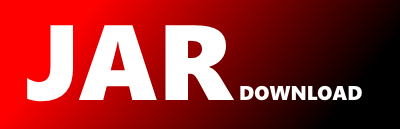
com.amazonaws.services.redshift.model.DescribeClusterVersionsRequest Maven / Gradle / Ivy
Show all versions of aws-java-sdk-redshift Show documentation
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.redshift.model;
import java.io.Serializable;
import javax.annotation.Generated;
import com.amazonaws.AmazonWebServiceRequest;
/**
*
*
* @see AWS
* API Documentation
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class DescribeClusterVersionsRequest extends com.amazonaws.AmazonWebServiceRequest implements Serializable, Cloneable {
/**
*
* The specific cluster version to return.
*
*
* Example: 1.0
*
*/
private String clusterVersion;
/**
*
* The name of a specific cluster parameter group family to return details for.
*
*
* Constraints:
*
*
* -
*
* Must be 1 to 255 alphanumeric characters
*
*
* -
*
* First character must be a letter
*
*
* -
*
* Cannot end with a hyphen or contain two consecutive hyphens
*
*
*
*/
private String clusterParameterGroupFamily;
/**
*
* The maximum number of response records to return in each call. If the number of remaining response records
* exceeds the specified MaxRecords
value, a value is returned in a marker
field of the
* response. You can retrieve the next set of records by retrying the command with the returned marker value.
*
*
* Default: 100
*
*
* Constraints: minimum 20, maximum 100.
*
*/
private Integer maxRecords;
/**
*
* An optional parameter that specifies the starting point to return a set of response records. When the results of
* a DescribeClusterVersions request exceed the value specified in MaxRecords
, Amazon Web
* Services returns a value in the Marker
field of the response. You can retrieve the next set of
* response records by providing the returned marker value in the Marker
parameter and retrying the
* request.
*
*/
private String marker;
/**
*
* The specific cluster version to return.
*
*
* Example: 1.0
*
*
* @param clusterVersion
* The specific cluster version to return.
*
* Example: 1.0
*/
public void setClusterVersion(String clusterVersion) {
this.clusterVersion = clusterVersion;
}
/**
*
* The specific cluster version to return.
*
*
* Example: 1.0
*
*
* @return The specific cluster version to return.
*
* Example: 1.0
*/
public String getClusterVersion() {
return this.clusterVersion;
}
/**
*
* The specific cluster version to return.
*
*
* Example: 1.0
*
*
* @param clusterVersion
* The specific cluster version to return.
*
* Example: 1.0
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DescribeClusterVersionsRequest withClusterVersion(String clusterVersion) {
setClusterVersion(clusterVersion);
return this;
}
/**
*
* The name of a specific cluster parameter group family to return details for.
*
*
* Constraints:
*
*
* -
*
* Must be 1 to 255 alphanumeric characters
*
*
* -
*
* First character must be a letter
*
*
* -
*
* Cannot end with a hyphen or contain two consecutive hyphens
*
*
*
*
* @param clusterParameterGroupFamily
* The name of a specific cluster parameter group family to return details for.
*
* Constraints:
*
*
* -
*
* Must be 1 to 255 alphanumeric characters
*
*
* -
*
* First character must be a letter
*
*
* -
*
* Cannot end with a hyphen or contain two consecutive hyphens
*
*
*/
public void setClusterParameterGroupFamily(String clusterParameterGroupFamily) {
this.clusterParameterGroupFamily = clusterParameterGroupFamily;
}
/**
*
* The name of a specific cluster parameter group family to return details for.
*
*
* Constraints:
*
*
* -
*
* Must be 1 to 255 alphanumeric characters
*
*
* -
*
* First character must be a letter
*
*
* -
*
* Cannot end with a hyphen or contain two consecutive hyphens
*
*
*
*
* @return The name of a specific cluster parameter group family to return details for.
*
* Constraints:
*
*
* -
*
* Must be 1 to 255 alphanumeric characters
*
*
* -
*
* First character must be a letter
*
*
* -
*
* Cannot end with a hyphen or contain two consecutive hyphens
*
*
*/
public String getClusterParameterGroupFamily() {
return this.clusterParameterGroupFamily;
}
/**
*
* The name of a specific cluster parameter group family to return details for.
*
*
* Constraints:
*
*
* -
*
* Must be 1 to 255 alphanumeric characters
*
*
* -
*
* First character must be a letter
*
*
* -
*
* Cannot end with a hyphen or contain two consecutive hyphens
*
*
*
*
* @param clusterParameterGroupFamily
* The name of a specific cluster parameter group family to return details for.
*
* Constraints:
*
*
* -
*
* Must be 1 to 255 alphanumeric characters
*
*
* -
*
* First character must be a letter
*
*
* -
*
* Cannot end with a hyphen or contain two consecutive hyphens
*
*
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DescribeClusterVersionsRequest withClusterParameterGroupFamily(String clusterParameterGroupFamily) {
setClusterParameterGroupFamily(clusterParameterGroupFamily);
return this;
}
/**
*
* The maximum number of response records to return in each call. If the number of remaining response records
* exceeds the specified MaxRecords
value, a value is returned in a marker
field of the
* response. You can retrieve the next set of records by retrying the command with the returned marker value.
*
*
* Default: 100
*
*
* Constraints: minimum 20, maximum 100.
*
*
* @param maxRecords
* The maximum number of response records to return in each call. If the number of remaining response records
* exceeds the specified MaxRecords
value, a value is returned in a marker
field of
* the response. You can retrieve the next set of records by retrying the command with the returned marker
* value.
*
* Default: 100
*
*
* Constraints: minimum 20, maximum 100.
*/
public void setMaxRecords(Integer maxRecords) {
this.maxRecords = maxRecords;
}
/**
*
* The maximum number of response records to return in each call. If the number of remaining response records
* exceeds the specified MaxRecords
value, a value is returned in a marker
field of the
* response. You can retrieve the next set of records by retrying the command with the returned marker value.
*
*
* Default: 100
*
*
* Constraints: minimum 20, maximum 100.
*
*
* @return The maximum number of response records to return in each call. If the number of remaining response
* records exceeds the specified MaxRecords
value, a value is returned in a marker
* field of the response. You can retrieve the next set of records by retrying the command with the returned
* marker value.
*
* Default: 100
*
*
* Constraints: minimum 20, maximum 100.
*/
public Integer getMaxRecords() {
return this.maxRecords;
}
/**
*
* The maximum number of response records to return in each call. If the number of remaining response records
* exceeds the specified MaxRecords
value, a value is returned in a marker
field of the
* response. You can retrieve the next set of records by retrying the command with the returned marker value.
*
*
* Default: 100
*
*
* Constraints: minimum 20, maximum 100.
*
*
* @param maxRecords
* The maximum number of response records to return in each call. If the number of remaining response records
* exceeds the specified MaxRecords
value, a value is returned in a marker
field of
* the response. You can retrieve the next set of records by retrying the command with the returned marker
* value.
*
* Default: 100
*
*
* Constraints: minimum 20, maximum 100.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DescribeClusterVersionsRequest withMaxRecords(Integer maxRecords) {
setMaxRecords(maxRecords);
return this;
}
/**
*
* An optional parameter that specifies the starting point to return a set of response records. When the results of
* a DescribeClusterVersions request exceed the value specified in MaxRecords
, Amazon Web
* Services returns a value in the Marker
field of the response. You can retrieve the next set of
* response records by providing the returned marker value in the Marker
parameter and retrying the
* request.
*
*
* @param marker
* An optional parameter that specifies the starting point to return a set of response records. When the
* results of a DescribeClusterVersions request exceed the value specified in MaxRecords
,
* Amazon Web Services returns a value in the Marker
field of the response. You can retrieve the
* next set of response records by providing the returned marker value in the Marker
parameter
* and retrying the request.
*/
public void setMarker(String marker) {
this.marker = marker;
}
/**
*
* An optional parameter that specifies the starting point to return a set of response records. When the results of
* a DescribeClusterVersions request exceed the value specified in MaxRecords
, Amazon Web
* Services returns a value in the Marker
field of the response. You can retrieve the next set of
* response records by providing the returned marker value in the Marker
parameter and retrying the
* request.
*
*
* @return An optional parameter that specifies the starting point to return a set of response records. When the
* results of a DescribeClusterVersions request exceed the value specified in MaxRecords
* , Amazon Web Services returns a value in the Marker
field of the response. You can retrieve
* the next set of response records by providing the returned marker value in the Marker
* parameter and retrying the request.
*/
public String getMarker() {
return this.marker;
}
/**
*
* An optional parameter that specifies the starting point to return a set of response records. When the results of
* a DescribeClusterVersions request exceed the value specified in MaxRecords
, Amazon Web
* Services returns a value in the Marker
field of the response. You can retrieve the next set of
* response records by providing the returned marker value in the Marker
parameter and retrying the
* request.
*
*
* @param marker
* An optional parameter that specifies the starting point to return a set of response records. When the
* results of a DescribeClusterVersions request exceed the value specified in MaxRecords
,
* Amazon Web Services returns a value in the Marker
field of the response. You can retrieve the
* next set of response records by providing the returned marker value in the Marker
parameter
* and retrying the request.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DescribeClusterVersionsRequest withMarker(String marker) {
setMarker(marker);
return this;
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getClusterVersion() != null)
sb.append("ClusterVersion: ").append(getClusterVersion()).append(",");
if (getClusterParameterGroupFamily() != null)
sb.append("ClusterParameterGroupFamily: ").append(getClusterParameterGroupFamily()).append(",");
if (getMaxRecords() != null)
sb.append("MaxRecords: ").append(getMaxRecords()).append(",");
if (getMarker() != null)
sb.append("Marker: ").append(getMarker());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof DescribeClusterVersionsRequest == false)
return false;
DescribeClusterVersionsRequest other = (DescribeClusterVersionsRequest) obj;
if (other.getClusterVersion() == null ^ this.getClusterVersion() == null)
return false;
if (other.getClusterVersion() != null && other.getClusterVersion().equals(this.getClusterVersion()) == false)
return false;
if (other.getClusterParameterGroupFamily() == null ^ this.getClusterParameterGroupFamily() == null)
return false;
if (other.getClusterParameterGroupFamily() != null && other.getClusterParameterGroupFamily().equals(this.getClusterParameterGroupFamily()) == false)
return false;
if (other.getMaxRecords() == null ^ this.getMaxRecords() == null)
return false;
if (other.getMaxRecords() != null && other.getMaxRecords().equals(this.getMaxRecords()) == false)
return false;
if (other.getMarker() == null ^ this.getMarker() == null)
return false;
if (other.getMarker() != null && other.getMarker().equals(this.getMarker()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getClusterVersion() == null) ? 0 : getClusterVersion().hashCode());
hashCode = prime * hashCode + ((getClusterParameterGroupFamily() == null) ? 0 : getClusterParameterGroupFamily().hashCode());
hashCode = prime * hashCode + ((getMaxRecords() == null) ? 0 : getMaxRecords().hashCode());
hashCode = prime * hashCode + ((getMarker() == null) ? 0 : getMarker().hashCode());
return hashCode;
}
@Override
public DescribeClusterVersionsRequest clone() {
return (DescribeClusterVersionsRequest) super.clone();
}
}