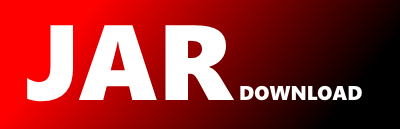
com.amazonaws.services.rds.model.DBProxyTarget Maven / Gradle / Ivy
Show all versions of aws-java-sdk-rds Show documentation
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.rds.model;
import java.io.Serializable;
import javax.annotation.Generated;
/**
*
* Contains the details for an RDS Proxy target. It represents an RDS DB instance or Aurora DB cluster that the proxy
* can connect to. One or more targets are associated with an RDS Proxy target group.
*
*
* This data type is used as a response element in the DescribeDBProxyTargets
action.
*
*
* @see AWS API
* Documentation
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class DBProxyTarget implements Serializable, Cloneable {
/**
*
* The Amazon Resource Name (ARN) for the RDS DB instance or Aurora DB cluster.
*
*/
private String targetArn;
/**
*
* The writer endpoint for the RDS DB instance or Aurora DB cluster.
*
*/
private String endpoint;
/**
*
* The DB cluster identifier when the target represents an Aurora DB cluster. This field is blank when the target
* represents an RDS DB instance.
*
*/
private String trackedClusterId;
/**
*
* The identifier representing the target. It can be the instance identifier for an RDS DB instance, or the cluster
* identifier for an Aurora DB cluster.
*
*/
private String rdsResourceId;
/**
*
* The port that the RDS Proxy uses to connect to the target RDS DB instance or Aurora DB cluster.
*
*/
private Integer port;
/**
*
* Specifies the kind of database, such as an RDS DB instance or an Aurora DB cluster, that the target represents.
*
*/
private String type;
/**
*
* A value that indicates whether the target of the proxy can be used for read/write or read-only operations.
*
*/
private String role;
/**
*
* Information about the connection health of the RDS Proxy target.
*
*/
private TargetHealth targetHealth;
/**
*
* The Amazon Resource Name (ARN) for the RDS DB instance or Aurora DB cluster.
*
*
* @param targetArn
* The Amazon Resource Name (ARN) for the RDS DB instance or Aurora DB cluster.
*/
public void setTargetArn(String targetArn) {
this.targetArn = targetArn;
}
/**
*
* The Amazon Resource Name (ARN) for the RDS DB instance or Aurora DB cluster.
*
*
* @return The Amazon Resource Name (ARN) for the RDS DB instance or Aurora DB cluster.
*/
public String getTargetArn() {
return this.targetArn;
}
/**
*
* The Amazon Resource Name (ARN) for the RDS DB instance or Aurora DB cluster.
*
*
* @param targetArn
* The Amazon Resource Name (ARN) for the RDS DB instance or Aurora DB cluster.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DBProxyTarget withTargetArn(String targetArn) {
setTargetArn(targetArn);
return this;
}
/**
*
* The writer endpoint for the RDS DB instance or Aurora DB cluster.
*
*
* @param endpoint
* The writer endpoint for the RDS DB instance or Aurora DB cluster.
*/
public void setEndpoint(String endpoint) {
this.endpoint = endpoint;
}
/**
*
* The writer endpoint for the RDS DB instance or Aurora DB cluster.
*
*
* @return The writer endpoint for the RDS DB instance or Aurora DB cluster.
*/
public String getEndpoint() {
return this.endpoint;
}
/**
*
* The writer endpoint for the RDS DB instance or Aurora DB cluster.
*
*
* @param endpoint
* The writer endpoint for the RDS DB instance or Aurora DB cluster.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DBProxyTarget withEndpoint(String endpoint) {
setEndpoint(endpoint);
return this;
}
/**
*
* The DB cluster identifier when the target represents an Aurora DB cluster. This field is blank when the target
* represents an RDS DB instance.
*
*
* @param trackedClusterId
* The DB cluster identifier when the target represents an Aurora DB cluster. This field is blank when the
* target represents an RDS DB instance.
*/
public void setTrackedClusterId(String trackedClusterId) {
this.trackedClusterId = trackedClusterId;
}
/**
*
* The DB cluster identifier when the target represents an Aurora DB cluster. This field is blank when the target
* represents an RDS DB instance.
*
*
* @return The DB cluster identifier when the target represents an Aurora DB cluster. This field is blank when the
* target represents an RDS DB instance.
*/
public String getTrackedClusterId() {
return this.trackedClusterId;
}
/**
*
* The DB cluster identifier when the target represents an Aurora DB cluster. This field is blank when the target
* represents an RDS DB instance.
*
*
* @param trackedClusterId
* The DB cluster identifier when the target represents an Aurora DB cluster. This field is blank when the
* target represents an RDS DB instance.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DBProxyTarget withTrackedClusterId(String trackedClusterId) {
setTrackedClusterId(trackedClusterId);
return this;
}
/**
*
* The identifier representing the target. It can be the instance identifier for an RDS DB instance, or the cluster
* identifier for an Aurora DB cluster.
*
*
* @param rdsResourceId
* The identifier representing the target. It can be the instance identifier for an RDS DB instance, or the
* cluster identifier for an Aurora DB cluster.
*/
public void setRdsResourceId(String rdsResourceId) {
this.rdsResourceId = rdsResourceId;
}
/**
*
* The identifier representing the target. It can be the instance identifier for an RDS DB instance, or the cluster
* identifier for an Aurora DB cluster.
*
*
* @return The identifier representing the target. It can be the instance identifier for an RDS DB instance, or the
* cluster identifier for an Aurora DB cluster.
*/
public String getRdsResourceId() {
return this.rdsResourceId;
}
/**
*
* The identifier representing the target. It can be the instance identifier for an RDS DB instance, or the cluster
* identifier for an Aurora DB cluster.
*
*
* @param rdsResourceId
* The identifier representing the target. It can be the instance identifier for an RDS DB instance, or the
* cluster identifier for an Aurora DB cluster.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DBProxyTarget withRdsResourceId(String rdsResourceId) {
setRdsResourceId(rdsResourceId);
return this;
}
/**
*
* The port that the RDS Proxy uses to connect to the target RDS DB instance or Aurora DB cluster.
*
*
* @param port
* The port that the RDS Proxy uses to connect to the target RDS DB instance or Aurora DB cluster.
*/
public void setPort(Integer port) {
this.port = port;
}
/**
*
* The port that the RDS Proxy uses to connect to the target RDS DB instance or Aurora DB cluster.
*
*
* @return The port that the RDS Proxy uses to connect to the target RDS DB instance or Aurora DB cluster.
*/
public Integer getPort() {
return this.port;
}
/**
*
* The port that the RDS Proxy uses to connect to the target RDS DB instance or Aurora DB cluster.
*
*
* @param port
* The port that the RDS Proxy uses to connect to the target RDS DB instance or Aurora DB cluster.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DBProxyTarget withPort(Integer port) {
setPort(port);
return this;
}
/**
*
* Specifies the kind of database, such as an RDS DB instance or an Aurora DB cluster, that the target represents.
*
*
* @param type
* Specifies the kind of database, such as an RDS DB instance or an Aurora DB cluster, that the target
* represents.
* @see TargetType
*/
public void setType(String type) {
this.type = type;
}
/**
*
* Specifies the kind of database, such as an RDS DB instance or an Aurora DB cluster, that the target represents.
*
*
* @return Specifies the kind of database, such as an RDS DB instance or an Aurora DB cluster, that the target
* represents.
* @see TargetType
*/
public String getType() {
return this.type;
}
/**
*
* Specifies the kind of database, such as an RDS DB instance or an Aurora DB cluster, that the target represents.
*
*
* @param type
* Specifies the kind of database, such as an RDS DB instance or an Aurora DB cluster, that the target
* represents.
* @return Returns a reference to this object so that method calls can be chained together.
* @see TargetType
*/
public DBProxyTarget withType(String type) {
setType(type);
return this;
}
/**
*
* Specifies the kind of database, such as an RDS DB instance or an Aurora DB cluster, that the target represents.
*
*
* @param type
* Specifies the kind of database, such as an RDS DB instance or an Aurora DB cluster, that the target
* represents.
* @return Returns a reference to this object so that method calls can be chained together.
* @see TargetType
*/
public DBProxyTarget withType(TargetType type) {
this.type = type.toString();
return this;
}
/**
*
* A value that indicates whether the target of the proxy can be used for read/write or read-only operations.
*
*
* @param role
* A value that indicates whether the target of the proxy can be used for read/write or read-only operations.
* @see TargetRole
*/
public void setRole(String role) {
this.role = role;
}
/**
*
* A value that indicates whether the target of the proxy can be used for read/write or read-only operations.
*
*
* @return A value that indicates whether the target of the proxy can be used for read/write or read-only
* operations.
* @see TargetRole
*/
public String getRole() {
return this.role;
}
/**
*
* A value that indicates whether the target of the proxy can be used for read/write or read-only operations.
*
*
* @param role
* A value that indicates whether the target of the proxy can be used for read/write or read-only operations.
* @return Returns a reference to this object so that method calls can be chained together.
* @see TargetRole
*/
public DBProxyTarget withRole(String role) {
setRole(role);
return this;
}
/**
*
* A value that indicates whether the target of the proxy can be used for read/write or read-only operations.
*
*
* @param role
* A value that indicates whether the target of the proxy can be used for read/write or read-only operations.
* @return Returns a reference to this object so that method calls can be chained together.
* @see TargetRole
*/
public DBProxyTarget withRole(TargetRole role) {
this.role = role.toString();
return this;
}
/**
*
* Information about the connection health of the RDS Proxy target.
*
*
* @param targetHealth
* Information about the connection health of the RDS Proxy target.
*/
public void setTargetHealth(TargetHealth targetHealth) {
this.targetHealth = targetHealth;
}
/**
*
* Information about the connection health of the RDS Proxy target.
*
*
* @return Information about the connection health of the RDS Proxy target.
*/
public TargetHealth getTargetHealth() {
return this.targetHealth;
}
/**
*
* Information about the connection health of the RDS Proxy target.
*
*
* @param targetHealth
* Information about the connection health of the RDS Proxy target.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DBProxyTarget withTargetHealth(TargetHealth targetHealth) {
setTargetHealth(targetHealth);
return this;
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getTargetArn() != null)
sb.append("TargetArn: ").append(getTargetArn()).append(",");
if (getEndpoint() != null)
sb.append("Endpoint: ").append(getEndpoint()).append(",");
if (getTrackedClusterId() != null)
sb.append("TrackedClusterId: ").append(getTrackedClusterId()).append(",");
if (getRdsResourceId() != null)
sb.append("RdsResourceId: ").append(getRdsResourceId()).append(",");
if (getPort() != null)
sb.append("Port: ").append(getPort()).append(",");
if (getType() != null)
sb.append("Type: ").append(getType()).append(",");
if (getRole() != null)
sb.append("Role: ").append(getRole()).append(",");
if (getTargetHealth() != null)
sb.append("TargetHealth: ").append(getTargetHealth());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof DBProxyTarget == false)
return false;
DBProxyTarget other = (DBProxyTarget) obj;
if (other.getTargetArn() == null ^ this.getTargetArn() == null)
return false;
if (other.getTargetArn() != null && other.getTargetArn().equals(this.getTargetArn()) == false)
return false;
if (other.getEndpoint() == null ^ this.getEndpoint() == null)
return false;
if (other.getEndpoint() != null && other.getEndpoint().equals(this.getEndpoint()) == false)
return false;
if (other.getTrackedClusterId() == null ^ this.getTrackedClusterId() == null)
return false;
if (other.getTrackedClusterId() != null && other.getTrackedClusterId().equals(this.getTrackedClusterId()) == false)
return false;
if (other.getRdsResourceId() == null ^ this.getRdsResourceId() == null)
return false;
if (other.getRdsResourceId() != null && other.getRdsResourceId().equals(this.getRdsResourceId()) == false)
return false;
if (other.getPort() == null ^ this.getPort() == null)
return false;
if (other.getPort() != null && other.getPort().equals(this.getPort()) == false)
return false;
if (other.getType() == null ^ this.getType() == null)
return false;
if (other.getType() != null && other.getType().equals(this.getType()) == false)
return false;
if (other.getRole() == null ^ this.getRole() == null)
return false;
if (other.getRole() != null && other.getRole().equals(this.getRole()) == false)
return false;
if (other.getTargetHealth() == null ^ this.getTargetHealth() == null)
return false;
if (other.getTargetHealth() != null && other.getTargetHealth().equals(this.getTargetHealth()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getTargetArn() == null) ? 0 : getTargetArn().hashCode());
hashCode = prime * hashCode + ((getEndpoint() == null) ? 0 : getEndpoint().hashCode());
hashCode = prime * hashCode + ((getTrackedClusterId() == null) ? 0 : getTrackedClusterId().hashCode());
hashCode = prime * hashCode + ((getRdsResourceId() == null) ? 0 : getRdsResourceId().hashCode());
hashCode = prime * hashCode + ((getPort() == null) ? 0 : getPort().hashCode());
hashCode = prime * hashCode + ((getType() == null) ? 0 : getType().hashCode());
hashCode = prime * hashCode + ((getRole() == null) ? 0 : getRole().hashCode());
hashCode = prime * hashCode + ((getTargetHealth() == null) ? 0 : getTargetHealth().hashCode());
return hashCode;
}
@Override
public DBProxyTarget clone() {
try {
return (DBProxyTarget) super.clone();
} catch (CloneNotSupportedException e) {
throw new IllegalStateException("Got a CloneNotSupportedException from Object.clone() " + "even though we're Cloneable!", e);
}
}
}