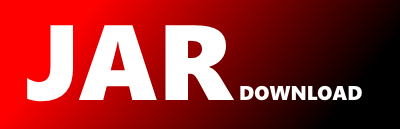
com.amazonaws.services.redshift.AmazonRedshiftClient Maven / Gradle / Ivy
Show all versions of aws-java-sdk-redshift Show documentation
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.redshift;
import org.w3c.dom.*;
import java.net.*;
import java.util.*;
import javax.annotation.Generated;
import org.apache.commons.logging.*;
import com.amazonaws.*;
import com.amazonaws.annotation.SdkInternalApi;
import com.amazonaws.auth.*;
import com.amazonaws.handlers.*;
import com.amazonaws.http.*;
import com.amazonaws.internal.*;
import com.amazonaws.internal.auth.*;
import com.amazonaws.metrics.*;
import com.amazonaws.regions.*;
import com.amazonaws.transform.*;
import com.amazonaws.util.*;
import com.amazonaws.protocol.json.*;
import com.amazonaws.util.AWSRequestMetrics.Field;
import com.amazonaws.annotation.ThreadSafe;
import com.amazonaws.client.AwsSyncClientParams;
import com.amazonaws.client.builder.AdvancedConfig;
import com.amazonaws.services.redshift.AmazonRedshiftClientBuilder;
import com.amazonaws.services.redshift.waiters.AmazonRedshiftWaiters;
import com.amazonaws.AmazonServiceException;
import com.amazonaws.services.redshift.model.*;
import com.amazonaws.services.redshift.model.transform.*;
/**
* Client for accessing Amazon Redshift. All service calls made using this client are blocking, and will not return
* until the service call completes.
*
* Amazon Redshift
*
* Overview
*
*
* This is an interface reference for Amazon Redshift. It contains documentation for one of the programming or command
* line interfaces you can use to manage Amazon Redshift clusters. Note that Amazon Redshift is asynchronous, which
* means that some interfaces may require techniques, such as polling or asynchronous callback handlers, to determine
* when a command has been applied. In this reference, the parameter descriptions indicate whether a change is applied
* immediately, on the next instance reboot, or during the next maintenance window. For a summary of the Amazon Redshift
* cluster management interfaces, go to Using the Amazon Redshift Management
* Interfaces.
*
*
* Amazon Redshift manages all the work of setting up, operating, and scaling a data warehouse: provisioning capacity,
* monitoring and backing up the cluster, and applying patches and upgrades to the Amazon Redshift engine. You can focus
* on using your data to acquire new insights for your business and customers.
*
*
* If you are a first-time user of Amazon Redshift, we recommend that you begin by reading the Amazon Redshift Getting Started
* Guide.
*
*
* If you are a database developer, the Amazon
* Redshift Database Developer Guide explains how to design, build, query, and maintain the databases that make up
* your data warehouse.
*
*/
@ThreadSafe
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class AmazonRedshiftClient extends AmazonWebServiceClient implements AmazonRedshift {
/** Provider for AWS credentials. */
private final AWSCredentialsProvider awsCredentialsProvider;
private static final Log log = LogFactory.getLog(AmazonRedshift.class);
/** Default signing name for the service. */
private static final String DEFAULT_SIGNING_NAME = "redshift";
private volatile AmazonRedshiftWaiters waiters;
/** Client configuration factory providing ClientConfigurations tailored to this client */
protected static final ClientConfigurationFactory configFactory = new ClientConfigurationFactory();
private final AdvancedConfig advancedConfig;
/**
* Map of exception unmarshallers for all modeled exceptions
*/
private final Map> exceptionUnmarshallersMap = new HashMap>();
/**
* List of exception unmarshallers for all modeled exceptions Even though this exceptionUnmarshallers is not used in
* Clients, this is not removed since this was directly used by Client extended classes. Using this list can cause
* performance impact.
*/
protected final List> exceptionUnmarshallers = new ArrayList>();
protected Unmarshaller defaultUnmarshaller;
/**
* Constructs a new client to invoke service methods on Amazon Redshift. A credentials provider chain will be used
* that searches for credentials in this order:
*
* - Environment Variables - AWS_ACCESS_KEY_ID and AWS_SECRET_KEY
* - Java System Properties - aws.accessKeyId and aws.secretKey
* - Instance profile credentials delivered through the Amazon EC2 metadata service
*
*
*
* All service calls made using this new client object are blocking, and will not return until the service call
* completes.
*
* @see DefaultAWSCredentialsProviderChain
* @deprecated use {@link AmazonRedshiftClientBuilder#defaultClient()}
*/
@Deprecated
public AmazonRedshiftClient() {
this(DefaultAWSCredentialsProviderChain.getInstance(), configFactory.getConfig());
}
/**
* Constructs a new client to invoke service methods on Amazon Redshift. A credentials provider chain will be used
* that searches for credentials in this order:
*
* - Environment Variables - AWS_ACCESS_KEY_ID and AWS_SECRET_KEY
* - Java System Properties - aws.accessKeyId and aws.secretKey
* - Instance profile credentials delivered through the Amazon EC2 metadata service
*
*
*
* All service calls made using this new client object are blocking, and will not return until the service call
* completes.
*
* @param clientConfiguration
* The client configuration options controlling how this client connects to Amazon Redshift (ex: proxy
* settings, retry counts, etc.).
*
* @see DefaultAWSCredentialsProviderChain
* @deprecated use {@link AmazonRedshiftClientBuilder#withClientConfiguration(ClientConfiguration)}
*/
@Deprecated
public AmazonRedshiftClient(ClientConfiguration clientConfiguration) {
this(DefaultAWSCredentialsProviderChain.getInstance(), clientConfiguration);
}
/**
* Constructs a new client to invoke service methods on Amazon Redshift using the specified AWS account credentials.
*
*
* All service calls made using this new client object are blocking, and will not return until the service call
* completes.
*
* @param awsCredentials
* The AWS credentials (access key ID and secret key) to use when authenticating with AWS services.
* @deprecated use {@link AmazonRedshiftClientBuilder#withCredentials(AWSCredentialsProvider)} for example:
* {@code AmazonRedshiftClientBuilder.standard().withCredentials(new AWSStaticCredentialsProvider(awsCredentials)).build();}
*/
@Deprecated
public AmazonRedshiftClient(AWSCredentials awsCredentials) {
this(awsCredentials, configFactory.getConfig());
}
/**
* Constructs a new client to invoke service methods on Amazon Redshift using the specified AWS account credentials
* and client configuration options.
*
*
* All service calls made using this new client object are blocking, and will not return until the service call
* completes.
*
* @param awsCredentials
* The AWS credentials (access key ID and secret key) to use when authenticating with AWS services.
* @param clientConfiguration
* The client configuration options controlling how this client connects to Amazon Redshift (ex: proxy
* settings, retry counts, etc.).
* @deprecated use {@link AmazonRedshiftClientBuilder#withCredentials(AWSCredentialsProvider)} and
* {@link AmazonRedshiftClientBuilder#withClientConfiguration(ClientConfiguration)}
*/
@Deprecated
public AmazonRedshiftClient(AWSCredentials awsCredentials, ClientConfiguration clientConfiguration) {
super(clientConfiguration);
this.awsCredentialsProvider = new StaticCredentialsProvider(awsCredentials);
this.advancedConfig = AdvancedConfig.EMPTY;
init();
}
/**
* Constructs a new client to invoke service methods on Amazon Redshift using the specified AWS account credentials
* provider.
*
*
* All service calls made using this new client object are blocking, and will not return until the service call
* completes.
*
* @param awsCredentialsProvider
* The AWS credentials provider which will provide credentials to authenticate requests with AWS services.
* @deprecated use {@link AmazonRedshiftClientBuilder#withCredentials(AWSCredentialsProvider)}
*/
@Deprecated
public AmazonRedshiftClient(AWSCredentialsProvider awsCredentialsProvider) {
this(awsCredentialsProvider, configFactory.getConfig());
}
/**
* Constructs a new client to invoke service methods on Amazon Redshift using the specified AWS account credentials
* provider and client configuration options.
*
*
* All service calls made using this new client object are blocking, and will not return until the service call
* completes.
*
* @param awsCredentialsProvider
* The AWS credentials provider which will provide credentials to authenticate requests with AWS services.
* @param clientConfiguration
* The client configuration options controlling how this client connects to Amazon Redshift (ex: proxy
* settings, retry counts, etc.).
* @deprecated use {@link AmazonRedshiftClientBuilder#withCredentials(AWSCredentialsProvider)} and
* {@link AmazonRedshiftClientBuilder#withClientConfiguration(ClientConfiguration)}
*/
@Deprecated
public AmazonRedshiftClient(AWSCredentialsProvider awsCredentialsProvider, ClientConfiguration clientConfiguration) {
this(awsCredentialsProvider, clientConfiguration, null);
}
/**
* Constructs a new client to invoke service methods on Amazon Redshift using the specified AWS account credentials
* provider, client configuration options, and request metric collector.
*
*
* All service calls made using this new client object are blocking, and will not return until the service call
* completes.
*
* @param awsCredentialsProvider
* The AWS credentials provider which will provide credentials to authenticate requests with AWS services.
* @param clientConfiguration
* The client configuration options controlling how this client connects to Amazon Redshift (ex: proxy
* settings, retry counts, etc.).
* @param requestMetricCollector
* optional request metric collector
* @deprecated use {@link AmazonRedshiftClientBuilder#withCredentials(AWSCredentialsProvider)} and
* {@link AmazonRedshiftClientBuilder#withClientConfiguration(ClientConfiguration)} and
* {@link AmazonRedshiftClientBuilder#withMetricsCollector(RequestMetricCollector)}
*/
@Deprecated
public AmazonRedshiftClient(AWSCredentialsProvider awsCredentialsProvider, ClientConfiguration clientConfiguration,
RequestMetricCollector requestMetricCollector) {
super(clientConfiguration, requestMetricCollector);
this.awsCredentialsProvider = awsCredentialsProvider;
this.advancedConfig = AdvancedConfig.EMPTY;
init();
}
public static AmazonRedshiftClientBuilder builder() {
return AmazonRedshiftClientBuilder.standard();
}
/**
* Constructs a new client to invoke service methods on Amazon Redshift using the specified parameters.
*
*
* All service calls made using this new client object are blocking, and will not return until the service call
* completes.
*
* @param clientParams
* Object providing client parameters.
*/
AmazonRedshiftClient(AwsSyncClientParams clientParams) {
this(clientParams, false);
}
/**
* Constructs a new client to invoke service methods on Amazon Redshift using the specified parameters.
*
*
* All service calls made using this new client object are blocking, and will not return until the service call
* completes.
*
* @param clientParams
* Object providing client parameters.
*/
AmazonRedshiftClient(AwsSyncClientParams clientParams, boolean endpointDiscoveryEnabled) {
super(clientParams);
this.awsCredentialsProvider = clientParams.getCredentialsProvider();
this.advancedConfig = clientParams.getAdvancedConfig();
init();
}
private void init() {
if (exceptionUnmarshallersMap.get("InvalidHsmConfigurationStateFault") == null) {
exceptionUnmarshallersMap.put("InvalidHsmConfigurationStateFault", new InvalidHsmConfigurationStateExceptionUnmarshaller());
}
exceptionUnmarshallers.add(new InvalidHsmConfigurationStateExceptionUnmarshaller());
if (exceptionUnmarshallersMap.get("SnapshotScheduleAlreadyExists") == null) {
exceptionUnmarshallersMap.put("SnapshotScheduleAlreadyExists", new SnapshotScheduleAlreadyExistsExceptionUnmarshaller());
}
exceptionUnmarshallers.add(new SnapshotScheduleAlreadyExistsExceptionUnmarshaller());
if (exceptionUnmarshallersMap.get("SnapshotScheduleQuotaExceeded") == null) {
exceptionUnmarshallersMap.put("SnapshotScheduleQuotaExceeded", new SnapshotScheduleQuotaExceededExceptionUnmarshaller());
}
exceptionUnmarshallers.add(new SnapshotScheduleQuotaExceededExceptionUnmarshaller());
if (exceptionUnmarshallersMap.get("InvalidSubscriptionStateFault") == null) {
exceptionUnmarshallersMap.put("InvalidSubscriptionStateFault", new InvalidSubscriptionStateExceptionUnmarshaller());
}
exceptionUnmarshallers.add(new InvalidSubscriptionStateExceptionUnmarshaller());
if (exceptionUnmarshallersMap.get("UnauthorizedPartnerIntegration") == null) {
exceptionUnmarshallersMap.put("UnauthorizedPartnerIntegration", new UnauthorizedPartnerIntegrationExceptionUnmarshaller());
}
exceptionUnmarshallers.add(new UnauthorizedPartnerIntegrationExceptionUnmarshaller());
if (exceptionUnmarshallersMap.get("InvalidUsageLimit") == null) {
exceptionUnmarshallersMap.put("InvalidUsageLimit", new InvalidUsageLimitExceptionUnmarshaller());
}
exceptionUnmarshallers.add(new InvalidUsageLimitExceptionUnmarshaller());
if (exceptionUnmarshallersMap.get("IntegrationNotFoundFault") == null) {
exceptionUnmarshallersMap.put("IntegrationNotFoundFault", new IntegrationNotFoundExceptionUnmarshaller());
}
exceptionUnmarshallers.add(new IntegrationNotFoundExceptionUnmarshaller());
if (exceptionUnmarshallersMap.get("InvalidRestore") == null) {
exceptionUnmarshallersMap.put("InvalidRestore", new InvalidRestoreExceptionUnmarshaller());
}
exceptionUnmarshallers.add(new InvalidRestoreExceptionUnmarshaller());
if (exceptionUnmarshallersMap.get("SnapshotCopyDisabledFault") == null) {
exceptionUnmarshallersMap.put("SnapshotCopyDisabledFault", new SnapshotCopyDisabledExceptionUnmarshaller());
}
exceptionUnmarshallers.add(new SnapshotCopyDisabledExceptionUnmarshaller());
if (exceptionUnmarshallersMap.get("ReservedNodeExchangeNotFond") == null) {
exceptionUnmarshallersMap.put("ReservedNodeExchangeNotFond", new ReservedNodeExchangeNotFoundExceptionUnmarshaller());
}
exceptionUnmarshallers.add(new ReservedNodeExchangeNotFoundExceptionUnmarshaller());
if (exceptionUnmarshallersMap.get("RedshiftIdcApplicationQuotaExceeded") == null) {
exceptionUnmarshallersMap.put("RedshiftIdcApplicationQuotaExceeded", new RedshiftIdcApplicationQuotaExceededExceptionUnmarshaller());
}
exceptionUnmarshallers.add(new RedshiftIdcApplicationQuotaExceededExceptionUnmarshaller());
if (exceptionUnmarshallersMap.get("ClusterSubnetGroupQuotaExceeded") == null) {
exceptionUnmarshallersMap.put("ClusterSubnetGroupQuotaExceeded", new ClusterSubnetGroupQuotaExceededExceptionUnmarshaller());
}
exceptionUnmarshallers.add(new ClusterSubnetGroupQuotaExceededExceptionUnmarshaller());
if (exceptionUnmarshallersMap.get("Ipv6CidrBlockNotFoundFault") == null) {
exceptionUnmarshallersMap.put("Ipv6CidrBlockNotFoundFault", new Ipv6CidrBlockNotFoundExceptionUnmarshaller());
}
exceptionUnmarshallers.add(new Ipv6CidrBlockNotFoundExceptionUnmarshaller());
if (exceptionUnmarshallersMap.get("InvalidSchedule") == null) {
exceptionUnmarshallersMap.put("InvalidSchedule", new InvalidScheduleExceptionUnmarshaller());
}
exceptionUnmarshallers.add(new InvalidScheduleExceptionUnmarshaller());
if (exceptionUnmarshallersMap.get("DependentServiceRequestThrottlingFault") == null) {
exceptionUnmarshallersMap.put("DependentServiceRequestThrottlingFault", new DependentServiceRequestThrottlingExceptionUnmarshaller());
}
exceptionUnmarshallers.add(new DependentServiceRequestThrottlingExceptionUnmarshaller());
if (exceptionUnmarshallersMap.get("RedshiftIdcApplicationNotExists") == null) {
exceptionUnmarshallersMap.put("RedshiftIdcApplicationNotExists", new RedshiftIdcApplicationNotExistsExceptionUnmarshaller());
}
exceptionUnmarshallers.add(new RedshiftIdcApplicationNotExistsExceptionUnmarshaller());
if (exceptionUnmarshallersMap.get("BucketNotFoundFault") == null) {
exceptionUnmarshallersMap.put("BucketNotFoundFault", new BucketNotFoundExceptionUnmarshaller());
}
exceptionUnmarshallers.add(new BucketNotFoundExceptionUnmarshaller());
if (exceptionUnmarshallersMap.get("LimitExceededFault") == null) {
exceptionUnmarshallersMap.put("LimitExceededFault", new LimitExceededExceptionUnmarshaller());
}
exceptionUnmarshallers.add(new LimitExceededExceptionUnmarshaller());
if (exceptionUnmarshallersMap.get("EndpointAlreadyExists") == null) {
exceptionUnmarshallersMap.put("EndpointAlreadyExists", new EndpointAlreadyExistsExceptionUnmarshaller());
}
exceptionUnmarshallers.add(new EndpointAlreadyExistsExceptionUnmarshaller());
if (exceptionUnmarshallersMap.get("PartnerNotFound") == null) {
exceptionUnmarshallersMap.put("PartnerNotFound", new PartnerNotFoundExceptionUnmarshaller());
}
exceptionUnmarshallers.add(new PartnerNotFoundExceptionUnmarshaller());
if (exceptionUnmarshallersMap.get("ScheduledActionQuotaExceeded") == null) {
exceptionUnmarshallersMap.put("ScheduledActionQuotaExceeded", new ScheduledActionQuotaExceededExceptionUnmarshaller());
}
exceptionUnmarshallers.add(new ScheduledActionQuotaExceededExceptionUnmarshaller());
if (exceptionUnmarshallersMap.get("InvalidTableRestoreArgument") == null) {
exceptionUnmarshallersMap.put("InvalidTableRestoreArgument", new InvalidTableRestoreArgumentExceptionUnmarshaller());
}
exceptionUnmarshallers.add(new InvalidTableRestoreArgumentExceptionUnmarshaller());
if (exceptionUnmarshallersMap.get("SnapshotCopyGrantAlreadyExistsFault") == null) {
exceptionUnmarshallersMap.put("SnapshotCopyGrantAlreadyExistsFault", new SnapshotCopyGrantAlreadyExistsExceptionUnmarshaller());
}
exceptionUnmarshallers.add(new SnapshotCopyGrantAlreadyExistsExceptionUnmarshaller());
if (exceptionUnmarshallersMap.get("SnapshotCopyGrantQuotaExceededFault") == null) {
exceptionUnmarshallersMap.put("SnapshotCopyGrantQuotaExceededFault", new SnapshotCopyGrantQuotaExceededExceptionUnmarshaller());
}
exceptionUnmarshallers.add(new SnapshotCopyGrantQuotaExceededExceptionUnmarshaller());
if (exceptionUnmarshallersMap.get("ClusterNotFound") == null) {
exceptionUnmarshallersMap.put("ClusterNotFound", new ClusterNotFoundExceptionUnmarshaller());
}
exceptionUnmarshallers.add(new ClusterNotFoundExceptionUnmarshaller());
if (exceptionUnmarshallersMap.get("ClusterQuotaExceeded") == null) {
exceptionUnmarshallersMap.put("ClusterQuotaExceeded", new ClusterQuotaExceededExceptionUnmarshaller());
}
exceptionUnmarshallers.add(new ClusterQuotaExceededExceptionUnmarshaller());
if (exceptionUnmarshallersMap.get("UnsupportedOperation") == null) {
exceptionUnmarshallersMap.put("UnsupportedOperation", new UnsupportedOperationExceptionUnmarshaller());
}
exceptionUnmarshallers.add(new UnsupportedOperationExceptionUnmarshaller());
if (exceptionUnmarshallersMap.get("ScheduledActionAlreadyExists") == null) {
exceptionUnmarshallersMap.put("ScheduledActionAlreadyExists", new ScheduledActionAlreadyExistsExceptionUnmarshaller());
}
exceptionUnmarshallers.add(new ScheduledActionAlreadyExistsExceptionUnmarshaller());
if (exceptionUnmarshallersMap.get("InvalidClusterState") == null) {
exceptionUnmarshallersMap.put("InvalidClusterState", new InvalidClusterStateExceptionUnmarshaller());
}
exceptionUnmarshallers.add(new InvalidClusterStateExceptionUnmarshaller());
if (exceptionUnmarshallersMap.get("ClusterSubnetQuotaExceededFault") == null) {
exceptionUnmarshallersMap.put("ClusterSubnetQuotaExceededFault", new ClusterSubnetQuotaExceededExceptionUnmarshaller());
}
exceptionUnmarshallers.add(new ClusterSubnetQuotaExceededExceptionUnmarshaller());
if (exceptionUnmarshallersMap.get("InvalidClusterParameterGroupState") == null) {
exceptionUnmarshallersMap.put("InvalidClusterParameterGroupState", new InvalidClusterParameterGroupStateExceptionUnmarshaller());
}
exceptionUnmarshallers.add(new InvalidClusterParameterGroupStateExceptionUnmarshaller());
if (exceptionUnmarshallersMap.get("ClusterSubnetGroupNotFoundFault") == null) {
exceptionUnmarshallersMap.put("ClusterSubnetGroupNotFoundFault", new ClusterSubnetGroupNotFoundExceptionUnmarshaller());
}
exceptionUnmarshallers.add(new ClusterSubnetGroupNotFoundExceptionUnmarshaller());
if (exceptionUnmarshallersMap.get("SnapshotCopyAlreadyDisabledFault") == null) {
exceptionUnmarshallersMap.put("SnapshotCopyAlreadyDisabledFault", new SnapshotCopyAlreadyDisabledExceptionUnmarshaller());
}
exceptionUnmarshallers.add(new SnapshotCopyAlreadyDisabledExceptionUnmarshaller());
if (exceptionUnmarshallersMap.get("ClusterSnapshotQuotaExceeded") == null) {
exceptionUnmarshallersMap.put("ClusterSnapshotQuotaExceeded", new ClusterSnapshotQuotaExceededExceptionUnmarshaller());
}
exceptionUnmarshallers.add(new ClusterSnapshotQuotaExceededExceptionUnmarshaller());
if (exceptionUnmarshallersMap.get("DependentServiceAccessDenied") == null) {
exceptionUnmarshallersMap.put("DependentServiceAccessDenied", new DependentServiceAccessDeniedExceptionUnmarshaller());
}
exceptionUnmarshallers.add(new DependentServiceAccessDeniedExceptionUnmarshaller());
if (exceptionUnmarshallersMap.get("EndpointAuthorizationAlreadyExists") == null) {
exceptionUnmarshallersMap.put("EndpointAuthorizationAlreadyExists", new EndpointAuthorizationAlreadyExistsExceptionUnmarshaller());
}
exceptionUnmarshallers.add(new EndpointAuthorizationAlreadyExistsExceptionUnmarshaller());
if (exceptionUnmarshallersMap.get("ClusterAlreadyExists") == null) {
exceptionUnmarshallersMap.put("ClusterAlreadyExists", new ClusterAlreadyExistsExceptionUnmarshaller());
}
exceptionUnmarshallers.add(new ClusterAlreadyExistsExceptionUnmarshaller());
if (exceptionUnmarshallersMap.get("ConflictPolicyUpdateFault") == null) {
exceptionUnmarshallersMap.put("ConflictPolicyUpdateFault", new ConflictPolicyUpdateExceptionUnmarshaller());
}
exceptionUnmarshallers.add(new ConflictPolicyUpdateExceptionUnmarshaller());
if (exceptionUnmarshallersMap.get("NumberOfNodesQuotaExceeded") == null) {
exceptionUnmarshallersMap.put("NumberOfNodesQuotaExceeded", new NumberOfNodesQuotaExceededExceptionUnmarshaller());
}
exceptionUnmarshallers.add(new NumberOfNodesQuotaExceededExceptionUnmarshaller());
if (exceptionUnmarshallersMap.get("ClusterSecurityGroupAlreadyExists") == null) {
exceptionUnmarshallersMap.put("ClusterSecurityGroupAlreadyExists", new ClusterSecurityGroupAlreadyExistsExceptionUnmarshaller());
}
exceptionUnmarshallers.add(new ClusterSecurityGroupAlreadyExistsExceptionUnmarshaller());
if (exceptionUnmarshallersMap.get("AuthorizationQuotaExceeded") == null) {
exceptionUnmarshallersMap.put("AuthorizationQuotaExceeded", new AuthorizationQuotaExceededExceptionUnmarshaller());
}
exceptionUnmarshallers.add(new AuthorizationQuotaExceededExceptionUnmarshaller());
if (exceptionUnmarshallersMap.get("ReservedNodeOfferingNotFound") == null) {
exceptionUnmarshallersMap.put("ReservedNodeOfferingNotFound", new ReservedNodeOfferingNotFoundExceptionUnmarshaller());
}
exceptionUnmarshallers.add(new ReservedNodeOfferingNotFoundExceptionUnmarshaller());
if (exceptionUnmarshallersMap.get("EndpointNotFound") == null) {
exceptionUnmarshallersMap.put("EndpointNotFound", new EndpointNotFoundExceptionUnmarshaller());
}
exceptionUnmarshallers.add(new EndpointNotFoundExceptionUnmarshaller());
if (exceptionUnmarshallersMap.get("InProgressTableRestoreQuotaExceededFault") == null) {
exceptionUnmarshallersMap.put("InProgressTableRestoreQuotaExceededFault", new InProgressTableRestoreQuotaExceededExceptionUnmarshaller());
}
exceptionUnmarshallers.add(new InProgressTableRestoreQuotaExceededExceptionUnmarshaller());
if (exceptionUnmarshallersMap.get("AccessToSnapshotDenied") == null) {
exceptionUnmarshallersMap.put("AccessToSnapshotDenied", new AccessToSnapshotDeniedExceptionUnmarshaller());
}
exceptionUnmarshallers.add(new AccessToSnapshotDeniedExceptionUnmarshaller());
if (exceptionUnmarshallersMap.get("InvalidElasticIpFault") == null) {
exceptionUnmarshallersMap.put("InvalidElasticIpFault", new InvalidElasticIpExceptionUnmarshaller());
}
exceptionUnmarshallers.add(new InvalidElasticIpExceptionUnmarshaller());
if (exceptionUnmarshallersMap.get("ResizeNotFound") == null) {
exceptionUnmarshallersMap.put("ResizeNotFound", new ResizeNotFoundExceptionUnmarshaller());
}
exceptionUnmarshallers.add(new ResizeNotFoundExceptionUnmarshaller());
if (exceptionUnmarshallersMap.get("ScheduleDefinitionTypeUnsupported") == null) {
exceptionUnmarshallersMap.put("ScheduleDefinitionTypeUnsupported", new ScheduleDefinitionTypeUnsupportedExceptionUnmarshaller());
}
exceptionUnmarshallers.add(new ScheduleDefinitionTypeUnsupportedExceptionUnmarshaller());
if (exceptionUnmarshallersMap.get("InvalidTagFault") == null) {
exceptionUnmarshallersMap.put("InvalidTagFault", new InvalidTagExceptionUnmarshaller());
}
exceptionUnmarshallers.add(new InvalidTagExceptionUnmarshaller());
if (exceptionUnmarshallersMap.get("HsmClientCertificateAlreadyExistsFault") == null) {
exceptionUnmarshallersMap.put("HsmClientCertificateAlreadyExistsFault", new HsmClientCertificateAlreadyExistsExceptionUnmarshaller());
}
exceptionUnmarshallers.add(new HsmClientCertificateAlreadyExistsExceptionUnmarshaller());
if (exceptionUnmarshallersMap.get("InvalidVPCNetworkStateFault") == null) {
exceptionUnmarshallersMap.put("InvalidVPCNetworkStateFault", new InvalidVPCNetworkStateExceptionUnmarshaller());
}
exceptionUnmarshallers.add(new InvalidVPCNetworkStateExceptionUnmarshaller());
if (exceptionUnmarshallersMap.get("SNSTopicArnNotFound") == null) {
exceptionUnmarshallersMap.put("SNSTopicArnNotFound", new SNSTopicArnNotFoundExceptionUnmarshaller());
}
exceptionUnmarshallers.add(new SNSTopicArnNotFoundExceptionUnmarshaller());
if (exceptionUnmarshallersMap.get("ScheduledActionTypeUnsupported") == null) {
exceptionUnmarshallersMap.put("ScheduledActionTypeUnsupported", new ScheduledActionTypeUnsupportedExceptionUnmarshaller());
}
exceptionUnmarshallers.add(new ScheduledActionTypeUnsupportedExceptionUnmarshaller());
if (exceptionUnmarshallersMap.get("ClusterParameterGroupQuotaExceeded") == null) {
exceptionUnmarshallersMap.put("ClusterParameterGroupQuotaExceeded", new ClusterParameterGroupQuotaExceededExceptionUnmarshaller());
}
exceptionUnmarshallers.add(new ClusterParameterGroupQuotaExceededExceptionUnmarshaller());
if (exceptionUnmarshallersMap.get("InvalidPolicyFault") == null) {
exceptionUnmarshallersMap.put("InvalidPolicyFault", new InvalidPolicyExceptionUnmarshaller());
}
exceptionUnmarshallers.add(new InvalidPolicyExceptionUnmarshaller());
if (exceptionUnmarshallersMap.get("UnauthorizedOperation") == null) {
exceptionUnmarshallersMap.put("UnauthorizedOperation", new UnauthorizedOperationExceptionUnmarshaller());
}
exceptionUnmarshallers.add(new UnauthorizedOperationExceptionUnmarshaller());
if (exceptionUnmarshallersMap.get("AuthenticationProfileNotFoundFault") == null) {
exceptionUnmarshallersMap.put("AuthenticationProfileNotFoundFault", new AuthenticationProfileNotFoundExceptionUnmarshaller());
}
exceptionUnmarshallers.add(new AuthenticationProfileNotFoundExceptionUnmarshaller());
if (exceptionUnmarshallersMap.get("SnapshotScheduleNotFound") == null) {
exceptionUnmarshallersMap.put("SnapshotScheduleNotFound", new SnapshotScheduleNotFoundExceptionUnmarshaller());
}
exceptionUnmarshallers.add(new SnapshotScheduleNotFoundExceptionUnmarshaller());
if (exceptionUnmarshallersMap.get("ReservedNodeAlreadyExists") == null) {
exceptionUnmarshallersMap.put("ReservedNodeAlreadyExists", new ReservedNodeAlreadyExistsExceptionUnmarshaller());
}
exceptionUnmarshallers.add(new ReservedNodeAlreadyExistsExceptionUnmarshaller());
if (exceptionUnmarshallersMap.get("InvalidS3BucketNameFault") == null) {
exceptionUnmarshallersMap.put("InvalidS3BucketNameFault", new InvalidS3BucketNameExceptionUnmarshaller());
}
exceptionUnmarshallers.add(new InvalidS3BucketNameExceptionUnmarshaller());
if (exceptionUnmarshallersMap.get("UnsupportedOptionFault") == null) {
exceptionUnmarshallersMap.put("UnsupportedOptionFault", new UnsupportedOptionExceptionUnmarshaller());
}
exceptionUnmarshallers.add(new UnsupportedOptionExceptionUnmarshaller());
if (exceptionUnmarshallersMap.get("InsufficientClusterCapacity") == null) {
exceptionUnmarshallersMap.put("InsufficientClusterCapacity", new InsufficientClusterCapacityExceptionUnmarshaller());
}
exceptionUnmarshallers.add(new InsufficientClusterCapacityExceptionUnmarshaller());
if (exceptionUnmarshallersMap.get("ClusterSubnetGroupAlreadyExists") == null) {
exceptionUnmarshallersMap.put("ClusterSubnetGroupAlreadyExists", new ClusterSubnetGroupAlreadyExistsExceptionUnmarshaller());
}
exceptionUnmarshallers.add(new ClusterSubnetGroupAlreadyExistsExceptionUnmarshaller());
if (exceptionUnmarshallersMap.get("TagLimitExceededFault") == null) {
exceptionUnmarshallersMap.put("TagLimitExceededFault", new TagLimitExceededExceptionUnmarshaller());
}
exceptionUnmarshallers.add(new TagLimitExceededExceptionUnmarshaller());
if (exceptionUnmarshallersMap.get("InvalidClusterSnapshotState") == null) {
exceptionUnmarshallersMap.put("InvalidClusterSnapshotState", new InvalidClusterSnapshotStateExceptionUnmarshaller());
}
exceptionUnmarshallers.add(new InvalidClusterSnapshotStateExceptionUnmarshaller());
if (exceptionUnmarshallersMap.get("InsufficientS3BucketPolicyFault") == null) {
exceptionUnmarshallersMap.put("InsufficientS3BucketPolicyFault", new InsufficientS3BucketPolicyExceptionUnmarshaller());
}
exceptionUnmarshallers.add(new InsufficientS3BucketPolicyExceptionUnmarshaller());
if (exceptionUnmarshallersMap.get("BatchDeleteRequestSizeExceeded") == null) {
exceptionUnmarshallersMap.put("BatchDeleteRequestSizeExceeded", new BatchDeleteRequestSizeExceededExceptionUnmarshaller());
}
exceptionUnmarshallers.add(new BatchDeleteRequestSizeExceededExceptionUnmarshaller());
if (exceptionUnmarshallersMap.get("InvalidS3KeyPrefixFault") == null) {
exceptionUnmarshallersMap.put("InvalidS3KeyPrefixFault", new InvalidS3KeyPrefixExceptionUnmarshaller());
}
exceptionUnmarshallers.add(new InvalidS3KeyPrefixExceptionUnmarshaller());
if (exceptionUnmarshallersMap.get("AuthorizationNotFound") == null) {
exceptionUnmarshallersMap.put("AuthorizationNotFound", new AuthorizationNotFoundExceptionUnmarshaller());
}
exceptionUnmarshallers.add(new AuthorizationNotFoundExceptionUnmarshaller());
if (exceptionUnmarshallersMap.get("ScheduledActionNotFound") == null) {
exceptionUnmarshallersMap.put("ScheduledActionNotFound", new ScheduledActionNotFoundExceptionUnmarshaller());
}
exceptionUnmarshallers.add(new ScheduledActionNotFoundExceptionUnmarshaller());
if (exceptionUnmarshallersMap.get("SubscriptionEventIdNotFound") == null) {
exceptionUnmarshallersMap.put("SubscriptionEventIdNotFound", new SubscriptionEventIdNotFoundExceptionUnmarshaller());
}
exceptionUnmarshallers.add(new SubscriptionEventIdNotFoundExceptionUnmarshaller());
if (exceptionUnmarshallersMap.get("InvalidClusterSnapshotScheduleState") == null) {
exceptionUnmarshallersMap.put("InvalidClusterSnapshotScheduleState", new InvalidClusterSnapshotScheduleStateExceptionUnmarshaller());
}
exceptionUnmarshallers.add(new InvalidClusterSnapshotScheduleStateExceptionUnmarshaller());
if (exceptionUnmarshallersMap.get("EndpointAuthorizationNotFound") == null) {
exceptionUnmarshallersMap.put("EndpointAuthorizationNotFound", new EndpointAuthorizationNotFoundExceptionUnmarshaller());
}
exceptionUnmarshallers.add(new EndpointAuthorizationNotFoundExceptionUnmarshaller());
if (exceptionUnmarshallersMap.get("InvalidClusterTrack") == null) {
exceptionUnmarshallersMap.put("InvalidClusterTrack", new InvalidClusterTrackExceptionUnmarshaller());
}
exceptionUnmarshallers.add(new InvalidClusterTrackExceptionUnmarshaller());
if (exceptionUnmarshallersMap.get("SubscriptionSeverityNotFound") == null) {
exceptionUnmarshallersMap.put("SubscriptionSeverityNotFound", new SubscriptionSeverityNotFoundExceptionUnmarshaller());
}
exceptionUnmarshallers.add(new SubscriptionSeverityNotFoundExceptionUnmarshaller());
if (exceptionUnmarshallersMap.get("InvalidNamespaceFault") == null) {
exceptionUnmarshallersMap.put("InvalidNamespaceFault", new InvalidNamespaceExceptionUnmarshaller());
}
exceptionUnmarshallers.add(new InvalidNamespaceExceptionUnmarshaller());
if (exceptionUnmarshallersMap.get("AccessToClusterDenied") == null) {
exceptionUnmarshallersMap.put("AccessToClusterDenied", new AccessToClusterDeniedExceptionUnmarshaller());
}
exceptionUnmarshallers.add(new AccessToClusterDeniedExceptionUnmarshaller());
if (exceptionUnmarshallersMap.get("CopyToRegionDisabledFault") == null) {
exceptionUnmarshallersMap.put("CopyToRegionDisabledFault", new CopyToRegionDisabledExceptionUnmarshaller());
}
exceptionUnmarshallers.add(new CopyToRegionDisabledExceptionUnmarshaller());
if (exceptionUnmarshallersMap.get("SubscriptionCategoryNotFound") == null) {
exceptionUnmarshallersMap.put("SubscriptionCategoryNotFound", new SubscriptionCategoryNotFoundExceptionUnmarshaller());
}
exceptionUnmarshallers.add(new SubscriptionCategoryNotFoundExceptionUnmarshaller());
if (exceptionUnmarshallersMap.get("SNSInvalidTopic") == null) {
exceptionUnmarshallersMap.put("SNSInvalidTopic", new SNSInvalidTopicExceptionUnmarshaller());
}
exceptionUnmarshallers.add(new SNSInvalidTopicExceptionUnmarshaller());
if (exceptionUnmarshallersMap.get("InvalidEndpointState") == null) {
exceptionUnmarshallersMap.put("InvalidEndpointState", new InvalidEndpointStateExceptionUnmarshaller());
}
exceptionUnmarshallers.add(new InvalidEndpointStateExceptionUnmarshaller());
if (exceptionUnmarshallersMap.get("UnknownSnapshotCopyRegionFault") == null) {
exceptionUnmarshallersMap.put("UnknownSnapshotCopyRegionFault", new UnknownSnapshotCopyRegionExceptionUnmarshaller());
}
exceptionUnmarshallers.add(new UnknownSnapshotCopyRegionExceptionUnmarshaller());
if (exceptionUnmarshallersMap.get("TableRestoreNotFoundFault") == null) {
exceptionUnmarshallersMap.put("TableRestoreNotFoundFault", new TableRestoreNotFoundExceptionUnmarshaller());
}
exceptionUnmarshallers.add(new TableRestoreNotFoundExceptionUnmarshaller());
if (exceptionUnmarshallersMap.get("ResourceNotFoundFault") == null) {
exceptionUnmarshallersMap.put("ResourceNotFoundFault", new ResourceNotFoundExceptionUnmarshaller());
}
exceptionUnmarshallers.add(new ResourceNotFoundExceptionUnmarshaller());
if (exceptionUnmarshallersMap.get("HsmConfigurationQuotaExceededFault") == null) {
exceptionUnmarshallersMap.put("HsmConfigurationQuotaExceededFault", new HsmConfigurationQuotaExceededExceptionUnmarshaller());
}
exceptionUnmarshallers.add(new HsmConfigurationQuotaExceededExceptionUnmarshaller());
if (exceptionUnmarshallersMap.get("SnapshotCopyGrantNotFoundFault") == null) {
exceptionUnmarshallersMap.put("SnapshotCopyGrantNotFoundFault", new SnapshotCopyGrantNotFoundExceptionUnmarshaller());
}
exceptionUnmarshallers.add(new SnapshotCopyGrantNotFoundExceptionUnmarshaller());
if (exceptionUnmarshallersMap.get("ReservedNodeAlreadyMigrated") == null) {
exceptionUnmarshallersMap.put("ReservedNodeAlreadyMigrated", new ReservedNodeAlreadyMigratedExceptionUnmarshaller());
}
exceptionUnmarshallers.add(new ReservedNodeAlreadyMigratedExceptionUnmarshaller());
if (exceptionUnmarshallersMap.get("HsmClientCertificateNotFoundFault") == null) {
exceptionUnmarshallersMap.put("HsmClientCertificateNotFoundFault", new HsmClientCertificateNotFoundExceptionUnmarshaller());
}
exceptionUnmarshallers.add(new HsmClientCertificateNotFoundExceptionUnmarshaller());
if (exceptionUnmarshallersMap.get("SnapshotCopyAlreadyEnabledFault") == null) {
exceptionUnmarshallersMap.put("SnapshotCopyAlreadyEnabledFault", new SnapshotCopyAlreadyEnabledExceptionUnmarshaller());
}
exceptionUnmarshallers.add(new SnapshotCopyAlreadyEnabledExceptionUnmarshaller());
if (exceptionUnmarshallersMap.get("HsmConfigurationAlreadyExistsFault") == null) {
exceptionUnmarshallersMap.put("HsmConfigurationAlreadyExistsFault", new HsmConfigurationAlreadyExistsExceptionUnmarshaller());
}
exceptionUnmarshallers.add(new HsmConfigurationAlreadyExistsExceptionUnmarshaller());
if (exceptionUnmarshallersMap.get("CustomCnameAssociationFault") == null) {
exceptionUnmarshallersMap.put("CustomCnameAssociationFault", new CustomCnameAssociationExceptionUnmarshaller());
}
exceptionUnmarshallers.add(new CustomCnameAssociationExceptionUnmarshaller());
if (exceptionUnmarshallersMap.get("SubscriptionNotFound") == null) {
exceptionUnmarshallersMap.put("SubscriptionNotFound", new SubscriptionNotFoundExceptionUnmarshaller());
}
exceptionUnmarshallers.add(new SubscriptionNotFoundExceptionUnmarshaller());
if (exceptionUnmarshallersMap.get("InvalidReservedNodeState") == null) {
exceptionUnmarshallersMap.put("InvalidReservedNodeState", new InvalidReservedNodeStateExceptionUnmarshaller());
}
exceptionUnmarshallers.add(new InvalidReservedNodeStateExceptionUnmarshaller());
if (exceptionUnmarshallersMap.get("CustomDomainAssociationNotFoundFault") == null) {
exceptionUnmarshallersMap.put("CustomDomainAssociationNotFoundFault", new CustomDomainAssociationNotFoundExceptionUnmarshaller());
}
exceptionUnmarshallers.add(new CustomDomainAssociationNotFoundExceptionUnmarshaller());
if (exceptionUnmarshallersMap.get("SubnetAlreadyInUse") == null) {
exceptionUnmarshallersMap.put("SubnetAlreadyInUse", new SubnetAlreadyInUseExceptionUnmarshaller());
}
exceptionUnmarshallers.add(new SubnetAlreadyInUseExceptionUnmarshaller());
if (exceptionUnmarshallersMap.get("DependentServiceUnavailableFault") == null) {
exceptionUnmarshallersMap.put("DependentServiceUnavailableFault", new DependentServiceUnavailableExceptionUnmarshaller());
}
exceptionUnmarshallers.add(new DependentServiceUnavailableExceptionUnmarshaller());
if (exceptionUnmarshallersMap.get("InvalidAuthorizationState") == null) {
exceptionUnmarshallersMap.put("InvalidAuthorizationState", new InvalidAuthorizationStateExceptionUnmarshaller());
}
exceptionUnmarshallers.add(new InvalidAuthorizationStateExceptionUnmarshaller());
if (exceptionUnmarshallersMap.get("ClusterSecurityGroupNotFound") == null) {
exceptionUnmarshallersMap.put("ClusterSecurityGroupNotFound", new ClusterSecurityGroupNotFoundExceptionUnmarshaller());
}
exceptionUnmarshallers.add(new ClusterSecurityGroupNotFoundExceptionUnmarshaller());
if (exceptionUnmarshallersMap.get("InvalidSubnet") == null) {
exceptionUnmarshallersMap.put("InvalidSubnet", new InvalidSubnetExceptionUnmarshaller());
}
exceptionUnmarshallers.add(new InvalidSubnetExceptionUnmarshaller());
if (exceptionUnmarshallersMap.get("HsmConfigurationNotFoundFault") == null) {
exceptionUnmarshallersMap.put("HsmConfigurationNotFoundFault", new HsmConfigurationNotFoundExceptionUnmarshaller());
}
exceptionUnmarshallers.add(new HsmConfigurationNotFoundExceptionUnmarshaller());
if (exceptionUnmarshallersMap.get("SNSNoAuthorization") == null) {
exceptionUnmarshallersMap.put("SNSNoAuthorization", new SNSNoAuthorizationExceptionUnmarshaller());
}
exceptionUnmarshallers.add(new SNSNoAuthorizationExceptionUnmarshaller());
if (exceptionUnmarshallersMap.get("NumberOfNodesPerClusterLimitExceeded") == null) {
exceptionUnmarshallersMap.put("NumberOfNodesPerClusterLimitExceeded", new NumberOfNodesPerClusterLimitExceededExceptionUnmarshaller());
}
exceptionUnmarshallers.add(new NumberOfNodesPerClusterLimitExceededExceptionUnmarshaller());
if (exceptionUnmarshallersMap.get("AuthenticationProfileQuotaExceededFault") == null) {
exceptionUnmarshallersMap.put("AuthenticationProfileQuotaExceededFault", new AuthenticationProfileQuotaExceededExceptionUnmarshaller());
}
exceptionUnmarshallers.add(new AuthenticationProfileQuotaExceededExceptionUnmarshaller());
if (exceptionUnmarshallersMap.get("EndpointAuthorizationsPerClusterLimitExceeded") == null) {
exceptionUnmarshallersMap.put("EndpointAuthorizationsPerClusterLimitExceeded",
new EndpointAuthorizationsPerClusterLimitExceededExceptionUnmarshaller());
}
exceptionUnmarshallers.add(new EndpointAuthorizationsPerClusterLimitExceededExceptionUnmarshaller());
if (exceptionUnmarshallersMap.get("ClusterSnapshotAlreadyExists") == null) {
exceptionUnmarshallersMap.put("ClusterSnapshotAlreadyExists", new ClusterSnapshotAlreadyExistsExceptionUnmarshaller());
}
exceptionUnmarshallers.add(new ClusterSnapshotAlreadyExistsExceptionUnmarshaller());
if (exceptionUnmarshallersMap.get("InvalidRetentionPeriodFault") == null) {
exceptionUnmarshallersMap.put("InvalidRetentionPeriodFault", new InvalidRetentionPeriodExceptionUnmarshaller());
}
exceptionUnmarshallers.add(new InvalidRetentionPeriodExceptionUnmarshaller());
if (exceptionUnmarshallersMap.get("AuthenticationProfileAlreadyExistsFault") == null) {
exceptionUnmarshallersMap.put("AuthenticationProfileAlreadyExistsFault", new AuthenticationProfileAlreadyExistsExceptionUnmarshaller());
}
exceptionUnmarshallers.add(new AuthenticationProfileAlreadyExistsExceptionUnmarshaller());
if (exceptionUnmarshallersMap.get("EndpointsPerAuthorizationLimitExceeded") == null) {
exceptionUnmarshallersMap.put("EndpointsPerAuthorizationLimitExceeded", new EndpointsPerAuthorizationLimitExceededExceptionUnmarshaller());
}
exceptionUnmarshallers.add(new EndpointsPerAuthorizationLimitExceededExceptionUnmarshaller());
if (exceptionUnmarshallersMap.get("UsageLimitNotFound") == null) {
exceptionUnmarshallersMap.put("UsageLimitNotFound", new UsageLimitNotFoundExceptionUnmarshaller());
}
exceptionUnmarshallers.add(new UsageLimitNotFoundExceptionUnmarshaller());
if (exceptionUnmarshallersMap.get("TableLimitExceeded") == null) {
exceptionUnmarshallersMap.put("TableLimitExceeded", new TableLimitExceededExceptionUnmarshaller());
}
exceptionUnmarshallers.add(new TableLimitExceededExceptionUnmarshaller());
if (exceptionUnmarshallersMap.get("SnapshotScheduleUpdateInProgress") == null) {
exceptionUnmarshallersMap.put("SnapshotScheduleUpdateInProgress", new SnapshotScheduleUpdateInProgressExceptionUnmarshaller());
}
exceptionUnmarshallers.add(new SnapshotScheduleUpdateInProgressExceptionUnmarshaller());
if (exceptionUnmarshallersMap.get("InvalidSnapshotCopyGrantStateFault") == null) {
exceptionUnmarshallersMap.put("InvalidSnapshotCopyGrantStateFault", new InvalidSnapshotCopyGrantStateExceptionUnmarshaller());
}
exceptionUnmarshallers.add(new InvalidSnapshotCopyGrantStateExceptionUnmarshaller());
if (exceptionUnmarshallersMap.get("InvalidHsmClientCertificateStateFault") == null) {
exceptionUnmarshallersMap.put("InvalidHsmClientCertificateStateFault", new InvalidHsmClientCertificateStateExceptionUnmarshaller());
}
exceptionUnmarshallers.add(new InvalidHsmClientCertificateStateExceptionUnmarshaller());
if (exceptionUnmarshallersMap.get("InvalidClusterSubnetGroupStateFault") == null) {
exceptionUnmarshallersMap.put("InvalidClusterSubnetGroupStateFault", new InvalidClusterSubnetGroupStateExceptionUnmarshaller());
}
exceptionUnmarshallers.add(new InvalidClusterSubnetGroupStateExceptionUnmarshaller());
if (exceptionUnmarshallersMap.get("ClusterOnLatestRevision") == null) {
exceptionUnmarshallersMap.put("ClusterOnLatestRevision", new ClusterOnLatestRevisionExceptionUnmarshaller());
}
exceptionUnmarshallers.add(new ClusterOnLatestRevisionExceptionUnmarshaller());
if (exceptionUnmarshallersMap.get("ClusterSnapshotNotFound") == null) {
exceptionUnmarshallersMap.put("ClusterSnapshotNotFound", new ClusterSnapshotNotFoundExceptionUnmarshaller());
}
exceptionUnmarshallers.add(new ClusterSnapshotNotFoundExceptionUnmarshaller());
if (exceptionUnmarshallersMap.get("InvalidDataShareFault") == null) {
exceptionUnmarshallersMap.put("InvalidDataShareFault", new InvalidDataShareExceptionUnmarshaller());
}
exceptionUnmarshallers.add(new InvalidDataShareExceptionUnmarshaller());
if (exceptionUnmarshallersMap.get("BatchModifyClusterSnapshotsLimitExceededFault") == null) {
exceptionUnmarshallersMap.put("BatchModifyClusterSnapshotsLimitExceededFault", new BatchModifyClusterSnapshotsLimitExceededExceptionUnmarshaller());
}
exceptionUnmarshallers.add(new BatchModifyClusterSnapshotsLimitExceededExceptionUnmarshaller());
if (exceptionUnmarshallersMap.get("HsmClientCertificateQuotaExceededFault") == null) {
exceptionUnmarshallersMap.put("HsmClientCertificateQuotaExceededFault", new HsmClientCertificateQuotaExceededExceptionUnmarshaller());
}
exceptionUnmarshallers.add(new HsmClientCertificateQuotaExceededExceptionUnmarshaller());
if (exceptionUnmarshallersMap.get("InvalidClusterSecurityGroupState") == null) {
exceptionUnmarshallersMap.put("InvalidClusterSecurityGroupState", new InvalidClusterSecurityGroupStateExceptionUnmarshaller());
}
exceptionUnmarshallers.add(new InvalidClusterSecurityGroupStateExceptionUnmarshaller());
if (exceptionUnmarshallersMap.get("ReservedNodeQuotaExceeded") == null) {
exceptionUnmarshallersMap.put("ReservedNodeQuotaExceeded", new ReservedNodeQuotaExceededExceptionUnmarshaller());
}
exceptionUnmarshallers.add(new ReservedNodeQuotaExceededExceptionUnmarshaller());
if (exceptionUnmarshallersMap.get("InvalidAuthenticationProfileRequestFault") == null) {
exceptionUnmarshallersMap.put("InvalidAuthenticationProfileRequestFault", new InvalidAuthenticationProfileRequestExceptionUnmarshaller());
}
exceptionUnmarshallers.add(new InvalidAuthenticationProfileRequestExceptionUnmarshaller());
if (exceptionUnmarshallersMap.get("InvalidClusterSubnetStateFault") == null) {
exceptionUnmarshallersMap.put("InvalidClusterSubnetStateFault", new InvalidClusterSubnetStateExceptionUnmarshaller());
}
exceptionUnmarshallers.add(new InvalidClusterSubnetStateExceptionUnmarshaller());
if (exceptionUnmarshallersMap.get("SubscriptionAlreadyExist") == null) {
exceptionUnmarshallersMap.put("SubscriptionAlreadyExist", new SubscriptionAlreadyExistExceptionUnmarshaller());
}
exceptionUnmarshallers.add(new SubscriptionAlreadyExistExceptionUnmarshaller());
if (exceptionUnmarshallersMap.get("ReservedNodeNotFound") == null) {
exceptionUnmarshallersMap.put("ReservedNodeNotFound", new ReservedNodeNotFoundExceptionUnmarshaller());
}
exceptionUnmarshallers.add(new ReservedNodeNotFoundExceptionUnmarshaller());
if (exceptionUnmarshallersMap.get("InvalidScheduledAction") == null) {
exceptionUnmarshallersMap.put("InvalidScheduledAction", new InvalidScheduledActionExceptionUnmarshaller());
}
exceptionUnmarshallers.add(new InvalidScheduledActionExceptionUnmarshaller());
if (exceptionUnmarshallersMap.get("IncompatibleOrderableOptions") == null) {
exceptionUnmarshallersMap.put("IncompatibleOrderableOptions", new IncompatibleOrderableOptionsExceptionUnmarshaller());
}
exceptionUnmarshallers.add(new IncompatibleOrderableOptionsExceptionUnmarshaller());
if (exceptionUnmarshallersMap.get("RedshiftIdcApplicationAlreadyExists") == null) {
exceptionUnmarshallersMap.put("RedshiftIdcApplicationAlreadyExists", new RedshiftIdcApplicationAlreadyExistsExceptionUnmarshaller());
}
exceptionUnmarshallers.add(new RedshiftIdcApplicationAlreadyExistsExceptionUnmarshaller());
if (exceptionUnmarshallersMap.get("EndpointsPerClusterLimitExceeded") == null) {
exceptionUnmarshallersMap.put("EndpointsPerClusterLimitExceeded", new EndpointsPerClusterLimitExceededExceptionUnmarshaller());
}
exceptionUnmarshallers.add(new EndpointsPerClusterLimitExceededExceptionUnmarshaller());
if (exceptionUnmarshallersMap.get("UsageLimitAlreadyExists") == null) {
exceptionUnmarshallersMap.put("UsageLimitAlreadyExists", new UsageLimitAlreadyExistsExceptionUnmarshaller());
}
exceptionUnmarshallers.add(new UsageLimitAlreadyExistsExceptionUnmarshaller());
if (exceptionUnmarshallersMap.get("SourceNotFound") == null) {
exceptionUnmarshallersMap.put("SourceNotFound", new SourceNotFoundExceptionUnmarshaller());
}
exceptionUnmarshallers.add(new SourceNotFoundExceptionUnmarshaller());
if (exceptionUnmarshallersMap.get("ClusterParameterGroupNotFound") == null) {
exceptionUnmarshallersMap.put("ClusterParameterGroupNotFound", new ClusterParameterGroupNotFoundExceptionUnmarshaller());
}
exceptionUnmarshallers.add(new ClusterParameterGroupNotFoundExceptionUnmarshaller());
if (exceptionUnmarshallersMap.get("QuotaExceeded.ClusterSecurityGroup") == null) {
exceptionUnmarshallersMap.put("QuotaExceeded.ClusterSecurityGroup", new ClusterSecurityGroupQuotaExceededExceptionUnmarshaller());
}
exceptionUnmarshallers.add(new ClusterSecurityGroupQuotaExceededExceptionUnmarshaller());
if (exceptionUnmarshallersMap.get("EventSubscriptionQuotaExceeded") == null) {
exceptionUnmarshallersMap.put("EventSubscriptionQuotaExceeded", new EventSubscriptionQuotaExceededExceptionUnmarshaller());
}
exceptionUnmarshallers.add(new EventSubscriptionQuotaExceededExceptionUnmarshaller());
if (exceptionUnmarshallersMap.get("AuthorizationAlreadyExists") == null) {
exceptionUnmarshallersMap.put("AuthorizationAlreadyExists", new AuthorizationAlreadyExistsExceptionUnmarshaller());
}
exceptionUnmarshallers.add(new AuthorizationAlreadyExistsExceptionUnmarshaller());
if (exceptionUnmarshallersMap.get("ClusterParameterGroupAlreadyExists") == null) {
exceptionUnmarshallersMap.put("ClusterParameterGroupAlreadyExists", new ClusterParameterGroupAlreadyExistsExceptionUnmarshaller());
}
exceptionUnmarshallers.add(new ClusterParameterGroupAlreadyExistsExceptionUnmarshaller());
defaultUnmarshaller = new StandardErrorUnmarshaller(com.amazonaws.services.redshift.model.AmazonRedshiftException.class);
exceptionUnmarshallers.add(new StandardErrorUnmarshaller(com.amazonaws.services.redshift.model.AmazonRedshiftException.class));
setServiceNameIntern(DEFAULT_SIGNING_NAME);
setEndpointPrefix(ENDPOINT_PREFIX);
// calling this.setEndPoint(...) will also modify the signer accordingly
this.setEndpoint("redshift.us-east-1.amazonaws.com");
HandlerChainFactory chainFactory = new HandlerChainFactory();
requestHandler2s.addAll(chainFactory.newRequestHandlerChain("/com/amazonaws/services/redshift/request.handlers"));
requestHandler2s.addAll(chainFactory.newRequestHandler2Chain("/com/amazonaws/services/redshift/request.handler2s"));
requestHandler2s.addAll(chainFactory.getGlobalHandlers());
}
/**
*
* Exchanges a DC1 Reserved Node for a DC2 Reserved Node with no changes to the configuration (term, payment type,
* or number of nodes) and no additional costs.
*
*
* @param acceptReservedNodeExchangeRequest
* @return Result of the AcceptReservedNodeExchange operation returned by the service.
* @throws ReservedNodeNotFoundException
* The specified reserved compute node not found.
* @throws InvalidReservedNodeStateException
* Indicates that the Reserved Node being exchanged is not in an active state.
* @throws ReservedNodeAlreadyMigratedException
* Indicates that the reserved node has already been exchanged.
* @throws ReservedNodeOfferingNotFoundException
* Specified offering does not exist.
* @throws UnsupportedOperationException
* The requested operation isn't supported.
* @throws DependentServiceUnavailableException
* Your request cannot be completed because a dependent internal service is temporarily unavailable. Wait 30
* to 60 seconds and try again.
* @throws ReservedNodeAlreadyExistsException
* User already has a reservation with the given identifier.
* @sample AmazonRedshift.AcceptReservedNodeExchange
* @see AWS API Documentation
*/
@Override
public ReservedNode acceptReservedNodeExchange(AcceptReservedNodeExchangeRequest request) {
request = beforeClientExecution(request);
return executeAcceptReservedNodeExchange(request);
}
@SdkInternalApi
final ReservedNode executeAcceptReservedNodeExchange(AcceptReservedNodeExchangeRequest acceptReservedNodeExchangeRequest) {
ExecutionContext executionContext = createExecutionContext(acceptReservedNodeExchangeRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new AcceptReservedNodeExchangeRequestMarshaller().marshall(super.beforeMarshalling(acceptReservedNodeExchangeRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Redshift");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "AcceptReservedNodeExchange");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
StaxResponseHandler responseHandler = new StaxResponseHandler(new ReservedNodeStaxUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Adds a partner integration to a cluster. This operation authorizes a partner to push status updates for the
* specified database. To complete the integration, you also set up the integration on the partner website.
*
*
* @param addPartnerRequest
* @return Result of the AddPartner operation returned by the service.
* @throws PartnerNotFoundException
* The name of the partner was not found.
* @throws ClusterNotFoundException
* The ClusterIdentifier
parameter does not refer to an existing cluster.
* @throws UnauthorizedPartnerIntegrationException
* The partner integration is not authorized.
* @throws UnsupportedOperationException
* The requested operation isn't supported.
* @sample AmazonRedshift.AddPartner
* @see AWS API
* Documentation
*/
@Override
public AddPartnerResult addPartner(AddPartnerRequest request) {
request = beforeClientExecution(request);
return executeAddPartner(request);
}
@SdkInternalApi
final AddPartnerResult executeAddPartner(AddPartnerRequest addPartnerRequest) {
ExecutionContext executionContext = createExecutionContext(addPartnerRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new AddPartnerRequestMarshaller().marshall(super.beforeMarshalling(addPartnerRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Redshift");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "AddPartner");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
StaxResponseHandler responseHandler = new StaxResponseHandler(new AddPartnerResultStaxUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* From a datashare consumer account, associates a datashare with the account (AssociateEntireAccount) or the
* specified namespace (ConsumerArn). If you make this association, the consumer can consume the datashare.
*
*
* @param associateDataShareConsumerRequest
* @return Result of the AssociateDataShareConsumer operation returned by the service.
* @throws InvalidDataShareException
* There is an error with the datashare.
* @throws InvalidNamespaceException
* The namespace isn't valid because the namespace doesn't exist. Provide a valid namespace.
* @sample AmazonRedshift.AssociateDataShareConsumer
* @see AWS API Documentation
*/
@Override
public AssociateDataShareConsumerResult associateDataShareConsumer(AssociateDataShareConsumerRequest request) {
request = beforeClientExecution(request);
return executeAssociateDataShareConsumer(request);
}
@SdkInternalApi
final AssociateDataShareConsumerResult executeAssociateDataShareConsumer(AssociateDataShareConsumerRequest associateDataShareConsumerRequest) {
ExecutionContext executionContext = createExecutionContext(associateDataShareConsumerRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new AssociateDataShareConsumerRequestMarshaller().marshall(super.beforeMarshalling(associateDataShareConsumerRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Redshift");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "AssociateDataShareConsumer");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
StaxResponseHandler responseHandler = new StaxResponseHandler(
new AssociateDataShareConsumerResultStaxUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Adds an inbound (ingress) rule to an Amazon Redshift security group. Depending on whether the application
* accessing your cluster is running on the Internet or an Amazon EC2 instance, you can authorize inbound access to
* either a Classless Interdomain Routing (CIDR)/Internet Protocol (IP) range or to an Amazon EC2 security group.
* You can add as many as 20 ingress rules to an Amazon Redshift security group.
*
*
* If you authorize access to an Amazon EC2 security group, specify EC2SecurityGroupName and
* EC2SecurityGroupOwnerId. The Amazon EC2 security group and Amazon Redshift cluster must be in the same
* Amazon Web Services Region.
*
*
* If you authorize access to a CIDR/IP address range, specify CIDRIP. For an overview of CIDR blocks, see
* the Wikipedia article on Classless
* Inter-Domain Routing.
*
*
* You must also associate the security group with a cluster so that clients running on these IP addresses or the
* EC2 instance are authorized to connect to the cluster. For information about managing security groups, go to Working with Security
* Groups in the Amazon Redshift Cluster Management Guide.
*
*
* @param authorizeClusterSecurityGroupIngressRequest
* @return Result of the AuthorizeClusterSecurityGroupIngress operation returned by the service.
* @throws ClusterSecurityGroupNotFoundException
* The cluster security group name does not refer to an existing cluster security group.
* @throws InvalidClusterSecurityGroupStateException
* The state of the cluster security group is not available
.
* @throws AuthorizationAlreadyExistsException
* The specified CIDR block or EC2 security group is already authorized for the specified cluster security
* group.
* @throws AuthorizationQuotaExceededException
* The authorization quota for the cluster security group has been reached.
* @sample AmazonRedshift.AuthorizeClusterSecurityGroupIngress
* @see AWS API Documentation
*/
@Override
public ClusterSecurityGroup authorizeClusterSecurityGroupIngress(AuthorizeClusterSecurityGroupIngressRequest request) {
request = beforeClientExecution(request);
return executeAuthorizeClusterSecurityGroupIngress(request);
}
@SdkInternalApi
final ClusterSecurityGroup executeAuthorizeClusterSecurityGroupIngress(
AuthorizeClusterSecurityGroupIngressRequest authorizeClusterSecurityGroupIngressRequest) {
ExecutionContext executionContext = createExecutionContext(authorizeClusterSecurityGroupIngressRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new AuthorizeClusterSecurityGroupIngressRequestMarshaller().marshall(super
.beforeMarshalling(authorizeClusterSecurityGroupIngressRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Redshift");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "AuthorizeClusterSecurityGroupIngress");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
StaxResponseHandler responseHandler = new StaxResponseHandler(
new ClusterSecurityGroupStaxUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* From a data producer account, authorizes the sharing of a datashare with one or more consumer accounts or
* managing entities. To authorize a datashare for a data consumer, the producer account must have the correct
* access permissions.
*
*
* @param authorizeDataShareRequest
* @return Result of the AuthorizeDataShare operation returned by the service.
* @throws InvalidDataShareException
* There is an error with the datashare.
* @sample AmazonRedshift.AuthorizeDataShare
* @see AWS
* API Documentation
*/
@Override
public AuthorizeDataShareResult authorizeDataShare(AuthorizeDataShareRequest request) {
request = beforeClientExecution(request);
return executeAuthorizeDataShare(request);
}
@SdkInternalApi
final AuthorizeDataShareResult executeAuthorizeDataShare(AuthorizeDataShareRequest authorizeDataShareRequest) {
ExecutionContext executionContext = createExecutionContext(authorizeDataShareRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new AuthorizeDataShareRequestMarshaller().marshall(super.beforeMarshalling(authorizeDataShareRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Redshift");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "AuthorizeDataShare");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
StaxResponseHandler responseHandler = new StaxResponseHandler(
new AuthorizeDataShareResultStaxUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Grants access to a cluster.
*
*
* @param authorizeEndpointAccessRequest
* @return Result of the AuthorizeEndpointAccess operation returned by the service.
* @throws ClusterNotFoundException
* The ClusterIdentifier
parameter does not refer to an existing cluster.
* @throws EndpointAuthorizationsPerClusterLimitExceededException
* The number of endpoint authorizations per cluster has exceeded its limit.
* @throws UnsupportedOperationException
* The requested operation isn't supported.
* @throws EndpointAuthorizationAlreadyExistsException
* The authorization already exists for this endpoint.
* @throws InvalidAuthorizationStateException
* The status of the authorization is not valid.
* @throws InvalidClusterStateException
* The specified cluster is not in the available
state.
* @sample AmazonRedshift.AuthorizeEndpointAccess
* @see AWS API Documentation
*/
@Override
public AuthorizeEndpointAccessResult authorizeEndpointAccess(AuthorizeEndpointAccessRequest request) {
request = beforeClientExecution(request);
return executeAuthorizeEndpointAccess(request);
}
@SdkInternalApi
final AuthorizeEndpointAccessResult executeAuthorizeEndpointAccess(AuthorizeEndpointAccessRequest authorizeEndpointAccessRequest) {
ExecutionContext executionContext = createExecutionContext(authorizeEndpointAccessRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new AuthorizeEndpointAccessRequestMarshaller().marshall(super.beforeMarshalling(authorizeEndpointAccessRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Redshift");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "AuthorizeEndpointAccess");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
StaxResponseHandler responseHandler = new StaxResponseHandler(
new AuthorizeEndpointAccessResultStaxUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Authorizes the specified Amazon Web Services account to restore the specified snapshot.
*
*
* For more information about working with snapshots, go to Amazon Redshift Snapshots
* in the Amazon Redshift Cluster Management Guide.
*
*
* @param authorizeSnapshotAccessRequest
* @return Result of the AuthorizeSnapshotAccess operation returned by the service.
* @throws ClusterSnapshotNotFoundException
* The snapshot identifier does not refer to an existing cluster snapshot.
* @throws AuthorizationAlreadyExistsException
* The specified CIDR block or EC2 security group is already authorized for the specified cluster security
* group.
* @throws AuthorizationQuotaExceededException
* The authorization quota for the cluster security group has been reached.
* @throws DependentServiceRequestThrottlingException
* The request cannot be completed because a dependent service is throttling requests made by Amazon
* Redshift on your behalf. Wait and retry the request.
* @throws InvalidClusterSnapshotStateException
* The specified cluster snapshot is not in the available
state, or other accounts are
* authorized to access the snapshot.
* @throws LimitExceededException
* The encryption key has exceeded its grant limit in Amazon Web Services KMS.
* @throws UnsupportedOperationException
* The requested operation isn't supported.
* @sample AmazonRedshift.AuthorizeSnapshotAccess
* @see AWS API Documentation
*/
@Override
public Snapshot authorizeSnapshotAccess(AuthorizeSnapshotAccessRequest request) {
request = beforeClientExecution(request);
return executeAuthorizeSnapshotAccess(request);
}
@SdkInternalApi
final Snapshot executeAuthorizeSnapshotAccess(AuthorizeSnapshotAccessRequest authorizeSnapshotAccessRequest) {
ExecutionContext executionContext = createExecutionContext(authorizeSnapshotAccessRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new AuthorizeSnapshotAccessRequestMarshaller().marshall(super.beforeMarshalling(authorizeSnapshotAccessRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Redshift");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "AuthorizeSnapshotAccess");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
StaxResponseHandler responseHandler = new StaxResponseHandler(new SnapshotStaxUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Deletes a set of cluster snapshots.
*
*
* @param batchDeleteClusterSnapshotsRequest
* @return Result of the BatchDeleteClusterSnapshots operation returned by the service.
* @throws BatchDeleteRequestSizeExceededException
* The maximum number for a batch delete of snapshots has been reached. The limit is 100.
* @sample AmazonRedshift.BatchDeleteClusterSnapshots
* @see AWS API Documentation
*/
@Override
public BatchDeleteClusterSnapshotsResult batchDeleteClusterSnapshots(BatchDeleteClusterSnapshotsRequest request) {
request = beforeClientExecution(request);
return executeBatchDeleteClusterSnapshots(request);
}
@SdkInternalApi
final BatchDeleteClusterSnapshotsResult executeBatchDeleteClusterSnapshots(BatchDeleteClusterSnapshotsRequest batchDeleteClusterSnapshotsRequest) {
ExecutionContext executionContext = createExecutionContext(batchDeleteClusterSnapshotsRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new BatchDeleteClusterSnapshotsRequestMarshaller().marshall(super.beforeMarshalling(batchDeleteClusterSnapshotsRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Redshift");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "BatchDeleteClusterSnapshots");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
StaxResponseHandler responseHandler = new StaxResponseHandler(
new BatchDeleteClusterSnapshotsResultStaxUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Modifies the settings for a set of cluster snapshots.
*
*
* @param batchModifyClusterSnapshotsRequest
* @return Result of the BatchModifyClusterSnapshots operation returned by the service.
* @throws InvalidRetentionPeriodException
* The retention period specified is either in the past or is not a valid value.
*
* The value must be either -1 or an integer between 1 and 3,653.
* @throws BatchModifyClusterSnapshotsLimitExceededException
* The maximum number for snapshot identifiers has been reached. The limit is 100.
* @sample AmazonRedshift.BatchModifyClusterSnapshots
* @see AWS API Documentation
*/
@Override
public BatchModifyClusterSnapshotsResult batchModifyClusterSnapshots(BatchModifyClusterSnapshotsRequest request) {
request = beforeClientExecution(request);
return executeBatchModifyClusterSnapshots(request);
}
@SdkInternalApi
final BatchModifyClusterSnapshotsResult executeBatchModifyClusterSnapshots(BatchModifyClusterSnapshotsRequest batchModifyClusterSnapshotsRequest) {
ExecutionContext executionContext = createExecutionContext(batchModifyClusterSnapshotsRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new BatchModifyClusterSnapshotsRequestMarshaller().marshall(super.beforeMarshalling(batchModifyClusterSnapshotsRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Redshift");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "BatchModifyClusterSnapshots");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
StaxResponseHandler responseHandler = new StaxResponseHandler(
new BatchModifyClusterSnapshotsResultStaxUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Cancels a resize operation for a cluster.
*
*
* @param cancelResizeRequest
* @return Result of the CancelResize operation returned by the service.
* @throws ClusterNotFoundException
* The ClusterIdentifier
parameter does not refer to an existing cluster.
* @throws ResizeNotFoundException
* A resize operation for the specified cluster is not found.
* @throws InvalidClusterStateException
* The specified cluster is not in the available
state.
* @throws UnsupportedOperationException
* The requested operation isn't supported.
* @sample AmazonRedshift.CancelResize
* @see AWS API
* Documentation
*/
@Override
public CancelResizeResult cancelResize(CancelResizeRequest request) {
request = beforeClientExecution(request);
return executeCancelResize(request);
}
@SdkInternalApi
final CancelResizeResult executeCancelResize(CancelResizeRequest cancelResizeRequest) {
ExecutionContext executionContext = createExecutionContext(cancelResizeRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new CancelResizeRequestMarshaller().marshall(super.beforeMarshalling(cancelResizeRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Redshift");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "CancelResize");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
StaxResponseHandler responseHandler = new StaxResponseHandler(new CancelResizeResultStaxUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Copies the specified automated cluster snapshot to a new manual cluster snapshot. The source must be an automated
* snapshot and it must be in the available state.
*
*
* When you delete a cluster, Amazon Redshift deletes any automated snapshots of the cluster. Also, when the
* retention period of the snapshot expires, Amazon Redshift automatically deletes it. If you want to keep an
* automated snapshot for a longer period, you can make a manual copy of the snapshot. Manual snapshots are retained
* until you delete them.
*
*
* For more information about working with snapshots, go to Amazon Redshift Snapshots
* in the Amazon Redshift Cluster Management Guide.
*
*
* @param copyClusterSnapshotRequest
* @return Result of the CopyClusterSnapshot operation returned by the service.
* @throws ClusterNotFoundException
* The ClusterIdentifier
parameter does not refer to an existing cluster.
* @throws ClusterSnapshotAlreadyExistsException
* The value specified as a snapshot identifier is already used by an existing snapshot.
* @throws ClusterSnapshotNotFoundException
* The snapshot identifier does not refer to an existing cluster snapshot.
* @throws InvalidClusterSnapshotStateException
* The specified cluster snapshot is not in the available
state, or other accounts are
* authorized to access the snapshot.
* @throws ClusterSnapshotQuotaExceededException
* The request would result in the user exceeding the allowed number of cluster snapshots.
* @throws InvalidRetentionPeriodException
* The retention period specified is either in the past or is not a valid value.
*
* The value must be either -1 or an integer between 1 and 3,653.
* @sample AmazonRedshift.CopyClusterSnapshot
* @see AWS
* API Documentation
*/
@Override
public Snapshot copyClusterSnapshot(CopyClusterSnapshotRequest request) {
request = beforeClientExecution(request);
return executeCopyClusterSnapshot(request);
}
@SdkInternalApi
final Snapshot executeCopyClusterSnapshot(CopyClusterSnapshotRequest copyClusterSnapshotRequest) {
ExecutionContext executionContext = createExecutionContext(copyClusterSnapshotRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new CopyClusterSnapshotRequestMarshaller().marshall(super.beforeMarshalling(copyClusterSnapshotRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Redshift");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "CopyClusterSnapshot");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
StaxResponseHandler responseHandler = new StaxResponseHandler(new SnapshotStaxUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Creates an authentication profile with the specified parameters.
*
*
* @param createAuthenticationProfileRequest
* @return Result of the CreateAuthenticationProfile operation returned by the service.
* @throws AuthenticationProfileAlreadyExistsException
* The authentication profile already exists.
* @throws AuthenticationProfileQuotaExceededException
* The size or number of authentication profiles has exceeded the quota. The maximum length of the JSON
* string and maximum number of authentication profiles is determined by a quota for your account.
* @throws InvalidAuthenticationProfileRequestException
* The authentication profile request is not valid. The profile name can't be null or empty. The
* authentication profile API operation must be available in the Amazon Web Services Region.
* @sample AmazonRedshift.CreateAuthenticationProfile
* @see AWS API Documentation
*/
@Override
public CreateAuthenticationProfileResult createAuthenticationProfile(CreateAuthenticationProfileRequest request) {
request = beforeClientExecution(request);
return executeCreateAuthenticationProfile(request);
}
@SdkInternalApi
final CreateAuthenticationProfileResult executeCreateAuthenticationProfile(CreateAuthenticationProfileRequest createAuthenticationProfileRequest) {
ExecutionContext executionContext = createExecutionContext(createAuthenticationProfileRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new CreateAuthenticationProfileRequestMarshaller().marshall(super.beforeMarshalling(createAuthenticationProfileRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Redshift");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "CreateAuthenticationProfile");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
StaxResponseHandler responseHandler = new StaxResponseHandler(
new CreateAuthenticationProfileResultStaxUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Creates a new cluster with the specified parameters.
*
*
* To create a cluster in Virtual Private Cloud (VPC), you must provide a cluster subnet group name. The cluster
* subnet group identifies the subnets of your VPC that Amazon Redshift uses when creating the cluster. For more
* information about managing clusters, go to Amazon Redshift Clusters
* in the Amazon Redshift Cluster Management Guide.
*
*
* @param createClusterRequest
* @return Result of the CreateCluster operation returned by the service.
* @throws ClusterAlreadyExistsException
* The account already has a cluster with the given identifier.
* @throws InsufficientClusterCapacityException
* The number of nodes specified exceeds the allotted capacity of the cluster.
* @throws ClusterParameterGroupNotFoundException
* The parameter group name does not refer to an existing parameter group.
* @throws ClusterSecurityGroupNotFoundException
* The cluster security group name does not refer to an existing cluster security group.
* @throws ClusterQuotaExceededException
* The request would exceed the allowed number of cluster instances for this account. For information about
* increasing your quota, go to Limits in Amazon
* Redshift in the Amazon Redshift Cluster Management Guide.
* @throws NumberOfNodesQuotaExceededException
* The operation would exceed the number of nodes allotted to the account. For information about increasing
* your quota, go to Limits in Amazon
* Redshift in the Amazon Redshift Cluster Management Guide.
* @throws NumberOfNodesPerClusterLimitExceededException
* The operation would exceed the number of nodes allowed for a cluster.
* @throws ClusterSubnetGroupNotFoundException
* The cluster subnet group name does not refer to an existing cluster subnet group.
* @throws InvalidVPCNetworkStateException
* The cluster subnet group does not cover all Availability Zones.
* @throws InvalidClusterSubnetGroupStateException
* The cluster subnet group cannot be deleted because it is in use.
* @throws InvalidSubnetException
* The requested subnet is not valid, or not all of the subnets are in the same VPC.
* @throws UnauthorizedOperationException
* Your account is not authorized to perform the requested operation.
* @throws HsmClientCertificateNotFoundException
* There is no Amazon Redshift HSM client certificate with the specified identifier.
* @throws HsmConfigurationNotFoundException
* There is no Amazon Redshift HSM configuration with the specified identifier.
* @throws InvalidElasticIpException
* The Elastic IP (EIP) is invalid or cannot be found.
* @throws TagLimitExceededException
* You have exceeded the number of tags allowed.
* @throws InvalidTagException
* The tag is invalid.
* @throws LimitExceededException
* The encryption key has exceeded its grant limit in Amazon Web Services KMS.
* @throws DependentServiceRequestThrottlingException
* The request cannot be completed because a dependent service is throttling requests made by Amazon
* Redshift on your behalf. Wait and retry the request.
* @throws InvalidClusterTrackException
* The provided cluster track name is not valid.
* @throws SnapshotScheduleNotFoundException
* We could not find the specified snapshot schedule.
* @throws InvalidRetentionPeriodException
* The retention period specified is either in the past or is not a valid value.
*
* The value must be either -1 or an integer between 1 and 3,653.
* @throws Ipv6CidrBlockNotFoundException
* There are no subnets in your VPC with associated IPv6 CIDR blocks. To use dual-stack mode, associate an
* IPv6 CIDR block with each subnet in your VPC.
* @throws UnsupportedOperationException
* The requested operation isn't supported.
* @throws RedshiftIdcApplicationNotExistsException
* The application you attempted to find doesn't exist.
* @sample AmazonRedshift.CreateCluster
* @see AWS API
* Documentation
*/
@Override
public Cluster createCluster(CreateClusterRequest request) {
request = beforeClientExecution(request);
return executeCreateCluster(request);
}
@SdkInternalApi
final Cluster executeCreateCluster(CreateClusterRequest createClusterRequest) {
ExecutionContext executionContext = createExecutionContext(createClusterRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new CreateClusterRequestMarshaller().marshall(super.beforeMarshalling(createClusterRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Redshift");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "CreateCluster");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
StaxResponseHandler responseHandler = new StaxResponseHandler(new ClusterStaxUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Creates an Amazon Redshift parameter group.
*
*
* Creating parameter groups is independent of creating clusters. You can associate a cluster with a parameter group
* when you create the cluster. You can also associate an existing cluster with a parameter group after the cluster
* is created by using ModifyCluster.
*
*
* Parameters in the parameter group define specific behavior that applies to the databases you create on the
* cluster. For more information about parameters and parameter groups, go to Amazon Redshift
* Parameter Groups in the Amazon Redshift Cluster Management Guide.
*
*
* @param createClusterParameterGroupRequest
* @return Result of the CreateClusterParameterGroup operation returned by the service.
* @throws ClusterParameterGroupQuotaExceededException
* The request would result in the user exceeding the allowed number of cluster parameter groups. For
* information about increasing your quota, go to Limits in Amazon
* Redshift in the Amazon Redshift Cluster Management Guide.
* @throws ClusterParameterGroupAlreadyExistsException
* A cluster parameter group with the same name already exists.
* @throws TagLimitExceededException
* You have exceeded the number of tags allowed.
* @throws InvalidTagException
* The tag is invalid.
* @sample AmazonRedshift.CreateClusterParameterGroup
* @see AWS API Documentation
*/
@Override
public ClusterParameterGroup createClusterParameterGroup(CreateClusterParameterGroupRequest request) {
request = beforeClientExecution(request);
return executeCreateClusterParameterGroup(request);
}
@SdkInternalApi
final ClusterParameterGroup executeCreateClusterParameterGroup(CreateClusterParameterGroupRequest createClusterParameterGroupRequest) {
ExecutionContext executionContext = createExecutionContext(createClusterParameterGroupRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new CreateClusterParameterGroupRequestMarshaller().marshall(super.beforeMarshalling(createClusterParameterGroupRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Redshift");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "CreateClusterParameterGroup");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
StaxResponseHandler responseHandler = new StaxResponseHandler(
new ClusterParameterGroupStaxUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Creates a new Amazon Redshift security group. You use security groups to control access to non-VPC clusters.
*
*
* For information about managing security groups, go to Amazon Redshift Cluster
* Security Groups in the Amazon Redshift Cluster Management Guide.
*
*
* @param createClusterSecurityGroupRequest
* @return Result of the CreateClusterSecurityGroup operation returned by the service.
* @throws ClusterSecurityGroupAlreadyExistsException
* A cluster security group with the same name already exists.
* @throws ClusterSecurityGroupQuotaExceededException
* The request would result in the user exceeding the allowed number of cluster security groups. For
* information about increasing your quota, go to Limits in Amazon
* Redshift in the Amazon Redshift Cluster Management Guide.
* @throws TagLimitExceededException
* You have exceeded the number of tags allowed.
* @throws InvalidTagException
* The tag is invalid.
* @sample AmazonRedshift.CreateClusterSecurityGroup
* @see AWS API Documentation
*/
@Override
public ClusterSecurityGroup createClusterSecurityGroup(CreateClusterSecurityGroupRequest request) {
request = beforeClientExecution(request);
return executeCreateClusterSecurityGroup(request);
}
@SdkInternalApi
final ClusterSecurityGroup executeCreateClusterSecurityGroup(CreateClusterSecurityGroupRequest createClusterSecurityGroupRequest) {
ExecutionContext executionContext = createExecutionContext(createClusterSecurityGroupRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new CreateClusterSecurityGroupRequestMarshaller().marshall(super.beforeMarshalling(createClusterSecurityGroupRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Redshift");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "CreateClusterSecurityGroup");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
StaxResponseHandler responseHandler = new StaxResponseHandler(
new ClusterSecurityGroupStaxUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Creates a manual snapshot of the specified cluster. The cluster must be in the available
state.
*
*
* For more information about working with snapshots, go to Amazon Redshift Snapshots
* in the Amazon Redshift Cluster Management Guide.
*
*
* @param createClusterSnapshotRequest
* @return Result of the CreateClusterSnapshot operation returned by the service.
* @throws ClusterSnapshotAlreadyExistsException
* The value specified as a snapshot identifier is already used by an existing snapshot.
* @throws InvalidClusterStateException
* The specified cluster is not in the available
state.
* @throws ClusterNotFoundException
* The ClusterIdentifier
parameter does not refer to an existing cluster.
* @throws ClusterSnapshotQuotaExceededException
* The request would result in the user exceeding the allowed number of cluster snapshots.
* @throws TagLimitExceededException
* You have exceeded the number of tags allowed.
* @throws InvalidTagException
* The tag is invalid.
* @throws InvalidRetentionPeriodException
* The retention period specified is either in the past or is not a valid value.
*
* The value must be either -1 or an integer between 1 and 3,653.
* @sample AmazonRedshift.CreateClusterSnapshot
* @see AWS
* API Documentation
*/
@Override
public Snapshot createClusterSnapshot(CreateClusterSnapshotRequest request) {
request = beforeClientExecution(request);
return executeCreateClusterSnapshot(request);
}
@SdkInternalApi
final Snapshot executeCreateClusterSnapshot(CreateClusterSnapshotRequest createClusterSnapshotRequest) {
ExecutionContext executionContext = createExecutionContext(createClusterSnapshotRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new CreateClusterSnapshotRequestMarshaller().marshall(super.beforeMarshalling(createClusterSnapshotRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Redshift");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "CreateClusterSnapshot");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
StaxResponseHandler responseHandler = new StaxResponseHandler(new SnapshotStaxUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Creates a new Amazon Redshift subnet group. You must provide a list of one or more subnets in your existing
* Amazon Virtual Private Cloud (Amazon VPC) when creating Amazon Redshift subnet group.
*
*
* For information about subnet groups, go to Amazon Redshift
* Cluster Subnet Groups in the Amazon Redshift Cluster Management Guide.
*
*
* @param createClusterSubnetGroupRequest
* @return Result of the CreateClusterSubnetGroup operation returned by the service.
* @throws ClusterSubnetGroupAlreadyExistsException
* A ClusterSubnetGroupName is already used by an existing cluster subnet group.
* @throws ClusterSubnetGroupQuotaExceededException
* The request would result in user exceeding the allowed number of cluster subnet groups. For information
* about increasing your quota, go to Limits in Amazon
* Redshift in the Amazon Redshift Cluster Management Guide.
* @throws ClusterSubnetQuotaExceededException
* The request would result in user exceeding the allowed number of subnets in a cluster subnet groups. For
* information about increasing your quota, go to Limits in Amazon
* Redshift in the Amazon Redshift Cluster Management Guide.
* @throws InvalidSubnetException
* The requested subnet is not valid, or not all of the subnets are in the same VPC.
* @throws UnauthorizedOperationException
* Your account is not authorized to perform the requested operation.
* @throws TagLimitExceededException
* You have exceeded the number of tags allowed.
* @throws InvalidTagException
* The tag is invalid.
* @throws DependentServiceRequestThrottlingException
* The request cannot be completed because a dependent service is throttling requests made by Amazon
* Redshift on your behalf. Wait and retry the request.
* @sample AmazonRedshift.CreateClusterSubnetGroup
* @see AWS API Documentation
*/
@Override
public ClusterSubnetGroup createClusterSubnetGroup(CreateClusterSubnetGroupRequest request) {
request = beforeClientExecution(request);
return executeCreateClusterSubnetGroup(request);
}
@SdkInternalApi
final ClusterSubnetGroup executeCreateClusterSubnetGroup(CreateClusterSubnetGroupRequest createClusterSubnetGroupRequest) {
ExecutionContext executionContext = createExecutionContext(createClusterSubnetGroupRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new CreateClusterSubnetGroupRequestMarshaller().marshall(super.beforeMarshalling(createClusterSubnetGroupRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Redshift");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "CreateClusterSubnetGroup");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
StaxResponseHandler responseHandler = new StaxResponseHandler(new ClusterSubnetGroupStaxUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Used to create a custom domain name for a cluster. Properties include the custom domain name, the cluster the
* custom domain is associated with, and the certificate Amazon Resource Name (ARN).
*
*
* @param createCustomDomainAssociationRequest
* @return Result of the CreateCustomDomainAssociation operation returned by the service.
* @throws UnsupportedOperationException
* The requested operation isn't supported.
* @throws ClusterNotFoundException
* The ClusterIdentifier
parameter does not refer to an existing cluster.
* @throws CustomCnameAssociationException
* An error occurred when an attempt was made to change the custom domain association.
* @sample AmazonRedshift.CreateCustomDomainAssociation
* @see AWS API Documentation
*/
@Override
public CreateCustomDomainAssociationResult createCustomDomainAssociation(CreateCustomDomainAssociationRequest request) {
request = beforeClientExecution(request);
return executeCreateCustomDomainAssociation(request);
}
@SdkInternalApi
final CreateCustomDomainAssociationResult executeCreateCustomDomainAssociation(CreateCustomDomainAssociationRequest createCustomDomainAssociationRequest) {
ExecutionContext executionContext = createExecutionContext(createCustomDomainAssociationRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new CreateCustomDomainAssociationRequestMarshaller().marshall(super.beforeMarshalling(createCustomDomainAssociationRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Redshift");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "CreateCustomDomainAssociation");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
StaxResponseHandler responseHandler = new StaxResponseHandler(
new CreateCustomDomainAssociationResultStaxUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Creates a Redshift-managed VPC endpoint.
*
*
* @param createEndpointAccessRequest
* @return Result of the CreateEndpointAccess operation returned by the service.
* @throws ClusterNotFoundException
* The ClusterIdentifier
parameter does not refer to an existing cluster.
* @throws AccessToClusterDeniedException
* You are not authorized to access the cluster.
* @throws EndpointsPerClusterLimitExceededException
* The number of Redshift-managed VPC endpoints per cluster has exceeded its limit.
* @throws EndpointsPerAuthorizationLimitExceededException
* The number of Redshift-managed VPC endpoints per authorization has exceeded its limit.
* @throws InvalidClusterSecurityGroupStateException
* The state of the cluster security group is not available
.
* @throws ClusterSubnetGroupNotFoundException
* The cluster subnet group name does not refer to an existing cluster subnet group.
* @throws EndpointAlreadyExistsException
* The account already has a Redshift-managed VPC endpoint with the given identifier.
* @throws UnsupportedOperationException
* The requested operation isn't supported.
* @throws InvalidClusterStateException
* The specified cluster is not in the available
state.
* @throws UnauthorizedOperationException
* Your account is not authorized to perform the requested operation.
* @sample AmazonRedshift.CreateEndpointAccess
* @see AWS
* API Documentation
*/
@Override
public CreateEndpointAccessResult createEndpointAccess(CreateEndpointAccessRequest request) {
request = beforeClientExecution(request);
return executeCreateEndpointAccess(request);
}
@SdkInternalApi
final CreateEndpointAccessResult executeCreateEndpointAccess(CreateEndpointAccessRequest createEndpointAccessRequest) {
ExecutionContext executionContext = createExecutionContext(createEndpointAccessRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new CreateEndpointAccessRequestMarshaller().marshall(super.beforeMarshalling(createEndpointAccessRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Redshift");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "CreateEndpointAccess");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
StaxResponseHandler responseHandler = new StaxResponseHandler(
new CreateEndpointAccessResultStaxUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Creates an Amazon Redshift event notification subscription. This action requires an ARN (Amazon Resource Name) of
* an Amazon SNS topic created by either the Amazon Redshift console, the Amazon SNS console, or the Amazon SNS API.
* To obtain an ARN with Amazon SNS, you must create a topic in Amazon SNS and subscribe to the topic. The ARN is
* displayed in the SNS console.
*
*
* You can specify the source type, and lists of Amazon Redshift source IDs, event categories, and event severities.
* Notifications will be sent for all events you want that match those criteria. For example, you can specify source
* type = cluster, source ID = my-cluster-1 and mycluster2, event categories = Availability, Backup, and severity =
* ERROR. The subscription will only send notifications for those ERROR events in the Availability and Backup
* categories for the specified clusters.
*
*
* If you specify both the source type and source IDs, such as source type = cluster and source identifier =
* my-cluster-1, notifications will be sent for all the cluster events for my-cluster-1. If you specify a source
* type but do not specify a source identifier, you will receive notice of the events for the objects of that type
* in your Amazon Web Services account. If you do not specify either the SourceType nor the SourceIdentifier, you
* will be notified of events generated from all Amazon Redshift sources belonging to your Amazon Web Services
* account. You must specify a source type if you specify a source ID.
*
*
* @param createEventSubscriptionRequest
* @return Result of the CreateEventSubscription operation returned by the service.
* @throws EventSubscriptionQuotaExceededException
* The request would exceed the allowed number of event subscriptions for this account. For information
* about increasing your quota, go to Limits in Amazon
* Redshift in the Amazon Redshift Cluster Management Guide.
* @throws SubscriptionAlreadyExistException
* There is already an existing event notification subscription with the specified name.
* @throws SNSInvalidTopicException
* Amazon SNS has responded that there is a problem with the specified Amazon SNS topic.
* @throws SNSNoAuthorizationException
* You do not have permission to publish to the specified Amazon SNS topic.
* @throws SNSTopicArnNotFoundException
* An Amazon SNS topic with the specified Amazon Resource Name (ARN) does not exist.
* @throws SubscriptionEventIdNotFoundException
* An Amazon Redshift event with the specified event ID does not exist.
* @throws SubscriptionCategoryNotFoundException
* The value specified for the event category was not one of the allowed values, or it specified a category
* that does not apply to the specified source type. The allowed values are Configuration, Management,
* Monitoring, and Security.
* @throws SubscriptionSeverityNotFoundException
* The value specified for the event severity was not one of the allowed values, or it specified a severity
* that does not apply to the specified source type. The allowed values are ERROR and INFO.
* @throws SourceNotFoundException
* The specified Amazon Redshift event source could not be found.
* @throws TagLimitExceededException
* You have exceeded the number of tags allowed.
* @throws InvalidTagException
* The tag is invalid.
* @sample AmazonRedshift.CreateEventSubscription
* @see AWS API Documentation
*/
@Override
public EventSubscription createEventSubscription(CreateEventSubscriptionRequest request) {
request = beforeClientExecution(request);
return executeCreateEventSubscription(request);
}
@SdkInternalApi
final EventSubscription executeCreateEventSubscription(CreateEventSubscriptionRequest createEventSubscriptionRequest) {
ExecutionContext executionContext = createExecutionContext(createEventSubscriptionRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new CreateEventSubscriptionRequestMarshaller().marshall(super.beforeMarshalling(createEventSubscriptionRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Redshift");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "CreateEventSubscription");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
StaxResponseHandler responseHandler = new StaxResponseHandler(new EventSubscriptionStaxUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Creates an HSM client certificate that an Amazon Redshift cluster will use to connect to the client's HSM in
* order to store and retrieve the keys used to encrypt the cluster databases.
*
*
* The command returns a public key, which you must store in the HSM. In addition to creating the HSM certificate,
* you must create an Amazon Redshift HSM configuration that provides a cluster the information needed to store and
* use encryption keys in the HSM. For more information, go to Hardware
* Security Modules in the Amazon Redshift Cluster Management Guide.
*
*
* @param createHsmClientCertificateRequest
* @return Result of the CreateHsmClientCertificate operation returned by the service.
* @throws HsmClientCertificateAlreadyExistsException
* There is already an existing Amazon Redshift HSM client certificate with the specified identifier.
* @throws HsmClientCertificateQuotaExceededException
* The quota for HSM client certificates has been reached. For information about increasing your quota, go
* to Limits in
* Amazon Redshift in the Amazon Redshift Cluster Management Guide.
* @throws TagLimitExceededException
* You have exceeded the number of tags allowed.
* @throws InvalidTagException
* The tag is invalid.
* @sample AmazonRedshift.CreateHsmClientCertificate
* @see AWS API Documentation
*/
@Override
public HsmClientCertificate createHsmClientCertificate(CreateHsmClientCertificateRequest request) {
request = beforeClientExecution(request);
return executeCreateHsmClientCertificate(request);
}
@SdkInternalApi
final HsmClientCertificate executeCreateHsmClientCertificate(CreateHsmClientCertificateRequest createHsmClientCertificateRequest) {
ExecutionContext executionContext = createExecutionContext(createHsmClientCertificateRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new CreateHsmClientCertificateRequestMarshaller().marshall(super.beforeMarshalling(createHsmClientCertificateRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Redshift");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "CreateHsmClientCertificate");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
StaxResponseHandler responseHandler = new StaxResponseHandler(
new HsmClientCertificateStaxUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Creates an HSM configuration that contains the information required by an Amazon Redshift cluster to store and
* use database encryption keys in a Hardware Security Module (HSM). After creating the HSM configuration, you can
* specify it as a parameter when creating a cluster. The cluster will then store its encryption keys in the HSM.
*
*
* In addition to creating an HSM configuration, you must also create an HSM client certificate. For more
* information, go to Hardware
* Security Modules in the Amazon Redshift Cluster Management Guide.
*
*
* @param createHsmConfigurationRequest
* @return Result of the CreateHsmConfiguration operation returned by the service.
* @throws HsmConfigurationAlreadyExistsException
* There is already an existing Amazon Redshift HSM configuration with the specified identifier.
* @throws HsmConfigurationQuotaExceededException
* The quota for HSM configurations has been reached. For information about increasing your quota, go to Limits in Amazon
* Redshift in the Amazon Redshift Cluster Management Guide.
* @throws TagLimitExceededException
* You have exceeded the number of tags allowed.
* @throws InvalidTagException
* The tag is invalid.
* @sample AmazonRedshift.CreateHsmConfiguration
* @see AWS API Documentation
*/
@Override
public HsmConfiguration createHsmConfiguration(CreateHsmConfigurationRequest request) {
request = beforeClientExecution(request);
return executeCreateHsmConfiguration(request);
}
@SdkInternalApi
final HsmConfiguration executeCreateHsmConfiguration(CreateHsmConfigurationRequest createHsmConfigurationRequest) {
ExecutionContext executionContext = createExecutionContext(createHsmConfigurationRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new CreateHsmConfigurationRequestMarshaller().marshall(super.beforeMarshalling(createHsmConfigurationRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Redshift");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "CreateHsmConfiguration");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
StaxResponseHandler responseHandler = new StaxResponseHandler(new HsmConfigurationStaxUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Creates an Amazon Redshift application for use with IAM Identity Center.
*
*
* @param createRedshiftIdcApplicationRequest
* @return Result of the CreateRedshiftIdcApplication operation returned by the service.
* @throws RedshiftIdcApplicationAlreadyExistsException
* The application you attempted to add already exists.
* @throws DependentServiceUnavailableException
* Your request cannot be completed because a dependent internal service is temporarily unavailable. Wait 30
* to 60 seconds and try again.
* @throws UnsupportedOperationException
* The requested operation isn't supported.
* @throws DependentServiceAccessDeniedException
* A dependent service denied access for the integration.
* @throws RedshiftIdcApplicationQuotaExceededException
* The maximum number of Redshift IAM Identity Center applications was exceeded.
* @sample AmazonRedshift.CreateRedshiftIdcApplication
* @see AWS API Documentation
*/
@Override
public RedshiftIdcApplication createRedshiftIdcApplication(CreateRedshiftIdcApplicationRequest request) {
request = beforeClientExecution(request);
return executeCreateRedshiftIdcApplication(request);
}
@SdkInternalApi
final RedshiftIdcApplication executeCreateRedshiftIdcApplication(CreateRedshiftIdcApplicationRequest createRedshiftIdcApplicationRequest) {
ExecutionContext executionContext = createExecutionContext(createRedshiftIdcApplicationRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new CreateRedshiftIdcApplicationRequestMarshaller().marshall(super.beforeMarshalling(createRedshiftIdcApplicationRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Redshift");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "CreateRedshiftIdcApplication");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
StaxResponseHandler responseHandler = new StaxResponseHandler(
new RedshiftIdcApplicationStaxUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Creates a scheduled action. A scheduled action contains a schedule and an Amazon Redshift API action. For
* example, you can create a schedule of when to run the ResizeCluster
API operation.
*
*
* @param createScheduledActionRequest
* @return Result of the CreateScheduledAction operation returned by the service.
* @throws ClusterNotFoundException
* The ClusterIdentifier
parameter does not refer to an existing cluster.
* @throws ScheduledActionAlreadyExistsException
* The scheduled action already exists.
* @throws ScheduledActionQuotaExceededException
* The quota for scheduled actions exceeded.
* @throws ScheduledActionTypeUnsupportedException
* The action type specified for a scheduled action is not supported.
* @throws InvalidScheduleException
* The schedule you submitted isn't valid.
* @throws InvalidScheduledActionException
* The scheduled action is not valid.
* @throws UnauthorizedOperationException
* Your account is not authorized to perform the requested operation.
* @throws UnsupportedOperationException
* The requested operation isn't supported.
* @sample AmazonRedshift.CreateScheduledAction
* @see AWS
* API Documentation
*/
@Override
public CreateScheduledActionResult createScheduledAction(CreateScheduledActionRequest request) {
request = beforeClientExecution(request);
return executeCreateScheduledAction(request);
}
@SdkInternalApi
final CreateScheduledActionResult executeCreateScheduledAction(CreateScheduledActionRequest createScheduledActionRequest) {
ExecutionContext executionContext = createExecutionContext(createScheduledActionRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new CreateScheduledActionRequestMarshaller().marshall(super.beforeMarshalling(createScheduledActionRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Redshift");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "CreateScheduledAction");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
StaxResponseHandler responseHandler = new StaxResponseHandler(
new CreateScheduledActionResultStaxUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Creates a snapshot copy grant that permits Amazon Redshift to use an encrypted symmetric key from Key Management
* Service (KMS) to encrypt copied snapshots in a destination region.
*
*
* For more information about managing snapshot copy grants, go to Amazon Redshift Database
* Encryption in the Amazon Redshift Cluster Management Guide.
*
*
* @param createSnapshotCopyGrantRequest
* The result of the CreateSnapshotCopyGrant
action.
* @return Result of the CreateSnapshotCopyGrant operation returned by the service.
* @throws SnapshotCopyGrantAlreadyExistsException
* The snapshot copy grant can't be created because a grant with the same name already exists.
* @throws SnapshotCopyGrantQuotaExceededException
* The Amazon Web Services account has exceeded the maximum number of snapshot copy grants in this region.
* @throws LimitExceededException
* The encryption key has exceeded its grant limit in Amazon Web Services KMS.
* @throws TagLimitExceededException
* You have exceeded the number of tags allowed.
* @throws InvalidTagException
* The tag is invalid.
* @throws DependentServiceRequestThrottlingException
* The request cannot be completed because a dependent service is throttling requests made by Amazon
* Redshift on your behalf. Wait and retry the request.
* @sample AmazonRedshift.CreateSnapshotCopyGrant
* @see AWS API Documentation
*/
@Override
public SnapshotCopyGrant createSnapshotCopyGrant(CreateSnapshotCopyGrantRequest request) {
request = beforeClientExecution(request);
return executeCreateSnapshotCopyGrant(request);
}
@SdkInternalApi
final SnapshotCopyGrant executeCreateSnapshotCopyGrant(CreateSnapshotCopyGrantRequest createSnapshotCopyGrantRequest) {
ExecutionContext executionContext = createExecutionContext(createSnapshotCopyGrantRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new CreateSnapshotCopyGrantRequestMarshaller().marshall(super.beforeMarshalling(createSnapshotCopyGrantRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Redshift");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "CreateSnapshotCopyGrant");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
StaxResponseHandler responseHandler = new StaxResponseHandler(new SnapshotCopyGrantStaxUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Create a snapshot schedule that can be associated to a cluster and which overrides the default system backup
* schedule.
*
*
* @param createSnapshotScheduleRequest
* @return Result of the CreateSnapshotSchedule operation returned by the service.
* @throws SnapshotScheduleAlreadyExistsException
* The specified snapshot schedule already exists.
* @throws InvalidScheduleException
* The schedule you submitted isn't valid.
* @throws SnapshotScheduleQuotaExceededException
* You have exceeded the quota of snapshot schedules.
* @throws TagLimitExceededException
* You have exceeded the number of tags allowed.
* @throws ScheduleDefinitionTypeUnsupportedException
* The definition you submitted is not supported.
* @throws InvalidTagException
* The tag is invalid.
* @sample AmazonRedshift.CreateSnapshotSchedule
* @see AWS API Documentation
*/
@Override
public CreateSnapshotScheduleResult createSnapshotSchedule(CreateSnapshotScheduleRequest request) {
request = beforeClientExecution(request);
return executeCreateSnapshotSchedule(request);
}
@SdkInternalApi
final CreateSnapshotScheduleResult executeCreateSnapshotSchedule(CreateSnapshotScheduleRequest createSnapshotScheduleRequest) {
ExecutionContext executionContext = createExecutionContext(createSnapshotScheduleRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new CreateSnapshotScheduleRequestMarshaller().marshall(super.beforeMarshalling(createSnapshotScheduleRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Redshift");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "CreateSnapshotSchedule");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
StaxResponseHandler responseHandler = new StaxResponseHandler(
new CreateSnapshotScheduleResultStaxUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Adds tags to a cluster.
*
*
* A resource can have up to 50 tags. If you try to create more than 50 tags for a resource, you will receive an
* error and the attempt will fail.
*
*
* If you specify a key that already exists for the resource, the value for that key will be updated with the new
* value.
*
*
* @param createTagsRequest
* Contains the output from the CreateTags
action.
* @return Result of the CreateTags operation returned by the service.
* @throws TagLimitExceededException
* You have exceeded the number of tags allowed.
* @throws ResourceNotFoundException
* The resource could not be found.
* @throws InvalidTagException
* The tag is invalid.
* @throws InvalidClusterStateException
* The specified cluster is not in the available
state.
* @sample AmazonRedshift.CreateTags
* @see AWS API
* Documentation
*/
@Override
public CreateTagsResult createTags(CreateTagsRequest request) {
request = beforeClientExecution(request);
return executeCreateTags(request);
}
@SdkInternalApi
final CreateTagsResult executeCreateTags(CreateTagsRequest createTagsRequest) {
ExecutionContext executionContext = createExecutionContext(createTagsRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new CreateTagsRequestMarshaller().marshall(super.beforeMarshalling(createTagsRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Redshift");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "CreateTags");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
StaxResponseHandler responseHandler = new StaxResponseHandler(new CreateTagsResultStaxUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Creates a usage limit for a specified Amazon Redshift feature on a cluster. The usage limit is identified by the
* returned usage limit identifier.
*
*
* @param createUsageLimitRequest
* @return Result of the CreateUsageLimit operation returned by the service.
* @throws ClusterNotFoundException
* The ClusterIdentifier
parameter does not refer to an existing cluster.
* @throws InvalidClusterStateException
* The specified cluster is not in the available
state.
* @throws LimitExceededException
* The encryption key has exceeded its grant limit in Amazon Web Services KMS.
* @throws UsageLimitAlreadyExistsException
* The usage limit already exists.
* @throws InvalidUsageLimitException
* The usage limit is not valid.
* @throws TagLimitExceededException
* You have exceeded the number of tags allowed.
* @throws UnsupportedOperationException
* The requested operation isn't supported.
* @sample AmazonRedshift.CreateUsageLimit
* @see AWS API
* Documentation
*/
@Override
public CreateUsageLimitResult createUsageLimit(CreateUsageLimitRequest request) {
request = beforeClientExecution(request);
return executeCreateUsageLimit(request);
}
@SdkInternalApi
final CreateUsageLimitResult executeCreateUsageLimit(CreateUsageLimitRequest createUsageLimitRequest) {
ExecutionContext executionContext = createExecutionContext(createUsageLimitRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new CreateUsageLimitRequestMarshaller().marshall(super.beforeMarshalling(createUsageLimitRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Redshift");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "CreateUsageLimit");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
StaxResponseHandler responseHandler = new StaxResponseHandler(
new CreateUsageLimitResultStaxUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* From a datashare producer account, removes authorization from the specified datashare.
*
*
* @param deauthorizeDataShareRequest
* @return Result of the DeauthorizeDataShare operation returned by the service.
* @throws InvalidDataShareException
* There is an error with the datashare.
* @sample AmazonRedshift.DeauthorizeDataShare
* @see AWS
* API Documentation
*/
@Override
public DeauthorizeDataShareResult deauthorizeDataShare(DeauthorizeDataShareRequest request) {
request = beforeClientExecution(request);
return executeDeauthorizeDataShare(request);
}
@SdkInternalApi
final DeauthorizeDataShareResult executeDeauthorizeDataShare(DeauthorizeDataShareRequest deauthorizeDataShareRequest) {
ExecutionContext executionContext = createExecutionContext(deauthorizeDataShareRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DeauthorizeDataShareRequestMarshaller().marshall(super.beforeMarshalling(deauthorizeDataShareRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Redshift");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DeauthorizeDataShare");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
StaxResponseHandler responseHandler = new StaxResponseHandler(
new DeauthorizeDataShareResultStaxUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Deletes an authentication profile.
*
*
* @param deleteAuthenticationProfileRequest
* @return Result of the DeleteAuthenticationProfile operation returned by the service.
* @throws AuthenticationProfileNotFoundException
* The authentication profile can't be found.
* @throws InvalidAuthenticationProfileRequestException
* The authentication profile request is not valid. The profile name can't be null or empty. The
* authentication profile API operation must be available in the Amazon Web Services Region.
* @sample AmazonRedshift.DeleteAuthenticationProfile
* @see AWS API Documentation
*/
@Override
public DeleteAuthenticationProfileResult deleteAuthenticationProfile(DeleteAuthenticationProfileRequest request) {
request = beforeClientExecution(request);
return executeDeleteAuthenticationProfile(request);
}
@SdkInternalApi
final DeleteAuthenticationProfileResult executeDeleteAuthenticationProfile(DeleteAuthenticationProfileRequest deleteAuthenticationProfileRequest) {
ExecutionContext executionContext = createExecutionContext(deleteAuthenticationProfileRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DeleteAuthenticationProfileRequestMarshaller().marshall(super.beforeMarshalling(deleteAuthenticationProfileRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Redshift");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DeleteAuthenticationProfile");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
StaxResponseHandler responseHandler = new StaxResponseHandler(
new DeleteAuthenticationProfileResultStaxUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Deletes a previously provisioned cluster without its final snapshot being created. A successful response from the
* web service indicates that the request was received correctly. Use DescribeClusters to monitor the status
* of the deletion. The delete operation cannot be canceled or reverted once submitted. For more information about
* managing clusters, go to Amazon Redshift Clusters
* in the Amazon Redshift Cluster Management Guide.
*
*
* If you want to shut down the cluster and retain it for future use, set SkipFinalClusterSnapshot to
* false
and specify a name for FinalClusterSnapshotIdentifier. You can later restore this
* snapshot to resume using the cluster. If a final cluster snapshot is requested, the status of the cluster will be
* "final-snapshot" while the snapshot is being taken, then it's "deleting" once Amazon Redshift begins deleting the
* cluster.
*
*
* For more information about managing clusters, go to Amazon Redshift Clusters
* in the Amazon Redshift Cluster Management Guide.
*
*
* @param deleteClusterRequest
* @return Result of the DeleteCluster operation returned by the service.
* @throws ClusterNotFoundException
* The ClusterIdentifier
parameter does not refer to an existing cluster.
* @throws InvalidClusterStateException
* The specified cluster is not in the available
state.
* @throws ClusterSnapshotAlreadyExistsException
* The value specified as a snapshot identifier is already used by an existing snapshot.
* @throws ClusterSnapshotQuotaExceededException
* The request would result in the user exceeding the allowed number of cluster snapshots.
* @throws InvalidRetentionPeriodException
* The retention period specified is either in the past or is not a valid value.
*
* The value must be either -1 or an integer between 1 and 3,653.
* @sample AmazonRedshift.DeleteCluster
* @see AWS API
* Documentation
*/
@Override
public Cluster deleteCluster(DeleteClusterRequest request) {
request = beforeClientExecution(request);
return executeDeleteCluster(request);
}
@SdkInternalApi
final Cluster executeDeleteCluster(DeleteClusterRequest deleteClusterRequest) {
ExecutionContext executionContext = createExecutionContext(deleteClusterRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DeleteClusterRequestMarshaller().marshall(super.beforeMarshalling(deleteClusterRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Redshift");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DeleteCluster");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
StaxResponseHandler responseHandler = new StaxResponseHandler(new ClusterStaxUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Deletes a specified Amazon Redshift parameter group.
*
*
*
* You cannot delete a parameter group if it is associated with a cluster.
*
*
*
* @param deleteClusterParameterGroupRequest
* @return Result of the DeleteClusterParameterGroup operation returned by the service.
* @throws InvalidClusterParameterGroupStateException
* The cluster parameter group action can not be completed because another task is in progress that involves
* the parameter group. Wait a few moments and try the operation again.
* @throws ClusterParameterGroupNotFoundException
* The parameter group name does not refer to an existing parameter group.
* @sample AmazonRedshift.DeleteClusterParameterGroup
* @see AWS API Documentation
*/
@Override
public DeleteClusterParameterGroupResult deleteClusterParameterGroup(DeleteClusterParameterGroupRequest request) {
request = beforeClientExecution(request);
return executeDeleteClusterParameterGroup(request);
}
@SdkInternalApi
final DeleteClusterParameterGroupResult executeDeleteClusterParameterGroup(DeleteClusterParameterGroupRequest deleteClusterParameterGroupRequest) {
ExecutionContext executionContext = createExecutionContext(deleteClusterParameterGroupRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DeleteClusterParameterGroupRequestMarshaller().marshall(super.beforeMarshalling(deleteClusterParameterGroupRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Redshift");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DeleteClusterParameterGroup");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
StaxResponseHandler responseHandler = new StaxResponseHandler(
new DeleteClusterParameterGroupResultStaxUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Deletes an Amazon Redshift security group.
*
*
*
* You cannot delete a security group that is associated with any clusters. You cannot delete the default security
* group.
*
*
*
* For information about managing security groups, go to Amazon Redshift Cluster
* Security Groups in the Amazon Redshift Cluster Management Guide.
*
*
* @param deleteClusterSecurityGroupRequest
* @return Result of the DeleteClusterSecurityGroup operation returned by the service.
* @throws InvalidClusterSecurityGroupStateException
* The state of the cluster security group is not available
.
* @throws ClusterSecurityGroupNotFoundException
* The cluster security group name does not refer to an existing cluster security group.
* @sample AmazonRedshift.DeleteClusterSecurityGroup
* @see AWS API Documentation
*/
@Override
public DeleteClusterSecurityGroupResult deleteClusterSecurityGroup(DeleteClusterSecurityGroupRequest request) {
request = beforeClientExecution(request);
return executeDeleteClusterSecurityGroup(request);
}
@SdkInternalApi
final DeleteClusterSecurityGroupResult executeDeleteClusterSecurityGroup(DeleteClusterSecurityGroupRequest deleteClusterSecurityGroupRequest) {
ExecutionContext executionContext = createExecutionContext(deleteClusterSecurityGroupRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DeleteClusterSecurityGroupRequestMarshaller().marshall(super.beforeMarshalling(deleteClusterSecurityGroupRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Redshift");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DeleteClusterSecurityGroup");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
StaxResponseHandler responseHandler = new StaxResponseHandler(
new DeleteClusterSecurityGroupResultStaxUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Deletes the specified manual snapshot. The snapshot must be in the available
state, with no other
* users authorized to access the snapshot.
*
*
* Unlike automated snapshots, manual snapshots are retained even after you delete your cluster. Amazon Redshift
* does not delete your manual snapshots. You must delete manual snapshot explicitly to avoid getting charged. If
* other accounts are authorized to access the snapshot, you must revoke all of the authorizations before you can
* delete the snapshot.
*
*
* @param deleteClusterSnapshotRequest
* @return Result of the DeleteClusterSnapshot operation returned by the service.
* @throws InvalidClusterSnapshotStateException
* The specified cluster snapshot is not in the available
state, or other accounts are
* authorized to access the snapshot.
* @throws ClusterSnapshotNotFoundException
* The snapshot identifier does not refer to an existing cluster snapshot.
* @sample AmazonRedshift.DeleteClusterSnapshot
* @see AWS
* API Documentation
*/
@Override
public Snapshot deleteClusterSnapshot(DeleteClusterSnapshotRequest request) {
request = beforeClientExecution(request);
return executeDeleteClusterSnapshot(request);
}
@SdkInternalApi
final Snapshot executeDeleteClusterSnapshot(DeleteClusterSnapshotRequest deleteClusterSnapshotRequest) {
ExecutionContext executionContext = createExecutionContext(deleteClusterSnapshotRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DeleteClusterSnapshotRequestMarshaller().marshall(super.beforeMarshalling(deleteClusterSnapshotRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Redshift");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DeleteClusterSnapshot");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
StaxResponseHandler responseHandler = new StaxResponseHandler(new SnapshotStaxUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Deletes the specified cluster subnet group.
*
*
* @param deleteClusterSubnetGroupRequest
* @return Result of the DeleteClusterSubnetGroup operation returned by the service.
* @throws InvalidClusterSubnetGroupStateException
* The cluster subnet group cannot be deleted because it is in use.
* @throws InvalidClusterSubnetStateException
* The state of the subnet is invalid.
* @throws ClusterSubnetGroupNotFoundException
* The cluster subnet group name does not refer to an existing cluster subnet group.
* @sample AmazonRedshift.DeleteClusterSubnetGroup
* @see AWS API Documentation
*/
@Override
public DeleteClusterSubnetGroupResult deleteClusterSubnetGroup(DeleteClusterSubnetGroupRequest request) {
request = beforeClientExecution(request);
return executeDeleteClusterSubnetGroup(request);
}
@SdkInternalApi
final DeleteClusterSubnetGroupResult executeDeleteClusterSubnetGroup(DeleteClusterSubnetGroupRequest deleteClusterSubnetGroupRequest) {
ExecutionContext executionContext = createExecutionContext(deleteClusterSubnetGroupRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DeleteClusterSubnetGroupRequestMarshaller().marshall(super.beforeMarshalling(deleteClusterSubnetGroupRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Redshift");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DeleteClusterSubnetGroup");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
StaxResponseHandler responseHandler = new StaxResponseHandler(
new DeleteClusterSubnetGroupResultStaxUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Contains information about deleting a custom domain association for a cluster.
*
*
* @param deleteCustomDomainAssociationRequest
* @return Result of the DeleteCustomDomainAssociation operation returned by the service.
* @throws UnsupportedOperationException
* The requested operation isn't supported.
* @throws ClusterNotFoundException
* The ClusterIdentifier
parameter does not refer to an existing cluster.
* @throws CustomCnameAssociationException
* An error occurred when an attempt was made to change the custom domain association.
* @throws CustomDomainAssociationNotFoundException
* An error occurred. The custom domain name couldn't be found.
* @sample AmazonRedshift.DeleteCustomDomainAssociation
* @see AWS API Documentation
*/
@Override
public DeleteCustomDomainAssociationResult deleteCustomDomainAssociation(DeleteCustomDomainAssociationRequest request) {
request = beforeClientExecution(request);
return executeDeleteCustomDomainAssociation(request);
}
@SdkInternalApi
final DeleteCustomDomainAssociationResult executeDeleteCustomDomainAssociation(DeleteCustomDomainAssociationRequest deleteCustomDomainAssociationRequest) {
ExecutionContext executionContext = createExecutionContext(deleteCustomDomainAssociationRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DeleteCustomDomainAssociationRequestMarshaller().marshall(super.beforeMarshalling(deleteCustomDomainAssociationRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Redshift");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DeleteCustomDomainAssociation");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
StaxResponseHandler responseHandler = new StaxResponseHandler(
new DeleteCustomDomainAssociationResultStaxUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Deletes a Redshift-managed VPC endpoint.
*
*
* @param deleteEndpointAccessRequest
* @return Result of the DeleteEndpointAccess operation returned by the service.
* @throws ClusterNotFoundException
* The ClusterIdentifier
parameter does not refer to an existing cluster.
* @throws InvalidEndpointStateException
* The status of the endpoint is not valid.
* @throws InvalidClusterSecurityGroupStateException
* The state of the cluster security group is not available
.
* @throws EndpointNotFoundException
* The endpoint name doesn't refer to an existing endpoint.
* @throws InvalidClusterStateException
* The specified cluster is not in the available
state.
* @sample AmazonRedshift.DeleteEndpointAccess
* @see AWS
* API Documentation
*/
@Override
public DeleteEndpointAccessResult deleteEndpointAccess(DeleteEndpointAccessRequest request) {
request = beforeClientExecution(request);
return executeDeleteEndpointAccess(request);
}
@SdkInternalApi
final DeleteEndpointAccessResult executeDeleteEndpointAccess(DeleteEndpointAccessRequest deleteEndpointAccessRequest) {
ExecutionContext executionContext = createExecutionContext(deleteEndpointAccessRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DeleteEndpointAccessRequestMarshaller().marshall(super.beforeMarshalling(deleteEndpointAccessRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Redshift");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DeleteEndpointAccess");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
StaxResponseHandler responseHandler = new StaxResponseHandler(
new DeleteEndpointAccessResultStaxUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Deletes an Amazon Redshift event notification subscription.
*
*
* @param deleteEventSubscriptionRequest
* @return Result of the DeleteEventSubscription operation returned by the service.
* @throws SubscriptionNotFoundException
* An Amazon Redshift event notification subscription with the specified name does not exist.
* @throws InvalidSubscriptionStateException
* The subscription request is invalid because it is a duplicate request. This subscription request is
* already in progress.
* @sample AmazonRedshift.DeleteEventSubscription
* @see AWS API Documentation
*/
@Override
public DeleteEventSubscriptionResult deleteEventSubscription(DeleteEventSubscriptionRequest request) {
request = beforeClientExecution(request);
return executeDeleteEventSubscription(request);
}
@SdkInternalApi
final DeleteEventSubscriptionResult executeDeleteEventSubscription(DeleteEventSubscriptionRequest deleteEventSubscriptionRequest) {
ExecutionContext executionContext = createExecutionContext(deleteEventSubscriptionRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DeleteEventSubscriptionRequestMarshaller().marshall(super.beforeMarshalling(deleteEventSubscriptionRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Redshift");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DeleteEventSubscription");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
StaxResponseHandler responseHandler = new StaxResponseHandler(
new DeleteEventSubscriptionResultStaxUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Deletes the specified HSM client certificate.
*
*
* @param deleteHsmClientCertificateRequest
* @return Result of the DeleteHsmClientCertificate operation returned by the service.
* @throws InvalidHsmClientCertificateStateException
* The specified HSM client certificate is not in the available
state, or it is still in use by
* one or more Amazon Redshift clusters.
* @throws HsmClientCertificateNotFoundException
* There is no Amazon Redshift HSM client certificate with the specified identifier.
* @sample AmazonRedshift.DeleteHsmClientCertificate
* @see AWS API Documentation
*/
@Override
public DeleteHsmClientCertificateResult deleteHsmClientCertificate(DeleteHsmClientCertificateRequest request) {
request = beforeClientExecution(request);
return executeDeleteHsmClientCertificate(request);
}
@SdkInternalApi
final DeleteHsmClientCertificateResult executeDeleteHsmClientCertificate(DeleteHsmClientCertificateRequest deleteHsmClientCertificateRequest) {
ExecutionContext executionContext = createExecutionContext(deleteHsmClientCertificateRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DeleteHsmClientCertificateRequestMarshaller().marshall(super.beforeMarshalling(deleteHsmClientCertificateRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Redshift");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DeleteHsmClientCertificate");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
StaxResponseHandler responseHandler = new StaxResponseHandler(
new DeleteHsmClientCertificateResultStaxUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Deletes the specified Amazon Redshift HSM configuration.
*
*
* @param deleteHsmConfigurationRequest
* @return Result of the DeleteHsmConfiguration operation returned by the service.
* @throws InvalidHsmConfigurationStateException
* The specified HSM configuration is not in the available
state, or it is still in use by one
* or more Amazon Redshift clusters.
* @throws HsmConfigurationNotFoundException
* There is no Amazon Redshift HSM configuration with the specified identifier.
* @sample AmazonRedshift.DeleteHsmConfiguration
* @see AWS API Documentation
*/
@Override
public DeleteHsmConfigurationResult deleteHsmConfiguration(DeleteHsmConfigurationRequest request) {
request = beforeClientExecution(request);
return executeDeleteHsmConfiguration(request);
}
@SdkInternalApi
final DeleteHsmConfigurationResult executeDeleteHsmConfiguration(DeleteHsmConfigurationRequest deleteHsmConfigurationRequest) {
ExecutionContext executionContext = createExecutionContext(deleteHsmConfigurationRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DeleteHsmConfigurationRequestMarshaller().marshall(super.beforeMarshalling(deleteHsmConfigurationRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Redshift");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DeleteHsmConfiguration");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
StaxResponseHandler responseHandler = new StaxResponseHandler(
new DeleteHsmConfigurationResultStaxUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Deletes a partner integration from a cluster. Data can still flow to the cluster until the integration is deleted
* at the partner's website.
*
*
* @param deletePartnerRequest
* @return Result of the DeletePartner operation returned by the service.
* @throws PartnerNotFoundException
* The name of the partner was not found.
* @throws ClusterNotFoundException
* The ClusterIdentifier
parameter does not refer to an existing cluster.
* @throws UnauthorizedPartnerIntegrationException
* The partner integration is not authorized.
* @throws UnsupportedOperationException
* The requested operation isn't supported.
* @sample AmazonRedshift.DeletePartner
* @see AWS API
* Documentation
*/
@Override
public DeletePartnerResult deletePartner(DeletePartnerRequest request) {
request = beforeClientExecution(request);
return executeDeletePartner(request);
}
@SdkInternalApi
final DeletePartnerResult executeDeletePartner(DeletePartnerRequest deletePartnerRequest) {
ExecutionContext executionContext = createExecutionContext(deletePartnerRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DeletePartnerRequestMarshaller().marshall(super.beforeMarshalling(deletePartnerRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Redshift");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DeletePartner");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
StaxResponseHandler responseHandler = new StaxResponseHandler(new DeletePartnerResultStaxUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Deletes an Amazon Redshift IAM Identity Center application.
*
*
* @param deleteRedshiftIdcApplicationRequest
* @return Result of the DeleteRedshiftIdcApplication operation returned by the service.
* @throws RedshiftIdcApplicationNotExistsException
* The application you attempted to find doesn't exist.
* @throws DependentServiceUnavailableException
* Your request cannot be completed because a dependent internal service is temporarily unavailable. Wait 30
* to 60 seconds and try again.
* @throws UnsupportedOperationException
* The requested operation isn't supported.
* @throws DependentServiceAccessDeniedException
* A dependent service denied access for the integration.
* @sample AmazonRedshift.DeleteRedshiftIdcApplication
* @see AWS API Documentation
*/
@Override
public DeleteRedshiftIdcApplicationResult deleteRedshiftIdcApplication(DeleteRedshiftIdcApplicationRequest request) {
request = beforeClientExecution(request);
return executeDeleteRedshiftIdcApplication(request);
}
@SdkInternalApi
final DeleteRedshiftIdcApplicationResult executeDeleteRedshiftIdcApplication(DeleteRedshiftIdcApplicationRequest deleteRedshiftIdcApplicationRequest) {
ExecutionContext executionContext = createExecutionContext(deleteRedshiftIdcApplicationRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DeleteRedshiftIdcApplicationRequestMarshaller().marshall(super.beforeMarshalling(deleteRedshiftIdcApplicationRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Redshift");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DeleteRedshiftIdcApplication");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
StaxResponseHandler responseHandler = new StaxResponseHandler(
new DeleteRedshiftIdcApplicationResultStaxUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Deletes the resource policy for a specified resource.
*
*
* @param deleteResourcePolicyRequest
* @return Result of the DeleteResourcePolicy operation returned by the service.
* @throws ResourceNotFoundException
* The resource could not be found.
* @throws UnsupportedOperationException
* The requested operation isn't supported.
* @sample AmazonRedshift.DeleteResourcePolicy
* @see AWS
* API Documentation
*/
@Override
public DeleteResourcePolicyResult deleteResourcePolicy(DeleteResourcePolicyRequest request) {
request = beforeClientExecution(request);
return executeDeleteResourcePolicy(request);
}
@SdkInternalApi
final DeleteResourcePolicyResult executeDeleteResourcePolicy(DeleteResourcePolicyRequest deleteResourcePolicyRequest) {
ExecutionContext executionContext = createExecutionContext(deleteResourcePolicyRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DeleteResourcePolicyRequestMarshaller().marshall(super.beforeMarshalling(deleteResourcePolicyRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Redshift");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DeleteResourcePolicy");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
StaxResponseHandler responseHandler = new StaxResponseHandler(
new DeleteResourcePolicyResultStaxUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Deletes a scheduled action.
*
*
* @param deleteScheduledActionRequest
* @return Result of the DeleteScheduledAction operation returned by the service.
* @throws ScheduledActionNotFoundException
* The scheduled action cannot be found.
* @throws UnauthorizedOperationException
* Your account is not authorized to perform the requested operation.
* @sample AmazonRedshift.DeleteScheduledAction
* @see AWS
* API Documentation
*/
@Override
public DeleteScheduledActionResult deleteScheduledAction(DeleteScheduledActionRequest request) {
request = beforeClientExecution(request);
return executeDeleteScheduledAction(request);
}
@SdkInternalApi
final DeleteScheduledActionResult executeDeleteScheduledAction(DeleteScheduledActionRequest deleteScheduledActionRequest) {
ExecutionContext executionContext = createExecutionContext(deleteScheduledActionRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DeleteScheduledActionRequestMarshaller().marshall(super.beforeMarshalling(deleteScheduledActionRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Redshift");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DeleteScheduledAction");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
StaxResponseHandler responseHandler = new StaxResponseHandler(
new DeleteScheduledActionResultStaxUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Deletes the specified snapshot copy grant.
*
*
* @param deleteSnapshotCopyGrantRequest
* The result of the DeleteSnapshotCopyGrant
action.
* @return Result of the DeleteSnapshotCopyGrant operation returned by the service.
* @throws InvalidSnapshotCopyGrantStateException
* The snapshot copy grant can't be deleted because it is used by one or more clusters.
* @throws SnapshotCopyGrantNotFoundException
* The specified snapshot copy grant can't be found. Make sure that the name is typed correctly and that the
* grant exists in the destination region.
* @sample AmazonRedshift.DeleteSnapshotCopyGrant
* @see AWS API Documentation
*/
@Override
public DeleteSnapshotCopyGrantResult deleteSnapshotCopyGrant(DeleteSnapshotCopyGrantRequest request) {
request = beforeClientExecution(request);
return executeDeleteSnapshotCopyGrant(request);
}
@SdkInternalApi
final DeleteSnapshotCopyGrantResult executeDeleteSnapshotCopyGrant(DeleteSnapshotCopyGrantRequest deleteSnapshotCopyGrantRequest) {
ExecutionContext executionContext = createExecutionContext(deleteSnapshotCopyGrantRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DeleteSnapshotCopyGrantRequestMarshaller().marshall(super.beforeMarshalling(deleteSnapshotCopyGrantRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Redshift");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DeleteSnapshotCopyGrant");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
StaxResponseHandler responseHandler = new StaxResponseHandler(
new DeleteSnapshotCopyGrantResultStaxUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Deletes a snapshot schedule.
*
*
* @param deleteSnapshotScheduleRequest
* @return Result of the DeleteSnapshotSchedule operation returned by the service.
* @throws InvalidClusterSnapshotScheduleStateException
* The cluster snapshot schedule state is not valid.
* @throws SnapshotScheduleNotFoundException
* We could not find the specified snapshot schedule.
* @sample AmazonRedshift.DeleteSnapshotSchedule
* @see AWS API Documentation
*/
@Override
public DeleteSnapshotScheduleResult deleteSnapshotSchedule(DeleteSnapshotScheduleRequest request) {
request = beforeClientExecution(request);
return executeDeleteSnapshotSchedule(request);
}
@SdkInternalApi
final DeleteSnapshotScheduleResult executeDeleteSnapshotSchedule(DeleteSnapshotScheduleRequest deleteSnapshotScheduleRequest) {
ExecutionContext executionContext = createExecutionContext(deleteSnapshotScheduleRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DeleteSnapshotScheduleRequestMarshaller().marshall(super.beforeMarshalling(deleteSnapshotScheduleRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Redshift");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DeleteSnapshotSchedule");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
StaxResponseHandler responseHandler = new StaxResponseHandler(
new DeleteSnapshotScheduleResultStaxUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Deletes tags from a resource. You must provide the ARN of the resource from which you want to delete the tag or
* tags.
*
*
* @param deleteTagsRequest
* Contains the output from the DeleteTags
action.
* @return Result of the DeleteTags operation returned by the service.
* @throws ResourceNotFoundException
* The resource could not be found.
* @throws InvalidTagException
* The tag is invalid.
* @sample AmazonRedshift.DeleteTags
* @see AWS API
* Documentation
*/
@Override
public DeleteTagsResult deleteTags(DeleteTagsRequest request) {
request = beforeClientExecution(request);
return executeDeleteTags(request);
}
@SdkInternalApi
final DeleteTagsResult executeDeleteTags(DeleteTagsRequest deleteTagsRequest) {
ExecutionContext executionContext = createExecutionContext(deleteTagsRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DeleteTagsRequestMarshaller().marshall(super.beforeMarshalling(deleteTagsRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Redshift");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DeleteTags");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
StaxResponseHandler responseHandler = new StaxResponseHandler(new DeleteTagsResultStaxUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Deletes a usage limit from a cluster.
*
*
* @param deleteUsageLimitRequest
* @return Result of the DeleteUsageLimit operation returned by the service.
* @throws UsageLimitNotFoundException
* The usage limit identifier can't be found.
* @throws UnsupportedOperationException
* The requested operation isn't supported.
* @sample AmazonRedshift.DeleteUsageLimit
* @see AWS API
* Documentation
*/
@Override
public DeleteUsageLimitResult deleteUsageLimit(DeleteUsageLimitRequest request) {
request = beforeClientExecution(request);
return executeDeleteUsageLimit(request);
}
@SdkInternalApi
final DeleteUsageLimitResult executeDeleteUsageLimit(DeleteUsageLimitRequest deleteUsageLimitRequest) {
ExecutionContext executionContext = createExecutionContext(deleteUsageLimitRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DeleteUsageLimitRequestMarshaller().marshall(super.beforeMarshalling(deleteUsageLimitRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Redshift");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DeleteUsageLimit");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
StaxResponseHandler responseHandler = new StaxResponseHandler(
new DeleteUsageLimitResultStaxUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Returns a list of attributes attached to an account
*
*
* @param describeAccountAttributesRequest
* @return Result of the DescribeAccountAttributes operation returned by the service.
* @sample AmazonRedshift.DescribeAccountAttributes
* @see AWS API Documentation
*/
@Override
public DescribeAccountAttributesResult describeAccountAttributes(DescribeAccountAttributesRequest request) {
request = beforeClientExecution(request);
return executeDescribeAccountAttributes(request);
}
@SdkInternalApi
final DescribeAccountAttributesResult executeDescribeAccountAttributes(DescribeAccountAttributesRequest describeAccountAttributesRequest) {
ExecutionContext executionContext = createExecutionContext(describeAccountAttributesRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DescribeAccountAttributesRequestMarshaller().marshall(super.beforeMarshalling(describeAccountAttributesRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Redshift");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DescribeAccountAttributes");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
StaxResponseHandler responseHandler = new StaxResponseHandler(
new DescribeAccountAttributesResultStaxUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Describes an authentication profile.
*
*
* @param describeAuthenticationProfilesRequest
* @return Result of the DescribeAuthenticationProfiles operation returned by the service.
* @throws AuthenticationProfileNotFoundException
* The authentication profile can't be found.
* @throws InvalidAuthenticationProfileRequestException
* The authentication profile request is not valid. The profile name can't be null or empty. The
* authentication profile API operation must be available in the Amazon Web Services Region.
* @sample AmazonRedshift.DescribeAuthenticationProfiles
* @see AWS API Documentation
*/
@Override
public DescribeAuthenticationProfilesResult describeAuthenticationProfiles(DescribeAuthenticationProfilesRequest request) {
request = beforeClientExecution(request);
return executeDescribeAuthenticationProfiles(request);
}
@SdkInternalApi
final DescribeAuthenticationProfilesResult executeDescribeAuthenticationProfiles(DescribeAuthenticationProfilesRequest describeAuthenticationProfilesRequest) {
ExecutionContext executionContext = createExecutionContext(describeAuthenticationProfilesRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DescribeAuthenticationProfilesRequestMarshaller().marshall(super.beforeMarshalling(describeAuthenticationProfilesRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Redshift");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DescribeAuthenticationProfiles");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
StaxResponseHandler responseHandler = new StaxResponseHandler(
new DescribeAuthenticationProfilesResultStaxUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Returns an array of ClusterDbRevision
objects.
*
*
* @param describeClusterDbRevisionsRequest
* @return Result of the DescribeClusterDbRevisions operation returned by the service.
* @throws ClusterNotFoundException
* The ClusterIdentifier
parameter does not refer to an existing cluster.
* @throws InvalidClusterStateException
* The specified cluster is not in the available
state.
* @sample AmazonRedshift.DescribeClusterDbRevisions
* @see AWS API Documentation
*/
@Override
public DescribeClusterDbRevisionsResult describeClusterDbRevisions(DescribeClusterDbRevisionsRequest request) {
request = beforeClientExecution(request);
return executeDescribeClusterDbRevisions(request);
}
@SdkInternalApi
final DescribeClusterDbRevisionsResult executeDescribeClusterDbRevisions(DescribeClusterDbRevisionsRequest describeClusterDbRevisionsRequest) {
ExecutionContext executionContext = createExecutionContext(describeClusterDbRevisionsRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DescribeClusterDbRevisionsRequestMarshaller().marshall(super.beforeMarshalling(describeClusterDbRevisionsRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Redshift");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DescribeClusterDbRevisions");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
StaxResponseHandler responseHandler = new StaxResponseHandler(
new DescribeClusterDbRevisionsResultStaxUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Returns a list of Amazon Redshift parameter groups, including parameter groups you created and the default
* parameter group. For each parameter group, the response includes the parameter group name, description, and
* parameter group family name. You can optionally specify a name to retrieve the description of a specific
* parameter group.
*
*
* For more information about parameters and parameter groups, go to Amazon Redshift
* Parameter Groups in the Amazon Redshift Cluster Management Guide.
*
*
* If you specify both tag keys and tag values in the same request, Amazon Redshift returns all parameter groups
* that match any combination of the specified keys and values. For example, if you have owner
and
* environment
for tag keys, and admin
and test
for tag values, all parameter
* groups that have any combination of those values are returned.
*
*
* If both tag keys and values are omitted from the request, parameter groups are returned regardless of whether
* they have tag keys or values associated with them.
*
*
* @param describeClusterParameterGroupsRequest
* @return Result of the DescribeClusterParameterGroups operation returned by the service.
* @throws ClusterParameterGroupNotFoundException
* The parameter group name does not refer to an existing parameter group.
* @throws InvalidTagException
* The tag is invalid.
* @sample AmazonRedshift.DescribeClusterParameterGroups
* @see AWS API Documentation
*/
@Override
public DescribeClusterParameterGroupsResult describeClusterParameterGroups(DescribeClusterParameterGroupsRequest request) {
request = beforeClientExecution(request);
return executeDescribeClusterParameterGroups(request);
}
@SdkInternalApi
final DescribeClusterParameterGroupsResult executeDescribeClusterParameterGroups(DescribeClusterParameterGroupsRequest describeClusterParameterGroupsRequest) {
ExecutionContext executionContext = createExecutionContext(describeClusterParameterGroupsRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DescribeClusterParameterGroupsRequestMarshaller().marshall(super.beforeMarshalling(describeClusterParameterGroupsRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Redshift");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DescribeClusterParameterGroups");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
StaxResponseHandler responseHandler = new StaxResponseHandler(
new DescribeClusterParameterGroupsResultStaxUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
@Override
public DescribeClusterParameterGroupsResult describeClusterParameterGroups() {
return describeClusterParameterGroups(new DescribeClusterParameterGroupsRequest());
}
/**
*
* Returns a detailed list of parameters contained within the specified Amazon Redshift parameter group. For each
* parameter the response includes information such as parameter name, description, data type, value, whether the
* parameter value is modifiable, and so on.
*
*
* You can specify source filter to retrieve parameters of only specific type. For example, to retrieve
* parameters that were modified by a user action such as from ModifyClusterParameterGroup, you can specify
* source equal to user.
*
*
* For more information about parameters and parameter groups, go to Amazon Redshift
* Parameter Groups in the Amazon Redshift Cluster Management Guide.
*
*
* @param describeClusterParametersRequest
* @return Result of the DescribeClusterParameters operation returned by the service.
* @throws ClusterParameterGroupNotFoundException
* The parameter group name does not refer to an existing parameter group.
* @sample AmazonRedshift.DescribeClusterParameters
* @see AWS API Documentation
*/
@Override
public DescribeClusterParametersResult describeClusterParameters(DescribeClusterParametersRequest request) {
request = beforeClientExecution(request);
return executeDescribeClusterParameters(request);
}
@SdkInternalApi
final DescribeClusterParametersResult executeDescribeClusterParameters(DescribeClusterParametersRequest describeClusterParametersRequest) {
ExecutionContext executionContext = createExecutionContext(describeClusterParametersRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DescribeClusterParametersRequestMarshaller().marshall(super.beforeMarshalling(describeClusterParametersRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Redshift");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DescribeClusterParameters");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
StaxResponseHandler responseHandler = new StaxResponseHandler(
new DescribeClusterParametersResultStaxUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Returns information about Amazon Redshift security groups. If the name of a security group is specified, the
* response will contain only information about only that security group.
*
*
* For information about managing security groups, go to Amazon Redshift Cluster
* Security Groups in the Amazon Redshift Cluster Management Guide.
*
*
* If you specify both tag keys and tag values in the same request, Amazon Redshift returns all security groups that
* match any combination of the specified keys and values. For example, if you have owner
and
* environment
for tag keys, and admin
and test
for tag values, all security
* groups that have any combination of those values are returned.
*
*
* If both tag keys and values are omitted from the request, security groups are returned regardless of whether they
* have tag keys or values associated with them.
*
*
* @param describeClusterSecurityGroupsRequest
* @return Result of the DescribeClusterSecurityGroups operation returned by the service.
* @throws ClusterSecurityGroupNotFoundException
* The cluster security group name does not refer to an existing cluster security group.
* @throws InvalidTagException
* The tag is invalid.
* @sample AmazonRedshift.DescribeClusterSecurityGroups
* @see AWS API Documentation
*/
@Override
public DescribeClusterSecurityGroupsResult describeClusterSecurityGroups(DescribeClusterSecurityGroupsRequest request) {
request = beforeClientExecution(request);
return executeDescribeClusterSecurityGroups(request);
}
@SdkInternalApi
final DescribeClusterSecurityGroupsResult executeDescribeClusterSecurityGroups(DescribeClusterSecurityGroupsRequest describeClusterSecurityGroupsRequest) {
ExecutionContext executionContext = createExecutionContext(describeClusterSecurityGroupsRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DescribeClusterSecurityGroupsRequestMarshaller().marshall(super.beforeMarshalling(describeClusterSecurityGroupsRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Redshift");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DescribeClusterSecurityGroups");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
StaxResponseHandler responseHandler = new StaxResponseHandler(
new DescribeClusterSecurityGroupsResultStaxUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
@Override
public DescribeClusterSecurityGroupsResult describeClusterSecurityGroups() {
return describeClusterSecurityGroups(new DescribeClusterSecurityGroupsRequest());
}
/**
*
* Returns one or more snapshot objects, which contain metadata about your cluster snapshots. By default, this
* operation returns information about all snapshots of all clusters that are owned by your Amazon Web Services
* account. No information is returned for snapshots owned by inactive Amazon Web Services accounts.
*
*
* If you specify both tag keys and tag values in the same request, Amazon Redshift returns all snapshots that match
* any combination of the specified keys and values. For example, if you have owner
and
* environment
for tag keys, and admin
and test
for tag values, all snapshots
* that have any combination of those values are returned. Only snapshots that you own are returned in the response;
* shared snapshots are not returned with the tag key and tag value request parameters.
*
*
* If both tag keys and values are omitted from the request, snapshots are returned regardless of whether they have
* tag keys or values associated with them.
*
*
* @param describeClusterSnapshotsRequest
* @return Result of the DescribeClusterSnapshots operation returned by the service.
* @throws ClusterNotFoundException
* The ClusterIdentifier
parameter does not refer to an existing cluster.
* @throws ClusterSnapshotNotFoundException
* The snapshot identifier does not refer to an existing cluster snapshot.
* @throws InvalidTagException
* The tag is invalid.
* @throws UnsupportedOperationException
* The requested operation isn't supported.
* @sample AmazonRedshift.DescribeClusterSnapshots
* @see AWS API Documentation
*/
@Override
public DescribeClusterSnapshotsResult describeClusterSnapshots(DescribeClusterSnapshotsRequest request) {
request = beforeClientExecution(request);
return executeDescribeClusterSnapshots(request);
}
@SdkInternalApi
final DescribeClusterSnapshotsResult executeDescribeClusterSnapshots(DescribeClusterSnapshotsRequest describeClusterSnapshotsRequest) {
ExecutionContext executionContext = createExecutionContext(describeClusterSnapshotsRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DescribeClusterSnapshotsRequestMarshaller().marshall(super.beforeMarshalling(describeClusterSnapshotsRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Redshift");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DescribeClusterSnapshots");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
StaxResponseHandler responseHandler = new StaxResponseHandler(
new DescribeClusterSnapshotsResultStaxUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
@Override
public DescribeClusterSnapshotsResult describeClusterSnapshots() {
return describeClusterSnapshots(new DescribeClusterSnapshotsRequest());
}
/**
*
* Returns one or more cluster subnet group objects, which contain metadata about your cluster subnet groups. By
* default, this operation returns information about all cluster subnet groups that are defined in your Amazon Web
* Services account.
*
*
* If you specify both tag keys and tag values in the same request, Amazon Redshift returns all subnet groups that
* match any combination of the specified keys and values. For example, if you have owner
and
* environment
for tag keys, and admin
and test
for tag values, all subnet
* groups that have any combination of those values are returned.
*
*
* If both tag keys and values are omitted from the request, subnet groups are returned regardless of whether they
* have tag keys or values associated with them.
*
*
* @param describeClusterSubnetGroupsRequest
* @return Result of the DescribeClusterSubnetGroups operation returned by the service.
* @throws ClusterSubnetGroupNotFoundException
* The cluster subnet group name does not refer to an existing cluster subnet group.
* @throws InvalidTagException
* The tag is invalid.
* @sample AmazonRedshift.DescribeClusterSubnetGroups
* @see AWS API Documentation
*/
@Override
public DescribeClusterSubnetGroupsResult describeClusterSubnetGroups(DescribeClusterSubnetGroupsRequest request) {
request = beforeClientExecution(request);
return executeDescribeClusterSubnetGroups(request);
}
@SdkInternalApi
final DescribeClusterSubnetGroupsResult executeDescribeClusterSubnetGroups(DescribeClusterSubnetGroupsRequest describeClusterSubnetGroupsRequest) {
ExecutionContext executionContext = createExecutionContext(describeClusterSubnetGroupsRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DescribeClusterSubnetGroupsRequestMarshaller().marshall(super.beforeMarshalling(describeClusterSubnetGroupsRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Redshift");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DescribeClusterSubnetGroups");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
StaxResponseHandler responseHandler = new StaxResponseHandler(
new DescribeClusterSubnetGroupsResultStaxUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
@Override
public DescribeClusterSubnetGroupsResult describeClusterSubnetGroups() {
return describeClusterSubnetGroups(new DescribeClusterSubnetGroupsRequest());
}
/**
*
* Returns a list of all the available maintenance tracks.
*
*
* @param describeClusterTracksRequest
* @return Result of the DescribeClusterTracks operation returned by the service.
* @throws InvalidClusterTrackException
* The provided cluster track name is not valid.
* @throws UnauthorizedOperationException
* Your account is not authorized to perform the requested operation.
* @sample AmazonRedshift.DescribeClusterTracks
* @see AWS
* API Documentation
*/
@Override
public DescribeClusterTracksResult describeClusterTracks(DescribeClusterTracksRequest request) {
request = beforeClientExecution(request);
return executeDescribeClusterTracks(request);
}
@SdkInternalApi
final DescribeClusterTracksResult executeDescribeClusterTracks(DescribeClusterTracksRequest describeClusterTracksRequest) {
ExecutionContext executionContext = createExecutionContext(describeClusterTracksRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DescribeClusterTracksRequestMarshaller().marshall(super.beforeMarshalling(describeClusterTracksRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Redshift");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DescribeClusterTracks");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
StaxResponseHandler responseHandler = new StaxResponseHandler(
new DescribeClusterTracksResultStaxUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Returns descriptions of the available Amazon Redshift cluster versions. You can call this operation even before
* creating any clusters to learn more about the Amazon Redshift versions. For more information about managing
* clusters, go to Amazon
* Redshift Clusters in the Amazon Redshift Cluster Management Guide.
*
*
* @param describeClusterVersionsRequest
* @return Result of the DescribeClusterVersions operation returned by the service.
* @sample AmazonRedshift.DescribeClusterVersions
* @see AWS API Documentation
*/
@Override
public DescribeClusterVersionsResult describeClusterVersions(DescribeClusterVersionsRequest request) {
request = beforeClientExecution(request);
return executeDescribeClusterVersions(request);
}
@SdkInternalApi
final DescribeClusterVersionsResult executeDescribeClusterVersions(DescribeClusterVersionsRequest describeClusterVersionsRequest) {
ExecutionContext executionContext = createExecutionContext(describeClusterVersionsRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DescribeClusterVersionsRequestMarshaller().marshall(super.beforeMarshalling(describeClusterVersionsRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Redshift");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DescribeClusterVersions");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
StaxResponseHandler responseHandler = new StaxResponseHandler(
new DescribeClusterVersionsResultStaxUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
@Override
public DescribeClusterVersionsResult describeClusterVersions() {
return describeClusterVersions(new DescribeClusterVersionsRequest());
}
/**
*
* Returns properties of provisioned clusters including general cluster properties, cluster database properties,
* maintenance and backup properties, and security and access properties. This operation supports pagination. For
* more information about managing clusters, go to Amazon Redshift Clusters
* in the Amazon Redshift Cluster Management Guide.
*
*
* If you specify both tag keys and tag values in the same request, Amazon Redshift returns all clusters that match
* any combination of the specified keys and values. For example, if you have owner
and
* environment
for tag keys, and admin
and test
for tag values, all clusters
* that have any combination of those values are returned.
*
*
* If both tag keys and values are omitted from the request, clusters are returned regardless of whether they have
* tag keys or values associated with them.
*
*
* @param describeClustersRequest
* @return Result of the DescribeClusters operation returned by the service.
* @throws ClusterNotFoundException
* The ClusterIdentifier
parameter does not refer to an existing cluster.
* @throws InvalidTagException
* The tag is invalid.
* @sample AmazonRedshift.DescribeClusters
* @see AWS API
* Documentation
*/
@Override
public DescribeClustersResult describeClusters(DescribeClustersRequest request) {
request = beforeClientExecution(request);
return executeDescribeClusters(request);
}
@SdkInternalApi
final DescribeClustersResult executeDescribeClusters(DescribeClustersRequest describeClustersRequest) {
ExecutionContext executionContext = createExecutionContext(describeClustersRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DescribeClustersRequestMarshaller().marshall(super.beforeMarshalling(describeClustersRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Redshift");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DescribeClusters");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
StaxResponseHandler responseHandler = new StaxResponseHandler(
new DescribeClustersResultStaxUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
@Override
public DescribeClustersResult describeClusters() {
return describeClusters(new DescribeClustersRequest());
}
/**
*
* Contains information about custom domain associations for a cluster.
*
*
* @param describeCustomDomainAssociationsRequest
* @return Result of the DescribeCustomDomainAssociations operation returned by the service.
* @throws CustomDomainAssociationNotFoundException
* An error occurred. The custom domain name couldn't be found.
* @throws UnsupportedOperationException
* The requested operation isn't supported.
* @sample AmazonRedshift.DescribeCustomDomainAssociations
* @see AWS API Documentation
*/
@Override
public DescribeCustomDomainAssociationsResult describeCustomDomainAssociations(DescribeCustomDomainAssociationsRequest request) {
request = beforeClientExecution(request);
return executeDescribeCustomDomainAssociations(request);
}
@SdkInternalApi
final DescribeCustomDomainAssociationsResult executeDescribeCustomDomainAssociations(
DescribeCustomDomainAssociationsRequest describeCustomDomainAssociationsRequest) {
ExecutionContext executionContext = createExecutionContext(describeCustomDomainAssociationsRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DescribeCustomDomainAssociationsRequestMarshaller().marshall(super.beforeMarshalling(describeCustomDomainAssociationsRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Redshift");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DescribeCustomDomainAssociations");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
StaxResponseHandler responseHandler = new StaxResponseHandler(
new DescribeCustomDomainAssociationsResultStaxUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Shows the status of any inbound or outbound datashares available in the specified account.
*
*
* @param describeDataSharesRequest
* @return Result of the DescribeDataShares operation returned by the service.
* @throws InvalidDataShareException
* There is an error with the datashare.
* @sample AmazonRedshift.DescribeDataShares
* @see AWS
* API Documentation
*/
@Override
public DescribeDataSharesResult describeDataShares(DescribeDataSharesRequest request) {
request = beforeClientExecution(request);
return executeDescribeDataShares(request);
}
@SdkInternalApi
final DescribeDataSharesResult executeDescribeDataShares(DescribeDataSharesRequest describeDataSharesRequest) {
ExecutionContext executionContext = createExecutionContext(describeDataSharesRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DescribeDataSharesRequestMarshaller().marshall(super.beforeMarshalling(describeDataSharesRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Redshift");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DescribeDataShares");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
StaxResponseHandler responseHandler = new StaxResponseHandler(
new DescribeDataSharesResultStaxUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Returns a list of datashares where the account identifier being called is a consumer account identifier.
*
*
* @param describeDataSharesForConsumerRequest
* @return Result of the DescribeDataSharesForConsumer operation returned by the service.
* @throws InvalidNamespaceException
* The namespace isn't valid because the namespace doesn't exist. Provide a valid namespace.
* @sample AmazonRedshift.DescribeDataSharesForConsumer
* @see AWS API Documentation
*/
@Override
public DescribeDataSharesForConsumerResult describeDataSharesForConsumer(DescribeDataSharesForConsumerRequest request) {
request = beforeClientExecution(request);
return executeDescribeDataSharesForConsumer(request);
}
@SdkInternalApi
final DescribeDataSharesForConsumerResult executeDescribeDataSharesForConsumer(DescribeDataSharesForConsumerRequest describeDataSharesForConsumerRequest) {
ExecutionContext executionContext = createExecutionContext(describeDataSharesForConsumerRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DescribeDataSharesForConsumerRequestMarshaller().marshall(super.beforeMarshalling(describeDataSharesForConsumerRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Redshift");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DescribeDataSharesForConsumer");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
StaxResponseHandler responseHandler = new StaxResponseHandler(
new DescribeDataSharesForConsumerResultStaxUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Returns a list of datashares when the account identifier being called is a producer account identifier.
*
*
* @param describeDataSharesForProducerRequest
* @return Result of the DescribeDataSharesForProducer operation returned by the service.
* @throws InvalidNamespaceException
* The namespace isn't valid because the namespace doesn't exist. Provide a valid namespace.
* @sample AmazonRedshift.DescribeDataSharesForProducer
* @see AWS API Documentation
*/
@Override
public DescribeDataSharesForProducerResult describeDataSharesForProducer(DescribeDataSharesForProducerRequest request) {
request = beforeClientExecution(request);
return executeDescribeDataSharesForProducer(request);
}
@SdkInternalApi
final DescribeDataSharesForProducerResult executeDescribeDataSharesForProducer(DescribeDataSharesForProducerRequest describeDataSharesForProducerRequest) {
ExecutionContext executionContext = createExecutionContext(describeDataSharesForProducerRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DescribeDataSharesForProducerRequestMarshaller().marshall(super.beforeMarshalling(describeDataSharesForProducerRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Redshift");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DescribeDataSharesForProducer");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
StaxResponseHandler responseHandler = new StaxResponseHandler(
new DescribeDataSharesForProducerResultStaxUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Returns a list of parameter settings for the specified parameter group family.
*
*
* For more information about parameters and parameter groups, go to Amazon Redshift
* Parameter Groups in the Amazon Redshift Cluster Management Guide.
*
*
* @param describeDefaultClusterParametersRequest
* @return Result of the DescribeDefaultClusterParameters operation returned by the service.
* @sample AmazonRedshift.DescribeDefaultClusterParameters
* @see AWS API Documentation
*/
@Override
public DefaultClusterParameters describeDefaultClusterParameters(DescribeDefaultClusterParametersRequest request) {
request = beforeClientExecution(request);
return executeDescribeDefaultClusterParameters(request);
}
@SdkInternalApi
final DefaultClusterParameters executeDescribeDefaultClusterParameters(DescribeDefaultClusterParametersRequest describeDefaultClusterParametersRequest) {
ExecutionContext executionContext = createExecutionContext(describeDefaultClusterParametersRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DescribeDefaultClusterParametersRequestMarshaller().marshall(super.beforeMarshalling(describeDefaultClusterParametersRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Redshift");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DescribeDefaultClusterParameters");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
StaxResponseHandler responseHandler = new StaxResponseHandler(
new DefaultClusterParametersStaxUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Describes a Redshift-managed VPC endpoint.
*
*
* @param describeEndpointAccessRequest
* @return Result of the DescribeEndpointAccess operation returned by the service.
* @throws ClusterNotFoundException
* The ClusterIdentifier
parameter does not refer to an existing cluster.
* @throws InvalidClusterStateException
* The specified cluster is not in the available
state.
* @throws EndpointNotFoundException
* The endpoint name doesn't refer to an existing endpoint.
* @sample AmazonRedshift.DescribeEndpointAccess
* @see AWS API Documentation
*/
@Override
public DescribeEndpointAccessResult describeEndpointAccess(DescribeEndpointAccessRequest request) {
request = beforeClientExecution(request);
return executeDescribeEndpointAccess(request);
}
@SdkInternalApi
final DescribeEndpointAccessResult executeDescribeEndpointAccess(DescribeEndpointAccessRequest describeEndpointAccessRequest) {
ExecutionContext executionContext = createExecutionContext(describeEndpointAccessRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DescribeEndpointAccessRequestMarshaller().marshall(super.beforeMarshalling(describeEndpointAccessRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Redshift");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DescribeEndpointAccess");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
StaxResponseHandler responseHandler = new StaxResponseHandler(
new DescribeEndpointAccessResultStaxUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Describes an endpoint authorization.
*
*
* @param describeEndpointAuthorizationRequest
* @return Result of the DescribeEndpointAuthorization operation returned by the service.
* @throws ClusterNotFoundException
* The ClusterIdentifier
parameter does not refer to an existing cluster.
* @throws UnsupportedOperationException
* The requested operation isn't supported.
* @sample AmazonRedshift.DescribeEndpointAuthorization
* @see AWS API Documentation
*/
@Override
public DescribeEndpointAuthorizationResult describeEndpointAuthorization(DescribeEndpointAuthorizationRequest request) {
request = beforeClientExecution(request);
return executeDescribeEndpointAuthorization(request);
}
@SdkInternalApi
final DescribeEndpointAuthorizationResult executeDescribeEndpointAuthorization(DescribeEndpointAuthorizationRequest describeEndpointAuthorizationRequest) {
ExecutionContext executionContext = createExecutionContext(describeEndpointAuthorizationRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DescribeEndpointAuthorizationRequestMarshaller().marshall(super.beforeMarshalling(describeEndpointAuthorizationRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Redshift");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DescribeEndpointAuthorization");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
StaxResponseHandler responseHandler = new StaxResponseHandler(
new DescribeEndpointAuthorizationResultStaxUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Displays a list of event categories for all event source types, or for a specified source type. For a list of the
* event categories and source types, go to Amazon Redshift
* Event Notifications.
*
*
* @param describeEventCategoriesRequest
* @return Result of the DescribeEventCategories operation returned by the service.
* @sample AmazonRedshift.DescribeEventCategories
* @see AWS API Documentation
*/
@Override
public DescribeEventCategoriesResult describeEventCategories(DescribeEventCategoriesRequest request) {
request = beforeClientExecution(request);
return executeDescribeEventCategories(request);
}
@SdkInternalApi
final DescribeEventCategoriesResult executeDescribeEventCategories(DescribeEventCategoriesRequest describeEventCategoriesRequest) {
ExecutionContext executionContext = createExecutionContext(describeEventCategoriesRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DescribeEventCategoriesRequestMarshaller().marshall(super.beforeMarshalling(describeEventCategoriesRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Redshift");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DescribeEventCategories");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
StaxResponseHandler responseHandler = new StaxResponseHandler(
new DescribeEventCategoriesResultStaxUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
@Override
public DescribeEventCategoriesResult describeEventCategories() {
return describeEventCategories(new DescribeEventCategoriesRequest());
}
/**
*
* Lists descriptions of all the Amazon Redshift event notification subscriptions for a customer account. If you
* specify a subscription name, lists the description for that subscription.
*
*
* If you specify both tag keys and tag values in the same request, Amazon Redshift returns all event notification
* subscriptions that match any combination of the specified keys and values. For example, if you have
* owner
and environment
for tag keys, and admin
and test
for
* tag values, all subscriptions that have any combination of those values are returned.
*
*
* If both tag keys and values are omitted from the request, subscriptions are returned regardless of whether they
* have tag keys or values associated with them.
*
*
* @param describeEventSubscriptionsRequest
* @return Result of the DescribeEventSubscriptions operation returned by the service.
* @throws SubscriptionNotFoundException
* An Amazon Redshift event notification subscription with the specified name does not exist.
* @throws InvalidTagException
* The tag is invalid.
* @sample AmazonRedshift.DescribeEventSubscriptions
* @see AWS API Documentation
*/
@Override
public DescribeEventSubscriptionsResult describeEventSubscriptions(DescribeEventSubscriptionsRequest request) {
request = beforeClientExecution(request);
return executeDescribeEventSubscriptions(request);
}
@SdkInternalApi
final DescribeEventSubscriptionsResult executeDescribeEventSubscriptions(DescribeEventSubscriptionsRequest describeEventSubscriptionsRequest) {
ExecutionContext executionContext = createExecutionContext(describeEventSubscriptionsRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DescribeEventSubscriptionsRequestMarshaller().marshall(super.beforeMarshalling(describeEventSubscriptionsRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Redshift");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DescribeEventSubscriptions");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
StaxResponseHandler responseHandler = new StaxResponseHandler(
new DescribeEventSubscriptionsResultStaxUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
@Override
public DescribeEventSubscriptionsResult describeEventSubscriptions() {
return describeEventSubscriptions(new DescribeEventSubscriptionsRequest());
}
/**
*
* Returns events related to clusters, security groups, snapshots, and parameter groups for the past 14 days. Events
* specific to a particular cluster, security group, snapshot or parameter group can be obtained by providing the
* name as a parameter. By default, the past hour of events are returned.
*
*
* @param describeEventsRequest
* @return Result of the DescribeEvents operation returned by the service.
* @sample AmazonRedshift.DescribeEvents
* @see AWS API
* Documentation
*/
@Override
public DescribeEventsResult describeEvents(DescribeEventsRequest request) {
request = beforeClientExecution(request);
return executeDescribeEvents(request);
}
@SdkInternalApi
final DescribeEventsResult executeDescribeEvents(DescribeEventsRequest describeEventsRequest) {
ExecutionContext executionContext = createExecutionContext(describeEventsRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DescribeEventsRequestMarshaller().marshall(super.beforeMarshalling(describeEventsRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Redshift");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DescribeEvents");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
StaxResponseHandler responseHandler = new StaxResponseHandler(
new DescribeEventsResultStaxUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
@Override
public DescribeEventsResult describeEvents() {
return describeEvents(new DescribeEventsRequest());
}
/**
*
* Returns information about the specified HSM client certificate. If no certificate ID is specified, returns
* information about all the HSM certificates owned by your Amazon Web Services account.
*
*
* If you specify both tag keys and tag values in the same request, Amazon Redshift returns all HSM client
* certificates that match any combination of the specified keys and values. For example, if you have
* owner
and environment
for tag keys, and admin
and test
for
* tag values, all HSM client certificates that have any combination of those values are returned.
*
*
* If both tag keys and values are omitted from the request, HSM client certificates are returned regardless of
* whether they have tag keys or values associated with them.
*
*
* @param describeHsmClientCertificatesRequest
* @return Result of the DescribeHsmClientCertificates operation returned by the service.
* @throws HsmClientCertificateNotFoundException
* There is no Amazon Redshift HSM client certificate with the specified identifier.
* @throws InvalidTagException
* The tag is invalid.
* @sample AmazonRedshift.DescribeHsmClientCertificates
* @see AWS API Documentation
*/
@Override
public DescribeHsmClientCertificatesResult describeHsmClientCertificates(DescribeHsmClientCertificatesRequest request) {
request = beforeClientExecution(request);
return executeDescribeHsmClientCertificates(request);
}
@SdkInternalApi
final DescribeHsmClientCertificatesResult executeDescribeHsmClientCertificates(DescribeHsmClientCertificatesRequest describeHsmClientCertificatesRequest) {
ExecutionContext executionContext = createExecutionContext(describeHsmClientCertificatesRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DescribeHsmClientCertificatesRequestMarshaller().marshall(super.beforeMarshalling(describeHsmClientCertificatesRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Redshift");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DescribeHsmClientCertificates");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
StaxResponseHandler responseHandler = new StaxResponseHandler(
new DescribeHsmClientCertificatesResultStaxUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
@Override
public DescribeHsmClientCertificatesResult describeHsmClientCertificates() {
return describeHsmClientCertificates(new DescribeHsmClientCertificatesRequest());
}
/**
*
* Returns information about the specified Amazon Redshift HSM configuration. If no configuration ID is specified,
* returns information about all the HSM configurations owned by your Amazon Web Services account.
*
*
* If you specify both tag keys and tag values in the same request, Amazon Redshift returns all HSM connections that
* match any combination of the specified keys and values. For example, if you have owner
and
* environment
for tag keys, and admin
and test
for tag values, all HSM
* connections that have any combination of those values are returned.
*
*
* If both tag keys and values are omitted from the request, HSM connections are returned regardless of whether they
* have tag keys or values associated with them.
*
*
* @param describeHsmConfigurationsRequest
* @return Result of the DescribeHsmConfigurations operation returned by the service.
* @throws HsmConfigurationNotFoundException
* There is no Amazon Redshift HSM configuration with the specified identifier.
* @throws InvalidTagException
* The tag is invalid.
* @sample AmazonRedshift.DescribeHsmConfigurations
* @see AWS API Documentation
*/
@Override
public DescribeHsmConfigurationsResult describeHsmConfigurations(DescribeHsmConfigurationsRequest request) {
request = beforeClientExecution(request);
return executeDescribeHsmConfigurations(request);
}
@SdkInternalApi
final DescribeHsmConfigurationsResult executeDescribeHsmConfigurations(DescribeHsmConfigurationsRequest describeHsmConfigurationsRequest) {
ExecutionContext executionContext = createExecutionContext(describeHsmConfigurationsRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DescribeHsmConfigurationsRequestMarshaller().marshall(super.beforeMarshalling(describeHsmConfigurationsRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Redshift");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DescribeHsmConfigurations");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
StaxResponseHandler responseHandler = new StaxResponseHandler(
new DescribeHsmConfigurationsResultStaxUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
@Override
public DescribeHsmConfigurationsResult describeHsmConfigurations() {
return describeHsmConfigurations(new DescribeHsmConfigurationsRequest());
}
/**
*
* Returns a list of inbound integrations.
*
*
* @param describeInboundIntegrationsRequest
* @return Result of the DescribeInboundIntegrations operation returned by the service.
* @throws IntegrationNotFoundException
* The integration can't be found.
* @throws InvalidNamespaceException
* The namespace isn't valid because the namespace doesn't exist. Provide a valid namespace.
* @throws UnsupportedOperationException
* The requested operation isn't supported.
* @sample AmazonRedshift.DescribeInboundIntegrations
* @see AWS API Documentation
*/
@Override
public DescribeInboundIntegrationsResult describeInboundIntegrations(DescribeInboundIntegrationsRequest request) {
request = beforeClientExecution(request);
return executeDescribeInboundIntegrations(request);
}
@SdkInternalApi
final DescribeInboundIntegrationsResult executeDescribeInboundIntegrations(DescribeInboundIntegrationsRequest describeInboundIntegrationsRequest) {
ExecutionContext executionContext = createExecutionContext(describeInboundIntegrationsRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DescribeInboundIntegrationsRequestMarshaller().marshall(super.beforeMarshalling(describeInboundIntegrationsRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Redshift");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DescribeInboundIntegrations");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
StaxResponseHandler responseHandler = new StaxResponseHandler(
new DescribeInboundIntegrationsResultStaxUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Describes whether information, such as queries and connection attempts, is being logged for the specified Amazon
* Redshift cluster.
*
*
* @param describeLoggingStatusRequest
* @return Result of the DescribeLoggingStatus operation returned by the service.
* @throws ClusterNotFoundException
* The ClusterIdentifier
parameter does not refer to an existing cluster.
* @throws UnsupportedOperationException
* The requested operation isn't supported.
* @sample AmazonRedshift.DescribeLoggingStatus
* @see AWS
* API Documentation
*/
@Override
public DescribeLoggingStatusResult describeLoggingStatus(DescribeLoggingStatusRequest request) {
request = beforeClientExecution(request);
return executeDescribeLoggingStatus(request);
}
@SdkInternalApi
final DescribeLoggingStatusResult executeDescribeLoggingStatus(DescribeLoggingStatusRequest describeLoggingStatusRequest) {
ExecutionContext executionContext = createExecutionContext(describeLoggingStatusRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DescribeLoggingStatusRequestMarshaller().marshall(super.beforeMarshalling(describeLoggingStatusRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Redshift");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DescribeLoggingStatus");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
StaxResponseHandler responseHandler = new StaxResponseHandler(
new DescribeLoggingStatusResultStaxUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Returns properties of possible node configurations such as node type, number of nodes, and disk usage for the
* specified action type.
*
*
* @param describeNodeConfigurationOptionsRequest
* @return Result of the DescribeNodeConfigurationOptions operation returned by the service.
* @throws ClusterSnapshotNotFoundException
* The snapshot identifier does not refer to an existing cluster snapshot.
* @throws InvalidClusterSnapshotStateException
* The specified cluster snapshot is not in the available
state, or other accounts are
* authorized to access the snapshot.
* @throws ClusterNotFoundException
* The ClusterIdentifier
parameter does not refer to an existing cluster.
* @throws AccessToSnapshotDeniedException
* The owner of the specified snapshot has not authorized your account to access the snapshot.
* @throws UnsupportedOperationException
* The requested operation isn't supported.
* @sample AmazonRedshift.DescribeNodeConfigurationOptions
* @see AWS API Documentation
*/
@Override
public DescribeNodeConfigurationOptionsResult describeNodeConfigurationOptions(DescribeNodeConfigurationOptionsRequest request) {
request = beforeClientExecution(request);
return executeDescribeNodeConfigurationOptions(request);
}
@SdkInternalApi
final DescribeNodeConfigurationOptionsResult executeDescribeNodeConfigurationOptions(
DescribeNodeConfigurationOptionsRequest describeNodeConfigurationOptionsRequest) {
ExecutionContext executionContext = createExecutionContext(describeNodeConfigurationOptionsRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DescribeNodeConfigurationOptionsRequestMarshaller().marshall(super.beforeMarshalling(describeNodeConfigurationOptionsRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Redshift");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DescribeNodeConfigurationOptions");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
StaxResponseHandler responseHandler = new StaxResponseHandler(
new DescribeNodeConfigurationOptionsResultStaxUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Returns a list of orderable cluster options. Before you create a new cluster you can use this operation to find
* what options are available, such as the EC2 Availability Zones (AZ) in the specific Amazon Web Services Region
* that you can specify, and the node types you can request. The node types differ by available storage, memory, CPU
* and price. With the cost involved you might want to obtain a list of cluster options in the specific region and
* specify values when creating a cluster. For more information about managing clusters, go to Amazon Redshift Clusters
* in the Amazon Redshift Cluster Management Guide.
*
*
* @param describeOrderableClusterOptionsRequest
* @return Result of the DescribeOrderableClusterOptions operation returned by the service.
* @sample AmazonRedshift.DescribeOrderableClusterOptions
* @see AWS API Documentation
*/
@Override
public DescribeOrderableClusterOptionsResult describeOrderableClusterOptions(DescribeOrderableClusterOptionsRequest request) {
request = beforeClientExecution(request);
return executeDescribeOrderableClusterOptions(request);
}
@SdkInternalApi
final DescribeOrderableClusterOptionsResult executeDescribeOrderableClusterOptions(
DescribeOrderableClusterOptionsRequest describeOrderableClusterOptionsRequest) {
ExecutionContext executionContext = createExecutionContext(describeOrderableClusterOptionsRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DescribeOrderableClusterOptionsRequestMarshaller().marshall(super.beforeMarshalling(describeOrderableClusterOptionsRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Redshift");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DescribeOrderableClusterOptions");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
StaxResponseHandler responseHandler = new StaxResponseHandler(
new DescribeOrderableClusterOptionsResultStaxUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
@Override
public DescribeOrderableClusterOptionsResult describeOrderableClusterOptions() {
return describeOrderableClusterOptions(new DescribeOrderableClusterOptionsRequest());
}
/**
*
* Returns information about the partner integrations defined for a cluster.
*
*
* @param describePartnersRequest
* @return Result of the DescribePartners operation returned by the service.
* @throws ClusterNotFoundException
* The ClusterIdentifier
parameter does not refer to an existing cluster.
* @throws UnauthorizedPartnerIntegrationException
* The partner integration is not authorized.
* @throws UnsupportedOperationException
* The requested operation isn't supported.
* @sample AmazonRedshift.DescribePartners
* @see AWS API
* Documentation
*/
@Override
public DescribePartnersResult describePartners(DescribePartnersRequest request) {
request = beforeClientExecution(request);
return executeDescribePartners(request);
}
@SdkInternalApi
final DescribePartnersResult executeDescribePartners(DescribePartnersRequest describePartnersRequest) {
ExecutionContext executionContext = createExecutionContext(describePartnersRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DescribePartnersRequestMarshaller().marshall(super.beforeMarshalling(describePartnersRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Redshift");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DescribePartners");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
StaxResponseHandler responseHandler = new StaxResponseHandler(
new DescribePartnersResultStaxUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Lists the Amazon Redshift IAM Identity Center applications.
*
*
* @param describeRedshiftIdcApplicationsRequest
* @return Result of the DescribeRedshiftIdcApplications operation returned by the service.
* @throws RedshiftIdcApplicationNotExistsException
* The application you attempted to find doesn't exist.
* @throws DependentServiceUnavailableException
* Your request cannot be completed because a dependent internal service is temporarily unavailable. Wait 30
* to 60 seconds and try again.
* @throws UnsupportedOperationException
* The requested operation isn't supported.
* @throws DependentServiceAccessDeniedException
* A dependent service denied access for the integration.
* @sample AmazonRedshift.DescribeRedshiftIdcApplications
* @see AWS API Documentation
*/
@Override
public DescribeRedshiftIdcApplicationsResult describeRedshiftIdcApplications(DescribeRedshiftIdcApplicationsRequest request) {
request = beforeClientExecution(request);
return executeDescribeRedshiftIdcApplications(request);
}
@SdkInternalApi
final DescribeRedshiftIdcApplicationsResult executeDescribeRedshiftIdcApplications(
DescribeRedshiftIdcApplicationsRequest describeRedshiftIdcApplicationsRequest) {
ExecutionContext executionContext = createExecutionContext(describeRedshiftIdcApplicationsRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DescribeRedshiftIdcApplicationsRequestMarshaller().marshall(super.beforeMarshalling(describeRedshiftIdcApplicationsRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Redshift");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DescribeRedshiftIdcApplications");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
StaxResponseHandler responseHandler = new StaxResponseHandler(
new DescribeRedshiftIdcApplicationsResultStaxUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Returns exchange status details and associated metadata for a reserved-node exchange. Statuses include such
* values as in progress and requested.
*
*
* @param describeReservedNodeExchangeStatusRequest
* @return Result of the DescribeReservedNodeExchangeStatus operation returned by the service.
* @throws ReservedNodeNotFoundException
* The specified reserved compute node not found.
* @throws ReservedNodeExchangeNotFoundException
* The reserved-node exchange status wasn't found.
* @throws UnsupportedOperationException
* The requested operation isn't supported.
* @sample AmazonRedshift.DescribeReservedNodeExchangeStatus
* @see AWS API Documentation
*/
@Override
public DescribeReservedNodeExchangeStatusResult describeReservedNodeExchangeStatus(DescribeReservedNodeExchangeStatusRequest request) {
request = beforeClientExecution(request);
return executeDescribeReservedNodeExchangeStatus(request);
}
@SdkInternalApi
final DescribeReservedNodeExchangeStatusResult executeDescribeReservedNodeExchangeStatus(
DescribeReservedNodeExchangeStatusRequest describeReservedNodeExchangeStatusRequest) {
ExecutionContext executionContext = createExecutionContext(describeReservedNodeExchangeStatusRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DescribeReservedNodeExchangeStatusRequestMarshaller()
.marshall(super.beforeMarshalling(describeReservedNodeExchangeStatusRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Redshift");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DescribeReservedNodeExchangeStatus");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
StaxResponseHandler responseHandler = new StaxResponseHandler(
new DescribeReservedNodeExchangeStatusResultStaxUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Returns a list of the available reserved node offerings by Amazon Redshift with their descriptions including the
* node type, the fixed and recurring costs of reserving the node and duration the node will be reserved for you.
* These descriptions help you determine which reserve node offering you want to purchase. You then use the unique
* offering ID in you call to PurchaseReservedNodeOffering to reserve one or more nodes for your Amazon
* Redshift cluster.
*
*
* For more information about reserved node offerings, go to Purchasing Reserved
* Nodes in the Amazon Redshift Cluster Management Guide.
*
*
* @param describeReservedNodeOfferingsRequest
* @return Result of the DescribeReservedNodeOfferings operation returned by the service.
* @throws ReservedNodeOfferingNotFoundException
* Specified offering does not exist.
* @throws UnsupportedOperationException
* The requested operation isn't supported.
* @throws DependentServiceUnavailableException
* Your request cannot be completed because a dependent internal service is temporarily unavailable. Wait 30
* to 60 seconds and try again.
* @sample AmazonRedshift.DescribeReservedNodeOfferings
* @see AWS API Documentation
*/
@Override
public DescribeReservedNodeOfferingsResult describeReservedNodeOfferings(DescribeReservedNodeOfferingsRequest request) {
request = beforeClientExecution(request);
return executeDescribeReservedNodeOfferings(request);
}
@SdkInternalApi
final DescribeReservedNodeOfferingsResult executeDescribeReservedNodeOfferings(DescribeReservedNodeOfferingsRequest describeReservedNodeOfferingsRequest) {
ExecutionContext executionContext = createExecutionContext(describeReservedNodeOfferingsRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DescribeReservedNodeOfferingsRequestMarshaller().marshall(super.beforeMarshalling(describeReservedNodeOfferingsRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Redshift");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DescribeReservedNodeOfferings");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
StaxResponseHandler responseHandler = new StaxResponseHandler(
new DescribeReservedNodeOfferingsResultStaxUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
@Override
public DescribeReservedNodeOfferingsResult describeReservedNodeOfferings() {
return describeReservedNodeOfferings(new DescribeReservedNodeOfferingsRequest());
}
/**
*
* Returns the descriptions of the reserved nodes.
*
*
* @param describeReservedNodesRequest
* @return Result of the DescribeReservedNodes operation returned by the service.
* @throws ReservedNodeNotFoundException
* The specified reserved compute node not found.
* @throws DependentServiceUnavailableException
* Your request cannot be completed because a dependent internal service is temporarily unavailable. Wait 30
* to 60 seconds and try again.
* @sample AmazonRedshift.DescribeReservedNodes
* @see AWS
* API Documentation
*/
@Override
public DescribeReservedNodesResult describeReservedNodes(DescribeReservedNodesRequest request) {
request = beforeClientExecution(request);
return executeDescribeReservedNodes(request);
}
@SdkInternalApi
final DescribeReservedNodesResult executeDescribeReservedNodes(DescribeReservedNodesRequest describeReservedNodesRequest) {
ExecutionContext executionContext = createExecutionContext(describeReservedNodesRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DescribeReservedNodesRequestMarshaller().marshall(super.beforeMarshalling(describeReservedNodesRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Redshift");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DescribeReservedNodes");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
StaxResponseHandler responseHandler = new StaxResponseHandler(
new DescribeReservedNodesResultStaxUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
@Override
public DescribeReservedNodesResult describeReservedNodes() {
return describeReservedNodes(new DescribeReservedNodesRequest());
}
/**
*
* Returns information about the last resize operation for the specified cluster. If no resize operation has ever
* been initiated for the specified cluster, a HTTP 404
error is returned. If a resize operation was
* initiated and completed, the status of the resize remains as SUCCEEDED
until the next resize.
*
*
* A resize operation can be requested using ModifyCluster and specifying a different number or type of nodes
* for the cluster.
*
*
* @param describeResizeRequest
* @return Result of the DescribeResize operation returned by the service.
* @throws ClusterNotFoundException
* The ClusterIdentifier
parameter does not refer to an existing cluster.
* @throws ResizeNotFoundException
* A resize operation for the specified cluster is not found.
* @throws UnsupportedOperationException
* The requested operation isn't supported.
* @sample AmazonRedshift.DescribeResize
* @see AWS API
* Documentation
*/
@Override
public DescribeResizeResult describeResize(DescribeResizeRequest request) {
request = beforeClientExecution(request);
return executeDescribeResize(request);
}
@SdkInternalApi
final DescribeResizeResult executeDescribeResize(DescribeResizeRequest describeResizeRequest) {
ExecutionContext executionContext = createExecutionContext(describeResizeRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DescribeResizeRequestMarshaller().marshall(super.beforeMarshalling(describeResizeRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Redshift");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DescribeResize");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
StaxResponseHandler responseHandler = new StaxResponseHandler(
new DescribeResizeResultStaxUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Describes properties of scheduled actions.
*
*
* @param describeScheduledActionsRequest
* @return Result of the DescribeScheduledActions operation returned by the service.
* @throws ScheduledActionNotFoundException
* The scheduled action cannot be found.
* @throws UnauthorizedOperationException
* Your account is not authorized to perform the requested operation.
* @sample AmazonRedshift.DescribeScheduledActions
* @see AWS API Documentation
*/
@Override
public DescribeScheduledActionsResult describeScheduledActions(DescribeScheduledActionsRequest request) {
request = beforeClientExecution(request);
return executeDescribeScheduledActions(request);
}
@SdkInternalApi
final DescribeScheduledActionsResult executeDescribeScheduledActions(DescribeScheduledActionsRequest describeScheduledActionsRequest) {
ExecutionContext executionContext = createExecutionContext(describeScheduledActionsRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DescribeScheduledActionsRequestMarshaller().marshall(super.beforeMarshalling(describeScheduledActionsRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Redshift");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DescribeScheduledActions");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
StaxResponseHandler responseHandler = new StaxResponseHandler(
new DescribeScheduledActionsResultStaxUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Returns a list of snapshot copy grants owned by the Amazon Web Services account in the destination region.
*
*
* For more information about managing snapshot copy grants, go to Amazon Redshift Database
* Encryption in the Amazon Redshift Cluster Management Guide.
*
*
* @param describeSnapshotCopyGrantsRequest
* The result of the DescribeSnapshotCopyGrants
action.
* @return Result of the DescribeSnapshotCopyGrants operation returned by the service.
* @throws SnapshotCopyGrantNotFoundException
* The specified snapshot copy grant can't be found. Make sure that the name is typed correctly and that the
* grant exists in the destination region.
* @throws InvalidTagException
* The tag is invalid.
* @sample AmazonRedshift.DescribeSnapshotCopyGrants
* @see AWS API Documentation
*/
@Override
public DescribeSnapshotCopyGrantsResult describeSnapshotCopyGrants(DescribeSnapshotCopyGrantsRequest request) {
request = beforeClientExecution(request);
return executeDescribeSnapshotCopyGrants(request);
}
@SdkInternalApi
final DescribeSnapshotCopyGrantsResult executeDescribeSnapshotCopyGrants(DescribeSnapshotCopyGrantsRequest describeSnapshotCopyGrantsRequest) {
ExecutionContext executionContext = createExecutionContext(describeSnapshotCopyGrantsRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DescribeSnapshotCopyGrantsRequestMarshaller().marshall(super.beforeMarshalling(describeSnapshotCopyGrantsRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Redshift");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DescribeSnapshotCopyGrants");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
StaxResponseHandler responseHandler = new StaxResponseHandler(
new DescribeSnapshotCopyGrantsResultStaxUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
@Override
public DescribeSnapshotCopyGrantsResult describeSnapshotCopyGrants() {
return describeSnapshotCopyGrants(new DescribeSnapshotCopyGrantsRequest());
}
/**
*