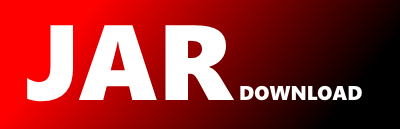
com.amazonaws.services.redshift.AmazonRedshift Maven / Gradle / Ivy
Show all versions of aws-java-sdk-redshift Show documentation
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.redshift;
import javax.annotation.Generated;
import com.amazonaws.*;
import com.amazonaws.regions.*;
import com.amazonaws.services.redshift.model.*;
import com.amazonaws.services.redshift.waiters.AmazonRedshiftWaiters;
/**
* Interface for accessing Amazon Redshift.
*
* Note: Do not directly implement this interface, new methods are added to it regularly. Extend from
* {@link com.amazonaws.services.redshift.AbstractAmazonRedshift} instead.
*
*
* Amazon Redshift
*
* Overview
*
*
* This is an interface reference for Amazon Redshift. It contains documentation for one of the programming or command
* line interfaces you can use to manage Amazon Redshift clusters. Note that Amazon Redshift is asynchronous, which
* means that some interfaces may require techniques, such as polling or asynchronous callback handlers, to determine
* when a command has been applied. In this reference, the parameter descriptions indicate whether a change is applied
* immediately, on the next instance reboot, or during the next maintenance window. For a summary of the Amazon Redshift
* cluster management interfaces, go to Using the Amazon Redshift Management
* Interfaces.
*
*
* Amazon Redshift manages all the work of setting up, operating, and scaling a data warehouse: provisioning capacity,
* monitoring and backing up the cluster, and applying patches and upgrades to the Amazon Redshift engine. You can focus
* on using your data to acquire new insights for your business and customers.
*
*
* If you are a first-time user of Amazon Redshift, we recommend that you begin by reading the Amazon Redshift Getting Started
* Guide.
*
*
* If you are a database developer, the Amazon
* Redshift Database Developer Guide explains how to design, build, query, and maintain the databases that make up
* your data warehouse.
*
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public interface AmazonRedshift {
/**
* The region metadata service name for computing region endpoints. You can use this value to retrieve metadata
* (such as supported regions) of the service.
*
* @see RegionUtils#getRegionsForService(String)
*/
String ENDPOINT_PREFIX = "redshift";
/**
* Overrides the default endpoint for this client ("redshift.us-east-1.amazonaws.com"). Callers can use this method
* to control which AWS region they want to work with.
*
* Callers can pass in just the endpoint (ex: "redshift.us-east-1.amazonaws.com") or a full URL, including the
* protocol (ex: "redshift.us-east-1.amazonaws.com"). If the protocol is not specified here, the default protocol
* from this client's {@link ClientConfiguration} will be used, which by default is HTTPS.
*
* For more information on using AWS regions with the AWS SDK for Java, and a complete list of all available
* endpoints for all AWS services, see: https://docs.aws.amazon.com/sdk-for-java/v1/developer-guide/java-dg-region-selection.html#region-selection-
* choose-endpoint
*
* This method is not threadsafe. An endpoint should be configured when the client is created and before any
* service requests are made. Changing it afterwards creates inevitable race conditions for any service requests in
* transit or retrying.
*
* @param endpoint
* The endpoint (ex: "redshift.us-east-1.amazonaws.com") or a full URL, including the protocol (ex:
* "redshift.us-east-1.amazonaws.com") of the region specific AWS endpoint this client will communicate with.
* @deprecated use {@link AwsClientBuilder#setEndpointConfiguration(AwsClientBuilder.EndpointConfiguration)} for
* example:
* {@code builder.setEndpointConfiguration(new EndpointConfiguration(endpoint, signingRegion));}
*/
@Deprecated
void setEndpoint(String endpoint);
/**
* An alternative to {@link AmazonRedshift#setEndpoint(String)}, sets the regional endpoint for this client's
* service calls. Callers can use this method to control which AWS region they want to work with.
*
* By default, all service endpoints in all regions use the https protocol. To use http instead, specify it in the
* {@link ClientConfiguration} supplied at construction.
*
* This method is not threadsafe. A region should be configured when the client is created and before any service
* requests are made. Changing it afterwards creates inevitable race conditions for any service requests in transit
* or retrying.
*
* @param region
* The region this client will communicate with. See {@link Region#getRegion(com.amazonaws.regions.Regions)}
* for accessing a given region. Must not be null and must be a region where the service is available.
*
* @see Region#getRegion(com.amazonaws.regions.Regions)
* @see Region#createClient(Class, com.amazonaws.auth.AWSCredentialsProvider, ClientConfiguration)
* @see Region#isServiceSupported(String)
* @deprecated use {@link AwsClientBuilder#setRegion(String)}
*/
@Deprecated
void setRegion(Region region);
/**
*
* Exchanges a DC1 Reserved Node for a DC2 Reserved Node with no changes to the configuration (term, payment type,
* or number of nodes) and no additional costs.
*
*
* @param acceptReservedNodeExchangeRequest
* @return Result of the AcceptReservedNodeExchange operation returned by the service.
* @throws ReservedNodeNotFoundException
* The specified reserved compute node not found.
* @throws InvalidReservedNodeStateException
* Indicates that the Reserved Node being exchanged is not in an active state.
* @throws ReservedNodeAlreadyMigratedException
* Indicates that the reserved node has already been exchanged.
* @throws ReservedNodeOfferingNotFoundException
* Specified offering does not exist.
* @throws UnsupportedOperationException
* The requested operation isn't supported.
* @throws DependentServiceUnavailableException
* Your request cannot be completed because a dependent internal service is temporarily unavailable. Wait 30
* to 60 seconds and try again.
* @throws ReservedNodeAlreadyExistsException
* User already has a reservation with the given identifier.
* @sample AmazonRedshift.AcceptReservedNodeExchange
* @see AWS API Documentation
*/
ReservedNode acceptReservedNodeExchange(AcceptReservedNodeExchangeRequest acceptReservedNodeExchangeRequest);
/**
*
* Adds a partner integration to a cluster. This operation authorizes a partner to push status updates for the
* specified database. To complete the integration, you also set up the integration on the partner website.
*
*
* @param addPartnerRequest
* @return Result of the AddPartner operation returned by the service.
* @throws PartnerNotFoundException
* The name of the partner was not found.
* @throws ClusterNotFoundException
* The ClusterIdentifier
parameter does not refer to an existing cluster.
* @throws UnauthorizedPartnerIntegrationException
* The partner integration is not authorized.
* @throws UnsupportedOperationException
* The requested operation isn't supported.
* @sample AmazonRedshift.AddPartner
* @see AWS API
* Documentation
*/
AddPartnerResult addPartner(AddPartnerRequest addPartnerRequest);
/**
*
* From a datashare consumer account, associates a datashare with the account (AssociateEntireAccount) or the
* specified namespace (ConsumerArn). If you make this association, the consumer can consume the datashare.
*
*
* @param associateDataShareConsumerRequest
* @return Result of the AssociateDataShareConsumer operation returned by the service.
* @throws InvalidDataShareException
* There is an error with the datashare.
* @throws InvalidNamespaceException
* The namespace isn't valid because the namespace doesn't exist. Provide a valid namespace.
* @sample AmazonRedshift.AssociateDataShareConsumer
* @see AWS API Documentation
*/
AssociateDataShareConsumerResult associateDataShareConsumer(AssociateDataShareConsumerRequest associateDataShareConsumerRequest);
/**
*
* Adds an inbound (ingress) rule to an Amazon Redshift security group. Depending on whether the application
* accessing your cluster is running on the Internet or an Amazon EC2 instance, you can authorize inbound access to
* either a Classless Interdomain Routing (CIDR)/Internet Protocol (IP) range or to an Amazon EC2 security group.
* You can add as many as 20 ingress rules to an Amazon Redshift security group.
*
*
* If you authorize access to an Amazon EC2 security group, specify EC2SecurityGroupName and
* EC2SecurityGroupOwnerId. The Amazon EC2 security group and Amazon Redshift cluster must be in the same
* Amazon Web Services Region.
*
*
* If you authorize access to a CIDR/IP address range, specify CIDRIP. For an overview of CIDR blocks, see
* the Wikipedia article on Classless
* Inter-Domain Routing.
*
*
* You must also associate the security group with a cluster so that clients running on these IP addresses or the
* EC2 instance are authorized to connect to the cluster. For information about managing security groups, go to Working with Security
* Groups in the Amazon Redshift Cluster Management Guide.
*
*
* @param authorizeClusterSecurityGroupIngressRequest
* @return Result of the AuthorizeClusterSecurityGroupIngress operation returned by the service.
* @throws ClusterSecurityGroupNotFoundException
* The cluster security group name does not refer to an existing cluster security group.
* @throws InvalidClusterSecurityGroupStateException
* The state of the cluster security group is not available
.
* @throws AuthorizationAlreadyExistsException
* The specified CIDR block or EC2 security group is already authorized for the specified cluster security
* group.
* @throws AuthorizationQuotaExceededException
* The authorization quota for the cluster security group has been reached.
* @sample AmazonRedshift.AuthorizeClusterSecurityGroupIngress
* @see AWS API Documentation
*/
ClusterSecurityGroup authorizeClusterSecurityGroupIngress(AuthorizeClusterSecurityGroupIngressRequest authorizeClusterSecurityGroupIngressRequest);
/**
*
* From a data producer account, authorizes the sharing of a datashare with one or more consumer accounts or
* managing entities. To authorize a datashare for a data consumer, the producer account must have the correct
* access permissions.
*
*
* @param authorizeDataShareRequest
* @return Result of the AuthorizeDataShare operation returned by the service.
* @throws InvalidDataShareException
* There is an error with the datashare.
* @sample AmazonRedshift.AuthorizeDataShare
* @see AWS
* API Documentation
*/
AuthorizeDataShareResult authorizeDataShare(AuthorizeDataShareRequest authorizeDataShareRequest);
/**
*
* Grants access to a cluster.
*
*
* @param authorizeEndpointAccessRequest
* @return Result of the AuthorizeEndpointAccess operation returned by the service.
* @throws ClusterNotFoundException
* The ClusterIdentifier
parameter does not refer to an existing cluster.
* @throws EndpointAuthorizationsPerClusterLimitExceededException
* The number of endpoint authorizations per cluster has exceeded its limit.
* @throws UnsupportedOperationException
* The requested operation isn't supported.
* @throws EndpointAuthorizationAlreadyExistsException
* The authorization already exists for this endpoint.
* @throws InvalidAuthorizationStateException
* The status of the authorization is not valid.
* @throws InvalidClusterStateException
* The specified cluster is not in the available
state.
* @sample AmazonRedshift.AuthorizeEndpointAccess
* @see AWS API Documentation
*/
AuthorizeEndpointAccessResult authorizeEndpointAccess(AuthorizeEndpointAccessRequest authorizeEndpointAccessRequest);
/**
*
* Authorizes the specified Amazon Web Services account to restore the specified snapshot.
*
*
* For more information about working with snapshots, go to Amazon Redshift Snapshots
* in the Amazon Redshift Cluster Management Guide.
*
*
* @param authorizeSnapshotAccessRequest
* @return Result of the AuthorizeSnapshotAccess operation returned by the service.
* @throws ClusterSnapshotNotFoundException
* The snapshot identifier does not refer to an existing cluster snapshot.
* @throws AuthorizationAlreadyExistsException
* The specified CIDR block or EC2 security group is already authorized for the specified cluster security
* group.
* @throws AuthorizationQuotaExceededException
* The authorization quota for the cluster security group has been reached.
* @throws DependentServiceRequestThrottlingException
* The request cannot be completed because a dependent service is throttling requests made by Amazon
* Redshift on your behalf. Wait and retry the request.
* @throws InvalidClusterSnapshotStateException
* The specified cluster snapshot is not in the available
state, or other accounts are
* authorized to access the snapshot.
* @throws LimitExceededException
* The encryption key has exceeded its grant limit in Amazon Web Services KMS.
* @throws UnsupportedOperationException
* The requested operation isn't supported.
* @sample AmazonRedshift.AuthorizeSnapshotAccess
* @see AWS API Documentation
*/
Snapshot authorizeSnapshotAccess(AuthorizeSnapshotAccessRequest authorizeSnapshotAccessRequest);
/**
*
* Deletes a set of cluster snapshots.
*
*
* @param batchDeleteClusterSnapshotsRequest
* @return Result of the BatchDeleteClusterSnapshots operation returned by the service.
* @throws BatchDeleteRequestSizeExceededException
* The maximum number for a batch delete of snapshots has been reached. The limit is 100.
* @sample AmazonRedshift.BatchDeleteClusterSnapshots
* @see AWS API Documentation
*/
BatchDeleteClusterSnapshotsResult batchDeleteClusterSnapshots(BatchDeleteClusterSnapshotsRequest batchDeleteClusterSnapshotsRequest);
/**
*
* Modifies the settings for a set of cluster snapshots.
*
*
* @param batchModifyClusterSnapshotsRequest
* @return Result of the BatchModifyClusterSnapshots operation returned by the service.
* @throws InvalidRetentionPeriodException
* The retention period specified is either in the past or is not a valid value.
*
* The value must be either -1 or an integer between 1 and 3,653.
* @throws BatchModifyClusterSnapshotsLimitExceededException
* The maximum number for snapshot identifiers has been reached. The limit is 100.
* @sample AmazonRedshift.BatchModifyClusterSnapshots
* @see AWS API Documentation
*/
BatchModifyClusterSnapshotsResult batchModifyClusterSnapshots(BatchModifyClusterSnapshotsRequest batchModifyClusterSnapshotsRequest);
/**
*
* Cancels a resize operation for a cluster.
*
*
* @param cancelResizeRequest
* @return Result of the CancelResize operation returned by the service.
* @throws ClusterNotFoundException
* The ClusterIdentifier
parameter does not refer to an existing cluster.
* @throws ResizeNotFoundException
* A resize operation for the specified cluster is not found.
* @throws InvalidClusterStateException
* The specified cluster is not in the available
state.
* @throws UnsupportedOperationException
* The requested operation isn't supported.
* @sample AmazonRedshift.CancelResize
* @see AWS API
* Documentation
*/
CancelResizeResult cancelResize(CancelResizeRequest cancelResizeRequest);
/**
*
* Copies the specified automated cluster snapshot to a new manual cluster snapshot. The source must be an automated
* snapshot and it must be in the available state.
*
*
* When you delete a cluster, Amazon Redshift deletes any automated snapshots of the cluster. Also, when the
* retention period of the snapshot expires, Amazon Redshift automatically deletes it. If you want to keep an
* automated snapshot for a longer period, you can make a manual copy of the snapshot. Manual snapshots are retained
* until you delete them.
*
*
* For more information about working with snapshots, go to Amazon Redshift Snapshots
* in the Amazon Redshift Cluster Management Guide.
*
*
* @param copyClusterSnapshotRequest
* @return Result of the CopyClusterSnapshot operation returned by the service.
* @throws ClusterNotFoundException
* The ClusterIdentifier
parameter does not refer to an existing cluster.
* @throws ClusterSnapshotAlreadyExistsException
* The value specified as a snapshot identifier is already used by an existing snapshot.
* @throws ClusterSnapshotNotFoundException
* The snapshot identifier does not refer to an existing cluster snapshot.
* @throws InvalidClusterSnapshotStateException
* The specified cluster snapshot is not in the available
state, or other accounts are
* authorized to access the snapshot.
* @throws ClusterSnapshotQuotaExceededException
* The request would result in the user exceeding the allowed number of cluster snapshots.
* @throws InvalidRetentionPeriodException
* The retention period specified is either in the past or is not a valid value.
*
* The value must be either -1 or an integer between 1 and 3,653.
* @sample AmazonRedshift.CopyClusterSnapshot
* @see AWS
* API Documentation
*/
Snapshot copyClusterSnapshot(CopyClusterSnapshotRequest copyClusterSnapshotRequest);
/**
*
* Creates an authentication profile with the specified parameters.
*
*
* @param createAuthenticationProfileRequest
* @return Result of the CreateAuthenticationProfile operation returned by the service.
* @throws AuthenticationProfileAlreadyExistsException
* The authentication profile already exists.
* @throws AuthenticationProfileQuotaExceededException
* The size or number of authentication profiles has exceeded the quota. The maximum length of the JSON
* string and maximum number of authentication profiles is determined by a quota for your account.
* @throws InvalidAuthenticationProfileRequestException
* The authentication profile request is not valid. The profile name can't be null or empty. The
* authentication profile API operation must be available in the Amazon Web Services Region.
* @sample AmazonRedshift.CreateAuthenticationProfile
* @see AWS API Documentation
*/
CreateAuthenticationProfileResult createAuthenticationProfile(CreateAuthenticationProfileRequest createAuthenticationProfileRequest);
/**
*
* Creates a new cluster with the specified parameters.
*
*
* To create a cluster in Virtual Private Cloud (VPC), you must provide a cluster subnet group name. The cluster
* subnet group identifies the subnets of your VPC that Amazon Redshift uses when creating the cluster. For more
* information about managing clusters, go to Amazon Redshift Clusters
* in the Amazon Redshift Cluster Management Guide.
*
*
* @param createClusterRequest
* @return Result of the CreateCluster operation returned by the service.
* @throws ClusterAlreadyExistsException
* The account already has a cluster with the given identifier.
* @throws InsufficientClusterCapacityException
* The number of nodes specified exceeds the allotted capacity of the cluster.
* @throws ClusterParameterGroupNotFoundException
* The parameter group name does not refer to an existing parameter group.
* @throws ClusterSecurityGroupNotFoundException
* The cluster security group name does not refer to an existing cluster security group.
* @throws ClusterQuotaExceededException
* The request would exceed the allowed number of cluster instances for this account. For information about
* increasing your quota, go to Limits in Amazon
* Redshift in the Amazon Redshift Cluster Management Guide.
* @throws NumberOfNodesQuotaExceededException
* The operation would exceed the number of nodes allotted to the account. For information about increasing
* your quota, go to Limits in Amazon
* Redshift in the Amazon Redshift Cluster Management Guide.
* @throws NumberOfNodesPerClusterLimitExceededException
* The operation would exceed the number of nodes allowed for a cluster.
* @throws ClusterSubnetGroupNotFoundException
* The cluster subnet group name does not refer to an existing cluster subnet group.
* @throws InvalidVPCNetworkStateException
* The cluster subnet group does not cover all Availability Zones.
* @throws InvalidClusterSubnetGroupStateException
* The cluster subnet group cannot be deleted because it is in use.
* @throws InvalidSubnetException
* The requested subnet is not valid, or not all of the subnets are in the same VPC.
* @throws UnauthorizedOperationException
* Your account is not authorized to perform the requested operation.
* @throws HsmClientCertificateNotFoundException
* There is no Amazon Redshift HSM client certificate with the specified identifier.
* @throws HsmConfigurationNotFoundException
* There is no Amazon Redshift HSM configuration with the specified identifier.
* @throws InvalidElasticIpException
* The Elastic IP (EIP) is invalid or cannot be found.
* @throws TagLimitExceededException
* You have exceeded the number of tags allowed.
* @throws InvalidTagException
* The tag is invalid.
* @throws LimitExceededException
* The encryption key has exceeded its grant limit in Amazon Web Services KMS.
* @throws DependentServiceRequestThrottlingException
* The request cannot be completed because a dependent service is throttling requests made by Amazon
* Redshift on your behalf. Wait and retry the request.
* @throws InvalidClusterTrackException
* The provided cluster track name is not valid.
* @throws SnapshotScheduleNotFoundException
* We could not find the specified snapshot schedule.
* @throws InvalidRetentionPeriodException
* The retention period specified is either in the past or is not a valid value.
*
* The value must be either -1 or an integer between 1 and 3,653.
* @throws Ipv6CidrBlockNotFoundException
* There are no subnets in your VPC with associated IPv6 CIDR blocks. To use dual-stack mode, associate an
* IPv6 CIDR block with each subnet in your VPC.
* @throws UnsupportedOperationException
* The requested operation isn't supported.
* @throws RedshiftIdcApplicationNotExistsException
* The application you attempted to find doesn't exist.
* @sample AmazonRedshift.CreateCluster
* @see AWS API
* Documentation
*/
Cluster createCluster(CreateClusterRequest createClusterRequest);
/**
*
* Creates an Amazon Redshift parameter group.
*
*
* Creating parameter groups is independent of creating clusters. You can associate a cluster with a parameter group
* when you create the cluster. You can also associate an existing cluster with a parameter group after the cluster
* is created by using ModifyCluster.
*
*
* Parameters in the parameter group define specific behavior that applies to the databases you create on the
* cluster. For more information about parameters and parameter groups, go to Amazon Redshift
* Parameter Groups in the Amazon Redshift Cluster Management Guide.
*
*
* @param createClusterParameterGroupRequest
* @return Result of the CreateClusterParameterGroup operation returned by the service.
* @throws ClusterParameterGroupQuotaExceededException
* The request would result in the user exceeding the allowed number of cluster parameter groups. For
* information about increasing your quota, go to Limits in Amazon
* Redshift in the Amazon Redshift Cluster Management Guide.
* @throws ClusterParameterGroupAlreadyExistsException
* A cluster parameter group with the same name already exists.
* @throws TagLimitExceededException
* You have exceeded the number of tags allowed.
* @throws InvalidTagException
* The tag is invalid.
* @sample AmazonRedshift.CreateClusterParameterGroup
* @see AWS API Documentation
*/
ClusterParameterGroup createClusterParameterGroup(CreateClusterParameterGroupRequest createClusterParameterGroupRequest);
/**
*
* Creates a new Amazon Redshift security group. You use security groups to control access to non-VPC clusters.
*
*
* For information about managing security groups, go to Amazon Redshift Cluster
* Security Groups in the Amazon Redshift Cluster Management Guide.
*
*
* @param createClusterSecurityGroupRequest
* @return Result of the CreateClusterSecurityGroup operation returned by the service.
* @throws ClusterSecurityGroupAlreadyExistsException
* A cluster security group with the same name already exists.
* @throws ClusterSecurityGroupQuotaExceededException
* The request would result in the user exceeding the allowed number of cluster security groups. For
* information about increasing your quota, go to Limits in Amazon
* Redshift in the Amazon Redshift Cluster Management Guide.
* @throws TagLimitExceededException
* You have exceeded the number of tags allowed.
* @throws InvalidTagException
* The tag is invalid.
* @sample AmazonRedshift.CreateClusterSecurityGroup
* @see AWS API Documentation
*/
ClusterSecurityGroup createClusterSecurityGroup(CreateClusterSecurityGroupRequest createClusterSecurityGroupRequest);
/**
*
* Creates a manual snapshot of the specified cluster. The cluster must be in the available
state.
*
*
* For more information about working with snapshots, go to Amazon Redshift Snapshots
* in the Amazon Redshift Cluster Management Guide.
*
*
* @param createClusterSnapshotRequest
* @return Result of the CreateClusterSnapshot operation returned by the service.
* @throws ClusterSnapshotAlreadyExistsException
* The value specified as a snapshot identifier is already used by an existing snapshot.
* @throws InvalidClusterStateException
* The specified cluster is not in the available
state.
* @throws ClusterNotFoundException
* The ClusterIdentifier
parameter does not refer to an existing cluster.
* @throws ClusterSnapshotQuotaExceededException
* The request would result in the user exceeding the allowed number of cluster snapshots.
* @throws TagLimitExceededException
* You have exceeded the number of tags allowed.
* @throws InvalidTagException
* The tag is invalid.
* @throws InvalidRetentionPeriodException
* The retention period specified is either in the past or is not a valid value.
*
* The value must be either -1 or an integer between 1 and 3,653.
* @sample AmazonRedshift.CreateClusterSnapshot
* @see AWS
* API Documentation
*/
Snapshot createClusterSnapshot(CreateClusterSnapshotRequest createClusterSnapshotRequest);
/**
*
* Creates a new Amazon Redshift subnet group. You must provide a list of one or more subnets in your existing
* Amazon Virtual Private Cloud (Amazon VPC) when creating Amazon Redshift subnet group.
*
*
* For information about subnet groups, go to Amazon Redshift
* Cluster Subnet Groups in the Amazon Redshift Cluster Management Guide.
*
*
* @param createClusterSubnetGroupRequest
* @return Result of the CreateClusterSubnetGroup operation returned by the service.
* @throws ClusterSubnetGroupAlreadyExistsException
* A ClusterSubnetGroupName is already used by an existing cluster subnet group.
* @throws ClusterSubnetGroupQuotaExceededException
* The request would result in user exceeding the allowed number of cluster subnet groups. For information
* about increasing your quota, go to Limits in Amazon
* Redshift in the Amazon Redshift Cluster Management Guide.
* @throws ClusterSubnetQuotaExceededException
* The request would result in user exceeding the allowed number of subnets in a cluster subnet groups. For
* information about increasing your quota, go to Limits in Amazon
* Redshift in the Amazon Redshift Cluster Management Guide.
* @throws InvalidSubnetException
* The requested subnet is not valid, or not all of the subnets are in the same VPC.
* @throws UnauthorizedOperationException
* Your account is not authorized to perform the requested operation.
* @throws TagLimitExceededException
* You have exceeded the number of tags allowed.
* @throws InvalidTagException
* The tag is invalid.
* @throws DependentServiceRequestThrottlingException
* The request cannot be completed because a dependent service is throttling requests made by Amazon
* Redshift on your behalf. Wait and retry the request.
* @sample AmazonRedshift.CreateClusterSubnetGroup
* @see AWS API Documentation
*/
ClusterSubnetGroup createClusterSubnetGroup(CreateClusterSubnetGroupRequest createClusterSubnetGroupRequest);
/**
*
* Used to create a custom domain name for a cluster. Properties include the custom domain name, the cluster the
* custom domain is associated with, and the certificate Amazon Resource Name (ARN).
*
*
* @param createCustomDomainAssociationRequest
* @return Result of the CreateCustomDomainAssociation operation returned by the service.
* @throws UnsupportedOperationException
* The requested operation isn't supported.
* @throws ClusterNotFoundException
* The ClusterIdentifier
parameter does not refer to an existing cluster.
* @throws CustomCnameAssociationException
* An error occurred when an attempt was made to change the custom domain association.
* @sample AmazonRedshift.CreateCustomDomainAssociation
* @see AWS API Documentation
*/
CreateCustomDomainAssociationResult createCustomDomainAssociation(CreateCustomDomainAssociationRequest createCustomDomainAssociationRequest);
/**
*
* Creates a Redshift-managed VPC endpoint.
*
*
* @param createEndpointAccessRequest
* @return Result of the CreateEndpointAccess operation returned by the service.
* @throws ClusterNotFoundException
* The ClusterIdentifier
parameter does not refer to an existing cluster.
* @throws AccessToClusterDeniedException
* You are not authorized to access the cluster.
* @throws EndpointsPerClusterLimitExceededException
* The number of Redshift-managed VPC endpoints per cluster has exceeded its limit.
* @throws EndpointsPerAuthorizationLimitExceededException
* The number of Redshift-managed VPC endpoints per authorization has exceeded its limit.
* @throws InvalidClusterSecurityGroupStateException
* The state of the cluster security group is not available
.
* @throws ClusterSubnetGroupNotFoundException
* The cluster subnet group name does not refer to an existing cluster subnet group.
* @throws EndpointAlreadyExistsException
* The account already has a Redshift-managed VPC endpoint with the given identifier.
* @throws UnsupportedOperationException
* The requested operation isn't supported.
* @throws InvalidClusterStateException
* The specified cluster is not in the available
state.
* @throws UnauthorizedOperationException
* Your account is not authorized to perform the requested operation.
* @sample AmazonRedshift.CreateEndpointAccess
* @see AWS
* API Documentation
*/
CreateEndpointAccessResult createEndpointAccess(CreateEndpointAccessRequest createEndpointAccessRequest);
/**
*
* Creates an Amazon Redshift event notification subscription. This action requires an ARN (Amazon Resource Name) of
* an Amazon SNS topic created by either the Amazon Redshift console, the Amazon SNS console, or the Amazon SNS API.
* To obtain an ARN with Amazon SNS, you must create a topic in Amazon SNS and subscribe to the topic. The ARN is
* displayed in the SNS console.
*
*
* You can specify the source type, and lists of Amazon Redshift source IDs, event categories, and event severities.
* Notifications will be sent for all events you want that match those criteria. For example, you can specify source
* type = cluster, source ID = my-cluster-1 and mycluster2, event categories = Availability, Backup, and severity =
* ERROR. The subscription will only send notifications for those ERROR events in the Availability and Backup
* categories for the specified clusters.
*
*
* If you specify both the source type and source IDs, such as source type = cluster and source identifier =
* my-cluster-1, notifications will be sent for all the cluster events for my-cluster-1. If you specify a source
* type but do not specify a source identifier, you will receive notice of the events for the objects of that type
* in your Amazon Web Services account. If you do not specify either the SourceType nor the SourceIdentifier, you
* will be notified of events generated from all Amazon Redshift sources belonging to your Amazon Web Services
* account. You must specify a source type if you specify a source ID.
*
*
* @param createEventSubscriptionRequest
* @return Result of the CreateEventSubscription operation returned by the service.
* @throws EventSubscriptionQuotaExceededException
* The request would exceed the allowed number of event subscriptions for this account. For information
* about increasing your quota, go to Limits in Amazon
* Redshift in the Amazon Redshift Cluster Management Guide.
* @throws SubscriptionAlreadyExistException
* There is already an existing event notification subscription with the specified name.
* @throws SNSInvalidTopicException
* Amazon SNS has responded that there is a problem with the specified Amazon SNS topic.
* @throws SNSNoAuthorizationException
* You do not have permission to publish to the specified Amazon SNS topic.
* @throws SNSTopicArnNotFoundException
* An Amazon SNS topic with the specified Amazon Resource Name (ARN) does not exist.
* @throws SubscriptionEventIdNotFoundException
* An Amazon Redshift event with the specified event ID does not exist.
* @throws SubscriptionCategoryNotFoundException
* The value specified for the event category was not one of the allowed values, or it specified a category
* that does not apply to the specified source type. The allowed values are Configuration, Management,
* Monitoring, and Security.
* @throws SubscriptionSeverityNotFoundException
* The value specified for the event severity was not one of the allowed values, or it specified a severity
* that does not apply to the specified source type. The allowed values are ERROR and INFO.
* @throws SourceNotFoundException
* The specified Amazon Redshift event source could not be found.
* @throws TagLimitExceededException
* You have exceeded the number of tags allowed.
* @throws InvalidTagException
* The tag is invalid.
* @sample AmazonRedshift.CreateEventSubscription
* @see AWS API Documentation
*/
EventSubscription createEventSubscription(CreateEventSubscriptionRequest createEventSubscriptionRequest);
/**
*
* Creates an HSM client certificate that an Amazon Redshift cluster will use to connect to the client's HSM in
* order to store and retrieve the keys used to encrypt the cluster databases.
*
*
* The command returns a public key, which you must store in the HSM. In addition to creating the HSM certificate,
* you must create an Amazon Redshift HSM configuration that provides a cluster the information needed to store and
* use encryption keys in the HSM. For more information, go to Hardware
* Security Modules in the Amazon Redshift Cluster Management Guide.
*
*
* @param createHsmClientCertificateRequest
* @return Result of the CreateHsmClientCertificate operation returned by the service.
* @throws HsmClientCertificateAlreadyExistsException
* There is already an existing Amazon Redshift HSM client certificate with the specified identifier.
* @throws HsmClientCertificateQuotaExceededException
* The quota for HSM client certificates has been reached. For information about increasing your quota, go
* to Limits in
* Amazon Redshift in the Amazon Redshift Cluster Management Guide.
* @throws TagLimitExceededException
* You have exceeded the number of tags allowed.
* @throws InvalidTagException
* The tag is invalid.
* @sample AmazonRedshift.CreateHsmClientCertificate
* @see AWS API Documentation
*/
HsmClientCertificate createHsmClientCertificate(CreateHsmClientCertificateRequest createHsmClientCertificateRequest);
/**
*
* Creates an HSM configuration that contains the information required by an Amazon Redshift cluster to store and
* use database encryption keys in a Hardware Security Module (HSM). After creating the HSM configuration, you can
* specify it as a parameter when creating a cluster. The cluster will then store its encryption keys in the HSM.
*
*
* In addition to creating an HSM configuration, you must also create an HSM client certificate. For more
* information, go to Hardware
* Security Modules in the Amazon Redshift Cluster Management Guide.
*
*
* @param createHsmConfigurationRequest
* @return Result of the CreateHsmConfiguration operation returned by the service.
* @throws HsmConfigurationAlreadyExistsException
* There is already an existing Amazon Redshift HSM configuration with the specified identifier.
* @throws HsmConfigurationQuotaExceededException
* The quota for HSM configurations has been reached. For information about increasing your quota, go to Limits in Amazon
* Redshift in the Amazon Redshift Cluster Management Guide.
* @throws TagLimitExceededException
* You have exceeded the number of tags allowed.
* @throws InvalidTagException
* The tag is invalid.
* @sample AmazonRedshift.CreateHsmConfiguration
* @see AWS API Documentation
*/
HsmConfiguration createHsmConfiguration(CreateHsmConfigurationRequest createHsmConfigurationRequest);
/**
*
* Creates an Amazon Redshift application for use with IAM Identity Center.
*
*
* @param createRedshiftIdcApplicationRequest
* @return Result of the CreateRedshiftIdcApplication operation returned by the service.
* @throws RedshiftIdcApplicationAlreadyExistsException
* The application you attempted to add already exists.
* @throws DependentServiceUnavailableException
* Your request cannot be completed because a dependent internal service is temporarily unavailable. Wait 30
* to 60 seconds and try again.
* @throws UnsupportedOperationException
* The requested operation isn't supported.
* @throws DependentServiceAccessDeniedException
* A dependent service denied access for the integration.
* @throws RedshiftIdcApplicationQuotaExceededException
* The maximum number of Redshift IAM Identity Center applications was exceeded.
* @sample AmazonRedshift.CreateRedshiftIdcApplication
* @see AWS API Documentation
*/
RedshiftIdcApplication createRedshiftIdcApplication(CreateRedshiftIdcApplicationRequest createRedshiftIdcApplicationRequest);
/**
*
* Creates a scheduled action. A scheduled action contains a schedule and an Amazon Redshift API action. For
* example, you can create a schedule of when to run the ResizeCluster
API operation.
*
*
* @param createScheduledActionRequest
* @return Result of the CreateScheduledAction operation returned by the service.
* @throws ClusterNotFoundException
* The ClusterIdentifier
parameter does not refer to an existing cluster.
* @throws ScheduledActionAlreadyExistsException
* The scheduled action already exists.
* @throws ScheduledActionQuotaExceededException
* The quota for scheduled actions exceeded.
* @throws ScheduledActionTypeUnsupportedException
* The action type specified for a scheduled action is not supported.
* @throws InvalidScheduleException
* The schedule you submitted isn't valid.
* @throws InvalidScheduledActionException
* The scheduled action is not valid.
* @throws UnauthorizedOperationException
* Your account is not authorized to perform the requested operation.
* @throws UnsupportedOperationException
* The requested operation isn't supported.
* @sample AmazonRedshift.CreateScheduledAction
* @see AWS
* API Documentation
*/
CreateScheduledActionResult createScheduledAction(CreateScheduledActionRequest createScheduledActionRequest);
/**
*
* Creates a snapshot copy grant that permits Amazon Redshift to use an encrypted symmetric key from Key Management
* Service (KMS) to encrypt copied snapshots in a destination region.
*
*
* For more information about managing snapshot copy grants, go to Amazon Redshift Database
* Encryption in the Amazon Redshift Cluster Management Guide.
*
*
* @param createSnapshotCopyGrantRequest
* The result of the CreateSnapshotCopyGrant
action.
* @return Result of the CreateSnapshotCopyGrant operation returned by the service.
* @throws SnapshotCopyGrantAlreadyExistsException
* The snapshot copy grant can't be created because a grant with the same name already exists.
* @throws SnapshotCopyGrantQuotaExceededException
* The Amazon Web Services account has exceeded the maximum number of snapshot copy grants in this region.
* @throws LimitExceededException
* The encryption key has exceeded its grant limit in Amazon Web Services KMS.
* @throws TagLimitExceededException
* You have exceeded the number of tags allowed.
* @throws InvalidTagException
* The tag is invalid.
* @throws DependentServiceRequestThrottlingException
* The request cannot be completed because a dependent service is throttling requests made by Amazon
* Redshift on your behalf. Wait and retry the request.
* @sample AmazonRedshift.CreateSnapshotCopyGrant
* @see AWS API Documentation
*/
SnapshotCopyGrant createSnapshotCopyGrant(CreateSnapshotCopyGrantRequest createSnapshotCopyGrantRequest);
/**
*
* Create a snapshot schedule that can be associated to a cluster and which overrides the default system backup
* schedule.
*
*
* @param createSnapshotScheduleRequest
* @return Result of the CreateSnapshotSchedule operation returned by the service.
* @throws SnapshotScheduleAlreadyExistsException
* The specified snapshot schedule already exists.
* @throws InvalidScheduleException
* The schedule you submitted isn't valid.
* @throws SnapshotScheduleQuotaExceededException
* You have exceeded the quota of snapshot schedules.
* @throws TagLimitExceededException
* You have exceeded the number of tags allowed.
* @throws ScheduleDefinitionTypeUnsupportedException
* The definition you submitted is not supported.
* @throws InvalidTagException
* The tag is invalid.
* @sample AmazonRedshift.CreateSnapshotSchedule
* @see AWS API Documentation
*/
CreateSnapshotScheduleResult createSnapshotSchedule(CreateSnapshotScheduleRequest createSnapshotScheduleRequest);
/**
*
* Adds tags to a cluster.
*
*
* A resource can have up to 50 tags. If you try to create more than 50 tags for a resource, you will receive an
* error and the attempt will fail.
*
*
* If you specify a key that already exists for the resource, the value for that key will be updated with the new
* value.
*
*
* @param createTagsRequest
* Contains the output from the CreateTags
action.
* @return Result of the CreateTags operation returned by the service.
* @throws TagLimitExceededException
* You have exceeded the number of tags allowed.
* @throws ResourceNotFoundException
* The resource could not be found.
* @throws InvalidTagException
* The tag is invalid.
* @throws InvalidClusterStateException
* The specified cluster is not in the available
state.
* @sample AmazonRedshift.CreateTags
* @see AWS API
* Documentation
*/
CreateTagsResult createTags(CreateTagsRequest createTagsRequest);
/**
*
* Creates a usage limit for a specified Amazon Redshift feature on a cluster. The usage limit is identified by the
* returned usage limit identifier.
*
*
* @param createUsageLimitRequest
* @return Result of the CreateUsageLimit operation returned by the service.
* @throws ClusterNotFoundException
* The ClusterIdentifier
parameter does not refer to an existing cluster.
* @throws InvalidClusterStateException
* The specified cluster is not in the available
state.
* @throws LimitExceededException
* The encryption key has exceeded its grant limit in Amazon Web Services KMS.
* @throws UsageLimitAlreadyExistsException
* The usage limit already exists.
* @throws InvalidUsageLimitException
* The usage limit is not valid.
* @throws TagLimitExceededException
* You have exceeded the number of tags allowed.
* @throws UnsupportedOperationException
* The requested operation isn't supported.
* @sample AmazonRedshift.CreateUsageLimit
* @see AWS API
* Documentation
*/
CreateUsageLimitResult createUsageLimit(CreateUsageLimitRequest createUsageLimitRequest);
/**
*
* From a datashare producer account, removes authorization from the specified datashare.
*
*
* @param deauthorizeDataShareRequest
* @return Result of the DeauthorizeDataShare operation returned by the service.
* @throws InvalidDataShareException
* There is an error with the datashare.
* @sample AmazonRedshift.DeauthorizeDataShare
* @see AWS
* API Documentation
*/
DeauthorizeDataShareResult deauthorizeDataShare(DeauthorizeDataShareRequest deauthorizeDataShareRequest);
/**
*
* Deletes an authentication profile.
*
*
* @param deleteAuthenticationProfileRequest
* @return Result of the DeleteAuthenticationProfile operation returned by the service.
* @throws AuthenticationProfileNotFoundException
* The authentication profile can't be found.
* @throws InvalidAuthenticationProfileRequestException
* The authentication profile request is not valid. The profile name can't be null or empty. The
* authentication profile API operation must be available in the Amazon Web Services Region.
* @sample AmazonRedshift.DeleteAuthenticationProfile
* @see AWS API Documentation
*/
DeleteAuthenticationProfileResult deleteAuthenticationProfile(DeleteAuthenticationProfileRequest deleteAuthenticationProfileRequest);
/**
*
* Deletes a previously provisioned cluster without its final snapshot being created. A successful response from the
* web service indicates that the request was received correctly. Use DescribeClusters to monitor the status
* of the deletion. The delete operation cannot be canceled or reverted once submitted. For more information about
* managing clusters, go to Amazon Redshift Clusters
* in the Amazon Redshift Cluster Management Guide.
*
*
* If you want to shut down the cluster and retain it for future use, set SkipFinalClusterSnapshot to
* false
and specify a name for FinalClusterSnapshotIdentifier. You can later restore this
* snapshot to resume using the cluster. If a final cluster snapshot is requested, the status of the cluster will be
* "final-snapshot" while the snapshot is being taken, then it's "deleting" once Amazon Redshift begins deleting the
* cluster.
*
*
* For more information about managing clusters, go to Amazon Redshift Clusters
* in the Amazon Redshift Cluster Management Guide.
*
*
* @param deleteClusterRequest
* @return Result of the DeleteCluster operation returned by the service.
* @throws ClusterNotFoundException
* The ClusterIdentifier
parameter does not refer to an existing cluster.
* @throws InvalidClusterStateException
* The specified cluster is not in the available
state.
* @throws ClusterSnapshotAlreadyExistsException
* The value specified as a snapshot identifier is already used by an existing snapshot.
* @throws ClusterSnapshotQuotaExceededException
* The request would result in the user exceeding the allowed number of cluster snapshots.
* @throws InvalidRetentionPeriodException
* The retention period specified is either in the past or is not a valid value.
*
* The value must be either -1 or an integer between 1 and 3,653.
* @sample AmazonRedshift.DeleteCluster
* @see AWS API
* Documentation
*/
Cluster deleteCluster(DeleteClusterRequest deleteClusterRequest);
/**
*
* Deletes a specified Amazon Redshift parameter group.
*
*
*
* You cannot delete a parameter group if it is associated with a cluster.
*
*
*
* @param deleteClusterParameterGroupRequest
* @return Result of the DeleteClusterParameterGroup operation returned by the service.
* @throws InvalidClusterParameterGroupStateException
* The cluster parameter group action can not be completed because another task is in progress that involves
* the parameter group. Wait a few moments and try the operation again.
* @throws ClusterParameterGroupNotFoundException
* The parameter group name does not refer to an existing parameter group.
* @sample AmazonRedshift.DeleteClusterParameterGroup
* @see AWS API Documentation
*/
DeleteClusterParameterGroupResult deleteClusterParameterGroup(DeleteClusterParameterGroupRequest deleteClusterParameterGroupRequest);
/**
*
* Deletes an Amazon Redshift security group.
*
*
*
* You cannot delete a security group that is associated with any clusters. You cannot delete the default security
* group.
*
*
*
* For information about managing security groups, go to Amazon Redshift Cluster
* Security Groups in the Amazon Redshift Cluster Management Guide.
*
*
* @param deleteClusterSecurityGroupRequest
* @return Result of the DeleteClusterSecurityGroup operation returned by the service.
* @throws InvalidClusterSecurityGroupStateException
* The state of the cluster security group is not available
.
* @throws ClusterSecurityGroupNotFoundException
* The cluster security group name does not refer to an existing cluster security group.
* @sample AmazonRedshift.DeleteClusterSecurityGroup
* @see AWS API Documentation
*/
DeleteClusterSecurityGroupResult deleteClusterSecurityGroup(DeleteClusterSecurityGroupRequest deleteClusterSecurityGroupRequest);
/**
*
* Deletes the specified manual snapshot. The snapshot must be in the available
state, with no other
* users authorized to access the snapshot.
*
*
* Unlike automated snapshots, manual snapshots are retained even after you delete your cluster. Amazon Redshift
* does not delete your manual snapshots. You must delete manual snapshot explicitly to avoid getting charged. If
* other accounts are authorized to access the snapshot, you must revoke all of the authorizations before you can
* delete the snapshot.
*
*
* @param deleteClusterSnapshotRequest
* @return Result of the DeleteClusterSnapshot operation returned by the service.
* @throws InvalidClusterSnapshotStateException
* The specified cluster snapshot is not in the available
state, or other accounts are
* authorized to access the snapshot.
* @throws ClusterSnapshotNotFoundException
* The snapshot identifier does not refer to an existing cluster snapshot.
* @sample AmazonRedshift.DeleteClusterSnapshot
* @see AWS
* API Documentation
*/
Snapshot deleteClusterSnapshot(DeleteClusterSnapshotRequest deleteClusterSnapshotRequest);
/**
*
* Deletes the specified cluster subnet group.
*
*
* @param deleteClusterSubnetGroupRequest
* @return Result of the DeleteClusterSubnetGroup operation returned by the service.
* @throws InvalidClusterSubnetGroupStateException
* The cluster subnet group cannot be deleted because it is in use.
* @throws InvalidClusterSubnetStateException
* The state of the subnet is invalid.
* @throws ClusterSubnetGroupNotFoundException
* The cluster subnet group name does not refer to an existing cluster subnet group.
* @sample AmazonRedshift.DeleteClusterSubnetGroup
* @see AWS API Documentation
*/
DeleteClusterSubnetGroupResult deleteClusterSubnetGroup(DeleteClusterSubnetGroupRequest deleteClusterSubnetGroupRequest);
/**
*
* Contains information about deleting a custom domain association for a cluster.
*
*
* @param deleteCustomDomainAssociationRequest
* @return Result of the DeleteCustomDomainAssociation operation returned by the service.
* @throws UnsupportedOperationException
* The requested operation isn't supported.
* @throws ClusterNotFoundException
* The ClusterIdentifier
parameter does not refer to an existing cluster.
* @throws CustomCnameAssociationException
* An error occurred when an attempt was made to change the custom domain association.
* @throws CustomDomainAssociationNotFoundException
* An error occurred. The custom domain name couldn't be found.
* @sample AmazonRedshift.DeleteCustomDomainAssociation
* @see AWS API Documentation
*/
DeleteCustomDomainAssociationResult deleteCustomDomainAssociation(DeleteCustomDomainAssociationRequest deleteCustomDomainAssociationRequest);
/**
*
* Deletes a Redshift-managed VPC endpoint.
*
*
* @param deleteEndpointAccessRequest
* @return Result of the DeleteEndpointAccess operation returned by the service.
* @throws ClusterNotFoundException
* The ClusterIdentifier
parameter does not refer to an existing cluster.
* @throws InvalidEndpointStateException
* The status of the endpoint is not valid.
* @throws InvalidClusterSecurityGroupStateException
* The state of the cluster security group is not available
.
* @throws EndpointNotFoundException
* The endpoint name doesn't refer to an existing endpoint.
* @throws InvalidClusterStateException
* The specified cluster is not in the available
state.
* @sample AmazonRedshift.DeleteEndpointAccess
* @see AWS
* API Documentation
*/
DeleteEndpointAccessResult deleteEndpointAccess(DeleteEndpointAccessRequest deleteEndpointAccessRequest);
/**
*
* Deletes an Amazon Redshift event notification subscription.
*
*
* @param deleteEventSubscriptionRequest
* @return Result of the DeleteEventSubscription operation returned by the service.
* @throws SubscriptionNotFoundException
* An Amazon Redshift event notification subscription with the specified name does not exist.
* @throws InvalidSubscriptionStateException
* The subscription request is invalid because it is a duplicate request. This subscription request is
* already in progress.
* @sample AmazonRedshift.DeleteEventSubscription
* @see AWS API Documentation
*/
DeleteEventSubscriptionResult deleteEventSubscription(DeleteEventSubscriptionRequest deleteEventSubscriptionRequest);
/**
*
* Deletes the specified HSM client certificate.
*
*
* @param deleteHsmClientCertificateRequest
* @return Result of the DeleteHsmClientCertificate operation returned by the service.
* @throws InvalidHsmClientCertificateStateException
* The specified HSM client certificate is not in the available
state, or it is still in use by
* one or more Amazon Redshift clusters.
* @throws HsmClientCertificateNotFoundException
* There is no Amazon Redshift HSM client certificate with the specified identifier.
* @sample AmazonRedshift.DeleteHsmClientCertificate
* @see AWS API Documentation
*/
DeleteHsmClientCertificateResult deleteHsmClientCertificate(DeleteHsmClientCertificateRequest deleteHsmClientCertificateRequest);
/**
*
* Deletes the specified Amazon Redshift HSM configuration.
*
*
* @param deleteHsmConfigurationRequest
* @return Result of the DeleteHsmConfiguration operation returned by the service.
* @throws InvalidHsmConfigurationStateException
* The specified HSM configuration is not in the available
state, or it is still in use by one
* or more Amazon Redshift clusters.
* @throws HsmConfigurationNotFoundException
* There is no Amazon Redshift HSM configuration with the specified identifier.
* @sample AmazonRedshift.DeleteHsmConfiguration
* @see AWS API Documentation
*/
DeleteHsmConfigurationResult deleteHsmConfiguration(DeleteHsmConfigurationRequest deleteHsmConfigurationRequest);
/**
*
* Deletes a partner integration from a cluster. Data can still flow to the cluster until the integration is deleted
* at the partner's website.
*
*
* @param deletePartnerRequest
* @return Result of the DeletePartner operation returned by the service.
* @throws PartnerNotFoundException
* The name of the partner was not found.
* @throws ClusterNotFoundException
* The ClusterIdentifier
parameter does not refer to an existing cluster.
* @throws UnauthorizedPartnerIntegrationException
* The partner integration is not authorized.
* @throws UnsupportedOperationException
* The requested operation isn't supported.
* @sample AmazonRedshift.DeletePartner
* @see AWS API
* Documentation
*/
DeletePartnerResult deletePartner(DeletePartnerRequest deletePartnerRequest);
/**
*
* Deletes an Amazon Redshift IAM Identity Center application.
*
*
* @param deleteRedshiftIdcApplicationRequest
* @return Result of the DeleteRedshiftIdcApplication operation returned by the service.
* @throws RedshiftIdcApplicationNotExistsException
* The application you attempted to find doesn't exist.
* @throws DependentServiceUnavailableException
* Your request cannot be completed because a dependent internal service is temporarily unavailable. Wait 30
* to 60 seconds and try again.
* @throws UnsupportedOperationException
* The requested operation isn't supported.
* @throws DependentServiceAccessDeniedException
* A dependent service denied access for the integration.
* @sample AmazonRedshift.DeleteRedshiftIdcApplication
* @see AWS API Documentation
*/
DeleteRedshiftIdcApplicationResult deleteRedshiftIdcApplication(DeleteRedshiftIdcApplicationRequest deleteRedshiftIdcApplicationRequest);
/**
*
* Deletes the resource policy for a specified resource.
*
*
* @param deleteResourcePolicyRequest
* @return Result of the DeleteResourcePolicy operation returned by the service.
* @throws ResourceNotFoundException
* The resource could not be found.
* @throws UnsupportedOperationException
* The requested operation isn't supported.
* @sample AmazonRedshift.DeleteResourcePolicy
* @see AWS
* API Documentation
*/
DeleteResourcePolicyResult deleteResourcePolicy(DeleteResourcePolicyRequest deleteResourcePolicyRequest);
/**
*
* Deletes a scheduled action.
*
*
* @param deleteScheduledActionRequest
* @return Result of the DeleteScheduledAction operation returned by the service.
* @throws ScheduledActionNotFoundException
* The scheduled action cannot be found.
* @throws UnauthorizedOperationException
* Your account is not authorized to perform the requested operation.
* @sample AmazonRedshift.DeleteScheduledAction
* @see AWS
* API Documentation
*/
DeleteScheduledActionResult deleteScheduledAction(DeleteScheduledActionRequest deleteScheduledActionRequest);
/**
*
* Deletes the specified snapshot copy grant.
*
*
* @param deleteSnapshotCopyGrantRequest
* The result of the DeleteSnapshotCopyGrant
action.
* @return Result of the DeleteSnapshotCopyGrant operation returned by the service.
* @throws InvalidSnapshotCopyGrantStateException
* The snapshot copy grant can't be deleted because it is used by one or more clusters.
* @throws SnapshotCopyGrantNotFoundException
* The specified snapshot copy grant can't be found. Make sure that the name is typed correctly and that the
* grant exists in the destination region.
* @sample AmazonRedshift.DeleteSnapshotCopyGrant
* @see AWS API Documentation
*/
DeleteSnapshotCopyGrantResult deleteSnapshotCopyGrant(DeleteSnapshotCopyGrantRequest deleteSnapshotCopyGrantRequest);
/**
*
* Deletes a snapshot schedule.
*
*
* @param deleteSnapshotScheduleRequest
* @return Result of the DeleteSnapshotSchedule operation returned by the service.
* @throws InvalidClusterSnapshotScheduleStateException
* The cluster snapshot schedule state is not valid.
* @throws SnapshotScheduleNotFoundException
* We could not find the specified snapshot schedule.
* @sample AmazonRedshift.DeleteSnapshotSchedule
* @see AWS API Documentation
*/
DeleteSnapshotScheduleResult deleteSnapshotSchedule(DeleteSnapshotScheduleRequest deleteSnapshotScheduleRequest);
/**
*
* Deletes tags from a resource. You must provide the ARN of the resource from which you want to delete the tag or
* tags.
*
*
* @param deleteTagsRequest
* Contains the output from the DeleteTags
action.
* @return Result of the DeleteTags operation returned by the service.
* @throws ResourceNotFoundException
* The resource could not be found.
* @throws InvalidTagException
* The tag is invalid.
* @sample AmazonRedshift.DeleteTags
* @see AWS API
* Documentation
*/
DeleteTagsResult deleteTags(DeleteTagsRequest deleteTagsRequest);
/**
*
* Deletes a usage limit from a cluster.
*
*
* @param deleteUsageLimitRequest
* @return Result of the DeleteUsageLimit operation returned by the service.
* @throws UsageLimitNotFoundException
* The usage limit identifier can't be found.
* @throws UnsupportedOperationException
* The requested operation isn't supported.
* @sample AmazonRedshift.DeleteUsageLimit
* @see AWS API
* Documentation
*/
DeleteUsageLimitResult deleteUsageLimit(DeleteUsageLimitRequest deleteUsageLimitRequest);
/**
*
* Returns a list of attributes attached to an account
*
*
* @param describeAccountAttributesRequest
* @return Result of the DescribeAccountAttributes operation returned by the service.
* @sample AmazonRedshift.DescribeAccountAttributes
* @see AWS API Documentation
*/
DescribeAccountAttributesResult describeAccountAttributes(DescribeAccountAttributesRequest describeAccountAttributesRequest);
/**
*
* Describes an authentication profile.
*
*
* @param describeAuthenticationProfilesRequest
* @return Result of the DescribeAuthenticationProfiles operation returned by the service.
* @throws AuthenticationProfileNotFoundException
* The authentication profile can't be found.
* @throws InvalidAuthenticationProfileRequestException
* The authentication profile request is not valid. The profile name can't be null or empty. The
* authentication profile API operation must be available in the Amazon Web Services Region.
* @sample AmazonRedshift.DescribeAuthenticationProfiles
* @see AWS API Documentation
*/
DescribeAuthenticationProfilesResult describeAuthenticationProfiles(DescribeAuthenticationProfilesRequest describeAuthenticationProfilesRequest);
/**
*
* Returns an array of ClusterDbRevision
objects.
*
*
* @param describeClusterDbRevisionsRequest
* @return Result of the DescribeClusterDbRevisions operation returned by the service.
* @throws ClusterNotFoundException
* The ClusterIdentifier
parameter does not refer to an existing cluster.
* @throws InvalidClusterStateException
* The specified cluster is not in the available
state.
* @sample AmazonRedshift.DescribeClusterDbRevisions
* @see AWS API Documentation
*/
DescribeClusterDbRevisionsResult describeClusterDbRevisions(DescribeClusterDbRevisionsRequest describeClusterDbRevisionsRequest);
/**
*
* Returns a list of Amazon Redshift parameter groups, including parameter groups you created and the default
* parameter group. For each parameter group, the response includes the parameter group name, description, and
* parameter group family name. You can optionally specify a name to retrieve the description of a specific
* parameter group.
*
*
* For more information about parameters and parameter groups, go to Amazon Redshift
* Parameter Groups in the Amazon Redshift Cluster Management Guide.
*
*
* If you specify both tag keys and tag values in the same request, Amazon Redshift returns all parameter groups
* that match any combination of the specified keys and values. For example, if you have owner
and
* environment
for tag keys, and admin
and test
for tag values, all parameter
* groups that have any combination of those values are returned.
*
*
* If both tag keys and values are omitted from the request, parameter groups are returned regardless of whether
* they have tag keys or values associated with them.
*
*
* @param describeClusterParameterGroupsRequest
* @return Result of the DescribeClusterParameterGroups operation returned by the service.
* @throws ClusterParameterGroupNotFoundException
* The parameter group name does not refer to an existing parameter group.
* @throws InvalidTagException
* The tag is invalid.
* @sample AmazonRedshift.DescribeClusterParameterGroups
* @see AWS API Documentation
*/
DescribeClusterParameterGroupsResult describeClusterParameterGroups(DescribeClusterParameterGroupsRequest describeClusterParameterGroupsRequest);
/**
* Simplified method form for invoking the DescribeClusterParameterGroups operation.
*
* @see #describeClusterParameterGroups(DescribeClusterParameterGroupsRequest)
*/
DescribeClusterParameterGroupsResult describeClusterParameterGroups();
/**
*
* Returns a detailed list of parameters contained within the specified Amazon Redshift parameter group. For each
* parameter the response includes information such as parameter name, description, data type, value, whether the
* parameter value is modifiable, and so on.
*
*
* You can specify source filter to retrieve parameters of only specific type. For example, to retrieve
* parameters that were modified by a user action such as from ModifyClusterParameterGroup, you can specify
* source equal to user.
*
*
* For more information about parameters and parameter groups, go to Amazon Redshift
* Parameter Groups in the Amazon Redshift Cluster Management Guide.
*
*
* @param describeClusterParametersRequest
* @return Result of the DescribeClusterParameters operation returned by the service.
* @throws ClusterParameterGroupNotFoundException
* The parameter group name does not refer to an existing parameter group.
* @sample AmazonRedshift.DescribeClusterParameters
* @see AWS API Documentation
*/
DescribeClusterParametersResult describeClusterParameters(DescribeClusterParametersRequest describeClusterParametersRequest);
/**
*
* Returns information about Amazon Redshift security groups. If the name of a security group is specified, the
* response will contain only information about only that security group.
*
*
* For information about managing security groups, go to Amazon Redshift Cluster
* Security Groups in the Amazon Redshift Cluster Management Guide.
*
*
* If you specify both tag keys and tag values in the same request, Amazon Redshift returns all security groups that
* match any combination of the specified keys and values. For example, if you have owner
and
* environment
for tag keys, and admin
and test
for tag values, all security
* groups that have any combination of those values are returned.
*
*
* If both tag keys and values are omitted from the request, security groups are returned regardless of whether they
* have tag keys or values associated with them.
*
*
* @param describeClusterSecurityGroupsRequest
* @return Result of the DescribeClusterSecurityGroups operation returned by the service.
* @throws ClusterSecurityGroupNotFoundException
* The cluster security group name does not refer to an existing cluster security group.
* @throws InvalidTagException
* The tag is invalid.
* @sample AmazonRedshift.DescribeClusterSecurityGroups
* @see AWS API Documentation
*/
DescribeClusterSecurityGroupsResult describeClusterSecurityGroups(DescribeClusterSecurityGroupsRequest describeClusterSecurityGroupsRequest);
/**
* Simplified method form for invoking the DescribeClusterSecurityGroups operation.
*
* @see #describeClusterSecurityGroups(DescribeClusterSecurityGroupsRequest)
*/
DescribeClusterSecurityGroupsResult describeClusterSecurityGroups();
/**
*
* Returns one or more snapshot objects, which contain metadata about your cluster snapshots. By default, this
* operation returns information about all snapshots of all clusters that are owned by your Amazon Web Services
* account. No information is returned for snapshots owned by inactive Amazon Web Services accounts.
*
*
* If you specify both tag keys and tag values in the same request, Amazon Redshift returns all snapshots that match
* any combination of the specified keys and values. For example, if you have owner
and
* environment
for tag keys, and admin
and test
for tag values, all snapshots
* that have any combination of those values are returned. Only snapshots that you own are returned in the response;
* shared snapshots are not returned with the tag key and tag value request parameters.
*
*
* If both tag keys and values are omitted from the request, snapshots are returned regardless of whether they have
* tag keys or values associated with them.
*
*
* @param describeClusterSnapshotsRequest
* @return Result of the DescribeClusterSnapshots operation returned by the service.
* @throws ClusterNotFoundException
* The ClusterIdentifier
parameter does not refer to an existing cluster.
* @throws ClusterSnapshotNotFoundException
* The snapshot identifier does not refer to an existing cluster snapshot.
* @throws InvalidTagException
* The tag is invalid.
* @throws UnsupportedOperationException
* The requested operation isn't supported.
* @sample AmazonRedshift.DescribeClusterSnapshots
* @see AWS API Documentation
*/
DescribeClusterSnapshotsResult describeClusterSnapshots(DescribeClusterSnapshotsRequest describeClusterSnapshotsRequest);
/**
* Simplified method form for invoking the DescribeClusterSnapshots operation.
*
* @see #describeClusterSnapshots(DescribeClusterSnapshotsRequest)
*/
DescribeClusterSnapshotsResult describeClusterSnapshots();
/**
*
* Returns one or more cluster subnet group objects, which contain metadata about your cluster subnet groups. By
* default, this operation returns information about all cluster subnet groups that are defined in your Amazon Web
* Services account.
*
*
* If you specify both tag keys and tag values in the same request, Amazon Redshift returns all subnet groups that
* match any combination of the specified keys and values. For example, if you have owner
and
* environment
for tag keys, and admin
and test
for tag values, all subnet
* groups that have any combination of those values are returned.
*
*
* If both tag keys and values are omitted from the request, subnet groups are returned regardless of whether they
* have tag keys or values associated with them.
*
*
* @param describeClusterSubnetGroupsRequest
* @return Result of the DescribeClusterSubnetGroups operation returned by the service.
* @throws ClusterSubnetGroupNotFoundException
* The cluster subnet group name does not refer to an existing cluster subnet group.
* @throws InvalidTagException
* The tag is invalid.
* @sample AmazonRedshift.DescribeClusterSubnetGroups
* @see AWS API Documentation
*/
DescribeClusterSubnetGroupsResult describeClusterSubnetGroups(DescribeClusterSubnetGroupsRequest describeClusterSubnetGroupsRequest);
/**
* Simplified method form for invoking the DescribeClusterSubnetGroups operation.
*
* @see #describeClusterSubnetGroups(DescribeClusterSubnetGroupsRequest)
*/
DescribeClusterSubnetGroupsResult describeClusterSubnetGroups();
/**
*
* Returns a list of all the available maintenance tracks.
*
*
* @param describeClusterTracksRequest
* @return Result of the DescribeClusterTracks operation returned by the service.
* @throws InvalidClusterTrackException
* The provided cluster track name is not valid.
* @throws UnauthorizedOperationException
* Your account is not authorized to perform the requested operation.
* @sample AmazonRedshift.DescribeClusterTracks
* @see AWS
* API Documentation
*/
DescribeClusterTracksResult describeClusterTracks(DescribeClusterTracksRequest describeClusterTracksRequest);
/**
*
* Returns descriptions of the available Amazon Redshift cluster versions. You can call this operation even before
* creating any clusters to learn more about the Amazon Redshift versions. For more information about managing
* clusters, go to Amazon
* Redshift Clusters in the Amazon Redshift Cluster Management Guide.
*
*
* @param describeClusterVersionsRequest
* @return Result of the DescribeClusterVersions operation returned by the service.
* @sample AmazonRedshift.DescribeClusterVersions
* @see AWS API Documentation
*/
DescribeClusterVersionsResult describeClusterVersions(DescribeClusterVersionsRequest describeClusterVersionsRequest);
/**
* Simplified method form for invoking the DescribeClusterVersions operation.
*
* @see #describeClusterVersions(DescribeClusterVersionsRequest)
*/
DescribeClusterVersionsResult describeClusterVersions();
/**
*
* Returns properties of provisioned clusters including general cluster properties, cluster database properties,
* maintenance and backup properties, and security and access properties. This operation supports pagination. For
* more information about managing clusters, go to Amazon Redshift Clusters
* in the Amazon Redshift Cluster Management Guide.
*
*
* If you specify both tag keys and tag values in the same request, Amazon Redshift returns all clusters that match
* any combination of the specified keys and values. For example, if you have owner
and
* environment
for tag keys, and admin
and test
for tag values, all clusters
* that have any combination of those values are returned.
*
*
* If both tag keys and values are omitted from the request, clusters are returned regardless of whether they have
* tag keys or values associated with them.
*
*
* @param describeClustersRequest
* @return Result of the DescribeClusters operation returned by the service.
* @throws ClusterNotFoundException
* The ClusterIdentifier
parameter does not refer to an existing cluster.
* @throws InvalidTagException
* The tag is invalid.
* @sample AmazonRedshift.DescribeClusters
* @see AWS API
* Documentation
*/
DescribeClustersResult describeClusters(DescribeClustersRequest describeClustersRequest);
/**
* Simplified method form for invoking the DescribeClusters operation.
*
* @see #describeClusters(DescribeClustersRequest)
*/
DescribeClustersResult describeClusters();
/**
*
* Contains information about custom domain associations for a cluster.
*
*
* @param describeCustomDomainAssociationsRequest
* @return Result of the DescribeCustomDomainAssociations operation returned by the service.
* @throws CustomDomainAssociationNotFoundException
* An error occurred. The custom domain name couldn't be found.
* @throws UnsupportedOperationException
* The requested operation isn't supported.
* @sample AmazonRedshift.DescribeCustomDomainAssociations
* @see AWS API Documentation
*/
DescribeCustomDomainAssociationsResult describeCustomDomainAssociations(DescribeCustomDomainAssociationsRequest describeCustomDomainAssociationsRequest);
/**
*
* Shows the status of any inbound or outbound datashares available in the specified account.
*
*
* @param describeDataSharesRequest
* @return Result of the DescribeDataShares operation returned by the service.
* @throws InvalidDataShareException
* There is an error with the datashare.
* @sample AmazonRedshift.DescribeDataShares
* @see AWS
* API Documentation
*/
DescribeDataSharesResult describeDataShares(DescribeDataSharesRequest describeDataSharesRequest);
/**
*
* Returns a list of datashares where the account identifier being called is a consumer account identifier.
*
*
* @param describeDataSharesForConsumerRequest
* @return Result of the DescribeDataSharesForConsumer operation returned by the service.
* @throws InvalidNamespaceException
* The namespace isn't valid because the namespace doesn't exist. Provide a valid namespace.
* @sample AmazonRedshift.DescribeDataSharesForConsumer
* @see AWS API Documentation
*/
DescribeDataSharesForConsumerResult describeDataSharesForConsumer(DescribeDataSharesForConsumerRequest describeDataSharesForConsumerRequest);
/**
*
* Returns a list of datashares when the account identifier being called is a producer account identifier.
*
*
* @param describeDataSharesForProducerRequest
* @return Result of the DescribeDataSharesForProducer operation returned by the service.
* @throws InvalidNamespaceException
* The namespace isn't valid because the namespace doesn't exist. Provide a valid namespace.
* @sample AmazonRedshift.DescribeDataSharesForProducer
* @see AWS API Documentation
*/
DescribeDataSharesForProducerResult describeDataSharesForProducer(DescribeDataSharesForProducerRequest describeDataSharesForProducerRequest);
/**
*
* Returns a list of parameter settings for the specified parameter group family.
*
*
* For more information about parameters and parameter groups, go to Amazon Redshift
* Parameter Groups in the Amazon Redshift Cluster Management Guide.
*
*
* @param describeDefaultClusterParametersRequest
* @return Result of the DescribeDefaultClusterParameters operation returned by the service.
* @sample AmazonRedshift.DescribeDefaultClusterParameters
* @see AWS API Documentation
*/
DefaultClusterParameters describeDefaultClusterParameters(DescribeDefaultClusterParametersRequest describeDefaultClusterParametersRequest);
/**
*
* Describes a Redshift-managed VPC endpoint.
*
*
* @param describeEndpointAccessRequest
* @return Result of the DescribeEndpointAccess operation returned by the service.
* @throws ClusterNotFoundException
* The ClusterIdentifier
parameter does not refer to an existing cluster.
* @throws InvalidClusterStateException
* The specified cluster is not in the available
state.
* @throws EndpointNotFoundException
* The endpoint name doesn't refer to an existing endpoint.
* @sample AmazonRedshift.DescribeEndpointAccess
* @see AWS API Documentation
*/
DescribeEndpointAccessResult describeEndpointAccess(DescribeEndpointAccessRequest describeEndpointAccessRequest);
/**
*
* Describes an endpoint authorization.
*
*
* @param describeEndpointAuthorizationRequest
* @return Result of the DescribeEndpointAuthorization operation returned by the service.
* @throws ClusterNotFoundException
* The ClusterIdentifier
parameter does not refer to an existing cluster.
* @throws UnsupportedOperationException
* The requested operation isn't supported.
* @sample AmazonRedshift.DescribeEndpointAuthorization
* @see AWS API Documentation
*/
DescribeEndpointAuthorizationResult describeEndpointAuthorization(DescribeEndpointAuthorizationRequest describeEndpointAuthorizationRequest);
/**
*
* Displays a list of event categories for all event source types, or for a specified source type. For a list of the
* event categories and source types, go to Amazon Redshift
* Event Notifications.
*
*
* @param describeEventCategoriesRequest
* @return Result of the DescribeEventCategories operation returned by the service.
* @sample AmazonRedshift.DescribeEventCategories
* @see AWS API Documentation
*/
DescribeEventCategoriesResult describeEventCategories(DescribeEventCategoriesRequest describeEventCategoriesRequest);
/**
* Simplified method form for invoking the DescribeEventCategories operation.
*
* @see #describeEventCategories(DescribeEventCategoriesRequest)
*/
DescribeEventCategoriesResult describeEventCategories();
/**
*
* Lists descriptions of all the Amazon Redshift event notification subscriptions for a customer account. If you
* specify a subscription name, lists the description for that subscription.
*
*
* If you specify both tag keys and tag values in the same request, Amazon Redshift returns all event notification
* subscriptions that match any combination of the specified keys and values. For example, if you have
* owner
and environment
for tag keys, and admin
and test
for
* tag values, all subscriptions that have any combination of those values are returned.
*
*
* If both tag keys and values are omitted from the request, subscriptions are returned regardless of whether they
* have tag keys or values associated with them.
*
*
* @param describeEventSubscriptionsRequest
* @return Result of the DescribeEventSubscriptions operation returned by the service.
* @throws SubscriptionNotFoundException
* An Amazon Redshift event notification subscription with the specified name does not exist.
* @throws InvalidTagException
* The tag is invalid.
* @sample AmazonRedshift.DescribeEventSubscriptions
* @see AWS API Documentation
*/
DescribeEventSubscriptionsResult describeEventSubscriptions(DescribeEventSubscriptionsRequest describeEventSubscriptionsRequest);
/**
* Simplified method form for invoking the DescribeEventSubscriptions operation.
*
* @see #describeEventSubscriptions(DescribeEventSubscriptionsRequest)
*/
DescribeEventSubscriptionsResult describeEventSubscriptions();
/**
*
* Returns events related to clusters, security groups, snapshots, and parameter groups for the past 14 days. Events
* specific to a particular cluster, security group, snapshot or parameter group can be obtained by providing the
* name as a parameter. By default, the past hour of events are returned.
*
*
* @param describeEventsRequest
* @return Result of the DescribeEvents operation returned by the service.
* @sample AmazonRedshift.DescribeEvents
* @see AWS API
* Documentation
*/
DescribeEventsResult describeEvents(DescribeEventsRequest describeEventsRequest);
/**
* Simplified method form for invoking the DescribeEvents operation.
*
* @see #describeEvents(DescribeEventsRequest)
*/
DescribeEventsResult describeEvents();
/**
*
* Returns information about the specified HSM client certificate. If no certificate ID is specified, returns
* information about all the HSM certificates owned by your Amazon Web Services account.
*
*
* If you specify both tag keys and tag values in the same request, Amazon Redshift returns all HSM client
* certificates that match any combination of the specified keys and values. For example, if you have
* owner
and environment
for tag keys, and admin
and test
for
* tag values, all HSM client certificates that have any combination of those values are returned.
*
*
* If both tag keys and values are omitted from the request, HSM client certificates are returned regardless of
* whether they have tag keys or values associated with them.
*
*
* @param describeHsmClientCertificatesRequest
* @return Result of the DescribeHsmClientCertificates operation returned by the service.
* @throws HsmClientCertificateNotFoundException
* There is no Amazon Redshift HSM client certificate with the specified identifier.
* @throws InvalidTagException
* The tag is invalid.
* @sample AmazonRedshift.DescribeHsmClientCertificates
* @see AWS API Documentation
*/
DescribeHsmClientCertificatesResult describeHsmClientCertificates(DescribeHsmClientCertificatesRequest describeHsmClientCertificatesRequest);
/**
* Simplified method form for invoking the DescribeHsmClientCertificates operation.
*
* @see #describeHsmClientCertificates(DescribeHsmClientCertificatesRequest)
*/
DescribeHsmClientCertificatesResult describeHsmClientCertificates();
/**
*
* Returns information about the specified Amazon Redshift HSM configuration. If no configuration ID is specified,
* returns information about all the HSM configurations owned by your Amazon Web Services account.
*
*
* If you specify both tag keys and tag values in the same request, Amazon Redshift returns all HSM connections that
* match any combination of the specified keys and values. For example, if you have owner
and
* environment
for tag keys, and admin
and test
for tag values, all HSM
* connections that have any combination of those values are returned.
*
*
* If both tag keys and values are omitted from the request, HSM connections are returned regardless of whether they
* have tag keys or values associated with them.
*
*
* @param describeHsmConfigurationsRequest
* @return Result of the DescribeHsmConfigurations operation returned by the service.
* @throws HsmConfigurationNotFoundException
* There is no Amazon Redshift HSM configuration with the specified identifier.
* @throws InvalidTagException
* The tag is invalid.
* @sample AmazonRedshift.DescribeHsmConfigurations
* @see AWS API Documentation
*/
DescribeHsmConfigurationsResult describeHsmConfigurations(DescribeHsmConfigurationsRequest describeHsmConfigurationsRequest);
/**
* Simplified method form for invoking the DescribeHsmConfigurations operation.
*
* @see #describeHsmConfigurations(DescribeHsmConfigurationsRequest)
*/
DescribeHsmConfigurationsResult describeHsmConfigurations();
/**
*
* Returns a list of inbound integrations.
*
*
* @param describeInboundIntegrationsRequest
* @return Result of the DescribeInboundIntegrations operation returned by the service.
* @throws IntegrationNotFoundException
* The integration can't be found.
* @throws InvalidNamespaceException
* The namespace isn't valid because the namespace doesn't exist. Provide a valid namespace.
* @throws UnsupportedOperationException
* The requested operation isn't supported.
* @sample AmazonRedshift.DescribeInboundIntegrations
* @see AWS API Documentation
*/
DescribeInboundIntegrationsResult describeInboundIntegrations(DescribeInboundIntegrationsRequest describeInboundIntegrationsRequest);
/**
*
* Describes whether information, such as queries and connection attempts, is being logged for the specified Amazon
* Redshift cluster.
*
*
* @param describeLoggingStatusRequest
* @return Result of the DescribeLoggingStatus operation returned by the service.
* @throws ClusterNotFoundException
* The ClusterIdentifier
parameter does not refer to an existing cluster.
* @throws UnsupportedOperationException
* The requested operation isn't supported.
* @sample AmazonRedshift.DescribeLoggingStatus
* @see AWS
* API Documentation
*/
DescribeLoggingStatusResult describeLoggingStatus(DescribeLoggingStatusRequest describeLoggingStatusRequest);
/**
*
* Returns properties of possible node configurations such as node type, number of nodes, and disk usage for the
* specified action type.
*
*
* @param describeNodeConfigurationOptionsRequest
* @return Result of the DescribeNodeConfigurationOptions operation returned by the service.
* @throws ClusterSnapshotNotFoundException
* The snapshot identifier does not refer to an existing cluster snapshot.
* @throws InvalidClusterSnapshotStateException
* The specified cluster snapshot is not in the available
state, or other accounts are
* authorized to access the snapshot.
* @throws ClusterNotFoundException
* The ClusterIdentifier
parameter does not refer to an existing cluster.
* @throws AccessToSnapshotDeniedException
* The owner of the specified snapshot has not authorized your account to access the snapshot.
* @throws UnsupportedOperationException
* The requested operation isn't supported.
* @sample AmazonRedshift.DescribeNodeConfigurationOptions
* @see AWS API Documentation
*/
DescribeNodeConfigurationOptionsResult describeNodeConfigurationOptions(DescribeNodeConfigurationOptionsRequest describeNodeConfigurationOptionsRequest);
/**
*
* Returns a list of orderable cluster options. Before you create a new cluster you can use this operation to find
* what options are available, such as the EC2 Availability Zones (AZ) in the specific Amazon Web Services Region
* that you can specify, and the node types you can request. The node types differ by available storage, memory, CPU
* and price. With the cost involved you might want to obtain a list of cluster options in the specific region and
* specify values when creating a cluster. For more information about managing clusters, go to Amazon Redshift Clusters
* in the Amazon Redshift Cluster Management Guide.
*
*
* @param describeOrderableClusterOptionsRequest
* @return Result of the DescribeOrderableClusterOptions operation returned by the service.
* @sample AmazonRedshift.DescribeOrderableClusterOptions
* @see AWS API Documentation
*/
DescribeOrderableClusterOptionsResult describeOrderableClusterOptions(DescribeOrderableClusterOptionsRequest describeOrderableClusterOptionsRequest);
/**
* Simplified method form for invoking the DescribeOrderableClusterOptions operation.
*
* @see #describeOrderableClusterOptions(DescribeOrderableClusterOptionsRequest)
*/
DescribeOrderableClusterOptionsResult describeOrderableClusterOptions();
/**
*
* Returns information about the partner integrations defined for a cluster.
*
*
* @param describePartnersRequest
* @return Result of the DescribePartners operation returned by the service.
* @throws ClusterNotFoundException
* The ClusterIdentifier
parameter does not refer to an existing cluster.
* @throws UnauthorizedPartnerIntegrationException
* The partner integration is not authorized.
* @throws UnsupportedOperationException
* The requested operation isn't supported.
* @sample AmazonRedshift.DescribePartners
* @see AWS API
* Documentation
*/
DescribePartnersResult describePartners(DescribePartnersRequest describePartnersRequest);
/**
*
* Lists the Amazon Redshift IAM Identity Center applications.
*
*
* @param describeRedshiftIdcApplicationsRequest
* @return Result of the DescribeRedshiftIdcApplications operation returned by the service.
* @throws RedshiftIdcApplicationNotExistsException
* The application you attempted to find doesn't exist.
* @throws DependentServiceUnavailableException
* Your request cannot be completed because a dependent internal service is temporarily unavailable. Wait 30
* to 60 seconds and try again.
* @throws UnsupportedOperationException
* The requested operation isn't supported.
* @throws DependentServiceAccessDeniedException
* A dependent service denied access for the integration.
* @sample AmazonRedshift.DescribeRedshiftIdcApplications
* @see AWS API Documentation
*/
DescribeRedshiftIdcApplicationsResult describeRedshiftIdcApplications(DescribeRedshiftIdcApplicationsRequest describeRedshiftIdcApplicationsRequest);
/**
*
* Returns exchange status details and associated metadata for a reserved-node exchange. Statuses include such
* values as in progress and requested.
*
*
* @param describeReservedNodeExchangeStatusRequest
* @return Result of the DescribeReservedNodeExchangeStatus operation returned by the service.
* @throws ReservedNodeNotFoundException
* The specified reserved compute node not found.
* @throws ReservedNodeExchangeNotFoundException
* The reserved-node exchange status wasn't found.
* @throws UnsupportedOperationException
* The requested operation isn't supported.
* @sample AmazonRedshift.DescribeReservedNodeExchangeStatus
* @see AWS API Documentation
*/
DescribeReservedNodeExchangeStatusResult describeReservedNodeExchangeStatus(
DescribeReservedNodeExchangeStatusRequest describeReservedNodeExchangeStatusRequest);
/**
*
* Returns a list of the available reserved node offerings by Amazon Redshift with their descriptions including the
* node type, the fixed and recurring costs of reserving the node and duration the node will be reserved for you.
* These descriptions help you determine which reserve node offering you want to purchase. You then use the unique
* offering ID in you call to PurchaseReservedNodeOffering to reserve one or more nodes for your Amazon
* Redshift cluster.
*
*
* For more information about reserved node offerings, go to Purchasing Reserved
* Nodes in the Amazon Redshift Cluster Management Guide.
*
*
* @param describeReservedNodeOfferingsRequest
* @return Result of the DescribeReservedNodeOfferings operation returned by the service.
* @throws ReservedNodeOfferingNotFoundException
* Specified offering does not exist.
* @throws UnsupportedOperationException
* The requested operation isn't supported.
* @throws DependentServiceUnavailableException
* Your request cannot be completed because a dependent internal service is temporarily unavailable. Wait 30
* to 60 seconds and try again.
* @sample AmazonRedshift.DescribeReservedNodeOfferings
* @see AWS API Documentation
*/
DescribeReservedNodeOfferingsResult describeReservedNodeOfferings(DescribeReservedNodeOfferingsRequest describeReservedNodeOfferingsRequest);
/**
* Simplified method form for invoking the DescribeReservedNodeOfferings operation.
*
* @see #describeReservedNodeOfferings(DescribeReservedNodeOfferingsRequest)
*/
DescribeReservedNodeOfferingsResult describeReservedNodeOfferings();
/**
*
* Returns the descriptions of the reserved nodes.
*
*
* @param describeReservedNodesRequest
* @return Result of the DescribeReservedNodes operation returned by the service.
* @throws ReservedNodeNotFoundException
* The specified reserved compute node not found.
* @throws DependentServiceUnavailableException
* Your request cannot be completed because a dependent internal service is temporarily unavailable. Wait 30
* to 60 seconds and try again.
* @sample AmazonRedshift.DescribeReservedNodes
* @see AWS
* API Documentation
*/
DescribeReservedNodesResult describeReservedNodes(DescribeReservedNodesRequest describeReservedNodesRequest);
/**
* Simplified method form for invoking the DescribeReservedNodes operation.
*
* @see #describeReservedNodes(DescribeReservedNodesRequest)
*/
DescribeReservedNodesResult describeReservedNodes();
/**
*
* Returns information about the last resize operation for the specified cluster. If no resize operation has ever
* been initiated for the specified cluster, a HTTP 404
error is returned. If a resize operation was
* initiated and completed, the status of the resize remains as SUCCEEDED
until the next resize.
*
*
* A resize operation can be requested using ModifyCluster and specifying a different number or type of nodes
* for the cluster.
*
*
* @param describeResizeRequest
* @return Result of the DescribeResize operation returned by the service.
* @throws ClusterNotFoundException
* The ClusterIdentifier
parameter does not refer to an existing cluster.
* @throws ResizeNotFoundException
* A resize operation for the specified cluster is not found.
* @throws UnsupportedOperationException
* The requested operation isn't supported.
* @sample AmazonRedshift.DescribeResize
* @see AWS API
* Documentation
*/
DescribeResizeResult describeResize(DescribeResizeRequest describeResizeRequest);
/**
*
* Describes properties of scheduled actions.
*
*
* @param describeScheduledActionsRequest
* @return Result of the DescribeScheduledActions operation returned by the service.
* @throws ScheduledActionNotFoundException
* The scheduled action cannot be found.
* @throws UnauthorizedOperationException
* Your account is not authorized to perform the requested operation.
* @sample AmazonRedshift.DescribeScheduledActions
* @see AWS API Documentation
*/
DescribeScheduledActionsResult describeScheduledActions(DescribeScheduledActionsRequest describeScheduledActionsRequest);
/**
*
* Returns a list of snapshot copy grants owned by the Amazon Web Services account in the destination region.
*
*
* For more information about managing snapshot copy grants, go to Amazon Redshift Database
* Encryption in the Amazon Redshift Cluster Management Guide.
*
*
* @param describeSnapshotCopyGrantsRequest
* The result of the DescribeSnapshotCopyGrants
action.
* @return Result of the DescribeSnapshotCopyGrants operation returned by the service.
* @throws SnapshotCopyGrantNotFoundException
* The specified snapshot copy grant can't be found. Make sure that the name is typed correctly and that the
* grant exists in the destination region.
* @throws InvalidTagException
* The tag is invalid.
* @sample AmazonRedshift.DescribeSnapshotCopyGrants
* @see AWS API Documentation
*/
DescribeSnapshotCopyGrantsResult describeSnapshotCopyGrants(DescribeSnapshotCopyGrantsRequest describeSnapshotCopyGrantsRequest);
/**
* Simplified method form for invoking the DescribeSnapshotCopyGrants operation.
*
* @see #describeSnapshotCopyGrants(DescribeSnapshotCopyGrantsRequest)
*/
DescribeSnapshotCopyGrantsResult describeSnapshotCopyGrants();
/**
*
* Returns a list of snapshot schedules.
*
*
* @param describeSnapshotSchedulesRequest
* @return Result of the DescribeSnapshotSchedules operation returned by the service.
* @sample AmazonRedshift.DescribeSnapshotSchedules
* @see AWS API Documentation
*/
DescribeSnapshotSchedulesResult describeSnapshotSchedules(DescribeSnapshotSchedulesRequest describeSnapshotSchedulesRequest);
/**
*
* Returns account level backups storage size and provisional storage.
*
*
* @param describeStorageRequest
* @return Result of the DescribeStorage operation returned by the service.
* @sample AmazonRedshift.DescribeStorage
* @see AWS API
* Documentation
*/
DescribeStorageResult describeStorage(DescribeStorageRequest describeStorageRequest);
/**
*
* Lists the status of one or more table restore requests made using the RestoreTableFromClusterSnapshot API
* action. If you don't specify a value for the TableRestoreRequestId
parameter, then
* DescribeTableRestoreStatus
returns the status of all table restore requests ordered by the date and
* time of the request in ascending order. Otherwise DescribeTableRestoreStatus
returns the status of
* the table specified by TableRestoreRequestId
.
*
*
* @param describeTableRestoreStatusRequest
* @return Result of the DescribeTableRestoreStatus operation returned by the service.
* @throws TableRestoreNotFoundException
* The specified TableRestoreRequestId
value was not found.
* @throws ClusterNotFoundException
* The ClusterIdentifier
parameter does not refer to an existing cluster.
* @sample AmazonRedshift.DescribeTableRestoreStatus
* @see AWS API Documentation
*/
DescribeTableRestoreStatusResult describeTableRestoreStatus(DescribeTableRestoreStatusRequest describeTableRestoreStatusRequest);
/**
* Simplified method form for invoking the DescribeTableRestoreStatus operation.
*
* @see #describeTableRestoreStatus(DescribeTableRestoreStatusRequest)
*/
DescribeTableRestoreStatusResult describeTableRestoreStatus();
/**
*
* Returns a list of tags. You can return tags from a specific resource by specifying an ARN, or you can return all
* tags for a given type of resource, such as clusters, snapshots, and so on.
*
*
* The following are limitations for DescribeTags
:
*
*
* -
*
* You cannot specify an ARN and a resource-type value together in the same request.
*
*
* -
*
* You cannot use the MaxRecords
and Marker
parameters together with the ARN parameter.
*
*
* -
*
* The MaxRecords
parameter can be a range from 10 to 50 results to return in a request.
*
*
*
*
* If you specify both tag keys and tag values in the same request, Amazon Redshift returns all resources that match
* any combination of the specified keys and values. For example, if you have owner
and
* environment
for tag keys, and admin
and test
for tag values, all resources
* that have any combination of those values are returned.
*
*
* If both tag keys and values are omitted from the request, resources are returned regardless of whether they have
* tag keys or values associated with them.
*
*
* @param describeTagsRequest
* @return Result of the DescribeTags operation returned by the service.
* @throws ResourceNotFoundException
* The resource could not be found.
* @throws InvalidTagException
* The tag is invalid.
* @sample AmazonRedshift.DescribeTags
* @see AWS API
* Documentation
*/
DescribeTagsResult describeTags(DescribeTagsRequest describeTagsRequest);
/**
* Simplified method form for invoking the DescribeTags operation.
*
* @see #describeTags(DescribeTagsRequest)
*/
DescribeTagsResult describeTags();
/**
*
* Shows usage limits on a cluster. Results are filtered based on the combination of input usage limit identifier,
* cluster identifier, and feature type parameters:
*
*
* -
*
* If usage limit identifier, cluster identifier, and feature type are not provided, then all usage limit objects
* for the current account in the current region are returned.
*
*
* -
*
* If usage limit identifier is provided, then the corresponding usage limit object is returned.
*
*
* -
*
* If cluster identifier is provided, then all usage limit objects for the specified cluster are returned.
*
*
* -
*
* If cluster identifier and feature type are provided, then all usage limit objects for the combination of cluster
* and feature are returned.
*
*
*
*
* @param describeUsageLimitsRequest
* @return Result of the DescribeUsageLimits operation returned by the service.
* @throws ClusterNotFoundException
* The ClusterIdentifier
parameter does not refer to an existing cluster.
* @throws UnsupportedOperationException
* The requested operation isn't supported.
* @sample AmazonRedshift.DescribeUsageLimits
* @see AWS
* API Documentation
*/
DescribeUsageLimitsResult describeUsageLimits(DescribeUsageLimitsRequest describeUsageLimitsRequest);
/**
*
* Stops logging information, such as queries and connection attempts, for the specified Amazon Redshift cluster.
*
*
* @param disableLoggingRequest
* @return Result of the DisableLogging operation returned by the service.
* @throws ClusterNotFoundException
* The ClusterIdentifier
parameter does not refer to an existing cluster.
* @throws InvalidClusterStateException
* The specified cluster is not in the available
state.
* @throws UnsupportedOperationException
* The requested operation isn't supported.
* @sample AmazonRedshift.DisableLogging
* @see AWS API
* Documentation
*/
DisableLoggingResult disableLogging(DisableLoggingRequest disableLoggingRequest);
/**
*
* Disables the automatic copying of snapshots from one region to another region for a specified cluster.
*
*
* If your cluster and its snapshots are encrypted using an encrypted symmetric key from Key Management Service, use
* DeleteSnapshotCopyGrant to delete the grant that grants Amazon Redshift permission to the key in the
* destination region.
*
*
* @param disableSnapshotCopyRequest
* @return Result of the DisableSnapshotCopy operation returned by the service.
* @throws ClusterNotFoundException
* The ClusterIdentifier
parameter does not refer to an existing cluster.
* @throws SnapshotCopyAlreadyDisabledException
* The cluster already has cross-region snapshot copy disabled.
* @throws InvalidClusterStateException
* The specified cluster is not in the available
state.
* @throws UnauthorizedOperationException
* Your account is not authorized to perform the requested operation.
* @throws UnsupportedOperationException
* The requested operation isn't supported.
* @sample AmazonRedshift.DisableSnapshotCopy
* @see AWS
* API Documentation
*/
Cluster disableSnapshotCopy(DisableSnapshotCopyRequest disableSnapshotCopyRequest);
/**
*
* From a datashare consumer account, remove association for the specified datashare.
*
*
* @param disassociateDataShareConsumerRequest
* @return Result of the DisassociateDataShareConsumer operation returned by the service.
* @throws InvalidDataShareException
* There is an error with the datashare.
* @throws InvalidNamespaceException
* The namespace isn't valid because the namespace doesn't exist. Provide a valid namespace.
* @sample AmazonRedshift.DisassociateDataShareConsumer
* @see AWS API Documentation
*/
DisassociateDataShareConsumerResult disassociateDataShareConsumer(DisassociateDataShareConsumerRequest disassociateDataShareConsumerRequest);
/**
*
* Starts logging information, such as queries and connection attempts, for the specified Amazon Redshift cluster.
*
*
* @param enableLoggingRequest
* @return Result of the EnableLogging operation returned by the service.
* @throws ClusterNotFoundException
* The ClusterIdentifier
parameter does not refer to an existing cluster.
* @throws BucketNotFoundException
* Could not find the specified S3 bucket.
* @throws InsufficientS3BucketPolicyException
* The cluster does not have read bucket or put object permissions on the S3 bucket specified when enabling
* logging.
* @throws InvalidS3KeyPrefixException
* The string specified for the logging S3 key prefix does not comply with the documented constraints.
* @throws InvalidS3BucketNameException
* The S3 bucket name is invalid. For more information about naming rules, go to Bucket Restrictions and
* Limitations in the Amazon Simple Storage Service (S3) Developer Guide.
* @throws InvalidClusterStateException
* The specified cluster is not in the available
state.
* @throws UnsupportedOperationException
* The requested operation isn't supported.
* @sample AmazonRedshift.EnableLogging
* @see AWS API
* Documentation
*/
EnableLoggingResult enableLogging(EnableLoggingRequest enableLoggingRequest);
/**
*
* Enables the automatic copy of snapshots from one region to another region for a specified cluster.
*
*
* @param enableSnapshotCopyRequest
* @return Result of the EnableSnapshotCopy operation returned by the service.
* @throws IncompatibleOrderableOptionsException
* The specified options are incompatible.
* @throws InvalidClusterStateException
* The specified cluster is not in the available
state.
* @throws ClusterNotFoundException
* The ClusterIdentifier
parameter does not refer to an existing cluster.
* @throws CopyToRegionDisabledException
* Cross-region snapshot copy was temporarily disabled. Try your request again.
* @throws SnapshotCopyAlreadyEnabledException
* The cluster already has cross-region snapshot copy enabled.
* @throws UnknownSnapshotCopyRegionException
* The specified region is incorrect or does not exist.
* @throws UnauthorizedOperationException
* Your account is not authorized to perform the requested operation.
* @throws SnapshotCopyGrantNotFoundException
* The specified snapshot copy grant can't be found. Make sure that the name is typed correctly and that the
* grant exists in the destination region.
* @throws LimitExceededException
* The encryption key has exceeded its grant limit in Amazon Web Services KMS.
* @throws DependentServiceRequestThrottlingException
* The request cannot be completed because a dependent service is throttling requests made by Amazon
* Redshift on your behalf. Wait and retry the request.
* @throws InvalidRetentionPeriodException
* The retention period specified is either in the past or is not a valid value.
*
* The value must be either -1 or an integer between 1 and 3,653.
* @sample AmazonRedshift.EnableSnapshotCopy
* @see AWS
* API Documentation
*/
Cluster enableSnapshotCopy(EnableSnapshotCopyRequest enableSnapshotCopyRequest);
/**
*
* Fails over the primary compute unit of the specified Multi-AZ cluster to another Availability Zone.
*
*
* @param failoverPrimaryComputeRequest
* @return Result of the FailoverPrimaryCompute operation returned by the service.
* @throws ClusterNotFoundException
* The ClusterIdentifier
parameter does not refer to an existing cluster.
* @throws UnsupportedOperationException
* The requested operation isn't supported.
* @throws UnauthorizedOperationException
* Your account is not authorized to perform the requested operation.
* @throws InvalidClusterStateException
* The specified cluster is not in the available
state.
* @sample AmazonRedshift.FailoverPrimaryCompute
* @see AWS API Documentation
*/
Cluster failoverPrimaryCompute(FailoverPrimaryComputeRequest failoverPrimaryComputeRequest);
/**
*
* Returns a database user name and temporary password with temporary authorization to log on to an Amazon Redshift
* database. The action returns the database user name prefixed with IAM:
if AutoCreate
is
* False
or IAMA:
if AutoCreate
is True
. You can optionally
* specify one or more database user groups that the user will join at log on. By default, the temporary credentials
* expire in 900 seconds. You can optionally specify a duration between 900 seconds (15 minutes) and 3600 seconds
* (60 minutes). For more information, see Using IAM Authentication
* to Generate Database User Credentials in the Amazon Redshift Cluster Management Guide.
*
*
* The Identity and Access Management (IAM) user or role that runs GetClusterCredentials must have an IAM policy
* attached that allows access to all necessary actions and resources. For more information about permissions, see
* Resource Policies for GetClusterCredentials in the Amazon Redshift Cluster Management Guide.
*
*
* If the DbGroups
parameter is specified, the IAM policy must allow the
* redshift:JoinGroup
action with access to the listed dbgroups
.
*
*
* In addition, if the AutoCreate
parameter is set to True
, then the policy must include
* the redshift:CreateClusterUser
permission.
*
*
* If the DbName
parameter is specified, the IAM policy must allow access to the resource
* dbname
for the specified database name.
*
*
* @param getClusterCredentialsRequest
* The request parameters to get cluster credentials.
* @return Result of the GetClusterCredentials operation returned by the service.
* @throws ClusterNotFoundException
* The ClusterIdentifier
parameter does not refer to an existing cluster.
* @throws UnsupportedOperationException
* The requested operation isn't supported.
* @sample AmazonRedshift.GetClusterCredentials
* @see AWS
* API Documentation
*/
GetClusterCredentialsResult getClusterCredentials(GetClusterCredentialsRequest getClusterCredentialsRequest);
/**
*
* Returns a database user name and temporary password with temporary authorization to log in to an Amazon Redshift
* database. The database user is mapped 1:1 to the source Identity and Access Management (IAM) identity. For more
* information about IAM identities, see IAM
* Identities (users, user groups, and roles) in the Amazon Web Services Identity and Access Management User
* Guide.
*
*
* The Identity and Access Management (IAM) identity that runs this operation must have an IAM policy attached that
* allows access to all necessary actions and resources. For more information about permissions, see Using
* identity-based policies (IAM policies) in the Amazon Redshift Cluster Management Guide.
*
*
* @param getClusterCredentialsWithIAMRequest
* @return Result of the GetClusterCredentialsWithIAM operation returned by the service.
* @throws ClusterNotFoundException
* The ClusterIdentifier
parameter does not refer to an existing cluster.
* @throws UnsupportedOperationException
* The requested operation isn't supported.
* @sample AmazonRedshift.GetClusterCredentialsWithIAM
* @see AWS API Documentation
*/
GetClusterCredentialsWithIAMResult getClusterCredentialsWithIAM(GetClusterCredentialsWithIAMRequest getClusterCredentialsWithIAMRequest);
/**
*
* Gets the configuration options for the reserved-node exchange. These options include information about the source
* reserved node and target reserved node offering. Details include the node type, the price, the node count, and
* the offering type.
*
*
* @param getReservedNodeExchangeConfigurationOptionsRequest
* @return Result of the GetReservedNodeExchangeConfigurationOptions operation returned by the service.
* @throws ReservedNodeNotFoundException
* The specified reserved compute node not found.
* @throws InvalidReservedNodeStateException
* Indicates that the Reserved Node being exchanged is not in an active state.
* @throws ReservedNodeAlreadyMigratedException
* Indicates that the reserved node has already been exchanged.
* @throws ReservedNodeOfferingNotFoundException
* Specified offering does not exist.
* @throws UnsupportedOperationException
* The requested operation isn't supported.
* @throws DependentServiceUnavailableException
* Your request cannot be completed because a dependent internal service is temporarily unavailable. Wait 30
* to 60 seconds and try again.
* @throws ClusterNotFoundException
* The ClusterIdentifier
parameter does not refer to an existing cluster.
* @throws ClusterSnapshotNotFoundException
* The snapshot identifier does not refer to an existing cluster snapshot.
* @sample AmazonRedshift.GetReservedNodeExchangeConfigurationOptions
* @see AWS API Documentation
*/
GetReservedNodeExchangeConfigurationOptionsResult getReservedNodeExchangeConfigurationOptions(
GetReservedNodeExchangeConfigurationOptionsRequest getReservedNodeExchangeConfigurationOptionsRequest);
/**
*
* Returns an array of DC2 ReservedNodeOfferings that matches the payment type, term, and usage price of the given
* DC1 reserved node.
*
*
* @param getReservedNodeExchangeOfferingsRequest
* @return Result of the GetReservedNodeExchangeOfferings operation returned by the service.
* @throws ReservedNodeNotFoundException
* The specified reserved compute node not found.
* @throws InvalidReservedNodeStateException
* Indicates that the Reserved Node being exchanged is not in an active state.
* @throws ReservedNodeAlreadyMigratedException
* Indicates that the reserved node has already been exchanged.
* @throws ReservedNodeOfferingNotFoundException
* Specified offering does not exist.
* @throws UnsupportedOperationException
* The requested operation isn't supported.
* @throws DependentServiceUnavailableException
* Your request cannot be completed because a dependent internal service is temporarily unavailable. Wait 30
* to 60 seconds and try again.
* @sample AmazonRedshift.GetReservedNodeExchangeOfferings
* @see AWS API Documentation
*/
GetReservedNodeExchangeOfferingsResult getReservedNodeExchangeOfferings(GetReservedNodeExchangeOfferingsRequest getReservedNodeExchangeOfferingsRequest);
/**
*
* Get the resource policy for a specified resource.
*
*
* @param getResourcePolicyRequest
* @return Result of the GetResourcePolicy operation returned by the service.
* @throws ResourceNotFoundException
* The resource could not be found.
* @throws InvalidPolicyException
* The resource policy isn't valid.
* @throws UnsupportedOperationException
* The requested operation isn't supported.
* @sample AmazonRedshift.GetResourcePolicy
* @see AWS API
* Documentation
*/
GetResourcePolicyResult getResourcePolicy(GetResourcePolicyRequest getResourcePolicyRequest);
/**
*
* List the Amazon Redshift Advisor recommendations for one or multiple Amazon Redshift clusters in an Amazon Web
* Services account.
*
*
* @param listRecommendationsRequest
* @return Result of the ListRecommendations operation returned by the service.
* @throws ClusterNotFoundException
* The ClusterIdentifier
parameter does not refer to an existing cluster.
* @throws UnsupportedOperationException
* The requested operation isn't supported.
* @sample AmazonRedshift.ListRecommendations
* @see AWS
* API Documentation
*/
ListRecommendationsResult listRecommendations(ListRecommendationsRequest listRecommendationsRequest);
/**
*
* This operation is retired. Calling this operation does not change AQUA configuration. Amazon Redshift
* automatically determines whether to use AQUA (Advanced Query Accelerator).
*
*
* @param modifyAquaConfigurationRequest
* @return Result of the ModifyAquaConfiguration operation returned by the service.
* @throws ClusterNotFoundException
* The ClusterIdentifier
parameter does not refer to an existing cluster.
* @throws InvalidClusterStateException
* The specified cluster is not in the available
state.
* @throws UnsupportedOperationException
* The requested operation isn't supported.
* @sample AmazonRedshift.ModifyAquaConfiguration
* @see AWS API Documentation
*/
ModifyAquaConfigurationResult modifyAquaConfiguration(ModifyAquaConfigurationRequest modifyAquaConfigurationRequest);
/**
*
* Modifies an authentication profile.
*
*
* @param modifyAuthenticationProfileRequest
* @return Result of the ModifyAuthenticationProfile operation returned by the service.
* @throws AuthenticationProfileNotFoundException
* The authentication profile can't be found.
* @throws AuthenticationProfileQuotaExceededException
* The size or number of authentication profiles has exceeded the quota. The maximum length of the JSON
* string and maximum number of authentication profiles is determined by a quota for your account.
* @throws InvalidAuthenticationProfileRequestException
* The authentication profile request is not valid. The profile name can't be null or empty. The
* authentication profile API operation must be available in the Amazon Web Services Region.
* @sample AmazonRedshift.ModifyAuthenticationProfile
* @see AWS API Documentation
*/
ModifyAuthenticationProfileResult modifyAuthenticationProfile(ModifyAuthenticationProfileRequest modifyAuthenticationProfileRequest);
/**
*
* Modifies the settings for a cluster.
*
*
* You can also change node type and the number of nodes to scale up or down the cluster. When resizing a cluster,
* you must specify both the number of nodes and the node type even if one of the parameters does not change.
*
*
* You can add another security or parameter group, or change the admin user password. Resetting a cluster password
* or modifying the security groups associated with a cluster do not need a reboot. However, modifying a parameter
* group requires a reboot for parameters to take effect. For more information about managing clusters, go to Amazon Redshift Clusters
* in the Amazon Redshift Cluster Management Guide.
*
*
* @param modifyClusterRequest
* @return Result of the ModifyCluster operation returned by the service.
* @throws InvalidClusterStateException
* The specified cluster is not in the available
state.
* @throws InvalidClusterSecurityGroupStateException
* The state of the cluster security group is not available
.
* @throws ClusterNotFoundException
* The ClusterIdentifier
parameter does not refer to an existing cluster.
* @throws NumberOfNodesQuotaExceededException
* The operation would exceed the number of nodes allotted to the account. For information about increasing
* your quota, go to Limits in Amazon
* Redshift in the Amazon Redshift Cluster Management Guide.
* @throws NumberOfNodesPerClusterLimitExceededException
* The operation would exceed the number of nodes allowed for a cluster.
* @throws ClusterSecurityGroupNotFoundException
* The cluster security group name does not refer to an existing cluster security group.
* @throws ClusterParameterGroupNotFoundException
* The parameter group name does not refer to an existing parameter group.
* @throws InsufficientClusterCapacityException
* The number of nodes specified exceeds the allotted capacity of the cluster.
* @throws UnsupportedOptionException
* A request option was specified that is not supported.
* @throws UnauthorizedOperationException
* Your account is not authorized to perform the requested operation.
* @throws HsmClientCertificateNotFoundException
* There is no Amazon Redshift HSM client certificate with the specified identifier.
* @throws HsmConfigurationNotFoundException
* There is no Amazon Redshift HSM configuration with the specified identifier.
* @throws ClusterAlreadyExistsException
* The account already has a cluster with the given identifier.
* @throws LimitExceededException
* The encryption key has exceeded its grant limit in Amazon Web Services KMS.
* @throws DependentServiceRequestThrottlingException
* The request cannot be completed because a dependent service is throttling requests made by Amazon
* Redshift on your behalf. Wait and retry the request.
* @throws InvalidElasticIpException
* The Elastic IP (EIP) is invalid or cannot be found.
* @throws TableLimitExceededException
* The number of tables in the cluster exceeds the limit for the requested new cluster node type.
* @throws InvalidClusterTrackException
* The provided cluster track name is not valid.
* @throws InvalidRetentionPeriodException
* The retention period specified is either in the past or is not a valid value.
*
* The value must be either -1 or an integer between 1 and 3,653.
* @throws UnsupportedOperationException
* The requested operation isn't supported.
* @throws CustomCnameAssociationException
* An error occurred when an attempt was made to change the custom domain association.
* @throws Ipv6CidrBlockNotFoundException
* There are no subnets in your VPC with associated IPv6 CIDR blocks. To use dual-stack mode, associate an
* IPv6 CIDR block with each subnet in your VPC.
* @sample AmazonRedshift.ModifyCluster
* @see AWS API
* Documentation
*/
Cluster modifyCluster(ModifyClusterRequest modifyClusterRequest);
/**
*
* Modifies the database revision of a cluster. The database revision is a unique revision of the database running
* in a cluster.
*
*
* @param modifyClusterDbRevisionRequest
* @return Result of the ModifyClusterDbRevision operation returned by the service.
* @throws ClusterNotFoundException
* The ClusterIdentifier
parameter does not refer to an existing cluster.
* @throws ClusterOnLatestRevisionException
* Cluster is already on the latest database revision.
* @throws InvalidClusterStateException
* The specified cluster is not in the available
state.
* @throws UnsupportedOperationException
* The requested operation isn't supported.
* @sample AmazonRedshift.ModifyClusterDbRevision
* @see AWS API Documentation
*/
Cluster modifyClusterDbRevision(ModifyClusterDbRevisionRequest modifyClusterDbRevisionRequest);
/**
*
* Modifies the list of Identity and Access Management (IAM) roles that can be used by the cluster to access other
* Amazon Web Services services.
*
*
* The maximum number of IAM roles that you can associate is subject to a quota. For more information, go to Quotas and limits in the
* Amazon Redshift Cluster Management Guide.
*
*
* @param modifyClusterIamRolesRequest
* @return Result of the ModifyClusterIamRoles operation returned by the service.
* @throws InvalidClusterStateException
* The specified cluster is not in the available
state.
* @throws ClusterNotFoundException
* The ClusterIdentifier
parameter does not refer to an existing cluster.
* @sample AmazonRedshift.ModifyClusterIamRoles
* @see AWS
* API Documentation
*/
Cluster modifyClusterIamRoles(ModifyClusterIamRolesRequest modifyClusterIamRolesRequest);
/**
*
* Modifies the maintenance settings of a cluster.
*
*
* @param modifyClusterMaintenanceRequest
* @return Result of the ModifyClusterMaintenance operation returned by the service.
* @throws ClusterNotFoundException
* The ClusterIdentifier
parameter does not refer to an existing cluster.
* @throws InvalidClusterStateException
* The specified cluster is not in the available
state.
* @sample AmazonRedshift.ModifyClusterMaintenance
* @see AWS API Documentation
*/
Cluster modifyClusterMaintenance(ModifyClusterMaintenanceRequest modifyClusterMaintenanceRequest);
/**
*
* Modifies the parameters of a parameter group. For the parameters parameter, it can't contain ASCII characters.
*
*
* For more information about parameters and parameter groups, go to Amazon Redshift
* Parameter Groups in the Amazon Redshift Cluster Management Guide.
*
*
* @param modifyClusterParameterGroupRequest
* Describes a modify cluster parameter group operation.
* @return Result of the ModifyClusterParameterGroup operation returned by the service.
* @throws ClusterParameterGroupNotFoundException
* The parameter group name does not refer to an existing parameter group.
* @throws InvalidClusterParameterGroupStateException
* The cluster parameter group action can not be completed because another task is in progress that involves
* the parameter group. Wait a few moments and try the operation again.
* @sample AmazonRedshift.ModifyClusterParameterGroup
* @see AWS API Documentation
*/
ModifyClusterParameterGroupResult modifyClusterParameterGroup(ModifyClusterParameterGroupRequest modifyClusterParameterGroupRequest);
/**
*
* Modifies the settings for a snapshot.
*
*
* This exanmple modifies the manual retention period setting for a cluster snapshot.
*
*
* @param modifyClusterSnapshotRequest
* @return Result of the ModifyClusterSnapshot operation returned by the service.
* @throws InvalidClusterSnapshotStateException
* The specified cluster snapshot is not in the available
state, or other accounts are
* authorized to access the snapshot.
* @throws ClusterSnapshotNotFoundException
* The snapshot identifier does not refer to an existing cluster snapshot.
* @throws InvalidRetentionPeriodException
* The retention period specified is either in the past or is not a valid value.
*
* The value must be either -1 or an integer between 1 and 3,653.
* @sample AmazonRedshift.ModifyClusterSnapshot
* @see AWS
* API Documentation
*/
Snapshot modifyClusterSnapshot(ModifyClusterSnapshotRequest modifyClusterSnapshotRequest);
/**
*
* Modifies a snapshot schedule for a cluster.
*
*
* @param modifyClusterSnapshotScheduleRequest
* @return Result of the ModifyClusterSnapshotSchedule operation returned by the service.
* @throws ClusterNotFoundException
* The ClusterIdentifier
parameter does not refer to an existing cluster.
* @throws SnapshotScheduleNotFoundException
* We could not find the specified snapshot schedule.
* @throws InvalidClusterSnapshotScheduleStateException
* The cluster snapshot schedule state is not valid.
* @sample AmazonRedshift.ModifyClusterSnapshotSchedule
* @see AWS API Documentation
*/
ModifyClusterSnapshotScheduleResult modifyClusterSnapshotSchedule(ModifyClusterSnapshotScheduleRequest modifyClusterSnapshotScheduleRequest);
/**
*
* Modifies a cluster subnet group to include the specified list of VPC subnets. The operation replaces the existing
* list of subnets with the new list of subnets.
*
*
* @param modifyClusterSubnetGroupRequest
* @return Result of the ModifyClusterSubnetGroup operation returned by the service.
* @throws ClusterSubnetGroupNotFoundException
* The cluster subnet group name does not refer to an existing cluster subnet group.
* @throws ClusterSubnetQuotaExceededException
* The request would result in user exceeding the allowed number of subnets in a cluster subnet groups. For
* information about increasing your quota, go to Limits in Amazon
* Redshift in the Amazon Redshift Cluster Management Guide.
* @throws SubnetAlreadyInUseException
* A specified subnet is already in use by another cluster.
* @throws InvalidSubnetException
* The requested subnet is not valid, or not all of the subnets are in the same VPC.
* @throws UnauthorizedOperationException
* Your account is not authorized to perform the requested operation.
* @throws DependentServiceRequestThrottlingException
* The request cannot be completed because a dependent service is throttling requests made by Amazon
* Redshift on your behalf. Wait and retry the request.
* @sample AmazonRedshift.ModifyClusterSubnetGroup
* @see AWS API Documentation
*/
ClusterSubnetGroup modifyClusterSubnetGroup(ModifyClusterSubnetGroupRequest modifyClusterSubnetGroupRequest);
/**
*
* Contains information for changing a custom domain association.
*
*
* @param modifyCustomDomainAssociationRequest
* @return Result of the ModifyCustomDomainAssociation operation returned by the service.
* @throws UnsupportedOperationException
* The requested operation isn't supported.
* @throws ClusterNotFoundException
* The ClusterIdentifier
parameter does not refer to an existing cluster.
* @throws CustomCnameAssociationException
* An error occurred when an attempt was made to change the custom domain association.
* @throws CustomDomainAssociationNotFoundException
* An error occurred. The custom domain name couldn't be found.
* @sample AmazonRedshift.ModifyCustomDomainAssociation
* @see AWS API Documentation
*/
ModifyCustomDomainAssociationResult modifyCustomDomainAssociation(ModifyCustomDomainAssociationRequest modifyCustomDomainAssociationRequest);
/**
*
* Modifies a Redshift-managed VPC endpoint.
*
*
* @param modifyEndpointAccessRequest
* @return Result of the ModifyEndpointAccess operation returned by the service.
* @throws InvalidClusterSecurityGroupStateException
* The state of the cluster security group is not available
.
* @throws ClusterNotFoundException
* The ClusterIdentifier
parameter does not refer to an existing cluster.
* @throws InvalidEndpointStateException
* The status of the endpoint is not valid.
* @throws EndpointNotFoundException
* The endpoint name doesn't refer to an existing endpoint.
* @throws InvalidClusterStateException
* The specified cluster is not in the available
state.
* @throws UnauthorizedOperationException
* Your account is not authorized to perform the requested operation.
* @sample AmazonRedshift.ModifyEndpointAccess
* @see AWS
* API Documentation
*/
ModifyEndpointAccessResult modifyEndpointAccess(ModifyEndpointAccessRequest modifyEndpointAccessRequest);
/**
*
* Modifies an existing Amazon Redshift event notification subscription.
*
*
* @param modifyEventSubscriptionRequest
* @return Result of the ModifyEventSubscription operation returned by the service.
* @throws SubscriptionNotFoundException
* An Amazon Redshift event notification subscription with the specified name does not exist.
* @throws SNSInvalidTopicException
* Amazon SNS has responded that there is a problem with the specified Amazon SNS topic.
* @throws SNSNoAuthorizationException
* You do not have permission to publish to the specified Amazon SNS topic.
* @throws SNSTopicArnNotFoundException
* An Amazon SNS topic with the specified Amazon Resource Name (ARN) does not exist.
* @throws SubscriptionEventIdNotFoundException
* An Amazon Redshift event with the specified event ID does not exist.
* @throws SubscriptionCategoryNotFoundException
* The value specified for the event category was not one of the allowed values, or it specified a category
* that does not apply to the specified source type. The allowed values are Configuration, Management,
* Monitoring, and Security.
* @throws SubscriptionSeverityNotFoundException
* The value specified for the event severity was not one of the allowed values, or it specified a severity
* that does not apply to the specified source type. The allowed values are ERROR and INFO.
* @throws SourceNotFoundException
* The specified Amazon Redshift event source could not be found.
* @throws InvalidSubscriptionStateException
* The subscription request is invalid because it is a duplicate request. This subscription request is
* already in progress.
* @sample AmazonRedshift.ModifyEventSubscription
* @see AWS API Documentation
*/
EventSubscription modifyEventSubscription(ModifyEventSubscriptionRequest modifyEventSubscriptionRequest);
/**
*
* Changes an existing Amazon Redshift IAM Identity Center application.
*
*
* @param modifyRedshiftIdcApplicationRequest
* @return Result of the ModifyRedshiftIdcApplication operation returned by the service.
* @throws RedshiftIdcApplicationNotExistsException
* The application you attempted to find doesn't exist.
* @throws DependentServiceUnavailableException
* Your request cannot be completed because a dependent internal service is temporarily unavailable. Wait 30
* to 60 seconds and try again.
* @throws UnsupportedOperationException
* The requested operation isn't supported.
* @throws DependentServiceAccessDeniedException
* A dependent service denied access for the integration.
* @sample AmazonRedshift.ModifyRedshiftIdcApplication
* @see AWS API Documentation
*/
RedshiftIdcApplication modifyRedshiftIdcApplication(ModifyRedshiftIdcApplicationRequest modifyRedshiftIdcApplicationRequest);
/**
*
* Modifies a scheduled action.
*
*
* @param modifyScheduledActionRequest
* @return Result of the ModifyScheduledAction operation returned by the service.
* @throws ClusterNotFoundException
* The ClusterIdentifier
parameter does not refer to an existing cluster.
* @throws ScheduledActionNotFoundException
* The scheduled action cannot be found.
* @throws ScheduledActionTypeUnsupportedException
* The action type specified for a scheduled action is not supported.
* @throws InvalidScheduleException
* The schedule you submitted isn't valid.
* @throws InvalidScheduledActionException
* The scheduled action is not valid.
* @throws UnauthorizedOperationException
* Your account is not authorized to perform the requested operation.
* @throws UnsupportedOperationException
* The requested operation isn't supported.
* @sample AmazonRedshift.ModifyScheduledAction
* @see AWS
* API Documentation
*/
ModifyScheduledActionResult modifyScheduledAction(ModifyScheduledActionRequest modifyScheduledActionRequest);
/**
*
* Modifies the number of days to retain snapshots in the destination Amazon Web Services Region after they are
* copied from the source Amazon Web Services Region. By default, this operation only changes the retention period
* of copied automated snapshots. The retention periods for both new and existing copied automated snapshots are
* updated with the new retention period. You can set the manual option to change only the retention periods of
* copied manual snapshots. If you set this option, only newly copied manual snapshots have the new retention
* period.
*
*
* @param modifySnapshotCopyRetentionPeriodRequest
* @return Result of the ModifySnapshotCopyRetentionPeriod operation returned by the service.
* @throws ClusterNotFoundException
* The ClusterIdentifier
parameter does not refer to an existing cluster.
* @throws SnapshotCopyDisabledException
* Cross-region snapshot copy was temporarily disabled. Try your request again.
* @throws UnauthorizedOperationException
* Your account is not authorized to perform the requested operation.
* @throws InvalidClusterStateException
* The specified cluster is not in the available
state.
* @throws InvalidRetentionPeriodException
* The retention period specified is either in the past or is not a valid value.
*
* The value must be either -1 or an integer between 1 and 3,653.
* @sample AmazonRedshift.ModifySnapshotCopyRetentionPeriod
* @see AWS API Documentation
*/
Cluster modifySnapshotCopyRetentionPeriod(ModifySnapshotCopyRetentionPeriodRequest modifySnapshotCopyRetentionPeriodRequest);
/**
*
* Modifies a snapshot schedule. Any schedule associated with a cluster is modified asynchronously.
*
*
* @param modifySnapshotScheduleRequest
* @return Result of the ModifySnapshotSchedule operation returned by the service.
* @throws InvalidScheduleException
* The schedule you submitted isn't valid.
* @throws SnapshotScheduleNotFoundException
* We could not find the specified snapshot schedule.
* @throws SnapshotScheduleUpdateInProgressException
* The specified snapshot schedule is already being updated.
* @sample AmazonRedshift.ModifySnapshotSchedule
* @see AWS API Documentation
*/
ModifySnapshotScheduleResult modifySnapshotSchedule(ModifySnapshotScheduleRequest modifySnapshotScheduleRequest);
/**
*
* Modifies a usage limit in a cluster. You can't modify the feature type or period of a usage limit.
*
*
* @param modifyUsageLimitRequest
* @return Result of the ModifyUsageLimit operation returned by the service.
* @throws InvalidUsageLimitException
* The usage limit is not valid.
* @throws UsageLimitNotFoundException
* The usage limit identifier can't be found.
* @throws UnsupportedOperationException
* The requested operation isn't supported.
* @sample AmazonRedshift.ModifyUsageLimit
* @see AWS API
* Documentation
*/
ModifyUsageLimitResult modifyUsageLimit(ModifyUsageLimitRequest modifyUsageLimitRequest);
/**
*
* Pauses a cluster.
*
*
* @param pauseClusterRequest
* Describes a pause cluster operation. For example, a scheduled action to run the PauseCluster
* API operation.
* @return Result of the PauseCluster operation returned by the service.
* @throws ClusterNotFoundException
* The ClusterIdentifier
parameter does not refer to an existing cluster.
* @throws InvalidClusterStateException
* The specified cluster is not in the available
state.
* @throws UnsupportedOperationException
* The requested operation isn't supported.
* @sample AmazonRedshift.PauseCluster
* @see AWS API
* Documentation
*/
Cluster pauseCluster(PauseClusterRequest pauseClusterRequest);
/**
*
* Allows you to purchase reserved nodes. Amazon Redshift offers a predefined set of reserved node offerings. You
* can purchase one or more of the offerings. You can call the DescribeReservedNodeOfferings API to obtain
* the available reserved node offerings. You can call this API by providing a specific reserved node offering and
* the number of nodes you want to reserve.
*
*
* For more information about reserved node offerings, go to Purchasing Reserved
* Nodes in the Amazon Redshift Cluster Management Guide.
*
*
* @param purchaseReservedNodeOfferingRequest
* @return Result of the PurchaseReservedNodeOffering operation returned by the service.
* @throws ReservedNodeOfferingNotFoundException
* Specified offering does not exist.
* @throws ReservedNodeAlreadyExistsException
* User already has a reservation with the given identifier.
* @throws ReservedNodeQuotaExceededException
* Request would exceed the user's compute node quota. For information about increasing your quota, go to Limits in Amazon
* Redshift in the Amazon Redshift Cluster Management Guide.
* @throws UnsupportedOperationException
* The requested operation isn't supported.
* @sample AmazonRedshift.PurchaseReservedNodeOffering
* @see AWS API Documentation
*/
ReservedNode purchaseReservedNodeOffering(PurchaseReservedNodeOfferingRequest purchaseReservedNodeOfferingRequest);
/**
*
* Updates the resource policy for a specified resource.
*
*
* @param putResourcePolicyRequest
* @return Result of the PutResourcePolicy operation returned by the service.
* @throws ResourceNotFoundException
* The resource could not be found.
* @throws InvalidPolicyException
* The resource policy isn't valid.
* @throws ConflictPolicyUpdateException
* There is a conflict while updating the resource policy.
* @throws UnsupportedOperationException
* The requested operation isn't supported.
* @sample AmazonRedshift.PutResourcePolicy
* @see AWS API
* Documentation
*/
PutResourcePolicyResult putResourcePolicy(PutResourcePolicyRequest putResourcePolicyRequest);
/**
*
* Reboots a cluster. This action is taken as soon as possible. It results in a momentary outage to the cluster,
* during which the cluster status is set to rebooting
. A cluster event is created when the reboot is
* completed. Any pending cluster modifications (see ModifyCluster) are applied at this reboot. For more
* information about managing clusters, go to Amazon Redshift Clusters
* in the Amazon Redshift Cluster Management Guide.
*
*
* @param rebootClusterRequest
* @return Result of the RebootCluster operation returned by the service.
* @throws InvalidClusterStateException
* The specified cluster is not in the available
state.
* @throws ClusterNotFoundException
* The ClusterIdentifier
parameter does not refer to an existing cluster.
* @sample AmazonRedshift.RebootCluster
* @see AWS API
* Documentation
*/
Cluster rebootCluster(RebootClusterRequest rebootClusterRequest);
/**
*
* From a datashare consumer account, rejects the specified datashare.
*
*
* @param rejectDataShareRequest
* @return Result of the RejectDataShare operation returned by the service.
* @throws InvalidDataShareException
* There is an error with the datashare.
* @sample AmazonRedshift.RejectDataShare
* @see AWS API
* Documentation
*/
RejectDataShareResult rejectDataShare(RejectDataShareRequest rejectDataShareRequest);
/**
*
* Sets one or more parameters of the specified parameter group to their default values and sets the source values
* of the parameters to "engine-default". To reset the entire parameter group specify the ResetAllParameters
* parameter. For parameter changes to take effect you must reboot any associated clusters.
*
*
* @param resetClusterParameterGroupRequest
* @return Result of the ResetClusterParameterGroup operation returned by the service.
* @throws InvalidClusterParameterGroupStateException
* The cluster parameter group action can not be completed because another task is in progress that involves
* the parameter group. Wait a few moments and try the operation again.
* @throws ClusterParameterGroupNotFoundException
* The parameter group name does not refer to an existing parameter group.
* @sample AmazonRedshift.ResetClusterParameterGroup
* @see AWS API Documentation
*/
ResetClusterParameterGroupResult resetClusterParameterGroup(ResetClusterParameterGroupRequest resetClusterParameterGroupRequest);
/**
*
* Changes the size of the cluster. You can change the cluster's type, or change the number or type of nodes. The
* default behavior is to use the elastic resize method. With an elastic resize, your cluster is available for read
* and write operations more quickly than with the classic resize method.
*
*
* Elastic resize operations have the following restrictions:
*
*
* -
*
* You can only resize clusters of the following types:
*
*
* -
*
* dc2.large
*
*
* -
*
* dc2.8xlarge
*
*
* -
*
* ra3.xlplus
*
*
* -
*
* ra3.4xlarge
*
*
* -
*
* ra3.16xlarge
*
*
*
*
* -
*
* The type of nodes that you add must match the node type for the cluster.
*
*
*
*
* @param resizeClusterRequest
* Describes a resize cluster operation. For example, a scheduled action to run the
* ResizeCluster
API operation.
* @return Result of the ResizeCluster operation returned by the service.
* @throws InvalidClusterStateException
* The specified cluster is not in the available
state.
* @throws ClusterNotFoundException
* The ClusterIdentifier
parameter does not refer to an existing cluster.
* @throws NumberOfNodesQuotaExceededException
* The operation would exceed the number of nodes allotted to the account. For information about increasing
* your quota, go to Limits in Amazon
* Redshift in the Amazon Redshift Cluster Management Guide.
* @throws NumberOfNodesPerClusterLimitExceededException
* The operation would exceed the number of nodes allowed for a cluster.
* @throws InsufficientClusterCapacityException
* The number of nodes specified exceeds the allotted capacity of the cluster.
* @throws UnsupportedOptionException
* A request option was specified that is not supported.
* @throws UnsupportedOperationException
* The requested operation isn't supported.
* @throws UnauthorizedOperationException
* Your account is not authorized to perform the requested operation.
* @throws LimitExceededException
* The encryption key has exceeded its grant limit in Amazon Web Services KMS.
* @throws ReservedNodeNotFoundException
* The specified reserved compute node not found.
* @throws InvalidReservedNodeStateException
* Indicates that the Reserved Node being exchanged is not in an active state.
* @throws ReservedNodeAlreadyMigratedException
* Indicates that the reserved node has already been exchanged.
* @throws ReservedNodeOfferingNotFoundException
* Specified offering does not exist.
* @throws DependentServiceUnavailableException
* Your request cannot be completed because a dependent internal service is temporarily unavailable. Wait 30
* to 60 seconds and try again.
* @throws ReservedNodeAlreadyExistsException
* User already has a reservation with the given identifier.
* @sample AmazonRedshift.ResizeCluster
* @see AWS API
* Documentation
*/
Cluster resizeCluster(ResizeClusterRequest resizeClusterRequest);
/**
*
* Creates a new cluster from a snapshot. By default, Amazon Redshift creates the resulting cluster with the same
* configuration as the original cluster from which the snapshot was created, except that the new cluster is created
* with the default cluster security and parameter groups. After Amazon Redshift creates the cluster, you can use
* the ModifyCluster API to associate a different security group and different parameter group with the
* restored cluster. If you are using a DS node type, you can also choose to change to another DS node type of the
* same size during restore.
*
*
* If you restore a cluster into a VPC, you must provide a cluster subnet group where you want the cluster restored.
*
*
* For more information about working with snapshots, go to Amazon Redshift Snapshots
* in the Amazon Redshift Cluster Management Guide.
*
*
* @param restoreFromClusterSnapshotRequest
* @return Result of the RestoreFromClusterSnapshot operation returned by the service.
* @throws AccessToSnapshotDeniedException
* The owner of the specified snapshot has not authorized your account to access the snapshot.
* @throws ClusterAlreadyExistsException
* The account already has a cluster with the given identifier.
* @throws ClusterSnapshotNotFoundException
* The snapshot identifier does not refer to an existing cluster snapshot.
* @throws ClusterQuotaExceededException
* The request would exceed the allowed number of cluster instances for this account. For information about
* increasing your quota, go to Limits in Amazon
* Redshift in the Amazon Redshift Cluster Management Guide.
* @throws InsufficientClusterCapacityException
* The number of nodes specified exceeds the allotted capacity of the cluster.
* @throws InvalidClusterSnapshotStateException
* The specified cluster snapshot is not in the available
state, or other accounts are
* authorized to access the snapshot.
* @throws InvalidRestoreException
* The restore is invalid.
* @throws NumberOfNodesQuotaExceededException
* The operation would exceed the number of nodes allotted to the account. For information about increasing
* your quota, go to Limits in Amazon
* Redshift in the Amazon Redshift Cluster Management Guide.
* @throws NumberOfNodesPerClusterLimitExceededException
* The operation would exceed the number of nodes allowed for a cluster.
* @throws InvalidVPCNetworkStateException
* The cluster subnet group does not cover all Availability Zones.
* @throws InvalidClusterSubnetGroupStateException
* The cluster subnet group cannot be deleted because it is in use.
* @throws InvalidSubnetException
* The requested subnet is not valid, or not all of the subnets are in the same VPC.
* @throws ClusterSubnetGroupNotFoundException
* The cluster subnet group name does not refer to an existing cluster subnet group.
* @throws UnauthorizedOperationException
* Your account is not authorized to perform the requested operation.
* @throws HsmClientCertificateNotFoundException
* There is no Amazon Redshift HSM client certificate with the specified identifier.
* @throws HsmConfigurationNotFoundException
* There is no Amazon Redshift HSM configuration with the specified identifier.
* @throws InvalidElasticIpException
* The Elastic IP (EIP) is invalid or cannot be found.
* @throws ClusterParameterGroupNotFoundException
* The parameter group name does not refer to an existing parameter group.
* @throws ClusterSecurityGroupNotFoundException
* The cluster security group name does not refer to an existing cluster security group.
* @throws LimitExceededException
* The encryption key has exceeded its grant limit in Amazon Web Services KMS.
* @throws DependentServiceRequestThrottlingException
* The request cannot be completed because a dependent service is throttling requests made by Amazon
* Redshift on your behalf. Wait and retry the request.
* @throws InvalidClusterTrackException
* The provided cluster track name is not valid.
* @throws SnapshotScheduleNotFoundException
* We could not find the specified snapshot schedule.
* @throws TagLimitExceededException
* You have exceeded the number of tags allowed.
* @throws InvalidTagException
* The tag is invalid.
* @throws ReservedNodeNotFoundException
* The specified reserved compute node not found.
* @throws InvalidReservedNodeStateException
* Indicates that the Reserved Node being exchanged is not in an active state.
* @throws ReservedNodeAlreadyMigratedException
* Indicates that the reserved node has already been exchanged.
* @throws ReservedNodeOfferingNotFoundException
* Specified offering does not exist.
* @throws DependentServiceUnavailableException
* Your request cannot be completed because a dependent internal service is temporarily unavailable. Wait 30
* to 60 seconds and try again.
* @throws ReservedNodeAlreadyExistsException
* User already has a reservation with the given identifier.
* @throws UnsupportedOperationException
* The requested operation isn't supported.
* @throws Ipv6CidrBlockNotFoundException
* There are no subnets in your VPC with associated IPv6 CIDR blocks. To use dual-stack mode, associate an
* IPv6 CIDR block with each subnet in your VPC.
* @sample AmazonRedshift.RestoreFromClusterSnapshot
* @see AWS API Documentation
*/
Cluster restoreFromClusterSnapshot(RestoreFromClusterSnapshotRequest restoreFromClusterSnapshotRequest);
/**
*
* Creates a new table from a table in an Amazon Redshift cluster snapshot. You must create the new table within the
* Amazon Redshift cluster that the snapshot was taken from.
*
*
* You cannot use RestoreTableFromClusterSnapshot
to restore a table with the same name as an existing
* table in an Amazon Redshift cluster. That is, you cannot overwrite an existing table in a cluster with a restored
* table. If you want to replace your original table with a new, restored table, then rename or drop your original
* table before you call RestoreTableFromClusterSnapshot
. When you have renamed your original table,
* then you can pass the original name of the table as the NewTableName
parameter value in the call to
* RestoreTableFromClusterSnapshot
. This way, you can replace the original table with the table created
* from the snapshot.
*
*
* You can't use this operation to restore tables with interleaved
* sort keys.
*
*
* @param restoreTableFromClusterSnapshotRequest
* @return Result of the RestoreTableFromClusterSnapshot operation returned by the service.
* @throws ClusterSnapshotNotFoundException
* The snapshot identifier does not refer to an existing cluster snapshot.
* @throws InProgressTableRestoreQuotaExceededException
* You have exceeded the allowed number of table restore requests. Wait for your current table restore
* requests to complete before making a new request.
* @throws InvalidClusterSnapshotStateException
* The specified cluster snapshot is not in the available
state, or other accounts are
* authorized to access the snapshot.
* @throws InvalidTableRestoreArgumentException
* The value specified for the sourceDatabaseName
, sourceSchemaName
, or
* sourceTableName
parameter, or a combination of these, doesn't exist in the snapshot.
* @throws ClusterNotFoundException
* The ClusterIdentifier
parameter does not refer to an existing cluster.
* @throws InvalidClusterStateException
* The specified cluster is not in the available
state.
* @throws UnsupportedOperationException
* The requested operation isn't supported.
* @sample AmazonRedshift.RestoreTableFromClusterSnapshot
* @see AWS API Documentation
*/
TableRestoreStatus restoreTableFromClusterSnapshot(RestoreTableFromClusterSnapshotRequest restoreTableFromClusterSnapshotRequest);
/**
*
* Resumes a paused cluster.
*
*
* @param resumeClusterRequest
* Describes a resume cluster operation. For example, a scheduled action to run the
* ResumeCluster
API operation.
* @return Result of the ResumeCluster operation returned by the service.
* @throws ClusterNotFoundException
* The ClusterIdentifier
parameter does not refer to an existing cluster.
* @throws InvalidClusterStateException
* The specified cluster is not in the available
state.
* @throws InsufficientClusterCapacityException
* The number of nodes specified exceeds the allotted capacity of the cluster.
* @throws UnsupportedOperationException
* The requested operation isn't supported.
* @sample AmazonRedshift.ResumeCluster
* @see AWS API
* Documentation
*/
Cluster resumeCluster(ResumeClusterRequest resumeClusterRequest);
/**
*
* Revokes an ingress rule in an Amazon Redshift security group for a previously authorized IP range or Amazon EC2
* security group. To add an ingress rule, see AuthorizeClusterSecurityGroupIngress. For information about
* managing security groups, go to Amazon Redshift Cluster
* Security Groups in the Amazon Redshift Cluster Management Guide.
*
*
* @param revokeClusterSecurityGroupIngressRequest
* @return Result of the RevokeClusterSecurityGroupIngress operation returned by the service.
* @throws ClusterSecurityGroupNotFoundException
* The cluster security group name does not refer to an existing cluster security group.
* @throws AuthorizationNotFoundException
* The specified CIDR IP range or EC2 security group is not authorized for the specified cluster security
* group.
* @throws InvalidClusterSecurityGroupStateException
* The state of the cluster security group is not available
.
* @sample AmazonRedshift.RevokeClusterSecurityGroupIngress
* @see AWS API Documentation
*/
ClusterSecurityGroup revokeClusterSecurityGroupIngress(RevokeClusterSecurityGroupIngressRequest revokeClusterSecurityGroupIngressRequest);
/**
*
* Revokes access to a cluster.
*
*
* @param revokeEndpointAccessRequest
* @return Result of the RevokeEndpointAccess operation returned by the service.
* @throws ClusterNotFoundException
* The ClusterIdentifier
parameter does not refer to an existing cluster.
* @throws InvalidEndpointStateException
* The status of the endpoint is not valid.
* @throws InvalidClusterSecurityGroupStateException
* The state of the cluster security group is not available
.
* @throws EndpointNotFoundException
* The endpoint name doesn't refer to an existing endpoint.
* @throws EndpointAuthorizationNotFoundException
* The authorization for this endpoint can't be found.
* @throws InvalidAuthorizationStateException
* The status of the authorization is not valid.
* @throws InvalidClusterStateException
* The specified cluster is not in the available
state.
* @sample AmazonRedshift.RevokeEndpointAccess
* @see AWS
* API Documentation
*/
RevokeEndpointAccessResult revokeEndpointAccess(RevokeEndpointAccessRequest revokeEndpointAccessRequest);
/**
*
* Removes the ability of the specified Amazon Web Services account to restore the specified snapshot. If the
* account is currently restoring the snapshot, the restore will run to completion.
*
*
* For more information about working with snapshots, go to Amazon Redshift Snapshots
* in the Amazon Redshift Cluster Management Guide.
*
*
* @param revokeSnapshotAccessRequest
* @return Result of the RevokeSnapshotAccess operation returned by the service.
* @throws AccessToSnapshotDeniedException
* The owner of the specified snapshot has not authorized your account to access the snapshot.
* @throws AuthorizationNotFoundException
* The specified CIDR IP range or EC2 security group is not authorized for the specified cluster security
* group.
* @throws ClusterSnapshotNotFoundException
* The snapshot identifier does not refer to an existing cluster snapshot.
* @throws UnsupportedOperationException
* The requested operation isn't supported.
* @sample AmazonRedshift.RevokeSnapshotAccess
* @see AWS
* API Documentation
*/
Snapshot revokeSnapshotAccess(RevokeSnapshotAccessRequest revokeSnapshotAccessRequest);
/**
*
* Rotates the encryption keys for a cluster.
*
*
* @param rotateEncryptionKeyRequest
* @return Result of the RotateEncryptionKey operation returned by the service.
* @throws ClusterNotFoundException
* The ClusterIdentifier
parameter does not refer to an existing cluster.
* @throws InvalidClusterStateException
* The specified cluster is not in the available
state.
* @throws DependentServiceRequestThrottlingException
* The request cannot be completed because a dependent service is throttling requests made by Amazon
* Redshift on your behalf. Wait and retry the request.
* @throws UnsupportedOperationException
* The requested operation isn't supported.
* @sample AmazonRedshift.RotateEncryptionKey
* @see AWS
* API Documentation
*/
Cluster rotateEncryptionKey(RotateEncryptionKeyRequest rotateEncryptionKeyRequest);
/**
*
* Updates the status of a partner integration.
*
*
* @param updatePartnerStatusRequest
* @return Result of the UpdatePartnerStatus operation returned by the service.
* @throws PartnerNotFoundException
* The name of the partner was not found.
* @throws ClusterNotFoundException
* The ClusterIdentifier
parameter does not refer to an existing cluster.
* @throws UnauthorizedPartnerIntegrationException
* The partner integration is not authorized.
* @throws UnsupportedOperationException
* The requested operation isn't supported.
* @sample AmazonRedshift.UpdatePartnerStatus
* @see AWS
* API Documentation
*/
UpdatePartnerStatusResult updatePartnerStatus(UpdatePartnerStatusRequest updatePartnerStatusRequest);
/**
* Shuts down this client object, releasing any resources that might be held open. This is an optional method, and
* callers are not expected to call it, but can if they want to explicitly release any open resources. Once a client
* has been shutdown, it should not be used to make any more requests.
*/
void shutdown();
/**
* Returns additional metadata for a previously executed successful request, typically used for debugging issues
* where a service isn't acting as expected. This data isn't considered part of the result data returned by an
* operation, so it's available through this separate, diagnostic interface.
*
* Response metadata is only cached for a limited period of time, so if you need to access this extra diagnostic
* information for an executed request, you should use this method to retrieve it as soon as possible after
* executing a request.
*
* @param request
* The originally executed request.
*
* @return The response metadata for the specified request, or null if none is available.
*/
ResponseMetadata getCachedResponseMetadata(AmazonWebServiceRequest request);
AmazonRedshiftWaiters waiters();
}