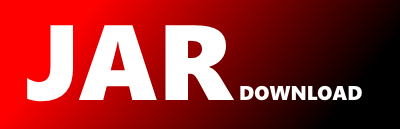
com.amazonaws.services.rds.model.StartExportTaskRequest Maven / Gradle / Ivy
/*
* Copyright 2016-2021 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.rds.model;
import java.io.Serializable;
import javax.annotation.Generated;
import com.amazonaws.AmazonWebServiceRequest;
/**
*
* @see AWS API
* Documentation
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class StartExportTaskRequest extends com.amazonaws.AmazonWebServiceRequest implements Serializable, Cloneable {
/**
*
* A unique identifier for the snapshot export task. This ID isn't an identifier for the Amazon S3 bucket where the
* snapshot is to be exported to.
*
*/
private String exportTaskIdentifier;
/**
*
* The Amazon Resource Name (ARN) of the snapshot to export to Amazon S3.
*
*/
private String sourceArn;
/**
*
* The name of the Amazon S3 bucket to export the snapshot to.
*
*/
private String s3BucketName;
/**
*
* The name of the IAM role to use for writing to the Amazon S3 bucket when exporting a snapshot.
*
*/
private String iamRoleArn;
/**
*
* The ID of the Amazon Web Services KMS key to use to encrypt the snapshot exported to Amazon S3. The Amazon Web
* Services KMS key identifier is the key ARN, key ID, alias ARN, or alias name for the KMS key. The caller of this
* operation must be authorized to execute the following operations. These can be set in the Amazon Web Services KMS
* key policy:
*
*
* -
*
* GrantOperation.Encrypt
*
*
* -
*
* GrantOperation.Decrypt
*
*
* -
*
* GrantOperation.GenerateDataKey
*
*
* -
*
* GrantOperation.GenerateDataKeyWithoutPlaintext
*
*
* -
*
* GrantOperation.ReEncryptFrom
*
*
* -
*
* GrantOperation.ReEncryptTo
*
*
* -
*
* GrantOperation.CreateGrant
*
*
* -
*
* GrantOperation.DescribeKey
*
*
* -
*
* GrantOperation.RetireGrant
*
*
*
*/
private String kmsKeyId;
/**
*
* The Amazon S3 bucket prefix to use as the file name and path of the exported snapshot.
*
*/
private String s3Prefix;
/**
*
* The data to be exported from the snapshot. If this parameter is not provided, all the snapshot data is exported.
* Valid values are the following:
*
*
* -
*
* database
- Export all the data from a specified database.
*
*
* -
*
* database.table
table-name - Export a table of the snapshot. This format is valid only for RDS
* for MySQL, RDS for MariaDB, and Aurora MySQL.
*
*
* -
*
* database.schema
schema-name - Export a database schema of the snapshot. This format is valid
* only for RDS for PostgreSQL and Aurora PostgreSQL.
*
*
* -
*
* database.schema.table
table-name - Export a table of the database schema. This format is
* valid only for RDS for PostgreSQL and Aurora PostgreSQL.
*
*
*
*/
private com.amazonaws.internal.SdkInternalList exportOnly;
/**
*
* A unique identifier for the snapshot export task. This ID isn't an identifier for the Amazon S3 bucket where the
* snapshot is to be exported to.
*
*
* @param exportTaskIdentifier
* A unique identifier for the snapshot export task. This ID isn't an identifier for the Amazon S3 bucket
* where the snapshot is to be exported to.
*/
public void setExportTaskIdentifier(String exportTaskIdentifier) {
this.exportTaskIdentifier = exportTaskIdentifier;
}
/**
*
* A unique identifier for the snapshot export task. This ID isn't an identifier for the Amazon S3 bucket where the
* snapshot is to be exported to.
*
*
* @return A unique identifier for the snapshot export task. This ID isn't an identifier for the Amazon S3 bucket
* where the snapshot is to be exported to.
*/
public String getExportTaskIdentifier() {
return this.exportTaskIdentifier;
}
/**
*
* A unique identifier for the snapshot export task. This ID isn't an identifier for the Amazon S3 bucket where the
* snapshot is to be exported to.
*
*
* @param exportTaskIdentifier
* A unique identifier for the snapshot export task. This ID isn't an identifier for the Amazon S3 bucket
* where the snapshot is to be exported to.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public StartExportTaskRequest withExportTaskIdentifier(String exportTaskIdentifier) {
setExportTaskIdentifier(exportTaskIdentifier);
return this;
}
/**
*
* The Amazon Resource Name (ARN) of the snapshot to export to Amazon S3.
*
*
* @param sourceArn
* The Amazon Resource Name (ARN) of the snapshot to export to Amazon S3.
*/
public void setSourceArn(String sourceArn) {
this.sourceArn = sourceArn;
}
/**
*
* The Amazon Resource Name (ARN) of the snapshot to export to Amazon S3.
*
*
* @return The Amazon Resource Name (ARN) of the snapshot to export to Amazon S3.
*/
public String getSourceArn() {
return this.sourceArn;
}
/**
*
* The Amazon Resource Name (ARN) of the snapshot to export to Amazon S3.
*
*
* @param sourceArn
* The Amazon Resource Name (ARN) of the snapshot to export to Amazon S3.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public StartExportTaskRequest withSourceArn(String sourceArn) {
setSourceArn(sourceArn);
return this;
}
/**
*
* The name of the Amazon S3 bucket to export the snapshot to.
*
*
* @param s3BucketName
* The name of the Amazon S3 bucket to export the snapshot to.
*/
public void setS3BucketName(String s3BucketName) {
this.s3BucketName = s3BucketName;
}
/**
*
* The name of the Amazon S3 bucket to export the snapshot to.
*
*
* @return The name of the Amazon S3 bucket to export the snapshot to.
*/
public String getS3BucketName() {
return this.s3BucketName;
}
/**
*
* The name of the Amazon S3 bucket to export the snapshot to.
*
*
* @param s3BucketName
* The name of the Amazon S3 bucket to export the snapshot to.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public StartExportTaskRequest withS3BucketName(String s3BucketName) {
setS3BucketName(s3BucketName);
return this;
}
/**
*
* The name of the IAM role to use for writing to the Amazon S3 bucket when exporting a snapshot.
*
*
* @param iamRoleArn
* The name of the IAM role to use for writing to the Amazon S3 bucket when exporting a snapshot.
*/
public void setIamRoleArn(String iamRoleArn) {
this.iamRoleArn = iamRoleArn;
}
/**
*
* The name of the IAM role to use for writing to the Amazon S3 bucket when exporting a snapshot.
*
*
* @return The name of the IAM role to use for writing to the Amazon S3 bucket when exporting a snapshot.
*/
public String getIamRoleArn() {
return this.iamRoleArn;
}
/**
*
* The name of the IAM role to use for writing to the Amazon S3 bucket when exporting a snapshot.
*
*
* @param iamRoleArn
* The name of the IAM role to use for writing to the Amazon S3 bucket when exporting a snapshot.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public StartExportTaskRequest withIamRoleArn(String iamRoleArn) {
setIamRoleArn(iamRoleArn);
return this;
}
/**
*
* The ID of the Amazon Web Services KMS key to use to encrypt the snapshot exported to Amazon S3. The Amazon Web
* Services KMS key identifier is the key ARN, key ID, alias ARN, or alias name for the KMS key. The caller of this
* operation must be authorized to execute the following operations. These can be set in the Amazon Web Services KMS
* key policy:
*
*
* -
*
* GrantOperation.Encrypt
*
*
* -
*
* GrantOperation.Decrypt
*
*
* -
*
* GrantOperation.GenerateDataKey
*
*
* -
*
* GrantOperation.GenerateDataKeyWithoutPlaintext
*
*
* -
*
* GrantOperation.ReEncryptFrom
*
*
* -
*
* GrantOperation.ReEncryptTo
*
*
* -
*
* GrantOperation.CreateGrant
*
*
* -
*
* GrantOperation.DescribeKey
*
*
* -
*
* GrantOperation.RetireGrant
*
*
*
*
* @param kmsKeyId
* The ID of the Amazon Web Services KMS key to use to encrypt the snapshot exported to Amazon S3. The Amazon
* Web Services KMS key identifier is the key ARN, key ID, alias ARN, or alias name for the KMS key. The
* caller of this operation must be authorized to execute the following operations. These can be set in the
* Amazon Web Services KMS key policy:
*
* -
*
* GrantOperation.Encrypt
*
*
* -
*
* GrantOperation.Decrypt
*
*
* -
*
* GrantOperation.GenerateDataKey
*
*
* -
*
* GrantOperation.GenerateDataKeyWithoutPlaintext
*
*
* -
*
* GrantOperation.ReEncryptFrom
*
*
* -
*
* GrantOperation.ReEncryptTo
*
*
* -
*
* GrantOperation.CreateGrant
*
*
* -
*
* GrantOperation.DescribeKey
*
*
* -
*
* GrantOperation.RetireGrant
*
*
*/
public void setKmsKeyId(String kmsKeyId) {
this.kmsKeyId = kmsKeyId;
}
/**
*
* The ID of the Amazon Web Services KMS key to use to encrypt the snapshot exported to Amazon S3. The Amazon Web
* Services KMS key identifier is the key ARN, key ID, alias ARN, or alias name for the KMS key. The caller of this
* operation must be authorized to execute the following operations. These can be set in the Amazon Web Services KMS
* key policy:
*
*
* -
*
* GrantOperation.Encrypt
*
*
* -
*
* GrantOperation.Decrypt
*
*
* -
*
* GrantOperation.GenerateDataKey
*
*
* -
*
* GrantOperation.GenerateDataKeyWithoutPlaintext
*
*
* -
*
* GrantOperation.ReEncryptFrom
*
*
* -
*
* GrantOperation.ReEncryptTo
*
*
* -
*
* GrantOperation.CreateGrant
*
*
* -
*
* GrantOperation.DescribeKey
*
*
* -
*
* GrantOperation.RetireGrant
*
*
*
*
* @return The ID of the Amazon Web Services KMS key to use to encrypt the snapshot exported to Amazon S3. The
* Amazon Web Services KMS key identifier is the key ARN, key ID, alias ARN, or alias name for the KMS key.
* The caller of this operation must be authorized to execute the following operations. These can be set in
* the Amazon Web Services KMS key policy:
*
* -
*
* GrantOperation.Encrypt
*
*
* -
*
* GrantOperation.Decrypt
*
*
* -
*
* GrantOperation.GenerateDataKey
*
*
* -
*
* GrantOperation.GenerateDataKeyWithoutPlaintext
*
*
* -
*
* GrantOperation.ReEncryptFrom
*
*
* -
*
* GrantOperation.ReEncryptTo
*
*
* -
*
* GrantOperation.CreateGrant
*
*
* -
*
* GrantOperation.DescribeKey
*
*
* -
*
* GrantOperation.RetireGrant
*
*
*/
public String getKmsKeyId() {
return this.kmsKeyId;
}
/**
*
* The ID of the Amazon Web Services KMS key to use to encrypt the snapshot exported to Amazon S3. The Amazon Web
* Services KMS key identifier is the key ARN, key ID, alias ARN, or alias name for the KMS key. The caller of this
* operation must be authorized to execute the following operations. These can be set in the Amazon Web Services KMS
* key policy:
*
*
* -
*
* GrantOperation.Encrypt
*
*
* -
*
* GrantOperation.Decrypt
*
*
* -
*
* GrantOperation.GenerateDataKey
*
*
* -
*
* GrantOperation.GenerateDataKeyWithoutPlaintext
*
*
* -
*
* GrantOperation.ReEncryptFrom
*
*
* -
*
* GrantOperation.ReEncryptTo
*
*
* -
*
* GrantOperation.CreateGrant
*
*
* -
*
* GrantOperation.DescribeKey
*
*
* -
*
* GrantOperation.RetireGrant
*
*
*
*
* @param kmsKeyId
* The ID of the Amazon Web Services KMS key to use to encrypt the snapshot exported to Amazon S3. The Amazon
* Web Services KMS key identifier is the key ARN, key ID, alias ARN, or alias name for the KMS key. The
* caller of this operation must be authorized to execute the following operations. These can be set in the
* Amazon Web Services KMS key policy:
*
* -
*
* GrantOperation.Encrypt
*
*
* -
*
* GrantOperation.Decrypt
*
*
* -
*
* GrantOperation.GenerateDataKey
*
*
* -
*
* GrantOperation.GenerateDataKeyWithoutPlaintext
*
*
* -
*
* GrantOperation.ReEncryptFrom
*
*
* -
*
* GrantOperation.ReEncryptTo
*
*
* -
*
* GrantOperation.CreateGrant
*
*
* -
*
* GrantOperation.DescribeKey
*
*
* -
*
* GrantOperation.RetireGrant
*
*
* @return Returns a reference to this object so that method calls can be chained together.
*/
public StartExportTaskRequest withKmsKeyId(String kmsKeyId) {
setKmsKeyId(kmsKeyId);
return this;
}
/**
*
* The Amazon S3 bucket prefix to use as the file name and path of the exported snapshot.
*
*
* @param s3Prefix
* The Amazon S3 bucket prefix to use as the file name and path of the exported snapshot.
*/
public void setS3Prefix(String s3Prefix) {
this.s3Prefix = s3Prefix;
}
/**
*
* The Amazon S3 bucket prefix to use as the file name and path of the exported snapshot.
*
*
* @return The Amazon S3 bucket prefix to use as the file name and path of the exported snapshot.
*/
public String getS3Prefix() {
return this.s3Prefix;
}
/**
*
* The Amazon S3 bucket prefix to use as the file name and path of the exported snapshot.
*
*
* @param s3Prefix
* The Amazon S3 bucket prefix to use as the file name and path of the exported snapshot.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public StartExportTaskRequest withS3Prefix(String s3Prefix) {
setS3Prefix(s3Prefix);
return this;
}
/**
*
* The data to be exported from the snapshot. If this parameter is not provided, all the snapshot data is exported.
* Valid values are the following:
*
*
* -
*
* database
- Export all the data from a specified database.
*
*
* -
*
* database.table
table-name - Export a table of the snapshot. This format is valid only for RDS
* for MySQL, RDS for MariaDB, and Aurora MySQL.
*
*
* -
*
* database.schema
schema-name - Export a database schema of the snapshot. This format is valid
* only for RDS for PostgreSQL and Aurora PostgreSQL.
*
*
* -
*
* database.schema.table
table-name - Export a table of the database schema. This format is
* valid only for RDS for PostgreSQL and Aurora PostgreSQL.
*
*
*
*
* @return The data to be exported from the snapshot. If this parameter is not provided, all the snapshot data is
* exported. Valid values are the following:
*
* -
*
* database
- Export all the data from a specified database.
*
*
* -
*
* database.table
table-name - Export a table of the snapshot. This format is valid only
* for RDS for MySQL, RDS for MariaDB, and Aurora MySQL.
*
*
* -
*
* database.schema
schema-name - Export a database schema of the snapshot. This format
* is valid only for RDS for PostgreSQL and Aurora PostgreSQL.
*
*
* -
*
* database.schema.table
table-name - Export a table of the database schema. This format
* is valid only for RDS for PostgreSQL and Aurora PostgreSQL.
*
*
*/
public java.util.List getExportOnly() {
if (exportOnly == null) {
exportOnly = new com.amazonaws.internal.SdkInternalList();
}
return exportOnly;
}
/**
*
* The data to be exported from the snapshot. If this parameter is not provided, all the snapshot data is exported.
* Valid values are the following:
*
*
* -
*
* database
- Export all the data from a specified database.
*
*
* -
*
* database.table
table-name - Export a table of the snapshot. This format is valid only for RDS
* for MySQL, RDS for MariaDB, and Aurora MySQL.
*
*
* -
*
* database.schema
schema-name - Export a database schema of the snapshot. This format is valid
* only for RDS for PostgreSQL and Aurora PostgreSQL.
*
*
* -
*
* database.schema.table
table-name - Export a table of the database schema. This format is
* valid only for RDS for PostgreSQL and Aurora PostgreSQL.
*
*
*
*
* @param exportOnly
* The data to be exported from the snapshot. If this parameter is not provided, all the snapshot data is
* exported. Valid values are the following:
*
* -
*
* database
- Export all the data from a specified database.
*
*
* -
*
* database.table
table-name - Export a table of the snapshot. This format is valid only
* for RDS for MySQL, RDS for MariaDB, and Aurora MySQL.
*
*
* -
*
* database.schema
schema-name - Export a database schema of the snapshot. This format is
* valid only for RDS for PostgreSQL and Aurora PostgreSQL.
*
*
* -
*
* database.schema.table
table-name - Export a table of the database schema. This format
* is valid only for RDS for PostgreSQL and Aurora PostgreSQL.
*
*
*/
public void setExportOnly(java.util.Collection exportOnly) {
if (exportOnly == null) {
this.exportOnly = null;
return;
}
this.exportOnly = new com.amazonaws.internal.SdkInternalList(exportOnly);
}
/**
*
* The data to be exported from the snapshot. If this parameter is not provided, all the snapshot data is exported.
* Valid values are the following:
*
*
* -
*
* database
- Export all the data from a specified database.
*
*
* -
*
* database.table
table-name - Export a table of the snapshot. This format is valid only for RDS
* for MySQL, RDS for MariaDB, and Aurora MySQL.
*
*
* -
*
* database.schema
schema-name - Export a database schema of the snapshot. This format is valid
* only for RDS for PostgreSQL and Aurora PostgreSQL.
*
*
* -
*
* database.schema.table
table-name - Export a table of the database schema. This format is
* valid only for RDS for PostgreSQL and Aurora PostgreSQL.
*
*
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setExportOnly(java.util.Collection)} or {@link #withExportOnly(java.util.Collection)} if you want to
* override the existing values.
*
*
* @param exportOnly
* The data to be exported from the snapshot. If this parameter is not provided, all the snapshot data is
* exported. Valid values are the following:
*
* -
*
* database
- Export all the data from a specified database.
*
*
* -
*
* database.table
table-name - Export a table of the snapshot. This format is valid only
* for RDS for MySQL, RDS for MariaDB, and Aurora MySQL.
*
*
* -
*
* database.schema
schema-name - Export a database schema of the snapshot. This format is
* valid only for RDS for PostgreSQL and Aurora PostgreSQL.
*
*
* -
*
* database.schema.table
table-name - Export a table of the database schema. This format
* is valid only for RDS for PostgreSQL and Aurora PostgreSQL.
*
*
* @return Returns a reference to this object so that method calls can be chained together.
*/
public StartExportTaskRequest withExportOnly(String... exportOnly) {
if (this.exportOnly == null) {
setExportOnly(new com.amazonaws.internal.SdkInternalList(exportOnly.length));
}
for (String ele : exportOnly) {
this.exportOnly.add(ele);
}
return this;
}
/**
*
* The data to be exported from the snapshot. If this parameter is not provided, all the snapshot data is exported.
* Valid values are the following:
*
*
* -
*
* database
- Export all the data from a specified database.
*
*
* -
*
* database.table
table-name - Export a table of the snapshot. This format is valid only for RDS
* for MySQL, RDS for MariaDB, and Aurora MySQL.
*
*
* -
*
* database.schema
schema-name - Export a database schema of the snapshot. This format is valid
* only for RDS for PostgreSQL and Aurora PostgreSQL.
*
*
* -
*
* database.schema.table
table-name - Export a table of the database schema. This format is
* valid only for RDS for PostgreSQL and Aurora PostgreSQL.
*
*
*
*
* @param exportOnly
* The data to be exported from the snapshot. If this parameter is not provided, all the snapshot data is
* exported. Valid values are the following:
*
* -
*
* database
- Export all the data from a specified database.
*
*
* -
*
* database.table
table-name - Export a table of the snapshot. This format is valid only
* for RDS for MySQL, RDS for MariaDB, and Aurora MySQL.
*
*
* -
*
* database.schema
schema-name - Export a database schema of the snapshot. This format is
* valid only for RDS for PostgreSQL and Aurora PostgreSQL.
*
*
* -
*
* database.schema.table
table-name - Export a table of the database schema. This format
* is valid only for RDS for PostgreSQL and Aurora PostgreSQL.
*
*
* @return Returns a reference to this object so that method calls can be chained together.
*/
public StartExportTaskRequest withExportOnly(java.util.Collection exportOnly) {
setExportOnly(exportOnly);
return this;
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getExportTaskIdentifier() != null)
sb.append("ExportTaskIdentifier: ").append(getExportTaskIdentifier()).append(",");
if (getSourceArn() != null)
sb.append("SourceArn: ").append(getSourceArn()).append(",");
if (getS3BucketName() != null)
sb.append("S3BucketName: ").append(getS3BucketName()).append(",");
if (getIamRoleArn() != null)
sb.append("IamRoleArn: ").append(getIamRoleArn()).append(",");
if (getKmsKeyId() != null)
sb.append("KmsKeyId: ").append(getKmsKeyId()).append(",");
if (getS3Prefix() != null)
sb.append("S3Prefix: ").append(getS3Prefix()).append(",");
if (getExportOnly() != null)
sb.append("ExportOnly: ").append(getExportOnly());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof StartExportTaskRequest == false)
return false;
StartExportTaskRequest other = (StartExportTaskRequest) obj;
if (other.getExportTaskIdentifier() == null ^ this.getExportTaskIdentifier() == null)
return false;
if (other.getExportTaskIdentifier() != null && other.getExportTaskIdentifier().equals(this.getExportTaskIdentifier()) == false)
return false;
if (other.getSourceArn() == null ^ this.getSourceArn() == null)
return false;
if (other.getSourceArn() != null && other.getSourceArn().equals(this.getSourceArn()) == false)
return false;
if (other.getS3BucketName() == null ^ this.getS3BucketName() == null)
return false;
if (other.getS3BucketName() != null && other.getS3BucketName().equals(this.getS3BucketName()) == false)
return false;
if (other.getIamRoleArn() == null ^ this.getIamRoleArn() == null)
return false;
if (other.getIamRoleArn() != null && other.getIamRoleArn().equals(this.getIamRoleArn()) == false)
return false;
if (other.getKmsKeyId() == null ^ this.getKmsKeyId() == null)
return false;
if (other.getKmsKeyId() != null && other.getKmsKeyId().equals(this.getKmsKeyId()) == false)
return false;
if (other.getS3Prefix() == null ^ this.getS3Prefix() == null)
return false;
if (other.getS3Prefix() != null && other.getS3Prefix().equals(this.getS3Prefix()) == false)
return false;
if (other.getExportOnly() == null ^ this.getExportOnly() == null)
return false;
if (other.getExportOnly() != null && other.getExportOnly().equals(this.getExportOnly()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getExportTaskIdentifier() == null) ? 0 : getExportTaskIdentifier().hashCode());
hashCode = prime * hashCode + ((getSourceArn() == null) ? 0 : getSourceArn().hashCode());
hashCode = prime * hashCode + ((getS3BucketName() == null) ? 0 : getS3BucketName().hashCode());
hashCode = prime * hashCode + ((getIamRoleArn() == null) ? 0 : getIamRoleArn().hashCode());
hashCode = prime * hashCode + ((getKmsKeyId() == null) ? 0 : getKmsKeyId().hashCode());
hashCode = prime * hashCode + ((getS3Prefix() == null) ? 0 : getS3Prefix().hashCode());
hashCode = prime * hashCode + ((getExportOnly() == null) ? 0 : getExportOnly().hashCode());
return hashCode;
}
@Override
public StartExportTaskRequest clone() {
return (StartExportTaskRequest) super.clone();
}
}