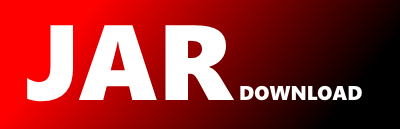
com.amazonaws.services.redshiftdataapi.model.StatementData Maven / Gradle / Ivy
Show all versions of aws-java-sdk-redshiftdataapi Show documentation
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.redshiftdataapi.model;
import java.io.Serializable;
import javax.annotation.Generated;
import com.amazonaws.protocol.StructuredPojo;
import com.amazonaws.protocol.ProtocolMarshaller;
/**
*
* The SQL statement to run.
*
*
* @see AWS API
* Documentation
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class StatementData implements Serializable, Cloneable, StructuredPojo {
/**
*
* The date and time (UTC) the statement was created.
*
*/
private java.util.Date createdAt;
/**
*
* The SQL statement identifier. This value is a universally unique identifier (UUID) generated by Amazon Redshift
* Data API.
*
*/
private String id;
/**
*
* A value that indicates whether the statement is a batch query request.
*
*/
private Boolean isBatchStatement;
/**
*
* The parameters used in a SQL statement.
*
*/
private java.util.List queryParameters;
/**
*
* The SQL statement.
*
*/
private String queryString;
/**
*
* One or more SQL statements. Each query string in the array corresponds to one of the queries in a batch query
* request.
*
*/
private java.util.List queryStrings;
/**
*
* The name or Amazon Resource Name (ARN) of the secret that enables access to the database.
*
*/
private String secretArn;
/**
*
* The name of the SQL statement.
*
*/
private String statementName;
/**
*
* The status of the SQL statement. An example is the that the SQL statement finished.
*
*/
private String status;
/**
*
* The date and time (UTC) that the statement metadata was last updated.
*
*/
private java.util.Date updatedAt;
/**
*
* The date and time (UTC) the statement was created.
*
*
* @param createdAt
* The date and time (UTC) the statement was created.
*/
public void setCreatedAt(java.util.Date createdAt) {
this.createdAt = createdAt;
}
/**
*
* The date and time (UTC) the statement was created.
*
*
* @return The date and time (UTC) the statement was created.
*/
public java.util.Date getCreatedAt() {
return this.createdAt;
}
/**
*
* The date and time (UTC) the statement was created.
*
*
* @param createdAt
* The date and time (UTC) the statement was created.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public StatementData withCreatedAt(java.util.Date createdAt) {
setCreatedAt(createdAt);
return this;
}
/**
*
* The SQL statement identifier. This value is a universally unique identifier (UUID) generated by Amazon Redshift
* Data API.
*
*
* @param id
* The SQL statement identifier. This value is a universally unique identifier (UUID) generated by Amazon
* Redshift Data API.
*/
public void setId(String id) {
this.id = id;
}
/**
*
* The SQL statement identifier. This value is a universally unique identifier (UUID) generated by Amazon Redshift
* Data API.
*
*
* @return The SQL statement identifier. This value is a universally unique identifier (UUID) generated by Amazon
* Redshift Data API.
*/
public String getId() {
return this.id;
}
/**
*
* The SQL statement identifier. This value is a universally unique identifier (UUID) generated by Amazon Redshift
* Data API.
*
*
* @param id
* The SQL statement identifier. This value is a universally unique identifier (UUID) generated by Amazon
* Redshift Data API.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public StatementData withId(String id) {
setId(id);
return this;
}
/**
*
* A value that indicates whether the statement is a batch query request.
*
*
* @param isBatchStatement
* A value that indicates whether the statement is a batch query request.
*/
public void setIsBatchStatement(Boolean isBatchStatement) {
this.isBatchStatement = isBatchStatement;
}
/**
*
* A value that indicates whether the statement is a batch query request.
*
*
* @return A value that indicates whether the statement is a batch query request.
*/
public Boolean getIsBatchStatement() {
return this.isBatchStatement;
}
/**
*
* A value that indicates whether the statement is a batch query request.
*
*
* @param isBatchStatement
* A value that indicates whether the statement is a batch query request.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public StatementData withIsBatchStatement(Boolean isBatchStatement) {
setIsBatchStatement(isBatchStatement);
return this;
}
/**
*
* A value that indicates whether the statement is a batch query request.
*
*
* @return A value that indicates whether the statement is a batch query request.
*/
public Boolean isBatchStatement() {
return this.isBatchStatement;
}
/**
*
* The parameters used in a SQL statement.
*
*
* @return The parameters used in a SQL statement.
*/
public java.util.List getQueryParameters() {
return queryParameters;
}
/**
*
* The parameters used in a SQL statement.
*
*
* @param queryParameters
* The parameters used in a SQL statement.
*/
public void setQueryParameters(java.util.Collection queryParameters) {
if (queryParameters == null) {
this.queryParameters = null;
return;
}
this.queryParameters = new java.util.ArrayList(queryParameters);
}
/**
*
* The parameters used in a SQL statement.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setQueryParameters(java.util.Collection)} or {@link #withQueryParameters(java.util.Collection)} if you
* want to override the existing values.
*
*
* @param queryParameters
* The parameters used in a SQL statement.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public StatementData withQueryParameters(SqlParameter... queryParameters) {
if (this.queryParameters == null) {
setQueryParameters(new java.util.ArrayList(queryParameters.length));
}
for (SqlParameter ele : queryParameters) {
this.queryParameters.add(ele);
}
return this;
}
/**
*
* The parameters used in a SQL statement.
*
*
* @param queryParameters
* The parameters used in a SQL statement.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public StatementData withQueryParameters(java.util.Collection queryParameters) {
setQueryParameters(queryParameters);
return this;
}
/**
*
* The SQL statement.
*
*
* @param queryString
* The SQL statement.
*/
public void setQueryString(String queryString) {
this.queryString = queryString;
}
/**
*
* The SQL statement.
*
*
* @return The SQL statement.
*/
public String getQueryString() {
return this.queryString;
}
/**
*
* The SQL statement.
*
*
* @param queryString
* The SQL statement.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public StatementData withQueryString(String queryString) {
setQueryString(queryString);
return this;
}
/**
*
* One or more SQL statements. Each query string in the array corresponds to one of the queries in a batch query
* request.
*
*
* @return One or more SQL statements. Each query string in the array corresponds to one of the queries in a batch
* query request.
*/
public java.util.List getQueryStrings() {
return queryStrings;
}
/**
*
* One or more SQL statements. Each query string in the array corresponds to one of the queries in a batch query
* request.
*
*
* @param queryStrings
* One or more SQL statements. Each query string in the array corresponds to one of the queries in a batch
* query request.
*/
public void setQueryStrings(java.util.Collection queryStrings) {
if (queryStrings == null) {
this.queryStrings = null;
return;
}
this.queryStrings = new java.util.ArrayList(queryStrings);
}
/**
*
* One or more SQL statements. Each query string in the array corresponds to one of the queries in a batch query
* request.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setQueryStrings(java.util.Collection)} or {@link #withQueryStrings(java.util.Collection)} if you want to
* override the existing values.
*
*
* @param queryStrings
* One or more SQL statements. Each query string in the array corresponds to one of the queries in a batch
* query request.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public StatementData withQueryStrings(String... queryStrings) {
if (this.queryStrings == null) {
setQueryStrings(new java.util.ArrayList(queryStrings.length));
}
for (String ele : queryStrings) {
this.queryStrings.add(ele);
}
return this;
}
/**
*
* One or more SQL statements. Each query string in the array corresponds to one of the queries in a batch query
* request.
*
*
* @param queryStrings
* One or more SQL statements. Each query string in the array corresponds to one of the queries in a batch
* query request.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public StatementData withQueryStrings(java.util.Collection queryStrings) {
setQueryStrings(queryStrings);
return this;
}
/**
*
* The name or Amazon Resource Name (ARN) of the secret that enables access to the database.
*
*
* @param secretArn
* The name or Amazon Resource Name (ARN) of the secret that enables access to the database.
*/
public void setSecretArn(String secretArn) {
this.secretArn = secretArn;
}
/**
*
* The name or Amazon Resource Name (ARN) of the secret that enables access to the database.
*
*
* @return The name or Amazon Resource Name (ARN) of the secret that enables access to the database.
*/
public String getSecretArn() {
return this.secretArn;
}
/**
*
* The name or Amazon Resource Name (ARN) of the secret that enables access to the database.
*
*
* @param secretArn
* The name or Amazon Resource Name (ARN) of the secret that enables access to the database.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public StatementData withSecretArn(String secretArn) {
setSecretArn(secretArn);
return this;
}
/**
*
* The name of the SQL statement.
*
*
* @param statementName
* The name of the SQL statement.
*/
public void setStatementName(String statementName) {
this.statementName = statementName;
}
/**
*
* The name of the SQL statement.
*
*
* @return The name of the SQL statement.
*/
public String getStatementName() {
return this.statementName;
}
/**
*
* The name of the SQL statement.
*
*
* @param statementName
* The name of the SQL statement.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public StatementData withStatementName(String statementName) {
setStatementName(statementName);
return this;
}
/**
*
* The status of the SQL statement. An example is the that the SQL statement finished.
*
*
* @param status
* The status of the SQL statement. An example is the that the SQL statement finished.
* @see StatusString
*/
public void setStatus(String status) {
this.status = status;
}
/**
*
* The status of the SQL statement. An example is the that the SQL statement finished.
*
*
* @return The status of the SQL statement. An example is the that the SQL statement finished.
* @see StatusString
*/
public String getStatus() {
return this.status;
}
/**
*
* The status of the SQL statement. An example is the that the SQL statement finished.
*
*
* @param status
* The status of the SQL statement. An example is the that the SQL statement finished.
* @return Returns a reference to this object so that method calls can be chained together.
* @see StatusString
*/
public StatementData withStatus(String status) {
setStatus(status);
return this;
}
/**
*
* The status of the SQL statement. An example is the that the SQL statement finished.
*
*
* @param status
* The status of the SQL statement. An example is the that the SQL statement finished.
* @return Returns a reference to this object so that method calls can be chained together.
* @see StatusString
*/
public StatementData withStatus(StatusString status) {
this.status = status.toString();
return this;
}
/**
*
* The date and time (UTC) that the statement metadata was last updated.
*
*
* @param updatedAt
* The date and time (UTC) that the statement metadata was last updated.
*/
public void setUpdatedAt(java.util.Date updatedAt) {
this.updatedAt = updatedAt;
}
/**
*
* The date and time (UTC) that the statement metadata was last updated.
*
*
* @return The date and time (UTC) that the statement metadata was last updated.
*/
public java.util.Date getUpdatedAt() {
return this.updatedAt;
}
/**
*
* The date and time (UTC) that the statement metadata was last updated.
*
*
* @param updatedAt
* The date and time (UTC) that the statement metadata was last updated.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public StatementData withUpdatedAt(java.util.Date updatedAt) {
setUpdatedAt(updatedAt);
return this;
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getCreatedAt() != null)
sb.append("CreatedAt: ").append(getCreatedAt()).append(",");
if (getId() != null)
sb.append("Id: ").append(getId()).append(",");
if (getIsBatchStatement() != null)
sb.append("IsBatchStatement: ").append(getIsBatchStatement()).append(",");
if (getQueryParameters() != null)
sb.append("QueryParameters: ").append(getQueryParameters()).append(",");
if (getQueryString() != null)
sb.append("QueryString: ").append(getQueryString()).append(",");
if (getQueryStrings() != null)
sb.append("QueryStrings: ").append(getQueryStrings()).append(",");
if (getSecretArn() != null)
sb.append("SecretArn: ").append(getSecretArn()).append(",");
if (getStatementName() != null)
sb.append("StatementName: ").append(getStatementName()).append(",");
if (getStatus() != null)
sb.append("Status: ").append(getStatus()).append(",");
if (getUpdatedAt() != null)
sb.append("UpdatedAt: ").append(getUpdatedAt());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof StatementData == false)
return false;
StatementData other = (StatementData) obj;
if (other.getCreatedAt() == null ^ this.getCreatedAt() == null)
return false;
if (other.getCreatedAt() != null && other.getCreatedAt().equals(this.getCreatedAt()) == false)
return false;
if (other.getId() == null ^ this.getId() == null)
return false;
if (other.getId() != null && other.getId().equals(this.getId()) == false)
return false;
if (other.getIsBatchStatement() == null ^ this.getIsBatchStatement() == null)
return false;
if (other.getIsBatchStatement() != null && other.getIsBatchStatement().equals(this.getIsBatchStatement()) == false)
return false;
if (other.getQueryParameters() == null ^ this.getQueryParameters() == null)
return false;
if (other.getQueryParameters() != null && other.getQueryParameters().equals(this.getQueryParameters()) == false)
return false;
if (other.getQueryString() == null ^ this.getQueryString() == null)
return false;
if (other.getQueryString() != null && other.getQueryString().equals(this.getQueryString()) == false)
return false;
if (other.getQueryStrings() == null ^ this.getQueryStrings() == null)
return false;
if (other.getQueryStrings() != null && other.getQueryStrings().equals(this.getQueryStrings()) == false)
return false;
if (other.getSecretArn() == null ^ this.getSecretArn() == null)
return false;
if (other.getSecretArn() != null && other.getSecretArn().equals(this.getSecretArn()) == false)
return false;
if (other.getStatementName() == null ^ this.getStatementName() == null)
return false;
if (other.getStatementName() != null && other.getStatementName().equals(this.getStatementName()) == false)
return false;
if (other.getStatus() == null ^ this.getStatus() == null)
return false;
if (other.getStatus() != null && other.getStatus().equals(this.getStatus()) == false)
return false;
if (other.getUpdatedAt() == null ^ this.getUpdatedAt() == null)
return false;
if (other.getUpdatedAt() != null && other.getUpdatedAt().equals(this.getUpdatedAt()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getCreatedAt() == null) ? 0 : getCreatedAt().hashCode());
hashCode = prime * hashCode + ((getId() == null) ? 0 : getId().hashCode());
hashCode = prime * hashCode + ((getIsBatchStatement() == null) ? 0 : getIsBatchStatement().hashCode());
hashCode = prime * hashCode + ((getQueryParameters() == null) ? 0 : getQueryParameters().hashCode());
hashCode = prime * hashCode + ((getQueryString() == null) ? 0 : getQueryString().hashCode());
hashCode = prime * hashCode + ((getQueryStrings() == null) ? 0 : getQueryStrings().hashCode());
hashCode = prime * hashCode + ((getSecretArn() == null) ? 0 : getSecretArn().hashCode());
hashCode = prime * hashCode + ((getStatementName() == null) ? 0 : getStatementName().hashCode());
hashCode = prime * hashCode + ((getStatus() == null) ? 0 : getStatus().hashCode());
hashCode = prime * hashCode + ((getUpdatedAt() == null) ? 0 : getUpdatedAt().hashCode());
return hashCode;
}
@Override
public StatementData clone() {
try {
return (StatementData) super.clone();
} catch (CloneNotSupportedException e) {
throw new IllegalStateException("Got a CloneNotSupportedException from Object.clone() " + "even though we're Cloneable!", e);
}
}
@com.amazonaws.annotation.SdkInternalApi
@Override
public void marshall(ProtocolMarshaller protocolMarshaller) {
com.amazonaws.services.redshiftdataapi.model.transform.StatementDataMarshaller.getInstance().marshall(this, protocolMarshaller);
}
}