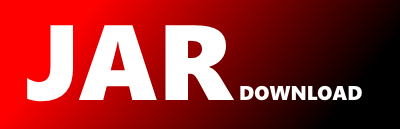
com.amazonaws.services.rds.model.ModifyCustomDBEngineVersionResult Maven / Gradle / Ivy
Show all versions of aws-java-sdk-rds Show documentation
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.rds.model;
import java.io.Serializable;
import javax.annotation.Generated;
/**
*
* This data type is used as a response element in the action DescribeDBEngineVersions
.
*
*
* @see AWS
* API Documentation
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class ModifyCustomDBEngineVersionResult extends com.amazonaws.AmazonWebServiceResult implements Serializable, Cloneable {
/**
*
* The name of the database engine.
*
*/
private String engine;
/**
*
* The version number of the database engine.
*
*/
private String engineVersion;
/**
*
* The name of the DB parameter group family for the database engine.
*
*/
private String dBParameterGroupFamily;
/**
*
* The description of the database engine.
*
*/
private String dBEngineDescription;
/**
*
* The description of the database engine version.
*
*/
private String dBEngineVersionDescription;
/**
*
* The default character set for new instances of this engine version, if the CharacterSetName
* parameter of the CreateDBInstance API isn't specified.
*
*/
private CharacterSet defaultCharacterSet;
/**
*
* The EC2 image
*
*/
private CustomDBEngineVersionAMI image;
/**
*
* A value that indicates the source media provider of the AMI based on the usage operation. Applicable for RDS
* Custom for SQL Server.
*
*/
private String dBEngineMediaType;
/**
*
* A list of the character sets supported by this engine for the CharacterSetName
parameter of the
* CreateDBInstance
operation.
*
*/
private com.amazonaws.internal.SdkInternalList supportedCharacterSets;
/**
*
* A list of the character sets supported by the Oracle DB engine for the NcharCharacterSetName
* parameter of the CreateDBInstance
operation.
*
*/
private com.amazonaws.internal.SdkInternalList supportedNcharCharacterSets;
/**
*
* A list of engine versions that this database engine version can be upgraded to.
*
*/
private com.amazonaws.internal.SdkInternalList validUpgradeTarget;
/**
*
* A list of the time zones supported by this engine for the Timezone
parameter of the
* CreateDBInstance
action.
*
*/
private com.amazonaws.internal.SdkInternalList supportedTimezones;
/**
*
* The types of logs that the database engine has available for export to CloudWatch Logs.
*
*/
private com.amazonaws.internal.SdkInternalList exportableLogTypes;
/**
*
* Indicates whether the engine version supports exporting the log types specified by ExportableLogTypes to
* CloudWatch Logs.
*
*/
private Boolean supportsLogExportsToCloudwatchLogs;
/**
*
* Indicates whether the database engine version supports read replicas.
*
*/
private Boolean supportsReadReplica;
/**
*
* A list of the supported DB engine modes.
*
*/
private com.amazonaws.internal.SdkInternalList supportedEngineModes;
/**
*
* A list of features supported by the DB engine.
*
*
* The supported features vary by DB engine and DB engine version.
*
*
* To determine the supported features for a specific DB engine and DB engine version using the CLI, use the
* following command:
*
*
* aws rds describe-db-engine-versions --engine <engine_name> --engine-version <engine_version>
*
*
* For example, to determine the supported features for RDS for PostgreSQL version 13.3 using the CLI, use the
* following command:
*
*
* aws rds describe-db-engine-versions --engine postgres --engine-version 13.3
*
*
* The supported features are listed under SupportedFeatureNames
in the output.
*
*/
private com.amazonaws.internal.SdkInternalList supportedFeatureNames;
/**
*
* The status of the DB engine version, either available
or deprecated
.
*
*/
private String status;
/**
*
* Indicates whether you can use Aurora parallel query with a specific DB engine version.
*
*/
private Boolean supportsParallelQuery;
/**
*
* Indicates whether you can use Aurora global databases with a specific DB engine version.
*
*/
private Boolean supportsGlobalDatabases;
/**
*
* The major engine version of the CEV.
*
*/
private String majorEngineVersion;
/**
*
* The name of the Amazon S3 bucket that contains your database installation files.
*
*/
private String databaseInstallationFilesS3BucketName;
/**
*
* The Amazon S3 directory that contains the database installation files. If not specified, then no prefix is
* assumed.
*
*/
private String databaseInstallationFilesS3Prefix;
/**
*
* The ARN of the custom engine version.
*
*/
private String dBEngineVersionArn;
/**
*
* The Amazon Web Services KMS key identifier for an encrypted CEV. This parameter is required for RDS Custom, but
* optional for Amazon RDS.
*
*/
private String kMSKeyId;
/**
*
* The creation time of the DB engine version.
*
*/
private java.util.Date createTime;
private com.amazonaws.internal.SdkInternalList tagList;
/**
*
* Indicates whether the engine version supports Babelfish for Aurora PostgreSQL.
*
*/
private Boolean supportsBabelfish;
/**
*
* JSON string that lists the installation files and parameters that RDS Custom uses to create a custom engine
* version (CEV). RDS Custom applies the patches in the order in which they're listed in the manifest. You can set
* the Oracle home, Oracle base, and UNIX/Linux user and group using the installation parameters. For more
* information, see JSON fields in the CEV manifest in the Amazon RDS User Guide.
*
*/
private String customDBEngineVersionManifest;
/**
*
* Indicates whether the DB engine version supports Aurora Limitless Database.
*
*/
private Boolean supportsLimitlessDatabase;
/**
*
* Indicates whether the engine version supports rotating the server certificate without rebooting the DB instance.
*
*/
private Boolean supportsCertificateRotationWithoutRestart;
/**
*
* A list of the supported CA certificate identifiers.
*
*
* For more information, see Using SSL/TLS to encrypt a
* connection to a DB instance in the Amazon RDS User Guide and Using SSL/TLS to
* encrypt a connection to a DB cluster in the Amazon Aurora User Guide.
*
*/
private com.amazonaws.internal.SdkInternalList supportedCACertificateIdentifiers;
/**
*
* Indicates whether the DB engine version supports forwarding write operations from reader DB instances to the
* writer DB instance in the DB cluster. By default, write operations aren't allowed on reader DB instances.
*
*
* Valid for: Aurora DB clusters only
*
*/
private Boolean supportsLocalWriteForwarding;
/**
*
* Indicates whether the DB engine version supports zero-ETL integrations with Amazon Redshift.
*
*/
private Boolean supportsIntegrations;
/**
*
* The name of the database engine.
*
*
* @param engine
* The name of the database engine.
*/
public void setEngine(String engine) {
this.engine = engine;
}
/**
*
* The name of the database engine.
*
*
* @return The name of the database engine.
*/
public String getEngine() {
return this.engine;
}
/**
*
* The name of the database engine.
*
*
* @param engine
* The name of the database engine.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ModifyCustomDBEngineVersionResult withEngine(String engine) {
setEngine(engine);
return this;
}
/**
*
* The version number of the database engine.
*
*
* @param engineVersion
* The version number of the database engine.
*/
public void setEngineVersion(String engineVersion) {
this.engineVersion = engineVersion;
}
/**
*
* The version number of the database engine.
*
*
* @return The version number of the database engine.
*/
public String getEngineVersion() {
return this.engineVersion;
}
/**
*
* The version number of the database engine.
*
*
* @param engineVersion
* The version number of the database engine.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ModifyCustomDBEngineVersionResult withEngineVersion(String engineVersion) {
setEngineVersion(engineVersion);
return this;
}
/**
*
* The name of the DB parameter group family for the database engine.
*
*
* @param dBParameterGroupFamily
* The name of the DB parameter group family for the database engine.
*/
public void setDBParameterGroupFamily(String dBParameterGroupFamily) {
this.dBParameterGroupFamily = dBParameterGroupFamily;
}
/**
*
* The name of the DB parameter group family for the database engine.
*
*
* @return The name of the DB parameter group family for the database engine.
*/
public String getDBParameterGroupFamily() {
return this.dBParameterGroupFamily;
}
/**
*
* The name of the DB parameter group family for the database engine.
*
*
* @param dBParameterGroupFamily
* The name of the DB parameter group family for the database engine.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ModifyCustomDBEngineVersionResult withDBParameterGroupFamily(String dBParameterGroupFamily) {
setDBParameterGroupFamily(dBParameterGroupFamily);
return this;
}
/**
*
* The description of the database engine.
*
*
* @param dBEngineDescription
* The description of the database engine.
*/
public void setDBEngineDescription(String dBEngineDescription) {
this.dBEngineDescription = dBEngineDescription;
}
/**
*
* The description of the database engine.
*
*
* @return The description of the database engine.
*/
public String getDBEngineDescription() {
return this.dBEngineDescription;
}
/**
*
* The description of the database engine.
*
*
* @param dBEngineDescription
* The description of the database engine.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ModifyCustomDBEngineVersionResult withDBEngineDescription(String dBEngineDescription) {
setDBEngineDescription(dBEngineDescription);
return this;
}
/**
*
* The description of the database engine version.
*
*
* @param dBEngineVersionDescription
* The description of the database engine version.
*/
public void setDBEngineVersionDescription(String dBEngineVersionDescription) {
this.dBEngineVersionDescription = dBEngineVersionDescription;
}
/**
*
* The description of the database engine version.
*
*
* @return The description of the database engine version.
*/
public String getDBEngineVersionDescription() {
return this.dBEngineVersionDescription;
}
/**
*
* The description of the database engine version.
*
*
* @param dBEngineVersionDescription
* The description of the database engine version.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ModifyCustomDBEngineVersionResult withDBEngineVersionDescription(String dBEngineVersionDescription) {
setDBEngineVersionDescription(dBEngineVersionDescription);
return this;
}
/**
*
* The default character set for new instances of this engine version, if the CharacterSetName
* parameter of the CreateDBInstance API isn't specified.
*
*
* @param defaultCharacterSet
* The default character set for new instances of this engine version, if the CharacterSetName
* parameter of the CreateDBInstance API isn't specified.
*/
public void setDefaultCharacterSet(CharacterSet defaultCharacterSet) {
this.defaultCharacterSet = defaultCharacterSet;
}
/**
*
* The default character set for new instances of this engine version, if the CharacterSetName
* parameter of the CreateDBInstance API isn't specified.
*
*
* @return The default character set for new instances of this engine version, if the CharacterSetName
* parameter of the CreateDBInstance API isn't specified.
*/
public CharacterSet getDefaultCharacterSet() {
return this.defaultCharacterSet;
}
/**
*
* The default character set for new instances of this engine version, if the CharacterSetName
* parameter of the CreateDBInstance API isn't specified.
*
*
* @param defaultCharacterSet
* The default character set for new instances of this engine version, if the CharacterSetName
* parameter of the CreateDBInstance API isn't specified.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ModifyCustomDBEngineVersionResult withDefaultCharacterSet(CharacterSet defaultCharacterSet) {
setDefaultCharacterSet(defaultCharacterSet);
return this;
}
/**
*
* The EC2 image
*
*
* @param image
* The EC2 image
*/
public void setImage(CustomDBEngineVersionAMI image) {
this.image = image;
}
/**
*
* The EC2 image
*
*
* @return The EC2 image
*/
public CustomDBEngineVersionAMI getImage() {
return this.image;
}
/**
*
* The EC2 image
*
*
* @param image
* The EC2 image
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ModifyCustomDBEngineVersionResult withImage(CustomDBEngineVersionAMI image) {
setImage(image);
return this;
}
/**
*
* A value that indicates the source media provider of the AMI based on the usage operation. Applicable for RDS
* Custom for SQL Server.
*
*
* @param dBEngineMediaType
* A value that indicates the source media provider of the AMI based on the usage operation. Applicable for
* RDS Custom for SQL Server.
*/
public void setDBEngineMediaType(String dBEngineMediaType) {
this.dBEngineMediaType = dBEngineMediaType;
}
/**
*
* A value that indicates the source media provider of the AMI based on the usage operation. Applicable for RDS
* Custom for SQL Server.
*
*
* @return A value that indicates the source media provider of the AMI based on the usage operation. Applicable for
* RDS Custom for SQL Server.
*/
public String getDBEngineMediaType() {
return this.dBEngineMediaType;
}
/**
*
* A value that indicates the source media provider of the AMI based on the usage operation. Applicable for RDS
* Custom for SQL Server.
*
*
* @param dBEngineMediaType
* A value that indicates the source media provider of the AMI based on the usage operation. Applicable for
* RDS Custom for SQL Server.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ModifyCustomDBEngineVersionResult withDBEngineMediaType(String dBEngineMediaType) {
setDBEngineMediaType(dBEngineMediaType);
return this;
}
/**
*
* A list of the character sets supported by this engine for the CharacterSetName
parameter of the
* CreateDBInstance
operation.
*
*
* @return A list of the character sets supported by this engine for the CharacterSetName
parameter of
* the CreateDBInstance
operation.
*/
public java.util.List getSupportedCharacterSets() {
if (supportedCharacterSets == null) {
supportedCharacterSets = new com.amazonaws.internal.SdkInternalList();
}
return supportedCharacterSets;
}
/**
*
* A list of the character sets supported by this engine for the CharacterSetName
parameter of the
* CreateDBInstance
operation.
*
*
* @param supportedCharacterSets
* A list of the character sets supported by this engine for the CharacterSetName
parameter of
* the CreateDBInstance
operation.
*/
public void setSupportedCharacterSets(java.util.Collection supportedCharacterSets) {
if (supportedCharacterSets == null) {
this.supportedCharacterSets = null;
return;
}
this.supportedCharacterSets = new com.amazonaws.internal.SdkInternalList(supportedCharacterSets);
}
/**
*
* A list of the character sets supported by this engine for the CharacterSetName
parameter of the
* CreateDBInstance
operation.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setSupportedCharacterSets(java.util.Collection)} or
* {@link #withSupportedCharacterSets(java.util.Collection)} if you want to override the existing values.
*
*
* @param supportedCharacterSets
* A list of the character sets supported by this engine for the CharacterSetName
parameter of
* the CreateDBInstance
operation.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ModifyCustomDBEngineVersionResult withSupportedCharacterSets(CharacterSet... supportedCharacterSets) {
if (this.supportedCharacterSets == null) {
setSupportedCharacterSets(new com.amazonaws.internal.SdkInternalList(supportedCharacterSets.length));
}
for (CharacterSet ele : supportedCharacterSets) {
this.supportedCharacterSets.add(ele);
}
return this;
}
/**
*
* A list of the character sets supported by this engine for the CharacterSetName
parameter of the
* CreateDBInstance
operation.
*
*
* @param supportedCharacterSets
* A list of the character sets supported by this engine for the CharacterSetName
parameter of
* the CreateDBInstance
operation.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ModifyCustomDBEngineVersionResult withSupportedCharacterSets(java.util.Collection supportedCharacterSets) {
setSupportedCharacterSets(supportedCharacterSets);
return this;
}
/**
*
* A list of the character sets supported by the Oracle DB engine for the NcharCharacterSetName
* parameter of the CreateDBInstance
operation.
*
*
* @return A list of the character sets supported by the Oracle DB engine for the NcharCharacterSetName
* parameter of the CreateDBInstance
operation.
*/
public java.util.List getSupportedNcharCharacterSets() {
if (supportedNcharCharacterSets == null) {
supportedNcharCharacterSets = new com.amazonaws.internal.SdkInternalList();
}
return supportedNcharCharacterSets;
}
/**
*
* A list of the character sets supported by the Oracle DB engine for the NcharCharacterSetName
* parameter of the CreateDBInstance
operation.
*
*
* @param supportedNcharCharacterSets
* A list of the character sets supported by the Oracle DB engine for the NcharCharacterSetName
* parameter of the CreateDBInstance
operation.
*/
public void setSupportedNcharCharacterSets(java.util.Collection supportedNcharCharacterSets) {
if (supportedNcharCharacterSets == null) {
this.supportedNcharCharacterSets = null;
return;
}
this.supportedNcharCharacterSets = new com.amazonaws.internal.SdkInternalList(supportedNcharCharacterSets);
}
/**
*
* A list of the character sets supported by the Oracle DB engine for the NcharCharacterSetName
* parameter of the CreateDBInstance
operation.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setSupportedNcharCharacterSets(java.util.Collection)} or
* {@link #withSupportedNcharCharacterSets(java.util.Collection)} if you want to override the existing values.
*
*
* @param supportedNcharCharacterSets
* A list of the character sets supported by the Oracle DB engine for the NcharCharacterSetName
* parameter of the CreateDBInstance
operation.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ModifyCustomDBEngineVersionResult withSupportedNcharCharacterSets(CharacterSet... supportedNcharCharacterSets) {
if (this.supportedNcharCharacterSets == null) {
setSupportedNcharCharacterSets(new com.amazonaws.internal.SdkInternalList(supportedNcharCharacterSets.length));
}
for (CharacterSet ele : supportedNcharCharacterSets) {
this.supportedNcharCharacterSets.add(ele);
}
return this;
}
/**
*
* A list of the character sets supported by the Oracle DB engine for the NcharCharacterSetName
* parameter of the CreateDBInstance
operation.
*
*
* @param supportedNcharCharacterSets
* A list of the character sets supported by the Oracle DB engine for the NcharCharacterSetName
* parameter of the CreateDBInstance
operation.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ModifyCustomDBEngineVersionResult withSupportedNcharCharacterSets(java.util.Collection supportedNcharCharacterSets) {
setSupportedNcharCharacterSets(supportedNcharCharacterSets);
return this;
}
/**
*
* A list of engine versions that this database engine version can be upgraded to.
*
*
* @return A list of engine versions that this database engine version can be upgraded to.
*/
public java.util.List getValidUpgradeTarget() {
if (validUpgradeTarget == null) {
validUpgradeTarget = new com.amazonaws.internal.SdkInternalList();
}
return validUpgradeTarget;
}
/**
*
* A list of engine versions that this database engine version can be upgraded to.
*
*
* @param validUpgradeTarget
* A list of engine versions that this database engine version can be upgraded to.
*/
public void setValidUpgradeTarget(java.util.Collection validUpgradeTarget) {
if (validUpgradeTarget == null) {
this.validUpgradeTarget = null;
return;
}
this.validUpgradeTarget = new com.amazonaws.internal.SdkInternalList(validUpgradeTarget);
}
/**
*
* A list of engine versions that this database engine version can be upgraded to.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setValidUpgradeTarget(java.util.Collection)} or {@link #withValidUpgradeTarget(java.util.Collection)} if
* you want to override the existing values.
*
*
* @param validUpgradeTarget
* A list of engine versions that this database engine version can be upgraded to.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ModifyCustomDBEngineVersionResult withValidUpgradeTarget(UpgradeTarget... validUpgradeTarget) {
if (this.validUpgradeTarget == null) {
setValidUpgradeTarget(new com.amazonaws.internal.SdkInternalList(validUpgradeTarget.length));
}
for (UpgradeTarget ele : validUpgradeTarget) {
this.validUpgradeTarget.add(ele);
}
return this;
}
/**
*
* A list of engine versions that this database engine version can be upgraded to.
*
*
* @param validUpgradeTarget
* A list of engine versions that this database engine version can be upgraded to.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ModifyCustomDBEngineVersionResult withValidUpgradeTarget(java.util.Collection validUpgradeTarget) {
setValidUpgradeTarget(validUpgradeTarget);
return this;
}
/**
*
* A list of the time zones supported by this engine for the Timezone
parameter of the
* CreateDBInstance
action.
*
*
* @return A list of the time zones supported by this engine for the Timezone
parameter of the
* CreateDBInstance
action.
*/
public java.util.List getSupportedTimezones() {
if (supportedTimezones == null) {
supportedTimezones = new com.amazonaws.internal.SdkInternalList();
}
return supportedTimezones;
}
/**
*
* A list of the time zones supported by this engine for the Timezone
parameter of the
* CreateDBInstance
action.
*
*
* @param supportedTimezones
* A list of the time zones supported by this engine for the Timezone
parameter of the
* CreateDBInstance
action.
*/
public void setSupportedTimezones(java.util.Collection supportedTimezones) {
if (supportedTimezones == null) {
this.supportedTimezones = null;
return;
}
this.supportedTimezones = new com.amazonaws.internal.SdkInternalList(supportedTimezones);
}
/**
*
* A list of the time zones supported by this engine for the Timezone
parameter of the
* CreateDBInstance
action.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setSupportedTimezones(java.util.Collection)} or {@link #withSupportedTimezones(java.util.Collection)} if
* you want to override the existing values.
*
*
* @param supportedTimezones
* A list of the time zones supported by this engine for the Timezone
parameter of the
* CreateDBInstance
action.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ModifyCustomDBEngineVersionResult withSupportedTimezones(Timezone... supportedTimezones) {
if (this.supportedTimezones == null) {
setSupportedTimezones(new com.amazonaws.internal.SdkInternalList(supportedTimezones.length));
}
for (Timezone ele : supportedTimezones) {
this.supportedTimezones.add(ele);
}
return this;
}
/**
*
* A list of the time zones supported by this engine for the Timezone
parameter of the
* CreateDBInstance
action.
*
*
* @param supportedTimezones
* A list of the time zones supported by this engine for the Timezone
parameter of the
* CreateDBInstance
action.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ModifyCustomDBEngineVersionResult withSupportedTimezones(java.util.Collection supportedTimezones) {
setSupportedTimezones(supportedTimezones);
return this;
}
/**
*
* The types of logs that the database engine has available for export to CloudWatch Logs.
*
*
* @return The types of logs that the database engine has available for export to CloudWatch Logs.
*/
public java.util.List getExportableLogTypes() {
if (exportableLogTypes == null) {
exportableLogTypes = new com.amazonaws.internal.SdkInternalList();
}
return exportableLogTypes;
}
/**
*
* The types of logs that the database engine has available for export to CloudWatch Logs.
*
*
* @param exportableLogTypes
* The types of logs that the database engine has available for export to CloudWatch Logs.
*/
public void setExportableLogTypes(java.util.Collection exportableLogTypes) {
if (exportableLogTypes == null) {
this.exportableLogTypes = null;
return;
}
this.exportableLogTypes = new com.amazonaws.internal.SdkInternalList(exportableLogTypes);
}
/**
*
* The types of logs that the database engine has available for export to CloudWatch Logs.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setExportableLogTypes(java.util.Collection)} or {@link #withExportableLogTypes(java.util.Collection)} if
* you want to override the existing values.
*
*
* @param exportableLogTypes
* The types of logs that the database engine has available for export to CloudWatch Logs.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ModifyCustomDBEngineVersionResult withExportableLogTypes(String... exportableLogTypes) {
if (this.exportableLogTypes == null) {
setExportableLogTypes(new com.amazonaws.internal.SdkInternalList(exportableLogTypes.length));
}
for (String ele : exportableLogTypes) {
this.exportableLogTypes.add(ele);
}
return this;
}
/**
*
* The types of logs that the database engine has available for export to CloudWatch Logs.
*
*
* @param exportableLogTypes
* The types of logs that the database engine has available for export to CloudWatch Logs.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ModifyCustomDBEngineVersionResult withExportableLogTypes(java.util.Collection exportableLogTypes) {
setExportableLogTypes(exportableLogTypes);
return this;
}
/**
*
* Indicates whether the engine version supports exporting the log types specified by ExportableLogTypes to
* CloudWatch Logs.
*
*
* @param supportsLogExportsToCloudwatchLogs
* Indicates whether the engine version supports exporting the log types specified by ExportableLogTypes to
* CloudWatch Logs.
*/
public void setSupportsLogExportsToCloudwatchLogs(Boolean supportsLogExportsToCloudwatchLogs) {
this.supportsLogExportsToCloudwatchLogs = supportsLogExportsToCloudwatchLogs;
}
/**
*
* Indicates whether the engine version supports exporting the log types specified by ExportableLogTypes to
* CloudWatch Logs.
*
*
* @return Indicates whether the engine version supports exporting the log types specified by ExportableLogTypes to
* CloudWatch Logs.
*/
public Boolean getSupportsLogExportsToCloudwatchLogs() {
return this.supportsLogExportsToCloudwatchLogs;
}
/**
*
* Indicates whether the engine version supports exporting the log types specified by ExportableLogTypes to
* CloudWatch Logs.
*
*
* @param supportsLogExportsToCloudwatchLogs
* Indicates whether the engine version supports exporting the log types specified by ExportableLogTypes to
* CloudWatch Logs.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ModifyCustomDBEngineVersionResult withSupportsLogExportsToCloudwatchLogs(Boolean supportsLogExportsToCloudwatchLogs) {
setSupportsLogExportsToCloudwatchLogs(supportsLogExportsToCloudwatchLogs);
return this;
}
/**
*
* Indicates whether the engine version supports exporting the log types specified by ExportableLogTypes to
* CloudWatch Logs.
*
*
* @return Indicates whether the engine version supports exporting the log types specified by ExportableLogTypes to
* CloudWatch Logs.
*/
public Boolean isSupportsLogExportsToCloudwatchLogs() {
return this.supportsLogExportsToCloudwatchLogs;
}
/**
*
* Indicates whether the database engine version supports read replicas.
*
*
* @param supportsReadReplica
* Indicates whether the database engine version supports read replicas.
*/
public void setSupportsReadReplica(Boolean supportsReadReplica) {
this.supportsReadReplica = supportsReadReplica;
}
/**
*
* Indicates whether the database engine version supports read replicas.
*
*
* @return Indicates whether the database engine version supports read replicas.
*/
public Boolean getSupportsReadReplica() {
return this.supportsReadReplica;
}
/**
*
* Indicates whether the database engine version supports read replicas.
*
*
* @param supportsReadReplica
* Indicates whether the database engine version supports read replicas.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ModifyCustomDBEngineVersionResult withSupportsReadReplica(Boolean supportsReadReplica) {
setSupportsReadReplica(supportsReadReplica);
return this;
}
/**
*
* Indicates whether the database engine version supports read replicas.
*
*
* @return Indicates whether the database engine version supports read replicas.
*/
public Boolean isSupportsReadReplica() {
return this.supportsReadReplica;
}
/**
*
* A list of the supported DB engine modes.
*
*
* @return A list of the supported DB engine modes.
*/
public java.util.List getSupportedEngineModes() {
if (supportedEngineModes == null) {
supportedEngineModes = new com.amazonaws.internal.SdkInternalList();
}
return supportedEngineModes;
}
/**
*
* A list of the supported DB engine modes.
*
*
* @param supportedEngineModes
* A list of the supported DB engine modes.
*/
public void setSupportedEngineModes(java.util.Collection supportedEngineModes) {
if (supportedEngineModes == null) {
this.supportedEngineModes = null;
return;
}
this.supportedEngineModes = new com.amazonaws.internal.SdkInternalList(supportedEngineModes);
}
/**
*
* A list of the supported DB engine modes.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setSupportedEngineModes(java.util.Collection)} or {@link #withSupportedEngineModes(java.util.Collection)}
* if you want to override the existing values.
*
*
* @param supportedEngineModes
* A list of the supported DB engine modes.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ModifyCustomDBEngineVersionResult withSupportedEngineModes(String... supportedEngineModes) {
if (this.supportedEngineModes == null) {
setSupportedEngineModes(new com.amazonaws.internal.SdkInternalList(supportedEngineModes.length));
}
for (String ele : supportedEngineModes) {
this.supportedEngineModes.add(ele);
}
return this;
}
/**
*
* A list of the supported DB engine modes.
*
*
* @param supportedEngineModes
* A list of the supported DB engine modes.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ModifyCustomDBEngineVersionResult withSupportedEngineModes(java.util.Collection supportedEngineModes) {
setSupportedEngineModes(supportedEngineModes);
return this;
}
/**
*
* A list of features supported by the DB engine.
*
*
* The supported features vary by DB engine and DB engine version.
*
*
* To determine the supported features for a specific DB engine and DB engine version using the CLI, use the
* following command:
*
*
* aws rds describe-db-engine-versions --engine <engine_name> --engine-version <engine_version>
*
*
* For example, to determine the supported features for RDS for PostgreSQL version 13.3 using the CLI, use the
* following command:
*
*
* aws rds describe-db-engine-versions --engine postgres --engine-version 13.3
*
*
* The supported features are listed under SupportedFeatureNames
in the output.
*
*
* @return A list of features supported by the DB engine.
*
* The supported features vary by DB engine and DB engine version.
*
*
* To determine the supported features for a specific DB engine and DB engine version using the CLI, use the
* following command:
*
*
* aws rds describe-db-engine-versions --engine <engine_name> --engine-version <engine_version>
*
*
* For example, to determine the supported features for RDS for PostgreSQL version 13.3 using the CLI, use
* the following command:
*
*
* aws rds describe-db-engine-versions --engine postgres --engine-version 13.3
*
*
* The supported features are listed under SupportedFeatureNames
in the output.
*/
public java.util.List getSupportedFeatureNames() {
if (supportedFeatureNames == null) {
supportedFeatureNames = new com.amazonaws.internal.SdkInternalList();
}
return supportedFeatureNames;
}
/**
*
* A list of features supported by the DB engine.
*
*
* The supported features vary by DB engine and DB engine version.
*
*
* To determine the supported features for a specific DB engine and DB engine version using the CLI, use the
* following command:
*
*
* aws rds describe-db-engine-versions --engine <engine_name> --engine-version <engine_version>
*
*
* For example, to determine the supported features for RDS for PostgreSQL version 13.3 using the CLI, use the
* following command:
*
*
* aws rds describe-db-engine-versions --engine postgres --engine-version 13.3
*
*
* The supported features are listed under SupportedFeatureNames
in the output.
*
*
* @param supportedFeatureNames
* A list of features supported by the DB engine.
*
* The supported features vary by DB engine and DB engine version.
*
*
* To determine the supported features for a specific DB engine and DB engine version using the CLI, use the
* following command:
*
*
* aws rds describe-db-engine-versions --engine <engine_name> --engine-version <engine_version>
*
*
* For example, to determine the supported features for RDS for PostgreSQL version 13.3 using the CLI, use
* the following command:
*
*
* aws rds describe-db-engine-versions --engine postgres --engine-version 13.3
*
*
* The supported features are listed under SupportedFeatureNames
in the output.
*/
public void setSupportedFeatureNames(java.util.Collection supportedFeatureNames) {
if (supportedFeatureNames == null) {
this.supportedFeatureNames = null;
return;
}
this.supportedFeatureNames = new com.amazonaws.internal.SdkInternalList(supportedFeatureNames);
}
/**
*
* A list of features supported by the DB engine.
*
*
* The supported features vary by DB engine and DB engine version.
*
*
* To determine the supported features for a specific DB engine and DB engine version using the CLI, use the
* following command:
*
*
* aws rds describe-db-engine-versions --engine <engine_name> --engine-version <engine_version>
*
*
* For example, to determine the supported features for RDS for PostgreSQL version 13.3 using the CLI, use the
* following command:
*
*
* aws rds describe-db-engine-versions --engine postgres --engine-version 13.3
*
*
* The supported features are listed under SupportedFeatureNames
in the output.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setSupportedFeatureNames(java.util.Collection)} or
* {@link #withSupportedFeatureNames(java.util.Collection)} if you want to override the existing values.
*
*
* @param supportedFeatureNames
* A list of features supported by the DB engine.
*
* The supported features vary by DB engine and DB engine version.
*
*
* To determine the supported features for a specific DB engine and DB engine version using the CLI, use the
* following command:
*
*
* aws rds describe-db-engine-versions --engine <engine_name> --engine-version <engine_version>
*
*
* For example, to determine the supported features for RDS for PostgreSQL version 13.3 using the CLI, use
* the following command:
*
*
* aws rds describe-db-engine-versions --engine postgres --engine-version 13.3
*
*
* The supported features are listed under SupportedFeatureNames
in the output.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ModifyCustomDBEngineVersionResult withSupportedFeatureNames(String... supportedFeatureNames) {
if (this.supportedFeatureNames == null) {
setSupportedFeatureNames(new com.amazonaws.internal.SdkInternalList(supportedFeatureNames.length));
}
for (String ele : supportedFeatureNames) {
this.supportedFeatureNames.add(ele);
}
return this;
}
/**
*
* A list of features supported by the DB engine.
*
*
* The supported features vary by DB engine and DB engine version.
*
*
* To determine the supported features for a specific DB engine and DB engine version using the CLI, use the
* following command:
*
*
* aws rds describe-db-engine-versions --engine <engine_name> --engine-version <engine_version>
*
*
* For example, to determine the supported features for RDS for PostgreSQL version 13.3 using the CLI, use the
* following command:
*
*
* aws rds describe-db-engine-versions --engine postgres --engine-version 13.3
*
*
* The supported features are listed under SupportedFeatureNames
in the output.
*
*
* @param supportedFeatureNames
* A list of features supported by the DB engine.
*
* The supported features vary by DB engine and DB engine version.
*
*
* To determine the supported features for a specific DB engine and DB engine version using the CLI, use the
* following command:
*
*
* aws rds describe-db-engine-versions --engine <engine_name> --engine-version <engine_version>
*
*
* For example, to determine the supported features for RDS for PostgreSQL version 13.3 using the CLI, use
* the following command:
*
*
* aws rds describe-db-engine-versions --engine postgres --engine-version 13.3
*
*
* The supported features are listed under SupportedFeatureNames
in the output.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ModifyCustomDBEngineVersionResult withSupportedFeatureNames(java.util.Collection supportedFeatureNames) {
setSupportedFeatureNames(supportedFeatureNames);
return this;
}
/**
*
* The status of the DB engine version, either available
or deprecated
.
*
*
* @param status
* The status of the DB engine version, either available
or deprecated
.
*/
public void setStatus(String status) {
this.status = status;
}
/**
*
* The status of the DB engine version, either available
or deprecated
.
*
*
* @return The status of the DB engine version, either available
or deprecated
.
*/
public String getStatus() {
return this.status;
}
/**
*
* The status of the DB engine version, either available
or deprecated
.
*
*
* @param status
* The status of the DB engine version, either available
or deprecated
.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ModifyCustomDBEngineVersionResult withStatus(String status) {
setStatus(status);
return this;
}
/**
*
* Indicates whether you can use Aurora parallel query with a specific DB engine version.
*
*
* @param supportsParallelQuery
* Indicates whether you can use Aurora parallel query with a specific DB engine version.
*/
public void setSupportsParallelQuery(Boolean supportsParallelQuery) {
this.supportsParallelQuery = supportsParallelQuery;
}
/**
*
* Indicates whether you can use Aurora parallel query with a specific DB engine version.
*
*
* @return Indicates whether you can use Aurora parallel query with a specific DB engine version.
*/
public Boolean getSupportsParallelQuery() {
return this.supportsParallelQuery;
}
/**
*
* Indicates whether you can use Aurora parallel query with a specific DB engine version.
*
*
* @param supportsParallelQuery
* Indicates whether you can use Aurora parallel query with a specific DB engine version.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ModifyCustomDBEngineVersionResult withSupportsParallelQuery(Boolean supportsParallelQuery) {
setSupportsParallelQuery(supportsParallelQuery);
return this;
}
/**
*
* Indicates whether you can use Aurora parallel query with a specific DB engine version.
*
*
* @return Indicates whether you can use Aurora parallel query with a specific DB engine version.
*/
public Boolean isSupportsParallelQuery() {
return this.supportsParallelQuery;
}
/**
*
* Indicates whether you can use Aurora global databases with a specific DB engine version.
*
*
* @param supportsGlobalDatabases
* Indicates whether you can use Aurora global databases with a specific DB engine version.
*/
public void setSupportsGlobalDatabases(Boolean supportsGlobalDatabases) {
this.supportsGlobalDatabases = supportsGlobalDatabases;
}
/**
*
* Indicates whether you can use Aurora global databases with a specific DB engine version.
*
*
* @return Indicates whether you can use Aurora global databases with a specific DB engine version.
*/
public Boolean getSupportsGlobalDatabases() {
return this.supportsGlobalDatabases;
}
/**
*
* Indicates whether you can use Aurora global databases with a specific DB engine version.
*
*
* @param supportsGlobalDatabases
* Indicates whether you can use Aurora global databases with a specific DB engine version.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ModifyCustomDBEngineVersionResult withSupportsGlobalDatabases(Boolean supportsGlobalDatabases) {
setSupportsGlobalDatabases(supportsGlobalDatabases);
return this;
}
/**
*
* Indicates whether you can use Aurora global databases with a specific DB engine version.
*
*
* @return Indicates whether you can use Aurora global databases with a specific DB engine version.
*/
public Boolean isSupportsGlobalDatabases() {
return this.supportsGlobalDatabases;
}
/**
*
* The major engine version of the CEV.
*
*
* @param majorEngineVersion
* The major engine version of the CEV.
*/
public void setMajorEngineVersion(String majorEngineVersion) {
this.majorEngineVersion = majorEngineVersion;
}
/**
*
* The major engine version of the CEV.
*
*
* @return The major engine version of the CEV.
*/
public String getMajorEngineVersion() {
return this.majorEngineVersion;
}
/**
*
* The major engine version of the CEV.
*
*
* @param majorEngineVersion
* The major engine version of the CEV.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ModifyCustomDBEngineVersionResult withMajorEngineVersion(String majorEngineVersion) {
setMajorEngineVersion(majorEngineVersion);
return this;
}
/**
*
* The name of the Amazon S3 bucket that contains your database installation files.
*
*
* @param databaseInstallationFilesS3BucketName
* The name of the Amazon S3 bucket that contains your database installation files.
*/
public void setDatabaseInstallationFilesS3BucketName(String databaseInstallationFilesS3BucketName) {
this.databaseInstallationFilesS3BucketName = databaseInstallationFilesS3BucketName;
}
/**
*
* The name of the Amazon S3 bucket that contains your database installation files.
*
*
* @return The name of the Amazon S3 bucket that contains your database installation files.
*/
public String getDatabaseInstallationFilesS3BucketName() {
return this.databaseInstallationFilesS3BucketName;
}
/**
*
* The name of the Amazon S3 bucket that contains your database installation files.
*
*
* @param databaseInstallationFilesS3BucketName
* The name of the Amazon S3 bucket that contains your database installation files.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ModifyCustomDBEngineVersionResult withDatabaseInstallationFilesS3BucketName(String databaseInstallationFilesS3BucketName) {
setDatabaseInstallationFilesS3BucketName(databaseInstallationFilesS3BucketName);
return this;
}
/**
*
* The Amazon S3 directory that contains the database installation files. If not specified, then no prefix is
* assumed.
*
*
* @param databaseInstallationFilesS3Prefix
* The Amazon S3 directory that contains the database installation files. If not specified, then no prefix is
* assumed.
*/
public void setDatabaseInstallationFilesS3Prefix(String databaseInstallationFilesS3Prefix) {
this.databaseInstallationFilesS3Prefix = databaseInstallationFilesS3Prefix;
}
/**
*
* The Amazon S3 directory that contains the database installation files. If not specified, then no prefix is
* assumed.
*
*
* @return The Amazon S3 directory that contains the database installation files. If not specified, then no prefix
* is assumed.
*/
public String getDatabaseInstallationFilesS3Prefix() {
return this.databaseInstallationFilesS3Prefix;
}
/**
*
* The Amazon S3 directory that contains the database installation files. If not specified, then no prefix is
* assumed.
*
*
* @param databaseInstallationFilesS3Prefix
* The Amazon S3 directory that contains the database installation files. If not specified, then no prefix is
* assumed.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ModifyCustomDBEngineVersionResult withDatabaseInstallationFilesS3Prefix(String databaseInstallationFilesS3Prefix) {
setDatabaseInstallationFilesS3Prefix(databaseInstallationFilesS3Prefix);
return this;
}
/**
*
* The ARN of the custom engine version.
*
*
* @param dBEngineVersionArn
* The ARN of the custom engine version.
*/
public void setDBEngineVersionArn(String dBEngineVersionArn) {
this.dBEngineVersionArn = dBEngineVersionArn;
}
/**
*
* The ARN of the custom engine version.
*
*
* @return The ARN of the custom engine version.
*/
public String getDBEngineVersionArn() {
return this.dBEngineVersionArn;
}
/**
*
* The ARN of the custom engine version.
*
*
* @param dBEngineVersionArn
* The ARN of the custom engine version.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ModifyCustomDBEngineVersionResult withDBEngineVersionArn(String dBEngineVersionArn) {
setDBEngineVersionArn(dBEngineVersionArn);
return this;
}
/**
*
* The Amazon Web Services KMS key identifier for an encrypted CEV. This parameter is required for RDS Custom, but
* optional for Amazon RDS.
*
*
* @param kMSKeyId
* The Amazon Web Services KMS key identifier for an encrypted CEV. This parameter is required for RDS
* Custom, but optional for Amazon RDS.
*/
public void setKMSKeyId(String kMSKeyId) {
this.kMSKeyId = kMSKeyId;
}
/**
*
* The Amazon Web Services KMS key identifier for an encrypted CEV. This parameter is required for RDS Custom, but
* optional for Amazon RDS.
*
*
* @return The Amazon Web Services KMS key identifier for an encrypted CEV. This parameter is required for RDS
* Custom, but optional for Amazon RDS.
*/
public String getKMSKeyId() {
return this.kMSKeyId;
}
/**
*
* The Amazon Web Services KMS key identifier for an encrypted CEV. This parameter is required for RDS Custom, but
* optional for Amazon RDS.
*
*
* @param kMSKeyId
* The Amazon Web Services KMS key identifier for an encrypted CEV. This parameter is required for RDS
* Custom, but optional for Amazon RDS.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ModifyCustomDBEngineVersionResult withKMSKeyId(String kMSKeyId) {
setKMSKeyId(kMSKeyId);
return this;
}
/**
*
* The creation time of the DB engine version.
*
*
* @param createTime
* The creation time of the DB engine version.
*/
public void setCreateTime(java.util.Date createTime) {
this.createTime = createTime;
}
/**
*
* The creation time of the DB engine version.
*
*
* @return The creation time of the DB engine version.
*/
public java.util.Date getCreateTime() {
return this.createTime;
}
/**
*
* The creation time of the DB engine version.
*
*
* @param createTime
* The creation time of the DB engine version.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ModifyCustomDBEngineVersionResult withCreateTime(java.util.Date createTime) {
setCreateTime(createTime);
return this;
}
/**
* @return
*/
public java.util.List getTagList() {
if (tagList == null) {
tagList = new com.amazonaws.internal.SdkInternalList();
}
return tagList;
}
/**
* @param tagList
*/
public void setTagList(java.util.Collection tagList) {
if (tagList == null) {
this.tagList = null;
return;
}
this.tagList = new com.amazonaws.internal.SdkInternalList(tagList);
}
/**
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setTagList(java.util.Collection)} or {@link #withTagList(java.util.Collection)} if you want to override
* the existing values.
*
*
* @param tagList
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ModifyCustomDBEngineVersionResult withTagList(Tag... tagList) {
if (this.tagList == null) {
setTagList(new com.amazonaws.internal.SdkInternalList(tagList.length));
}
for (Tag ele : tagList) {
this.tagList.add(ele);
}
return this;
}
/**
* @param tagList
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ModifyCustomDBEngineVersionResult withTagList(java.util.Collection tagList) {
setTagList(tagList);
return this;
}
/**
*
* Indicates whether the engine version supports Babelfish for Aurora PostgreSQL.
*
*
* @param supportsBabelfish
* Indicates whether the engine version supports Babelfish for Aurora PostgreSQL.
*/
public void setSupportsBabelfish(Boolean supportsBabelfish) {
this.supportsBabelfish = supportsBabelfish;
}
/**
*
* Indicates whether the engine version supports Babelfish for Aurora PostgreSQL.
*
*
* @return Indicates whether the engine version supports Babelfish for Aurora PostgreSQL.
*/
public Boolean getSupportsBabelfish() {
return this.supportsBabelfish;
}
/**
*
* Indicates whether the engine version supports Babelfish for Aurora PostgreSQL.
*
*
* @param supportsBabelfish
* Indicates whether the engine version supports Babelfish for Aurora PostgreSQL.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ModifyCustomDBEngineVersionResult withSupportsBabelfish(Boolean supportsBabelfish) {
setSupportsBabelfish(supportsBabelfish);
return this;
}
/**
*
* Indicates whether the engine version supports Babelfish for Aurora PostgreSQL.
*
*
* @return Indicates whether the engine version supports Babelfish for Aurora PostgreSQL.
*/
public Boolean isSupportsBabelfish() {
return this.supportsBabelfish;
}
/**
*
* JSON string that lists the installation files and parameters that RDS Custom uses to create a custom engine
* version (CEV). RDS Custom applies the patches in the order in which they're listed in the manifest. You can set
* the Oracle home, Oracle base, and UNIX/Linux user and group using the installation parameters. For more
* information, see JSON fields in the CEV manifest in the Amazon RDS User Guide.
*
*
* @param customDBEngineVersionManifest
* JSON string that lists the installation files and parameters that RDS Custom uses to create a custom
* engine version (CEV). RDS Custom applies the patches in the order in which they're listed in the manifest.
* You can set the Oracle home, Oracle base, and UNIX/Linux user and group using the installation parameters.
* For more information, see JSON fields in the CEV manifest in the Amazon RDS User Guide.
*/
public void setCustomDBEngineVersionManifest(String customDBEngineVersionManifest) {
this.customDBEngineVersionManifest = customDBEngineVersionManifest;
}
/**
*
* JSON string that lists the installation files and parameters that RDS Custom uses to create a custom engine
* version (CEV). RDS Custom applies the patches in the order in which they're listed in the manifest. You can set
* the Oracle home, Oracle base, and UNIX/Linux user and group using the installation parameters. For more
* information, see JSON fields in the CEV manifest in the Amazon RDS User Guide.
*
*
* @return JSON string that lists the installation files and parameters that RDS Custom uses to create a custom
* engine version (CEV). RDS Custom applies the patches in the order in which they're listed in the
* manifest. You can set the Oracle home, Oracle base, and UNIX/Linux user and group using the installation
* parameters. For more information, see JSON fields in the CEV manifest in the Amazon RDS User Guide.
*/
public String getCustomDBEngineVersionManifest() {
return this.customDBEngineVersionManifest;
}
/**
*
* JSON string that lists the installation files and parameters that RDS Custom uses to create a custom engine
* version (CEV). RDS Custom applies the patches in the order in which they're listed in the manifest. You can set
* the Oracle home, Oracle base, and UNIX/Linux user and group using the installation parameters. For more
* information, see JSON fields in the CEV manifest in the Amazon RDS User Guide.
*
*
* @param customDBEngineVersionManifest
* JSON string that lists the installation files and parameters that RDS Custom uses to create a custom
* engine version (CEV). RDS Custom applies the patches in the order in which they're listed in the manifest.
* You can set the Oracle home, Oracle base, and UNIX/Linux user and group using the installation parameters.
* For more information, see JSON fields in the CEV manifest in the Amazon RDS User Guide.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ModifyCustomDBEngineVersionResult withCustomDBEngineVersionManifest(String customDBEngineVersionManifest) {
setCustomDBEngineVersionManifest(customDBEngineVersionManifest);
return this;
}
/**
*
* Indicates whether the DB engine version supports Aurora Limitless Database.
*
*
* @param supportsLimitlessDatabase
* Indicates whether the DB engine version supports Aurora Limitless Database.
*/
public void setSupportsLimitlessDatabase(Boolean supportsLimitlessDatabase) {
this.supportsLimitlessDatabase = supportsLimitlessDatabase;
}
/**
*
* Indicates whether the DB engine version supports Aurora Limitless Database.
*
*
* @return Indicates whether the DB engine version supports Aurora Limitless Database.
*/
public Boolean getSupportsLimitlessDatabase() {
return this.supportsLimitlessDatabase;
}
/**
*
* Indicates whether the DB engine version supports Aurora Limitless Database.
*
*
* @param supportsLimitlessDatabase
* Indicates whether the DB engine version supports Aurora Limitless Database.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ModifyCustomDBEngineVersionResult withSupportsLimitlessDatabase(Boolean supportsLimitlessDatabase) {
setSupportsLimitlessDatabase(supportsLimitlessDatabase);
return this;
}
/**
*
* Indicates whether the DB engine version supports Aurora Limitless Database.
*
*
* @return Indicates whether the DB engine version supports Aurora Limitless Database.
*/
public Boolean isSupportsLimitlessDatabase() {
return this.supportsLimitlessDatabase;
}
/**
*
* Indicates whether the engine version supports rotating the server certificate without rebooting the DB instance.
*
*
* @param supportsCertificateRotationWithoutRestart
* Indicates whether the engine version supports rotating the server certificate without rebooting the DB
* instance.
*/
public void setSupportsCertificateRotationWithoutRestart(Boolean supportsCertificateRotationWithoutRestart) {
this.supportsCertificateRotationWithoutRestart = supportsCertificateRotationWithoutRestart;
}
/**
*
* Indicates whether the engine version supports rotating the server certificate without rebooting the DB instance.
*
*
* @return Indicates whether the engine version supports rotating the server certificate without rebooting the DB
* instance.
*/
public Boolean getSupportsCertificateRotationWithoutRestart() {
return this.supportsCertificateRotationWithoutRestart;
}
/**
*
* Indicates whether the engine version supports rotating the server certificate without rebooting the DB instance.
*
*
* @param supportsCertificateRotationWithoutRestart
* Indicates whether the engine version supports rotating the server certificate without rebooting the DB
* instance.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ModifyCustomDBEngineVersionResult withSupportsCertificateRotationWithoutRestart(Boolean supportsCertificateRotationWithoutRestart) {
setSupportsCertificateRotationWithoutRestart(supportsCertificateRotationWithoutRestart);
return this;
}
/**
*
* Indicates whether the engine version supports rotating the server certificate without rebooting the DB instance.
*
*
* @return Indicates whether the engine version supports rotating the server certificate without rebooting the DB
* instance.
*/
public Boolean isSupportsCertificateRotationWithoutRestart() {
return this.supportsCertificateRotationWithoutRestart;
}
/**
*
* A list of the supported CA certificate identifiers.
*
*
* For more information, see Using SSL/TLS to encrypt a
* connection to a DB instance in the Amazon RDS User Guide and Using SSL/TLS to
* encrypt a connection to a DB cluster in the Amazon Aurora User Guide.
*
*
* @return A list of the supported CA certificate identifiers.
*
* For more information, see Using SSL/TLS to
* encrypt a connection to a DB instance in the Amazon RDS User Guide and Using SSL/TLS
* to encrypt a connection to a DB cluster in the Amazon Aurora User Guide.
*/
public java.util.List getSupportedCACertificateIdentifiers() {
if (supportedCACertificateIdentifiers == null) {
supportedCACertificateIdentifiers = new com.amazonaws.internal.SdkInternalList();
}
return supportedCACertificateIdentifiers;
}
/**
*
* A list of the supported CA certificate identifiers.
*
*
* For more information, see Using SSL/TLS to encrypt a
* connection to a DB instance in the Amazon RDS User Guide and Using SSL/TLS to
* encrypt a connection to a DB cluster in the Amazon Aurora User Guide.
*
*
* @param supportedCACertificateIdentifiers
* A list of the supported CA certificate identifiers.
*
* For more information, see Using SSL/TLS to
* encrypt a connection to a DB instance in the Amazon RDS User Guide and Using SSL/TLS
* to encrypt a connection to a DB cluster in the Amazon Aurora User Guide.
*/
public void setSupportedCACertificateIdentifiers(java.util.Collection supportedCACertificateIdentifiers) {
if (supportedCACertificateIdentifiers == null) {
this.supportedCACertificateIdentifiers = null;
return;
}
this.supportedCACertificateIdentifiers = new com.amazonaws.internal.SdkInternalList(supportedCACertificateIdentifiers);
}
/**
*
* A list of the supported CA certificate identifiers.
*
*
* For more information, see Using SSL/TLS to encrypt a
* connection to a DB instance in the Amazon RDS User Guide and Using SSL/TLS to
* encrypt a connection to a DB cluster in the Amazon Aurora User Guide.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setSupportedCACertificateIdentifiers(java.util.Collection)} or
* {@link #withSupportedCACertificateIdentifiers(java.util.Collection)} if you want to override the existing values.
*
*
* @param supportedCACertificateIdentifiers
* A list of the supported CA certificate identifiers.
*
* For more information, see Using SSL/TLS to
* encrypt a connection to a DB instance in the Amazon RDS User Guide and Using SSL/TLS
* to encrypt a connection to a DB cluster in the Amazon Aurora User Guide.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ModifyCustomDBEngineVersionResult withSupportedCACertificateIdentifiers(String... supportedCACertificateIdentifiers) {
if (this.supportedCACertificateIdentifiers == null) {
setSupportedCACertificateIdentifiers(new com.amazonaws.internal.SdkInternalList(supportedCACertificateIdentifiers.length));
}
for (String ele : supportedCACertificateIdentifiers) {
this.supportedCACertificateIdentifiers.add(ele);
}
return this;
}
/**
*
* A list of the supported CA certificate identifiers.
*
*
* For more information, see Using SSL/TLS to encrypt a
* connection to a DB instance in the Amazon RDS User Guide and Using SSL/TLS to
* encrypt a connection to a DB cluster in the Amazon Aurora User Guide.
*
*
* @param supportedCACertificateIdentifiers
* A list of the supported CA certificate identifiers.
*
* For more information, see Using SSL/TLS to
* encrypt a connection to a DB instance in the Amazon RDS User Guide and Using SSL/TLS
* to encrypt a connection to a DB cluster in the Amazon Aurora User Guide.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ModifyCustomDBEngineVersionResult withSupportedCACertificateIdentifiers(java.util.Collection supportedCACertificateIdentifiers) {
setSupportedCACertificateIdentifiers(supportedCACertificateIdentifiers);
return this;
}
/**
*
* Indicates whether the DB engine version supports forwarding write operations from reader DB instances to the
* writer DB instance in the DB cluster. By default, write operations aren't allowed on reader DB instances.
*
*
* Valid for: Aurora DB clusters only
*
*
* @param supportsLocalWriteForwarding
* Indicates whether the DB engine version supports forwarding write operations from reader DB instances to
* the writer DB instance in the DB cluster. By default, write operations aren't allowed on reader DB
* instances.
*
* Valid for: Aurora DB clusters only
*/
public void setSupportsLocalWriteForwarding(Boolean supportsLocalWriteForwarding) {
this.supportsLocalWriteForwarding = supportsLocalWriteForwarding;
}
/**
*
* Indicates whether the DB engine version supports forwarding write operations from reader DB instances to the
* writer DB instance in the DB cluster. By default, write operations aren't allowed on reader DB instances.
*
*
* Valid for: Aurora DB clusters only
*
*
* @return Indicates whether the DB engine version supports forwarding write operations from reader DB instances to
* the writer DB instance in the DB cluster. By default, write operations aren't allowed on reader DB
* instances.
*
* Valid for: Aurora DB clusters only
*/
public Boolean getSupportsLocalWriteForwarding() {
return this.supportsLocalWriteForwarding;
}
/**
*
* Indicates whether the DB engine version supports forwarding write operations from reader DB instances to the
* writer DB instance in the DB cluster. By default, write operations aren't allowed on reader DB instances.
*
*
* Valid for: Aurora DB clusters only
*
*
* @param supportsLocalWriteForwarding
* Indicates whether the DB engine version supports forwarding write operations from reader DB instances to
* the writer DB instance in the DB cluster. By default, write operations aren't allowed on reader DB
* instances.
*
* Valid for: Aurora DB clusters only
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ModifyCustomDBEngineVersionResult withSupportsLocalWriteForwarding(Boolean supportsLocalWriteForwarding) {
setSupportsLocalWriteForwarding(supportsLocalWriteForwarding);
return this;
}
/**
*
* Indicates whether the DB engine version supports forwarding write operations from reader DB instances to the
* writer DB instance in the DB cluster. By default, write operations aren't allowed on reader DB instances.
*
*
* Valid for: Aurora DB clusters only
*
*
* @return Indicates whether the DB engine version supports forwarding write operations from reader DB instances to
* the writer DB instance in the DB cluster. By default, write operations aren't allowed on reader DB
* instances.
*
* Valid for: Aurora DB clusters only
*/
public Boolean isSupportsLocalWriteForwarding() {
return this.supportsLocalWriteForwarding;
}
/**
*
* Indicates whether the DB engine version supports zero-ETL integrations with Amazon Redshift.
*
*
* @param supportsIntegrations
* Indicates whether the DB engine version supports zero-ETL integrations with Amazon Redshift.
*/
public void setSupportsIntegrations(Boolean supportsIntegrations) {
this.supportsIntegrations = supportsIntegrations;
}
/**
*
* Indicates whether the DB engine version supports zero-ETL integrations with Amazon Redshift.
*
*
* @return Indicates whether the DB engine version supports zero-ETL integrations with Amazon Redshift.
*/
public Boolean getSupportsIntegrations() {
return this.supportsIntegrations;
}
/**
*
* Indicates whether the DB engine version supports zero-ETL integrations with Amazon Redshift.
*
*
* @param supportsIntegrations
* Indicates whether the DB engine version supports zero-ETL integrations with Amazon Redshift.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ModifyCustomDBEngineVersionResult withSupportsIntegrations(Boolean supportsIntegrations) {
setSupportsIntegrations(supportsIntegrations);
return this;
}
/**
*
* Indicates whether the DB engine version supports zero-ETL integrations with Amazon Redshift.
*
*
* @return Indicates whether the DB engine version supports zero-ETL integrations with Amazon Redshift.
*/
public Boolean isSupportsIntegrations() {
return this.supportsIntegrations;
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getEngine() != null)
sb.append("Engine: ").append(getEngine()).append(",");
if (getEngineVersion() != null)
sb.append("EngineVersion: ").append(getEngineVersion()).append(",");
if (getDBParameterGroupFamily() != null)
sb.append("DBParameterGroupFamily: ").append(getDBParameterGroupFamily()).append(",");
if (getDBEngineDescription() != null)
sb.append("DBEngineDescription: ").append(getDBEngineDescription()).append(",");
if (getDBEngineVersionDescription() != null)
sb.append("DBEngineVersionDescription: ").append(getDBEngineVersionDescription()).append(",");
if (getDefaultCharacterSet() != null)
sb.append("DefaultCharacterSet: ").append(getDefaultCharacterSet()).append(",");
if (getImage() != null)
sb.append("Image: ").append(getImage()).append(",");
if (getDBEngineMediaType() != null)
sb.append("DBEngineMediaType: ").append(getDBEngineMediaType()).append(",");
if (getSupportedCharacterSets() != null)
sb.append("SupportedCharacterSets: ").append(getSupportedCharacterSets()).append(",");
if (getSupportedNcharCharacterSets() != null)
sb.append("SupportedNcharCharacterSets: ").append(getSupportedNcharCharacterSets()).append(",");
if (getValidUpgradeTarget() != null)
sb.append("ValidUpgradeTarget: ").append(getValidUpgradeTarget()).append(",");
if (getSupportedTimezones() != null)
sb.append("SupportedTimezones: ").append(getSupportedTimezones()).append(",");
if (getExportableLogTypes() != null)
sb.append("ExportableLogTypes: ").append(getExportableLogTypes()).append(",");
if (getSupportsLogExportsToCloudwatchLogs() != null)
sb.append("SupportsLogExportsToCloudwatchLogs: ").append(getSupportsLogExportsToCloudwatchLogs()).append(",");
if (getSupportsReadReplica() != null)
sb.append("SupportsReadReplica: ").append(getSupportsReadReplica()).append(",");
if (getSupportedEngineModes() != null)
sb.append("SupportedEngineModes: ").append(getSupportedEngineModes()).append(",");
if (getSupportedFeatureNames() != null)
sb.append("SupportedFeatureNames: ").append(getSupportedFeatureNames()).append(",");
if (getStatus() != null)
sb.append("Status: ").append(getStatus()).append(",");
if (getSupportsParallelQuery() != null)
sb.append("SupportsParallelQuery: ").append(getSupportsParallelQuery()).append(",");
if (getSupportsGlobalDatabases() != null)
sb.append("SupportsGlobalDatabases: ").append(getSupportsGlobalDatabases()).append(",");
if (getMajorEngineVersion() != null)
sb.append("MajorEngineVersion: ").append(getMajorEngineVersion()).append(",");
if (getDatabaseInstallationFilesS3BucketName() != null)
sb.append("DatabaseInstallationFilesS3BucketName: ").append(getDatabaseInstallationFilesS3BucketName()).append(",");
if (getDatabaseInstallationFilesS3Prefix() != null)
sb.append("DatabaseInstallationFilesS3Prefix: ").append(getDatabaseInstallationFilesS3Prefix()).append(",");
if (getDBEngineVersionArn() != null)
sb.append("DBEngineVersionArn: ").append(getDBEngineVersionArn()).append(",");
if (getKMSKeyId() != null)
sb.append("KMSKeyId: ").append(getKMSKeyId()).append(",");
if (getCreateTime() != null)
sb.append("CreateTime: ").append(getCreateTime()).append(",");
if (getTagList() != null)
sb.append("TagList: ").append(getTagList()).append(",");
if (getSupportsBabelfish() != null)
sb.append("SupportsBabelfish: ").append(getSupportsBabelfish()).append(",");
if (getCustomDBEngineVersionManifest() != null)
sb.append("CustomDBEngineVersionManifest: ").append(getCustomDBEngineVersionManifest()).append(",");
if (getSupportsLimitlessDatabase() != null)
sb.append("SupportsLimitlessDatabase: ").append(getSupportsLimitlessDatabase()).append(",");
if (getSupportsCertificateRotationWithoutRestart() != null)
sb.append("SupportsCertificateRotationWithoutRestart: ").append(getSupportsCertificateRotationWithoutRestart()).append(",");
if (getSupportedCACertificateIdentifiers() != null)
sb.append("SupportedCACertificateIdentifiers: ").append(getSupportedCACertificateIdentifiers()).append(",");
if (getSupportsLocalWriteForwarding() != null)
sb.append("SupportsLocalWriteForwarding: ").append(getSupportsLocalWriteForwarding()).append(",");
if (getSupportsIntegrations() != null)
sb.append("SupportsIntegrations: ").append(getSupportsIntegrations());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof ModifyCustomDBEngineVersionResult == false)
return false;
ModifyCustomDBEngineVersionResult other = (ModifyCustomDBEngineVersionResult) obj;
if (other.getEngine() == null ^ this.getEngine() == null)
return false;
if (other.getEngine() != null && other.getEngine().equals(this.getEngine()) == false)
return false;
if (other.getEngineVersion() == null ^ this.getEngineVersion() == null)
return false;
if (other.getEngineVersion() != null && other.getEngineVersion().equals(this.getEngineVersion()) == false)
return false;
if (other.getDBParameterGroupFamily() == null ^ this.getDBParameterGroupFamily() == null)
return false;
if (other.getDBParameterGroupFamily() != null && other.getDBParameterGroupFamily().equals(this.getDBParameterGroupFamily()) == false)
return false;
if (other.getDBEngineDescription() == null ^ this.getDBEngineDescription() == null)
return false;
if (other.getDBEngineDescription() != null && other.getDBEngineDescription().equals(this.getDBEngineDescription()) == false)
return false;
if (other.getDBEngineVersionDescription() == null ^ this.getDBEngineVersionDescription() == null)
return false;
if (other.getDBEngineVersionDescription() != null && other.getDBEngineVersionDescription().equals(this.getDBEngineVersionDescription()) == false)
return false;
if (other.getDefaultCharacterSet() == null ^ this.getDefaultCharacterSet() == null)
return false;
if (other.getDefaultCharacterSet() != null && other.getDefaultCharacterSet().equals(this.getDefaultCharacterSet()) == false)
return false;
if (other.getImage() == null ^ this.getImage() == null)
return false;
if (other.getImage() != null && other.getImage().equals(this.getImage()) == false)
return false;
if (other.getDBEngineMediaType() == null ^ this.getDBEngineMediaType() == null)
return false;
if (other.getDBEngineMediaType() != null && other.getDBEngineMediaType().equals(this.getDBEngineMediaType()) == false)
return false;
if (other.getSupportedCharacterSets() == null ^ this.getSupportedCharacterSets() == null)
return false;
if (other.getSupportedCharacterSets() != null && other.getSupportedCharacterSets().equals(this.getSupportedCharacterSets()) == false)
return false;
if (other.getSupportedNcharCharacterSets() == null ^ this.getSupportedNcharCharacterSets() == null)
return false;
if (other.getSupportedNcharCharacterSets() != null && other.getSupportedNcharCharacterSets().equals(this.getSupportedNcharCharacterSets()) == false)
return false;
if (other.getValidUpgradeTarget() == null ^ this.getValidUpgradeTarget() == null)
return false;
if (other.getValidUpgradeTarget() != null && other.getValidUpgradeTarget().equals(this.getValidUpgradeTarget()) == false)
return false;
if (other.getSupportedTimezones() == null ^ this.getSupportedTimezones() == null)
return false;
if (other.getSupportedTimezones() != null && other.getSupportedTimezones().equals(this.getSupportedTimezones()) == false)
return false;
if (other.getExportableLogTypes() == null ^ this.getExportableLogTypes() == null)
return false;
if (other.getExportableLogTypes() != null && other.getExportableLogTypes().equals(this.getExportableLogTypes()) == false)
return false;
if (other.getSupportsLogExportsToCloudwatchLogs() == null ^ this.getSupportsLogExportsToCloudwatchLogs() == null)
return false;
if (other.getSupportsLogExportsToCloudwatchLogs() != null
&& other.getSupportsLogExportsToCloudwatchLogs().equals(this.getSupportsLogExportsToCloudwatchLogs()) == false)
return false;
if (other.getSupportsReadReplica() == null ^ this.getSupportsReadReplica() == null)
return false;
if (other.getSupportsReadReplica() != null && other.getSupportsReadReplica().equals(this.getSupportsReadReplica()) == false)
return false;
if (other.getSupportedEngineModes() == null ^ this.getSupportedEngineModes() == null)
return false;
if (other.getSupportedEngineModes() != null && other.getSupportedEngineModes().equals(this.getSupportedEngineModes()) == false)
return false;
if (other.getSupportedFeatureNames() == null ^ this.getSupportedFeatureNames() == null)
return false;
if (other.getSupportedFeatureNames() != null && other.getSupportedFeatureNames().equals(this.getSupportedFeatureNames()) == false)
return false;
if (other.getStatus() == null ^ this.getStatus() == null)
return false;
if (other.getStatus() != null && other.getStatus().equals(this.getStatus()) == false)
return false;
if (other.getSupportsParallelQuery() == null ^ this.getSupportsParallelQuery() == null)
return false;
if (other.getSupportsParallelQuery() != null && other.getSupportsParallelQuery().equals(this.getSupportsParallelQuery()) == false)
return false;
if (other.getSupportsGlobalDatabases() == null ^ this.getSupportsGlobalDatabases() == null)
return false;
if (other.getSupportsGlobalDatabases() != null && other.getSupportsGlobalDatabases().equals(this.getSupportsGlobalDatabases()) == false)
return false;
if (other.getMajorEngineVersion() == null ^ this.getMajorEngineVersion() == null)
return false;
if (other.getMajorEngineVersion() != null && other.getMajorEngineVersion().equals(this.getMajorEngineVersion()) == false)
return false;
if (other.getDatabaseInstallationFilesS3BucketName() == null ^ this.getDatabaseInstallationFilesS3BucketName() == null)
return false;
if (other.getDatabaseInstallationFilesS3BucketName() != null
&& other.getDatabaseInstallationFilesS3BucketName().equals(this.getDatabaseInstallationFilesS3BucketName()) == false)
return false;
if (other.getDatabaseInstallationFilesS3Prefix() == null ^ this.getDatabaseInstallationFilesS3Prefix() == null)
return false;
if (other.getDatabaseInstallationFilesS3Prefix() != null
&& other.getDatabaseInstallationFilesS3Prefix().equals(this.getDatabaseInstallationFilesS3Prefix()) == false)
return false;
if (other.getDBEngineVersionArn() == null ^ this.getDBEngineVersionArn() == null)
return false;
if (other.getDBEngineVersionArn() != null && other.getDBEngineVersionArn().equals(this.getDBEngineVersionArn()) == false)
return false;
if (other.getKMSKeyId() == null ^ this.getKMSKeyId() == null)
return false;
if (other.getKMSKeyId() != null && other.getKMSKeyId().equals(this.getKMSKeyId()) == false)
return false;
if (other.getCreateTime() == null ^ this.getCreateTime() == null)
return false;
if (other.getCreateTime() != null && other.getCreateTime().equals(this.getCreateTime()) == false)
return false;
if (other.getTagList() == null ^ this.getTagList() == null)
return false;
if (other.getTagList() != null && other.getTagList().equals(this.getTagList()) == false)
return false;
if (other.getSupportsBabelfish() == null ^ this.getSupportsBabelfish() == null)
return false;
if (other.getSupportsBabelfish() != null && other.getSupportsBabelfish().equals(this.getSupportsBabelfish()) == false)
return false;
if (other.getCustomDBEngineVersionManifest() == null ^ this.getCustomDBEngineVersionManifest() == null)
return false;
if (other.getCustomDBEngineVersionManifest() != null
&& other.getCustomDBEngineVersionManifest().equals(this.getCustomDBEngineVersionManifest()) == false)
return false;
if (other.getSupportsLimitlessDatabase() == null ^ this.getSupportsLimitlessDatabase() == null)
return false;
if (other.getSupportsLimitlessDatabase() != null && other.getSupportsLimitlessDatabase().equals(this.getSupportsLimitlessDatabase()) == false)
return false;
if (other.getSupportsCertificateRotationWithoutRestart() == null ^ this.getSupportsCertificateRotationWithoutRestart() == null)
return false;
if (other.getSupportsCertificateRotationWithoutRestart() != null
&& other.getSupportsCertificateRotationWithoutRestart().equals(this.getSupportsCertificateRotationWithoutRestart()) == false)
return false;
if (other.getSupportedCACertificateIdentifiers() == null ^ this.getSupportedCACertificateIdentifiers() == null)
return false;
if (other.getSupportedCACertificateIdentifiers() != null
&& other.getSupportedCACertificateIdentifiers().equals(this.getSupportedCACertificateIdentifiers()) == false)
return false;
if (other.getSupportsLocalWriteForwarding() == null ^ this.getSupportsLocalWriteForwarding() == null)
return false;
if (other.getSupportsLocalWriteForwarding() != null && other.getSupportsLocalWriteForwarding().equals(this.getSupportsLocalWriteForwarding()) == false)
return false;
if (other.getSupportsIntegrations() == null ^ this.getSupportsIntegrations() == null)
return false;
if (other.getSupportsIntegrations() != null && other.getSupportsIntegrations().equals(this.getSupportsIntegrations()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getEngine() == null) ? 0 : getEngine().hashCode());
hashCode = prime * hashCode + ((getEngineVersion() == null) ? 0 : getEngineVersion().hashCode());
hashCode = prime * hashCode + ((getDBParameterGroupFamily() == null) ? 0 : getDBParameterGroupFamily().hashCode());
hashCode = prime * hashCode + ((getDBEngineDescription() == null) ? 0 : getDBEngineDescription().hashCode());
hashCode = prime * hashCode + ((getDBEngineVersionDescription() == null) ? 0 : getDBEngineVersionDescription().hashCode());
hashCode = prime * hashCode + ((getDefaultCharacterSet() == null) ? 0 : getDefaultCharacterSet().hashCode());
hashCode = prime * hashCode + ((getImage() == null) ? 0 : getImage().hashCode());
hashCode = prime * hashCode + ((getDBEngineMediaType() == null) ? 0 : getDBEngineMediaType().hashCode());
hashCode = prime * hashCode + ((getSupportedCharacterSets() == null) ? 0 : getSupportedCharacterSets().hashCode());
hashCode = prime * hashCode + ((getSupportedNcharCharacterSets() == null) ? 0 : getSupportedNcharCharacterSets().hashCode());
hashCode = prime * hashCode + ((getValidUpgradeTarget() == null) ? 0 : getValidUpgradeTarget().hashCode());
hashCode = prime * hashCode + ((getSupportedTimezones() == null) ? 0 : getSupportedTimezones().hashCode());
hashCode = prime * hashCode + ((getExportableLogTypes() == null) ? 0 : getExportableLogTypes().hashCode());
hashCode = prime * hashCode + ((getSupportsLogExportsToCloudwatchLogs() == null) ? 0 : getSupportsLogExportsToCloudwatchLogs().hashCode());
hashCode = prime * hashCode + ((getSupportsReadReplica() == null) ? 0 : getSupportsReadReplica().hashCode());
hashCode = prime * hashCode + ((getSupportedEngineModes() == null) ? 0 : getSupportedEngineModes().hashCode());
hashCode = prime * hashCode + ((getSupportedFeatureNames() == null) ? 0 : getSupportedFeatureNames().hashCode());
hashCode = prime * hashCode + ((getStatus() == null) ? 0 : getStatus().hashCode());
hashCode = prime * hashCode + ((getSupportsParallelQuery() == null) ? 0 : getSupportsParallelQuery().hashCode());
hashCode = prime * hashCode + ((getSupportsGlobalDatabases() == null) ? 0 : getSupportsGlobalDatabases().hashCode());
hashCode = prime * hashCode + ((getMajorEngineVersion() == null) ? 0 : getMajorEngineVersion().hashCode());
hashCode = prime * hashCode + ((getDatabaseInstallationFilesS3BucketName() == null) ? 0 : getDatabaseInstallationFilesS3BucketName().hashCode());
hashCode = prime * hashCode + ((getDatabaseInstallationFilesS3Prefix() == null) ? 0 : getDatabaseInstallationFilesS3Prefix().hashCode());
hashCode = prime * hashCode + ((getDBEngineVersionArn() == null) ? 0 : getDBEngineVersionArn().hashCode());
hashCode = prime * hashCode + ((getKMSKeyId() == null) ? 0 : getKMSKeyId().hashCode());
hashCode = prime * hashCode + ((getCreateTime() == null) ? 0 : getCreateTime().hashCode());
hashCode = prime * hashCode + ((getTagList() == null) ? 0 : getTagList().hashCode());
hashCode = prime * hashCode + ((getSupportsBabelfish() == null) ? 0 : getSupportsBabelfish().hashCode());
hashCode = prime * hashCode + ((getCustomDBEngineVersionManifest() == null) ? 0 : getCustomDBEngineVersionManifest().hashCode());
hashCode = prime * hashCode + ((getSupportsLimitlessDatabase() == null) ? 0 : getSupportsLimitlessDatabase().hashCode());
hashCode = prime * hashCode
+ ((getSupportsCertificateRotationWithoutRestart() == null) ? 0 : getSupportsCertificateRotationWithoutRestart().hashCode());
hashCode = prime * hashCode + ((getSupportedCACertificateIdentifiers() == null) ? 0 : getSupportedCACertificateIdentifiers().hashCode());
hashCode = prime * hashCode + ((getSupportsLocalWriteForwarding() == null) ? 0 : getSupportsLocalWriteForwarding().hashCode());
hashCode = prime * hashCode + ((getSupportsIntegrations() == null) ? 0 : getSupportsIntegrations().hashCode());
return hashCode;
}
@Override
public ModifyCustomDBEngineVersionResult clone() {
try {
return (ModifyCustomDBEngineVersionResult) super.clone();
} catch (CloneNotSupportedException e) {
throw new IllegalStateException("Got a CloneNotSupportedException from Object.clone() " + "even though we're Cloneable!", e);
}
}
}