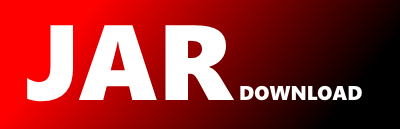
com.amazonaws.services.redshiftserverless.model.UpdateNamespaceRequest Maven / Gradle / Ivy
Show all versions of aws-java-sdk-redshiftserverless Show documentation
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.redshiftserverless.model;
import java.io.Serializable;
import javax.annotation.Generated;
import com.amazonaws.AmazonWebServiceRequest;
/**
*
* @see AWS API Documentation
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class UpdateNamespaceRequest extends com.amazonaws.AmazonWebServiceRequest implements Serializable, Cloneable {
/**
*
* The ID of the Key Management Service (KMS) key used to encrypt and store the namespace's admin credentials
* secret. You can only use this parameter if manageAdminPassword
is true.
*
*/
private String adminPasswordSecretKmsKeyId;
/**
*
* The password of the administrator for the first database created in the namespace. This parameter must be updated
* together with adminUsername
.
*
*
* You can't use adminUserPassword
if manageAdminPassword
is true.
*
*/
private String adminUserPassword;
/**
*
* The username of the administrator for the first database created in the namespace. This parameter must be updated
* together with adminUserPassword
.
*
*/
private String adminUsername;
/**
*
* The Amazon Resource Name (ARN) of the IAM role to set as a default in the namespace. This parameter must be
* updated together with iamRoles
.
*
*/
private String defaultIamRoleArn;
/**
*
* A list of IAM roles to associate with the namespace. This parameter must be updated together with
* defaultIamRoleArn
.
*
*/
private java.util.List iamRoles;
/**
*
* The ID of the Amazon Web Services Key Management Service key used to encrypt your data.
*
*/
private String kmsKeyId;
/**
*
* The types of logs the namespace can export. The export types are userlog
, connectionlog
* , and useractivitylog
.
*
*/
private java.util.List logExports;
/**
*
* If true
, Amazon Redshift uses Secrets Manager to manage the namespace's admin credentials. You can't
* use adminUserPassword
if manageAdminPassword
is true. If
* manageAdminPassword
is false or not set, Amazon Redshift uses adminUserPassword
for the
* admin user account's password.
*
*/
private Boolean manageAdminPassword;
/**
*
* The name of the namespace to update. You can't update the name of a namespace once it is created.
*
*/
private String namespaceName;
/**
*
* The ID of the Key Management Service (KMS) key used to encrypt and store the namespace's admin credentials
* secret. You can only use this parameter if manageAdminPassword
is true.
*
*
* @param adminPasswordSecretKmsKeyId
* The ID of the Key Management Service (KMS) key used to encrypt and store the namespace's admin credentials
* secret. You can only use this parameter if manageAdminPassword
is true.
*/
public void setAdminPasswordSecretKmsKeyId(String adminPasswordSecretKmsKeyId) {
this.adminPasswordSecretKmsKeyId = adminPasswordSecretKmsKeyId;
}
/**
*
* The ID of the Key Management Service (KMS) key used to encrypt and store the namespace's admin credentials
* secret. You can only use this parameter if manageAdminPassword
is true.
*
*
* @return The ID of the Key Management Service (KMS) key used to encrypt and store the namespace's admin
* credentials secret. You can only use this parameter if manageAdminPassword
is true.
*/
public String getAdminPasswordSecretKmsKeyId() {
return this.adminPasswordSecretKmsKeyId;
}
/**
*
* The ID of the Key Management Service (KMS) key used to encrypt and store the namespace's admin credentials
* secret. You can only use this parameter if manageAdminPassword
is true.
*
*
* @param adminPasswordSecretKmsKeyId
* The ID of the Key Management Service (KMS) key used to encrypt and store the namespace's admin credentials
* secret. You can only use this parameter if manageAdminPassword
is true.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public UpdateNamespaceRequest withAdminPasswordSecretKmsKeyId(String adminPasswordSecretKmsKeyId) {
setAdminPasswordSecretKmsKeyId(adminPasswordSecretKmsKeyId);
return this;
}
/**
*
* The password of the administrator for the first database created in the namespace. This parameter must be updated
* together with adminUsername
.
*
*
* You can't use adminUserPassword
if manageAdminPassword
is true.
*
*
* @param adminUserPassword
* The password of the administrator for the first database created in the namespace. This parameter must be
* updated together with adminUsername
.
*
* You can't use adminUserPassword
if manageAdminPassword
is true.
*/
public void setAdminUserPassword(String adminUserPassword) {
this.adminUserPassword = adminUserPassword;
}
/**
*
* The password of the administrator for the first database created in the namespace. This parameter must be updated
* together with adminUsername
.
*
*
* You can't use adminUserPassword
if manageAdminPassword
is true.
*
*
* @return The password of the administrator for the first database created in the namespace. This parameter must be
* updated together with adminUsername
.
*
* You can't use adminUserPassword
if manageAdminPassword
is true.
*/
public String getAdminUserPassword() {
return this.adminUserPassword;
}
/**
*
* The password of the administrator for the first database created in the namespace. This parameter must be updated
* together with adminUsername
.
*
*
* You can't use adminUserPassword
if manageAdminPassword
is true.
*
*
* @param adminUserPassword
* The password of the administrator for the first database created in the namespace. This parameter must be
* updated together with adminUsername
.
*
* You can't use adminUserPassword
if manageAdminPassword
is true.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public UpdateNamespaceRequest withAdminUserPassword(String adminUserPassword) {
setAdminUserPassword(adminUserPassword);
return this;
}
/**
*
* The username of the administrator for the first database created in the namespace. This parameter must be updated
* together with adminUserPassword
.
*
*
* @param adminUsername
* The username of the administrator for the first database created in the namespace. This parameter must be
* updated together with adminUserPassword
.
*/
public void setAdminUsername(String adminUsername) {
this.adminUsername = adminUsername;
}
/**
*
* The username of the administrator for the first database created in the namespace. This parameter must be updated
* together with adminUserPassword
.
*
*
* @return The username of the administrator for the first database created in the namespace. This parameter must be
* updated together with adminUserPassword
.
*/
public String getAdminUsername() {
return this.adminUsername;
}
/**
*
* The username of the administrator for the first database created in the namespace. This parameter must be updated
* together with adminUserPassword
.
*
*
* @param adminUsername
* The username of the administrator for the first database created in the namespace. This parameter must be
* updated together with adminUserPassword
.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public UpdateNamespaceRequest withAdminUsername(String adminUsername) {
setAdminUsername(adminUsername);
return this;
}
/**
*
* The Amazon Resource Name (ARN) of the IAM role to set as a default in the namespace. This parameter must be
* updated together with iamRoles
.
*
*
* @param defaultIamRoleArn
* The Amazon Resource Name (ARN) of the IAM role to set as a default in the namespace. This parameter must
* be updated together with iamRoles
.
*/
public void setDefaultIamRoleArn(String defaultIamRoleArn) {
this.defaultIamRoleArn = defaultIamRoleArn;
}
/**
*
* The Amazon Resource Name (ARN) of the IAM role to set as a default in the namespace. This parameter must be
* updated together with iamRoles
.
*
*
* @return The Amazon Resource Name (ARN) of the IAM role to set as a default in the namespace. This parameter must
* be updated together with iamRoles
.
*/
public String getDefaultIamRoleArn() {
return this.defaultIamRoleArn;
}
/**
*
* The Amazon Resource Name (ARN) of the IAM role to set as a default in the namespace. This parameter must be
* updated together with iamRoles
.
*
*
* @param defaultIamRoleArn
* The Amazon Resource Name (ARN) of the IAM role to set as a default in the namespace. This parameter must
* be updated together with iamRoles
.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public UpdateNamespaceRequest withDefaultIamRoleArn(String defaultIamRoleArn) {
setDefaultIamRoleArn(defaultIamRoleArn);
return this;
}
/**
*
* A list of IAM roles to associate with the namespace. This parameter must be updated together with
* defaultIamRoleArn
.
*
*
* @return A list of IAM roles to associate with the namespace. This parameter must be updated together with
* defaultIamRoleArn
.
*/
public java.util.List getIamRoles() {
return iamRoles;
}
/**
*
* A list of IAM roles to associate with the namespace. This parameter must be updated together with
* defaultIamRoleArn
.
*
*
* @param iamRoles
* A list of IAM roles to associate with the namespace. This parameter must be updated together with
* defaultIamRoleArn
.
*/
public void setIamRoles(java.util.Collection iamRoles) {
if (iamRoles == null) {
this.iamRoles = null;
return;
}
this.iamRoles = new java.util.ArrayList(iamRoles);
}
/**
*
* A list of IAM roles to associate with the namespace. This parameter must be updated together with
* defaultIamRoleArn
.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setIamRoles(java.util.Collection)} or {@link #withIamRoles(java.util.Collection)} if you want to override
* the existing values.
*
*
* @param iamRoles
* A list of IAM roles to associate with the namespace. This parameter must be updated together with
* defaultIamRoleArn
.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public UpdateNamespaceRequest withIamRoles(String... iamRoles) {
if (this.iamRoles == null) {
setIamRoles(new java.util.ArrayList(iamRoles.length));
}
for (String ele : iamRoles) {
this.iamRoles.add(ele);
}
return this;
}
/**
*
* A list of IAM roles to associate with the namespace. This parameter must be updated together with
* defaultIamRoleArn
.
*
*
* @param iamRoles
* A list of IAM roles to associate with the namespace. This parameter must be updated together with
* defaultIamRoleArn
.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public UpdateNamespaceRequest withIamRoles(java.util.Collection iamRoles) {
setIamRoles(iamRoles);
return this;
}
/**
*
* The ID of the Amazon Web Services Key Management Service key used to encrypt your data.
*
*
* @param kmsKeyId
* The ID of the Amazon Web Services Key Management Service key used to encrypt your data.
*/
public void setKmsKeyId(String kmsKeyId) {
this.kmsKeyId = kmsKeyId;
}
/**
*
* The ID of the Amazon Web Services Key Management Service key used to encrypt your data.
*
*
* @return The ID of the Amazon Web Services Key Management Service key used to encrypt your data.
*/
public String getKmsKeyId() {
return this.kmsKeyId;
}
/**
*
* The ID of the Amazon Web Services Key Management Service key used to encrypt your data.
*
*
* @param kmsKeyId
* The ID of the Amazon Web Services Key Management Service key used to encrypt your data.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public UpdateNamespaceRequest withKmsKeyId(String kmsKeyId) {
setKmsKeyId(kmsKeyId);
return this;
}
/**
*
* The types of logs the namespace can export. The export types are userlog
, connectionlog
* , and useractivitylog
.
*
*
* @return The types of logs the namespace can export. The export types are userlog
,
* connectionlog
, and useractivitylog
.
* @see LogExport
*/
public java.util.List getLogExports() {
return logExports;
}
/**
*
* The types of logs the namespace can export. The export types are userlog
, connectionlog
* , and useractivitylog
.
*
*
* @param logExports
* The types of logs the namespace can export. The export types are userlog
,
* connectionlog
, and useractivitylog
.
* @see LogExport
*/
public void setLogExports(java.util.Collection logExports) {
if (logExports == null) {
this.logExports = null;
return;
}
this.logExports = new java.util.ArrayList(logExports);
}
/**
*
* The types of logs the namespace can export. The export types are userlog
, connectionlog
* , and useractivitylog
.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setLogExports(java.util.Collection)} or {@link #withLogExports(java.util.Collection)} if you want to
* override the existing values.
*
*
* @param logExports
* The types of logs the namespace can export. The export types are userlog
,
* connectionlog
, and useractivitylog
.
* @return Returns a reference to this object so that method calls can be chained together.
* @see LogExport
*/
public UpdateNamespaceRequest withLogExports(String... logExports) {
if (this.logExports == null) {
setLogExports(new java.util.ArrayList(logExports.length));
}
for (String ele : logExports) {
this.logExports.add(ele);
}
return this;
}
/**
*
* The types of logs the namespace can export. The export types are userlog
, connectionlog
* , and useractivitylog
.
*
*
* @param logExports
* The types of logs the namespace can export. The export types are userlog
,
* connectionlog
, and useractivitylog
.
* @return Returns a reference to this object so that method calls can be chained together.
* @see LogExport
*/
public UpdateNamespaceRequest withLogExports(java.util.Collection logExports) {
setLogExports(logExports);
return this;
}
/**
*
* The types of logs the namespace can export. The export types are userlog
, connectionlog
* , and useractivitylog
.
*
*
* @param logExports
* The types of logs the namespace can export. The export types are userlog
,
* connectionlog
, and useractivitylog
.
* @return Returns a reference to this object so that method calls can be chained together.
* @see LogExport
*/
public UpdateNamespaceRequest withLogExports(LogExport... logExports) {
java.util.ArrayList logExportsCopy = new java.util.ArrayList(logExports.length);
for (LogExport value : logExports) {
logExportsCopy.add(value.toString());
}
if (getLogExports() == null) {
setLogExports(logExportsCopy);
} else {
getLogExports().addAll(logExportsCopy);
}
return this;
}
/**
*
* If true
, Amazon Redshift uses Secrets Manager to manage the namespace's admin credentials. You can't
* use adminUserPassword
if manageAdminPassword
is true. If
* manageAdminPassword
is false or not set, Amazon Redshift uses adminUserPassword
for the
* admin user account's password.
*
*
* @param manageAdminPassword
* If true
, Amazon Redshift uses Secrets Manager to manage the namespace's admin credentials.
* You can't use adminUserPassword
if manageAdminPassword
is true. If
* manageAdminPassword
is false or not set, Amazon Redshift uses adminUserPassword
* for the admin user account's password.
*/
public void setManageAdminPassword(Boolean manageAdminPassword) {
this.manageAdminPassword = manageAdminPassword;
}
/**
*
* If true
, Amazon Redshift uses Secrets Manager to manage the namespace's admin credentials. You can't
* use adminUserPassword
if manageAdminPassword
is true. If
* manageAdminPassword
is false or not set, Amazon Redshift uses adminUserPassword
for the
* admin user account's password.
*
*
* @return If true
, Amazon Redshift uses Secrets Manager to manage the namespace's admin credentials.
* You can't use adminUserPassword
if manageAdminPassword
is true. If
* manageAdminPassword
is false or not set, Amazon Redshift uses adminUserPassword
* for the admin user account's password.
*/
public Boolean getManageAdminPassword() {
return this.manageAdminPassword;
}
/**
*
* If true
, Amazon Redshift uses Secrets Manager to manage the namespace's admin credentials. You can't
* use adminUserPassword
if manageAdminPassword
is true. If
* manageAdminPassword
is false or not set, Amazon Redshift uses adminUserPassword
for the
* admin user account's password.
*
*
* @param manageAdminPassword
* If true
, Amazon Redshift uses Secrets Manager to manage the namespace's admin credentials.
* You can't use adminUserPassword
if manageAdminPassword
is true. If
* manageAdminPassword
is false or not set, Amazon Redshift uses adminUserPassword
* for the admin user account's password.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public UpdateNamespaceRequest withManageAdminPassword(Boolean manageAdminPassword) {
setManageAdminPassword(manageAdminPassword);
return this;
}
/**
*
* If true
, Amazon Redshift uses Secrets Manager to manage the namespace's admin credentials. You can't
* use adminUserPassword
if manageAdminPassword
is true. If
* manageAdminPassword
is false or not set, Amazon Redshift uses adminUserPassword
for the
* admin user account's password.
*
*
* @return If true
, Amazon Redshift uses Secrets Manager to manage the namespace's admin credentials.
* You can't use adminUserPassword
if manageAdminPassword
is true. If
* manageAdminPassword
is false or not set, Amazon Redshift uses adminUserPassword
* for the admin user account's password.
*/
public Boolean isManageAdminPassword() {
return this.manageAdminPassword;
}
/**
*
* The name of the namespace to update. You can't update the name of a namespace once it is created.
*
*
* @param namespaceName
* The name of the namespace to update. You can't update the name of a namespace once it is created.
*/
public void setNamespaceName(String namespaceName) {
this.namespaceName = namespaceName;
}
/**
*
* The name of the namespace to update. You can't update the name of a namespace once it is created.
*
*
* @return The name of the namespace to update. You can't update the name of a namespace once it is created.
*/
public String getNamespaceName() {
return this.namespaceName;
}
/**
*
* The name of the namespace to update. You can't update the name of a namespace once it is created.
*
*
* @param namespaceName
* The name of the namespace to update. You can't update the name of a namespace once it is created.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public UpdateNamespaceRequest withNamespaceName(String namespaceName) {
setNamespaceName(namespaceName);
return this;
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getAdminPasswordSecretKmsKeyId() != null)
sb.append("AdminPasswordSecretKmsKeyId: ").append(getAdminPasswordSecretKmsKeyId()).append(",");
if (getAdminUserPassword() != null)
sb.append("AdminUserPassword: ").append("***Sensitive Data Redacted***").append(",");
if (getAdminUsername() != null)
sb.append("AdminUsername: ").append("***Sensitive Data Redacted***").append(",");
if (getDefaultIamRoleArn() != null)
sb.append("DefaultIamRoleArn: ").append(getDefaultIamRoleArn()).append(",");
if (getIamRoles() != null)
sb.append("IamRoles: ").append(getIamRoles()).append(",");
if (getKmsKeyId() != null)
sb.append("KmsKeyId: ").append(getKmsKeyId()).append(",");
if (getLogExports() != null)
sb.append("LogExports: ").append(getLogExports()).append(",");
if (getManageAdminPassword() != null)
sb.append("ManageAdminPassword: ").append(getManageAdminPassword()).append(",");
if (getNamespaceName() != null)
sb.append("NamespaceName: ").append(getNamespaceName());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof UpdateNamespaceRequest == false)
return false;
UpdateNamespaceRequest other = (UpdateNamespaceRequest) obj;
if (other.getAdminPasswordSecretKmsKeyId() == null ^ this.getAdminPasswordSecretKmsKeyId() == null)
return false;
if (other.getAdminPasswordSecretKmsKeyId() != null && other.getAdminPasswordSecretKmsKeyId().equals(this.getAdminPasswordSecretKmsKeyId()) == false)
return false;
if (other.getAdminUserPassword() == null ^ this.getAdminUserPassword() == null)
return false;
if (other.getAdminUserPassword() != null && other.getAdminUserPassword().equals(this.getAdminUserPassword()) == false)
return false;
if (other.getAdminUsername() == null ^ this.getAdminUsername() == null)
return false;
if (other.getAdminUsername() != null && other.getAdminUsername().equals(this.getAdminUsername()) == false)
return false;
if (other.getDefaultIamRoleArn() == null ^ this.getDefaultIamRoleArn() == null)
return false;
if (other.getDefaultIamRoleArn() != null && other.getDefaultIamRoleArn().equals(this.getDefaultIamRoleArn()) == false)
return false;
if (other.getIamRoles() == null ^ this.getIamRoles() == null)
return false;
if (other.getIamRoles() != null && other.getIamRoles().equals(this.getIamRoles()) == false)
return false;
if (other.getKmsKeyId() == null ^ this.getKmsKeyId() == null)
return false;
if (other.getKmsKeyId() != null && other.getKmsKeyId().equals(this.getKmsKeyId()) == false)
return false;
if (other.getLogExports() == null ^ this.getLogExports() == null)
return false;
if (other.getLogExports() != null && other.getLogExports().equals(this.getLogExports()) == false)
return false;
if (other.getManageAdminPassword() == null ^ this.getManageAdminPassword() == null)
return false;
if (other.getManageAdminPassword() != null && other.getManageAdminPassword().equals(this.getManageAdminPassword()) == false)
return false;
if (other.getNamespaceName() == null ^ this.getNamespaceName() == null)
return false;
if (other.getNamespaceName() != null && other.getNamespaceName().equals(this.getNamespaceName()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getAdminPasswordSecretKmsKeyId() == null) ? 0 : getAdminPasswordSecretKmsKeyId().hashCode());
hashCode = prime * hashCode + ((getAdminUserPassword() == null) ? 0 : getAdminUserPassword().hashCode());
hashCode = prime * hashCode + ((getAdminUsername() == null) ? 0 : getAdminUsername().hashCode());
hashCode = prime * hashCode + ((getDefaultIamRoleArn() == null) ? 0 : getDefaultIamRoleArn().hashCode());
hashCode = prime * hashCode + ((getIamRoles() == null) ? 0 : getIamRoles().hashCode());
hashCode = prime * hashCode + ((getKmsKeyId() == null) ? 0 : getKmsKeyId().hashCode());
hashCode = prime * hashCode + ((getLogExports() == null) ? 0 : getLogExports().hashCode());
hashCode = prime * hashCode + ((getManageAdminPassword() == null) ? 0 : getManageAdminPassword().hashCode());
hashCode = prime * hashCode + ((getNamespaceName() == null) ? 0 : getNamespaceName().hashCode());
return hashCode;
}
@Override
public UpdateNamespaceRequest clone() {
return (UpdateNamespaceRequest) super.clone();
}
}