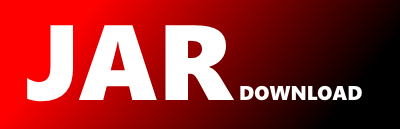
com.amazonaws.services.rds.model.CopyDBSnapshotRequest Maven / Gradle / Ivy
Show all versions of aws-java-sdk-rds Show documentation
/*
* Copyright 2016-2021 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.rds.model;
import java.io.Serializable;
import javax.annotation.Generated;
import com.amazonaws.AmazonWebServiceRequest;
/**
*
*
* @see AWS API
* Documentation
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class CopyDBSnapshotRequest extends com.amazonaws.AmazonWebServiceRequest implements Serializable, Cloneable {
/**
*
* The identifier for the source DB snapshot.
*
*
* If the source snapshot is in the same Amazon Web Services Region as the copy, specify a valid DB snapshot
* identifier. For example, you might specify rds:mysql-instance1-snapshot-20130805
.
*
*
* If the source snapshot is in a different Amazon Web Services Region than the copy, specify a valid DB snapshot
* ARN. For example, you might specify
* arn:aws:rds:us-west-2:123456789012:snapshot:mysql-instance1-snapshot-20130805
.
*
*
* If you are copying from a shared manual DB snapshot, this parameter must be the Amazon Resource Name (ARN) of the
* shared DB snapshot.
*
*
* If you are copying an encrypted snapshot this parameter must be in the ARN format for the source Amazon Web
* Services Region, and must match the SourceDBSnapshotIdentifier
in the PreSignedUrl
* parameter.
*
*
* Constraints:
*
*
* -
*
* Must specify a valid system snapshot in the "available" state.
*
*
*
*
* Example: rds:mydb-2012-04-02-00-01
*
*
* Example: arn:aws:rds:us-west-2:123456789012:snapshot:mysql-instance1-snapshot-20130805
*
*/
private String sourceDBSnapshotIdentifier;
/**
*
* The identifier for the copy of the snapshot.
*
*
* Constraints:
*
*
* -
*
* Can't be null, empty, or blank
*
*
* -
*
* Must contain from 1 to 255 letters, numbers, or hyphens
*
*
* -
*
* First character must be a letter
*
*
* -
*
* Can't end with a hyphen or contain two consecutive hyphens
*
*
*
*
* Example: my-db-snapshot
*
*/
private String targetDBSnapshotIdentifier;
/**
*
* The Amazon Web Services KMS key identifier for an encrypted DB snapshot. The Amazon Web Services KMS key
* identifier is the key ARN, key ID, alias ARN, or alias name for the KMS key.
*
*
* If you copy an encrypted DB snapshot from your Amazon Web Services account, you can specify a value for this
* parameter to encrypt the copy with a new KMS key. If you don't specify a value for this parameter, then the copy
* of the DB snapshot is encrypted with the same Amazon Web Services KMS key as the source DB snapshot.
*
*
* If you copy an encrypted DB snapshot that is shared from another Amazon Web Services account, then you must
* specify a value for this parameter.
*
*
* If you specify this parameter when you copy an unencrypted snapshot, the copy is encrypted.
*
*
* If you copy an encrypted snapshot to a different Amazon Web Services Region, then you must specify an Amazon Web
* Services KMS key identifier for the destination Amazon Web Services Region. KMS keys are specific to the Amazon
* Web Services Region that they are created in, and you can't use KMS keys from one Amazon Web Services Region in
* another Amazon Web Services Region.
*
*/
private String kmsKeyId;
private com.amazonaws.internal.SdkInternalList tags;
/**
*
* A value that indicates whether to copy all tags from the source DB snapshot to the target DB snapshot. By
* default, tags are not copied.
*
*/
private Boolean copyTags;
/**
*
* The URL that contains a Signature Version 4 signed request for the CopyDBSnapshot
API action in the
* source Amazon Web Services Region that contains the source DB snapshot to copy.
*
*
* You must specify this parameter when you copy an encrypted DB snapshot from another Amazon Web Services Region by
* using the Amazon RDS API. Don't specify PreSignedUrl
when you are copying an encrypted DB snapshot
* in the same Amazon Web Services Region.
*
*
* The presigned URL must be a valid request for the CopyDBSnapshot
API action that can be executed in
* the source Amazon Web Services Region that contains the encrypted DB snapshot to be copied. The presigned URL
* request must contain the following parameter values:
*
*
* -
*
* DestinationRegion
- The Amazon Web Services Region that the encrypted DB snapshot is copied to. This
* Amazon Web Services Region is the same one where the CopyDBSnapshot
action is called that contains
* this presigned URL.
*
*
* For example, if you copy an encrypted DB snapshot from the us-west-2 Amazon Web Services Region to the us-east-1
* Amazon Web Services Region, then you call the CopyDBSnapshot
action in the us-east-1 Amazon Web
* Services Region and provide a presigned URL that contains a call to the CopyDBSnapshot
action in the
* us-west-2 Amazon Web Services Region. For this example, the DestinationRegion
in the presigned URL
* must be set to the us-east-1 Amazon Web Services Region.
*
*
* -
*
* KmsKeyId
- The Amazon Web Services KMS key identifier for the KMS key to use to encrypt the copy of
* the DB snapshot in the destination Amazon Web Services Region. This is the same identifier for both the
* CopyDBSnapshot
action that is called in the destination Amazon Web Services Region, and the action
* contained in the presigned URL.
*
*
* -
*
* SourceDBSnapshotIdentifier
- The DB snapshot identifier for the encrypted snapshot to be copied.
* This identifier must be in the Amazon Resource Name (ARN) format for the source Amazon Web Services Region. For
* example, if you are copying an encrypted DB snapshot from the us-west-2 Amazon Web Services Region, then your
* SourceDBSnapshotIdentifier
looks like the following example:
* arn:aws:rds:us-west-2:123456789012:snapshot:mysql-instance1-snapshot-20161115
.
*
*
*
*
* To learn how to generate a Signature Version 4 signed request, see Authenticating Requests:
* Using Query Parameters (Amazon Web Services Signature Version 4) and Signature Version 4 Signing
* Process.
*
*
*
* If you are using an Amazon Web Services SDK tool or the CLI, you can specify SourceRegion
(or
* --source-region
for the CLI) instead of specifying PreSignedUrl
manually. Specifying
* SourceRegion
autogenerates a pre-signed URL that is a valid request for the operation that can be
* executed in the source Amazon Web Services Region.
*
*
*/
private String preSignedUrl;
/**
*
* The name of an option group to associate with the copy of the snapshot.
*
*
* Specify this option if you are copying a snapshot from one Amazon Web Services Region to another, and your DB
* instance uses a nondefault option group. If your source DB instance uses Transparent Data Encryption for Oracle
* or Microsoft SQL Server, you must specify this option when copying across Amazon Web Services Regions. For more
* information, see Option group considerations in the Amazon RDS User Guide.
*
*/
private String optionGroupName;
/**
*
* The external custom Availability Zone (CAZ) identifier for the target CAZ.
*
*
* Example: rds-caz-aiqhTgQv
.
*
*/
private String targetCustomAvailabilityZone;
/** The region where the source snapshot is located. */
private String sourceRegion;
/**
*
* The identifier for the source DB snapshot.
*
*
* If the source snapshot is in the same Amazon Web Services Region as the copy, specify a valid DB snapshot
* identifier. For example, you might specify rds:mysql-instance1-snapshot-20130805
.
*
*
* If the source snapshot is in a different Amazon Web Services Region than the copy, specify a valid DB snapshot
* ARN. For example, you might specify
* arn:aws:rds:us-west-2:123456789012:snapshot:mysql-instance1-snapshot-20130805
.
*
*
* If you are copying from a shared manual DB snapshot, this parameter must be the Amazon Resource Name (ARN) of the
* shared DB snapshot.
*
*
* If you are copying an encrypted snapshot this parameter must be in the ARN format for the source Amazon Web
* Services Region, and must match the SourceDBSnapshotIdentifier
in the PreSignedUrl
* parameter.
*
*
* Constraints:
*
*
* -
*
* Must specify a valid system snapshot in the "available" state.
*
*
*
*
* Example: rds:mydb-2012-04-02-00-01
*
*
* Example: arn:aws:rds:us-west-2:123456789012:snapshot:mysql-instance1-snapshot-20130805
*
*
* @param sourceDBSnapshotIdentifier
* The identifier for the source DB snapshot.
*
* If the source snapshot is in the same Amazon Web Services Region as the copy, specify a valid DB snapshot
* identifier. For example, you might specify rds:mysql-instance1-snapshot-20130805
.
*
*
* If the source snapshot is in a different Amazon Web Services Region than the copy, specify a valid DB
* snapshot ARN. For example, you might specify
* arn:aws:rds:us-west-2:123456789012:snapshot:mysql-instance1-snapshot-20130805
.
*
*
* If you are copying from a shared manual DB snapshot, this parameter must be the Amazon Resource Name (ARN)
* of the shared DB snapshot.
*
*
* If you are copying an encrypted snapshot this parameter must be in the ARN format for the source Amazon
* Web Services Region, and must match the SourceDBSnapshotIdentifier
in the
* PreSignedUrl
parameter.
*
*
* Constraints:
*
*
* -
*
* Must specify a valid system snapshot in the "available" state.
*
*
*
*
* Example: rds:mydb-2012-04-02-00-01
*
*
* Example: arn:aws:rds:us-west-2:123456789012:snapshot:mysql-instance1-snapshot-20130805
*/
public void setSourceDBSnapshotIdentifier(String sourceDBSnapshotIdentifier) {
this.sourceDBSnapshotIdentifier = sourceDBSnapshotIdentifier;
}
/**
*
* The identifier for the source DB snapshot.
*
*
* If the source snapshot is in the same Amazon Web Services Region as the copy, specify a valid DB snapshot
* identifier. For example, you might specify rds:mysql-instance1-snapshot-20130805
.
*
*
* If the source snapshot is in a different Amazon Web Services Region than the copy, specify a valid DB snapshot
* ARN. For example, you might specify
* arn:aws:rds:us-west-2:123456789012:snapshot:mysql-instance1-snapshot-20130805
.
*
*
* If you are copying from a shared manual DB snapshot, this parameter must be the Amazon Resource Name (ARN) of the
* shared DB snapshot.
*
*
* If you are copying an encrypted snapshot this parameter must be in the ARN format for the source Amazon Web
* Services Region, and must match the SourceDBSnapshotIdentifier
in the PreSignedUrl
* parameter.
*
*
* Constraints:
*
*
* -
*
* Must specify a valid system snapshot in the "available" state.
*
*
*
*
* Example: rds:mydb-2012-04-02-00-01
*
*
* Example: arn:aws:rds:us-west-2:123456789012:snapshot:mysql-instance1-snapshot-20130805
*
*
* @return The identifier for the source DB snapshot.
*
* If the source snapshot is in the same Amazon Web Services Region as the copy, specify a valid DB snapshot
* identifier. For example, you might specify rds:mysql-instance1-snapshot-20130805
.
*
*
* If the source snapshot is in a different Amazon Web Services Region than the copy, specify a valid DB
* snapshot ARN. For example, you might specify
* arn:aws:rds:us-west-2:123456789012:snapshot:mysql-instance1-snapshot-20130805
.
*
*
* If you are copying from a shared manual DB snapshot, this parameter must be the Amazon Resource Name
* (ARN) of the shared DB snapshot.
*
*
* If you are copying an encrypted snapshot this parameter must be in the ARN format for the source Amazon
* Web Services Region, and must match the SourceDBSnapshotIdentifier
in the
* PreSignedUrl
parameter.
*
*
* Constraints:
*
*
* -
*
* Must specify a valid system snapshot in the "available" state.
*
*
*
*
* Example: rds:mydb-2012-04-02-00-01
*
*
* Example: arn:aws:rds:us-west-2:123456789012:snapshot:mysql-instance1-snapshot-20130805
*/
public String getSourceDBSnapshotIdentifier() {
return this.sourceDBSnapshotIdentifier;
}
/**
*
* The identifier for the source DB snapshot.
*
*
* If the source snapshot is in the same Amazon Web Services Region as the copy, specify a valid DB snapshot
* identifier. For example, you might specify rds:mysql-instance1-snapshot-20130805
.
*
*
* If the source snapshot is in a different Amazon Web Services Region than the copy, specify a valid DB snapshot
* ARN. For example, you might specify
* arn:aws:rds:us-west-2:123456789012:snapshot:mysql-instance1-snapshot-20130805
.
*
*
* If you are copying from a shared manual DB snapshot, this parameter must be the Amazon Resource Name (ARN) of the
* shared DB snapshot.
*
*
* If you are copying an encrypted snapshot this parameter must be in the ARN format for the source Amazon Web
* Services Region, and must match the SourceDBSnapshotIdentifier
in the PreSignedUrl
* parameter.
*
*
* Constraints:
*
*
* -
*
* Must specify a valid system snapshot in the "available" state.
*
*
*
*
* Example: rds:mydb-2012-04-02-00-01
*
*
* Example: arn:aws:rds:us-west-2:123456789012:snapshot:mysql-instance1-snapshot-20130805
*
*
* @param sourceDBSnapshotIdentifier
* The identifier for the source DB snapshot.
*
* If the source snapshot is in the same Amazon Web Services Region as the copy, specify a valid DB snapshot
* identifier. For example, you might specify rds:mysql-instance1-snapshot-20130805
.
*
*
* If the source snapshot is in a different Amazon Web Services Region than the copy, specify a valid DB
* snapshot ARN. For example, you might specify
* arn:aws:rds:us-west-2:123456789012:snapshot:mysql-instance1-snapshot-20130805
.
*
*
* If you are copying from a shared manual DB snapshot, this parameter must be the Amazon Resource Name (ARN)
* of the shared DB snapshot.
*
*
* If you are copying an encrypted snapshot this parameter must be in the ARN format for the source Amazon
* Web Services Region, and must match the SourceDBSnapshotIdentifier
in the
* PreSignedUrl
parameter.
*
*
* Constraints:
*
*
* -
*
* Must specify a valid system snapshot in the "available" state.
*
*
*
*
* Example: rds:mydb-2012-04-02-00-01
*
*
* Example: arn:aws:rds:us-west-2:123456789012:snapshot:mysql-instance1-snapshot-20130805
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CopyDBSnapshotRequest withSourceDBSnapshotIdentifier(String sourceDBSnapshotIdentifier) {
setSourceDBSnapshotIdentifier(sourceDBSnapshotIdentifier);
return this;
}
/**
*
* The identifier for the copy of the snapshot.
*
*
* Constraints:
*
*
* -
*
* Can't be null, empty, or blank
*
*
* -
*
* Must contain from 1 to 255 letters, numbers, or hyphens
*
*
* -
*
* First character must be a letter
*
*
* -
*
* Can't end with a hyphen or contain two consecutive hyphens
*
*
*
*
* Example: my-db-snapshot
*
*
* @param targetDBSnapshotIdentifier
* The identifier for the copy of the snapshot.
*
* Constraints:
*
*
* -
*
* Can't be null, empty, or blank
*
*
* -
*
* Must contain from 1 to 255 letters, numbers, or hyphens
*
*
* -
*
* First character must be a letter
*
*
* -
*
* Can't end with a hyphen or contain two consecutive hyphens
*
*
*
*
* Example: my-db-snapshot
*/
public void setTargetDBSnapshotIdentifier(String targetDBSnapshotIdentifier) {
this.targetDBSnapshotIdentifier = targetDBSnapshotIdentifier;
}
/**
*
* The identifier for the copy of the snapshot.
*
*
* Constraints:
*
*
* -
*
* Can't be null, empty, or blank
*
*
* -
*
* Must contain from 1 to 255 letters, numbers, or hyphens
*
*
* -
*
* First character must be a letter
*
*
* -
*
* Can't end with a hyphen or contain two consecutive hyphens
*
*
*
*
* Example: my-db-snapshot
*
*
* @return The identifier for the copy of the snapshot.
*
* Constraints:
*
*
* -
*
* Can't be null, empty, or blank
*
*
* -
*
* Must contain from 1 to 255 letters, numbers, or hyphens
*
*
* -
*
* First character must be a letter
*
*
* -
*
* Can't end with a hyphen or contain two consecutive hyphens
*
*
*
*
* Example: my-db-snapshot
*/
public String getTargetDBSnapshotIdentifier() {
return this.targetDBSnapshotIdentifier;
}
/**
*
* The identifier for the copy of the snapshot.
*
*
* Constraints:
*
*
* -
*
* Can't be null, empty, or blank
*
*
* -
*
* Must contain from 1 to 255 letters, numbers, or hyphens
*
*
* -
*
* First character must be a letter
*
*
* -
*
* Can't end with a hyphen or contain two consecutive hyphens
*
*
*
*
* Example: my-db-snapshot
*
*
* @param targetDBSnapshotIdentifier
* The identifier for the copy of the snapshot.
*
* Constraints:
*
*
* -
*
* Can't be null, empty, or blank
*
*
* -
*
* Must contain from 1 to 255 letters, numbers, or hyphens
*
*
* -
*
* First character must be a letter
*
*
* -
*
* Can't end with a hyphen or contain two consecutive hyphens
*
*
*
*
* Example: my-db-snapshot
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CopyDBSnapshotRequest withTargetDBSnapshotIdentifier(String targetDBSnapshotIdentifier) {
setTargetDBSnapshotIdentifier(targetDBSnapshotIdentifier);
return this;
}
/**
*
* The Amazon Web Services KMS key identifier for an encrypted DB snapshot. The Amazon Web Services KMS key
* identifier is the key ARN, key ID, alias ARN, or alias name for the KMS key.
*
*
* If you copy an encrypted DB snapshot from your Amazon Web Services account, you can specify a value for this
* parameter to encrypt the copy with a new KMS key. If you don't specify a value for this parameter, then the copy
* of the DB snapshot is encrypted with the same Amazon Web Services KMS key as the source DB snapshot.
*
*
* If you copy an encrypted DB snapshot that is shared from another Amazon Web Services account, then you must
* specify a value for this parameter.
*
*
* If you specify this parameter when you copy an unencrypted snapshot, the copy is encrypted.
*
*
* If you copy an encrypted snapshot to a different Amazon Web Services Region, then you must specify an Amazon Web
* Services KMS key identifier for the destination Amazon Web Services Region. KMS keys are specific to the Amazon
* Web Services Region that they are created in, and you can't use KMS keys from one Amazon Web Services Region in
* another Amazon Web Services Region.
*
*
* @param kmsKeyId
* The Amazon Web Services KMS key identifier for an encrypted DB snapshot. The Amazon Web Services KMS key
* identifier is the key ARN, key ID, alias ARN, or alias name for the KMS key.
*
* If you copy an encrypted DB snapshot from your Amazon Web Services account, you can specify a value for
* this parameter to encrypt the copy with a new KMS key. If you don't specify a value for this parameter,
* then the copy of the DB snapshot is encrypted with the same Amazon Web Services KMS key as the source DB
* snapshot.
*
*
* If you copy an encrypted DB snapshot that is shared from another Amazon Web Services account, then you
* must specify a value for this parameter.
*
*
* If you specify this parameter when you copy an unencrypted snapshot, the copy is encrypted.
*
*
* If you copy an encrypted snapshot to a different Amazon Web Services Region, then you must specify an
* Amazon Web Services KMS key identifier for the destination Amazon Web Services Region. KMS keys are
* specific to the Amazon Web Services Region that they are created in, and you can't use KMS keys from one
* Amazon Web Services Region in another Amazon Web Services Region.
*/
public void setKmsKeyId(String kmsKeyId) {
this.kmsKeyId = kmsKeyId;
}
/**
*
* The Amazon Web Services KMS key identifier for an encrypted DB snapshot. The Amazon Web Services KMS key
* identifier is the key ARN, key ID, alias ARN, or alias name for the KMS key.
*
*
* If you copy an encrypted DB snapshot from your Amazon Web Services account, you can specify a value for this
* parameter to encrypt the copy with a new KMS key. If you don't specify a value for this parameter, then the copy
* of the DB snapshot is encrypted with the same Amazon Web Services KMS key as the source DB snapshot.
*
*
* If you copy an encrypted DB snapshot that is shared from another Amazon Web Services account, then you must
* specify a value for this parameter.
*
*
* If you specify this parameter when you copy an unencrypted snapshot, the copy is encrypted.
*
*
* If you copy an encrypted snapshot to a different Amazon Web Services Region, then you must specify an Amazon Web
* Services KMS key identifier for the destination Amazon Web Services Region. KMS keys are specific to the Amazon
* Web Services Region that they are created in, and you can't use KMS keys from one Amazon Web Services Region in
* another Amazon Web Services Region.
*
*
* @return The Amazon Web Services KMS key identifier for an encrypted DB snapshot. The Amazon Web Services KMS key
* identifier is the key ARN, key ID, alias ARN, or alias name for the KMS key.
*
* If you copy an encrypted DB snapshot from your Amazon Web Services account, you can specify a value for
* this parameter to encrypt the copy with a new KMS key. If you don't specify a value for this parameter,
* then the copy of the DB snapshot is encrypted with the same Amazon Web Services KMS key as the source DB
* snapshot.
*
*
* If you copy an encrypted DB snapshot that is shared from another Amazon Web Services account, then you
* must specify a value for this parameter.
*
*
* If you specify this parameter when you copy an unencrypted snapshot, the copy is encrypted.
*
*
* If you copy an encrypted snapshot to a different Amazon Web Services Region, then you must specify an
* Amazon Web Services KMS key identifier for the destination Amazon Web Services Region. KMS keys are
* specific to the Amazon Web Services Region that they are created in, and you can't use KMS keys from one
* Amazon Web Services Region in another Amazon Web Services Region.
*/
public String getKmsKeyId() {
return this.kmsKeyId;
}
/**
*
* The Amazon Web Services KMS key identifier for an encrypted DB snapshot. The Amazon Web Services KMS key
* identifier is the key ARN, key ID, alias ARN, or alias name for the KMS key.
*
*
* If you copy an encrypted DB snapshot from your Amazon Web Services account, you can specify a value for this
* parameter to encrypt the copy with a new KMS key. If you don't specify a value for this parameter, then the copy
* of the DB snapshot is encrypted with the same Amazon Web Services KMS key as the source DB snapshot.
*
*
* If you copy an encrypted DB snapshot that is shared from another Amazon Web Services account, then you must
* specify a value for this parameter.
*
*
* If you specify this parameter when you copy an unencrypted snapshot, the copy is encrypted.
*
*
* If you copy an encrypted snapshot to a different Amazon Web Services Region, then you must specify an Amazon Web
* Services KMS key identifier for the destination Amazon Web Services Region. KMS keys are specific to the Amazon
* Web Services Region that they are created in, and you can't use KMS keys from one Amazon Web Services Region in
* another Amazon Web Services Region.
*
*
* @param kmsKeyId
* The Amazon Web Services KMS key identifier for an encrypted DB snapshot. The Amazon Web Services KMS key
* identifier is the key ARN, key ID, alias ARN, or alias name for the KMS key.
*
* If you copy an encrypted DB snapshot from your Amazon Web Services account, you can specify a value for
* this parameter to encrypt the copy with a new KMS key. If you don't specify a value for this parameter,
* then the copy of the DB snapshot is encrypted with the same Amazon Web Services KMS key as the source DB
* snapshot.
*
*
* If you copy an encrypted DB snapshot that is shared from another Amazon Web Services account, then you
* must specify a value for this parameter.
*
*
* If you specify this parameter when you copy an unencrypted snapshot, the copy is encrypted.
*
*
* If you copy an encrypted snapshot to a different Amazon Web Services Region, then you must specify an
* Amazon Web Services KMS key identifier for the destination Amazon Web Services Region. KMS keys are
* specific to the Amazon Web Services Region that they are created in, and you can't use KMS keys from one
* Amazon Web Services Region in another Amazon Web Services Region.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CopyDBSnapshotRequest withKmsKeyId(String kmsKeyId) {
setKmsKeyId(kmsKeyId);
return this;
}
/**
* @return
*/
public java.util.List getTags() {
if (tags == null) {
tags = new com.amazonaws.internal.SdkInternalList();
}
return tags;
}
/**
* @param tags
*/
public void setTags(java.util.Collection tags) {
if (tags == null) {
this.tags = null;
return;
}
this.tags = new com.amazonaws.internal.SdkInternalList(tags);
}
/**
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setTags(java.util.Collection)} or {@link #withTags(java.util.Collection)} if you want to override the
* existing values.
*
*
* @param tags
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CopyDBSnapshotRequest withTags(Tag... tags) {
if (this.tags == null) {
setTags(new com.amazonaws.internal.SdkInternalList(tags.length));
}
for (Tag ele : tags) {
this.tags.add(ele);
}
return this;
}
/**
* @param tags
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CopyDBSnapshotRequest withTags(java.util.Collection tags) {
setTags(tags);
return this;
}
/**
*
* A value that indicates whether to copy all tags from the source DB snapshot to the target DB snapshot. By
* default, tags are not copied.
*
*
* @param copyTags
* A value that indicates whether to copy all tags from the source DB snapshot to the target DB snapshot. By
* default, tags are not copied.
*/
public void setCopyTags(Boolean copyTags) {
this.copyTags = copyTags;
}
/**
*
* A value that indicates whether to copy all tags from the source DB snapshot to the target DB snapshot. By
* default, tags are not copied.
*
*
* @return A value that indicates whether to copy all tags from the source DB snapshot to the target DB snapshot. By
* default, tags are not copied.
*/
public Boolean getCopyTags() {
return this.copyTags;
}
/**
*
* A value that indicates whether to copy all tags from the source DB snapshot to the target DB snapshot. By
* default, tags are not copied.
*
*
* @param copyTags
* A value that indicates whether to copy all tags from the source DB snapshot to the target DB snapshot. By
* default, tags are not copied.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CopyDBSnapshotRequest withCopyTags(Boolean copyTags) {
setCopyTags(copyTags);
return this;
}
/**
*
* A value that indicates whether to copy all tags from the source DB snapshot to the target DB snapshot. By
* default, tags are not copied.
*
*
* @return A value that indicates whether to copy all tags from the source DB snapshot to the target DB snapshot. By
* default, tags are not copied.
*/
public Boolean isCopyTags() {
return this.copyTags;
}
/**
*
* The URL that contains a Signature Version 4 signed request for the CopyDBSnapshot
API action in the
* source Amazon Web Services Region that contains the source DB snapshot to copy.
*
*
* You must specify this parameter when you copy an encrypted DB snapshot from another Amazon Web Services Region by
* using the Amazon RDS API. Don't specify PreSignedUrl
when you are copying an encrypted DB snapshot
* in the same Amazon Web Services Region.
*
*
* The presigned URL must be a valid request for the CopyDBSnapshot
API action that can be executed in
* the source Amazon Web Services Region that contains the encrypted DB snapshot to be copied. The presigned URL
* request must contain the following parameter values:
*
*
* -
*
* DestinationRegion
- The Amazon Web Services Region that the encrypted DB snapshot is copied to. This
* Amazon Web Services Region is the same one where the CopyDBSnapshot
action is called that contains
* this presigned URL.
*
*
* For example, if you copy an encrypted DB snapshot from the us-west-2 Amazon Web Services Region to the us-east-1
* Amazon Web Services Region, then you call the CopyDBSnapshot
action in the us-east-1 Amazon Web
* Services Region and provide a presigned URL that contains a call to the CopyDBSnapshot
action in the
* us-west-2 Amazon Web Services Region. For this example, the DestinationRegion
in the presigned URL
* must be set to the us-east-1 Amazon Web Services Region.
*
*
* -
*
* KmsKeyId
- The Amazon Web Services KMS key identifier for the KMS key to use to encrypt the copy of
* the DB snapshot in the destination Amazon Web Services Region. This is the same identifier for both the
* CopyDBSnapshot
action that is called in the destination Amazon Web Services Region, and the action
* contained in the presigned URL.
*
*
* -
*
* SourceDBSnapshotIdentifier
- The DB snapshot identifier for the encrypted snapshot to be copied.
* This identifier must be in the Amazon Resource Name (ARN) format for the source Amazon Web Services Region. For
* example, if you are copying an encrypted DB snapshot from the us-west-2 Amazon Web Services Region, then your
* SourceDBSnapshotIdentifier
looks like the following example:
* arn:aws:rds:us-west-2:123456789012:snapshot:mysql-instance1-snapshot-20161115
.
*
*
*
*
* To learn how to generate a Signature Version 4 signed request, see Authenticating Requests:
* Using Query Parameters (Amazon Web Services Signature Version 4) and Signature Version 4 Signing
* Process.
*
*
*
* If you are using an Amazon Web Services SDK tool or the CLI, you can specify SourceRegion
(or
* --source-region
for the CLI) instead of specifying PreSignedUrl
manually. Specifying
* SourceRegion
autogenerates a pre-signed URL that is a valid request for the operation that can be
* executed in the source Amazon Web Services Region.
*
*
*
* @param preSignedUrl
* The URL that contains a Signature Version 4 signed request for the CopyDBSnapshot
API action
* in the source Amazon Web Services Region that contains the source DB snapshot to copy.
*
* You must specify this parameter when you copy an encrypted DB snapshot from another Amazon Web Services
* Region by using the Amazon RDS API. Don't specify PreSignedUrl
when you are copying an
* encrypted DB snapshot in the same Amazon Web Services Region.
*
*
* The presigned URL must be a valid request for the CopyDBSnapshot
API action that can be
* executed in the source Amazon Web Services Region that contains the encrypted DB snapshot to be copied.
* The presigned URL request must contain the following parameter values:
*
*
* -
*
* DestinationRegion
- The Amazon Web Services Region that the encrypted DB snapshot is copied
* to. This Amazon Web Services Region is the same one where the CopyDBSnapshot
action is called
* that contains this presigned URL.
*
*
* For example, if you copy an encrypted DB snapshot from the us-west-2 Amazon Web Services Region to the
* us-east-1 Amazon Web Services Region, then you call the CopyDBSnapshot
action in the
* us-east-1 Amazon Web Services Region and provide a presigned URL that contains a call to the
* CopyDBSnapshot
action in the us-west-2 Amazon Web Services Region. For this example, the
* DestinationRegion
in the presigned URL must be set to the us-east-1 Amazon Web Services
* Region.
*
*
* -
*
* KmsKeyId
- The Amazon Web Services KMS key identifier for the KMS key to use to encrypt the
* copy of the DB snapshot in the destination Amazon Web Services Region. This is the same identifier for
* both the CopyDBSnapshot
action that is called in the destination Amazon Web Services Region,
* and the action contained in the presigned URL.
*
*
* -
*
* SourceDBSnapshotIdentifier
- The DB snapshot identifier for the encrypted snapshot to be
* copied. This identifier must be in the Amazon Resource Name (ARN) format for the source Amazon Web
* Services Region. For example, if you are copying an encrypted DB snapshot from the us-west-2 Amazon Web
* Services Region, then your SourceDBSnapshotIdentifier
looks like the following example:
* arn:aws:rds:us-west-2:123456789012:snapshot:mysql-instance1-snapshot-20161115
.
*
*
*
*
* To learn how to generate a Signature Version 4 signed request, see Authenticating
* Requests: Using Query Parameters (Amazon Web Services Signature Version 4) and Signature Version 4 Signing
* Process.
*
*
*
* If you are using an Amazon Web Services SDK tool or the CLI, you can specify SourceRegion
(or
* --source-region
for the CLI) instead of specifying PreSignedUrl
manually.
* Specifying SourceRegion
autogenerates a pre-signed URL that is a valid request for the
* operation that can be executed in the source Amazon Web Services Region.
*
*/
public void setPreSignedUrl(String preSignedUrl) {
this.preSignedUrl = preSignedUrl;
}
/**
*
* The URL that contains a Signature Version 4 signed request for the CopyDBSnapshot
API action in the
* source Amazon Web Services Region that contains the source DB snapshot to copy.
*
*
* You must specify this parameter when you copy an encrypted DB snapshot from another Amazon Web Services Region by
* using the Amazon RDS API. Don't specify PreSignedUrl
when you are copying an encrypted DB snapshot
* in the same Amazon Web Services Region.
*
*
* The presigned URL must be a valid request for the CopyDBSnapshot
API action that can be executed in
* the source Amazon Web Services Region that contains the encrypted DB snapshot to be copied. The presigned URL
* request must contain the following parameter values:
*
*
* -
*
* DestinationRegion
- The Amazon Web Services Region that the encrypted DB snapshot is copied to. This
* Amazon Web Services Region is the same one where the CopyDBSnapshot
action is called that contains
* this presigned URL.
*
*
* For example, if you copy an encrypted DB snapshot from the us-west-2 Amazon Web Services Region to the us-east-1
* Amazon Web Services Region, then you call the CopyDBSnapshot
action in the us-east-1 Amazon Web
* Services Region and provide a presigned URL that contains a call to the CopyDBSnapshot
action in the
* us-west-2 Amazon Web Services Region. For this example, the DestinationRegion
in the presigned URL
* must be set to the us-east-1 Amazon Web Services Region.
*
*
* -
*
* KmsKeyId
- The Amazon Web Services KMS key identifier for the KMS key to use to encrypt the copy of
* the DB snapshot in the destination Amazon Web Services Region. This is the same identifier for both the
* CopyDBSnapshot
action that is called in the destination Amazon Web Services Region, and the action
* contained in the presigned URL.
*
*
* -
*
* SourceDBSnapshotIdentifier
- The DB snapshot identifier for the encrypted snapshot to be copied.
* This identifier must be in the Amazon Resource Name (ARN) format for the source Amazon Web Services Region. For
* example, if you are copying an encrypted DB snapshot from the us-west-2 Amazon Web Services Region, then your
* SourceDBSnapshotIdentifier
looks like the following example:
* arn:aws:rds:us-west-2:123456789012:snapshot:mysql-instance1-snapshot-20161115
.
*
*
*
*
* To learn how to generate a Signature Version 4 signed request, see Authenticating Requests:
* Using Query Parameters (Amazon Web Services Signature Version 4) and Signature Version 4 Signing
* Process.
*
*
*
* If you are using an Amazon Web Services SDK tool or the CLI, you can specify SourceRegion
(or
* --source-region
for the CLI) instead of specifying PreSignedUrl
manually. Specifying
* SourceRegion
autogenerates a pre-signed URL that is a valid request for the operation that can be
* executed in the source Amazon Web Services Region.
*
*
*
* @return The URL that contains a Signature Version 4 signed request for the CopyDBSnapshot
API action
* in the source Amazon Web Services Region that contains the source DB snapshot to copy.
*
* You must specify this parameter when you copy an encrypted DB snapshot from another Amazon Web Services
* Region by using the Amazon RDS API. Don't specify PreSignedUrl
when you are copying an
* encrypted DB snapshot in the same Amazon Web Services Region.
*
*
* The presigned URL must be a valid request for the CopyDBSnapshot
API action that can be
* executed in the source Amazon Web Services Region that contains the encrypted DB snapshot to be copied.
* The presigned URL request must contain the following parameter values:
*
*
* -
*
* DestinationRegion
- The Amazon Web Services Region that the encrypted DB snapshot is copied
* to. This Amazon Web Services Region is the same one where the CopyDBSnapshot
action is
* called that contains this presigned URL.
*
*
* For example, if you copy an encrypted DB snapshot from the us-west-2 Amazon Web Services Region to the
* us-east-1 Amazon Web Services Region, then you call the CopyDBSnapshot
action in the
* us-east-1 Amazon Web Services Region and provide a presigned URL that contains a call to the
* CopyDBSnapshot
action in the us-west-2 Amazon Web Services Region. For this example, the
* DestinationRegion
in the presigned URL must be set to the us-east-1 Amazon Web Services
* Region.
*
*
* -
*
* KmsKeyId
- The Amazon Web Services KMS key identifier for the KMS key to use to encrypt the
* copy of the DB snapshot in the destination Amazon Web Services Region. This is the same identifier for
* both the CopyDBSnapshot
action that is called in the destination Amazon Web Services Region,
* and the action contained in the presigned URL.
*
*
* -
*
* SourceDBSnapshotIdentifier
- The DB snapshot identifier for the encrypted snapshot to be
* copied. This identifier must be in the Amazon Resource Name (ARN) format for the source Amazon Web
* Services Region. For example, if you are copying an encrypted DB snapshot from the us-west-2 Amazon Web
* Services Region, then your SourceDBSnapshotIdentifier
looks like the following example:
* arn:aws:rds:us-west-2:123456789012:snapshot:mysql-instance1-snapshot-20161115
.
*
*
*
*
* To learn how to generate a Signature Version 4 signed request, see Authenticating
* Requests: Using Query Parameters (Amazon Web Services Signature Version 4) and Signature Version 4 Signing
* Process.
*
*
*
* If you are using an Amazon Web Services SDK tool or the CLI, you can specify SourceRegion
* (or --source-region
for the CLI) instead of specifying PreSignedUrl
manually.
* Specifying SourceRegion
autogenerates a pre-signed URL that is a valid request for the
* operation that can be executed in the source Amazon Web Services Region.
*
*/
public String getPreSignedUrl() {
return this.preSignedUrl;
}
/**
*
* The URL that contains a Signature Version 4 signed request for the CopyDBSnapshot
API action in the
* source Amazon Web Services Region that contains the source DB snapshot to copy.
*
*
* You must specify this parameter when you copy an encrypted DB snapshot from another Amazon Web Services Region by
* using the Amazon RDS API. Don't specify PreSignedUrl
when you are copying an encrypted DB snapshot
* in the same Amazon Web Services Region.
*
*
* The presigned URL must be a valid request for the CopyDBSnapshot
API action that can be executed in
* the source Amazon Web Services Region that contains the encrypted DB snapshot to be copied. The presigned URL
* request must contain the following parameter values:
*
*
* -
*
* DestinationRegion
- The Amazon Web Services Region that the encrypted DB snapshot is copied to. This
* Amazon Web Services Region is the same one where the CopyDBSnapshot
action is called that contains
* this presigned URL.
*
*
* For example, if you copy an encrypted DB snapshot from the us-west-2 Amazon Web Services Region to the us-east-1
* Amazon Web Services Region, then you call the CopyDBSnapshot
action in the us-east-1 Amazon Web
* Services Region and provide a presigned URL that contains a call to the CopyDBSnapshot
action in the
* us-west-2 Amazon Web Services Region. For this example, the DestinationRegion
in the presigned URL
* must be set to the us-east-1 Amazon Web Services Region.
*
*
* -
*
* KmsKeyId
- The Amazon Web Services KMS key identifier for the KMS key to use to encrypt the copy of
* the DB snapshot in the destination Amazon Web Services Region. This is the same identifier for both the
* CopyDBSnapshot
action that is called in the destination Amazon Web Services Region, and the action
* contained in the presigned URL.
*
*
* -
*
* SourceDBSnapshotIdentifier
- The DB snapshot identifier for the encrypted snapshot to be copied.
* This identifier must be in the Amazon Resource Name (ARN) format for the source Amazon Web Services Region. For
* example, if you are copying an encrypted DB snapshot from the us-west-2 Amazon Web Services Region, then your
* SourceDBSnapshotIdentifier
looks like the following example:
* arn:aws:rds:us-west-2:123456789012:snapshot:mysql-instance1-snapshot-20161115
.
*
*
*
*
* To learn how to generate a Signature Version 4 signed request, see Authenticating Requests:
* Using Query Parameters (Amazon Web Services Signature Version 4) and Signature Version 4 Signing
* Process.
*
*
*
* If you are using an Amazon Web Services SDK tool or the CLI, you can specify SourceRegion
(or
* --source-region
for the CLI) instead of specifying PreSignedUrl
manually. Specifying
* SourceRegion
autogenerates a pre-signed URL that is a valid request for the operation that can be
* executed in the source Amazon Web Services Region.
*
*
*
* @param preSignedUrl
* The URL that contains a Signature Version 4 signed request for the CopyDBSnapshot
API action
* in the source Amazon Web Services Region that contains the source DB snapshot to copy.
*
* You must specify this parameter when you copy an encrypted DB snapshot from another Amazon Web Services
* Region by using the Amazon RDS API. Don't specify PreSignedUrl
when you are copying an
* encrypted DB snapshot in the same Amazon Web Services Region.
*
*
* The presigned URL must be a valid request for the CopyDBSnapshot
API action that can be
* executed in the source Amazon Web Services Region that contains the encrypted DB snapshot to be copied.
* The presigned URL request must contain the following parameter values:
*
*
* -
*
* DestinationRegion
- The Amazon Web Services Region that the encrypted DB snapshot is copied
* to. This Amazon Web Services Region is the same one where the CopyDBSnapshot
action is called
* that contains this presigned URL.
*
*
* For example, if you copy an encrypted DB snapshot from the us-west-2 Amazon Web Services Region to the
* us-east-1 Amazon Web Services Region, then you call the CopyDBSnapshot
action in the
* us-east-1 Amazon Web Services Region and provide a presigned URL that contains a call to the
* CopyDBSnapshot
action in the us-west-2 Amazon Web Services Region. For this example, the
* DestinationRegion
in the presigned URL must be set to the us-east-1 Amazon Web Services
* Region.
*
*
* -
*
* KmsKeyId
- The Amazon Web Services KMS key identifier for the KMS key to use to encrypt the
* copy of the DB snapshot in the destination Amazon Web Services Region. This is the same identifier for
* both the CopyDBSnapshot
action that is called in the destination Amazon Web Services Region,
* and the action contained in the presigned URL.
*
*
* -
*
* SourceDBSnapshotIdentifier
- The DB snapshot identifier for the encrypted snapshot to be
* copied. This identifier must be in the Amazon Resource Name (ARN) format for the source Amazon Web
* Services Region. For example, if you are copying an encrypted DB snapshot from the us-west-2 Amazon Web
* Services Region, then your SourceDBSnapshotIdentifier
looks like the following example:
* arn:aws:rds:us-west-2:123456789012:snapshot:mysql-instance1-snapshot-20161115
.
*
*
*
*
* To learn how to generate a Signature Version 4 signed request, see Authenticating
* Requests: Using Query Parameters (Amazon Web Services Signature Version 4) and Signature Version 4 Signing
* Process.
*
*
*
* If you are using an Amazon Web Services SDK tool or the CLI, you can specify SourceRegion
(or
* --source-region
for the CLI) instead of specifying PreSignedUrl
manually.
* Specifying SourceRegion
autogenerates a pre-signed URL that is a valid request for the
* operation that can be executed in the source Amazon Web Services Region.
*
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CopyDBSnapshotRequest withPreSignedUrl(String preSignedUrl) {
setPreSignedUrl(preSignedUrl);
return this;
}
/**
*
* The name of an option group to associate with the copy of the snapshot.
*
*
* Specify this option if you are copying a snapshot from one Amazon Web Services Region to another, and your DB
* instance uses a nondefault option group. If your source DB instance uses Transparent Data Encryption for Oracle
* or Microsoft SQL Server, you must specify this option when copying across Amazon Web Services Regions. For more
* information, see Option group considerations in the Amazon RDS User Guide.
*
*
* @param optionGroupName
* The name of an option group to associate with the copy of the snapshot.
*
* Specify this option if you are copying a snapshot from one Amazon Web Services Region to another, and your
* DB instance uses a nondefault option group. If your source DB instance uses Transparent Data Encryption
* for Oracle or Microsoft SQL Server, you must specify this option when copying across Amazon Web Services
* Regions. For more information, see Option group considerations in the Amazon RDS User Guide.
*/
public void setOptionGroupName(String optionGroupName) {
this.optionGroupName = optionGroupName;
}
/**
*
* The name of an option group to associate with the copy of the snapshot.
*
*
* Specify this option if you are copying a snapshot from one Amazon Web Services Region to another, and your DB
* instance uses a nondefault option group. If your source DB instance uses Transparent Data Encryption for Oracle
* or Microsoft SQL Server, you must specify this option when copying across Amazon Web Services Regions. For more
* information, see Option group considerations in the Amazon RDS User Guide.
*
*
* @return The name of an option group to associate with the copy of the snapshot.
*
* Specify this option if you are copying a snapshot from one Amazon Web Services Region to another, and
* your DB instance uses a nondefault option group. If your source DB instance uses Transparent Data
* Encryption for Oracle or Microsoft SQL Server, you must specify this option when copying across Amazon
* Web Services Regions. For more information, see Option group considerations in the Amazon RDS User Guide.
*/
public String getOptionGroupName() {
return this.optionGroupName;
}
/**
*
* The name of an option group to associate with the copy of the snapshot.
*
*
* Specify this option if you are copying a snapshot from one Amazon Web Services Region to another, and your DB
* instance uses a nondefault option group. If your source DB instance uses Transparent Data Encryption for Oracle
* or Microsoft SQL Server, you must specify this option when copying across Amazon Web Services Regions. For more
* information, see Option group considerations in the Amazon RDS User Guide.
*
*
* @param optionGroupName
* The name of an option group to associate with the copy of the snapshot.
*
* Specify this option if you are copying a snapshot from one Amazon Web Services Region to another, and your
* DB instance uses a nondefault option group. If your source DB instance uses Transparent Data Encryption
* for Oracle or Microsoft SQL Server, you must specify this option when copying across Amazon Web Services
* Regions. For more information, see Option group considerations in the Amazon RDS User Guide.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CopyDBSnapshotRequest withOptionGroupName(String optionGroupName) {
setOptionGroupName(optionGroupName);
return this;
}
/**
*
* The external custom Availability Zone (CAZ) identifier for the target CAZ.
*
*
* Example: rds-caz-aiqhTgQv
.
*
*
* @param targetCustomAvailabilityZone
* The external custom Availability Zone (CAZ) identifier for the target CAZ.
*
* Example: rds-caz-aiqhTgQv
.
*/
public void setTargetCustomAvailabilityZone(String targetCustomAvailabilityZone) {
this.targetCustomAvailabilityZone = targetCustomAvailabilityZone;
}
/**
*
* The external custom Availability Zone (CAZ) identifier for the target CAZ.
*
*
* Example: rds-caz-aiqhTgQv
.
*
*
* @return The external custom Availability Zone (CAZ) identifier for the target CAZ.
*
* Example: rds-caz-aiqhTgQv
.
*/
public String getTargetCustomAvailabilityZone() {
return this.targetCustomAvailabilityZone;
}
/**
*
* The external custom Availability Zone (CAZ) identifier for the target CAZ.
*
*
* Example: rds-caz-aiqhTgQv
.
*
*
* @param targetCustomAvailabilityZone
* The external custom Availability Zone (CAZ) identifier for the target CAZ.
*
* Example: rds-caz-aiqhTgQv
.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CopyDBSnapshotRequest withTargetCustomAvailabilityZone(String targetCustomAvailabilityZone) {
setTargetCustomAvailabilityZone(targetCustomAvailabilityZone);
return this;
}
/**
* The region where the source snapshot is located.
*
* @param sourceRegion
* The region where the source snapshot is located.
*/
public void setSourceRegion(String sourceRegion) {
this.sourceRegion = sourceRegion;
}
/**
* The region where the source snapshot is located.
*
* @return The region where the source snapshot is located.
*/
public String getSourceRegion() {
return this.sourceRegion;
}
/**
* The region where the source snapshot is located.
*
* @param sourceRegion
* The region where the source snapshot is located.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CopyDBSnapshotRequest withSourceRegion(String sourceRegion) {
setSourceRegion(sourceRegion);
return this;
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getSourceDBSnapshotIdentifier() != null)
sb.append("SourceDBSnapshotIdentifier: ").append(getSourceDBSnapshotIdentifier()).append(",");
if (getTargetDBSnapshotIdentifier() != null)
sb.append("TargetDBSnapshotIdentifier: ").append(getTargetDBSnapshotIdentifier()).append(",");
if (getKmsKeyId() != null)
sb.append("KmsKeyId: ").append(getKmsKeyId()).append(",");
if (getTags() != null)
sb.append("Tags: ").append(getTags()).append(",");
if (getCopyTags() != null)
sb.append("CopyTags: ").append(getCopyTags()).append(",");
if (getPreSignedUrl() != null)
sb.append("PreSignedUrl: ").append(getPreSignedUrl()).append(",");
if (getOptionGroupName() != null)
sb.append("OptionGroupName: ").append(getOptionGroupName()).append(",");
if (getTargetCustomAvailabilityZone() != null)
sb.append("TargetCustomAvailabilityZone: ").append(getTargetCustomAvailabilityZone()).append(",");
if (getSourceRegion() != null)
sb.append("SourceRegion: ").append(getSourceRegion());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof CopyDBSnapshotRequest == false)
return false;
CopyDBSnapshotRequest other = (CopyDBSnapshotRequest) obj;
if (other.getSourceDBSnapshotIdentifier() == null ^ this.getSourceDBSnapshotIdentifier() == null)
return false;
if (other.getSourceDBSnapshotIdentifier() != null && other.getSourceDBSnapshotIdentifier().equals(this.getSourceDBSnapshotIdentifier()) == false)
return false;
if (other.getTargetDBSnapshotIdentifier() == null ^ this.getTargetDBSnapshotIdentifier() == null)
return false;
if (other.getTargetDBSnapshotIdentifier() != null && other.getTargetDBSnapshotIdentifier().equals(this.getTargetDBSnapshotIdentifier()) == false)
return false;
if (other.getKmsKeyId() == null ^ this.getKmsKeyId() == null)
return false;
if (other.getKmsKeyId() != null && other.getKmsKeyId().equals(this.getKmsKeyId()) == false)
return false;
if (other.getTags() == null ^ this.getTags() == null)
return false;
if (other.getTags() != null && other.getTags().equals(this.getTags()) == false)
return false;
if (other.getCopyTags() == null ^ this.getCopyTags() == null)
return false;
if (other.getCopyTags() != null && other.getCopyTags().equals(this.getCopyTags()) == false)
return false;
if (other.getPreSignedUrl() == null ^ this.getPreSignedUrl() == null)
return false;
if (other.getPreSignedUrl() != null && other.getPreSignedUrl().equals(this.getPreSignedUrl()) == false)
return false;
if (other.getOptionGroupName() == null ^ this.getOptionGroupName() == null)
return false;
if (other.getOptionGroupName() != null && other.getOptionGroupName().equals(this.getOptionGroupName()) == false)
return false;
if (other.getTargetCustomAvailabilityZone() == null ^ this.getTargetCustomAvailabilityZone() == null)
return false;
if (other.getTargetCustomAvailabilityZone() != null && other.getTargetCustomAvailabilityZone().equals(this.getTargetCustomAvailabilityZone()) == false)
return false;
if (other.getSourceRegion() == null ^ this.getSourceRegion() == null)
return false;
if (other.getSourceRegion() != null && other.getSourceRegion().equals(this.getSourceRegion()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getSourceDBSnapshotIdentifier() == null) ? 0 : getSourceDBSnapshotIdentifier().hashCode());
hashCode = prime * hashCode + ((getTargetDBSnapshotIdentifier() == null) ? 0 : getTargetDBSnapshotIdentifier().hashCode());
hashCode = prime * hashCode + ((getKmsKeyId() == null) ? 0 : getKmsKeyId().hashCode());
hashCode = prime * hashCode + ((getTags() == null) ? 0 : getTags().hashCode());
hashCode = prime * hashCode + ((getCopyTags() == null) ? 0 : getCopyTags().hashCode());
hashCode = prime * hashCode + ((getPreSignedUrl() == null) ? 0 : getPreSignedUrl().hashCode());
hashCode = prime * hashCode + ((getOptionGroupName() == null) ? 0 : getOptionGroupName().hashCode());
hashCode = prime * hashCode + ((getTargetCustomAvailabilityZone() == null) ? 0 : getTargetCustomAvailabilityZone().hashCode());
hashCode = prime * hashCode + ((getSourceRegion() == null) ? 0 : getSourceRegion().hashCode());
return hashCode;
}
@Override
public CopyDBSnapshotRequest clone() {
return (CopyDBSnapshotRequest) super.clone();
}
}