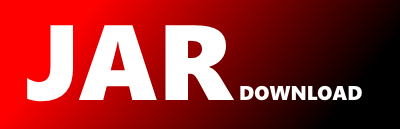
com.amazonaws.services.redshiftserverless.model.Workgroup Maven / Gradle / Ivy
Show all versions of aws-java-sdk-redshiftserverless Show documentation
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.redshiftserverless.model;
import java.io.Serializable;
import javax.annotation.Generated;
import com.amazonaws.protocol.StructuredPojo;
import com.amazonaws.protocol.ProtocolMarshaller;
/**
*
* The collection of computing resources from which an endpoint is created.
*
*
* @see AWS API
* Documentation
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class Workgroup implements Serializable, Cloneable, StructuredPojo {
/**
*
* The base data warehouse capacity of the workgroup in Redshift Processing Units (RPUs).
*
*/
private Integer baseCapacity;
/**
*
* An array of parameters to set for advanced control over a database. The options are auto_mv
,
* datestyle
, enable_case_sensitive_identifier
, enable_user_activity_logging
,
* query_group
, search_path
, require_ssl
, use_fips_ssl
, and
* query monitoring metrics that let you define performance boundaries. For more information about query monitoring
* rules and available metrics, see Query monitoring metrics for Amazon Redshift Serverless.
*
*/
private java.util.List configParameters;
/**
*
* The creation date of the workgroup.
*
*/
private java.util.Date creationDate;
/**
*
* A list of VPCs. Each entry is the unique identifier of a virtual private cloud with access to Amazon Redshift
* Serverless. If all of the VPCs for the grantee are allowed, it shows an asterisk.
*
*/
private java.util.List crossAccountVpcs;
/**
*
* The custom domain name’s certificate Amazon resource name (ARN).
*
*/
private String customDomainCertificateArn;
/**
*
* The expiration time for the certificate.
*
*/
private java.util.Date customDomainCertificateExpiryTime;
/**
*
* The custom domain name associated with the workgroup.
*
*/
private String customDomainName;
/**
*
* The endpoint that is created from the workgroup.
*
*/
private Endpoint endpoint;
/**
*
* The value that specifies whether to enable enhanced virtual private cloud (VPC) routing, which forces Amazon
* Redshift Serverless to route traffic through your VPC.
*
*/
private Boolean enhancedVpcRouting;
/**
*
* The IP address type that the workgroup supports. Possible values are ipv4
and dualstack
* .
*
*/
private String ipAddressType;
/**
*
* The maximum data-warehouse capacity Amazon Redshift Serverless uses to serve queries. The max capacity is
* specified in RPUs.
*
*/
private Integer maxCapacity;
/**
*
* The namespace the workgroup is associated with.
*
*/
private String namespaceName;
/**
*
* The patch version of your Amazon Redshift Serverless workgroup. For more information about patch versions, see Cluster versions for Amazon
* Redshift.
*
*/
private String patchVersion;
/**
*
* The custom port to use when connecting to a workgroup. Valid port ranges are 5431-5455 and 8191-8215. The default
* is 5439.
*
*/
private Integer port;
/**
*
* A value that specifies whether the workgroup can be accessible from a public network.
*
*/
private Boolean publiclyAccessible;
/**
*
* An array of security group IDs to associate with the workgroup.
*
*/
private java.util.List securityGroupIds;
/**
*
* The status of the workgroup.
*
*/
private String status;
/**
*
* An array of subnet IDs the workgroup is associated with.
*
*/
private java.util.List subnetIds;
/**
*
* The Amazon Resource Name (ARN) that links to the workgroup.
*
*/
private String workgroupArn;
/**
*
* The unique identifier of the workgroup.
*
*/
private String workgroupId;
/**
*
* The name of the workgroup.
*
*/
private String workgroupName;
/**
*
* The Amazon Redshift Serverless version of your workgroup. For more information about Amazon Redshift Serverless
* versions, seeCluster versions
* for Amazon Redshift.
*
*/
private String workgroupVersion;
/**
*
* The base data warehouse capacity of the workgroup in Redshift Processing Units (RPUs).
*
*
* @param baseCapacity
* The base data warehouse capacity of the workgroup in Redshift Processing Units (RPUs).
*/
public void setBaseCapacity(Integer baseCapacity) {
this.baseCapacity = baseCapacity;
}
/**
*
* The base data warehouse capacity of the workgroup in Redshift Processing Units (RPUs).
*
*
* @return The base data warehouse capacity of the workgroup in Redshift Processing Units (RPUs).
*/
public Integer getBaseCapacity() {
return this.baseCapacity;
}
/**
*
* The base data warehouse capacity of the workgroup in Redshift Processing Units (RPUs).
*
*
* @param baseCapacity
* The base data warehouse capacity of the workgroup in Redshift Processing Units (RPUs).
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Workgroup withBaseCapacity(Integer baseCapacity) {
setBaseCapacity(baseCapacity);
return this;
}
/**
*
* An array of parameters to set for advanced control over a database. The options are auto_mv
,
* datestyle
, enable_case_sensitive_identifier
, enable_user_activity_logging
,
* query_group
, search_path
, require_ssl
, use_fips_ssl
, and
* query monitoring metrics that let you define performance boundaries. For more information about query monitoring
* rules and available metrics, see Query monitoring metrics for Amazon Redshift Serverless.
*
*
* @return An array of parameters to set for advanced control over a database. The options are auto_mv
,
* datestyle
, enable_case_sensitive_identifier
,
* enable_user_activity_logging
, query_group
, search_path
,
* require_ssl
, use_fips_ssl
, and query monitoring metrics that let you define
* performance boundaries. For more information about query monitoring rules and available metrics, see Query monitoring metrics for Amazon Redshift Serverless.
*/
public java.util.List getConfigParameters() {
return configParameters;
}
/**
*
* An array of parameters to set for advanced control over a database. The options are auto_mv
,
* datestyle
, enable_case_sensitive_identifier
, enable_user_activity_logging
,
* query_group
, search_path
, require_ssl
, use_fips_ssl
, and
* query monitoring metrics that let you define performance boundaries. For more information about query monitoring
* rules and available metrics, see Query monitoring metrics for Amazon Redshift Serverless.
*
*
* @param configParameters
* An array of parameters to set for advanced control over a database. The options are auto_mv
,
* datestyle
, enable_case_sensitive_identifier
,
* enable_user_activity_logging
, query_group
, search_path
,
* require_ssl
, use_fips_ssl
, and query monitoring metrics that let you define
* performance boundaries. For more information about query monitoring rules and available metrics, see Query monitoring metrics for Amazon Redshift Serverless.
*/
public void setConfigParameters(java.util.Collection configParameters) {
if (configParameters == null) {
this.configParameters = null;
return;
}
this.configParameters = new java.util.ArrayList(configParameters);
}
/**
*
* An array of parameters to set for advanced control over a database. The options are auto_mv
,
* datestyle
, enable_case_sensitive_identifier
, enable_user_activity_logging
,
* query_group
, search_path
, require_ssl
, use_fips_ssl
, and
* query monitoring metrics that let you define performance boundaries. For more information about query monitoring
* rules and available metrics, see Query monitoring metrics for Amazon Redshift Serverless.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setConfigParameters(java.util.Collection)} or {@link #withConfigParameters(java.util.Collection)} if you
* want to override the existing values.
*
*
* @param configParameters
* An array of parameters to set for advanced control over a database. The options are auto_mv
,
* datestyle
, enable_case_sensitive_identifier
,
* enable_user_activity_logging
, query_group
, search_path
,
* require_ssl
, use_fips_ssl
, and query monitoring metrics that let you define
* performance boundaries. For more information about query monitoring rules and available metrics, see Query monitoring metrics for Amazon Redshift Serverless.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Workgroup withConfigParameters(ConfigParameter... configParameters) {
if (this.configParameters == null) {
setConfigParameters(new java.util.ArrayList(configParameters.length));
}
for (ConfigParameter ele : configParameters) {
this.configParameters.add(ele);
}
return this;
}
/**
*
* An array of parameters to set for advanced control over a database. The options are auto_mv
,
* datestyle
, enable_case_sensitive_identifier
, enable_user_activity_logging
,
* query_group
, search_path
, require_ssl
, use_fips_ssl
, and
* query monitoring metrics that let you define performance boundaries. For more information about query monitoring
* rules and available metrics, see Query monitoring metrics for Amazon Redshift Serverless.
*
*
* @param configParameters
* An array of parameters to set for advanced control over a database. The options are auto_mv
,
* datestyle
, enable_case_sensitive_identifier
,
* enable_user_activity_logging
, query_group
, search_path
,
* require_ssl
, use_fips_ssl
, and query monitoring metrics that let you define
* performance boundaries. For more information about query monitoring rules and available metrics, see Query monitoring metrics for Amazon Redshift Serverless.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Workgroup withConfigParameters(java.util.Collection configParameters) {
setConfigParameters(configParameters);
return this;
}
/**
*
* The creation date of the workgroup.
*
*
* @param creationDate
* The creation date of the workgroup.
*/
public void setCreationDate(java.util.Date creationDate) {
this.creationDate = creationDate;
}
/**
*
* The creation date of the workgroup.
*
*
* @return The creation date of the workgroup.
*/
public java.util.Date getCreationDate() {
return this.creationDate;
}
/**
*
* The creation date of the workgroup.
*
*
* @param creationDate
* The creation date of the workgroup.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Workgroup withCreationDate(java.util.Date creationDate) {
setCreationDate(creationDate);
return this;
}
/**
*
* A list of VPCs. Each entry is the unique identifier of a virtual private cloud with access to Amazon Redshift
* Serverless. If all of the VPCs for the grantee are allowed, it shows an asterisk.
*
*
* @return A list of VPCs. Each entry is the unique identifier of a virtual private cloud with access to Amazon
* Redshift Serverless. If all of the VPCs for the grantee are allowed, it shows an asterisk.
*/
public java.util.List getCrossAccountVpcs() {
return crossAccountVpcs;
}
/**
*
* A list of VPCs. Each entry is the unique identifier of a virtual private cloud with access to Amazon Redshift
* Serverless. If all of the VPCs for the grantee are allowed, it shows an asterisk.
*
*
* @param crossAccountVpcs
* A list of VPCs. Each entry is the unique identifier of a virtual private cloud with access to Amazon
* Redshift Serverless. If all of the VPCs for the grantee are allowed, it shows an asterisk.
*/
public void setCrossAccountVpcs(java.util.Collection crossAccountVpcs) {
if (crossAccountVpcs == null) {
this.crossAccountVpcs = null;
return;
}
this.crossAccountVpcs = new java.util.ArrayList(crossAccountVpcs);
}
/**
*
* A list of VPCs. Each entry is the unique identifier of a virtual private cloud with access to Amazon Redshift
* Serverless. If all of the VPCs for the grantee are allowed, it shows an asterisk.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setCrossAccountVpcs(java.util.Collection)} or {@link #withCrossAccountVpcs(java.util.Collection)} if you
* want to override the existing values.
*
*
* @param crossAccountVpcs
* A list of VPCs. Each entry is the unique identifier of a virtual private cloud with access to Amazon
* Redshift Serverless. If all of the VPCs for the grantee are allowed, it shows an asterisk.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Workgroup withCrossAccountVpcs(String... crossAccountVpcs) {
if (this.crossAccountVpcs == null) {
setCrossAccountVpcs(new java.util.ArrayList(crossAccountVpcs.length));
}
for (String ele : crossAccountVpcs) {
this.crossAccountVpcs.add(ele);
}
return this;
}
/**
*
* A list of VPCs. Each entry is the unique identifier of a virtual private cloud with access to Amazon Redshift
* Serverless. If all of the VPCs for the grantee are allowed, it shows an asterisk.
*
*
* @param crossAccountVpcs
* A list of VPCs. Each entry is the unique identifier of a virtual private cloud with access to Amazon
* Redshift Serverless. If all of the VPCs for the grantee are allowed, it shows an asterisk.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Workgroup withCrossAccountVpcs(java.util.Collection crossAccountVpcs) {
setCrossAccountVpcs(crossAccountVpcs);
return this;
}
/**
*
* The custom domain name’s certificate Amazon resource name (ARN).
*
*
* @param customDomainCertificateArn
* The custom domain name’s certificate Amazon resource name (ARN).
*/
public void setCustomDomainCertificateArn(String customDomainCertificateArn) {
this.customDomainCertificateArn = customDomainCertificateArn;
}
/**
*
* The custom domain name’s certificate Amazon resource name (ARN).
*
*
* @return The custom domain name’s certificate Amazon resource name (ARN).
*/
public String getCustomDomainCertificateArn() {
return this.customDomainCertificateArn;
}
/**
*
* The custom domain name’s certificate Amazon resource name (ARN).
*
*
* @param customDomainCertificateArn
* The custom domain name’s certificate Amazon resource name (ARN).
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Workgroup withCustomDomainCertificateArn(String customDomainCertificateArn) {
setCustomDomainCertificateArn(customDomainCertificateArn);
return this;
}
/**
*
* The expiration time for the certificate.
*
*
* @param customDomainCertificateExpiryTime
* The expiration time for the certificate.
*/
public void setCustomDomainCertificateExpiryTime(java.util.Date customDomainCertificateExpiryTime) {
this.customDomainCertificateExpiryTime = customDomainCertificateExpiryTime;
}
/**
*
* The expiration time for the certificate.
*
*
* @return The expiration time for the certificate.
*/
public java.util.Date getCustomDomainCertificateExpiryTime() {
return this.customDomainCertificateExpiryTime;
}
/**
*
* The expiration time for the certificate.
*
*
* @param customDomainCertificateExpiryTime
* The expiration time for the certificate.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Workgroup withCustomDomainCertificateExpiryTime(java.util.Date customDomainCertificateExpiryTime) {
setCustomDomainCertificateExpiryTime(customDomainCertificateExpiryTime);
return this;
}
/**
*
* The custom domain name associated with the workgroup.
*
*
* @param customDomainName
* The custom domain name associated with the workgroup.
*/
public void setCustomDomainName(String customDomainName) {
this.customDomainName = customDomainName;
}
/**
*
* The custom domain name associated with the workgroup.
*
*
* @return The custom domain name associated with the workgroup.
*/
public String getCustomDomainName() {
return this.customDomainName;
}
/**
*
* The custom domain name associated with the workgroup.
*
*
* @param customDomainName
* The custom domain name associated with the workgroup.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Workgroup withCustomDomainName(String customDomainName) {
setCustomDomainName(customDomainName);
return this;
}
/**
*
* The endpoint that is created from the workgroup.
*
*
* @param endpoint
* The endpoint that is created from the workgroup.
*/
public void setEndpoint(Endpoint endpoint) {
this.endpoint = endpoint;
}
/**
*
* The endpoint that is created from the workgroup.
*
*
* @return The endpoint that is created from the workgroup.
*/
public Endpoint getEndpoint() {
return this.endpoint;
}
/**
*
* The endpoint that is created from the workgroup.
*
*
* @param endpoint
* The endpoint that is created from the workgroup.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Workgroup withEndpoint(Endpoint endpoint) {
setEndpoint(endpoint);
return this;
}
/**
*
* The value that specifies whether to enable enhanced virtual private cloud (VPC) routing, which forces Amazon
* Redshift Serverless to route traffic through your VPC.
*
*
* @param enhancedVpcRouting
* The value that specifies whether to enable enhanced virtual private cloud (VPC) routing, which forces
* Amazon Redshift Serverless to route traffic through your VPC.
*/
public void setEnhancedVpcRouting(Boolean enhancedVpcRouting) {
this.enhancedVpcRouting = enhancedVpcRouting;
}
/**
*
* The value that specifies whether to enable enhanced virtual private cloud (VPC) routing, which forces Amazon
* Redshift Serverless to route traffic through your VPC.
*
*
* @return The value that specifies whether to enable enhanced virtual private cloud (VPC) routing, which forces
* Amazon Redshift Serverless to route traffic through your VPC.
*/
public Boolean getEnhancedVpcRouting() {
return this.enhancedVpcRouting;
}
/**
*
* The value that specifies whether to enable enhanced virtual private cloud (VPC) routing, which forces Amazon
* Redshift Serverless to route traffic through your VPC.
*
*
* @param enhancedVpcRouting
* The value that specifies whether to enable enhanced virtual private cloud (VPC) routing, which forces
* Amazon Redshift Serverless to route traffic through your VPC.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Workgroup withEnhancedVpcRouting(Boolean enhancedVpcRouting) {
setEnhancedVpcRouting(enhancedVpcRouting);
return this;
}
/**
*
* The value that specifies whether to enable enhanced virtual private cloud (VPC) routing, which forces Amazon
* Redshift Serverless to route traffic through your VPC.
*
*
* @return The value that specifies whether to enable enhanced virtual private cloud (VPC) routing, which forces
* Amazon Redshift Serverless to route traffic through your VPC.
*/
public Boolean isEnhancedVpcRouting() {
return this.enhancedVpcRouting;
}
/**
*
* The IP address type that the workgroup supports. Possible values are ipv4
and dualstack
* .
*
*
* @param ipAddressType
* The IP address type that the workgroup supports. Possible values are ipv4
and
* dualstack
.
*/
public void setIpAddressType(String ipAddressType) {
this.ipAddressType = ipAddressType;
}
/**
*
* The IP address type that the workgroup supports. Possible values are ipv4
and dualstack
* .
*
*
* @return The IP address type that the workgroup supports. Possible values are ipv4
and
* dualstack
.
*/
public String getIpAddressType() {
return this.ipAddressType;
}
/**
*
* The IP address type that the workgroup supports. Possible values are ipv4
and dualstack
* .
*
*
* @param ipAddressType
* The IP address type that the workgroup supports. Possible values are ipv4
and
* dualstack
.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Workgroup withIpAddressType(String ipAddressType) {
setIpAddressType(ipAddressType);
return this;
}
/**
*
* The maximum data-warehouse capacity Amazon Redshift Serverless uses to serve queries. The max capacity is
* specified in RPUs.
*
*
* @param maxCapacity
* The maximum data-warehouse capacity Amazon Redshift Serverless uses to serve queries. The max capacity is
* specified in RPUs.
*/
public void setMaxCapacity(Integer maxCapacity) {
this.maxCapacity = maxCapacity;
}
/**
*
* The maximum data-warehouse capacity Amazon Redshift Serverless uses to serve queries. The max capacity is
* specified in RPUs.
*
*
* @return The maximum data-warehouse capacity Amazon Redshift Serverless uses to serve queries. The max capacity is
* specified in RPUs.
*/
public Integer getMaxCapacity() {
return this.maxCapacity;
}
/**
*
* The maximum data-warehouse capacity Amazon Redshift Serverless uses to serve queries. The max capacity is
* specified in RPUs.
*
*
* @param maxCapacity
* The maximum data-warehouse capacity Amazon Redshift Serverless uses to serve queries. The max capacity is
* specified in RPUs.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Workgroup withMaxCapacity(Integer maxCapacity) {
setMaxCapacity(maxCapacity);
return this;
}
/**
*
* The namespace the workgroup is associated with.
*
*
* @param namespaceName
* The namespace the workgroup is associated with.
*/
public void setNamespaceName(String namespaceName) {
this.namespaceName = namespaceName;
}
/**
*
* The namespace the workgroup is associated with.
*
*
* @return The namespace the workgroup is associated with.
*/
public String getNamespaceName() {
return this.namespaceName;
}
/**
*
* The namespace the workgroup is associated with.
*
*
* @param namespaceName
* The namespace the workgroup is associated with.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Workgroup withNamespaceName(String namespaceName) {
setNamespaceName(namespaceName);
return this;
}
/**
*
* The patch version of your Amazon Redshift Serverless workgroup. For more information about patch versions, see Cluster versions for Amazon
* Redshift.
*
*
* @param patchVersion
* The patch version of your Amazon Redshift Serverless workgroup. For more information about patch versions,
* see Cluster versions for
* Amazon Redshift.
*/
public void setPatchVersion(String patchVersion) {
this.patchVersion = patchVersion;
}
/**
*
* The patch version of your Amazon Redshift Serverless workgroup. For more information about patch versions, see Cluster versions for Amazon
* Redshift.
*
*
* @return The patch version of your Amazon Redshift Serverless workgroup. For more information about patch
* versions, see Cluster
* versions for Amazon Redshift.
*/
public String getPatchVersion() {
return this.patchVersion;
}
/**
*
* The patch version of your Amazon Redshift Serverless workgroup. For more information about patch versions, see Cluster versions for Amazon
* Redshift.
*
*
* @param patchVersion
* The patch version of your Amazon Redshift Serverless workgroup. For more information about patch versions,
* see Cluster versions for
* Amazon Redshift.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Workgroup withPatchVersion(String patchVersion) {
setPatchVersion(patchVersion);
return this;
}
/**
*
* The custom port to use when connecting to a workgroup. Valid port ranges are 5431-5455 and 8191-8215. The default
* is 5439.
*
*
* @param port
* The custom port to use when connecting to a workgroup. Valid port ranges are 5431-5455 and 8191-8215. The
* default is 5439.
*/
public void setPort(Integer port) {
this.port = port;
}
/**
*
* The custom port to use when connecting to a workgroup. Valid port ranges are 5431-5455 and 8191-8215. The default
* is 5439.
*
*
* @return The custom port to use when connecting to a workgroup. Valid port ranges are 5431-5455 and 8191-8215. The
* default is 5439.
*/
public Integer getPort() {
return this.port;
}
/**
*
* The custom port to use when connecting to a workgroup. Valid port ranges are 5431-5455 and 8191-8215. The default
* is 5439.
*
*
* @param port
* The custom port to use when connecting to a workgroup. Valid port ranges are 5431-5455 and 8191-8215. The
* default is 5439.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Workgroup withPort(Integer port) {
setPort(port);
return this;
}
/**
*
* A value that specifies whether the workgroup can be accessible from a public network.
*
*
* @param publiclyAccessible
* A value that specifies whether the workgroup can be accessible from a public network.
*/
public void setPubliclyAccessible(Boolean publiclyAccessible) {
this.publiclyAccessible = publiclyAccessible;
}
/**
*
* A value that specifies whether the workgroup can be accessible from a public network.
*
*
* @return A value that specifies whether the workgroup can be accessible from a public network.
*/
public Boolean getPubliclyAccessible() {
return this.publiclyAccessible;
}
/**
*
* A value that specifies whether the workgroup can be accessible from a public network.
*
*
* @param publiclyAccessible
* A value that specifies whether the workgroup can be accessible from a public network.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Workgroup withPubliclyAccessible(Boolean publiclyAccessible) {
setPubliclyAccessible(publiclyAccessible);
return this;
}
/**
*
* A value that specifies whether the workgroup can be accessible from a public network.
*
*
* @return A value that specifies whether the workgroup can be accessible from a public network.
*/
public Boolean isPubliclyAccessible() {
return this.publiclyAccessible;
}
/**
*
* An array of security group IDs to associate with the workgroup.
*
*
* @return An array of security group IDs to associate with the workgroup.
*/
public java.util.List getSecurityGroupIds() {
return securityGroupIds;
}
/**
*
* An array of security group IDs to associate with the workgroup.
*
*
* @param securityGroupIds
* An array of security group IDs to associate with the workgroup.
*/
public void setSecurityGroupIds(java.util.Collection securityGroupIds) {
if (securityGroupIds == null) {
this.securityGroupIds = null;
return;
}
this.securityGroupIds = new java.util.ArrayList(securityGroupIds);
}
/**
*
* An array of security group IDs to associate with the workgroup.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setSecurityGroupIds(java.util.Collection)} or {@link #withSecurityGroupIds(java.util.Collection)} if you
* want to override the existing values.
*
*
* @param securityGroupIds
* An array of security group IDs to associate with the workgroup.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Workgroup withSecurityGroupIds(String... securityGroupIds) {
if (this.securityGroupIds == null) {
setSecurityGroupIds(new java.util.ArrayList(securityGroupIds.length));
}
for (String ele : securityGroupIds) {
this.securityGroupIds.add(ele);
}
return this;
}
/**
*
* An array of security group IDs to associate with the workgroup.
*
*
* @param securityGroupIds
* An array of security group IDs to associate with the workgroup.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Workgroup withSecurityGroupIds(java.util.Collection securityGroupIds) {
setSecurityGroupIds(securityGroupIds);
return this;
}
/**
*
* The status of the workgroup.
*
*
* @param status
* The status of the workgroup.
* @see WorkgroupStatus
*/
public void setStatus(String status) {
this.status = status;
}
/**
*
* The status of the workgroup.
*
*
* @return The status of the workgroup.
* @see WorkgroupStatus
*/
public String getStatus() {
return this.status;
}
/**
*
* The status of the workgroup.
*
*
* @param status
* The status of the workgroup.
* @return Returns a reference to this object so that method calls can be chained together.
* @see WorkgroupStatus
*/
public Workgroup withStatus(String status) {
setStatus(status);
return this;
}
/**
*
* The status of the workgroup.
*
*
* @param status
* The status of the workgroup.
* @return Returns a reference to this object so that method calls can be chained together.
* @see WorkgroupStatus
*/
public Workgroup withStatus(WorkgroupStatus status) {
this.status = status.toString();
return this;
}
/**
*
* An array of subnet IDs the workgroup is associated with.
*
*
* @return An array of subnet IDs the workgroup is associated with.
*/
public java.util.List getSubnetIds() {
return subnetIds;
}
/**
*
* An array of subnet IDs the workgroup is associated with.
*
*
* @param subnetIds
* An array of subnet IDs the workgroup is associated with.
*/
public void setSubnetIds(java.util.Collection subnetIds) {
if (subnetIds == null) {
this.subnetIds = null;
return;
}
this.subnetIds = new java.util.ArrayList(subnetIds);
}
/**
*
* An array of subnet IDs the workgroup is associated with.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setSubnetIds(java.util.Collection)} or {@link #withSubnetIds(java.util.Collection)} if you want to
* override the existing values.
*
*
* @param subnetIds
* An array of subnet IDs the workgroup is associated with.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Workgroup withSubnetIds(String... subnetIds) {
if (this.subnetIds == null) {
setSubnetIds(new java.util.ArrayList(subnetIds.length));
}
for (String ele : subnetIds) {
this.subnetIds.add(ele);
}
return this;
}
/**
*
* An array of subnet IDs the workgroup is associated with.
*
*
* @param subnetIds
* An array of subnet IDs the workgroup is associated with.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Workgroup withSubnetIds(java.util.Collection subnetIds) {
setSubnetIds(subnetIds);
return this;
}
/**
*
* The Amazon Resource Name (ARN) that links to the workgroup.
*
*
* @param workgroupArn
* The Amazon Resource Name (ARN) that links to the workgroup.
*/
public void setWorkgroupArn(String workgroupArn) {
this.workgroupArn = workgroupArn;
}
/**
*
* The Amazon Resource Name (ARN) that links to the workgroup.
*
*
* @return The Amazon Resource Name (ARN) that links to the workgroup.
*/
public String getWorkgroupArn() {
return this.workgroupArn;
}
/**
*
* The Amazon Resource Name (ARN) that links to the workgroup.
*
*
* @param workgroupArn
* The Amazon Resource Name (ARN) that links to the workgroup.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Workgroup withWorkgroupArn(String workgroupArn) {
setWorkgroupArn(workgroupArn);
return this;
}
/**
*
* The unique identifier of the workgroup.
*
*
* @param workgroupId
* The unique identifier of the workgroup.
*/
public void setWorkgroupId(String workgroupId) {
this.workgroupId = workgroupId;
}
/**
*
* The unique identifier of the workgroup.
*
*
* @return The unique identifier of the workgroup.
*/
public String getWorkgroupId() {
return this.workgroupId;
}
/**
*
* The unique identifier of the workgroup.
*
*
* @param workgroupId
* The unique identifier of the workgroup.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Workgroup withWorkgroupId(String workgroupId) {
setWorkgroupId(workgroupId);
return this;
}
/**
*
* The name of the workgroup.
*
*
* @param workgroupName
* The name of the workgroup.
*/
public void setWorkgroupName(String workgroupName) {
this.workgroupName = workgroupName;
}
/**
*
* The name of the workgroup.
*
*
* @return The name of the workgroup.
*/
public String getWorkgroupName() {
return this.workgroupName;
}
/**
*
* The name of the workgroup.
*
*
* @param workgroupName
* The name of the workgroup.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Workgroup withWorkgroupName(String workgroupName) {
setWorkgroupName(workgroupName);
return this;
}
/**
*
* The Amazon Redshift Serverless version of your workgroup. For more information about Amazon Redshift Serverless
* versions, seeCluster versions
* for Amazon Redshift.
*
*
* @param workgroupVersion
* The Amazon Redshift Serverless version of your workgroup. For more information about Amazon Redshift
* Serverless versions, seeCluster versions for Amazon
* Redshift.
*/
public void setWorkgroupVersion(String workgroupVersion) {
this.workgroupVersion = workgroupVersion;
}
/**
*
* The Amazon Redshift Serverless version of your workgroup. For more information about Amazon Redshift Serverless
* versions, seeCluster versions
* for Amazon Redshift.
*
*
* @return The Amazon Redshift Serverless version of your workgroup. For more information about Amazon Redshift
* Serverless versions, seeCluster versions for Amazon
* Redshift.
*/
public String getWorkgroupVersion() {
return this.workgroupVersion;
}
/**
*
* The Amazon Redshift Serverless version of your workgroup. For more information about Amazon Redshift Serverless
* versions, seeCluster versions
* for Amazon Redshift.
*
*
* @param workgroupVersion
* The Amazon Redshift Serverless version of your workgroup. For more information about Amazon Redshift
* Serverless versions, seeCluster versions for Amazon
* Redshift.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Workgroup withWorkgroupVersion(String workgroupVersion) {
setWorkgroupVersion(workgroupVersion);
return this;
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getBaseCapacity() != null)
sb.append("BaseCapacity: ").append(getBaseCapacity()).append(",");
if (getConfigParameters() != null)
sb.append("ConfigParameters: ").append(getConfigParameters()).append(",");
if (getCreationDate() != null)
sb.append("CreationDate: ").append(getCreationDate()).append(",");
if (getCrossAccountVpcs() != null)
sb.append("CrossAccountVpcs: ").append(getCrossAccountVpcs()).append(",");
if (getCustomDomainCertificateArn() != null)
sb.append("CustomDomainCertificateArn: ").append(getCustomDomainCertificateArn()).append(",");
if (getCustomDomainCertificateExpiryTime() != null)
sb.append("CustomDomainCertificateExpiryTime: ").append(getCustomDomainCertificateExpiryTime()).append(",");
if (getCustomDomainName() != null)
sb.append("CustomDomainName: ").append(getCustomDomainName()).append(",");
if (getEndpoint() != null)
sb.append("Endpoint: ").append(getEndpoint()).append(",");
if (getEnhancedVpcRouting() != null)
sb.append("EnhancedVpcRouting: ").append(getEnhancedVpcRouting()).append(",");
if (getIpAddressType() != null)
sb.append("IpAddressType: ").append(getIpAddressType()).append(",");
if (getMaxCapacity() != null)
sb.append("MaxCapacity: ").append(getMaxCapacity()).append(",");
if (getNamespaceName() != null)
sb.append("NamespaceName: ").append(getNamespaceName()).append(",");
if (getPatchVersion() != null)
sb.append("PatchVersion: ").append(getPatchVersion()).append(",");
if (getPort() != null)
sb.append("Port: ").append(getPort()).append(",");
if (getPubliclyAccessible() != null)
sb.append("PubliclyAccessible: ").append(getPubliclyAccessible()).append(",");
if (getSecurityGroupIds() != null)
sb.append("SecurityGroupIds: ").append(getSecurityGroupIds()).append(",");
if (getStatus() != null)
sb.append("Status: ").append(getStatus()).append(",");
if (getSubnetIds() != null)
sb.append("SubnetIds: ").append(getSubnetIds()).append(",");
if (getWorkgroupArn() != null)
sb.append("WorkgroupArn: ").append(getWorkgroupArn()).append(",");
if (getWorkgroupId() != null)
sb.append("WorkgroupId: ").append(getWorkgroupId()).append(",");
if (getWorkgroupName() != null)
sb.append("WorkgroupName: ").append(getWorkgroupName()).append(",");
if (getWorkgroupVersion() != null)
sb.append("WorkgroupVersion: ").append(getWorkgroupVersion());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof Workgroup == false)
return false;
Workgroup other = (Workgroup) obj;
if (other.getBaseCapacity() == null ^ this.getBaseCapacity() == null)
return false;
if (other.getBaseCapacity() != null && other.getBaseCapacity().equals(this.getBaseCapacity()) == false)
return false;
if (other.getConfigParameters() == null ^ this.getConfigParameters() == null)
return false;
if (other.getConfigParameters() != null && other.getConfigParameters().equals(this.getConfigParameters()) == false)
return false;
if (other.getCreationDate() == null ^ this.getCreationDate() == null)
return false;
if (other.getCreationDate() != null && other.getCreationDate().equals(this.getCreationDate()) == false)
return false;
if (other.getCrossAccountVpcs() == null ^ this.getCrossAccountVpcs() == null)
return false;
if (other.getCrossAccountVpcs() != null && other.getCrossAccountVpcs().equals(this.getCrossAccountVpcs()) == false)
return false;
if (other.getCustomDomainCertificateArn() == null ^ this.getCustomDomainCertificateArn() == null)
return false;
if (other.getCustomDomainCertificateArn() != null && other.getCustomDomainCertificateArn().equals(this.getCustomDomainCertificateArn()) == false)
return false;
if (other.getCustomDomainCertificateExpiryTime() == null ^ this.getCustomDomainCertificateExpiryTime() == null)
return false;
if (other.getCustomDomainCertificateExpiryTime() != null
&& other.getCustomDomainCertificateExpiryTime().equals(this.getCustomDomainCertificateExpiryTime()) == false)
return false;
if (other.getCustomDomainName() == null ^ this.getCustomDomainName() == null)
return false;
if (other.getCustomDomainName() != null && other.getCustomDomainName().equals(this.getCustomDomainName()) == false)
return false;
if (other.getEndpoint() == null ^ this.getEndpoint() == null)
return false;
if (other.getEndpoint() != null && other.getEndpoint().equals(this.getEndpoint()) == false)
return false;
if (other.getEnhancedVpcRouting() == null ^ this.getEnhancedVpcRouting() == null)
return false;
if (other.getEnhancedVpcRouting() != null && other.getEnhancedVpcRouting().equals(this.getEnhancedVpcRouting()) == false)
return false;
if (other.getIpAddressType() == null ^ this.getIpAddressType() == null)
return false;
if (other.getIpAddressType() != null && other.getIpAddressType().equals(this.getIpAddressType()) == false)
return false;
if (other.getMaxCapacity() == null ^ this.getMaxCapacity() == null)
return false;
if (other.getMaxCapacity() != null && other.getMaxCapacity().equals(this.getMaxCapacity()) == false)
return false;
if (other.getNamespaceName() == null ^ this.getNamespaceName() == null)
return false;
if (other.getNamespaceName() != null && other.getNamespaceName().equals(this.getNamespaceName()) == false)
return false;
if (other.getPatchVersion() == null ^ this.getPatchVersion() == null)
return false;
if (other.getPatchVersion() != null && other.getPatchVersion().equals(this.getPatchVersion()) == false)
return false;
if (other.getPort() == null ^ this.getPort() == null)
return false;
if (other.getPort() != null && other.getPort().equals(this.getPort()) == false)
return false;
if (other.getPubliclyAccessible() == null ^ this.getPubliclyAccessible() == null)
return false;
if (other.getPubliclyAccessible() != null && other.getPubliclyAccessible().equals(this.getPubliclyAccessible()) == false)
return false;
if (other.getSecurityGroupIds() == null ^ this.getSecurityGroupIds() == null)
return false;
if (other.getSecurityGroupIds() != null && other.getSecurityGroupIds().equals(this.getSecurityGroupIds()) == false)
return false;
if (other.getStatus() == null ^ this.getStatus() == null)
return false;
if (other.getStatus() != null && other.getStatus().equals(this.getStatus()) == false)
return false;
if (other.getSubnetIds() == null ^ this.getSubnetIds() == null)
return false;
if (other.getSubnetIds() != null && other.getSubnetIds().equals(this.getSubnetIds()) == false)
return false;
if (other.getWorkgroupArn() == null ^ this.getWorkgroupArn() == null)
return false;
if (other.getWorkgroupArn() != null && other.getWorkgroupArn().equals(this.getWorkgroupArn()) == false)
return false;
if (other.getWorkgroupId() == null ^ this.getWorkgroupId() == null)
return false;
if (other.getWorkgroupId() != null && other.getWorkgroupId().equals(this.getWorkgroupId()) == false)
return false;
if (other.getWorkgroupName() == null ^ this.getWorkgroupName() == null)
return false;
if (other.getWorkgroupName() != null && other.getWorkgroupName().equals(this.getWorkgroupName()) == false)
return false;
if (other.getWorkgroupVersion() == null ^ this.getWorkgroupVersion() == null)
return false;
if (other.getWorkgroupVersion() != null && other.getWorkgroupVersion().equals(this.getWorkgroupVersion()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getBaseCapacity() == null) ? 0 : getBaseCapacity().hashCode());
hashCode = prime * hashCode + ((getConfigParameters() == null) ? 0 : getConfigParameters().hashCode());
hashCode = prime * hashCode + ((getCreationDate() == null) ? 0 : getCreationDate().hashCode());
hashCode = prime * hashCode + ((getCrossAccountVpcs() == null) ? 0 : getCrossAccountVpcs().hashCode());
hashCode = prime * hashCode + ((getCustomDomainCertificateArn() == null) ? 0 : getCustomDomainCertificateArn().hashCode());
hashCode = prime * hashCode + ((getCustomDomainCertificateExpiryTime() == null) ? 0 : getCustomDomainCertificateExpiryTime().hashCode());
hashCode = prime * hashCode + ((getCustomDomainName() == null) ? 0 : getCustomDomainName().hashCode());
hashCode = prime * hashCode + ((getEndpoint() == null) ? 0 : getEndpoint().hashCode());
hashCode = prime * hashCode + ((getEnhancedVpcRouting() == null) ? 0 : getEnhancedVpcRouting().hashCode());
hashCode = prime * hashCode + ((getIpAddressType() == null) ? 0 : getIpAddressType().hashCode());
hashCode = prime * hashCode + ((getMaxCapacity() == null) ? 0 : getMaxCapacity().hashCode());
hashCode = prime * hashCode + ((getNamespaceName() == null) ? 0 : getNamespaceName().hashCode());
hashCode = prime * hashCode + ((getPatchVersion() == null) ? 0 : getPatchVersion().hashCode());
hashCode = prime * hashCode + ((getPort() == null) ? 0 : getPort().hashCode());
hashCode = prime * hashCode + ((getPubliclyAccessible() == null) ? 0 : getPubliclyAccessible().hashCode());
hashCode = prime * hashCode + ((getSecurityGroupIds() == null) ? 0 : getSecurityGroupIds().hashCode());
hashCode = prime * hashCode + ((getStatus() == null) ? 0 : getStatus().hashCode());
hashCode = prime * hashCode + ((getSubnetIds() == null) ? 0 : getSubnetIds().hashCode());
hashCode = prime * hashCode + ((getWorkgroupArn() == null) ? 0 : getWorkgroupArn().hashCode());
hashCode = prime * hashCode + ((getWorkgroupId() == null) ? 0 : getWorkgroupId().hashCode());
hashCode = prime * hashCode + ((getWorkgroupName() == null) ? 0 : getWorkgroupName().hashCode());
hashCode = prime * hashCode + ((getWorkgroupVersion() == null) ? 0 : getWorkgroupVersion().hashCode());
return hashCode;
}
@Override
public Workgroup clone() {
try {
return (Workgroup) super.clone();
} catch (CloneNotSupportedException e) {
throw new IllegalStateException("Got a CloneNotSupportedException from Object.clone() " + "even though we're Cloneable!", e);
}
}
@com.amazonaws.annotation.SdkInternalApi
@Override
public void marshall(ProtocolMarshaller protocolMarshaller) {
com.amazonaws.services.redshiftserverless.model.transform.WorkgroupMarshaller.getInstance().marshall(this, protocolMarshaller);
}
}