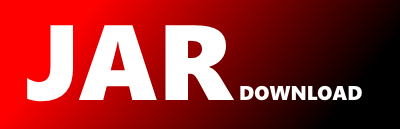
com.amazonaws.services.rds.model.CreateTenantDatabaseRequest Maven / Gradle / Ivy
Show all versions of aws-java-sdk-rds Show documentation
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.rds.model;
import java.io.Serializable;
import javax.annotation.Generated;
import com.amazonaws.AmazonWebServiceRequest;
/**
*
* @see AWS API
* Documentation
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class CreateTenantDatabaseRequest extends com.amazonaws.AmazonWebServiceRequest implements Serializable, Cloneable {
/**
*
* The user-supplied DB instance identifier. RDS creates your tenant database in this DB instance. This parameter
* isn't case-sensitive.
*
*/
private String dBInstanceIdentifier;
/**
*
* The user-supplied name of the tenant database that you want to create in your DB instance. This parameter has the
* same constraints as DBName
in CreateDBInstance
.
*
*/
private String tenantDBName;
/**
*
* The name for the master user account in your tenant database. RDS creates this user account in the tenant
* database and grants privileges to the master user. This parameter is case-sensitive.
*
*
* Constraints:
*
*
* -
*
* Must be 1 to 16 letters, numbers, or underscores.
*
*
* -
*
* First character must be a letter.
*
*
* -
*
* Can't be a reserved word for the chosen database engine.
*
*
*
*/
private String masterUsername;
/**
*
* The password for the master user in your tenant database.
*
*
* Constraints:
*
*
* -
*
* Must be 8 to 30 characters.
*
*
* -
*
* Can include any printable ASCII character except forward slash (/
), double quote ("
),
* at symbol (@
), ampersand (&
), or single quote ('
).
*
*
*
*/
private String masterUserPassword;
/**
*
* The character set for your tenant database. If you don't specify a value, the character set name defaults to
* AL32UTF8
.
*
*/
private String characterSetName;
/**
*
* The NCHAR
value for the tenant database.
*
*/
private String ncharCharacterSetName;
private com.amazonaws.internal.SdkInternalList tags;
/**
*
* The user-supplied DB instance identifier. RDS creates your tenant database in this DB instance. This parameter
* isn't case-sensitive.
*
*
* @param dBInstanceIdentifier
* The user-supplied DB instance identifier. RDS creates your tenant database in this DB instance. This
* parameter isn't case-sensitive.
*/
public void setDBInstanceIdentifier(String dBInstanceIdentifier) {
this.dBInstanceIdentifier = dBInstanceIdentifier;
}
/**
*
* The user-supplied DB instance identifier. RDS creates your tenant database in this DB instance. This parameter
* isn't case-sensitive.
*
*
* @return The user-supplied DB instance identifier. RDS creates your tenant database in this DB instance. This
* parameter isn't case-sensitive.
*/
public String getDBInstanceIdentifier() {
return this.dBInstanceIdentifier;
}
/**
*
* The user-supplied DB instance identifier. RDS creates your tenant database in this DB instance. This parameter
* isn't case-sensitive.
*
*
* @param dBInstanceIdentifier
* The user-supplied DB instance identifier. RDS creates your tenant database in this DB instance. This
* parameter isn't case-sensitive.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateTenantDatabaseRequest withDBInstanceIdentifier(String dBInstanceIdentifier) {
setDBInstanceIdentifier(dBInstanceIdentifier);
return this;
}
/**
*
* The user-supplied name of the tenant database that you want to create in your DB instance. This parameter has the
* same constraints as DBName
in CreateDBInstance
.
*
*
* @param tenantDBName
* The user-supplied name of the tenant database that you want to create in your DB instance. This parameter
* has the same constraints as DBName
in CreateDBInstance
.
*/
public void setTenantDBName(String tenantDBName) {
this.tenantDBName = tenantDBName;
}
/**
*
* The user-supplied name of the tenant database that you want to create in your DB instance. This parameter has the
* same constraints as DBName
in CreateDBInstance
.
*
*
* @return The user-supplied name of the tenant database that you want to create in your DB instance. This parameter
* has the same constraints as DBName
in CreateDBInstance
.
*/
public String getTenantDBName() {
return this.tenantDBName;
}
/**
*
* The user-supplied name of the tenant database that you want to create in your DB instance. This parameter has the
* same constraints as DBName
in CreateDBInstance
.
*
*
* @param tenantDBName
* The user-supplied name of the tenant database that you want to create in your DB instance. This parameter
* has the same constraints as DBName
in CreateDBInstance
.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateTenantDatabaseRequest withTenantDBName(String tenantDBName) {
setTenantDBName(tenantDBName);
return this;
}
/**
*
* The name for the master user account in your tenant database. RDS creates this user account in the tenant
* database and grants privileges to the master user. This parameter is case-sensitive.
*
*
* Constraints:
*
*
* -
*
* Must be 1 to 16 letters, numbers, or underscores.
*
*
* -
*
* First character must be a letter.
*
*
* -
*
* Can't be a reserved word for the chosen database engine.
*
*
*
*
* @param masterUsername
* The name for the master user account in your tenant database. RDS creates this user account in the tenant
* database and grants privileges to the master user. This parameter is case-sensitive.
*
* Constraints:
*
*
* -
*
* Must be 1 to 16 letters, numbers, or underscores.
*
*
* -
*
* First character must be a letter.
*
*
* -
*
* Can't be a reserved word for the chosen database engine.
*
*
*/
public void setMasterUsername(String masterUsername) {
this.masterUsername = masterUsername;
}
/**
*
* The name for the master user account in your tenant database. RDS creates this user account in the tenant
* database and grants privileges to the master user. This parameter is case-sensitive.
*
*
* Constraints:
*
*
* -
*
* Must be 1 to 16 letters, numbers, or underscores.
*
*
* -
*
* First character must be a letter.
*
*
* -
*
* Can't be a reserved word for the chosen database engine.
*
*
*
*
* @return The name for the master user account in your tenant database. RDS creates this user account in the tenant
* database and grants privileges to the master user. This parameter is case-sensitive.
*
* Constraints:
*
*
* -
*
* Must be 1 to 16 letters, numbers, or underscores.
*
*
* -
*
* First character must be a letter.
*
*
* -
*
* Can't be a reserved word for the chosen database engine.
*
*
*/
public String getMasterUsername() {
return this.masterUsername;
}
/**
*
* The name for the master user account in your tenant database. RDS creates this user account in the tenant
* database and grants privileges to the master user. This parameter is case-sensitive.
*
*
* Constraints:
*
*
* -
*
* Must be 1 to 16 letters, numbers, or underscores.
*
*
* -
*
* First character must be a letter.
*
*
* -
*
* Can't be a reserved word for the chosen database engine.
*
*
*
*
* @param masterUsername
* The name for the master user account in your tenant database. RDS creates this user account in the tenant
* database and grants privileges to the master user. This parameter is case-sensitive.
*
* Constraints:
*
*
* -
*
* Must be 1 to 16 letters, numbers, or underscores.
*
*
* -
*
* First character must be a letter.
*
*
* -
*
* Can't be a reserved word for the chosen database engine.
*
*
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateTenantDatabaseRequest withMasterUsername(String masterUsername) {
setMasterUsername(masterUsername);
return this;
}
/**
*
* The password for the master user in your tenant database.
*
*
* Constraints:
*
*
* -
*
* Must be 8 to 30 characters.
*
*
* -
*
* Can include any printable ASCII character except forward slash (/
), double quote ("
),
* at symbol (@
), ampersand (&
), or single quote ('
).
*
*
*
*
* @param masterUserPassword
* The password for the master user in your tenant database.
*
* Constraints:
*
*
* -
*
* Must be 8 to 30 characters.
*
*
* -
*
* Can include any printable ASCII character except forward slash (/
), double quote (
* "
), at symbol (@
), ampersand (&
), or single quote (
* '
).
*
*
*/
public void setMasterUserPassword(String masterUserPassword) {
this.masterUserPassword = masterUserPassword;
}
/**
*
* The password for the master user in your tenant database.
*
*
* Constraints:
*
*
* -
*
* Must be 8 to 30 characters.
*
*
* -
*
* Can include any printable ASCII character except forward slash (/
), double quote ("
),
* at symbol (@
), ampersand (&
), or single quote ('
).
*
*
*
*
* @return The password for the master user in your tenant database.
*
* Constraints:
*
*
* -
*
* Must be 8 to 30 characters.
*
*
* -
*
* Can include any printable ASCII character except forward slash (/
), double quote (
* "
), at symbol (@
), ampersand (&
), or single quote (
* '
).
*
*
*/
public String getMasterUserPassword() {
return this.masterUserPassword;
}
/**
*
* The password for the master user in your tenant database.
*
*
* Constraints:
*
*
* -
*
* Must be 8 to 30 characters.
*
*
* -
*
* Can include any printable ASCII character except forward slash (/
), double quote ("
),
* at symbol (@
), ampersand (&
), or single quote ('
).
*
*
*
*
* @param masterUserPassword
* The password for the master user in your tenant database.
*
* Constraints:
*
*
* -
*
* Must be 8 to 30 characters.
*
*
* -
*
* Can include any printable ASCII character except forward slash (/
), double quote (
* "
), at symbol (@
), ampersand (&
), or single quote (
* '
).
*
*
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateTenantDatabaseRequest withMasterUserPassword(String masterUserPassword) {
setMasterUserPassword(masterUserPassword);
return this;
}
/**
*
* The character set for your tenant database. If you don't specify a value, the character set name defaults to
* AL32UTF8
.
*
*
* @param characterSetName
* The character set for your tenant database. If you don't specify a value, the character set name defaults
* to AL32UTF8
.
*/
public void setCharacterSetName(String characterSetName) {
this.characterSetName = characterSetName;
}
/**
*
* The character set for your tenant database. If you don't specify a value, the character set name defaults to
* AL32UTF8
.
*
*
* @return The character set for your tenant database. If you don't specify a value, the character set name defaults
* to AL32UTF8
.
*/
public String getCharacterSetName() {
return this.characterSetName;
}
/**
*
* The character set for your tenant database. If you don't specify a value, the character set name defaults to
* AL32UTF8
.
*
*
* @param characterSetName
* The character set for your tenant database. If you don't specify a value, the character set name defaults
* to AL32UTF8
.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateTenantDatabaseRequest withCharacterSetName(String characterSetName) {
setCharacterSetName(characterSetName);
return this;
}
/**
*
* The NCHAR
value for the tenant database.
*
*
* @param ncharCharacterSetName
* The NCHAR
value for the tenant database.
*/
public void setNcharCharacterSetName(String ncharCharacterSetName) {
this.ncharCharacterSetName = ncharCharacterSetName;
}
/**
*
* The NCHAR
value for the tenant database.
*
*
* @return The NCHAR
value for the tenant database.
*/
public String getNcharCharacterSetName() {
return this.ncharCharacterSetName;
}
/**
*
* The NCHAR
value for the tenant database.
*
*
* @param ncharCharacterSetName
* The NCHAR
value for the tenant database.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateTenantDatabaseRequest withNcharCharacterSetName(String ncharCharacterSetName) {
setNcharCharacterSetName(ncharCharacterSetName);
return this;
}
/**
* @return
*/
public java.util.List getTags() {
if (tags == null) {
tags = new com.amazonaws.internal.SdkInternalList();
}
return tags;
}
/**
* @param tags
*/
public void setTags(java.util.Collection tags) {
if (tags == null) {
this.tags = null;
return;
}
this.tags = new com.amazonaws.internal.SdkInternalList(tags);
}
/**
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setTags(java.util.Collection)} or {@link #withTags(java.util.Collection)} if you want to override the
* existing values.
*
*
* @param tags
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateTenantDatabaseRequest withTags(Tag... tags) {
if (this.tags == null) {
setTags(new com.amazonaws.internal.SdkInternalList(tags.length));
}
for (Tag ele : tags) {
this.tags.add(ele);
}
return this;
}
/**
* @param tags
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateTenantDatabaseRequest withTags(java.util.Collection tags) {
setTags(tags);
return this;
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getDBInstanceIdentifier() != null)
sb.append("DBInstanceIdentifier: ").append(getDBInstanceIdentifier()).append(",");
if (getTenantDBName() != null)
sb.append("TenantDBName: ").append(getTenantDBName()).append(",");
if (getMasterUsername() != null)
sb.append("MasterUsername: ").append(getMasterUsername()).append(",");
if (getMasterUserPassword() != null)
sb.append("MasterUserPassword: ").append("***Sensitive Data Redacted***").append(",");
if (getCharacterSetName() != null)
sb.append("CharacterSetName: ").append(getCharacterSetName()).append(",");
if (getNcharCharacterSetName() != null)
sb.append("NcharCharacterSetName: ").append(getNcharCharacterSetName()).append(",");
if (getTags() != null)
sb.append("Tags: ").append(getTags());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof CreateTenantDatabaseRequest == false)
return false;
CreateTenantDatabaseRequest other = (CreateTenantDatabaseRequest) obj;
if (other.getDBInstanceIdentifier() == null ^ this.getDBInstanceIdentifier() == null)
return false;
if (other.getDBInstanceIdentifier() != null && other.getDBInstanceIdentifier().equals(this.getDBInstanceIdentifier()) == false)
return false;
if (other.getTenantDBName() == null ^ this.getTenantDBName() == null)
return false;
if (other.getTenantDBName() != null && other.getTenantDBName().equals(this.getTenantDBName()) == false)
return false;
if (other.getMasterUsername() == null ^ this.getMasterUsername() == null)
return false;
if (other.getMasterUsername() != null && other.getMasterUsername().equals(this.getMasterUsername()) == false)
return false;
if (other.getMasterUserPassword() == null ^ this.getMasterUserPassword() == null)
return false;
if (other.getMasterUserPassword() != null && other.getMasterUserPassword().equals(this.getMasterUserPassword()) == false)
return false;
if (other.getCharacterSetName() == null ^ this.getCharacterSetName() == null)
return false;
if (other.getCharacterSetName() != null && other.getCharacterSetName().equals(this.getCharacterSetName()) == false)
return false;
if (other.getNcharCharacterSetName() == null ^ this.getNcharCharacterSetName() == null)
return false;
if (other.getNcharCharacterSetName() != null && other.getNcharCharacterSetName().equals(this.getNcharCharacterSetName()) == false)
return false;
if (other.getTags() == null ^ this.getTags() == null)
return false;
if (other.getTags() != null && other.getTags().equals(this.getTags()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getDBInstanceIdentifier() == null) ? 0 : getDBInstanceIdentifier().hashCode());
hashCode = prime * hashCode + ((getTenantDBName() == null) ? 0 : getTenantDBName().hashCode());
hashCode = prime * hashCode + ((getMasterUsername() == null) ? 0 : getMasterUsername().hashCode());
hashCode = prime * hashCode + ((getMasterUserPassword() == null) ? 0 : getMasterUserPassword().hashCode());
hashCode = prime * hashCode + ((getCharacterSetName() == null) ? 0 : getCharacterSetName().hashCode());
hashCode = prime * hashCode + ((getNcharCharacterSetName() == null) ? 0 : getNcharCharacterSetName().hashCode());
hashCode = prime * hashCode + ((getTags() == null) ? 0 : getTags().hashCode());
return hashCode;
}
@Override
public CreateTenantDatabaseRequest clone() {
return (CreateTenantDatabaseRequest) super.clone();
}
}