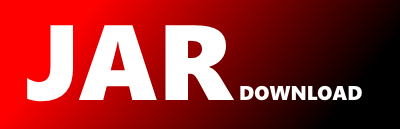
com.amazonaws.services.redshiftdataapi.AWSRedshiftDataAPI Maven / Gradle / Ivy
Show all versions of aws-java-sdk-redshiftdataapi Show documentation
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.redshiftdataapi;
import javax.annotation.Generated;
import com.amazonaws.*;
import com.amazonaws.regions.*;
import com.amazonaws.services.redshiftdataapi.model.*;
/**
* Interface for accessing Redshift Data API Service.
*
* Note: Do not directly implement this interface, new methods are added to it regularly. Extend from
* {@link com.amazonaws.services.redshiftdataapi.AbstractAWSRedshiftDataAPI} instead.
*
*
*
* You can use the Amazon Redshift Data API to run queries on Amazon Redshift tables. You can run SQL statements, which
* are committed if the statement succeeds.
*
*
* For more information about the Amazon Redshift Data API and CLI usage examples, see Using the Amazon Redshift Data API in the
* Amazon Redshift Management Guide.
*
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public interface AWSRedshiftDataAPI {
/**
* The region metadata service name for computing region endpoints. You can use this value to retrieve metadata
* (such as supported regions) of the service.
*
* @see RegionUtils#getRegionsForService(String)
*/
String ENDPOINT_PREFIX = "redshift-data";
/**
*
* Runs one or more SQL statements, which can be data manipulation language (DML) or data definition language (DDL).
* Depending on the authorization method, use one of the following combinations of request parameters:
*
*
* -
*
* Secrets Manager - when connecting to a cluster, provide the secret-arn
of a secret stored in Secrets
* Manager which has username
and password
. The specified secret contains credentials to
* connect to the database
you specify. When you are connecting to a cluster, you also supply the
* database name, If you provide a cluster identifier (dbClusterIdentifier
), it must match the cluster
* identifier stored in the secret. When you are connecting to a serverless workgroup, you also supply the database
* name.
*
*
* -
*
* Temporary credentials - when connecting to your data warehouse, choose one of the following options:
*
*
* -
*
* When connecting to a serverless workgroup, specify the workgroup name and database name. The database user name
* is derived from the IAM identity. For example, arn:iam::123456789012:user:foo
has the database user
* name IAM:foo
. Also, permission to call the redshift-serverless:GetCredentials
operation
* is required.
*
*
* -
*
* When connecting to a cluster as an IAM identity, specify the cluster identifier and the database name. The
* database user name is derived from the IAM identity. For example, arn:iam::123456789012:user:foo
has
* the database user name IAM:foo
. Also, permission to call the
* redshift:GetClusterCredentialsWithIAM
operation is required.
*
*
* -
*
* When connecting to a cluster as a database user, specify the cluster identifier, the database name, and the
* database user name. Also, permission to call the redshift:GetClusterCredentials
operation is
* required.
*
*
*
*
*
*
* For more information about the Amazon Redshift Data API and CLI usage examples, see Using the Amazon Redshift Data API in
* the Amazon Redshift Management Guide.
*
*
* @param batchExecuteStatementRequest
* @return Result of the BatchExecuteStatement operation returned by the service.
* @throws ValidationException
* The Amazon Redshift Data API operation failed due to invalid input.
* @throws ActiveStatementsExceededException
* The number of active statements exceeds the limit.
* @throws BatchExecuteStatementException
* An SQL statement encountered an environmental error while running.
* @sample AWSRedshiftDataAPI.BatchExecuteStatement
* @see AWS API Documentation
*/
BatchExecuteStatementResult batchExecuteStatement(BatchExecuteStatementRequest batchExecuteStatementRequest);
/**
*
* Cancels a running query. To be canceled, a query must be running.
*
*
* For more information about the Amazon Redshift Data API and CLI usage examples, see Using the Amazon Redshift Data API in
* the Amazon Redshift Management Guide.
*
*
* @param cancelStatementRequest
* @return Result of the CancelStatement operation returned by the service.
* @throws ValidationException
* The Amazon Redshift Data API operation failed due to invalid input.
* @throws ResourceNotFoundException
* The Amazon Redshift Data API operation failed due to a missing resource.
* @throws InternalServerException
* The Amazon Redshift Data API operation failed due to invalid input.
* @throws DatabaseConnectionException
* Connection to a database failed.
* @sample AWSRedshiftDataAPI.CancelStatement
* @see AWS
* API Documentation
*/
CancelStatementResult cancelStatement(CancelStatementRequest cancelStatementRequest);
/**
*
* Describes the details about a specific instance when a query was run by the Amazon Redshift Data API. The
* information includes when the query started, when it finished, the query status, the number of rows returned, and
* the SQL statement.
*
*
* For more information about the Amazon Redshift Data API and CLI usage examples, see Using the Amazon Redshift Data API in
* the Amazon Redshift Management Guide.
*
*
* @param describeStatementRequest
* @return Result of the DescribeStatement operation returned by the service.
* @throws ValidationException
* The Amazon Redshift Data API operation failed due to invalid input.
* @throws ResourceNotFoundException
* The Amazon Redshift Data API operation failed due to a missing resource.
* @throws InternalServerException
* The Amazon Redshift Data API operation failed due to invalid input.
* @sample AWSRedshiftDataAPI.DescribeStatement
* @see AWS API Documentation
*/
DescribeStatementResult describeStatement(DescribeStatementRequest describeStatementRequest);
/**
*
* Describes the detailed information about a table from metadata in the cluster. The information includes its
* columns. A token is returned to page through the column list. Depending on the authorization method, use one of
* the following combinations of request parameters:
*
*
* -
*
* Secrets Manager - when connecting to a cluster, provide the secret-arn
of a secret stored in Secrets
* Manager which has username
and password
. The specified secret contains credentials to
* connect to the database
you specify. When you are connecting to a cluster, you also supply the
* database name, If you provide a cluster identifier (dbClusterIdentifier
), it must match the cluster
* identifier stored in the secret. When you are connecting to a serverless workgroup, you also supply the database
* name.
*
*
* -
*
* Temporary credentials - when connecting to your data warehouse, choose one of the following options:
*
*
* -
*
* When connecting to a serverless workgroup, specify the workgroup name and database name. The database user name
* is derived from the IAM identity. For example, arn:iam::123456789012:user:foo
has the database user
* name IAM:foo
. Also, permission to call the redshift-serverless:GetCredentials
operation
* is required.
*
*
* -
*
* When connecting to a cluster as an IAM identity, specify the cluster identifier and the database name. The
* database user name is derived from the IAM identity. For example, arn:iam::123456789012:user:foo
has
* the database user name IAM:foo
. Also, permission to call the
* redshift:GetClusterCredentialsWithIAM
operation is required.
*
*
* -
*
* When connecting to a cluster as a database user, specify the cluster identifier, the database name, and the
* database user name. Also, permission to call the redshift:GetClusterCredentials
operation is
* required.
*
*
*
*
*
*
* For more information about the Amazon Redshift Data API and CLI usage examples, see Using the Amazon Redshift Data API in
* the Amazon Redshift Management Guide.
*
*
* @param describeTableRequest
* @return Result of the DescribeTable operation returned by the service.
* @throws ValidationException
* The Amazon Redshift Data API operation failed due to invalid input.
* @throws InternalServerException
* The Amazon Redshift Data API operation failed due to invalid input.
* @throws DatabaseConnectionException
* Connection to a database failed.
* @sample AWSRedshiftDataAPI.DescribeTable
* @see AWS
* API Documentation
*/
DescribeTableResult describeTable(DescribeTableRequest describeTableRequest);
/**
*
* Runs an SQL statement, which can be data manipulation language (DML) or data definition language (DDL). This
* statement must be a single SQL statement. Depending on the authorization method, use one of the following
* combinations of request parameters:
*
*
* -
*
* Secrets Manager - when connecting to a cluster, provide the secret-arn
of a secret stored in Secrets
* Manager which has username
and password
. The specified secret contains credentials to
* connect to the database
you specify. When you are connecting to a cluster, you also supply the
* database name, If you provide a cluster identifier (dbClusterIdentifier
), it must match the cluster
* identifier stored in the secret. When you are connecting to a serverless workgroup, you also supply the database
* name.
*
*
* -
*
* Temporary credentials - when connecting to your data warehouse, choose one of the following options:
*
*
* -
*
* When connecting to a serverless workgroup, specify the workgroup name and database name. The database user name
* is derived from the IAM identity. For example, arn:iam::123456789012:user:foo
has the database user
* name IAM:foo
. Also, permission to call the redshift-serverless:GetCredentials
operation
* is required.
*
*
* -
*
* When connecting to a cluster as an IAM identity, specify the cluster identifier and the database name. The
* database user name is derived from the IAM identity. For example, arn:iam::123456789012:user:foo
has
* the database user name IAM:foo
. Also, permission to call the
* redshift:GetClusterCredentialsWithIAM
operation is required.
*
*
* -
*
* When connecting to a cluster as a database user, specify the cluster identifier, the database name, and the
* database user name. Also, permission to call the redshift:GetClusterCredentials
operation is
* required.
*
*
*
*
*
*
* For more information about the Amazon Redshift Data API and CLI usage examples, see Using the Amazon Redshift Data API in
* the Amazon Redshift Management Guide.
*
*
* @param executeStatementRequest
* @return Result of the ExecuteStatement operation returned by the service.
* @throws ValidationException
* The Amazon Redshift Data API operation failed due to invalid input.
* @throws ExecuteStatementException
* The SQL statement encountered an environmental error while running.
* @throws ActiveStatementsExceededException
* The number of active statements exceeds the limit.
* @sample AWSRedshiftDataAPI.ExecuteStatement
* @see AWS
* API Documentation
*/
ExecuteStatementResult executeStatement(ExecuteStatementRequest executeStatementRequest);
/**
*
* Fetches the temporarily cached result of an SQL statement. A token is returned to page through the statement
* results.
*
*
* For more information about the Amazon Redshift Data API and CLI usage examples, see Using the Amazon Redshift Data API in
* the Amazon Redshift Management Guide.
*
*
* @param getStatementResultRequest
* @return Result of the GetStatementResult operation returned by the service.
* @throws ValidationException
* The Amazon Redshift Data API operation failed due to invalid input.
* @throws ResourceNotFoundException
* The Amazon Redshift Data API operation failed due to a missing resource.
* @throws InternalServerException
* The Amazon Redshift Data API operation failed due to invalid input.
* @sample AWSRedshiftDataAPI.GetStatementResult
* @see AWS API Documentation
*/
GetStatementResultResult getStatementResult(GetStatementResultRequest getStatementResultRequest);
/**
*
* List the databases in a cluster. A token is returned to page through the database list. Depending on the
* authorization method, use one of the following combinations of request parameters:
*
*
* -
*
* Secrets Manager - when connecting to a cluster, provide the secret-arn
of a secret stored in Secrets
* Manager which has username
and password
. The specified secret contains credentials to
* connect to the database
you specify. When you are connecting to a cluster, you also supply the
* database name, If you provide a cluster identifier (dbClusterIdentifier
), it must match the cluster
* identifier stored in the secret. When you are connecting to a serverless workgroup, you also supply the database
* name.
*
*
* -
*
* Temporary credentials - when connecting to your data warehouse, choose one of the following options:
*
*
* -
*
* When connecting to a serverless workgroup, specify the workgroup name and database name. The database user name
* is derived from the IAM identity. For example, arn:iam::123456789012:user:foo
has the database user
* name IAM:foo
. Also, permission to call the redshift-serverless:GetCredentials
operation
* is required.
*
*
* -
*
* When connecting to a cluster as an IAM identity, specify the cluster identifier and the database name. The
* database user name is derived from the IAM identity. For example, arn:iam::123456789012:user:foo
has
* the database user name IAM:foo
. Also, permission to call the
* redshift:GetClusterCredentialsWithIAM
operation is required.
*
*
* -
*
* When connecting to a cluster as a database user, specify the cluster identifier, the database name, and the
* database user name. Also, permission to call the redshift:GetClusterCredentials
operation is
* required.
*
*
*
*
*
*
* For more information about the Amazon Redshift Data API and CLI usage examples, see Using the Amazon Redshift Data API in
* the Amazon Redshift Management Guide.
*
*
* @param listDatabasesRequest
* @return Result of the ListDatabases operation returned by the service.
* @throws ValidationException
* The Amazon Redshift Data API operation failed due to invalid input.
* @throws InternalServerException
* The Amazon Redshift Data API operation failed due to invalid input.
* @throws DatabaseConnectionException
* Connection to a database failed.
* @sample AWSRedshiftDataAPI.ListDatabases
* @see AWS
* API Documentation
*/
ListDatabasesResult listDatabases(ListDatabasesRequest listDatabasesRequest);
/**
*
* Lists the schemas in a database. A token is returned to page through the schema list. Depending on the
* authorization method, use one of the following combinations of request parameters:
*
*
* -
*
* Secrets Manager - when connecting to a cluster, provide the secret-arn
of a secret stored in Secrets
* Manager which has username
and password
. The specified secret contains credentials to
* connect to the database
you specify. When you are connecting to a cluster, you also supply the
* database name, If you provide a cluster identifier (dbClusterIdentifier
), it must match the cluster
* identifier stored in the secret. When you are connecting to a serverless workgroup, you also supply the database
* name.
*
*
* -
*
* Temporary credentials - when connecting to your data warehouse, choose one of the following options:
*
*
* -
*
* When connecting to a serverless workgroup, specify the workgroup name and database name. The database user name
* is derived from the IAM identity. For example, arn:iam::123456789012:user:foo
has the database user
* name IAM:foo
. Also, permission to call the redshift-serverless:GetCredentials
operation
* is required.
*
*
* -
*
* When connecting to a cluster as an IAM identity, specify the cluster identifier and the database name. The
* database user name is derived from the IAM identity. For example, arn:iam::123456789012:user:foo
has
* the database user name IAM:foo
. Also, permission to call the
* redshift:GetClusterCredentialsWithIAM
operation is required.
*
*
* -
*
* When connecting to a cluster as a database user, specify the cluster identifier, the database name, and the
* database user name. Also, permission to call the redshift:GetClusterCredentials
operation is
* required.
*
*
*
*
*
*
* For more information about the Amazon Redshift Data API and CLI usage examples, see Using the Amazon Redshift Data API in
* the Amazon Redshift Management Guide.
*
*
* @param listSchemasRequest
* @return Result of the ListSchemas operation returned by the service.
* @throws ValidationException
* The Amazon Redshift Data API operation failed due to invalid input.
* @throws InternalServerException
* The Amazon Redshift Data API operation failed due to invalid input.
* @throws DatabaseConnectionException
* Connection to a database failed.
* @sample AWSRedshiftDataAPI.ListSchemas
* @see AWS API
* Documentation
*/
ListSchemasResult listSchemas(ListSchemasRequest listSchemasRequest);
/**
*
* List of SQL statements. By default, only finished statements are shown. A token is returned to page through the
* statement list.
*
*
* For more information about the Amazon Redshift Data API and CLI usage examples, see Using the Amazon Redshift Data API in
* the Amazon Redshift Management Guide.
*
*
* @param listStatementsRequest
* @return Result of the ListStatements operation returned by the service.
* @throws ValidationException
* The Amazon Redshift Data API operation failed due to invalid input.
* @throws InternalServerException
* The Amazon Redshift Data API operation failed due to invalid input.
* @sample AWSRedshiftDataAPI.ListStatements
* @see AWS
* API Documentation
*/
ListStatementsResult listStatements(ListStatementsRequest listStatementsRequest);
/**
*
* List the tables in a database. If neither SchemaPattern
nor TablePattern
are specified,
* then all tables in the database are returned. A token is returned to page through the table list. Depending on
* the authorization method, use one of the following combinations of request parameters:
*
*
* -
*
* Secrets Manager - when connecting to a cluster, provide the secret-arn
of a secret stored in Secrets
* Manager which has username
and password
. The specified secret contains credentials to
* connect to the database
you specify. When you are connecting to a cluster, you also supply the
* database name, If you provide a cluster identifier (dbClusterIdentifier
), it must match the cluster
* identifier stored in the secret. When you are connecting to a serverless workgroup, you also supply the database
* name.
*
*
* -
*
* Temporary credentials - when connecting to your data warehouse, choose one of the following options:
*
*
* -
*
* When connecting to a serverless workgroup, specify the workgroup name and database name. The database user name
* is derived from the IAM identity. For example, arn:iam::123456789012:user:foo
has the database user
* name IAM:foo
. Also, permission to call the redshift-serverless:GetCredentials
operation
* is required.
*
*
* -
*
* When connecting to a cluster as an IAM identity, specify the cluster identifier and the database name. The
* database user name is derived from the IAM identity. For example, arn:iam::123456789012:user:foo
has
* the database user name IAM:foo
. Also, permission to call the
* redshift:GetClusterCredentialsWithIAM
operation is required.
*
*
* -
*
* When connecting to a cluster as a database user, specify the cluster identifier, the database name, and the
* database user name. Also, permission to call the redshift:GetClusterCredentials
operation is
* required.
*
*
*
*
*
*
* For more information about the Amazon Redshift Data API and CLI usage examples, see Using the Amazon Redshift Data API in
* the Amazon Redshift Management Guide.
*
*
* @param listTablesRequest
* @return Result of the ListTables operation returned by the service.
* @throws ValidationException
* The Amazon Redshift Data API operation failed due to invalid input.
* @throws InternalServerException
* The Amazon Redshift Data API operation failed due to invalid input.
* @throws DatabaseConnectionException
* Connection to a database failed.
* @sample AWSRedshiftDataAPI.ListTables
* @see AWS API
* Documentation
*/
ListTablesResult listTables(ListTablesRequest listTablesRequest);
/**
* Shuts down this client object, releasing any resources that might be held open. This is an optional method, and
* callers are not expected to call it, but can if they want to explicitly release any open resources. Once a client
* has been shutdown, it should not be used to make any more requests.
*/
void shutdown();
/**
* Returns additional metadata for a previously executed successful request, typically used for debugging issues
* where a service isn't acting as expected. This data isn't considered part of the result data returned by an
* operation, so it's available through this separate, diagnostic interface.
*
* Response metadata is only cached for a limited period of time, so if you need to access this extra diagnostic
* information for an executed request, you should use this method to retrieve it as soon as possible after
* executing a request.
*
* @param request
* The originally executed request.
*
* @return The response metadata for the specified request, or null if none is available.
*/
ResponseMetadata getCachedResponseMetadata(AmazonWebServiceRequest request);
}