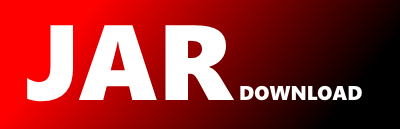
com.amazonaws.services.redshift.model.RevokeEndpointAccessResult Maven / Gradle / Ivy
Show all versions of aws-java-sdk-redshift Show documentation
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.redshift.model;
import java.io.Serializable;
import javax.annotation.Generated;
/**
*
* Describes an endpoint authorization for authorizing Redshift-managed VPC endpoint access to a cluster across Amazon
* Web Services accounts.
*
*
* @see AWS API
* Documentation
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class RevokeEndpointAccessResult extends com.amazonaws.AmazonWebServiceResult implements Serializable, Cloneable {
/**
*
* The Amazon Web Services account ID of the cluster owner.
*
*/
private String grantor;
/**
*
* The Amazon Web Services account ID of the grantee of the cluster.
*
*/
private String grantee;
/**
*
* The cluster identifier.
*
*/
private String clusterIdentifier;
/**
*
* The time (UTC) when the authorization was created.
*
*/
private java.util.Date authorizeTime;
/**
*
* The status of the cluster.
*
*/
private String clusterStatus;
/**
*
* The status of the authorization action.
*
*/
private String status;
/**
*
* Indicates whether all VPCs in the grantee account are allowed access to the cluster.
*
*/
private Boolean allowedAllVPCs;
/**
*
* The VPCs allowed access to the cluster.
*
*/
private com.amazonaws.internal.SdkInternalList allowedVPCs;
/**
*
* The number of Redshift-managed VPC endpoints created for the authorization.
*
*/
private Integer endpointCount;
/**
*
* The Amazon Web Services account ID of the cluster owner.
*
*
* @param grantor
* The Amazon Web Services account ID of the cluster owner.
*/
public void setGrantor(String grantor) {
this.grantor = grantor;
}
/**
*
* The Amazon Web Services account ID of the cluster owner.
*
*
* @return The Amazon Web Services account ID of the cluster owner.
*/
public String getGrantor() {
return this.grantor;
}
/**
*
* The Amazon Web Services account ID of the cluster owner.
*
*
* @param grantor
* The Amazon Web Services account ID of the cluster owner.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public RevokeEndpointAccessResult withGrantor(String grantor) {
setGrantor(grantor);
return this;
}
/**
*
* The Amazon Web Services account ID of the grantee of the cluster.
*
*
* @param grantee
* The Amazon Web Services account ID of the grantee of the cluster.
*/
public void setGrantee(String grantee) {
this.grantee = grantee;
}
/**
*
* The Amazon Web Services account ID of the grantee of the cluster.
*
*
* @return The Amazon Web Services account ID of the grantee of the cluster.
*/
public String getGrantee() {
return this.grantee;
}
/**
*
* The Amazon Web Services account ID of the grantee of the cluster.
*
*
* @param grantee
* The Amazon Web Services account ID of the grantee of the cluster.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public RevokeEndpointAccessResult withGrantee(String grantee) {
setGrantee(grantee);
return this;
}
/**
*
* The cluster identifier.
*
*
* @param clusterIdentifier
* The cluster identifier.
*/
public void setClusterIdentifier(String clusterIdentifier) {
this.clusterIdentifier = clusterIdentifier;
}
/**
*
* The cluster identifier.
*
*
* @return The cluster identifier.
*/
public String getClusterIdentifier() {
return this.clusterIdentifier;
}
/**
*
* The cluster identifier.
*
*
* @param clusterIdentifier
* The cluster identifier.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public RevokeEndpointAccessResult withClusterIdentifier(String clusterIdentifier) {
setClusterIdentifier(clusterIdentifier);
return this;
}
/**
*
* The time (UTC) when the authorization was created.
*
*
* @param authorizeTime
* The time (UTC) when the authorization was created.
*/
public void setAuthorizeTime(java.util.Date authorizeTime) {
this.authorizeTime = authorizeTime;
}
/**
*
* The time (UTC) when the authorization was created.
*
*
* @return The time (UTC) when the authorization was created.
*/
public java.util.Date getAuthorizeTime() {
return this.authorizeTime;
}
/**
*
* The time (UTC) when the authorization was created.
*
*
* @param authorizeTime
* The time (UTC) when the authorization was created.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public RevokeEndpointAccessResult withAuthorizeTime(java.util.Date authorizeTime) {
setAuthorizeTime(authorizeTime);
return this;
}
/**
*
* The status of the cluster.
*
*
* @param clusterStatus
* The status of the cluster.
*/
public void setClusterStatus(String clusterStatus) {
this.clusterStatus = clusterStatus;
}
/**
*
* The status of the cluster.
*
*
* @return The status of the cluster.
*/
public String getClusterStatus() {
return this.clusterStatus;
}
/**
*
* The status of the cluster.
*
*
* @param clusterStatus
* The status of the cluster.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public RevokeEndpointAccessResult withClusterStatus(String clusterStatus) {
setClusterStatus(clusterStatus);
return this;
}
/**
*
* The status of the authorization action.
*
*
* @param status
* The status of the authorization action.
* @see AuthorizationStatus
*/
public void setStatus(String status) {
this.status = status;
}
/**
*
* The status of the authorization action.
*
*
* @return The status of the authorization action.
* @see AuthorizationStatus
*/
public String getStatus() {
return this.status;
}
/**
*
* The status of the authorization action.
*
*
* @param status
* The status of the authorization action.
* @return Returns a reference to this object so that method calls can be chained together.
* @see AuthorizationStatus
*/
public RevokeEndpointAccessResult withStatus(String status) {
setStatus(status);
return this;
}
/**
*
* The status of the authorization action.
*
*
* @param status
* The status of the authorization action.
* @return Returns a reference to this object so that method calls can be chained together.
* @see AuthorizationStatus
*/
public RevokeEndpointAccessResult withStatus(AuthorizationStatus status) {
this.status = status.toString();
return this;
}
/**
*
* Indicates whether all VPCs in the grantee account are allowed access to the cluster.
*
*
* @param allowedAllVPCs
* Indicates whether all VPCs in the grantee account are allowed access to the cluster.
*/
public void setAllowedAllVPCs(Boolean allowedAllVPCs) {
this.allowedAllVPCs = allowedAllVPCs;
}
/**
*
* Indicates whether all VPCs in the grantee account are allowed access to the cluster.
*
*
* @return Indicates whether all VPCs in the grantee account are allowed access to the cluster.
*/
public Boolean getAllowedAllVPCs() {
return this.allowedAllVPCs;
}
/**
*
* Indicates whether all VPCs in the grantee account are allowed access to the cluster.
*
*
* @param allowedAllVPCs
* Indicates whether all VPCs in the grantee account are allowed access to the cluster.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public RevokeEndpointAccessResult withAllowedAllVPCs(Boolean allowedAllVPCs) {
setAllowedAllVPCs(allowedAllVPCs);
return this;
}
/**
*
* Indicates whether all VPCs in the grantee account are allowed access to the cluster.
*
*
* @return Indicates whether all VPCs in the grantee account are allowed access to the cluster.
*/
public Boolean isAllowedAllVPCs() {
return this.allowedAllVPCs;
}
/**
*
* The VPCs allowed access to the cluster.
*
*
* @return The VPCs allowed access to the cluster.
*/
public java.util.List getAllowedVPCs() {
if (allowedVPCs == null) {
allowedVPCs = new com.amazonaws.internal.SdkInternalList();
}
return allowedVPCs;
}
/**
*
* The VPCs allowed access to the cluster.
*
*
* @param allowedVPCs
* The VPCs allowed access to the cluster.
*/
public void setAllowedVPCs(java.util.Collection allowedVPCs) {
if (allowedVPCs == null) {
this.allowedVPCs = null;
return;
}
this.allowedVPCs = new com.amazonaws.internal.SdkInternalList(allowedVPCs);
}
/**
*
* The VPCs allowed access to the cluster.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setAllowedVPCs(java.util.Collection)} or {@link #withAllowedVPCs(java.util.Collection)} if you want to
* override the existing values.
*
*
* @param allowedVPCs
* The VPCs allowed access to the cluster.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public RevokeEndpointAccessResult withAllowedVPCs(String... allowedVPCs) {
if (this.allowedVPCs == null) {
setAllowedVPCs(new com.amazonaws.internal.SdkInternalList(allowedVPCs.length));
}
for (String ele : allowedVPCs) {
this.allowedVPCs.add(ele);
}
return this;
}
/**
*
* The VPCs allowed access to the cluster.
*
*
* @param allowedVPCs
* The VPCs allowed access to the cluster.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public RevokeEndpointAccessResult withAllowedVPCs(java.util.Collection allowedVPCs) {
setAllowedVPCs(allowedVPCs);
return this;
}
/**
*
* The number of Redshift-managed VPC endpoints created for the authorization.
*
*
* @param endpointCount
* The number of Redshift-managed VPC endpoints created for the authorization.
*/
public void setEndpointCount(Integer endpointCount) {
this.endpointCount = endpointCount;
}
/**
*
* The number of Redshift-managed VPC endpoints created for the authorization.
*
*
* @return The number of Redshift-managed VPC endpoints created for the authorization.
*/
public Integer getEndpointCount() {
return this.endpointCount;
}
/**
*
* The number of Redshift-managed VPC endpoints created for the authorization.
*
*
* @param endpointCount
* The number of Redshift-managed VPC endpoints created for the authorization.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public RevokeEndpointAccessResult withEndpointCount(Integer endpointCount) {
setEndpointCount(endpointCount);
return this;
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getGrantor() != null)
sb.append("Grantor: ").append(getGrantor()).append(",");
if (getGrantee() != null)
sb.append("Grantee: ").append(getGrantee()).append(",");
if (getClusterIdentifier() != null)
sb.append("ClusterIdentifier: ").append(getClusterIdentifier()).append(",");
if (getAuthorizeTime() != null)
sb.append("AuthorizeTime: ").append(getAuthorizeTime()).append(",");
if (getClusterStatus() != null)
sb.append("ClusterStatus: ").append(getClusterStatus()).append(",");
if (getStatus() != null)
sb.append("Status: ").append(getStatus()).append(",");
if (getAllowedAllVPCs() != null)
sb.append("AllowedAllVPCs: ").append(getAllowedAllVPCs()).append(",");
if (getAllowedVPCs() != null)
sb.append("AllowedVPCs: ").append(getAllowedVPCs()).append(",");
if (getEndpointCount() != null)
sb.append("EndpointCount: ").append(getEndpointCount());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof RevokeEndpointAccessResult == false)
return false;
RevokeEndpointAccessResult other = (RevokeEndpointAccessResult) obj;
if (other.getGrantor() == null ^ this.getGrantor() == null)
return false;
if (other.getGrantor() != null && other.getGrantor().equals(this.getGrantor()) == false)
return false;
if (other.getGrantee() == null ^ this.getGrantee() == null)
return false;
if (other.getGrantee() != null && other.getGrantee().equals(this.getGrantee()) == false)
return false;
if (other.getClusterIdentifier() == null ^ this.getClusterIdentifier() == null)
return false;
if (other.getClusterIdentifier() != null && other.getClusterIdentifier().equals(this.getClusterIdentifier()) == false)
return false;
if (other.getAuthorizeTime() == null ^ this.getAuthorizeTime() == null)
return false;
if (other.getAuthorizeTime() != null && other.getAuthorizeTime().equals(this.getAuthorizeTime()) == false)
return false;
if (other.getClusterStatus() == null ^ this.getClusterStatus() == null)
return false;
if (other.getClusterStatus() != null && other.getClusterStatus().equals(this.getClusterStatus()) == false)
return false;
if (other.getStatus() == null ^ this.getStatus() == null)
return false;
if (other.getStatus() != null && other.getStatus().equals(this.getStatus()) == false)
return false;
if (other.getAllowedAllVPCs() == null ^ this.getAllowedAllVPCs() == null)
return false;
if (other.getAllowedAllVPCs() != null && other.getAllowedAllVPCs().equals(this.getAllowedAllVPCs()) == false)
return false;
if (other.getAllowedVPCs() == null ^ this.getAllowedVPCs() == null)
return false;
if (other.getAllowedVPCs() != null && other.getAllowedVPCs().equals(this.getAllowedVPCs()) == false)
return false;
if (other.getEndpointCount() == null ^ this.getEndpointCount() == null)
return false;
if (other.getEndpointCount() != null && other.getEndpointCount().equals(this.getEndpointCount()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getGrantor() == null) ? 0 : getGrantor().hashCode());
hashCode = prime * hashCode + ((getGrantee() == null) ? 0 : getGrantee().hashCode());
hashCode = prime * hashCode + ((getClusterIdentifier() == null) ? 0 : getClusterIdentifier().hashCode());
hashCode = prime * hashCode + ((getAuthorizeTime() == null) ? 0 : getAuthorizeTime().hashCode());
hashCode = prime * hashCode + ((getClusterStatus() == null) ? 0 : getClusterStatus().hashCode());
hashCode = prime * hashCode + ((getStatus() == null) ? 0 : getStatus().hashCode());
hashCode = prime * hashCode + ((getAllowedAllVPCs() == null) ? 0 : getAllowedAllVPCs().hashCode());
hashCode = prime * hashCode + ((getAllowedVPCs() == null) ? 0 : getAllowedVPCs().hashCode());
hashCode = prime * hashCode + ((getEndpointCount() == null) ? 0 : getEndpointCount().hashCode());
return hashCode;
}
@Override
public RevokeEndpointAccessResult clone() {
try {
return (RevokeEndpointAccessResult) super.clone();
} catch (CloneNotSupportedException e) {
throw new IllegalStateException("Got a CloneNotSupportedException from Object.clone() " + "even though we're Cloneable!", e);
}
}
}