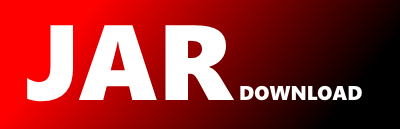
com.amazonaws.services.redshift.model.ReservedNodeExchangeStatus Maven / Gradle / Ivy
Show all versions of aws-java-sdk-redshift Show documentation
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.redshift.model;
import java.io.Serializable;
import javax.annotation.Generated;
/**
*
* Reserved-node status details, such as the source reserved-node identifier, the target reserved-node identifier, the
* node type, the node count, and other details.
*
*
* @see AWS API Documentation
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class ReservedNodeExchangeStatus implements Serializable, Cloneable {
/**
*
* The identifier of the reserved-node exchange request.
*
*/
private String reservedNodeExchangeRequestId;
/**
*
* The status of the reserved-node exchange request. Statuses include in-progress and requested.
*
*/
private String status;
/**
*
* A date and time that indicate when the reserved-node exchange was requested.
*
*/
private java.util.Date requestTime;
/**
*
* The identifier of the source reserved node.
*
*/
private String sourceReservedNodeId;
/**
*
* The source reserved-node type, for example ra3.4xlarge.
*
*/
private String sourceReservedNodeType;
/**
*
* The source reserved-node count in the cluster.
*
*/
private Integer sourceReservedNodeCount;
/**
*
* The identifier of the target reserved node offering.
*
*/
private String targetReservedNodeOfferingId;
/**
*
* The node type of the target reserved node, for example ra3.4xlarge.
*
*/
private String targetReservedNodeType;
/**
*
* The count of target reserved nodes in the cluster.
*
*/
private Integer targetReservedNodeCount;
/**
*
* The identifier of the reserved-node exchange request.
*
*
* @param reservedNodeExchangeRequestId
* The identifier of the reserved-node exchange request.
*/
public void setReservedNodeExchangeRequestId(String reservedNodeExchangeRequestId) {
this.reservedNodeExchangeRequestId = reservedNodeExchangeRequestId;
}
/**
*
* The identifier of the reserved-node exchange request.
*
*
* @return The identifier of the reserved-node exchange request.
*/
public String getReservedNodeExchangeRequestId() {
return this.reservedNodeExchangeRequestId;
}
/**
*
* The identifier of the reserved-node exchange request.
*
*
* @param reservedNodeExchangeRequestId
* The identifier of the reserved-node exchange request.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ReservedNodeExchangeStatus withReservedNodeExchangeRequestId(String reservedNodeExchangeRequestId) {
setReservedNodeExchangeRequestId(reservedNodeExchangeRequestId);
return this;
}
/**
*
* The status of the reserved-node exchange request. Statuses include in-progress and requested.
*
*
* @param status
* The status of the reserved-node exchange request. Statuses include in-progress and requested.
* @see ReservedNodeExchangeStatusType
*/
public void setStatus(String status) {
this.status = status;
}
/**
*
* The status of the reserved-node exchange request. Statuses include in-progress and requested.
*
*
* @return The status of the reserved-node exchange request. Statuses include in-progress and requested.
* @see ReservedNodeExchangeStatusType
*/
public String getStatus() {
return this.status;
}
/**
*
* The status of the reserved-node exchange request. Statuses include in-progress and requested.
*
*
* @param status
* The status of the reserved-node exchange request. Statuses include in-progress and requested.
* @return Returns a reference to this object so that method calls can be chained together.
* @see ReservedNodeExchangeStatusType
*/
public ReservedNodeExchangeStatus withStatus(String status) {
setStatus(status);
return this;
}
/**
*
* The status of the reserved-node exchange request. Statuses include in-progress and requested.
*
*
* @param status
* The status of the reserved-node exchange request. Statuses include in-progress and requested.
* @return Returns a reference to this object so that method calls can be chained together.
* @see ReservedNodeExchangeStatusType
*/
public ReservedNodeExchangeStatus withStatus(ReservedNodeExchangeStatusType status) {
this.status = status.toString();
return this;
}
/**
*
* A date and time that indicate when the reserved-node exchange was requested.
*
*
* @param requestTime
* A date and time that indicate when the reserved-node exchange was requested.
*/
public void setRequestTime(java.util.Date requestTime) {
this.requestTime = requestTime;
}
/**
*
* A date and time that indicate when the reserved-node exchange was requested.
*
*
* @return A date and time that indicate when the reserved-node exchange was requested.
*/
public java.util.Date getRequestTime() {
return this.requestTime;
}
/**
*
* A date and time that indicate when the reserved-node exchange was requested.
*
*
* @param requestTime
* A date and time that indicate when the reserved-node exchange was requested.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ReservedNodeExchangeStatus withRequestTime(java.util.Date requestTime) {
setRequestTime(requestTime);
return this;
}
/**
*
* The identifier of the source reserved node.
*
*
* @param sourceReservedNodeId
* The identifier of the source reserved node.
*/
public void setSourceReservedNodeId(String sourceReservedNodeId) {
this.sourceReservedNodeId = sourceReservedNodeId;
}
/**
*
* The identifier of the source reserved node.
*
*
* @return The identifier of the source reserved node.
*/
public String getSourceReservedNodeId() {
return this.sourceReservedNodeId;
}
/**
*
* The identifier of the source reserved node.
*
*
* @param sourceReservedNodeId
* The identifier of the source reserved node.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ReservedNodeExchangeStatus withSourceReservedNodeId(String sourceReservedNodeId) {
setSourceReservedNodeId(sourceReservedNodeId);
return this;
}
/**
*
* The source reserved-node type, for example ra3.4xlarge.
*
*
* @param sourceReservedNodeType
* The source reserved-node type, for example ra3.4xlarge.
*/
public void setSourceReservedNodeType(String sourceReservedNodeType) {
this.sourceReservedNodeType = sourceReservedNodeType;
}
/**
*
* The source reserved-node type, for example ra3.4xlarge.
*
*
* @return The source reserved-node type, for example ra3.4xlarge.
*/
public String getSourceReservedNodeType() {
return this.sourceReservedNodeType;
}
/**
*
* The source reserved-node type, for example ra3.4xlarge.
*
*
* @param sourceReservedNodeType
* The source reserved-node type, for example ra3.4xlarge.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ReservedNodeExchangeStatus withSourceReservedNodeType(String sourceReservedNodeType) {
setSourceReservedNodeType(sourceReservedNodeType);
return this;
}
/**
*
* The source reserved-node count in the cluster.
*
*
* @param sourceReservedNodeCount
* The source reserved-node count in the cluster.
*/
public void setSourceReservedNodeCount(Integer sourceReservedNodeCount) {
this.sourceReservedNodeCount = sourceReservedNodeCount;
}
/**
*
* The source reserved-node count in the cluster.
*
*
* @return The source reserved-node count in the cluster.
*/
public Integer getSourceReservedNodeCount() {
return this.sourceReservedNodeCount;
}
/**
*
* The source reserved-node count in the cluster.
*
*
* @param sourceReservedNodeCount
* The source reserved-node count in the cluster.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ReservedNodeExchangeStatus withSourceReservedNodeCount(Integer sourceReservedNodeCount) {
setSourceReservedNodeCount(sourceReservedNodeCount);
return this;
}
/**
*
* The identifier of the target reserved node offering.
*
*
* @param targetReservedNodeOfferingId
* The identifier of the target reserved node offering.
*/
public void setTargetReservedNodeOfferingId(String targetReservedNodeOfferingId) {
this.targetReservedNodeOfferingId = targetReservedNodeOfferingId;
}
/**
*
* The identifier of the target reserved node offering.
*
*
* @return The identifier of the target reserved node offering.
*/
public String getTargetReservedNodeOfferingId() {
return this.targetReservedNodeOfferingId;
}
/**
*
* The identifier of the target reserved node offering.
*
*
* @param targetReservedNodeOfferingId
* The identifier of the target reserved node offering.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ReservedNodeExchangeStatus withTargetReservedNodeOfferingId(String targetReservedNodeOfferingId) {
setTargetReservedNodeOfferingId(targetReservedNodeOfferingId);
return this;
}
/**
*
* The node type of the target reserved node, for example ra3.4xlarge.
*
*
* @param targetReservedNodeType
* The node type of the target reserved node, for example ra3.4xlarge.
*/
public void setTargetReservedNodeType(String targetReservedNodeType) {
this.targetReservedNodeType = targetReservedNodeType;
}
/**
*
* The node type of the target reserved node, for example ra3.4xlarge.
*
*
* @return The node type of the target reserved node, for example ra3.4xlarge.
*/
public String getTargetReservedNodeType() {
return this.targetReservedNodeType;
}
/**
*
* The node type of the target reserved node, for example ra3.4xlarge.
*
*
* @param targetReservedNodeType
* The node type of the target reserved node, for example ra3.4xlarge.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ReservedNodeExchangeStatus withTargetReservedNodeType(String targetReservedNodeType) {
setTargetReservedNodeType(targetReservedNodeType);
return this;
}
/**
*
* The count of target reserved nodes in the cluster.
*
*
* @param targetReservedNodeCount
* The count of target reserved nodes in the cluster.
*/
public void setTargetReservedNodeCount(Integer targetReservedNodeCount) {
this.targetReservedNodeCount = targetReservedNodeCount;
}
/**
*
* The count of target reserved nodes in the cluster.
*
*
* @return The count of target reserved nodes in the cluster.
*/
public Integer getTargetReservedNodeCount() {
return this.targetReservedNodeCount;
}
/**
*
* The count of target reserved nodes in the cluster.
*
*
* @param targetReservedNodeCount
* The count of target reserved nodes in the cluster.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ReservedNodeExchangeStatus withTargetReservedNodeCount(Integer targetReservedNodeCount) {
setTargetReservedNodeCount(targetReservedNodeCount);
return this;
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getReservedNodeExchangeRequestId() != null)
sb.append("ReservedNodeExchangeRequestId: ").append(getReservedNodeExchangeRequestId()).append(",");
if (getStatus() != null)
sb.append("Status: ").append(getStatus()).append(",");
if (getRequestTime() != null)
sb.append("RequestTime: ").append(getRequestTime()).append(",");
if (getSourceReservedNodeId() != null)
sb.append("SourceReservedNodeId: ").append(getSourceReservedNodeId()).append(",");
if (getSourceReservedNodeType() != null)
sb.append("SourceReservedNodeType: ").append(getSourceReservedNodeType()).append(",");
if (getSourceReservedNodeCount() != null)
sb.append("SourceReservedNodeCount: ").append(getSourceReservedNodeCount()).append(",");
if (getTargetReservedNodeOfferingId() != null)
sb.append("TargetReservedNodeOfferingId: ").append(getTargetReservedNodeOfferingId()).append(",");
if (getTargetReservedNodeType() != null)
sb.append("TargetReservedNodeType: ").append(getTargetReservedNodeType()).append(",");
if (getTargetReservedNodeCount() != null)
sb.append("TargetReservedNodeCount: ").append(getTargetReservedNodeCount());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof ReservedNodeExchangeStatus == false)
return false;
ReservedNodeExchangeStatus other = (ReservedNodeExchangeStatus) obj;
if (other.getReservedNodeExchangeRequestId() == null ^ this.getReservedNodeExchangeRequestId() == null)
return false;
if (other.getReservedNodeExchangeRequestId() != null
&& other.getReservedNodeExchangeRequestId().equals(this.getReservedNodeExchangeRequestId()) == false)
return false;
if (other.getStatus() == null ^ this.getStatus() == null)
return false;
if (other.getStatus() != null && other.getStatus().equals(this.getStatus()) == false)
return false;
if (other.getRequestTime() == null ^ this.getRequestTime() == null)
return false;
if (other.getRequestTime() != null && other.getRequestTime().equals(this.getRequestTime()) == false)
return false;
if (other.getSourceReservedNodeId() == null ^ this.getSourceReservedNodeId() == null)
return false;
if (other.getSourceReservedNodeId() != null && other.getSourceReservedNodeId().equals(this.getSourceReservedNodeId()) == false)
return false;
if (other.getSourceReservedNodeType() == null ^ this.getSourceReservedNodeType() == null)
return false;
if (other.getSourceReservedNodeType() != null && other.getSourceReservedNodeType().equals(this.getSourceReservedNodeType()) == false)
return false;
if (other.getSourceReservedNodeCount() == null ^ this.getSourceReservedNodeCount() == null)
return false;
if (other.getSourceReservedNodeCount() != null && other.getSourceReservedNodeCount().equals(this.getSourceReservedNodeCount()) == false)
return false;
if (other.getTargetReservedNodeOfferingId() == null ^ this.getTargetReservedNodeOfferingId() == null)
return false;
if (other.getTargetReservedNodeOfferingId() != null && other.getTargetReservedNodeOfferingId().equals(this.getTargetReservedNodeOfferingId()) == false)
return false;
if (other.getTargetReservedNodeType() == null ^ this.getTargetReservedNodeType() == null)
return false;
if (other.getTargetReservedNodeType() != null && other.getTargetReservedNodeType().equals(this.getTargetReservedNodeType()) == false)
return false;
if (other.getTargetReservedNodeCount() == null ^ this.getTargetReservedNodeCount() == null)
return false;
if (other.getTargetReservedNodeCount() != null && other.getTargetReservedNodeCount().equals(this.getTargetReservedNodeCount()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getReservedNodeExchangeRequestId() == null) ? 0 : getReservedNodeExchangeRequestId().hashCode());
hashCode = prime * hashCode + ((getStatus() == null) ? 0 : getStatus().hashCode());
hashCode = prime * hashCode + ((getRequestTime() == null) ? 0 : getRequestTime().hashCode());
hashCode = prime * hashCode + ((getSourceReservedNodeId() == null) ? 0 : getSourceReservedNodeId().hashCode());
hashCode = prime * hashCode + ((getSourceReservedNodeType() == null) ? 0 : getSourceReservedNodeType().hashCode());
hashCode = prime * hashCode + ((getSourceReservedNodeCount() == null) ? 0 : getSourceReservedNodeCount().hashCode());
hashCode = prime * hashCode + ((getTargetReservedNodeOfferingId() == null) ? 0 : getTargetReservedNodeOfferingId().hashCode());
hashCode = prime * hashCode + ((getTargetReservedNodeType() == null) ? 0 : getTargetReservedNodeType().hashCode());
hashCode = prime * hashCode + ((getTargetReservedNodeCount() == null) ? 0 : getTargetReservedNodeCount().hashCode());
return hashCode;
}
@Override
public ReservedNodeExchangeStatus clone() {
try {
return (ReservedNodeExchangeStatus) super.clone();
} catch (CloneNotSupportedException e) {
throw new IllegalStateException("Got a CloneNotSupportedException from Object.clone() " + "even though we're Cloneable!", e);
}
}
}