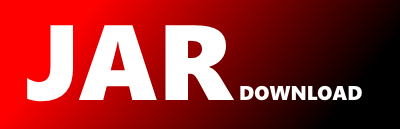
com.amazonaws.services.rds.model.ModifyDBClusterRequest Maven / Gradle / Ivy
Show all versions of aws-java-sdk-rds Show documentation
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.rds.model;
import java.io.Serializable;
import javax.annotation.Generated;
import com.amazonaws.AmazonWebServiceRequest;
/**
*
*
* @see AWS API
* Documentation
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class ModifyDBClusterRequest extends com.amazonaws.AmazonWebServiceRequest implements Serializable, Cloneable {
/**
*
* The DB cluster identifier for the cluster being modified. This parameter isn't case-sensitive.
*
*
* Valid for Cluster Type: Aurora DB clusters and Multi-AZ DB clusters
*
*
* Constraints:
*
*
* -
*
* Must match the identifier of an existing DB cluster.
*
*
*
*/
private String dBClusterIdentifier;
/**
*
* The new DB cluster identifier for the DB cluster when renaming a DB cluster. This value is stored as a lowercase
* string.
*
*
* Valid for Cluster Type: Aurora DB clusters and Multi-AZ DB clusters
*
*
* Constraints:
*
*
* -
*
* Must contain from 1 to 63 letters, numbers, or hyphens.
*
*
* -
*
* The first character must be a letter.
*
*
* -
*
* Can't end with a hyphen or contain two consecutive hyphens.
*
*
*
*
* Example: my-cluster2
*
*/
private String newDBClusterIdentifier;
/**
*
* Specifies whether the modifications in this request and any pending modifications are asynchronously applied as
* soon as possible, regardless of the PreferredMaintenanceWindow
setting for the DB cluster. If this
* parameter is disabled, changes to the DB cluster are applied during the next maintenance window.
*
*
* Most modifications can be applied immediately or during the next scheduled maintenance window. Some
* modifications, such as turning on deletion protection and changing the master password, are applied
* immediately—regardless of when you choose to apply them.
*
*
* By default, this parameter is disabled.
*
*
* Valid for Cluster Type: Aurora DB clusters and Multi-AZ DB clusters
*
*/
private Boolean applyImmediately;
/**
*
* The number of days for which automated backups are retained. Specify a minimum value of 1
.
*
*
* Valid for Cluster Type: Aurora DB clusters and Multi-AZ DB clusters
*
*
* Default: 1
*
*
* Constraints:
*
*
* -
*
* Must be a value from 1 to 35.
*
*
*
*/
private Integer backupRetentionPeriod;
/**
*
* The name of the DB cluster parameter group to use for the DB cluster.
*
*
* Valid for Cluster Type: Aurora DB clusters and Multi-AZ DB clusters
*
*/
private String dBClusterParameterGroupName;
/**
*
* A list of EC2 VPC security groups to associate with this DB cluster.
*
*
* Valid for Cluster Type: Aurora DB clusters and Multi-AZ DB clusters
*
*/
private com.amazonaws.internal.SdkInternalList vpcSecurityGroupIds;
/**
*
* The port number on which the DB cluster accepts connections.
*
*
* Valid for Cluster Type: Aurora DB clusters only
*
*
* Valid Values: 1150-65535
*
*
* Default: The same port as the original DB cluster.
*
*/
private Integer port;
/**
*
* The new password for the master database user.
*
*
* Valid for Cluster Type: Aurora DB clusters and Multi-AZ DB clusters
*
*
* Constraints:
*
*
* -
*
* Must contain from 8 to 41 characters.
*
*
* -
*
* Can contain any printable ASCII character except "/", """, or "@".
*
*
* -
*
* Can't be specified if ManageMasterUserPassword
is turned on.
*
*
*
*/
private String masterUserPassword;
/**
*
* The option group to associate the DB cluster with.
*
*
* DB clusters are associated with a default option group that can't be modified.
*
*/
private String optionGroupName;
/**
*
* The daily time range during which automated backups are created if automated backups are enabled, using the
* BackupRetentionPeriod
parameter.
*
*
* The default is a 30-minute window selected at random from an 8-hour block of time for each Amazon Web Services
* Region. To view the time blocks available, see Backup window in the Amazon Aurora User Guide.
*
*
* Valid for Cluster Type: Aurora DB clusters and Multi-AZ DB clusters
*
*
* Constraints:
*
*
* -
*
* Must be in the format hh24:mi-hh24:mi
.
*
*
* -
*
* Must be in Universal Coordinated Time (UTC).
*
*
* -
*
* Must not conflict with the preferred maintenance window.
*
*
* -
*
* Must be at least 30 minutes.
*
*
*
*/
private String preferredBackupWindow;
/**
*
* The weekly time range during which system maintenance can occur, in Universal Coordinated Time (UTC).
*
*
* Valid for Cluster Type: Aurora DB clusters and Multi-AZ DB clusters
*
*
* The default is a 30-minute window selected at random from an 8-hour block of time for each Amazon Web Services
* Region, occurring on a random day of the week. To see the time blocks available, see Adjusting the Preferred DB Cluster Maintenance Window in the Amazon Aurora User Guide.
*
*
* Constraints:
*
*
* -
*
* Must be in the format ddd:hh24:mi-ddd:hh24:mi
.
*
*
* -
*
* Days must be one of Mon | Tue | Wed | Thu | Fri | Sat | Sun
.
*
*
* -
*
* Must be in Universal Coordinated Time (UTC).
*
*
* -
*
* Must be at least 30 minutes.
*
*
*
*/
private String preferredMaintenanceWindow;
/**
*
* Specifies whether to enable mapping of Amazon Web Services Identity and Access Management (IAM) accounts to
* database accounts. By default, mapping isn't enabled.
*
*
* For more information, see IAM Database
* Authentication in the Amazon Aurora User Guide.
*
*
* Valid for Cluster Type: Aurora DB clusters only
*
*/
private Boolean enableIAMDatabaseAuthentication;
/**
*
* The target backtrack window, in seconds. To disable backtracking, set this value to 0
.
*
*
* Valid for Cluster Type: Aurora MySQL DB clusters only
*
*
* Default: 0
*
*
* Constraints:
*
*
* -
*
* If specified, this value must be set to a number from 0 to 259,200 (72 hours).
*
*
*
*/
private Long backtrackWindow;
/**
*
* The configuration setting for the log types to be enabled for export to CloudWatch Logs for a specific DB
* cluster.
*
*
* Valid for Cluster Type: Aurora DB clusters and Multi-AZ DB clusters
*
*
* The following values are valid for each DB engine:
*
*
* -
*
* Aurora MySQL - audit | error | general | slowquery
*
*
* -
*
* Aurora PostgreSQL - postgresql
*
*
* -
*
* RDS for MySQL - error | general | slowquery
*
*
* -
*
* RDS for PostgreSQL - postgresql | upgrade
*
*
*
*
* For more information about exporting CloudWatch Logs for Amazon RDS, see Publishing Database Logs to Amazon CloudWatch Logs in the Amazon RDS User Guide.
*
*
* For more information about exporting CloudWatch Logs for Amazon Aurora, see Publishing Database Logs to Amazon CloudWatch Logs in the Amazon Aurora User Guide.
*
*/
private CloudwatchLogsExportConfiguration cloudwatchLogsExportConfiguration;
/**
*
* The version number of the database engine to which you want to upgrade. Changing this parameter results in an
* outage. The change is applied during the next maintenance window unless ApplyImmediately
is enabled.
*
*
* If the cluster that you're modifying has one or more read replicas, all replicas must be running an engine
* version that's the same or later than the version you specify.
*
*
* To list all of the available engine versions for Aurora MySQL, use the following command:
*
*
* aws rds describe-db-engine-versions --engine aurora-mysql --query "DBEngineVersions[].EngineVersion"
*
*
* To list all of the available engine versions for Aurora PostgreSQL, use the following command:
*
*
* aws rds describe-db-engine-versions --engine aurora-postgresql --query "DBEngineVersions[].EngineVersion"
*
*
* To list all of the available engine versions for RDS for MySQL, use the following command:
*
*
* aws rds describe-db-engine-versions --engine mysql --query "DBEngineVersions[].EngineVersion"
*
*
* To list all of the available engine versions for RDS for PostgreSQL, use the following command:
*
*
* aws rds describe-db-engine-versions --engine postgres --query "DBEngineVersions[].EngineVersion"
*
*
* Valid for Cluster Type: Aurora DB clusters and Multi-AZ DB clusters
*
*/
private String engineVersion;
/**
*
* Specifies whether major version upgrades are allowed.
*
*
* Valid for Cluster Type: Aurora DB clusters and Multi-AZ DB clusters
*
*
* Constraints:
*
*
* -
*
* You must allow major version upgrades when specifying a value for the EngineVersion
parameter that
* is a different major version than the DB cluster's current version.
*
*
*
*/
private Boolean allowMajorVersionUpgrade;
/**
*
* The name of the DB parameter group to apply to all instances of the DB cluster.
*
*
*
* When you apply a parameter group using the DBInstanceParameterGroupName
parameter, the DB cluster
* isn't rebooted automatically. Also, parameter changes are applied immediately rather than during the next
* maintenance window.
*
*
*
* Valid for Cluster Type: Aurora DB clusters only
*
*
* Default: The existing name setting
*
*
* Constraints:
*
*
* -
*
* The DB parameter group must be in the same DB parameter group family as this DB cluster.
*
*
* -
*
* The DBInstanceParameterGroupName
parameter is valid in combination with the
* AllowMajorVersionUpgrade
parameter for a major version upgrade only.
*
*
*
*/
private String dBInstanceParameterGroupName;
/**
*
* The Active Directory directory ID to move the DB cluster to. Specify none
to remove the cluster from
* its current domain. The domain must be created prior to this operation.
*
*
* For more information, see Kerberos
* Authentication in the Amazon Aurora User Guide.
*
*
* Valid for Cluster Type: Aurora DB clusters only
*
*/
private String domain;
/**
*
* The name of the IAM role to use when making API calls to the Directory Service.
*
*
* Valid for Cluster Type: Aurora DB clusters only
*
*/
private String domainIAMRoleName;
/**
*
* The scaling properties of the DB cluster. You can only modify scaling properties for DB clusters in
* serverless
DB engine mode.
*
*
* Valid for Cluster Type: Aurora DB clusters only
*
*/
private ScalingConfiguration scalingConfiguration;
/**
*
* Specifies whether the DB cluster has deletion protection enabled. The database can't be deleted when deletion
* protection is enabled. By default, deletion protection isn't enabled.
*
*
* Valid for Cluster Type: Aurora DB clusters and Multi-AZ DB clusters
*
*/
private Boolean deletionProtection;
/**
*
* Specifies whether to enable the HTTP endpoint for an Aurora Serverless v1 DB cluster. By default, the HTTP
* endpoint isn't enabled.
*
*
* When enabled, the HTTP endpoint provides a connectionless web service API (RDS Data API) for running SQL queries
* on the Aurora Serverless v1 DB cluster. You can also query your database from inside the RDS console with the RDS
* query editor.
*
*
* For more information, see Using RDS Data API in the
* Amazon Aurora User Guide.
*
*
*
* This parameter applies only to Aurora Serverless v1 DB clusters. To enable or disable the HTTP endpoint for an
* Aurora PostgreSQL Serverless v2 or provisioned DB cluster, use the EnableHttpEndpoint
and
* DisableHttpEndpoint
operations.
*
*
*
* Valid for Cluster Type: Aurora DB clusters only
*
*/
private Boolean enableHttpEndpoint;
/**
*
* Specifies whether to copy all tags from the DB cluster to snapshots of the DB cluster. The default is not to copy
* them.
*
*
* Valid for Cluster Type: Aurora DB clusters and Multi-AZ DB clusters
*
*/
private Boolean copyTagsToSnapshot;
/**
*
* Specifies whether to enable this DB cluster to forward write operations to the primary cluster of a global
* cluster (Aurora global database). By default, write operations are not allowed on Aurora DB clusters that are
* secondary clusters in an Aurora global database.
*
*
* You can set this value only on Aurora DB clusters that are members of an Aurora global database. With this
* parameter enabled, a secondary cluster can forward writes to the current primary cluster, and the resulting
* changes are replicated back to this cluster. For the primary DB cluster of an Aurora global database, this value
* is used immediately if the primary is demoted by a global cluster API operation, but it does nothing until then.
*
*
* Valid for Cluster Type: Aurora DB clusters only
*
*/
private Boolean enableGlobalWriteForwarding;
/**
*
* The compute and memory capacity of each DB instance in the Multi-AZ DB cluster, for example
* db.m6gd.xlarge
. Not all DB instance classes are available in all Amazon Web Services Regions, or for
* all database engines.
*
*
* For the full list of DB instance classes and availability for your engine, see DB Instance
* Class in the Amazon RDS User Guide.
*
*
* Valid for Cluster Type: Multi-AZ DB clusters only
*
*/
private String dBClusterInstanceClass;
/**
*
* The amount of storage in gibibytes (GiB) to allocate to each DB instance in the Multi-AZ DB cluster.
*
*
* Valid for Cluster Type: Multi-AZ DB clusters only
*
*/
private Integer allocatedStorage;
/**
*
* The storage type to associate with the DB cluster.
*
*
* For information on storage types for Aurora DB clusters, see Storage configurations for Amazon Aurora DB clusters. For information on storage types for Multi-AZ DB
* clusters, see Settings for creating Multi-AZ DB clusters.
*
*
* When specified for a Multi-AZ DB cluster, a value for the Iops
parameter is required.
*
*
* Valid for Cluster Type: Aurora DB clusters and Multi-AZ DB clusters
*
*
* Valid Values:
*
*
* -
*
* Aurora DB clusters - aurora | aurora-iopt1
*
*
* -
*
* Multi-AZ DB clusters - io1 | io2 | gp3
*
*
*
*
* Default:
*
*
* -
*
* Aurora DB clusters - aurora
*
*
* -
*
* Multi-AZ DB clusters - io1
*
*
*
*/
private String storageType;
/**
*
* The amount of Provisioned IOPS (input/output operations per second) to be initially allocated for each DB
* instance in the Multi-AZ DB cluster.
*
*
* For information about valid IOPS values, see Amazon RDS Provisioned
* IOPS storage in the Amazon RDS User Guide.
*
*
* Valid for Cluster Type: Multi-AZ DB clusters only
*
*
* Constraints:
*
*
* -
*
* Must be a multiple between .5 and 50 of the storage amount for the DB cluster.
*
*
*
*/
private Integer iops;
/**
*
* Specifies whether minor engine upgrades are applied automatically to the DB cluster during the maintenance
* window. By default, minor engine upgrades are applied automatically.
*
*
* Valid for Cluster Type: Multi-AZ DB clusters only
*
*/
private Boolean autoMinorVersionUpgrade;
/**
*
* The interval, in seconds, between points when Enhanced Monitoring metrics are collected for the DB cluster. To
* turn off collecting Enhanced Monitoring metrics, specify 0
.
*
*
* If MonitoringRoleArn
is specified, also set MonitoringInterval
to a value other than
* 0
.
*
*
* Valid for Cluster Type: Multi-AZ DB clusters only
*
*
* Valid Values: 0 | 1 | 5 | 10 | 15 | 30 | 60
*
*
* Default: 0
*
*/
private Integer monitoringInterval;
/**
*
* The Amazon Resource Name (ARN) for the IAM role that permits RDS to send Enhanced Monitoring metrics to Amazon
* CloudWatch Logs. An example is arn:aws:iam:123456789012:role/emaccess
. For information on creating a
* monitoring role, see To
* create an IAM role for Amazon RDS Enhanced Monitoring in the Amazon RDS User Guide.
*
*
* If MonitoringInterval
is set to a value other than 0
, supply a
* MonitoringRoleArn
value.
*
*
* Valid for Cluster Type: Multi-AZ DB clusters only
*
*/
private String monitoringRoleArn;
/**
*
* Specifies whether to turn on Performance Insights for the DB cluster.
*
*
* For more information, see Using Amazon Performance
* Insights in the Amazon RDS User Guide.
*
*
* Valid for Cluster Type: Multi-AZ DB clusters only
*
*/
private Boolean enablePerformanceInsights;
/**
*
* The Amazon Web Services KMS key identifier for encryption of Performance Insights data.
*
*
* The Amazon Web Services KMS key identifier is the key ARN, key ID, alias ARN, or alias name for the KMS key.
*
*
* If you don't specify a value for PerformanceInsightsKMSKeyId
, then Amazon RDS uses your default KMS
* key. There is a default KMS key for your Amazon Web Services account. Your Amazon Web Services account has a
* different default KMS key for each Amazon Web Services Region.
*
*
* Valid for Cluster Type: Multi-AZ DB clusters only
*
*/
private String performanceInsightsKMSKeyId;
/**
*
* The number of days to retain Performance Insights data.
*
*
* Valid for Cluster Type: Multi-AZ DB clusters only
*
*
* Valid Values:
*
*
* -
*
* 7
*
*
* -
*
* month * 31, where month is a number of months from 1-23. Examples: 93
(3 months * 31),
* 341
(11 months * 31), 589
(19 months * 31)
*
*
* -
*
* 731
*
*
*
*
* Default: 7
days
*
*
* If you specify a retention period that isn't valid, such as 94
, Amazon RDS issues an error.
*
*/
private Integer performanceInsightsRetentionPeriod;
private ServerlessV2ScalingConfiguration serverlessV2ScalingConfiguration;
/**
*
* The network type of the DB cluster.
*
*
* The network type is determined by the DBSubnetGroup
specified for the DB cluster. A
* DBSubnetGroup
can support only the IPv4 protocol or the IPv4 and the IPv6 protocols (
* DUAL
).
*
*
* For more information, see
* Working with a DB instance in a VPC in the Amazon Aurora User Guide.
*
*
* Valid for Cluster Type: Aurora DB clusters only
*
*
* Valid Values: IPV4 | DUAL
*
*/
private String networkType;
/**
*
* Specifies whether to manage the master user password with Amazon Web Services Secrets Manager.
*
*
* If the DB cluster doesn't manage the master user password with Amazon Web Services Secrets Manager, you can turn
* on this management. In this case, you can't specify MasterUserPassword
.
*
*
* If the DB cluster already manages the master user password with Amazon Web Services Secrets Manager, and you
* specify that the master user password is not managed with Amazon Web Services Secrets Manager, then you must
* specify MasterUserPassword
. In this case, RDS deletes the secret and uses the new password for the
* master user specified by MasterUserPassword
.
*
*
* For more information, see Password management with
* Amazon Web Services Secrets Manager in the Amazon RDS User Guide and Password management
* with Amazon Web Services Secrets Manager in the Amazon Aurora User Guide.
*
*
* Valid for Cluster Type: Aurora DB clusters and Multi-AZ DB clusters
*
*/
private Boolean manageMasterUserPassword;
/**
*
* Specifies whether to rotate the secret managed by Amazon Web Services Secrets Manager for the master user
* password.
*
*
* This setting is valid only if the master user password is managed by RDS in Amazon Web Services Secrets Manager
* for the DB cluster. The secret value contains the updated password.
*
*
* For more information, see Password management with
* Amazon Web Services Secrets Manager in the Amazon RDS User Guide and Password management
* with Amazon Web Services Secrets Manager in the Amazon Aurora User Guide.
*
*
* Valid for Cluster Type: Aurora DB clusters and Multi-AZ DB clusters
*
*
* Constraints:
*
*
* -
*
* You must apply the change immediately when rotating the master user password.
*
*
*
*/
private Boolean rotateMasterUserPassword;
/**
*
* The Amazon Web Services KMS key identifier to encrypt a secret that is automatically generated and managed in
* Amazon Web Services Secrets Manager.
*
*
* This setting is valid only if both of the following conditions are met:
*
*
* -
*
* The DB cluster doesn't manage the master user password in Amazon Web Services Secrets Manager.
*
*
* If the DB cluster already manages the master user password in Amazon Web Services Secrets Manager, you can't
* change the KMS key that is used to encrypt the secret.
*
*
* -
*
* You are turning on ManageMasterUserPassword
to manage the master user password in Amazon Web
* Services Secrets Manager.
*
*
* If you are turning on ManageMasterUserPassword
and don't specify
* MasterUserSecretKmsKeyId
, then the aws/secretsmanager
KMS key is used to encrypt the
* secret. If the secret is in a different Amazon Web Services account, then you can't use the
* aws/secretsmanager
KMS key to encrypt the secret, and you must use a customer managed KMS key.
*
*
*
*
* The Amazon Web Services KMS key identifier is the key ARN, key ID, alias ARN, or alias name for the KMS key. To
* use a KMS key in a different Amazon Web Services account, specify the key ARN or alias ARN.
*
*
* There is a default KMS key for your Amazon Web Services account. Your Amazon Web Services account has a different
* default KMS key for each Amazon Web Services Region.
*
*
* Valid for Cluster Type: Aurora DB clusters and Multi-AZ DB clusters
*
*/
private String masterUserSecretKmsKeyId;
/**
*
* The DB engine mode of the DB cluster, either provisioned
or serverless
.
*
*
*
* The DB engine mode can be modified only from serverless
to provisioned
.
*
*
*
* For more information, see CreateDBCluster.
*
*
* Valid for Cluster Type: Aurora DB clusters only
*
*/
private String engineMode;
/**
*
* Specifies whether engine mode changes from serverless
to provisioned
are allowed.
*
*
* Valid for Cluster Type: Aurora Serverless v1 DB clusters only
*
*
* Constraints:
*
*
* -
*
* You must allow engine mode changes when specifying a different value for the EngineMode
parameter
* from the DB cluster's current engine mode.
*
*
*
*/
private Boolean allowEngineModeChange;
/**
*
* Specifies whether read replicas can forward write operations to the writer DB instance in the DB cluster. By
* default, write operations aren't allowed on reader DB instances.
*
*
* Valid for: Aurora DB clusters only
*
*/
private Boolean enableLocalWriteForwarding;
/**
*
* The Amazon Resource Name (ARN) of the recovery point in Amazon Web Services Backup.
*
*/
private String awsBackupRecoveryPointArn;
/**
*
* Specifies whether to enable Aurora Limitless Database. You must enable Aurora Limitless Database to create a DB
* shard group.
*
*
* Valid for: Aurora DB clusters only
*
*/
private Boolean enableLimitlessDatabase;
/**
*
* The CA certificate identifier to use for the DB cluster's server certificate.
*
*
* For more information, see Using SSL/TLS to encrypt a
* connection to a DB instance in the Amazon RDS User Guide.
*
*
* Valid for Cluster Type: Multi-AZ DB clusters
*
*/
private String cACertificateIdentifier;
/**
*
* The DB cluster identifier for the cluster being modified. This parameter isn't case-sensitive.
*
*
* Valid for Cluster Type: Aurora DB clusters and Multi-AZ DB clusters
*
*
* Constraints:
*
*
* -
*
* Must match the identifier of an existing DB cluster.
*
*
*
*
* @param dBClusterIdentifier
* The DB cluster identifier for the cluster being modified. This parameter isn't case-sensitive.
*
* Valid for Cluster Type: Aurora DB clusters and Multi-AZ DB clusters
*
*
* Constraints:
*
*
* -
*
* Must match the identifier of an existing DB cluster.
*
*
*/
public void setDBClusterIdentifier(String dBClusterIdentifier) {
this.dBClusterIdentifier = dBClusterIdentifier;
}
/**
*
* The DB cluster identifier for the cluster being modified. This parameter isn't case-sensitive.
*
*
* Valid for Cluster Type: Aurora DB clusters and Multi-AZ DB clusters
*
*
* Constraints:
*
*
* -
*
* Must match the identifier of an existing DB cluster.
*
*
*
*
* @return The DB cluster identifier for the cluster being modified. This parameter isn't case-sensitive.
*
* Valid for Cluster Type: Aurora DB clusters and Multi-AZ DB clusters
*
*
* Constraints:
*
*
* -
*
* Must match the identifier of an existing DB cluster.
*
*
*/
public String getDBClusterIdentifier() {
return this.dBClusterIdentifier;
}
/**
*
* The DB cluster identifier for the cluster being modified. This parameter isn't case-sensitive.
*
*
* Valid for Cluster Type: Aurora DB clusters and Multi-AZ DB clusters
*
*
* Constraints:
*
*
* -
*
* Must match the identifier of an existing DB cluster.
*
*
*
*
* @param dBClusterIdentifier
* The DB cluster identifier for the cluster being modified. This parameter isn't case-sensitive.
*
* Valid for Cluster Type: Aurora DB clusters and Multi-AZ DB clusters
*
*
* Constraints:
*
*
* -
*
* Must match the identifier of an existing DB cluster.
*
*
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ModifyDBClusterRequest withDBClusterIdentifier(String dBClusterIdentifier) {
setDBClusterIdentifier(dBClusterIdentifier);
return this;
}
/**
*
* The new DB cluster identifier for the DB cluster when renaming a DB cluster. This value is stored as a lowercase
* string.
*
*
* Valid for Cluster Type: Aurora DB clusters and Multi-AZ DB clusters
*
*
* Constraints:
*
*
* -
*
* Must contain from 1 to 63 letters, numbers, or hyphens.
*
*
* -
*
* The first character must be a letter.
*
*
* -
*
* Can't end with a hyphen or contain two consecutive hyphens.
*
*
*
*
* Example: my-cluster2
*
*
* @param newDBClusterIdentifier
* The new DB cluster identifier for the DB cluster when renaming a DB cluster. This value is stored as a
* lowercase string.
*
* Valid for Cluster Type: Aurora DB clusters and Multi-AZ DB clusters
*
*
* Constraints:
*
*
* -
*
* Must contain from 1 to 63 letters, numbers, or hyphens.
*
*
* -
*
* The first character must be a letter.
*
*
* -
*
* Can't end with a hyphen or contain two consecutive hyphens.
*
*
*
*
* Example: my-cluster2
*/
public void setNewDBClusterIdentifier(String newDBClusterIdentifier) {
this.newDBClusterIdentifier = newDBClusterIdentifier;
}
/**
*
* The new DB cluster identifier for the DB cluster when renaming a DB cluster. This value is stored as a lowercase
* string.
*
*
* Valid for Cluster Type: Aurora DB clusters and Multi-AZ DB clusters
*
*
* Constraints:
*
*
* -
*
* Must contain from 1 to 63 letters, numbers, or hyphens.
*
*
* -
*
* The first character must be a letter.
*
*
* -
*
* Can't end with a hyphen or contain two consecutive hyphens.
*
*
*
*
* Example: my-cluster2
*
*
* @return The new DB cluster identifier for the DB cluster when renaming a DB cluster. This value is stored as a
* lowercase string.
*
* Valid for Cluster Type: Aurora DB clusters and Multi-AZ DB clusters
*
*
* Constraints:
*
*
* -
*
* Must contain from 1 to 63 letters, numbers, or hyphens.
*
*
* -
*
* The first character must be a letter.
*
*
* -
*
* Can't end with a hyphen or contain two consecutive hyphens.
*
*
*
*
* Example: my-cluster2
*/
public String getNewDBClusterIdentifier() {
return this.newDBClusterIdentifier;
}
/**
*
* The new DB cluster identifier for the DB cluster when renaming a DB cluster. This value is stored as a lowercase
* string.
*
*
* Valid for Cluster Type: Aurora DB clusters and Multi-AZ DB clusters
*
*
* Constraints:
*
*
* -
*
* Must contain from 1 to 63 letters, numbers, or hyphens.
*
*
* -
*
* The first character must be a letter.
*
*
* -
*
* Can't end with a hyphen or contain two consecutive hyphens.
*
*
*
*
* Example: my-cluster2
*
*
* @param newDBClusterIdentifier
* The new DB cluster identifier for the DB cluster when renaming a DB cluster. This value is stored as a
* lowercase string.
*
* Valid for Cluster Type: Aurora DB clusters and Multi-AZ DB clusters
*
*
* Constraints:
*
*
* -
*
* Must contain from 1 to 63 letters, numbers, or hyphens.
*
*
* -
*
* The first character must be a letter.
*
*
* -
*
* Can't end with a hyphen or contain two consecutive hyphens.
*
*
*
*
* Example: my-cluster2
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ModifyDBClusterRequest withNewDBClusterIdentifier(String newDBClusterIdentifier) {
setNewDBClusterIdentifier(newDBClusterIdentifier);
return this;
}
/**
*
* Specifies whether the modifications in this request and any pending modifications are asynchronously applied as
* soon as possible, regardless of the PreferredMaintenanceWindow
setting for the DB cluster. If this
* parameter is disabled, changes to the DB cluster are applied during the next maintenance window.
*
*
* Most modifications can be applied immediately or during the next scheduled maintenance window. Some
* modifications, such as turning on deletion protection and changing the master password, are applied
* immediately—regardless of when you choose to apply them.
*
*
* By default, this parameter is disabled.
*
*
* Valid for Cluster Type: Aurora DB clusters and Multi-AZ DB clusters
*
*
* @param applyImmediately
* Specifies whether the modifications in this request and any pending modifications are asynchronously
* applied as soon as possible, regardless of the PreferredMaintenanceWindow
setting for the DB
* cluster. If this parameter is disabled, changes to the DB cluster are applied during the next maintenance
* window.
*
* Most modifications can be applied immediately or during the next scheduled maintenance window. Some
* modifications, such as turning on deletion protection and changing the master password, are applied
* immediately—regardless of when you choose to apply them.
*
*
* By default, this parameter is disabled.
*
*
* Valid for Cluster Type: Aurora DB clusters and Multi-AZ DB clusters
*/
public void setApplyImmediately(Boolean applyImmediately) {
this.applyImmediately = applyImmediately;
}
/**
*
* Specifies whether the modifications in this request and any pending modifications are asynchronously applied as
* soon as possible, regardless of the PreferredMaintenanceWindow
setting for the DB cluster. If this
* parameter is disabled, changes to the DB cluster are applied during the next maintenance window.
*
*
* Most modifications can be applied immediately or during the next scheduled maintenance window. Some
* modifications, such as turning on deletion protection and changing the master password, are applied
* immediately—regardless of when you choose to apply them.
*
*
* By default, this parameter is disabled.
*
*
* Valid for Cluster Type: Aurora DB clusters and Multi-AZ DB clusters
*
*
* @return Specifies whether the modifications in this request and any pending modifications are asynchronously
* applied as soon as possible, regardless of the PreferredMaintenanceWindow
setting for the DB
* cluster. If this parameter is disabled, changes to the DB cluster are applied during the next maintenance
* window.
*
* Most modifications can be applied immediately or during the next scheduled maintenance window. Some
* modifications, such as turning on deletion protection and changing the master password, are applied
* immediately—regardless of when you choose to apply them.
*
*
* By default, this parameter is disabled.
*
*
* Valid for Cluster Type: Aurora DB clusters and Multi-AZ DB clusters
*/
public Boolean getApplyImmediately() {
return this.applyImmediately;
}
/**
*
* Specifies whether the modifications in this request and any pending modifications are asynchronously applied as
* soon as possible, regardless of the PreferredMaintenanceWindow
setting for the DB cluster. If this
* parameter is disabled, changes to the DB cluster are applied during the next maintenance window.
*
*
* Most modifications can be applied immediately or during the next scheduled maintenance window. Some
* modifications, such as turning on deletion protection and changing the master password, are applied
* immediately—regardless of when you choose to apply them.
*
*
* By default, this parameter is disabled.
*
*
* Valid for Cluster Type: Aurora DB clusters and Multi-AZ DB clusters
*
*
* @param applyImmediately
* Specifies whether the modifications in this request and any pending modifications are asynchronously
* applied as soon as possible, regardless of the PreferredMaintenanceWindow
setting for the DB
* cluster. If this parameter is disabled, changes to the DB cluster are applied during the next maintenance
* window.
*
* Most modifications can be applied immediately or during the next scheduled maintenance window. Some
* modifications, such as turning on deletion protection and changing the master password, are applied
* immediately—regardless of when you choose to apply them.
*
*
* By default, this parameter is disabled.
*
*
* Valid for Cluster Type: Aurora DB clusters and Multi-AZ DB clusters
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ModifyDBClusterRequest withApplyImmediately(Boolean applyImmediately) {
setApplyImmediately(applyImmediately);
return this;
}
/**
*
* Specifies whether the modifications in this request and any pending modifications are asynchronously applied as
* soon as possible, regardless of the PreferredMaintenanceWindow
setting for the DB cluster. If this
* parameter is disabled, changes to the DB cluster are applied during the next maintenance window.
*
*
* Most modifications can be applied immediately or during the next scheduled maintenance window. Some
* modifications, such as turning on deletion protection and changing the master password, are applied
* immediately—regardless of when you choose to apply them.
*
*
* By default, this parameter is disabled.
*
*
* Valid for Cluster Type: Aurora DB clusters and Multi-AZ DB clusters
*
*
* @return Specifies whether the modifications in this request and any pending modifications are asynchronously
* applied as soon as possible, regardless of the PreferredMaintenanceWindow
setting for the DB
* cluster. If this parameter is disabled, changes to the DB cluster are applied during the next maintenance
* window.
*
* Most modifications can be applied immediately or during the next scheduled maintenance window. Some
* modifications, such as turning on deletion protection and changing the master password, are applied
* immediately—regardless of when you choose to apply them.
*
*
* By default, this parameter is disabled.
*
*
* Valid for Cluster Type: Aurora DB clusters and Multi-AZ DB clusters
*/
public Boolean isApplyImmediately() {
return this.applyImmediately;
}
/**
*
* The number of days for which automated backups are retained. Specify a minimum value of 1
.
*
*
* Valid for Cluster Type: Aurora DB clusters and Multi-AZ DB clusters
*
*
* Default: 1
*
*
* Constraints:
*
*
* -
*
* Must be a value from 1 to 35.
*
*
*
*
* @param backupRetentionPeriod
* The number of days for which automated backups are retained. Specify a minimum value of 1
* .
*
* Valid for Cluster Type: Aurora DB clusters and Multi-AZ DB clusters
*
*
* Default: 1
*
*
* Constraints:
*
*
* -
*
* Must be a value from 1 to 35.
*
*
*/
public void setBackupRetentionPeriod(Integer backupRetentionPeriod) {
this.backupRetentionPeriod = backupRetentionPeriod;
}
/**
*
* The number of days for which automated backups are retained. Specify a minimum value of 1
.
*
*
* Valid for Cluster Type: Aurora DB clusters and Multi-AZ DB clusters
*
*
* Default: 1
*
*
* Constraints:
*
*
* -
*
* Must be a value from 1 to 35.
*
*
*
*
* @return The number of days for which automated backups are retained. Specify a minimum value of 1
* .
*
* Valid for Cluster Type: Aurora DB clusters and Multi-AZ DB clusters
*
*
* Default: 1
*
*
* Constraints:
*
*
* -
*
* Must be a value from 1 to 35.
*
*
*/
public Integer getBackupRetentionPeriod() {
return this.backupRetentionPeriod;
}
/**
*
* The number of days for which automated backups are retained. Specify a minimum value of 1
.
*
*
* Valid for Cluster Type: Aurora DB clusters and Multi-AZ DB clusters
*
*
* Default: 1
*
*
* Constraints:
*
*
* -
*
* Must be a value from 1 to 35.
*
*
*
*
* @param backupRetentionPeriod
* The number of days for which automated backups are retained. Specify a minimum value of 1
* .
*
* Valid for Cluster Type: Aurora DB clusters and Multi-AZ DB clusters
*
*
* Default: 1
*
*
* Constraints:
*
*
* -
*
* Must be a value from 1 to 35.
*
*
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ModifyDBClusterRequest withBackupRetentionPeriod(Integer backupRetentionPeriod) {
setBackupRetentionPeriod(backupRetentionPeriod);
return this;
}
/**
*
* The name of the DB cluster parameter group to use for the DB cluster.
*
*
* Valid for Cluster Type: Aurora DB clusters and Multi-AZ DB clusters
*
*
* @param dBClusterParameterGroupName
* The name of the DB cluster parameter group to use for the DB cluster.
*
* Valid for Cluster Type: Aurora DB clusters and Multi-AZ DB clusters
*/
public void setDBClusterParameterGroupName(String dBClusterParameterGroupName) {
this.dBClusterParameterGroupName = dBClusterParameterGroupName;
}
/**
*
* The name of the DB cluster parameter group to use for the DB cluster.
*
*
* Valid for Cluster Type: Aurora DB clusters and Multi-AZ DB clusters
*
*
* @return The name of the DB cluster parameter group to use for the DB cluster.
*
* Valid for Cluster Type: Aurora DB clusters and Multi-AZ DB clusters
*/
public String getDBClusterParameterGroupName() {
return this.dBClusterParameterGroupName;
}
/**
*
* The name of the DB cluster parameter group to use for the DB cluster.
*
*
* Valid for Cluster Type: Aurora DB clusters and Multi-AZ DB clusters
*
*
* @param dBClusterParameterGroupName
* The name of the DB cluster parameter group to use for the DB cluster.
*
* Valid for Cluster Type: Aurora DB clusters and Multi-AZ DB clusters
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ModifyDBClusterRequest withDBClusterParameterGroupName(String dBClusterParameterGroupName) {
setDBClusterParameterGroupName(dBClusterParameterGroupName);
return this;
}
/**
*
* A list of EC2 VPC security groups to associate with this DB cluster.
*
*
* Valid for Cluster Type: Aurora DB clusters and Multi-AZ DB clusters
*
*
* @return A list of EC2 VPC security groups to associate with this DB cluster.
*
* Valid for Cluster Type: Aurora DB clusters and Multi-AZ DB clusters
*/
public java.util.List getVpcSecurityGroupIds() {
if (vpcSecurityGroupIds == null) {
vpcSecurityGroupIds = new com.amazonaws.internal.SdkInternalList();
}
return vpcSecurityGroupIds;
}
/**
*
* A list of EC2 VPC security groups to associate with this DB cluster.
*
*
* Valid for Cluster Type: Aurora DB clusters and Multi-AZ DB clusters
*
*
* @param vpcSecurityGroupIds
* A list of EC2 VPC security groups to associate with this DB cluster.
*
* Valid for Cluster Type: Aurora DB clusters and Multi-AZ DB clusters
*/
public void setVpcSecurityGroupIds(java.util.Collection vpcSecurityGroupIds) {
if (vpcSecurityGroupIds == null) {
this.vpcSecurityGroupIds = null;
return;
}
this.vpcSecurityGroupIds = new com.amazonaws.internal.SdkInternalList(vpcSecurityGroupIds);
}
/**
*
* A list of EC2 VPC security groups to associate with this DB cluster.
*
*
* Valid for Cluster Type: Aurora DB clusters and Multi-AZ DB clusters
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setVpcSecurityGroupIds(java.util.Collection)} or {@link #withVpcSecurityGroupIds(java.util.Collection)}
* if you want to override the existing values.
*
*
* @param vpcSecurityGroupIds
* A list of EC2 VPC security groups to associate with this DB cluster.
*
* Valid for Cluster Type: Aurora DB clusters and Multi-AZ DB clusters
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ModifyDBClusterRequest withVpcSecurityGroupIds(String... vpcSecurityGroupIds) {
if (this.vpcSecurityGroupIds == null) {
setVpcSecurityGroupIds(new com.amazonaws.internal.SdkInternalList(vpcSecurityGroupIds.length));
}
for (String ele : vpcSecurityGroupIds) {
this.vpcSecurityGroupIds.add(ele);
}
return this;
}
/**
*
* A list of EC2 VPC security groups to associate with this DB cluster.
*
*
* Valid for Cluster Type: Aurora DB clusters and Multi-AZ DB clusters
*
*
* @param vpcSecurityGroupIds
* A list of EC2 VPC security groups to associate with this DB cluster.
*
* Valid for Cluster Type: Aurora DB clusters and Multi-AZ DB clusters
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ModifyDBClusterRequest withVpcSecurityGroupIds(java.util.Collection vpcSecurityGroupIds) {
setVpcSecurityGroupIds(vpcSecurityGroupIds);
return this;
}
/**
*
* The port number on which the DB cluster accepts connections.
*
*
* Valid for Cluster Type: Aurora DB clusters only
*
*
* Valid Values: 1150-65535
*
*
* Default: The same port as the original DB cluster.
*
*
* @param port
* The port number on which the DB cluster accepts connections.
*
* Valid for Cluster Type: Aurora DB clusters only
*
*
* Valid Values: 1150-65535
*
*
* Default: The same port as the original DB cluster.
*/
public void setPort(Integer port) {
this.port = port;
}
/**
*
* The port number on which the DB cluster accepts connections.
*
*
* Valid for Cluster Type: Aurora DB clusters only
*
*
* Valid Values: 1150-65535
*
*
* Default: The same port as the original DB cluster.
*
*
* @return The port number on which the DB cluster accepts connections.
*
* Valid for Cluster Type: Aurora DB clusters only
*
*
* Valid Values: 1150-65535
*
*
* Default: The same port as the original DB cluster.
*/
public Integer getPort() {
return this.port;
}
/**
*
* The port number on which the DB cluster accepts connections.
*
*
* Valid for Cluster Type: Aurora DB clusters only
*
*
* Valid Values: 1150-65535
*
*
* Default: The same port as the original DB cluster.
*
*
* @param port
* The port number on which the DB cluster accepts connections.
*
* Valid for Cluster Type: Aurora DB clusters only
*
*
* Valid Values: 1150-65535
*
*
* Default: The same port as the original DB cluster.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ModifyDBClusterRequest withPort(Integer port) {
setPort(port);
return this;
}
/**
*
* The new password for the master database user.
*
*
* Valid for Cluster Type: Aurora DB clusters and Multi-AZ DB clusters
*
*
* Constraints:
*
*
* -
*
* Must contain from 8 to 41 characters.
*
*
* -
*
* Can contain any printable ASCII character except "/", """, or "@".
*
*
* -
*
* Can't be specified if ManageMasterUserPassword
is turned on.
*
*
*
*
* @param masterUserPassword
* The new password for the master database user.
*
* Valid for Cluster Type: Aurora DB clusters and Multi-AZ DB clusters
*
*
* Constraints:
*
*
* -
*
* Must contain from 8 to 41 characters.
*
*
* -
*
* Can contain any printable ASCII character except "/", """, or "@".
*
*
* -
*
* Can't be specified if ManageMasterUserPassword
is turned on.
*
*
*/
public void setMasterUserPassword(String masterUserPassword) {
this.masterUserPassword = masterUserPassword;
}
/**
*
* The new password for the master database user.
*
*
* Valid for Cluster Type: Aurora DB clusters and Multi-AZ DB clusters
*
*
* Constraints:
*
*
* -
*
* Must contain from 8 to 41 characters.
*
*
* -
*
* Can contain any printable ASCII character except "/", """, or "@".
*
*
* -
*
* Can't be specified if ManageMasterUserPassword
is turned on.
*
*
*
*
* @return The new password for the master database user.
*
* Valid for Cluster Type: Aurora DB clusters and Multi-AZ DB clusters
*
*
* Constraints:
*
*
* -
*
* Must contain from 8 to 41 characters.
*
*
* -
*
* Can contain any printable ASCII character except "/", """, or "@".
*
*
* -
*
* Can't be specified if ManageMasterUserPassword
is turned on.
*
*
*/
public String getMasterUserPassword() {
return this.masterUserPassword;
}
/**
*
* The new password for the master database user.
*
*
* Valid for Cluster Type: Aurora DB clusters and Multi-AZ DB clusters
*
*
* Constraints:
*
*
* -
*
* Must contain from 8 to 41 characters.
*
*
* -
*
* Can contain any printable ASCII character except "/", """, or "@".
*
*
* -
*
* Can't be specified if ManageMasterUserPassword
is turned on.
*
*
*
*
* @param masterUserPassword
* The new password for the master database user.
*
* Valid for Cluster Type: Aurora DB clusters and Multi-AZ DB clusters
*
*
* Constraints:
*
*
* -
*
* Must contain from 8 to 41 characters.
*
*
* -
*
* Can contain any printable ASCII character except "/", """, or "@".
*
*
* -
*
* Can't be specified if ManageMasterUserPassword
is turned on.
*
*
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ModifyDBClusterRequest withMasterUserPassword(String masterUserPassword) {
setMasterUserPassword(masterUserPassword);
return this;
}
/**
*
* The option group to associate the DB cluster with.
*
*
* DB clusters are associated with a default option group that can't be modified.
*
*
* @param optionGroupName
* The option group to associate the DB cluster with.
*
* DB clusters are associated with a default option group that can't be modified.
*/
public void setOptionGroupName(String optionGroupName) {
this.optionGroupName = optionGroupName;
}
/**
*
* The option group to associate the DB cluster with.
*
*
* DB clusters are associated with a default option group that can't be modified.
*
*
* @return The option group to associate the DB cluster with.
*
* DB clusters are associated with a default option group that can't be modified.
*/
public String getOptionGroupName() {
return this.optionGroupName;
}
/**
*
* The option group to associate the DB cluster with.
*
*
* DB clusters are associated with a default option group that can't be modified.
*
*
* @param optionGroupName
* The option group to associate the DB cluster with.
*
* DB clusters are associated with a default option group that can't be modified.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ModifyDBClusterRequest withOptionGroupName(String optionGroupName) {
setOptionGroupName(optionGroupName);
return this;
}
/**
*
* The daily time range during which automated backups are created if automated backups are enabled, using the
* BackupRetentionPeriod
parameter.
*
*
* The default is a 30-minute window selected at random from an 8-hour block of time for each Amazon Web Services
* Region. To view the time blocks available, see Backup window in the Amazon Aurora User Guide.
*
*
* Valid for Cluster Type: Aurora DB clusters and Multi-AZ DB clusters
*
*
* Constraints:
*
*
* -
*
* Must be in the format hh24:mi-hh24:mi
.
*
*
* -
*
* Must be in Universal Coordinated Time (UTC).
*
*
* -
*
* Must not conflict with the preferred maintenance window.
*
*
* -
*
* Must be at least 30 minutes.
*
*
*
*
* @param preferredBackupWindow
* The daily time range during which automated backups are created if automated backups are enabled, using
* the BackupRetentionPeriod
parameter.
*
* The default is a 30-minute window selected at random from an 8-hour block of time for each Amazon Web
* Services Region. To view the time blocks available, see Backup window in the Amazon Aurora User Guide.
*
*
* Valid for Cluster Type: Aurora DB clusters and Multi-AZ DB clusters
*
*
* Constraints:
*
*
* -
*
* Must be in the format hh24:mi-hh24:mi
.
*
*
* -
*
* Must be in Universal Coordinated Time (UTC).
*
*
* -
*
* Must not conflict with the preferred maintenance window.
*
*
* -
*
* Must be at least 30 minutes.
*
*
*/
public void setPreferredBackupWindow(String preferredBackupWindow) {
this.preferredBackupWindow = preferredBackupWindow;
}
/**
*
* The daily time range during which automated backups are created if automated backups are enabled, using the
* BackupRetentionPeriod
parameter.
*
*
* The default is a 30-minute window selected at random from an 8-hour block of time for each Amazon Web Services
* Region. To view the time blocks available, see Backup window in the Amazon Aurora User Guide.
*
*
* Valid for Cluster Type: Aurora DB clusters and Multi-AZ DB clusters
*
*
* Constraints:
*
*
* -
*
* Must be in the format hh24:mi-hh24:mi
.
*
*
* -
*
* Must be in Universal Coordinated Time (UTC).
*
*
* -
*
* Must not conflict with the preferred maintenance window.
*
*
* -
*
* Must be at least 30 minutes.
*
*
*
*
* @return The daily time range during which automated backups are created if automated backups are enabled, using
* the BackupRetentionPeriod
parameter.
*
* The default is a 30-minute window selected at random from an 8-hour block of time for each Amazon Web
* Services Region. To view the time blocks available, see Backup window in the Amazon Aurora User Guide.
*
*
* Valid for Cluster Type: Aurora DB clusters and Multi-AZ DB clusters
*
*
* Constraints:
*
*
* -
*
* Must be in the format hh24:mi-hh24:mi
.
*
*
* -
*
* Must be in Universal Coordinated Time (UTC).
*
*
* -
*
* Must not conflict with the preferred maintenance window.
*
*
* -
*
* Must be at least 30 minutes.
*
*
*/
public String getPreferredBackupWindow() {
return this.preferredBackupWindow;
}
/**
*
* The daily time range during which automated backups are created if automated backups are enabled, using the
* BackupRetentionPeriod
parameter.
*
*
* The default is a 30-minute window selected at random from an 8-hour block of time for each Amazon Web Services
* Region. To view the time blocks available, see Backup window in the Amazon Aurora User Guide.
*
*
* Valid for Cluster Type: Aurora DB clusters and Multi-AZ DB clusters
*
*
* Constraints:
*
*
* -
*
* Must be in the format hh24:mi-hh24:mi
.
*
*
* -
*
* Must be in Universal Coordinated Time (UTC).
*
*
* -
*
* Must not conflict with the preferred maintenance window.
*
*
* -
*
* Must be at least 30 minutes.
*
*
*
*
* @param preferredBackupWindow
* The daily time range during which automated backups are created if automated backups are enabled, using
* the BackupRetentionPeriod
parameter.
*
* The default is a 30-minute window selected at random from an 8-hour block of time for each Amazon Web
* Services Region. To view the time blocks available, see Backup window in the Amazon Aurora User Guide.
*
*
* Valid for Cluster Type: Aurora DB clusters and Multi-AZ DB clusters
*
*
* Constraints:
*
*
* -
*
* Must be in the format hh24:mi-hh24:mi
.
*
*
* -
*
* Must be in Universal Coordinated Time (UTC).
*
*
* -
*
* Must not conflict with the preferred maintenance window.
*
*
* -
*
* Must be at least 30 minutes.
*
*
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ModifyDBClusterRequest withPreferredBackupWindow(String preferredBackupWindow) {
setPreferredBackupWindow(preferredBackupWindow);
return this;
}
/**
*
* The weekly time range during which system maintenance can occur, in Universal Coordinated Time (UTC).
*
*
* Valid for Cluster Type: Aurora DB clusters and Multi-AZ DB clusters
*
*
* The default is a 30-minute window selected at random from an 8-hour block of time for each Amazon Web Services
* Region, occurring on a random day of the week. To see the time blocks available, see Adjusting the Preferred DB Cluster Maintenance Window in the Amazon Aurora User Guide.
*
*
* Constraints:
*
*
* -
*
* Must be in the format ddd:hh24:mi-ddd:hh24:mi
.
*
*
* -
*
* Days must be one of Mon | Tue | Wed | Thu | Fri | Sat | Sun
.
*
*
* -
*
* Must be in Universal Coordinated Time (UTC).
*
*
* -
*
* Must be at least 30 minutes.
*
*
*
*
* @param preferredMaintenanceWindow
* The weekly time range during which system maintenance can occur, in Universal Coordinated Time (UTC).
*
* Valid for Cluster Type: Aurora DB clusters and Multi-AZ DB clusters
*
*
* The default is a 30-minute window selected at random from an 8-hour block of time for each Amazon Web
* Services Region, occurring on a random day of the week. To see the time blocks available, see Adjusting the Preferred DB Cluster Maintenance Window in the Amazon Aurora User Guide.
*
*
* Constraints:
*
*
* -
*
* Must be in the format ddd:hh24:mi-ddd:hh24:mi
.
*
*
* -
*
* Days must be one of Mon | Tue | Wed | Thu | Fri | Sat | Sun
.
*
*
* -
*
* Must be in Universal Coordinated Time (UTC).
*
*
* -
*
* Must be at least 30 minutes.
*
*
*/
public void setPreferredMaintenanceWindow(String preferredMaintenanceWindow) {
this.preferredMaintenanceWindow = preferredMaintenanceWindow;
}
/**
*
* The weekly time range during which system maintenance can occur, in Universal Coordinated Time (UTC).
*
*
* Valid for Cluster Type: Aurora DB clusters and Multi-AZ DB clusters
*
*
* The default is a 30-minute window selected at random from an 8-hour block of time for each Amazon Web Services
* Region, occurring on a random day of the week. To see the time blocks available, see Adjusting the Preferred DB Cluster Maintenance Window in the Amazon Aurora User Guide.
*
*
* Constraints:
*
*
* -
*
* Must be in the format ddd:hh24:mi-ddd:hh24:mi
.
*
*
* -
*
* Days must be one of Mon | Tue | Wed | Thu | Fri | Sat | Sun
.
*
*
* -
*
* Must be in Universal Coordinated Time (UTC).
*
*
* -
*
* Must be at least 30 minutes.
*
*
*
*
* @return The weekly time range during which system maintenance can occur, in Universal Coordinated Time (UTC).
*
* Valid for Cluster Type: Aurora DB clusters and Multi-AZ DB clusters
*
*
* The default is a 30-minute window selected at random from an 8-hour block of time for each Amazon Web
* Services Region, occurring on a random day of the week. To see the time blocks available, see Adjusting the Preferred DB Cluster Maintenance Window in the Amazon Aurora User Guide.
*
*
* Constraints:
*
*
* -
*
* Must be in the format ddd:hh24:mi-ddd:hh24:mi
.
*
*
* -
*
* Days must be one of Mon | Tue | Wed | Thu | Fri | Sat | Sun
.
*
*
* -
*
* Must be in Universal Coordinated Time (UTC).
*
*
* -
*
* Must be at least 30 minutes.
*
*
*/
public String getPreferredMaintenanceWindow() {
return this.preferredMaintenanceWindow;
}
/**
*
* The weekly time range during which system maintenance can occur, in Universal Coordinated Time (UTC).
*
*
* Valid for Cluster Type: Aurora DB clusters and Multi-AZ DB clusters
*
*
* The default is a 30-minute window selected at random from an 8-hour block of time for each Amazon Web Services
* Region, occurring on a random day of the week. To see the time blocks available, see Adjusting the Preferred DB Cluster Maintenance Window in the Amazon Aurora User Guide.
*
*
* Constraints:
*
*
* -
*
* Must be in the format ddd:hh24:mi-ddd:hh24:mi
.
*
*
* -
*
* Days must be one of Mon | Tue | Wed | Thu | Fri | Sat | Sun
.
*
*
* -
*
* Must be in Universal Coordinated Time (UTC).
*
*
* -
*
* Must be at least 30 minutes.
*
*
*
*
* @param preferredMaintenanceWindow
* The weekly time range during which system maintenance can occur, in Universal Coordinated Time (UTC).
*
* Valid for Cluster Type: Aurora DB clusters and Multi-AZ DB clusters
*
*
* The default is a 30-minute window selected at random from an 8-hour block of time for each Amazon Web
* Services Region, occurring on a random day of the week. To see the time blocks available, see Adjusting the Preferred DB Cluster Maintenance Window in the Amazon Aurora User Guide.
*
*
* Constraints:
*
*
* -
*
* Must be in the format ddd:hh24:mi-ddd:hh24:mi
.
*
*
* -
*
* Days must be one of Mon | Tue | Wed | Thu | Fri | Sat | Sun
.
*
*
* -
*
* Must be in Universal Coordinated Time (UTC).
*
*
* -
*
* Must be at least 30 minutes.
*
*
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ModifyDBClusterRequest withPreferredMaintenanceWindow(String preferredMaintenanceWindow) {
setPreferredMaintenanceWindow(preferredMaintenanceWindow);
return this;
}
/**
*
* Specifies whether to enable mapping of Amazon Web Services Identity and Access Management (IAM) accounts to
* database accounts. By default, mapping isn't enabled.
*
*
* For more information, see IAM Database
* Authentication in the Amazon Aurora User Guide.
*
*
* Valid for Cluster Type: Aurora DB clusters only
*
*
* @param enableIAMDatabaseAuthentication
* Specifies whether to enable mapping of Amazon Web Services Identity and Access Management (IAM) accounts
* to database accounts. By default, mapping isn't enabled.
*
* For more information, see IAM
* Database Authentication in the Amazon Aurora User Guide.
*
*
* Valid for Cluster Type: Aurora DB clusters only
*/
public void setEnableIAMDatabaseAuthentication(Boolean enableIAMDatabaseAuthentication) {
this.enableIAMDatabaseAuthentication = enableIAMDatabaseAuthentication;
}
/**
*
* Specifies whether to enable mapping of Amazon Web Services Identity and Access Management (IAM) accounts to
* database accounts. By default, mapping isn't enabled.
*
*
* For more information, see IAM Database
* Authentication in the Amazon Aurora User Guide.
*
*
* Valid for Cluster Type: Aurora DB clusters only
*
*
* @return Specifies whether to enable mapping of Amazon Web Services Identity and Access Management (IAM) accounts
* to database accounts. By default, mapping isn't enabled.
*
* For more information, see IAM
* Database Authentication in the Amazon Aurora User Guide.
*
*
* Valid for Cluster Type: Aurora DB clusters only
*/
public Boolean getEnableIAMDatabaseAuthentication() {
return this.enableIAMDatabaseAuthentication;
}
/**
*
* Specifies whether to enable mapping of Amazon Web Services Identity and Access Management (IAM) accounts to
* database accounts. By default, mapping isn't enabled.
*
*
* For more information, see IAM Database
* Authentication in the Amazon Aurora User Guide.
*
*
* Valid for Cluster Type: Aurora DB clusters only
*
*
* @param enableIAMDatabaseAuthentication
* Specifies whether to enable mapping of Amazon Web Services Identity and Access Management (IAM) accounts
* to database accounts. By default, mapping isn't enabled.
*
* For more information, see IAM
* Database Authentication in the Amazon Aurora User Guide.
*
*
* Valid for Cluster Type: Aurora DB clusters only
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ModifyDBClusterRequest withEnableIAMDatabaseAuthentication(Boolean enableIAMDatabaseAuthentication) {
setEnableIAMDatabaseAuthentication(enableIAMDatabaseAuthentication);
return this;
}
/**
*
* Specifies whether to enable mapping of Amazon Web Services Identity and Access Management (IAM) accounts to
* database accounts. By default, mapping isn't enabled.
*
*
* For more information, see IAM Database
* Authentication in the Amazon Aurora User Guide.
*
*
* Valid for Cluster Type: Aurora DB clusters only
*
*
* @return Specifies whether to enable mapping of Amazon Web Services Identity and Access Management (IAM) accounts
* to database accounts. By default, mapping isn't enabled.
*
* For more information, see IAM
* Database Authentication in the Amazon Aurora User Guide.
*
*
* Valid for Cluster Type: Aurora DB clusters only
*/
public Boolean isEnableIAMDatabaseAuthentication() {
return this.enableIAMDatabaseAuthentication;
}
/**
*
* The target backtrack window, in seconds. To disable backtracking, set this value to 0
.
*
*
* Valid for Cluster Type: Aurora MySQL DB clusters only
*
*
* Default: 0
*
*
* Constraints:
*
*
* -
*
* If specified, this value must be set to a number from 0 to 259,200 (72 hours).
*
*
*
*
* @param backtrackWindow
* The target backtrack window, in seconds. To disable backtracking, set this value to 0
.
*
* Valid for Cluster Type: Aurora MySQL DB clusters only
*
*
* Default: 0
*
*
* Constraints:
*
*
* -
*
* If specified, this value must be set to a number from 0 to 259,200 (72 hours).
*
*
*/
public void setBacktrackWindow(Long backtrackWindow) {
this.backtrackWindow = backtrackWindow;
}
/**
*
* The target backtrack window, in seconds. To disable backtracking, set this value to 0
.
*
*
* Valid for Cluster Type: Aurora MySQL DB clusters only
*
*
* Default: 0
*
*
* Constraints:
*
*
* -
*
* If specified, this value must be set to a number from 0 to 259,200 (72 hours).
*
*
*
*
* @return The target backtrack window, in seconds. To disable backtracking, set this value to 0
.
*
* Valid for Cluster Type: Aurora MySQL DB clusters only
*
*
* Default: 0
*
*
* Constraints:
*
*
* -
*
* If specified, this value must be set to a number from 0 to 259,200 (72 hours).
*
*
*/
public Long getBacktrackWindow() {
return this.backtrackWindow;
}
/**
*
* The target backtrack window, in seconds. To disable backtracking, set this value to 0
.
*
*
* Valid for Cluster Type: Aurora MySQL DB clusters only
*
*
* Default: 0
*
*
* Constraints:
*
*
* -
*
* If specified, this value must be set to a number from 0 to 259,200 (72 hours).
*
*
*
*
* @param backtrackWindow
* The target backtrack window, in seconds. To disable backtracking, set this value to 0
.
*
* Valid for Cluster Type: Aurora MySQL DB clusters only
*
*
* Default: 0
*
*
* Constraints:
*
*
* -
*
* If specified, this value must be set to a number from 0 to 259,200 (72 hours).
*
*
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ModifyDBClusterRequest withBacktrackWindow(Long backtrackWindow) {
setBacktrackWindow(backtrackWindow);
return this;
}
/**
*
* The configuration setting for the log types to be enabled for export to CloudWatch Logs for a specific DB
* cluster.
*
*
* Valid for Cluster Type: Aurora DB clusters and Multi-AZ DB clusters
*
*
* The following values are valid for each DB engine:
*
*
* -
*
* Aurora MySQL - audit | error | general | slowquery
*
*
* -
*
* Aurora PostgreSQL - postgresql
*
*
* -
*
* RDS for MySQL - error | general | slowquery
*
*
* -
*
* RDS for PostgreSQL - postgresql | upgrade
*
*
*
*
* For more information about exporting CloudWatch Logs for Amazon RDS, see Publishing Database Logs to Amazon CloudWatch Logs in the Amazon RDS User Guide.
*
*
* For more information about exporting CloudWatch Logs for Amazon Aurora, see Publishing Database Logs to Amazon CloudWatch Logs in the Amazon Aurora User Guide.
*
*
* @param cloudwatchLogsExportConfiguration
* The configuration setting for the log types to be enabled for export to CloudWatch Logs for a specific DB
* cluster.
*
* Valid for Cluster Type: Aurora DB clusters and Multi-AZ DB clusters
*
*
* The following values are valid for each DB engine:
*
*
* -
*
* Aurora MySQL - audit | error | general | slowquery
*
*
* -
*
* Aurora PostgreSQL - postgresql
*
*
* -
*
* RDS for MySQL - error | general | slowquery
*
*
* -
*
* RDS for PostgreSQL - postgresql | upgrade
*
*
*
*
* For more information about exporting CloudWatch Logs for Amazon RDS, see Publishing Database Logs to Amazon CloudWatch Logs in the Amazon RDS User Guide.
*
*
* For more information about exporting CloudWatch Logs for Amazon Aurora, see Publishing Database Logs to Amazon CloudWatch Logs in the Amazon Aurora User Guide.
*/
public void setCloudwatchLogsExportConfiguration(CloudwatchLogsExportConfiguration cloudwatchLogsExportConfiguration) {
this.cloudwatchLogsExportConfiguration = cloudwatchLogsExportConfiguration;
}
/**
*
* The configuration setting for the log types to be enabled for export to CloudWatch Logs for a specific DB
* cluster.
*
*
* Valid for Cluster Type: Aurora DB clusters and Multi-AZ DB clusters
*
*
* The following values are valid for each DB engine:
*
*
* -
*
* Aurora MySQL - audit | error | general | slowquery
*
*
* -
*
* Aurora PostgreSQL - postgresql
*
*
* -
*
* RDS for MySQL - error | general | slowquery
*
*
* -
*
* RDS for PostgreSQL - postgresql | upgrade
*
*
*
*
* For more information about exporting CloudWatch Logs for Amazon RDS, see Publishing Database Logs to Amazon CloudWatch Logs in the Amazon RDS User Guide.
*
*
* For more information about exporting CloudWatch Logs for Amazon Aurora, see Publishing Database Logs to Amazon CloudWatch Logs in the Amazon Aurora User Guide.
*
*
* @return The configuration setting for the log types to be enabled for export to CloudWatch Logs for a specific DB
* cluster.
*
* Valid for Cluster Type: Aurora DB clusters and Multi-AZ DB clusters
*
*
* The following values are valid for each DB engine:
*
*
* -
*
* Aurora MySQL - audit | error | general | slowquery
*
*
* -
*
* Aurora PostgreSQL - postgresql
*
*
* -
*
* RDS for MySQL - error | general | slowquery
*
*
* -
*
* RDS for PostgreSQL - postgresql | upgrade
*
*
*
*
* For more information about exporting CloudWatch Logs for Amazon RDS, see Publishing Database Logs to Amazon CloudWatch Logs in the Amazon RDS User Guide.
*
*
* For more information about exporting CloudWatch Logs for Amazon Aurora, see Publishing Database Logs to Amazon CloudWatch Logs in the Amazon Aurora User Guide.
*/
public CloudwatchLogsExportConfiguration getCloudwatchLogsExportConfiguration() {
return this.cloudwatchLogsExportConfiguration;
}
/**
*
* The configuration setting for the log types to be enabled for export to CloudWatch Logs for a specific DB
* cluster.
*
*
* Valid for Cluster Type: Aurora DB clusters and Multi-AZ DB clusters
*
*
* The following values are valid for each DB engine:
*
*
* -
*
* Aurora MySQL - audit | error | general | slowquery
*
*
* -
*
* Aurora PostgreSQL - postgresql
*
*
* -
*
* RDS for MySQL - error | general | slowquery
*
*
* -
*
* RDS for PostgreSQL - postgresql | upgrade
*
*
*
*
* For more information about exporting CloudWatch Logs for Amazon RDS, see Publishing Database Logs to Amazon CloudWatch Logs in the Amazon RDS User Guide.
*
*
* For more information about exporting CloudWatch Logs for Amazon Aurora, see Publishing Database Logs to Amazon CloudWatch Logs in the Amazon Aurora User Guide.
*
*
* @param cloudwatchLogsExportConfiguration
* The configuration setting for the log types to be enabled for export to CloudWatch Logs for a specific DB
* cluster.
*
* Valid for Cluster Type: Aurora DB clusters and Multi-AZ DB clusters
*
*
* The following values are valid for each DB engine:
*
*
* -
*
* Aurora MySQL - audit | error | general | slowquery
*
*
* -
*
* Aurora PostgreSQL - postgresql
*
*
* -
*
* RDS for MySQL - error | general | slowquery
*
*
* -
*
* RDS for PostgreSQL - postgresql | upgrade
*
*
*
*
* For more information about exporting CloudWatch Logs for Amazon RDS, see Publishing Database Logs to Amazon CloudWatch Logs in the Amazon RDS User Guide.
*
*
* For more information about exporting CloudWatch Logs for Amazon Aurora, see Publishing Database Logs to Amazon CloudWatch Logs in the Amazon Aurora User Guide.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ModifyDBClusterRequest withCloudwatchLogsExportConfiguration(CloudwatchLogsExportConfiguration cloudwatchLogsExportConfiguration) {
setCloudwatchLogsExportConfiguration(cloudwatchLogsExportConfiguration);
return this;
}
/**
*
* The version number of the database engine to which you want to upgrade. Changing this parameter results in an
* outage. The change is applied during the next maintenance window unless ApplyImmediately
is enabled.
*
*
* If the cluster that you're modifying has one or more read replicas, all replicas must be running an engine
* version that's the same or later than the version you specify.
*
*
* To list all of the available engine versions for Aurora MySQL, use the following command:
*
*
* aws rds describe-db-engine-versions --engine aurora-mysql --query "DBEngineVersions[].EngineVersion"
*
*
* To list all of the available engine versions for Aurora PostgreSQL, use the following command:
*
*
* aws rds describe-db-engine-versions --engine aurora-postgresql --query "DBEngineVersions[].EngineVersion"
*
*
* To list all of the available engine versions for RDS for MySQL, use the following command:
*
*
* aws rds describe-db-engine-versions --engine mysql --query "DBEngineVersions[].EngineVersion"
*
*
* To list all of the available engine versions for RDS for PostgreSQL, use the following command:
*
*
* aws rds describe-db-engine-versions --engine postgres --query "DBEngineVersions[].EngineVersion"
*
*
* Valid for Cluster Type: Aurora DB clusters and Multi-AZ DB clusters
*
*
* @param engineVersion
* The version number of the database engine to which you want to upgrade. Changing this parameter results in
* an outage. The change is applied during the next maintenance window unless ApplyImmediately
* is enabled.
*
* If the cluster that you're modifying has one or more read replicas, all replicas must be running an engine
* version that's the same or later than the version you specify.
*
*
* To list all of the available engine versions for Aurora MySQL, use the following command:
*
*
* aws rds describe-db-engine-versions --engine aurora-mysql --query "DBEngineVersions[].EngineVersion"
*
*
* To list all of the available engine versions for Aurora PostgreSQL, use the following command:
*
*
* aws rds describe-db-engine-versions --engine aurora-postgresql --query "DBEngineVersions[].EngineVersion"
*
*
* To list all of the available engine versions for RDS for MySQL, use the following command:
*
*
* aws rds describe-db-engine-versions --engine mysql --query "DBEngineVersions[].EngineVersion"
*
*
* To list all of the available engine versions for RDS for PostgreSQL, use the following command:
*
*
* aws rds describe-db-engine-versions --engine postgres --query "DBEngineVersions[].EngineVersion"
*
*
* Valid for Cluster Type: Aurora DB clusters and Multi-AZ DB clusters
*/
public void setEngineVersion(String engineVersion) {
this.engineVersion = engineVersion;
}
/**
*
* The version number of the database engine to which you want to upgrade. Changing this parameter results in an
* outage. The change is applied during the next maintenance window unless ApplyImmediately
is enabled.
*
*
* If the cluster that you're modifying has one or more read replicas, all replicas must be running an engine
* version that's the same or later than the version you specify.
*
*
* To list all of the available engine versions for Aurora MySQL, use the following command:
*
*
* aws rds describe-db-engine-versions --engine aurora-mysql --query "DBEngineVersions[].EngineVersion"
*
*
* To list all of the available engine versions for Aurora PostgreSQL, use the following command:
*
*
* aws rds describe-db-engine-versions --engine aurora-postgresql --query "DBEngineVersions[].EngineVersion"
*
*
* To list all of the available engine versions for RDS for MySQL, use the following command:
*
*
* aws rds describe-db-engine-versions --engine mysql --query "DBEngineVersions[].EngineVersion"
*
*
* To list all of the available engine versions for RDS for PostgreSQL, use the following command:
*
*
* aws rds describe-db-engine-versions --engine postgres --query "DBEngineVersions[].EngineVersion"
*
*
* Valid for Cluster Type: Aurora DB clusters and Multi-AZ DB clusters
*
*
* @return The version number of the database engine to which you want to upgrade. Changing this parameter results
* in an outage. The change is applied during the next maintenance window unless
* ApplyImmediately
is enabled.
*
* If the cluster that you're modifying has one or more read replicas, all replicas must be running an
* engine version that's the same or later than the version you specify.
*
*
* To list all of the available engine versions for Aurora MySQL, use the following command:
*
*
* aws rds describe-db-engine-versions --engine aurora-mysql --query "DBEngineVersions[].EngineVersion"
*
*
* To list all of the available engine versions for Aurora PostgreSQL, use the following command:
*
*
* aws rds describe-db-engine-versions --engine aurora-postgresql --query "DBEngineVersions[].EngineVersion"
*
*
* To list all of the available engine versions for RDS for MySQL, use the following command:
*
*
* aws rds describe-db-engine-versions --engine mysql --query "DBEngineVersions[].EngineVersion"
*
*
* To list all of the available engine versions for RDS for PostgreSQL, use the following command:
*
*
* aws rds describe-db-engine-versions --engine postgres --query "DBEngineVersions[].EngineVersion"
*
*
* Valid for Cluster Type: Aurora DB clusters and Multi-AZ DB clusters
*/
public String getEngineVersion() {
return this.engineVersion;
}
/**
*
* The version number of the database engine to which you want to upgrade. Changing this parameter results in an
* outage. The change is applied during the next maintenance window unless ApplyImmediately
is enabled.
*
*
* If the cluster that you're modifying has one or more read replicas, all replicas must be running an engine
* version that's the same or later than the version you specify.
*
*
* To list all of the available engine versions for Aurora MySQL, use the following command:
*
*
* aws rds describe-db-engine-versions --engine aurora-mysql --query "DBEngineVersions[].EngineVersion"
*
*
* To list all of the available engine versions for Aurora PostgreSQL, use the following command:
*
*
* aws rds describe-db-engine-versions --engine aurora-postgresql --query "DBEngineVersions[].EngineVersion"
*
*
* To list all of the available engine versions for RDS for MySQL, use the following command:
*
*
* aws rds describe-db-engine-versions --engine mysql --query "DBEngineVersions[].EngineVersion"
*
*
* To list all of the available engine versions for RDS for PostgreSQL, use the following command:
*
*
* aws rds describe-db-engine-versions --engine postgres --query "DBEngineVersions[].EngineVersion"
*
*
* Valid for Cluster Type: Aurora DB clusters and Multi-AZ DB clusters
*
*
* @param engineVersion
* The version number of the database engine to which you want to upgrade. Changing this parameter results in
* an outage. The change is applied during the next maintenance window unless ApplyImmediately
* is enabled.
*
* If the cluster that you're modifying has one or more read replicas, all replicas must be running an engine
* version that's the same or later than the version you specify.
*
*
* To list all of the available engine versions for Aurora MySQL, use the following command:
*
*
* aws rds describe-db-engine-versions --engine aurora-mysql --query "DBEngineVersions[].EngineVersion"
*
*
* To list all of the available engine versions for Aurora PostgreSQL, use the following command:
*
*
* aws rds describe-db-engine-versions --engine aurora-postgresql --query "DBEngineVersions[].EngineVersion"
*
*
* To list all of the available engine versions for RDS for MySQL, use the following command:
*
*
* aws rds describe-db-engine-versions --engine mysql --query "DBEngineVersions[].EngineVersion"
*
*
* To list all of the available engine versions for RDS for PostgreSQL, use the following command:
*
*
* aws rds describe-db-engine-versions --engine postgres --query "DBEngineVersions[].EngineVersion"
*
*
* Valid for Cluster Type: Aurora DB clusters and Multi-AZ DB clusters
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ModifyDBClusterRequest withEngineVersion(String engineVersion) {
setEngineVersion(engineVersion);
return this;
}
/**
*
* Specifies whether major version upgrades are allowed.
*
*
* Valid for Cluster Type: Aurora DB clusters and Multi-AZ DB clusters
*
*
* Constraints:
*
*
* -
*
* You must allow major version upgrades when specifying a value for the EngineVersion
parameter that
* is a different major version than the DB cluster's current version.
*
*
*
*
* @param allowMajorVersionUpgrade
* Specifies whether major version upgrades are allowed.
*
* Valid for Cluster Type: Aurora DB clusters and Multi-AZ DB clusters
*
*
* Constraints:
*
*
* -
*
* You must allow major version upgrades when specifying a value for the EngineVersion
parameter
* that is a different major version than the DB cluster's current version.
*
*
*/
public void setAllowMajorVersionUpgrade(Boolean allowMajorVersionUpgrade) {
this.allowMajorVersionUpgrade = allowMajorVersionUpgrade;
}
/**
*
* Specifies whether major version upgrades are allowed.
*
*
* Valid for Cluster Type: Aurora DB clusters and Multi-AZ DB clusters
*
*
* Constraints:
*
*
* -
*
* You must allow major version upgrades when specifying a value for the EngineVersion
parameter that
* is a different major version than the DB cluster's current version.
*
*
*
*
* @return Specifies whether major version upgrades are allowed.
*
* Valid for Cluster Type: Aurora DB clusters and Multi-AZ DB clusters
*
*
* Constraints:
*
*
* -
*
* You must allow major version upgrades when specifying a value for the EngineVersion
* parameter that is a different major version than the DB cluster's current version.
*
*
*/
public Boolean getAllowMajorVersionUpgrade() {
return this.allowMajorVersionUpgrade;
}
/**
*
* Specifies whether major version upgrades are allowed.
*
*
* Valid for Cluster Type: Aurora DB clusters and Multi-AZ DB clusters
*
*
* Constraints:
*
*
* -
*
* You must allow major version upgrades when specifying a value for the EngineVersion
parameter that
* is a different major version than the DB cluster's current version.
*
*
*
*
* @param allowMajorVersionUpgrade
* Specifies whether major version upgrades are allowed.
*
* Valid for Cluster Type: Aurora DB clusters and Multi-AZ DB clusters
*
*
* Constraints:
*
*
* -
*
* You must allow major version upgrades when specifying a value for the EngineVersion
parameter
* that is a different major version than the DB cluster's current version.
*
*
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ModifyDBClusterRequest withAllowMajorVersionUpgrade(Boolean allowMajorVersionUpgrade) {
setAllowMajorVersionUpgrade(allowMajorVersionUpgrade);
return this;
}
/**
*
* Specifies whether major version upgrades are allowed.
*
*
* Valid for Cluster Type: Aurora DB clusters and Multi-AZ DB clusters
*
*
* Constraints:
*
*
* -
*
* You must allow major version upgrades when specifying a value for the EngineVersion
parameter that
* is a different major version than the DB cluster's current version.
*
*
*
*
* @return Specifies whether major version upgrades are allowed.
*
* Valid for Cluster Type: Aurora DB clusters and Multi-AZ DB clusters
*
*
* Constraints:
*
*
* -
*
* You must allow major version upgrades when specifying a value for the EngineVersion
* parameter that is a different major version than the DB cluster's current version.
*
*
*/
public Boolean isAllowMajorVersionUpgrade() {
return this.allowMajorVersionUpgrade;
}
/**
*
* The name of the DB parameter group to apply to all instances of the DB cluster.
*
*
*
* When you apply a parameter group using the DBInstanceParameterGroupName
parameter, the DB cluster
* isn't rebooted automatically. Also, parameter changes are applied immediately rather than during the next
* maintenance window.
*
*
*
* Valid for Cluster Type: Aurora DB clusters only
*
*
* Default: The existing name setting
*
*
* Constraints:
*
*
* -
*
* The DB parameter group must be in the same DB parameter group family as this DB cluster.
*
*
* -
*
* The DBInstanceParameterGroupName
parameter is valid in combination with the
* AllowMajorVersionUpgrade
parameter for a major version upgrade only.
*
*
*
*
* @param dBInstanceParameterGroupName
* The name of the DB parameter group to apply to all instances of the DB cluster.
*
* When you apply a parameter group using the DBInstanceParameterGroupName
parameter, the DB
* cluster isn't rebooted automatically. Also, parameter changes are applied immediately rather than during
* the next maintenance window.
*
*
*
* Valid for Cluster Type: Aurora DB clusters only
*
*
* Default: The existing name setting
*
*
* Constraints:
*
*
* -
*
* The DB parameter group must be in the same DB parameter group family as this DB cluster.
*
*
* -
*
* The DBInstanceParameterGroupName
parameter is valid in combination with the
* AllowMajorVersionUpgrade
parameter for a major version upgrade only.
*
*
*/
public void setDBInstanceParameterGroupName(String dBInstanceParameterGroupName) {
this.dBInstanceParameterGroupName = dBInstanceParameterGroupName;
}
/**
*
* The name of the DB parameter group to apply to all instances of the DB cluster.
*
*
*
* When you apply a parameter group using the DBInstanceParameterGroupName
parameter, the DB cluster
* isn't rebooted automatically. Also, parameter changes are applied immediately rather than during the next
* maintenance window.
*
*
*
* Valid for Cluster Type: Aurora DB clusters only
*
*
* Default: The existing name setting
*
*
* Constraints:
*
*
* -
*
* The DB parameter group must be in the same DB parameter group family as this DB cluster.
*
*
* -
*
* The DBInstanceParameterGroupName
parameter is valid in combination with the
* AllowMajorVersionUpgrade
parameter for a major version upgrade only.
*
*
*
*
* @return The name of the DB parameter group to apply to all instances of the DB cluster.
*
* When you apply a parameter group using the DBInstanceParameterGroupName
parameter, the DB
* cluster isn't rebooted automatically. Also, parameter changes are applied immediately rather than during
* the next maintenance window.
*
*
*
* Valid for Cluster Type: Aurora DB clusters only
*
*
* Default: The existing name setting
*
*
* Constraints:
*
*
* -
*
* The DB parameter group must be in the same DB parameter group family as this DB cluster.
*
*
* -
*
* The DBInstanceParameterGroupName
parameter is valid in combination with the
* AllowMajorVersionUpgrade
parameter for a major version upgrade only.
*
*
*/
public String getDBInstanceParameterGroupName() {
return this.dBInstanceParameterGroupName;
}
/**
*
* The name of the DB parameter group to apply to all instances of the DB cluster.
*
*
*
* When you apply a parameter group using the DBInstanceParameterGroupName
parameter, the DB cluster
* isn't rebooted automatically. Also, parameter changes are applied immediately rather than during the next
* maintenance window.
*
*
*
* Valid for Cluster Type: Aurora DB clusters only
*
*
* Default: The existing name setting
*
*
* Constraints:
*
*
* -
*
* The DB parameter group must be in the same DB parameter group family as this DB cluster.
*
*
* -
*
* The DBInstanceParameterGroupName
parameter is valid in combination with the
* AllowMajorVersionUpgrade
parameter for a major version upgrade only.
*
*
*
*
* @param dBInstanceParameterGroupName
* The name of the DB parameter group to apply to all instances of the DB cluster.
*
* When you apply a parameter group using the DBInstanceParameterGroupName
parameter, the DB
* cluster isn't rebooted automatically. Also, parameter changes are applied immediately rather than during
* the next maintenance window.
*
*
*
* Valid for Cluster Type: Aurora DB clusters only
*
*
* Default: The existing name setting
*
*
* Constraints:
*
*
* -
*
* The DB parameter group must be in the same DB parameter group family as this DB cluster.
*
*
* -
*
* The DBInstanceParameterGroupName
parameter is valid in combination with the
* AllowMajorVersionUpgrade
parameter for a major version upgrade only.
*
*
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ModifyDBClusterRequest withDBInstanceParameterGroupName(String dBInstanceParameterGroupName) {
setDBInstanceParameterGroupName(dBInstanceParameterGroupName);
return this;
}
/**
*
* The Active Directory directory ID to move the DB cluster to. Specify none
to remove the cluster from
* its current domain. The domain must be created prior to this operation.
*
*
* For more information, see Kerberos
* Authentication in the Amazon Aurora User Guide.
*
*
* Valid for Cluster Type: Aurora DB clusters only
*
*
* @param domain
* The Active Directory directory ID to move the DB cluster to. Specify none
to remove the
* cluster from its current domain. The domain must be created prior to this operation.
*
* For more information, see Kerberos
* Authentication in the Amazon Aurora User Guide.
*
*
* Valid for Cluster Type: Aurora DB clusters only
*/
public void setDomain(String domain) {
this.domain = domain;
}
/**
*
* The Active Directory directory ID to move the DB cluster to. Specify none
to remove the cluster from
* its current domain. The domain must be created prior to this operation.
*
*
* For more information, see Kerberos
* Authentication in the Amazon Aurora User Guide.
*
*
* Valid for Cluster Type: Aurora DB clusters only
*
*
* @return The Active Directory directory ID to move the DB cluster to. Specify none
to remove the
* cluster from its current domain. The domain must be created prior to this operation.
*
* For more information, see Kerberos
* Authentication in the Amazon Aurora User Guide.
*
*
* Valid for Cluster Type: Aurora DB clusters only
*/
public String getDomain() {
return this.domain;
}
/**
*
* The Active Directory directory ID to move the DB cluster to. Specify none
to remove the cluster from
* its current domain. The domain must be created prior to this operation.
*
*
* For more information, see Kerberos
* Authentication in the Amazon Aurora User Guide.
*
*
* Valid for Cluster Type: Aurora DB clusters only
*
*
* @param domain
* The Active Directory directory ID to move the DB cluster to. Specify none
to remove the
* cluster from its current domain. The domain must be created prior to this operation.
*
* For more information, see Kerberos
* Authentication in the Amazon Aurora User Guide.
*
*
* Valid for Cluster Type: Aurora DB clusters only
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ModifyDBClusterRequest withDomain(String domain) {
setDomain(domain);
return this;
}
/**
*
* The name of the IAM role to use when making API calls to the Directory Service.
*
*
* Valid for Cluster Type: Aurora DB clusters only
*
*
* @param domainIAMRoleName
* The name of the IAM role to use when making API calls to the Directory Service.
*
* Valid for Cluster Type: Aurora DB clusters only
*/
public void setDomainIAMRoleName(String domainIAMRoleName) {
this.domainIAMRoleName = domainIAMRoleName;
}
/**
*
* The name of the IAM role to use when making API calls to the Directory Service.
*
*
* Valid for Cluster Type: Aurora DB clusters only
*
*
* @return The name of the IAM role to use when making API calls to the Directory Service.
*
* Valid for Cluster Type: Aurora DB clusters only
*/
public String getDomainIAMRoleName() {
return this.domainIAMRoleName;
}
/**
*
* The name of the IAM role to use when making API calls to the Directory Service.
*
*
* Valid for Cluster Type: Aurora DB clusters only
*
*
* @param domainIAMRoleName
* The name of the IAM role to use when making API calls to the Directory Service.
*
* Valid for Cluster Type: Aurora DB clusters only
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ModifyDBClusterRequest withDomainIAMRoleName(String domainIAMRoleName) {
setDomainIAMRoleName(domainIAMRoleName);
return this;
}
/**
*
* The scaling properties of the DB cluster. You can only modify scaling properties for DB clusters in
* serverless
DB engine mode.
*
*
* Valid for Cluster Type: Aurora DB clusters only
*
*
* @param scalingConfiguration
* The scaling properties of the DB cluster. You can only modify scaling properties for DB clusters in
* serverless
DB engine mode.
*
* Valid for Cluster Type: Aurora DB clusters only
*/
public void setScalingConfiguration(ScalingConfiguration scalingConfiguration) {
this.scalingConfiguration = scalingConfiguration;
}
/**
*
* The scaling properties of the DB cluster. You can only modify scaling properties for DB clusters in
* serverless
DB engine mode.
*
*
* Valid for Cluster Type: Aurora DB clusters only
*
*
* @return The scaling properties of the DB cluster. You can only modify scaling properties for DB clusters in
* serverless
DB engine mode.
*
* Valid for Cluster Type: Aurora DB clusters only
*/
public ScalingConfiguration getScalingConfiguration() {
return this.scalingConfiguration;
}
/**
*
* The scaling properties of the DB cluster. You can only modify scaling properties for DB clusters in
* serverless
DB engine mode.
*
*
* Valid for Cluster Type: Aurora DB clusters only
*
*
* @param scalingConfiguration
* The scaling properties of the DB cluster. You can only modify scaling properties for DB clusters in
* serverless
DB engine mode.
*
* Valid for Cluster Type: Aurora DB clusters only
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ModifyDBClusterRequest withScalingConfiguration(ScalingConfiguration scalingConfiguration) {
setScalingConfiguration(scalingConfiguration);
return this;
}
/**
*
* Specifies whether the DB cluster has deletion protection enabled. The database can't be deleted when deletion
* protection is enabled. By default, deletion protection isn't enabled.
*
*
* Valid for Cluster Type: Aurora DB clusters and Multi-AZ DB clusters
*
*
* @param deletionProtection
* Specifies whether the DB cluster has deletion protection enabled. The database can't be deleted when
* deletion protection is enabled. By default, deletion protection isn't enabled.
*
* Valid for Cluster Type: Aurora DB clusters and Multi-AZ DB clusters
*/
public void setDeletionProtection(Boolean deletionProtection) {
this.deletionProtection = deletionProtection;
}
/**
*
* Specifies whether the DB cluster has deletion protection enabled. The database can't be deleted when deletion
* protection is enabled. By default, deletion protection isn't enabled.
*
*
* Valid for Cluster Type: Aurora DB clusters and Multi-AZ DB clusters
*
*
* @return Specifies whether the DB cluster has deletion protection enabled. The database can't be deleted when
* deletion protection is enabled. By default, deletion protection isn't enabled.
*
* Valid for Cluster Type: Aurora DB clusters and Multi-AZ DB clusters
*/
public Boolean getDeletionProtection() {
return this.deletionProtection;
}
/**
*
* Specifies whether the DB cluster has deletion protection enabled. The database can't be deleted when deletion
* protection is enabled. By default, deletion protection isn't enabled.
*
*
* Valid for Cluster Type: Aurora DB clusters and Multi-AZ DB clusters
*
*
* @param deletionProtection
* Specifies whether the DB cluster has deletion protection enabled. The database can't be deleted when
* deletion protection is enabled. By default, deletion protection isn't enabled.
*
* Valid for Cluster Type: Aurora DB clusters and Multi-AZ DB clusters
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ModifyDBClusterRequest withDeletionProtection(Boolean deletionProtection) {
setDeletionProtection(deletionProtection);
return this;
}
/**
*
* Specifies whether the DB cluster has deletion protection enabled. The database can't be deleted when deletion
* protection is enabled. By default, deletion protection isn't enabled.
*
*
* Valid for Cluster Type: Aurora DB clusters and Multi-AZ DB clusters
*
*
* @return Specifies whether the DB cluster has deletion protection enabled. The database can't be deleted when
* deletion protection is enabled. By default, deletion protection isn't enabled.
*
* Valid for Cluster Type: Aurora DB clusters and Multi-AZ DB clusters
*/
public Boolean isDeletionProtection() {
return this.deletionProtection;
}
/**
*
* Specifies whether to enable the HTTP endpoint for an Aurora Serverless v1 DB cluster. By default, the HTTP
* endpoint isn't enabled.
*
*
* When enabled, the HTTP endpoint provides a connectionless web service API (RDS Data API) for running SQL queries
* on the Aurora Serverless v1 DB cluster. You can also query your database from inside the RDS console with the RDS
* query editor.
*
*
* For more information, see Using RDS Data API in the
* Amazon Aurora User Guide.
*
*
*
* This parameter applies only to Aurora Serverless v1 DB clusters. To enable or disable the HTTP endpoint for an
* Aurora PostgreSQL Serverless v2 or provisioned DB cluster, use the EnableHttpEndpoint
and
* DisableHttpEndpoint
operations.
*
*
*
* Valid for Cluster Type: Aurora DB clusters only
*
*
* @param enableHttpEndpoint
* Specifies whether to enable the HTTP endpoint for an Aurora Serverless v1 DB cluster. By default, the HTTP
* endpoint isn't enabled.
*
* When enabled, the HTTP endpoint provides a connectionless web service API (RDS Data API) for running SQL
* queries on the Aurora Serverless v1 DB cluster. You can also query your database from inside the RDS
* console with the RDS query editor.
*
*
* For more information, see Using RDS Data API
* in the Amazon Aurora User Guide.
*
*
*
* This parameter applies only to Aurora Serverless v1 DB clusters. To enable or disable the HTTP endpoint
* for an Aurora PostgreSQL Serverless v2 or provisioned DB cluster, use the EnableHttpEndpoint
* and DisableHttpEndpoint
operations.
*
*
*
* Valid for Cluster Type: Aurora DB clusters only
*/
public void setEnableHttpEndpoint(Boolean enableHttpEndpoint) {
this.enableHttpEndpoint = enableHttpEndpoint;
}
/**
*
* Specifies whether to enable the HTTP endpoint for an Aurora Serverless v1 DB cluster. By default, the HTTP
* endpoint isn't enabled.
*
*
* When enabled, the HTTP endpoint provides a connectionless web service API (RDS Data API) for running SQL queries
* on the Aurora Serverless v1 DB cluster. You can also query your database from inside the RDS console with the RDS
* query editor.
*
*
* For more information, see Using RDS Data API in the
* Amazon Aurora User Guide.
*
*
*
* This parameter applies only to Aurora Serverless v1 DB clusters. To enable or disable the HTTP endpoint for an
* Aurora PostgreSQL Serverless v2 or provisioned DB cluster, use the EnableHttpEndpoint
and
* DisableHttpEndpoint
operations.
*
*
*
* Valid for Cluster Type: Aurora DB clusters only
*
*
* @return Specifies whether to enable the HTTP endpoint for an Aurora Serverless v1 DB cluster. By default, the
* HTTP endpoint isn't enabled.
*
* When enabled, the HTTP endpoint provides a connectionless web service API (RDS Data API) for running SQL
* queries on the Aurora Serverless v1 DB cluster. You can also query your database from inside the RDS
* console with the RDS query editor.
*
*
* For more information, see Using RDS Data API
* in the Amazon Aurora User Guide.
*
*
*
* This parameter applies only to Aurora Serverless v1 DB clusters. To enable or disable the HTTP endpoint
* for an Aurora PostgreSQL Serverless v2 or provisioned DB cluster, use the EnableHttpEndpoint
* and DisableHttpEndpoint
operations.
*
*
*
* Valid for Cluster Type: Aurora DB clusters only
*/
public Boolean getEnableHttpEndpoint() {
return this.enableHttpEndpoint;
}
/**
*
* Specifies whether to enable the HTTP endpoint for an Aurora Serverless v1 DB cluster. By default, the HTTP
* endpoint isn't enabled.
*
*
* When enabled, the HTTP endpoint provides a connectionless web service API (RDS Data API) for running SQL queries
* on the Aurora Serverless v1 DB cluster. You can also query your database from inside the RDS console with the RDS
* query editor.
*
*
* For more information, see Using RDS Data API in the
* Amazon Aurora User Guide.
*
*
*
* This parameter applies only to Aurora Serverless v1 DB clusters. To enable or disable the HTTP endpoint for an
* Aurora PostgreSQL Serverless v2 or provisioned DB cluster, use the EnableHttpEndpoint
and
* DisableHttpEndpoint
operations.
*
*
*
* Valid for Cluster Type: Aurora DB clusters only
*
*
* @param enableHttpEndpoint
* Specifies whether to enable the HTTP endpoint for an Aurora Serverless v1 DB cluster. By default, the HTTP
* endpoint isn't enabled.
*
* When enabled, the HTTP endpoint provides a connectionless web service API (RDS Data API) for running SQL
* queries on the Aurora Serverless v1 DB cluster. You can also query your database from inside the RDS
* console with the RDS query editor.
*
*
* For more information, see Using RDS Data API
* in the Amazon Aurora User Guide.
*
*
*
* This parameter applies only to Aurora Serverless v1 DB clusters. To enable or disable the HTTP endpoint
* for an Aurora PostgreSQL Serverless v2 or provisioned DB cluster, use the EnableHttpEndpoint
* and DisableHttpEndpoint
operations.
*
*
*
* Valid for Cluster Type: Aurora DB clusters only
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ModifyDBClusterRequest withEnableHttpEndpoint(Boolean enableHttpEndpoint) {
setEnableHttpEndpoint(enableHttpEndpoint);
return this;
}
/**
*
* Specifies whether to enable the HTTP endpoint for an Aurora Serverless v1 DB cluster. By default, the HTTP
* endpoint isn't enabled.
*
*
* When enabled, the HTTP endpoint provides a connectionless web service API (RDS Data API) for running SQL queries
* on the Aurora Serverless v1 DB cluster. You can also query your database from inside the RDS console with the RDS
* query editor.
*
*
* For more information, see Using RDS Data API in the
* Amazon Aurora User Guide.
*
*
*
* This parameter applies only to Aurora Serverless v1 DB clusters. To enable or disable the HTTP endpoint for an
* Aurora PostgreSQL Serverless v2 or provisioned DB cluster, use the EnableHttpEndpoint
and
* DisableHttpEndpoint
operations.
*
*
*
* Valid for Cluster Type: Aurora DB clusters only
*
*
* @return Specifies whether to enable the HTTP endpoint for an Aurora Serverless v1 DB cluster. By default, the
* HTTP endpoint isn't enabled.
*
* When enabled, the HTTP endpoint provides a connectionless web service API (RDS Data API) for running SQL
* queries on the Aurora Serverless v1 DB cluster. You can also query your database from inside the RDS
* console with the RDS query editor.
*
*
* For more information, see Using RDS Data API
* in the Amazon Aurora User Guide.
*
*
*
* This parameter applies only to Aurora Serverless v1 DB clusters. To enable or disable the HTTP endpoint
* for an Aurora PostgreSQL Serverless v2 or provisioned DB cluster, use the EnableHttpEndpoint
* and DisableHttpEndpoint
operations.
*
*
*
* Valid for Cluster Type: Aurora DB clusters only
*/
public Boolean isEnableHttpEndpoint() {
return this.enableHttpEndpoint;
}
/**
*
* Specifies whether to copy all tags from the DB cluster to snapshots of the DB cluster. The default is not to copy
* them.
*
*
* Valid for Cluster Type: Aurora DB clusters and Multi-AZ DB clusters
*
*
* @param copyTagsToSnapshot
* Specifies whether to copy all tags from the DB cluster to snapshots of the DB cluster. The default is not
* to copy them.
*
* Valid for Cluster Type: Aurora DB clusters and Multi-AZ DB clusters
*/
public void setCopyTagsToSnapshot(Boolean copyTagsToSnapshot) {
this.copyTagsToSnapshot = copyTagsToSnapshot;
}
/**
*
* Specifies whether to copy all tags from the DB cluster to snapshots of the DB cluster. The default is not to copy
* them.
*
*
* Valid for Cluster Type: Aurora DB clusters and Multi-AZ DB clusters
*
*
* @return Specifies whether to copy all tags from the DB cluster to snapshots of the DB cluster. The default is not
* to copy them.
*
* Valid for Cluster Type: Aurora DB clusters and Multi-AZ DB clusters
*/
public Boolean getCopyTagsToSnapshot() {
return this.copyTagsToSnapshot;
}
/**
*
* Specifies whether to copy all tags from the DB cluster to snapshots of the DB cluster. The default is not to copy
* them.
*
*
* Valid for Cluster Type: Aurora DB clusters and Multi-AZ DB clusters
*
*
* @param copyTagsToSnapshot
* Specifies whether to copy all tags from the DB cluster to snapshots of the DB cluster. The default is not
* to copy them.
*
* Valid for Cluster Type: Aurora DB clusters and Multi-AZ DB clusters
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ModifyDBClusterRequest withCopyTagsToSnapshot(Boolean copyTagsToSnapshot) {
setCopyTagsToSnapshot(copyTagsToSnapshot);
return this;
}
/**
*
* Specifies whether to copy all tags from the DB cluster to snapshots of the DB cluster. The default is not to copy
* them.
*
*
* Valid for Cluster Type: Aurora DB clusters and Multi-AZ DB clusters
*
*
* @return Specifies whether to copy all tags from the DB cluster to snapshots of the DB cluster. The default is not
* to copy them.
*
* Valid for Cluster Type: Aurora DB clusters and Multi-AZ DB clusters
*/
public Boolean isCopyTagsToSnapshot() {
return this.copyTagsToSnapshot;
}
/**
*
* Specifies whether to enable this DB cluster to forward write operations to the primary cluster of a global
* cluster (Aurora global database). By default, write operations are not allowed on Aurora DB clusters that are
* secondary clusters in an Aurora global database.
*
*
* You can set this value only on Aurora DB clusters that are members of an Aurora global database. With this
* parameter enabled, a secondary cluster can forward writes to the current primary cluster, and the resulting
* changes are replicated back to this cluster. For the primary DB cluster of an Aurora global database, this value
* is used immediately if the primary is demoted by a global cluster API operation, but it does nothing until then.
*
*
* Valid for Cluster Type: Aurora DB clusters only
*
*
* @param enableGlobalWriteForwarding
* Specifies whether to enable this DB cluster to forward write operations to the primary cluster of a global
* cluster (Aurora global database). By default, write operations are not allowed on Aurora DB clusters that
* are secondary clusters in an Aurora global database.
*
* You can set this value only on Aurora DB clusters that are members of an Aurora global database. With this
* parameter enabled, a secondary cluster can forward writes to the current primary cluster, and the
* resulting changes are replicated back to this cluster. For the primary DB cluster of an Aurora global
* database, this value is used immediately if the primary is demoted by a global cluster API operation, but
* it does nothing until then.
*
*
* Valid for Cluster Type: Aurora DB clusters only
*/
public void setEnableGlobalWriteForwarding(Boolean enableGlobalWriteForwarding) {
this.enableGlobalWriteForwarding = enableGlobalWriteForwarding;
}
/**
*
* Specifies whether to enable this DB cluster to forward write operations to the primary cluster of a global
* cluster (Aurora global database). By default, write operations are not allowed on Aurora DB clusters that are
* secondary clusters in an Aurora global database.
*
*
* You can set this value only on Aurora DB clusters that are members of an Aurora global database. With this
* parameter enabled, a secondary cluster can forward writes to the current primary cluster, and the resulting
* changes are replicated back to this cluster. For the primary DB cluster of an Aurora global database, this value
* is used immediately if the primary is demoted by a global cluster API operation, but it does nothing until then.
*
*
* Valid for Cluster Type: Aurora DB clusters only
*
*
* @return Specifies whether to enable this DB cluster to forward write operations to the primary cluster of a
* global cluster (Aurora global database). By default, write operations are not allowed on Aurora DB
* clusters that are secondary clusters in an Aurora global database.
*
* You can set this value only on Aurora DB clusters that are members of an Aurora global database. With
* this parameter enabled, a secondary cluster can forward writes to the current primary cluster, and the
* resulting changes are replicated back to this cluster. For the primary DB cluster of an Aurora global
* database, this value is used immediately if the primary is demoted by a global cluster API operation, but
* it does nothing until then.
*
*
* Valid for Cluster Type: Aurora DB clusters only
*/
public Boolean getEnableGlobalWriteForwarding() {
return this.enableGlobalWriteForwarding;
}
/**
*
* Specifies whether to enable this DB cluster to forward write operations to the primary cluster of a global
* cluster (Aurora global database). By default, write operations are not allowed on Aurora DB clusters that are
* secondary clusters in an Aurora global database.
*
*
* You can set this value only on Aurora DB clusters that are members of an Aurora global database. With this
* parameter enabled, a secondary cluster can forward writes to the current primary cluster, and the resulting
* changes are replicated back to this cluster. For the primary DB cluster of an Aurora global database, this value
* is used immediately if the primary is demoted by a global cluster API operation, but it does nothing until then.
*
*
* Valid for Cluster Type: Aurora DB clusters only
*
*
* @param enableGlobalWriteForwarding
* Specifies whether to enable this DB cluster to forward write operations to the primary cluster of a global
* cluster (Aurora global database). By default, write operations are not allowed on Aurora DB clusters that
* are secondary clusters in an Aurora global database.
*
* You can set this value only on Aurora DB clusters that are members of an Aurora global database. With this
* parameter enabled, a secondary cluster can forward writes to the current primary cluster, and the
* resulting changes are replicated back to this cluster. For the primary DB cluster of an Aurora global
* database, this value is used immediately if the primary is demoted by a global cluster API operation, but
* it does nothing until then.
*
*
* Valid for Cluster Type: Aurora DB clusters only
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ModifyDBClusterRequest withEnableGlobalWriteForwarding(Boolean enableGlobalWriteForwarding) {
setEnableGlobalWriteForwarding(enableGlobalWriteForwarding);
return this;
}
/**
*
* Specifies whether to enable this DB cluster to forward write operations to the primary cluster of a global
* cluster (Aurora global database). By default, write operations are not allowed on Aurora DB clusters that are
* secondary clusters in an Aurora global database.
*
*
* You can set this value only on Aurora DB clusters that are members of an Aurora global database. With this
* parameter enabled, a secondary cluster can forward writes to the current primary cluster, and the resulting
* changes are replicated back to this cluster. For the primary DB cluster of an Aurora global database, this value
* is used immediately if the primary is demoted by a global cluster API operation, but it does nothing until then.
*
*
* Valid for Cluster Type: Aurora DB clusters only
*
*
* @return Specifies whether to enable this DB cluster to forward write operations to the primary cluster of a
* global cluster (Aurora global database). By default, write operations are not allowed on Aurora DB
* clusters that are secondary clusters in an Aurora global database.
*
* You can set this value only on Aurora DB clusters that are members of an Aurora global database. With
* this parameter enabled, a secondary cluster can forward writes to the current primary cluster, and the
* resulting changes are replicated back to this cluster. For the primary DB cluster of an Aurora global
* database, this value is used immediately if the primary is demoted by a global cluster API operation, but
* it does nothing until then.
*
*
* Valid for Cluster Type: Aurora DB clusters only
*/
public Boolean isEnableGlobalWriteForwarding() {
return this.enableGlobalWriteForwarding;
}
/**
*
* The compute and memory capacity of each DB instance in the Multi-AZ DB cluster, for example
* db.m6gd.xlarge
. Not all DB instance classes are available in all Amazon Web Services Regions, or for
* all database engines.
*
*
* For the full list of DB instance classes and availability for your engine, see DB Instance
* Class in the Amazon RDS User Guide.
*
*
* Valid for Cluster Type: Multi-AZ DB clusters only
*
*
* @param dBClusterInstanceClass
* The compute and memory capacity of each DB instance in the Multi-AZ DB cluster, for example
* db.m6gd.xlarge
. Not all DB instance classes are available in all Amazon Web Services Regions,
* or for all database engines.
*
* For the full list of DB instance classes and availability for your engine, see DB Instance
* Class in the Amazon RDS User Guide.
*
*
* Valid for Cluster Type: Multi-AZ DB clusters only
*/
public void setDBClusterInstanceClass(String dBClusterInstanceClass) {
this.dBClusterInstanceClass = dBClusterInstanceClass;
}
/**
*
* The compute and memory capacity of each DB instance in the Multi-AZ DB cluster, for example
* db.m6gd.xlarge
. Not all DB instance classes are available in all Amazon Web Services Regions, or for
* all database engines.
*
*
* For the full list of DB instance classes and availability for your engine, see DB Instance
* Class in the Amazon RDS User Guide.
*
*
* Valid for Cluster Type: Multi-AZ DB clusters only
*
*
* @return The compute and memory capacity of each DB instance in the Multi-AZ DB cluster, for example
* db.m6gd.xlarge
. Not all DB instance classes are available in all Amazon Web Services
* Regions, or for all database engines.
*
* For the full list of DB instance classes and availability for your engine, see DB Instance
* Class in the Amazon RDS User Guide.
*
*
* Valid for Cluster Type: Multi-AZ DB clusters only
*/
public String getDBClusterInstanceClass() {
return this.dBClusterInstanceClass;
}
/**
*
* The compute and memory capacity of each DB instance in the Multi-AZ DB cluster, for example
* db.m6gd.xlarge
. Not all DB instance classes are available in all Amazon Web Services Regions, or for
* all database engines.
*
*
* For the full list of DB instance classes and availability for your engine, see DB Instance
* Class in the Amazon RDS User Guide.
*
*
* Valid for Cluster Type: Multi-AZ DB clusters only
*
*
* @param dBClusterInstanceClass
* The compute and memory capacity of each DB instance in the Multi-AZ DB cluster, for example
* db.m6gd.xlarge
. Not all DB instance classes are available in all Amazon Web Services Regions,
* or for all database engines.
*
* For the full list of DB instance classes and availability for your engine, see DB Instance
* Class in the Amazon RDS User Guide.
*
*
* Valid for Cluster Type: Multi-AZ DB clusters only
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ModifyDBClusterRequest withDBClusterInstanceClass(String dBClusterInstanceClass) {
setDBClusterInstanceClass(dBClusterInstanceClass);
return this;
}
/**
*
* The amount of storage in gibibytes (GiB) to allocate to each DB instance in the Multi-AZ DB cluster.
*
*
* Valid for Cluster Type: Multi-AZ DB clusters only
*
*
* @param allocatedStorage
* The amount of storage in gibibytes (GiB) to allocate to each DB instance in the Multi-AZ DB cluster.
*
* Valid for Cluster Type: Multi-AZ DB clusters only
*/
public void setAllocatedStorage(Integer allocatedStorage) {
this.allocatedStorage = allocatedStorage;
}
/**
*
* The amount of storage in gibibytes (GiB) to allocate to each DB instance in the Multi-AZ DB cluster.
*
*
* Valid for Cluster Type: Multi-AZ DB clusters only
*
*
* @return The amount of storage in gibibytes (GiB) to allocate to each DB instance in the Multi-AZ DB cluster.
*
* Valid for Cluster Type: Multi-AZ DB clusters only
*/
public Integer getAllocatedStorage() {
return this.allocatedStorage;
}
/**
*
* The amount of storage in gibibytes (GiB) to allocate to each DB instance in the Multi-AZ DB cluster.
*
*
* Valid for Cluster Type: Multi-AZ DB clusters only
*
*
* @param allocatedStorage
* The amount of storage in gibibytes (GiB) to allocate to each DB instance in the Multi-AZ DB cluster.
*
* Valid for Cluster Type: Multi-AZ DB clusters only
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ModifyDBClusterRequest withAllocatedStorage(Integer allocatedStorage) {
setAllocatedStorage(allocatedStorage);
return this;
}
/**
*
* The storage type to associate with the DB cluster.
*
*
* For information on storage types for Aurora DB clusters, see Storage configurations for Amazon Aurora DB clusters. For information on storage types for Multi-AZ DB
* clusters, see Settings for creating Multi-AZ DB clusters.
*
*
* When specified for a Multi-AZ DB cluster, a value for the Iops
parameter is required.
*
*
* Valid for Cluster Type: Aurora DB clusters and Multi-AZ DB clusters
*
*
* Valid Values:
*
*
* -
*
* Aurora DB clusters - aurora | aurora-iopt1
*
*
* -
*
* Multi-AZ DB clusters - io1 | io2 | gp3
*
*
*
*
* Default:
*
*
* -
*
* Aurora DB clusters - aurora
*
*
* -
*
* Multi-AZ DB clusters - io1
*
*
*
*
* @param storageType
* The storage type to associate with the DB cluster.
*
* For information on storage types for Aurora DB clusters, see Storage configurations for Amazon Aurora DB clusters. For information on storage types for Multi-AZ
* DB clusters, see Settings for creating Multi-AZ DB clusters.
*
*
* When specified for a Multi-AZ DB cluster, a value for the Iops
parameter is required.
*
*
* Valid for Cluster Type: Aurora DB clusters and Multi-AZ DB clusters
*
*
* Valid Values:
*
*
* -
*
* Aurora DB clusters - aurora | aurora-iopt1
*
*
* -
*
* Multi-AZ DB clusters - io1 | io2 | gp3
*
*
*
*
* Default:
*
*
* -
*
* Aurora DB clusters - aurora
*
*
* -
*
* Multi-AZ DB clusters - io1
*
*
*/
public void setStorageType(String storageType) {
this.storageType = storageType;
}
/**
*
* The storage type to associate with the DB cluster.
*
*
* For information on storage types for Aurora DB clusters, see Storage configurations for Amazon Aurora DB clusters. For information on storage types for Multi-AZ DB
* clusters, see Settings for creating Multi-AZ DB clusters.
*
*
* When specified for a Multi-AZ DB cluster, a value for the Iops
parameter is required.
*
*
* Valid for Cluster Type: Aurora DB clusters and Multi-AZ DB clusters
*
*
* Valid Values:
*
*
* -
*
* Aurora DB clusters - aurora | aurora-iopt1
*
*
* -
*
* Multi-AZ DB clusters - io1 | io2 | gp3
*
*
*
*
* Default:
*
*
* -
*
* Aurora DB clusters - aurora
*
*
* -
*
* Multi-AZ DB clusters - io1
*
*
*
*
* @return The storage type to associate with the DB cluster.
*
* For information on storage types for Aurora DB clusters, see Storage configurations for Amazon Aurora DB clusters. For information on storage types for Multi-AZ
* DB clusters, see Settings for creating Multi-AZ DB clusters.
*
*
* When specified for a Multi-AZ DB cluster, a value for the Iops
parameter is required.
*
*
* Valid for Cluster Type: Aurora DB clusters and Multi-AZ DB clusters
*
*
* Valid Values:
*
*
* -
*
* Aurora DB clusters - aurora | aurora-iopt1
*
*
* -
*
* Multi-AZ DB clusters - io1 | io2 | gp3
*
*
*
*
* Default:
*
*
* -
*
* Aurora DB clusters - aurora
*
*
* -
*
* Multi-AZ DB clusters - io1
*
*
*/
public String getStorageType() {
return this.storageType;
}
/**
*
* The storage type to associate with the DB cluster.
*
*
* For information on storage types for Aurora DB clusters, see Storage configurations for Amazon Aurora DB clusters. For information on storage types for Multi-AZ DB
* clusters, see Settings for creating Multi-AZ DB clusters.
*
*
* When specified for a Multi-AZ DB cluster, a value for the Iops
parameter is required.
*
*
* Valid for Cluster Type: Aurora DB clusters and Multi-AZ DB clusters
*
*
* Valid Values:
*
*
* -
*
* Aurora DB clusters - aurora | aurora-iopt1
*
*
* -
*
* Multi-AZ DB clusters - io1 | io2 | gp3
*
*
*
*
* Default:
*
*
* -
*
* Aurora DB clusters - aurora
*
*
* -
*
* Multi-AZ DB clusters - io1
*
*
*
*
* @param storageType
* The storage type to associate with the DB cluster.
*
* For information on storage types for Aurora DB clusters, see Storage configurations for Amazon Aurora DB clusters. For information on storage types for Multi-AZ
* DB clusters, see Settings for creating Multi-AZ DB clusters.
*
*
* When specified for a Multi-AZ DB cluster, a value for the Iops
parameter is required.
*
*
* Valid for Cluster Type: Aurora DB clusters and Multi-AZ DB clusters
*
*
* Valid Values:
*
*
* -
*
* Aurora DB clusters - aurora | aurora-iopt1
*
*
* -
*
* Multi-AZ DB clusters - io1 | io2 | gp3
*
*
*
*
* Default:
*
*
* -
*
* Aurora DB clusters - aurora
*
*
* -
*
* Multi-AZ DB clusters - io1
*
*
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ModifyDBClusterRequest withStorageType(String storageType) {
setStorageType(storageType);
return this;
}
/**
*
* The amount of Provisioned IOPS (input/output operations per second) to be initially allocated for each DB
* instance in the Multi-AZ DB cluster.
*
*
* For information about valid IOPS values, see Amazon RDS Provisioned
* IOPS storage in the Amazon RDS User Guide.
*
*
* Valid for Cluster Type: Multi-AZ DB clusters only
*
*
* Constraints:
*
*
* -
*
* Must be a multiple between .5 and 50 of the storage amount for the DB cluster.
*
*
*
*
* @param iops
* The amount of Provisioned IOPS (input/output operations per second) to be initially allocated for each DB
* instance in the Multi-AZ DB cluster.
*
* For information about valid IOPS values, see Amazon RDS
* Provisioned IOPS storage in the Amazon RDS User Guide.
*
*
* Valid for Cluster Type: Multi-AZ DB clusters only
*
*
* Constraints:
*
*
* -
*
* Must be a multiple between .5 and 50 of the storage amount for the DB cluster.
*
*
*/
public void setIops(Integer iops) {
this.iops = iops;
}
/**
*
* The amount of Provisioned IOPS (input/output operations per second) to be initially allocated for each DB
* instance in the Multi-AZ DB cluster.
*
*
* For information about valid IOPS values, see Amazon RDS Provisioned
* IOPS storage in the Amazon RDS User Guide.
*
*
* Valid for Cluster Type: Multi-AZ DB clusters only
*
*
* Constraints:
*
*
* -
*
* Must be a multiple between .5 and 50 of the storage amount for the DB cluster.
*
*
*
*
* @return The amount of Provisioned IOPS (input/output operations per second) to be initially allocated for each DB
* instance in the Multi-AZ DB cluster.
*
* For information about valid IOPS values, see Amazon RDS
* Provisioned IOPS storage in the Amazon RDS User Guide.
*
*
* Valid for Cluster Type: Multi-AZ DB clusters only
*
*
* Constraints:
*
*
* -
*
* Must be a multiple between .5 and 50 of the storage amount for the DB cluster.
*
*
*/
public Integer getIops() {
return this.iops;
}
/**
*
* The amount of Provisioned IOPS (input/output operations per second) to be initially allocated for each DB
* instance in the Multi-AZ DB cluster.
*
*
* For information about valid IOPS values, see Amazon RDS Provisioned
* IOPS storage in the Amazon RDS User Guide.
*
*
* Valid for Cluster Type: Multi-AZ DB clusters only
*
*
* Constraints:
*
*
* -
*
* Must be a multiple between .5 and 50 of the storage amount for the DB cluster.
*
*
*
*
* @param iops
* The amount of Provisioned IOPS (input/output operations per second) to be initially allocated for each DB
* instance in the Multi-AZ DB cluster.
*
* For information about valid IOPS values, see Amazon RDS
* Provisioned IOPS storage in the Amazon RDS User Guide.
*
*
* Valid for Cluster Type: Multi-AZ DB clusters only
*
*
* Constraints:
*
*
* -
*
* Must be a multiple between .5 and 50 of the storage amount for the DB cluster.
*
*
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ModifyDBClusterRequest withIops(Integer iops) {
setIops(iops);
return this;
}
/**
*
* Specifies whether minor engine upgrades are applied automatically to the DB cluster during the maintenance
* window. By default, minor engine upgrades are applied automatically.
*
*
* Valid for Cluster Type: Multi-AZ DB clusters only
*
*
* @param autoMinorVersionUpgrade
* Specifies whether minor engine upgrades are applied automatically to the DB cluster during the maintenance
* window. By default, minor engine upgrades are applied automatically.
*
* Valid for Cluster Type: Multi-AZ DB clusters only
*/
public void setAutoMinorVersionUpgrade(Boolean autoMinorVersionUpgrade) {
this.autoMinorVersionUpgrade = autoMinorVersionUpgrade;
}
/**
*
* Specifies whether minor engine upgrades are applied automatically to the DB cluster during the maintenance
* window. By default, minor engine upgrades are applied automatically.
*
*
* Valid for Cluster Type: Multi-AZ DB clusters only
*
*
* @return Specifies whether minor engine upgrades are applied automatically to the DB cluster during the
* maintenance window. By default, minor engine upgrades are applied automatically.
*
* Valid for Cluster Type: Multi-AZ DB clusters only
*/
public Boolean getAutoMinorVersionUpgrade() {
return this.autoMinorVersionUpgrade;
}
/**
*
* Specifies whether minor engine upgrades are applied automatically to the DB cluster during the maintenance
* window. By default, minor engine upgrades are applied automatically.
*
*
* Valid for Cluster Type: Multi-AZ DB clusters only
*
*
* @param autoMinorVersionUpgrade
* Specifies whether minor engine upgrades are applied automatically to the DB cluster during the maintenance
* window. By default, minor engine upgrades are applied automatically.
*
* Valid for Cluster Type: Multi-AZ DB clusters only
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ModifyDBClusterRequest withAutoMinorVersionUpgrade(Boolean autoMinorVersionUpgrade) {
setAutoMinorVersionUpgrade(autoMinorVersionUpgrade);
return this;
}
/**
*
* Specifies whether minor engine upgrades are applied automatically to the DB cluster during the maintenance
* window. By default, minor engine upgrades are applied automatically.
*
*
* Valid for Cluster Type: Multi-AZ DB clusters only
*
*
* @return Specifies whether minor engine upgrades are applied automatically to the DB cluster during the
* maintenance window. By default, minor engine upgrades are applied automatically.
*
* Valid for Cluster Type: Multi-AZ DB clusters only
*/
public Boolean isAutoMinorVersionUpgrade() {
return this.autoMinorVersionUpgrade;
}
/**
*
* The interval, in seconds, between points when Enhanced Monitoring metrics are collected for the DB cluster. To
* turn off collecting Enhanced Monitoring metrics, specify 0
.
*
*
* If MonitoringRoleArn
is specified, also set MonitoringInterval
to a value other than
* 0
.
*
*
* Valid for Cluster Type: Multi-AZ DB clusters only
*
*
* Valid Values: 0 | 1 | 5 | 10 | 15 | 30 | 60
*
*
* Default: 0
*
*
* @param monitoringInterval
* The interval, in seconds, between points when Enhanced Monitoring metrics are collected for the DB
* cluster. To turn off collecting Enhanced Monitoring metrics, specify 0
.
*
* If MonitoringRoleArn
is specified, also set MonitoringInterval
to a value other
* than 0
.
*
*
* Valid for Cluster Type: Multi-AZ DB clusters only
*
*
* Valid Values: 0 | 1 | 5 | 10 | 15 | 30 | 60
*
*
* Default: 0
*/
public void setMonitoringInterval(Integer monitoringInterval) {
this.monitoringInterval = monitoringInterval;
}
/**
*
* The interval, in seconds, between points when Enhanced Monitoring metrics are collected for the DB cluster. To
* turn off collecting Enhanced Monitoring metrics, specify 0
.
*
*
* If MonitoringRoleArn
is specified, also set MonitoringInterval
to a value other than
* 0
.
*
*
* Valid for Cluster Type: Multi-AZ DB clusters only
*
*
* Valid Values: 0 | 1 | 5 | 10 | 15 | 30 | 60
*
*
* Default: 0
*
*
* @return The interval, in seconds, between points when Enhanced Monitoring metrics are collected for the DB
* cluster. To turn off collecting Enhanced Monitoring metrics, specify 0
.
*
* If MonitoringRoleArn
is specified, also set MonitoringInterval
to a value other
* than 0
.
*
*
* Valid for Cluster Type: Multi-AZ DB clusters only
*
*
* Valid Values: 0 | 1 | 5 | 10 | 15 | 30 | 60
*
*
* Default: 0
*/
public Integer getMonitoringInterval() {
return this.monitoringInterval;
}
/**
*
* The interval, in seconds, between points when Enhanced Monitoring metrics are collected for the DB cluster. To
* turn off collecting Enhanced Monitoring metrics, specify 0
.
*
*
* If MonitoringRoleArn
is specified, also set MonitoringInterval
to a value other than
* 0
.
*
*
* Valid for Cluster Type: Multi-AZ DB clusters only
*
*
* Valid Values: 0 | 1 | 5 | 10 | 15 | 30 | 60
*
*
* Default: 0
*
*
* @param monitoringInterval
* The interval, in seconds, between points when Enhanced Monitoring metrics are collected for the DB
* cluster. To turn off collecting Enhanced Monitoring metrics, specify 0
.
*
* If MonitoringRoleArn
is specified, also set MonitoringInterval
to a value other
* than 0
.
*
*
* Valid for Cluster Type: Multi-AZ DB clusters only
*
*
* Valid Values: 0 | 1 | 5 | 10 | 15 | 30 | 60
*
*
* Default: 0
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ModifyDBClusterRequest withMonitoringInterval(Integer monitoringInterval) {
setMonitoringInterval(monitoringInterval);
return this;
}
/**
*
* The Amazon Resource Name (ARN) for the IAM role that permits RDS to send Enhanced Monitoring metrics to Amazon
* CloudWatch Logs. An example is arn:aws:iam:123456789012:role/emaccess
. For information on creating a
* monitoring role, see To
* create an IAM role for Amazon RDS Enhanced Monitoring in the Amazon RDS User Guide.
*
*
* If MonitoringInterval
is set to a value other than 0
, supply a
* MonitoringRoleArn
value.
*
*
* Valid for Cluster Type: Multi-AZ DB clusters only
*
*
* @param monitoringRoleArn
* The Amazon Resource Name (ARN) for the IAM role that permits RDS to send Enhanced Monitoring metrics to
* Amazon CloudWatch Logs. An example is arn:aws:iam:123456789012:role/emaccess
. For information
* on creating a monitoring role, see To create an IAM role for Amazon RDS Enhanced Monitoring in the Amazon RDS User Guide.
*
* If MonitoringInterval
is set to a value other than 0
, supply a
* MonitoringRoleArn
value.
*
*
* Valid for Cluster Type: Multi-AZ DB clusters only
*/
public void setMonitoringRoleArn(String monitoringRoleArn) {
this.monitoringRoleArn = monitoringRoleArn;
}
/**
*
* The Amazon Resource Name (ARN) for the IAM role that permits RDS to send Enhanced Monitoring metrics to Amazon
* CloudWatch Logs. An example is arn:aws:iam:123456789012:role/emaccess
. For information on creating a
* monitoring role, see To
* create an IAM role for Amazon RDS Enhanced Monitoring in the Amazon RDS User Guide.
*
*
* If MonitoringInterval
is set to a value other than 0
, supply a
* MonitoringRoleArn
value.
*
*
* Valid for Cluster Type: Multi-AZ DB clusters only
*
*
* @return The Amazon Resource Name (ARN) for the IAM role that permits RDS to send Enhanced Monitoring metrics to
* Amazon CloudWatch Logs. An example is arn:aws:iam:123456789012:role/emaccess
. For
* information on creating a monitoring role, see To create an IAM role for Amazon RDS Enhanced Monitoring in the Amazon RDS User Guide.
*
* If MonitoringInterval
is set to a value other than 0
, supply a
* MonitoringRoleArn
value.
*
*
* Valid for Cluster Type: Multi-AZ DB clusters only
*/
public String getMonitoringRoleArn() {
return this.monitoringRoleArn;
}
/**
*
* The Amazon Resource Name (ARN) for the IAM role that permits RDS to send Enhanced Monitoring metrics to Amazon
* CloudWatch Logs. An example is arn:aws:iam:123456789012:role/emaccess
. For information on creating a
* monitoring role, see To
* create an IAM role for Amazon RDS Enhanced Monitoring in the Amazon RDS User Guide.
*
*
* If MonitoringInterval
is set to a value other than 0
, supply a
* MonitoringRoleArn
value.
*
*
* Valid for Cluster Type: Multi-AZ DB clusters only
*
*
* @param monitoringRoleArn
* The Amazon Resource Name (ARN) for the IAM role that permits RDS to send Enhanced Monitoring metrics to
* Amazon CloudWatch Logs. An example is arn:aws:iam:123456789012:role/emaccess
. For information
* on creating a monitoring role, see To create an IAM role for Amazon RDS Enhanced Monitoring in the Amazon RDS User Guide.
*
* If MonitoringInterval
is set to a value other than 0
, supply a
* MonitoringRoleArn
value.
*
*
* Valid for Cluster Type: Multi-AZ DB clusters only
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ModifyDBClusterRequest withMonitoringRoleArn(String monitoringRoleArn) {
setMonitoringRoleArn(monitoringRoleArn);
return this;
}
/**
*
* Specifies whether to turn on Performance Insights for the DB cluster.
*
*
* For more information, see Using Amazon Performance
* Insights in the Amazon RDS User Guide.
*
*
* Valid for Cluster Type: Multi-AZ DB clusters only
*
*
* @param enablePerformanceInsights
* Specifies whether to turn on Performance Insights for the DB cluster.
*
* For more information, see Using Amazon
* Performance Insights in the Amazon RDS User Guide.
*
*
* Valid for Cluster Type: Multi-AZ DB clusters only
*/
public void setEnablePerformanceInsights(Boolean enablePerformanceInsights) {
this.enablePerformanceInsights = enablePerformanceInsights;
}
/**
*
* Specifies whether to turn on Performance Insights for the DB cluster.
*
*
* For more information, see Using Amazon Performance
* Insights in the Amazon RDS User Guide.
*
*
* Valid for Cluster Type: Multi-AZ DB clusters only
*
*
* @return Specifies whether to turn on Performance Insights for the DB cluster.
*
* For more information, see Using Amazon
* Performance Insights in the Amazon RDS User Guide.
*
*
* Valid for Cluster Type: Multi-AZ DB clusters only
*/
public Boolean getEnablePerformanceInsights() {
return this.enablePerformanceInsights;
}
/**
*
* Specifies whether to turn on Performance Insights for the DB cluster.
*
*
* For more information, see Using Amazon Performance
* Insights in the Amazon RDS User Guide.
*
*
* Valid for Cluster Type: Multi-AZ DB clusters only
*
*
* @param enablePerformanceInsights
* Specifies whether to turn on Performance Insights for the DB cluster.
*
* For more information, see Using Amazon
* Performance Insights in the Amazon RDS User Guide.
*
*
* Valid for Cluster Type: Multi-AZ DB clusters only
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ModifyDBClusterRequest withEnablePerformanceInsights(Boolean enablePerformanceInsights) {
setEnablePerformanceInsights(enablePerformanceInsights);
return this;
}
/**
*
* Specifies whether to turn on Performance Insights for the DB cluster.
*
*
* For more information, see Using Amazon Performance
* Insights in the Amazon RDS User Guide.
*
*
* Valid for Cluster Type: Multi-AZ DB clusters only
*
*
* @return Specifies whether to turn on Performance Insights for the DB cluster.
*
* For more information, see Using Amazon
* Performance Insights in the Amazon RDS User Guide.
*
*
* Valid for Cluster Type: Multi-AZ DB clusters only
*/
public Boolean isEnablePerformanceInsights() {
return this.enablePerformanceInsights;
}
/**
*
* The Amazon Web Services KMS key identifier for encryption of Performance Insights data.
*
*
* The Amazon Web Services KMS key identifier is the key ARN, key ID, alias ARN, or alias name for the KMS key.
*
*
* If you don't specify a value for PerformanceInsightsKMSKeyId
, then Amazon RDS uses your default KMS
* key. There is a default KMS key for your Amazon Web Services account. Your Amazon Web Services account has a
* different default KMS key for each Amazon Web Services Region.
*
*
* Valid for Cluster Type: Multi-AZ DB clusters only
*
*
* @param performanceInsightsKMSKeyId
* The Amazon Web Services KMS key identifier for encryption of Performance Insights data.
*
* The Amazon Web Services KMS key identifier is the key ARN, key ID, alias ARN, or alias name for the KMS
* key.
*
*
* If you don't specify a value for PerformanceInsightsKMSKeyId
, then Amazon RDS uses your
* default KMS key. There is a default KMS key for your Amazon Web Services account. Your Amazon Web Services
* account has a different default KMS key for each Amazon Web Services Region.
*
*
* Valid for Cluster Type: Multi-AZ DB clusters only
*/
public void setPerformanceInsightsKMSKeyId(String performanceInsightsKMSKeyId) {
this.performanceInsightsKMSKeyId = performanceInsightsKMSKeyId;
}
/**
*
* The Amazon Web Services KMS key identifier for encryption of Performance Insights data.
*
*
* The Amazon Web Services KMS key identifier is the key ARN, key ID, alias ARN, or alias name for the KMS key.
*
*
* If you don't specify a value for PerformanceInsightsKMSKeyId
, then Amazon RDS uses your default KMS
* key. There is a default KMS key for your Amazon Web Services account. Your Amazon Web Services account has a
* different default KMS key for each Amazon Web Services Region.
*
*
* Valid for Cluster Type: Multi-AZ DB clusters only
*
*
* @return The Amazon Web Services KMS key identifier for encryption of Performance Insights data.
*
* The Amazon Web Services KMS key identifier is the key ARN, key ID, alias ARN, or alias name for the KMS
* key.
*
*
* If you don't specify a value for PerformanceInsightsKMSKeyId
, then Amazon RDS uses your
* default KMS key. There is a default KMS key for your Amazon Web Services account. Your Amazon Web
* Services account has a different default KMS key for each Amazon Web Services Region.
*
*
* Valid for Cluster Type: Multi-AZ DB clusters only
*/
public String getPerformanceInsightsKMSKeyId() {
return this.performanceInsightsKMSKeyId;
}
/**
*
* The Amazon Web Services KMS key identifier for encryption of Performance Insights data.
*
*
* The Amazon Web Services KMS key identifier is the key ARN, key ID, alias ARN, or alias name for the KMS key.
*
*
* If you don't specify a value for PerformanceInsightsKMSKeyId
, then Amazon RDS uses your default KMS
* key. There is a default KMS key for your Amazon Web Services account. Your Amazon Web Services account has a
* different default KMS key for each Amazon Web Services Region.
*
*
* Valid for Cluster Type: Multi-AZ DB clusters only
*
*
* @param performanceInsightsKMSKeyId
* The Amazon Web Services KMS key identifier for encryption of Performance Insights data.
*
* The Amazon Web Services KMS key identifier is the key ARN, key ID, alias ARN, or alias name for the KMS
* key.
*
*
* If you don't specify a value for PerformanceInsightsKMSKeyId
, then Amazon RDS uses your
* default KMS key. There is a default KMS key for your Amazon Web Services account. Your Amazon Web Services
* account has a different default KMS key for each Amazon Web Services Region.
*
*
* Valid for Cluster Type: Multi-AZ DB clusters only
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ModifyDBClusterRequest withPerformanceInsightsKMSKeyId(String performanceInsightsKMSKeyId) {
setPerformanceInsightsKMSKeyId(performanceInsightsKMSKeyId);
return this;
}
/**
*
* The number of days to retain Performance Insights data.
*
*
* Valid for Cluster Type: Multi-AZ DB clusters only
*
*
* Valid Values:
*
*
* -
*
* 7
*
*
* -
*
* month * 31, where month is a number of months from 1-23. Examples: 93
(3 months * 31),
* 341
(11 months * 31), 589
(19 months * 31)
*
*
* -
*
* 731
*
*
*
*
* Default: 7
days
*
*
* If you specify a retention period that isn't valid, such as 94
, Amazon RDS issues an error.
*
*
* @param performanceInsightsRetentionPeriod
* The number of days to retain Performance Insights data.
*
* Valid for Cluster Type: Multi-AZ DB clusters only
*
*
* Valid Values:
*
*
* -
*
* 7
*
*
* -
*
* month * 31, where month is a number of months from 1-23. Examples: 93
(3 months
* * 31), 341
(11 months * 31), 589
(19 months * 31)
*
*
* -
*
* 731
*
*
*
*
* Default: 7
days
*
*
* If you specify a retention period that isn't valid, such as 94
, Amazon RDS issues an error.
*/
public void setPerformanceInsightsRetentionPeriod(Integer performanceInsightsRetentionPeriod) {
this.performanceInsightsRetentionPeriod = performanceInsightsRetentionPeriod;
}
/**
*
* The number of days to retain Performance Insights data.
*
*
* Valid for Cluster Type: Multi-AZ DB clusters only
*
*
* Valid Values:
*
*
* -
*
* 7
*
*
* -
*
* month * 31, where month is a number of months from 1-23. Examples: 93
(3 months * 31),
* 341
(11 months * 31), 589
(19 months * 31)
*
*
* -
*
* 731
*
*
*
*
* Default: 7
days
*
*
* If you specify a retention period that isn't valid, such as 94
, Amazon RDS issues an error.
*
*
* @return The number of days to retain Performance Insights data.
*
* Valid for Cluster Type: Multi-AZ DB clusters only
*
*
* Valid Values:
*
*
* -
*
* 7
*
*
* -
*
* month * 31, where month is a number of months from 1-23. Examples: 93
(3
* months * 31), 341
(11 months * 31), 589
(19 months * 31)
*
*
* -
*
* 731
*
*
*
*
* Default: 7
days
*
*
* If you specify a retention period that isn't valid, such as 94
, Amazon RDS issues an error.
*/
public Integer getPerformanceInsightsRetentionPeriod() {
return this.performanceInsightsRetentionPeriod;
}
/**
*
* The number of days to retain Performance Insights data.
*
*
* Valid for Cluster Type: Multi-AZ DB clusters only
*
*
* Valid Values:
*
*
* -
*
* 7
*
*
* -
*
* month * 31, where month is a number of months from 1-23. Examples: 93
(3 months * 31),
* 341
(11 months * 31), 589
(19 months * 31)
*
*
* -
*
* 731
*
*
*
*
* Default: 7
days
*
*
* If you specify a retention period that isn't valid, such as 94
, Amazon RDS issues an error.
*
*
* @param performanceInsightsRetentionPeriod
* The number of days to retain Performance Insights data.
*
* Valid for Cluster Type: Multi-AZ DB clusters only
*
*
* Valid Values:
*
*
* -
*
* 7
*
*
* -
*
* month * 31, where month is a number of months from 1-23. Examples: 93
(3 months
* * 31), 341
(11 months * 31), 589
(19 months * 31)
*
*
* -
*
* 731
*
*
*
*
* Default: 7
days
*
*
* If you specify a retention period that isn't valid, such as 94
, Amazon RDS issues an error.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ModifyDBClusterRequest withPerformanceInsightsRetentionPeriod(Integer performanceInsightsRetentionPeriod) {
setPerformanceInsightsRetentionPeriod(performanceInsightsRetentionPeriod);
return this;
}
/**
* @param serverlessV2ScalingConfiguration
*/
public void setServerlessV2ScalingConfiguration(ServerlessV2ScalingConfiguration serverlessV2ScalingConfiguration) {
this.serverlessV2ScalingConfiguration = serverlessV2ScalingConfiguration;
}
/**
* @return
*/
public ServerlessV2ScalingConfiguration getServerlessV2ScalingConfiguration() {
return this.serverlessV2ScalingConfiguration;
}
/**
* @param serverlessV2ScalingConfiguration
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ModifyDBClusterRequest withServerlessV2ScalingConfiguration(ServerlessV2ScalingConfiguration serverlessV2ScalingConfiguration) {
setServerlessV2ScalingConfiguration(serverlessV2ScalingConfiguration);
return this;
}
/**
*
* The network type of the DB cluster.
*
*
* The network type is determined by the DBSubnetGroup
specified for the DB cluster. A
* DBSubnetGroup
can support only the IPv4 protocol or the IPv4 and the IPv6 protocols (
* DUAL
).
*
*
* For more information, see
* Working with a DB instance in a VPC in the Amazon Aurora User Guide.
*
*
* Valid for Cluster Type: Aurora DB clusters only
*
*
* Valid Values: IPV4 | DUAL
*
*
* @param networkType
* The network type of the DB cluster.
*
* The network type is determined by the DBSubnetGroup
specified for the DB cluster. A
* DBSubnetGroup
can support only the IPv4 protocol or the IPv4 and the IPv6 protocols (
* DUAL
).
*
*
* For more information, see
* Working with a DB instance in a VPC in the Amazon Aurora User Guide.
*
*
* Valid for Cluster Type: Aurora DB clusters only
*
*
* Valid Values: IPV4 | DUAL
*/
public void setNetworkType(String networkType) {
this.networkType = networkType;
}
/**
*
* The network type of the DB cluster.
*
*
* The network type is determined by the DBSubnetGroup
specified for the DB cluster. A
* DBSubnetGroup
can support only the IPv4 protocol or the IPv4 and the IPv6 protocols (
* DUAL
).
*
*
* For more information, see
* Working with a DB instance in a VPC in the Amazon Aurora User Guide.
*
*
* Valid for Cluster Type: Aurora DB clusters only
*
*
* Valid Values: IPV4 | DUAL
*
*
* @return The network type of the DB cluster.
*
* The network type is determined by the DBSubnetGroup
specified for the DB cluster. A
* DBSubnetGroup
can support only the IPv4 protocol or the IPv4 and the IPv6 protocols (
* DUAL
).
*
*
* For more information, see Working with a DB instance in a VPC in the Amazon Aurora User Guide.
*
*
* Valid for Cluster Type: Aurora DB clusters only
*
*
* Valid Values: IPV4 | DUAL
*/
public String getNetworkType() {
return this.networkType;
}
/**
*
* The network type of the DB cluster.
*
*
* The network type is determined by the DBSubnetGroup
specified for the DB cluster. A
* DBSubnetGroup
can support only the IPv4 protocol or the IPv4 and the IPv6 protocols (
* DUAL
).
*
*
* For more information, see
* Working with a DB instance in a VPC in the Amazon Aurora User Guide.
*
*
* Valid for Cluster Type: Aurora DB clusters only
*
*
* Valid Values: IPV4 | DUAL
*
*
* @param networkType
* The network type of the DB cluster.
*
* The network type is determined by the DBSubnetGroup
specified for the DB cluster. A
* DBSubnetGroup
can support only the IPv4 protocol or the IPv4 and the IPv6 protocols (
* DUAL
).
*
*
* For more information, see
* Working with a DB instance in a VPC in the Amazon Aurora User Guide.
*
*
* Valid for Cluster Type: Aurora DB clusters only
*
*
* Valid Values: IPV4 | DUAL
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ModifyDBClusterRequest withNetworkType(String networkType) {
setNetworkType(networkType);
return this;
}
/**
*
* Specifies whether to manage the master user password with Amazon Web Services Secrets Manager.
*
*
* If the DB cluster doesn't manage the master user password with Amazon Web Services Secrets Manager, you can turn
* on this management. In this case, you can't specify MasterUserPassword
.
*
*
* If the DB cluster already manages the master user password with Amazon Web Services Secrets Manager, and you
* specify that the master user password is not managed with Amazon Web Services Secrets Manager, then you must
* specify MasterUserPassword
. In this case, RDS deletes the secret and uses the new password for the
* master user specified by MasterUserPassword
.
*
*
* For more information, see Password management with
* Amazon Web Services Secrets Manager in the Amazon RDS User Guide and Password management
* with Amazon Web Services Secrets Manager in the Amazon Aurora User Guide.
*
*
* Valid for Cluster Type: Aurora DB clusters and Multi-AZ DB clusters
*
*
* @param manageMasterUserPassword
* Specifies whether to manage the master user password with Amazon Web Services Secrets Manager.
*
* If the DB cluster doesn't manage the master user password with Amazon Web Services Secrets Manager, you
* can turn on this management. In this case, you can't specify MasterUserPassword
.
*
*
* If the DB cluster already manages the master user password with Amazon Web Services Secrets Manager, and
* you specify that the master user password is not managed with Amazon Web Services Secrets Manager, then
* you must specify MasterUserPassword
. In this case, RDS deletes the secret and uses the new
* password for the master user specified by MasterUserPassword
.
*
*
* For more information, see Password management
* with Amazon Web Services Secrets Manager in the Amazon RDS User Guide and Password
* management with Amazon Web Services Secrets Manager in the Amazon Aurora User Guide.
*
*
* Valid for Cluster Type: Aurora DB clusters and Multi-AZ DB clusters
*/
public void setManageMasterUserPassword(Boolean manageMasterUserPassword) {
this.manageMasterUserPassword = manageMasterUserPassword;
}
/**
*
* Specifies whether to manage the master user password with Amazon Web Services Secrets Manager.
*
*
* If the DB cluster doesn't manage the master user password with Amazon Web Services Secrets Manager, you can turn
* on this management. In this case, you can't specify MasterUserPassword
.
*
*
* If the DB cluster already manages the master user password with Amazon Web Services Secrets Manager, and you
* specify that the master user password is not managed with Amazon Web Services Secrets Manager, then you must
* specify MasterUserPassword
. In this case, RDS deletes the secret and uses the new password for the
* master user specified by MasterUserPassword
.
*
*
* For more information, see Password management with
* Amazon Web Services Secrets Manager in the Amazon RDS User Guide and Password management
* with Amazon Web Services Secrets Manager in the Amazon Aurora User Guide.
*
*
* Valid for Cluster Type: Aurora DB clusters and Multi-AZ DB clusters
*
*
* @return Specifies whether to manage the master user password with Amazon Web Services Secrets Manager.
*
* If the DB cluster doesn't manage the master user password with Amazon Web Services Secrets Manager, you
* can turn on this management. In this case, you can't specify MasterUserPassword
.
*
*
* If the DB cluster already manages the master user password with Amazon Web Services Secrets Manager, and
* you specify that the master user password is not managed with Amazon Web Services Secrets Manager, then
* you must specify MasterUserPassword
. In this case, RDS deletes the secret and uses the new
* password for the master user specified by MasterUserPassword
.
*
*
* For more information, see Password
* management with Amazon Web Services Secrets Manager in the Amazon RDS User Guide and Password
* management with Amazon Web Services Secrets Manager in the Amazon Aurora User Guide.
*
*
* Valid for Cluster Type: Aurora DB clusters and Multi-AZ DB clusters
*/
public Boolean getManageMasterUserPassword() {
return this.manageMasterUserPassword;
}
/**
*
* Specifies whether to manage the master user password with Amazon Web Services Secrets Manager.
*
*
* If the DB cluster doesn't manage the master user password with Amazon Web Services Secrets Manager, you can turn
* on this management. In this case, you can't specify MasterUserPassword
.
*
*
* If the DB cluster already manages the master user password with Amazon Web Services Secrets Manager, and you
* specify that the master user password is not managed with Amazon Web Services Secrets Manager, then you must
* specify MasterUserPassword
. In this case, RDS deletes the secret and uses the new password for the
* master user specified by MasterUserPassword
.
*
*
* For more information, see Password management with
* Amazon Web Services Secrets Manager in the Amazon RDS User Guide and Password management
* with Amazon Web Services Secrets Manager in the Amazon Aurora User Guide.
*
*
* Valid for Cluster Type: Aurora DB clusters and Multi-AZ DB clusters
*
*
* @param manageMasterUserPassword
* Specifies whether to manage the master user password with Amazon Web Services Secrets Manager.
*
* If the DB cluster doesn't manage the master user password with Amazon Web Services Secrets Manager, you
* can turn on this management. In this case, you can't specify MasterUserPassword
.
*
*
* If the DB cluster already manages the master user password with Amazon Web Services Secrets Manager, and
* you specify that the master user password is not managed with Amazon Web Services Secrets Manager, then
* you must specify MasterUserPassword
. In this case, RDS deletes the secret and uses the new
* password for the master user specified by MasterUserPassword
.
*
*
* For more information, see Password management
* with Amazon Web Services Secrets Manager in the Amazon RDS User Guide and Password
* management with Amazon Web Services Secrets Manager in the Amazon Aurora User Guide.
*
*
* Valid for Cluster Type: Aurora DB clusters and Multi-AZ DB clusters
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ModifyDBClusterRequest withManageMasterUserPassword(Boolean manageMasterUserPassword) {
setManageMasterUserPassword(manageMasterUserPassword);
return this;
}
/**
*
* Specifies whether to manage the master user password with Amazon Web Services Secrets Manager.
*
*
* If the DB cluster doesn't manage the master user password with Amazon Web Services Secrets Manager, you can turn
* on this management. In this case, you can't specify MasterUserPassword
.
*
*
* If the DB cluster already manages the master user password with Amazon Web Services Secrets Manager, and you
* specify that the master user password is not managed with Amazon Web Services Secrets Manager, then you must
* specify MasterUserPassword
. In this case, RDS deletes the secret and uses the new password for the
* master user specified by MasterUserPassword
.
*
*
* For more information, see Password management with
* Amazon Web Services Secrets Manager in the Amazon RDS User Guide and Password management
* with Amazon Web Services Secrets Manager in the Amazon Aurora User Guide.
*
*
* Valid for Cluster Type: Aurora DB clusters and Multi-AZ DB clusters
*
*
* @return Specifies whether to manage the master user password with Amazon Web Services Secrets Manager.
*
* If the DB cluster doesn't manage the master user password with Amazon Web Services Secrets Manager, you
* can turn on this management. In this case, you can't specify MasterUserPassword
.
*
*
* If the DB cluster already manages the master user password with Amazon Web Services Secrets Manager, and
* you specify that the master user password is not managed with Amazon Web Services Secrets Manager, then
* you must specify MasterUserPassword
. In this case, RDS deletes the secret and uses the new
* password for the master user specified by MasterUserPassword
.
*
*
* For more information, see Password
* management with Amazon Web Services Secrets Manager in the Amazon RDS User Guide and Password
* management with Amazon Web Services Secrets Manager in the Amazon Aurora User Guide.
*
*
* Valid for Cluster Type: Aurora DB clusters and Multi-AZ DB clusters
*/
public Boolean isManageMasterUserPassword() {
return this.manageMasterUserPassword;
}
/**
*
* Specifies whether to rotate the secret managed by Amazon Web Services Secrets Manager for the master user
* password.
*
*
* This setting is valid only if the master user password is managed by RDS in Amazon Web Services Secrets Manager
* for the DB cluster. The secret value contains the updated password.
*
*
* For more information, see Password management with
* Amazon Web Services Secrets Manager in the Amazon RDS User Guide and Password management
* with Amazon Web Services Secrets Manager in the Amazon Aurora User Guide.
*
*
* Valid for Cluster Type: Aurora DB clusters and Multi-AZ DB clusters
*
*
* Constraints:
*
*
* -
*
* You must apply the change immediately when rotating the master user password.
*
*
*
*
* @param rotateMasterUserPassword
* Specifies whether to rotate the secret managed by Amazon Web Services Secrets Manager for the master user
* password.
*
* This setting is valid only if the master user password is managed by RDS in Amazon Web Services Secrets
* Manager for the DB cluster. The secret value contains the updated password.
*
*
* For more information, see Password management
* with Amazon Web Services Secrets Manager in the Amazon RDS User Guide and Password
* management with Amazon Web Services Secrets Manager in the Amazon Aurora User Guide.
*
*
* Valid for Cluster Type: Aurora DB clusters and Multi-AZ DB clusters
*
*
* Constraints:
*
*
* -
*
* You must apply the change immediately when rotating the master user password.
*
*
*/
public void setRotateMasterUserPassword(Boolean rotateMasterUserPassword) {
this.rotateMasterUserPassword = rotateMasterUserPassword;
}
/**
*
* Specifies whether to rotate the secret managed by Amazon Web Services Secrets Manager for the master user
* password.
*
*
* This setting is valid only if the master user password is managed by RDS in Amazon Web Services Secrets Manager
* for the DB cluster. The secret value contains the updated password.
*
*
* For more information, see Password management with
* Amazon Web Services Secrets Manager in the Amazon RDS User Guide and Password management
* with Amazon Web Services Secrets Manager in the Amazon Aurora User Guide.
*
*
* Valid for Cluster Type: Aurora DB clusters and Multi-AZ DB clusters
*
*
* Constraints:
*
*
* -
*
* You must apply the change immediately when rotating the master user password.
*
*
*
*
* @return Specifies whether to rotate the secret managed by Amazon Web Services Secrets Manager for the master user
* password.
*
* This setting is valid only if the master user password is managed by RDS in Amazon Web Services Secrets
* Manager for the DB cluster. The secret value contains the updated password.
*
*
* For more information, see Password
* management with Amazon Web Services Secrets Manager in the Amazon RDS User Guide and Password
* management with Amazon Web Services Secrets Manager in the Amazon Aurora User Guide.
*
*
* Valid for Cluster Type: Aurora DB clusters and Multi-AZ DB clusters
*
*
* Constraints:
*
*
* -
*
* You must apply the change immediately when rotating the master user password.
*
*
*/
public Boolean getRotateMasterUserPassword() {
return this.rotateMasterUserPassword;
}
/**
*
* Specifies whether to rotate the secret managed by Amazon Web Services Secrets Manager for the master user
* password.
*
*
* This setting is valid only if the master user password is managed by RDS in Amazon Web Services Secrets Manager
* for the DB cluster. The secret value contains the updated password.
*
*
* For more information, see Password management with
* Amazon Web Services Secrets Manager in the Amazon RDS User Guide and Password management
* with Amazon Web Services Secrets Manager in the Amazon Aurora User Guide.
*
*
* Valid for Cluster Type: Aurora DB clusters and Multi-AZ DB clusters
*
*
* Constraints:
*
*
* -
*
* You must apply the change immediately when rotating the master user password.
*
*
*
*
* @param rotateMasterUserPassword
* Specifies whether to rotate the secret managed by Amazon Web Services Secrets Manager for the master user
* password.
*
* This setting is valid only if the master user password is managed by RDS in Amazon Web Services Secrets
* Manager for the DB cluster. The secret value contains the updated password.
*
*
* For more information, see Password management
* with Amazon Web Services Secrets Manager in the Amazon RDS User Guide and Password
* management with Amazon Web Services Secrets Manager in the Amazon Aurora User Guide.
*
*
* Valid for Cluster Type: Aurora DB clusters and Multi-AZ DB clusters
*
*
* Constraints:
*
*
* -
*
* You must apply the change immediately when rotating the master user password.
*
*
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ModifyDBClusterRequest withRotateMasterUserPassword(Boolean rotateMasterUserPassword) {
setRotateMasterUserPassword(rotateMasterUserPassword);
return this;
}
/**
*
* Specifies whether to rotate the secret managed by Amazon Web Services Secrets Manager for the master user
* password.
*
*
* This setting is valid only if the master user password is managed by RDS in Amazon Web Services Secrets Manager
* for the DB cluster. The secret value contains the updated password.
*
*
* For more information, see Password management with
* Amazon Web Services Secrets Manager in the Amazon RDS User Guide and Password management
* with Amazon Web Services Secrets Manager in the Amazon Aurora User Guide.
*
*
* Valid for Cluster Type: Aurora DB clusters and Multi-AZ DB clusters
*
*
* Constraints:
*
*
* -
*
* You must apply the change immediately when rotating the master user password.
*
*
*
*
* @return Specifies whether to rotate the secret managed by Amazon Web Services Secrets Manager for the master user
* password.
*
* This setting is valid only if the master user password is managed by RDS in Amazon Web Services Secrets
* Manager for the DB cluster. The secret value contains the updated password.
*
*
* For more information, see Password
* management with Amazon Web Services Secrets Manager in the Amazon RDS User Guide and Password
* management with Amazon Web Services Secrets Manager in the Amazon Aurora User Guide.
*
*
* Valid for Cluster Type: Aurora DB clusters and Multi-AZ DB clusters
*
*
* Constraints:
*
*
* -
*
* You must apply the change immediately when rotating the master user password.
*
*
*/
public Boolean isRotateMasterUserPassword() {
return this.rotateMasterUserPassword;
}
/**
*
* The Amazon Web Services KMS key identifier to encrypt a secret that is automatically generated and managed in
* Amazon Web Services Secrets Manager.
*
*
* This setting is valid only if both of the following conditions are met:
*
*
* -
*
* The DB cluster doesn't manage the master user password in Amazon Web Services Secrets Manager.
*
*
* If the DB cluster already manages the master user password in Amazon Web Services Secrets Manager, you can't
* change the KMS key that is used to encrypt the secret.
*
*
* -
*
* You are turning on ManageMasterUserPassword
to manage the master user password in Amazon Web
* Services Secrets Manager.
*
*
* If you are turning on ManageMasterUserPassword
and don't specify
* MasterUserSecretKmsKeyId
, then the aws/secretsmanager
KMS key is used to encrypt the
* secret. If the secret is in a different Amazon Web Services account, then you can't use the
* aws/secretsmanager
KMS key to encrypt the secret, and you must use a customer managed KMS key.
*
*
*
*
* The Amazon Web Services KMS key identifier is the key ARN, key ID, alias ARN, or alias name for the KMS key. To
* use a KMS key in a different Amazon Web Services account, specify the key ARN or alias ARN.
*
*
* There is a default KMS key for your Amazon Web Services account. Your Amazon Web Services account has a different
* default KMS key for each Amazon Web Services Region.
*
*
* Valid for Cluster Type: Aurora DB clusters and Multi-AZ DB clusters
*
*
* @param masterUserSecretKmsKeyId
* The Amazon Web Services KMS key identifier to encrypt a secret that is automatically generated and managed
* in Amazon Web Services Secrets Manager.
*
* This setting is valid only if both of the following conditions are met:
*
*
* -
*
* The DB cluster doesn't manage the master user password in Amazon Web Services Secrets Manager.
*
*
* If the DB cluster already manages the master user password in Amazon Web Services Secrets Manager, you
* can't change the KMS key that is used to encrypt the secret.
*
*
* -
*
* You are turning on ManageMasterUserPassword
to manage the master user password in Amazon Web
* Services Secrets Manager.
*
*
* If you are turning on ManageMasterUserPassword
and don't specify
* MasterUserSecretKmsKeyId
, then the aws/secretsmanager
KMS key is used to encrypt
* the secret. If the secret is in a different Amazon Web Services account, then you can't use the
* aws/secretsmanager
KMS key to encrypt the secret, and you must use a customer managed KMS
* key.
*
*
*
*
* The Amazon Web Services KMS key identifier is the key ARN, key ID, alias ARN, or alias name for the KMS
* key. To use a KMS key in a different Amazon Web Services account, specify the key ARN or alias ARN.
*
*
* There is a default KMS key for your Amazon Web Services account. Your Amazon Web Services account has a
* different default KMS key for each Amazon Web Services Region.
*
*
* Valid for Cluster Type: Aurora DB clusters and Multi-AZ DB clusters
*/
public void setMasterUserSecretKmsKeyId(String masterUserSecretKmsKeyId) {
this.masterUserSecretKmsKeyId = masterUserSecretKmsKeyId;
}
/**
*
* The Amazon Web Services KMS key identifier to encrypt a secret that is automatically generated and managed in
* Amazon Web Services Secrets Manager.
*
*
* This setting is valid only if both of the following conditions are met:
*
*
* -
*
* The DB cluster doesn't manage the master user password in Amazon Web Services Secrets Manager.
*
*
* If the DB cluster already manages the master user password in Amazon Web Services Secrets Manager, you can't
* change the KMS key that is used to encrypt the secret.
*
*
* -
*
* You are turning on ManageMasterUserPassword
to manage the master user password in Amazon Web
* Services Secrets Manager.
*
*
* If you are turning on ManageMasterUserPassword
and don't specify
* MasterUserSecretKmsKeyId
, then the aws/secretsmanager
KMS key is used to encrypt the
* secret. If the secret is in a different Amazon Web Services account, then you can't use the
* aws/secretsmanager
KMS key to encrypt the secret, and you must use a customer managed KMS key.
*
*
*
*
* The Amazon Web Services KMS key identifier is the key ARN, key ID, alias ARN, or alias name for the KMS key. To
* use a KMS key in a different Amazon Web Services account, specify the key ARN or alias ARN.
*
*
* There is a default KMS key for your Amazon Web Services account. Your Amazon Web Services account has a different
* default KMS key for each Amazon Web Services Region.
*
*
* Valid for Cluster Type: Aurora DB clusters and Multi-AZ DB clusters
*
*
* @return The Amazon Web Services KMS key identifier to encrypt a secret that is automatically generated and
* managed in Amazon Web Services Secrets Manager.
*
* This setting is valid only if both of the following conditions are met:
*
*
* -
*
* The DB cluster doesn't manage the master user password in Amazon Web Services Secrets Manager.
*
*
* If the DB cluster already manages the master user password in Amazon Web Services Secrets Manager, you
* can't change the KMS key that is used to encrypt the secret.
*
*
* -
*
* You are turning on ManageMasterUserPassword
to manage the master user password in Amazon Web
* Services Secrets Manager.
*
*
* If you are turning on ManageMasterUserPassword
and don't specify
* MasterUserSecretKmsKeyId
, then the aws/secretsmanager
KMS key is used to
* encrypt the secret. If the secret is in a different Amazon Web Services account, then you can't use the
* aws/secretsmanager
KMS key to encrypt the secret, and you must use a customer managed KMS
* key.
*
*
*
*
* The Amazon Web Services KMS key identifier is the key ARN, key ID, alias ARN, or alias name for the KMS
* key. To use a KMS key in a different Amazon Web Services account, specify the key ARN or alias ARN.
*
*
* There is a default KMS key for your Amazon Web Services account. Your Amazon Web Services account has a
* different default KMS key for each Amazon Web Services Region.
*
*
* Valid for Cluster Type: Aurora DB clusters and Multi-AZ DB clusters
*/
public String getMasterUserSecretKmsKeyId() {
return this.masterUserSecretKmsKeyId;
}
/**
*
* The Amazon Web Services KMS key identifier to encrypt a secret that is automatically generated and managed in
* Amazon Web Services Secrets Manager.
*
*
* This setting is valid only if both of the following conditions are met:
*
*
* -
*
* The DB cluster doesn't manage the master user password in Amazon Web Services Secrets Manager.
*
*
* If the DB cluster already manages the master user password in Amazon Web Services Secrets Manager, you can't
* change the KMS key that is used to encrypt the secret.
*
*
* -
*
* You are turning on ManageMasterUserPassword
to manage the master user password in Amazon Web
* Services Secrets Manager.
*
*
* If you are turning on ManageMasterUserPassword
and don't specify
* MasterUserSecretKmsKeyId
, then the aws/secretsmanager
KMS key is used to encrypt the
* secret. If the secret is in a different Amazon Web Services account, then you can't use the
* aws/secretsmanager
KMS key to encrypt the secret, and you must use a customer managed KMS key.
*
*
*
*
* The Amazon Web Services KMS key identifier is the key ARN, key ID, alias ARN, or alias name for the KMS key. To
* use a KMS key in a different Amazon Web Services account, specify the key ARN or alias ARN.
*
*
* There is a default KMS key for your Amazon Web Services account. Your Amazon Web Services account has a different
* default KMS key for each Amazon Web Services Region.
*
*
* Valid for Cluster Type: Aurora DB clusters and Multi-AZ DB clusters
*
*
* @param masterUserSecretKmsKeyId
* The Amazon Web Services KMS key identifier to encrypt a secret that is automatically generated and managed
* in Amazon Web Services Secrets Manager.
*
* This setting is valid only if both of the following conditions are met:
*
*
* -
*
* The DB cluster doesn't manage the master user password in Amazon Web Services Secrets Manager.
*
*
* If the DB cluster already manages the master user password in Amazon Web Services Secrets Manager, you
* can't change the KMS key that is used to encrypt the secret.
*
*
* -
*
* You are turning on ManageMasterUserPassword
to manage the master user password in Amazon Web
* Services Secrets Manager.
*
*
* If you are turning on ManageMasterUserPassword
and don't specify
* MasterUserSecretKmsKeyId
, then the aws/secretsmanager
KMS key is used to encrypt
* the secret. If the secret is in a different Amazon Web Services account, then you can't use the
* aws/secretsmanager
KMS key to encrypt the secret, and you must use a customer managed KMS
* key.
*
*
*
*
* The Amazon Web Services KMS key identifier is the key ARN, key ID, alias ARN, or alias name for the KMS
* key. To use a KMS key in a different Amazon Web Services account, specify the key ARN or alias ARN.
*
*
* There is a default KMS key for your Amazon Web Services account. Your Amazon Web Services account has a
* different default KMS key for each Amazon Web Services Region.
*
*
* Valid for Cluster Type: Aurora DB clusters and Multi-AZ DB clusters
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ModifyDBClusterRequest withMasterUserSecretKmsKeyId(String masterUserSecretKmsKeyId) {
setMasterUserSecretKmsKeyId(masterUserSecretKmsKeyId);
return this;
}
/**
*
* The DB engine mode of the DB cluster, either provisioned
or serverless
.
*
*
*
* The DB engine mode can be modified only from serverless
to provisioned
.
*
*
*
* For more information, see CreateDBCluster.
*
*
* Valid for Cluster Type: Aurora DB clusters only
*
*
* @param engineMode
* The DB engine mode of the DB cluster, either provisioned
or serverless
.
*
*
* The DB engine mode can be modified only from serverless
to provisioned
.
*
*
*
* For more information, see
* CreateDBCluster.
*
*
* Valid for Cluster Type: Aurora DB clusters only
*/
public void setEngineMode(String engineMode) {
this.engineMode = engineMode;
}
/**
*
* The DB engine mode of the DB cluster, either provisioned
or serverless
.
*
*
*
* The DB engine mode can be modified only from serverless
to provisioned
.
*
*
*
* For more information, see CreateDBCluster.
*
*
* Valid for Cluster Type: Aurora DB clusters only
*
*
* @return The DB engine mode of the DB cluster, either provisioned
or serverless
.
*
*
* The DB engine mode can be modified only from serverless
to provisioned
.
*
*
*
* For more information, see
* CreateDBCluster.
*
*
* Valid for Cluster Type: Aurora DB clusters only
*/
public String getEngineMode() {
return this.engineMode;
}
/**
*
* The DB engine mode of the DB cluster, either provisioned
or serverless
.
*
*
*
* The DB engine mode can be modified only from serverless
to provisioned
.
*
*
*
* For more information, see CreateDBCluster.
*
*
* Valid for Cluster Type: Aurora DB clusters only
*
*
* @param engineMode
* The DB engine mode of the DB cluster, either provisioned
or serverless
.
*
*
* The DB engine mode can be modified only from serverless
to provisioned
.
*
*
*
* For more information, see
* CreateDBCluster.
*
*
* Valid for Cluster Type: Aurora DB clusters only
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ModifyDBClusterRequest withEngineMode(String engineMode) {
setEngineMode(engineMode);
return this;
}
/**
*
* Specifies whether engine mode changes from serverless
to provisioned
are allowed.
*
*
* Valid for Cluster Type: Aurora Serverless v1 DB clusters only
*
*
* Constraints:
*
*
* -
*
* You must allow engine mode changes when specifying a different value for the EngineMode
parameter
* from the DB cluster's current engine mode.
*
*
*
*
* @param allowEngineModeChange
* Specifies whether engine mode changes from serverless
to provisioned
are
* allowed.
*
* Valid for Cluster Type: Aurora Serverless v1 DB clusters only
*
*
* Constraints:
*
*
* -
*
* You must allow engine mode changes when specifying a different value for the EngineMode
* parameter from the DB cluster's current engine mode.
*
*
*/
public void setAllowEngineModeChange(Boolean allowEngineModeChange) {
this.allowEngineModeChange = allowEngineModeChange;
}
/**
*
* Specifies whether engine mode changes from serverless
to provisioned
are allowed.
*
*
* Valid for Cluster Type: Aurora Serverless v1 DB clusters only
*
*
* Constraints:
*
*
* -
*
* You must allow engine mode changes when specifying a different value for the EngineMode
parameter
* from the DB cluster's current engine mode.
*
*
*
*
* @return Specifies whether engine mode changes from serverless
to provisioned
are
* allowed.
*
* Valid for Cluster Type: Aurora Serverless v1 DB clusters only
*
*
* Constraints:
*
*
* -
*
* You must allow engine mode changes when specifying a different value for the EngineMode
* parameter from the DB cluster's current engine mode.
*
*
*/
public Boolean getAllowEngineModeChange() {
return this.allowEngineModeChange;
}
/**
*
* Specifies whether engine mode changes from serverless
to provisioned
are allowed.
*
*
* Valid for Cluster Type: Aurora Serverless v1 DB clusters only
*
*
* Constraints:
*
*
* -
*
* You must allow engine mode changes when specifying a different value for the EngineMode
parameter
* from the DB cluster's current engine mode.
*
*
*
*
* @param allowEngineModeChange
* Specifies whether engine mode changes from serverless
to provisioned
are
* allowed.
*
* Valid for Cluster Type: Aurora Serverless v1 DB clusters only
*
*
* Constraints:
*
*
* -
*
* You must allow engine mode changes when specifying a different value for the EngineMode
* parameter from the DB cluster's current engine mode.
*
*
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ModifyDBClusterRequest withAllowEngineModeChange(Boolean allowEngineModeChange) {
setAllowEngineModeChange(allowEngineModeChange);
return this;
}
/**
*
* Specifies whether engine mode changes from serverless
to provisioned
are allowed.
*
*
* Valid for Cluster Type: Aurora Serverless v1 DB clusters only
*
*
* Constraints:
*
*
* -
*
* You must allow engine mode changes when specifying a different value for the EngineMode
parameter
* from the DB cluster's current engine mode.
*
*
*
*
* @return Specifies whether engine mode changes from serverless
to provisioned
are
* allowed.
*
* Valid for Cluster Type: Aurora Serverless v1 DB clusters only
*
*
* Constraints:
*
*
* -
*
* You must allow engine mode changes when specifying a different value for the EngineMode
* parameter from the DB cluster's current engine mode.
*
*
*/
public Boolean isAllowEngineModeChange() {
return this.allowEngineModeChange;
}
/**
*
* Specifies whether read replicas can forward write operations to the writer DB instance in the DB cluster. By
* default, write operations aren't allowed on reader DB instances.
*
*
* Valid for: Aurora DB clusters only
*
*
* @param enableLocalWriteForwarding
* Specifies whether read replicas can forward write operations to the writer DB instance in the DB cluster.
* By default, write operations aren't allowed on reader DB instances.
*
* Valid for: Aurora DB clusters only
*/
public void setEnableLocalWriteForwarding(Boolean enableLocalWriteForwarding) {
this.enableLocalWriteForwarding = enableLocalWriteForwarding;
}
/**
*
* Specifies whether read replicas can forward write operations to the writer DB instance in the DB cluster. By
* default, write operations aren't allowed on reader DB instances.
*
*
* Valid for: Aurora DB clusters only
*
*
* @return Specifies whether read replicas can forward write operations to the writer DB instance in the DB cluster.
* By default, write operations aren't allowed on reader DB instances.
*
* Valid for: Aurora DB clusters only
*/
public Boolean getEnableLocalWriteForwarding() {
return this.enableLocalWriteForwarding;
}
/**
*
* Specifies whether read replicas can forward write operations to the writer DB instance in the DB cluster. By
* default, write operations aren't allowed on reader DB instances.
*
*
* Valid for: Aurora DB clusters only
*
*
* @param enableLocalWriteForwarding
* Specifies whether read replicas can forward write operations to the writer DB instance in the DB cluster.
* By default, write operations aren't allowed on reader DB instances.
*
* Valid for: Aurora DB clusters only
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ModifyDBClusterRequest withEnableLocalWriteForwarding(Boolean enableLocalWriteForwarding) {
setEnableLocalWriteForwarding(enableLocalWriteForwarding);
return this;
}
/**
*
* Specifies whether read replicas can forward write operations to the writer DB instance in the DB cluster. By
* default, write operations aren't allowed on reader DB instances.
*
*
* Valid for: Aurora DB clusters only
*
*
* @return Specifies whether read replicas can forward write operations to the writer DB instance in the DB cluster.
* By default, write operations aren't allowed on reader DB instances.
*
* Valid for: Aurora DB clusters only
*/
public Boolean isEnableLocalWriteForwarding() {
return this.enableLocalWriteForwarding;
}
/**
*
* The Amazon Resource Name (ARN) of the recovery point in Amazon Web Services Backup.
*
*
* @param awsBackupRecoveryPointArn
* The Amazon Resource Name (ARN) of the recovery point in Amazon Web Services Backup.
*/
public void setAwsBackupRecoveryPointArn(String awsBackupRecoveryPointArn) {
this.awsBackupRecoveryPointArn = awsBackupRecoveryPointArn;
}
/**
*
* The Amazon Resource Name (ARN) of the recovery point in Amazon Web Services Backup.
*
*
* @return The Amazon Resource Name (ARN) of the recovery point in Amazon Web Services Backup.
*/
public String getAwsBackupRecoveryPointArn() {
return this.awsBackupRecoveryPointArn;
}
/**
*
* The Amazon Resource Name (ARN) of the recovery point in Amazon Web Services Backup.
*
*
* @param awsBackupRecoveryPointArn
* The Amazon Resource Name (ARN) of the recovery point in Amazon Web Services Backup.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ModifyDBClusterRequest withAwsBackupRecoveryPointArn(String awsBackupRecoveryPointArn) {
setAwsBackupRecoveryPointArn(awsBackupRecoveryPointArn);
return this;
}
/**
*
* Specifies whether to enable Aurora Limitless Database. You must enable Aurora Limitless Database to create a DB
* shard group.
*
*
* Valid for: Aurora DB clusters only
*
*
* @param enableLimitlessDatabase
* Specifies whether to enable Aurora Limitless Database. You must enable Aurora Limitless Database to create
* a DB shard group.
*
* Valid for: Aurora DB clusters only
*/
public void setEnableLimitlessDatabase(Boolean enableLimitlessDatabase) {
this.enableLimitlessDatabase = enableLimitlessDatabase;
}
/**
*
* Specifies whether to enable Aurora Limitless Database. You must enable Aurora Limitless Database to create a DB
* shard group.
*
*
* Valid for: Aurora DB clusters only
*
*
* @return Specifies whether to enable Aurora Limitless Database. You must enable Aurora Limitless Database to
* create a DB shard group.
*
* Valid for: Aurora DB clusters only
*/
public Boolean getEnableLimitlessDatabase() {
return this.enableLimitlessDatabase;
}
/**
*
* Specifies whether to enable Aurora Limitless Database. You must enable Aurora Limitless Database to create a DB
* shard group.
*
*
* Valid for: Aurora DB clusters only
*
*
* @param enableLimitlessDatabase
* Specifies whether to enable Aurora Limitless Database. You must enable Aurora Limitless Database to create
* a DB shard group.
*
* Valid for: Aurora DB clusters only
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ModifyDBClusterRequest withEnableLimitlessDatabase(Boolean enableLimitlessDatabase) {
setEnableLimitlessDatabase(enableLimitlessDatabase);
return this;
}
/**
*
* Specifies whether to enable Aurora Limitless Database. You must enable Aurora Limitless Database to create a DB
* shard group.
*
*
* Valid for: Aurora DB clusters only
*
*
* @return Specifies whether to enable Aurora Limitless Database. You must enable Aurora Limitless Database to
* create a DB shard group.
*
* Valid for: Aurora DB clusters only
*/
public Boolean isEnableLimitlessDatabase() {
return this.enableLimitlessDatabase;
}
/**
*
* The CA certificate identifier to use for the DB cluster's server certificate.
*
*
* For more information, see Using SSL/TLS to encrypt a
* connection to a DB instance in the Amazon RDS User Guide.
*
*
* Valid for Cluster Type: Multi-AZ DB clusters
*
*
* @param cACertificateIdentifier
* The CA certificate identifier to use for the DB cluster's server certificate.
*
* For more information, see Using SSL/TLS to
* encrypt a connection to a DB instance in the Amazon RDS User Guide.
*
*
* Valid for Cluster Type: Multi-AZ DB clusters
*/
public void setCACertificateIdentifier(String cACertificateIdentifier) {
this.cACertificateIdentifier = cACertificateIdentifier;
}
/**
*
* The CA certificate identifier to use for the DB cluster's server certificate.
*
*
* For more information, see Using SSL/TLS to encrypt a
* connection to a DB instance in the Amazon RDS User Guide.
*
*
* Valid for Cluster Type: Multi-AZ DB clusters
*
*
* @return The CA certificate identifier to use for the DB cluster's server certificate.
*
* For more information, see Using SSL/TLS to
* encrypt a connection to a DB instance in the Amazon RDS User Guide.
*
*
* Valid for Cluster Type: Multi-AZ DB clusters
*/
public String getCACertificateIdentifier() {
return this.cACertificateIdentifier;
}
/**
*
* The CA certificate identifier to use for the DB cluster's server certificate.
*
*
* For more information, see Using SSL/TLS to encrypt a
* connection to a DB instance in the Amazon RDS User Guide.
*
*
* Valid for Cluster Type: Multi-AZ DB clusters
*
*
* @param cACertificateIdentifier
* The CA certificate identifier to use for the DB cluster's server certificate.
*
* For more information, see Using SSL/TLS to
* encrypt a connection to a DB instance in the Amazon RDS User Guide.
*
*
* Valid for Cluster Type: Multi-AZ DB clusters
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ModifyDBClusterRequest withCACertificateIdentifier(String cACertificateIdentifier) {
setCACertificateIdentifier(cACertificateIdentifier);
return this;
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getDBClusterIdentifier() != null)
sb.append("DBClusterIdentifier: ").append(getDBClusterIdentifier()).append(",");
if (getNewDBClusterIdentifier() != null)
sb.append("NewDBClusterIdentifier: ").append(getNewDBClusterIdentifier()).append(",");
if (getApplyImmediately() != null)
sb.append("ApplyImmediately: ").append(getApplyImmediately()).append(",");
if (getBackupRetentionPeriod() != null)
sb.append("BackupRetentionPeriod: ").append(getBackupRetentionPeriod()).append(",");
if (getDBClusterParameterGroupName() != null)
sb.append("DBClusterParameterGroupName: ").append(getDBClusterParameterGroupName()).append(",");
if (getVpcSecurityGroupIds() != null)
sb.append("VpcSecurityGroupIds: ").append(getVpcSecurityGroupIds()).append(",");
if (getPort() != null)
sb.append("Port: ").append(getPort()).append(",");
if (getMasterUserPassword() != null)
sb.append("MasterUserPassword: ").append(getMasterUserPassword()).append(",");
if (getOptionGroupName() != null)
sb.append("OptionGroupName: ").append(getOptionGroupName()).append(",");
if (getPreferredBackupWindow() != null)
sb.append("PreferredBackupWindow: ").append(getPreferredBackupWindow()).append(",");
if (getPreferredMaintenanceWindow() != null)
sb.append("PreferredMaintenanceWindow: ").append(getPreferredMaintenanceWindow()).append(",");
if (getEnableIAMDatabaseAuthentication() != null)
sb.append("EnableIAMDatabaseAuthentication: ").append(getEnableIAMDatabaseAuthentication()).append(",");
if (getBacktrackWindow() != null)
sb.append("BacktrackWindow: ").append(getBacktrackWindow()).append(",");
if (getCloudwatchLogsExportConfiguration() != null)
sb.append("CloudwatchLogsExportConfiguration: ").append(getCloudwatchLogsExportConfiguration()).append(",");
if (getEngineVersion() != null)
sb.append("EngineVersion: ").append(getEngineVersion()).append(",");
if (getAllowMajorVersionUpgrade() != null)
sb.append("AllowMajorVersionUpgrade: ").append(getAllowMajorVersionUpgrade()).append(",");
if (getDBInstanceParameterGroupName() != null)
sb.append("DBInstanceParameterGroupName: ").append(getDBInstanceParameterGroupName()).append(",");
if (getDomain() != null)
sb.append("Domain: ").append(getDomain()).append(",");
if (getDomainIAMRoleName() != null)
sb.append("DomainIAMRoleName: ").append(getDomainIAMRoleName()).append(",");
if (getScalingConfiguration() != null)
sb.append("ScalingConfiguration: ").append(getScalingConfiguration()).append(",");
if (getDeletionProtection() != null)
sb.append("DeletionProtection: ").append(getDeletionProtection()).append(",");
if (getEnableHttpEndpoint() != null)
sb.append("EnableHttpEndpoint: ").append(getEnableHttpEndpoint()).append(",");
if (getCopyTagsToSnapshot() != null)
sb.append("CopyTagsToSnapshot: ").append(getCopyTagsToSnapshot()).append(",");
if (getEnableGlobalWriteForwarding() != null)
sb.append("EnableGlobalWriteForwarding: ").append(getEnableGlobalWriteForwarding()).append(",");
if (getDBClusterInstanceClass() != null)
sb.append("DBClusterInstanceClass: ").append(getDBClusterInstanceClass()).append(",");
if (getAllocatedStorage() != null)
sb.append("AllocatedStorage: ").append(getAllocatedStorage()).append(",");
if (getStorageType() != null)
sb.append("StorageType: ").append(getStorageType()).append(",");
if (getIops() != null)
sb.append("Iops: ").append(getIops()).append(",");
if (getAutoMinorVersionUpgrade() != null)
sb.append("AutoMinorVersionUpgrade: ").append(getAutoMinorVersionUpgrade()).append(",");
if (getMonitoringInterval() != null)
sb.append("MonitoringInterval: ").append(getMonitoringInterval()).append(",");
if (getMonitoringRoleArn() != null)
sb.append("MonitoringRoleArn: ").append(getMonitoringRoleArn()).append(",");
if (getEnablePerformanceInsights() != null)
sb.append("EnablePerformanceInsights: ").append(getEnablePerformanceInsights()).append(",");
if (getPerformanceInsightsKMSKeyId() != null)
sb.append("PerformanceInsightsKMSKeyId: ").append(getPerformanceInsightsKMSKeyId()).append(",");
if (getPerformanceInsightsRetentionPeriod() != null)
sb.append("PerformanceInsightsRetentionPeriod: ").append(getPerformanceInsightsRetentionPeriod()).append(",");
if (getServerlessV2ScalingConfiguration() != null)
sb.append("ServerlessV2ScalingConfiguration: ").append(getServerlessV2ScalingConfiguration()).append(",");
if (getNetworkType() != null)
sb.append("NetworkType: ").append(getNetworkType()).append(",");
if (getManageMasterUserPassword() != null)
sb.append("ManageMasterUserPassword: ").append(getManageMasterUserPassword()).append(",");
if (getRotateMasterUserPassword() != null)
sb.append("RotateMasterUserPassword: ").append(getRotateMasterUserPassword()).append(",");
if (getMasterUserSecretKmsKeyId() != null)
sb.append("MasterUserSecretKmsKeyId: ").append(getMasterUserSecretKmsKeyId()).append(",");
if (getEngineMode() != null)
sb.append("EngineMode: ").append(getEngineMode()).append(",");
if (getAllowEngineModeChange() != null)
sb.append("AllowEngineModeChange: ").append(getAllowEngineModeChange()).append(",");
if (getEnableLocalWriteForwarding() != null)
sb.append("EnableLocalWriteForwarding: ").append(getEnableLocalWriteForwarding()).append(",");
if (getAwsBackupRecoveryPointArn() != null)
sb.append("AwsBackupRecoveryPointArn: ").append(getAwsBackupRecoveryPointArn()).append(",");
if (getEnableLimitlessDatabase() != null)
sb.append("EnableLimitlessDatabase: ").append(getEnableLimitlessDatabase()).append(",");
if (getCACertificateIdentifier() != null)
sb.append("CACertificateIdentifier: ").append(getCACertificateIdentifier());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof ModifyDBClusterRequest == false)
return false;
ModifyDBClusterRequest other = (ModifyDBClusterRequest) obj;
if (other.getDBClusterIdentifier() == null ^ this.getDBClusterIdentifier() == null)
return false;
if (other.getDBClusterIdentifier() != null && other.getDBClusterIdentifier().equals(this.getDBClusterIdentifier()) == false)
return false;
if (other.getNewDBClusterIdentifier() == null ^ this.getNewDBClusterIdentifier() == null)
return false;
if (other.getNewDBClusterIdentifier() != null && other.getNewDBClusterIdentifier().equals(this.getNewDBClusterIdentifier()) == false)
return false;
if (other.getApplyImmediately() == null ^ this.getApplyImmediately() == null)
return false;
if (other.getApplyImmediately() != null && other.getApplyImmediately().equals(this.getApplyImmediately()) == false)
return false;
if (other.getBackupRetentionPeriod() == null ^ this.getBackupRetentionPeriod() == null)
return false;
if (other.getBackupRetentionPeriod() != null && other.getBackupRetentionPeriod().equals(this.getBackupRetentionPeriod()) == false)
return false;
if (other.getDBClusterParameterGroupName() == null ^ this.getDBClusterParameterGroupName() == null)
return false;
if (other.getDBClusterParameterGroupName() != null && other.getDBClusterParameterGroupName().equals(this.getDBClusterParameterGroupName()) == false)
return false;
if (other.getVpcSecurityGroupIds() == null ^ this.getVpcSecurityGroupIds() == null)
return false;
if (other.getVpcSecurityGroupIds() != null && other.getVpcSecurityGroupIds().equals(this.getVpcSecurityGroupIds()) == false)
return false;
if (other.getPort() == null ^ this.getPort() == null)
return false;
if (other.getPort() != null && other.getPort().equals(this.getPort()) == false)
return false;
if (other.getMasterUserPassword() == null ^ this.getMasterUserPassword() == null)
return false;
if (other.getMasterUserPassword() != null && other.getMasterUserPassword().equals(this.getMasterUserPassword()) == false)
return false;
if (other.getOptionGroupName() == null ^ this.getOptionGroupName() == null)
return false;
if (other.getOptionGroupName() != null && other.getOptionGroupName().equals(this.getOptionGroupName()) == false)
return false;
if (other.getPreferredBackupWindow() == null ^ this.getPreferredBackupWindow() == null)
return false;
if (other.getPreferredBackupWindow() != null && other.getPreferredBackupWindow().equals(this.getPreferredBackupWindow()) == false)
return false;
if (other.getPreferredMaintenanceWindow() == null ^ this.getPreferredMaintenanceWindow() == null)
return false;
if (other.getPreferredMaintenanceWindow() != null && other.getPreferredMaintenanceWindow().equals(this.getPreferredMaintenanceWindow()) == false)
return false;
if (other.getEnableIAMDatabaseAuthentication() == null ^ this.getEnableIAMDatabaseAuthentication() == null)
return false;
if (other.getEnableIAMDatabaseAuthentication() != null
&& other.getEnableIAMDatabaseAuthentication().equals(this.getEnableIAMDatabaseAuthentication()) == false)
return false;
if (other.getBacktrackWindow() == null ^ this.getBacktrackWindow() == null)
return false;
if (other.getBacktrackWindow() != null && other.getBacktrackWindow().equals(this.getBacktrackWindow()) == false)
return false;
if (other.getCloudwatchLogsExportConfiguration() == null ^ this.getCloudwatchLogsExportConfiguration() == null)
return false;
if (other.getCloudwatchLogsExportConfiguration() != null
&& other.getCloudwatchLogsExportConfiguration().equals(this.getCloudwatchLogsExportConfiguration()) == false)
return false;
if (other.getEngineVersion() == null ^ this.getEngineVersion() == null)
return false;
if (other.getEngineVersion() != null && other.getEngineVersion().equals(this.getEngineVersion()) == false)
return false;
if (other.getAllowMajorVersionUpgrade() == null ^ this.getAllowMajorVersionUpgrade() == null)
return false;
if (other.getAllowMajorVersionUpgrade() != null && other.getAllowMajorVersionUpgrade().equals(this.getAllowMajorVersionUpgrade()) == false)
return false;
if (other.getDBInstanceParameterGroupName() == null ^ this.getDBInstanceParameterGroupName() == null)
return false;
if (other.getDBInstanceParameterGroupName() != null && other.getDBInstanceParameterGroupName().equals(this.getDBInstanceParameterGroupName()) == false)
return false;
if (other.getDomain() == null ^ this.getDomain() == null)
return false;
if (other.getDomain() != null && other.getDomain().equals(this.getDomain()) == false)
return false;
if (other.getDomainIAMRoleName() == null ^ this.getDomainIAMRoleName() == null)
return false;
if (other.getDomainIAMRoleName() != null && other.getDomainIAMRoleName().equals(this.getDomainIAMRoleName()) == false)
return false;
if (other.getScalingConfiguration() == null ^ this.getScalingConfiguration() == null)
return false;
if (other.getScalingConfiguration() != null && other.getScalingConfiguration().equals(this.getScalingConfiguration()) == false)
return false;
if (other.getDeletionProtection() == null ^ this.getDeletionProtection() == null)
return false;
if (other.getDeletionProtection() != null && other.getDeletionProtection().equals(this.getDeletionProtection()) == false)
return false;
if (other.getEnableHttpEndpoint() == null ^ this.getEnableHttpEndpoint() == null)
return false;
if (other.getEnableHttpEndpoint() != null && other.getEnableHttpEndpoint().equals(this.getEnableHttpEndpoint()) == false)
return false;
if (other.getCopyTagsToSnapshot() == null ^ this.getCopyTagsToSnapshot() == null)
return false;
if (other.getCopyTagsToSnapshot() != null && other.getCopyTagsToSnapshot().equals(this.getCopyTagsToSnapshot()) == false)
return false;
if (other.getEnableGlobalWriteForwarding() == null ^ this.getEnableGlobalWriteForwarding() == null)
return false;
if (other.getEnableGlobalWriteForwarding() != null && other.getEnableGlobalWriteForwarding().equals(this.getEnableGlobalWriteForwarding()) == false)
return false;
if (other.getDBClusterInstanceClass() == null ^ this.getDBClusterInstanceClass() == null)
return false;
if (other.getDBClusterInstanceClass() != null && other.getDBClusterInstanceClass().equals(this.getDBClusterInstanceClass()) == false)
return false;
if (other.getAllocatedStorage() == null ^ this.getAllocatedStorage() == null)
return false;
if (other.getAllocatedStorage() != null && other.getAllocatedStorage().equals(this.getAllocatedStorage()) == false)
return false;
if (other.getStorageType() == null ^ this.getStorageType() == null)
return false;
if (other.getStorageType() != null && other.getStorageType().equals(this.getStorageType()) == false)
return false;
if (other.getIops() == null ^ this.getIops() == null)
return false;
if (other.getIops() != null && other.getIops().equals(this.getIops()) == false)
return false;
if (other.getAutoMinorVersionUpgrade() == null ^ this.getAutoMinorVersionUpgrade() == null)
return false;
if (other.getAutoMinorVersionUpgrade() != null && other.getAutoMinorVersionUpgrade().equals(this.getAutoMinorVersionUpgrade()) == false)
return false;
if (other.getMonitoringInterval() == null ^ this.getMonitoringInterval() == null)
return false;
if (other.getMonitoringInterval() != null && other.getMonitoringInterval().equals(this.getMonitoringInterval()) == false)
return false;
if (other.getMonitoringRoleArn() == null ^ this.getMonitoringRoleArn() == null)
return false;
if (other.getMonitoringRoleArn() != null && other.getMonitoringRoleArn().equals(this.getMonitoringRoleArn()) == false)
return false;
if (other.getEnablePerformanceInsights() == null ^ this.getEnablePerformanceInsights() == null)
return false;
if (other.getEnablePerformanceInsights() != null && other.getEnablePerformanceInsights().equals(this.getEnablePerformanceInsights()) == false)
return false;
if (other.getPerformanceInsightsKMSKeyId() == null ^ this.getPerformanceInsightsKMSKeyId() == null)
return false;
if (other.getPerformanceInsightsKMSKeyId() != null && other.getPerformanceInsightsKMSKeyId().equals(this.getPerformanceInsightsKMSKeyId()) == false)
return false;
if (other.getPerformanceInsightsRetentionPeriod() == null ^ this.getPerformanceInsightsRetentionPeriod() == null)
return false;
if (other.getPerformanceInsightsRetentionPeriod() != null
&& other.getPerformanceInsightsRetentionPeriod().equals(this.getPerformanceInsightsRetentionPeriod()) == false)
return false;
if (other.getServerlessV2ScalingConfiguration() == null ^ this.getServerlessV2ScalingConfiguration() == null)
return false;
if (other.getServerlessV2ScalingConfiguration() != null
&& other.getServerlessV2ScalingConfiguration().equals(this.getServerlessV2ScalingConfiguration()) == false)
return false;
if (other.getNetworkType() == null ^ this.getNetworkType() == null)
return false;
if (other.getNetworkType() != null && other.getNetworkType().equals(this.getNetworkType()) == false)
return false;
if (other.getManageMasterUserPassword() == null ^ this.getManageMasterUserPassword() == null)
return false;
if (other.getManageMasterUserPassword() != null && other.getManageMasterUserPassword().equals(this.getManageMasterUserPassword()) == false)
return false;
if (other.getRotateMasterUserPassword() == null ^ this.getRotateMasterUserPassword() == null)
return false;
if (other.getRotateMasterUserPassword() != null && other.getRotateMasterUserPassword().equals(this.getRotateMasterUserPassword()) == false)
return false;
if (other.getMasterUserSecretKmsKeyId() == null ^ this.getMasterUserSecretKmsKeyId() == null)
return false;
if (other.getMasterUserSecretKmsKeyId() != null && other.getMasterUserSecretKmsKeyId().equals(this.getMasterUserSecretKmsKeyId()) == false)
return false;
if (other.getEngineMode() == null ^ this.getEngineMode() == null)
return false;
if (other.getEngineMode() != null && other.getEngineMode().equals(this.getEngineMode()) == false)
return false;
if (other.getAllowEngineModeChange() == null ^ this.getAllowEngineModeChange() == null)
return false;
if (other.getAllowEngineModeChange() != null && other.getAllowEngineModeChange().equals(this.getAllowEngineModeChange()) == false)
return false;
if (other.getEnableLocalWriteForwarding() == null ^ this.getEnableLocalWriteForwarding() == null)
return false;
if (other.getEnableLocalWriteForwarding() != null && other.getEnableLocalWriteForwarding().equals(this.getEnableLocalWriteForwarding()) == false)
return false;
if (other.getAwsBackupRecoveryPointArn() == null ^ this.getAwsBackupRecoveryPointArn() == null)
return false;
if (other.getAwsBackupRecoveryPointArn() != null && other.getAwsBackupRecoveryPointArn().equals(this.getAwsBackupRecoveryPointArn()) == false)
return false;
if (other.getEnableLimitlessDatabase() == null ^ this.getEnableLimitlessDatabase() == null)
return false;
if (other.getEnableLimitlessDatabase() != null && other.getEnableLimitlessDatabase().equals(this.getEnableLimitlessDatabase()) == false)
return false;
if (other.getCACertificateIdentifier() == null ^ this.getCACertificateIdentifier() == null)
return false;
if (other.getCACertificateIdentifier() != null && other.getCACertificateIdentifier().equals(this.getCACertificateIdentifier()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getDBClusterIdentifier() == null) ? 0 : getDBClusterIdentifier().hashCode());
hashCode = prime * hashCode + ((getNewDBClusterIdentifier() == null) ? 0 : getNewDBClusterIdentifier().hashCode());
hashCode = prime * hashCode + ((getApplyImmediately() == null) ? 0 : getApplyImmediately().hashCode());
hashCode = prime * hashCode + ((getBackupRetentionPeriod() == null) ? 0 : getBackupRetentionPeriod().hashCode());
hashCode = prime * hashCode + ((getDBClusterParameterGroupName() == null) ? 0 : getDBClusterParameterGroupName().hashCode());
hashCode = prime * hashCode + ((getVpcSecurityGroupIds() == null) ? 0 : getVpcSecurityGroupIds().hashCode());
hashCode = prime * hashCode + ((getPort() == null) ? 0 : getPort().hashCode());
hashCode = prime * hashCode + ((getMasterUserPassword() == null) ? 0 : getMasterUserPassword().hashCode());
hashCode = prime * hashCode + ((getOptionGroupName() == null) ? 0 : getOptionGroupName().hashCode());
hashCode = prime * hashCode + ((getPreferredBackupWindow() == null) ? 0 : getPreferredBackupWindow().hashCode());
hashCode = prime * hashCode + ((getPreferredMaintenanceWindow() == null) ? 0 : getPreferredMaintenanceWindow().hashCode());
hashCode = prime * hashCode + ((getEnableIAMDatabaseAuthentication() == null) ? 0 : getEnableIAMDatabaseAuthentication().hashCode());
hashCode = prime * hashCode + ((getBacktrackWindow() == null) ? 0 : getBacktrackWindow().hashCode());
hashCode = prime * hashCode + ((getCloudwatchLogsExportConfiguration() == null) ? 0 : getCloudwatchLogsExportConfiguration().hashCode());
hashCode = prime * hashCode + ((getEngineVersion() == null) ? 0 : getEngineVersion().hashCode());
hashCode = prime * hashCode + ((getAllowMajorVersionUpgrade() == null) ? 0 : getAllowMajorVersionUpgrade().hashCode());
hashCode = prime * hashCode + ((getDBInstanceParameterGroupName() == null) ? 0 : getDBInstanceParameterGroupName().hashCode());
hashCode = prime * hashCode + ((getDomain() == null) ? 0 : getDomain().hashCode());
hashCode = prime * hashCode + ((getDomainIAMRoleName() == null) ? 0 : getDomainIAMRoleName().hashCode());
hashCode = prime * hashCode + ((getScalingConfiguration() == null) ? 0 : getScalingConfiguration().hashCode());
hashCode = prime * hashCode + ((getDeletionProtection() == null) ? 0 : getDeletionProtection().hashCode());
hashCode = prime * hashCode + ((getEnableHttpEndpoint() == null) ? 0 : getEnableHttpEndpoint().hashCode());
hashCode = prime * hashCode + ((getCopyTagsToSnapshot() == null) ? 0 : getCopyTagsToSnapshot().hashCode());
hashCode = prime * hashCode + ((getEnableGlobalWriteForwarding() == null) ? 0 : getEnableGlobalWriteForwarding().hashCode());
hashCode = prime * hashCode + ((getDBClusterInstanceClass() == null) ? 0 : getDBClusterInstanceClass().hashCode());
hashCode = prime * hashCode + ((getAllocatedStorage() == null) ? 0 : getAllocatedStorage().hashCode());
hashCode = prime * hashCode + ((getStorageType() == null) ? 0 : getStorageType().hashCode());
hashCode = prime * hashCode + ((getIops() == null) ? 0 : getIops().hashCode());
hashCode = prime * hashCode + ((getAutoMinorVersionUpgrade() == null) ? 0 : getAutoMinorVersionUpgrade().hashCode());
hashCode = prime * hashCode + ((getMonitoringInterval() == null) ? 0 : getMonitoringInterval().hashCode());
hashCode = prime * hashCode + ((getMonitoringRoleArn() == null) ? 0 : getMonitoringRoleArn().hashCode());
hashCode = prime * hashCode + ((getEnablePerformanceInsights() == null) ? 0 : getEnablePerformanceInsights().hashCode());
hashCode = prime * hashCode + ((getPerformanceInsightsKMSKeyId() == null) ? 0 : getPerformanceInsightsKMSKeyId().hashCode());
hashCode = prime * hashCode + ((getPerformanceInsightsRetentionPeriod() == null) ? 0 : getPerformanceInsightsRetentionPeriod().hashCode());
hashCode = prime * hashCode + ((getServerlessV2ScalingConfiguration() == null) ? 0 : getServerlessV2ScalingConfiguration().hashCode());
hashCode = prime * hashCode + ((getNetworkType() == null) ? 0 : getNetworkType().hashCode());
hashCode = prime * hashCode + ((getManageMasterUserPassword() == null) ? 0 : getManageMasterUserPassword().hashCode());
hashCode = prime * hashCode + ((getRotateMasterUserPassword() == null) ? 0 : getRotateMasterUserPassword().hashCode());
hashCode = prime * hashCode + ((getMasterUserSecretKmsKeyId() == null) ? 0 : getMasterUserSecretKmsKeyId().hashCode());
hashCode = prime * hashCode + ((getEngineMode() == null) ? 0 : getEngineMode().hashCode());
hashCode = prime * hashCode + ((getAllowEngineModeChange() == null) ? 0 : getAllowEngineModeChange().hashCode());
hashCode = prime * hashCode + ((getEnableLocalWriteForwarding() == null) ? 0 : getEnableLocalWriteForwarding().hashCode());
hashCode = prime * hashCode + ((getAwsBackupRecoveryPointArn() == null) ? 0 : getAwsBackupRecoveryPointArn().hashCode());
hashCode = prime * hashCode + ((getEnableLimitlessDatabase() == null) ? 0 : getEnableLimitlessDatabase().hashCode());
hashCode = prime * hashCode + ((getCACertificateIdentifier() == null) ? 0 : getCACertificateIdentifier().hashCode());
return hashCode;
}
@Override
public ModifyDBClusterRequest clone() {
return (ModifyDBClusterRequest) super.clone();
}
}