstance withMasterUsername(String masterUsername) {
setMasterUsername(masterUsername);
return this;
}
/**
*
* Contains the initial database name that you provided (if required) when you created the DB instance. This name is
* returned for the life of your DB instance. For an RDS for Oracle CDB instance, the name identifies the PDB rather
* than the CDB.
*
*
* @param dBName
* Contains the initial database name that you provided (if required) when you created the DB instance. This
* name is returned for the life of your DB instance. For an RDS for Oracle CDB instance, the name identifies
* the PDB rather than the CDB.
*/
public void setDBName(String dBName) {
this.dBName = dBName;
}
/**
*
* Contains the initial database name that you provided (if required) when you created the DB instance. This name is
* returned for the life of your DB instance. For an RDS for Oracle CDB instance, the name identifies the PDB rather
* than the CDB.
*
*
* @return Contains the initial database name that you provided (if required) when you created the DB instance. This
* name is returned for the life of your DB instance. For an RDS for Oracle CDB instance, the name
* identifies the PDB rather than the CDB.
*/
public String getDBName() {
return this.dBName;
}
/**
*
* Contains the initial database name that you provided (if required) when you created the DB instance. This name is
* returned for the life of your DB instance. For an RDS for Oracle CDB instance, the name identifies the PDB rather
* than the CDB.
*
*
* @param dBName
* Contains the initial database name that you provided (if required) when you created the DB instance. This
* name is returned for the life of your DB instance. For an RDS for Oracle CDB instance, the name identifies
* the PDB rather than the CDB.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DBInstance withDBName(String dBName) {
setDBName(dBName);
return this;
}
/**
*
* The connection endpoint for the DB instance.
*
*
*
* The endpoint might not be shown for instances with the status of creating
.
*
*
*
* @param endpoint
* The connection endpoint for the DB instance.
*
* The endpoint might not be shown for instances with the status of creating
.
*
*/
public void setEndpoint(Endpoint endpoint) {
this.endpoint = endpoint;
}
/**
*
* The connection endpoint for the DB instance.
*
*
*
* The endpoint might not be shown for instances with the status of creating
.
*
*
*
* @return The connection endpoint for the DB instance.
*
* The endpoint might not be shown for instances with the status of creating
.
*
*/
public Endpoint getEndpoint() {
return this.endpoint;
}
/**
*
* The connection endpoint for the DB instance.
*
*
*
* The endpoint might not be shown for instances with the status of creating
.
*
*
*
* @param endpoint
* The connection endpoint for the DB instance.
*
* The endpoint might not be shown for instances with the status of creating
.
*
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DBInstance withEndpoint(Endpoint endpoint) {
setEndpoint(endpoint);
return this;
}
/**
*
* The amount of storage in gibibytes (GiB) allocated for the DB instance.
*
*
* @param allocatedStorage
* The amount of storage in gibibytes (GiB) allocated for the DB instance.
*/
public void setAllocatedStorage(Integer allocatedStorage) {
this.allocatedStorage = allocatedStorage;
}
/**
*
* The amount of storage in gibibytes (GiB) allocated for the DB instance.
*
*
* @return The amount of storage in gibibytes (GiB) allocated for the DB instance.
*/
public Integer getAllocatedStorage() {
return this.allocatedStorage;
}
/**
*
* The amount of storage in gibibytes (GiB) allocated for the DB instance.
*
*
* @param allocatedStorage
* The amount of storage in gibibytes (GiB) allocated for the DB instance.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DBInstance withAllocatedStorage(Integer allocatedStorage) {
setAllocatedStorage(allocatedStorage);
return this;
}
/**
*
* The date and time when the DB instance was created.
*
*
* @param instanceCreateTime
* The date and time when the DB instance was created.
*/
public void setInstanceCreateTime(java.util.Date instanceCreateTime) {
this.instanceCreateTime = instanceCreateTime;
}
/**
*
* The date and time when the DB instance was created.
*
*
* @return The date and time when the DB instance was created.
*/
public java.util.Date getInstanceCreateTime() {
return this.instanceCreateTime;
}
/**
*
* The date and time when the DB instance was created.
*
*
* @param instanceCreateTime
* The date and time when the DB instance was created.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DBInstance withInstanceCreateTime(java.util.Date instanceCreateTime) {
setInstanceCreateTime(instanceCreateTime);
return this;
}
/**
*
* The daily time range during which automated backups are created if automated backups are enabled, as determined
* by the BackupRetentionPeriod
.
*
*
* @param preferredBackupWindow
* The daily time range during which automated backups are created if automated backups are enabled, as
* determined by the BackupRetentionPeriod
.
*/
public void setPreferredBackupWindow(String preferredBackupWindow) {
this.preferredBackupWindow = preferredBackupWindow;
}
/**
*
* The daily time range during which automated backups are created if automated backups are enabled, as determined
* by the BackupRetentionPeriod
.
*
*
* @return The daily time range during which automated backups are created if automated backups are enabled, as
* determined by the BackupRetentionPeriod
.
*/
public String getPreferredBackupWindow() {
return this.preferredBackupWindow;
}
/**
*
* The daily time range during which automated backups are created if automated backups are enabled, as determined
* by the BackupRetentionPeriod
.
*
*
* @param preferredBackupWindow
* The daily time range during which automated backups are created if automated backups are enabled, as
* determined by the BackupRetentionPeriod
.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DBInstance withPreferredBackupWindow(String preferredBackupWindow) {
setPreferredBackupWindow(preferredBackupWindow);
return this;
}
/**
*
* The number of days for which automatic DB snapshots are retained.
*
*
* @param backupRetentionPeriod
* The number of days for which automatic DB snapshots are retained.
*/
public void setBackupRetentionPeriod(Integer backupRetentionPeriod) {
this.backupRetentionPeriod = backupRetentionPeriod;
}
/**
*
* The number of days for which automatic DB snapshots are retained.
*
*
* @return The number of days for which automatic DB snapshots are retained.
*/
public Integer getBackupRetentionPeriod() {
return this.backupRetentionPeriod;
}
/**
*
* The number of days for which automatic DB snapshots are retained.
*
*
* @param backupRetentionPeriod
* The number of days for which automatic DB snapshots are retained.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DBInstance withBackupRetentionPeriod(Integer backupRetentionPeriod) {
setBackupRetentionPeriod(backupRetentionPeriod);
return this;
}
/**
*
* A list of DB security group elements containing DBSecurityGroup.Name
and
* DBSecurityGroup.Status
subelements.
*
*
* @return A list of DB security group elements containing DBSecurityGroup.Name
and
* DBSecurityGroup.Status
subelements.
*/
public java.util.List getDBSecurityGroups() {
if (dBSecurityGroups == null) {
dBSecurityGroups = new com.amazonaws.internal.SdkInternalList();
}
return dBSecurityGroups;
}
/**
*
* A list of DB security group elements containing DBSecurityGroup.Name
and
* DBSecurityGroup.Status
subelements.
*
*
* @param dBSecurityGroups
* A list of DB security group elements containing DBSecurityGroup.Name
and
* DBSecurityGroup.Status
subelements.
*/
public void setDBSecurityGroups(java.util.Collection dBSecurityGroups) {
if (dBSecurityGroups == null) {
this.dBSecurityGroups = null;
return;
}
this.dBSecurityGroups = new com.amazonaws.internal.SdkInternalList(dBSecurityGroups);
}
/**
*
* A list of DB security group elements containing DBSecurityGroup.Name
and
* DBSecurityGroup.Status
subelements.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setDBSecurityGroups(java.util.Collection)} or {@link #withDBSecurityGroups(java.util.Collection)} if you
* want to override the existing values.
*
*
* @param dBSecurityGroups
* A list of DB security group elements containing DBSecurityGroup.Name
and
* DBSecurityGroup.Status
subelements.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DBInstance withDBSecurityGroups(DBSecurityGroupMembership... dBSecurityGroups) {
if (this.dBSecurityGroups == null) {
setDBSecurityGroups(new com.amazonaws.internal.SdkInternalList(dBSecurityGroups.length));
}
for (DBSecurityGroupMembership ele : dBSecurityGroups) {
this.dBSecurityGroups.add(ele);
}
return this;
}
/**
*
* A list of DB security group elements containing DBSecurityGroup.Name
and
* DBSecurityGroup.Status
subelements.
*
*
* @param dBSecurityGroups
* A list of DB security group elements containing DBSecurityGroup.Name
and
* DBSecurityGroup.Status
subelements.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DBInstance withDBSecurityGroups(java.util.Collection dBSecurityGroups) {
setDBSecurityGroups(dBSecurityGroups);
return this;
}
/**
*
* The list of Amazon EC2 VPC security groups that the DB instance belongs to.
*
*
* @return The list of Amazon EC2 VPC security groups that the DB instance belongs to.
*/
public java.util.List getVpcSecurityGroups() {
if (vpcSecurityGroups == null) {
vpcSecurityGroups = new com.amazonaws.internal.SdkInternalList();
}
return vpcSecurityGroups;
}
/**
*
* The list of Amazon EC2 VPC security groups that the DB instance belongs to.
*
*
* @param vpcSecurityGroups
* The list of Amazon EC2 VPC security groups that the DB instance belongs to.
*/
public void setVpcSecurityGroups(java.util.Collection vpcSecurityGroups) {
if (vpcSecurityGroups == null) {
this.vpcSecurityGroups = null;
return;
}
this.vpcSecurityGroups = new com.amazonaws.internal.SdkInternalList(vpcSecurityGroups);
}
/**
*
* The list of Amazon EC2 VPC security groups that the DB instance belongs to.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setVpcSecurityGroups(java.util.Collection)} or {@link #withVpcSecurityGroups(java.util.Collection)} if
* you want to override the existing values.
*
*
* @param vpcSecurityGroups
* The list of Amazon EC2 VPC security groups that the DB instance belongs to.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DBInstance withVpcSecurityGroups(VpcSecurityGroupMembership... vpcSecurityGroups) {
if (this.vpcSecurityGroups == null) {
setVpcSecurityGroups(new com.amazonaws.internal.SdkInternalList(vpcSecurityGroups.length));
}
for (VpcSecurityGroupMembership ele : vpcSecurityGroups) {
this.vpcSecurityGroups.add(ele);
}
return this;
}
/**
*
* The list of Amazon EC2 VPC security groups that the DB instance belongs to.
*
*
* @param vpcSecurityGroups
* The list of Amazon EC2 VPC security groups that the DB instance belongs to.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DBInstance withVpcSecurityGroups(java.util.Collection vpcSecurityGroups) {
setVpcSecurityGroups(vpcSecurityGroups);
return this;
}
/**
*
* The list of DB parameter groups applied to this DB instance.
*
*
* @return The list of DB parameter groups applied to this DB instance.
*/
public java.util.List getDBParameterGroups() {
if (dBParameterGroups == null) {
dBParameterGroups = new com.amazonaws.internal.SdkInternalList();
}
return dBParameterGroups;
}
/**
*
* The list of DB parameter groups applied to this DB instance.
*
*
* @param dBParameterGroups
* The list of DB parameter groups applied to this DB instance.
*/
public void setDBParameterGroups(java.util.Collection dBParameterGroups) {
if (dBParameterGroups == null) {
this.dBParameterGroups = null;
return;
}
this.dBParameterGroups = new com.amazonaws.internal.SdkInternalList(dBParameterGroups);
}
/**
*
* The list of DB parameter groups applied to this DB instance.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setDBParameterGroups(java.util.Collection)} or {@link #withDBParameterGroups(java.util.Collection)} if
* you want to override the existing values.
*
*
* @param dBParameterGroups
* The list of DB parameter groups applied to this DB instance.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DBInstance withDBParameterGroups(DBParameterGroupStatus... dBParameterGroups) {
if (this.dBParameterGroups == null) {
setDBParameterGroups(new com.amazonaws.internal.SdkInternalList(dBParameterGroups.length));
}
for (DBParameterGroupStatus ele : dBParameterGroups) {
this.dBParameterGroups.add(ele);
}
return this;
}
/**
*
* The list of DB parameter groups applied to this DB instance.
*
*
* @param dBParameterGroups
* The list of DB parameter groups applied to this DB instance.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DBInstance withDBParameterGroups(java.util.Collection dBParameterGroups) {
setDBParameterGroups(dBParameterGroups);
return this;
}
/**
*
* The name of the Availability Zone where the DB instance is located.
*
*
* @param availabilityZone
* The name of the Availability Zone where the DB instance is located.
*/
public void setAvailabilityZone(String availabilityZone) {
this.availabilityZone = availabilityZone;
}
/**
*
* The name of the Availability Zone where the DB instance is located.
*
*
* @return The name of the Availability Zone where the DB instance is located.
*/
public String getAvailabilityZone() {
return this.availabilityZone;
}
/**
*
* The name of the Availability Zone where the DB instance is located.
*
*
* @param availabilityZone
* The name of the Availability Zone where the DB instance is located.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DBInstance withAvailabilityZone(String availabilityZone) {
setAvailabilityZone(availabilityZone);
return this;
}
/**
*
* Information about the subnet group associated with the DB instance, including the name, description, and subnets
* in the subnet group.
*
*
* @param dBSubnetGroup
* Information about the subnet group associated with the DB instance, including the name, description, and
* subnets in the subnet group.
*/
public void setDBSubnetGroup(DBSubnetGroup dBSubnetGroup) {
this.dBSubnetGroup = dBSubnetGroup;
}
/**
*
* Information about the subnet group associated with the DB instance, including the name, description, and subnets
* in the subnet group.
*
*
* @return Information about the subnet group associated with the DB instance, including the name, description, and
* subnets in the subnet group.
*/
public DBSubnetGroup getDBSubnetGroup() {
return this.dBSubnetGroup;
}
/**
*
* Information about the subnet group associated with the DB instance, including the name, description, and subnets
* in the subnet group.
*
*
* @param dBSubnetGroup
* Information about the subnet group associated with the DB instance, including the name, description, and
* subnets in the subnet group.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DBInstance withDBSubnetGroup(DBSubnetGroup dBSubnetGroup) {
setDBSubnetGroup(dBSubnetGroup);
return this;
}
/**
*
* The weekly time range during which system maintenance can occur, in Universal Coordinated Time (UTC).
*
*
* @param preferredMaintenanceWindow
* The weekly time range during which system maintenance can occur, in Universal Coordinated Time (UTC).
*/
public void setPreferredMaintenanceWindow(String preferredMaintenanceWindow) {
this.preferredMaintenanceWindow = preferredMaintenanceWindow;
}
/**
*
* The weekly time range during which system maintenance can occur, in Universal Coordinated Time (UTC).
*
*
* @return The weekly time range during which system maintenance can occur, in Universal Coordinated Time (UTC).
*/
public String getPreferredMaintenanceWindow() {
return this.preferredMaintenanceWindow;
}
/**
*
* The weekly time range during which system maintenance can occur, in Universal Coordinated Time (UTC).
*
*
* @param preferredMaintenanceWindow
* The weekly time range during which system maintenance can occur, in Universal Coordinated Time (UTC).
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DBInstance withPreferredMaintenanceWindow(String preferredMaintenanceWindow) {
setPreferredMaintenanceWindow(preferredMaintenanceWindow);
return this;
}
/**
*
* Information about pending changes to the DB instance. This information is returned only when there are pending
* changes. Specific changes are identified by subelements.
*
*
* @param pendingModifiedValues
* Information about pending changes to the DB instance. This information is returned only when there are
* pending changes. Specific changes are identified by subelements.
*/
public void setPendingModifiedValues(PendingModifiedValues pendingModifiedValues) {
this.pendingModifiedValues = pendingModifiedValues;
}
/**
*
* Information about pending changes to the DB instance. This information is returned only when there are pending
* changes. Specific changes are identified by subelements.
*
*
* @return Information about pending changes to the DB instance. This information is returned only when there are
* pending changes. Specific changes are identified by subelements.
*/
public PendingModifiedValues getPendingModifiedValues() {
return this.pendingModifiedValues;
}
/**
*
* Information about pending changes to the DB instance. This information is returned only when there are pending
* changes. Specific changes are identified by subelements.
*
*
* @param pendingModifiedValues
* Information about pending changes to the DB instance. This information is returned only when there are
* pending changes. Specific changes are identified by subelements.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DBInstance withPendingModifiedValues(PendingModifiedValues pendingModifiedValues) {
setPendingModifiedValues(pendingModifiedValues);
return this;
}
/**
*
* The latest time to which a database in this DB instance can be restored with point-in-time restore.
*
*
* @param latestRestorableTime
* The latest time to which a database in this DB instance can be restored with point-in-time restore.
*/
public void setLatestRestorableTime(java.util.Date latestRestorableTime) {
this.latestRestorableTime = latestRestorableTime;
}
/**
*
* The latest time to which a database in this DB instance can be restored with point-in-time restore.
*
*
* @return The latest time to which a database in this DB instance can be restored with point-in-time restore.
*/
public java.util.Date getLatestRestorableTime() {
return this.latestRestorableTime;
}
/**
*
* The latest time to which a database in this DB instance can be restored with point-in-time restore.
*
*
* @param latestRestorableTime
* The latest time to which a database in this DB instance can be restored with point-in-time restore.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DBInstance withLatestRestorableTime(java.util.Date latestRestorableTime) {
setLatestRestorableTime(latestRestorableTime);
return this;
}
/**
*
* Indicates whether the DB instance is a Multi-AZ deployment. This setting doesn't apply to RDS Custom DB
* instances.
*
*
* @param multiAZ
* Indicates whether the DB instance is a Multi-AZ deployment. This setting doesn't apply to RDS Custom DB
* instances.
*/
public void setMultiAZ(Boolean multiAZ) {
this.multiAZ = multiAZ;
}
/**
*
* Indicates whether the DB instance is a Multi-AZ deployment. This setting doesn't apply to RDS Custom DB
* instances.
*
*
* @return Indicates whether the DB instance is a Multi-AZ deployment. This setting doesn't apply to RDS Custom DB
* instances.
*/
public Boolean getMultiAZ() {
return this.multiAZ;
}
/**
*
* Indicates whether the DB instance is a Multi-AZ deployment. This setting doesn't apply to RDS Custom DB
* instances.
*
*
* @param multiAZ
* Indicates whether the DB instance is a Multi-AZ deployment. This setting doesn't apply to RDS Custom DB
* instances.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DBInstance withMultiAZ(Boolean multiAZ) {
setMultiAZ(multiAZ);
return this;
}
/**
*
* Indicates whether the DB instance is a Multi-AZ deployment. This setting doesn't apply to RDS Custom DB
* instances.
*
*
* @return Indicates whether the DB instance is a Multi-AZ deployment. This setting doesn't apply to RDS Custom DB
* instances.
*/
public Boolean isMultiAZ() {
return this.multiAZ;
}
/**
*
* The version of the database engine.
*
*
* @param engineVersion
* The version of the database engine.
*/
public void setEngineVersion(String engineVersion) {
this.engineVersion = engineVersion;
}
/**
*
* The version of the database engine.
*
*
* @return The version of the database engine.
*/
public String getEngineVersion() {
return this.engineVersion;
}
/**
*
* The version of the database engine.
*
*
* @param engineVersion
* The version of the database engine.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DBInstance withEngineVersion(String engineVersion) {
setEngineVersion(engineVersion);
return this;
}
/**
*
* Indicates whether minor version patches are applied automatically.
*
*
* @param autoMinorVersionUpgrade
* Indicates whether minor version patches are applied automatically.
*/
public void setAutoMinorVersionUpgrade(Boolean autoMinorVersionUpgrade) {
this.autoMinorVersionUpgrade = autoMinorVersionUpgrade;
}
/**
*
* Indicates whether minor version patches are applied automatically.
*
*
* @return Indicates whether minor version patches are applied automatically.
*/
public Boolean getAutoMinorVersionUpgrade() {
return this.autoMinorVersionUpgrade;
}
/**
*
* Indicates whether minor version patches are applied automatically.
*
*
* @param autoMinorVersionUpgrade
* Indicates whether minor version patches are applied automatically.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DBInstance withAutoMinorVersionUpgrade(Boolean autoMinorVersionUpgrade) {
setAutoMinorVersionUpgrade(autoMinorVersionUpgrade);
return this;
}
/**
*
* Indicates whether minor version patches are applied automatically.
*
*
* @return Indicates whether minor version patches are applied automatically.
*/
public Boolean isAutoMinorVersionUpgrade() {
return this.autoMinorVersionUpgrade;
}
/**
*
* The identifier of the source DB instance if this DB instance is a read replica.
*
*
* @param readReplicaSourceDBInstanceIdentifier
* The identifier of the source DB instance if this DB instance is a read replica.
*/
public void setReadReplicaSourceDBInstanceIdentifier(String readReplicaSourceDBInstanceIdentifier) {
this.readReplicaSourceDBInstanceIdentifier = readReplicaSourceDBInstanceIdentifier;
}
/**
*
* The identifier of the source DB instance if this DB instance is a read replica.
*
*
* @return The identifier of the source DB instance if this DB instance is a read replica.
*/
public String getReadReplicaSourceDBInstanceIdentifier() {
return this.readReplicaSourceDBInstanceIdentifier;
}
/**
*
* The identifier of the source DB instance if this DB instance is a read replica.
*
*
* @param readReplicaSourceDBInstanceIdentifier
* The identifier of the source DB instance if this DB instance is a read replica.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DBInstance withReadReplicaSourceDBInstanceIdentifier(String readReplicaSourceDBInstanceIdentifier) {
setReadReplicaSourceDBInstanceIdentifier(readReplicaSourceDBInstanceIdentifier);
return this;
}
/**
*
* The identifiers of the read replicas associated with this DB instance.
*
*
* @return The identifiers of the read replicas associated with this DB instance.
*/
public java.util.List getReadReplicaDBInstanceIdentifiers() {
if (readReplicaDBInstanceIdentifiers == null) {
readReplicaDBInstanceIdentifiers = new com.amazonaws.internal.SdkInternalList();
}
return readReplicaDBInstanceIdentifiers;
}
/**
*
* The identifiers of the read replicas associated with this DB instance.
*
*
* @param readReplicaDBInstanceIdentifiers
* The identifiers of the read replicas associated with this DB instance.
*/
public void setReadReplicaDBInstanceIdentifiers(java.util.Collection readReplicaDBInstanceIdentifiers) {
if (readReplicaDBInstanceIdentifiers == null) {
this.readReplicaDBInstanceIdentifiers = null;
return;
}
this.readReplicaDBInstanceIdentifiers = new com.amazonaws.internal.SdkInternalList(readReplicaDBInstanceIdentifiers);
}
/**
*
* The identifiers of the read replicas associated with this DB instance.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setReadReplicaDBInstanceIdentifiers(java.util.Collection)} or
* {@link #withReadReplicaDBInstanceIdentifiers(java.util.Collection)} if you want to override the existing values.
*
*
* @param readReplicaDBInstanceIdentifiers
* The identifiers of the read replicas associated with this DB instance.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DBInstance withReadReplicaDBInstanceIdentifiers(String... readReplicaDBInstanceIdentifiers) {
if (this.readReplicaDBInstanceIdentifiers == null) {
setReadReplicaDBInstanceIdentifiers(new com.amazonaws.internal.SdkInternalList(readReplicaDBInstanceIdentifiers.length));
}
for (String ele : readReplicaDBInstanceIdentifiers) {
this.readReplicaDBInstanceIdentifiers.add(ele);
}
return this;
}
/**
*
* The identifiers of the read replicas associated with this DB instance.
*
*
* @param readReplicaDBInstanceIdentifiers
* The identifiers of the read replicas associated with this DB instance.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DBInstance withReadReplicaDBInstanceIdentifiers(java.util.Collection readReplicaDBInstanceIdentifiers) {
setReadReplicaDBInstanceIdentifiers(readReplicaDBInstanceIdentifiers);
return this;
}
/**
*
* The identifiers of Aurora DB clusters to which the RDS DB instance is replicated as a read replica. For example,
* when you create an Aurora read replica of an RDS for MySQL DB instance, the Aurora MySQL DB cluster for the
* Aurora read replica is shown. This output doesn't contain information about cross-Region Aurora read replicas.
*
*
*
* Currently, each RDS DB instance can have only one Aurora read replica.
*
*
*
* @return The identifiers of Aurora DB clusters to which the RDS DB instance is replicated as a read replica. For
* example, when you create an Aurora read replica of an RDS for MySQL DB instance, the Aurora MySQL DB
* cluster for the Aurora read replica is shown. This output doesn't contain information about cross-Region
* Aurora read replicas.
*
* Currently, each RDS DB instance can have only one Aurora read replica.
*
*/
public java.util.List getReadReplicaDBClusterIdentifiers() {
if (readReplicaDBClusterIdentifiers == null) {
readReplicaDBClusterIdentifiers = new com.amazonaws.internal.SdkInternalList();
}
return readReplicaDBClusterIdentifiers;
}
/**
*
* The identifiers of Aurora DB clusters to which the RDS DB instance is replicated as a read replica. For example,
* when you create an Aurora read replica of an RDS for MySQL DB instance, the Aurora MySQL DB cluster for the
* Aurora read replica is shown. This output doesn't contain information about cross-Region Aurora read replicas.
*
*
*
* Currently, each RDS DB instance can have only one Aurora read replica.
*
*
*
* @param readReplicaDBClusterIdentifiers
* The identifiers of Aurora DB clusters to which the RDS DB instance is replicated as a read replica. For
* example, when you create an Aurora read replica of an RDS for MySQL DB instance, the Aurora MySQL DB
* cluster for the Aurora read replica is shown. This output doesn't contain information about cross-Region
* Aurora read replicas.
*
* Currently, each RDS DB instance can have only one Aurora read replica.
*
*/
public void setReadReplicaDBClusterIdentifiers(java.util.Collection readReplicaDBClusterIdentifiers) {
if (readReplicaDBClusterIdentifiers == null) {
this.readReplicaDBClusterIdentifiers = null;
return;
}
this.readReplicaDBClusterIdentifiers = new com.amazonaws.internal.SdkInternalList(readReplicaDBClusterIdentifiers);
}
/**
*
* The identifiers of Aurora DB clusters to which the RDS DB instance is replicated as a read replica. For example,
* when you create an Aurora read replica of an RDS for MySQL DB instance, the Aurora MySQL DB cluster for the
* Aurora read replica is shown. This output doesn't contain information about cross-Region Aurora read replicas.
*
*
*
* Currently, each RDS DB instance can have only one Aurora read replica.
*
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setReadReplicaDBClusterIdentifiers(java.util.Collection)} or
* {@link #withReadReplicaDBClusterIdentifiers(java.util.Collection)} if you want to override the existing values.
*
*
* @param readReplicaDBClusterIdentifiers
* The identifiers of Aurora DB clusters to which the RDS DB instance is replicated as a read replica. For
* example, when you create an Aurora read replica of an RDS for MySQL DB instance, the Aurora MySQL DB
* cluster for the Aurora read replica is shown. This output doesn't contain information about cross-Region
* Aurora read replicas.
*
* Currently, each RDS DB instance can have only one Aurora read replica.
*
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DBInstance withReadReplicaDBClusterIdentifiers(String... readReplicaDBClusterIdentifiers) {
if (this.readReplicaDBClusterIdentifiers == null) {
setReadReplicaDBClusterIdentifiers(new com.amazonaws.internal.SdkInternalList(readReplicaDBClusterIdentifiers.length));
}
for (String ele : readReplicaDBClusterIdentifiers) {
this.readReplicaDBClusterIdentifiers.add(ele);
}
return this;
}
/**
*
* The identifiers of Aurora DB clusters to which the RDS DB instance is replicated as a read replica. For example,
* when you create an Aurora read replica of an RDS for MySQL DB instance, the Aurora MySQL DB cluster for the
* Aurora read replica is shown. This output doesn't contain information about cross-Region Aurora read replicas.
*
*
*
* Currently, each RDS DB instance can have only one Aurora read replica.
*
*
*
* @param readReplicaDBClusterIdentifiers
* The identifiers of Aurora DB clusters to which the RDS DB instance is replicated as a read replica. For
* example, when you create an Aurora read replica of an RDS for MySQL DB instance, the Aurora MySQL DB
* cluster for the Aurora read replica is shown. This output doesn't contain information about cross-Region
* Aurora read replicas.
*
* Currently, each RDS DB instance can have only one Aurora read replica.
*
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DBInstance withReadReplicaDBClusterIdentifiers(java.util.Collection readReplicaDBClusterIdentifiers) {
setReadReplicaDBClusterIdentifiers(readReplicaDBClusterIdentifiers);
return this;
}
/**
*
* The open mode of an Oracle read replica. The default is open-read-only
. For more information, see Working with Oracle Read
* Replicas for Amazon RDS in the Amazon RDS User Guide.
*
*
*
* This attribute is only supported in RDS for Oracle.
*
*
*
* @param replicaMode
* The open mode of an Oracle read replica. The default is open-read-only
. For more information,
* see Working
* with Oracle Read Replicas for Amazon RDS in the Amazon RDS User Guide.
*
* This attribute is only supported in RDS for Oracle.
*
* @see ReplicaMode
*/
public void setReplicaMode(String replicaMode) {
this.replicaMode = replicaMode;
}
/**
*
* The open mode of an Oracle read replica. The default is open-read-only
. For more information, see Working with Oracle Read
* Replicas for Amazon RDS in the Amazon RDS User Guide.
*
*
*
* This attribute is only supported in RDS for Oracle.
*
*
*
* @return The open mode of an Oracle read replica. The default is open-read-only
. For more
* information, see Working with
* Oracle Read Replicas for Amazon RDS in the Amazon RDS User Guide.
*
* This attribute is only supported in RDS for Oracle.
*
* @see ReplicaMode
*/
public String getReplicaMode() {
return this.replicaMode;
}
/**
*
* The open mode of an Oracle read replica. The default is open-read-only
. For more information, see Working with Oracle Read
* Replicas for Amazon RDS in the Amazon RDS User Guide.
*
*
*
* This attribute is only supported in RDS for Oracle.
*
*
*
* @param replicaMode
* The open mode of an Oracle read replica. The default is open-read-only
. For more information,
* see Working
* with Oracle Read Replicas for Amazon RDS in the Amazon RDS User Guide.
*
* This attribute is only supported in RDS for Oracle.
*
* @return Returns a reference to this object so that method calls can be chained together.
* @see ReplicaMode
*/
public DBInstance withReplicaMode(String replicaMode) {
setReplicaMode(replicaMode);
return this;
}
/**
*
* The open mode of an Oracle read replica. The default is open-read-only
. For more information, see Working with Oracle Read
* Replicas for Amazon RDS in the Amazon RDS User Guide.
*
*
*
* This attribute is only supported in RDS for Oracle.
*
*
*
* @param replicaMode
* The open mode of an Oracle read replica. The default is open-read-only
. For more information,
* see Working
* with Oracle Read Replicas for Amazon RDS in the Amazon RDS User Guide.
*
* This attribute is only supported in RDS for Oracle.
*
* @return Returns a reference to this object so that method calls can be chained together.
* @see ReplicaMode
*/
public DBInstance withReplicaMode(ReplicaMode replicaMode) {
this.replicaMode = replicaMode.toString();
return this;
}
/**
*
* The license model information for this DB instance. This setting doesn't apply to RDS Custom DB instances.
*
*
* @param licenseModel
* The license model information for this DB instance. This setting doesn't apply to RDS Custom DB instances.
*/
public void setLicenseModel(String licenseModel) {
this.licenseModel = licenseModel;
}
/**
*
* The license model information for this DB instance. This setting doesn't apply to RDS Custom DB instances.
*
*
* @return The license model information for this DB instance. This setting doesn't apply to RDS Custom DB
* instances.
*/
public String getLicenseModel() {
return this.licenseModel;
}
/**
*
* The license model information for this DB instance. This setting doesn't apply to RDS Custom DB instances.
*
*
* @param licenseModel
* The license model information for this DB instance. This setting doesn't apply to RDS Custom DB instances.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DBInstance withLicenseModel(String licenseModel) {
setLicenseModel(licenseModel);
return this;
}
/**
*
* The Provisioned IOPS (I/O operations per second) value for the DB instance.
*
*
* @param iops
* The Provisioned IOPS (I/O operations per second) value for the DB instance.
*/
public void setIops(Integer iops) {
this.iops = iops;
}
/**
*
* The Provisioned IOPS (I/O operations per second) value for the DB instance.
*
*
* @return The Provisioned IOPS (I/O operations per second) value for the DB instance.
*/
public Integer getIops() {
return this.iops;
}
/**
*
* The Provisioned IOPS (I/O operations per second) value for the DB instance.
*
*
* @param iops
* The Provisioned IOPS (I/O operations per second) value for the DB instance.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DBInstance withIops(Integer iops) {
setIops(iops);
return this;
}
/**
*
* The list of option group memberships for this DB instance.
*
*
* @return The list of option group memberships for this DB instance.
*/
public java.util.List getOptionGroupMemberships() {
if (optionGroupMemberships == null) {
optionGroupMemberships = new com.amazonaws.internal.SdkInternalList();
}
return optionGroupMemberships;
}
/**
*
* The list of option group memberships for this DB instance.
*
*
* @param optionGroupMemberships
* The list of option group memberships for this DB instance.
*/
public void setOptionGroupMemberships(java.util.Collection optionGroupMemberships) {
if (optionGroupMemberships == null) {
this.optionGroupMemberships = null;
return;
}
this.optionGroupMemberships = new com.amazonaws.internal.SdkInternalList(optionGroupMemberships);
}
/**
*
* The list of option group memberships for this DB instance.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setOptionGroupMemberships(java.util.Collection)} or
* {@link #withOptionGroupMemberships(java.util.Collection)} if you want to override the existing values.
*
*
* @param optionGroupMemberships
* The list of option group memberships for this DB instance.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DBInstance withOptionGroupMemberships(OptionGroupMembership... optionGroupMemberships) {
if (this.optionGroupMemberships == null) {
setOptionGroupMemberships(new com.amazonaws.internal.SdkInternalList(optionGroupMemberships.length));
}
for (OptionGroupMembership ele : optionGroupMemberships) {
this.optionGroupMemberships.add(ele);
}
return this;
}
/**
*
* The list of option group memberships for this DB instance.
*
*
* @param optionGroupMemberships
* The list of option group memberships for this DB instance.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DBInstance withOptionGroupMemberships(java.util.Collection optionGroupMemberships) {
setOptionGroupMemberships(optionGroupMemberships);
return this;
}
/**
*
* If present, specifies the name of the character set that this instance is associated with.
*
*
* @param characterSetName
* If present, specifies the name of the character set that this instance is associated with.
*/
public void setCharacterSetName(String characterSetName) {
this.characterSetName = characterSetName;
}
/**
*
* If present, specifies the name of the character set that this instance is associated with.
*
*
* @return If present, specifies the name of the character set that this instance is associated with.
*/
public String getCharacterSetName() {
return this.characterSetName;
}
/**
*
* If present, specifies the name of the character set that this instance is associated with.
*
*
* @param characterSetName
* If present, specifies the name of the character set that this instance is associated with.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DBInstance withCharacterSetName(String characterSetName) {
setCharacterSetName(characterSetName);
return this;
}
/**
*
* The name of the NCHAR character set for the Oracle DB instance. This character set specifies the Unicode encoding
* for data stored in table columns of type NCHAR, NCLOB, or NVARCHAR2.
*
*
* @param ncharCharacterSetName
* The name of the NCHAR character set for the Oracle DB instance. This character set specifies the Unicode
* encoding for data stored in table columns of type NCHAR, NCLOB, or NVARCHAR2.
*/
public void setNcharCharacterSetName(String ncharCharacterSetName) {
this.ncharCharacterSetName = ncharCharacterSetName;
}
/**
*
* The name of the NCHAR character set for the Oracle DB instance. This character set specifies the Unicode encoding
* for data stored in table columns of type NCHAR, NCLOB, or NVARCHAR2.
*
*
* @return The name of the NCHAR character set for the Oracle DB instance. This character set specifies the Unicode
* encoding for data stored in table columns of type NCHAR, NCLOB, or NVARCHAR2.
*/
public String getNcharCharacterSetName() {
return this.ncharCharacterSetName;
}
/**
*
* The name of the NCHAR character set for the Oracle DB instance. This character set specifies the Unicode encoding
* for data stored in table columns of type NCHAR, NCLOB, or NVARCHAR2.
*
*
* @param ncharCharacterSetName
* The name of the NCHAR character set for the Oracle DB instance. This character set specifies the Unicode
* encoding for data stored in table columns of type NCHAR, NCLOB, or NVARCHAR2.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DBInstance withNcharCharacterSetName(String ncharCharacterSetName) {
setNcharCharacterSetName(ncharCharacterSetName);
return this;
}
/**
*
* If present, specifies the name of the secondary Availability Zone for a DB instance with multi-AZ support.
*
*
* @param secondaryAvailabilityZone
* If present, specifies the name of the secondary Availability Zone for a DB instance with multi-AZ support.
*/
public void setSecondaryAvailabilityZone(String secondaryAvailabilityZone) {
this.secondaryAvailabilityZone = secondaryAvailabilityZone;
}
/**
*
* If present, specifies the name of the secondary Availability Zone for a DB instance with multi-AZ support.
*
*
* @return If present, specifies the name of the secondary Availability Zone for a DB instance with multi-AZ
* support.
*/
public String getSecondaryAvailabilityZone() {
return this.secondaryAvailabilityZone;
}
/**
*
* If present, specifies the name of the secondary Availability Zone for a DB instance with multi-AZ support.
*
*
* @param secondaryAvailabilityZone
* If present, specifies the name of the secondary Availability Zone for a DB instance with multi-AZ support.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DBInstance withSecondaryAvailabilityZone(String secondaryAvailabilityZone) {
setSecondaryAvailabilityZone(secondaryAvailabilityZone);
return this;
}
/**
*
* Indicates whether the DB instance is publicly accessible.
*
*
* When the DB cluster is publicly accessible, its Domain Name System (DNS) endpoint resolves to the private IP
* address from within the DB cluster's virtual private cloud (VPC). It resolves to the public IP address from
* outside of the DB cluster's VPC. Access to the DB cluster is ultimately controlled by the security group it uses.
* That public access isn't permitted if the security group assigned to the DB cluster doesn't permit it.
*
*
* When the DB instance isn't publicly accessible, it is an internal DB instance with a DNS name that resolves to a
* private IP address.
*
*
* For more information, see CreateDBInstance.
*
*
* @param publiclyAccessible
* Indicates whether the DB instance is publicly accessible.
*
* When the DB cluster is publicly accessible, its Domain Name System (DNS) endpoint resolves to the private
* IP address from within the DB cluster's virtual private cloud (VPC). It resolves to the public IP address
* from outside of the DB cluster's VPC. Access to the DB cluster is ultimately controlled by the security
* group it uses. That public access isn't permitted if the security group assigned to the DB cluster doesn't
* permit it.
*
*
* When the DB instance isn't publicly accessible, it is an internal DB instance with a DNS name that
* resolves to a private IP address.
*
*
* For more information, see CreateDBInstance.
*/
public void setPubliclyAccessible(Boolean publiclyAccessible) {
this.publiclyAccessible = publiclyAccessible;
}
/**
*
* Indicates whether the DB instance is publicly accessible.
*
*
* When the DB cluster is publicly accessible, its Domain Name System (DNS) endpoint resolves to the private IP
* address from within the DB cluster's virtual private cloud (VPC). It resolves to the public IP address from
* outside of the DB cluster's VPC. Access to the DB cluster is ultimately controlled by the security group it uses.
* That public access isn't permitted if the security group assigned to the DB cluster doesn't permit it.
*
*
* When the DB instance isn't publicly accessible, it is an internal DB instance with a DNS name that resolves to a
* private IP address.
*
*
* For more information, see CreateDBInstance.
*
*
* @return Indicates whether the DB instance is publicly accessible.
*
* When the DB cluster is publicly accessible, its Domain Name System (DNS) endpoint resolves to the private
* IP address from within the DB cluster's virtual private cloud (VPC). It resolves to the public IP address
* from outside of the DB cluster's VPC. Access to the DB cluster is ultimately controlled by the security
* group it uses. That public access isn't permitted if the security group assigned to the DB cluster
* doesn't permit it.
*
*
* When the DB instance isn't publicly accessible, it is an internal DB instance with a DNS name that
* resolves to a private IP address.
*
*
* For more information, see CreateDBInstance.
*/
public Boolean getPubliclyAccessible() {
return this.publiclyAccessible;
}
/**
*
* Indicates whether the DB instance is publicly accessible.
*
*
* When the DB cluster is publicly accessible, its Domain Name System (DNS) endpoint resolves to the private IP
* address from within the DB cluster's virtual private cloud (VPC). It resolves to the public IP address from
* outside of the DB cluster's VPC. Access to the DB cluster is ultimately controlled by the security group it uses.
* That public access isn't permitted if the security group assigned to the DB cluster doesn't permit it.
*
*
* When the DB instance isn't publicly accessible, it is an internal DB instance with a DNS name that resolves to a
* private IP address.
*
*
* For more information, see CreateDBInstance.
*
*
* @param publiclyAccessible
* Indicates whether the DB instance is publicly accessible.
*
* When the DB cluster is publicly accessible, its Domain Name System (DNS) endpoint resolves to the private
* IP address from within the DB cluster's virtual private cloud (VPC). It resolves to the public IP address
* from outside of the DB cluster's VPC. Access to the DB cluster is ultimately controlled by the security
* group it uses. That public access isn't permitted if the security group assigned to the DB cluster doesn't
* permit it.
*
*
* When the DB instance isn't publicly accessible, it is an internal DB instance with a DNS name that
* resolves to a private IP address.
*
*
* For more information, see CreateDBInstance.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DBInstance withPubliclyAccessible(Boolean publiclyAccessible) {
setPubliclyAccessible(publiclyAccessible);
return this;
}
/**
*
* Indicates whether the DB instance is publicly accessible.
*
*
* When the DB cluster is publicly accessible, its Domain Name System (DNS) endpoint resolves to the private IP
* address from within the DB cluster's virtual private cloud (VPC). It resolves to the public IP address from
* outside of the DB cluster's VPC. Access to the DB cluster is ultimately controlled by the security group it uses.
* That public access isn't permitted if the security group assigned to the DB cluster doesn't permit it.
*
*
* When the DB instance isn't publicly accessible, it is an internal DB instance with a DNS name that resolves to a
* private IP address.
*
*
* For more information, see CreateDBInstance.
*
*
* @return Indicates whether the DB instance is publicly accessible.
*
* When the DB cluster is publicly accessible, its Domain Name System (DNS) endpoint resolves to the private
* IP address from within the DB cluster's virtual private cloud (VPC). It resolves to the public IP address
* from outside of the DB cluster's VPC. Access to the DB cluster is ultimately controlled by the security
* group it uses. That public access isn't permitted if the security group assigned to the DB cluster
* doesn't permit it.
*
*
* When the DB instance isn't publicly accessible, it is an internal DB instance with a DNS name that
* resolves to a private IP address.
*
*
* For more information, see CreateDBInstance.
*/
public Boolean isPubliclyAccessible() {
return this.publiclyAccessible;
}
/**
*
* The status of a read replica. If the DB instance isn't a read replica, the value is blank.
*
*
* @return The status of a read replica. If the DB instance isn't a read replica, the value is blank.
*/
public java.util.List getStatusInfos() {
if (statusInfos == null) {
statusInfos = new com.amazonaws.internal.SdkInternalList();
}
return statusInfos;
}
/**
*
* The status of a read replica. If the DB instance isn't a read replica, the value is blank.
*
*
* @param statusInfos
* The status of a read replica. If the DB instance isn't a read replica, the value is blank.
*/
public void setStatusInfos(java.util.Collection statusInfos) {
if (statusInfos == null) {
this.statusInfos = null;
return;
}
this.statusInfos = new com.amazonaws.internal.SdkInternalList(statusInfos);
}
/**
*
* The status of a read replica. If the DB instance isn't a read replica, the value is blank.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setStatusInfos(java.util.Collection)} or {@link #withStatusInfos(java.util.Collection)} if you want to
* override the existing values.
*
*
* @param statusInfos
* The status of a read replica. If the DB instance isn't a read replica, the value is blank.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DBInstance withStatusInfos(DBInstanceStatusInfo... statusInfos) {
if (this.statusInfos == null) {
setStatusInfos(new com.amazonaws.internal.SdkInternalList(statusInfos.length));
}
for (DBInstanceStatusInfo ele : statusInfos) {
this.statusInfos.add(ele);
}
return this;
}
/**
*
* The status of a read replica. If the DB instance isn't a read replica, the value is blank.
*
*
* @param statusInfos
* The status of a read replica. If the DB instance isn't a read replica, the value is blank.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DBInstance withStatusInfos(java.util.Collection statusInfos) {
setStatusInfos(statusInfos);
return this;
}
/**
*
* The storage type associated with the DB instance.
*
*
* @param storageType
* The storage type associated with the DB instance.
*/
public void setStorageType(String storageType) {
this.storageType = storageType;
}
/**
*
* The storage type associated with the DB instance.
*
*
* @return The storage type associated with the DB instance.
*/
public String getStorageType() {
return this.storageType;
}
/**
*
* The storage type associated with the DB instance.
*
*
* @param storageType
* The storage type associated with the DB instance.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DBInstance withStorageType(String storageType) {
setStorageType(storageType);
return this;
}
/**
*
* The ARN from the key store with which the instance is associated for TDE encryption.
*
*
* @param tdeCredentialArn
* The ARN from the key store with which the instance is associated for TDE encryption.
*/
public void setTdeCredentialArn(String tdeCredentialArn) {
this.tdeCredentialArn = tdeCredentialArn;
}
/**
*
* The ARN from the key store with which the instance is associated for TDE encryption.
*
*
* @return The ARN from the key store with which the instance is associated for TDE encryption.
*/
public String getTdeCredentialArn() {
return this.tdeCredentialArn;
}
/**
*
* The ARN from the key store with which the instance is associated for TDE encryption.
*
*
* @param tdeCredentialArn
* The ARN from the key store with which the instance is associated for TDE encryption.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DBInstance withTdeCredentialArn(String tdeCredentialArn) {
setTdeCredentialArn(tdeCredentialArn);
return this;
}
/**
*
* The port that the DB instance listens on. If the DB instance is part of a DB cluster, this can be a different
* port than the DB cluster port.
*
*
* @param dbInstancePort
* The port that the DB instance listens on. If the DB instance is part of a DB cluster, this can be a
* different port than the DB cluster port.
*/
public void setDbInstancePort(Integer dbInstancePort) {
this.dbInstancePort = dbInstancePort;
}
/**
*
* The port that the DB instance listens on. If the DB instance is part of a DB cluster, this can be a different
* port than the DB cluster port.
*
*
* @return The port that the DB instance listens on. If the DB instance is part of a DB cluster, this can be a
* different port than the DB cluster port.
*/
public Integer getDbInstancePort() {
return this.dbInstancePort;
}
/**
*
* The port that the DB instance listens on. If the DB instance is part of a DB cluster, this can be a different
* port than the DB cluster port.
*
*
* @param dbInstancePort
* The port that the DB instance listens on. If the DB instance is part of a DB cluster, this can be a
* different port than the DB cluster port.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DBInstance withDbInstancePort(Integer dbInstancePort) {
setDbInstancePort(dbInstancePort);
return this;
}
/**
*
* If the DB instance is a member of a DB cluster, indicates the name of the DB cluster that the DB instance is a
* member of.
*
*
* @param dBClusterIdentifier
* If the DB instance is a member of a DB cluster, indicates the name of the DB cluster that the DB instance
* is a member of.
*/
public void setDBClusterIdentifier(String dBClusterIdentifier) {
this.dBClusterIdentifier = dBClusterIdentifier;
}
/**
*
* If the DB instance is a member of a DB cluster, indicates the name of the DB cluster that the DB instance is a
* member of.
*
*
* @return If the DB instance is a member of a DB cluster, indicates the name of the DB cluster that the DB instance
* is a member of.
*/
public String getDBClusterIdentifier() {
return this.dBClusterIdentifier;
}
/**
*
* If the DB instance is a member of a DB cluster, indicates the name of the DB cluster that the DB instance is a
* member of.
*
*
* @param dBClusterIdentifier
* If the DB instance is a member of a DB cluster, indicates the name of the DB cluster that the DB instance
* is a member of.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DBInstance withDBClusterIdentifier(String dBClusterIdentifier) {
setDBClusterIdentifier(dBClusterIdentifier);
return this;
}
/**
*
* Indicates whether the DB instance is encrypted.
*
*
* @param storageEncrypted
* Indicates whether the DB instance is encrypted.
*/
public void setStorageEncrypted(Boolean storageEncrypted) {
this.storageEncrypted = storageEncrypted;
}
/**
*
* Indicates whether the DB instance is encrypted.
*
*
* @return Indicates whether the DB instance is encrypted.
*/
public Boolean getStorageEncrypted() {
return this.storageEncrypted;
}
/**
*
* Indicates whether the DB instance is encrypted.
*
*
* @param storageEncrypted
* Indicates whether the DB instance is encrypted.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DBInstance withStorageEncrypted(Boolean storageEncrypted) {
setStorageEncrypted(storageEncrypted);
return this;
}
/**
*
* Indicates whether the DB instance is encrypted.
*
*
* @return Indicates whether the DB instance is encrypted.
*/
public Boolean isStorageEncrypted() {
return this.storageEncrypted;
}
/**
*
* If StorageEncrypted
is enabled, the Amazon Web Services KMS key identifier for the encrypted DB
* instance.
*
*
* The Amazon Web Services KMS key identifier is the key ARN, key ID, alias ARN, or alias name for the KMS key.
*
*
* @param kmsKeyId
* If StorageEncrypted
is enabled, the Amazon Web Services KMS key identifier for the encrypted
* DB instance.
*
* The Amazon Web Services KMS key identifier is the key ARN, key ID, alias ARN, or alias name for the KMS
* key.
*/
public void setKmsKeyId(String kmsKeyId) {
this.kmsKeyId = kmsKeyId;
}
/**
*
* If StorageEncrypted
is enabled, the Amazon Web Services KMS key identifier for the encrypted DB
* instance.
*
*
* The Amazon Web Services KMS key identifier is the key ARN, key ID, alias ARN, or alias name for the KMS key.
*
*
* @return If StorageEncrypted
is enabled, the Amazon Web Services KMS key identifier for the encrypted
* DB instance.
*
* The Amazon Web Services KMS key identifier is the key ARN, key ID, alias ARN, or alias name for the KMS
* key.
*/
public String getKmsKeyId() {
return this.kmsKeyId;
}
/**
*
* If StorageEncrypted
is enabled, the Amazon Web Services KMS key identifier for the encrypted DB
* instance.
*
*
* The Amazon Web Services KMS key identifier is the key ARN, key ID, alias ARN, or alias name for the KMS key.
*
*
* @param kmsKeyId
* If StorageEncrypted
is enabled, the Amazon Web Services KMS key identifier for the encrypted
* DB instance.
*
* The Amazon Web Services KMS key identifier is the key ARN, key ID, alias ARN, or alias name for the KMS
* key.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DBInstance withKmsKeyId(String kmsKeyId) {
setKmsKeyId(kmsKeyId);
return this;
}
/**
*
* The Amazon Web Services Region-unique, immutable identifier for the DB instance. This identifier is found in
* Amazon Web Services CloudTrail log entries whenever the Amazon Web Services KMS key for the DB instance is
* accessed.
*
*
* @param dbiResourceId
* The Amazon Web Services Region-unique, immutable identifier for the DB instance. This identifier is found
* in Amazon Web Services CloudTrail log entries whenever the Amazon Web Services KMS key for the DB instance
* is accessed.
*/
public void setDbiResourceId(String dbiResourceId) {
this.dbiResourceId = dbiResourceId;
}
/**
*
* The Amazon Web Services Region-unique, immutable identifier for the DB instance. This identifier is found in
* Amazon Web Services CloudTrail log entries whenever the Amazon Web Services KMS key for the DB instance is
* accessed.
*
*
* @return The Amazon Web Services Region-unique, immutable identifier for the DB instance. This identifier is found
* in Amazon Web Services CloudTrail log entries whenever the Amazon Web Services KMS key for the DB
* instance is accessed.
*/
public String getDbiResourceId() {
return this.dbiResourceId;
}
/**
*
* The Amazon Web Services Region-unique, immutable identifier for the DB instance. This identifier is found in
* Amazon Web Services CloudTrail log entries whenever the Amazon Web Services KMS key for the DB instance is
* accessed.
*
*
* @param dbiResourceId
* The Amazon Web Services Region-unique, immutable identifier for the DB instance. This identifier is found
* in Amazon Web Services CloudTrail log entries whenever the Amazon Web Services KMS key for the DB instance
* is accessed.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DBInstance withDbiResourceId(String dbiResourceId) {
setDbiResourceId(dbiResourceId);
return this;
}
/**
*
* The identifier of the CA certificate for this DB instance.
*
*
* For more information, see Using SSL/TLS to encrypt a
* connection to a DB instance in the Amazon RDS User Guide and Using SSL/TLS to
* encrypt a connection to a DB cluster in the Amazon Aurora User Guide.
*
*
* @param cACertificateIdentifier
* The identifier of the CA certificate for this DB instance.
*
* For more information, see Using SSL/TLS to
* encrypt a connection to a DB instance in the Amazon RDS User Guide and Using SSL/TLS
* to encrypt a connection to a DB cluster in the Amazon Aurora User Guide.
*/
public void setCACertificateIdentifier(String cACertificateIdentifier) {
this.cACertificateIdentifier = cACertificateIdentifier;
}
/**
*
* The identifier of the CA certificate for this DB instance.
*
*
* For more information, see Using SSL/TLS to encrypt a
* connection to a DB instance in the Amazon RDS User Guide and Using SSL/TLS to
* encrypt a connection to a DB cluster in the Amazon Aurora User Guide.
*
*
* @return The identifier of the CA certificate for this DB instance.
*
* For more information, see Using SSL/TLS to
* encrypt a connection to a DB instance in the Amazon RDS User Guide and Using SSL/TLS
* to encrypt a connection to a DB cluster in the Amazon Aurora User Guide.
*/
public String getCACertificateIdentifier() {
return this.cACertificateIdentifier;
}
/**
*
* The identifier of the CA certificate for this DB instance.
*
*
* For more information, see Using SSL/TLS to encrypt a
* connection to a DB instance in the Amazon RDS User Guide and Using SSL/TLS to
* encrypt a connection to a DB cluster in the Amazon Aurora User Guide.
*
*
* @param cACertificateIdentifier
* The identifier of the CA certificate for this DB instance.
*
* For more information, see Using SSL/TLS to
* encrypt a connection to a DB instance in the Amazon RDS User Guide and Using SSL/TLS
* to encrypt a connection to a DB cluster in the Amazon Aurora User Guide.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DBInstance withCACertificateIdentifier(String cACertificateIdentifier) {
setCACertificateIdentifier(cACertificateIdentifier);
return this;
}
/**
*
* The Active Directory Domain membership records associated with the DB instance.
*
*
* @return The Active Directory Domain membership records associated with the DB instance.
*/
public java.util.List getDomainMemberships() {
if (domainMemberships == null) {
domainMemberships = new com.amazonaws.internal.SdkInternalList();
}
return domainMemberships;
}
/**
*
* The Active Directory Domain membership records associated with the DB instance.
*
*
* @param domainMemberships
* The Active Directory Domain membership records associated with the DB instance.
*/
public void setDomainMemberships(java.util.Collection domainMemberships) {
if (domainMemberships == null) {
this.domainMemberships = null;
return;
}
this.domainMemberships = new com.amazonaws.internal.SdkInternalList(domainMemberships);
}
/**
*
* The Active Directory Domain membership records associated with the DB instance.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setDomainMemberships(java.util.Collection)} or {@link #withDomainMemberships(java.util.Collection)} if
* you want to override the existing values.
*
*
* @param domainMemberships
* The Active Directory Domain membership records associated with the DB instance.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DBInstance withDomainMemberships(DomainMembership... domainMemberships) {
if (this.domainMemberships == null) {
setDomainMemberships(new com.amazonaws.internal.SdkInternalList(domainMemberships.length));
}
for (DomainMembership ele : domainMemberships) {
this.domainMemberships.add(ele);
}
return this;
}
/**
*
* The Active Directory Domain membership records associated with the DB instance.
*
*
* @param domainMemberships
* The Active Directory Domain membership records associated with the DB instance.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DBInstance withDomainMemberships(java.util.Collection domainMemberships) {
setDomainMemberships(domainMemberships);
return this;
}
/**
*
* Indicates whether tags are copied from the DB instance to snapshots of the DB instance.
*
*
* This setting doesn't apply to Amazon Aurora DB instances. Copying tags to snapshots is managed by the DB cluster.
* Setting this value for an Aurora DB instance has no effect on the DB cluster setting. For more information, see
* DBCluster
.
*
*
* @param copyTagsToSnapshot
* Indicates whether tags are copied from the DB instance to snapshots of the DB instance.
*
* This setting doesn't apply to Amazon Aurora DB instances. Copying tags to snapshots is managed by the DB
* cluster. Setting this value for an Aurora DB instance has no effect on the DB cluster setting. For more
* information, see DBCluster
.
*/
public void setCopyTagsToSnapshot(Boolean copyTagsToSnapshot) {
this.copyTagsToSnapshot = copyTagsToSnapshot;
}
/**
*
* Indicates whether tags are copied from the DB instance to snapshots of the DB instance.
*
*
* This setting doesn't apply to Amazon Aurora DB instances. Copying tags to snapshots is managed by the DB cluster.
* Setting this value for an Aurora DB instance has no effect on the DB cluster setting. For more information, see
* DBCluster
.
*
*
* @return Indicates whether tags are copied from the DB instance to snapshots of the DB instance.
*
* This setting doesn't apply to Amazon Aurora DB instances. Copying tags to snapshots is managed by the DB
* cluster. Setting this value for an Aurora DB instance has no effect on the DB cluster setting. For more
* information, see DBCluster
.
*/
public Boolean getCopyTagsToSnapshot() {
return this.copyTagsToSnapshot;
}
/**
*
* Indicates whether tags are copied from the DB instance to snapshots of the DB instance.
*
*
* This setting doesn't apply to Amazon Aurora DB instances. Copying tags to snapshots is managed by the DB cluster.
* Setting this value for an Aurora DB instance has no effect on the DB cluster setting. For more information, see
* DBCluster
.
*
*
* @param copyTagsToSnapshot
* Indicates whether tags are copied from the DB instance to snapshots of the DB instance.
*
* This setting doesn't apply to Amazon Aurora DB instances. Copying tags to snapshots is managed by the DB
* cluster. Setting this value for an Aurora DB instance has no effect on the DB cluster setting. For more
* information, see DBCluster
.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DBInstance withCopyTagsToSnapshot(Boolean copyTagsToSnapshot) {
setCopyTagsToSnapshot(copyTagsToSnapshot);
return this;
}
/**
*
* Indicates whether tags are copied from the DB instance to snapshots of the DB instance.
*
*
* This setting doesn't apply to Amazon Aurora DB instances. Copying tags to snapshots is managed by the DB cluster.
* Setting this value for an Aurora DB instance has no effect on the DB cluster setting. For more information, see
* DBCluster
.
*
*
* @return Indicates whether tags are copied from the DB instance to snapshots of the DB instance.
*
* This setting doesn't apply to Amazon Aurora DB instances. Copying tags to snapshots is managed by the DB
* cluster. Setting this value for an Aurora DB instance has no effect on the DB cluster setting. For more
* information, see DBCluster
.
*/
public Boolean isCopyTagsToSnapshot() {
return this.copyTagsToSnapshot;
}
/**
*
* The interval, in seconds, between points when Enhanced Monitoring metrics are collected for the DB instance.
*
*
* @param monitoringInterval
* The interval, in seconds, between points when Enhanced Monitoring metrics are collected for the DB
* instance.
*/
public void setMonitoringInterval(Integer monitoringInterval) {
this.monitoringInterval = monitoringInterval;
}
/**
*
* The interval, in seconds, between points when Enhanced Monitoring metrics are collected for the DB instance.
*
*
* @return The interval, in seconds, between points when Enhanced Monitoring metrics are collected for the DB
* instance.
*/
public Integer getMonitoringInterval() {
return this.monitoringInterval;
}
/**
*
* The interval, in seconds, between points when Enhanced Monitoring metrics are collected for the DB instance.
*
*
* @param monitoringInterval
* The interval, in seconds, between points when Enhanced Monitoring metrics are collected for the DB
* instance.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DBInstance withMonitoringInterval(Integer monitoringInterval) {
setMonitoringInterval(monitoringInterval);
return this;
}
/**
*
* The Amazon Resource Name (ARN) of the Amazon CloudWatch Logs log stream that receives the Enhanced Monitoring
* metrics data for the DB instance.
*
*
* @param enhancedMonitoringResourceArn
* The Amazon Resource Name (ARN) of the Amazon CloudWatch Logs log stream that receives the Enhanced
* Monitoring metrics data for the DB instance.
*/
public void setEnhancedMonitoringResourceArn(String enhancedMonitoringResourceArn) {
this.enhancedMonitoringResourceArn = enhancedMonitoringResourceArn;
}
/**
*
* The Amazon Resource Name (ARN) of the Amazon CloudWatch Logs log stream that receives the Enhanced Monitoring
* metrics data for the DB instance.
*
*
* @return The Amazon Resource Name (ARN) of the Amazon CloudWatch Logs log stream that receives the Enhanced
* Monitoring metrics data for the DB instance.
*/
public String getEnhancedMonitoringResourceArn() {
return this.enhancedMonitoringResourceArn;
}
/**
*
* The Amazon Resource Name (ARN) of the Amazon CloudWatch Logs log stream that receives the Enhanced Monitoring
* metrics data for the DB instance.
*
*
* @param enhancedMonitoringResourceArn
* The Amazon Resource Name (ARN) of the Amazon CloudWatch Logs log stream that receives the Enhanced
* Monitoring metrics data for the DB instance.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DBInstance withEnhancedMonitoringResourceArn(String enhancedMonitoringResourceArn) {
setEnhancedMonitoringResourceArn(enhancedMonitoringResourceArn);
return this;
}
/**
*
* The ARN for the IAM role that permits RDS to send Enhanced Monitoring metrics to Amazon CloudWatch Logs.
*
*
* @param monitoringRoleArn
* The ARN for the IAM role that permits RDS to send Enhanced Monitoring metrics to Amazon CloudWatch Logs.
*/
public void setMonitoringRoleArn(String monitoringRoleArn) {
this.monitoringRoleArn = monitoringRoleArn;
}
/**
*
* The ARN for the IAM role that permits RDS to send Enhanced Monitoring metrics to Amazon CloudWatch Logs.
*
*
* @return The ARN for the IAM role that permits RDS to send Enhanced Monitoring metrics to Amazon CloudWatch Logs.
*/
public String getMonitoringRoleArn() {
return this.monitoringRoleArn;
}
/**
*
* The ARN for the IAM role that permits RDS to send Enhanced Monitoring metrics to Amazon CloudWatch Logs.
*
*
* @param monitoringRoleArn
* The ARN for the IAM role that permits RDS to send Enhanced Monitoring metrics to Amazon CloudWatch Logs.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DBInstance withMonitoringRoleArn(String monitoringRoleArn) {
setMonitoringRoleArn(monitoringRoleArn);
return this;
}
/**
*
* The order of priority in which an Aurora Replica is promoted to the primary instance after a failure of the
* existing primary instance. For more information, see Fault Tolerance for an Aurora DB Cluster in the Amazon Aurora User Guide.
*
*
* @param promotionTier
* The order of priority in which an Aurora Replica is promoted to the primary instance after a failure of
* the existing primary instance. For more information, see Fault Tolerance for an Aurora DB Cluster in the Amazon Aurora User Guide.
*/
public void setPromotionTier(Integer promotionTier) {
this.promotionTier = promotionTier;
}
/**
*
* The order of priority in which an Aurora Replica is promoted to the primary instance after a failure of the
* existing primary instance. For more information, see Fault Tolerance for an Aurora DB Cluster in the Amazon Aurora User Guide.
*
*
* @return The order of priority in which an Aurora Replica is promoted to the primary instance after a failure of
* the existing primary instance. For more information, see Fault Tolerance for an Aurora DB Cluster in the Amazon Aurora User Guide.
*/
public Integer getPromotionTier() {
return this.promotionTier;
}
/**
*
* The order of priority in which an Aurora Replica is promoted to the primary instance after a failure of the
* existing primary instance. For more information, see Fault Tolerance for an Aurora DB Cluster in the Amazon Aurora User Guide.
*
*
* @param promotionTier
* The order of priority in which an Aurora Replica is promoted to the primary instance after a failure of
* the existing primary instance. For more information, see Fault Tolerance for an Aurora DB Cluster in the Amazon Aurora User Guide.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DBInstance withPromotionTier(Integer promotionTier) {
setPromotionTier(promotionTier);
return this;
}
/**
*
* The Amazon Resource Name (ARN) for the DB instance.
*
*
* @param dBInstanceArn
* The Amazon Resource Name (ARN) for the DB instance.
*/
public void setDBInstanceArn(String dBInstanceArn) {
this.dBInstanceArn = dBInstanceArn;
}
/**
*
* The Amazon Resource Name (ARN) for the DB instance.
*
*
* @return The Amazon Resource Name (ARN) for the DB instance.
*/
public String getDBInstanceArn() {
return this.dBInstanceArn;
}
/**
*
* The Amazon Resource Name (ARN) for the DB instance.
*
*
* @param dBInstanceArn
* The Amazon Resource Name (ARN) for the DB instance.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DBInstance withDBInstanceArn(String dBInstanceArn) {
setDBInstanceArn(dBInstanceArn);
return this;
}
/**
*
* The time zone of the DB instance. In most cases, the Timezone
element is empty.
* Timezone
content appears only for Microsoft SQL Server DB instances that were created with a time
* zone specified.
*
*
* @param timezone
* The time zone of the DB instance. In most cases, the Timezone
element is empty.
* Timezone
content appears only for Microsoft SQL Server DB instances that were created with a
* time zone specified.
*/
public void setTimezone(String timezone) {
this.timezone = timezone;
}
/**
*
* The time zone of the DB instance. In most cases, the Timezone
element is empty.
* Timezone
content appears only for Microsoft SQL Server DB instances that were created with a time
* zone specified.
*
*
* @return The time zone of the DB instance. In most cases, the Timezone
element is empty.
* Timezone
content appears only for Microsoft SQL Server DB instances that were created with a
* time zone specified.
*/
public String getTimezone() {
return this.timezone;
}
/**
*
* The time zone of the DB instance. In most cases, the Timezone
element is empty.
* Timezone
content appears only for Microsoft SQL Server DB instances that were created with a time
* zone specified.
*
*
* @param timezone
* The time zone of the DB instance. In most cases, the Timezone
element is empty.
* Timezone
content appears only for Microsoft SQL Server DB instances that were created with a
* time zone specified.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DBInstance withTimezone(String timezone) {
setTimezone(timezone);
return this;
}
/**
*
* Indicates whether mapping of Amazon Web Services Identity and Access Management (IAM) accounts to database
* accounts is enabled for the DB instance.
*
*
* For a list of engine versions that support IAM database authentication, see IAM database authentication in the Amazon RDS User Guide and IAM database authentication in Aurora in the Amazon Aurora User Guide.
*
*
* @param iAMDatabaseAuthenticationEnabled
* Indicates whether mapping of Amazon Web Services Identity and Access Management (IAM) accounts to database
* accounts is enabled for the DB instance.
*
* For a list of engine versions that support IAM database authentication, see IAM database authentication in the Amazon RDS User Guide and IAM database authentication in Aurora in the Amazon Aurora User Guide.
*/
public void setIAMDatabaseAuthenticationEnabled(Boolean iAMDatabaseAuthenticationEnabled) {
this.iAMDatabaseAuthenticationEnabled = iAMDatabaseAuthenticationEnabled;
}
/**
*
* Indicates whether mapping of Amazon Web Services Identity and Access Management (IAM) accounts to database
* accounts is enabled for the DB instance.
*
*
* For a list of engine versions that support IAM database authentication, see IAM database authentication in the Amazon RDS User Guide and IAM database authentication in Aurora in the Amazon Aurora User Guide.
*
*
* @return Indicates whether mapping of Amazon Web Services Identity and Access Management (IAM) accounts to
* database accounts is enabled for the DB instance.
*
* For a list of engine versions that support IAM database authentication, see IAM database authentication in the Amazon RDS User Guide and IAM database authentication in Aurora in the Amazon Aurora User Guide.
*/
public Boolean getIAMDatabaseAuthenticationEnabled() {
return this.iAMDatabaseAuthenticationEnabled;
}
/**
*
* Indicates whether mapping of Amazon Web Services Identity and Access Management (IAM) accounts to database
* accounts is enabled for the DB instance.
*
*
* For a list of engine versions that support IAM database authentication, see IAM database authentication in the Amazon RDS User Guide and IAM database authentication in Aurora in the Amazon Aurora User Guide.
*
*
* @param iAMDatabaseAuthenticationEnabled
* Indicates whether mapping of Amazon Web Services Identity and Access Management (IAM) accounts to database
* accounts is enabled for the DB instance.
*
* For a list of engine versions that support IAM database authentication, see IAM database authentication in the Amazon RDS User Guide and IAM database authentication in Aurora in the Amazon Aurora User Guide.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DBInstance withIAMDatabaseAuthenticationEnabled(Boolean iAMDatabaseAuthenticationEnabled) {
setIAMDatabaseAuthenticationEnabled(iAMDatabaseAuthenticationEnabled);
return this;
}
/**
*
* Indicates whether mapping of Amazon Web Services Identity and Access Management (IAM) accounts to database
* accounts is enabled for the DB instance.
*
*
* For a list of engine versions that support IAM database authentication, see IAM database authentication in the Amazon RDS User Guide and IAM database authentication in Aurora in the Amazon Aurora User Guide.
*
*
* @return Indicates whether mapping of Amazon Web Services Identity and Access Management (IAM) accounts to
* database accounts is enabled for the DB instance.
*
* For a list of engine versions that support IAM database authentication, see IAM database authentication in the Amazon RDS User Guide and IAM database authentication in Aurora in the Amazon Aurora User Guide.
*/
public Boolean isIAMDatabaseAuthenticationEnabled() {
return this.iAMDatabaseAuthenticationEnabled;
}
/**
*
* Indicates whether Performance Insights is enabled for the DB instance.
*
*
* @param performanceInsightsEnabled
* Indicates whether Performance Insights is enabled for the DB instance.
*/
public void setPerformanceInsightsEnabled(Boolean performanceInsightsEnabled) {
this.performanceInsightsEnabled = performanceInsightsEnabled;
}
/**
*
* Indicates whether Performance Insights is enabled for the DB instance.
*
*
* @return Indicates whether Performance Insights is enabled for the DB instance.
*/
public Boolean getPerformanceInsightsEnabled() {
return this.performanceInsightsEnabled;
}
/**
*
* Indicates whether Performance Insights is enabled for the DB instance.
*
*
* @param performanceInsightsEnabled
* Indicates whether Performance Insights is enabled for the DB instance.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DBInstance withPerformanceInsightsEnabled(Boolean performanceInsightsEnabled) {
setPerformanceInsightsEnabled(performanceInsightsEnabled);
return this;
}
/**
*
* Indicates whether Performance Insights is enabled for the DB instance.
*
*
* @return Indicates whether Performance Insights is enabled for the DB instance.
*/
public Boolean isPerformanceInsightsEnabled() {
return this.performanceInsightsEnabled;
}
/**
*
* The Amazon Web Services KMS key identifier for encryption of Performance Insights data.
*
*
* The Amazon Web Services KMS key identifier is the key ARN, key ID, alias ARN, or alias name for the KMS key.
*
*
* @param performanceInsightsKMSKeyId
* The Amazon Web Services KMS key identifier for encryption of Performance Insights data.
*
* The Amazon Web Services KMS key identifier is the key ARN, key ID, alias ARN, or alias name for the KMS
* key.
*/
public void setPerformanceInsightsKMSKeyId(String performanceInsightsKMSKeyId) {
this.performanceInsightsKMSKeyId = performanceInsightsKMSKeyId;
}
/**
*
* The Amazon Web Services KMS key identifier for encryption of Performance Insights data.
*
*
* The Amazon Web Services KMS key identifier is the key ARN, key ID, alias ARN, or alias name for the KMS key.
*
*
* @return The Amazon Web Services KMS key identifier for encryption of Performance Insights data.
*
* The Amazon Web Services KMS key identifier is the key ARN, key ID, alias ARN, or alias name for the KMS
* key.
*/
public String getPerformanceInsightsKMSKeyId() {
return this.performanceInsightsKMSKeyId;
}
/**
*
* The Amazon Web Services KMS key identifier for encryption of Performance Insights data.
*
*
* The Amazon Web Services KMS key identifier is the key ARN, key ID, alias ARN, or alias name for the KMS key.
*
*
* @param performanceInsightsKMSKeyId
* The Amazon Web Services KMS key identifier for encryption of Performance Insights data.
*
* The Amazon Web Services KMS key identifier is the key ARN, key ID, alias ARN, or alias name for the KMS
* key.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DBInstance withPerformanceInsightsKMSKeyId(String performanceInsightsKMSKeyId) {
setPerformanceInsightsKMSKeyId(performanceInsightsKMSKeyId);
return this;
}
/**
*
* The number of days to retain Performance Insights data.
*
*
* Valid Values:
*
*
* -
*
* 7
*
*
* -
*
* month * 31, where month is a number of months from 1-23. Examples: 93
(3 months * 31),
* 341
(11 months * 31), 589
(19 months * 31)
*
*
* -
*
* 731
*
*
*
*
* Default: 7
days
*
*
* @param performanceInsightsRetentionPeriod
* The number of days to retain Performance Insights data.
*
* Valid Values:
*
*
* -
*
* 7
*
*
* -
*
* month * 31, where month is a number of months from 1-23. Examples: 93
(3 months
* * 31), 341
(11 months * 31), 589
(19 months * 31)
*
*
* -
*
* 731
*
*
*
*
* Default: 7
days
*/
public void setPerformanceInsightsRetentionPeriod(Integer performanceInsightsRetentionPeriod) {
this.performanceInsightsRetentionPeriod = performanceInsightsRetentionPeriod;
}
/**
*
* The number of days to retain Performance Insights data.
*
*
* Valid Values:
*
*
* -
*
* 7
*
*
* -
*
* month * 31, where month is a number of months from 1-23. Examples: 93
(3 months * 31),
* 341
(11 months * 31), 589
(19 months * 31)
*
*
* -
*
* 731
*
*
*
*
* Default: 7
days
*
*
* @return The number of days to retain Performance Insights data.
*
* Valid Values:
*
*
* -
*
* 7
*
*
* -
*
* month * 31, where month is a number of months from 1-23. Examples: 93
(3
* months * 31), 341
(11 months * 31), 589
(19 months * 31)
*
*
* -
*
* 731
*
*
*
*
* Default: 7
days
*/
public Integer getPerformanceInsightsRetentionPeriod() {
return this.performanceInsightsRetentionPeriod;
}
/**
*
* The number of days to retain Performance Insights data.
*
*
* Valid Values:
*
*
* -
*
* 7
*
*
* -
*
* month * 31, where month is a number of months from 1-23. Examples: 93
(3 months * 31),
* 341
(11 months * 31), 589
(19 months * 31)
*
*
* -
*
* 731
*
*
*
*
* Default: 7
days
*
*
* @param performanceInsightsRetentionPeriod
* The number of days to retain Performance Insights data.
*
* Valid Values:
*
*
* -
*
* 7
*
*
* -
*
* month * 31, where month is a number of months from 1-23. Examples: 93
(3 months
* * 31), 341
(11 months * 31), 589
(19 months * 31)
*
*
* -
*
* 731
*
*
*
*
* Default: 7
days
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DBInstance withPerformanceInsightsRetentionPeriod(Integer performanceInsightsRetentionPeriod) {
setPerformanceInsightsRetentionPeriod(performanceInsightsRetentionPeriod);
return this;
}
/**
*
* A list of log types that this DB instance is configured to export to CloudWatch Logs.
*
*
* Log types vary by DB engine. For information about the log types for each DB engine, see Monitoring Amazon RDS log
* files in the Amazon RDS User Guide.
*
*
* @return A list of log types that this DB instance is configured to export to CloudWatch Logs.
*
* Log types vary by DB engine. For information about the log types for each DB engine, see Monitoring Amazon RDS
* log files in the Amazon RDS User Guide.
*/
public java.util.List getEnabledCloudwatchLogsExports() {
if (enabledCloudwatchLogsExports == null) {
enabledCloudwatchLogsExports = new com.amazonaws.internal.SdkInternalList();
}
return enabledCloudwatchLogsExports;
}
/**
*
* A list of log types that this DB instance is configured to export to CloudWatch Logs.
*
*
* Log types vary by DB engine. For information about the log types for each DB engine, see Monitoring Amazon RDS log
* files in the Amazon RDS User Guide.
*
*
* @param enabledCloudwatchLogsExports
* A list of log types that this DB instance is configured to export to CloudWatch Logs.
*
* Log types vary by DB engine. For information about the log types for each DB engine, see Monitoring Amazon RDS
* log files in the Amazon RDS User Guide.
*/
public void setEnabledCloudwatchLogsExports(java.util.Collection enabledCloudwatchLogsExports) {
if (enabledCloudwatchLogsExports == null) {
this.enabledCloudwatchLogsExports = null;
return;
}
this.enabledCloudwatchLogsExports = new com.amazonaws.internal.SdkInternalList(enabledCloudwatchLogsExports);
}
/**
*
* A list of log types that this DB instance is configured to export to CloudWatch Logs.
*
*
* Log types vary by DB engine. For information about the log types for each DB engine, see Monitoring Amazon RDS log
* files in the Amazon RDS User Guide.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setEnabledCloudwatchLogsExports(java.util.Collection)} or
* {@link #withEnabledCloudwatchLogsExports(java.util.Collection)} if you want to override the existing values.
*
*
* @param enabledCloudwatchLogsExports
* A list of log types that this DB instance is configured to export to CloudWatch Logs.
*
* Log types vary by DB engine. For information about the log types for each DB engine, see Monitoring Amazon RDS
* log files in the Amazon RDS User Guide.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DBInstance withEnabledCloudwatchLogsExports(String... enabledCloudwatchLogsExports) {
if (this.enabledCloudwatchLogsExports == null) {
setEnabledCloudwatchLogsExports(new com.amazonaws.internal.SdkInternalList(enabledCloudwatchLogsExports.length));
}
for (String ele : enabledCloudwatchLogsExports) {
this.enabledCloudwatchLogsExports.add(ele);
}
return this;
}
/**
*
* A list of log types that this DB instance is configured to export to CloudWatch Logs.
*
*
* Log types vary by DB engine. For information about the log types for each DB engine, see Monitoring Amazon RDS log
* files in the Amazon RDS User Guide.
*
*
* @param enabledCloudwatchLogsExports
* A list of log types that this DB instance is configured to export to CloudWatch Logs.
*
* Log types vary by DB engine. For information about the log types for each DB engine, see Monitoring Amazon RDS
* log files in the Amazon RDS User Guide.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DBInstance withEnabledCloudwatchLogsExports(java.util.Collection enabledCloudwatchLogsExports) {
setEnabledCloudwatchLogsExports(enabledCloudwatchLogsExports);
return this;
}
/**
*
* The number of CPU cores and the number of threads per core for the DB instance class of the DB instance.
*
*
* @return The number of CPU cores and the number of threads per core for the DB instance class of the DB instance.
*/
public java.util.List getProcessorFeatures() {
if (processorFeatures == null) {
processorFeatures = new com.amazonaws.internal.SdkInternalList();
}
return processorFeatures;
}
/**
*
* The number of CPU cores and the number of threads per core for the DB instance class of the DB instance.
*
*
* @param processorFeatures
* The number of CPU cores and the number of threads per core for the DB instance class of the DB instance.
*/
public void setProcessorFeatures(java.util.Collection processorFeatures) {
if (processorFeatures == null) {
this.processorFeatures = null;
return;
}
this.processorFeatures = new com.amazonaws.internal.SdkInternalList(processorFeatures);
}
/**
*
* The number of CPU cores and the number of threads per core for the DB instance class of the DB instance.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setProcessorFeatures(java.util.Collection)} or {@link #withProcessorFeatures(java.util.Collection)} if
* you want to override the existing values.
*
*
* @param processorFeatures
* The number of CPU cores and the number of threads per core for the DB instance class of the DB instance.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DBInstance withProcessorFeatures(ProcessorFeature... processorFeatures) {
if (this.processorFeatures == null) {
setProcessorFeatures(new com.amazonaws.internal.SdkInternalList(processorFeatures.length));
}
for (ProcessorFeature ele : processorFeatures) {
this.processorFeatures.add(ele);
}
return this;
}
/**
*
* The number of CPU cores and the number of threads per core for the DB instance class of the DB instance.
*
*
* @param processorFeatures
* The number of CPU cores and the number of threads per core for the DB instance class of the DB instance.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DBInstance withProcessorFeatures(java.util.Collection processorFeatures) {
setProcessorFeatures(processorFeatures);
return this;
}
/**
*
* Indicates whether the DB instance has deletion protection enabled. The database can't be deleted when deletion
* protection is enabled. For more information, see Deleting a DB
* Instance.
*
*
* @param deletionProtection
* Indicates whether the DB instance has deletion protection enabled. The database can't be deleted when
* deletion protection is enabled. For more information, see Deleting a DB
* Instance.
*/
public void setDeletionProtection(Boolean deletionProtection) {
this.deletionProtection = deletionProtection;
}
/**
*
* Indicates whether the DB instance has deletion protection enabled. The database can't be deleted when deletion
* protection is enabled. For more information, see Deleting a DB
* Instance.
*
*
* @return Indicates whether the DB instance has deletion protection enabled. The database can't be deleted when
* deletion protection is enabled. For more information, see Deleting a DB
* Instance.
*/
public Boolean getDeletionProtection() {
return this.deletionProtection;
}
/**
*
* Indicates whether the DB instance has deletion protection enabled. The database can't be deleted when deletion
* protection is enabled. For more information, see Deleting a DB
* Instance.
*
*
* @param deletionProtection
* Indicates whether the DB instance has deletion protection enabled. The database can't be deleted when
* deletion protection is enabled. For more information, see Deleting a DB
* Instance.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DBInstance withDeletionProtection(Boolean deletionProtection) {
setDeletionProtection(deletionProtection);
return this;
}
/**
*
* Indicates whether the DB instance has deletion protection enabled. The database can't be deleted when deletion
* protection is enabled. For more information, see Deleting a DB
* Instance.
*
*
* @return Indicates whether the DB instance has deletion protection enabled. The database can't be deleted when
* deletion protection is enabled. For more information, see Deleting a DB
* Instance.
*/
public Boolean isDeletionProtection() {
return this.deletionProtection;
}
/**
*
* The Amazon Web Services Identity and Access Management (IAM) roles associated with the DB instance.
*
*
* @return The Amazon Web Services Identity and Access Management (IAM) roles associated with the DB instance.
*/
public java.util.List getAssociatedRoles() {
if (associatedRoles == null) {
associatedRoles = new com.amazonaws.internal.SdkInternalList();
}
return associatedRoles;
}
/**
*
* The Amazon Web Services Identity and Access Management (IAM) roles associated with the DB instance.
*
*
* @param associatedRoles
* The Amazon Web Services Identity and Access Management (IAM) roles associated with the DB instance.
*/
public void setAssociatedRoles(java.util.Collection associatedRoles) {
if (associatedRoles == null) {
this.associatedRoles = null;
return;
}
this.associatedRoles = new com.amazonaws.internal.SdkInternalList(associatedRoles);
}
/**
*
* The Amazon Web Services Identity and Access Management (IAM) roles associated with the DB instance.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setAssociatedRoles(java.util.Collection)} or {@link #withAssociatedRoles(java.util.Collection)} if you
* want to override the existing values.
*
*
* @param associatedRoles
* The Amazon Web Services Identity and Access Management (IAM) roles associated with the DB instance.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DBInstance withAssociatedRoles(DBInstanceRole... associatedRoles) {
if (this.associatedRoles == null) {
setAssociatedRoles(new com.amazonaws.internal.SdkInternalList(associatedRoles.length));
}
for (DBInstanceRole ele : associatedRoles) {
this.associatedRoles.add(ele);
}
return this;
}
/**
*
* The Amazon Web Services Identity and Access Management (IAM) roles associated with the DB instance.
*
*
* @param associatedRoles
* The Amazon Web Services Identity and Access Management (IAM) roles associated with the DB instance.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DBInstance withAssociatedRoles(java.util.Collection associatedRoles) {
setAssociatedRoles(associatedRoles);
return this;
}
/**
*
* The listener connection endpoint for SQL Server Always On.
*
*
* @param listenerEndpoint
* The listener connection endpoint for SQL Server Always On.
*/
public void setListenerEndpoint(Endpoint listenerEndpoint) {
this.listenerEndpoint = listenerEndpoint;
}
/**
*
* The listener connection endpoint for SQL Server Always On.
*
*
* @return The listener connection endpoint for SQL Server Always On.
*/
public Endpoint getListenerEndpoint() {
return this.listenerEndpoint;
}
/**
*
* The listener connection endpoint for SQL Server Always On.
*
*
* @param listenerEndpoint
* The listener connection endpoint for SQL Server Always On.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DBInstance withListenerEndpoint(Endpoint listenerEndpoint) {
setListenerEndpoint(listenerEndpoint);
return this;
}
/**
*
* The upper limit in gibibytes (GiB) to which Amazon RDS can automatically scale the storage of the DB instance.
*
*
* @param maxAllocatedStorage
* The upper limit in gibibytes (GiB) to which Amazon RDS can automatically scale the storage of the DB
* instance.
*/
public void setMaxAllocatedStorage(Integer maxAllocatedStorage) {
this.maxAllocatedStorage = maxAllocatedStorage;
}
/**
*
* The upper limit in gibibytes (GiB) to which Amazon RDS can automatically scale the storage of the DB instance.
*
*
* @return The upper limit in gibibytes (GiB) to which Amazon RDS can automatically scale the storage of the DB
* instance.
*/
public Integer getMaxAllocatedStorage() {
return this.maxAllocatedStorage;
}
/**
*
* The upper limit in gibibytes (GiB) to which Amazon RDS can automatically scale the storage of the DB instance.
*
*
* @param maxAllocatedStorage
* The upper limit in gibibytes (GiB) to which Amazon RDS can automatically scale the storage of the DB
* instance.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DBInstance withMaxAllocatedStorage(Integer maxAllocatedStorage) {
setMaxAllocatedStorage(maxAllocatedStorage);
return this;
}
/**
* @return
*/
public java.util.List getTagList() {
if (tagList == null) {
tagList = new com.amazonaws.internal.SdkInternalList();
}
return tagList;
}
/**
* @param tagList
*/
public void setTagList(java.util.Collection tagList) {
if (tagList == null) {
this.tagList = null;
return;
}
this.tagList = new com.amazonaws.internal.SdkInternalList(tagList);
}
/**
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setTagList(java.util.Collection)} or {@link #withTagList(java.util.Collection)} if you want to override
* the existing values.
*
*
* @param tagList
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DBInstance withTagList(Tag... tagList) {
if (this.tagList == null) {
setTagList(new com.amazonaws.internal.SdkInternalList(tagList.length));
}
for (Tag ele : tagList) {
this.tagList.add(ele);
}
return this;
}
/**
* @param tagList
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DBInstance withTagList(java.util.Collection tagList) {
setTagList(tagList);
return this;
}
/**
*
* The list of replicated automated backups associated with the DB instance.
*
*
* @return The list of replicated automated backups associated with the DB instance.
*/
public java.util.List getDBInstanceAutomatedBackupsReplications() {
if (dBInstanceAutomatedBackupsReplications == null) {
dBInstanceAutomatedBackupsReplications = new com.amazonaws.internal.SdkInternalList();
}
return dBInstanceAutomatedBackupsReplications;
}
/**
*
* The list of replicated automated backups associated with the DB instance.
*
*
* @param dBInstanceAutomatedBackupsReplications
* The list of replicated automated backups associated with the DB instance.
*/
public void setDBInstanceAutomatedBackupsReplications(java.util.Collection dBInstanceAutomatedBackupsReplications) {
if (dBInstanceAutomatedBackupsReplications == null) {
this.dBInstanceAutomatedBackupsReplications = null;
return;
}
this.dBInstanceAutomatedBackupsReplications = new com.amazonaws.internal.SdkInternalList(
dBInstanceAutomatedBackupsReplications);
}
/**
*
* The list of replicated automated backups associated with the DB instance.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setDBInstanceAutomatedBackupsReplications(java.util.Collection)} or
* {@link #withDBInstanceAutomatedBackupsReplications(java.util.Collection)} if you want to override the existing
* values.
*
*
* @param dBInstanceAutomatedBackupsReplications
* The list of replicated automated backups associated with the DB instance.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DBInstance withDBInstanceAutomatedBackupsReplications(DBInstanceAutomatedBackupsReplication... dBInstanceAutomatedBackupsReplications) {
if (this.dBInstanceAutomatedBackupsReplications == null) {
setDBInstanceAutomatedBackupsReplications(new com.amazonaws.internal.SdkInternalList(
dBInstanceAutomatedBackupsReplications.length));
}
for (DBInstanceAutomatedBackupsReplication ele : dBInstanceAutomatedBackupsReplications) {
this.dBInstanceAutomatedBackupsReplications.add(ele);
}
return this;
}
/**
*
* The list of replicated automated backups associated with the DB instance.
*
*
* @param dBInstanceAutomatedBackupsReplications
* The list of replicated automated backups associated with the DB instance.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DBInstance withDBInstanceAutomatedBackupsReplications(
java.util.Collection dBInstanceAutomatedBackupsReplications) {
setDBInstanceAutomatedBackupsReplications(dBInstanceAutomatedBackupsReplications);
return this;
}
/**
*
* Indicates whether a customer-owned IP address (CoIP) is enabled for an RDS on Outposts DB instance.
*
*
* A CoIP provides local or external connectivity to resources in your Outpost subnets through your
* on-premises network. For some use cases, a CoIP can provide lower latency for connections to the DB instance from
* outside of its virtual private cloud (VPC) on your local network.
*
*
* For more information about RDS on Outposts, see Working with Amazon RDS on
* Amazon Web Services Outposts in the Amazon RDS User Guide.
*
*
* For more information about CoIPs, see Customer-owned IP
* addresses in the Amazon Web Services Outposts User Guide.
*
*
* @param customerOwnedIpEnabled
* Indicates whether a customer-owned IP address (CoIP) is enabled for an RDS on Outposts DB instance.
*
* A CoIP provides local or external connectivity to resources in your Outpost subnets through your
* on-premises network. For some use cases, a CoIP can provide lower latency for connections to the DB
* instance from outside of its virtual private cloud (VPC) on your local network.
*
*
* For more information about RDS on Outposts, see Working with Amazon RDS
* on Amazon Web Services Outposts in the Amazon RDS User Guide.
*
*
* For more information about CoIPs, see Customer-owned IP
* addresses in the Amazon Web Services Outposts User Guide.
*/
public void setCustomerOwnedIpEnabled(Boolean customerOwnedIpEnabled) {
this.customerOwnedIpEnabled = customerOwnedIpEnabled;
}
/**
*
* Indicates whether a customer-owned IP address (CoIP) is enabled for an RDS on Outposts DB instance.
*
*
* A CoIP provides local or external connectivity to resources in your Outpost subnets through your
* on-premises network. For some use cases, a CoIP can provide lower latency for connections to the DB instance from
* outside of its virtual private cloud (VPC) on your local network.
*
*
* For more information about RDS on Outposts, see Working with Amazon RDS on
* Amazon Web Services Outposts in the Amazon RDS User Guide.
*
*
* For more information about CoIPs, see Customer-owned IP
* addresses in the Amazon Web Services Outposts User Guide.
*
*
* @return Indicates whether a customer-owned IP address (CoIP) is enabled for an RDS on Outposts DB instance.
*
* A CoIP provides local or external connectivity to resources in your Outpost subnets through your
* on-premises network. For some use cases, a CoIP can provide lower latency for connections to the DB
* instance from outside of its virtual private cloud (VPC) on your local network.
*
*
* For more information about RDS on Outposts, see Working with Amazon
* RDS on Amazon Web Services Outposts in the Amazon RDS User Guide.
*
*
* For more information about CoIPs, see Customer-owned IP
* addresses in the Amazon Web Services Outposts User Guide.
*/
public Boolean getCustomerOwnedIpEnabled() {
return this.customerOwnedIpEnabled;
}
/**
*
* Indicates whether a customer-owned IP address (CoIP) is enabled for an RDS on Outposts DB instance.
*
*
* A CoIP provides local or external connectivity to resources in your Outpost subnets through your
* on-premises network. For some use cases, a CoIP can provide lower latency for connections to the DB instance from
* outside of its virtual private cloud (VPC) on your local network.
*
*
* For more information about RDS on Outposts, see Working with Amazon RDS on
* Amazon Web Services Outposts in the Amazon RDS User Guide.
*
*
* For more information about CoIPs, see Customer-owned IP
* addresses in the Amazon Web Services Outposts User Guide.
*
*
* @param customerOwnedIpEnabled
* Indicates whether a customer-owned IP address (CoIP) is enabled for an RDS on Outposts DB instance.
*
* A CoIP provides local or external connectivity to resources in your Outpost subnets through your
* on-premises network. For some use cases, a CoIP can provide lower latency for connections to the DB
* instance from outside of its virtual private cloud (VPC) on your local network.
*
*
* For more information about RDS on Outposts, see Working with Amazon RDS
* on Amazon Web Services Outposts in the Amazon RDS User Guide.
*
*
* For more information about CoIPs, see Customer-owned IP
* addresses in the Amazon Web Services Outposts User Guide.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DBInstance withCustomerOwnedIpEnabled(Boolean customerOwnedIpEnabled) {
setCustomerOwnedIpEnabled(customerOwnedIpEnabled);
return this;
}
/**
*
* Indicates whether a customer-owned IP address (CoIP) is enabled for an RDS on Outposts DB instance.
*
*
* A CoIP provides local or external connectivity to resources in your Outpost subnets through your
* on-premises network. For some use cases, a CoIP can provide lower latency for connections to the DB instance from
* outside of its virtual private cloud (VPC) on your local network.
*
*
* For more information about RDS on Outposts, see Working with Amazon RDS on
* Amazon Web Services Outposts in the Amazon RDS User Guide.
*
*
* For more information about CoIPs, see Customer-owned IP
* addresses in the Amazon Web Services Outposts User Guide.
*
*
* @return Indicates whether a customer-owned IP address (CoIP) is enabled for an RDS on Outposts DB instance.
*
* A CoIP provides local or external connectivity to resources in your Outpost subnets through your
* on-premises network. For some use cases, a CoIP can provide lower latency for connections to the DB
* instance from outside of its virtual private cloud (VPC) on your local network.
*
*
* For more information about RDS on Outposts, see Working with Amazon
* RDS on Amazon Web Services Outposts in the Amazon RDS User Guide.
*
*
* For more information about CoIPs, see Customer-owned IP
* addresses in the Amazon Web Services Outposts User Guide.
*/
public Boolean isCustomerOwnedIpEnabled() {
return this.customerOwnedIpEnabled;
}
/**
*
* The Amazon Resource Name (ARN) of the recovery point in Amazon Web Services Backup.
*
*
* @param awsBackupRecoveryPointArn
* The Amazon Resource Name (ARN) of the recovery point in Amazon Web Services Backup.
*/
public void setAwsBackupRecoveryPointArn(String awsBackupRecoveryPointArn) {
this.awsBackupRecoveryPointArn = awsBackupRecoveryPointArn;
}
/**
*
* The Amazon Resource Name (ARN) of the recovery point in Amazon Web Services Backup.
*
*
* @return The Amazon Resource Name (ARN) of the recovery point in Amazon Web Services Backup.
*/
public String getAwsBackupRecoveryPointArn() {
return this.awsBackupRecoveryPointArn;
}
/**
*
* The Amazon Resource Name (ARN) of the recovery point in Amazon Web Services Backup.
*
*
* @param awsBackupRecoveryPointArn
* The Amazon Resource Name (ARN) of the recovery point in Amazon Web Services Backup.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DBInstance withAwsBackupRecoveryPointArn(String awsBackupRecoveryPointArn) {
setAwsBackupRecoveryPointArn(awsBackupRecoveryPointArn);
return this;
}
/**
*
* The status of the database activity stream.
*
*
* @param activityStreamStatus
* The status of the database activity stream.
* @see ActivityStreamStatus
*/
public void setActivityStreamStatus(String activityStreamStatus) {
this.activityStreamStatus = activityStreamStatus;
}
/**
*
* The status of the database activity stream.
*
*
* @return The status of the database activity stream.
* @see ActivityStreamStatus
*/
public String getActivityStreamStatus() {
return this.activityStreamStatus;
}
/**
*
* The status of the database activity stream.
*
*
* @param activityStreamStatus
* The status of the database activity stream.
* @return Returns a reference to this object so that method calls can be chained together.
* @see ActivityStreamStatus
*/
public DBInstance withActivityStreamStatus(String activityStreamStatus) {
setActivityStreamStatus(activityStreamStatus);
return this;
}
/**
*
* The status of the database activity stream.
*
*
* @param activityStreamStatus
* The status of the database activity stream.
* @return Returns a reference to this object so that method calls can be chained together.
* @see ActivityStreamStatus
*/
public DBInstance withActivityStreamStatus(ActivityStreamStatus activityStreamStatus) {
this.activityStreamStatus = activityStreamStatus.toString();
return this;
}
/**
*
* The Amazon Web Services KMS key identifier used for encrypting messages in the database activity stream. The
* Amazon Web Services KMS key identifier is the key ARN, key ID, alias ARN, or alias name for the KMS key.
*
*
* @param activityStreamKmsKeyId
* The Amazon Web Services KMS key identifier used for encrypting messages in the database activity stream.
* The Amazon Web Services KMS key identifier is the key ARN, key ID, alias ARN, or alias name for the KMS
* key.
*/
public void setActivityStreamKmsKeyId(String activityStreamKmsKeyId) {
this.activityStreamKmsKeyId = activityStreamKmsKeyId;
}
/**
*
* The Amazon Web Services KMS key identifier used for encrypting messages in the database activity stream. The
* Amazon Web Services KMS key identifier is the key ARN, key ID, alias ARN, or alias name for the KMS key.
*
*
* @return The Amazon Web Services KMS key identifier used for encrypting messages in the database activity stream.
* The Amazon Web Services KMS key identifier is the key ARN, key ID, alias ARN, or alias name for the KMS
* key.
*/
public String getActivityStreamKmsKeyId() {
return this.activityStreamKmsKeyId;
}
/**
*
* The Amazon Web Services KMS key identifier used for encrypting messages in the database activity stream. The
* Amazon Web Services KMS key identifier is the key ARN, key ID, alias ARN, or alias name for the KMS key.
*
*
* @param activityStreamKmsKeyId
* The Amazon Web Services KMS key identifier used for encrypting messages in the database activity stream.
* The Amazon Web Services KMS key identifier is the key ARN, key ID, alias ARN, or alias name for the KMS
* key.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DBInstance withActivityStreamKmsKeyId(String activityStreamKmsKeyId) {
setActivityStreamKmsKeyId(activityStreamKmsKeyId);
return this;
}
/**
*
* The name of the Amazon Kinesis data stream used for the database activity stream.
*
*
* @param activityStreamKinesisStreamName
* The name of the Amazon Kinesis data stream used for the database activity stream.
*/
public void setActivityStreamKinesisStreamName(String activityStreamKinesisStreamName) {
this.activityStreamKinesisStreamName = activityStreamKinesisStreamName;
}
/**
*
* The name of the Amazon Kinesis data stream used for the database activity stream.
*
*
* @return The name of the Amazon Kinesis data stream used for the database activity stream.
*/
public String getActivityStreamKinesisStreamName() {
return this.activityStreamKinesisStreamName;
}
/**
*
* The name of the Amazon Kinesis data stream used for the database activity stream.
*
*
* @param activityStreamKinesisStreamName
* The name of the Amazon Kinesis data stream used for the database activity stream.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DBInstance withActivityStreamKinesisStreamName(String activityStreamKinesisStreamName) {
setActivityStreamKinesisStreamName(activityStreamKinesisStreamName);
return this;
}
/**
*
* The mode of the database activity stream. Database events such as a change or access generate an activity stream
* event. RDS for Oracle always handles these events asynchronously.
*
*
* @param activityStreamMode
* The mode of the database activity stream. Database events such as a change or access generate an activity
* stream event. RDS for Oracle always handles these events asynchronously.
* @see ActivityStreamMode
*/
public void setActivityStreamMode(String activityStreamMode) {
this.activityStreamMode = activityStreamMode;
}
/**
*
* The mode of the database activity stream. Database events such as a change or access generate an activity stream
* event. RDS for Oracle always handles these events asynchronously.
*
*
* @return The mode of the database activity stream. Database events such as a change or access generate an activity
* stream event. RDS for Oracle always handles these events asynchronously.
* @see ActivityStreamMode
*/
public String getActivityStreamMode() {
return this.activityStreamMode;
}
/**
*
* The mode of the database activity stream. Database events such as a change or access generate an activity stream
* event. RDS for Oracle always handles these events asynchronously.
*
*
* @param activityStreamMode
* The mode of the database activity stream. Database events such as a change or access generate an activity
* stream event. RDS for Oracle always handles these events asynchronously.
* @return Returns a reference to this object so that method calls can be chained together.
* @see ActivityStreamMode
*/
public DBInstance withActivityStreamMode(String activityStreamMode) {
setActivityStreamMode(activityStreamMode);
return this;
}
/**
*
* The mode of the database activity stream. Database events such as a change or access generate an activity stream
* event. RDS for Oracle always handles these events asynchronously.
*
*
* @param activityStreamMode
* The mode of the database activity stream. Database events such as a change or access generate an activity
* stream event. RDS for Oracle always handles these events asynchronously.
* @return Returns a reference to this object so that method calls can be chained together.
* @see ActivityStreamMode
*/
public DBInstance withActivityStreamMode(ActivityStreamMode activityStreamMode) {
this.activityStreamMode = activityStreamMode.toString();
return this;
}
/**
*
* Indicates whether engine-native audit fields are included in the database activity stream.
*
*
* @param activityStreamEngineNativeAuditFieldsIncluded
* Indicates whether engine-native audit fields are included in the database activity stream.
*/
public void setActivityStreamEngineNativeAuditFieldsIncluded(Boolean activityStreamEngineNativeAuditFieldsIncluded) {
this.activityStreamEngineNativeAuditFieldsIncluded = activityStreamEngineNativeAuditFieldsIncluded;
}
/**
*
* Indicates whether engine-native audit fields are included in the database activity stream.
*
*
* @return Indicates whether engine-native audit fields are included in the database activity stream.
*/
public Boolean getActivityStreamEngineNativeAuditFieldsIncluded() {
return this.activityStreamEngineNativeAuditFieldsIncluded;
}
/**
*
* Indicates whether engine-native audit fields are included in the database activity stream.
*
*
* @param activityStreamEngineNativeAuditFieldsIncluded
* Indicates whether engine-native audit fields are included in the database activity stream.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DBInstance withActivityStreamEngineNativeAuditFieldsIncluded(Boolean activityStreamEngineNativeAuditFieldsIncluded) {
setActivityStreamEngineNativeAuditFieldsIncluded(activityStreamEngineNativeAuditFieldsIncluded);
return this;
}
/**
*
* Indicates whether engine-native audit fields are included in the database activity stream.
*
*
* @return Indicates whether engine-native audit fields are included in the database activity stream.
*/
public Boolean isActivityStreamEngineNativeAuditFieldsIncluded() {
return this.activityStreamEngineNativeAuditFieldsIncluded;
}
/**
*
* The automation mode of the RDS Custom DB instance: full
or all paused
. If
* full
, the DB instance automates monitoring and instance recovery. If all paused
, the
* instance pauses automation for the duration set by --resume-full-automation-mode-minutes
.
*
*
* @param automationMode
* The automation mode of the RDS Custom DB instance: full
or all paused
. If
* full
, the DB instance automates monitoring and instance recovery. If all paused
,
* the instance pauses automation for the duration set by --resume-full-automation-mode-minutes
.
* @see AutomationMode
*/
public void setAutomationMode(String automationMode) {
this.automationMode = automationMode;
}
/**
*
* The automation mode of the RDS Custom DB instance: full
or all paused
. If
* full
, the DB instance automates monitoring and instance recovery. If all paused
, the
* instance pauses automation for the duration set by --resume-full-automation-mode-minutes
.
*
*
* @return The automation mode of the RDS Custom DB instance: full
or all paused
. If
* full
, the DB instance automates monitoring and instance recovery. If all paused
* , the instance pauses automation for the duration set by
* --resume-full-automation-mode-minutes
.
* @see AutomationMode
*/
public String getAutomationMode() {
return this.automationMode;
}
/**
*
* The automation mode of the RDS Custom DB instance: full
or all paused
. If
* full
, the DB instance automates monitoring and instance recovery. If all paused
, the
* instance pauses automation for the duration set by --resume-full-automation-mode-minutes
.
*
*
* @param automationMode
* The automation mode of the RDS Custom DB instance: full
or all paused
. If
* full
, the DB instance automates monitoring and instance recovery. If all paused
,
* the instance pauses automation for the duration set by --resume-full-automation-mode-minutes
.
* @return Returns a reference to this object so that method calls can be chained together.
* @see AutomationMode
*/
public DBInstance withAutomationMode(String automationMode) {
setAutomationMode(automationMode);
return this;
}
/**
*
* The automation mode of the RDS Custom DB instance: full
or all paused
. If
* full
, the DB instance automates monitoring and instance recovery. If all paused
, the
* instance pauses automation for the duration set by --resume-full-automation-mode-minutes
.
*
*
* @param automationMode
* The automation mode of the RDS Custom DB instance: full
or all paused
. If
* full
, the DB instance automates monitoring and instance recovery. If all paused
,
* the instance pauses automation for the duration set by --resume-full-automation-mode-minutes
.
* @return Returns a reference to this object so that method calls can be chained together.
* @see AutomationMode
*/
public DBInstance withAutomationMode(AutomationMode automationMode) {
this.automationMode = automationMode.toString();
return this;
}
/**
*
* The number of minutes to pause the automation. When the time period ends, RDS Custom resumes full automation. The
* minimum value is 60 (default). The maximum value is 1,440.
*
*
* @param resumeFullAutomationModeTime
* The number of minutes to pause the automation. When the time period ends, RDS Custom resumes full
* automation. The minimum value is 60 (default). The maximum value is 1,440.
*/
public void setResumeFullAutomationModeTime(java.util.Date resumeFullAutomationModeTime) {
this.resumeFullAutomationModeTime = resumeFullAutomationModeTime;
}
/**
*
* The number of minutes to pause the automation. When the time period ends, RDS Custom resumes full automation. The
* minimum value is 60 (default). The maximum value is 1,440.
*
*
* @return The number of minutes to pause the automation. When the time period ends, RDS Custom resumes full
* automation. The minimum value is 60 (default). The maximum value is 1,440.
*/
public java.util.Date getResumeFullAutomationModeTime() {
return this.resumeFullAutomationModeTime;
}
/**
*
* The number of minutes to pause the automation. When the time period ends, RDS Custom resumes full automation. The
* minimum value is 60 (default). The maximum value is 1,440.
*
*
* @param resumeFullAutomationModeTime
* The number of minutes to pause the automation. When the time period ends, RDS Custom resumes full
* automation. The minimum value is 60 (default). The maximum value is 1,440.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DBInstance withResumeFullAutomationModeTime(java.util.Date resumeFullAutomationModeTime) {
setResumeFullAutomationModeTime(resumeFullAutomationModeTime);
return this;
}
/**
*
* The instance profile associated with the underlying Amazon EC2 instance of an RDS Custom DB instance. The
* instance profile must meet the following requirements:
*
*
* -
*
* The profile must exist in your account.
*
*
* -
*
* The profile must have an IAM role that Amazon EC2 has permissions to assume.
*
*
* -
*
* The instance profile name and the associated IAM role name must start with the prefix AWSRDSCustom
.
*
*
*
*
* For the list of permissions required for the IAM role, see
* Configure IAM and your VPC in the Amazon RDS User Guide.
*
*
* @param customIamInstanceProfile
* The instance profile associated with the underlying Amazon EC2 instance of an RDS Custom DB instance. The
* instance profile must meet the following requirements:
*
* -
*
* The profile must exist in your account.
*
*
* -
*
* The profile must have an IAM role that Amazon EC2 has permissions to assume.
*
*
* -
*
* The instance profile name and the associated IAM role name must start with the prefix
* AWSRDSCustom
.
*
*
*
*
* For the list of permissions required for the IAM role, see
* Configure IAM and your VPC in the Amazon RDS User Guide.
*/
public void setCustomIamInstanceProfile(String customIamInstanceProfile) {
this.customIamInstanceProfile = customIamInstanceProfile;
}
/**
*
* The instance profile associated with the underlying Amazon EC2 instance of an RDS Custom DB instance. The
* instance profile must meet the following requirements:
*
*
* -
*
* The profile must exist in your account.
*
*
* -
*
* The profile must have an IAM role that Amazon EC2 has permissions to assume.
*
*
* -
*
* The instance profile name and the associated IAM role name must start with the prefix AWSRDSCustom
.
*
*
*
*
* For the list of permissions required for the IAM role, see
* Configure IAM and your VPC in the Amazon RDS User Guide.
*
*
* @return The instance profile associated with the underlying Amazon EC2 instance of an RDS Custom DB instance. The
* instance profile must meet the following requirements:
*
* -
*
* The profile must exist in your account.
*
*
* -
*
* The profile must have an IAM role that Amazon EC2 has permissions to assume.
*
*
* -
*
* The instance profile name and the associated IAM role name must start with the prefix
* AWSRDSCustom
.
*
*
*
*
* For the list of permissions required for the IAM role, see Configure IAM and your VPC in the Amazon RDS User Guide.
*/
public String getCustomIamInstanceProfile() {
return this.customIamInstanceProfile;
}
/**
*
* The instance profile associated with the underlying Amazon EC2 instance of an RDS Custom DB instance. The
* instance profile must meet the following requirements:
*
*
* -
*
* The profile must exist in your account.
*
*
* -
*
* The profile must have an IAM role that Amazon EC2 has permissions to assume.
*
*
* -
*
* The instance profile name and the associated IAM role name must start with the prefix AWSRDSCustom
.
*
*
*
*
* For the list of permissions required for the IAM role, see
* Configure IAM and your VPC in the Amazon RDS User Guide.
*
*
* @param customIamInstanceProfile
* The instance profile associated with the underlying Amazon EC2 instance of an RDS Custom DB instance. The
* instance profile must meet the following requirements:
*
* -
*
* The profile must exist in your account.
*
*
* -
*
* The profile must have an IAM role that Amazon EC2 has permissions to assume.
*
*
* -
*
* The instance profile name and the associated IAM role name must start with the prefix
* AWSRDSCustom
.
*
*
*
*
* For the list of permissions required for the IAM role, see
* Configure IAM and your VPC in the Amazon RDS User Guide.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DBInstance withCustomIamInstanceProfile(String customIamInstanceProfile) {
setCustomIamInstanceProfile(customIamInstanceProfile);
return this;
}
/**
*
* The location where automated backups and manual snapshots are stored: Amazon Web Services Outposts or the Amazon
* Web Services Region.
*
*
* @param backupTarget
* The location where automated backups and manual snapshots are stored: Amazon Web Services Outposts or the
* Amazon Web Services Region.
*/
public void setBackupTarget(String backupTarget) {
this.backupTarget = backupTarget;
}
/**
*
* The location where automated backups and manual snapshots are stored: Amazon Web Services Outposts or the Amazon
* Web Services Region.
*
*
* @return The location where automated backups and manual snapshots are stored: Amazon Web Services Outposts or the
* Amazon Web Services Region.
*/
public String getBackupTarget() {
return this.backupTarget;
}
/**
*
* The location where automated backups and manual snapshots are stored: Amazon Web Services Outposts or the Amazon
* Web Services Region.
*
*
* @param backupTarget
* The location where automated backups and manual snapshots are stored: Amazon Web Services Outposts or the
* Amazon Web Services Region.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DBInstance withBackupTarget(String backupTarget) {
setBackupTarget(backupTarget);
return this;
}
/**
*
* The network type of the DB instance.
*
*
* The network type is determined by the DBSubnetGroup
specified for the DB instance. A
* DBSubnetGroup
can support only the IPv4 protocol or the IPv4 and the IPv6 protocols (
* DUAL
).
*
*
* For more information, see Working
* with a DB instance in a VPC in the Amazon RDS User Guide and
* Working with a DB instance in a VPC in the Amazon Aurora User Guide.
*
*
* Valid Values: IPV4 | DUAL
*
*
* @param networkType
* The network type of the DB instance.
*
* The network type is determined by the DBSubnetGroup
specified for the DB instance. A
* DBSubnetGroup
can support only the IPv4 protocol or the IPv4 and the IPv6 protocols (
* DUAL
).
*
*
* For more information, see
* Working with a DB instance in a VPC in the Amazon RDS User Guide and
* Working with a DB instance in a VPC in the Amazon Aurora User Guide.
*
*
* Valid Values: IPV4 | DUAL
*/
public void setNetworkType(String networkType) {
this.networkType = networkType;
}
/**
*
* The network type of the DB instance.
*
*
* The network type is determined by the DBSubnetGroup
specified for the DB instance. A
* DBSubnetGroup
can support only the IPv4 protocol or the IPv4 and the IPv6 protocols (
* DUAL
).
*
*
* For more information, see Working
* with a DB instance in a VPC in the Amazon RDS User Guide and
* Working with a DB instance in a VPC in the Amazon Aurora User Guide.
*
*
* Valid Values: IPV4 | DUAL
*
*
* @return The network type of the DB instance.
*
* The network type is determined by the DBSubnetGroup
specified for the DB instance. A
* DBSubnetGroup
can support only the IPv4 protocol or the IPv4 and the IPv6 protocols (
* DUAL
).
*
*
* For more information, see
* Working with a DB instance in a VPC in the Amazon RDS User Guide and Working with a DB instance in a VPC in the Amazon Aurora User Guide.
*
*
* Valid Values: IPV4 | DUAL
*/
public String getNetworkType() {
return this.networkType;
}
/**
*
* The network type of the DB instance.
*
*
* The network type is determined by the DBSubnetGroup
specified for the DB instance. A
* DBSubnetGroup
can support only the IPv4 protocol or the IPv4 and the IPv6 protocols (
* DUAL
).
*
*
* For more information, see Working
* with a DB instance in a VPC in the Amazon RDS User Guide and
* Working with a DB instance in a VPC in the Amazon Aurora User Guide.
*
*
* Valid Values: IPV4 | DUAL
*
*
* @param networkType
* The network type of the DB instance.
*
* The network type is determined by the DBSubnetGroup
specified for the DB instance. A
* DBSubnetGroup
can support only the IPv4 protocol or the IPv4 and the IPv6 protocols (
* DUAL
).
*
*
* For more information, see
* Working with a DB instance in a VPC in the Amazon RDS User Guide and
* Working with a DB instance in a VPC in the Amazon Aurora User Guide.
*
*
* Valid Values: IPV4 | DUAL
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DBInstance withNetworkType(String networkType) {
setNetworkType(networkType);
return this;
}
/**
*
* The status of the policy state of the activity stream.
*
*
* @param activityStreamPolicyStatus
* The status of the policy state of the activity stream.
* @see ActivityStreamPolicyStatus
*/
public void setActivityStreamPolicyStatus(String activityStreamPolicyStatus) {
this.activityStreamPolicyStatus = activityStreamPolicyStatus;
}
/**
*
* The status of the policy state of the activity stream.
*
*
* @return The status of the policy state of the activity stream.
* @see ActivityStreamPolicyStatus
*/
public String getActivityStreamPolicyStatus() {
return this.activityStreamPolicyStatus;
}
/**
*
* The status of the policy state of the activity stream.
*
*
* @param activityStreamPolicyStatus
* The status of the policy state of the activity stream.
* @return Returns a reference to this object so that method calls can be chained together.
* @see ActivityStreamPolicyStatus
*/
public DBInstance withActivityStreamPolicyStatus(String activityStreamPolicyStatus) {
setActivityStreamPolicyStatus(activityStreamPolicyStatus);
return this;
}
/**
*
* The status of the policy state of the activity stream.
*
*
* @param activityStreamPolicyStatus
* The status of the policy state of the activity stream.
* @return Returns a reference to this object so that method calls can be chained together.
* @see ActivityStreamPolicyStatus
*/
public DBInstance withActivityStreamPolicyStatus(ActivityStreamPolicyStatus activityStreamPolicyStatus) {
this.activityStreamPolicyStatus = activityStreamPolicyStatus.toString();
return this;
}
/**
*
* The storage throughput for the DB instance.
*
*
* This setting applies only to the gp3
storage type.
*
*
* @param storageThroughput
* The storage throughput for the DB instance.
*
* This setting applies only to the gp3
storage type.
*/
public void setStorageThroughput(Integer storageThroughput) {
this.storageThroughput = storageThroughput;
}
/**
*
* The storage throughput for the DB instance.
*
*
* This setting applies only to the gp3
storage type.
*
*
* @return The storage throughput for the DB instance.
*
* This setting applies only to the gp3
storage type.
*/
public Integer getStorageThroughput() {
return this.storageThroughput;
}
/**
*
* The storage throughput for the DB instance.
*
*
* This setting applies only to the gp3
storage type.
*
*
* @param storageThroughput
* The storage throughput for the DB instance.
*
* This setting applies only to the gp3
storage type.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DBInstance withStorageThroughput(Integer storageThroughput) {
setStorageThroughput(storageThroughput);
return this;
}
/**
*
* The Oracle system ID (Oracle SID) for a container database (CDB). The Oracle SID is also the name of the CDB.
* This setting is only valid for RDS Custom DB instances.
*
*
* @param dBSystemId
* The Oracle system ID (Oracle SID) for a container database (CDB). The Oracle SID is also the name of the
* CDB. This setting is only valid for RDS Custom DB instances.
*/
public void setDBSystemId(String dBSystemId) {
this.dBSystemId = dBSystemId;
}
/**
*
* The Oracle system ID (Oracle SID) for a container database (CDB). The Oracle SID is also the name of the CDB.
* This setting is only valid for RDS Custom DB instances.
*
*
* @return The Oracle system ID (Oracle SID) for a container database (CDB). The Oracle SID is also the name of the
* CDB. This setting is only valid for RDS Custom DB instances.
*/
public String getDBSystemId() {
return this.dBSystemId;
}
/**
*
* The Oracle system ID (Oracle SID) for a container database (CDB). The Oracle SID is also the name of the CDB.
* This setting is only valid for RDS Custom DB instances.
*
*
* @param dBSystemId
* The Oracle system ID (Oracle SID) for a container database (CDB). The Oracle SID is also the name of the
* CDB. This setting is only valid for RDS Custom DB instances.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DBInstance withDBSystemId(String dBSystemId) {
setDBSystemId(dBSystemId);
return this;
}
/**
*
* The secret managed by RDS in Amazon Web Services Secrets Manager for the master user password.
*
*
* For more information, see Password management with
* Amazon Web Services Secrets Manager in the Amazon RDS User Guide.
*
*
* @param masterUserSecret
* The secret managed by RDS in Amazon Web Services Secrets Manager for the master user password.
*
* For more information, see Password management
* with Amazon Web Services Secrets Manager in the Amazon RDS User Guide.
*/
public void setMasterUserSecret(MasterUserSecret masterUserSecret) {
this.masterUserSecret = masterUserSecret;
}
/**
*
* The secret managed by RDS in Amazon Web Services Secrets Manager for the master user password.
*
*
* For more information, see Password management with
* Amazon Web Services Secrets Manager in the Amazon RDS User Guide.
*
*
* @return The secret managed by RDS in Amazon Web Services Secrets Manager for the master user password.
*
* For more information, see Password
* management with Amazon Web Services Secrets Manager in the Amazon RDS User Guide.
*/
public MasterUserSecret getMasterUserSecret() {
return this.masterUserSecret;
}
/**
*
* The secret managed by RDS in Amazon Web Services Secrets Manager for the master user password.
*
*
* For more information, see Password management with
* Amazon Web Services Secrets Manager in the Amazon RDS User Guide.
*
*
* @param masterUserSecret
* The secret managed by RDS in Amazon Web Services Secrets Manager for the master user password.
*
* For more information, see Password management
* with Amazon Web Services Secrets Manager in the Amazon RDS User Guide.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DBInstance withMasterUserSecret(MasterUserSecret masterUserSecret) {
setMasterUserSecret(masterUserSecret);
return this;
}
/**
*
* The details of the DB instance's server certificate.
*
*
* @param certificateDetails
* The details of the DB instance's server certificate.
*/
public void setCertificateDetails(CertificateDetails certificateDetails) {
this.certificateDetails = certificateDetails;
}
/**
*
* The details of the DB instance's server certificate.
*
*
* @return The details of the DB instance's server certificate.
*/
public CertificateDetails getCertificateDetails() {
return this.certificateDetails;
}
/**
*
* The details of the DB instance's server certificate.
*
*
* @param certificateDetails
* The details of the DB instance's server certificate.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DBInstance withCertificateDetails(CertificateDetails certificateDetails) {
setCertificateDetails(certificateDetails);
return this;
}
/**
*
* The identifier of the source DB cluster if this DB instance is a read replica.
*
*
* @param readReplicaSourceDBClusterIdentifier
* The identifier of the source DB cluster if this DB instance is a read replica.
*/
public void setReadReplicaSourceDBClusterIdentifier(String readReplicaSourceDBClusterIdentifier) {
this.readReplicaSourceDBClusterIdentifier = readReplicaSourceDBClusterIdentifier;
}
/**
*
* The identifier of the source DB cluster if this DB instance is a read replica.
*
*
* @return The identifier of the source DB cluster if this DB instance is a read replica.
*/
public String getReadReplicaSourceDBClusterIdentifier() {
return this.readReplicaSourceDBClusterIdentifier;
}
/**
*
* The identifier of the source DB cluster if this DB instance is a read replica.
*
*
* @param readReplicaSourceDBClusterIdentifier
* The identifier of the source DB cluster if this DB instance is a read replica.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DBInstance withReadReplicaSourceDBClusterIdentifier(String readReplicaSourceDBClusterIdentifier) {
setReadReplicaSourceDBClusterIdentifier(readReplicaSourceDBClusterIdentifier);
return this;
}
/**
*
* The progress of the storage optimization operation as a percentage.
*
*
* @param percentProgress
* The progress of the storage optimization operation as a percentage.
*/
public void setPercentProgress(String percentProgress) {
this.percentProgress = percentProgress;
}
/**
*
* The progress of the storage optimization operation as a percentage.
*
*
* @return The progress of the storage optimization operation as a percentage.
*/
public String getPercentProgress() {
return this.percentProgress;
}
/**
*
* The progress of the storage optimization operation as a percentage.
*
*
* @param percentProgress
* The progress of the storage optimization operation as a percentage.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DBInstance withPercentProgress(String percentProgress) {
setPercentProgress(percentProgress);
return this;
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getDBInstanceIdentifier() != null)
sb.append("DBInstanceIdentifier: ").append(getDBInstanceIdentifier()).append(",");
if (getDBInstanceClass() != null)
sb.append("DBInstanceClass: ").append(getDBInstanceClass()).append(",");
if (getEngine() != null)
sb.append("Engine: ").append(getEngine()).append(",");
if (getDBInstanceStatus() != null)
sb.append("DBInstanceStatus: ").append(getDBInstanceStatus()).append(",");
if (getAutomaticRestartTime() != null)
sb.append("AutomaticRestartTime: ").append(getAutomaticRestartTime()).append(",");
if (getMasterUsername() != null)
sb.append("MasterUsername: ").append(getMasterUsername()).append(",");
if (getDBName() != null)
sb.append("DBName: ").append(getDBName()).append(",");
if (getEndpoint() != null)
sb.append("Endpoint: ").append(getEndpoint()).append(",");
if (getAllocatedStorage() != null)
sb.append("AllocatedStorage: ").append(getAllocatedStorage()).append(",");
if (getInstanceCreateTime() != null)
sb.append("InstanceCreateTime: ").append(getInstanceCreateTime()).append(",");
if (getPreferredBackupWindow() != null)
sb.append("PreferredBackupWindow: ").append(getPreferredBackupWindow()).append(",");
if (getBackupRetentionPeriod() != null)
sb.append("BackupRetentionPeriod: ").append(getBackupRetentionPeriod()).append(",");
if (getDBSecurityGroups() != null)
sb.append("DBSecurityGroups: ").append(getDBSecurityGroups()).append(",");
if (getVpcSecurityGroups() != null)
sb.append("VpcSecurityGroups: ").append(getVpcSecurityGroups()).append(",");
if (getDBParameterGroups() != null)
sb.append("DBParameterGroups: ").append(getDBParameterGroups()).append(",");
if (getAvailabilityZone() != null)
sb.append("AvailabilityZone: ").append(getAvailabilityZone()).append(",");
if (getDBSubnetGroup() != null)
sb.append("DBSubnetGroup: ").append(getDBSubnetGroup()).append(",");
if (getPreferredMaintenanceWindow() != null)
sb.append("PreferredMaintenanceWindow: ").append(getPreferredMaintenanceWindow()).append(",");
if (getPendingModifiedValues() != null)
sb.append("PendingModifiedValues: ").append(getPendingModifiedValues()).append(",");
if (getLatestRestorableTime() != null)
sb.append("LatestRestorableTime: ").append(getLatestRestorableTime()).append(",");
if (getMultiAZ() != null)
sb.append("MultiAZ: ").append(getMultiAZ()).append(",");
if (getEngineVersion() != null)
sb.append("EngineVersion: ").append(getEngineVersion()).append(",");
if (getAutoMinorVersionUpgrade() != null)
sb.append("AutoMinorVersionUpgrade: ").append(getAutoMinorVersionUpgrade()).append(",");
if (getReadReplicaSourceDBInstanceIdentifier() != null)
sb.append("ReadReplicaSourceDBInstanceIdentifier: ").append(getReadReplicaSourceDBInstanceIdentifier()).append(",");
if (getReadReplicaDBInstanceIdentifiers() != null)
sb.append("ReadReplicaDBInstanceIdentifiers: ").append(getReadReplicaDBInstanceIdentifiers()).append(",");
if (getReadReplicaDBClusterIdentifiers() != null)
sb.append("ReadReplicaDBClusterIdentifiers: ").append(getReadReplicaDBClusterIdentifiers()).append(",");
if (getReplicaMode() != null)
sb.append("ReplicaMode: ").append(getReplicaMode()).append(",");
if (getLicenseModel() != null)
sb.append("LicenseModel: ").append(getLicenseModel()).append(",");
if (getIops() != null)
sb.append("Iops: ").append(getIops()).append(",");
if (getOptionGroupMemberships() != null)
sb.append("OptionGroupMemberships: ").append(getOptionGroupMemberships()).append(",");
if (getCharacterSetName() != null)
sb.append("CharacterSetName: ").append(getCharacterSetName()).append(",");
if (getNcharCharacterSetName() != null)
sb.append("NcharCharacterSetName: ").append(getNcharCharacterSetName()).append(",");
if (getSecondaryAvailabilityZone() != null)
sb.append("SecondaryAvailabilityZone: ").append(getSecondaryAvailabilityZone()).append(",");
if (getPubliclyAccessible() != null)
sb.append("PubliclyAccessible: ").append(getPubliclyAccessible()).append(",");
if (getStatusInfos() != null)
sb.append("StatusInfos: ").append(getStatusInfos()).append(",");
if (getStorageType() != null)
sb.append("StorageType: ").append(getStorageType()).append(",");
if (getTdeCredentialArn() != null)
sb.append("TdeCredentialArn: ").append(getTdeCredentialArn()).append(",");
if (getDbInstancePort() != null)
sb.append("DbInstancePort: ").append(getDbInstancePort()).append(",");
if (getDBClusterIdentifier() != null)
sb.append("DBClusterIdentifier: ").append(getDBClusterIdentifier()).append(",");
if (getStorageEncrypted() != null)
sb.append("StorageEncrypted: ").append(getStorageEncrypted()).append(",");
if (getKmsKeyId() != null)
sb.append("KmsKeyId: ").append(getKmsKeyId()).append(",");
if (getDbiResourceId() != null)
sb.append("DbiResourceId: ").append(getDbiResourceId()).append(",");
if (getCACertificateIdentifier() != null)
sb.append("CACertificateIdentifier: ").append(getCACertificateIdentifier()).append(",");
if (getDomainMemberships() != null)
sb.append("DomainMemberships: ").append(getDomainMemberships()).append(",");
if (getCopyTagsToSnapshot() != null)
sb.append("CopyTagsToSnapshot: ").append(getCopyTagsToSnapshot()).append(",");
if (getMonitoringInterval() != null)
sb.append("MonitoringInterval: ").append(getMonitoringInterval()).append(",");
if (getEnhancedMonitoringResourceArn() != null)
sb.append("EnhancedMonitoringResourceArn: ").append(getEnhancedMonitoringResourceArn()).append(",");
if (getMonitoringRoleArn() != null)
sb.append("MonitoringRoleArn: ").append(getMonitoringRoleArn()).append(",");
if (getPromotionTier() != null)
sb.append("PromotionTier: ").append(getPromotionTier()).append(",");
if (getDBInstanceArn() != null)
sb.append("DBInstanceArn: ").append(getDBInstanceArn()).append(",");
if (getTimezone() != null)
sb.append("Timezone: ").append(getTimezone()).append(",");
if (getIAMDatabaseAuthenticationEnabled() != null)
sb.append("IAMDatabaseAuthenticationEnabled: ").append(getIAMDatabaseAuthenticationEnabled()).append(",");
if (getPerformanceInsightsEnabled() != null)
sb.append("PerformanceInsightsEnabled: ").append(getPerformanceInsightsEnabled()).append(",");
if (getPerformanceInsightsKMSKeyId() != null)
sb.append("PerformanceInsightsKMSKeyId: ").append(getPerformanceInsightsKMSKeyId()).append(",");
if (getPerformanceInsightsRetentionPeriod() != null)
sb.append("PerformanceInsightsRetentionPeriod: ").append(getPerformanceInsightsRetentionPeriod()).append(",");
if (getEnabledCloudwatchLogsExports() != null)
sb.append("EnabledCloudwatchLogsExports: ").append(getEnabledCloudwatchLogsExports()).append(",");
if (getProcessorFeatures() != null)
sb.append("ProcessorFeatures: ").append(getProcessorFeatures()).append(",");
if (getDeletionProtection() != null)
sb.append("DeletionProtection: ").append(getDeletionProtection()).append(",");
if (getAssociatedRoles() != null)
sb.append("AssociatedRoles: ").append(getAssociatedRoles()).append(",");
if (getListenerEndpoint() != null)
sb.append("ListenerEndpoint: ").append(getListenerEndpoint()).append(",");
if (getMaxAllocatedStorage() != null)
sb.append("MaxAllocatedStorage: ").append(getMaxAllocatedStorage()).append(",");
if (getTagList() != null)
sb.append("TagList: ").append(getTagList()).append(",");
if (getDBInstanceAutomatedBackupsReplications() != null)
sb.append("DBInstanceAutomatedBackupsReplications: ").append(getDBInstanceAutomatedBackupsReplications()).append(",");
if (getCustomerOwnedIpEnabled() != null)
sb.append("CustomerOwnedIpEnabled: ").append(getCustomerOwnedIpEnabled()).append(",");
if (getAwsBackupRecoveryPointArn() != null)
sb.append("AwsBackupRecoveryPointArn: ").append(getAwsBackupRecoveryPointArn()).append(",");
if (getActivityStreamStatus() != null)
sb.append("ActivityStreamStatus: ").append(getActivityStreamStatus()).append(",");
if (getActivityStreamKmsKeyId() != null)
sb.append("ActivityStreamKmsKeyId: ").append(getActivityStreamKmsKeyId()).append(",");
if (getActivityStreamKinesisStreamName() != null)
sb.append("ActivityStreamKinesisStreamName: ").append(getActivityStreamKinesisStreamName()).append(",");
if (getActivityStreamMode() != null)
sb.append("ActivityStreamMode: ").append(getActivityStreamMode()).append(",");
if (getActivityStreamEngineNativeAuditFieldsIncluded() != null)
sb.append("ActivityStreamEngineNativeAuditFieldsIncluded: ").append(getActivityStreamEngineNativeAuditFieldsIncluded()).append(",");
if (getAutomationMode() != null)
sb.append("AutomationMode: ").append(getAutomationMode()).append(",");
if (getResumeFullAutomationModeTime() != null)
sb.append("ResumeFullAutomationModeTime: ").append(getResumeFullAutomationModeTime()).append(",");
if (getCustomIamInstanceProfile() != null)
sb.append("CustomIamInstanceProfile: ").append(getCustomIamInstanceProfile()).append(",");
if (getBackupTarget() != null)
sb.append("BackupTarget: ").append(getBackupTarget()).append(",");
if (getNetworkType() != null)
sb.append("NetworkType: ").append(getNetworkType()).append(",");
if (getActivityStreamPolicyStatus() != null)
sb.append("ActivityStreamPolicyStatus: ").append(getActivityStreamPolicyStatus()).append(",");
if (getStorageThroughput() != null)
sb.append("StorageThroughput: ").append(getStorageThroughput()).append(",");
if (getDBSystemId() != null)
sb.append("DBSystemId: ").append(getDBSystemId()).append(",");
if (getMasterUserSecret() != null)
sb.append("MasterUserSecret: ").append(getMasterUserSecret()).append(",");
if (getCertificateDetails() != null)
sb.append("CertificateDetails: ").append(getCertificateDetails()).append(",");
if (getReadReplicaSourceDBClusterIdentifier() != null)
sb.append("ReadReplicaSourceDBClusterIdentifier: ").append(getReadReplicaSourceDBClusterIdentifier()).append(",");
if (getPercentProgress() != null)
sb.append("PercentProgress: ").append(getPercentProgress());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof DBInstance == false)
return false;
DBInstance other = (DBInstance) obj;
if (other.getDBInstanceIdentifier() == null ^ this.getDBInstanceIdentifier() == null)
return false;
if (other.getDBInstanceIdentifier() != null && other.getDBInstanceIdentifier().equals(this.getDBInstanceIdentifier()) == false)
return false;
if (other.getDBInstanceClass() == null ^ this.getDBInstanceClass() == null)
return false;
if (other.getDBInstanceClass() != null && other.getDBInstanceClass().equals(this.getDBInstanceClass()) == false)
return false;
if (other.getEngine() == null ^ this.getEngine() == null)
return false;
if (other.getEngine() != null && other.getEngine().equals(this.getEngine()) == false)
return false;
if (other.getDBInstanceStatus() == null ^ this.getDBInstanceStatus() == null)
return false;
if (other.getDBInstanceStatus() != null && other.getDBInstanceStatus().equals(this.getDBInstanceStatus()) == false)
return false;
if (other.getAutomaticRestartTime() == null ^ this.getAutomaticRestartTime() == null)
return false;
if (other.getAutomaticRestartTime() != null && other.getAutomaticRestartTime().equals(this.getAutomaticRestartTime()) == false)
return false;
if (other.getMasterUsername() == null ^ this.getMasterUsername() == null)
return false;
if (other.getMasterUsername() != null && other.getMasterUsername().equals(this.getMasterUsername()) == false)
return false;
if (other.getDBName() == null ^ this.getDBName() == null)
return false;
if (other.getDBName() != null && other.getDBName().equals(this.getDBName()) == false)
return false;
if (other.getEndpoint() == null ^ this.getEndpoint() == null)
return false;
if (other.getEndpoint() != null && other.getEndpoint().equals(this.getEndpoint()) == false)
return false;
if (other.getAllocatedStorage() == null ^ this.getAllocatedStorage() == null)
return false;
if (other.getAllocatedStorage() != null && other.getAllocatedStorage().equals(this.getAllocatedStorage()) == false)
return false;
if (other.getInstanceCreateTime() == null ^ this.getInstanceCreateTime() == null)
return false;
if (other.getInstanceCreateTime() != null && other.getInstanceCreateTime().equals(this.getInstanceCreateTime()) == false)
return false;
if (other.getPreferredBackupWindow() == null ^ this.getPreferredBackupWindow() == null)
return false;
if (other.getPreferredBackupWindow() != null && other.getPreferredBackupWindow().equals(this.getPreferredBackupWindow()) == false)
return false;
if (other.getBackupRetentionPeriod() == null ^ this.getBackupRetentionPeriod() == null)
return false;
if (other.getBackupRetentionPeriod() != null && other.getBackupRetentionPeriod().equals(this.getBackupRetentionPeriod()) == false)
return false;
if (other.getDBSecurityGroups() == null ^ this.getDBSecurityGroups() == null)
return false;
if (other.getDBSecurityGroups() != null && other.getDBSecurityGroups().equals(this.getDBSecurityGroups()) == false)
return false;
if (other.getVpcSecurityGroups() == null ^ this.getVpcSecurityGroups() == null)
return false;
if (other.getVpcSecurityGroups() != null && other.getVpcSecurityGroups().equals(this.getVpcSecurityGroups()) == false)
return false;
if (other.getDBParameterGroups() == null ^ this.getDBParameterGroups() == null)
return false;
if (other.getDBParameterGroups() != null && other.getDBParameterGroups().equals(this.getDBParameterGroups()) == false)
return false;
if (other.getAvailabilityZone() == null ^ this.getAvailabilityZone() == null)
return false;
if (other.getAvailabilityZone() != null && other.getAvailabilityZone().equals(this.getAvailabilityZone()) == false)
return false;
if (other.getDBSubnetGroup() == null ^ this.getDBSubnetGroup() == null)
return false;
if (other.getDBSubnetGroup() != null && other.getDBSubnetGroup().equals(this.getDBSubnetGroup()) == false)
return false;
if (other.getPreferredMaintenanceWindow() == null ^ this.getPreferredMaintenanceWindow() == null)
return false;
if (other.getPreferredMaintenanceWindow() != null && other.getPreferredMaintenanceWindow().equals(this.getPreferredMaintenanceWindow()) == false)
return false;
if (other.getPendingModifiedValues() == null ^ this.getPendingModifiedValues() == null)
return false;
if (other.getPendingModifiedValues() != null && other.getPendingModifiedValues().equals(this.getPendingModifiedValues()) == false)
return false;
if (other.getLatestRestorableTime() == null ^ this.getLatestRestorableTime() == null)
return false;
if (other.getLatestRestorableTime() != null && other.getLatestRestorableTime().equals(this.getLatestRestorableTime()) == false)
return false;
if (other.getMultiAZ() == null ^ this.getMultiAZ() == null)
return false;
if (other.getMultiAZ() != null && other.getMultiAZ().equals(this.getMultiAZ()) == false)
return false;
if (other.getEngineVersion() == null ^ this.getEngineVersion() == null)
return false;
if (other.getEngineVersion() != null && other.getEngineVersion().equals(this.getEngineVersion()) == false)
return false;
if (other.getAutoMinorVersionUpgrade() == null ^ this.getAutoMinorVersionUpgrade() == null)
return false;
if (other.getAutoMinorVersionUpgrade() != null && other.getAutoMinorVersionUpgrade().equals(this.getAutoMinorVersionUpgrade()) == false)
return false;
if (other.getReadReplicaSourceDBInstanceIdentifier() == null ^ this.getReadReplicaSourceDBInstanceIdentifier() == null)
return false;
if (other.getReadReplicaSourceDBInstanceIdentifier() != null
&& other.getReadReplicaSourceDBInstanceIdentifier().equals(this.getReadReplicaSourceDBInstanceIdentifier()) == false)
return false;
if (other.getReadReplicaDBInstanceIdentifiers() == null ^ this.getReadReplicaDBInstanceIdentifiers() == null)
return false;
if (other.getReadReplicaDBInstanceIdentifiers() != null
&& other.getReadReplicaDBInstanceIdentifiers().equals(this.getReadReplicaDBInstanceIdentifiers()) == false)
return false;
if (other.getReadReplicaDBClusterIdentifiers() == null ^ this.getReadReplicaDBClusterIdentifiers() == null)
return false;
if (other.getReadReplicaDBClusterIdentifiers() != null
&& other.getReadReplicaDBClusterIdentifiers().equals(this.getReadReplicaDBClusterIdentifiers()) == false)
return false;
if (other.getReplicaMode() == null ^ this.getReplicaMode() == null)
return false;
if (other.getReplicaMode() != null && other.getReplicaMode().equals(this.getReplicaMode()) == false)
return false;
if (other.getLicenseModel() == null ^ this.getLicenseModel() == null)
return false;
if (other.getLicenseModel() != null && other.getLicenseModel().equals(this.getLicenseModel()) == false)
return false;
if (other.getIops() == null ^ this.getIops() == null)
return false;
if (other.getIops() != null && other.getIops().equals(this.getIops()) == false)
return false;
if (other.getOptionGroupMemberships() == null ^ this.getOptionGroupMemberships() == null)
return false;
if (other.getOptionGroupMemberships() != null && other.getOptionGroupMemberships().equals(this.getOptionGroupMemberships()) == false)
return false;
if (other.getCharacterSetName() == null ^ this.getCharacterSetName() == null)
return false;
if (other.getCharacterSetName() != null && other.getCharacterSetName().equals(this.getCharacterSetName()) == false)
return false;
if (other.getNcharCharacterSetName() == null ^ this.getNcharCharacterSetName() == null)
return false;
if (other.getNcharCharacterSetName() != null && other.getNcharCharacterSetName().equals(this.getNcharCharacterSetName()) == false)
return false;
if (other.getSecondaryAvailabilityZone() == null ^ this.getSecondaryAvailabilityZone() == null)
return false;
if (other.getSecondaryAvailabilityZone() != null && other.getSecondaryAvailabilityZone().equals(this.getSecondaryAvailabilityZone()) == false)
return false;
if (other.getPubliclyAccessible() == null ^ this.getPubliclyAccessible() == null)
return false;
if (other.getPubliclyAccessible() != null && other.getPubliclyAccessible().equals(this.getPubliclyAccessible()) == false)
return false;
if (other.getStatusInfos() == null ^ this.getStatusInfos() == null)
return false;
if (other.getStatusInfos() != null && other.getStatusInfos().equals(this.getStatusInfos()) == false)
return false;
if (other.getStorageType() == null ^ this.getStorageType() == null)
return false;
if (other.getStorageType() != null && other.getStorageType().equals(this.getStorageType()) == false)
return false;
if (other.getTdeCredentialArn() == null ^ this.getTdeCredentialArn() == null)
return false;
if (other.getTdeCredentialArn() != null && other.getTdeCredentialArn().equals(this.getTdeCredentialArn()) == false)
return false;
if (other.getDbInstancePort() == null ^ this.getDbInstancePort() == null)
return false;
if (other.getDbInstancePort() != null && other.getDbInstancePort().equals(this.getDbInstancePort()) == false)
return false;
if (other.getDBClusterIdentifier() == null ^ this.getDBClusterIdentifier() == null)
return false;
if (other.getDBClusterIdentifier() != null && other.getDBClusterIdentifier().equals(this.getDBClusterIdentifier()) == false)
return false;
if (other.getStorageEncrypted() == null ^ this.getStorageEncrypted() == null)
return false;
if (other.getStorageEncrypted() != null && other.getStorageEncrypted().equals(this.getStorageEncrypted()) == false)
return false;
if (other.getKmsKeyId() == null ^ this.getKmsKeyId() == null)
return false;
if (other.getKmsKeyId() != null && other.getKmsKeyId().equals(this.getKmsKeyId()) == false)
return false;
if (other.getDbiResourceId() == null ^ this.getDbiResourceId() == null)
return false;
if (other.getDbiResourceId() != null && other.getDbiResourceId().equals(this.getDbiResourceId()) == false)
return false;
if (other.getCACertificateIdentifier() == null ^ this.getCACertificateIdentifier() == null)
return false;
if (other.getCACertificateIdentifier() != null && other.getCACertificateIdentifier().equals(this.getCACertificateIdentifier()) == false)
return false;
if (other.getDomainMemberships() == null ^ this.getDomainMemberships() == null)
return false;
if (other.getDomainMemberships() != null && other.getDomainMemberships().equals(this.getDomainMemberships()) == false)
return false;
if (other.getCopyTagsToSnapshot() == null ^ this.getCopyTagsToSnapshot() == null)
return false;
if (other.getCopyTagsToSnapshot() != null && other.getCopyTagsToSnapshot().equals(this.getCopyTagsToSnapshot()) == false)
return false;
if (other.getMonitoringInterval() == null ^ this.getMonitoringInterval() == null)
return false;
if (other.getMonitoringInterval() != null && other.getMonitoringInterval().equals(this.getMonitoringInterval()) == false)
return false;
if (other.getEnhancedMonitoringResourceArn() == null ^ this.getEnhancedMonitoringResourceArn() == null)
return false;
if (other.getEnhancedMonitoringResourceArn() != null
&& other.getEnhancedMonitoringResourceArn().equals(this.getEnhancedMonitoringResourceArn()) == false)
return false;
if (other.getMonitoringRoleArn() == null ^ this.getMonitoringRoleArn() == null)
return false;
if (other.getMonitoringRoleArn() != null && other.getMonitoringRoleArn().equals(this.getMonitoringRoleArn()) == false)
return false;
if (other.getPromotionTier() == null ^ this.getPromotionTier() == null)
return false;
if (other.getPromotionTier() != null && other.getPromotionTier().equals(this.getPromotionTier()) == false)
return false;
if (other.getDBInstanceArn() == null ^ this.getDBInstanceArn() == null)
return false;
if (other.getDBInstanceArn() != null && other.getDBInstanceArn().equals(this.getDBInstanceArn()) == false)
return false;
if (other.getTimezone() == null ^ this.getTimezone() == null)
return false;
if (other.getTimezone() != null && other.getTimezone().equals(this.getTimezone()) == false)
return false;
if (other.getIAMDatabaseAuthenticationEnabled() == null ^ this.getIAMDatabaseAuthenticationEnabled() == null)
return false;
if (other.getIAMDatabaseAuthenticationEnabled() != null
&& other.getIAMDatabaseAuthenticationEnabled().equals(this.getIAMDatabaseAuthenticationEnabled()) == false)
return false;
if (other.getPerformanceInsightsEnabled() == null ^ this.getPerformanceInsightsEnabled() == null)
return false;
if (other.getPerformanceInsightsEnabled() != null && other.getPerformanceInsightsEnabled().equals(this.getPerformanceInsightsEnabled()) == false)
return false;
if (other.getPerformanceInsightsKMSKeyId() == null ^ this.getPerformanceInsightsKMSKeyId() == null)
return false;
if (other.getPerformanceInsightsKMSKeyId() != null && other.getPerformanceInsightsKMSKeyId().equals(this.getPerformanceInsightsKMSKeyId()) == false)
return false;
if (other.getPerformanceInsightsRetentionPeriod() == null ^ this.getPerformanceInsightsRetentionPeriod() == null)
return false;
if (other.getPerformanceInsightsRetentionPeriod() != null
&& other.getPerformanceInsightsRetentionPeriod().equals(this.getPerformanceInsightsRetentionPeriod()) == false)
return false;
if (other.getEnabledCloudwatchLogsExports() == null ^ this.getEnabledCloudwatchLogsExports() == null)
return false;
if (other.getEnabledCloudwatchLogsExports() != null && other.getEnabledCloudwatchLogsExports().equals(this.getEnabledCloudwatchLogsExports()) == false)
return false;
if (other.getProcessorFeatures() == null ^ this.getProcessorFeatures() == null)
return false;
if (other.getProcessorFeatures() != null && other.getProcessorFeatures().equals(this.getProcessorFeatures()) == false)
return false;
if (other.getDeletionProtection() == null ^ this.getDeletionProtection() == null)
return false;
if (other.getDeletionProtection() != null && other.getDeletionProtection().equals(this.getDeletionProtection()) == false)
return false;
if (other.getAssociatedRoles() == null ^ this.getAssociatedRoles() == null)
return false;
if (other.getAssociatedRoles() != null && other.getAssociatedRoles().equals(this.getAssociatedRoles()) == false)
return false;
if (other.getListenerEndpoint() == null ^ this.getListenerEndpoint() == null)
return false;
if (other.getListenerEndpoint() != null && other.getListenerEndpoint().equals(this.getListenerEndpoint()) == false)
return false;
if (other.getMaxAllocatedStorage() == null ^ this.getMaxAllocatedStorage() == null)
return false;
if (other.getMaxAllocatedStorage() != null && other.getMaxAllocatedStorage().equals(this.getMaxAllocatedStorage()) == false)
return false;
if (other.getTagList() == null ^ this.getTagList() == null)
return false;
if (other.getTagList() != null && other.getTagList().equals(this.getTagList()) == false)
return false;
if (other.getDBInstanceAutomatedBackupsReplications() == null ^ this.getDBInstanceAutomatedBackupsReplications() == null)
return false;
if (other.getDBInstanceAutomatedBackupsReplications() != null
&& other.getDBInstanceAutomatedBackupsReplications().equals(this.getDBInstanceAutomatedBackupsReplications()) == false)
return false;
if (other.getCustomerOwnedIpEnabled() == null ^ this.getCustomerOwnedIpEnabled() == null)
return false;
if (other.getCustomerOwnedIpEnabled() != null && other.getCustomerOwnedIpEnabled().equals(this.getCustomerOwnedIpEnabled()) == false)
return false;
if (other.getAwsBackupRecoveryPointArn() == null ^ this.getAwsBackupRecoveryPointArn() == null)
return false;
if (other.getAwsBackupRecoveryPointArn() != null && other.getAwsBackupRecoveryPointArn().equals(this.getAwsBackupRecoveryPointArn()) == false)
return false;
if (other.getActivityStreamStatus() == null ^ this.getActivityStreamStatus() == null)
return false;
if (other.getActivityStreamStatus() != null && other.getActivityStreamStatus().equals(this.getActivityStreamStatus()) == false)
return false;
if (other.getActivityStreamKmsKeyId() == null ^ this.getActivityStreamKmsKeyId() == null)
return false;
if (other.getActivityStreamKmsKeyId() != null && other.getActivityStreamKmsKeyId().equals(this.getActivityStreamKmsKeyId()) == false)
return false;
if (other.getActivityStreamKinesisStreamName() == null ^ this.getActivityStreamKinesisStreamName() == null)
return false;
if (other.getActivityStreamKinesisStreamName() != null
&& other.getActivityStreamKinesisStreamName().equals(this.getActivityStreamKinesisStreamName()) == false)
return false;
if (other.getActivityStreamMode() == null ^ this.getActivityStreamMode() == null)
return false;
if (other.getActivityStreamMode() != null && other.getActivityStreamMode().equals(this.getActivityStreamMode()) == false)
return false;
if (other.getActivityStreamEngineNativeAuditFieldsIncluded() == null ^ this.getActivityStreamEngineNativeAuditFieldsIncluded() == null)
return false;
if (other.getActivityStreamEngineNativeAuditFieldsIncluded() != null
&& other.getActivityStreamEngineNativeAuditFieldsIncluded().equals(this.getActivityStreamEngineNativeAuditFieldsIncluded()) == false)
return false;
if (other.getAutomationMode() == null ^ this.getAutomationMode() == null)
return false;
if (other.getAutomationMode() != null && other.getAutomationMode().equals(this.getAutomationMode()) == false)
return false;
if (other.getResumeFullAutomationModeTime() == null ^ this.getResumeFullAutomationModeTime() == null)
return false;
if (other.getResumeFullAutomationModeTime() != null && other.getResumeFullAutomationModeTime().equals(this.getResumeFullAutomationModeTime()) == false)
return false;
if (other.getCustomIamInstanceProfile() == null ^ this.getCustomIamInstanceProfile() == null)
return false;
if (other.getCustomIamInstanceProfile() != null && other.getCustomIamInstanceProfile().equals(this.getCustomIamInstanceProfile()) == false)
return false;
if (other.getBackupTarget() == null ^ this.getBackupTarget() == null)
return false;
if (other.getBackupTarget() != null && other.getBackupTarget().equals(this.getBackupTarget()) == false)
return false;
if (other.getNetworkType() == null ^ this.getNetworkType() == null)
return false;
if (other.getNetworkType() != null && other.getNetworkType().equals(this.getNetworkType()) == false)
return false;
if (other.getActivityStreamPolicyStatus() == null ^ this.getActivityStreamPolicyStatus() == null)
return false;
if (other.getActivityStreamPolicyStatus() != null && other.getActivityStreamPolicyStatus().equals(this.getActivityStreamPolicyStatus()) == false)
return false;
if (other.getStorageThroughput() == null ^ this.getStorageThroughput() == null)
return false;
if (other.getStorageThroughput() != null && other.getStorageThroughput().equals(this.getStorageThroughput()) == false)
return false;
if (other.getDBSystemId() == null ^ this.getDBSystemId() == null)
return false;
if (other.getDBSystemId() != null && other.getDBSystemId().equals(this.getDBSystemId()) == false)
return false;
if (other.getMasterUserSecret() == null ^ this.getMasterUserSecret() == null)
return false;
if (other.getMasterUserSecret() != null && other.getMasterUserSecret().equals(this.getMasterUserSecret()) == false)
return false;
if (other.getCertificateDetails() == null ^ this.getCertificateDetails() == null)
return false;
if (other.getCertificateDetails() != null && other.getCertificateDetails().equals(this.getCertificateDetails()) == false)
return false;
if (other.getReadReplicaSourceDBClusterIdentifier() == null ^ this.getReadReplicaSourceDBClusterIdentifier() == null)
return false;
if (other.getReadReplicaSourceDBClusterIdentifier() != null
&& other.getReadReplicaSourceDBClusterIdentifier().equals(this.getReadReplicaSourceDBClusterIdentifier()) == false)
return false;
if (other.getPercentProgress() == null ^ this.getPercentProgress() == null)
return false;
if (other.getPercentProgress() != null && other.getPercentProgress().equals(this.getPercentProgress()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getDBInstanceIdentifier() == null) ? 0 : getDBInstanceIdentifier().hashCode());
hashCode = prime * hashCode + ((getDBInstanceClass() == null) ? 0 : getDBInstanceClass().hashCode());
hashCode = prime * hashCode + ((getEngine() == null) ? 0 : getEngine().hashCode());
hashCode = prime * hashCode + ((getDBInstanceStatus() == null) ? 0 : getDBInstanceStatus().hashCode());
hashCode = prime * hashCode + ((getAutomaticRestartTime() == null) ? 0 : getAutomaticRestartTime().hashCode());
hashCode = prime * hashCode + ((getMasterUsername() == null) ? 0 : getMasterUsername().hashCode());
hashCode = prime * hashCode + ((getDBName() == null) ? 0 : getDBName().hashCode());
hashCode = prime * hashCode + ((getEndpoint() == null) ? 0 : getEndpoint().hashCode());
hashCode = prime * hashCode + ((getAllocatedStorage() == null) ? 0 : getAllocatedStorage().hashCode());
hashCode = prime * hashCode + ((getInstanceCreateTime() == null) ? 0 : getInstanceCreateTime().hashCode());
hashCode = prime * hashCode + ((getPreferredBackupWindow() == null) ? 0 : getPreferredBackupWindow().hashCode());
hashCode = prime * hashCode + ((getBackupRetentionPeriod() == null) ? 0 : getBackupRetentionPeriod().hashCode());
hashCode = prime * hashCode + ((getDBSecurityGroups() == null) ? 0 : getDBSecurityGroups().hashCode());
hashCode = prime * hashCode + ((getVpcSecurityGroups() == null) ? 0 : getVpcSecurityGroups().hashCode());
hashCode = prime * hashCode + ((getDBParameterGroups() == null) ? 0 : getDBParameterGroups().hashCode());
hashCode = prime * hashCode + ((getAvailabilityZone() == null) ? 0 : getAvailabilityZone().hashCode());
hashCode = prime * hashCode + ((getDBSubnetGroup() == null) ? 0 : getDBSubnetGroup().hashCode());
hashCode = prime * hashCode + ((getPreferredMaintenanceWindow() == null) ? 0 : getPreferredMaintenanceWindow().hashCode());
hashCode = prime * hashCode + ((getPendingModifiedValues() == null) ? 0 : getPendingModifiedValues().hashCode());
hashCode = prime * hashCode + ((getLatestRestorableTime() == null) ? 0 : getLatestRestorableTime().hashCode());
hashCode = prime * hashCode + ((getMultiAZ() == null) ? 0 : getMultiAZ().hashCode());
hashCode = prime * hashCode + ((getEngineVersion() == null) ? 0 : getEngineVersion().hashCode());
hashCode = prime * hashCode + ((getAutoMinorVersionUpgrade() == null) ? 0 : getAutoMinorVersionUpgrade().hashCode());
hashCode = prime * hashCode + ((getReadReplicaSourceDBInstanceIdentifier() == null) ? 0 : getReadReplicaSourceDBInstanceIdentifier().hashCode());
hashCode = prime * hashCode + ((getReadReplicaDBInstanceIdentifiers() == null) ? 0 : getReadReplicaDBInstanceIdentifiers().hashCode());
hashCode = prime * hashCode + ((getReadReplicaDBClusterIdentifiers() == null) ? 0 : getReadReplicaDBClusterIdentifiers().hashCode());
hashCode = prime * hashCode + ((getReplicaMode() == null) ? 0 : getReplicaMode().hashCode());
hashCode = prime * hashCode + ((getLicenseModel() == null) ? 0 : getLicenseModel().hashCode());
hashCode = prime * hashCode + ((getIops() == null) ? 0 : getIops().hashCode());
hashCode = prime * hashCode + ((getOptionGroupMemberships() == null) ? 0 : getOptionGroupMemberships().hashCode());
hashCode = prime * hashCode + ((getCharacterSetName() == null) ? 0 : getCharacterSetName().hashCode());
hashCode = prime * hashCode + ((getNcharCharacterSetName() == null) ? 0 : getNcharCharacterSetName().hashCode());
hashCode = prime * hashCode + ((getSecondaryAvailabilityZone() == null) ? 0 : getSecondaryAvailabilityZone().hashCode());
hashCode = prime * hashCode + ((getPubliclyAccessible() == null) ? 0 : getPubliclyAccessible().hashCode());
hashCode = prime * hashCode + ((getStatusInfos() == null) ? 0 : getStatusInfos().hashCode());
hashCode = prime * hashCode + ((getStorageType() == null) ? 0 : getStorageType().hashCode());
hashCode = prime * hashCode + ((getTdeCredentialArn() == null) ? 0 : getTdeCredentialArn().hashCode());
hashCode = prime * hashCode + ((getDbInstancePort() == null) ? 0 : getDbInstancePort().hashCode());
hashCode = prime * hashCode + ((getDBClusterIdentifier() == null) ? 0 : getDBClusterIdentifier().hashCode());
hashCode = prime * hashCode + ((getStorageEncrypted() == null) ? 0 : getStorageEncrypted().hashCode());
hashCode = prime * hashCode + ((getKmsKeyId() == null) ? 0 : getKmsKeyId().hashCode());
hashCode = prime * hashCode + ((getDbiResourceId() == null) ? 0 : getDbiResourceId().hashCode());
hashCode = prime * hashCode + ((getCACertificateIdentifier() == null) ? 0 : getCACertificateIdentifier().hashCode());
hashCode = prime * hashCode + ((getDomainMemberships() == null) ? 0 : getDomainMemberships().hashCode());
hashCode = prime * hashCode + ((getCopyTagsToSnapshot() == null) ? 0 : getCopyTagsToSnapshot().hashCode());
hashCode = prime * hashCode + ((getMonitoringInterval() == null) ? 0 : getMonitoringInterval().hashCode());
hashCode = prime * hashCode + ((getEnhancedMonitoringResourceArn() == null) ? 0 : getEnhancedMonitoringResourceArn().hashCode());
hashCode = prime * hashCode + ((getMonitoringRoleArn() == null) ? 0 : getMonitoringRoleArn().hashCode());
hashCode = prime * hashCode + ((getPromotionTier() == null) ? 0 : getPromotionTier().hashCode());
hashCode = prime * hashCode + ((getDBInstanceArn() == null) ? 0 : getDBInstanceArn().hashCode());
hashCode = prime * hashCode + ((getTimezone() == null) ? 0 : getTimezone().hashCode());
hashCode = prime * hashCode + ((getIAMDatabaseAuthenticationEnabled() == null) ? 0 : getIAMDatabaseAuthenticationEnabled().hashCode());
hashCode = prime * hashCode + ((getPerformanceInsightsEnabled() == null) ? 0 : getPerformanceInsightsEnabled().hashCode());
hashCode = prime * hashCode + ((getPerformanceInsightsKMSKeyId() == null) ? 0 : getPerformanceInsightsKMSKeyId().hashCode());
hashCode = prime * hashCode + ((getPerformanceInsightsRetentionPeriod() == null) ? 0 : getPerformanceInsightsRetentionPeriod().hashCode());
hashCode = prime * hashCode + ((getEnabledCloudwatchLogsExports() == null) ? 0 : getEnabledCloudwatchLogsExports().hashCode());
hashCode = prime * hashCode + ((getProcessorFeatures() == null) ? 0 : getProcessorFeatures().hashCode());
hashCode = prime * hashCode + ((getDeletionProtection() == null) ? 0 : getDeletionProtection().hashCode());
hashCode = prime * hashCode + ((getAssociatedRoles() == null) ? 0 : getAssociatedRoles().hashCode());
hashCode = prime * hashCode + ((getListenerEndpoint() == null) ? 0 : getListenerEndpoint().hashCode());
hashCode = prime * hashCode + ((getMaxAllocatedStorage() == null) ? 0 : getMaxAllocatedStorage().hashCode());
hashCode = prime * hashCode + ((getTagList() == null) ? 0 : getTagList().hashCode());
hashCode = prime * hashCode + ((getDBInstanceAutomatedBackupsReplications() == null) ? 0 : getDBInstanceAutomatedBackupsReplications().hashCode());
hashCode = prime * hashCode + ((getCustomerOwnedIpEnabled() == null) ? 0 : getCustomerOwnedIpEnabled().hashCode());
hashCode = prime * hashCode + ((getAwsBackupRecoveryPointArn() == null) ? 0 : getAwsBackupRecoveryPointArn().hashCode());
hashCode = prime * hashCode + ((getActivityStreamStatus() == null) ? 0 : getActivityStreamStatus().hashCode());
hashCode = prime * hashCode + ((getActivityStreamKmsKeyId() == null) ? 0 : getActivityStreamKmsKeyId().hashCode());
hashCode = prime * hashCode + ((getActivityStreamKinesisStreamName() == null) ? 0 : getActivityStreamKinesisStreamName().hashCode());
hashCode = prime * hashCode + ((getActivityStreamMode() == null) ? 0 : getActivityStreamMode().hashCode());
hashCode = prime * hashCode
+ ((getActivityStreamEngineNativeAuditFieldsIncluded() == null) ? 0 : getActivityStreamEngineNativeAuditFieldsIncluded().hashCode());
hashCode = prime * hashCode + ((getAutomationMode() == null) ? 0 : getAutomationMode().hashCode());
hashCode = prime * hashCode + ((getResumeFullAutomationModeTime() == null) ? 0 : getResumeFullAutomationModeTime().hashCode());
hashCode = prime * hashCode + ((getCustomIamInstanceProfile() == null) ? 0 : getCustomIamInstanceProfile().hashCode());
hashCode = prime * hashCode + ((getBackupTarget() == null) ? 0 : getBackupTarget().hashCode());
hashCode = prime * hashCode + ((getNetworkType() == null) ? 0 : getNetworkType().hashCode());
hashCode = prime * hashCode + ((getActivityStreamPolicyStatus() == null) ? 0 : getActivityStreamPolicyStatus().hashCode());
hashCode = prime * hashCode + ((getStorageThroughput() == null) ? 0 : getStorageThroughput().hashCode());
hashCode = prime * hashCode + ((getDBSystemId() == null) ? 0 : getDBSystemId().hashCode());
hashCode = prime * hashCode + ((getMasterUserSecret() == null) ? 0 : getMasterUserSecret().hashCode());
hashCode = prime * hashCode + ((getCertificateDetails() == null) ? 0 : getCertificateDetails().hashCode());
hashCode = prime * hashCode + ((getReadReplicaSourceDBClusterIdentifier() == null) ? 0 : getReadReplicaSourceDBClusterIdentifier().hashCode());
hashCode = prime * hashCode + ((getPercentProgress() == null) ? 0 : getPercentProgress().hashCode());
return hashCode;
}
@Override
public DBInstance clone() {
try {
return (DBInstance) super.clone();
} catch (CloneNotSupportedException e) {
throw new IllegalStateException("Got a CloneNotSupportedException from Object.clone() " + "even though we're Cloneable!", e);
}
}
}