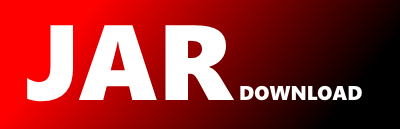
com.amazonaws.services.redshift.AmazonRedshiftAsync Maven / Gradle / Ivy
Show all versions of aws-java-sdk-redshift Show documentation
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.redshift;
import javax.annotation.Generated;
import com.amazonaws.services.redshift.model.*;
/**
* Interface for accessing Amazon Redshift asynchronously. Each asynchronous method will return a Java Future object
* representing the asynchronous operation; overloads which accept an {@code AsyncHandler} can be used to receive
* notification when an asynchronous operation completes.
*
* Note: Do not directly implement this interface, new methods are added to it regularly. Extend from
* {@link com.amazonaws.services.redshift.AbstractAmazonRedshiftAsync} instead.
*
*
* Amazon Redshift
*
* Overview
*
*
* This is an interface reference for Amazon Redshift. It contains documentation for one of the programming or command
* line interfaces you can use to manage Amazon Redshift clusters. Note that Amazon Redshift is asynchronous, which
* means that some interfaces may require techniques, such as polling or asynchronous callback handlers, to determine
* when a command has been applied. In this reference, the parameter descriptions indicate whether a change is applied
* immediately, on the next instance reboot, or during the next maintenance window. For a summary of the Amazon Redshift
* cluster management interfaces, go to Using the Amazon Redshift Management
* Interfaces.
*
*
* Amazon Redshift manages all the work of setting up, operating, and scaling a data warehouse: provisioning capacity,
* monitoring and backing up the cluster, and applying patches and upgrades to the Amazon Redshift engine. You can focus
* on using your data to acquire new insights for your business and customers.
*
*
* If you are a first-time user of Amazon Redshift, we recommend that you begin by reading the Amazon Redshift Getting Started
* Guide.
*
*
* If you are a database developer, the Amazon
* Redshift Database Developer Guide explains how to design, build, query, and maintain the databases that make up
* your data warehouse.
*
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public interface AmazonRedshiftAsync extends AmazonRedshift {
/**
*
* Exchanges a DC1 Reserved Node for a DC2 Reserved Node with no changes to the configuration (term, payment type,
* or number of nodes) and no additional costs.
*
*
* @param acceptReservedNodeExchangeRequest
* @return A Java Future containing the result of the AcceptReservedNodeExchange operation returned by the service.
* @sample AmazonRedshiftAsync.AcceptReservedNodeExchange
* @see AWS API Documentation
*/
java.util.concurrent.Future acceptReservedNodeExchangeAsync(AcceptReservedNodeExchangeRequest acceptReservedNodeExchangeRequest);
/**
*
* Exchanges a DC1 Reserved Node for a DC2 Reserved Node with no changes to the configuration (term, payment type,
* or number of nodes) and no additional costs.
*
*
* @param acceptReservedNodeExchangeRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the AcceptReservedNodeExchange operation returned by the service.
* @sample AmazonRedshiftAsyncHandler.AcceptReservedNodeExchange
* @see AWS API Documentation
*/
java.util.concurrent.Future acceptReservedNodeExchangeAsync(AcceptReservedNodeExchangeRequest acceptReservedNodeExchangeRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Adds a partner integration to a cluster. This operation authorizes a partner to push status updates for the
* specified database. To complete the integration, you also set up the integration on the partner website.
*
*
* @param addPartnerRequest
* @return A Java Future containing the result of the AddPartner operation returned by the service.
* @sample AmazonRedshiftAsync.AddPartner
* @see AWS API
* Documentation
*/
java.util.concurrent.Future addPartnerAsync(AddPartnerRequest addPartnerRequest);
/**
*
* Adds a partner integration to a cluster. This operation authorizes a partner to push status updates for the
* specified database. To complete the integration, you also set up the integration on the partner website.
*
*
* @param addPartnerRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the AddPartner operation returned by the service.
* @sample AmazonRedshiftAsyncHandler.AddPartner
* @see AWS API
* Documentation
*/
java.util.concurrent.Future addPartnerAsync(AddPartnerRequest addPartnerRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* From a datashare consumer account, associates a datashare with the account (AssociateEntireAccount) or the
* specified namespace (ConsumerArn). If you make this association, the consumer can consume the datashare.
*
*
* @param associateDataShareConsumerRequest
* @return A Java Future containing the result of the AssociateDataShareConsumer operation returned by the service.
* @sample AmazonRedshiftAsync.AssociateDataShareConsumer
* @see AWS API Documentation
*/
java.util.concurrent.Future associateDataShareConsumerAsync(
AssociateDataShareConsumerRequest associateDataShareConsumerRequest);
/**
*
* From a datashare consumer account, associates a datashare with the account (AssociateEntireAccount) or the
* specified namespace (ConsumerArn). If you make this association, the consumer can consume the datashare.
*
*
* @param associateDataShareConsumerRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the AssociateDataShareConsumer operation returned by the service.
* @sample AmazonRedshiftAsyncHandler.AssociateDataShareConsumer
* @see AWS API Documentation
*/
java.util.concurrent.Future associateDataShareConsumerAsync(
AssociateDataShareConsumerRequest associateDataShareConsumerRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Adds an inbound (ingress) rule to an Amazon Redshift security group. Depending on whether the application
* accessing your cluster is running on the Internet or an Amazon EC2 instance, you can authorize inbound access to
* either a Classless Interdomain Routing (CIDR)/Internet Protocol (IP) range or to an Amazon EC2 security group.
* You can add as many as 20 ingress rules to an Amazon Redshift security group.
*
*
* If you authorize access to an Amazon EC2 security group, specify EC2SecurityGroupName and
* EC2SecurityGroupOwnerId. The Amazon EC2 security group and Amazon Redshift cluster must be in the same
* Amazon Web Services Region.
*
*
* If you authorize access to a CIDR/IP address range, specify CIDRIP. For an overview of CIDR blocks, see
* the Wikipedia article on Classless
* Inter-Domain Routing.
*
*
* You must also associate the security group with a cluster so that clients running on these IP addresses or the
* EC2 instance are authorized to connect to the cluster. For information about managing security groups, go to Working with Security
* Groups in the Amazon Redshift Cluster Management Guide.
*
*
* @param authorizeClusterSecurityGroupIngressRequest
* @return A Java Future containing the result of the AuthorizeClusterSecurityGroupIngress operation returned by the
* service.
* @sample AmazonRedshiftAsync.AuthorizeClusterSecurityGroupIngress
* @see AWS API Documentation
*/
java.util.concurrent.Future authorizeClusterSecurityGroupIngressAsync(
AuthorizeClusterSecurityGroupIngressRequest authorizeClusterSecurityGroupIngressRequest);
/**
*
* Adds an inbound (ingress) rule to an Amazon Redshift security group. Depending on whether the application
* accessing your cluster is running on the Internet or an Amazon EC2 instance, you can authorize inbound access to
* either a Classless Interdomain Routing (CIDR)/Internet Protocol (IP) range or to an Amazon EC2 security group.
* You can add as many as 20 ingress rules to an Amazon Redshift security group.
*
*
* If you authorize access to an Amazon EC2 security group, specify EC2SecurityGroupName and
* EC2SecurityGroupOwnerId. The Amazon EC2 security group and Amazon Redshift cluster must be in the same
* Amazon Web Services Region.
*
*
* If you authorize access to a CIDR/IP address range, specify CIDRIP. For an overview of CIDR blocks, see
* the Wikipedia article on Classless
* Inter-Domain Routing.
*
*
* You must also associate the security group with a cluster so that clients running on these IP addresses or the
* EC2 instance are authorized to connect to the cluster. For information about managing security groups, go to Working with Security
* Groups in the Amazon Redshift Cluster Management Guide.
*
*
* @param authorizeClusterSecurityGroupIngressRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the AuthorizeClusterSecurityGroupIngress operation returned by the
* service.
* @sample AmazonRedshiftAsyncHandler.AuthorizeClusterSecurityGroupIngress
* @see AWS API Documentation
*/
java.util.concurrent.Future authorizeClusterSecurityGroupIngressAsync(
AuthorizeClusterSecurityGroupIngressRequest authorizeClusterSecurityGroupIngressRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* From a data producer account, authorizes the sharing of a datashare with one or more consumer accounts or
* managing entities. To authorize a datashare for a data consumer, the producer account must have the correct
* access permissions.
*
*
* @param authorizeDataShareRequest
* @return A Java Future containing the result of the AuthorizeDataShare operation returned by the service.
* @sample AmazonRedshiftAsync.AuthorizeDataShare
* @see AWS
* API Documentation
*/
java.util.concurrent.Future authorizeDataShareAsync(AuthorizeDataShareRequest authorizeDataShareRequest);
/**
*
* From a data producer account, authorizes the sharing of a datashare with one or more consumer accounts or
* managing entities. To authorize a datashare for a data consumer, the producer account must have the correct
* access permissions.
*
*
* @param authorizeDataShareRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the AuthorizeDataShare operation returned by the service.
* @sample AmazonRedshiftAsyncHandler.AuthorizeDataShare
* @see AWS
* API Documentation
*/
java.util.concurrent.Future authorizeDataShareAsync(AuthorizeDataShareRequest authorizeDataShareRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Grants access to a cluster.
*
*
* @param authorizeEndpointAccessRequest
* @return A Java Future containing the result of the AuthorizeEndpointAccess operation returned by the service.
* @sample AmazonRedshiftAsync.AuthorizeEndpointAccess
* @see AWS API Documentation
*/
java.util.concurrent.Future authorizeEndpointAccessAsync(AuthorizeEndpointAccessRequest authorizeEndpointAccessRequest);
/**
*
* Grants access to a cluster.
*
*
* @param authorizeEndpointAccessRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the AuthorizeEndpointAccess operation returned by the service.
* @sample AmazonRedshiftAsyncHandler.AuthorizeEndpointAccess
* @see AWS API Documentation
*/
java.util.concurrent.Future authorizeEndpointAccessAsync(AuthorizeEndpointAccessRequest authorizeEndpointAccessRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Authorizes the specified Amazon Web Services account to restore the specified snapshot.
*
*
* For more information about working with snapshots, go to Amazon Redshift Snapshots
* in the Amazon Redshift Cluster Management Guide.
*
*
* @param authorizeSnapshotAccessRequest
* @return A Java Future containing the result of the AuthorizeSnapshotAccess operation returned by the service.
* @sample AmazonRedshiftAsync.AuthorizeSnapshotAccess
* @see AWS API Documentation
*/
java.util.concurrent.Future authorizeSnapshotAccessAsync(AuthorizeSnapshotAccessRequest authorizeSnapshotAccessRequest);
/**
*
* Authorizes the specified Amazon Web Services account to restore the specified snapshot.
*
*
* For more information about working with snapshots, go to Amazon Redshift Snapshots
* in the Amazon Redshift Cluster Management Guide.
*
*
* @param authorizeSnapshotAccessRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the AuthorizeSnapshotAccess operation returned by the service.
* @sample AmazonRedshiftAsyncHandler.AuthorizeSnapshotAccess
* @see AWS API Documentation
*/
java.util.concurrent.Future authorizeSnapshotAccessAsync(AuthorizeSnapshotAccessRequest authorizeSnapshotAccessRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deletes a set of cluster snapshots.
*
*
* @param batchDeleteClusterSnapshotsRequest
* @return A Java Future containing the result of the BatchDeleteClusterSnapshots operation returned by the service.
* @sample AmazonRedshiftAsync.BatchDeleteClusterSnapshots
* @see AWS API Documentation
*/
java.util.concurrent.Future batchDeleteClusterSnapshotsAsync(
BatchDeleteClusterSnapshotsRequest batchDeleteClusterSnapshotsRequest);
/**
*
* Deletes a set of cluster snapshots.
*
*
* @param batchDeleteClusterSnapshotsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the BatchDeleteClusterSnapshots operation returned by the service.
* @sample AmazonRedshiftAsyncHandler.BatchDeleteClusterSnapshots
* @see AWS API Documentation
*/
java.util.concurrent.Future batchDeleteClusterSnapshotsAsync(
BatchDeleteClusterSnapshotsRequest batchDeleteClusterSnapshotsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Modifies the settings for a set of cluster snapshots.
*
*
* @param batchModifyClusterSnapshotsRequest
* @return A Java Future containing the result of the BatchModifyClusterSnapshots operation returned by the service.
* @sample AmazonRedshiftAsync.BatchModifyClusterSnapshots
* @see AWS API Documentation
*/
java.util.concurrent.Future batchModifyClusterSnapshotsAsync(
BatchModifyClusterSnapshotsRequest batchModifyClusterSnapshotsRequest);
/**
*
* Modifies the settings for a set of cluster snapshots.
*
*
* @param batchModifyClusterSnapshotsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the BatchModifyClusterSnapshots operation returned by the service.
* @sample AmazonRedshiftAsyncHandler.BatchModifyClusterSnapshots
* @see AWS API Documentation
*/
java.util.concurrent.Future batchModifyClusterSnapshotsAsync(
BatchModifyClusterSnapshotsRequest batchModifyClusterSnapshotsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Cancels a resize operation for a cluster.
*
*
* @param cancelResizeRequest
* @return A Java Future containing the result of the CancelResize operation returned by the service.
* @sample AmazonRedshiftAsync.CancelResize
* @see AWS API
* Documentation
*/
java.util.concurrent.Future cancelResizeAsync(CancelResizeRequest cancelResizeRequest);
/**
*
* Cancels a resize operation for a cluster.
*
*
* @param cancelResizeRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CancelResize operation returned by the service.
* @sample AmazonRedshiftAsyncHandler.CancelResize
* @see AWS API
* Documentation
*/
java.util.concurrent.Future cancelResizeAsync(CancelResizeRequest cancelResizeRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Copies the specified automated cluster snapshot to a new manual cluster snapshot. The source must be an automated
* snapshot and it must be in the available state.
*
*
* When you delete a cluster, Amazon Redshift deletes any automated snapshots of the cluster. Also, when the
* retention period of the snapshot expires, Amazon Redshift automatically deletes it. If you want to keep an
* automated snapshot for a longer period, you can make a manual copy of the snapshot. Manual snapshots are retained
* until you delete them.
*
*
* For more information about working with snapshots, go to Amazon Redshift Snapshots
* in the Amazon Redshift Cluster Management Guide.
*
*
* @param copyClusterSnapshotRequest
* @return A Java Future containing the result of the CopyClusterSnapshot operation returned by the service.
* @sample AmazonRedshiftAsync.CopyClusterSnapshot
* @see AWS
* API Documentation
*/
java.util.concurrent.Future copyClusterSnapshotAsync(CopyClusterSnapshotRequest copyClusterSnapshotRequest);
/**
*
* Copies the specified automated cluster snapshot to a new manual cluster snapshot. The source must be an automated
* snapshot and it must be in the available state.
*
*
* When you delete a cluster, Amazon Redshift deletes any automated snapshots of the cluster. Also, when the
* retention period of the snapshot expires, Amazon Redshift automatically deletes it. If you want to keep an
* automated snapshot for a longer period, you can make a manual copy of the snapshot. Manual snapshots are retained
* until you delete them.
*
*
* For more information about working with snapshots, go to Amazon Redshift Snapshots
* in the Amazon Redshift Cluster Management Guide.
*
*
* @param copyClusterSnapshotRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CopyClusterSnapshot operation returned by the service.
* @sample AmazonRedshiftAsyncHandler.CopyClusterSnapshot
* @see AWS
* API Documentation
*/
java.util.concurrent.Future copyClusterSnapshotAsync(CopyClusterSnapshotRequest copyClusterSnapshotRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates an authentication profile with the specified parameters.
*
*
* @param createAuthenticationProfileRequest
* @return A Java Future containing the result of the CreateAuthenticationProfile operation returned by the service.
* @sample AmazonRedshiftAsync.CreateAuthenticationProfile
* @see AWS API Documentation
*/
java.util.concurrent.Future createAuthenticationProfileAsync(
CreateAuthenticationProfileRequest createAuthenticationProfileRequest);
/**
*
* Creates an authentication profile with the specified parameters.
*
*
* @param createAuthenticationProfileRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateAuthenticationProfile operation returned by the service.
* @sample AmazonRedshiftAsyncHandler.CreateAuthenticationProfile
* @see AWS API Documentation
*/
java.util.concurrent.Future createAuthenticationProfileAsync(
CreateAuthenticationProfileRequest createAuthenticationProfileRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates a new cluster with the specified parameters.
*
*
* To create a cluster in Virtual Private Cloud (VPC), you must provide a cluster subnet group name. The cluster
* subnet group identifies the subnets of your VPC that Amazon Redshift uses when creating the cluster. For more
* information about managing clusters, go to Amazon Redshift Clusters
* in the Amazon Redshift Cluster Management Guide.
*
*
* @param createClusterRequest
* @return A Java Future containing the result of the CreateCluster operation returned by the service.
* @sample AmazonRedshiftAsync.CreateCluster
* @see AWS API
* Documentation
*/
java.util.concurrent.Future createClusterAsync(CreateClusterRequest createClusterRequest);
/**
*
* Creates a new cluster with the specified parameters.
*
*
* To create a cluster in Virtual Private Cloud (VPC), you must provide a cluster subnet group name. The cluster
* subnet group identifies the subnets of your VPC that Amazon Redshift uses when creating the cluster. For more
* information about managing clusters, go to Amazon Redshift Clusters
* in the Amazon Redshift Cluster Management Guide.
*
*
* @param createClusterRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateCluster operation returned by the service.
* @sample AmazonRedshiftAsyncHandler.CreateCluster
* @see AWS API
* Documentation
*/
java.util.concurrent.Future createClusterAsync(CreateClusterRequest createClusterRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates an Amazon Redshift parameter group.
*
*
* Creating parameter groups is independent of creating clusters. You can associate a cluster with a parameter group
* when you create the cluster. You can also associate an existing cluster with a parameter group after the cluster
* is created by using ModifyCluster.
*
*
* Parameters in the parameter group define specific behavior that applies to the databases you create on the
* cluster. For more information about parameters and parameter groups, go to Amazon Redshift
* Parameter Groups in the Amazon Redshift Cluster Management Guide.
*
*
* @param createClusterParameterGroupRequest
* @return A Java Future containing the result of the CreateClusterParameterGroup operation returned by the service.
* @sample AmazonRedshiftAsync.CreateClusterParameterGroup
* @see AWS API Documentation
*/
java.util.concurrent.Future createClusterParameterGroupAsync(CreateClusterParameterGroupRequest createClusterParameterGroupRequest);
/**
*
* Creates an Amazon Redshift parameter group.
*
*
* Creating parameter groups is independent of creating clusters. You can associate a cluster with a parameter group
* when you create the cluster. You can also associate an existing cluster with a parameter group after the cluster
* is created by using ModifyCluster.
*
*
* Parameters in the parameter group define specific behavior that applies to the databases you create on the
* cluster. For more information about parameters and parameter groups, go to Amazon Redshift
* Parameter Groups in the Amazon Redshift Cluster Management Guide.
*
*
* @param createClusterParameterGroupRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateClusterParameterGroup operation returned by the service.
* @sample AmazonRedshiftAsyncHandler.CreateClusterParameterGroup
* @see AWS API Documentation
*/
java.util.concurrent.Future createClusterParameterGroupAsync(CreateClusterParameterGroupRequest createClusterParameterGroupRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates a new Amazon Redshift security group. You use security groups to control access to non-VPC clusters.
*
*
* For information about managing security groups, go to Amazon Redshift Cluster
* Security Groups in the Amazon Redshift Cluster Management Guide.
*
*
* @param createClusterSecurityGroupRequest
* @return A Java Future containing the result of the CreateClusterSecurityGroup operation returned by the service.
* @sample AmazonRedshiftAsync.CreateClusterSecurityGroup
* @see AWS API Documentation
*/
java.util.concurrent.Future createClusterSecurityGroupAsync(CreateClusterSecurityGroupRequest createClusterSecurityGroupRequest);
/**
*
* Creates a new Amazon Redshift security group. You use security groups to control access to non-VPC clusters.
*
*
* For information about managing security groups, go to Amazon Redshift Cluster
* Security Groups in the Amazon Redshift Cluster Management Guide.
*
*
* @param createClusterSecurityGroupRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateClusterSecurityGroup operation returned by the service.
* @sample AmazonRedshiftAsyncHandler.CreateClusterSecurityGroup
* @see AWS API Documentation
*/
java.util.concurrent.Future createClusterSecurityGroupAsync(CreateClusterSecurityGroupRequest createClusterSecurityGroupRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates a manual snapshot of the specified cluster. The cluster must be in the available
state.
*
*
* For more information about working with snapshots, go to Amazon Redshift Snapshots
* in the Amazon Redshift Cluster Management Guide.
*
*
* @param createClusterSnapshotRequest
* @return A Java Future containing the result of the CreateClusterSnapshot operation returned by the service.
* @sample AmazonRedshiftAsync.CreateClusterSnapshot
* @see AWS
* API Documentation
*/
java.util.concurrent.Future createClusterSnapshotAsync(CreateClusterSnapshotRequest createClusterSnapshotRequest);
/**
*
* Creates a manual snapshot of the specified cluster. The cluster must be in the available
state.
*
*
* For more information about working with snapshots, go to Amazon Redshift Snapshots
* in the Amazon Redshift Cluster Management Guide.
*
*
* @param createClusterSnapshotRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateClusterSnapshot operation returned by the service.
* @sample AmazonRedshiftAsyncHandler.CreateClusterSnapshot
* @see AWS
* API Documentation
*/
java.util.concurrent.Future createClusterSnapshotAsync(CreateClusterSnapshotRequest createClusterSnapshotRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates a new Amazon Redshift subnet group. You must provide a list of one or more subnets in your existing
* Amazon Virtual Private Cloud (Amazon VPC) when creating Amazon Redshift subnet group.
*
*
* For information about subnet groups, go to Amazon Redshift
* Cluster Subnet Groups in the Amazon Redshift Cluster Management Guide.
*
*
* @param createClusterSubnetGroupRequest
* @return A Java Future containing the result of the CreateClusterSubnetGroup operation returned by the service.
* @sample AmazonRedshiftAsync.CreateClusterSubnetGroup
* @see AWS API Documentation
*/
java.util.concurrent.Future createClusterSubnetGroupAsync(CreateClusterSubnetGroupRequest createClusterSubnetGroupRequest);
/**
*
* Creates a new Amazon Redshift subnet group. You must provide a list of one or more subnets in your existing
* Amazon Virtual Private Cloud (Amazon VPC) when creating Amazon Redshift subnet group.
*
*
* For information about subnet groups, go to Amazon Redshift
* Cluster Subnet Groups in the Amazon Redshift Cluster Management Guide.
*
*
* @param createClusterSubnetGroupRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateClusterSubnetGroup operation returned by the service.
* @sample AmazonRedshiftAsyncHandler.CreateClusterSubnetGroup
* @see AWS API Documentation
*/
java.util.concurrent.Future createClusterSubnetGroupAsync(CreateClusterSubnetGroupRequest createClusterSubnetGroupRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Used to create a custom domain name for a cluster. Properties include the custom domain name, the cluster the
* custom domain is associated with, and the certificate Amazon Resource Name (ARN).
*
*
* @param createCustomDomainAssociationRequest
* @return A Java Future containing the result of the CreateCustomDomainAssociation operation returned by the
* service.
* @sample AmazonRedshiftAsync.CreateCustomDomainAssociation
* @see AWS API Documentation
*/
java.util.concurrent.Future createCustomDomainAssociationAsync(
CreateCustomDomainAssociationRequest createCustomDomainAssociationRequest);
/**
*
* Used to create a custom domain name for a cluster. Properties include the custom domain name, the cluster the
* custom domain is associated with, and the certificate Amazon Resource Name (ARN).
*
*
* @param createCustomDomainAssociationRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateCustomDomainAssociation operation returned by the
* service.
* @sample AmazonRedshiftAsyncHandler.CreateCustomDomainAssociation
* @see AWS API Documentation
*/
java.util.concurrent.Future createCustomDomainAssociationAsync(
CreateCustomDomainAssociationRequest createCustomDomainAssociationRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates a Redshift-managed VPC endpoint.
*
*
* @param createEndpointAccessRequest
* @return A Java Future containing the result of the CreateEndpointAccess operation returned by the service.
* @sample AmazonRedshiftAsync.CreateEndpointAccess
* @see AWS
* API Documentation
*/
java.util.concurrent.Future createEndpointAccessAsync(CreateEndpointAccessRequest createEndpointAccessRequest);
/**
*
* Creates a Redshift-managed VPC endpoint.
*
*
* @param createEndpointAccessRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateEndpointAccess operation returned by the service.
* @sample AmazonRedshiftAsyncHandler.CreateEndpointAccess
* @see AWS
* API Documentation
*/
java.util.concurrent.Future createEndpointAccessAsync(CreateEndpointAccessRequest createEndpointAccessRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates an Amazon Redshift event notification subscription. This action requires an ARN (Amazon Resource Name) of
* an Amazon SNS topic created by either the Amazon Redshift console, the Amazon SNS console, or the Amazon SNS API.
* To obtain an ARN with Amazon SNS, you must create a topic in Amazon SNS and subscribe to the topic. The ARN is
* displayed in the SNS console.
*
*
* You can specify the source type, and lists of Amazon Redshift source IDs, event categories, and event severities.
* Notifications will be sent for all events you want that match those criteria. For example, you can specify source
* type = cluster, source ID = my-cluster-1 and mycluster2, event categories = Availability, Backup, and severity =
* ERROR. The subscription will only send notifications for those ERROR events in the Availability and Backup
* categories for the specified clusters.
*
*
* If you specify both the source type and source IDs, such as source type = cluster and source identifier =
* my-cluster-1, notifications will be sent for all the cluster events for my-cluster-1. If you specify a source
* type but do not specify a source identifier, you will receive notice of the events for the objects of that type
* in your Amazon Web Services account. If you do not specify either the SourceType nor the SourceIdentifier, you
* will be notified of events generated from all Amazon Redshift sources belonging to your Amazon Web Services
* account. You must specify a source type if you specify a source ID.
*
*
* @param createEventSubscriptionRequest
* @return A Java Future containing the result of the CreateEventSubscription operation returned by the service.
* @sample AmazonRedshiftAsync.CreateEventSubscription
* @see AWS API Documentation
*/
java.util.concurrent.Future createEventSubscriptionAsync(CreateEventSubscriptionRequest createEventSubscriptionRequest);
/**
*
* Creates an Amazon Redshift event notification subscription. This action requires an ARN (Amazon Resource Name) of
* an Amazon SNS topic created by either the Amazon Redshift console, the Amazon SNS console, or the Amazon SNS API.
* To obtain an ARN with Amazon SNS, you must create a topic in Amazon SNS and subscribe to the topic. The ARN is
* displayed in the SNS console.
*
*
* You can specify the source type, and lists of Amazon Redshift source IDs, event categories, and event severities.
* Notifications will be sent for all events you want that match those criteria. For example, you can specify source
* type = cluster, source ID = my-cluster-1 and mycluster2, event categories = Availability, Backup, and severity =
* ERROR. The subscription will only send notifications for those ERROR events in the Availability and Backup
* categories for the specified clusters.
*
*
* If you specify both the source type and source IDs, such as source type = cluster and source identifier =
* my-cluster-1, notifications will be sent for all the cluster events for my-cluster-1. If you specify a source
* type but do not specify a source identifier, you will receive notice of the events for the objects of that type
* in your Amazon Web Services account. If you do not specify either the SourceType nor the SourceIdentifier, you
* will be notified of events generated from all Amazon Redshift sources belonging to your Amazon Web Services
* account. You must specify a source type if you specify a source ID.
*
*
* @param createEventSubscriptionRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateEventSubscription operation returned by the service.
* @sample AmazonRedshiftAsyncHandler.CreateEventSubscription
* @see AWS API Documentation
*/
java.util.concurrent.Future createEventSubscriptionAsync(CreateEventSubscriptionRequest createEventSubscriptionRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates an HSM client certificate that an Amazon Redshift cluster will use to connect to the client's HSM in
* order to store and retrieve the keys used to encrypt the cluster databases.
*
*
* The command returns a public key, which you must store in the HSM. In addition to creating the HSM certificate,
* you must create an Amazon Redshift HSM configuration that provides a cluster the information needed to store and
* use encryption keys in the HSM. For more information, go to Hardware
* Security Modules in the Amazon Redshift Cluster Management Guide.
*
*
* @param createHsmClientCertificateRequest
* @return A Java Future containing the result of the CreateHsmClientCertificate operation returned by the service.
* @sample AmazonRedshiftAsync.CreateHsmClientCertificate
* @see AWS API Documentation
*/
java.util.concurrent.Future createHsmClientCertificateAsync(CreateHsmClientCertificateRequest createHsmClientCertificateRequest);
/**
*
* Creates an HSM client certificate that an Amazon Redshift cluster will use to connect to the client's HSM in
* order to store and retrieve the keys used to encrypt the cluster databases.
*
*
* The command returns a public key, which you must store in the HSM. In addition to creating the HSM certificate,
* you must create an Amazon Redshift HSM configuration that provides a cluster the information needed to store and
* use encryption keys in the HSM. For more information, go to Hardware
* Security Modules in the Amazon Redshift Cluster Management Guide.
*
*
* @param createHsmClientCertificateRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateHsmClientCertificate operation returned by the service.
* @sample AmazonRedshiftAsyncHandler.CreateHsmClientCertificate
* @see AWS API Documentation
*/
java.util.concurrent.Future createHsmClientCertificateAsync(CreateHsmClientCertificateRequest createHsmClientCertificateRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates an HSM configuration that contains the information required by an Amazon Redshift cluster to store and
* use database encryption keys in a Hardware Security Module (HSM). After creating the HSM configuration, you can
* specify it as a parameter when creating a cluster. The cluster will then store its encryption keys in the HSM.
*
*
* In addition to creating an HSM configuration, you must also create an HSM client certificate. For more
* information, go to Hardware
* Security Modules in the Amazon Redshift Cluster Management Guide.
*
*
* @param createHsmConfigurationRequest
* @return A Java Future containing the result of the CreateHsmConfiguration operation returned by the service.
* @sample AmazonRedshiftAsync.CreateHsmConfiguration
* @see AWS API Documentation
*/
java.util.concurrent.Future createHsmConfigurationAsync(CreateHsmConfigurationRequest createHsmConfigurationRequest);
/**
*
* Creates an HSM configuration that contains the information required by an Amazon Redshift cluster to store and
* use database encryption keys in a Hardware Security Module (HSM). After creating the HSM configuration, you can
* specify it as a parameter when creating a cluster. The cluster will then store its encryption keys in the HSM.
*
*
* In addition to creating an HSM configuration, you must also create an HSM client certificate. For more
* information, go to Hardware
* Security Modules in the Amazon Redshift Cluster Management Guide.
*
*
* @param createHsmConfigurationRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateHsmConfiguration operation returned by the service.
* @sample AmazonRedshiftAsyncHandler.CreateHsmConfiguration
* @see AWS API Documentation
*/
java.util.concurrent.Future createHsmConfigurationAsync(CreateHsmConfigurationRequest createHsmConfigurationRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates an Amazon Redshift application for use with IAM Identity Center.
*
*
* @param createRedshiftIdcApplicationRequest
* @return A Java Future containing the result of the CreateRedshiftIdcApplication operation returned by the
* service.
* @sample AmazonRedshiftAsync.CreateRedshiftIdcApplication
* @see AWS API Documentation
*/
java.util.concurrent.Future createRedshiftIdcApplicationAsync(
CreateRedshiftIdcApplicationRequest createRedshiftIdcApplicationRequest);
/**
*
* Creates an Amazon Redshift application for use with IAM Identity Center.
*
*
* @param createRedshiftIdcApplicationRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateRedshiftIdcApplication operation returned by the
* service.
* @sample AmazonRedshiftAsyncHandler.CreateRedshiftIdcApplication
* @see AWS API Documentation
*/
java.util.concurrent.Future createRedshiftIdcApplicationAsync(
CreateRedshiftIdcApplicationRequest createRedshiftIdcApplicationRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates a scheduled action. A scheduled action contains a schedule and an Amazon Redshift API action. For
* example, you can create a schedule of when to run the ResizeCluster
API operation.
*
*
* @param createScheduledActionRequest
* @return A Java Future containing the result of the CreateScheduledAction operation returned by the service.
* @sample AmazonRedshiftAsync.CreateScheduledAction
* @see AWS
* API Documentation
*/
java.util.concurrent.Future createScheduledActionAsync(CreateScheduledActionRequest createScheduledActionRequest);
/**
*
* Creates a scheduled action. A scheduled action contains a schedule and an Amazon Redshift API action. For
* example, you can create a schedule of when to run the ResizeCluster
API operation.
*
*
* @param createScheduledActionRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateScheduledAction operation returned by the service.
* @sample AmazonRedshiftAsyncHandler.CreateScheduledAction
* @see AWS
* API Documentation
*/
java.util.concurrent.Future createScheduledActionAsync(CreateScheduledActionRequest createScheduledActionRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates a snapshot copy grant that permits Amazon Redshift to use an encrypted symmetric key from Key Management
* Service (KMS) to encrypt copied snapshots in a destination region.
*
*
* For more information about managing snapshot copy grants, go to Amazon Redshift Database
* Encryption in the Amazon Redshift Cluster Management Guide.
*
*
* @param createSnapshotCopyGrantRequest
* The result of the CreateSnapshotCopyGrant
action.
* @return A Java Future containing the result of the CreateSnapshotCopyGrant operation returned by the service.
* @sample AmazonRedshiftAsync.CreateSnapshotCopyGrant
* @see AWS API Documentation
*/
java.util.concurrent.Future createSnapshotCopyGrantAsync(CreateSnapshotCopyGrantRequest createSnapshotCopyGrantRequest);
/**
*
* Creates a snapshot copy grant that permits Amazon Redshift to use an encrypted symmetric key from Key Management
* Service (KMS) to encrypt copied snapshots in a destination region.
*
*
* For more information about managing snapshot copy grants, go to Amazon Redshift Database
* Encryption in the Amazon Redshift Cluster Management Guide.
*
*
* @param createSnapshotCopyGrantRequest
* The result of the CreateSnapshotCopyGrant
action.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateSnapshotCopyGrant operation returned by the service.
* @sample AmazonRedshiftAsyncHandler.CreateSnapshotCopyGrant
* @see AWS API Documentation
*/
java.util.concurrent.Future createSnapshotCopyGrantAsync(CreateSnapshotCopyGrantRequest createSnapshotCopyGrantRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Create a snapshot schedule that can be associated to a cluster and which overrides the default system backup
* schedule.
*
*
* @param createSnapshotScheduleRequest
* @return A Java Future containing the result of the CreateSnapshotSchedule operation returned by the service.
* @sample AmazonRedshiftAsync.CreateSnapshotSchedule
* @see AWS API Documentation
*/
java.util.concurrent.Future createSnapshotScheduleAsync(CreateSnapshotScheduleRequest createSnapshotScheduleRequest);
/**
*
* Create a snapshot schedule that can be associated to a cluster and which overrides the default system backup
* schedule.
*
*
* @param createSnapshotScheduleRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateSnapshotSchedule operation returned by the service.
* @sample AmazonRedshiftAsyncHandler.CreateSnapshotSchedule
* @see AWS API Documentation
*/
java.util.concurrent.Future createSnapshotScheduleAsync(CreateSnapshotScheduleRequest createSnapshotScheduleRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Adds tags to a cluster.
*
*
* A resource can have up to 50 tags. If you try to create more than 50 tags for a resource, you will receive an
* error and the attempt will fail.
*
*
* If you specify a key that already exists for the resource, the value for that key will be updated with the new
* value.
*
*
* @param createTagsRequest
* Contains the output from the CreateTags
action.
* @return A Java Future containing the result of the CreateTags operation returned by the service.
* @sample AmazonRedshiftAsync.CreateTags
* @see AWS API
* Documentation
*/
java.util.concurrent.Future createTagsAsync(CreateTagsRequest createTagsRequest);
/**
*
* Adds tags to a cluster.
*
*
* A resource can have up to 50 tags. If you try to create more than 50 tags for a resource, you will receive an
* error and the attempt will fail.
*
*
* If you specify a key that already exists for the resource, the value for that key will be updated with the new
* value.
*
*
* @param createTagsRequest
* Contains the output from the CreateTags
action.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateTags operation returned by the service.
* @sample AmazonRedshiftAsyncHandler.CreateTags
* @see AWS API
* Documentation
*/
java.util.concurrent.Future createTagsAsync(CreateTagsRequest createTagsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates a usage limit for a specified Amazon Redshift feature on a cluster. The usage limit is identified by the
* returned usage limit identifier.
*
*
* @param createUsageLimitRequest
* @return A Java Future containing the result of the CreateUsageLimit operation returned by the service.
* @sample AmazonRedshiftAsync.CreateUsageLimit
* @see AWS API
* Documentation
*/
java.util.concurrent.Future createUsageLimitAsync(CreateUsageLimitRequest createUsageLimitRequest);
/**
*
* Creates a usage limit for a specified Amazon Redshift feature on a cluster. The usage limit is identified by the
* returned usage limit identifier.
*
*
* @param createUsageLimitRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateUsageLimit operation returned by the service.
* @sample AmazonRedshiftAsyncHandler.CreateUsageLimit
* @see AWS API
* Documentation
*/
java.util.concurrent.Future createUsageLimitAsync(CreateUsageLimitRequest createUsageLimitRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* From a datashare producer account, removes authorization from the specified datashare.
*
*
* @param deauthorizeDataShareRequest
* @return A Java Future containing the result of the DeauthorizeDataShare operation returned by the service.
* @sample AmazonRedshiftAsync.DeauthorizeDataShare
* @see AWS
* API Documentation
*/
java.util.concurrent.Future deauthorizeDataShareAsync(DeauthorizeDataShareRequest deauthorizeDataShareRequest);
/**
*
* From a datashare producer account, removes authorization from the specified datashare.
*
*
* @param deauthorizeDataShareRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeauthorizeDataShare operation returned by the service.
* @sample AmazonRedshiftAsyncHandler.DeauthorizeDataShare
* @see AWS
* API Documentation
*/
java.util.concurrent.Future deauthorizeDataShareAsync(DeauthorizeDataShareRequest deauthorizeDataShareRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deletes an authentication profile.
*
*
* @param deleteAuthenticationProfileRequest
* @return A Java Future containing the result of the DeleteAuthenticationProfile operation returned by the service.
* @sample AmazonRedshiftAsync.DeleteAuthenticationProfile
* @see AWS API Documentation
*/
java.util.concurrent.Future deleteAuthenticationProfileAsync(
DeleteAuthenticationProfileRequest deleteAuthenticationProfileRequest);
/**
*
* Deletes an authentication profile.
*
*
* @param deleteAuthenticationProfileRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteAuthenticationProfile operation returned by the service.
* @sample AmazonRedshiftAsyncHandler.DeleteAuthenticationProfile
* @see AWS API Documentation
*/
java.util.concurrent.Future deleteAuthenticationProfileAsync(
DeleteAuthenticationProfileRequest deleteAuthenticationProfileRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deletes a previously provisioned cluster without its final snapshot being created. A successful response from the
* web service indicates that the request was received correctly. Use DescribeClusters to monitor the status
* of the deletion. The delete operation cannot be canceled or reverted once submitted. For more information about
* managing clusters, go to Amazon Redshift Clusters
* in the Amazon Redshift Cluster Management Guide.
*
*
* If you want to shut down the cluster and retain it for future use, set SkipFinalClusterSnapshot to
* false
and specify a name for FinalClusterSnapshotIdentifier. You can later restore this
* snapshot to resume using the cluster. If a final cluster snapshot is requested, the status of the cluster will be
* "final-snapshot" while the snapshot is being taken, then it's "deleting" once Amazon Redshift begins deleting the
* cluster.
*
*
* For more information about managing clusters, go to Amazon Redshift Clusters
* in the Amazon Redshift Cluster Management Guide.
*
*
* @param deleteClusterRequest
* @return A Java Future containing the result of the DeleteCluster operation returned by the service.
* @sample AmazonRedshiftAsync.DeleteCluster
* @see AWS API
* Documentation
*/
java.util.concurrent.Future deleteClusterAsync(DeleteClusterRequest deleteClusterRequest);
/**
*
* Deletes a previously provisioned cluster without its final snapshot being created. A successful response from the
* web service indicates that the request was received correctly. Use DescribeClusters to monitor the status
* of the deletion. The delete operation cannot be canceled or reverted once submitted. For more information about
* managing clusters, go to Amazon Redshift Clusters
* in the Amazon Redshift Cluster Management Guide.
*
*
* If you want to shut down the cluster and retain it for future use, set SkipFinalClusterSnapshot to
* false
and specify a name for FinalClusterSnapshotIdentifier. You can later restore this
* snapshot to resume using the cluster. If a final cluster snapshot is requested, the status of the cluster will be
* "final-snapshot" while the snapshot is being taken, then it's "deleting" once Amazon Redshift begins deleting the
* cluster.
*
*
* For more information about managing clusters, go to Amazon Redshift Clusters
* in the Amazon Redshift Cluster Management Guide.
*
*
* @param deleteClusterRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteCluster operation returned by the service.
* @sample AmazonRedshiftAsyncHandler.DeleteCluster
* @see AWS API
* Documentation
*/
java.util.concurrent.Future deleteClusterAsync(DeleteClusterRequest deleteClusterRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deletes a specified Amazon Redshift parameter group.
*
*
*
* You cannot delete a parameter group if it is associated with a cluster.
*
*
*
* @param deleteClusterParameterGroupRequest
* @return A Java Future containing the result of the DeleteClusterParameterGroup operation returned by the service.
* @sample AmazonRedshiftAsync.DeleteClusterParameterGroup
* @see AWS API Documentation
*/
java.util.concurrent.Future deleteClusterParameterGroupAsync(
DeleteClusterParameterGroupRequest deleteClusterParameterGroupRequest);
/**
*
* Deletes a specified Amazon Redshift parameter group.
*
*
*
* You cannot delete a parameter group if it is associated with a cluster.
*
*
*
* @param deleteClusterParameterGroupRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteClusterParameterGroup operation returned by the service.
* @sample AmazonRedshiftAsyncHandler.DeleteClusterParameterGroup
* @see AWS API Documentation
*/
java.util.concurrent.Future deleteClusterParameterGroupAsync(
DeleteClusterParameterGroupRequest deleteClusterParameterGroupRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deletes an Amazon Redshift security group.
*
*
*
* You cannot delete a security group that is associated with any clusters. You cannot delete the default security
* group.
*
*
*
* For information about managing security groups, go to Amazon Redshift Cluster
* Security Groups in the Amazon Redshift Cluster Management Guide.
*
*
* @param deleteClusterSecurityGroupRequest
* @return A Java Future containing the result of the DeleteClusterSecurityGroup operation returned by the service.
* @sample AmazonRedshiftAsync.DeleteClusterSecurityGroup
* @see AWS API Documentation
*/
java.util.concurrent.Future deleteClusterSecurityGroupAsync(
DeleteClusterSecurityGroupRequest deleteClusterSecurityGroupRequest);
/**
*
* Deletes an Amazon Redshift security group.
*
*
*
* You cannot delete a security group that is associated with any clusters. You cannot delete the default security
* group.
*
*
*
* For information about managing security groups, go to Amazon Redshift Cluster
* Security Groups in the Amazon Redshift Cluster Management Guide.
*
*
* @param deleteClusterSecurityGroupRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteClusterSecurityGroup operation returned by the service.
* @sample AmazonRedshiftAsyncHandler.DeleteClusterSecurityGroup
* @see AWS API Documentation
*/
java.util.concurrent.Future deleteClusterSecurityGroupAsync(
DeleteClusterSecurityGroupRequest deleteClusterSecurityGroupRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deletes the specified manual snapshot. The snapshot must be in the available
state, with no other
* users authorized to access the snapshot.
*
*
* Unlike automated snapshots, manual snapshots are retained even after you delete your cluster. Amazon Redshift
* does not delete your manual snapshots. You must delete manual snapshot explicitly to avoid getting charged. If
* other accounts are authorized to access the snapshot, you must revoke all of the authorizations before you can
* delete the snapshot.
*
*
* @param deleteClusterSnapshotRequest
* @return A Java Future containing the result of the DeleteClusterSnapshot operation returned by the service.
* @sample AmazonRedshiftAsync.DeleteClusterSnapshot
* @see AWS
* API Documentation
*/
java.util.concurrent.Future deleteClusterSnapshotAsync(DeleteClusterSnapshotRequest deleteClusterSnapshotRequest);
/**
*
* Deletes the specified manual snapshot. The snapshot must be in the available
state, with no other
* users authorized to access the snapshot.
*
*
* Unlike automated snapshots, manual snapshots are retained even after you delete your cluster. Amazon Redshift
* does not delete your manual snapshots. You must delete manual snapshot explicitly to avoid getting charged. If
* other accounts are authorized to access the snapshot, you must revoke all of the authorizations before you can
* delete the snapshot.
*
*
* @param deleteClusterSnapshotRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteClusterSnapshot operation returned by the service.
* @sample AmazonRedshiftAsyncHandler.DeleteClusterSnapshot
* @see AWS
* API Documentation
*/
java.util.concurrent.Future deleteClusterSnapshotAsync(DeleteClusterSnapshotRequest deleteClusterSnapshotRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deletes the specified cluster subnet group.
*
*
* @param deleteClusterSubnetGroupRequest
* @return A Java Future containing the result of the DeleteClusterSubnetGroup operation returned by the service.
* @sample AmazonRedshiftAsync.DeleteClusterSubnetGroup
* @see AWS API Documentation
*/
java.util.concurrent.Future deleteClusterSubnetGroupAsync(DeleteClusterSubnetGroupRequest deleteClusterSubnetGroupRequest);
/**
*
* Deletes the specified cluster subnet group.
*
*
* @param deleteClusterSubnetGroupRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteClusterSubnetGroup operation returned by the service.
* @sample AmazonRedshiftAsyncHandler.DeleteClusterSubnetGroup
* @see AWS API Documentation
*/
java.util.concurrent.Future deleteClusterSubnetGroupAsync(DeleteClusterSubnetGroupRequest deleteClusterSubnetGroupRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Contains information about deleting a custom domain association for a cluster.
*
*
* @param deleteCustomDomainAssociationRequest
* @return A Java Future containing the result of the DeleteCustomDomainAssociation operation returned by the
* service.
* @sample AmazonRedshiftAsync.DeleteCustomDomainAssociation
* @see AWS API Documentation
*/
java.util.concurrent.Future deleteCustomDomainAssociationAsync(
DeleteCustomDomainAssociationRequest deleteCustomDomainAssociationRequest);
/**
*
* Contains information about deleting a custom domain association for a cluster.
*
*
* @param deleteCustomDomainAssociationRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteCustomDomainAssociation operation returned by the
* service.
* @sample AmazonRedshiftAsyncHandler.DeleteCustomDomainAssociation
* @see AWS API Documentation
*/
java.util.concurrent.Future deleteCustomDomainAssociationAsync(
DeleteCustomDomainAssociationRequest deleteCustomDomainAssociationRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deletes a Redshift-managed VPC endpoint.
*
*
* @param deleteEndpointAccessRequest
* @return A Java Future containing the result of the DeleteEndpointAccess operation returned by the service.
* @sample AmazonRedshiftAsync.DeleteEndpointAccess
* @see AWS
* API Documentation
*/
java.util.concurrent.Future deleteEndpointAccessAsync(DeleteEndpointAccessRequest deleteEndpointAccessRequest);
/**
*
* Deletes a Redshift-managed VPC endpoint.
*
*
* @param deleteEndpointAccessRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteEndpointAccess operation returned by the service.
* @sample AmazonRedshiftAsyncHandler.DeleteEndpointAccess
* @see AWS
* API Documentation
*/
java.util.concurrent.Future deleteEndpointAccessAsync(DeleteEndpointAccessRequest deleteEndpointAccessRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deletes an Amazon Redshift event notification subscription.
*
*
* @param deleteEventSubscriptionRequest
* @return A Java Future containing the result of the DeleteEventSubscription operation returned by the service.
* @sample AmazonRedshiftAsync.DeleteEventSubscription
* @see AWS API Documentation
*/
java.util.concurrent.Future deleteEventSubscriptionAsync(DeleteEventSubscriptionRequest deleteEventSubscriptionRequest);
/**
*
* Deletes an Amazon Redshift event notification subscription.
*
*
* @param deleteEventSubscriptionRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteEventSubscription operation returned by the service.
* @sample AmazonRedshiftAsyncHandler.DeleteEventSubscription
* @see AWS API Documentation
*/
java.util.concurrent.Future deleteEventSubscriptionAsync(DeleteEventSubscriptionRequest deleteEventSubscriptionRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deletes the specified HSM client certificate.
*
*
* @param deleteHsmClientCertificateRequest
* @return A Java Future containing the result of the DeleteHsmClientCertificate operation returned by the service.
* @sample AmazonRedshiftAsync.DeleteHsmClientCertificate
* @see AWS API Documentation
*/
java.util.concurrent.Future deleteHsmClientCertificateAsync(
DeleteHsmClientCertificateRequest deleteHsmClientCertificateRequest);
/**
*
* Deletes the specified HSM client certificate.
*
*
* @param deleteHsmClientCertificateRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteHsmClientCertificate operation returned by the service.
* @sample AmazonRedshiftAsyncHandler.DeleteHsmClientCertificate
* @see AWS API Documentation
*/
java.util.concurrent.Future deleteHsmClientCertificateAsync(
DeleteHsmClientCertificateRequest deleteHsmClientCertificateRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deletes the specified Amazon Redshift HSM configuration.
*
*
* @param deleteHsmConfigurationRequest
* @return A Java Future containing the result of the DeleteHsmConfiguration operation returned by the service.
* @sample AmazonRedshiftAsync.DeleteHsmConfiguration
* @see AWS API Documentation
*/
java.util.concurrent.Future deleteHsmConfigurationAsync(DeleteHsmConfigurationRequest deleteHsmConfigurationRequest);
/**
*
* Deletes the specified Amazon Redshift HSM configuration.
*
*
* @param deleteHsmConfigurationRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteHsmConfiguration operation returned by the service.
* @sample AmazonRedshiftAsyncHandler.DeleteHsmConfiguration
* @see AWS API Documentation
*/
java.util.concurrent.Future deleteHsmConfigurationAsync(DeleteHsmConfigurationRequest deleteHsmConfigurationRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deletes a partner integration from a cluster. Data can still flow to the cluster until the integration is deleted
* at the partner's website.
*
*
* @param deletePartnerRequest
* @return A Java Future containing the result of the DeletePartner operation returned by the service.
* @sample AmazonRedshiftAsync.DeletePartner
* @see AWS API
* Documentation
*/
java.util.concurrent.Future deletePartnerAsync(DeletePartnerRequest deletePartnerRequest);
/**
*
* Deletes a partner integration from a cluster. Data can still flow to the cluster until the integration is deleted
* at the partner's website.
*
*
* @param deletePartnerRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeletePartner operation returned by the service.
* @sample AmazonRedshiftAsyncHandler.DeletePartner
* @see AWS API
* Documentation
*/
java.util.concurrent.Future deletePartnerAsync(DeletePartnerRequest deletePartnerRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deletes an Amazon Redshift IAM Identity Center application.
*
*
* @param deleteRedshiftIdcApplicationRequest
* @return A Java Future containing the result of the DeleteRedshiftIdcApplication operation returned by the
* service.
* @sample AmazonRedshiftAsync.DeleteRedshiftIdcApplication
* @see AWS API Documentation
*/
java.util.concurrent.Future deleteRedshiftIdcApplicationAsync(
DeleteRedshiftIdcApplicationRequest deleteRedshiftIdcApplicationRequest);
/**
*
* Deletes an Amazon Redshift IAM Identity Center application.
*
*
* @param deleteRedshiftIdcApplicationRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteRedshiftIdcApplication operation returned by the
* service.
* @sample AmazonRedshiftAsyncHandler.DeleteRedshiftIdcApplication
* @see AWS API Documentation
*/
java.util.concurrent.Future deleteRedshiftIdcApplicationAsync(
DeleteRedshiftIdcApplicationRequest deleteRedshiftIdcApplicationRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deletes the resource policy for a specified resource.
*
*
* @param deleteResourcePolicyRequest
* @return A Java Future containing the result of the DeleteResourcePolicy operation returned by the service.
* @sample AmazonRedshiftAsync.DeleteResourcePolicy
* @see AWS
* API Documentation
*/
java.util.concurrent.Future deleteResourcePolicyAsync(DeleteResourcePolicyRequest deleteResourcePolicyRequest);
/**
*
* Deletes the resource policy for a specified resource.
*
*
* @param deleteResourcePolicyRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteResourcePolicy operation returned by the service.
* @sample AmazonRedshiftAsyncHandler.DeleteResourcePolicy
* @see AWS
* API Documentation
*/
java.util.concurrent.Future deleteResourcePolicyAsync(DeleteResourcePolicyRequest deleteResourcePolicyRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deletes a scheduled action.
*
*
* @param deleteScheduledActionRequest
* @return A Java Future containing the result of the DeleteScheduledAction operation returned by the service.
* @sample AmazonRedshiftAsync.DeleteScheduledAction
* @see AWS
* API Documentation
*/
java.util.concurrent.Future deleteScheduledActionAsync(DeleteScheduledActionRequest deleteScheduledActionRequest);
/**
*
* Deletes a scheduled action.
*
*
* @param deleteScheduledActionRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteScheduledAction operation returned by the service.
* @sample AmazonRedshiftAsyncHandler.DeleteScheduledAction
* @see AWS
* API Documentation
*/
java.util.concurrent.Future deleteScheduledActionAsync(DeleteScheduledActionRequest deleteScheduledActionRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deletes the specified snapshot copy grant.
*
*
* @param deleteSnapshotCopyGrantRequest
* The result of the DeleteSnapshotCopyGrant
action.
* @return A Java Future containing the result of the DeleteSnapshotCopyGrant operation returned by the service.
* @sample AmazonRedshiftAsync.DeleteSnapshotCopyGrant
* @see AWS API Documentation
*/
java.util.concurrent.Future deleteSnapshotCopyGrantAsync(DeleteSnapshotCopyGrantRequest deleteSnapshotCopyGrantRequest);
/**
*
* Deletes the specified snapshot copy grant.
*
*
* @param deleteSnapshotCopyGrantRequest
* The result of the DeleteSnapshotCopyGrant
action.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteSnapshotCopyGrant operation returned by the service.
* @sample AmazonRedshiftAsyncHandler.DeleteSnapshotCopyGrant
* @see AWS API Documentation
*/
java.util.concurrent.Future deleteSnapshotCopyGrantAsync(DeleteSnapshotCopyGrantRequest deleteSnapshotCopyGrantRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deletes a snapshot schedule.
*
*
* @param deleteSnapshotScheduleRequest
* @return A Java Future containing the result of the DeleteSnapshotSchedule operation returned by the service.
* @sample AmazonRedshiftAsync.DeleteSnapshotSchedule
* @see AWS API Documentation
*/
java.util.concurrent.Future deleteSnapshotScheduleAsync(DeleteSnapshotScheduleRequest deleteSnapshotScheduleRequest);
/**
*
* Deletes a snapshot schedule.
*
*
* @param deleteSnapshotScheduleRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteSnapshotSchedule operation returned by the service.
* @sample AmazonRedshiftAsyncHandler.DeleteSnapshotSchedule
* @see AWS API Documentation
*/
java.util.concurrent.Future deleteSnapshotScheduleAsync(DeleteSnapshotScheduleRequest deleteSnapshotScheduleRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deletes tags from a resource. You must provide the ARN of the resource from which you want to delete the tag or
* tags.
*
*
* @param deleteTagsRequest
* Contains the output from the DeleteTags
action.
* @return A Java Future containing the result of the DeleteTags operation returned by the service.
* @sample AmazonRedshiftAsync.DeleteTags
* @see AWS API
* Documentation
*/
java.util.concurrent.Future deleteTagsAsync(DeleteTagsRequest deleteTagsRequest);
/**
*
* Deletes tags from a resource. You must provide the ARN of the resource from which you want to delete the tag or
* tags.
*
*
* @param deleteTagsRequest
* Contains the output from the DeleteTags
action.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteTags operation returned by the service.
* @sample AmazonRedshiftAsyncHandler.DeleteTags
* @see AWS API
* Documentation
*/
java.util.concurrent.Future deleteTagsAsync(DeleteTagsRequest deleteTagsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deletes a usage limit from a cluster.
*
*
* @param deleteUsageLimitRequest
* @return A Java Future containing the result of the DeleteUsageLimit operation returned by the service.
* @sample AmazonRedshiftAsync.DeleteUsageLimit
* @see AWS API
* Documentation
*/
java.util.concurrent.Future deleteUsageLimitAsync(DeleteUsageLimitRequest deleteUsageLimitRequest);
/**
*
* Deletes a usage limit from a cluster.
*
*
* @param deleteUsageLimitRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteUsageLimit operation returned by the service.
* @sample AmazonRedshiftAsyncHandler.DeleteUsageLimit
* @see AWS API
* Documentation
*/
java.util.concurrent.Future deleteUsageLimitAsync(DeleteUsageLimitRequest deleteUsageLimitRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns a list of attributes attached to an account
*
*
* @param describeAccountAttributesRequest
* @return A Java Future containing the result of the DescribeAccountAttributes operation returned by the service.
* @sample AmazonRedshiftAsync.DescribeAccountAttributes
* @see AWS API Documentation
*/
java.util.concurrent.Future describeAccountAttributesAsync(
DescribeAccountAttributesRequest describeAccountAttributesRequest);
/**
*
* Returns a list of attributes attached to an account
*
*
* @param describeAccountAttributesRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeAccountAttributes operation returned by the service.
* @sample AmazonRedshiftAsyncHandler.DescribeAccountAttributes
* @see AWS API Documentation
*/
java.util.concurrent.Future describeAccountAttributesAsync(
DescribeAccountAttributesRequest describeAccountAttributesRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Describes an authentication profile.
*
*
* @param describeAuthenticationProfilesRequest
* @return A Java Future containing the result of the DescribeAuthenticationProfiles operation returned by the
* service.
* @sample AmazonRedshiftAsync.DescribeAuthenticationProfiles
* @see AWS API Documentation
*/
java.util.concurrent.Future describeAuthenticationProfilesAsync(
DescribeAuthenticationProfilesRequest describeAuthenticationProfilesRequest);
/**
*
* Describes an authentication profile.
*
*
* @param describeAuthenticationProfilesRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeAuthenticationProfiles operation returned by the
* service.
* @sample AmazonRedshiftAsyncHandler.DescribeAuthenticationProfiles
* @see AWS API Documentation
*/
java.util.concurrent.Future describeAuthenticationProfilesAsync(
DescribeAuthenticationProfilesRequest describeAuthenticationProfilesRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns an array of ClusterDbRevision
objects.
*
*
* @param describeClusterDbRevisionsRequest
* @return A Java Future containing the result of the DescribeClusterDbRevisions operation returned by the service.
* @sample AmazonRedshiftAsync.DescribeClusterDbRevisions
* @see AWS API Documentation
*/
java.util.concurrent.Future describeClusterDbRevisionsAsync(
DescribeClusterDbRevisionsRequest describeClusterDbRevisionsRequest);
/**
*
* Returns an array of ClusterDbRevision
objects.
*
*
* @param describeClusterDbRevisionsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeClusterDbRevisions operation returned by the service.
* @sample AmazonRedshiftAsyncHandler.DescribeClusterDbRevisions
* @see AWS API Documentation
*/
java.util.concurrent.Future describeClusterDbRevisionsAsync(
DescribeClusterDbRevisionsRequest describeClusterDbRevisionsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns a list of Amazon Redshift parameter groups, including parameter groups you created and the default
* parameter group. For each parameter group, the response includes the parameter group name, description, and
* parameter group family name. You can optionally specify a name to retrieve the description of a specific
* parameter group.
*
*
* For more information about parameters and parameter groups, go to Amazon Redshift
* Parameter Groups in the Amazon Redshift Cluster Management Guide.
*
*
* If you specify both tag keys and tag values in the same request, Amazon Redshift returns all parameter groups
* that match any combination of the specified keys and values. For example, if you have owner
and
* environment
for tag keys, and admin
and test
for tag values, all parameter
* groups that have any combination of those values are returned.
*
*
* If both tag keys and values are omitted from the request, parameter groups are returned regardless of whether
* they have tag keys or values associated with them.
*
*
* @param describeClusterParameterGroupsRequest
* @return A Java Future containing the result of the DescribeClusterParameterGroups operation returned by the
* service.
* @sample AmazonRedshiftAsync.DescribeClusterParameterGroups
* @see AWS API Documentation
*/
java.util.concurrent.Future describeClusterParameterGroupsAsync(
DescribeClusterParameterGroupsRequest describeClusterParameterGroupsRequest);
/**
*
* Returns a list of Amazon Redshift parameter groups, including parameter groups you created and the default
* parameter group. For each parameter group, the response includes the parameter group name, description, and
* parameter group family name. You can optionally specify a name to retrieve the description of a specific
* parameter group.
*
*
* For more information about parameters and parameter groups, go to Amazon Redshift
* Parameter Groups in the Amazon Redshift Cluster Management Guide.
*
*
* If you specify both tag keys and tag values in the same request, Amazon Redshift returns all parameter groups
* that match any combination of the specified keys and values. For example, if you have owner
and
* environment
for tag keys, and admin
and test
for tag values, all parameter
* groups that have any combination of those values are returned.
*
*
* If both tag keys and values are omitted from the request, parameter groups are returned regardless of whether
* they have tag keys or values associated with them.
*
*
* @param describeClusterParameterGroupsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeClusterParameterGroups operation returned by the
* service.
* @sample AmazonRedshiftAsyncHandler.DescribeClusterParameterGroups
* @see AWS API Documentation
*/
java.util.concurrent.Future describeClusterParameterGroupsAsync(
DescribeClusterParameterGroupsRequest describeClusterParameterGroupsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
* Simplified method form for invoking the DescribeClusterParameterGroups operation.
*
* @see #describeClusterParameterGroupsAsync(DescribeClusterParameterGroupsRequest)
*/
java.util.concurrent.Future describeClusterParameterGroupsAsync();
/**
* Simplified method form for invoking the DescribeClusterParameterGroups operation with an AsyncHandler.
*
* @see #describeClusterParameterGroupsAsync(DescribeClusterParameterGroupsRequest,
* com.amazonaws.handlers.AsyncHandler)
*/
java.util.concurrent.Future describeClusterParameterGroupsAsync(
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns a detailed list of parameters contained within the specified Amazon Redshift parameter group. For each
* parameter the response includes information such as parameter name, description, data type, value, whether the
* parameter value is modifiable, and so on.
*
*
* You can specify source filter to retrieve parameters of only specific type. For example, to retrieve
* parameters that were modified by a user action such as from ModifyClusterParameterGroup, you can specify
* source equal to user.
*
*
* For more information about parameters and parameter groups, go to Amazon Redshift
* Parameter Groups in the Amazon Redshift Cluster Management Guide.
*
*
* @param describeClusterParametersRequest
* @return A Java Future containing the result of the DescribeClusterParameters operation returned by the service.
* @sample AmazonRedshiftAsync.DescribeClusterParameters
* @see AWS API Documentation
*/
java.util.concurrent.Future describeClusterParametersAsync(
DescribeClusterParametersRequest describeClusterParametersRequest);
/**
*
* Returns a detailed list of parameters contained within the specified Amazon Redshift parameter group. For each
* parameter the response includes information such as parameter name, description, data type, value, whether the
* parameter value is modifiable, and so on.
*
*
* You can specify source filter to retrieve parameters of only specific type. For example, to retrieve
* parameters that were modified by a user action such as from ModifyClusterParameterGroup, you can specify
* source equal to user.
*
*
* For more information about parameters and parameter groups, go to Amazon Redshift
* Parameter Groups in the Amazon Redshift Cluster Management Guide.
*
*
* @param describeClusterParametersRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeClusterParameters operation returned by the service.
* @sample AmazonRedshiftAsyncHandler.DescribeClusterParameters
* @see AWS API Documentation
*/
java.util.concurrent.Future describeClusterParametersAsync(
DescribeClusterParametersRequest describeClusterParametersRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns information about Amazon Redshift security groups. If the name of a security group is specified, the
* response will contain only information about only that security group.
*
*
* For information about managing security groups, go to Amazon Redshift Cluster
* Security Groups in the Amazon Redshift Cluster Management Guide.
*
*
* If you specify both tag keys and tag values in the same request, Amazon Redshift returns all security groups that
* match any combination of the specified keys and values. For example, if you have owner
and
* environment
for tag keys, and admin
and test
for tag values, all security
* groups that have any combination of those values are returned.
*
*
* If both tag keys and values are omitted from the request, security groups are returned regardless of whether they
* have tag keys or values associated with them.
*
*
* @param describeClusterSecurityGroupsRequest
* @return A Java Future containing the result of the DescribeClusterSecurityGroups operation returned by the
* service.
* @sample AmazonRedshiftAsync.DescribeClusterSecurityGroups
* @see AWS API Documentation
*/
java.util.concurrent.Future describeClusterSecurityGroupsAsync(
DescribeClusterSecurityGroupsRequest describeClusterSecurityGroupsRequest);
/**
*
* Returns information about Amazon Redshift security groups. If the name of a security group is specified, the
* response will contain only information about only that security group.
*
*
* For information about managing security groups, go to Amazon Redshift Cluster
* Security Groups in the Amazon Redshift Cluster Management Guide.
*
*
* If you specify both tag keys and tag values in the same request, Amazon Redshift returns all security groups that
* match any combination of the specified keys and values. For example, if you have owner
and
* environment
for tag keys, and admin
and test
for tag values, all security
* groups that have any combination of those values are returned.
*
*
* If both tag keys and values are omitted from the request, security groups are returned regardless of whether they
* have tag keys or values associated with them.
*
*
* @param describeClusterSecurityGroupsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeClusterSecurityGroups operation returned by the
* service.
* @sample AmazonRedshiftAsyncHandler.DescribeClusterSecurityGroups
* @see AWS API Documentation
*/
java.util.concurrent.Future describeClusterSecurityGroupsAsync(
DescribeClusterSecurityGroupsRequest describeClusterSecurityGroupsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
* Simplified method form for invoking the DescribeClusterSecurityGroups operation.
*
* @see #describeClusterSecurityGroupsAsync(DescribeClusterSecurityGroupsRequest)
*/
java.util.concurrent.Future describeClusterSecurityGroupsAsync();
/**
* Simplified method form for invoking the DescribeClusterSecurityGroups operation with an AsyncHandler.
*
* @see #describeClusterSecurityGroupsAsync(DescribeClusterSecurityGroupsRequest,
* com.amazonaws.handlers.AsyncHandler)
*/
java.util.concurrent.Future describeClusterSecurityGroupsAsync(
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns one or more snapshot objects, which contain metadata about your cluster snapshots. By default, this
* operation returns information about all snapshots of all clusters that are owned by your Amazon Web Services
* account. No information is returned for snapshots owned by inactive Amazon Web Services accounts.
*
*
* If you specify both tag keys and tag values in the same request, Amazon Redshift returns all snapshots that match
* any combination of the specified keys and values. For example, if you have owner
and
* environment
for tag keys, and admin
and test
for tag values, all snapshots
* that have any combination of those values are returned. Only snapshots that you own are returned in the response;
* shared snapshots are not returned with the tag key and tag value request parameters.
*
*
* If both tag keys and values are omitted from the request, snapshots are returned regardless of whether they have
* tag keys or values associated with them.
*
*
* @param describeClusterSnapshotsRequest
* @return A Java Future containing the result of the DescribeClusterSnapshots operation returned by the service.
* @sample AmazonRedshiftAsync.DescribeClusterSnapshots
* @see AWS API Documentation
*/
java.util.concurrent.Future describeClusterSnapshotsAsync(DescribeClusterSnapshotsRequest describeClusterSnapshotsRequest);
/**
*
* Returns one or more snapshot objects, which contain metadata about your cluster snapshots. By default, this
* operation returns information about all snapshots of all clusters that are owned by your Amazon Web Services
* account. No information is returned for snapshots owned by inactive Amazon Web Services accounts.
*
*
* If you specify both tag keys and tag values in the same request, Amazon Redshift returns all snapshots that match
* any combination of the specified keys and values. For example, if you have owner
and
* environment
for tag keys, and admin
and test
for tag values, all snapshots
* that have any combination of those values are returned. Only snapshots that you own are returned in the response;
* shared snapshots are not returned with the tag key and tag value request parameters.
*
*
* If both tag keys and values are omitted from the request, snapshots are returned regardless of whether they have
* tag keys or values associated with them.
*
*
* @param describeClusterSnapshotsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeClusterSnapshots operation returned by the service.
* @sample AmazonRedshiftAsyncHandler.DescribeClusterSnapshots
* @see AWS API Documentation
*/
java.util.concurrent.Future describeClusterSnapshotsAsync(DescribeClusterSnapshotsRequest describeClusterSnapshotsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
* Simplified method form for invoking the DescribeClusterSnapshots operation.
*
* @see #describeClusterSnapshotsAsync(DescribeClusterSnapshotsRequest)
*/
java.util.concurrent.Future describeClusterSnapshotsAsync();
/**
* Simplified method form for invoking the DescribeClusterSnapshots operation with an AsyncHandler.
*
* @see #describeClusterSnapshotsAsync(DescribeClusterSnapshotsRequest, com.amazonaws.handlers.AsyncHandler)
*/
java.util.concurrent.Future describeClusterSnapshotsAsync(
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns one or more cluster subnet group objects, which contain metadata about your cluster subnet groups. By
* default, this operation returns information about all cluster subnet groups that are defined in your Amazon Web
* Services account.
*
*
* If you specify both tag keys and tag values in the same request, Amazon Redshift returns all subnet groups that
* match any combination of the specified keys and values. For example, if you have owner
and
* environment
for tag keys, and admin
and test
for tag values, all subnet
* groups that have any combination of those values are returned.
*
*
* If both tag keys and values are omitted from the request, subnet groups are returned regardless of whether they
* have tag keys or values associated with them.
*
*
* @param describeClusterSubnetGroupsRequest
* @return A Java Future containing the result of the DescribeClusterSubnetGroups operation returned by the service.
* @sample AmazonRedshiftAsync.DescribeClusterSubnetGroups
* @see AWS API Documentation
*/
java.util.concurrent.Future describeClusterSubnetGroupsAsync(
DescribeClusterSubnetGroupsRequest describeClusterSubnetGroupsRequest);
/**
*
* Returns one or more cluster subnet group objects, which contain metadata about your cluster subnet groups. By
* default, this operation returns information about all cluster subnet groups that are defined in your Amazon Web
* Services account.
*
*
* If you specify both tag keys and tag values in the same request, Amazon Redshift returns all subnet groups that
* match any combination of the specified keys and values. For example, if you have owner
and
* environment
for tag keys, and admin
and test
for tag values, all subnet
* groups that have any combination of those values are returned.
*
*
* If both tag keys and values are omitted from the request, subnet groups are returned regardless of whether they
* have tag keys or values associated with them.
*
*
* @param describeClusterSubnetGroupsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeClusterSubnetGroups operation returned by the service.
* @sample AmazonRedshiftAsyncHandler.DescribeClusterSubnetGroups
* @see AWS API Documentation
*/
java.util.concurrent.Future describeClusterSubnetGroupsAsync(
DescribeClusterSubnetGroupsRequest describeClusterSubnetGroupsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
* Simplified method form for invoking the DescribeClusterSubnetGroups operation.
*
* @see #describeClusterSubnetGroupsAsync(DescribeClusterSubnetGroupsRequest)
*/
java.util.concurrent.Future describeClusterSubnetGroupsAsync();
/**
* Simplified method form for invoking the DescribeClusterSubnetGroups operation with an AsyncHandler.
*
* @see #describeClusterSubnetGroupsAsync(DescribeClusterSubnetGroupsRequest, com.amazonaws.handlers.AsyncHandler)
*/
java.util.concurrent.Future describeClusterSubnetGroupsAsync(
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns a list of all the available maintenance tracks.
*
*
* @param describeClusterTracksRequest
* @return A Java Future containing the result of the DescribeClusterTracks operation returned by the service.
* @sample AmazonRedshiftAsync.DescribeClusterTracks
* @see AWS
* API Documentation
*/
java.util.concurrent.Future describeClusterTracksAsync(DescribeClusterTracksRequest describeClusterTracksRequest);
/**
*
* Returns a list of all the available maintenance tracks.
*
*
* @param describeClusterTracksRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeClusterTracks operation returned by the service.
* @sample AmazonRedshiftAsyncHandler.DescribeClusterTracks
* @see AWS
* API Documentation
*/
java.util.concurrent.Future describeClusterTracksAsync(DescribeClusterTracksRequest describeClusterTracksRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns descriptions of the available Amazon Redshift cluster versions. You can call this operation even before
* creating any clusters to learn more about the Amazon Redshift versions. For more information about managing
* clusters, go to Amazon
* Redshift Clusters in the Amazon Redshift Cluster Management Guide.
*
*
* @param describeClusterVersionsRequest
* @return A Java Future containing the result of the DescribeClusterVersions operation returned by the service.
* @sample AmazonRedshiftAsync.DescribeClusterVersions
* @see AWS API Documentation
*/
java.util.concurrent.Future describeClusterVersionsAsync(DescribeClusterVersionsRequest describeClusterVersionsRequest);
/**
*
* Returns descriptions of the available Amazon Redshift cluster versions. You can call this operation even before
* creating any clusters to learn more about the Amazon Redshift versions. For more information about managing
* clusters, go to Amazon
* Redshift Clusters in the Amazon Redshift Cluster Management Guide.
*
*
* @param describeClusterVersionsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeClusterVersions operation returned by the service.
* @sample AmazonRedshiftAsyncHandler.DescribeClusterVersions
* @see AWS API Documentation
*/
java.util.concurrent.Future describeClusterVersionsAsync(DescribeClusterVersionsRequest describeClusterVersionsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
* Simplified method form for invoking the DescribeClusterVersions operation.
*
* @see #describeClusterVersionsAsync(DescribeClusterVersionsRequest)
*/
java.util.concurrent.Future describeClusterVersionsAsync();
/**
* Simplified method form for invoking the DescribeClusterVersions operation with an AsyncHandler.
*
* @see #describeClusterVersionsAsync(DescribeClusterVersionsRequest, com.amazonaws.handlers.AsyncHandler)
*/
java.util.concurrent.Future describeClusterVersionsAsync(
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns properties of provisioned clusters including general cluster properties, cluster database properties,
* maintenance and backup properties, and security and access properties. This operation supports pagination. For
* more information about managing clusters, go to Amazon Redshift Clusters
* in the Amazon Redshift Cluster Management Guide.
*
*
* If you specify both tag keys and tag values in the same request, Amazon Redshift returns all clusters that match
* any combination of the specified keys and values. For example, if you have owner
and
* environment
for tag keys, and admin
and test
for tag values, all clusters
* that have any combination of those values are returned.
*
*
* If both tag keys and values are omitted from the request, clusters are returned regardless of whether they have
* tag keys or values associated with them.
*
*
* @param describeClustersRequest
* @return A Java Future containing the result of the DescribeClusters operation returned by the service.
* @sample AmazonRedshiftAsync.DescribeClusters
* @see AWS API
* Documentation
*/
java.util.concurrent.Future describeClustersAsync(DescribeClustersRequest describeClustersRequest);
/**
*
* Returns properties of provisioned clusters including general cluster properties, cluster database properties,
* maintenance and backup properties, and security and access properties. This operation supports pagination. For
* more information about managing clusters, go to Amazon Redshift Clusters
* in the Amazon Redshift Cluster Management Guide.
*
*
* If you specify both tag keys and tag values in the same request, Amazon Redshift returns all clusters that match
* any combination of the specified keys and values. For example, if you have owner
and
* environment
for tag keys, and admin
and test
for tag values, all clusters
* that have any combination of those values are returned.
*
*
* If both tag keys and values are omitted from the request, clusters are returned regardless of whether they have
* tag keys or values associated with them.
*
*
* @param describeClustersRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeClusters operation returned by the service.
* @sample AmazonRedshiftAsyncHandler.DescribeClusters
* @see AWS API
* Documentation
*/
java.util.concurrent.Future describeClustersAsync(DescribeClustersRequest describeClustersRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
* Simplified method form for invoking the DescribeClusters operation.
*
* @see #describeClustersAsync(DescribeClustersRequest)
*/
java.util.concurrent.Future describeClustersAsync();
/**
* Simplified method form for invoking the DescribeClusters operation with an AsyncHandler.
*
* @see #describeClustersAsync(DescribeClustersRequest, com.amazonaws.handlers.AsyncHandler)
*/
java.util.concurrent.Future describeClustersAsync(
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Contains information about custom domain associations for a cluster.
*
*
* @param describeCustomDomainAssociationsRequest
* @return A Java Future containing the result of the DescribeCustomDomainAssociations operation returned by the
* service.
* @sample AmazonRedshiftAsync.DescribeCustomDomainAssociations
* @see AWS API Documentation
*/
java.util.concurrent.Future describeCustomDomainAssociationsAsync(
DescribeCustomDomainAssociationsRequest describeCustomDomainAssociationsRequest);
/**
*
* Contains information about custom domain associations for a cluster.
*
*
* @param describeCustomDomainAssociationsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeCustomDomainAssociations operation returned by the
* service.
* @sample AmazonRedshiftAsyncHandler.DescribeCustomDomainAssociations
* @see AWS API Documentation
*/
java.util.concurrent.Future describeCustomDomainAssociationsAsync(
DescribeCustomDomainAssociationsRequest describeCustomDomainAssociationsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Shows the status of any inbound or outbound datashares available in the specified account.
*
*
* @param describeDataSharesRequest
* @return A Java Future containing the result of the DescribeDataShares operation returned by the service.
* @sample AmazonRedshiftAsync.DescribeDataShares
* @see AWS
* API Documentation
*/
java.util.concurrent.Future describeDataSharesAsync(DescribeDataSharesRequest describeDataSharesRequest);
/**
*
* Shows the status of any inbound or outbound datashares available in the specified account.
*
*
* @param describeDataSharesRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeDataShares operation returned by the service.
* @sample AmazonRedshiftAsyncHandler.DescribeDataShares
* @see AWS
* API Documentation
*/
java.util.concurrent.Future describeDataSharesAsync(DescribeDataSharesRequest describeDataSharesRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns a list of datashares where the account identifier being called is a consumer account identifier.
*
*
* @param describeDataSharesForConsumerRequest
* @return A Java Future containing the result of the DescribeDataSharesForConsumer operation returned by the
* service.
* @sample AmazonRedshiftAsync.DescribeDataSharesForConsumer
* @see AWS API Documentation
*/
java.util.concurrent.Future describeDataSharesForConsumerAsync(
DescribeDataSharesForConsumerRequest describeDataSharesForConsumerRequest);
/**
*
* Returns a list of datashares where the account identifier being called is a consumer account identifier.
*
*
* @param describeDataSharesForConsumerRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeDataSharesForConsumer operation returned by the
* service.
* @sample AmazonRedshiftAsyncHandler.DescribeDataSharesForConsumer
* @see AWS API Documentation
*/
java.util.concurrent.Future describeDataSharesForConsumerAsync(
DescribeDataSharesForConsumerRequest describeDataSharesForConsumerRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns a list of datashares when the account identifier being called is a producer account identifier.
*
*
* @param describeDataSharesForProducerRequest
* @return A Java Future containing the result of the DescribeDataSharesForProducer operation returned by the
* service.
* @sample AmazonRedshiftAsync.DescribeDataSharesForProducer
* @see AWS API Documentation
*/
java.util.concurrent.Future describeDataSharesForProducerAsync(
DescribeDataSharesForProducerRequest describeDataSharesForProducerRequest);
/**
*
* Returns a list of datashares when the account identifier being called is a producer account identifier.
*
*
* @param describeDataSharesForProducerRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeDataSharesForProducer operation returned by the
* service.
* @sample AmazonRedshiftAsyncHandler.DescribeDataSharesForProducer
* @see AWS API Documentation
*/
java.util.concurrent.Future describeDataSharesForProducerAsync(
DescribeDataSharesForProducerRequest describeDataSharesForProducerRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns a list of parameter settings for the specified parameter group family.
*
*
* For more information about parameters and parameter groups, go to Amazon Redshift
* Parameter Groups in the Amazon Redshift Cluster Management Guide.
*
*
* @param describeDefaultClusterParametersRequest
* @return A Java Future containing the result of the DescribeDefaultClusterParameters operation returned by the
* service.
* @sample AmazonRedshiftAsync.DescribeDefaultClusterParameters
* @see AWS API Documentation
*/
java.util.concurrent.Future describeDefaultClusterParametersAsync(
DescribeDefaultClusterParametersRequest describeDefaultClusterParametersRequest);
/**
*
* Returns a list of parameter settings for the specified parameter group family.
*
*
* For more information about parameters and parameter groups, go to Amazon Redshift
* Parameter Groups in the Amazon Redshift Cluster Management Guide.
*
*
* @param describeDefaultClusterParametersRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeDefaultClusterParameters operation returned by the
* service.
* @sample AmazonRedshiftAsyncHandler.DescribeDefaultClusterParameters
* @see AWS API Documentation
*/
java.util.concurrent.Future describeDefaultClusterParametersAsync(
DescribeDefaultClusterParametersRequest describeDefaultClusterParametersRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Describes a Redshift-managed VPC endpoint.
*
*
* @param describeEndpointAccessRequest
* @return A Java Future containing the result of the DescribeEndpointAccess operation returned by the service.
* @sample AmazonRedshiftAsync.DescribeEndpointAccess
* @see AWS API Documentation
*/
java.util.concurrent.Future describeEndpointAccessAsync(DescribeEndpointAccessRequest describeEndpointAccessRequest);
/**
*
* Describes a Redshift-managed VPC endpoint.
*
*
* @param describeEndpointAccessRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeEndpointAccess operation returned by the service.
* @sample AmazonRedshiftAsyncHandler.DescribeEndpointAccess
* @see AWS API Documentation
*/
java.util.concurrent.Future describeEndpointAccessAsync(DescribeEndpointAccessRequest describeEndpointAccessRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Describes an endpoint authorization.
*
*
* @param describeEndpointAuthorizationRequest
* @return A Java Future containing the result of the DescribeEndpointAuthorization operation returned by the
* service.
* @sample AmazonRedshiftAsync.DescribeEndpointAuthorization
* @see AWS API Documentation
*/
java.util.concurrent.Future describeEndpointAuthorizationAsync(
DescribeEndpointAuthorizationRequest describeEndpointAuthorizationRequest);
/**
*
* Describes an endpoint authorization.
*
*
* @param describeEndpointAuthorizationRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeEndpointAuthorization operation returned by the
* service.
* @sample AmazonRedshiftAsyncHandler.DescribeEndpointAuthorization
* @see AWS API Documentation
*/
java.util.concurrent.Future describeEndpointAuthorizationAsync(
DescribeEndpointAuthorizationRequest describeEndpointAuthorizationRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Displays a list of event categories for all event source types, or for a specified source type. For a list of the
* event categories and source types, go to Amazon Redshift
* Event Notifications.
*
*
* @param describeEventCategoriesRequest
* @return A Java Future containing the result of the DescribeEventCategories operation returned by the service.
* @sample AmazonRedshiftAsync.DescribeEventCategories
* @see AWS API Documentation
*/
java.util.concurrent.Future describeEventCategoriesAsync(DescribeEventCategoriesRequest describeEventCategoriesRequest);
/**
*
* Displays a list of event categories for all event source types, or for a specified source type. For a list of the
* event categories and source types, go to Amazon Redshift
* Event Notifications.
*
*
* @param describeEventCategoriesRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeEventCategories operation returned by the service.
* @sample AmazonRedshiftAsyncHandler.DescribeEventCategories
* @see AWS API Documentation
*/
java.util.concurrent.Future describeEventCategoriesAsync(DescribeEventCategoriesRequest describeEventCategoriesRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
* Simplified method form for invoking the DescribeEventCategories operation.
*
* @see #describeEventCategoriesAsync(DescribeEventCategoriesRequest)
*/
java.util.concurrent.Future describeEventCategoriesAsync();
/**
* Simplified method form for invoking the DescribeEventCategories operation with an AsyncHandler.
*
* @see #describeEventCategoriesAsync(DescribeEventCategoriesRequest, com.amazonaws.handlers.AsyncHandler)
*/
java.util.concurrent.Future describeEventCategoriesAsync(
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Lists descriptions of all the Amazon Redshift event notification subscriptions for a customer account. If you
* specify a subscription name, lists the description for that subscription.
*
*
* If you specify both tag keys and tag values in the same request, Amazon Redshift returns all event notification
* subscriptions that match any combination of the specified keys and values. For example, if you have
* owner
and environment
for tag keys, and admin
and test
for
* tag values, all subscriptions that have any combination of those values are returned.
*
*
* If both tag keys and values are omitted from the request, subscriptions are returned regardless of whether they
* have tag keys or values associated with them.
*
*
* @param describeEventSubscriptionsRequest
* @return A Java Future containing the result of the DescribeEventSubscriptions operation returned by the service.
* @sample AmazonRedshiftAsync.DescribeEventSubscriptions
* @see AWS API Documentation
*/
java.util.concurrent.Future describeEventSubscriptionsAsync(
DescribeEventSubscriptionsRequest describeEventSubscriptionsRequest);
/**
*
* Lists descriptions of all the Amazon Redshift event notification subscriptions for a customer account. If you
* specify a subscription name, lists the description for that subscription.
*
*
* If you specify both tag keys and tag values in the same request, Amazon Redshift returns all event notification
* subscriptions that match any combination of the specified keys and values. For example, if you have
* owner
and environment
for tag keys, and admin
and test
for
* tag values, all subscriptions that have any combination of those values are returned.
*
*
* If both tag keys and values are omitted from the request, subscriptions are returned regardless of whether they
* have tag keys or values associated with them.
*
*
* @param describeEventSubscriptionsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeEventSubscriptions operation returned by the service.
* @sample AmazonRedshiftAsyncHandler.DescribeEventSubscriptions
* @see AWS API Documentation
*/
java.util.concurrent.Future describeEventSubscriptionsAsync(
DescribeEventSubscriptionsRequest describeEventSubscriptionsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
* Simplified method form for invoking the DescribeEventSubscriptions operation.
*
* @see #describeEventSubscriptionsAsync(DescribeEventSubscriptionsRequest)
*/
java.util.concurrent.Future describeEventSubscriptionsAsync();
/**
* Simplified method form for invoking the DescribeEventSubscriptions operation with an AsyncHandler.
*
* @see #describeEventSubscriptionsAsync(DescribeEventSubscriptionsRequest, com.amazonaws.handlers.AsyncHandler)
*/
java.util.concurrent.Future describeEventSubscriptionsAsync(
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns events related to clusters, security groups, snapshots, and parameter groups for the past 14 days. Events
* specific to a particular cluster, security group, snapshot or parameter group can be obtained by providing the
* name as a parameter. By default, the past hour of events are returned.
*
*
* @param describeEventsRequest
* @return A Java Future containing the result of the DescribeEvents operation returned by the service.
* @sample AmazonRedshiftAsync.DescribeEvents
* @see AWS API
* Documentation
*/
java.util.concurrent.Future describeEventsAsync(DescribeEventsRequest describeEventsRequest);
/**
*
* Returns events related to clusters, security groups, snapshots, and parameter groups for the past 14 days. Events
* specific to a particular cluster, security group, snapshot or parameter group can be obtained by providing the
* name as a parameter. By default, the past hour of events are returned.
*
*
* @param describeEventsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeEvents operation returned by the service.
* @sample AmazonRedshiftAsyncHandler.DescribeEvents
* @see AWS API
* Documentation
*/
java.util.concurrent.Future describeEventsAsync(DescribeEventsRequest describeEventsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
* Simplified method form for invoking the DescribeEvents operation.
*
* @see #describeEventsAsync(DescribeEventsRequest)
*/
java.util.concurrent.Future describeEventsAsync();
/**
* Simplified method form for invoking the DescribeEvents operation with an AsyncHandler.
*
* @see #describeEventsAsync(DescribeEventsRequest, com.amazonaws.handlers.AsyncHandler)
*/
java.util.concurrent.Future describeEventsAsync(
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns information about the specified HSM client certificate. If no certificate ID is specified, returns
* information about all the HSM certificates owned by your Amazon Web Services account.
*
*
* If you specify both tag keys and tag values in the same request, Amazon Redshift returns all HSM client
* certificates that match any combination of the specified keys and values. For example, if you have
* owner
and environment
for tag keys, and admin
and test
for
* tag values, all HSM client certificates that have any combination of those values are returned.
*
*
* If both tag keys and values are omitted from the request, HSM client certificates are returned regardless of
* whether they have tag keys or values associated with them.
*
*
* @param describeHsmClientCertificatesRequest
* @return A Java Future containing the result of the DescribeHsmClientCertificates operation returned by the
* service.
* @sample AmazonRedshiftAsync.DescribeHsmClientCertificates
* @see AWS API Documentation
*/
java.util.concurrent.Future describeHsmClientCertificatesAsync(
DescribeHsmClientCertificatesRequest describeHsmClientCertificatesRequest);
/**
*
* Returns information about the specified HSM client certificate. If no certificate ID is specified, returns
* information about all the HSM certificates owned by your Amazon Web Services account.
*
*
* If you specify both tag keys and tag values in the same request, Amazon Redshift returns all HSM client
* certificates that match any combination of the specified keys and values. For example, if you have
* owner
and environment
for tag keys, and admin
and test
for
* tag values, all HSM client certificates that have any combination of those values are returned.
*
*
* If both tag keys and values are omitted from the request, HSM client certificates are returned regardless of
* whether they have tag keys or values associated with them.
*
*
* @param describeHsmClientCertificatesRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeHsmClientCertificates operation returned by the
* service.
* @sample AmazonRedshiftAsyncHandler.DescribeHsmClientCertificates
* @see AWS API Documentation
*/
java.util.concurrent.Future describeHsmClientCertificatesAsync(
DescribeHsmClientCertificatesRequest describeHsmClientCertificatesRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
* Simplified method form for invoking the DescribeHsmClientCertificates operation.
*
* @see #describeHsmClientCertificatesAsync(DescribeHsmClientCertificatesRequest)
*/
java.util.concurrent.Future describeHsmClientCertificatesAsync();
/**
* Simplified method form for invoking the DescribeHsmClientCertificates operation with an AsyncHandler.
*
* @see #describeHsmClientCertificatesAsync(DescribeHsmClientCertificatesRequest,
* com.amazonaws.handlers.AsyncHandler)
*/
java.util.concurrent.Future describeHsmClientCertificatesAsync(
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns information about the specified Amazon Redshift HSM configuration. If no configuration ID is specified,
* returns information about all the HSM configurations owned by your Amazon Web Services account.
*
*
* If you specify both tag keys and tag values in the same request, Amazon Redshift returns all HSM connections that
* match any combination of the specified keys and values. For example, if you have owner
and
* environment
for tag keys, and admin
and test
for tag values, all HSM
* connections that have any combination of those values are returned.
*
*
* If both tag keys and values are omitted from the request, HSM connections are returned regardless of whether they
* have tag keys or values associated with them.
*
*
* @param describeHsmConfigurationsRequest
* @return A Java Future containing the result of the DescribeHsmConfigurations operation returned by the service.
* @sample AmazonRedshiftAsync.DescribeHsmConfigurations
* @see AWS API Documentation
*/
java.util.concurrent.Future describeHsmConfigurationsAsync(
DescribeHsmConfigurationsRequest describeHsmConfigurationsRequest);
/**
*
* Returns information about the specified Amazon Redshift HSM configuration. If no configuration ID is specified,
* returns information about all the HSM configurations owned by your Amazon Web Services account.
*
*
* If you specify both tag keys and tag values in the same request, Amazon Redshift returns all HSM connections that
* match any combination of the specified keys and values. For example, if you have owner
and
* environment
for tag keys, and admin
and test
for tag values, all HSM
* connections that have any combination of those values are returned.
*
*
* If both tag keys and values are omitted from the request, HSM connections are returned regardless of whether they
* have tag keys or values associated with them.
*
*
* @param describeHsmConfigurationsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeHsmConfigurations operation returned by the service.
* @sample AmazonRedshiftAsyncHandler.DescribeHsmConfigurations
* @see AWS API Documentation
*/
java.util.concurrent.Future describeHsmConfigurationsAsync(
DescribeHsmConfigurationsRequest describeHsmConfigurationsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
* Simplified method form for invoking the DescribeHsmConfigurations operation.
*
* @see #describeHsmConfigurationsAsync(DescribeHsmConfigurationsRequest)
*/
java.util.concurrent.Future describeHsmConfigurationsAsync();
/**
* Simplified method form for invoking the DescribeHsmConfigurations operation with an AsyncHandler.
*
* @see #describeHsmConfigurationsAsync(DescribeHsmConfigurationsRequest, com.amazonaws.handlers.AsyncHandler)
*/
java.util.concurrent.Future describeHsmConfigurationsAsync(
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns a list of inbound integrations.
*
*
* @param describeInboundIntegrationsRequest
* @return A Java Future containing the result of the DescribeInboundIntegrations operation returned by the service.
* @sample AmazonRedshiftAsync.DescribeInboundIntegrations
* @see AWS API Documentation
*/
java.util.concurrent.Future describeInboundIntegrationsAsync(
DescribeInboundIntegrationsRequest describeInboundIntegrationsRequest);
/**
*
* Returns a list of inbound integrations.
*
*
* @param describeInboundIntegrationsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeInboundIntegrations operation returned by the service.
* @sample AmazonRedshiftAsyncHandler.DescribeInboundIntegrations
* @see AWS API Documentation
*/
java.util.concurrent.Future describeInboundIntegrationsAsync(
DescribeInboundIntegrationsRequest describeInboundIntegrationsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Describes whether information, such as queries and connection attempts, is being logged for the specified Amazon
* Redshift cluster.
*
*
* @param describeLoggingStatusRequest
* @return A Java Future containing the result of the DescribeLoggingStatus operation returned by the service.
* @sample AmazonRedshiftAsync.DescribeLoggingStatus
* @see AWS
* API Documentation
*/
java.util.concurrent.Future describeLoggingStatusAsync(DescribeLoggingStatusRequest describeLoggingStatusRequest);
/**
*