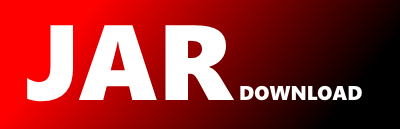
com.amazonaws.services.redshiftserverless.model.UpdateWorkgroupRequest Maven / Gradle / Ivy
Show all versions of aws-java-sdk-redshiftserverless Show documentation
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.redshiftserverless.model;
import java.io.Serializable;
import javax.annotation.Generated;
import com.amazonaws.AmazonWebServiceRequest;
/**
*
* @see AWS API Documentation
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class UpdateWorkgroupRequest extends com.amazonaws.AmazonWebServiceRequest implements Serializable, Cloneable {
/**
*
* The new base data warehouse capacity in Redshift Processing Units (RPUs).
*
*/
private Integer baseCapacity;
/**
*
* An array of parameters to set for advanced control over a database. The options are auto_mv
,
* datestyle
, enable_case_sensitive_identifier
, enable_user_activity_logging
,
* query_group
, search_path
, require_ssl
, use_fips_ssl
, and
* query monitoring metrics that let you define performance boundaries. For more information about query monitoring
* rules and available metrics, see Query monitoring metrics for Amazon Redshift Serverless.
*
*/
private java.util.List configParameters;
/**
*
* The value that specifies whether to turn on enhanced virtual private cloud (VPC) routing, which forces Amazon
* Redshift Serverless to route traffic through your VPC.
*
*/
private Boolean enhancedVpcRouting;
/**
*
* The IP address type that the workgroup supports. Possible values are ipv4
and dualstack
* .
*
*/
private String ipAddressType;
/**
*
* The maximum data-warehouse capacity Amazon Redshift Serverless uses to serve queries. The max capacity is
* specified in RPUs.
*
*/
private Integer maxCapacity;
/**
*
* The custom port to use when connecting to a workgroup. Valid port ranges are 5431-5455 and 8191-8215. The default
* is 5439.
*
*/
private Integer port;
/**
*
* A value that specifies whether the workgroup can be accessible from a public network.
*
*/
private Boolean publiclyAccessible;
/**
*
* An array of security group IDs to associate with the workgroup.
*
*/
private java.util.List securityGroupIds;
/**
*
* An array of VPC subnet IDs to associate with the workgroup.
*
*/
private java.util.List subnetIds;
/**
*
* The name of the workgroup to update. You can't update the name of a workgroup once it is created.
*
*/
private String workgroupName;
/**
*
* The new base data warehouse capacity in Redshift Processing Units (RPUs).
*
*
* @param baseCapacity
* The new base data warehouse capacity in Redshift Processing Units (RPUs).
*/
public void setBaseCapacity(Integer baseCapacity) {
this.baseCapacity = baseCapacity;
}
/**
*
* The new base data warehouse capacity in Redshift Processing Units (RPUs).
*
*
* @return The new base data warehouse capacity in Redshift Processing Units (RPUs).
*/
public Integer getBaseCapacity() {
return this.baseCapacity;
}
/**
*
* The new base data warehouse capacity in Redshift Processing Units (RPUs).
*
*
* @param baseCapacity
* The new base data warehouse capacity in Redshift Processing Units (RPUs).
* @return Returns a reference to this object so that method calls can be chained together.
*/
public UpdateWorkgroupRequest withBaseCapacity(Integer baseCapacity) {
setBaseCapacity(baseCapacity);
return this;
}
/**
*
* An array of parameters to set for advanced control over a database. The options are auto_mv
,
* datestyle
, enable_case_sensitive_identifier
, enable_user_activity_logging
,
* query_group
, search_path
, require_ssl
, use_fips_ssl
, and
* query monitoring metrics that let you define performance boundaries. For more information about query monitoring
* rules and available metrics, see Query monitoring metrics for Amazon Redshift Serverless.
*
*
* @return An array of parameters to set for advanced control over a database. The options are auto_mv
,
* datestyle
, enable_case_sensitive_identifier
,
* enable_user_activity_logging
, query_group
, search_path
,
* require_ssl
, use_fips_ssl
, and query monitoring metrics that let you define
* performance boundaries. For more information about query monitoring rules and available metrics, see Query monitoring metrics for Amazon Redshift Serverless.
*/
public java.util.List getConfigParameters() {
return configParameters;
}
/**
*
* An array of parameters to set for advanced control over a database. The options are auto_mv
,
* datestyle
, enable_case_sensitive_identifier
, enable_user_activity_logging
,
* query_group
, search_path
, require_ssl
, use_fips_ssl
, and
* query monitoring metrics that let you define performance boundaries. For more information about query monitoring
* rules and available metrics, see Query monitoring metrics for Amazon Redshift Serverless.
*
*
* @param configParameters
* An array of parameters to set for advanced control over a database. The options are auto_mv
,
* datestyle
, enable_case_sensitive_identifier
,
* enable_user_activity_logging
, query_group
, search_path
,
* require_ssl
, use_fips_ssl
, and query monitoring metrics that let you define
* performance boundaries. For more information about query monitoring rules and available metrics, see Query monitoring metrics for Amazon Redshift Serverless.
*/
public void setConfigParameters(java.util.Collection configParameters) {
if (configParameters == null) {
this.configParameters = null;
return;
}
this.configParameters = new java.util.ArrayList(configParameters);
}
/**
*
* An array of parameters to set for advanced control over a database. The options are auto_mv
,
* datestyle
, enable_case_sensitive_identifier
, enable_user_activity_logging
,
* query_group
, search_path
, require_ssl
, use_fips_ssl
, and
* query monitoring metrics that let you define performance boundaries. For more information about query monitoring
* rules and available metrics, see Query monitoring metrics for Amazon Redshift Serverless.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setConfigParameters(java.util.Collection)} or {@link #withConfigParameters(java.util.Collection)} if you
* want to override the existing values.
*
*
* @param configParameters
* An array of parameters to set for advanced control over a database. The options are auto_mv
,
* datestyle
, enable_case_sensitive_identifier
,
* enable_user_activity_logging
, query_group
, search_path
,
* require_ssl
, use_fips_ssl
, and query monitoring metrics that let you define
* performance boundaries. For more information about query monitoring rules and available metrics, see Query monitoring metrics for Amazon Redshift Serverless.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public UpdateWorkgroupRequest withConfigParameters(ConfigParameter... configParameters) {
if (this.configParameters == null) {
setConfigParameters(new java.util.ArrayList(configParameters.length));
}
for (ConfigParameter ele : configParameters) {
this.configParameters.add(ele);
}
return this;
}
/**
*
* An array of parameters to set for advanced control over a database. The options are auto_mv
,
* datestyle
, enable_case_sensitive_identifier
, enable_user_activity_logging
,
* query_group
, search_path
, require_ssl
, use_fips_ssl
, and
* query monitoring metrics that let you define performance boundaries. For more information about query monitoring
* rules and available metrics, see Query monitoring metrics for Amazon Redshift Serverless.
*
*
* @param configParameters
* An array of parameters to set for advanced control over a database. The options are auto_mv
,
* datestyle
, enable_case_sensitive_identifier
,
* enable_user_activity_logging
, query_group
, search_path
,
* require_ssl
, use_fips_ssl
, and query monitoring metrics that let you define
* performance boundaries. For more information about query monitoring rules and available metrics, see Query monitoring metrics for Amazon Redshift Serverless.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public UpdateWorkgroupRequest withConfigParameters(java.util.Collection configParameters) {
setConfigParameters(configParameters);
return this;
}
/**
*
* The value that specifies whether to turn on enhanced virtual private cloud (VPC) routing, which forces Amazon
* Redshift Serverless to route traffic through your VPC.
*
*
* @param enhancedVpcRouting
* The value that specifies whether to turn on enhanced virtual private cloud (VPC) routing, which forces
* Amazon Redshift Serverless to route traffic through your VPC.
*/
public void setEnhancedVpcRouting(Boolean enhancedVpcRouting) {
this.enhancedVpcRouting = enhancedVpcRouting;
}
/**
*
* The value that specifies whether to turn on enhanced virtual private cloud (VPC) routing, which forces Amazon
* Redshift Serverless to route traffic through your VPC.
*
*
* @return The value that specifies whether to turn on enhanced virtual private cloud (VPC) routing, which forces
* Amazon Redshift Serverless to route traffic through your VPC.
*/
public Boolean getEnhancedVpcRouting() {
return this.enhancedVpcRouting;
}
/**
*
* The value that specifies whether to turn on enhanced virtual private cloud (VPC) routing, which forces Amazon
* Redshift Serverless to route traffic through your VPC.
*
*
* @param enhancedVpcRouting
* The value that specifies whether to turn on enhanced virtual private cloud (VPC) routing, which forces
* Amazon Redshift Serverless to route traffic through your VPC.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public UpdateWorkgroupRequest withEnhancedVpcRouting(Boolean enhancedVpcRouting) {
setEnhancedVpcRouting(enhancedVpcRouting);
return this;
}
/**
*
* The value that specifies whether to turn on enhanced virtual private cloud (VPC) routing, which forces Amazon
* Redshift Serverless to route traffic through your VPC.
*
*
* @return The value that specifies whether to turn on enhanced virtual private cloud (VPC) routing, which forces
* Amazon Redshift Serverless to route traffic through your VPC.
*/
public Boolean isEnhancedVpcRouting() {
return this.enhancedVpcRouting;
}
/**
*
* The IP address type that the workgroup supports. Possible values are ipv4
and dualstack
* .
*
*
* @param ipAddressType
* The IP address type that the workgroup supports. Possible values are ipv4
and
* dualstack
.
*/
public void setIpAddressType(String ipAddressType) {
this.ipAddressType = ipAddressType;
}
/**
*
* The IP address type that the workgroup supports. Possible values are ipv4
and dualstack
* .
*
*
* @return The IP address type that the workgroup supports. Possible values are ipv4
and
* dualstack
.
*/
public String getIpAddressType() {
return this.ipAddressType;
}
/**
*
* The IP address type that the workgroup supports. Possible values are ipv4
and dualstack
* .
*
*
* @param ipAddressType
* The IP address type that the workgroup supports. Possible values are ipv4
and
* dualstack
.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public UpdateWorkgroupRequest withIpAddressType(String ipAddressType) {
setIpAddressType(ipAddressType);
return this;
}
/**
*
* The maximum data-warehouse capacity Amazon Redshift Serverless uses to serve queries. The max capacity is
* specified in RPUs.
*
*
* @param maxCapacity
* The maximum data-warehouse capacity Amazon Redshift Serverless uses to serve queries. The max capacity is
* specified in RPUs.
*/
public void setMaxCapacity(Integer maxCapacity) {
this.maxCapacity = maxCapacity;
}
/**
*
* The maximum data-warehouse capacity Amazon Redshift Serverless uses to serve queries. The max capacity is
* specified in RPUs.
*
*
* @return The maximum data-warehouse capacity Amazon Redshift Serverless uses to serve queries. The max capacity is
* specified in RPUs.
*/
public Integer getMaxCapacity() {
return this.maxCapacity;
}
/**
*
* The maximum data-warehouse capacity Amazon Redshift Serverless uses to serve queries. The max capacity is
* specified in RPUs.
*
*
* @param maxCapacity
* The maximum data-warehouse capacity Amazon Redshift Serverless uses to serve queries. The max capacity is
* specified in RPUs.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public UpdateWorkgroupRequest withMaxCapacity(Integer maxCapacity) {
setMaxCapacity(maxCapacity);
return this;
}
/**
*
* The custom port to use when connecting to a workgroup. Valid port ranges are 5431-5455 and 8191-8215. The default
* is 5439.
*
*
* @param port
* The custom port to use when connecting to a workgroup. Valid port ranges are 5431-5455 and 8191-8215. The
* default is 5439.
*/
public void setPort(Integer port) {
this.port = port;
}
/**
*
* The custom port to use when connecting to a workgroup. Valid port ranges are 5431-5455 and 8191-8215. The default
* is 5439.
*
*
* @return The custom port to use when connecting to a workgroup. Valid port ranges are 5431-5455 and 8191-8215. The
* default is 5439.
*/
public Integer getPort() {
return this.port;
}
/**
*
* The custom port to use when connecting to a workgroup. Valid port ranges are 5431-5455 and 8191-8215. The default
* is 5439.
*
*
* @param port
* The custom port to use when connecting to a workgroup. Valid port ranges are 5431-5455 and 8191-8215. The
* default is 5439.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public UpdateWorkgroupRequest withPort(Integer port) {
setPort(port);
return this;
}
/**
*
* A value that specifies whether the workgroup can be accessible from a public network.
*
*
* @param publiclyAccessible
* A value that specifies whether the workgroup can be accessible from a public network.
*/
public void setPubliclyAccessible(Boolean publiclyAccessible) {
this.publiclyAccessible = publiclyAccessible;
}
/**
*
* A value that specifies whether the workgroup can be accessible from a public network.
*
*
* @return A value that specifies whether the workgroup can be accessible from a public network.
*/
public Boolean getPubliclyAccessible() {
return this.publiclyAccessible;
}
/**
*
* A value that specifies whether the workgroup can be accessible from a public network.
*
*
* @param publiclyAccessible
* A value that specifies whether the workgroup can be accessible from a public network.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public UpdateWorkgroupRequest withPubliclyAccessible(Boolean publiclyAccessible) {
setPubliclyAccessible(publiclyAccessible);
return this;
}
/**
*
* A value that specifies whether the workgroup can be accessible from a public network.
*
*
* @return A value that specifies whether the workgroup can be accessible from a public network.
*/
public Boolean isPubliclyAccessible() {
return this.publiclyAccessible;
}
/**
*
* An array of security group IDs to associate with the workgroup.
*
*
* @return An array of security group IDs to associate with the workgroup.
*/
public java.util.List getSecurityGroupIds() {
return securityGroupIds;
}
/**
*
* An array of security group IDs to associate with the workgroup.
*
*
* @param securityGroupIds
* An array of security group IDs to associate with the workgroup.
*/
public void setSecurityGroupIds(java.util.Collection securityGroupIds) {
if (securityGroupIds == null) {
this.securityGroupIds = null;
return;
}
this.securityGroupIds = new java.util.ArrayList(securityGroupIds);
}
/**
*
* An array of security group IDs to associate with the workgroup.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setSecurityGroupIds(java.util.Collection)} or {@link #withSecurityGroupIds(java.util.Collection)} if you
* want to override the existing values.
*
*
* @param securityGroupIds
* An array of security group IDs to associate with the workgroup.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public UpdateWorkgroupRequest withSecurityGroupIds(String... securityGroupIds) {
if (this.securityGroupIds == null) {
setSecurityGroupIds(new java.util.ArrayList(securityGroupIds.length));
}
for (String ele : securityGroupIds) {
this.securityGroupIds.add(ele);
}
return this;
}
/**
*
* An array of security group IDs to associate with the workgroup.
*
*
* @param securityGroupIds
* An array of security group IDs to associate with the workgroup.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public UpdateWorkgroupRequest withSecurityGroupIds(java.util.Collection securityGroupIds) {
setSecurityGroupIds(securityGroupIds);
return this;
}
/**
*
* An array of VPC subnet IDs to associate with the workgroup.
*
*
* @return An array of VPC subnet IDs to associate with the workgroup.
*/
public java.util.List getSubnetIds() {
return subnetIds;
}
/**
*
* An array of VPC subnet IDs to associate with the workgroup.
*
*
* @param subnetIds
* An array of VPC subnet IDs to associate with the workgroup.
*/
public void setSubnetIds(java.util.Collection subnetIds) {
if (subnetIds == null) {
this.subnetIds = null;
return;
}
this.subnetIds = new java.util.ArrayList(subnetIds);
}
/**
*
* An array of VPC subnet IDs to associate with the workgroup.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setSubnetIds(java.util.Collection)} or {@link #withSubnetIds(java.util.Collection)} if you want to
* override the existing values.
*
*
* @param subnetIds
* An array of VPC subnet IDs to associate with the workgroup.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public UpdateWorkgroupRequest withSubnetIds(String... subnetIds) {
if (this.subnetIds == null) {
setSubnetIds(new java.util.ArrayList(subnetIds.length));
}
for (String ele : subnetIds) {
this.subnetIds.add(ele);
}
return this;
}
/**
*
* An array of VPC subnet IDs to associate with the workgroup.
*
*
* @param subnetIds
* An array of VPC subnet IDs to associate with the workgroup.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public UpdateWorkgroupRequest withSubnetIds(java.util.Collection subnetIds) {
setSubnetIds(subnetIds);
return this;
}
/**
*
* The name of the workgroup to update. You can't update the name of a workgroup once it is created.
*
*
* @param workgroupName
* The name of the workgroup to update. You can't update the name of a workgroup once it is created.
*/
public void setWorkgroupName(String workgroupName) {
this.workgroupName = workgroupName;
}
/**
*
* The name of the workgroup to update. You can't update the name of a workgroup once it is created.
*
*
* @return The name of the workgroup to update. You can't update the name of a workgroup once it is created.
*/
public String getWorkgroupName() {
return this.workgroupName;
}
/**
*
* The name of the workgroup to update. You can't update the name of a workgroup once it is created.
*
*
* @param workgroupName
* The name of the workgroup to update. You can't update the name of a workgroup once it is created.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public UpdateWorkgroupRequest withWorkgroupName(String workgroupName) {
setWorkgroupName(workgroupName);
return this;
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getBaseCapacity() != null)
sb.append("BaseCapacity: ").append(getBaseCapacity()).append(",");
if (getConfigParameters() != null)
sb.append("ConfigParameters: ").append(getConfigParameters()).append(",");
if (getEnhancedVpcRouting() != null)
sb.append("EnhancedVpcRouting: ").append(getEnhancedVpcRouting()).append(",");
if (getIpAddressType() != null)
sb.append("IpAddressType: ").append(getIpAddressType()).append(",");
if (getMaxCapacity() != null)
sb.append("MaxCapacity: ").append(getMaxCapacity()).append(",");
if (getPort() != null)
sb.append("Port: ").append(getPort()).append(",");
if (getPubliclyAccessible() != null)
sb.append("PubliclyAccessible: ").append(getPubliclyAccessible()).append(",");
if (getSecurityGroupIds() != null)
sb.append("SecurityGroupIds: ").append(getSecurityGroupIds()).append(",");
if (getSubnetIds() != null)
sb.append("SubnetIds: ").append(getSubnetIds()).append(",");
if (getWorkgroupName() != null)
sb.append("WorkgroupName: ").append(getWorkgroupName());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof UpdateWorkgroupRequest == false)
return false;
UpdateWorkgroupRequest other = (UpdateWorkgroupRequest) obj;
if (other.getBaseCapacity() == null ^ this.getBaseCapacity() == null)
return false;
if (other.getBaseCapacity() != null && other.getBaseCapacity().equals(this.getBaseCapacity()) == false)
return false;
if (other.getConfigParameters() == null ^ this.getConfigParameters() == null)
return false;
if (other.getConfigParameters() != null && other.getConfigParameters().equals(this.getConfigParameters()) == false)
return false;
if (other.getEnhancedVpcRouting() == null ^ this.getEnhancedVpcRouting() == null)
return false;
if (other.getEnhancedVpcRouting() != null && other.getEnhancedVpcRouting().equals(this.getEnhancedVpcRouting()) == false)
return false;
if (other.getIpAddressType() == null ^ this.getIpAddressType() == null)
return false;
if (other.getIpAddressType() != null && other.getIpAddressType().equals(this.getIpAddressType()) == false)
return false;
if (other.getMaxCapacity() == null ^ this.getMaxCapacity() == null)
return false;
if (other.getMaxCapacity() != null && other.getMaxCapacity().equals(this.getMaxCapacity()) == false)
return false;
if (other.getPort() == null ^ this.getPort() == null)
return false;
if (other.getPort() != null && other.getPort().equals(this.getPort()) == false)
return false;
if (other.getPubliclyAccessible() == null ^ this.getPubliclyAccessible() == null)
return false;
if (other.getPubliclyAccessible() != null && other.getPubliclyAccessible().equals(this.getPubliclyAccessible()) == false)
return false;
if (other.getSecurityGroupIds() == null ^ this.getSecurityGroupIds() == null)
return false;
if (other.getSecurityGroupIds() != null && other.getSecurityGroupIds().equals(this.getSecurityGroupIds()) == false)
return false;
if (other.getSubnetIds() == null ^ this.getSubnetIds() == null)
return false;
if (other.getSubnetIds() != null && other.getSubnetIds().equals(this.getSubnetIds()) == false)
return false;
if (other.getWorkgroupName() == null ^ this.getWorkgroupName() == null)
return false;
if (other.getWorkgroupName() != null && other.getWorkgroupName().equals(this.getWorkgroupName()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getBaseCapacity() == null) ? 0 : getBaseCapacity().hashCode());
hashCode = prime * hashCode + ((getConfigParameters() == null) ? 0 : getConfigParameters().hashCode());
hashCode = prime * hashCode + ((getEnhancedVpcRouting() == null) ? 0 : getEnhancedVpcRouting().hashCode());
hashCode = prime * hashCode + ((getIpAddressType() == null) ? 0 : getIpAddressType().hashCode());
hashCode = prime * hashCode + ((getMaxCapacity() == null) ? 0 : getMaxCapacity().hashCode());
hashCode = prime * hashCode + ((getPort() == null) ? 0 : getPort().hashCode());
hashCode = prime * hashCode + ((getPubliclyAccessible() == null) ? 0 : getPubliclyAccessible().hashCode());
hashCode = prime * hashCode + ((getSecurityGroupIds() == null) ? 0 : getSecurityGroupIds().hashCode());
hashCode = prime * hashCode + ((getSubnetIds() == null) ? 0 : getSubnetIds().hashCode());
hashCode = prime * hashCode + ((getWorkgroupName() == null) ? 0 : getWorkgroupName().hashCode());
return hashCode;
}
@Override
public UpdateWorkgroupRequest clone() {
return (UpdateWorkgroupRequest) super.clone();
}
}