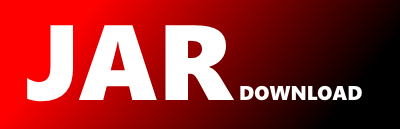
com.amazonaws.services.redshift.model.EndpointAccess Maven / Gradle / Ivy
Show all versions of aws-java-sdk-redshift Show documentation
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.redshift.model;
import java.io.Serializable;
import javax.annotation.Generated;
/**
*
* Describes a Redshift-managed VPC endpoint.
*
*
* @see AWS API
* Documentation
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class EndpointAccess implements Serializable, Cloneable {
/**
*
* The cluster identifier of the cluster associated with the endpoint.
*
*/
private String clusterIdentifier;
/**
*
* The Amazon Web Services account ID of the owner of the cluster.
*
*/
private String resourceOwner;
/**
*
* The subnet group name where Amazon Redshift chooses to deploy the endpoint.
*
*/
private String subnetGroupName;
/**
*
* The status of the endpoint.
*
*/
private String endpointStatus;
/**
*
* The name of the endpoint.
*
*/
private String endpointName;
/**
*
* The time (UTC) that the endpoint was created.
*
*/
private java.util.Date endpointCreateTime;
/**
*
* The port number on which the cluster accepts incoming connections.
*
*/
private Integer port;
/**
*
* The DNS address of the endpoint.
*
*/
private String address;
/**
*
* The security groups associated with the endpoint.
*
*/
private com.amazonaws.internal.SdkInternalList vpcSecurityGroups;
private VpcEndpoint vpcEndpoint;
/**
*
* The cluster identifier of the cluster associated with the endpoint.
*
*
* @param clusterIdentifier
* The cluster identifier of the cluster associated with the endpoint.
*/
public void setClusterIdentifier(String clusterIdentifier) {
this.clusterIdentifier = clusterIdentifier;
}
/**
*
* The cluster identifier of the cluster associated with the endpoint.
*
*
* @return The cluster identifier of the cluster associated with the endpoint.
*/
public String getClusterIdentifier() {
return this.clusterIdentifier;
}
/**
*
* The cluster identifier of the cluster associated with the endpoint.
*
*
* @param clusterIdentifier
* The cluster identifier of the cluster associated with the endpoint.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public EndpointAccess withClusterIdentifier(String clusterIdentifier) {
setClusterIdentifier(clusterIdentifier);
return this;
}
/**
*
* The Amazon Web Services account ID of the owner of the cluster.
*
*
* @param resourceOwner
* The Amazon Web Services account ID of the owner of the cluster.
*/
public void setResourceOwner(String resourceOwner) {
this.resourceOwner = resourceOwner;
}
/**
*
* The Amazon Web Services account ID of the owner of the cluster.
*
*
* @return The Amazon Web Services account ID of the owner of the cluster.
*/
public String getResourceOwner() {
return this.resourceOwner;
}
/**
*
* The Amazon Web Services account ID of the owner of the cluster.
*
*
* @param resourceOwner
* The Amazon Web Services account ID of the owner of the cluster.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public EndpointAccess withResourceOwner(String resourceOwner) {
setResourceOwner(resourceOwner);
return this;
}
/**
*
* The subnet group name where Amazon Redshift chooses to deploy the endpoint.
*
*
* @param subnetGroupName
* The subnet group name where Amazon Redshift chooses to deploy the endpoint.
*/
public void setSubnetGroupName(String subnetGroupName) {
this.subnetGroupName = subnetGroupName;
}
/**
*
* The subnet group name where Amazon Redshift chooses to deploy the endpoint.
*
*
* @return The subnet group name where Amazon Redshift chooses to deploy the endpoint.
*/
public String getSubnetGroupName() {
return this.subnetGroupName;
}
/**
*
* The subnet group name where Amazon Redshift chooses to deploy the endpoint.
*
*
* @param subnetGroupName
* The subnet group name where Amazon Redshift chooses to deploy the endpoint.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public EndpointAccess withSubnetGroupName(String subnetGroupName) {
setSubnetGroupName(subnetGroupName);
return this;
}
/**
*
* The status of the endpoint.
*
*
* @param endpointStatus
* The status of the endpoint.
*/
public void setEndpointStatus(String endpointStatus) {
this.endpointStatus = endpointStatus;
}
/**
*
* The status of the endpoint.
*
*
* @return The status of the endpoint.
*/
public String getEndpointStatus() {
return this.endpointStatus;
}
/**
*
* The status of the endpoint.
*
*
* @param endpointStatus
* The status of the endpoint.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public EndpointAccess withEndpointStatus(String endpointStatus) {
setEndpointStatus(endpointStatus);
return this;
}
/**
*
* The name of the endpoint.
*
*
* @param endpointName
* The name of the endpoint.
*/
public void setEndpointName(String endpointName) {
this.endpointName = endpointName;
}
/**
*
* The name of the endpoint.
*
*
* @return The name of the endpoint.
*/
public String getEndpointName() {
return this.endpointName;
}
/**
*
* The name of the endpoint.
*
*
* @param endpointName
* The name of the endpoint.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public EndpointAccess withEndpointName(String endpointName) {
setEndpointName(endpointName);
return this;
}
/**
*
* The time (UTC) that the endpoint was created.
*
*
* @param endpointCreateTime
* The time (UTC) that the endpoint was created.
*/
public void setEndpointCreateTime(java.util.Date endpointCreateTime) {
this.endpointCreateTime = endpointCreateTime;
}
/**
*
* The time (UTC) that the endpoint was created.
*
*
* @return The time (UTC) that the endpoint was created.
*/
public java.util.Date getEndpointCreateTime() {
return this.endpointCreateTime;
}
/**
*
* The time (UTC) that the endpoint was created.
*
*
* @param endpointCreateTime
* The time (UTC) that the endpoint was created.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public EndpointAccess withEndpointCreateTime(java.util.Date endpointCreateTime) {
setEndpointCreateTime(endpointCreateTime);
return this;
}
/**
*
* The port number on which the cluster accepts incoming connections.
*
*
* @param port
* The port number on which the cluster accepts incoming connections.
*/
public void setPort(Integer port) {
this.port = port;
}
/**
*
* The port number on which the cluster accepts incoming connections.
*
*
* @return The port number on which the cluster accepts incoming connections.
*/
public Integer getPort() {
return this.port;
}
/**
*
* The port number on which the cluster accepts incoming connections.
*
*
* @param port
* The port number on which the cluster accepts incoming connections.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public EndpointAccess withPort(Integer port) {
setPort(port);
return this;
}
/**
*
* The DNS address of the endpoint.
*
*
* @param address
* The DNS address of the endpoint.
*/
public void setAddress(String address) {
this.address = address;
}
/**
*
* The DNS address of the endpoint.
*
*
* @return The DNS address of the endpoint.
*/
public String getAddress() {
return this.address;
}
/**
*
* The DNS address of the endpoint.
*
*
* @param address
* The DNS address of the endpoint.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public EndpointAccess withAddress(String address) {
setAddress(address);
return this;
}
/**
*
* The security groups associated with the endpoint.
*
*
* @return The security groups associated with the endpoint.
*/
public java.util.List getVpcSecurityGroups() {
if (vpcSecurityGroups == null) {
vpcSecurityGroups = new com.amazonaws.internal.SdkInternalList();
}
return vpcSecurityGroups;
}
/**
*
* The security groups associated with the endpoint.
*
*
* @param vpcSecurityGroups
* The security groups associated with the endpoint.
*/
public void setVpcSecurityGroups(java.util.Collection vpcSecurityGroups) {
if (vpcSecurityGroups == null) {
this.vpcSecurityGroups = null;
return;
}
this.vpcSecurityGroups = new com.amazonaws.internal.SdkInternalList(vpcSecurityGroups);
}
/**
*
* The security groups associated with the endpoint.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setVpcSecurityGroups(java.util.Collection)} or {@link #withVpcSecurityGroups(java.util.Collection)} if
* you want to override the existing values.
*
*
* @param vpcSecurityGroups
* The security groups associated with the endpoint.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public EndpointAccess withVpcSecurityGroups(VpcSecurityGroupMembership... vpcSecurityGroups) {
if (this.vpcSecurityGroups == null) {
setVpcSecurityGroups(new com.amazonaws.internal.SdkInternalList(vpcSecurityGroups.length));
}
for (VpcSecurityGroupMembership ele : vpcSecurityGroups) {
this.vpcSecurityGroups.add(ele);
}
return this;
}
/**
*
* The security groups associated with the endpoint.
*
*
* @param vpcSecurityGroups
* The security groups associated with the endpoint.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public EndpointAccess withVpcSecurityGroups(java.util.Collection vpcSecurityGroups) {
setVpcSecurityGroups(vpcSecurityGroups);
return this;
}
/**
* @param vpcEndpoint
*/
public void setVpcEndpoint(VpcEndpoint vpcEndpoint) {
this.vpcEndpoint = vpcEndpoint;
}
/**
* @return
*/
public VpcEndpoint getVpcEndpoint() {
return this.vpcEndpoint;
}
/**
* @param vpcEndpoint
* @return Returns a reference to this object so that method calls can be chained together.
*/
public EndpointAccess withVpcEndpoint(VpcEndpoint vpcEndpoint) {
setVpcEndpoint(vpcEndpoint);
return this;
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getClusterIdentifier() != null)
sb.append("ClusterIdentifier: ").append(getClusterIdentifier()).append(",");
if (getResourceOwner() != null)
sb.append("ResourceOwner: ").append(getResourceOwner()).append(",");
if (getSubnetGroupName() != null)
sb.append("SubnetGroupName: ").append(getSubnetGroupName()).append(",");
if (getEndpointStatus() != null)
sb.append("EndpointStatus: ").append(getEndpointStatus()).append(",");
if (getEndpointName() != null)
sb.append("EndpointName: ").append(getEndpointName()).append(",");
if (getEndpointCreateTime() != null)
sb.append("EndpointCreateTime: ").append(getEndpointCreateTime()).append(",");
if (getPort() != null)
sb.append("Port: ").append(getPort()).append(",");
if (getAddress() != null)
sb.append("Address: ").append(getAddress()).append(",");
if (getVpcSecurityGroups() != null)
sb.append("VpcSecurityGroups: ").append(getVpcSecurityGroups()).append(",");
if (getVpcEndpoint() != null)
sb.append("VpcEndpoint: ").append(getVpcEndpoint());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof EndpointAccess == false)
return false;
EndpointAccess other = (EndpointAccess) obj;
if (other.getClusterIdentifier() == null ^ this.getClusterIdentifier() == null)
return false;
if (other.getClusterIdentifier() != null && other.getClusterIdentifier().equals(this.getClusterIdentifier()) == false)
return false;
if (other.getResourceOwner() == null ^ this.getResourceOwner() == null)
return false;
if (other.getResourceOwner() != null && other.getResourceOwner().equals(this.getResourceOwner()) == false)
return false;
if (other.getSubnetGroupName() == null ^ this.getSubnetGroupName() == null)
return false;
if (other.getSubnetGroupName() != null && other.getSubnetGroupName().equals(this.getSubnetGroupName()) == false)
return false;
if (other.getEndpointStatus() == null ^ this.getEndpointStatus() == null)
return false;
if (other.getEndpointStatus() != null && other.getEndpointStatus().equals(this.getEndpointStatus()) == false)
return false;
if (other.getEndpointName() == null ^ this.getEndpointName() == null)
return false;
if (other.getEndpointName() != null && other.getEndpointName().equals(this.getEndpointName()) == false)
return false;
if (other.getEndpointCreateTime() == null ^ this.getEndpointCreateTime() == null)
return false;
if (other.getEndpointCreateTime() != null && other.getEndpointCreateTime().equals(this.getEndpointCreateTime()) == false)
return false;
if (other.getPort() == null ^ this.getPort() == null)
return false;
if (other.getPort() != null && other.getPort().equals(this.getPort()) == false)
return false;
if (other.getAddress() == null ^ this.getAddress() == null)
return false;
if (other.getAddress() != null && other.getAddress().equals(this.getAddress()) == false)
return false;
if (other.getVpcSecurityGroups() == null ^ this.getVpcSecurityGroups() == null)
return false;
if (other.getVpcSecurityGroups() != null && other.getVpcSecurityGroups().equals(this.getVpcSecurityGroups()) == false)
return false;
if (other.getVpcEndpoint() == null ^ this.getVpcEndpoint() == null)
return false;
if (other.getVpcEndpoint() != null && other.getVpcEndpoint().equals(this.getVpcEndpoint()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getClusterIdentifier() == null) ? 0 : getClusterIdentifier().hashCode());
hashCode = prime * hashCode + ((getResourceOwner() == null) ? 0 : getResourceOwner().hashCode());
hashCode = prime * hashCode + ((getSubnetGroupName() == null) ? 0 : getSubnetGroupName().hashCode());
hashCode = prime * hashCode + ((getEndpointStatus() == null) ? 0 : getEndpointStatus().hashCode());
hashCode = prime * hashCode + ((getEndpointName() == null) ? 0 : getEndpointName().hashCode());
hashCode = prime * hashCode + ((getEndpointCreateTime() == null) ? 0 : getEndpointCreateTime().hashCode());
hashCode = prime * hashCode + ((getPort() == null) ? 0 : getPort().hashCode());
hashCode = prime * hashCode + ((getAddress() == null) ? 0 : getAddress().hashCode());
hashCode = prime * hashCode + ((getVpcSecurityGroups() == null) ? 0 : getVpcSecurityGroups().hashCode());
hashCode = prime * hashCode + ((getVpcEndpoint() == null) ? 0 : getVpcEndpoint().hashCode());
return hashCode;
}
@Override
public EndpointAccess clone() {
try {
return (EndpointAccess) super.clone();
} catch (CloneNotSupportedException e) {
throw new IllegalStateException("Got a CloneNotSupportedException from Object.clone() " + "even though we're Cloneable!", e);
}
}
}