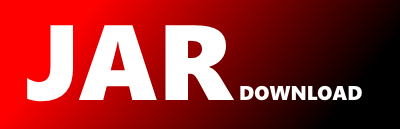
com.amazonaws.services.rds.model.CreateBlueGreenDeploymentRequest Maven / Gradle / Ivy
Show all versions of aws-java-sdk-rds Show documentation
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.rds.model;
import java.io.Serializable;
import javax.annotation.Generated;
import com.amazonaws.AmazonWebServiceRequest;
/**
*
* @see AWS API
* Documentation
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class CreateBlueGreenDeploymentRequest extends com.amazonaws.AmazonWebServiceRequest implements Serializable, Cloneable {
/**
*
* The name of the blue/green deployment.
*
*
* Constraints:
*
*
* -
*
* Can't be the same as an existing blue/green deployment name in the same account and Amazon Web Services Region.
*
*
*
*/
private String blueGreenDeploymentName;
/**
*
* The Amazon Resource Name (ARN) of the source production database.
*
*
* Specify the database that you want to clone. The blue/green deployment creates this database in the green
* environment. You can make updates to the database in the green environment, such as an engine version upgrade.
* When you are ready, you can switch the database in the green environment to be the production database.
*
*/
private String source;
/**
*
* The engine version of the database in the green environment.
*
*
* Specify the engine version to upgrade to in the green environment.
*
*/
private String targetEngineVersion;
/**
*
* The DB parameter group associated with the DB instance in the green environment.
*
*
* To test parameter changes, specify a DB parameter group that is different from the one associated with the source
* DB instance.
*
*/
private String targetDBParameterGroupName;
/**
*
* The DB cluster parameter group associated with the Aurora DB cluster in the green environment.
*
*
* To test parameter changes, specify a DB cluster parameter group that is different from the one associated with
* the source DB cluster.
*
*/
private String targetDBClusterParameterGroupName;
/**
*
* Tags to assign to the blue/green deployment.
*
*/
private com.amazonaws.internal.SdkInternalList tags;
/**
*
* Specify the DB instance class for the databases in the green environment.
*
*
* This parameter only applies to RDS DB instances, because DB instances within an Aurora DB cluster can have
* multiple different instance classes. If you're creating a blue/green deployment from an Aurora DB cluster, don't
* specify this parameter. After the green environment is created, you can individually modify the instance classes
* of the DB instances within the green DB cluster.
*
*/
private String targetDBInstanceClass;
/**
*
* Whether to upgrade the storage file system configuration on the green database. This option migrates the green DB
* instance from the older 32-bit file system to the preferred configuration. For more information, see Upgrading the storage file system for a DB instance.
*
*/
private Boolean upgradeTargetStorageConfig;
/**
*
* The name of the blue/green deployment.
*
*
* Constraints:
*
*
* -
*
* Can't be the same as an existing blue/green deployment name in the same account and Amazon Web Services Region.
*
*
*
*
* @param blueGreenDeploymentName
* The name of the blue/green deployment.
*
* Constraints:
*
*
* -
*
* Can't be the same as an existing blue/green deployment name in the same account and Amazon Web Services
* Region.
*
*
*/
public void setBlueGreenDeploymentName(String blueGreenDeploymentName) {
this.blueGreenDeploymentName = blueGreenDeploymentName;
}
/**
*
* The name of the blue/green deployment.
*
*
* Constraints:
*
*
* -
*
* Can't be the same as an existing blue/green deployment name in the same account and Amazon Web Services Region.
*
*
*
*
* @return The name of the blue/green deployment.
*
* Constraints:
*
*
* -
*
* Can't be the same as an existing blue/green deployment name in the same account and Amazon Web Services
* Region.
*
*
*/
public String getBlueGreenDeploymentName() {
return this.blueGreenDeploymentName;
}
/**
*
* The name of the blue/green deployment.
*
*
* Constraints:
*
*
* -
*
* Can't be the same as an existing blue/green deployment name in the same account and Amazon Web Services Region.
*
*
*
*
* @param blueGreenDeploymentName
* The name of the blue/green deployment.
*
* Constraints:
*
*
* -
*
* Can't be the same as an existing blue/green deployment name in the same account and Amazon Web Services
* Region.
*
*
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateBlueGreenDeploymentRequest withBlueGreenDeploymentName(String blueGreenDeploymentName) {
setBlueGreenDeploymentName(blueGreenDeploymentName);
return this;
}
/**
*
* The Amazon Resource Name (ARN) of the source production database.
*
*
* Specify the database that you want to clone. The blue/green deployment creates this database in the green
* environment. You can make updates to the database in the green environment, such as an engine version upgrade.
* When you are ready, you can switch the database in the green environment to be the production database.
*
*
* @param source
* The Amazon Resource Name (ARN) of the source production database.
*
* Specify the database that you want to clone. The blue/green deployment creates this database in the green
* environment. You can make updates to the database in the green environment, such as an engine version
* upgrade. When you are ready, you can switch the database in the green environment to be the production
* database.
*/
public void setSource(String source) {
this.source = source;
}
/**
*
* The Amazon Resource Name (ARN) of the source production database.
*
*
* Specify the database that you want to clone. The blue/green deployment creates this database in the green
* environment. You can make updates to the database in the green environment, such as an engine version upgrade.
* When you are ready, you can switch the database in the green environment to be the production database.
*
*
* @return The Amazon Resource Name (ARN) of the source production database.
*
* Specify the database that you want to clone. The blue/green deployment creates this database in the green
* environment. You can make updates to the database in the green environment, such as an engine version
* upgrade. When you are ready, you can switch the database in the green environment to be the production
* database.
*/
public String getSource() {
return this.source;
}
/**
*
* The Amazon Resource Name (ARN) of the source production database.
*
*
* Specify the database that you want to clone. The blue/green deployment creates this database in the green
* environment. You can make updates to the database in the green environment, such as an engine version upgrade.
* When you are ready, you can switch the database in the green environment to be the production database.
*
*
* @param source
* The Amazon Resource Name (ARN) of the source production database.
*
* Specify the database that you want to clone. The blue/green deployment creates this database in the green
* environment. You can make updates to the database in the green environment, such as an engine version
* upgrade. When you are ready, you can switch the database in the green environment to be the production
* database.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateBlueGreenDeploymentRequest withSource(String source) {
setSource(source);
return this;
}
/**
*
* The engine version of the database in the green environment.
*
*
* Specify the engine version to upgrade to in the green environment.
*
*
* @param targetEngineVersion
* The engine version of the database in the green environment.
*
* Specify the engine version to upgrade to in the green environment.
*/
public void setTargetEngineVersion(String targetEngineVersion) {
this.targetEngineVersion = targetEngineVersion;
}
/**
*
* The engine version of the database in the green environment.
*
*
* Specify the engine version to upgrade to in the green environment.
*
*
* @return The engine version of the database in the green environment.
*
* Specify the engine version to upgrade to in the green environment.
*/
public String getTargetEngineVersion() {
return this.targetEngineVersion;
}
/**
*
* The engine version of the database in the green environment.
*
*
* Specify the engine version to upgrade to in the green environment.
*
*
* @param targetEngineVersion
* The engine version of the database in the green environment.
*
* Specify the engine version to upgrade to in the green environment.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateBlueGreenDeploymentRequest withTargetEngineVersion(String targetEngineVersion) {
setTargetEngineVersion(targetEngineVersion);
return this;
}
/**
*
* The DB parameter group associated with the DB instance in the green environment.
*
*
* To test parameter changes, specify a DB parameter group that is different from the one associated with the source
* DB instance.
*
*
* @param targetDBParameterGroupName
* The DB parameter group associated with the DB instance in the green environment.
*
* To test parameter changes, specify a DB parameter group that is different from the one associated with the
* source DB instance.
*/
public void setTargetDBParameterGroupName(String targetDBParameterGroupName) {
this.targetDBParameterGroupName = targetDBParameterGroupName;
}
/**
*
* The DB parameter group associated with the DB instance in the green environment.
*
*
* To test parameter changes, specify a DB parameter group that is different from the one associated with the source
* DB instance.
*
*
* @return The DB parameter group associated with the DB instance in the green environment.
*
* To test parameter changes, specify a DB parameter group that is different from the one associated with
* the source DB instance.
*/
public String getTargetDBParameterGroupName() {
return this.targetDBParameterGroupName;
}
/**
*
* The DB parameter group associated with the DB instance in the green environment.
*
*
* To test parameter changes, specify a DB parameter group that is different from the one associated with the source
* DB instance.
*
*
* @param targetDBParameterGroupName
* The DB parameter group associated with the DB instance in the green environment.
*
* To test parameter changes, specify a DB parameter group that is different from the one associated with the
* source DB instance.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateBlueGreenDeploymentRequest withTargetDBParameterGroupName(String targetDBParameterGroupName) {
setTargetDBParameterGroupName(targetDBParameterGroupName);
return this;
}
/**
*
* The DB cluster parameter group associated with the Aurora DB cluster in the green environment.
*
*
* To test parameter changes, specify a DB cluster parameter group that is different from the one associated with
* the source DB cluster.
*
*
* @param targetDBClusterParameterGroupName
* The DB cluster parameter group associated with the Aurora DB cluster in the green environment.
*
* To test parameter changes, specify a DB cluster parameter group that is different from the one associated
* with the source DB cluster.
*/
public void setTargetDBClusterParameterGroupName(String targetDBClusterParameterGroupName) {
this.targetDBClusterParameterGroupName = targetDBClusterParameterGroupName;
}
/**
*
* The DB cluster parameter group associated with the Aurora DB cluster in the green environment.
*
*
* To test parameter changes, specify a DB cluster parameter group that is different from the one associated with
* the source DB cluster.
*
*
* @return The DB cluster parameter group associated with the Aurora DB cluster in the green environment.
*
* To test parameter changes, specify a DB cluster parameter group that is different from the one associated
* with the source DB cluster.
*/
public String getTargetDBClusterParameterGroupName() {
return this.targetDBClusterParameterGroupName;
}
/**
*
* The DB cluster parameter group associated with the Aurora DB cluster in the green environment.
*
*
* To test parameter changes, specify a DB cluster parameter group that is different from the one associated with
* the source DB cluster.
*
*
* @param targetDBClusterParameterGroupName
* The DB cluster parameter group associated with the Aurora DB cluster in the green environment.
*
* To test parameter changes, specify a DB cluster parameter group that is different from the one associated
* with the source DB cluster.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateBlueGreenDeploymentRequest withTargetDBClusterParameterGroupName(String targetDBClusterParameterGroupName) {
setTargetDBClusterParameterGroupName(targetDBClusterParameterGroupName);
return this;
}
/**
*
* Tags to assign to the blue/green deployment.
*
*
* @return Tags to assign to the blue/green deployment.
*/
public java.util.List getTags() {
if (tags == null) {
tags = new com.amazonaws.internal.SdkInternalList();
}
return tags;
}
/**
*
* Tags to assign to the blue/green deployment.
*
*
* @param tags
* Tags to assign to the blue/green deployment.
*/
public void setTags(java.util.Collection tags) {
if (tags == null) {
this.tags = null;
return;
}
this.tags = new com.amazonaws.internal.SdkInternalList(tags);
}
/**
*
* Tags to assign to the blue/green deployment.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setTags(java.util.Collection)} or {@link #withTags(java.util.Collection)} if you want to override the
* existing values.
*
*
* @param tags
* Tags to assign to the blue/green deployment.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateBlueGreenDeploymentRequest withTags(Tag... tags) {
if (this.tags == null) {
setTags(new com.amazonaws.internal.SdkInternalList(tags.length));
}
for (Tag ele : tags) {
this.tags.add(ele);
}
return this;
}
/**
*
* Tags to assign to the blue/green deployment.
*
*
* @param tags
* Tags to assign to the blue/green deployment.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateBlueGreenDeploymentRequest withTags(java.util.Collection tags) {
setTags(tags);
return this;
}
/**
*
* Specify the DB instance class for the databases in the green environment.
*
*
* This parameter only applies to RDS DB instances, because DB instances within an Aurora DB cluster can have
* multiple different instance classes. If you're creating a blue/green deployment from an Aurora DB cluster, don't
* specify this parameter. After the green environment is created, you can individually modify the instance classes
* of the DB instances within the green DB cluster.
*
*
* @param targetDBInstanceClass
* Specify the DB instance class for the databases in the green environment.
*
* This parameter only applies to RDS DB instances, because DB instances within an Aurora DB cluster can have
* multiple different instance classes. If you're creating a blue/green deployment from an Aurora DB cluster,
* don't specify this parameter. After the green environment is created, you can individually modify the
* instance classes of the DB instances within the green DB cluster.
*/
public void setTargetDBInstanceClass(String targetDBInstanceClass) {
this.targetDBInstanceClass = targetDBInstanceClass;
}
/**
*
* Specify the DB instance class for the databases in the green environment.
*
*
* This parameter only applies to RDS DB instances, because DB instances within an Aurora DB cluster can have
* multiple different instance classes. If you're creating a blue/green deployment from an Aurora DB cluster, don't
* specify this parameter. After the green environment is created, you can individually modify the instance classes
* of the DB instances within the green DB cluster.
*
*
* @return Specify the DB instance class for the databases in the green environment.
*
* This parameter only applies to RDS DB instances, because DB instances within an Aurora DB cluster can
* have multiple different instance classes. If you're creating a blue/green deployment from an Aurora DB
* cluster, don't specify this parameter. After the green environment is created, you can individually
* modify the instance classes of the DB instances within the green DB cluster.
*/
public String getTargetDBInstanceClass() {
return this.targetDBInstanceClass;
}
/**
*
* Specify the DB instance class for the databases in the green environment.
*
*
* This parameter only applies to RDS DB instances, because DB instances within an Aurora DB cluster can have
* multiple different instance classes. If you're creating a blue/green deployment from an Aurora DB cluster, don't
* specify this parameter. After the green environment is created, you can individually modify the instance classes
* of the DB instances within the green DB cluster.
*
*
* @param targetDBInstanceClass
* Specify the DB instance class for the databases in the green environment.
*
* This parameter only applies to RDS DB instances, because DB instances within an Aurora DB cluster can have
* multiple different instance classes. If you're creating a blue/green deployment from an Aurora DB cluster,
* don't specify this parameter. After the green environment is created, you can individually modify the
* instance classes of the DB instances within the green DB cluster.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateBlueGreenDeploymentRequest withTargetDBInstanceClass(String targetDBInstanceClass) {
setTargetDBInstanceClass(targetDBInstanceClass);
return this;
}
/**
*
* Whether to upgrade the storage file system configuration on the green database. This option migrates the green DB
* instance from the older 32-bit file system to the preferred configuration. For more information, see Upgrading the storage file system for a DB instance.
*
*
* @param upgradeTargetStorageConfig
* Whether to upgrade the storage file system configuration on the green database. This option migrates the
* green DB instance from the older 32-bit file system to the preferred configuration. For more information,
* see Upgrading the storage file system for a DB instance.
*/
public void setUpgradeTargetStorageConfig(Boolean upgradeTargetStorageConfig) {
this.upgradeTargetStorageConfig = upgradeTargetStorageConfig;
}
/**
*
* Whether to upgrade the storage file system configuration on the green database. This option migrates the green DB
* instance from the older 32-bit file system to the preferred configuration. For more information, see Upgrading the storage file system for a DB instance.
*
*
* @return Whether to upgrade the storage file system configuration on the green database. This option migrates the
* green DB instance from the older 32-bit file system to the preferred configuration. For more information,
* see Upgrading the storage file system for a DB instance.
*/
public Boolean getUpgradeTargetStorageConfig() {
return this.upgradeTargetStorageConfig;
}
/**
*
* Whether to upgrade the storage file system configuration on the green database. This option migrates the green DB
* instance from the older 32-bit file system to the preferred configuration. For more information, see Upgrading the storage file system for a DB instance.
*
*
* @param upgradeTargetStorageConfig
* Whether to upgrade the storage file system configuration on the green database. This option migrates the
* green DB instance from the older 32-bit file system to the preferred configuration. For more information,
* see Upgrading the storage file system for a DB instance.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateBlueGreenDeploymentRequest withUpgradeTargetStorageConfig(Boolean upgradeTargetStorageConfig) {
setUpgradeTargetStorageConfig(upgradeTargetStorageConfig);
return this;
}
/**
*
* Whether to upgrade the storage file system configuration on the green database. This option migrates the green DB
* instance from the older 32-bit file system to the preferred configuration. For more information, see Upgrading the storage file system for a DB instance.
*
*
* @return Whether to upgrade the storage file system configuration on the green database. This option migrates the
* green DB instance from the older 32-bit file system to the preferred configuration. For more information,
* see Upgrading the storage file system for a DB instance.
*/
public Boolean isUpgradeTargetStorageConfig() {
return this.upgradeTargetStorageConfig;
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getBlueGreenDeploymentName() != null)
sb.append("BlueGreenDeploymentName: ").append(getBlueGreenDeploymentName()).append(",");
if (getSource() != null)
sb.append("Source: ").append(getSource()).append(",");
if (getTargetEngineVersion() != null)
sb.append("TargetEngineVersion: ").append(getTargetEngineVersion()).append(",");
if (getTargetDBParameterGroupName() != null)
sb.append("TargetDBParameterGroupName: ").append(getTargetDBParameterGroupName()).append(",");
if (getTargetDBClusterParameterGroupName() != null)
sb.append("TargetDBClusterParameterGroupName: ").append(getTargetDBClusterParameterGroupName()).append(",");
if (getTags() != null)
sb.append("Tags: ").append(getTags()).append(",");
if (getTargetDBInstanceClass() != null)
sb.append("TargetDBInstanceClass: ").append(getTargetDBInstanceClass()).append(",");
if (getUpgradeTargetStorageConfig() != null)
sb.append("UpgradeTargetStorageConfig: ").append(getUpgradeTargetStorageConfig());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof CreateBlueGreenDeploymentRequest == false)
return false;
CreateBlueGreenDeploymentRequest other = (CreateBlueGreenDeploymentRequest) obj;
if (other.getBlueGreenDeploymentName() == null ^ this.getBlueGreenDeploymentName() == null)
return false;
if (other.getBlueGreenDeploymentName() != null && other.getBlueGreenDeploymentName().equals(this.getBlueGreenDeploymentName()) == false)
return false;
if (other.getSource() == null ^ this.getSource() == null)
return false;
if (other.getSource() != null && other.getSource().equals(this.getSource()) == false)
return false;
if (other.getTargetEngineVersion() == null ^ this.getTargetEngineVersion() == null)
return false;
if (other.getTargetEngineVersion() != null && other.getTargetEngineVersion().equals(this.getTargetEngineVersion()) == false)
return false;
if (other.getTargetDBParameterGroupName() == null ^ this.getTargetDBParameterGroupName() == null)
return false;
if (other.getTargetDBParameterGroupName() != null && other.getTargetDBParameterGroupName().equals(this.getTargetDBParameterGroupName()) == false)
return false;
if (other.getTargetDBClusterParameterGroupName() == null ^ this.getTargetDBClusterParameterGroupName() == null)
return false;
if (other.getTargetDBClusterParameterGroupName() != null
&& other.getTargetDBClusterParameterGroupName().equals(this.getTargetDBClusterParameterGroupName()) == false)
return false;
if (other.getTags() == null ^ this.getTags() == null)
return false;
if (other.getTags() != null && other.getTags().equals(this.getTags()) == false)
return false;
if (other.getTargetDBInstanceClass() == null ^ this.getTargetDBInstanceClass() == null)
return false;
if (other.getTargetDBInstanceClass() != null && other.getTargetDBInstanceClass().equals(this.getTargetDBInstanceClass()) == false)
return false;
if (other.getUpgradeTargetStorageConfig() == null ^ this.getUpgradeTargetStorageConfig() == null)
return false;
if (other.getUpgradeTargetStorageConfig() != null && other.getUpgradeTargetStorageConfig().equals(this.getUpgradeTargetStorageConfig()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getBlueGreenDeploymentName() == null) ? 0 : getBlueGreenDeploymentName().hashCode());
hashCode = prime * hashCode + ((getSource() == null) ? 0 : getSource().hashCode());
hashCode = prime * hashCode + ((getTargetEngineVersion() == null) ? 0 : getTargetEngineVersion().hashCode());
hashCode = prime * hashCode + ((getTargetDBParameterGroupName() == null) ? 0 : getTargetDBParameterGroupName().hashCode());
hashCode = prime * hashCode + ((getTargetDBClusterParameterGroupName() == null) ? 0 : getTargetDBClusterParameterGroupName().hashCode());
hashCode = prime * hashCode + ((getTags() == null) ? 0 : getTags().hashCode());
hashCode = prime * hashCode + ((getTargetDBInstanceClass() == null) ? 0 : getTargetDBInstanceClass().hashCode());
hashCode = prime * hashCode + ((getUpgradeTargetStorageConfig() == null) ? 0 : getUpgradeTargetStorageConfig().hashCode());
return hashCode;
}
@Override
public CreateBlueGreenDeploymentRequest clone() {
return (CreateBlueGreenDeploymentRequest) super.clone();
}
}