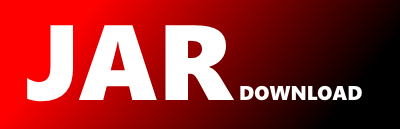
com.amazonaws.services.rds.model.DBRecommendation Maven / Gradle / Ivy
Show all versions of aws-java-sdk-rds Show documentation
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.rds.model;
import java.io.Serializable;
import javax.annotation.Generated;
/**
*
* The recommendation for your DB instances, DB clusters, and DB parameter groups.
*
*
* @see AWS API
* Documentation
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class DBRecommendation implements Serializable, Cloneable {
/**
*
* The unique identifier of the recommendation.
*
*/
private String recommendationId;
/**
*
* A value that indicates the type of recommendation. This value determines how the description is rendered.
*
*/
private String typeId;
/**
*
* The severity level of the recommendation. The severity level can help you decide the urgency with which to
* address the recommendation.
*
*
* Valid values:
*
*
* -
*
* high
*
*
* -
*
* medium
*
*
* -
*
* low
*
*
* -
*
* informational
*
*
*
*/
private String severity;
/**
*
* The Amazon Resource Name (ARN) of the RDS resource associated with the recommendation.
*
*/
private String resourceArn;
/**
*
* The current status of the recommendation.
*
*
* Valid values:
*
*
* -
*
* active
- The recommendations which are ready for you to apply.
*
*
* -
*
* pending
- The applied or scheduled recommendations which are in progress.
*
*
* -
*
* resolved
- The recommendations which are completed.
*
*
* -
*
* dismissed
- The recommendations that you dismissed.
*
*
*
*/
private String status;
/**
*
* The time when the recommendation was created. For example, 2023-09-28T01:13:53.931000+00:00
.
*
*/
private java.util.Date createdTime;
/**
*
* The time when the recommendation was last updated.
*
*/
private java.util.Date updatedTime;
/**
*
* A short description of the issue identified for this recommendation. The description might contain markdown.
*
*/
private String detection;
/**
*
* A short description of the recommendation to resolve an issue. The description might contain markdown.
*
*/
private String recommendation;
/**
*
* A detailed description of the recommendation. The description might contain markdown.
*
*/
private String description;
/**
*
* The reason why this recommendation was created. The information might contain markdown.
*
*/
private String reason;
/**
*
* A list of recommended actions.
*
*/
private com.amazonaws.internal.SdkInternalList recommendedActions;
/**
*
* The category of the recommendation.
*
*
* Valid values:
*
*
* -
*
* performance efficiency
*
*
* -
*
* security
*
*
* -
*
* reliability
*
*
* -
*
* cost optimization
*
*
* -
*
* operational excellence
*
*
* -
*
* sustainability
*
*
*
*/
private String category;
/**
*
* The Amazon Web Services service that generated the recommendations.
*
*/
private String source;
/**
*
* A short description of the recommendation type. The description might contain markdown.
*
*/
private String typeDetection;
/**
*
* A short description that summarizes the recommendation to fix all the issues of the recommendation type. The
* description might contain markdown.
*
*/
private String typeRecommendation;
/**
*
* A short description that explains the possible impact of an issue.
*
*/
private String impact;
/**
*
* Additional information about the recommendation. The information might contain markdown.
*
*/
private String additionalInfo;
/**
*
* A link to documentation that provides additional information about the recommendation.
*
*/
private com.amazonaws.internal.SdkInternalList links;
/**
*
* Details of the issue that caused the recommendation.
*
*/
private IssueDetails issueDetails;
/**
*
* The unique identifier of the recommendation.
*
*
* @param recommendationId
* The unique identifier of the recommendation.
*/
public void setRecommendationId(String recommendationId) {
this.recommendationId = recommendationId;
}
/**
*
* The unique identifier of the recommendation.
*
*
* @return The unique identifier of the recommendation.
*/
public String getRecommendationId() {
return this.recommendationId;
}
/**
*
* The unique identifier of the recommendation.
*
*
* @param recommendationId
* The unique identifier of the recommendation.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DBRecommendation withRecommendationId(String recommendationId) {
setRecommendationId(recommendationId);
return this;
}
/**
*
* A value that indicates the type of recommendation. This value determines how the description is rendered.
*
*
* @param typeId
* A value that indicates the type of recommendation. This value determines how the description is rendered.
*/
public void setTypeId(String typeId) {
this.typeId = typeId;
}
/**
*
* A value that indicates the type of recommendation. This value determines how the description is rendered.
*
*
* @return A value that indicates the type of recommendation. This value determines how the description is rendered.
*/
public String getTypeId() {
return this.typeId;
}
/**
*
* A value that indicates the type of recommendation. This value determines how the description is rendered.
*
*
* @param typeId
* A value that indicates the type of recommendation. This value determines how the description is rendered.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DBRecommendation withTypeId(String typeId) {
setTypeId(typeId);
return this;
}
/**
*
* The severity level of the recommendation. The severity level can help you decide the urgency with which to
* address the recommendation.
*
*
* Valid values:
*
*
* -
*
* high
*
*
* -
*
* medium
*
*
* -
*
* low
*
*
* -
*
* informational
*
*
*
*
* @param severity
* The severity level of the recommendation. The severity level can help you decide the urgency with which to
* address the recommendation.
*
* Valid values:
*
*
* -
*
* high
*
*
* -
*
* medium
*
*
* -
*
* low
*
*
* -
*
* informational
*
*
*/
public void setSeverity(String severity) {
this.severity = severity;
}
/**
*
* The severity level of the recommendation. The severity level can help you decide the urgency with which to
* address the recommendation.
*
*
* Valid values:
*
*
* -
*
* high
*
*
* -
*
* medium
*
*
* -
*
* low
*
*
* -
*
* informational
*
*
*
*
* @return The severity level of the recommendation. The severity level can help you decide the urgency with which
* to address the recommendation.
*
* Valid values:
*
*
* -
*
* high
*
*
* -
*
* medium
*
*
* -
*
* low
*
*
* -
*
* informational
*
*
*/
public String getSeverity() {
return this.severity;
}
/**
*
* The severity level of the recommendation. The severity level can help you decide the urgency with which to
* address the recommendation.
*
*
* Valid values:
*
*
* -
*
* high
*
*
* -
*
* medium
*
*
* -
*
* low
*
*
* -
*
* informational
*
*
*
*
* @param severity
* The severity level of the recommendation. The severity level can help you decide the urgency with which to
* address the recommendation.
*
* Valid values:
*
*
* -
*
* high
*
*
* -
*
* medium
*
*
* -
*
* low
*
*
* -
*
* informational
*
*
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DBRecommendation withSeverity(String severity) {
setSeverity(severity);
return this;
}
/**
*
* The Amazon Resource Name (ARN) of the RDS resource associated with the recommendation.
*
*
* @param resourceArn
* The Amazon Resource Name (ARN) of the RDS resource associated with the recommendation.
*/
public void setResourceArn(String resourceArn) {
this.resourceArn = resourceArn;
}
/**
*
* The Amazon Resource Name (ARN) of the RDS resource associated with the recommendation.
*
*
* @return The Amazon Resource Name (ARN) of the RDS resource associated with the recommendation.
*/
public String getResourceArn() {
return this.resourceArn;
}
/**
*
* The Amazon Resource Name (ARN) of the RDS resource associated with the recommendation.
*
*
* @param resourceArn
* The Amazon Resource Name (ARN) of the RDS resource associated with the recommendation.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DBRecommendation withResourceArn(String resourceArn) {
setResourceArn(resourceArn);
return this;
}
/**
*
* The current status of the recommendation.
*
*
* Valid values:
*
*
* -
*
* active
- The recommendations which are ready for you to apply.
*
*
* -
*
* pending
- The applied or scheduled recommendations which are in progress.
*
*
* -
*
* resolved
- The recommendations which are completed.
*
*
* -
*
* dismissed
- The recommendations that you dismissed.
*
*
*
*
* @param status
* The current status of the recommendation.
*
* Valid values:
*
*
* -
*
* active
- The recommendations which are ready for you to apply.
*
*
* -
*
* pending
- The applied or scheduled recommendations which are in progress.
*
*
* -
*
* resolved
- The recommendations which are completed.
*
*
* -
*
* dismissed
- The recommendations that you dismissed.
*
*
*/
public void setStatus(String status) {
this.status = status;
}
/**
*
* The current status of the recommendation.
*
*
* Valid values:
*
*
* -
*
* active
- The recommendations which are ready for you to apply.
*
*
* -
*
* pending
- The applied or scheduled recommendations which are in progress.
*
*
* -
*
* resolved
- The recommendations which are completed.
*
*
* -
*
* dismissed
- The recommendations that you dismissed.
*
*
*
*
* @return The current status of the recommendation.
*
* Valid values:
*
*
* -
*
* active
- The recommendations which are ready for you to apply.
*
*
* -
*
* pending
- The applied or scheduled recommendations which are in progress.
*
*
* -
*
* resolved
- The recommendations which are completed.
*
*
* -
*
* dismissed
- The recommendations that you dismissed.
*
*
*/
public String getStatus() {
return this.status;
}
/**
*
* The current status of the recommendation.
*
*
* Valid values:
*
*
* -
*
* active
- The recommendations which are ready for you to apply.
*
*
* -
*
* pending
- The applied or scheduled recommendations which are in progress.
*
*
* -
*
* resolved
- The recommendations which are completed.
*
*
* -
*
* dismissed
- The recommendations that you dismissed.
*
*
*
*
* @param status
* The current status of the recommendation.
*
* Valid values:
*
*
* -
*
* active
- The recommendations which are ready for you to apply.
*
*
* -
*
* pending
- The applied or scheduled recommendations which are in progress.
*
*
* -
*
* resolved
- The recommendations which are completed.
*
*
* -
*
* dismissed
- The recommendations that you dismissed.
*
*
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DBRecommendation withStatus(String status) {
setStatus(status);
return this;
}
/**
*
* The time when the recommendation was created. For example, 2023-09-28T01:13:53.931000+00:00
.
*
*
* @param createdTime
* The time when the recommendation was created. For example, 2023-09-28T01:13:53.931000+00:00
.
*/
public void setCreatedTime(java.util.Date createdTime) {
this.createdTime = createdTime;
}
/**
*
* The time when the recommendation was created. For example, 2023-09-28T01:13:53.931000+00:00
.
*
*
* @return The time when the recommendation was created. For example, 2023-09-28T01:13:53.931000+00:00
.
*/
public java.util.Date getCreatedTime() {
return this.createdTime;
}
/**
*
* The time when the recommendation was created. For example, 2023-09-28T01:13:53.931000+00:00
.
*
*
* @param createdTime
* The time when the recommendation was created. For example, 2023-09-28T01:13:53.931000+00:00
.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DBRecommendation withCreatedTime(java.util.Date createdTime) {
setCreatedTime(createdTime);
return this;
}
/**
*
* The time when the recommendation was last updated.
*
*
* @param updatedTime
* The time when the recommendation was last updated.
*/
public void setUpdatedTime(java.util.Date updatedTime) {
this.updatedTime = updatedTime;
}
/**
*
* The time when the recommendation was last updated.
*
*
* @return The time when the recommendation was last updated.
*/
public java.util.Date getUpdatedTime() {
return this.updatedTime;
}
/**
*
* The time when the recommendation was last updated.
*
*
* @param updatedTime
* The time when the recommendation was last updated.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DBRecommendation withUpdatedTime(java.util.Date updatedTime) {
setUpdatedTime(updatedTime);
return this;
}
/**
*
* A short description of the issue identified for this recommendation. The description might contain markdown.
*
*
* @param detection
* A short description of the issue identified for this recommendation. The description might contain
* markdown.
*/
public void setDetection(String detection) {
this.detection = detection;
}
/**
*
* A short description of the issue identified for this recommendation. The description might contain markdown.
*
*
* @return A short description of the issue identified for this recommendation. The description might contain
* markdown.
*/
public String getDetection() {
return this.detection;
}
/**
*
* A short description of the issue identified for this recommendation. The description might contain markdown.
*
*
* @param detection
* A short description of the issue identified for this recommendation. The description might contain
* markdown.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DBRecommendation withDetection(String detection) {
setDetection(detection);
return this;
}
/**
*
* A short description of the recommendation to resolve an issue. The description might contain markdown.
*
*
* @param recommendation
* A short description of the recommendation to resolve an issue. The description might contain markdown.
*/
public void setRecommendation(String recommendation) {
this.recommendation = recommendation;
}
/**
*
* A short description of the recommendation to resolve an issue. The description might contain markdown.
*
*
* @return A short description of the recommendation to resolve an issue. The description might contain markdown.
*/
public String getRecommendation() {
return this.recommendation;
}
/**
*
* A short description of the recommendation to resolve an issue. The description might contain markdown.
*
*
* @param recommendation
* A short description of the recommendation to resolve an issue. The description might contain markdown.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DBRecommendation withRecommendation(String recommendation) {
setRecommendation(recommendation);
return this;
}
/**
*
* A detailed description of the recommendation. The description might contain markdown.
*
*
* @param description
* A detailed description of the recommendation. The description might contain markdown.
*/
public void setDescription(String description) {
this.description = description;
}
/**
*
* A detailed description of the recommendation. The description might contain markdown.
*
*
* @return A detailed description of the recommendation. The description might contain markdown.
*/
public String getDescription() {
return this.description;
}
/**
*
* A detailed description of the recommendation. The description might contain markdown.
*
*
* @param description
* A detailed description of the recommendation. The description might contain markdown.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DBRecommendation withDescription(String description) {
setDescription(description);
return this;
}
/**
*
* The reason why this recommendation was created. The information might contain markdown.
*
*
* @param reason
* The reason why this recommendation was created. The information might contain markdown.
*/
public void setReason(String reason) {
this.reason = reason;
}
/**
*
* The reason why this recommendation was created. The information might contain markdown.
*
*
* @return The reason why this recommendation was created. The information might contain markdown.
*/
public String getReason() {
return this.reason;
}
/**
*
* The reason why this recommendation was created. The information might contain markdown.
*
*
* @param reason
* The reason why this recommendation was created. The information might contain markdown.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DBRecommendation withReason(String reason) {
setReason(reason);
return this;
}
/**
*
* A list of recommended actions.
*
*
* @return A list of recommended actions.
*/
public java.util.List getRecommendedActions() {
if (recommendedActions == null) {
recommendedActions = new com.amazonaws.internal.SdkInternalList();
}
return recommendedActions;
}
/**
*
* A list of recommended actions.
*
*
* @param recommendedActions
* A list of recommended actions.
*/
public void setRecommendedActions(java.util.Collection recommendedActions) {
if (recommendedActions == null) {
this.recommendedActions = null;
return;
}
this.recommendedActions = new com.amazonaws.internal.SdkInternalList(recommendedActions);
}
/**
*
* A list of recommended actions.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setRecommendedActions(java.util.Collection)} or {@link #withRecommendedActions(java.util.Collection)} if
* you want to override the existing values.
*
*
* @param recommendedActions
* A list of recommended actions.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DBRecommendation withRecommendedActions(RecommendedAction... recommendedActions) {
if (this.recommendedActions == null) {
setRecommendedActions(new com.amazonaws.internal.SdkInternalList(recommendedActions.length));
}
for (RecommendedAction ele : recommendedActions) {
this.recommendedActions.add(ele);
}
return this;
}
/**
*
* A list of recommended actions.
*
*
* @param recommendedActions
* A list of recommended actions.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DBRecommendation withRecommendedActions(java.util.Collection recommendedActions) {
setRecommendedActions(recommendedActions);
return this;
}
/**
*
* The category of the recommendation.
*
*
* Valid values:
*
*
* -
*
* performance efficiency
*
*
* -
*
* security
*
*
* -
*
* reliability
*
*
* -
*
* cost optimization
*
*
* -
*
* operational excellence
*
*
* -
*
* sustainability
*
*
*
*
* @param category
* The category of the recommendation.
*
* Valid values:
*
*
* -
*
* performance efficiency
*
*
* -
*
* security
*
*
* -
*
* reliability
*
*
* -
*
* cost optimization
*
*
* -
*
* operational excellence
*
*
* -
*
* sustainability
*
*
*/
public void setCategory(String category) {
this.category = category;
}
/**
*
* The category of the recommendation.
*
*
* Valid values:
*
*
* -
*
* performance efficiency
*
*
* -
*
* security
*
*
* -
*
* reliability
*
*
* -
*
* cost optimization
*
*
* -
*
* operational excellence
*
*
* -
*
* sustainability
*
*
*
*
* @return The category of the recommendation.
*
* Valid values:
*
*
* -
*
* performance efficiency
*
*
* -
*
* security
*
*
* -
*
* reliability
*
*
* -
*
* cost optimization
*
*
* -
*
* operational excellence
*
*
* -
*
* sustainability
*
*
*/
public String getCategory() {
return this.category;
}
/**
*
* The category of the recommendation.
*
*
* Valid values:
*
*
* -
*
* performance efficiency
*
*
* -
*
* security
*
*
* -
*
* reliability
*
*
* -
*
* cost optimization
*
*
* -
*
* operational excellence
*
*
* -
*
* sustainability
*
*
*
*
* @param category
* The category of the recommendation.
*
* Valid values:
*
*
* -
*
* performance efficiency
*
*
* -
*
* security
*
*
* -
*
* reliability
*
*
* -
*
* cost optimization
*
*
* -
*
* operational excellence
*
*
* -
*
* sustainability
*
*
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DBRecommendation withCategory(String category) {
setCategory(category);
return this;
}
/**
*
* The Amazon Web Services service that generated the recommendations.
*
*
* @param source
* The Amazon Web Services service that generated the recommendations.
*/
public void setSource(String source) {
this.source = source;
}
/**
*
* The Amazon Web Services service that generated the recommendations.
*
*
* @return The Amazon Web Services service that generated the recommendations.
*/
public String getSource() {
return this.source;
}
/**
*
* The Amazon Web Services service that generated the recommendations.
*
*
* @param source
* The Amazon Web Services service that generated the recommendations.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DBRecommendation withSource(String source) {
setSource(source);
return this;
}
/**
*
* A short description of the recommendation type. The description might contain markdown.
*
*
* @param typeDetection
* A short description of the recommendation type. The description might contain markdown.
*/
public void setTypeDetection(String typeDetection) {
this.typeDetection = typeDetection;
}
/**
*
* A short description of the recommendation type. The description might contain markdown.
*
*
* @return A short description of the recommendation type. The description might contain markdown.
*/
public String getTypeDetection() {
return this.typeDetection;
}
/**
*
* A short description of the recommendation type. The description might contain markdown.
*
*
* @param typeDetection
* A short description of the recommendation type. The description might contain markdown.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DBRecommendation withTypeDetection(String typeDetection) {
setTypeDetection(typeDetection);
return this;
}
/**
*
* A short description that summarizes the recommendation to fix all the issues of the recommendation type. The
* description might contain markdown.
*
*
* @param typeRecommendation
* A short description that summarizes the recommendation to fix all the issues of the recommendation type.
* The description might contain markdown.
*/
public void setTypeRecommendation(String typeRecommendation) {
this.typeRecommendation = typeRecommendation;
}
/**
*
* A short description that summarizes the recommendation to fix all the issues of the recommendation type. The
* description might contain markdown.
*
*
* @return A short description that summarizes the recommendation to fix all the issues of the recommendation type.
* The description might contain markdown.
*/
public String getTypeRecommendation() {
return this.typeRecommendation;
}
/**
*
* A short description that summarizes the recommendation to fix all the issues of the recommendation type. The
* description might contain markdown.
*
*
* @param typeRecommendation
* A short description that summarizes the recommendation to fix all the issues of the recommendation type.
* The description might contain markdown.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DBRecommendation withTypeRecommendation(String typeRecommendation) {
setTypeRecommendation(typeRecommendation);
return this;
}
/**
*
* A short description that explains the possible impact of an issue.
*
*
* @param impact
* A short description that explains the possible impact of an issue.
*/
public void setImpact(String impact) {
this.impact = impact;
}
/**
*
* A short description that explains the possible impact of an issue.
*
*
* @return A short description that explains the possible impact of an issue.
*/
public String getImpact() {
return this.impact;
}
/**
*
* A short description that explains the possible impact of an issue.
*
*
* @param impact
* A short description that explains the possible impact of an issue.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DBRecommendation withImpact(String impact) {
setImpact(impact);
return this;
}
/**
*
* Additional information about the recommendation. The information might contain markdown.
*
*
* @param additionalInfo
* Additional information about the recommendation. The information might contain markdown.
*/
public void setAdditionalInfo(String additionalInfo) {
this.additionalInfo = additionalInfo;
}
/**
*
* Additional information about the recommendation. The information might contain markdown.
*
*
* @return Additional information about the recommendation. The information might contain markdown.
*/
public String getAdditionalInfo() {
return this.additionalInfo;
}
/**
*
* Additional information about the recommendation. The information might contain markdown.
*
*
* @param additionalInfo
* Additional information about the recommendation. The information might contain markdown.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DBRecommendation withAdditionalInfo(String additionalInfo) {
setAdditionalInfo(additionalInfo);
return this;
}
/**
*
* A link to documentation that provides additional information about the recommendation.
*
*
* @return A link to documentation that provides additional information about the recommendation.
*/
public java.util.List getLinks() {
if (links == null) {
links = new com.amazonaws.internal.SdkInternalList();
}
return links;
}
/**
*
* A link to documentation that provides additional information about the recommendation.
*
*
* @param links
* A link to documentation that provides additional information about the recommendation.
*/
public void setLinks(java.util.Collection links) {
if (links == null) {
this.links = null;
return;
}
this.links = new com.amazonaws.internal.SdkInternalList(links);
}
/**
*
* A link to documentation that provides additional information about the recommendation.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setLinks(java.util.Collection)} or {@link #withLinks(java.util.Collection)} if you want to override the
* existing values.
*
*
* @param links
* A link to documentation that provides additional information about the recommendation.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DBRecommendation withLinks(DocLink... links) {
if (this.links == null) {
setLinks(new com.amazonaws.internal.SdkInternalList(links.length));
}
for (DocLink ele : links) {
this.links.add(ele);
}
return this;
}
/**
*
* A link to documentation that provides additional information about the recommendation.
*
*
* @param links
* A link to documentation that provides additional information about the recommendation.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DBRecommendation withLinks(java.util.Collection links) {
setLinks(links);
return this;
}
/**
*
* Details of the issue that caused the recommendation.
*
*
* @param issueDetails
* Details of the issue that caused the recommendation.
*/
public void setIssueDetails(IssueDetails issueDetails) {
this.issueDetails = issueDetails;
}
/**
*
* Details of the issue that caused the recommendation.
*
*
* @return Details of the issue that caused the recommendation.
*/
public IssueDetails getIssueDetails() {
return this.issueDetails;
}
/**
*
* Details of the issue that caused the recommendation.
*
*
* @param issueDetails
* Details of the issue that caused the recommendation.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DBRecommendation withIssueDetails(IssueDetails issueDetails) {
setIssueDetails(issueDetails);
return this;
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getRecommendationId() != null)
sb.append("RecommendationId: ").append(getRecommendationId()).append(",");
if (getTypeId() != null)
sb.append("TypeId: ").append(getTypeId()).append(",");
if (getSeverity() != null)
sb.append("Severity: ").append(getSeverity()).append(",");
if (getResourceArn() != null)
sb.append("ResourceArn: ").append(getResourceArn()).append(",");
if (getStatus() != null)
sb.append("Status: ").append(getStatus()).append(",");
if (getCreatedTime() != null)
sb.append("CreatedTime: ").append(getCreatedTime()).append(",");
if (getUpdatedTime() != null)
sb.append("UpdatedTime: ").append(getUpdatedTime()).append(",");
if (getDetection() != null)
sb.append("Detection: ").append(getDetection()).append(",");
if (getRecommendation() != null)
sb.append("Recommendation: ").append(getRecommendation()).append(",");
if (getDescription() != null)
sb.append("Description: ").append(getDescription()).append(",");
if (getReason() != null)
sb.append("Reason: ").append(getReason()).append(",");
if (getRecommendedActions() != null)
sb.append("RecommendedActions: ").append(getRecommendedActions()).append(",");
if (getCategory() != null)
sb.append("Category: ").append(getCategory()).append(",");
if (getSource() != null)
sb.append("Source: ").append(getSource()).append(",");
if (getTypeDetection() != null)
sb.append("TypeDetection: ").append(getTypeDetection()).append(",");
if (getTypeRecommendation() != null)
sb.append("TypeRecommendation: ").append(getTypeRecommendation()).append(",");
if (getImpact() != null)
sb.append("Impact: ").append(getImpact()).append(",");
if (getAdditionalInfo() != null)
sb.append("AdditionalInfo: ").append(getAdditionalInfo()).append(",");
if (getLinks() != null)
sb.append("Links: ").append(getLinks()).append(",");
if (getIssueDetails() != null)
sb.append("IssueDetails: ").append(getIssueDetails());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof DBRecommendation == false)
return false;
DBRecommendation other = (DBRecommendation) obj;
if (other.getRecommendationId() == null ^ this.getRecommendationId() == null)
return false;
if (other.getRecommendationId() != null && other.getRecommendationId().equals(this.getRecommendationId()) == false)
return false;
if (other.getTypeId() == null ^ this.getTypeId() == null)
return false;
if (other.getTypeId() != null && other.getTypeId().equals(this.getTypeId()) == false)
return false;
if (other.getSeverity() == null ^ this.getSeverity() == null)
return false;
if (other.getSeverity() != null && other.getSeverity().equals(this.getSeverity()) == false)
return false;
if (other.getResourceArn() == null ^ this.getResourceArn() == null)
return false;
if (other.getResourceArn() != null && other.getResourceArn().equals(this.getResourceArn()) == false)
return false;
if (other.getStatus() == null ^ this.getStatus() == null)
return false;
if (other.getStatus() != null && other.getStatus().equals(this.getStatus()) == false)
return false;
if (other.getCreatedTime() == null ^ this.getCreatedTime() == null)
return false;
if (other.getCreatedTime() != null && other.getCreatedTime().equals(this.getCreatedTime()) == false)
return false;
if (other.getUpdatedTime() == null ^ this.getUpdatedTime() == null)
return false;
if (other.getUpdatedTime() != null && other.getUpdatedTime().equals(this.getUpdatedTime()) == false)
return false;
if (other.getDetection() == null ^ this.getDetection() == null)
return false;
if (other.getDetection() != null && other.getDetection().equals(this.getDetection()) == false)
return false;
if (other.getRecommendation() == null ^ this.getRecommendation() == null)
return false;
if (other.getRecommendation() != null && other.getRecommendation().equals(this.getRecommendation()) == false)
return false;
if (other.getDescription() == null ^ this.getDescription() == null)
return false;
if (other.getDescription() != null && other.getDescription().equals(this.getDescription()) == false)
return false;
if (other.getReason() == null ^ this.getReason() == null)
return false;
if (other.getReason() != null && other.getReason().equals(this.getReason()) == false)
return false;
if (other.getRecommendedActions() == null ^ this.getRecommendedActions() == null)
return false;
if (other.getRecommendedActions() != null && other.getRecommendedActions().equals(this.getRecommendedActions()) == false)
return false;
if (other.getCategory() == null ^ this.getCategory() == null)
return false;
if (other.getCategory() != null && other.getCategory().equals(this.getCategory()) == false)
return false;
if (other.getSource() == null ^ this.getSource() == null)
return false;
if (other.getSource() != null && other.getSource().equals(this.getSource()) == false)
return false;
if (other.getTypeDetection() == null ^ this.getTypeDetection() == null)
return false;
if (other.getTypeDetection() != null && other.getTypeDetection().equals(this.getTypeDetection()) == false)
return false;
if (other.getTypeRecommendation() == null ^ this.getTypeRecommendation() == null)
return false;
if (other.getTypeRecommendation() != null && other.getTypeRecommendation().equals(this.getTypeRecommendation()) == false)
return false;
if (other.getImpact() == null ^ this.getImpact() == null)
return false;
if (other.getImpact() != null && other.getImpact().equals(this.getImpact()) == false)
return false;
if (other.getAdditionalInfo() == null ^ this.getAdditionalInfo() == null)
return false;
if (other.getAdditionalInfo() != null && other.getAdditionalInfo().equals(this.getAdditionalInfo()) == false)
return false;
if (other.getLinks() == null ^ this.getLinks() == null)
return false;
if (other.getLinks() != null && other.getLinks().equals(this.getLinks()) == false)
return false;
if (other.getIssueDetails() == null ^ this.getIssueDetails() == null)
return false;
if (other.getIssueDetails() != null && other.getIssueDetails().equals(this.getIssueDetails()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getRecommendationId() == null) ? 0 : getRecommendationId().hashCode());
hashCode = prime * hashCode + ((getTypeId() == null) ? 0 : getTypeId().hashCode());
hashCode = prime * hashCode + ((getSeverity() == null) ? 0 : getSeverity().hashCode());
hashCode = prime * hashCode + ((getResourceArn() == null) ? 0 : getResourceArn().hashCode());
hashCode = prime * hashCode + ((getStatus() == null) ? 0 : getStatus().hashCode());
hashCode = prime * hashCode + ((getCreatedTime() == null) ? 0 : getCreatedTime().hashCode());
hashCode = prime * hashCode + ((getUpdatedTime() == null) ? 0 : getUpdatedTime().hashCode());
hashCode = prime * hashCode + ((getDetection() == null) ? 0 : getDetection().hashCode());
hashCode = prime * hashCode + ((getRecommendation() == null) ? 0 : getRecommendation().hashCode());
hashCode = prime * hashCode + ((getDescription() == null) ? 0 : getDescription().hashCode());
hashCode = prime * hashCode + ((getReason() == null) ? 0 : getReason().hashCode());
hashCode = prime * hashCode + ((getRecommendedActions() == null) ? 0 : getRecommendedActions().hashCode());
hashCode = prime * hashCode + ((getCategory() == null) ? 0 : getCategory().hashCode());
hashCode = prime * hashCode + ((getSource() == null) ? 0 : getSource().hashCode());
hashCode = prime * hashCode + ((getTypeDetection() == null) ? 0 : getTypeDetection().hashCode());
hashCode = prime * hashCode + ((getTypeRecommendation() == null) ? 0 : getTypeRecommendation().hashCode());
hashCode = prime * hashCode + ((getImpact() == null) ? 0 : getImpact().hashCode());
hashCode = prime * hashCode + ((getAdditionalInfo() == null) ? 0 : getAdditionalInfo().hashCode());
hashCode = prime * hashCode + ((getLinks() == null) ? 0 : getLinks().hashCode());
hashCode = prime * hashCode + ((getIssueDetails() == null) ? 0 : getIssueDetails().hashCode());
return hashCode;
}
@Override
public DBRecommendation clone() {
try {
return (DBRecommendation) super.clone();
} catch (CloneNotSupportedException e) {
throw new IllegalStateException("Got a CloneNotSupportedException from Object.clone() " + "even though we're Cloneable!", e);
}
}
}