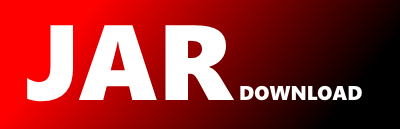
com.amazonaws.services.rds.model.StartExportTaskResult Maven / Gradle / Ivy
Show all versions of aws-java-sdk-rds Show documentation
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.rds.model;
import java.io.Serializable;
import javax.annotation.Generated;
/**
*
* Contains the details of a snapshot or cluster export to Amazon S3.
*
*
* This data type is used as a response element in the DescribeExportTasks
operation.
*
*
* @see AWS API
* Documentation
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class StartExportTaskResult extends com.amazonaws.AmazonWebServiceResult implements Serializable, Cloneable {
/**
*
* A unique identifier for the snapshot or cluster export task. This ID isn't an identifier for the Amazon S3 bucket
* where the data is exported.
*
*/
private String exportTaskIdentifier;
/**
*
* The Amazon Resource Name (ARN) of the snapshot or cluster exported to Amazon S3.
*
*/
private String sourceArn;
/**
*
* The data exported from the snapshot or cluster.
*
*
* Valid Values:
*
*
* -
*
* database
- Export all the data from a specified database.
*
*
* -
*
* database.table
table-name - Export a table of the snapshot or cluster. This format is valid
* only for RDS for MySQL, RDS for MariaDB, and Aurora MySQL.
*
*
* -
*
* database.schema
schema-name - Export a database schema of the snapshot or cluster. This
* format is valid only for RDS for PostgreSQL and Aurora PostgreSQL.
*
*
* -
*
* database.schema.table
table-name - Export a table of the database schema. This format is
* valid only for RDS for PostgreSQL and Aurora PostgreSQL.
*
*
*
*/
private com.amazonaws.internal.SdkInternalList exportOnly;
/**
*
* The time when the snapshot was created.
*
*/
private java.util.Date snapshotTime;
/**
*
* The time when the snapshot or cluster export task started.
*
*/
private java.util.Date taskStartTime;
/**
*
* The time when the snapshot or cluster export task ended.
*
*/
private java.util.Date taskEndTime;
/**
*
* The Amazon S3 bucket where the snapshot or cluster is exported to.
*
*/
private String s3Bucket;
/**
*
* The Amazon S3 bucket prefix that is the file name and path of the exported data.
*
*/
private String s3Prefix;
/**
*
* The name of the IAM role that is used to write to Amazon S3 when exporting a snapshot or cluster.
*
*/
private String iamRoleArn;
/**
*
* The key identifier of the Amazon Web Services KMS key that is used to encrypt the data when it's exported to
* Amazon S3. The KMS key identifier is its key ARN, key ID, alias ARN, or alias name. The IAM role used for the
* export must have encryption and decryption permissions to use this KMS key.
*
*/
private String kmsKeyId;
/**
*
* The progress status of the export task. The status can be one of the following:
*
*
* -
*
* CANCELED
*
*
* -
*
* CANCELING
*
*
* -
*
* COMPLETE
*
*
* -
*
* FAILED
*
*
* -
*
* IN_PROGRESS
*
*
* -
*
* STARTING
*
*
*
*/
private String status;
/**
*
* The progress of the snapshot or cluster export task as a percentage.
*
*/
private Integer percentProgress;
/**
*
* The total amount of data exported, in gigabytes.
*
*/
private Integer totalExtractedDataInGB;
/**
*
* The reason the export failed, if it failed.
*
*/
private String failureCause;
/**
*
* A warning about the snapshot or cluster export task.
*
*/
private String warningMessage;
/**
*
* The type of source for the export.
*
*/
private String sourceType;
/**
*
* A unique identifier for the snapshot or cluster export task. This ID isn't an identifier for the Amazon S3 bucket
* where the data is exported.
*
*
* @param exportTaskIdentifier
* A unique identifier for the snapshot or cluster export task. This ID isn't an identifier for the Amazon S3
* bucket where the data is exported.
*/
public void setExportTaskIdentifier(String exportTaskIdentifier) {
this.exportTaskIdentifier = exportTaskIdentifier;
}
/**
*
* A unique identifier for the snapshot or cluster export task. This ID isn't an identifier for the Amazon S3 bucket
* where the data is exported.
*
*
* @return A unique identifier for the snapshot or cluster export task. This ID isn't an identifier for the Amazon
* S3 bucket where the data is exported.
*/
public String getExportTaskIdentifier() {
return this.exportTaskIdentifier;
}
/**
*
* A unique identifier for the snapshot or cluster export task. This ID isn't an identifier for the Amazon S3 bucket
* where the data is exported.
*
*
* @param exportTaskIdentifier
* A unique identifier for the snapshot or cluster export task. This ID isn't an identifier for the Amazon S3
* bucket where the data is exported.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public StartExportTaskResult withExportTaskIdentifier(String exportTaskIdentifier) {
setExportTaskIdentifier(exportTaskIdentifier);
return this;
}
/**
*
* The Amazon Resource Name (ARN) of the snapshot or cluster exported to Amazon S3.
*
*
* @param sourceArn
* The Amazon Resource Name (ARN) of the snapshot or cluster exported to Amazon S3.
*/
public void setSourceArn(String sourceArn) {
this.sourceArn = sourceArn;
}
/**
*
* The Amazon Resource Name (ARN) of the snapshot or cluster exported to Amazon S3.
*
*
* @return The Amazon Resource Name (ARN) of the snapshot or cluster exported to Amazon S3.
*/
public String getSourceArn() {
return this.sourceArn;
}
/**
*
* The Amazon Resource Name (ARN) of the snapshot or cluster exported to Amazon S3.
*
*
* @param sourceArn
* The Amazon Resource Name (ARN) of the snapshot or cluster exported to Amazon S3.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public StartExportTaskResult withSourceArn(String sourceArn) {
setSourceArn(sourceArn);
return this;
}
/**
*
* The data exported from the snapshot or cluster.
*
*
* Valid Values:
*
*
* -
*
* database
- Export all the data from a specified database.
*
*
* -
*
* database.table
table-name - Export a table of the snapshot or cluster. This format is valid
* only for RDS for MySQL, RDS for MariaDB, and Aurora MySQL.
*
*
* -
*
* database.schema
schema-name - Export a database schema of the snapshot or cluster. This
* format is valid only for RDS for PostgreSQL and Aurora PostgreSQL.
*
*
* -
*
* database.schema.table
table-name - Export a table of the database schema. This format is
* valid only for RDS for PostgreSQL and Aurora PostgreSQL.
*
*
*
*
* @return The data exported from the snapshot or cluster.
*
* Valid Values:
*
*
* -
*
* database
- Export all the data from a specified database.
*
*
* -
*
* database.table
table-name - Export a table of the snapshot or cluster. This format is
* valid only for RDS for MySQL, RDS for MariaDB, and Aurora MySQL.
*
*
* -
*
* database.schema
schema-name - Export a database schema of the snapshot or cluster.
* This format is valid only for RDS for PostgreSQL and Aurora PostgreSQL.
*
*
* -
*
* database.schema.table
table-name - Export a table of the database schema. This format
* is valid only for RDS for PostgreSQL and Aurora PostgreSQL.
*
*
*/
public java.util.List getExportOnly() {
if (exportOnly == null) {
exportOnly = new com.amazonaws.internal.SdkInternalList();
}
return exportOnly;
}
/**
*
* The data exported from the snapshot or cluster.
*
*
* Valid Values:
*
*
* -
*
* database
- Export all the data from a specified database.
*
*
* -
*
* database.table
table-name - Export a table of the snapshot or cluster. This format is valid
* only for RDS for MySQL, RDS for MariaDB, and Aurora MySQL.
*
*
* -
*
* database.schema
schema-name - Export a database schema of the snapshot or cluster. This
* format is valid only for RDS for PostgreSQL and Aurora PostgreSQL.
*
*
* -
*
* database.schema.table
table-name - Export a table of the database schema. This format is
* valid only for RDS for PostgreSQL and Aurora PostgreSQL.
*
*
*
*
* @param exportOnly
* The data exported from the snapshot or cluster.
*
* Valid Values:
*
*
* -
*
* database
- Export all the data from a specified database.
*
*
* -
*
* database.table
table-name - Export a table of the snapshot or cluster. This format is
* valid only for RDS for MySQL, RDS for MariaDB, and Aurora MySQL.
*
*
* -
*
* database.schema
schema-name - Export a database schema of the snapshot or cluster.
* This format is valid only for RDS for PostgreSQL and Aurora PostgreSQL.
*
*
* -
*
* database.schema.table
table-name - Export a table of the database schema. This format
* is valid only for RDS for PostgreSQL and Aurora PostgreSQL.
*
*
*/
public void setExportOnly(java.util.Collection exportOnly) {
if (exportOnly == null) {
this.exportOnly = null;
return;
}
this.exportOnly = new com.amazonaws.internal.SdkInternalList(exportOnly);
}
/**
*
* The data exported from the snapshot or cluster.
*
*
* Valid Values:
*
*
* -
*
* database
- Export all the data from a specified database.
*
*
* -
*
* database.table
table-name - Export a table of the snapshot or cluster. This format is valid
* only for RDS for MySQL, RDS for MariaDB, and Aurora MySQL.
*
*
* -
*
* database.schema
schema-name - Export a database schema of the snapshot or cluster. This
* format is valid only for RDS for PostgreSQL and Aurora PostgreSQL.
*
*
* -
*
* database.schema.table
table-name - Export a table of the database schema. This format is
* valid only for RDS for PostgreSQL and Aurora PostgreSQL.
*
*
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setExportOnly(java.util.Collection)} or {@link #withExportOnly(java.util.Collection)} if you want to
* override the existing values.
*
*
* @param exportOnly
* The data exported from the snapshot or cluster.
*
* Valid Values:
*
*
* -
*
* database
- Export all the data from a specified database.
*
*
* -
*
* database.table
table-name - Export a table of the snapshot or cluster. This format is
* valid only for RDS for MySQL, RDS for MariaDB, and Aurora MySQL.
*
*
* -
*
* database.schema
schema-name - Export a database schema of the snapshot or cluster.
* This format is valid only for RDS for PostgreSQL and Aurora PostgreSQL.
*
*
* -
*
* database.schema.table
table-name - Export a table of the database schema. This format
* is valid only for RDS for PostgreSQL and Aurora PostgreSQL.
*
*
* @return Returns a reference to this object so that method calls can be chained together.
*/
public StartExportTaskResult withExportOnly(String... exportOnly) {
if (this.exportOnly == null) {
setExportOnly(new com.amazonaws.internal.SdkInternalList(exportOnly.length));
}
for (String ele : exportOnly) {
this.exportOnly.add(ele);
}
return this;
}
/**
*
* The data exported from the snapshot or cluster.
*
*
* Valid Values:
*
*
* -
*
* database
- Export all the data from a specified database.
*
*
* -
*
* database.table
table-name - Export a table of the snapshot or cluster. This format is valid
* only for RDS for MySQL, RDS for MariaDB, and Aurora MySQL.
*
*
* -
*
* database.schema
schema-name - Export a database schema of the snapshot or cluster. This
* format is valid only for RDS for PostgreSQL and Aurora PostgreSQL.
*
*
* -
*
* database.schema.table
table-name - Export a table of the database schema. This format is
* valid only for RDS for PostgreSQL and Aurora PostgreSQL.
*
*
*
*
* @param exportOnly
* The data exported from the snapshot or cluster.
*
* Valid Values:
*
*
* -
*
* database
- Export all the data from a specified database.
*
*
* -
*
* database.table
table-name - Export a table of the snapshot or cluster. This format is
* valid only for RDS for MySQL, RDS for MariaDB, and Aurora MySQL.
*
*
* -
*
* database.schema
schema-name - Export a database schema of the snapshot or cluster.
* This format is valid only for RDS for PostgreSQL and Aurora PostgreSQL.
*
*
* -
*
* database.schema.table
table-name - Export a table of the database schema. This format
* is valid only for RDS for PostgreSQL and Aurora PostgreSQL.
*
*
* @return Returns a reference to this object so that method calls can be chained together.
*/
public StartExportTaskResult withExportOnly(java.util.Collection exportOnly) {
setExportOnly(exportOnly);
return this;
}
/**
*
* The time when the snapshot was created.
*
*
* @param snapshotTime
* The time when the snapshot was created.
*/
public void setSnapshotTime(java.util.Date snapshotTime) {
this.snapshotTime = snapshotTime;
}
/**
*
* The time when the snapshot was created.
*
*
* @return The time when the snapshot was created.
*/
public java.util.Date getSnapshotTime() {
return this.snapshotTime;
}
/**
*
* The time when the snapshot was created.
*
*
* @param snapshotTime
* The time when the snapshot was created.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public StartExportTaskResult withSnapshotTime(java.util.Date snapshotTime) {
setSnapshotTime(snapshotTime);
return this;
}
/**
*
* The time when the snapshot or cluster export task started.
*
*
* @param taskStartTime
* The time when the snapshot or cluster export task started.
*/
public void setTaskStartTime(java.util.Date taskStartTime) {
this.taskStartTime = taskStartTime;
}
/**
*
* The time when the snapshot or cluster export task started.
*
*
* @return The time when the snapshot or cluster export task started.
*/
public java.util.Date getTaskStartTime() {
return this.taskStartTime;
}
/**
*
* The time when the snapshot or cluster export task started.
*
*
* @param taskStartTime
* The time when the snapshot or cluster export task started.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public StartExportTaskResult withTaskStartTime(java.util.Date taskStartTime) {
setTaskStartTime(taskStartTime);
return this;
}
/**
*
* The time when the snapshot or cluster export task ended.
*
*
* @param taskEndTime
* The time when the snapshot or cluster export task ended.
*/
public void setTaskEndTime(java.util.Date taskEndTime) {
this.taskEndTime = taskEndTime;
}
/**
*
* The time when the snapshot or cluster export task ended.
*
*
* @return The time when the snapshot or cluster export task ended.
*/
public java.util.Date getTaskEndTime() {
return this.taskEndTime;
}
/**
*
* The time when the snapshot or cluster export task ended.
*
*
* @param taskEndTime
* The time when the snapshot or cluster export task ended.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public StartExportTaskResult withTaskEndTime(java.util.Date taskEndTime) {
setTaskEndTime(taskEndTime);
return this;
}
/**
*
* The Amazon S3 bucket where the snapshot or cluster is exported to.
*
*
* @param s3Bucket
* The Amazon S3 bucket where the snapshot or cluster is exported to.
*/
public void setS3Bucket(String s3Bucket) {
this.s3Bucket = s3Bucket;
}
/**
*
* The Amazon S3 bucket where the snapshot or cluster is exported to.
*
*
* @return The Amazon S3 bucket where the snapshot or cluster is exported to.
*/
public String getS3Bucket() {
return this.s3Bucket;
}
/**
*
* The Amazon S3 bucket where the snapshot or cluster is exported to.
*
*
* @param s3Bucket
* The Amazon S3 bucket where the snapshot or cluster is exported to.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public StartExportTaskResult withS3Bucket(String s3Bucket) {
setS3Bucket(s3Bucket);
return this;
}
/**
*
* The Amazon S3 bucket prefix that is the file name and path of the exported data.
*
*
* @param s3Prefix
* The Amazon S3 bucket prefix that is the file name and path of the exported data.
*/
public void setS3Prefix(String s3Prefix) {
this.s3Prefix = s3Prefix;
}
/**
*
* The Amazon S3 bucket prefix that is the file name and path of the exported data.
*
*
* @return The Amazon S3 bucket prefix that is the file name and path of the exported data.
*/
public String getS3Prefix() {
return this.s3Prefix;
}
/**
*
* The Amazon S3 bucket prefix that is the file name and path of the exported data.
*
*
* @param s3Prefix
* The Amazon S3 bucket prefix that is the file name and path of the exported data.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public StartExportTaskResult withS3Prefix(String s3Prefix) {
setS3Prefix(s3Prefix);
return this;
}
/**
*
* The name of the IAM role that is used to write to Amazon S3 when exporting a snapshot or cluster.
*
*
* @param iamRoleArn
* The name of the IAM role that is used to write to Amazon S3 when exporting a snapshot or cluster.
*/
public void setIamRoleArn(String iamRoleArn) {
this.iamRoleArn = iamRoleArn;
}
/**
*
* The name of the IAM role that is used to write to Amazon S3 when exporting a snapshot or cluster.
*
*
* @return The name of the IAM role that is used to write to Amazon S3 when exporting a snapshot or cluster.
*/
public String getIamRoleArn() {
return this.iamRoleArn;
}
/**
*
* The name of the IAM role that is used to write to Amazon S3 when exporting a snapshot or cluster.
*
*
* @param iamRoleArn
* The name of the IAM role that is used to write to Amazon S3 when exporting a snapshot or cluster.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public StartExportTaskResult withIamRoleArn(String iamRoleArn) {
setIamRoleArn(iamRoleArn);
return this;
}
/**
*
* The key identifier of the Amazon Web Services KMS key that is used to encrypt the data when it's exported to
* Amazon S3. The KMS key identifier is its key ARN, key ID, alias ARN, or alias name. The IAM role used for the
* export must have encryption and decryption permissions to use this KMS key.
*
*
* @param kmsKeyId
* The key identifier of the Amazon Web Services KMS key that is used to encrypt the data when it's exported
* to Amazon S3. The KMS key identifier is its key ARN, key ID, alias ARN, or alias name. The IAM role used
* for the export must have encryption and decryption permissions to use this KMS key.
*/
public void setKmsKeyId(String kmsKeyId) {
this.kmsKeyId = kmsKeyId;
}
/**
*
* The key identifier of the Amazon Web Services KMS key that is used to encrypt the data when it's exported to
* Amazon S3. The KMS key identifier is its key ARN, key ID, alias ARN, or alias name. The IAM role used for the
* export must have encryption and decryption permissions to use this KMS key.
*
*
* @return The key identifier of the Amazon Web Services KMS key that is used to encrypt the data when it's exported
* to Amazon S3. The KMS key identifier is its key ARN, key ID, alias ARN, or alias name. The IAM role used
* for the export must have encryption and decryption permissions to use this KMS key.
*/
public String getKmsKeyId() {
return this.kmsKeyId;
}
/**
*
* The key identifier of the Amazon Web Services KMS key that is used to encrypt the data when it's exported to
* Amazon S3. The KMS key identifier is its key ARN, key ID, alias ARN, or alias name. The IAM role used for the
* export must have encryption and decryption permissions to use this KMS key.
*
*
* @param kmsKeyId
* The key identifier of the Amazon Web Services KMS key that is used to encrypt the data when it's exported
* to Amazon S3. The KMS key identifier is its key ARN, key ID, alias ARN, or alias name. The IAM role used
* for the export must have encryption and decryption permissions to use this KMS key.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public StartExportTaskResult withKmsKeyId(String kmsKeyId) {
setKmsKeyId(kmsKeyId);
return this;
}
/**
*
* The progress status of the export task. The status can be one of the following:
*
*
* -
*
* CANCELED
*
*
* -
*
* CANCELING
*
*
* -
*
* COMPLETE
*
*
* -
*
* FAILED
*
*
* -
*
* IN_PROGRESS
*
*
* -
*
* STARTING
*
*
*
*
* @param status
* The progress status of the export task. The status can be one of the following:
*
* -
*
* CANCELED
*
*
* -
*
* CANCELING
*
*
* -
*
* COMPLETE
*
*
* -
*
* FAILED
*
*
* -
*
* IN_PROGRESS
*
*
* -
*
* STARTING
*
*
*/
public void setStatus(String status) {
this.status = status;
}
/**
*
* The progress status of the export task. The status can be one of the following:
*
*
* -
*
* CANCELED
*
*
* -
*
* CANCELING
*
*
* -
*
* COMPLETE
*
*
* -
*
* FAILED
*
*
* -
*
* IN_PROGRESS
*
*
* -
*
* STARTING
*
*
*
*
* @return The progress status of the export task. The status can be one of the following:
*
* -
*
* CANCELED
*
*
* -
*
* CANCELING
*
*
* -
*
* COMPLETE
*
*
* -
*
* FAILED
*
*
* -
*
* IN_PROGRESS
*
*
* -
*
* STARTING
*
*
*/
public String getStatus() {
return this.status;
}
/**
*
* The progress status of the export task. The status can be one of the following:
*
*
* -
*
* CANCELED
*
*
* -
*
* CANCELING
*
*
* -
*
* COMPLETE
*
*
* -
*
* FAILED
*
*
* -
*
* IN_PROGRESS
*
*
* -
*
* STARTING
*
*
*
*
* @param status
* The progress status of the export task. The status can be one of the following:
*
* -
*
* CANCELED
*
*
* -
*
* CANCELING
*
*
* -
*
* COMPLETE
*
*
* -
*
* FAILED
*
*
* -
*
* IN_PROGRESS
*
*
* -
*
* STARTING
*
*
* @return Returns a reference to this object so that method calls can be chained together.
*/
public StartExportTaskResult withStatus(String status) {
setStatus(status);
return this;
}
/**
*
* The progress of the snapshot or cluster export task as a percentage.
*
*
* @param percentProgress
* The progress of the snapshot or cluster export task as a percentage.
*/
public void setPercentProgress(Integer percentProgress) {
this.percentProgress = percentProgress;
}
/**
*
* The progress of the snapshot or cluster export task as a percentage.
*
*
* @return The progress of the snapshot or cluster export task as a percentage.
*/
public Integer getPercentProgress() {
return this.percentProgress;
}
/**
*
* The progress of the snapshot or cluster export task as a percentage.
*
*
* @param percentProgress
* The progress of the snapshot or cluster export task as a percentage.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public StartExportTaskResult withPercentProgress(Integer percentProgress) {
setPercentProgress(percentProgress);
return this;
}
/**
*
* The total amount of data exported, in gigabytes.
*
*
* @param totalExtractedDataInGB
* The total amount of data exported, in gigabytes.
*/
public void setTotalExtractedDataInGB(Integer totalExtractedDataInGB) {
this.totalExtractedDataInGB = totalExtractedDataInGB;
}
/**
*
* The total amount of data exported, in gigabytes.
*
*
* @return The total amount of data exported, in gigabytes.
*/
public Integer getTotalExtractedDataInGB() {
return this.totalExtractedDataInGB;
}
/**
*
* The total amount of data exported, in gigabytes.
*
*
* @param totalExtractedDataInGB
* The total amount of data exported, in gigabytes.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public StartExportTaskResult withTotalExtractedDataInGB(Integer totalExtractedDataInGB) {
setTotalExtractedDataInGB(totalExtractedDataInGB);
return this;
}
/**
*
* The reason the export failed, if it failed.
*
*
* @param failureCause
* The reason the export failed, if it failed.
*/
public void setFailureCause(String failureCause) {
this.failureCause = failureCause;
}
/**
*
* The reason the export failed, if it failed.
*
*
* @return The reason the export failed, if it failed.
*/
public String getFailureCause() {
return this.failureCause;
}
/**
*
* The reason the export failed, if it failed.
*
*
* @param failureCause
* The reason the export failed, if it failed.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public StartExportTaskResult withFailureCause(String failureCause) {
setFailureCause(failureCause);
return this;
}
/**
*
* A warning about the snapshot or cluster export task.
*
*
* @param warningMessage
* A warning about the snapshot or cluster export task.
*/
public void setWarningMessage(String warningMessage) {
this.warningMessage = warningMessage;
}
/**
*
* A warning about the snapshot or cluster export task.
*
*
* @return A warning about the snapshot or cluster export task.
*/
public String getWarningMessage() {
return this.warningMessage;
}
/**
*
* A warning about the snapshot or cluster export task.
*
*
* @param warningMessage
* A warning about the snapshot or cluster export task.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public StartExportTaskResult withWarningMessage(String warningMessage) {
setWarningMessage(warningMessage);
return this;
}
/**
*
* The type of source for the export.
*
*
* @param sourceType
* The type of source for the export.
* @see ExportSourceType
*/
public void setSourceType(String sourceType) {
this.sourceType = sourceType;
}
/**
*
* The type of source for the export.
*
*
* @return The type of source for the export.
* @see ExportSourceType
*/
public String getSourceType() {
return this.sourceType;
}
/**
*
* The type of source for the export.
*
*
* @param sourceType
* The type of source for the export.
* @return Returns a reference to this object so that method calls can be chained together.
* @see ExportSourceType
*/
public StartExportTaskResult withSourceType(String sourceType) {
setSourceType(sourceType);
return this;
}
/**
*
* The type of source for the export.
*
*
* @param sourceType
* The type of source for the export.
* @return Returns a reference to this object so that method calls can be chained together.
* @see ExportSourceType
*/
public StartExportTaskResult withSourceType(ExportSourceType sourceType) {
this.sourceType = sourceType.toString();
return this;
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getExportTaskIdentifier() != null)
sb.append("ExportTaskIdentifier: ").append(getExportTaskIdentifier()).append(",");
if (getSourceArn() != null)
sb.append("SourceArn: ").append(getSourceArn()).append(",");
if (getExportOnly() != null)
sb.append("ExportOnly: ").append(getExportOnly()).append(",");
if (getSnapshotTime() != null)
sb.append("SnapshotTime: ").append(getSnapshotTime()).append(",");
if (getTaskStartTime() != null)
sb.append("TaskStartTime: ").append(getTaskStartTime()).append(",");
if (getTaskEndTime() != null)
sb.append("TaskEndTime: ").append(getTaskEndTime()).append(",");
if (getS3Bucket() != null)
sb.append("S3Bucket: ").append(getS3Bucket()).append(",");
if (getS3Prefix() != null)
sb.append("S3Prefix: ").append(getS3Prefix()).append(",");
if (getIamRoleArn() != null)
sb.append("IamRoleArn: ").append(getIamRoleArn()).append(",");
if (getKmsKeyId() != null)
sb.append("KmsKeyId: ").append(getKmsKeyId()).append(",");
if (getStatus() != null)
sb.append("Status: ").append(getStatus()).append(",");
if (getPercentProgress() != null)
sb.append("PercentProgress: ").append(getPercentProgress()).append(",");
if (getTotalExtractedDataInGB() != null)
sb.append("TotalExtractedDataInGB: ").append(getTotalExtractedDataInGB()).append(",");
if (getFailureCause() != null)
sb.append("FailureCause: ").append(getFailureCause()).append(",");
if (getWarningMessage() != null)
sb.append("WarningMessage: ").append(getWarningMessage()).append(",");
if (getSourceType() != null)
sb.append("SourceType: ").append(getSourceType());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof StartExportTaskResult == false)
return false;
StartExportTaskResult other = (StartExportTaskResult) obj;
if (other.getExportTaskIdentifier() == null ^ this.getExportTaskIdentifier() == null)
return false;
if (other.getExportTaskIdentifier() != null && other.getExportTaskIdentifier().equals(this.getExportTaskIdentifier()) == false)
return false;
if (other.getSourceArn() == null ^ this.getSourceArn() == null)
return false;
if (other.getSourceArn() != null && other.getSourceArn().equals(this.getSourceArn()) == false)
return false;
if (other.getExportOnly() == null ^ this.getExportOnly() == null)
return false;
if (other.getExportOnly() != null && other.getExportOnly().equals(this.getExportOnly()) == false)
return false;
if (other.getSnapshotTime() == null ^ this.getSnapshotTime() == null)
return false;
if (other.getSnapshotTime() != null && other.getSnapshotTime().equals(this.getSnapshotTime()) == false)
return false;
if (other.getTaskStartTime() == null ^ this.getTaskStartTime() == null)
return false;
if (other.getTaskStartTime() != null && other.getTaskStartTime().equals(this.getTaskStartTime()) == false)
return false;
if (other.getTaskEndTime() == null ^ this.getTaskEndTime() == null)
return false;
if (other.getTaskEndTime() != null && other.getTaskEndTime().equals(this.getTaskEndTime()) == false)
return false;
if (other.getS3Bucket() == null ^ this.getS3Bucket() == null)
return false;
if (other.getS3Bucket() != null && other.getS3Bucket().equals(this.getS3Bucket()) == false)
return false;
if (other.getS3Prefix() == null ^ this.getS3Prefix() == null)
return false;
if (other.getS3Prefix() != null && other.getS3Prefix().equals(this.getS3Prefix()) == false)
return false;
if (other.getIamRoleArn() == null ^ this.getIamRoleArn() == null)
return false;
if (other.getIamRoleArn() != null && other.getIamRoleArn().equals(this.getIamRoleArn()) == false)
return false;
if (other.getKmsKeyId() == null ^ this.getKmsKeyId() == null)
return false;
if (other.getKmsKeyId() != null && other.getKmsKeyId().equals(this.getKmsKeyId()) == false)
return false;
if (other.getStatus() == null ^ this.getStatus() == null)
return false;
if (other.getStatus() != null && other.getStatus().equals(this.getStatus()) == false)
return false;
if (other.getPercentProgress() == null ^ this.getPercentProgress() == null)
return false;
if (other.getPercentProgress() != null && other.getPercentProgress().equals(this.getPercentProgress()) == false)
return false;
if (other.getTotalExtractedDataInGB() == null ^ this.getTotalExtractedDataInGB() == null)
return false;
if (other.getTotalExtractedDataInGB() != null && other.getTotalExtractedDataInGB().equals(this.getTotalExtractedDataInGB()) == false)
return false;
if (other.getFailureCause() == null ^ this.getFailureCause() == null)
return false;
if (other.getFailureCause() != null && other.getFailureCause().equals(this.getFailureCause()) == false)
return false;
if (other.getWarningMessage() == null ^ this.getWarningMessage() == null)
return false;
if (other.getWarningMessage() != null && other.getWarningMessage().equals(this.getWarningMessage()) == false)
return false;
if (other.getSourceType() == null ^ this.getSourceType() == null)
return false;
if (other.getSourceType() != null && other.getSourceType().equals(this.getSourceType()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getExportTaskIdentifier() == null) ? 0 : getExportTaskIdentifier().hashCode());
hashCode = prime * hashCode + ((getSourceArn() == null) ? 0 : getSourceArn().hashCode());
hashCode = prime * hashCode + ((getExportOnly() == null) ? 0 : getExportOnly().hashCode());
hashCode = prime * hashCode + ((getSnapshotTime() == null) ? 0 : getSnapshotTime().hashCode());
hashCode = prime * hashCode + ((getTaskStartTime() == null) ? 0 : getTaskStartTime().hashCode());
hashCode = prime * hashCode + ((getTaskEndTime() == null) ? 0 : getTaskEndTime().hashCode());
hashCode = prime * hashCode + ((getS3Bucket() == null) ? 0 : getS3Bucket().hashCode());
hashCode = prime * hashCode + ((getS3Prefix() == null) ? 0 : getS3Prefix().hashCode());
hashCode = prime * hashCode + ((getIamRoleArn() == null) ? 0 : getIamRoleArn().hashCode());
hashCode = prime * hashCode + ((getKmsKeyId() == null) ? 0 : getKmsKeyId().hashCode());
hashCode = prime * hashCode + ((getStatus() == null) ? 0 : getStatus().hashCode());
hashCode = prime * hashCode + ((getPercentProgress() == null) ? 0 : getPercentProgress().hashCode());
hashCode = prime * hashCode + ((getTotalExtractedDataInGB() == null) ? 0 : getTotalExtractedDataInGB().hashCode());
hashCode = prime * hashCode + ((getFailureCause() == null) ? 0 : getFailureCause().hashCode());
hashCode = prime * hashCode + ((getWarningMessage() == null) ? 0 : getWarningMessage().hashCode());
hashCode = prime * hashCode + ((getSourceType() == null) ? 0 : getSourceType().hashCode());
return hashCode;
}
@Override
public StartExportTaskResult clone() {
try {
return (StartExportTaskResult) super.clone();
} catch (CloneNotSupportedException e) {
throw new IllegalStateException("Got a CloneNotSupportedException from Object.clone() " + "even though we're Cloneable!", e);
}
}
}