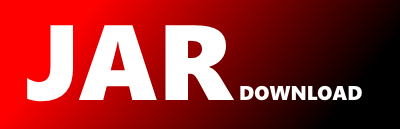
com.amazonaws.services.redshiftdataapi.model.ListSchemasRequest Maven / Gradle / Ivy
Show all versions of aws-java-sdk-redshiftdataapi Show documentation
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.redshiftdataapi.model;
import java.io.Serializable;
import javax.annotation.Generated;
import com.amazonaws.AmazonWebServiceRequest;
/**
*
* @see AWS API
* Documentation
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class ListSchemasRequest extends com.amazonaws.AmazonWebServiceRequest implements Serializable, Cloneable {
/**
*
* The cluster identifier. This parameter is required when connecting to a cluster and authenticating using either
* Secrets Manager or temporary credentials.
*
*/
private String clusterIdentifier;
/**
*
* A database name. The connected database is specified when you connect with your authentication credentials.
*
*/
private String connectedDatabase;
/**
*
* The name of the database that contains the schemas to list. If ConnectedDatabase
is not specified,
* this is also the database to connect to with your authentication credentials.
*
*/
private String database;
/**
*
* The database user name. This parameter is required when connecting to a cluster as a database user and
* authenticating using temporary credentials.
*
*/
private String dbUser;
/**
*
* The maximum number of schemas to return in the response. If more schemas exist than fit in one response, then
* NextToken
is returned to page through the results.
*
*/
private Integer maxResults;
/**
*
* A value that indicates the starting point for the next set of response records in a subsequent request. If a
* value is returned in a response, you can retrieve the next set of records by providing this returned NextToken
* value in the next NextToken parameter and retrying the command. If the NextToken field is empty, all response
* records have been retrieved for the request.
*
*/
private String nextToken;
/**
*
* A pattern to filter results by schema name. Within a schema pattern, "%" means match any substring of 0 or more
* characters and "_" means match any one character. Only schema name entries matching the search pattern are
* returned.
*
*/
private String schemaPattern;
/**
*
* The name or ARN of the secret that enables access to the database. This parameter is required when authenticating
* using Secrets Manager.
*
*/
private String secretArn;
/**
*
* The serverless workgroup name or Amazon Resource Name (ARN). This parameter is required when connecting to a
* serverless workgroup and authenticating using either Secrets Manager or temporary credentials.
*
*/
private String workgroupName;
/**
*
* The cluster identifier. This parameter is required when connecting to a cluster and authenticating using either
* Secrets Manager or temporary credentials.
*
*
* @param clusterIdentifier
* The cluster identifier. This parameter is required when connecting to a cluster and authenticating using
* either Secrets Manager or temporary credentials.
*/
public void setClusterIdentifier(String clusterIdentifier) {
this.clusterIdentifier = clusterIdentifier;
}
/**
*
* The cluster identifier. This parameter is required when connecting to a cluster and authenticating using either
* Secrets Manager or temporary credentials.
*
*
* @return The cluster identifier. This parameter is required when connecting to a cluster and authenticating using
* either Secrets Manager or temporary credentials.
*/
public String getClusterIdentifier() {
return this.clusterIdentifier;
}
/**
*
* The cluster identifier. This parameter is required when connecting to a cluster and authenticating using either
* Secrets Manager or temporary credentials.
*
*
* @param clusterIdentifier
* The cluster identifier. This parameter is required when connecting to a cluster and authenticating using
* either Secrets Manager or temporary credentials.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ListSchemasRequest withClusterIdentifier(String clusterIdentifier) {
setClusterIdentifier(clusterIdentifier);
return this;
}
/**
*
* A database name. The connected database is specified when you connect with your authentication credentials.
*
*
* @param connectedDatabase
* A database name. The connected database is specified when you connect with your authentication
* credentials.
*/
public void setConnectedDatabase(String connectedDatabase) {
this.connectedDatabase = connectedDatabase;
}
/**
*
* A database name. The connected database is specified when you connect with your authentication credentials.
*
*
* @return A database name. The connected database is specified when you connect with your authentication
* credentials.
*/
public String getConnectedDatabase() {
return this.connectedDatabase;
}
/**
*
* A database name. The connected database is specified when you connect with your authentication credentials.
*
*
* @param connectedDatabase
* A database name. The connected database is specified when you connect with your authentication
* credentials.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ListSchemasRequest withConnectedDatabase(String connectedDatabase) {
setConnectedDatabase(connectedDatabase);
return this;
}
/**
*
* The name of the database that contains the schemas to list. If ConnectedDatabase
is not specified,
* this is also the database to connect to with your authentication credentials.
*
*
* @param database
* The name of the database that contains the schemas to list. If ConnectedDatabase
is not
* specified, this is also the database to connect to with your authentication credentials.
*/
public void setDatabase(String database) {
this.database = database;
}
/**
*
* The name of the database that contains the schemas to list. If ConnectedDatabase
is not specified,
* this is also the database to connect to with your authentication credentials.
*
*
* @return The name of the database that contains the schemas to list. If ConnectedDatabase
is not
* specified, this is also the database to connect to with your authentication credentials.
*/
public String getDatabase() {
return this.database;
}
/**
*
* The name of the database that contains the schemas to list. If ConnectedDatabase
is not specified,
* this is also the database to connect to with your authentication credentials.
*
*
* @param database
* The name of the database that contains the schemas to list. If ConnectedDatabase
is not
* specified, this is also the database to connect to with your authentication credentials.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ListSchemasRequest withDatabase(String database) {
setDatabase(database);
return this;
}
/**
*
* The database user name. This parameter is required when connecting to a cluster as a database user and
* authenticating using temporary credentials.
*
*
* @param dbUser
* The database user name. This parameter is required when connecting to a cluster as a database user and
* authenticating using temporary credentials.
*/
public void setDbUser(String dbUser) {
this.dbUser = dbUser;
}
/**
*
* The database user name. This parameter is required when connecting to a cluster as a database user and
* authenticating using temporary credentials.
*
*
* @return The database user name. This parameter is required when connecting to a cluster as a database user and
* authenticating using temporary credentials.
*/
public String getDbUser() {
return this.dbUser;
}
/**
*
* The database user name. This parameter is required when connecting to a cluster as a database user and
* authenticating using temporary credentials.
*
*
* @param dbUser
* The database user name. This parameter is required when connecting to a cluster as a database user and
* authenticating using temporary credentials.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ListSchemasRequest withDbUser(String dbUser) {
setDbUser(dbUser);
return this;
}
/**
*
* The maximum number of schemas to return in the response. If more schemas exist than fit in one response, then
* NextToken
is returned to page through the results.
*
*
* @param maxResults
* The maximum number of schemas to return in the response. If more schemas exist than fit in one response,
* then NextToken
is returned to page through the results.
*/
public void setMaxResults(Integer maxResults) {
this.maxResults = maxResults;
}
/**
*
* The maximum number of schemas to return in the response. If more schemas exist than fit in one response, then
* NextToken
is returned to page through the results.
*
*
* @return The maximum number of schemas to return in the response. If more schemas exist than fit in one response,
* then NextToken
is returned to page through the results.
*/
public Integer getMaxResults() {
return this.maxResults;
}
/**
*
* The maximum number of schemas to return in the response. If more schemas exist than fit in one response, then
* NextToken
is returned to page through the results.
*
*
* @param maxResults
* The maximum number of schemas to return in the response. If more schemas exist than fit in one response,
* then NextToken
is returned to page through the results.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ListSchemasRequest withMaxResults(Integer maxResults) {
setMaxResults(maxResults);
return this;
}
/**
*
* A value that indicates the starting point for the next set of response records in a subsequent request. If a
* value is returned in a response, you can retrieve the next set of records by providing this returned NextToken
* value in the next NextToken parameter and retrying the command. If the NextToken field is empty, all response
* records have been retrieved for the request.
*
*
* @param nextToken
* A value that indicates the starting point for the next set of response records in a subsequent request. If
* a value is returned in a response, you can retrieve the next set of records by providing this returned
* NextToken value in the next NextToken parameter and retrying the command. If the NextToken field is empty,
* all response records have been retrieved for the request.
*/
public void setNextToken(String nextToken) {
this.nextToken = nextToken;
}
/**
*
* A value that indicates the starting point for the next set of response records in a subsequent request. If a
* value is returned in a response, you can retrieve the next set of records by providing this returned NextToken
* value in the next NextToken parameter and retrying the command. If the NextToken field is empty, all response
* records have been retrieved for the request.
*
*
* @return A value that indicates the starting point for the next set of response records in a subsequent request.
* If a value is returned in a response, you can retrieve the next set of records by providing this returned
* NextToken value in the next NextToken parameter and retrying the command. If the NextToken field is
* empty, all response records have been retrieved for the request.
*/
public String getNextToken() {
return this.nextToken;
}
/**
*
* A value that indicates the starting point for the next set of response records in a subsequent request. If a
* value is returned in a response, you can retrieve the next set of records by providing this returned NextToken
* value in the next NextToken parameter and retrying the command. If the NextToken field is empty, all response
* records have been retrieved for the request.
*
*
* @param nextToken
* A value that indicates the starting point for the next set of response records in a subsequent request. If
* a value is returned in a response, you can retrieve the next set of records by providing this returned
* NextToken value in the next NextToken parameter and retrying the command. If the NextToken field is empty,
* all response records have been retrieved for the request.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ListSchemasRequest withNextToken(String nextToken) {
setNextToken(nextToken);
return this;
}
/**
*
* A pattern to filter results by schema name. Within a schema pattern, "%" means match any substring of 0 or more
* characters and "_" means match any one character. Only schema name entries matching the search pattern are
* returned.
*
*
* @param schemaPattern
* A pattern to filter results by schema name. Within a schema pattern, "%" means match any substring of 0 or
* more characters and "_" means match any one character. Only schema name entries matching the search
* pattern are returned.
*/
public void setSchemaPattern(String schemaPattern) {
this.schemaPattern = schemaPattern;
}
/**
*
* A pattern to filter results by schema name. Within a schema pattern, "%" means match any substring of 0 or more
* characters and "_" means match any one character. Only schema name entries matching the search pattern are
* returned.
*
*
* @return A pattern to filter results by schema name. Within a schema pattern, "%" means match any substring of 0
* or more characters and "_" means match any one character. Only schema name entries matching the search
* pattern are returned.
*/
public String getSchemaPattern() {
return this.schemaPattern;
}
/**
*
* A pattern to filter results by schema name. Within a schema pattern, "%" means match any substring of 0 or more
* characters and "_" means match any one character. Only schema name entries matching the search pattern are
* returned.
*
*
* @param schemaPattern
* A pattern to filter results by schema name. Within a schema pattern, "%" means match any substring of 0 or
* more characters and "_" means match any one character. Only schema name entries matching the search
* pattern are returned.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ListSchemasRequest withSchemaPattern(String schemaPattern) {
setSchemaPattern(schemaPattern);
return this;
}
/**
*
* The name or ARN of the secret that enables access to the database. This parameter is required when authenticating
* using Secrets Manager.
*
*
* @param secretArn
* The name or ARN of the secret that enables access to the database. This parameter is required when
* authenticating using Secrets Manager.
*/
public void setSecretArn(String secretArn) {
this.secretArn = secretArn;
}
/**
*
* The name or ARN of the secret that enables access to the database. This parameter is required when authenticating
* using Secrets Manager.
*
*
* @return The name or ARN of the secret that enables access to the database. This parameter is required when
* authenticating using Secrets Manager.
*/
public String getSecretArn() {
return this.secretArn;
}
/**
*
* The name or ARN of the secret that enables access to the database. This parameter is required when authenticating
* using Secrets Manager.
*
*
* @param secretArn
* The name or ARN of the secret that enables access to the database. This parameter is required when
* authenticating using Secrets Manager.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ListSchemasRequest withSecretArn(String secretArn) {
setSecretArn(secretArn);
return this;
}
/**
*
* The serverless workgroup name or Amazon Resource Name (ARN). This parameter is required when connecting to a
* serverless workgroup and authenticating using either Secrets Manager or temporary credentials.
*
*
* @param workgroupName
* The serverless workgroup name or Amazon Resource Name (ARN). This parameter is required when connecting to
* a serverless workgroup and authenticating using either Secrets Manager or temporary credentials.
*/
public void setWorkgroupName(String workgroupName) {
this.workgroupName = workgroupName;
}
/**
*
* The serverless workgroup name or Amazon Resource Name (ARN). This parameter is required when connecting to a
* serverless workgroup and authenticating using either Secrets Manager or temporary credentials.
*
*
* @return The serverless workgroup name or Amazon Resource Name (ARN). This parameter is required when connecting
* to a serverless workgroup and authenticating using either Secrets Manager or temporary credentials.
*/
public String getWorkgroupName() {
return this.workgroupName;
}
/**
*
* The serverless workgroup name or Amazon Resource Name (ARN). This parameter is required when connecting to a
* serverless workgroup and authenticating using either Secrets Manager or temporary credentials.
*
*
* @param workgroupName
* The serverless workgroup name or Amazon Resource Name (ARN). This parameter is required when connecting to
* a serverless workgroup and authenticating using either Secrets Manager or temporary credentials.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ListSchemasRequest withWorkgroupName(String workgroupName) {
setWorkgroupName(workgroupName);
return this;
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getClusterIdentifier() != null)
sb.append("ClusterIdentifier: ").append(getClusterIdentifier()).append(",");
if (getConnectedDatabase() != null)
sb.append("ConnectedDatabase: ").append(getConnectedDatabase()).append(",");
if (getDatabase() != null)
sb.append("Database: ").append(getDatabase()).append(",");
if (getDbUser() != null)
sb.append("DbUser: ").append(getDbUser()).append(",");
if (getMaxResults() != null)
sb.append("MaxResults: ").append(getMaxResults()).append(",");
if (getNextToken() != null)
sb.append("NextToken: ").append(getNextToken()).append(",");
if (getSchemaPattern() != null)
sb.append("SchemaPattern: ").append(getSchemaPattern()).append(",");
if (getSecretArn() != null)
sb.append("SecretArn: ").append(getSecretArn()).append(",");
if (getWorkgroupName() != null)
sb.append("WorkgroupName: ").append(getWorkgroupName());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof ListSchemasRequest == false)
return false;
ListSchemasRequest other = (ListSchemasRequest) obj;
if (other.getClusterIdentifier() == null ^ this.getClusterIdentifier() == null)
return false;
if (other.getClusterIdentifier() != null && other.getClusterIdentifier().equals(this.getClusterIdentifier()) == false)
return false;
if (other.getConnectedDatabase() == null ^ this.getConnectedDatabase() == null)
return false;
if (other.getConnectedDatabase() != null && other.getConnectedDatabase().equals(this.getConnectedDatabase()) == false)
return false;
if (other.getDatabase() == null ^ this.getDatabase() == null)
return false;
if (other.getDatabase() != null && other.getDatabase().equals(this.getDatabase()) == false)
return false;
if (other.getDbUser() == null ^ this.getDbUser() == null)
return false;
if (other.getDbUser() != null && other.getDbUser().equals(this.getDbUser()) == false)
return false;
if (other.getMaxResults() == null ^ this.getMaxResults() == null)
return false;
if (other.getMaxResults() != null && other.getMaxResults().equals(this.getMaxResults()) == false)
return false;
if (other.getNextToken() == null ^ this.getNextToken() == null)
return false;
if (other.getNextToken() != null && other.getNextToken().equals(this.getNextToken()) == false)
return false;
if (other.getSchemaPattern() == null ^ this.getSchemaPattern() == null)
return false;
if (other.getSchemaPattern() != null && other.getSchemaPattern().equals(this.getSchemaPattern()) == false)
return false;
if (other.getSecretArn() == null ^ this.getSecretArn() == null)
return false;
if (other.getSecretArn() != null && other.getSecretArn().equals(this.getSecretArn()) == false)
return false;
if (other.getWorkgroupName() == null ^ this.getWorkgroupName() == null)
return false;
if (other.getWorkgroupName() != null && other.getWorkgroupName().equals(this.getWorkgroupName()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getClusterIdentifier() == null) ? 0 : getClusterIdentifier().hashCode());
hashCode = prime * hashCode + ((getConnectedDatabase() == null) ? 0 : getConnectedDatabase().hashCode());
hashCode = prime * hashCode + ((getDatabase() == null) ? 0 : getDatabase().hashCode());
hashCode = prime * hashCode + ((getDbUser() == null) ? 0 : getDbUser().hashCode());
hashCode = prime * hashCode + ((getMaxResults() == null) ? 0 : getMaxResults().hashCode());
hashCode = prime * hashCode + ((getNextToken() == null) ? 0 : getNextToken().hashCode());
hashCode = prime * hashCode + ((getSchemaPattern() == null) ? 0 : getSchemaPattern().hashCode());
hashCode = prime * hashCode + ((getSecretArn() == null) ? 0 : getSecretArn().hashCode());
hashCode = prime * hashCode + ((getWorkgroupName() == null) ? 0 : getWorkgroupName().hashCode());
return hashCode;
}
@Override
public ListSchemasRequest clone() {
return (ListSchemasRequest) super.clone();
}
}