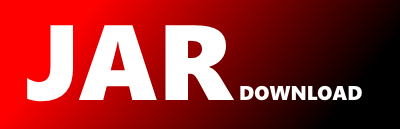
com.amazonaws.services.rds.model.DeleteIntegrationResult Maven / Gradle / Ivy
Show all versions of aws-java-sdk-rds Show documentation
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.rds.model;
import java.io.Serializable;
import javax.annotation.Generated;
/**
*
* A zero-ETL integration with Amazon Redshift.
*
*
* @see AWS API
* Documentation
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class DeleteIntegrationResult extends com.amazonaws.AmazonWebServiceResult implements Serializable, Cloneable {
/**
*
* The Amazon Resource Name (ARN) of the database used as the source for replication.
*
*/
private String sourceArn;
/**
*
* The ARN of the Redshift data warehouse used as the target for replication.
*
*/
private String targetArn;
/**
*
* The name of the integration.
*
*/
private String integrationName;
/**
*
* The ARN of the integration.
*
*/
private String integrationArn;
/**
*
* The Amazon Web Services Key Management System (Amazon Web Services KMS) key identifier for the key used to to
* encrypt the integration.
*
*/
private String kMSKeyId;
/**
*
* The encryption context for the integration. For more information, see Encryption context
* in the Amazon Web Services Key Management Service Developer Guide.
*
*/
private com.amazonaws.internal.SdkInternalMap additionalEncryptionContext;
/**
*
* The current status of the integration.
*
*/
private String status;
private com.amazonaws.internal.SdkInternalList tags;
/**
*
* The time when the integration was created, in Universal Coordinated Time (UTC).
*
*/
private java.util.Date createTime;
/**
*
* Any errors associated with the integration.
*
*/
private com.amazonaws.internal.SdkInternalList errors;
/**
*
* Data filters for the integration. These filters determine which tables from the source database are sent to the
* target Amazon Redshift data warehouse.
*
*/
private String dataFilter;
/**
*
* A description of the integration.
*
*/
private String description;
/**
*
* The Amazon Resource Name (ARN) of the database used as the source for replication.
*
*
* @param sourceArn
* The Amazon Resource Name (ARN) of the database used as the source for replication.
*/
public void setSourceArn(String sourceArn) {
this.sourceArn = sourceArn;
}
/**
*
* The Amazon Resource Name (ARN) of the database used as the source for replication.
*
*
* @return The Amazon Resource Name (ARN) of the database used as the source for replication.
*/
public String getSourceArn() {
return this.sourceArn;
}
/**
*
* The Amazon Resource Name (ARN) of the database used as the source for replication.
*
*
* @param sourceArn
* The Amazon Resource Name (ARN) of the database used as the source for replication.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DeleteIntegrationResult withSourceArn(String sourceArn) {
setSourceArn(sourceArn);
return this;
}
/**
*
* The ARN of the Redshift data warehouse used as the target for replication.
*
*
* @param targetArn
* The ARN of the Redshift data warehouse used as the target for replication.
*/
public void setTargetArn(String targetArn) {
this.targetArn = targetArn;
}
/**
*
* The ARN of the Redshift data warehouse used as the target for replication.
*
*
* @return The ARN of the Redshift data warehouse used as the target for replication.
*/
public String getTargetArn() {
return this.targetArn;
}
/**
*
* The ARN of the Redshift data warehouse used as the target for replication.
*
*
* @param targetArn
* The ARN of the Redshift data warehouse used as the target for replication.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DeleteIntegrationResult withTargetArn(String targetArn) {
setTargetArn(targetArn);
return this;
}
/**
*
* The name of the integration.
*
*
* @param integrationName
* The name of the integration.
*/
public void setIntegrationName(String integrationName) {
this.integrationName = integrationName;
}
/**
*
* The name of the integration.
*
*
* @return The name of the integration.
*/
public String getIntegrationName() {
return this.integrationName;
}
/**
*
* The name of the integration.
*
*
* @param integrationName
* The name of the integration.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DeleteIntegrationResult withIntegrationName(String integrationName) {
setIntegrationName(integrationName);
return this;
}
/**
*
* The ARN of the integration.
*
*
* @param integrationArn
* The ARN of the integration.
*/
public void setIntegrationArn(String integrationArn) {
this.integrationArn = integrationArn;
}
/**
*
* The ARN of the integration.
*
*
* @return The ARN of the integration.
*/
public String getIntegrationArn() {
return this.integrationArn;
}
/**
*
* The ARN of the integration.
*
*
* @param integrationArn
* The ARN of the integration.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DeleteIntegrationResult withIntegrationArn(String integrationArn) {
setIntegrationArn(integrationArn);
return this;
}
/**
*
* The Amazon Web Services Key Management System (Amazon Web Services KMS) key identifier for the key used to to
* encrypt the integration.
*
*
* @param kMSKeyId
* The Amazon Web Services Key Management System (Amazon Web Services KMS) key identifier for the key used to
* to encrypt the integration.
*/
public void setKMSKeyId(String kMSKeyId) {
this.kMSKeyId = kMSKeyId;
}
/**
*
* The Amazon Web Services Key Management System (Amazon Web Services KMS) key identifier for the key used to to
* encrypt the integration.
*
*
* @return The Amazon Web Services Key Management System (Amazon Web Services KMS) key identifier for the key used
* to to encrypt the integration.
*/
public String getKMSKeyId() {
return this.kMSKeyId;
}
/**
*
* The Amazon Web Services Key Management System (Amazon Web Services KMS) key identifier for the key used to to
* encrypt the integration.
*
*
* @param kMSKeyId
* The Amazon Web Services Key Management System (Amazon Web Services KMS) key identifier for the key used to
* to encrypt the integration.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DeleteIntegrationResult withKMSKeyId(String kMSKeyId) {
setKMSKeyId(kMSKeyId);
return this;
}
/**
*
* The encryption context for the integration. For more information, see Encryption context
* in the Amazon Web Services Key Management Service Developer Guide.
*
*
* @return The encryption context for the integration. For more information, see Encryption
* context in the Amazon Web Services Key Management Service Developer Guide.
*/
public java.util.Map getAdditionalEncryptionContext() {
if (additionalEncryptionContext == null) {
additionalEncryptionContext = new com.amazonaws.internal.SdkInternalMap();
}
return additionalEncryptionContext;
}
/**
*
* The encryption context for the integration. For more information, see Encryption context
* in the Amazon Web Services Key Management Service Developer Guide.
*
*
* @param additionalEncryptionContext
* The encryption context for the integration. For more information, see Encryption
* context in the Amazon Web Services Key Management Service Developer Guide.
*/
public void setAdditionalEncryptionContext(java.util.Map additionalEncryptionContext) {
this.additionalEncryptionContext = additionalEncryptionContext == null ? null : new com.amazonaws.internal.SdkInternalMap(
additionalEncryptionContext);
}
/**
*
* The encryption context for the integration. For more information, see Encryption context
* in the Amazon Web Services Key Management Service Developer Guide.
*
*
* @param additionalEncryptionContext
* The encryption context for the integration. For more information, see Encryption
* context in the Amazon Web Services Key Management Service Developer Guide.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DeleteIntegrationResult withAdditionalEncryptionContext(java.util.Map additionalEncryptionContext) {
setAdditionalEncryptionContext(additionalEncryptionContext);
return this;
}
/**
* Add a single AdditionalEncryptionContext entry
*
* @see DeleteIntegrationResult#withAdditionalEncryptionContext
* @returns a reference to this object so that method calls can be chained together.
*/
public DeleteIntegrationResult addAdditionalEncryptionContextEntry(String key, String value) {
if (null == this.additionalEncryptionContext) {
this.additionalEncryptionContext = new com.amazonaws.internal.SdkInternalMap();
}
if (this.additionalEncryptionContext.containsKey(key))
throw new IllegalArgumentException("Duplicated keys (" + key.toString() + ") are provided.");
this.additionalEncryptionContext.put(key, value);
return this;
}
/**
* Removes all the entries added into AdditionalEncryptionContext.
*
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DeleteIntegrationResult clearAdditionalEncryptionContextEntries() {
this.additionalEncryptionContext = null;
return this;
}
/**
*
* The current status of the integration.
*
*
* @param status
* The current status of the integration.
* @see IntegrationStatus
*/
public void setStatus(String status) {
this.status = status;
}
/**
*
* The current status of the integration.
*
*
* @return The current status of the integration.
* @see IntegrationStatus
*/
public String getStatus() {
return this.status;
}
/**
*
* The current status of the integration.
*
*
* @param status
* The current status of the integration.
* @return Returns a reference to this object so that method calls can be chained together.
* @see IntegrationStatus
*/
public DeleteIntegrationResult withStatus(String status) {
setStatus(status);
return this;
}
/**
*
* The current status of the integration.
*
*
* @param status
* The current status of the integration.
* @return Returns a reference to this object so that method calls can be chained together.
* @see IntegrationStatus
*/
public DeleteIntegrationResult withStatus(IntegrationStatus status) {
this.status = status.toString();
return this;
}
/**
* @return
*/
public java.util.List getTags() {
if (tags == null) {
tags = new com.amazonaws.internal.SdkInternalList();
}
return tags;
}
/**
* @param tags
*/
public void setTags(java.util.Collection tags) {
if (tags == null) {
this.tags = null;
return;
}
this.tags = new com.amazonaws.internal.SdkInternalList(tags);
}
/**
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setTags(java.util.Collection)} or {@link #withTags(java.util.Collection)} if you want to override the
* existing values.
*
*
* @param tags
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DeleteIntegrationResult withTags(Tag... tags) {
if (this.tags == null) {
setTags(new com.amazonaws.internal.SdkInternalList(tags.length));
}
for (Tag ele : tags) {
this.tags.add(ele);
}
return this;
}
/**
* @param tags
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DeleteIntegrationResult withTags(java.util.Collection tags) {
setTags(tags);
return this;
}
/**
*
* The time when the integration was created, in Universal Coordinated Time (UTC).
*
*
* @param createTime
* The time when the integration was created, in Universal Coordinated Time (UTC).
*/
public void setCreateTime(java.util.Date createTime) {
this.createTime = createTime;
}
/**
*
* The time when the integration was created, in Universal Coordinated Time (UTC).
*
*
* @return The time when the integration was created, in Universal Coordinated Time (UTC).
*/
public java.util.Date getCreateTime() {
return this.createTime;
}
/**
*
* The time when the integration was created, in Universal Coordinated Time (UTC).
*
*
* @param createTime
* The time when the integration was created, in Universal Coordinated Time (UTC).
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DeleteIntegrationResult withCreateTime(java.util.Date createTime) {
setCreateTime(createTime);
return this;
}
/**
*
* Any errors associated with the integration.
*
*
* @return Any errors associated with the integration.
*/
public java.util.List getErrors() {
if (errors == null) {
errors = new com.amazonaws.internal.SdkInternalList();
}
return errors;
}
/**
*
* Any errors associated with the integration.
*
*
* @param errors
* Any errors associated with the integration.
*/
public void setErrors(java.util.Collection errors) {
if (errors == null) {
this.errors = null;
return;
}
this.errors = new com.amazonaws.internal.SdkInternalList(errors);
}
/**
*
* Any errors associated with the integration.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setErrors(java.util.Collection)} or {@link #withErrors(java.util.Collection)} if you want to override the
* existing values.
*
*
* @param errors
* Any errors associated with the integration.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DeleteIntegrationResult withErrors(IntegrationError... errors) {
if (this.errors == null) {
setErrors(new com.amazonaws.internal.SdkInternalList(errors.length));
}
for (IntegrationError ele : errors) {
this.errors.add(ele);
}
return this;
}
/**
*
* Any errors associated with the integration.
*
*
* @param errors
* Any errors associated with the integration.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DeleteIntegrationResult withErrors(java.util.Collection errors) {
setErrors(errors);
return this;
}
/**
*
* Data filters for the integration. These filters determine which tables from the source database are sent to the
* target Amazon Redshift data warehouse.
*
*
* @param dataFilter
* Data filters for the integration. These filters determine which tables from the source database are sent
* to the target Amazon Redshift data warehouse.
*/
public void setDataFilter(String dataFilter) {
this.dataFilter = dataFilter;
}
/**
*
* Data filters for the integration. These filters determine which tables from the source database are sent to the
* target Amazon Redshift data warehouse.
*
*
* @return Data filters for the integration. These filters determine which tables from the source database are sent
* to the target Amazon Redshift data warehouse.
*/
public String getDataFilter() {
return this.dataFilter;
}
/**
*
* Data filters for the integration. These filters determine which tables from the source database are sent to the
* target Amazon Redshift data warehouse.
*
*
* @param dataFilter
* Data filters for the integration. These filters determine which tables from the source database are sent
* to the target Amazon Redshift data warehouse.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DeleteIntegrationResult withDataFilter(String dataFilter) {
setDataFilter(dataFilter);
return this;
}
/**
*
* A description of the integration.
*
*
* @param description
* A description of the integration.
*/
public void setDescription(String description) {
this.description = description;
}
/**
*
* A description of the integration.
*
*
* @return A description of the integration.
*/
public String getDescription() {
return this.description;
}
/**
*
* A description of the integration.
*
*
* @param description
* A description of the integration.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DeleteIntegrationResult withDescription(String description) {
setDescription(description);
return this;
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getSourceArn() != null)
sb.append("SourceArn: ").append(getSourceArn()).append(",");
if (getTargetArn() != null)
sb.append("TargetArn: ").append(getTargetArn()).append(",");
if (getIntegrationName() != null)
sb.append("IntegrationName: ").append(getIntegrationName()).append(",");
if (getIntegrationArn() != null)
sb.append("IntegrationArn: ").append(getIntegrationArn()).append(",");
if (getKMSKeyId() != null)
sb.append("KMSKeyId: ").append(getKMSKeyId()).append(",");
if (getAdditionalEncryptionContext() != null)
sb.append("AdditionalEncryptionContext: ").append(getAdditionalEncryptionContext()).append(",");
if (getStatus() != null)
sb.append("Status: ").append(getStatus()).append(",");
if (getTags() != null)
sb.append("Tags: ").append(getTags()).append(",");
if (getCreateTime() != null)
sb.append("CreateTime: ").append(getCreateTime()).append(",");
if (getErrors() != null)
sb.append("Errors: ").append(getErrors()).append(",");
if (getDataFilter() != null)
sb.append("DataFilter: ").append(getDataFilter()).append(",");
if (getDescription() != null)
sb.append("Description: ").append(getDescription());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof DeleteIntegrationResult == false)
return false;
DeleteIntegrationResult other = (DeleteIntegrationResult) obj;
if (other.getSourceArn() == null ^ this.getSourceArn() == null)
return false;
if (other.getSourceArn() != null && other.getSourceArn().equals(this.getSourceArn()) == false)
return false;
if (other.getTargetArn() == null ^ this.getTargetArn() == null)
return false;
if (other.getTargetArn() != null && other.getTargetArn().equals(this.getTargetArn()) == false)
return false;
if (other.getIntegrationName() == null ^ this.getIntegrationName() == null)
return false;
if (other.getIntegrationName() != null && other.getIntegrationName().equals(this.getIntegrationName()) == false)
return false;
if (other.getIntegrationArn() == null ^ this.getIntegrationArn() == null)
return false;
if (other.getIntegrationArn() != null && other.getIntegrationArn().equals(this.getIntegrationArn()) == false)
return false;
if (other.getKMSKeyId() == null ^ this.getKMSKeyId() == null)
return false;
if (other.getKMSKeyId() != null && other.getKMSKeyId().equals(this.getKMSKeyId()) == false)
return false;
if (other.getAdditionalEncryptionContext() == null ^ this.getAdditionalEncryptionContext() == null)
return false;
if (other.getAdditionalEncryptionContext() != null && other.getAdditionalEncryptionContext().equals(this.getAdditionalEncryptionContext()) == false)
return false;
if (other.getStatus() == null ^ this.getStatus() == null)
return false;
if (other.getStatus() != null && other.getStatus().equals(this.getStatus()) == false)
return false;
if (other.getTags() == null ^ this.getTags() == null)
return false;
if (other.getTags() != null && other.getTags().equals(this.getTags()) == false)
return false;
if (other.getCreateTime() == null ^ this.getCreateTime() == null)
return false;
if (other.getCreateTime() != null && other.getCreateTime().equals(this.getCreateTime()) == false)
return false;
if (other.getErrors() == null ^ this.getErrors() == null)
return false;
if (other.getErrors() != null && other.getErrors().equals(this.getErrors()) == false)
return false;
if (other.getDataFilter() == null ^ this.getDataFilter() == null)
return false;
if (other.getDataFilter() != null && other.getDataFilter().equals(this.getDataFilter()) == false)
return false;
if (other.getDescription() == null ^ this.getDescription() == null)
return false;
if (other.getDescription() != null && other.getDescription().equals(this.getDescription()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getSourceArn() == null) ? 0 : getSourceArn().hashCode());
hashCode = prime * hashCode + ((getTargetArn() == null) ? 0 : getTargetArn().hashCode());
hashCode = prime * hashCode + ((getIntegrationName() == null) ? 0 : getIntegrationName().hashCode());
hashCode = prime * hashCode + ((getIntegrationArn() == null) ? 0 : getIntegrationArn().hashCode());
hashCode = prime * hashCode + ((getKMSKeyId() == null) ? 0 : getKMSKeyId().hashCode());
hashCode = prime * hashCode + ((getAdditionalEncryptionContext() == null) ? 0 : getAdditionalEncryptionContext().hashCode());
hashCode = prime * hashCode + ((getStatus() == null) ? 0 : getStatus().hashCode());
hashCode = prime * hashCode + ((getTags() == null) ? 0 : getTags().hashCode());
hashCode = prime * hashCode + ((getCreateTime() == null) ? 0 : getCreateTime().hashCode());
hashCode = prime * hashCode + ((getErrors() == null) ? 0 : getErrors().hashCode());
hashCode = prime * hashCode + ((getDataFilter() == null) ? 0 : getDataFilter().hashCode());
hashCode = prime * hashCode + ((getDescription() == null) ? 0 : getDescription().hashCode());
return hashCode;
}
@Override
public DeleteIntegrationResult clone() {
try {
return (DeleteIntegrationResult) super.clone();
} catch (CloneNotSupportedException e) {
throw new IllegalStateException("Got a CloneNotSupportedException from Object.clone() " + "even though we're Cloneable!", e);
}
}
}