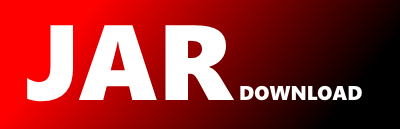
com.amazonaws.services.redshift.model.RejectDataShareResult Maven / Gradle / Ivy
Show all versions of aws-java-sdk-redshift Show documentation
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.redshift.model;
import java.io.Serializable;
import javax.annotation.Generated;
/**
*
* @see AWS API
* Documentation
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class RejectDataShareResult extends com.amazonaws.AmazonWebServiceResult implements Serializable, Cloneable {
/**
*
* The Amazon Resource Name (ARN) of the datashare that the consumer is to use.
*
*/
private String dataShareArn;
/**
*
* The Amazon Resource Name (ARN) of the producer namespace.
*
*/
private String producerArn;
/**
*
* A value that specifies whether the datashare can be shared to a publicly accessible cluster.
*
*/
private Boolean allowPubliclyAccessibleConsumers;
/**
*
* A value that specifies when the datashare has an association between producer and data consumers.
*
*/
private com.amazonaws.internal.SdkInternalList dataShareAssociations;
/**
*
* The identifier of a datashare to show its managing entity.
*
*/
private String managedBy;
/**
*
* The Amazon Resource Name (ARN) of the datashare that the consumer is to use.
*
*
* @param dataShareArn
* The Amazon Resource Name (ARN) of the datashare that the consumer is to use.
*/
public void setDataShareArn(String dataShareArn) {
this.dataShareArn = dataShareArn;
}
/**
*
* The Amazon Resource Name (ARN) of the datashare that the consumer is to use.
*
*
* @return The Amazon Resource Name (ARN) of the datashare that the consumer is to use.
*/
public String getDataShareArn() {
return this.dataShareArn;
}
/**
*
* The Amazon Resource Name (ARN) of the datashare that the consumer is to use.
*
*
* @param dataShareArn
* The Amazon Resource Name (ARN) of the datashare that the consumer is to use.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public RejectDataShareResult withDataShareArn(String dataShareArn) {
setDataShareArn(dataShareArn);
return this;
}
/**
*
* The Amazon Resource Name (ARN) of the producer namespace.
*
*
* @param producerArn
* The Amazon Resource Name (ARN) of the producer namespace.
*/
public void setProducerArn(String producerArn) {
this.producerArn = producerArn;
}
/**
*
* The Amazon Resource Name (ARN) of the producer namespace.
*
*
* @return The Amazon Resource Name (ARN) of the producer namespace.
*/
public String getProducerArn() {
return this.producerArn;
}
/**
*
* The Amazon Resource Name (ARN) of the producer namespace.
*
*
* @param producerArn
* The Amazon Resource Name (ARN) of the producer namespace.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public RejectDataShareResult withProducerArn(String producerArn) {
setProducerArn(producerArn);
return this;
}
/**
*
* A value that specifies whether the datashare can be shared to a publicly accessible cluster.
*
*
* @param allowPubliclyAccessibleConsumers
* A value that specifies whether the datashare can be shared to a publicly accessible cluster.
*/
public void setAllowPubliclyAccessibleConsumers(Boolean allowPubliclyAccessibleConsumers) {
this.allowPubliclyAccessibleConsumers = allowPubliclyAccessibleConsumers;
}
/**
*
* A value that specifies whether the datashare can be shared to a publicly accessible cluster.
*
*
* @return A value that specifies whether the datashare can be shared to a publicly accessible cluster.
*/
public Boolean getAllowPubliclyAccessibleConsumers() {
return this.allowPubliclyAccessibleConsumers;
}
/**
*
* A value that specifies whether the datashare can be shared to a publicly accessible cluster.
*
*
* @param allowPubliclyAccessibleConsumers
* A value that specifies whether the datashare can be shared to a publicly accessible cluster.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public RejectDataShareResult withAllowPubliclyAccessibleConsumers(Boolean allowPubliclyAccessibleConsumers) {
setAllowPubliclyAccessibleConsumers(allowPubliclyAccessibleConsumers);
return this;
}
/**
*
* A value that specifies whether the datashare can be shared to a publicly accessible cluster.
*
*
* @return A value that specifies whether the datashare can be shared to a publicly accessible cluster.
*/
public Boolean isAllowPubliclyAccessibleConsumers() {
return this.allowPubliclyAccessibleConsumers;
}
/**
*
* A value that specifies when the datashare has an association between producer and data consumers.
*
*
* @return A value that specifies when the datashare has an association between producer and data consumers.
*/
public java.util.List getDataShareAssociations() {
if (dataShareAssociations == null) {
dataShareAssociations = new com.amazonaws.internal.SdkInternalList();
}
return dataShareAssociations;
}
/**
*
* A value that specifies when the datashare has an association between producer and data consumers.
*
*
* @param dataShareAssociations
* A value that specifies when the datashare has an association between producer and data consumers.
*/
public void setDataShareAssociations(java.util.Collection dataShareAssociations) {
if (dataShareAssociations == null) {
this.dataShareAssociations = null;
return;
}
this.dataShareAssociations = new com.amazonaws.internal.SdkInternalList(dataShareAssociations);
}
/**
*
* A value that specifies when the datashare has an association between producer and data consumers.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setDataShareAssociations(java.util.Collection)} or
* {@link #withDataShareAssociations(java.util.Collection)} if you want to override the existing values.
*
*
* @param dataShareAssociations
* A value that specifies when the datashare has an association between producer and data consumers.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public RejectDataShareResult withDataShareAssociations(DataShareAssociation... dataShareAssociations) {
if (this.dataShareAssociations == null) {
setDataShareAssociations(new com.amazonaws.internal.SdkInternalList(dataShareAssociations.length));
}
for (DataShareAssociation ele : dataShareAssociations) {
this.dataShareAssociations.add(ele);
}
return this;
}
/**
*
* A value that specifies when the datashare has an association between producer and data consumers.
*
*
* @param dataShareAssociations
* A value that specifies when the datashare has an association between producer and data consumers.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public RejectDataShareResult withDataShareAssociations(java.util.Collection dataShareAssociations) {
setDataShareAssociations(dataShareAssociations);
return this;
}
/**
*
* The identifier of a datashare to show its managing entity.
*
*
* @param managedBy
* The identifier of a datashare to show its managing entity.
*/
public void setManagedBy(String managedBy) {
this.managedBy = managedBy;
}
/**
*
* The identifier of a datashare to show its managing entity.
*
*
* @return The identifier of a datashare to show its managing entity.
*/
public String getManagedBy() {
return this.managedBy;
}
/**
*
* The identifier of a datashare to show its managing entity.
*
*
* @param managedBy
* The identifier of a datashare to show its managing entity.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public RejectDataShareResult withManagedBy(String managedBy) {
setManagedBy(managedBy);
return this;
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getDataShareArn() != null)
sb.append("DataShareArn: ").append(getDataShareArn()).append(",");
if (getProducerArn() != null)
sb.append("ProducerArn: ").append(getProducerArn()).append(",");
if (getAllowPubliclyAccessibleConsumers() != null)
sb.append("AllowPubliclyAccessibleConsumers: ").append(getAllowPubliclyAccessibleConsumers()).append(",");
if (getDataShareAssociations() != null)
sb.append("DataShareAssociations: ").append(getDataShareAssociations()).append(",");
if (getManagedBy() != null)
sb.append("ManagedBy: ").append(getManagedBy());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof RejectDataShareResult == false)
return false;
RejectDataShareResult other = (RejectDataShareResult) obj;
if (other.getDataShareArn() == null ^ this.getDataShareArn() == null)
return false;
if (other.getDataShareArn() != null && other.getDataShareArn().equals(this.getDataShareArn()) == false)
return false;
if (other.getProducerArn() == null ^ this.getProducerArn() == null)
return false;
if (other.getProducerArn() != null && other.getProducerArn().equals(this.getProducerArn()) == false)
return false;
if (other.getAllowPubliclyAccessibleConsumers() == null ^ this.getAllowPubliclyAccessibleConsumers() == null)
return false;
if (other.getAllowPubliclyAccessibleConsumers() != null
&& other.getAllowPubliclyAccessibleConsumers().equals(this.getAllowPubliclyAccessibleConsumers()) == false)
return false;
if (other.getDataShareAssociations() == null ^ this.getDataShareAssociations() == null)
return false;
if (other.getDataShareAssociations() != null && other.getDataShareAssociations().equals(this.getDataShareAssociations()) == false)
return false;
if (other.getManagedBy() == null ^ this.getManagedBy() == null)
return false;
if (other.getManagedBy() != null && other.getManagedBy().equals(this.getManagedBy()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getDataShareArn() == null) ? 0 : getDataShareArn().hashCode());
hashCode = prime * hashCode + ((getProducerArn() == null) ? 0 : getProducerArn().hashCode());
hashCode = prime * hashCode + ((getAllowPubliclyAccessibleConsumers() == null) ? 0 : getAllowPubliclyAccessibleConsumers().hashCode());
hashCode = prime * hashCode + ((getDataShareAssociations() == null) ? 0 : getDataShareAssociations().hashCode());
hashCode = prime * hashCode + ((getManagedBy() == null) ? 0 : getManagedBy().hashCode());
return hashCode;
}
@Override
public RejectDataShareResult clone() {
try {
return (RejectDataShareResult) super.clone();
} catch (CloneNotSupportedException e) {
throw new IllegalStateException("Got a CloneNotSupportedException from Object.clone() " + "even though we're Cloneable!", e);
}
}
}