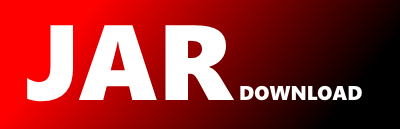
com.amazonaws.services.redshift.model.GetClusterCredentialsRequest Maven / Gradle / Ivy
Show all versions of aws-java-sdk-redshift Show documentation
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.redshift.model;
import java.io.Serializable;
import javax.annotation.Generated;
import com.amazonaws.AmazonWebServiceRequest;
/**
*
* The request parameters to get cluster credentials.
*
*
* @see AWS API
* Documentation
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class GetClusterCredentialsRequest extends com.amazonaws.AmazonWebServiceRequest implements Serializable, Cloneable {
/**
*
* The name of a database user. If a user name matching DbUser
exists in the database, the temporary
* user credentials have the same permissions as the existing user. If DbUser
doesn't exist in the
* database and Autocreate
is True
, a new user is created using the value for
* DbUser
with PUBLIC permissions. If a database user matching the value for DbUser
* doesn't exist and Autocreate
is False
, then the command succeeds but the connection
* attempt will fail because the user doesn't exist in the database.
*
*
* For more information, see CREATE
* USER in the Amazon Redshift Database Developer Guide.
*
*
* Constraints:
*
*
* -
*
* Must be 1 to 64 alphanumeric characters or hyphens. The user name can't be PUBLIC
.
*
*
* -
*
* Must contain uppercase or lowercase letters, numbers, underscore, plus sign, period (dot), at symbol (@), or
* hyphen.
*
*
* -
*
* First character must be a letter.
*
*
* -
*
* Must not contain a colon ( : ) or slash ( / ).
*
*
* -
*
* Cannot be a reserved word. A list of reserved words can be found in Reserved Words in the Amazon Redshift
* Database Developer Guide.
*
*
*
*/
private String dbUser;
/**
*
* The name of a database that DbUser
is authorized to log on to. If DbName
is not
* specified, DbUser
can log on to any existing database.
*
*
* Constraints:
*
*
* -
*
* Must be 1 to 64 alphanumeric characters or hyphens
*
*
* -
*
* Must contain uppercase or lowercase letters, numbers, underscore, plus sign, period (dot), at symbol (@), or
* hyphen.
*
*
* -
*
* First character must be a letter.
*
*
* -
*
* Must not contain a colon ( : ) or slash ( / ).
*
*
* -
*
* Cannot be a reserved word. A list of reserved words can be found in Reserved Words in the Amazon Redshift
* Database Developer Guide.
*
*
*
*/
private String dbName;
/**
*
* The unique identifier of the cluster that contains the database for which you are requesting credentials. This
* parameter is case sensitive.
*
*/
private String clusterIdentifier;
/**
*
* The number of seconds until the returned temporary password expires.
*
*
* Constraint: minimum 900, maximum 3600.
*
*
* Default: 900
*
*/
private Integer durationSeconds;
/**
*
* Create a database user with the name specified for the user named in DbUser
if one does not exist.
*
*/
private Boolean autoCreate;
/**
*
* A list of the names of existing database groups that the user named in DbUser
will join for the
* current session, in addition to any group memberships for an existing user. If not specified, a new user is added
* only to PUBLIC.
*
*
* Database group name constraints
*
*
* -
*
* Must be 1 to 64 alphanumeric characters or hyphens
*
*
* -
*
* Must contain only lowercase letters, numbers, underscore, plus sign, period (dot), at symbol (@), or hyphen.
*
*
* -
*
* First character must be a letter.
*
*
* -
*
* Must not contain a colon ( : ) or slash ( / ).
*
*
* -
*
* Cannot be a reserved word. A list of reserved words can be found in Reserved Words in the Amazon Redshift
* Database Developer Guide.
*
*
*
*/
private com.amazonaws.internal.SdkInternalList dbGroups;
/**
*
* The custom domain name for the cluster credentials.
*
*/
private String customDomainName;
/**
*
* The name of a database user. If a user name matching DbUser
exists in the database, the temporary
* user credentials have the same permissions as the existing user. If DbUser
doesn't exist in the
* database and Autocreate
is True
, a new user is created using the value for
* DbUser
with PUBLIC permissions. If a database user matching the value for DbUser
* doesn't exist and Autocreate
is False
, then the command succeeds but the connection
* attempt will fail because the user doesn't exist in the database.
*
*
* For more information, see CREATE
* USER in the Amazon Redshift Database Developer Guide.
*
*
* Constraints:
*
*
* -
*
* Must be 1 to 64 alphanumeric characters or hyphens. The user name can't be PUBLIC
.
*
*
* -
*
* Must contain uppercase or lowercase letters, numbers, underscore, plus sign, period (dot), at symbol (@), or
* hyphen.
*
*
* -
*
* First character must be a letter.
*
*
* -
*
* Must not contain a colon ( : ) or slash ( / ).
*
*
* -
*
* Cannot be a reserved word. A list of reserved words can be found in Reserved Words in the Amazon Redshift
* Database Developer Guide.
*
*
*
*
* @param dbUser
* The name of a database user. If a user name matching DbUser
exists in the database, the
* temporary user credentials have the same permissions as the existing user. If DbUser
doesn't
* exist in the database and Autocreate
is True
, a new user is created using the
* value for DbUser
with PUBLIC permissions. If a database user matching the value for
* DbUser
doesn't exist and Autocreate
is False
, then the command
* succeeds but the connection attempt will fail because the user doesn't exist in the database.
*
* For more information, see CREATE USER in the Amazon
* Redshift Database Developer Guide.
*
*
* Constraints:
*
*
* -
*
* Must be 1 to 64 alphanumeric characters or hyphens. The user name can't be PUBLIC
.
*
*
* -
*
* Must contain uppercase or lowercase letters, numbers, underscore, plus sign, period (dot), at symbol (@),
* or hyphen.
*
*
* -
*
* First character must be a letter.
*
*
* -
*
* Must not contain a colon ( : ) or slash ( / ).
*
*
* -
*
* Cannot be a reserved word. A list of reserved words can be found in Reserved Words in the Amazon
* Redshift Database Developer Guide.
*
*
*/
public void setDbUser(String dbUser) {
this.dbUser = dbUser;
}
/**
*
* The name of a database user. If a user name matching DbUser
exists in the database, the temporary
* user credentials have the same permissions as the existing user. If DbUser
doesn't exist in the
* database and Autocreate
is True
, a new user is created using the value for
* DbUser
with PUBLIC permissions. If a database user matching the value for DbUser
* doesn't exist and Autocreate
is False
, then the command succeeds but the connection
* attempt will fail because the user doesn't exist in the database.
*
*
* For more information, see CREATE
* USER in the Amazon Redshift Database Developer Guide.
*
*
* Constraints:
*
*
* -
*
* Must be 1 to 64 alphanumeric characters or hyphens. The user name can't be PUBLIC
.
*
*
* -
*
* Must contain uppercase or lowercase letters, numbers, underscore, plus sign, period (dot), at symbol (@), or
* hyphen.
*
*
* -
*
* First character must be a letter.
*
*
* -
*
* Must not contain a colon ( : ) or slash ( / ).
*
*
* -
*
* Cannot be a reserved word. A list of reserved words can be found in Reserved Words in the Amazon Redshift
* Database Developer Guide.
*
*
*
*
* @return The name of a database user. If a user name matching DbUser
exists in the database, the
* temporary user credentials have the same permissions as the existing user. If DbUser
doesn't
* exist in the database and Autocreate
is True
, a new user is created using the
* value for DbUser
with PUBLIC permissions. If a database user matching the value for
* DbUser
doesn't exist and Autocreate
is False
, then the command
* succeeds but the connection attempt will fail because the user doesn't exist in the database.
*
* For more information, see CREATE USER in the Amazon
* Redshift Database Developer Guide.
*
*
* Constraints:
*
*
* -
*
* Must be 1 to 64 alphanumeric characters or hyphens. The user name can't be PUBLIC
.
*
*
* -
*
* Must contain uppercase or lowercase letters, numbers, underscore, plus sign, period (dot), at symbol (@),
* or hyphen.
*
*
* -
*
* First character must be a letter.
*
*
* -
*
* Must not contain a colon ( : ) or slash ( / ).
*
*
* -
*
* Cannot be a reserved word. A list of reserved words can be found in Reserved Words in the Amazon
* Redshift Database Developer Guide.
*
*
*/
public String getDbUser() {
return this.dbUser;
}
/**
*
* The name of a database user. If a user name matching DbUser
exists in the database, the temporary
* user credentials have the same permissions as the existing user. If DbUser
doesn't exist in the
* database and Autocreate
is True
, a new user is created using the value for
* DbUser
with PUBLIC permissions. If a database user matching the value for DbUser
* doesn't exist and Autocreate
is False
, then the command succeeds but the connection
* attempt will fail because the user doesn't exist in the database.
*
*
* For more information, see CREATE
* USER in the Amazon Redshift Database Developer Guide.
*
*
* Constraints:
*
*
* -
*
* Must be 1 to 64 alphanumeric characters or hyphens. The user name can't be PUBLIC
.
*
*
* -
*
* Must contain uppercase or lowercase letters, numbers, underscore, plus sign, period (dot), at symbol (@), or
* hyphen.
*
*
* -
*
* First character must be a letter.
*
*
* -
*
* Must not contain a colon ( : ) or slash ( / ).
*
*
* -
*
* Cannot be a reserved word. A list of reserved words can be found in Reserved Words in the Amazon Redshift
* Database Developer Guide.
*
*
*
*
* @param dbUser
* The name of a database user. If a user name matching DbUser
exists in the database, the
* temporary user credentials have the same permissions as the existing user. If DbUser
doesn't
* exist in the database and Autocreate
is True
, a new user is created using the
* value for DbUser
with PUBLIC permissions. If a database user matching the value for
* DbUser
doesn't exist and Autocreate
is False
, then the command
* succeeds but the connection attempt will fail because the user doesn't exist in the database.
*
* For more information, see CREATE USER in the Amazon
* Redshift Database Developer Guide.
*
*
* Constraints:
*
*
* -
*
* Must be 1 to 64 alphanumeric characters or hyphens. The user name can't be PUBLIC
.
*
*
* -
*
* Must contain uppercase or lowercase letters, numbers, underscore, plus sign, period (dot), at symbol (@),
* or hyphen.
*
*
* -
*
* First character must be a letter.
*
*
* -
*
* Must not contain a colon ( : ) or slash ( / ).
*
*
* -
*
* Cannot be a reserved word. A list of reserved words can be found in Reserved Words in the Amazon
* Redshift Database Developer Guide.
*
*
* @return Returns a reference to this object so that method calls can be chained together.
*/
public GetClusterCredentialsRequest withDbUser(String dbUser) {
setDbUser(dbUser);
return this;
}
/**
*
* The name of a database that DbUser
is authorized to log on to. If DbName
is not
* specified, DbUser
can log on to any existing database.
*
*
* Constraints:
*
*
* -
*
* Must be 1 to 64 alphanumeric characters or hyphens
*
*
* -
*
* Must contain uppercase or lowercase letters, numbers, underscore, plus sign, period (dot), at symbol (@), or
* hyphen.
*
*
* -
*
* First character must be a letter.
*
*
* -
*
* Must not contain a colon ( : ) or slash ( / ).
*
*
* -
*
* Cannot be a reserved word. A list of reserved words can be found in Reserved Words in the Amazon Redshift
* Database Developer Guide.
*
*
*
*
* @param dbName
* The name of a database that DbUser
is authorized to log on to. If DbName
is not
* specified, DbUser
can log on to any existing database.
*
* Constraints:
*
*
* -
*
* Must be 1 to 64 alphanumeric characters or hyphens
*
*
* -
*
* Must contain uppercase or lowercase letters, numbers, underscore, plus sign, period (dot), at symbol (@),
* or hyphen.
*
*
* -
*
* First character must be a letter.
*
*
* -
*
* Must not contain a colon ( : ) or slash ( / ).
*
*
* -
*
* Cannot be a reserved word. A list of reserved words can be found in Reserved Words in the Amazon
* Redshift Database Developer Guide.
*
*
*/
public void setDbName(String dbName) {
this.dbName = dbName;
}
/**
*
* The name of a database that DbUser
is authorized to log on to. If DbName
is not
* specified, DbUser
can log on to any existing database.
*
*
* Constraints:
*
*
* -
*
* Must be 1 to 64 alphanumeric characters or hyphens
*
*
* -
*
* Must contain uppercase or lowercase letters, numbers, underscore, plus sign, period (dot), at symbol (@), or
* hyphen.
*
*
* -
*
* First character must be a letter.
*
*
* -
*
* Must not contain a colon ( : ) or slash ( / ).
*
*
* -
*
* Cannot be a reserved word. A list of reserved words can be found in Reserved Words in the Amazon Redshift
* Database Developer Guide.
*
*
*
*
* @return The name of a database that DbUser
is authorized to log on to. If DbName
is not
* specified, DbUser
can log on to any existing database.
*
* Constraints:
*
*
* -
*
* Must be 1 to 64 alphanumeric characters or hyphens
*
*
* -
*
* Must contain uppercase or lowercase letters, numbers, underscore, plus sign, period (dot), at symbol (@),
* or hyphen.
*
*
* -
*
* First character must be a letter.
*
*
* -
*
* Must not contain a colon ( : ) or slash ( / ).
*
*
* -
*
* Cannot be a reserved word. A list of reserved words can be found in Reserved Words in the Amazon
* Redshift Database Developer Guide.
*
*
*/
public String getDbName() {
return this.dbName;
}
/**
*
* The name of a database that DbUser
is authorized to log on to. If DbName
is not
* specified, DbUser
can log on to any existing database.
*
*
* Constraints:
*
*
* -
*
* Must be 1 to 64 alphanumeric characters or hyphens
*
*
* -
*
* Must contain uppercase or lowercase letters, numbers, underscore, plus sign, period (dot), at symbol (@), or
* hyphen.
*
*
* -
*
* First character must be a letter.
*
*
* -
*
* Must not contain a colon ( : ) or slash ( / ).
*
*
* -
*
* Cannot be a reserved word. A list of reserved words can be found in Reserved Words in the Amazon Redshift
* Database Developer Guide.
*
*
*
*
* @param dbName
* The name of a database that DbUser
is authorized to log on to. If DbName
is not
* specified, DbUser
can log on to any existing database.
*
* Constraints:
*
*
* -
*
* Must be 1 to 64 alphanumeric characters or hyphens
*
*
* -
*
* Must contain uppercase or lowercase letters, numbers, underscore, plus sign, period (dot), at symbol (@),
* or hyphen.
*
*
* -
*
* First character must be a letter.
*
*
* -
*
* Must not contain a colon ( : ) or slash ( / ).
*
*
* -
*
* Cannot be a reserved word. A list of reserved words can be found in Reserved Words in the Amazon
* Redshift Database Developer Guide.
*
*
* @return Returns a reference to this object so that method calls can be chained together.
*/
public GetClusterCredentialsRequest withDbName(String dbName) {
setDbName(dbName);
return this;
}
/**
*
* The unique identifier of the cluster that contains the database for which you are requesting credentials. This
* parameter is case sensitive.
*
*
* @param clusterIdentifier
* The unique identifier of the cluster that contains the database for which you are requesting credentials.
* This parameter is case sensitive.
*/
public void setClusterIdentifier(String clusterIdentifier) {
this.clusterIdentifier = clusterIdentifier;
}
/**
*
* The unique identifier of the cluster that contains the database for which you are requesting credentials. This
* parameter is case sensitive.
*
*
* @return The unique identifier of the cluster that contains the database for which you are requesting credentials.
* This parameter is case sensitive.
*/
public String getClusterIdentifier() {
return this.clusterIdentifier;
}
/**
*
* The unique identifier of the cluster that contains the database for which you are requesting credentials. This
* parameter is case sensitive.
*
*
* @param clusterIdentifier
* The unique identifier of the cluster that contains the database for which you are requesting credentials.
* This parameter is case sensitive.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public GetClusterCredentialsRequest withClusterIdentifier(String clusterIdentifier) {
setClusterIdentifier(clusterIdentifier);
return this;
}
/**
*
* The number of seconds until the returned temporary password expires.
*
*
* Constraint: minimum 900, maximum 3600.
*
*
* Default: 900
*
*
* @param durationSeconds
* The number of seconds until the returned temporary password expires.
*
* Constraint: minimum 900, maximum 3600.
*
*
* Default: 900
*/
public void setDurationSeconds(Integer durationSeconds) {
this.durationSeconds = durationSeconds;
}
/**
*
* The number of seconds until the returned temporary password expires.
*
*
* Constraint: minimum 900, maximum 3600.
*
*
* Default: 900
*
*
* @return The number of seconds until the returned temporary password expires.
*
* Constraint: minimum 900, maximum 3600.
*
*
* Default: 900
*/
public Integer getDurationSeconds() {
return this.durationSeconds;
}
/**
*
* The number of seconds until the returned temporary password expires.
*
*
* Constraint: minimum 900, maximum 3600.
*
*
* Default: 900
*
*
* @param durationSeconds
* The number of seconds until the returned temporary password expires.
*
* Constraint: minimum 900, maximum 3600.
*
*
* Default: 900
* @return Returns a reference to this object so that method calls can be chained together.
*/
public GetClusterCredentialsRequest withDurationSeconds(Integer durationSeconds) {
setDurationSeconds(durationSeconds);
return this;
}
/**
*
* Create a database user with the name specified for the user named in DbUser
if one does not exist.
*
*
* @param autoCreate
* Create a database user with the name specified for the user named in DbUser
if one does not
* exist.
*/
public void setAutoCreate(Boolean autoCreate) {
this.autoCreate = autoCreate;
}
/**
*
* Create a database user with the name specified for the user named in DbUser
if one does not exist.
*
*
* @return Create a database user with the name specified for the user named in DbUser
if one does not
* exist.
*/
public Boolean getAutoCreate() {
return this.autoCreate;
}
/**
*
* Create a database user with the name specified for the user named in DbUser
if one does not exist.
*
*
* @param autoCreate
* Create a database user with the name specified for the user named in DbUser
if one does not
* exist.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public GetClusterCredentialsRequest withAutoCreate(Boolean autoCreate) {
setAutoCreate(autoCreate);
return this;
}
/**
*
* Create a database user with the name specified for the user named in DbUser
if one does not exist.
*
*
* @return Create a database user with the name specified for the user named in DbUser
if one does not
* exist.
*/
public Boolean isAutoCreate() {
return this.autoCreate;
}
/**
*
* A list of the names of existing database groups that the user named in DbUser
will join for the
* current session, in addition to any group memberships for an existing user. If not specified, a new user is added
* only to PUBLIC.
*
*
* Database group name constraints
*
*
* -
*
* Must be 1 to 64 alphanumeric characters or hyphens
*
*
* -
*
* Must contain only lowercase letters, numbers, underscore, plus sign, period (dot), at symbol (@), or hyphen.
*
*
* -
*
* First character must be a letter.
*
*
* -
*
* Must not contain a colon ( : ) or slash ( / ).
*
*
* -
*
* Cannot be a reserved word. A list of reserved words can be found in Reserved Words in the Amazon Redshift
* Database Developer Guide.
*
*
*
*
* @return A list of the names of existing database groups that the user named in DbUser
will join for
* the current session, in addition to any group memberships for an existing user. If not specified, a new
* user is added only to PUBLIC.
*
* Database group name constraints
*
*
* -
*
* Must be 1 to 64 alphanumeric characters or hyphens
*
*
* -
*
* Must contain only lowercase letters, numbers, underscore, plus sign, period (dot), at symbol (@), or
* hyphen.
*
*
* -
*
* First character must be a letter.
*
*
* -
*
* Must not contain a colon ( : ) or slash ( / ).
*
*
* -
*
* Cannot be a reserved word. A list of reserved words can be found in Reserved Words in the Amazon
* Redshift Database Developer Guide.
*
*
*/
public java.util.List getDbGroups() {
if (dbGroups == null) {
dbGroups = new com.amazonaws.internal.SdkInternalList();
}
return dbGroups;
}
/**
*
* A list of the names of existing database groups that the user named in DbUser
will join for the
* current session, in addition to any group memberships for an existing user. If not specified, a new user is added
* only to PUBLIC.
*
*
* Database group name constraints
*
*
* -
*
* Must be 1 to 64 alphanumeric characters or hyphens
*
*
* -
*
* Must contain only lowercase letters, numbers, underscore, plus sign, period (dot), at symbol (@), or hyphen.
*
*
* -
*
* First character must be a letter.
*
*
* -
*
* Must not contain a colon ( : ) or slash ( / ).
*
*
* -
*
* Cannot be a reserved word. A list of reserved words can be found in Reserved Words in the Amazon Redshift
* Database Developer Guide.
*
*
*
*
* @param dbGroups
* A list of the names of existing database groups that the user named in DbUser
will join for
* the current session, in addition to any group memberships for an existing user. If not specified, a new
* user is added only to PUBLIC.
*
* Database group name constraints
*
*
* -
*
* Must be 1 to 64 alphanumeric characters or hyphens
*
*
* -
*
* Must contain only lowercase letters, numbers, underscore, plus sign, period (dot), at symbol (@), or
* hyphen.
*
*
* -
*
* First character must be a letter.
*
*
* -
*
* Must not contain a colon ( : ) or slash ( / ).
*
*
* -
*
* Cannot be a reserved word. A list of reserved words can be found in Reserved Words in the Amazon
* Redshift Database Developer Guide.
*
*
*/
public void setDbGroups(java.util.Collection dbGroups) {
if (dbGroups == null) {
this.dbGroups = null;
return;
}
this.dbGroups = new com.amazonaws.internal.SdkInternalList(dbGroups);
}
/**
*
* A list of the names of existing database groups that the user named in DbUser
will join for the
* current session, in addition to any group memberships for an existing user. If not specified, a new user is added
* only to PUBLIC.
*
*
* Database group name constraints
*
*
* -
*
* Must be 1 to 64 alphanumeric characters or hyphens
*
*
* -
*
* Must contain only lowercase letters, numbers, underscore, plus sign, period (dot), at symbol (@), or hyphen.
*
*
* -
*
* First character must be a letter.
*
*
* -
*
* Must not contain a colon ( : ) or slash ( / ).
*
*
* -
*
* Cannot be a reserved word. A list of reserved words can be found in Reserved Words in the Amazon Redshift
* Database Developer Guide.
*
*
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setDbGroups(java.util.Collection)} or {@link #withDbGroups(java.util.Collection)} if you want to override
* the existing values.
*
*
* @param dbGroups
* A list of the names of existing database groups that the user named in DbUser
will join for
* the current session, in addition to any group memberships for an existing user. If not specified, a new
* user is added only to PUBLIC.
*
* Database group name constraints
*
*
* -
*
* Must be 1 to 64 alphanumeric characters or hyphens
*
*
* -
*
* Must contain only lowercase letters, numbers, underscore, plus sign, period (dot), at symbol (@), or
* hyphen.
*
*
* -
*
* First character must be a letter.
*
*
* -
*
* Must not contain a colon ( : ) or slash ( / ).
*
*
* -
*
* Cannot be a reserved word. A list of reserved words can be found in Reserved Words in the Amazon
* Redshift Database Developer Guide.
*
*
* @return Returns a reference to this object so that method calls can be chained together.
*/
public GetClusterCredentialsRequest withDbGroups(String... dbGroups) {
if (this.dbGroups == null) {
setDbGroups(new com.amazonaws.internal.SdkInternalList(dbGroups.length));
}
for (String ele : dbGroups) {
this.dbGroups.add(ele);
}
return this;
}
/**
*
* A list of the names of existing database groups that the user named in DbUser
will join for the
* current session, in addition to any group memberships for an existing user. If not specified, a new user is added
* only to PUBLIC.
*
*
* Database group name constraints
*
*
* -
*
* Must be 1 to 64 alphanumeric characters or hyphens
*
*
* -
*
* Must contain only lowercase letters, numbers, underscore, plus sign, period (dot), at symbol (@), or hyphen.
*
*
* -
*
* First character must be a letter.
*
*
* -
*
* Must not contain a colon ( : ) or slash ( / ).
*
*
* -
*
* Cannot be a reserved word. A list of reserved words can be found in Reserved Words in the Amazon Redshift
* Database Developer Guide.
*
*
*
*
* @param dbGroups
* A list of the names of existing database groups that the user named in DbUser
will join for
* the current session, in addition to any group memberships for an existing user. If not specified, a new
* user is added only to PUBLIC.
*
* Database group name constraints
*
*
* -
*
* Must be 1 to 64 alphanumeric characters or hyphens
*
*
* -
*
* Must contain only lowercase letters, numbers, underscore, plus sign, period (dot), at symbol (@), or
* hyphen.
*
*
* -
*
* First character must be a letter.
*
*
* -
*
* Must not contain a colon ( : ) or slash ( / ).
*
*
* -
*
* Cannot be a reserved word. A list of reserved words can be found in Reserved Words in the Amazon
* Redshift Database Developer Guide.
*
*
* @return Returns a reference to this object so that method calls can be chained together.
*/
public GetClusterCredentialsRequest withDbGroups(java.util.Collection dbGroups) {
setDbGroups(dbGroups);
return this;
}
/**
*
* The custom domain name for the cluster credentials.
*
*
* @param customDomainName
* The custom domain name for the cluster credentials.
*/
public void setCustomDomainName(String customDomainName) {
this.customDomainName = customDomainName;
}
/**
*
* The custom domain name for the cluster credentials.
*
*
* @return The custom domain name for the cluster credentials.
*/
public String getCustomDomainName() {
return this.customDomainName;
}
/**
*
* The custom domain name for the cluster credentials.
*
*
* @param customDomainName
* The custom domain name for the cluster credentials.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public GetClusterCredentialsRequest withCustomDomainName(String customDomainName) {
setCustomDomainName(customDomainName);
return this;
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getDbUser() != null)
sb.append("DbUser: ").append(getDbUser()).append(",");
if (getDbName() != null)
sb.append("DbName: ").append(getDbName()).append(",");
if (getClusterIdentifier() != null)
sb.append("ClusterIdentifier: ").append(getClusterIdentifier()).append(",");
if (getDurationSeconds() != null)
sb.append("DurationSeconds: ").append(getDurationSeconds()).append(",");
if (getAutoCreate() != null)
sb.append("AutoCreate: ").append(getAutoCreate()).append(",");
if (getDbGroups() != null)
sb.append("DbGroups: ").append(getDbGroups()).append(",");
if (getCustomDomainName() != null)
sb.append("CustomDomainName: ").append(getCustomDomainName());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof GetClusterCredentialsRequest == false)
return false;
GetClusterCredentialsRequest other = (GetClusterCredentialsRequest) obj;
if (other.getDbUser() == null ^ this.getDbUser() == null)
return false;
if (other.getDbUser() != null && other.getDbUser().equals(this.getDbUser()) == false)
return false;
if (other.getDbName() == null ^ this.getDbName() == null)
return false;
if (other.getDbName() != null && other.getDbName().equals(this.getDbName()) == false)
return false;
if (other.getClusterIdentifier() == null ^ this.getClusterIdentifier() == null)
return false;
if (other.getClusterIdentifier() != null && other.getClusterIdentifier().equals(this.getClusterIdentifier()) == false)
return false;
if (other.getDurationSeconds() == null ^ this.getDurationSeconds() == null)
return false;
if (other.getDurationSeconds() != null && other.getDurationSeconds().equals(this.getDurationSeconds()) == false)
return false;
if (other.getAutoCreate() == null ^ this.getAutoCreate() == null)
return false;
if (other.getAutoCreate() != null && other.getAutoCreate().equals(this.getAutoCreate()) == false)
return false;
if (other.getDbGroups() == null ^ this.getDbGroups() == null)
return false;
if (other.getDbGroups() != null && other.getDbGroups().equals(this.getDbGroups()) == false)
return false;
if (other.getCustomDomainName() == null ^ this.getCustomDomainName() == null)
return false;
if (other.getCustomDomainName() != null && other.getCustomDomainName().equals(this.getCustomDomainName()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getDbUser() == null) ? 0 : getDbUser().hashCode());
hashCode = prime * hashCode + ((getDbName() == null) ? 0 : getDbName().hashCode());
hashCode = prime * hashCode + ((getClusterIdentifier() == null) ? 0 : getClusterIdentifier().hashCode());
hashCode = prime * hashCode + ((getDurationSeconds() == null) ? 0 : getDurationSeconds().hashCode());
hashCode = prime * hashCode + ((getAutoCreate() == null) ? 0 : getAutoCreate().hashCode());
hashCode = prime * hashCode + ((getDbGroups() == null) ? 0 : getDbGroups().hashCode());
hashCode = prime * hashCode + ((getCustomDomainName() == null) ? 0 : getCustomDomainName().hashCode());
return hashCode;
}
@Override
public GetClusterCredentialsRequest clone() {
return (GetClusterCredentialsRequest) super.clone();
}
}