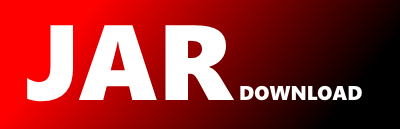
com.amazonaws.services.rds.model.CreateCustomDBEngineVersionRequest Maven / Gradle / Ivy
Show all versions of aws-java-sdk-rds Show documentation
/*
* Copyright 2016-2021 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.rds.model;
import java.io.Serializable;
import javax.annotation.Generated;
import com.amazonaws.AmazonWebServiceRequest;
/**
*
* @see AWS
* API Documentation
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class CreateCustomDBEngineVersionRequest extends com.amazonaws.AmazonWebServiceRequest implements Serializable, Cloneable {
/**
*
* The database engine to use for your custom engine version (CEV). The only supported value is
* custom-oracle-ee
.
*
*/
private String engine;
/**
*
* The name of your CEV. The name format is 19.customized_string
. For example, a valid name is
* 19.my_cev1
. This setting is required for RDS Custom, but optional for Amazon RDS. The combination of
* Engine
and EngineVersion
is unique per customer per Region.
*
*/
private String engineVersion;
/**
*
* The name of an Amazon S3 bucket that contains database installation files for your CEV. For example, a valid
* bucket name is my-custom-installation-files
.
*
*/
private String databaseInstallationFilesS3BucketName;
/**
*
* The Amazon S3 directory that contains the database installation files for your CEV. For example, a valid bucket
* name is 123456789012/cev1
. If this setting isn't specified, no prefix is assumed.
*
*/
private String databaseInstallationFilesS3Prefix;
/**
*
* The Amazon Web Services KMS key identifier for an encrypted CEV. A symmetric KMS key is required for RDS Custom,
* but optional for Amazon RDS.
*
*
* If you have an existing symmetric KMS key in your account, you can use it with RDS Custom. No further action is
* necessary. If you don't already have a symmetric KMS key in your account, follow the instructions in Creating
* symmetric KMS keys in the Amazon Web Services Key Management Service Developer Guide.
*
*
* You can choose the same symmetric key when you create a CEV and a DB instance, or choose different keys.
*
*/
private String kMSKeyId;
/**
*
* An optional description of your CEV.
*
*/
private String description;
/**
*
* The CEV manifest, which is a JSON document that describes the installation .zip files stored in Amazon S3.
* Specify the name/value pairs in a file or a quoted string. RDS Custom applies the patches in the order in which
* they are listed.
*
*
* The following JSON fields are valid:
*
*
* - MediaImportTemplateVersion
* -
*
* Version of the CEV manifest. The date is in the format YYYY-MM-DD
.
*
*
* - databaseInstallationFileNames
* -
*
* Ordered list of installation files for the CEV.
*
*
* - opatchFileNames
* -
*
* Ordered list of OPatch installers used for the Oracle DB engine.
*
*
* - psuRuPatchFileNames
* -
*
* The PSU and RU patches for this CEV.
*
*
* - OtherPatchFileNames
* -
*
* The patches that are not in the list of PSU and RU patches. Amazon RDS applies these patches after applying the
* PSU and RU patches.
*
*
*
*
* For more information, see
* Creating the CEV manifest in the Amazon RDS User Guide.
*
*/
private String manifest;
private com.amazonaws.internal.SdkInternalList tags;
/**
*
* The database engine to use for your custom engine version (CEV). The only supported value is
* custom-oracle-ee
.
*
*
* @param engine
* The database engine to use for your custom engine version (CEV). The only supported value is
* custom-oracle-ee
.
*/
public void setEngine(String engine) {
this.engine = engine;
}
/**
*
* The database engine to use for your custom engine version (CEV). The only supported value is
* custom-oracle-ee
.
*
*
* @return The database engine to use for your custom engine version (CEV). The only supported value is
* custom-oracle-ee
.
*/
public String getEngine() {
return this.engine;
}
/**
*
* The database engine to use for your custom engine version (CEV). The only supported value is
* custom-oracle-ee
.
*
*
* @param engine
* The database engine to use for your custom engine version (CEV). The only supported value is
* custom-oracle-ee
.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateCustomDBEngineVersionRequest withEngine(String engine) {
setEngine(engine);
return this;
}
/**
*
* The name of your CEV. The name format is 19.customized_string
. For example, a valid name is
* 19.my_cev1
. This setting is required for RDS Custom, but optional for Amazon RDS. The combination of
* Engine
and EngineVersion
is unique per customer per Region.
*
*
* @param engineVersion
* The name of your CEV. The name format is 19.customized_string
. For example, a valid
* name is 19.my_cev1
. This setting is required for RDS Custom, but optional for Amazon RDS. The
* combination of Engine
and EngineVersion
is unique per customer per Region.
*/
public void setEngineVersion(String engineVersion) {
this.engineVersion = engineVersion;
}
/**
*
* The name of your CEV. The name format is 19.customized_string
. For example, a valid name is
* 19.my_cev1
. This setting is required for RDS Custom, but optional for Amazon RDS. The combination of
* Engine
and EngineVersion
is unique per customer per Region.
*
*
* @return The name of your CEV. The name format is 19.customized_string
. For example, a valid
* name is 19.my_cev1
. This setting is required for RDS Custom, but optional for Amazon RDS.
* The combination of Engine
and EngineVersion
is unique per customer per Region.
*/
public String getEngineVersion() {
return this.engineVersion;
}
/**
*
* The name of your CEV. The name format is 19.customized_string
. For example, a valid name is
* 19.my_cev1
. This setting is required for RDS Custom, but optional for Amazon RDS. The combination of
* Engine
and EngineVersion
is unique per customer per Region.
*
*
* @param engineVersion
* The name of your CEV. The name format is 19.customized_string
. For example, a valid
* name is 19.my_cev1
. This setting is required for RDS Custom, but optional for Amazon RDS. The
* combination of Engine
and EngineVersion
is unique per customer per Region.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateCustomDBEngineVersionRequest withEngineVersion(String engineVersion) {
setEngineVersion(engineVersion);
return this;
}
/**
*
* The name of an Amazon S3 bucket that contains database installation files for your CEV. For example, a valid
* bucket name is my-custom-installation-files
.
*
*
* @param databaseInstallationFilesS3BucketName
* The name of an Amazon S3 bucket that contains database installation files for your CEV. For example, a
* valid bucket name is my-custom-installation-files
.
*/
public void setDatabaseInstallationFilesS3BucketName(String databaseInstallationFilesS3BucketName) {
this.databaseInstallationFilesS3BucketName = databaseInstallationFilesS3BucketName;
}
/**
*
* The name of an Amazon S3 bucket that contains database installation files for your CEV. For example, a valid
* bucket name is my-custom-installation-files
.
*
*
* @return The name of an Amazon S3 bucket that contains database installation files for your CEV. For example, a
* valid bucket name is my-custom-installation-files
.
*/
public String getDatabaseInstallationFilesS3BucketName() {
return this.databaseInstallationFilesS3BucketName;
}
/**
*
* The name of an Amazon S3 bucket that contains database installation files for your CEV. For example, a valid
* bucket name is my-custom-installation-files
.
*
*
* @param databaseInstallationFilesS3BucketName
* The name of an Amazon S3 bucket that contains database installation files for your CEV. For example, a
* valid bucket name is my-custom-installation-files
.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateCustomDBEngineVersionRequest withDatabaseInstallationFilesS3BucketName(String databaseInstallationFilesS3BucketName) {
setDatabaseInstallationFilesS3BucketName(databaseInstallationFilesS3BucketName);
return this;
}
/**
*
* The Amazon S3 directory that contains the database installation files for your CEV. For example, a valid bucket
* name is 123456789012/cev1
. If this setting isn't specified, no prefix is assumed.
*
*
* @param databaseInstallationFilesS3Prefix
* The Amazon S3 directory that contains the database installation files for your CEV. For example, a valid
* bucket name is 123456789012/cev1
. If this setting isn't specified, no prefix is assumed.
*/
public void setDatabaseInstallationFilesS3Prefix(String databaseInstallationFilesS3Prefix) {
this.databaseInstallationFilesS3Prefix = databaseInstallationFilesS3Prefix;
}
/**
*
* The Amazon S3 directory that contains the database installation files for your CEV. For example, a valid bucket
* name is 123456789012/cev1
. If this setting isn't specified, no prefix is assumed.
*
*
* @return The Amazon S3 directory that contains the database installation files for your CEV. For example, a valid
* bucket name is 123456789012/cev1
. If this setting isn't specified, no prefix is assumed.
*/
public String getDatabaseInstallationFilesS3Prefix() {
return this.databaseInstallationFilesS3Prefix;
}
/**
*
* The Amazon S3 directory that contains the database installation files for your CEV. For example, a valid bucket
* name is 123456789012/cev1
. If this setting isn't specified, no prefix is assumed.
*
*
* @param databaseInstallationFilesS3Prefix
* The Amazon S3 directory that contains the database installation files for your CEV. For example, a valid
* bucket name is 123456789012/cev1
. If this setting isn't specified, no prefix is assumed.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateCustomDBEngineVersionRequest withDatabaseInstallationFilesS3Prefix(String databaseInstallationFilesS3Prefix) {
setDatabaseInstallationFilesS3Prefix(databaseInstallationFilesS3Prefix);
return this;
}
/**
*
* The Amazon Web Services KMS key identifier for an encrypted CEV. A symmetric KMS key is required for RDS Custom,
* but optional for Amazon RDS.
*
*
* If you have an existing symmetric KMS key in your account, you can use it with RDS Custom. No further action is
* necessary. If you don't already have a symmetric KMS key in your account, follow the instructions in Creating
* symmetric KMS keys in the Amazon Web Services Key Management Service Developer Guide.
*
*
* You can choose the same symmetric key when you create a CEV and a DB instance, or choose different keys.
*
*
* @param kMSKeyId
* The Amazon Web Services KMS key identifier for an encrypted CEV. A symmetric KMS key is required for RDS
* Custom, but optional for Amazon RDS.
*
* If you have an existing symmetric KMS key in your account, you can use it with RDS Custom. No further
* action is necessary. If you don't already have a symmetric KMS key in your account, follow the
* instructions in
* Creating symmetric KMS keys in the Amazon Web Services Key Management Service Developer Guide.
*
*
* You can choose the same symmetric key when you create a CEV and a DB instance, or choose different keys.
*/
public void setKMSKeyId(String kMSKeyId) {
this.kMSKeyId = kMSKeyId;
}
/**
*
* The Amazon Web Services KMS key identifier for an encrypted CEV. A symmetric KMS key is required for RDS Custom,
* but optional for Amazon RDS.
*
*
* If you have an existing symmetric KMS key in your account, you can use it with RDS Custom. No further action is
* necessary. If you don't already have a symmetric KMS key in your account, follow the instructions in Creating
* symmetric KMS keys in the Amazon Web Services Key Management Service Developer Guide.
*
*
* You can choose the same symmetric key when you create a CEV and a DB instance, or choose different keys.
*
*
* @return The Amazon Web Services KMS key identifier for an encrypted CEV. A symmetric KMS key is required for RDS
* Custom, but optional for Amazon RDS.
*
* If you have an existing symmetric KMS key in your account, you can use it with RDS Custom. No further
* action is necessary. If you don't already have a symmetric KMS key in your account, follow the
* instructions in
* Creating symmetric KMS keys in the Amazon Web Services Key Management Service Developer Guide.
*
*
* You can choose the same symmetric key when you create a CEV and a DB instance, or choose different keys.
*/
public String getKMSKeyId() {
return this.kMSKeyId;
}
/**
*
* The Amazon Web Services KMS key identifier for an encrypted CEV. A symmetric KMS key is required for RDS Custom,
* but optional for Amazon RDS.
*
*
* If you have an existing symmetric KMS key in your account, you can use it with RDS Custom. No further action is
* necessary. If you don't already have a symmetric KMS key in your account, follow the instructions in Creating
* symmetric KMS keys in the Amazon Web Services Key Management Service Developer Guide.
*
*
* You can choose the same symmetric key when you create a CEV and a DB instance, or choose different keys.
*
*
* @param kMSKeyId
* The Amazon Web Services KMS key identifier for an encrypted CEV. A symmetric KMS key is required for RDS
* Custom, but optional for Amazon RDS.
*
* If you have an existing symmetric KMS key in your account, you can use it with RDS Custom. No further
* action is necessary. If you don't already have a symmetric KMS key in your account, follow the
* instructions in
* Creating symmetric KMS keys in the Amazon Web Services Key Management Service Developer Guide.
*
*
* You can choose the same symmetric key when you create a CEV and a DB instance, or choose different keys.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateCustomDBEngineVersionRequest withKMSKeyId(String kMSKeyId) {
setKMSKeyId(kMSKeyId);
return this;
}
/**
*
* An optional description of your CEV.
*
*
* @param description
* An optional description of your CEV.
*/
public void setDescription(String description) {
this.description = description;
}
/**
*
* An optional description of your CEV.
*
*
* @return An optional description of your CEV.
*/
public String getDescription() {
return this.description;
}
/**
*
* An optional description of your CEV.
*
*
* @param description
* An optional description of your CEV.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateCustomDBEngineVersionRequest withDescription(String description) {
setDescription(description);
return this;
}
/**
*
* The CEV manifest, which is a JSON document that describes the installation .zip files stored in Amazon S3.
* Specify the name/value pairs in a file or a quoted string. RDS Custom applies the patches in the order in which
* they are listed.
*
*
* The following JSON fields are valid:
*
*
* - MediaImportTemplateVersion
* -
*
* Version of the CEV manifest. The date is in the format YYYY-MM-DD
.
*
*
* - databaseInstallationFileNames
* -
*
* Ordered list of installation files for the CEV.
*
*
* - opatchFileNames
* -
*
* Ordered list of OPatch installers used for the Oracle DB engine.
*
*
* - psuRuPatchFileNames
* -
*
* The PSU and RU patches for this CEV.
*
*
* - OtherPatchFileNames
* -
*
* The patches that are not in the list of PSU and RU patches. Amazon RDS applies these patches after applying the
* PSU and RU patches.
*
*
*
*
* For more information, see
* Creating the CEV manifest in the Amazon RDS User Guide.
*
*
* @param manifest
* The CEV manifest, which is a JSON document that describes the installation .zip files stored in Amazon S3.
* Specify the name/value pairs in a file or a quoted string. RDS Custom applies the patches in the order in
* which they are listed.
*
* The following JSON fields are valid:
*
*
* - MediaImportTemplateVersion
* -
*
* Version of the CEV manifest. The date is in the format YYYY-MM-DD
.
*
*
* - databaseInstallationFileNames
* -
*
* Ordered list of installation files for the CEV.
*
*
* - opatchFileNames
* -
*
* Ordered list of OPatch installers used for the Oracle DB engine.
*
*
* - psuRuPatchFileNames
* -
*
* The PSU and RU patches for this CEV.
*
*
* - OtherPatchFileNames
* -
*
* The patches that are not in the list of PSU and RU patches. Amazon RDS applies these patches after
* applying the PSU and RU patches.
*
*
*
*
* For more information, see
* Creating the CEV manifest in the Amazon RDS User Guide.
*/
public void setManifest(String manifest) {
this.manifest = manifest;
}
/**
*
* The CEV manifest, which is a JSON document that describes the installation .zip files stored in Amazon S3.
* Specify the name/value pairs in a file or a quoted string. RDS Custom applies the patches in the order in which
* they are listed.
*
*
* The following JSON fields are valid:
*
*
* - MediaImportTemplateVersion
* -
*
* Version of the CEV manifest. The date is in the format YYYY-MM-DD
.
*
*
* - databaseInstallationFileNames
* -
*
* Ordered list of installation files for the CEV.
*
*
* - opatchFileNames
* -
*
* Ordered list of OPatch installers used for the Oracle DB engine.
*
*
* - psuRuPatchFileNames
* -
*
* The PSU and RU patches for this CEV.
*
*
* - OtherPatchFileNames
* -
*
* The patches that are not in the list of PSU and RU patches. Amazon RDS applies these patches after applying the
* PSU and RU patches.
*
*
*
*
* For more information, see
* Creating the CEV manifest in the Amazon RDS User Guide.
*
*
* @return The CEV manifest, which is a JSON document that describes the installation .zip files stored in Amazon
* S3. Specify the name/value pairs in a file or a quoted string. RDS Custom applies the patches in the
* order in which they are listed.
*
* The following JSON fields are valid:
*
*
* - MediaImportTemplateVersion
* -
*
* Version of the CEV manifest. The date is in the format YYYY-MM-DD
.
*
*
* - databaseInstallationFileNames
* -
*
* Ordered list of installation files for the CEV.
*
*
* - opatchFileNames
* -
*
* Ordered list of OPatch installers used for the Oracle DB engine.
*
*
* - psuRuPatchFileNames
* -
*
* The PSU and RU patches for this CEV.
*
*
* - OtherPatchFileNames
* -
*
* The patches that are not in the list of PSU and RU patches. Amazon RDS applies these patches after
* applying the PSU and RU patches.
*
*
*
*
* For more information, see
* Creating the CEV manifest in the Amazon RDS User Guide.
*/
public String getManifest() {
return this.manifest;
}
/**
*
* The CEV manifest, which is a JSON document that describes the installation .zip files stored in Amazon S3.
* Specify the name/value pairs in a file or a quoted string. RDS Custom applies the patches in the order in which
* they are listed.
*
*
* The following JSON fields are valid:
*
*
* - MediaImportTemplateVersion
* -
*
* Version of the CEV manifest. The date is in the format YYYY-MM-DD
.
*
*
* - databaseInstallationFileNames
* -
*
* Ordered list of installation files for the CEV.
*
*
* - opatchFileNames
* -
*
* Ordered list of OPatch installers used for the Oracle DB engine.
*
*
* - psuRuPatchFileNames
* -
*
* The PSU and RU patches for this CEV.
*
*
* - OtherPatchFileNames
* -
*
* The patches that are not in the list of PSU and RU patches. Amazon RDS applies these patches after applying the
* PSU and RU patches.
*
*
*
*
* For more information, see
* Creating the CEV manifest in the Amazon RDS User Guide.
*
*
* @param manifest
* The CEV manifest, which is a JSON document that describes the installation .zip files stored in Amazon S3.
* Specify the name/value pairs in a file or a quoted string. RDS Custom applies the patches in the order in
* which they are listed.
*
* The following JSON fields are valid:
*
*
* - MediaImportTemplateVersion
* -
*
* Version of the CEV manifest. The date is in the format YYYY-MM-DD
.
*
*
* - databaseInstallationFileNames
* -
*
* Ordered list of installation files for the CEV.
*
*
* - opatchFileNames
* -
*
* Ordered list of OPatch installers used for the Oracle DB engine.
*
*
* - psuRuPatchFileNames
* -
*
* The PSU and RU patches for this CEV.
*
*
* - OtherPatchFileNames
* -
*
* The patches that are not in the list of PSU and RU patches. Amazon RDS applies these patches after
* applying the PSU and RU patches.
*
*
*
*
* For more information, see
* Creating the CEV manifest in the Amazon RDS User Guide.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateCustomDBEngineVersionRequest withManifest(String manifest) {
setManifest(manifest);
return this;
}
/**
* @return
*/
public java.util.List getTags() {
if (tags == null) {
tags = new com.amazonaws.internal.SdkInternalList();
}
return tags;
}
/**
* @param tags
*/
public void setTags(java.util.Collection tags) {
if (tags == null) {
this.tags = null;
return;
}
this.tags = new com.amazonaws.internal.SdkInternalList(tags);
}
/**
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setTags(java.util.Collection)} or {@link #withTags(java.util.Collection)} if you want to override the
* existing values.
*
*
* @param tags
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateCustomDBEngineVersionRequest withTags(Tag... tags) {
if (this.tags == null) {
setTags(new com.amazonaws.internal.SdkInternalList(tags.length));
}
for (Tag ele : tags) {
this.tags.add(ele);
}
return this;
}
/**
* @param tags
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateCustomDBEngineVersionRequest withTags(java.util.Collection tags) {
setTags(tags);
return this;
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getEngine() != null)
sb.append("Engine: ").append(getEngine()).append(",");
if (getEngineVersion() != null)
sb.append("EngineVersion: ").append(getEngineVersion()).append(",");
if (getDatabaseInstallationFilesS3BucketName() != null)
sb.append("DatabaseInstallationFilesS3BucketName: ").append(getDatabaseInstallationFilesS3BucketName()).append(",");
if (getDatabaseInstallationFilesS3Prefix() != null)
sb.append("DatabaseInstallationFilesS3Prefix: ").append(getDatabaseInstallationFilesS3Prefix()).append(",");
if (getKMSKeyId() != null)
sb.append("KMSKeyId: ").append(getKMSKeyId()).append(",");
if (getDescription() != null)
sb.append("Description: ").append(getDescription()).append(",");
if (getManifest() != null)
sb.append("Manifest: ").append(getManifest()).append(",");
if (getTags() != null)
sb.append("Tags: ").append(getTags());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof CreateCustomDBEngineVersionRequest == false)
return false;
CreateCustomDBEngineVersionRequest other = (CreateCustomDBEngineVersionRequest) obj;
if (other.getEngine() == null ^ this.getEngine() == null)
return false;
if (other.getEngine() != null && other.getEngine().equals(this.getEngine()) == false)
return false;
if (other.getEngineVersion() == null ^ this.getEngineVersion() == null)
return false;
if (other.getEngineVersion() != null && other.getEngineVersion().equals(this.getEngineVersion()) == false)
return false;
if (other.getDatabaseInstallationFilesS3BucketName() == null ^ this.getDatabaseInstallationFilesS3BucketName() == null)
return false;
if (other.getDatabaseInstallationFilesS3BucketName() != null
&& other.getDatabaseInstallationFilesS3BucketName().equals(this.getDatabaseInstallationFilesS3BucketName()) == false)
return false;
if (other.getDatabaseInstallationFilesS3Prefix() == null ^ this.getDatabaseInstallationFilesS3Prefix() == null)
return false;
if (other.getDatabaseInstallationFilesS3Prefix() != null
&& other.getDatabaseInstallationFilesS3Prefix().equals(this.getDatabaseInstallationFilesS3Prefix()) == false)
return false;
if (other.getKMSKeyId() == null ^ this.getKMSKeyId() == null)
return false;
if (other.getKMSKeyId() != null && other.getKMSKeyId().equals(this.getKMSKeyId()) == false)
return false;
if (other.getDescription() == null ^ this.getDescription() == null)
return false;
if (other.getDescription() != null && other.getDescription().equals(this.getDescription()) == false)
return false;
if (other.getManifest() == null ^ this.getManifest() == null)
return false;
if (other.getManifest() != null && other.getManifest().equals(this.getManifest()) == false)
return false;
if (other.getTags() == null ^ this.getTags() == null)
return false;
if (other.getTags() != null && other.getTags().equals(this.getTags()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getEngine() == null) ? 0 : getEngine().hashCode());
hashCode = prime * hashCode + ((getEngineVersion() == null) ? 0 : getEngineVersion().hashCode());
hashCode = prime * hashCode + ((getDatabaseInstallationFilesS3BucketName() == null) ? 0 : getDatabaseInstallationFilesS3BucketName().hashCode());
hashCode = prime * hashCode + ((getDatabaseInstallationFilesS3Prefix() == null) ? 0 : getDatabaseInstallationFilesS3Prefix().hashCode());
hashCode = prime * hashCode + ((getKMSKeyId() == null) ? 0 : getKMSKeyId().hashCode());
hashCode = prime * hashCode + ((getDescription() == null) ? 0 : getDescription().hashCode());
hashCode = prime * hashCode + ((getManifest() == null) ? 0 : getManifest().hashCode());
hashCode = prime * hashCode + ((getTags() == null) ? 0 : getTags().hashCode());
return hashCode;
}
@Override
public CreateCustomDBEngineVersionRequest clone() {
return (CreateCustomDBEngineVersionRequest) super.clone();
}
}