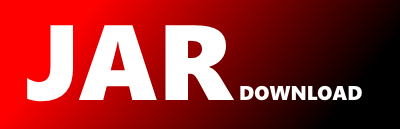
com.amazonaws.services.redshift.AmazonRedshiftAsync Maven / Gradle / Ivy
Show all versions of aws-java-sdk-redshift Show documentation
/*
* Copyright 2010-2016 Amazon.com, Inc. or its affiliates. All Rights
* Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License").
* You may not use this file except in compliance with the License.
* A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed
* on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either
* express or implied. See the License for the specific language governing
* permissions and limitations under the License.
*/
package com.amazonaws.services.redshift;
import com.amazonaws.services.redshift.model.*;
/**
* Interface for accessing Amazon Redshift asynchronously. Each asynchronous
* method will return a Java Future object representing the asynchronous
* operation; overloads which accept an {@code AsyncHandler} can be used to
* receive notification when an asynchronous operation completes.
*
* Amazon Redshift Overview
*
* This is an interface reference for Amazon Redshift. It contains documentation
* for one of the programming or command line interfaces you can use to manage
* Amazon Redshift clusters. Note that Amazon Redshift is asynchronous, which
* means that some interfaces may require techniques, such as polling or
* asynchronous callback handlers, to determine when a command has been applied.
* In this reference, the parameter descriptions indicate whether a change is
* applied immediately, on the next instance reboot, or during the next
* maintenance window. For a summary of the Amazon Redshift cluster management
* interfaces, go to Using the Amazon Redshift Management Interfaces .
*
*
* Amazon Redshift manages all the work of setting up, operating, and scaling a
* data warehouse: provisioning capacity, monitoring and backing up the cluster,
* and applying patches and upgrades to the Amazon Redshift engine. You can
* focus on using your data to acquire new insights for your business and
* customers.
*
*
* If you are a first-time user of Amazon Redshift, we recommend that you begin
* by reading the The Amazon Redshift Getting Started Guide
*
*
* If you are a database developer, the Amazon
* Redshift Database Developer Guide explains how to design, build, query,
* and maintain the databases that make up your data warehouse.
*
*/
public interface AmazonRedshiftAsync extends AmazonRedshift {
/**
*
* Adds an inbound (ingress) rule to an Amazon Redshift security group.
* Depending on whether the application accessing your cluster is running on
* the Internet or an Amazon EC2 instance, you can authorize inbound access
* to either a Classless Interdomain Routing (CIDR)/Internet Protocol (IP)
* range or to an Amazon EC2 security group. You can add as many as 20
* ingress rules to an Amazon Redshift security group.
*
*
* If you authorize access to an Amazon EC2 security group, specify
* EC2SecurityGroupName and EC2SecurityGroupOwnerId. The
* Amazon EC2 security group and Amazon Redshift cluster must be in the same
* AWS region.
*
*
* If you authorize access to a CIDR/IP address range, specify
* CIDRIP. For an overview of CIDR blocks, see the Wikipedia article
* on
* Classless Inter-Domain Routing.
*
*
* You must also associate the security group with a cluster so that clients
* running on these IP addresses or the EC2 instance are authorized to
* connect to the cluster. For information about managing security groups,
* go to Working with Security Groups in the Amazon Redshift Cluster
* Management Guide.
*
*
* @param authorizeClusterSecurityGroupIngressRequest
* @return A Java Future containing the result of the
* AuthorizeClusterSecurityGroupIngress operation returned by the
* service.
* @sample AmazonRedshiftAsync.AuthorizeClusterSecurityGroupIngress
*/
java.util.concurrent.Future authorizeClusterSecurityGroupIngressAsync(
AuthorizeClusterSecurityGroupIngressRequest authorizeClusterSecurityGroupIngressRequest);
/**
*
* Adds an inbound (ingress) rule to an Amazon Redshift security group.
* Depending on whether the application accessing your cluster is running on
* the Internet or an Amazon EC2 instance, you can authorize inbound access
* to either a Classless Interdomain Routing (CIDR)/Internet Protocol (IP)
* range or to an Amazon EC2 security group. You can add as many as 20
* ingress rules to an Amazon Redshift security group.
*
*
* If you authorize access to an Amazon EC2 security group, specify
* EC2SecurityGroupName and EC2SecurityGroupOwnerId. The
* Amazon EC2 security group and Amazon Redshift cluster must be in the same
* AWS region.
*
*
* If you authorize access to a CIDR/IP address range, specify
* CIDRIP. For an overview of CIDR blocks, see the Wikipedia article
* on
* Classless Inter-Domain Routing.
*
*
* You must also associate the security group with a cluster so that clients
* running on these IP addresses or the EC2 instance are authorized to
* connect to the cluster. For information about managing security groups,
* go to Working with Security Groups in the Amazon Redshift Cluster
* Management Guide.
*
*
* @param authorizeClusterSecurityGroupIngressRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the
* request. Users can provide an implementation of the callback
* methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the
* AuthorizeClusterSecurityGroupIngress operation returned by the
* service.
* @sample AmazonRedshiftAsyncHandler.AuthorizeClusterSecurityGroupIngress
*/
java.util.concurrent.Future authorizeClusterSecurityGroupIngressAsync(
AuthorizeClusterSecurityGroupIngressRequest authorizeClusterSecurityGroupIngressRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Authorizes the specified AWS customer account to restore the specified
* snapshot.
*
*
* For more information about working with snapshots, go to Amazon Redshift Snapshots in the Amazon Redshift Cluster
* Management Guide.
*
*
* @param authorizeSnapshotAccessRequest
* @return A Java Future containing the result of the
* AuthorizeSnapshotAccess operation returned by the service.
* @sample AmazonRedshiftAsync.AuthorizeSnapshotAccess
*/
java.util.concurrent.Future authorizeSnapshotAccessAsync(
AuthorizeSnapshotAccessRequest authorizeSnapshotAccessRequest);
/**
*
* Authorizes the specified AWS customer account to restore the specified
* snapshot.
*
*
* For more information about working with snapshots, go to Amazon Redshift Snapshots in the Amazon Redshift Cluster
* Management Guide.
*
*
* @param authorizeSnapshotAccessRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the
* request. Users can provide an implementation of the callback
* methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the
* AuthorizeSnapshotAccess operation returned by the service.
* @sample AmazonRedshiftAsyncHandler.AuthorizeSnapshotAccess
*/
java.util.concurrent.Future authorizeSnapshotAccessAsync(
AuthorizeSnapshotAccessRequest authorizeSnapshotAccessRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Copies the specified automated cluster snapshot to a new manual cluster
* snapshot. The source must be an automated snapshot and it must be in the
* available state.
*
*
* When you delete a cluster, Amazon Redshift deletes any automated
* snapshots of the cluster. Also, when the retention period of the snapshot
* expires, Amazon Redshift automatically deletes it. If you want to keep an
* automated snapshot for a longer period, you can make a manual copy of the
* snapshot. Manual snapshots are retained until you delete them.
*
*
* For more information about working with snapshots, go to Amazon Redshift Snapshots in the Amazon Redshift Cluster
* Management Guide.
*
*
* @param copyClusterSnapshotRequest
* @return A Java Future containing the result of the CopyClusterSnapshot
* operation returned by the service.
* @sample AmazonRedshiftAsync.CopyClusterSnapshot
*/
java.util.concurrent.Future copyClusterSnapshotAsync(
CopyClusterSnapshotRequest copyClusterSnapshotRequest);
/**
*
* Copies the specified automated cluster snapshot to a new manual cluster
* snapshot. The source must be an automated snapshot and it must be in the
* available state.
*
*
* When you delete a cluster, Amazon Redshift deletes any automated
* snapshots of the cluster. Also, when the retention period of the snapshot
* expires, Amazon Redshift automatically deletes it. If you want to keep an
* automated snapshot for a longer period, you can make a manual copy of the
* snapshot. Manual snapshots are retained until you delete them.
*
*
* For more information about working with snapshots, go to Amazon Redshift Snapshots in the Amazon Redshift Cluster
* Management Guide.
*
*
* @param copyClusterSnapshotRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the
* request. Users can provide an implementation of the callback
* methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CopyClusterSnapshot
* operation returned by the service.
* @sample AmazonRedshiftAsyncHandler.CopyClusterSnapshot
*/
java.util.concurrent.Future copyClusterSnapshotAsync(
CopyClusterSnapshotRequest copyClusterSnapshotRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates a new cluster. To create the cluster in virtual private cloud
* (VPC), you must provide cluster subnet group name. If you don't provide a
* cluster subnet group name or the cluster security group parameter, Amazon
* Redshift creates a non-VPC cluster, it associates the default cluster
* security group with the cluster. For more information about managing
* clusters, go to Amazon Redshift Clusters in the Amazon Redshift Cluster
* Management Guide .
*
*
* @param createClusterRequest
* @return A Java Future containing the result of the CreateCluster
* operation returned by the service.
* @sample AmazonRedshiftAsync.CreateCluster
*/
java.util.concurrent.Future createClusterAsync(
CreateClusterRequest createClusterRequest);
/**
*
* Creates a new cluster. To create the cluster in virtual private cloud
* (VPC), you must provide cluster subnet group name. If you don't provide a
* cluster subnet group name or the cluster security group parameter, Amazon
* Redshift creates a non-VPC cluster, it associates the default cluster
* security group with the cluster. For more information about managing
* clusters, go to Amazon Redshift Clusters in the Amazon Redshift Cluster
* Management Guide .
*
*
* @param createClusterRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the
* request. Users can provide an implementation of the callback
* methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateCluster
* operation returned by the service.
* @sample AmazonRedshiftAsyncHandler.CreateCluster
*/
java.util.concurrent.Future createClusterAsync(
CreateClusterRequest createClusterRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates an Amazon Redshift parameter group.
*
*
* Creating parameter groups is independent of creating clusters. You can
* associate a cluster with a parameter group when you create the cluster.
* You can also associate an existing cluster with a parameter group after
* the cluster is created by using ModifyCluster.
*
*
* Parameters in the parameter group define specific behavior that applies
* to the databases you create on the cluster. For more information about
* parameters and parameter groups, go to Amazon Redshift Parameter Groups in the Amazon Redshift Cluster
* Management Guide.
*
*
* @param createClusterParameterGroupRequest
* @return A Java Future containing the result of the
* CreateClusterParameterGroup operation returned by the service.
* @sample AmazonRedshiftAsync.CreateClusterParameterGroup
*/
java.util.concurrent.Future createClusterParameterGroupAsync(
CreateClusterParameterGroupRequest createClusterParameterGroupRequest);
/**
*
* Creates an Amazon Redshift parameter group.
*
*
* Creating parameter groups is independent of creating clusters. You can
* associate a cluster with a parameter group when you create the cluster.
* You can also associate an existing cluster with a parameter group after
* the cluster is created by using ModifyCluster.
*
*
* Parameters in the parameter group define specific behavior that applies
* to the databases you create on the cluster. For more information about
* parameters and parameter groups, go to Amazon Redshift Parameter Groups in the Amazon Redshift Cluster
* Management Guide.
*
*
* @param createClusterParameterGroupRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the
* request. Users can provide an implementation of the callback
* methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the
* CreateClusterParameterGroup operation returned by the service.
* @sample AmazonRedshiftAsyncHandler.CreateClusterParameterGroup
*/
java.util.concurrent.Future createClusterParameterGroupAsync(
CreateClusterParameterGroupRequest createClusterParameterGroupRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates a new Amazon Redshift security group. You use security groups to
* control access to non-VPC clusters.
*
*
* For information about managing security groups, go to Amazon Redshift Cluster Security Groups in the Amazon Redshift
* Cluster Management Guide.
*
*
* @param createClusterSecurityGroupRequest
* @return A Java Future containing the result of the
* CreateClusterSecurityGroup operation returned by the service.
* @sample AmazonRedshiftAsync.CreateClusterSecurityGroup
*/
java.util.concurrent.Future createClusterSecurityGroupAsync(
CreateClusterSecurityGroupRequest createClusterSecurityGroupRequest);
/**
*
* Creates a new Amazon Redshift security group. You use security groups to
* control access to non-VPC clusters.
*
*
* For information about managing security groups, go to Amazon Redshift Cluster Security Groups in the Amazon Redshift
* Cluster Management Guide.
*
*
* @param createClusterSecurityGroupRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the
* request. Users can provide an implementation of the callback
* methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the
* CreateClusterSecurityGroup operation returned by the service.
* @sample AmazonRedshiftAsyncHandler.CreateClusterSecurityGroup
*/
java.util.concurrent.Future createClusterSecurityGroupAsync(
CreateClusterSecurityGroupRequest createClusterSecurityGroupRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates a manual snapshot of the specified cluster. The cluster must be
* in the available
state.
*
*
* For more information about working with snapshots, go to Amazon Redshift Snapshots in the Amazon Redshift Cluster
* Management Guide.
*
*
* @param createClusterSnapshotRequest
* @return A Java Future containing the result of the CreateClusterSnapshot
* operation returned by the service.
* @sample AmazonRedshiftAsync.CreateClusterSnapshot
*/
java.util.concurrent.Future createClusterSnapshotAsync(
CreateClusterSnapshotRequest createClusterSnapshotRequest);
/**
*
* Creates a manual snapshot of the specified cluster. The cluster must be
* in the available
state.
*
*
* For more information about working with snapshots, go to Amazon Redshift Snapshots in the Amazon Redshift Cluster
* Management Guide.
*
*
* @param createClusterSnapshotRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the
* request. Users can provide an implementation of the callback
* methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateClusterSnapshot
* operation returned by the service.
* @sample AmazonRedshiftAsyncHandler.CreateClusterSnapshot
*/
java.util.concurrent.Future createClusterSnapshotAsync(
CreateClusterSnapshotRequest createClusterSnapshotRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates a new Amazon Redshift subnet group. You must provide a list of
* one or more subnets in your existing Amazon Virtual Private Cloud (Amazon
* VPC) when creating Amazon Redshift subnet group.
*
*
* For information about subnet groups, go to Amazon Redshift Cluster Subnet Groups in the Amazon Redshift
* Cluster Management Guide.
*
*
* @param createClusterSubnetGroupRequest
* @return A Java Future containing the result of the
* CreateClusterSubnetGroup operation returned by the service.
* @sample AmazonRedshiftAsync.CreateClusterSubnetGroup
*/
java.util.concurrent.Future createClusterSubnetGroupAsync(
CreateClusterSubnetGroupRequest createClusterSubnetGroupRequest);
/**
*
* Creates a new Amazon Redshift subnet group. You must provide a list of
* one or more subnets in your existing Amazon Virtual Private Cloud (Amazon
* VPC) when creating Amazon Redshift subnet group.
*
*
* For information about subnet groups, go to Amazon Redshift Cluster Subnet Groups in the Amazon Redshift
* Cluster Management Guide.
*
*
* @param createClusterSubnetGroupRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the
* request. Users can provide an implementation of the callback
* methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the
* CreateClusterSubnetGroup operation returned by the service.
* @sample AmazonRedshiftAsyncHandler.CreateClusterSubnetGroup
*/
java.util.concurrent.Future createClusterSubnetGroupAsync(
CreateClusterSubnetGroupRequest createClusterSubnetGroupRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates an Amazon Redshift event notification subscription. This action
* requires an ARN (Amazon Resource Name) of an Amazon SNS topic created by
* either the Amazon Redshift console, the Amazon SNS console, or the Amazon
* SNS API. To obtain an ARN with Amazon SNS, you must create a topic in
* Amazon SNS and subscribe to the topic. The ARN is displayed in the SNS
* console.
*
*
* You can specify the source type, and lists of Amazon Redshift source IDs,
* event categories, and event severities. Notifications will be sent for
* all events you want that match those criteria. For example, you can
* specify source type = cluster, source ID = my-cluster-1 and mycluster2,
* event categories = Availability, Backup, and severity = ERROR. The
* subscription will only send notifications for those ERROR events in the
* Availability and Backup categories for the specified clusters.
*
*
* If you specify both the source type and source IDs, such as source type =
* cluster and source identifier = my-cluster-1, notifications will be sent
* for all the cluster events for my-cluster-1. If you specify a source type
* but do not specify a source identifier, you will receive notice of the
* events for the objects of that type in your AWS account. If you do not
* specify either the SourceType nor the SourceIdentifier, you will be
* notified of events generated from all Amazon Redshift sources belonging
* to your AWS account. You must specify a source type if you specify a
* source ID.
*
*
* @param createEventSubscriptionRequest
* @return A Java Future containing the result of the
* CreateEventSubscription operation returned by the service.
* @sample AmazonRedshiftAsync.CreateEventSubscription
*/
java.util.concurrent.Future createEventSubscriptionAsync(
CreateEventSubscriptionRequest createEventSubscriptionRequest);
/**
*
* Creates an Amazon Redshift event notification subscription. This action
* requires an ARN (Amazon Resource Name) of an Amazon SNS topic created by
* either the Amazon Redshift console, the Amazon SNS console, or the Amazon
* SNS API. To obtain an ARN with Amazon SNS, you must create a topic in
* Amazon SNS and subscribe to the topic. The ARN is displayed in the SNS
* console.
*
*
* You can specify the source type, and lists of Amazon Redshift source IDs,
* event categories, and event severities. Notifications will be sent for
* all events you want that match those criteria. For example, you can
* specify source type = cluster, source ID = my-cluster-1 and mycluster2,
* event categories = Availability, Backup, and severity = ERROR. The
* subscription will only send notifications for those ERROR events in the
* Availability and Backup categories for the specified clusters.
*
*
* If you specify both the source type and source IDs, such as source type =
* cluster and source identifier = my-cluster-1, notifications will be sent
* for all the cluster events for my-cluster-1. If you specify a source type
* but do not specify a source identifier, you will receive notice of the
* events for the objects of that type in your AWS account. If you do not
* specify either the SourceType nor the SourceIdentifier, you will be
* notified of events generated from all Amazon Redshift sources belonging
* to your AWS account. You must specify a source type if you specify a
* source ID.
*
*
* @param createEventSubscriptionRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the
* request. Users can provide an implementation of the callback
* methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the
* CreateEventSubscription operation returned by the service.
* @sample AmazonRedshiftAsyncHandler.CreateEventSubscription
*/
java.util.concurrent.Future createEventSubscriptionAsync(
CreateEventSubscriptionRequest createEventSubscriptionRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates an HSM client certificate that an Amazon Redshift cluster will
* use to connect to the client's HSM in order to store and retrieve the
* keys used to encrypt the cluster databases.
*
*
* The command returns a public key, which you must store in the HSM. In
* addition to creating the HSM certificate, you must create an Amazon
* Redshift HSM configuration that provides a cluster the information needed
* to store and use encryption keys in the HSM. For more information, go to
* Hardware Security Modules in the Amazon Redshift Cluster Management
* Guide.
*
*
* @param createHsmClientCertificateRequest
* @return A Java Future containing the result of the
* CreateHsmClientCertificate operation returned by the service.
* @sample AmazonRedshiftAsync.CreateHsmClientCertificate
*/
java.util.concurrent.Future createHsmClientCertificateAsync(
CreateHsmClientCertificateRequest createHsmClientCertificateRequest);
/**
*
* Creates an HSM client certificate that an Amazon Redshift cluster will
* use to connect to the client's HSM in order to store and retrieve the
* keys used to encrypt the cluster databases.
*
*
* The command returns a public key, which you must store in the HSM. In
* addition to creating the HSM certificate, you must create an Amazon
* Redshift HSM configuration that provides a cluster the information needed
* to store and use encryption keys in the HSM. For more information, go to
* Hardware Security Modules in the Amazon Redshift Cluster Management
* Guide.
*
*
* @param createHsmClientCertificateRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the
* request. Users can provide an implementation of the callback
* methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the
* CreateHsmClientCertificate operation returned by the service.
* @sample AmazonRedshiftAsyncHandler.CreateHsmClientCertificate
*/
java.util.concurrent.Future createHsmClientCertificateAsync(
CreateHsmClientCertificateRequest createHsmClientCertificateRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates an HSM configuration that contains the information required by an
* Amazon Redshift cluster to store and use database encryption keys in a
* Hardware Security Module (HSM). After creating the HSM configuration, you
* can specify it as a parameter when creating a cluster. The cluster will
* then store its encryption keys in the HSM.
*
*
* In addition to creating an HSM configuration, you must also create an HSM
* client certificate. For more information, go to Hardware Security Modules in the Amazon Redshift Cluster Management
* Guide.
*
*
* @param createHsmConfigurationRequest
* @return A Java Future containing the result of the CreateHsmConfiguration
* operation returned by the service.
* @sample AmazonRedshiftAsync.CreateHsmConfiguration
*/
java.util.concurrent.Future createHsmConfigurationAsync(
CreateHsmConfigurationRequest createHsmConfigurationRequest);
/**
*
* Creates an HSM configuration that contains the information required by an
* Amazon Redshift cluster to store and use database encryption keys in a
* Hardware Security Module (HSM). After creating the HSM configuration, you
* can specify it as a parameter when creating a cluster. The cluster will
* then store its encryption keys in the HSM.
*
*
* In addition to creating an HSM configuration, you must also create an HSM
* client certificate. For more information, go to Hardware Security Modules in the Amazon Redshift Cluster Management
* Guide.
*
*
* @param createHsmConfigurationRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the
* request. Users can provide an implementation of the callback
* methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateHsmConfiguration
* operation returned by the service.
* @sample AmazonRedshiftAsyncHandler.CreateHsmConfiguration
*/
java.util.concurrent.Future createHsmConfigurationAsync(
CreateHsmConfigurationRequest createHsmConfigurationRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates a snapshot copy grant that permits Amazon Redshift to use a
* customer master key (CMK) from AWS Key Management Service (AWS KMS) to
* encrypt copied snapshots in a destination region.
*
*
* For more information about managing snapshot copy grants, go to Amazon Redshift Database Encryption in the Amazon Redshift
* Cluster Management Guide.
*
*
* @param createSnapshotCopyGrantRequest
* The result of the CreateSnapshotCopyGrant
action.
* @return A Java Future containing the result of the
* CreateSnapshotCopyGrant operation returned by the service.
* @sample AmazonRedshiftAsync.CreateSnapshotCopyGrant
*/
java.util.concurrent.Future createSnapshotCopyGrantAsync(
CreateSnapshotCopyGrantRequest createSnapshotCopyGrantRequest);
/**
*
* Creates a snapshot copy grant that permits Amazon Redshift to use a
* customer master key (CMK) from AWS Key Management Service (AWS KMS) to
* encrypt copied snapshots in a destination region.
*
*
* For more information about managing snapshot copy grants, go to Amazon Redshift Database Encryption in the Amazon Redshift
* Cluster Management Guide.
*
*
* @param createSnapshotCopyGrantRequest
* The result of the CreateSnapshotCopyGrant
action.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the
* request. Users can provide an implementation of the callback
* methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the
* CreateSnapshotCopyGrant operation returned by the service.
* @sample AmazonRedshiftAsyncHandler.CreateSnapshotCopyGrant
*/
java.util.concurrent.Future createSnapshotCopyGrantAsync(
CreateSnapshotCopyGrantRequest createSnapshotCopyGrantRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Adds one or more tags to a specified resource.
*
*
* A resource can have up to 10 tags. If you try to create more than 10 tags
* for a resource, you will receive an error and the attempt will fail.
*
*
* If you specify a key that already exists for the resource, the value for
* that key will be updated with the new value.
*
*
* @param createTagsRequest
* Contains the output from the CreateTags
action.
* @return A Java Future containing the result of the CreateTags operation
* returned by the service.
* @sample AmazonRedshiftAsync.CreateTags
*/
java.util.concurrent.Future createTagsAsync(
CreateTagsRequest createTagsRequest);
/**
*
* Adds one or more tags to a specified resource.
*
*
* A resource can have up to 10 tags. If you try to create more than 10 tags
* for a resource, you will receive an error and the attempt will fail.
*
*
* If you specify a key that already exists for the resource, the value for
* that key will be updated with the new value.
*
*
* @param createTagsRequest
* Contains the output from the CreateTags
action.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the
* request. Users can provide an implementation of the callback
* methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateTags operation
* returned by the service.
* @sample AmazonRedshiftAsyncHandler.CreateTags
*/
java.util.concurrent.Future createTagsAsync(
CreateTagsRequest createTagsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deletes a previously provisioned cluster. A successful response from the
* web service indicates that the request was received correctly. Use
* DescribeClusters to monitor the status of the deletion. The delete
* operation cannot be canceled or reverted once submitted. For more
* information about managing clusters, go to Amazon Redshift Clusters in the Amazon Redshift Cluster
* Management Guide .
*
*
* If you want to shut down the cluster and retain it for future use, set
* SkipFinalClusterSnapshot to false
and specify a name
* for FinalClusterSnapshotIdentifier. You can later restore this
* snapshot to resume using the cluster. If a final cluster snapshot is
* requested, the status of the cluster will be "final-snapshot" while the
* snapshot is being taken, then it's "deleting" once Amazon Redshift begins
* deleting the cluster.
*
*
* For more information about managing clusters, go to Amazon Redshift Clusters in the Amazon Redshift Cluster
* Management Guide .
*
*
* @param deleteClusterRequest
* @return A Java Future containing the result of the DeleteCluster
* operation returned by the service.
* @sample AmazonRedshiftAsync.DeleteCluster
*/
java.util.concurrent.Future deleteClusterAsync(
DeleteClusterRequest deleteClusterRequest);
/**
*
* Deletes a previously provisioned cluster. A successful response from the
* web service indicates that the request was received correctly. Use
* DescribeClusters to monitor the status of the deletion. The delete
* operation cannot be canceled or reverted once submitted. For more
* information about managing clusters, go to Amazon Redshift Clusters in the Amazon Redshift Cluster
* Management Guide .
*
*
* If you want to shut down the cluster and retain it for future use, set
* SkipFinalClusterSnapshot to false
and specify a name
* for FinalClusterSnapshotIdentifier. You can later restore this
* snapshot to resume using the cluster. If a final cluster snapshot is
* requested, the status of the cluster will be "final-snapshot" while the
* snapshot is being taken, then it's "deleting" once Amazon Redshift begins
* deleting the cluster.
*
*
* For more information about managing clusters, go to Amazon Redshift Clusters in the Amazon Redshift Cluster
* Management Guide .
*
*
* @param deleteClusterRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the
* request. Users can provide an implementation of the callback
* methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteCluster
* operation returned by the service.
* @sample AmazonRedshiftAsyncHandler.DeleteCluster
*/
java.util.concurrent.Future deleteClusterAsync(
DeleteClusterRequest deleteClusterRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deletes a specified Amazon Redshift parameter group. You cannot
* delete a parameter group if it is associated with a cluster.
*
*
* @param deleteClusterParameterGroupRequest
* @return A Java Future containing the result of the
* DeleteClusterParameterGroup operation returned by the service.
* @sample AmazonRedshiftAsync.DeleteClusterParameterGroup
*/
java.util.concurrent.Future deleteClusterParameterGroupAsync(
DeleteClusterParameterGroupRequest deleteClusterParameterGroupRequest);
/**
*
* Deletes a specified Amazon Redshift parameter group. You cannot
* delete a parameter group if it is associated with a cluster.
*
*
* @param deleteClusterParameterGroupRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the
* request. Users can provide an implementation of the callback
* methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the
* DeleteClusterParameterGroup operation returned by the service.
* @sample AmazonRedshiftAsyncHandler.DeleteClusterParameterGroup
*/
java.util.concurrent.Future deleteClusterParameterGroupAsync(
DeleteClusterParameterGroupRequest deleteClusterParameterGroupRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deletes an Amazon Redshift security group.
*
* You cannot delete a security group that is associated with any
* clusters. You cannot delete the default security group.
*
* For information about managing security groups, go to Amazon Redshift Cluster Security Groups in the Amazon Redshift
* Cluster Management Guide.
*
*
* @param deleteClusterSecurityGroupRequest
* @return A Java Future containing the result of the
* DeleteClusterSecurityGroup operation returned by the service.
* @sample AmazonRedshiftAsync.DeleteClusterSecurityGroup
*/
java.util.concurrent.Future deleteClusterSecurityGroupAsync(
DeleteClusterSecurityGroupRequest deleteClusterSecurityGroupRequest);
/**
*
* Deletes an Amazon Redshift security group.
*
* You cannot delete a security group that is associated with any
* clusters. You cannot delete the default security group.
*
* For information about managing security groups, go to Amazon Redshift Cluster Security Groups in the Amazon Redshift
* Cluster Management Guide.
*
*
* @param deleteClusterSecurityGroupRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the
* request. Users can provide an implementation of the callback
* methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the
* DeleteClusterSecurityGroup operation returned by the service.
* @sample AmazonRedshiftAsyncHandler.DeleteClusterSecurityGroup
*/
java.util.concurrent.Future deleteClusterSecurityGroupAsync(
DeleteClusterSecurityGroupRequest deleteClusterSecurityGroupRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deletes the specified manual snapshot. The snapshot must be in the
* available
state, with no other users authorized to access
* the snapshot.
*
*
* Unlike automated snapshots, manual snapshots are retained even after you
* delete your cluster. Amazon Redshift does not delete your manual
* snapshots. You must delete manual snapshot explicitly to avoid getting
* charged. If other accounts are authorized to access the snapshot, you
* must revoke all of the authorizations before you can delete the snapshot.
*
*
* @param deleteClusterSnapshotRequest
* @return A Java Future containing the result of the DeleteClusterSnapshot
* operation returned by the service.
* @sample AmazonRedshiftAsync.DeleteClusterSnapshot
*/
java.util.concurrent.Future deleteClusterSnapshotAsync(
DeleteClusterSnapshotRequest deleteClusterSnapshotRequest);
/**
*
* Deletes the specified manual snapshot. The snapshot must be in the
* available
state, with no other users authorized to access
* the snapshot.
*
*
* Unlike automated snapshots, manual snapshots are retained even after you
* delete your cluster. Amazon Redshift does not delete your manual
* snapshots. You must delete manual snapshot explicitly to avoid getting
* charged. If other accounts are authorized to access the snapshot, you
* must revoke all of the authorizations before you can delete the snapshot.
*
*
* @param deleteClusterSnapshotRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the
* request. Users can provide an implementation of the callback
* methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteClusterSnapshot
* operation returned by the service.
* @sample AmazonRedshiftAsyncHandler.DeleteClusterSnapshot
*/
java.util.concurrent.Future deleteClusterSnapshotAsync(
DeleteClusterSnapshotRequest deleteClusterSnapshotRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deletes the specified cluster subnet group.
*
*
* @param deleteClusterSubnetGroupRequest
* @return A Java Future containing the result of the
* DeleteClusterSubnetGroup operation returned by the service.
* @sample AmazonRedshiftAsync.DeleteClusterSubnetGroup
*/
java.util.concurrent.Future deleteClusterSubnetGroupAsync(
DeleteClusterSubnetGroupRequest deleteClusterSubnetGroupRequest);
/**
*
* Deletes the specified cluster subnet group.
*
*
* @param deleteClusterSubnetGroupRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the
* request. Users can provide an implementation of the callback
* methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the
* DeleteClusterSubnetGroup operation returned by the service.
* @sample AmazonRedshiftAsyncHandler.DeleteClusterSubnetGroup
*/
java.util.concurrent.Future deleteClusterSubnetGroupAsync(
DeleteClusterSubnetGroupRequest deleteClusterSubnetGroupRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deletes an Amazon Redshift event notification subscription.
*
*
* @param deleteEventSubscriptionRequest
* @return A Java Future containing the result of the
* DeleteEventSubscription operation returned by the service.
* @sample AmazonRedshiftAsync.DeleteEventSubscription
*/
java.util.concurrent.Future deleteEventSubscriptionAsync(
DeleteEventSubscriptionRequest deleteEventSubscriptionRequest);
/**
*
* Deletes an Amazon Redshift event notification subscription.
*
*
* @param deleteEventSubscriptionRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the
* request. Users can provide an implementation of the callback
* methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the
* DeleteEventSubscription operation returned by the service.
* @sample AmazonRedshiftAsyncHandler.DeleteEventSubscription
*/
java.util.concurrent.Future deleteEventSubscriptionAsync(
DeleteEventSubscriptionRequest deleteEventSubscriptionRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deletes the specified HSM client certificate.
*
*
* @param deleteHsmClientCertificateRequest
* @return A Java Future containing the result of the
* DeleteHsmClientCertificate operation returned by the service.
* @sample AmazonRedshiftAsync.DeleteHsmClientCertificate
*/
java.util.concurrent.Future deleteHsmClientCertificateAsync(
DeleteHsmClientCertificateRequest deleteHsmClientCertificateRequest);
/**
*
* Deletes the specified HSM client certificate.
*
*
* @param deleteHsmClientCertificateRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the
* request. Users can provide an implementation of the callback
* methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the
* DeleteHsmClientCertificate operation returned by the service.
* @sample AmazonRedshiftAsyncHandler.DeleteHsmClientCertificate
*/
java.util.concurrent.Future deleteHsmClientCertificateAsync(
DeleteHsmClientCertificateRequest deleteHsmClientCertificateRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deletes the specified Amazon Redshift HSM configuration.
*
*
* @param deleteHsmConfigurationRequest
* @return A Java Future containing the result of the DeleteHsmConfiguration
* operation returned by the service.
* @sample AmazonRedshiftAsync.DeleteHsmConfiguration
*/
java.util.concurrent.Future deleteHsmConfigurationAsync(
DeleteHsmConfigurationRequest deleteHsmConfigurationRequest);
/**
*
* Deletes the specified Amazon Redshift HSM configuration.
*
*
* @param deleteHsmConfigurationRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the
* request. Users can provide an implementation of the callback
* methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteHsmConfiguration
* operation returned by the service.
* @sample AmazonRedshiftAsyncHandler.DeleteHsmConfiguration
*/
java.util.concurrent.Future deleteHsmConfigurationAsync(
DeleteHsmConfigurationRequest deleteHsmConfigurationRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deletes the specified snapshot copy grant.
*
*
* @param deleteSnapshotCopyGrantRequest
* The result of the DeleteSnapshotCopyGrant
action.
* @return A Java Future containing the result of the
* DeleteSnapshotCopyGrant operation returned by the service.
* @sample AmazonRedshiftAsync.DeleteSnapshotCopyGrant
*/
java.util.concurrent.Future deleteSnapshotCopyGrantAsync(
DeleteSnapshotCopyGrantRequest deleteSnapshotCopyGrantRequest);
/**
*
* Deletes the specified snapshot copy grant.
*
*
* @param deleteSnapshotCopyGrantRequest
* The result of the DeleteSnapshotCopyGrant
action.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the
* request. Users can provide an implementation of the callback
* methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the
* DeleteSnapshotCopyGrant operation returned by the service.
* @sample AmazonRedshiftAsyncHandler.DeleteSnapshotCopyGrant
*/
java.util.concurrent.Future deleteSnapshotCopyGrantAsync(
DeleteSnapshotCopyGrantRequest deleteSnapshotCopyGrantRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deletes a tag or tags from a resource. You must provide the ARN of the
* resource from which you want to delete the tag or tags.
*
*
* @param deleteTagsRequest
* Contains the output from the DeleteTags
action.
* @return A Java Future containing the result of the DeleteTags operation
* returned by the service.
* @sample AmazonRedshiftAsync.DeleteTags
*/
java.util.concurrent.Future deleteTagsAsync(
DeleteTagsRequest deleteTagsRequest);
/**
*
* Deletes a tag or tags from a resource. You must provide the ARN of the
* resource from which you want to delete the tag or tags.
*
*
* @param deleteTagsRequest
* Contains the output from the DeleteTags
action.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the
* request. Users can provide an implementation of the callback
* methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteTags operation
* returned by the service.
* @sample AmazonRedshiftAsyncHandler.DeleteTags
*/
java.util.concurrent.Future deleteTagsAsync(
DeleteTagsRequest deleteTagsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns a list of Amazon Redshift parameter groups, including parameter
* groups you created and the default parameter group. For each parameter
* group, the response includes the parameter group name, description, and
* parameter group family name. You can optionally specify a name to
* retrieve the description of a specific parameter group.
*
*
* For more information about parameters and parameter groups, go to Amazon Redshift Parameter Groups in the Amazon Redshift Cluster
* Management Guide.
*
*
* If you specify both tag keys and tag values in the same request, Amazon
* Redshift returns all parameter groups that match any combination of the
* specified keys and values. For example, if you have owner
* and environment
for tag keys, and admin
and
* test
for tag values, all parameter groups that have any
* combination of those values are returned.
*
*
* If both tag keys and values are omitted from the request, parameter
* groups are returned regardless of whether they have tag keys or values
* associated with them.
*
*
* @param describeClusterParameterGroupsRequest
* @return A Java Future containing the result of the
* DescribeClusterParameterGroups operation returned by the service.
* @sample AmazonRedshiftAsync.DescribeClusterParameterGroups
*/
java.util.concurrent.Future describeClusterParameterGroupsAsync(
DescribeClusterParameterGroupsRequest describeClusterParameterGroupsRequest);
/**
*
* Returns a list of Amazon Redshift parameter groups, including parameter
* groups you created and the default parameter group. For each parameter
* group, the response includes the parameter group name, description, and
* parameter group family name. You can optionally specify a name to
* retrieve the description of a specific parameter group.
*
*
* For more information about parameters and parameter groups, go to Amazon Redshift Parameter Groups in the Amazon Redshift Cluster
* Management Guide.
*
*
* If you specify both tag keys and tag values in the same request, Amazon
* Redshift returns all parameter groups that match any combination of the
* specified keys and values. For example, if you have owner
* and environment
for tag keys, and admin
and
* test
for tag values, all parameter groups that have any
* combination of those values are returned.
*
*
* If both tag keys and values are omitted from the request, parameter
* groups are returned regardless of whether they have tag keys or values
* associated with them.
*
*
* @param describeClusterParameterGroupsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the
* request. Users can provide an implementation of the callback
* methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the
* DescribeClusterParameterGroups operation returned by the service.
* @sample AmazonRedshiftAsyncHandler.DescribeClusterParameterGroups
*/
java.util.concurrent.Future describeClusterParameterGroupsAsync(
DescribeClusterParameterGroupsRequest describeClusterParameterGroupsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
* Simplified method form for invoking the DescribeClusterParameterGroups
* operation.
*
* @see #describeClusterParameterGroupsAsync(DescribeClusterParameterGroupsRequest)
*/
java.util.concurrent.Future describeClusterParameterGroupsAsync();
/**
* Simplified method form for invoking the DescribeClusterParameterGroups
* operation with an AsyncHandler.
*
* @see #describeClusterParameterGroupsAsync(DescribeClusterParameterGroupsRequest,
* com.amazonaws.handlers.AsyncHandler)
*/
java.util.concurrent.Future describeClusterParameterGroupsAsync(
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns a detailed list of parameters contained within the specified
* Amazon Redshift parameter group. For each parameter the response includes
* information such as parameter name, description, data type, value,
* whether the parameter value is modifiable, and so on.
*
*
* You can specify source filter to retrieve parameters of only
* specific type. For example, to retrieve parameters that were modified by
* a user action such as from ModifyClusterParameterGroup, you can
* specify source equal to user.
*
*
* For more information about parameters and parameter groups, go to Amazon Redshift Parameter Groups in the Amazon Redshift Cluster
* Management Guide.
*
*
* @param describeClusterParametersRequest
* @return A Java Future containing the result of the
* DescribeClusterParameters operation returned by the service.
* @sample AmazonRedshiftAsync.DescribeClusterParameters
*/
java.util.concurrent.Future describeClusterParametersAsync(
DescribeClusterParametersRequest describeClusterParametersRequest);
/**
*
* Returns a detailed list of parameters contained within the specified
* Amazon Redshift parameter group. For each parameter the response includes
* information such as parameter name, description, data type, value,
* whether the parameter value is modifiable, and so on.
*
*
* You can specify source filter to retrieve parameters of only
* specific type. For example, to retrieve parameters that were modified by
* a user action such as from ModifyClusterParameterGroup, you can
* specify source equal to user.
*
*
* For more information about parameters and parameter groups, go to Amazon Redshift Parameter Groups in the Amazon Redshift Cluster
* Management Guide.
*
*
* @param describeClusterParametersRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the
* request. Users can provide an implementation of the callback
* methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the
* DescribeClusterParameters operation returned by the service.
* @sample AmazonRedshiftAsyncHandler.DescribeClusterParameters
*/
java.util.concurrent.Future describeClusterParametersAsync(
DescribeClusterParametersRequest describeClusterParametersRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns information about Amazon Redshift security groups. If the name of
* a security group is specified, the response will contain only information
* about only that security group.
*
*
* For information about managing security groups, go to Amazon Redshift Cluster Security Groups in the Amazon Redshift
* Cluster Management Guide.
*
*
* If you specify both tag keys and tag values in the same request, Amazon
* Redshift returns all security groups that match any combination of the
* specified keys and values. For example, if you have owner
* and environment
for tag keys, and admin
and
* test
for tag values, all security groups that have any
* combination of those values are returned.
*
*
* If both tag keys and values are omitted from the request, security groups
* are returned regardless of whether they have tag keys or values
* associated with them.
*
*
* @param describeClusterSecurityGroupsRequest
* @return A Java Future containing the result of the
* DescribeClusterSecurityGroups operation returned by the service.
* @sample AmazonRedshiftAsync.DescribeClusterSecurityGroups
*/
java.util.concurrent.Future describeClusterSecurityGroupsAsync(
DescribeClusterSecurityGroupsRequest describeClusterSecurityGroupsRequest);
/**
*
* Returns information about Amazon Redshift security groups. If the name of
* a security group is specified, the response will contain only information
* about only that security group.
*
*
* For information about managing security groups, go to Amazon Redshift Cluster Security Groups in the Amazon Redshift
* Cluster Management Guide.
*
*
* If you specify both tag keys and tag values in the same request, Amazon
* Redshift returns all security groups that match any combination of the
* specified keys and values. For example, if you have owner
* and environment
for tag keys, and admin
and
* test
for tag values, all security groups that have any
* combination of those values are returned.
*
*
* If both tag keys and values are omitted from the request, security groups
* are returned regardless of whether they have tag keys or values
* associated with them.
*
*
* @param describeClusterSecurityGroupsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the
* request. Users can provide an implementation of the callback
* methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the
* DescribeClusterSecurityGroups operation returned by the service.
* @sample AmazonRedshiftAsyncHandler.DescribeClusterSecurityGroups
*/
java.util.concurrent.Future describeClusterSecurityGroupsAsync(
DescribeClusterSecurityGroupsRequest describeClusterSecurityGroupsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
* Simplified method form for invoking the DescribeClusterSecurityGroups
* operation.
*
* @see #describeClusterSecurityGroupsAsync(DescribeClusterSecurityGroupsRequest)
*/
java.util.concurrent.Future describeClusterSecurityGroupsAsync();
/**
* Simplified method form for invoking the DescribeClusterSecurityGroups
* operation with an AsyncHandler.
*
* @see #describeClusterSecurityGroupsAsync(DescribeClusterSecurityGroupsRequest,
* com.amazonaws.handlers.AsyncHandler)
*/
java.util.concurrent.Future describeClusterSecurityGroupsAsync(
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns one or more snapshot objects, which contain metadata about your
* cluster snapshots. By default, this operation returns information about
* all snapshots of all clusters that are owned by you AWS customer account.
* No information is returned for snapshots owned by inactive AWS customer
* accounts.
*
*
* If you specify both tag keys and tag values in the same request, Amazon
* Redshift returns all snapshots that match any combination of the
* specified keys and values. For example, if you have owner
* and environment
for tag keys, and admin
and
* test
for tag values, all snapshots that have any combination
* of those values are returned. Only snapshots that you own are returned in
* the response; shared snapshots are not returned with the tag key and tag
* value request parameters.
*
*
* If both tag keys and values are omitted from the request, snapshots are
* returned regardless of whether they have tag keys or values associated
* with them.
*
*
* @param describeClusterSnapshotsRequest
* @return A Java Future containing the result of the
* DescribeClusterSnapshots operation returned by the service.
* @sample AmazonRedshiftAsync.DescribeClusterSnapshots
*/
java.util.concurrent.Future describeClusterSnapshotsAsync(
DescribeClusterSnapshotsRequest describeClusterSnapshotsRequest);
/**
*
* Returns one or more snapshot objects, which contain metadata about your
* cluster snapshots. By default, this operation returns information about
* all snapshots of all clusters that are owned by you AWS customer account.
* No information is returned for snapshots owned by inactive AWS customer
* accounts.
*
*
* If you specify both tag keys and tag values in the same request, Amazon
* Redshift returns all snapshots that match any combination of the
* specified keys and values. For example, if you have owner
* and environment
for tag keys, and admin
and
* test
for tag values, all snapshots that have any combination
* of those values are returned. Only snapshots that you own are returned in
* the response; shared snapshots are not returned with the tag key and tag
* value request parameters.
*
*
* If both tag keys and values are omitted from the request, snapshots are
* returned regardless of whether they have tag keys or values associated
* with them.
*
*
* @param describeClusterSnapshotsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the
* request. Users can provide an implementation of the callback
* methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the
* DescribeClusterSnapshots operation returned by the service.
* @sample AmazonRedshiftAsyncHandler.DescribeClusterSnapshots
*/
java.util.concurrent.Future describeClusterSnapshotsAsync(
DescribeClusterSnapshotsRequest describeClusterSnapshotsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
* Simplified method form for invoking the DescribeClusterSnapshots
* operation.
*
* @see #describeClusterSnapshotsAsync(DescribeClusterSnapshotsRequest)
*/
java.util.concurrent.Future describeClusterSnapshotsAsync();
/**
* Simplified method form for invoking the DescribeClusterSnapshots
* operation with an AsyncHandler.
*
* @see #describeClusterSnapshotsAsync(DescribeClusterSnapshotsRequest,
* com.amazonaws.handlers.AsyncHandler)
*/
java.util.concurrent.Future describeClusterSnapshotsAsync(
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns one or more cluster subnet group objects, which contain metadata
* about your cluster subnet groups. By default, this operation returns
* information about all cluster subnet groups that are defined in you AWS
* account.
*
*
* If you specify both tag keys and tag values in the same request, Amazon
* Redshift returns all subnet groups that match any combination of the
* specified keys and values. For example, if you have owner
* and environment
for tag keys, and admin
and
* test
for tag values, all subnet groups that have any
* combination of those values are returned.
*
*
* If both tag keys and values are omitted from the request, subnet groups
* are returned regardless of whether they have tag keys or values
* associated with them.
*
*
* @param describeClusterSubnetGroupsRequest
* @return A Java Future containing the result of the
* DescribeClusterSubnetGroups operation returned by the service.
* @sample AmazonRedshiftAsync.DescribeClusterSubnetGroups
*/
java.util.concurrent.Future describeClusterSubnetGroupsAsync(
DescribeClusterSubnetGroupsRequest describeClusterSubnetGroupsRequest);
/**
*
* Returns one or more cluster subnet group objects, which contain metadata
* about your cluster subnet groups. By default, this operation returns
* information about all cluster subnet groups that are defined in you AWS
* account.
*
*
* If you specify both tag keys and tag values in the same request, Amazon
* Redshift returns all subnet groups that match any combination of the
* specified keys and values. For example, if you have owner
* and environment
for tag keys, and admin
and
* test
for tag values, all subnet groups that have any
* combination of those values are returned.
*
*
* If both tag keys and values are omitted from the request, subnet groups
* are returned regardless of whether they have tag keys or values
* associated with them.
*
*
* @param describeClusterSubnetGroupsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the
* request. Users can provide an implementation of the callback
* methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the
* DescribeClusterSubnetGroups operation returned by the service.
* @sample AmazonRedshiftAsyncHandler.DescribeClusterSubnetGroups
*/
java.util.concurrent.Future describeClusterSubnetGroupsAsync(
DescribeClusterSubnetGroupsRequest describeClusterSubnetGroupsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
* Simplified method form for invoking the DescribeClusterSubnetGroups
* operation.
*
* @see #describeClusterSubnetGroupsAsync(DescribeClusterSubnetGroupsRequest)
*/
java.util.concurrent.Future describeClusterSubnetGroupsAsync();
/**
* Simplified method form for invoking the DescribeClusterSubnetGroups
* operation with an AsyncHandler.
*
* @see #describeClusterSubnetGroupsAsync(DescribeClusterSubnetGroupsRequest,
* com.amazonaws.handlers.AsyncHandler)
*/
java.util.concurrent.Future describeClusterSubnetGroupsAsync(
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns descriptions of the available Amazon Redshift cluster versions.
* You can call this operation even before creating any clusters to learn
* more about the Amazon Redshift versions. For more information about
* managing clusters, go to Amazon Redshift Clusters in the Amazon Redshift Cluster
* Management Guide
*
*
* @param describeClusterVersionsRequest
* @return A Java Future containing the result of the
* DescribeClusterVersions operation returned by the service.
* @sample AmazonRedshiftAsync.DescribeClusterVersions
*/
java.util.concurrent.Future describeClusterVersionsAsync(
DescribeClusterVersionsRequest describeClusterVersionsRequest);
/**
*
* Returns descriptions of the available Amazon Redshift cluster versions.
* You can call this operation even before creating any clusters to learn
* more about the Amazon Redshift versions. For more information about
* managing clusters, go to Amazon Redshift Clusters in the Amazon Redshift Cluster
* Management Guide
*
*
* @param describeClusterVersionsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the
* request. Users can provide an implementation of the callback
* methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the
* DescribeClusterVersions operation returned by the service.
* @sample AmazonRedshiftAsyncHandler.DescribeClusterVersions
*/
java.util.concurrent.Future describeClusterVersionsAsync(
DescribeClusterVersionsRequest describeClusterVersionsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
* Simplified method form for invoking the DescribeClusterVersions
* operation.
*
* @see #describeClusterVersionsAsync(DescribeClusterVersionsRequest)
*/
java.util.concurrent.Future describeClusterVersionsAsync();
/**
* Simplified method form for invoking the DescribeClusterVersions operation
* with an AsyncHandler.
*
* @see #describeClusterVersionsAsync(DescribeClusterVersionsRequest,
* com.amazonaws.handlers.AsyncHandler)
*/
java.util.concurrent.Future describeClusterVersionsAsync(
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns properties of provisioned clusters including general cluster
* properties, cluster database properties, maintenance and backup
* properties, and security and access properties. This operation supports
* pagination. For more information about managing clusters, go to Amazon Redshift Clusters in the Amazon Redshift Cluster
* Management Guide .
*
*
* If you specify both tag keys and tag values in the same request, Amazon
* Redshift returns all clusters that match any combination of the specified
* keys and values. For example, if you have owner
and
* environment
for tag keys, and admin
and
* test
for tag values, all clusters that have any combination
* of those values are returned.
*
*
* If both tag keys and values are omitted from the request, clusters are
* returned regardless of whether they have tag keys or values associated
* with them.
*
*
* @param describeClustersRequest
* @return A Java Future containing the result of the DescribeClusters
* operation returned by the service.
* @sample AmazonRedshiftAsync.DescribeClusters
*/
java.util.concurrent.Future describeClustersAsync(
DescribeClustersRequest describeClustersRequest);
/**
*
* Returns properties of provisioned clusters including general cluster
* properties, cluster database properties, maintenance and backup
* properties, and security and access properties. This operation supports
* pagination. For more information about managing clusters, go to Amazon Redshift Clusters in the Amazon Redshift Cluster
* Management Guide .
*
*
* If you specify both tag keys and tag values in the same request, Amazon
* Redshift returns all clusters that match any combination of the specified
* keys and values. For example, if you have owner
and
* environment
for tag keys, and admin
and
* test
for tag values, all clusters that have any combination
* of those values are returned.
*
*
* If both tag keys and values are omitted from the request, clusters are
* returned regardless of whether they have tag keys or values associated
* with them.
*
*
* @param describeClustersRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the
* request. Users can provide an implementation of the callback
* methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeClusters
* operation returned by the service.
* @sample AmazonRedshiftAsyncHandler.DescribeClusters
*/
java.util.concurrent.Future describeClustersAsync(
DescribeClustersRequest describeClustersRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
* Simplified method form for invoking the DescribeClusters operation.
*
* @see #describeClustersAsync(DescribeClustersRequest)
*/
java.util.concurrent.Future describeClustersAsync();
/**
* Simplified method form for invoking the DescribeClusters operation with
* an AsyncHandler.
*
* @see #describeClustersAsync(DescribeClustersRequest,
* com.amazonaws.handlers.AsyncHandler)
*/
java.util.concurrent.Future describeClustersAsync(
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns a list of parameter settings for the specified parameter group
* family.
*
*
* For more information about parameters and parameter groups, go to Amazon Redshift Parameter Groups in the Amazon Redshift Cluster
* Management Guide.
*
*
* @param describeDefaultClusterParametersRequest
* @return A Java Future containing the result of the
* DescribeDefaultClusterParameters operation returned by the
* service.
* @sample AmazonRedshiftAsync.DescribeDefaultClusterParameters
*/
java.util.concurrent.Future describeDefaultClusterParametersAsync(
DescribeDefaultClusterParametersRequest describeDefaultClusterParametersRequest);
/**
*
* Returns a list of parameter settings for the specified parameter group
* family.
*
*
* For more information about parameters and parameter groups, go to Amazon Redshift Parameter Groups in the Amazon Redshift Cluster
* Management Guide.
*
*
* @param describeDefaultClusterParametersRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the
* request. Users can provide an implementation of the callback
* methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the
* DescribeDefaultClusterParameters operation returned by the
* service.
* @sample AmazonRedshiftAsyncHandler.DescribeDefaultClusterParameters
*/
java.util.concurrent.Future describeDefaultClusterParametersAsync(
DescribeDefaultClusterParametersRequest describeDefaultClusterParametersRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Displays a list of event categories for all event source types, or for a
* specified source type. For a list of the event categories and source
* types, go to Amazon Redshift Event Notifications.
*
*
* @param describeEventCategoriesRequest
* @return A Java Future containing the result of the
* DescribeEventCategories operation returned by the service.
* @sample AmazonRedshiftAsync.DescribeEventCategories
*/
java.util.concurrent.Future describeEventCategoriesAsync(
DescribeEventCategoriesRequest describeEventCategoriesRequest);
/**
*
* Displays a list of event categories for all event source types, or for a
* specified source type. For a list of the event categories and source
* types, go to Amazon Redshift Event Notifications.
*
*
* @param describeEventCategoriesRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the
* request. Users can provide an implementation of the callback
* methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the
* DescribeEventCategories operation returned by the service.
* @sample AmazonRedshiftAsyncHandler.DescribeEventCategories
*/
java.util.concurrent.Future describeEventCategoriesAsync(
DescribeEventCategoriesRequest describeEventCategoriesRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
* Simplified method form for invoking the DescribeEventCategories
* operation.
*
* @see #describeEventCategoriesAsync(DescribeEventCategoriesRequest)
*/
java.util.concurrent.Future describeEventCategoriesAsync();
/**
* Simplified method form for invoking the DescribeEventCategories operation
* with an AsyncHandler.
*
* @see #describeEventCategoriesAsync(DescribeEventCategoriesRequest,
* com.amazonaws.handlers.AsyncHandler)
*/
java.util.concurrent.Future describeEventCategoriesAsync(
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Lists descriptions of all the Amazon Redshift event notifications
* subscription for a customer account. If you specify a subscription name,
* lists the description for that subscription.
*
*
* @param describeEventSubscriptionsRequest
* @return A Java Future containing the result of the
* DescribeEventSubscriptions operation returned by the service.
* @sample AmazonRedshiftAsync.DescribeEventSubscriptions
*/
java.util.concurrent.Future describeEventSubscriptionsAsync(
DescribeEventSubscriptionsRequest describeEventSubscriptionsRequest);
/**
*
* Lists descriptions of all the Amazon Redshift event notifications
* subscription for a customer account. If you specify a subscription name,
* lists the description for that subscription.
*
*
* @param describeEventSubscriptionsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the
* request. Users can provide an implementation of the callback
* methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the
* DescribeEventSubscriptions operation returned by the service.
* @sample AmazonRedshiftAsyncHandler.DescribeEventSubscriptions
*/
java.util.concurrent.Future describeEventSubscriptionsAsync(
DescribeEventSubscriptionsRequest describeEventSubscriptionsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
* Simplified method form for invoking the DescribeEventSubscriptions
* operation.
*
* @see #describeEventSubscriptionsAsync(DescribeEventSubscriptionsRequest)
*/
java.util.concurrent.Future describeEventSubscriptionsAsync();
/**
* Simplified method form for invoking the DescribeEventSubscriptions
* operation with an AsyncHandler.
*
* @see #describeEventSubscriptionsAsync(DescribeEventSubscriptionsRequest,
* com.amazonaws.handlers.AsyncHandler)
*/
java.util.concurrent.Future describeEventSubscriptionsAsync(
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns events related to clusters, security groups, snapshots, and
* parameter groups for the past 14 days. Events specific to a particular
* cluster, security group, snapshot or parameter group can be obtained by
* providing the name as a parameter. By default, the past hour of events
* are returned.
*
*
* @param describeEventsRequest
* @return A Java Future containing the result of the DescribeEvents
* operation returned by the service.
* @sample AmazonRedshiftAsync.DescribeEvents
*/
java.util.concurrent.Future describeEventsAsync(
DescribeEventsRequest describeEventsRequest);
/**
*
* Returns events related to clusters, security groups, snapshots, and
* parameter groups for the past 14 days. Events specific to a particular
* cluster, security group, snapshot or parameter group can be obtained by
* providing the name as a parameter. By default, the past hour of events
* are returned.
*
*
* @param describeEventsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the
* request. Users can provide an implementation of the callback
* methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeEvents
* operation returned by the service.
* @sample AmazonRedshiftAsyncHandler.DescribeEvents
*/
java.util.concurrent.Future describeEventsAsync(
DescribeEventsRequest describeEventsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
* Simplified method form for invoking the DescribeEvents operation.
*
* @see #describeEventsAsync(DescribeEventsRequest)
*/
java.util.concurrent.Future describeEventsAsync();
/**
* Simplified method form for invoking the DescribeEvents operation with an
* AsyncHandler.
*
* @see #describeEventsAsync(DescribeEventsRequest,
* com.amazonaws.handlers.AsyncHandler)
*/
java.util.concurrent.Future describeEventsAsync(
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns information about the specified HSM client certificate. If no
* certificate ID is specified, returns information about all the HSM
* certificates owned by your AWS customer account.
*
*
* If you specify both tag keys and tag values in the same request, Amazon
* Redshift returns all HSM client certificates that match any combination
* of the specified keys and values. For example, if you have
* owner
and environment
for tag keys, and
* admin
and test
for tag values, all HSM client
* certificates that have any combination of those values are returned.
*
*
* If both tag keys and values are omitted from the request, HSM client
* certificates are returned regardless of whether they have tag keys or
* values associated with them.
*
*
* @param describeHsmClientCertificatesRequest
* @return A Java Future containing the result of the
* DescribeHsmClientCertificates operation returned by the service.
* @sample AmazonRedshiftAsync.DescribeHsmClientCertificates
*/
java.util.concurrent.Future describeHsmClientCertificatesAsync(
DescribeHsmClientCertificatesRequest describeHsmClientCertificatesRequest);
/**
*
* Returns information about the specified HSM client certificate. If no
* certificate ID is specified, returns information about all the HSM
* certificates owned by your AWS customer account.
*
*
* If you specify both tag keys and tag values in the same request, Amazon
* Redshift returns all HSM client certificates that match any combination
* of the specified keys and values. For example, if you have
* owner
and environment
for tag keys, and
* admin
and test
for tag values, all HSM client
* certificates that have any combination of those values are returned.
*
*
* If both tag keys and values are omitted from the request, HSM client
* certificates are returned regardless of whether they have tag keys or
* values associated with them.
*
*
* @param describeHsmClientCertificatesRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the
* request. Users can provide an implementation of the callback
* methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the
* DescribeHsmClientCertificates operation returned by the service.
* @sample AmazonRedshiftAsyncHandler.DescribeHsmClientCertificates
*/
java.util.concurrent.Future describeHsmClientCertificatesAsync(
DescribeHsmClientCertificatesRequest describeHsmClientCertificatesRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
* Simplified method form for invoking the DescribeHsmClientCertificates
* operation.
*
* @see #describeHsmClientCertificatesAsync(DescribeHsmClientCertificatesRequest)
*/
java.util.concurrent.Future describeHsmClientCertificatesAsync();
/**
* Simplified method form for invoking the DescribeHsmClientCertificates
* operation with an AsyncHandler.
*
* @see #describeHsmClientCertificatesAsync(DescribeHsmClientCertificatesRequest,
* com.amazonaws.handlers.AsyncHandler)
*/
java.util.concurrent.Future describeHsmClientCertificatesAsync(
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns information about the specified Amazon Redshift HSM
* configuration. If no configuration ID is specified, returns information
* about all the HSM configurations owned by your AWS customer account.
*
*
* If you specify both tag keys and tag values in the same request, Amazon
* Redshift returns all HSM connections that match any combination of the
* specified keys and values. For example, if you have owner
* and environment
for tag keys, and admin
and
* test
for tag values, all HSM connections that have any
* combination of those values are returned.
*
*
* If both tag keys and values are omitted from the request, HSM connections
* are returned regardless of whether they have tag keys or values
* associated with them.
*
*
* @param describeHsmConfigurationsRequest
* @return A Java Future containing the result of the
* DescribeHsmConfigurations operation returned by the service.
* @sample AmazonRedshiftAsync.DescribeHsmConfigurations
*/
java.util.concurrent.Future describeHsmConfigurationsAsync(
DescribeHsmConfigurationsRequest describeHsmConfigurationsRequest);
/**
*
* Returns information about the specified Amazon Redshift HSM
* configuration. If no configuration ID is specified, returns information
* about all the HSM configurations owned by your AWS customer account.
*
*
* If you specify both tag keys and tag values in the same request, Amazon
* Redshift returns all HSM connections that match any combination of the
* specified keys and values. For example, if you have owner
* and environment
for tag keys, and admin
and
* test
for tag values, all HSM connections that have any
* combination of those values are returned.
*
*
* If both tag keys and values are omitted from the request, HSM connections
* are returned regardless of whether they have tag keys or values
* associated with them.
*
*
* @param describeHsmConfigurationsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the
* request. Users can provide an implementation of the callback
* methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the
* DescribeHsmConfigurations operation returned by the service.
* @sample AmazonRedshiftAsyncHandler.DescribeHsmConfigurations
*/
java.util.concurrent.Future describeHsmConfigurationsAsync(
DescribeHsmConfigurationsRequest describeHsmConfigurationsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
* Simplified method form for invoking the DescribeHsmConfigurations
* operation.
*
* @see #describeHsmConfigurationsAsync(DescribeHsmConfigurationsRequest)
*/
java.util.concurrent.Future describeHsmConfigurationsAsync();
/**
* Simplified method form for invoking the DescribeHsmConfigurations
* operation with an AsyncHandler.
*
* @see #describeHsmConfigurationsAsync(DescribeHsmConfigurationsRequest,
* com.amazonaws.handlers.AsyncHandler)
*/
java.util.concurrent.Future describeHsmConfigurationsAsync(
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Describes whether information, such as queries and connection attempts,
* is being logged for the specified Amazon Redshift cluster.
*
*
* @param describeLoggingStatusRequest
* @return A Java Future containing the result of the DescribeLoggingStatus
* operation returned by the service.
* @sample AmazonRedshiftAsync.DescribeLoggingStatus
*/
java.util.concurrent.Future describeLoggingStatusAsync(
DescribeLoggingStatusRequest describeLoggingStatusRequest);
/**
*
* Describes whether information, such as queries and connection attempts,
* is being logged for the specified Amazon Redshift cluster.
*
*
* @param describeLoggingStatusRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the
* request. Users can provide an implementation of the callback
* methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeLoggingStatus
* operation returned by the service.
* @sample AmazonRedshiftAsyncHandler.DescribeLoggingStatus
*/
java.util.concurrent.Future describeLoggingStatusAsync(
DescribeLoggingStatusRequest describeLoggingStatusRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns a list of orderable cluster options. Before you create a new
* cluster you can use this operation to find what options are available,
* such as the EC2 Availability Zones (AZ) in the specific AWS region that
* you can specify, and the node types you can request. The node types
* differ by available storage, memory, CPU and price. With the cost
* involved you might want to obtain a list of cluster options in the
* specific region and specify values when creating a cluster. For more
* information about managing clusters, go to Amazon Redshift Clusters in the Amazon Redshift Cluster
* Management Guide
*
*
* @param describeOrderableClusterOptionsRequest
* @return A Java Future containing the result of the
* DescribeOrderableClusterOptions operation returned by the
* service.
* @sample AmazonRedshiftAsync.DescribeOrderableClusterOptions
*/
java.util.concurrent.Future describeOrderableClusterOptionsAsync(
DescribeOrderableClusterOptionsRequest describeOrderableClusterOptionsRequest);
/**
*
* Returns a list of orderable cluster options. Before you create a new
* cluster you can use this operation to find what options are available,
* such as the EC2 Availability Zones (AZ) in the specific AWS region that
* you can specify, and the node types you can request. The node types
* differ by available storage, memory, CPU and price. With the cost
* involved you might want to obtain a list of cluster options in the
* specific region and specify values when creating a cluster. For more
* information about managing clusters, go to Amazon Redshift Clusters in the Amazon Redshift Cluster
* Management Guide
*
*
* @param describeOrderableClusterOptionsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the
* request. Users can provide an implementation of the callback
* methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the
* DescribeOrderableClusterOptions operation returned by the
* service.
* @sample AmazonRedshiftAsyncHandler.DescribeOrderableClusterOptions
*/
java.util.concurrent.Future describeOrderableClusterOptionsAsync(
DescribeOrderableClusterOptionsRequest describeOrderableClusterOptionsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
* Simplified method form for invoking the DescribeOrderableClusterOptions
* operation.
*
* @see #describeOrderableClusterOptionsAsync(DescribeOrderableClusterOptionsRequest)
*/
java.util.concurrent.Future describeOrderableClusterOptionsAsync();
/**
* Simplified method form for invoking the DescribeOrderableClusterOptions
* operation with an AsyncHandler.
*
* @see #describeOrderableClusterOptionsAsync(DescribeOrderableClusterOptionsRequest,
* com.amazonaws.handlers.AsyncHandler)
*/
java.util.concurrent.Future describeOrderableClusterOptionsAsync(
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns a list of the available reserved node offerings by Amazon
* Redshift with their descriptions including the node type, the fixed and
* recurring costs of reserving the node and duration the node will be
* reserved for you. These descriptions help you determine which reserve
* node offering you want to purchase. You then use the unique offering ID
* in you call to PurchaseReservedNodeOffering to reserve one or more
* nodes for your Amazon Redshift cluster.
*
*
* For more information about reserved node offerings, go to Purchasing Reserved Nodes in the Amazon Redshift Cluster
* Management Guide.
*
*
* @param describeReservedNodeOfferingsRequest
* @return A Java Future containing the result of the
* DescribeReservedNodeOfferings operation returned by the service.
* @sample AmazonRedshiftAsync.DescribeReservedNodeOfferings
*/
java.util.concurrent.Future describeReservedNodeOfferingsAsync(
DescribeReservedNodeOfferingsRequest describeReservedNodeOfferingsRequest);
/**
*
* Returns a list of the available reserved node offerings by Amazon
* Redshift with their descriptions including the node type, the fixed and
* recurring costs of reserving the node and duration the node will be
* reserved for you. These descriptions help you determine which reserve
* node offering you want to purchase. You then use the unique offering ID
* in you call to PurchaseReservedNodeOffering to reserve one or more
* nodes for your Amazon Redshift cluster.
*
*
* For more information about reserved node offerings, go to Purchasing Reserved Nodes in the Amazon Redshift Cluster
* Management Guide.
*
*
* @param describeReservedNodeOfferingsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the
* request. Users can provide an implementation of the callback
* methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the
* DescribeReservedNodeOfferings operation returned by the service.
* @sample AmazonRedshiftAsyncHandler.DescribeReservedNodeOfferings
*/
java.util.concurrent.Future describeReservedNodeOfferingsAsync(
DescribeReservedNodeOfferingsRequest describeReservedNodeOfferingsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
* Simplified method form for invoking the DescribeReservedNodeOfferings
* operation.
*
* @see #describeReservedNodeOfferingsAsync(DescribeReservedNodeOfferingsRequest)
*/
java.util.concurrent.Future describeReservedNodeOfferingsAsync();
/**
* Simplified method form for invoking the DescribeReservedNodeOfferings
* operation with an AsyncHandler.
*
* @see #describeReservedNodeOfferingsAsync(DescribeReservedNodeOfferingsRequest,
* com.amazonaws.handlers.AsyncHandler)
*/
java.util.concurrent.Future describeReservedNodeOfferingsAsync(
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns the descriptions of the reserved nodes.
*
*
* @param describeReservedNodesRequest
* @return A Java Future containing the result of the DescribeReservedNodes
* operation returned by the service.
* @sample AmazonRedshiftAsync.DescribeReservedNodes
*/
java.util.concurrent.Future describeReservedNodesAsync(
DescribeReservedNodesRequest describeReservedNodesRequest);
/**
*
* Returns the descriptions of the reserved nodes.
*
*
* @param describeReservedNodesRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the
* request. Users can provide an implementation of the callback
* methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeReservedNodes
* operation returned by the service.
* @sample AmazonRedshiftAsyncHandler.DescribeReservedNodes
*/
java.util.concurrent.Future describeReservedNodesAsync(
DescribeReservedNodesRequest describeReservedNodesRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
* Simplified method form for invoking the DescribeReservedNodes operation.
*
* @see #describeReservedNodesAsync(DescribeReservedNodesRequest)
*/
java.util.concurrent.Future describeReservedNodesAsync();
/**
* Simplified method form for invoking the DescribeReservedNodes operation
* with an AsyncHandler.
*
* @see #describeReservedNodesAsync(DescribeReservedNodesRequest,
* com.amazonaws.handlers.AsyncHandler)
*/
java.util.concurrent.Future describeReservedNodesAsync(
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns information about the last resize operation for the specified
* cluster. If no resize operation has ever been initiated for the specified
* cluster, a HTTP 404
error is returned. If a resize operation
* was initiated and completed, the status of the resize remains as
* SUCCEEDED
until the next resize.
*
*
* A resize operation can be requested using ModifyCluster and
* specifying a different number or type of nodes for the cluster.
*
*
* @param describeResizeRequest
* @return A Java Future containing the result of the DescribeResize
* operation returned by the service.
* @sample AmazonRedshiftAsync.DescribeResize
*/
java.util.concurrent.Future describeResizeAsync(
DescribeResizeRequest describeResizeRequest);
/**
*
* Returns information about the last resize operation for the specified
* cluster. If no resize operation has ever been initiated for the specified
* cluster, a HTTP 404
error is returned. If a resize operation
* was initiated and completed, the status of the resize remains as
* SUCCEEDED
until the next resize.
*
*
* A resize operation can be requested using ModifyCluster and
* specifying a different number or type of nodes for the cluster.
*
*
* @param describeResizeRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the
* request. Users can provide an implementation of the callback
* methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeResize
* operation returned by the service.
* @sample AmazonRedshiftAsyncHandler.DescribeResize
*/
java.util.concurrent.Future describeResizeAsync(
DescribeResizeRequest describeResizeRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns a list of snapshot copy grants owned by the AWS account in the
* destination region.
*
*
* For more information about managing snapshot copy grants, go to Amazon Redshift Database Encryption in the Amazon Redshift
* Cluster Management Guide.
*
*
* @param describeSnapshotCopyGrantsRequest
* The result of the DescribeSnapshotCopyGrants
action.
* @return A Java Future containing the result of the
* DescribeSnapshotCopyGrants operation returned by the service.
* @sample AmazonRedshiftAsync.DescribeSnapshotCopyGrants
*/
java.util.concurrent.Future describeSnapshotCopyGrantsAsync(
DescribeSnapshotCopyGrantsRequest describeSnapshotCopyGrantsRequest);
/**
*
* Returns a list of snapshot copy grants owned by the AWS account in the
* destination region.
*
*
* For more information about managing snapshot copy grants, go to Amazon Redshift Database Encryption in the Amazon Redshift
* Cluster Management Guide.
*
*
* @param describeSnapshotCopyGrantsRequest
* The result of the DescribeSnapshotCopyGrants
action.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the
* request. Users can provide an implementation of the callback
* methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the
* DescribeSnapshotCopyGrants operation returned by the service.
* @sample AmazonRedshiftAsyncHandler.DescribeSnapshotCopyGrants
*/
java.util.concurrent.Future describeSnapshotCopyGrantsAsync(
DescribeSnapshotCopyGrantsRequest describeSnapshotCopyGrantsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
* Simplified method form for invoking the DescribeSnapshotCopyGrants
* operation.
*
* @see #describeSnapshotCopyGrantsAsync(DescribeSnapshotCopyGrantsRequest)
*/
java.util.concurrent.Future describeSnapshotCopyGrantsAsync();
/**
* Simplified method form for invoking the DescribeSnapshotCopyGrants
* operation with an AsyncHandler.
*
* @see #describeSnapshotCopyGrantsAsync(DescribeSnapshotCopyGrantsRequest,
* com.amazonaws.handlers.AsyncHandler)
*/
java.util.concurrent.Future describeSnapshotCopyGrantsAsync(
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Lists the status of one or more table restore requests made using the
* RestoreTableFromClusterSnapshot API action. If you don't specify a
* value for the TableRestoreRequestId
parameter, then
* DescribeTableRestoreStatus
returns the status of all table
* restore requests ordered by the date and time of the request in ascending
* order. Otherwise DescribeTableRestoreStatus
returns the
* status of the table specified by TableRestoreRequestId
.
*
*
* @param describeTableRestoreStatusRequest
* @return A Java Future containing the result of the
* DescribeTableRestoreStatus operation returned by the service.
* @sample AmazonRedshiftAsync.DescribeTableRestoreStatus
*/
java.util.concurrent.Future describeTableRestoreStatusAsync(
DescribeTableRestoreStatusRequest describeTableRestoreStatusRequest);
/**
*
* Lists the status of one or more table restore requests made using the
* RestoreTableFromClusterSnapshot API action. If you don't specify a
* value for the TableRestoreRequestId
parameter, then
* DescribeTableRestoreStatus
returns the status of all table
* restore requests ordered by the date and time of the request in ascending
* order. Otherwise DescribeTableRestoreStatus
returns the
* status of the table specified by TableRestoreRequestId
.
*
*
* @param describeTableRestoreStatusRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the
* request. Users can provide an implementation of the callback
* methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the
* DescribeTableRestoreStatus operation returned by the service.
* @sample AmazonRedshiftAsyncHandler.DescribeTableRestoreStatus
*/
java.util.concurrent.Future describeTableRestoreStatusAsync(
DescribeTableRestoreStatusRequest describeTableRestoreStatusRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
* Simplified method form for invoking the DescribeTableRestoreStatus
* operation.
*
* @see #describeTableRestoreStatusAsync(DescribeTableRestoreStatusRequest)
*/
java.util.concurrent.Future describeTableRestoreStatusAsync();
/**
* Simplified method form for invoking the DescribeTableRestoreStatus
* operation with an AsyncHandler.
*
* @see #describeTableRestoreStatusAsync(DescribeTableRestoreStatusRequest,
* com.amazonaws.handlers.AsyncHandler)
*/
java.util.concurrent.Future describeTableRestoreStatusAsync(
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns a list of tags. You can return tags from a specific resource by
* specifying an ARN, or you can return all tags for a given type of
* resource, such as clusters, snapshots, and so on.
*
*
* The following are limitations for DescribeTags
:
*
* - You cannot specify an ARN and a resource-type value together in the
* same request.
* - You cannot use the
MaxRecords
and Marker
* parameters together with the ARN parameter.
* - The
MaxRecords
parameter can be a range from 10 to 50
* results to return in a request.
*
*
*
* If you specify both tag keys and tag values in the same request, Amazon
* Redshift returns all resources that match any combination of the
* specified keys and values. For example, if you have owner
* and environment
for tag keys, and admin
and
* test
for tag values, all resources that have any combination
* of those values are returned.
*
*
* If both tag keys and values are omitted from the request, resources are
* returned regardless of whether they have tag keys or values associated
* with them.
*
*
* @param describeTagsRequest
* @return A Java Future containing the result of the DescribeTags operation
* returned by the service.
* @sample AmazonRedshiftAsync.DescribeTags
*/
java.util.concurrent.Future describeTagsAsync(
DescribeTagsRequest describeTagsRequest);
/**
*
* Returns a list of tags. You can return tags from a specific resource by
* specifying an ARN, or you can return all tags for a given type of
* resource, such as clusters, snapshots, and so on.
*
*
* The following are limitations for DescribeTags
:
*
* - You cannot specify an ARN and a resource-type value together in the
* same request.
* - You cannot use the
MaxRecords
and Marker
* parameters together with the ARN parameter.
* - The
MaxRecords
parameter can be a range from 10 to 50
* results to return in a request.
*
*
*
* If you specify both tag keys and tag values in the same request, Amazon
* Redshift returns all resources that match any combination of the
* specified keys and values. For example, if you have owner
* and environment
for tag keys, and admin
and
* test
for tag values, all resources that have any combination
* of those values are returned.
*
*
* If both tag keys and values are omitted from the request, resources are
* returned regardless of whether they have tag keys or values associated
* with them.
*
*
* @param describeTagsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the
* request. Users can provide an implementation of the callback
* methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeTags operation
* returned by the service.
* @sample AmazonRedshiftAsyncHandler.DescribeTags
*/
java.util.concurrent.Future describeTagsAsync(
DescribeTagsRequest describeTagsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
* Simplified method form for invoking the DescribeTags operation.
*
* @see #describeTagsAsync(DescribeTagsRequest)
*/
java.util.concurrent.Future describeTagsAsync();
/**
* Simplified method form for invoking the DescribeTags operation with an
* AsyncHandler.
*
* @see #describeTagsAsync(DescribeTagsRequest,
* com.amazonaws.handlers.AsyncHandler)
*/
java.util.concurrent.Future describeTagsAsync(
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Stops logging information, such as queries and connection attempts, for
* the specified Amazon Redshift cluster.
*
*
* @param disableLoggingRequest
* @return A Java Future containing the result of the DisableLogging
* operation returned by the service.
* @sample AmazonRedshiftAsync.DisableLogging
*/
java.util.concurrent.Future disableLoggingAsync(
DisableLoggingRequest disableLoggingRequest);
/**
*
* Stops logging information, such as queries and connection attempts, for
* the specified Amazon Redshift cluster.
*
*
* @param disableLoggingRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the
* request. Users can provide an implementation of the callback
* methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DisableLogging
* operation returned by the service.
* @sample AmazonRedshiftAsyncHandler.DisableLogging
*/
java.util.concurrent.Future disableLoggingAsync(
DisableLoggingRequest disableLoggingRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Disables the automatic copying of snapshots from one region to another
* region for a specified cluster.
*
*
* If your cluster and its snapshots are encrypted using a customer master
* key (CMK) from AWS KMS, use DeleteSnapshotCopyGrant to delete the
* grant that grants Amazon Redshift permission to the CMK in the
* destination region.
*
*
* @param disableSnapshotCopyRequest
* @return A Java Future containing the result of the DisableSnapshotCopy
* operation returned by the service.
* @sample AmazonRedshiftAsync.DisableSnapshotCopy
*/
java.util.concurrent.Future disableSnapshotCopyAsync(
DisableSnapshotCopyRequest disableSnapshotCopyRequest);
/**
*
* Disables the automatic copying of snapshots from one region to another
* region for a specified cluster.
*
*
* If your cluster and its snapshots are encrypted using a customer master
* key (CMK) from AWS KMS, use DeleteSnapshotCopyGrant to delete the
* grant that grants Amazon Redshift permission to the CMK in the
* destination region.
*
*
* @param disableSnapshotCopyRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the
* request. Users can provide an implementation of the callback
* methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DisableSnapshotCopy
* operation returned by the service.
* @sample AmazonRedshiftAsyncHandler.DisableSnapshotCopy
*/
java.util.concurrent.Future disableSnapshotCopyAsync(
DisableSnapshotCopyRequest disableSnapshotCopyRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Starts logging information, such as queries and connection attempts, for
* the specified Amazon Redshift cluster.
*
*
* @param enableLoggingRequest
* @return A Java Future containing the result of the EnableLogging
* operation returned by the service.
* @sample AmazonRedshiftAsync.EnableLogging
*/
java.util.concurrent.Future enableLoggingAsync(
EnableLoggingRequest enableLoggingRequest);
/**
*
* Starts logging information, such as queries and connection attempts, for
* the specified Amazon Redshift cluster.
*
*
* @param enableLoggingRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the
* request. Users can provide an implementation of the callback
* methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the EnableLogging
* operation returned by the service.
* @sample AmazonRedshiftAsyncHandler.EnableLogging
*/
java.util.concurrent.Future enableLoggingAsync(
EnableLoggingRequest enableLoggingRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Enables the automatic copy of snapshots from one region to another region
* for a specified cluster.
*
*
* @param enableSnapshotCopyRequest
* @return A Java Future containing the result of the EnableSnapshotCopy
* operation returned by the service.
* @sample AmazonRedshiftAsync.EnableSnapshotCopy
*/
java.util.concurrent.Future enableSnapshotCopyAsync(
EnableSnapshotCopyRequest enableSnapshotCopyRequest);
/**
*
* Enables the automatic copy of snapshots from one region to another region
* for a specified cluster.
*
*
* @param enableSnapshotCopyRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the
* request. Users can provide an implementation of the callback
* methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the EnableSnapshotCopy
* operation returned by the service.
* @sample AmazonRedshiftAsyncHandler.EnableSnapshotCopy
*/
java.util.concurrent.Future enableSnapshotCopyAsync(
EnableSnapshotCopyRequest enableSnapshotCopyRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Modifies the settings for a cluster. For example, you can add another
* security or parameter group, update the preferred maintenance window, or
* change the master user password. Resetting a cluster password or
* modifying the security groups associated with a cluster do not need a
* reboot. However, modifying a parameter group requires a reboot for
* parameters to take effect. For more information about managing clusters,
* go to Amazon Redshift Clusters in the Amazon Redshift Cluster
* Management Guide .
*
*
* You can also change node type and the number of nodes to scale up or down
* the cluster. When resizing a cluster, you must specify both the number of
* nodes and the node type even if one of the parameters does not change.
*
*
* @param modifyClusterRequest
* @return A Java Future containing the result of the ModifyCluster
* operation returned by the service.
* @sample AmazonRedshiftAsync.ModifyCluster
*/
java.util.concurrent.Future modifyClusterAsync(
ModifyClusterRequest modifyClusterRequest);
/**
*
* Modifies the settings for a cluster. For example, you can add another
* security or parameter group, update the preferred maintenance window, or
* change the master user password. Resetting a cluster password or
* modifying the security groups associated with a cluster do not need a
* reboot. However, modifying a parameter group requires a reboot for
* parameters to take effect. For more information about managing clusters,
* go to Amazon Redshift Clusters in the Amazon Redshift Cluster
* Management Guide .
*
*
* You can also change node type and the number of nodes to scale up or down
* the cluster. When resizing a cluster, you must specify both the number of
* nodes and the node type even if one of the parameters does not change.
*
*
* @param modifyClusterRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the
* request. Users can provide an implementation of the callback
* methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ModifyCluster
* operation returned by the service.
* @sample AmazonRedshiftAsyncHandler.ModifyCluster
*/
java.util.concurrent.Future modifyClusterAsync(
ModifyClusterRequest modifyClusterRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Modifies the list of AWS Identity and Access Management (IAM) roles that
* can be used by the cluster to access other AWS services.
*
*
* A cluster can have up to 10 IAM roles associated at any time.
*
*
* @param modifyClusterIamRolesRequest
* @return A Java Future containing the result of the ModifyClusterIamRoles
* operation returned by the service.
* @sample AmazonRedshiftAsync.ModifyClusterIamRoles
*/
java.util.concurrent.Future modifyClusterIamRolesAsync(
ModifyClusterIamRolesRequest modifyClusterIamRolesRequest);
/**
*
* Modifies the list of AWS Identity and Access Management (IAM) roles that
* can be used by the cluster to access other AWS services.
*
*
* A cluster can have up to 10 IAM roles associated at any time.
*
*
* @param modifyClusterIamRolesRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the
* request. Users can provide an implementation of the callback
* methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ModifyClusterIamRoles
* operation returned by the service.
* @sample AmazonRedshiftAsyncHandler.ModifyClusterIamRoles
*/
java.util.concurrent.Future modifyClusterIamRolesAsync(
ModifyClusterIamRolesRequest modifyClusterIamRolesRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Modifies the parameters of a parameter group.
*
*
* For more information about parameters and parameter groups, go to Amazon Redshift Parameter Groups in the Amazon Redshift Cluster
* Management Guide.
*
*
* @param modifyClusterParameterGroupRequest
* @return A Java Future containing the result of the
* ModifyClusterParameterGroup operation returned by the service.
* @sample AmazonRedshiftAsync.ModifyClusterParameterGroup
*/
java.util.concurrent.Future modifyClusterParameterGroupAsync(
ModifyClusterParameterGroupRequest modifyClusterParameterGroupRequest);
/**
*
* Modifies the parameters of a parameter group.
*
*
* For more information about parameters and parameter groups, go to Amazon Redshift Parameter Groups in the Amazon Redshift Cluster
* Management Guide.
*
*
* @param modifyClusterParameterGroupRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the
* request. Users can provide an implementation of the callback
* methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the
* ModifyClusterParameterGroup operation returned by the service.
* @sample AmazonRedshiftAsyncHandler.ModifyClusterParameterGroup
*/
java.util.concurrent.Future modifyClusterParameterGroupAsync(
ModifyClusterParameterGroupRequest modifyClusterParameterGroupRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Modifies a cluster subnet group to include the specified list of VPC
* subnets. The operation replaces the existing list of subnets with the new
* list of subnets.
*
*
* @param modifyClusterSubnetGroupRequest
* @return A Java Future containing the result of the
* ModifyClusterSubnetGroup operation returned by the service.
* @sample AmazonRedshiftAsync.ModifyClusterSubnetGroup
*/
java.util.concurrent.Future modifyClusterSubnetGroupAsync(
ModifyClusterSubnetGroupRequest modifyClusterSubnetGroupRequest);
/**
*
* Modifies a cluster subnet group to include the specified list of VPC
* subnets. The operation replaces the existing list of subnets with the new
* list of subnets.
*
*
* @param modifyClusterSubnetGroupRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the
* request. Users can provide an implementation of the callback
* methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the
* ModifyClusterSubnetGroup operation returned by the service.
* @sample AmazonRedshiftAsyncHandler.ModifyClusterSubnetGroup
*/
java.util.concurrent.Future modifyClusterSubnetGroupAsync(
ModifyClusterSubnetGroupRequest modifyClusterSubnetGroupRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Modifies an existing Amazon Redshift event notification subscription.
*
*
* @param modifyEventSubscriptionRequest
* @return A Java Future containing the result of the
* ModifyEventSubscription operation returned by the service.
* @sample AmazonRedshiftAsync.ModifyEventSubscription
*/
java.util.concurrent.Future modifyEventSubscriptionAsync(
ModifyEventSubscriptionRequest modifyEventSubscriptionRequest);
/**
*
* Modifies an existing Amazon Redshift event notification subscription.
*
*
* @param modifyEventSubscriptionRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the
* request. Users can provide an implementation of the callback
* methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the
* ModifyEventSubscription operation returned by the service.
* @sample AmazonRedshiftAsyncHandler.ModifyEventSubscription
*/
java.util.concurrent.Future modifyEventSubscriptionAsync(
ModifyEventSubscriptionRequest modifyEventSubscriptionRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Modifies the number of days to retain automated snapshots in the
* destination region after they are copied from the source region.
*
*
* @param modifySnapshotCopyRetentionPeriodRequest
* @return A Java Future containing the result of the
* ModifySnapshotCopyRetentionPeriod operation returned by the
* service.
* @sample AmazonRedshiftAsync.ModifySnapshotCopyRetentionPeriod
*/
java.util.concurrent.Future modifySnapshotCopyRetentionPeriodAsync(
ModifySnapshotCopyRetentionPeriodRequest modifySnapshotCopyRetentionPeriodRequest);
/**
*
* Modifies the number of days to retain automated snapshots in the
* destination region after they are copied from the source region.
*
*
* @param modifySnapshotCopyRetentionPeriodRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the
* request. Users can provide an implementation of the callback
* methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the
* ModifySnapshotCopyRetentionPeriod operation returned by the
* service.
* @sample AmazonRedshiftAsyncHandler.ModifySnapshotCopyRetentionPeriod
*/
java.util.concurrent.Future modifySnapshotCopyRetentionPeriodAsync(
ModifySnapshotCopyRetentionPeriodRequest modifySnapshotCopyRetentionPeriodRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Allows you to purchase reserved nodes. Amazon Redshift offers a
* predefined set of reserved node offerings. You can purchase one or more
* of the offerings. You can call the DescribeReservedNodeOfferings
* API to obtain the available reserved node offerings. You can call this
* API by providing a specific reserved node offering and the number of
* nodes you want to reserve.
*
*
* For more information about reserved node offerings, go to Purchasing Reserved Nodes in the Amazon Redshift Cluster
* Management Guide.
*
*
* @param purchaseReservedNodeOfferingRequest
* @return A Java Future containing the result of the
* PurchaseReservedNodeOffering operation returned by the service.
* @sample AmazonRedshiftAsync.PurchaseReservedNodeOffering
*/
java.util.concurrent.Future purchaseReservedNodeOfferingAsync(
PurchaseReservedNodeOfferingRequest purchaseReservedNodeOfferingRequest);
/**
*
* Allows you to purchase reserved nodes. Amazon Redshift offers a
* predefined set of reserved node offerings. You can purchase one or more
* of the offerings. You can call the DescribeReservedNodeOfferings
* API to obtain the available reserved node offerings. You can call this
* API by providing a specific reserved node offering and the number of
* nodes you want to reserve.
*
*
* For more information about reserved node offerings, go to Purchasing Reserved Nodes in the Amazon Redshift Cluster
* Management Guide.
*
*
* @param purchaseReservedNodeOfferingRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the
* request. Users can provide an implementation of the callback
* methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the
* PurchaseReservedNodeOffering operation returned by the service.
* @sample AmazonRedshiftAsyncHandler.PurchaseReservedNodeOffering
*/
java.util.concurrent.Future purchaseReservedNodeOfferingAsync(
PurchaseReservedNodeOfferingRequest purchaseReservedNodeOfferingRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Reboots a cluster. This action is taken as soon as possible. It results
* in a momentary outage to the cluster, during which the cluster status is
* set to rebooting
. A cluster event is created when the reboot
* is completed. Any pending cluster modifications (see
* ModifyCluster) are applied at this reboot. For more information
* about managing clusters, go to Amazon Redshift Clusters in the Amazon Redshift Cluster
* Management Guide
*
*
* @param rebootClusterRequest
* @return A Java Future containing the result of the RebootCluster
* operation returned by the service.
* @sample AmazonRedshiftAsync.RebootCluster
*/
java.util.concurrent.Future rebootClusterAsync(
RebootClusterRequest rebootClusterRequest);
/**
*
* Reboots a cluster. This action is taken as soon as possible. It results
* in a momentary outage to the cluster, during which the cluster status is
* set to rebooting
. A cluster event is created when the reboot
* is completed. Any pending cluster modifications (see
* ModifyCluster) are applied at this reboot. For more information
* about managing clusters, go to Amazon Redshift Clusters in the Amazon Redshift Cluster
* Management Guide
*
*
* @param rebootClusterRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the
* request. Users can provide an implementation of the callback
* methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the RebootCluster
* operation returned by the service.
* @sample AmazonRedshiftAsyncHandler.RebootCluster
*/
java.util.concurrent.Future rebootClusterAsync(
RebootClusterRequest rebootClusterRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Sets one or more parameters of the specified parameter group to their
* default values and sets the source values of the parameters to
* "engine-default". To reset the entire parameter group specify the
* ResetAllParameters parameter. For parameter changes to take effect
* you must reboot any associated clusters.
*
*
* @param resetClusterParameterGroupRequest
* @return A Java Future containing the result of the
* ResetClusterParameterGroup operation returned by the service.
* @sample AmazonRedshiftAsync.ResetClusterParameterGroup
*/
java.util.concurrent.Future resetClusterParameterGroupAsync(
ResetClusterParameterGroupRequest resetClusterParameterGroupRequest);
/**
*
* Sets one or more parameters of the specified parameter group to their
* default values and sets the source values of the parameters to
* "engine-default". To reset the entire parameter group specify the
* ResetAllParameters parameter. For parameter changes to take effect
* you must reboot any associated clusters.
*
*
* @param resetClusterParameterGroupRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the
* request. Users can provide an implementation of the callback
* methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the
* ResetClusterParameterGroup operation returned by the service.
* @sample AmazonRedshiftAsyncHandler.ResetClusterParameterGroup
*/
java.util.concurrent.Future resetClusterParameterGroupAsync(
ResetClusterParameterGroupRequest resetClusterParameterGroupRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates a new cluster from a snapshot. By default, Amazon Redshift
* creates the resulting cluster with the same configuration as the original
* cluster from which the snapshot was created, except that the new cluster
* is created with the default cluster security and parameter groups. After
* Amazon Redshift creates the cluster, you can use the ModifyCluster
* API to associate a different security group and different parameter group
* with the restored cluster. If you are using a DS node type, you can also
* choose to change to another DS node type of the same size during restore.
*
*
* If you restore a cluster into a VPC, you must provide a cluster subnet
* group where you want the cluster restored.
*
*
* For more information about working with snapshots, go to Amazon Redshift Snapshots in the Amazon Redshift Cluster
* Management Guide.
*
*
* @param restoreFromClusterSnapshotRequest
* @return A Java Future containing the result of the
* RestoreFromClusterSnapshot operation returned by the service.
* @sample AmazonRedshiftAsync.RestoreFromClusterSnapshot
*/
java.util.concurrent.Future restoreFromClusterSnapshotAsync(
RestoreFromClusterSnapshotRequest restoreFromClusterSnapshotRequest);
/**
*
* Creates a new cluster from a snapshot. By default, Amazon Redshift
* creates the resulting cluster with the same configuration as the original
* cluster from which the snapshot was created, except that the new cluster
* is created with the default cluster security and parameter groups. After
* Amazon Redshift creates the cluster, you can use the ModifyCluster
* API to associate a different security group and different parameter group
* with the restored cluster. If you are using a DS node type, you can also
* choose to change to another DS node type of the same size during restore.
*
*
* If you restore a cluster into a VPC, you must provide a cluster subnet
* group where you want the cluster restored.
*
*
* For more information about working with snapshots, go to Amazon Redshift Snapshots in the Amazon Redshift Cluster
* Management Guide.
*
*
* @param restoreFromClusterSnapshotRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the
* request. Users can provide an implementation of the callback
* methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the
* RestoreFromClusterSnapshot operation returned by the service.
* @sample AmazonRedshiftAsyncHandler.RestoreFromClusterSnapshot
*/
java.util.concurrent.Future restoreFromClusterSnapshotAsync(
RestoreFromClusterSnapshotRequest restoreFromClusterSnapshotRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates a new table from a table in an Amazon Redshift cluster snapshot.
* You must create the new table within the Amazon Redshift cluster that the
* snapshot was taken from.
*
*
* You cannot use RestoreTableFromClusterSnapshot
to restore a
* table with the same name as an existing table in an Amazon Redshift
* cluster. That is, you cannot overwrite an existing table in a cluster
* with a restored table. If you want to replace your original table with a
* new, restored table, then rename or drop your original table before you
* call RestoreTableFromClusterSnapshot
. When you have renamed
* your original table, then you can pass the original name of the table as
* the NewTableName
parameter value in the call to
* RestoreTableFromClusterSnapshot
. This way, you can replace
* the original table with the table created from the snapshot.
*
*
* @param restoreTableFromClusterSnapshotRequest
* @return A Java Future containing the result of the
* RestoreTableFromClusterSnapshot operation returned by the
* service.
* @sample AmazonRedshiftAsync.RestoreTableFromClusterSnapshot
*/
java.util.concurrent.Future restoreTableFromClusterSnapshotAsync(
RestoreTableFromClusterSnapshotRequest restoreTableFromClusterSnapshotRequest);
/**
*
* Creates a new table from a table in an Amazon Redshift cluster snapshot.
* You must create the new table within the Amazon Redshift cluster that the
* snapshot was taken from.
*
*
* You cannot use RestoreTableFromClusterSnapshot
to restore a
* table with the same name as an existing table in an Amazon Redshift
* cluster. That is, you cannot overwrite an existing table in a cluster
* with a restored table. If you want to replace your original table with a
* new, restored table, then rename or drop your original table before you
* call RestoreTableFromClusterSnapshot
. When you have renamed
* your original table, then you can pass the original name of the table as
* the NewTableName
parameter value in the call to
* RestoreTableFromClusterSnapshot
. This way, you can replace
* the original table with the table created from the snapshot.
*
*
* @param restoreTableFromClusterSnapshotRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the
* request. Users can provide an implementation of the callback
* methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the
* RestoreTableFromClusterSnapshot operation returned by the
* service.
* @sample AmazonRedshiftAsyncHandler.RestoreTableFromClusterSnapshot
*/
java.util.concurrent.Future restoreTableFromClusterSnapshotAsync(
RestoreTableFromClusterSnapshotRequest restoreTableFromClusterSnapshotRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Revokes an ingress rule in an Amazon Redshift security group for a
* previously authorized IP range or Amazon EC2 security group. To add an
* ingress rule, see AuthorizeClusterSecurityGroupIngress. For
* information about managing security groups, go to Amazon Redshift Cluster Security Groups in the Amazon Redshift
* Cluster Management Guide.
*
*
* @param revokeClusterSecurityGroupIngressRequest
* @return A Java Future containing the result of the
* RevokeClusterSecurityGroupIngress operation returned by the
* service.
* @sample AmazonRedshiftAsync.RevokeClusterSecurityGroupIngress
*/
java.util.concurrent.Future revokeClusterSecurityGroupIngressAsync(
RevokeClusterSecurityGroupIngressRequest revokeClusterSecurityGroupIngressRequest);
/**
*
* Revokes an ingress rule in an Amazon Redshift security group for a
* previously authorized IP range or Amazon EC2 security group. To add an
* ingress rule, see AuthorizeClusterSecurityGroupIngress. For
* information about managing security groups, go to Amazon Redshift Cluster Security Groups in the Amazon Redshift
* Cluster Management Guide.
*
*
* @param revokeClusterSecurityGroupIngressRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the
* request. Users can provide an implementation of the callback
* methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the
* RevokeClusterSecurityGroupIngress operation returned by the
* service.
* @sample AmazonRedshiftAsyncHandler.RevokeClusterSecurityGroupIngress
*/
java.util.concurrent.Future revokeClusterSecurityGroupIngressAsync(
RevokeClusterSecurityGroupIngressRequest revokeClusterSecurityGroupIngressRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Removes the ability of the specified AWS customer account to restore the
* specified snapshot. If the account is currently restoring the snapshot,
* the restore will run to completion.
*
*
* For more information about working with snapshots, go to Amazon Redshift Snapshots in the Amazon Redshift Cluster
* Management Guide.
*
*
* @param revokeSnapshotAccessRequest
* @return A Java Future containing the result of the RevokeSnapshotAccess
* operation returned by the service.
* @sample AmazonRedshiftAsync.RevokeSnapshotAccess
*/
java.util.concurrent.Future revokeSnapshotAccessAsync(
RevokeSnapshotAccessRequest revokeSnapshotAccessRequest);
/**
*
* Removes the ability of the specified AWS customer account to restore the
* specified snapshot. If the account is currently restoring the snapshot,
* the restore will run to completion.
*
*
* For more information about working with snapshots, go to Amazon Redshift Snapshots in the Amazon Redshift Cluster
* Management Guide.
*
*
* @param revokeSnapshotAccessRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the
* request. Users can provide an implementation of the callback
* methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the RevokeSnapshotAccess
* operation returned by the service.
* @sample AmazonRedshiftAsyncHandler.RevokeSnapshotAccess
*/
java.util.concurrent.Future revokeSnapshotAccessAsync(
RevokeSnapshotAccessRequest revokeSnapshotAccessRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Rotates the encryption keys for a cluster.
*
*
* @param rotateEncryptionKeyRequest
* @return A Java Future containing the result of the RotateEncryptionKey
* operation returned by the service.
* @sample AmazonRedshiftAsync.RotateEncryptionKey
*/
java.util.concurrent.Future rotateEncryptionKeyAsync(
RotateEncryptionKeyRequest rotateEncryptionKeyRequest);
/**
*
* Rotates the encryption keys for a cluster.
*
*
* @param rotateEncryptionKeyRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the
* request. Users can provide an implementation of the callback
* methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the RotateEncryptionKey
* operation returned by the service.
* @sample AmazonRedshiftAsyncHandler.RotateEncryptionKey
*/
java.util.concurrent.Future rotateEncryptionKeyAsync(
RotateEncryptionKeyRequest rotateEncryptionKeyRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
}