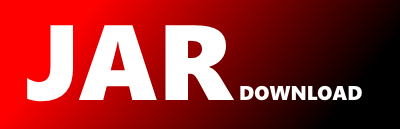
com.amazonaws.services.redshift.model.CancelResizeResult Maven / Gradle / Ivy
Show all versions of aws-java-sdk-redshift Show documentation
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.redshift.model;
import java.io.Serializable;
import javax.annotation.Generated;
/**
*
* Describes the result of a cluster resize operation.
*
*
* @see AWS API
* Documentation
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class CancelResizeResult extends com.amazonaws.AmazonWebServiceResult implements Serializable, Cloneable {
/**
*
* The node type that the cluster will have after the resize operation is complete.
*
*/
private String targetNodeType;
/**
*
* The number of nodes that the cluster will have after the resize operation is complete.
*
*/
private Integer targetNumberOfNodes;
/**
*
* The cluster type after the resize operation is complete.
*
*
* Valid Values: multi-node
| single-node
*
*/
private String targetClusterType;
/**
*
* The status of the resize operation.
*
*
* Valid Values: NONE
| IN_PROGRESS
| FAILED
| SUCCEEDED
|
* CANCELLING
*
*/
private String status;
/**
*
* The names of tables that have been completely imported .
*
*
* Valid Values: List of table names.
*
*/
private com.amazonaws.internal.SdkInternalList importTablesCompleted;
/**
*
* The names of tables that are being currently imported.
*
*
* Valid Values: List of table names.
*
*/
private com.amazonaws.internal.SdkInternalList importTablesInProgress;
/**
*
* The names of tables that have not been yet imported.
*
*
* Valid Values: List of table names
*
*/
private com.amazonaws.internal.SdkInternalList importTablesNotStarted;
/**
*
* The average rate of the resize operation over the last few minutes, measured in megabytes per second. After the
* resize operation completes, this value shows the average rate of the entire resize operation.
*
*/
private Double avgResizeRateInMegaBytesPerSecond;
/**
*
* The estimated total amount of data, in megabytes, on the cluster before the resize operation began.
*
*/
private Long totalResizeDataInMegaBytes;
/**
*
* While the resize operation is in progress, this value shows the current amount of data, in megabytes, that has
* been processed so far. When the resize operation is complete, this value shows the total amount of data, in
* megabytes, on the cluster, which may be more or less than TotalResizeDataInMegaBytes (the estimated total amount
* of data before resize).
*
*/
private Long progressInMegaBytes;
/**
*
* The amount of seconds that have elapsed since the resize operation began. After the resize operation completes,
* this value shows the total actual time, in seconds, for the resize operation.
*
*/
private Long elapsedTimeInSeconds;
/**
*
* The estimated time remaining, in seconds, until the resize operation is complete. This value is calculated based
* on the average resize rate and the estimated amount of data remaining to be processed. Once the resize operation
* is complete, this value will be 0.
*
*/
private Long estimatedTimeToCompletionInSeconds;
/**
*
* An enum with possible values of ClassicResize
and ElasticResize
. These values describe
* the type of resize operation being performed.
*
*/
private String resizeType;
/**
*
* An optional string to provide additional details about the resize action.
*
*/
private String message;
/**
*
* The type of encryption for the cluster after the resize is complete.
*
*
* Possible values are KMS
and None
.
*
*/
private String targetEncryptionType;
/**
*
* The percent of data transferred from source cluster to target cluster.
*
*/
private Double dataTransferProgressPercent;
/**
*
* The node type that the cluster will have after the resize operation is complete.
*
*
* @param targetNodeType
* The node type that the cluster will have after the resize operation is complete.
*/
public void setTargetNodeType(String targetNodeType) {
this.targetNodeType = targetNodeType;
}
/**
*
* The node type that the cluster will have after the resize operation is complete.
*
*
* @return The node type that the cluster will have after the resize operation is complete.
*/
public String getTargetNodeType() {
return this.targetNodeType;
}
/**
*
* The node type that the cluster will have after the resize operation is complete.
*
*
* @param targetNodeType
* The node type that the cluster will have after the resize operation is complete.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CancelResizeResult withTargetNodeType(String targetNodeType) {
setTargetNodeType(targetNodeType);
return this;
}
/**
*
* The number of nodes that the cluster will have after the resize operation is complete.
*
*
* @param targetNumberOfNodes
* The number of nodes that the cluster will have after the resize operation is complete.
*/
public void setTargetNumberOfNodes(Integer targetNumberOfNodes) {
this.targetNumberOfNodes = targetNumberOfNodes;
}
/**
*
* The number of nodes that the cluster will have after the resize operation is complete.
*
*
* @return The number of nodes that the cluster will have after the resize operation is complete.
*/
public Integer getTargetNumberOfNodes() {
return this.targetNumberOfNodes;
}
/**
*
* The number of nodes that the cluster will have after the resize operation is complete.
*
*
* @param targetNumberOfNodes
* The number of nodes that the cluster will have after the resize operation is complete.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CancelResizeResult withTargetNumberOfNodes(Integer targetNumberOfNodes) {
setTargetNumberOfNodes(targetNumberOfNodes);
return this;
}
/**
*
* The cluster type after the resize operation is complete.
*
*
* Valid Values: multi-node
| single-node
*
*
* @param targetClusterType
* The cluster type after the resize operation is complete.
*
* Valid Values: multi-node
| single-node
*/
public void setTargetClusterType(String targetClusterType) {
this.targetClusterType = targetClusterType;
}
/**
*
* The cluster type after the resize operation is complete.
*
*
* Valid Values: multi-node
| single-node
*
*
* @return The cluster type after the resize operation is complete.
*
* Valid Values: multi-node
| single-node
*/
public String getTargetClusterType() {
return this.targetClusterType;
}
/**
*
* The cluster type after the resize operation is complete.
*
*
* Valid Values: multi-node
| single-node
*
*
* @param targetClusterType
* The cluster type after the resize operation is complete.
*
* Valid Values: multi-node
| single-node
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CancelResizeResult withTargetClusterType(String targetClusterType) {
setTargetClusterType(targetClusterType);
return this;
}
/**
*
* The status of the resize operation.
*
*
* Valid Values: NONE
| IN_PROGRESS
| FAILED
| SUCCEEDED
|
* CANCELLING
*
*
* @param status
* The status of the resize operation.
*
* Valid Values: NONE
| IN_PROGRESS
| FAILED
| SUCCEEDED
* | CANCELLING
*/
public void setStatus(String status) {
this.status = status;
}
/**
*
* The status of the resize operation.
*
*
* Valid Values: NONE
| IN_PROGRESS
| FAILED
| SUCCEEDED
|
* CANCELLING
*
*
* @return The status of the resize operation.
*
* Valid Values: NONE
| IN_PROGRESS
| FAILED
| SUCCEEDED
* | CANCELLING
*/
public String getStatus() {
return this.status;
}
/**
*
* The status of the resize operation.
*
*
* Valid Values: NONE
| IN_PROGRESS
| FAILED
| SUCCEEDED
|
* CANCELLING
*
*
* @param status
* The status of the resize operation.
*
* Valid Values: NONE
| IN_PROGRESS
| FAILED
| SUCCEEDED
* | CANCELLING
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CancelResizeResult withStatus(String status) {
setStatus(status);
return this;
}
/**
*
* The names of tables that have been completely imported .
*
*
* Valid Values: List of table names.
*
*
* @return The names of tables that have been completely imported .
*
* Valid Values: List of table names.
*/
public java.util.List getImportTablesCompleted() {
if (importTablesCompleted == null) {
importTablesCompleted = new com.amazonaws.internal.SdkInternalList();
}
return importTablesCompleted;
}
/**
*
* The names of tables that have been completely imported .
*
*
* Valid Values: List of table names.
*
*
* @param importTablesCompleted
* The names of tables that have been completely imported .
*
* Valid Values: List of table names.
*/
public void setImportTablesCompleted(java.util.Collection importTablesCompleted) {
if (importTablesCompleted == null) {
this.importTablesCompleted = null;
return;
}
this.importTablesCompleted = new com.amazonaws.internal.SdkInternalList(importTablesCompleted);
}
/**
*
* The names of tables that have been completely imported .
*
*
* Valid Values: List of table names.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setImportTablesCompleted(java.util.Collection)} or
* {@link #withImportTablesCompleted(java.util.Collection)} if you want to override the existing values.
*
*
* @param importTablesCompleted
* The names of tables that have been completely imported .
*
* Valid Values: List of table names.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CancelResizeResult withImportTablesCompleted(String... importTablesCompleted) {
if (this.importTablesCompleted == null) {
setImportTablesCompleted(new com.amazonaws.internal.SdkInternalList(importTablesCompleted.length));
}
for (String ele : importTablesCompleted) {
this.importTablesCompleted.add(ele);
}
return this;
}
/**
*
* The names of tables that have been completely imported .
*
*
* Valid Values: List of table names.
*
*
* @param importTablesCompleted
* The names of tables that have been completely imported .
*
* Valid Values: List of table names.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CancelResizeResult withImportTablesCompleted(java.util.Collection importTablesCompleted) {
setImportTablesCompleted(importTablesCompleted);
return this;
}
/**
*
* The names of tables that are being currently imported.
*
*
* Valid Values: List of table names.
*
*
* @return The names of tables that are being currently imported.
*
* Valid Values: List of table names.
*/
public java.util.List getImportTablesInProgress() {
if (importTablesInProgress == null) {
importTablesInProgress = new com.amazonaws.internal.SdkInternalList();
}
return importTablesInProgress;
}
/**
*
* The names of tables that are being currently imported.
*
*
* Valid Values: List of table names.
*
*
* @param importTablesInProgress
* The names of tables that are being currently imported.
*
* Valid Values: List of table names.
*/
public void setImportTablesInProgress(java.util.Collection importTablesInProgress) {
if (importTablesInProgress == null) {
this.importTablesInProgress = null;
return;
}
this.importTablesInProgress = new com.amazonaws.internal.SdkInternalList(importTablesInProgress);
}
/**
*
* The names of tables that are being currently imported.
*
*
* Valid Values: List of table names.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setImportTablesInProgress(java.util.Collection)} or
* {@link #withImportTablesInProgress(java.util.Collection)} if you want to override the existing values.
*
*
* @param importTablesInProgress
* The names of tables that are being currently imported.
*
* Valid Values: List of table names.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CancelResizeResult withImportTablesInProgress(String... importTablesInProgress) {
if (this.importTablesInProgress == null) {
setImportTablesInProgress(new com.amazonaws.internal.SdkInternalList(importTablesInProgress.length));
}
for (String ele : importTablesInProgress) {
this.importTablesInProgress.add(ele);
}
return this;
}
/**
*
* The names of tables that are being currently imported.
*
*
* Valid Values: List of table names.
*
*
* @param importTablesInProgress
* The names of tables that are being currently imported.
*
* Valid Values: List of table names.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CancelResizeResult withImportTablesInProgress(java.util.Collection importTablesInProgress) {
setImportTablesInProgress(importTablesInProgress);
return this;
}
/**
*
* The names of tables that have not been yet imported.
*
*
* Valid Values: List of table names
*
*
* @return The names of tables that have not been yet imported.
*
* Valid Values: List of table names
*/
public java.util.List getImportTablesNotStarted() {
if (importTablesNotStarted == null) {
importTablesNotStarted = new com.amazonaws.internal.SdkInternalList();
}
return importTablesNotStarted;
}
/**
*
* The names of tables that have not been yet imported.
*
*
* Valid Values: List of table names
*
*
* @param importTablesNotStarted
* The names of tables that have not been yet imported.
*
* Valid Values: List of table names
*/
public void setImportTablesNotStarted(java.util.Collection importTablesNotStarted) {
if (importTablesNotStarted == null) {
this.importTablesNotStarted = null;
return;
}
this.importTablesNotStarted = new com.amazonaws.internal.SdkInternalList(importTablesNotStarted);
}
/**
*
* The names of tables that have not been yet imported.
*
*
* Valid Values: List of table names
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setImportTablesNotStarted(java.util.Collection)} or
* {@link #withImportTablesNotStarted(java.util.Collection)} if you want to override the existing values.
*
*
* @param importTablesNotStarted
* The names of tables that have not been yet imported.
*
* Valid Values: List of table names
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CancelResizeResult withImportTablesNotStarted(String... importTablesNotStarted) {
if (this.importTablesNotStarted == null) {
setImportTablesNotStarted(new com.amazonaws.internal.SdkInternalList(importTablesNotStarted.length));
}
for (String ele : importTablesNotStarted) {
this.importTablesNotStarted.add(ele);
}
return this;
}
/**
*
* The names of tables that have not been yet imported.
*
*
* Valid Values: List of table names
*
*
* @param importTablesNotStarted
* The names of tables that have not been yet imported.
*
* Valid Values: List of table names
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CancelResizeResult withImportTablesNotStarted(java.util.Collection importTablesNotStarted) {
setImportTablesNotStarted(importTablesNotStarted);
return this;
}
/**
*
* The average rate of the resize operation over the last few minutes, measured in megabytes per second. After the
* resize operation completes, this value shows the average rate of the entire resize operation.
*
*
* @param avgResizeRateInMegaBytesPerSecond
* The average rate of the resize operation over the last few minutes, measured in megabytes per second.
* After the resize operation completes, this value shows the average rate of the entire resize operation.
*/
public void setAvgResizeRateInMegaBytesPerSecond(Double avgResizeRateInMegaBytesPerSecond) {
this.avgResizeRateInMegaBytesPerSecond = avgResizeRateInMegaBytesPerSecond;
}
/**
*
* The average rate of the resize operation over the last few minutes, measured in megabytes per second. After the
* resize operation completes, this value shows the average rate of the entire resize operation.
*
*
* @return The average rate of the resize operation over the last few minutes, measured in megabytes per second.
* After the resize operation completes, this value shows the average rate of the entire resize operation.
*/
public Double getAvgResizeRateInMegaBytesPerSecond() {
return this.avgResizeRateInMegaBytesPerSecond;
}
/**
*
* The average rate of the resize operation over the last few minutes, measured in megabytes per second. After the
* resize operation completes, this value shows the average rate of the entire resize operation.
*
*
* @param avgResizeRateInMegaBytesPerSecond
* The average rate of the resize operation over the last few minutes, measured in megabytes per second.
* After the resize operation completes, this value shows the average rate of the entire resize operation.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CancelResizeResult withAvgResizeRateInMegaBytesPerSecond(Double avgResizeRateInMegaBytesPerSecond) {
setAvgResizeRateInMegaBytesPerSecond(avgResizeRateInMegaBytesPerSecond);
return this;
}
/**
*
* The estimated total amount of data, in megabytes, on the cluster before the resize operation began.
*
*
* @param totalResizeDataInMegaBytes
* The estimated total amount of data, in megabytes, on the cluster before the resize operation began.
*/
public void setTotalResizeDataInMegaBytes(Long totalResizeDataInMegaBytes) {
this.totalResizeDataInMegaBytes = totalResizeDataInMegaBytes;
}
/**
*
* The estimated total amount of data, in megabytes, on the cluster before the resize operation began.
*
*
* @return The estimated total amount of data, in megabytes, on the cluster before the resize operation began.
*/
public Long getTotalResizeDataInMegaBytes() {
return this.totalResizeDataInMegaBytes;
}
/**
*
* The estimated total amount of data, in megabytes, on the cluster before the resize operation began.
*
*
* @param totalResizeDataInMegaBytes
* The estimated total amount of data, in megabytes, on the cluster before the resize operation began.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CancelResizeResult withTotalResizeDataInMegaBytes(Long totalResizeDataInMegaBytes) {
setTotalResizeDataInMegaBytes(totalResizeDataInMegaBytes);
return this;
}
/**
*
* While the resize operation is in progress, this value shows the current amount of data, in megabytes, that has
* been processed so far. When the resize operation is complete, this value shows the total amount of data, in
* megabytes, on the cluster, which may be more or less than TotalResizeDataInMegaBytes (the estimated total amount
* of data before resize).
*
*
* @param progressInMegaBytes
* While the resize operation is in progress, this value shows the current amount of data, in megabytes, that
* has been processed so far. When the resize operation is complete, this value shows the total amount of
* data, in megabytes, on the cluster, which may be more or less than TotalResizeDataInMegaBytes (the
* estimated total amount of data before resize).
*/
public void setProgressInMegaBytes(Long progressInMegaBytes) {
this.progressInMegaBytes = progressInMegaBytes;
}
/**
*
* While the resize operation is in progress, this value shows the current amount of data, in megabytes, that has
* been processed so far. When the resize operation is complete, this value shows the total amount of data, in
* megabytes, on the cluster, which may be more or less than TotalResizeDataInMegaBytes (the estimated total amount
* of data before resize).
*
*
* @return While the resize operation is in progress, this value shows the current amount of data, in megabytes,
* that has been processed so far. When the resize operation is complete, this value shows the total amount
* of data, in megabytes, on the cluster, which may be more or less than TotalResizeDataInMegaBytes (the
* estimated total amount of data before resize).
*/
public Long getProgressInMegaBytes() {
return this.progressInMegaBytes;
}
/**
*
* While the resize operation is in progress, this value shows the current amount of data, in megabytes, that has
* been processed so far. When the resize operation is complete, this value shows the total amount of data, in
* megabytes, on the cluster, which may be more or less than TotalResizeDataInMegaBytes (the estimated total amount
* of data before resize).
*
*
* @param progressInMegaBytes
* While the resize operation is in progress, this value shows the current amount of data, in megabytes, that
* has been processed so far. When the resize operation is complete, this value shows the total amount of
* data, in megabytes, on the cluster, which may be more or less than TotalResizeDataInMegaBytes (the
* estimated total amount of data before resize).
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CancelResizeResult withProgressInMegaBytes(Long progressInMegaBytes) {
setProgressInMegaBytes(progressInMegaBytes);
return this;
}
/**
*
* The amount of seconds that have elapsed since the resize operation began. After the resize operation completes,
* this value shows the total actual time, in seconds, for the resize operation.
*
*
* @param elapsedTimeInSeconds
* The amount of seconds that have elapsed since the resize operation began. After the resize operation
* completes, this value shows the total actual time, in seconds, for the resize operation.
*/
public void setElapsedTimeInSeconds(Long elapsedTimeInSeconds) {
this.elapsedTimeInSeconds = elapsedTimeInSeconds;
}
/**
*
* The amount of seconds that have elapsed since the resize operation began. After the resize operation completes,
* this value shows the total actual time, in seconds, for the resize operation.
*
*
* @return The amount of seconds that have elapsed since the resize operation began. After the resize operation
* completes, this value shows the total actual time, in seconds, for the resize operation.
*/
public Long getElapsedTimeInSeconds() {
return this.elapsedTimeInSeconds;
}
/**
*
* The amount of seconds that have elapsed since the resize operation began. After the resize operation completes,
* this value shows the total actual time, in seconds, for the resize operation.
*
*
* @param elapsedTimeInSeconds
* The amount of seconds that have elapsed since the resize operation began. After the resize operation
* completes, this value shows the total actual time, in seconds, for the resize operation.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CancelResizeResult withElapsedTimeInSeconds(Long elapsedTimeInSeconds) {
setElapsedTimeInSeconds(elapsedTimeInSeconds);
return this;
}
/**
*
* The estimated time remaining, in seconds, until the resize operation is complete. This value is calculated based
* on the average resize rate and the estimated amount of data remaining to be processed. Once the resize operation
* is complete, this value will be 0.
*
*
* @param estimatedTimeToCompletionInSeconds
* The estimated time remaining, in seconds, until the resize operation is complete. This value is calculated
* based on the average resize rate and the estimated amount of data remaining to be processed. Once the
* resize operation is complete, this value will be 0.
*/
public void setEstimatedTimeToCompletionInSeconds(Long estimatedTimeToCompletionInSeconds) {
this.estimatedTimeToCompletionInSeconds = estimatedTimeToCompletionInSeconds;
}
/**
*
* The estimated time remaining, in seconds, until the resize operation is complete. This value is calculated based
* on the average resize rate and the estimated amount of data remaining to be processed. Once the resize operation
* is complete, this value will be 0.
*
*
* @return The estimated time remaining, in seconds, until the resize operation is complete. This value is
* calculated based on the average resize rate and the estimated amount of data remaining to be processed.
* Once the resize operation is complete, this value will be 0.
*/
public Long getEstimatedTimeToCompletionInSeconds() {
return this.estimatedTimeToCompletionInSeconds;
}
/**
*
* The estimated time remaining, in seconds, until the resize operation is complete. This value is calculated based
* on the average resize rate and the estimated amount of data remaining to be processed. Once the resize operation
* is complete, this value will be 0.
*
*
* @param estimatedTimeToCompletionInSeconds
* The estimated time remaining, in seconds, until the resize operation is complete. This value is calculated
* based on the average resize rate and the estimated amount of data remaining to be processed. Once the
* resize operation is complete, this value will be 0.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CancelResizeResult withEstimatedTimeToCompletionInSeconds(Long estimatedTimeToCompletionInSeconds) {
setEstimatedTimeToCompletionInSeconds(estimatedTimeToCompletionInSeconds);
return this;
}
/**
*
* An enum with possible values of ClassicResize
and ElasticResize
. These values describe
* the type of resize operation being performed.
*
*
* @param resizeType
* An enum with possible values of ClassicResize
and ElasticResize
. These values
* describe the type of resize operation being performed.
*/
public void setResizeType(String resizeType) {
this.resizeType = resizeType;
}
/**
*
* An enum with possible values of ClassicResize
and ElasticResize
. These values describe
* the type of resize operation being performed.
*
*
* @return An enum with possible values of ClassicResize
and ElasticResize
. These values
* describe the type of resize operation being performed.
*/
public String getResizeType() {
return this.resizeType;
}
/**
*
* An enum with possible values of ClassicResize
and ElasticResize
. These values describe
* the type of resize operation being performed.
*
*
* @param resizeType
* An enum with possible values of ClassicResize
and ElasticResize
. These values
* describe the type of resize operation being performed.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CancelResizeResult withResizeType(String resizeType) {
setResizeType(resizeType);
return this;
}
/**
*
* An optional string to provide additional details about the resize action.
*
*
* @param message
* An optional string to provide additional details about the resize action.
*/
public void setMessage(String message) {
this.message = message;
}
/**
*
* An optional string to provide additional details about the resize action.
*
*
* @return An optional string to provide additional details about the resize action.
*/
public String getMessage() {
return this.message;
}
/**
*
* An optional string to provide additional details about the resize action.
*
*
* @param message
* An optional string to provide additional details about the resize action.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CancelResizeResult withMessage(String message) {
setMessage(message);
return this;
}
/**
*
* The type of encryption for the cluster after the resize is complete.
*
*
* Possible values are KMS
and None
.
*
*
* @param targetEncryptionType
* The type of encryption for the cluster after the resize is complete.
*
* Possible values are KMS
and None
.
*/
public void setTargetEncryptionType(String targetEncryptionType) {
this.targetEncryptionType = targetEncryptionType;
}
/**
*
* The type of encryption for the cluster after the resize is complete.
*
*
* Possible values are KMS
and None
.
*
*
* @return The type of encryption for the cluster after the resize is complete.
*
* Possible values are KMS
and None
.
*/
public String getTargetEncryptionType() {
return this.targetEncryptionType;
}
/**
*
* The type of encryption for the cluster after the resize is complete.
*
*
* Possible values are KMS
and None
.
*
*
* @param targetEncryptionType
* The type of encryption for the cluster after the resize is complete.
*
* Possible values are KMS
and None
.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CancelResizeResult withTargetEncryptionType(String targetEncryptionType) {
setTargetEncryptionType(targetEncryptionType);
return this;
}
/**
*
* The percent of data transferred from source cluster to target cluster.
*
*
* @param dataTransferProgressPercent
* The percent of data transferred from source cluster to target cluster.
*/
public void setDataTransferProgressPercent(Double dataTransferProgressPercent) {
this.dataTransferProgressPercent = dataTransferProgressPercent;
}
/**
*
* The percent of data transferred from source cluster to target cluster.
*
*
* @return The percent of data transferred from source cluster to target cluster.
*/
public Double getDataTransferProgressPercent() {
return this.dataTransferProgressPercent;
}
/**
*
* The percent of data transferred from source cluster to target cluster.
*
*
* @param dataTransferProgressPercent
* The percent of data transferred from source cluster to target cluster.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CancelResizeResult withDataTransferProgressPercent(Double dataTransferProgressPercent) {
setDataTransferProgressPercent(dataTransferProgressPercent);
return this;
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getTargetNodeType() != null)
sb.append("TargetNodeType: ").append(getTargetNodeType()).append(",");
if (getTargetNumberOfNodes() != null)
sb.append("TargetNumberOfNodes: ").append(getTargetNumberOfNodes()).append(",");
if (getTargetClusterType() != null)
sb.append("TargetClusterType: ").append(getTargetClusterType()).append(",");
if (getStatus() != null)
sb.append("Status: ").append(getStatus()).append(",");
if (getImportTablesCompleted() != null)
sb.append("ImportTablesCompleted: ").append(getImportTablesCompleted()).append(",");
if (getImportTablesInProgress() != null)
sb.append("ImportTablesInProgress: ").append(getImportTablesInProgress()).append(",");
if (getImportTablesNotStarted() != null)
sb.append("ImportTablesNotStarted: ").append(getImportTablesNotStarted()).append(",");
if (getAvgResizeRateInMegaBytesPerSecond() != null)
sb.append("AvgResizeRateInMegaBytesPerSecond: ").append(getAvgResizeRateInMegaBytesPerSecond()).append(",");
if (getTotalResizeDataInMegaBytes() != null)
sb.append("TotalResizeDataInMegaBytes: ").append(getTotalResizeDataInMegaBytes()).append(",");
if (getProgressInMegaBytes() != null)
sb.append("ProgressInMegaBytes: ").append(getProgressInMegaBytes()).append(",");
if (getElapsedTimeInSeconds() != null)
sb.append("ElapsedTimeInSeconds: ").append(getElapsedTimeInSeconds()).append(",");
if (getEstimatedTimeToCompletionInSeconds() != null)
sb.append("EstimatedTimeToCompletionInSeconds: ").append(getEstimatedTimeToCompletionInSeconds()).append(",");
if (getResizeType() != null)
sb.append("ResizeType: ").append(getResizeType()).append(",");
if (getMessage() != null)
sb.append("Message: ").append(getMessage()).append(",");
if (getTargetEncryptionType() != null)
sb.append("TargetEncryptionType: ").append(getTargetEncryptionType()).append(",");
if (getDataTransferProgressPercent() != null)
sb.append("DataTransferProgressPercent: ").append(getDataTransferProgressPercent());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof CancelResizeResult == false)
return false;
CancelResizeResult other = (CancelResizeResult) obj;
if (other.getTargetNodeType() == null ^ this.getTargetNodeType() == null)
return false;
if (other.getTargetNodeType() != null && other.getTargetNodeType().equals(this.getTargetNodeType()) == false)
return false;
if (other.getTargetNumberOfNodes() == null ^ this.getTargetNumberOfNodes() == null)
return false;
if (other.getTargetNumberOfNodes() != null && other.getTargetNumberOfNodes().equals(this.getTargetNumberOfNodes()) == false)
return false;
if (other.getTargetClusterType() == null ^ this.getTargetClusterType() == null)
return false;
if (other.getTargetClusterType() != null && other.getTargetClusterType().equals(this.getTargetClusterType()) == false)
return false;
if (other.getStatus() == null ^ this.getStatus() == null)
return false;
if (other.getStatus() != null && other.getStatus().equals(this.getStatus()) == false)
return false;
if (other.getImportTablesCompleted() == null ^ this.getImportTablesCompleted() == null)
return false;
if (other.getImportTablesCompleted() != null && other.getImportTablesCompleted().equals(this.getImportTablesCompleted()) == false)
return false;
if (other.getImportTablesInProgress() == null ^ this.getImportTablesInProgress() == null)
return false;
if (other.getImportTablesInProgress() != null && other.getImportTablesInProgress().equals(this.getImportTablesInProgress()) == false)
return false;
if (other.getImportTablesNotStarted() == null ^ this.getImportTablesNotStarted() == null)
return false;
if (other.getImportTablesNotStarted() != null && other.getImportTablesNotStarted().equals(this.getImportTablesNotStarted()) == false)
return false;
if (other.getAvgResizeRateInMegaBytesPerSecond() == null ^ this.getAvgResizeRateInMegaBytesPerSecond() == null)
return false;
if (other.getAvgResizeRateInMegaBytesPerSecond() != null
&& other.getAvgResizeRateInMegaBytesPerSecond().equals(this.getAvgResizeRateInMegaBytesPerSecond()) == false)
return false;
if (other.getTotalResizeDataInMegaBytes() == null ^ this.getTotalResizeDataInMegaBytes() == null)
return false;
if (other.getTotalResizeDataInMegaBytes() != null && other.getTotalResizeDataInMegaBytes().equals(this.getTotalResizeDataInMegaBytes()) == false)
return false;
if (other.getProgressInMegaBytes() == null ^ this.getProgressInMegaBytes() == null)
return false;
if (other.getProgressInMegaBytes() != null && other.getProgressInMegaBytes().equals(this.getProgressInMegaBytes()) == false)
return false;
if (other.getElapsedTimeInSeconds() == null ^ this.getElapsedTimeInSeconds() == null)
return false;
if (other.getElapsedTimeInSeconds() != null && other.getElapsedTimeInSeconds().equals(this.getElapsedTimeInSeconds()) == false)
return false;
if (other.getEstimatedTimeToCompletionInSeconds() == null ^ this.getEstimatedTimeToCompletionInSeconds() == null)
return false;
if (other.getEstimatedTimeToCompletionInSeconds() != null
&& other.getEstimatedTimeToCompletionInSeconds().equals(this.getEstimatedTimeToCompletionInSeconds()) == false)
return false;
if (other.getResizeType() == null ^ this.getResizeType() == null)
return false;
if (other.getResizeType() != null && other.getResizeType().equals(this.getResizeType()) == false)
return false;
if (other.getMessage() == null ^ this.getMessage() == null)
return false;
if (other.getMessage() != null && other.getMessage().equals(this.getMessage()) == false)
return false;
if (other.getTargetEncryptionType() == null ^ this.getTargetEncryptionType() == null)
return false;
if (other.getTargetEncryptionType() != null && other.getTargetEncryptionType().equals(this.getTargetEncryptionType()) == false)
return false;
if (other.getDataTransferProgressPercent() == null ^ this.getDataTransferProgressPercent() == null)
return false;
if (other.getDataTransferProgressPercent() != null && other.getDataTransferProgressPercent().equals(this.getDataTransferProgressPercent()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getTargetNodeType() == null) ? 0 : getTargetNodeType().hashCode());
hashCode = prime * hashCode + ((getTargetNumberOfNodes() == null) ? 0 : getTargetNumberOfNodes().hashCode());
hashCode = prime * hashCode + ((getTargetClusterType() == null) ? 0 : getTargetClusterType().hashCode());
hashCode = prime * hashCode + ((getStatus() == null) ? 0 : getStatus().hashCode());
hashCode = prime * hashCode + ((getImportTablesCompleted() == null) ? 0 : getImportTablesCompleted().hashCode());
hashCode = prime * hashCode + ((getImportTablesInProgress() == null) ? 0 : getImportTablesInProgress().hashCode());
hashCode = prime * hashCode + ((getImportTablesNotStarted() == null) ? 0 : getImportTablesNotStarted().hashCode());
hashCode = prime * hashCode + ((getAvgResizeRateInMegaBytesPerSecond() == null) ? 0 : getAvgResizeRateInMegaBytesPerSecond().hashCode());
hashCode = prime * hashCode + ((getTotalResizeDataInMegaBytes() == null) ? 0 : getTotalResizeDataInMegaBytes().hashCode());
hashCode = prime * hashCode + ((getProgressInMegaBytes() == null) ? 0 : getProgressInMegaBytes().hashCode());
hashCode = prime * hashCode + ((getElapsedTimeInSeconds() == null) ? 0 : getElapsedTimeInSeconds().hashCode());
hashCode = prime * hashCode + ((getEstimatedTimeToCompletionInSeconds() == null) ? 0 : getEstimatedTimeToCompletionInSeconds().hashCode());
hashCode = prime * hashCode + ((getResizeType() == null) ? 0 : getResizeType().hashCode());
hashCode = prime * hashCode + ((getMessage() == null) ? 0 : getMessage().hashCode());
hashCode = prime * hashCode + ((getTargetEncryptionType() == null) ? 0 : getTargetEncryptionType().hashCode());
hashCode = prime * hashCode + ((getDataTransferProgressPercent() == null) ? 0 : getDataTransferProgressPercent().hashCode());
return hashCode;
}
@Override
public CancelResizeResult clone() {
try {
return (CancelResizeResult) super.clone();
} catch (CloneNotSupportedException e) {
throw new IllegalStateException("Got a CloneNotSupportedException from Object.clone() " + "even though we're Cloneable!", e);
}
}
}