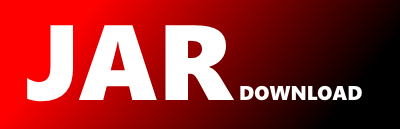
com.amazonaws.services.redshiftserverless.AWSRedshiftServerless Maven / Gradle / Ivy
Show all versions of aws-java-sdk-redshiftserverless Show documentation
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.redshiftserverless;
import javax.annotation.Generated;
import com.amazonaws.*;
import com.amazonaws.regions.*;
import com.amazonaws.services.redshiftserverless.model.*;
/**
* Interface for accessing Redshift Serverless.
*
* Note: Do not directly implement this interface, new methods are added to it regularly. Extend from
* {@link com.amazonaws.services.redshiftserverless.AbstractAWSRedshiftServerless} instead.
*
*
*
* This is an interface reference for Amazon Redshift Serverless. It contains documentation for one of the programming
* or command line interfaces you can use to manage Amazon Redshift Serverless.
*
*
* Amazon Redshift Serverless automatically provisions data warehouse capacity and intelligently scales the underlying
* resources based on workload demands. Amazon Redshift Serverless adjusts capacity in seconds to deliver consistently
* high performance and simplified operations for even the most demanding and volatile workloads. Amazon Redshift
* Serverless lets you focus on using your data to acquire new insights for your business and customers.
*
*
* To learn more about Amazon Redshift Serverless, see What is Amazon Redshift
* Serverless.
*
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public interface AWSRedshiftServerless {
/**
* The region metadata service name for computing region endpoints. You can use this value to retrieve metadata
* (such as supported regions) of the service.
*
* @see RegionUtils#getRegionsForService(String)
*/
String ENDPOINT_PREFIX = "redshift-serverless";
/**
*
* Converts a recovery point to a snapshot. For more information about recovery points and snapshots, see Working with snapshots
* and recovery points.
*
*
* @param convertRecoveryPointToSnapshotRequest
* @return Result of the ConvertRecoveryPointToSnapshot operation returned by the service.
* @throws InternalServerException
* The request processing has failed because of an unknown error, exception or failure.
* @throws ResourceNotFoundException
* The resource could not be found.
* @throws ConflictException
* The submitted action has conflicts.
* @throws ValidationException
* The input failed to satisfy the constraints specified by an AWS service.
* @throws TooManyTagsException
* The request exceeded the number of tags allowed for a resource.
* @throws ServiceQuotaExceededException
* The service limit was exceeded.
* @sample AWSRedshiftServerless.ConvertRecoveryPointToSnapshot
* @see AWS API Documentation
*/
ConvertRecoveryPointToSnapshotResult convertRecoveryPointToSnapshot(ConvertRecoveryPointToSnapshotRequest convertRecoveryPointToSnapshotRequest);
/**
*
* Creates a custom domain association for Amazon Redshift Serverless.
*
*
* @param createCustomDomainAssociationRequest
* @return Result of the CreateCustomDomainAssociation operation returned by the service.
* @throws InternalServerException
* The request processing has failed because of an unknown error, exception or failure.
* @throws ResourceNotFoundException
* The resource could not be found.
* @throws ConflictException
* The submitted action has conflicts.
* @throws ValidationException
* The input failed to satisfy the constraints specified by an AWS service.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @sample AWSRedshiftServerless.CreateCustomDomainAssociation
* @see AWS API Documentation
*/
CreateCustomDomainAssociationResult createCustomDomainAssociation(CreateCustomDomainAssociationRequest createCustomDomainAssociationRequest);
/**
*
* Creates an Amazon Redshift Serverless managed VPC endpoint.
*
*
* @param createEndpointAccessRequest
* @return Result of the CreateEndpointAccess operation returned by the service.
* @throws InternalServerException
* The request processing has failed because of an unknown error, exception or failure.
* @throws ResourceNotFoundException
* The resource could not be found.
* @throws ConflictException
* The submitted action has conflicts.
* @throws ValidationException
* The input failed to satisfy the constraints specified by an AWS service.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ServiceQuotaExceededException
* The service limit was exceeded.
* @sample AWSRedshiftServerless.CreateEndpointAccess
* @see AWS API Documentation
*/
CreateEndpointAccessResult createEndpointAccess(CreateEndpointAccessRequest createEndpointAccessRequest);
/**
*
* Creates a namespace in Amazon Redshift Serverless.
*
*
* @param createNamespaceRequest
* @return Result of the CreateNamespace operation returned by the service.
* @throws InternalServerException
* The request processing has failed because of an unknown error, exception or failure.
* @throws ConflictException
* The submitted action has conflicts.
* @throws ValidationException
* The input failed to satisfy the constraints specified by an AWS service.
* @throws TooManyTagsException
* The request exceeded the number of tags allowed for a resource.
* @sample AWSRedshiftServerless.CreateNamespace
* @see AWS API Documentation
*/
CreateNamespaceResult createNamespace(CreateNamespaceRequest createNamespaceRequest);
/**
*
* Creates a scheduled action. A scheduled action contains a schedule and an Amazon Redshift API action. For
* example, you can create a schedule of when to run the CreateSnapshot
API operation.
*
*
* @param createScheduledActionRequest
* @return Result of the CreateScheduledAction operation returned by the service.
* @throws InternalServerException
* The request processing has failed because of an unknown error, exception or failure.
* @throws ResourceNotFoundException
* The resource could not be found.
* @throws ConflictException
* The submitted action has conflicts.
* @throws ValidationException
* The input failed to satisfy the constraints specified by an AWS service.
* @sample AWSRedshiftServerless.CreateScheduledAction
* @see AWS API Documentation
*/
CreateScheduledActionResult createScheduledAction(CreateScheduledActionRequest createScheduledActionRequest);
/**
*
* Creates a snapshot of all databases in a namespace. For more information about snapshots, see Working with
* snapshots and recovery points.
*
*
* @param createSnapshotRequest
* @return Result of the CreateSnapshot operation returned by the service.
* @throws InternalServerException
* The request processing has failed because of an unknown error, exception or failure.
* @throws ResourceNotFoundException
* The resource could not be found.
* @throws ConflictException
* The submitted action has conflicts.
* @throws ValidationException
* The input failed to satisfy the constraints specified by an AWS service.
* @throws TooManyTagsException
* The request exceeded the number of tags allowed for a resource.
* @throws ServiceQuotaExceededException
* The service limit was exceeded.
* @sample AWSRedshiftServerless.CreateSnapshot
* @see AWS API Documentation
*/
CreateSnapshotResult createSnapshot(CreateSnapshotRequest createSnapshotRequest);
/**
*
* Creates a snapshot copy configuration that lets you copy snapshots to another Amazon Web Services Region.
*
*
* @param createSnapshotCopyConfigurationRequest
* @return Result of the CreateSnapshotCopyConfiguration operation returned by the service.
* @throws InternalServerException
* The request processing has failed because of an unknown error, exception or failure.
* @throws ResourceNotFoundException
* The resource could not be found.
* @throws ConflictException
* The submitted action has conflicts.
* @throws ValidationException
* The input failed to satisfy the constraints specified by an AWS service.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ServiceQuotaExceededException
* The service limit was exceeded.
* @sample AWSRedshiftServerless.CreateSnapshotCopyConfiguration
* @see AWS API Documentation
*/
CreateSnapshotCopyConfigurationResult createSnapshotCopyConfiguration(CreateSnapshotCopyConfigurationRequest createSnapshotCopyConfigurationRequest);
/**
*
* Creates a usage limit for a specified Amazon Redshift Serverless usage type. The usage limit is identified by the
* returned usage limit identifier.
*
*
* @param createUsageLimitRequest
* @return Result of the CreateUsageLimit operation returned by the service.
* @throws InternalServerException
* The request processing has failed because of an unknown error, exception or failure.
* @throws ResourceNotFoundException
* The resource could not be found.
* @throws ConflictException
* The submitted action has conflicts.
* @throws ValidationException
* The input failed to satisfy the constraints specified by an AWS service.
* @throws ServiceQuotaExceededException
* The service limit was exceeded.
* @sample AWSRedshiftServerless.CreateUsageLimit
* @see AWS API Documentation
*/
CreateUsageLimitResult createUsageLimit(CreateUsageLimitRequest createUsageLimitRequest);
/**
*
* Creates an workgroup in Amazon Redshift Serverless.
*
*
* @param createWorkgroupRequest
* @return Result of the CreateWorkgroup operation returned by the service.
* @throws InternalServerException
* The request processing has failed because of an unknown error, exception or failure.
* @throws InsufficientCapacityException
* There is an insufficient capacity to perform the action.
* @throws ResourceNotFoundException
* The resource could not be found.
* @throws ConflictException
* The submitted action has conflicts.
* @throws ValidationException
* The input failed to satisfy the constraints specified by an AWS service.
* @throws TooManyTagsException
* The request exceeded the number of tags allowed for a resource.
* @throws Ipv6CidrBlockNotFoundException
* There are no subnets in your VPC with associated IPv6 CIDR blocks. To use dual-stack mode, associate an
* IPv6 CIDR block with each subnet in your VPC.
* @sample AWSRedshiftServerless.CreateWorkgroup
* @see AWS API Documentation
*/
CreateWorkgroupResult createWorkgroup(CreateWorkgroupRequest createWorkgroupRequest);
/**
*
* Deletes a custom domain association for Amazon Redshift Serverless.
*
*
* @param deleteCustomDomainAssociationRequest
* @return Result of the DeleteCustomDomainAssociation operation returned by the service.
* @throws InternalServerException
* The request processing has failed because of an unknown error, exception or failure.
* @throws ResourceNotFoundException
* The resource could not be found.
* @throws ConflictException
* The submitted action has conflicts.
* @throws ValidationException
* The input failed to satisfy the constraints specified by an AWS service.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @sample AWSRedshiftServerless.DeleteCustomDomainAssociation
* @see AWS API Documentation
*/
DeleteCustomDomainAssociationResult deleteCustomDomainAssociation(DeleteCustomDomainAssociationRequest deleteCustomDomainAssociationRequest);
/**
*
* Deletes an Amazon Redshift Serverless managed VPC endpoint.
*
*
* @param deleteEndpointAccessRequest
* @return Result of the DeleteEndpointAccess operation returned by the service.
* @throws InternalServerException
* The request processing has failed because of an unknown error, exception or failure.
* @throws ResourceNotFoundException
* The resource could not be found.
* @throws ConflictException
* The submitted action has conflicts.
* @throws ValidationException
* The input failed to satisfy the constraints specified by an AWS service.
* @sample AWSRedshiftServerless.DeleteEndpointAccess
* @see AWS API Documentation
*/
DeleteEndpointAccessResult deleteEndpointAccess(DeleteEndpointAccessRequest deleteEndpointAccessRequest);
/**
*
* Deletes a namespace from Amazon Redshift Serverless. Before you delete the namespace, you can create a final
* snapshot that has all of the data within the namespace.
*
*
* @param deleteNamespaceRequest
* @return Result of the DeleteNamespace operation returned by the service.
* @throws InternalServerException
* The request processing has failed because of an unknown error, exception or failure.
* @throws ResourceNotFoundException
* The resource could not be found.
* @throws ConflictException
* The submitted action has conflicts.
* @throws ValidationException
* The input failed to satisfy the constraints specified by an AWS service.
* @sample AWSRedshiftServerless.DeleteNamespace
* @see AWS API Documentation
*/
DeleteNamespaceResult deleteNamespace(DeleteNamespaceRequest deleteNamespaceRequest);
/**
*
* Deletes the specified resource policy.
*
*
* @param deleteResourcePolicyRequest
* @return Result of the DeleteResourcePolicy operation returned by the service.
* @throws InternalServerException
* The request processing has failed because of an unknown error, exception or failure.
* @throws ResourceNotFoundException
* The resource could not be found.
* @throws ValidationException
* The input failed to satisfy the constraints specified by an AWS service.
* @sample AWSRedshiftServerless.DeleteResourcePolicy
* @see AWS API Documentation
*/
DeleteResourcePolicyResult deleteResourcePolicy(DeleteResourcePolicyRequest deleteResourcePolicyRequest);
/**
*
* Deletes a scheduled action.
*
*
* @param deleteScheduledActionRequest
* @return Result of the DeleteScheduledAction operation returned by the service.
* @throws InternalServerException
* The request processing has failed because of an unknown error, exception or failure.
* @throws ResourceNotFoundException
* The resource could not be found.
* @throws ValidationException
* The input failed to satisfy the constraints specified by an AWS service.
* @sample AWSRedshiftServerless.DeleteScheduledAction
* @see AWS API Documentation
*/
DeleteScheduledActionResult deleteScheduledAction(DeleteScheduledActionRequest deleteScheduledActionRequest);
/**
*
* Deletes a snapshot from Amazon Redshift Serverless.
*
*
* @param deleteSnapshotRequest
* @return Result of the DeleteSnapshot operation returned by the service.
* @throws InternalServerException
* The request processing has failed because of an unknown error, exception or failure.
* @throws ResourceNotFoundException
* The resource could not be found.
* @throws ConflictException
* The submitted action has conflicts.
* @throws ValidationException
* The input failed to satisfy the constraints specified by an AWS service.
* @sample AWSRedshiftServerless.DeleteSnapshot
* @see AWS API Documentation
*/
DeleteSnapshotResult deleteSnapshot(DeleteSnapshotRequest deleteSnapshotRequest);
/**
*
* Deletes a snapshot copy configuration
*
*
* @param deleteSnapshotCopyConfigurationRequest
* @return Result of the DeleteSnapshotCopyConfiguration operation returned by the service.
* @throws InternalServerException
* The request processing has failed because of an unknown error, exception or failure.
* @throws ResourceNotFoundException
* The resource could not be found.
* @throws ConflictException
* The submitted action has conflicts.
* @throws ValidationException
* The input failed to satisfy the constraints specified by an AWS service.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @sample AWSRedshiftServerless.DeleteSnapshotCopyConfiguration
* @see AWS API Documentation
*/
DeleteSnapshotCopyConfigurationResult deleteSnapshotCopyConfiguration(DeleteSnapshotCopyConfigurationRequest deleteSnapshotCopyConfigurationRequest);
/**
*
* Deletes a usage limit from Amazon Redshift Serverless.
*
*
* @param deleteUsageLimitRequest
* @return Result of the DeleteUsageLimit operation returned by the service.
* @throws InternalServerException
* The request processing has failed because of an unknown error, exception or failure.
* @throws ResourceNotFoundException
* The resource could not be found.
* @throws ConflictException
* The submitted action has conflicts.
* @throws ValidationException
* The input failed to satisfy the constraints specified by an AWS service.
* @sample AWSRedshiftServerless.DeleteUsageLimit
* @see AWS API Documentation
*/
DeleteUsageLimitResult deleteUsageLimit(DeleteUsageLimitRequest deleteUsageLimitRequest);
/**
*
* Deletes a workgroup.
*
*
* @param deleteWorkgroupRequest
* @return Result of the DeleteWorkgroup operation returned by the service.
* @throws InternalServerException
* The request processing has failed because of an unknown error, exception or failure.
* @throws ResourceNotFoundException
* The resource could not be found.
* @throws ConflictException
* The submitted action has conflicts.
* @throws ValidationException
* The input failed to satisfy the constraints specified by an AWS service.
* @sample AWSRedshiftServerless.DeleteWorkgroup
* @see AWS API Documentation
*/
DeleteWorkgroupResult deleteWorkgroup(DeleteWorkgroupRequest deleteWorkgroupRequest);
/**
*
* Returns a database user name and temporary password with temporary authorization to log in to Amazon Redshift
* Serverless.
*
*
* By default, the temporary credentials expire in 900 seconds. You can optionally specify a duration between 900
* seconds (15 minutes) and 3600 seconds (60 minutes).
*
*
*
* <p>The Identity and Access Management (IAM) user or role that runs GetCredentials must have an IAM policy attached that allows access to all necessary actions and resources.</p> <p>If the <code>DbName</code> parameter is specified, the IAM policy must allow access to the resource dbname for the specified database name.</p>
*
*
* @param getCredentialsRequest
* @return Result of the GetCredentials operation returned by the service.
* @throws InternalServerException
* The request processing has failed because of an unknown error, exception or failure.
* @throws ResourceNotFoundException
* The resource could not be found.
* @throws ValidationException
* The input failed to satisfy the constraints specified by an AWS service.
* @sample AWSRedshiftServerless.GetCredentials
* @see AWS API Documentation
*/
GetCredentialsResult getCredentials(GetCredentialsRequest getCredentialsRequest);
/**
*
* Gets information about a specific custom domain association.
*
*
* @param getCustomDomainAssociationRequest
* @return Result of the GetCustomDomainAssociation operation returned by the service.
* @throws InternalServerException
* The request processing has failed because of an unknown error, exception or failure.
* @throws ResourceNotFoundException
* The resource could not be found.
* @throws ConflictException
* The submitted action has conflicts.
* @throws ValidationException
* The input failed to satisfy the constraints specified by an AWS service.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @sample AWSRedshiftServerless.GetCustomDomainAssociation
* @see AWS API Documentation
*/
GetCustomDomainAssociationResult getCustomDomainAssociation(GetCustomDomainAssociationRequest getCustomDomainAssociationRequest);
/**
*
* Returns information, such as the name, about a VPC endpoint.
*
*
* @param getEndpointAccessRequest
* @return Result of the GetEndpointAccess operation returned by the service.
* @throws InternalServerException
* The request processing has failed because of an unknown error, exception or failure.
* @throws ResourceNotFoundException
* The resource could not be found.
* @throws ConflictException
* The submitted action has conflicts.
* @throws ValidationException
* The input failed to satisfy the constraints specified by an AWS service.
* @sample AWSRedshiftServerless.GetEndpointAccess
* @see AWS API Documentation
*/
GetEndpointAccessResult getEndpointAccess(GetEndpointAccessRequest getEndpointAccessRequest);
/**
*
* Returns information about a namespace in Amazon Redshift Serverless.
*
*
* @param getNamespaceRequest
* @return Result of the GetNamespace operation returned by the service.
* @throws InternalServerException
* The request processing has failed because of an unknown error, exception or failure.
* @throws ResourceNotFoundException
* The resource could not be found.
* @throws ValidationException
* The input failed to satisfy the constraints specified by an AWS service.
* @sample AWSRedshiftServerless.GetNamespace
* @see AWS API Documentation
*/
GetNamespaceResult getNamespace(GetNamespaceRequest getNamespaceRequest);
/**
*
* Returns information about a recovery point.
*
*
* @param getRecoveryPointRequest
* @return Result of the GetRecoveryPoint operation returned by the service.
* @throws InternalServerException
* The request processing has failed because of an unknown error, exception or failure.
* @throws ResourceNotFoundException
* The resource could not be found.
* @throws ConflictException
* The submitted action has conflicts.
* @throws ValidationException
* The input failed to satisfy the constraints specified by an AWS service.
* @sample AWSRedshiftServerless.GetRecoveryPoint
* @see AWS API Documentation
*/
GetRecoveryPointResult getRecoveryPoint(GetRecoveryPointRequest getRecoveryPointRequest);
/**
*
* Returns a resource policy.
*
*
* @param getResourcePolicyRequest
* @return Result of the GetResourcePolicy operation returned by the service.
* @throws InternalServerException
* The request processing has failed because of an unknown error, exception or failure.
* @throws ResourceNotFoundException
* The resource could not be found.
* @throws ValidationException
* The input failed to satisfy the constraints specified by an AWS service.
* @sample AWSRedshiftServerless.GetResourcePolicy
* @see AWS API Documentation
*/
GetResourcePolicyResult getResourcePolicy(GetResourcePolicyRequest getResourcePolicyRequest);
/**
*
* Returns information about a scheduled action.
*
*
* @param getScheduledActionRequest
* @return Result of the GetScheduledAction operation returned by the service.
* @throws InternalServerException
* The request processing has failed because of an unknown error, exception or failure.
* @throws ResourceNotFoundException
* The resource could not be found.
* @throws ValidationException
* The input failed to satisfy the constraints specified by an AWS service.
* @sample AWSRedshiftServerless.GetScheduledAction
* @see AWS API Documentation
*/
GetScheduledActionResult getScheduledAction(GetScheduledActionRequest getScheduledActionRequest);
/**
*
* Returns information about a specific snapshot.
*
*
* @param getSnapshotRequest
* @return Result of the GetSnapshot operation returned by the service.
* @throws InternalServerException
* The request processing has failed because of an unknown error, exception or failure.
* @throws ResourceNotFoundException
* The resource could not be found.
* @throws ValidationException
* The input failed to satisfy the constraints specified by an AWS service.
* @sample AWSRedshiftServerless.GetSnapshot
* @see AWS API Documentation
*/
GetSnapshotResult getSnapshot(GetSnapshotRequest getSnapshotRequest);
/**
*
* Returns information about a TableRestoreStatus
object.
*
*
* @param getTableRestoreStatusRequest
* @return Result of the GetTableRestoreStatus operation returned by the service.
* @throws ResourceNotFoundException
* The resource could not be found.
* @throws ValidationException
* The input failed to satisfy the constraints specified by an AWS service.
* @sample AWSRedshiftServerless.GetTableRestoreStatus
* @see AWS API Documentation
*/
GetTableRestoreStatusResult getTableRestoreStatus(GetTableRestoreStatusRequest getTableRestoreStatusRequest);
/**
*
* Returns information about a usage limit.
*
*
* @param getUsageLimitRequest
* @return Result of the GetUsageLimit operation returned by the service.
* @throws InternalServerException
* The request processing has failed because of an unknown error, exception or failure.
* @throws ResourceNotFoundException
* The resource could not be found.
* @throws ConflictException
* The submitted action has conflicts.
* @throws ValidationException
* The input failed to satisfy the constraints specified by an AWS service.
* @sample AWSRedshiftServerless.GetUsageLimit
* @see AWS API Documentation
*/
GetUsageLimitResult getUsageLimit(GetUsageLimitRequest getUsageLimitRequest);
/**
*
* Returns information about a specific workgroup.
*
*
* @param getWorkgroupRequest
* @return Result of the GetWorkgroup operation returned by the service.
* @throws InternalServerException
* The request processing has failed because of an unknown error, exception or failure.
* @throws ResourceNotFoundException
* The resource could not be found.
* @throws ValidationException
* The input failed to satisfy the constraints specified by an AWS service.
* @sample AWSRedshiftServerless.GetWorkgroup
* @see AWS API Documentation
*/
GetWorkgroupResult getWorkgroup(GetWorkgroupRequest getWorkgroupRequest);
/**
*
* Lists custom domain associations for Amazon Redshift Serverless.
*
*
* @param listCustomDomainAssociationsRequest
* @return Result of the ListCustomDomainAssociations operation returned by the service.
* @throws InternalServerException
* The request processing has failed because of an unknown error, exception or failure.
* @throws InvalidPaginationException
* The provided pagination token is invalid.
* @throws ValidationException
* The input failed to satisfy the constraints specified by an AWS service.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @sample AWSRedshiftServerless.ListCustomDomainAssociations
* @see AWS API Documentation
*/
ListCustomDomainAssociationsResult listCustomDomainAssociations(ListCustomDomainAssociationsRequest listCustomDomainAssociationsRequest);
/**
*
* Returns an array of EndpointAccess
objects and relevant information.
*
*
* @param listEndpointAccessRequest
* @return Result of the ListEndpointAccess operation returned by the service.
* @throws InternalServerException
* The request processing has failed because of an unknown error, exception or failure.
* @throws ResourceNotFoundException
* The resource could not be found.
* @throws ConflictException
* The submitted action has conflicts.
* @throws ValidationException
* The input failed to satisfy the constraints specified by an AWS service.
* @sample AWSRedshiftServerless.ListEndpointAccess
* @see AWS API Documentation
*/
ListEndpointAccessResult listEndpointAccess(ListEndpointAccessRequest listEndpointAccessRequest);
/**
*
* Returns information about a list of specified namespaces.
*
*
* @param listNamespacesRequest
* @return Result of the ListNamespaces operation returned by the service.
* @throws InternalServerException
* The request processing has failed because of an unknown error, exception or failure.
* @throws ValidationException
* The input failed to satisfy the constraints specified by an AWS service.
* @sample AWSRedshiftServerless.ListNamespaces
* @see AWS API Documentation
*/
ListNamespacesResult listNamespaces(ListNamespacesRequest listNamespacesRequest);
/**
*
* Returns an array of recovery points.
*
*
* @param listRecoveryPointsRequest
* @return Result of the ListRecoveryPoints operation returned by the service.
* @throws InternalServerException
* The request processing has failed because of an unknown error, exception or failure.
* @throws ValidationException
* The input failed to satisfy the constraints specified by an AWS service.
* @sample AWSRedshiftServerless.ListRecoveryPoints
* @see AWS API Documentation
*/
ListRecoveryPointsResult listRecoveryPoints(ListRecoveryPointsRequest listRecoveryPointsRequest);
/**
*
* Returns a list of scheduled actions. You can use the flags to filter the list of returned scheduled actions.
*
*
* @param listScheduledActionsRequest
* @return Result of the ListScheduledActions operation returned by the service.
* @throws InternalServerException
* The request processing has failed because of an unknown error, exception or failure.
* @throws InvalidPaginationException
* The provided pagination token is invalid.
* @throws ResourceNotFoundException
* The resource could not be found.
* @throws ValidationException
* The input failed to satisfy the constraints specified by an AWS service.
* @sample AWSRedshiftServerless.ListScheduledActions
* @see AWS API Documentation
*/
ListScheduledActionsResult listScheduledActions(ListScheduledActionsRequest listScheduledActionsRequest);
/**
*
* Returns a list of snapshot copy configurations.
*
*
* @param listSnapshotCopyConfigurationsRequest
* @return Result of the ListSnapshotCopyConfigurations operation returned by the service.
* @throws InternalServerException
* The request processing has failed because of an unknown error, exception or failure.
* @throws InvalidPaginationException
* The provided pagination token is invalid.
* @throws ResourceNotFoundException
* The resource could not be found.
* @throws ConflictException
* The submitted action has conflicts.
* @throws ValidationException
* The input failed to satisfy the constraints specified by an AWS service.
* @sample AWSRedshiftServerless.ListSnapshotCopyConfigurations
* @see AWS API Documentation
*/
ListSnapshotCopyConfigurationsResult listSnapshotCopyConfigurations(ListSnapshotCopyConfigurationsRequest listSnapshotCopyConfigurationsRequest);
/**
*
* Returns a list of snapshots.
*
*
* @param listSnapshotsRequest
* @return Result of the ListSnapshots operation returned by the service.
* @throws InternalServerException
* The request processing has failed because of an unknown error, exception or failure.
* @throws ResourceNotFoundException
* The resource could not be found.
* @throws ValidationException
* The input failed to satisfy the constraints specified by an AWS service.
* @sample AWSRedshiftServerless.ListSnapshots
* @see AWS API Documentation
*/
ListSnapshotsResult listSnapshots(ListSnapshotsRequest listSnapshotsRequest);
/**
*
* Returns information about an array of TableRestoreStatus
objects.
*
*
* @param listTableRestoreStatusRequest
* @return Result of the ListTableRestoreStatus operation returned by the service.
* @throws InvalidPaginationException
* The provided pagination token is invalid.
* @throws ResourceNotFoundException
* The resource could not be found.
* @throws ValidationException
* The input failed to satisfy the constraints specified by an AWS service.
* @sample AWSRedshiftServerless.ListTableRestoreStatus
* @see AWS API Documentation
*/
ListTableRestoreStatusResult listTableRestoreStatus(ListTableRestoreStatusRequest listTableRestoreStatusRequest);
/**
*
* Lists the tags assigned to a resource.
*
*
* @param listTagsForResourceRequest
* @return Result of the ListTagsForResource operation returned by the service.
* @throws InternalServerException
* The request processing has failed because of an unknown error, exception or failure.
* @throws ResourceNotFoundException
* The resource could not be found.
* @throws ValidationException
* The input failed to satisfy the constraints specified by an AWS service.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @sample AWSRedshiftServerless.ListTagsForResource
* @see AWS API Documentation
*/
ListTagsForResourceResult listTagsForResource(ListTagsForResourceRequest listTagsForResourceRequest);
/**
*
* Lists all usage limits within Amazon Redshift Serverless.
*
*
* @param listUsageLimitsRequest
* @return Result of the ListUsageLimits operation returned by the service.
* @throws InternalServerException
* The request processing has failed because of an unknown error, exception or failure.
* @throws InvalidPaginationException
* The provided pagination token is invalid.
* @throws ResourceNotFoundException
* The resource could not be found.
* @throws ConflictException
* The submitted action has conflicts.
* @throws ValidationException
* The input failed to satisfy the constraints specified by an AWS service.
* @sample AWSRedshiftServerless.ListUsageLimits
* @see AWS API Documentation
*/
ListUsageLimitsResult listUsageLimits(ListUsageLimitsRequest listUsageLimitsRequest);
/**
*
* Returns information about a list of specified workgroups.
*
*
* @param listWorkgroupsRequest
* @return Result of the ListWorkgroups operation returned by the service.
* @throws InternalServerException
* The request processing has failed because of an unknown error, exception or failure.
* @throws ValidationException
* The input failed to satisfy the constraints specified by an AWS service.
* @sample AWSRedshiftServerless.ListWorkgroups
* @see AWS API Documentation
*/
ListWorkgroupsResult listWorkgroups(ListWorkgroupsRequest listWorkgroupsRequest);
/**
*
* Creates or updates a resource policy. Currently, you can use policies to share snapshots across Amazon Web
* Services accounts.
*
*
* @param putResourcePolicyRequest
* @return Result of the PutResourcePolicy operation returned by the service.
* @throws InternalServerException
* The request processing has failed because of an unknown error, exception or failure.
* @throws ResourceNotFoundException
* The resource could not be found.
* @throws ConflictException
* The submitted action has conflicts.
* @throws ValidationException
* The input failed to satisfy the constraints specified by an AWS service.
* @throws ServiceQuotaExceededException
* The service limit was exceeded.
* @sample AWSRedshiftServerless.PutResourcePolicy
* @see AWS API Documentation
*/
PutResourcePolicyResult putResourcePolicy(PutResourcePolicyRequest putResourcePolicyRequest);
/**
*
* Restore the data from a recovery point.
*
*
* @param restoreFromRecoveryPointRequest
* @return Result of the RestoreFromRecoveryPoint operation returned by the service.
* @throws InternalServerException
* The request processing has failed because of an unknown error, exception or failure.
* @throws ResourceNotFoundException
* The resource could not be found.
* @throws ConflictException
* The submitted action has conflicts.
* @throws ValidationException
* The input failed to satisfy the constraints specified by an AWS service.
* @sample AWSRedshiftServerless.RestoreFromRecoveryPoint
* @see AWS API Documentation
*/
RestoreFromRecoveryPointResult restoreFromRecoveryPoint(RestoreFromRecoveryPointRequest restoreFromRecoveryPointRequest);
/**
*
* Restores a namespace from a snapshot.
*
*
* @param restoreFromSnapshotRequest
* @return Result of the RestoreFromSnapshot operation returned by the service.
* @throws InternalServerException
* The request processing has failed because of an unknown error, exception or failure.
* @throws ResourceNotFoundException
* The resource could not be found.
* @throws ConflictException
* The submitted action has conflicts.
* @throws ValidationException
* The input failed to satisfy the constraints specified by an AWS service.
* @throws ServiceQuotaExceededException
* The service limit was exceeded.
* @sample AWSRedshiftServerless.RestoreFromSnapshot
* @see AWS API Documentation
*/
RestoreFromSnapshotResult restoreFromSnapshot(RestoreFromSnapshotRequest restoreFromSnapshotRequest);
/**
*
* Restores a table from a recovery point to your Amazon Redshift Serverless instance. You can't use this operation
* to restore tables with interleaved sort keys.
*
*
* @param restoreTableFromRecoveryPointRequest
* @return Result of the RestoreTableFromRecoveryPoint operation returned by the service.
* @throws InternalServerException
* The request processing has failed because of an unknown error, exception or failure.
* @throws ResourceNotFoundException
* The resource could not be found.
* @throws ConflictException
* The submitted action has conflicts.
* @throws ValidationException
* The input failed to satisfy the constraints specified by an AWS service.
* @sample AWSRedshiftServerless.RestoreTableFromRecoveryPoint
* @see AWS API Documentation
*/
RestoreTableFromRecoveryPointResult restoreTableFromRecoveryPoint(RestoreTableFromRecoveryPointRequest restoreTableFromRecoveryPointRequest);
/**
*
* Restores a table from a snapshot to your Amazon Redshift Serverless instance. You can't use this operation to
* restore tables with interleaved
* sort keys.
*
*
* @param restoreTableFromSnapshotRequest
* @return Result of the RestoreTableFromSnapshot operation returned by the service.
* @throws InternalServerException
* The request processing has failed because of an unknown error, exception or failure.
* @throws ResourceNotFoundException
* The resource could not be found.
* @throws ConflictException
* The submitted action has conflicts.
* @throws ValidationException
* The input failed to satisfy the constraints specified by an AWS service.
* @sample AWSRedshiftServerless.RestoreTableFromSnapshot
* @see AWS API Documentation
*/
RestoreTableFromSnapshotResult restoreTableFromSnapshot(RestoreTableFromSnapshotRequest restoreTableFromSnapshotRequest);
/**
*
* Assigns one or more tags to a resource.
*
*
* @param tagResourceRequest
* @return Result of the TagResource operation returned by the service.
* @throws InternalServerException
* The request processing has failed because of an unknown error, exception or failure.
* @throws ResourceNotFoundException
* The resource could not be found.
* @throws ValidationException
* The input failed to satisfy the constraints specified by an AWS service.
* @throws TooManyTagsException
* The request exceeded the number of tags allowed for a resource.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @sample AWSRedshiftServerless.TagResource
* @see AWS API Documentation
*/
TagResourceResult tagResource(TagResourceRequest tagResourceRequest);
/**
*
* Removes a tag or set of tags from a resource.
*
*
* @param untagResourceRequest
* @return Result of the UntagResource operation returned by the service.
* @throws InternalServerException
* The request processing has failed because of an unknown error, exception or failure.
* @throws ResourceNotFoundException
* The resource could not be found.
* @throws ValidationException
* The input failed to satisfy the constraints specified by an AWS service.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @sample AWSRedshiftServerless.UntagResource
* @see AWS API Documentation
*/
UntagResourceResult untagResource(UntagResourceRequest untagResourceRequest);
/**
*
* Updates an Amazon Redshift Serverless certificate associated with a custom domain.
*
*
* @param updateCustomDomainAssociationRequest
* @return Result of the UpdateCustomDomainAssociation operation returned by the service.
* @throws InternalServerException
* The request processing has failed because of an unknown error, exception or failure.
* @throws ResourceNotFoundException
* The resource could not be found.
* @throws ConflictException
* The submitted action has conflicts.
* @throws ValidationException
* The input failed to satisfy the constraints specified by an AWS service.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @sample AWSRedshiftServerless.UpdateCustomDomainAssociation
* @see AWS API Documentation
*/
UpdateCustomDomainAssociationResult updateCustomDomainAssociation(UpdateCustomDomainAssociationRequest updateCustomDomainAssociationRequest);
/**
*
* Updates an Amazon Redshift Serverless managed endpoint.
*
*
* @param updateEndpointAccessRequest
* @return Result of the UpdateEndpointAccess operation returned by the service.
* @throws InternalServerException
* The request processing has failed because of an unknown error, exception or failure.
* @throws ResourceNotFoundException
* The resource could not be found.
* @throws ConflictException
* The submitted action has conflicts.
* @throws ValidationException
* The input failed to satisfy the constraints specified by an AWS service.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @sample AWSRedshiftServerless.UpdateEndpointAccess
* @see AWS API Documentation
*/
UpdateEndpointAccessResult updateEndpointAccess(UpdateEndpointAccessRequest updateEndpointAccessRequest);
/**
*
* Updates a namespace with the specified settings. Unless required, you can't update multiple parameters in one
* request. For example, you must specify both adminUsername
and adminUserPassword
to
* update either field, but you can't update both kmsKeyId
and logExports
in a single
* request.
*
*
* @param updateNamespaceRequest
* @return Result of the UpdateNamespace operation returned by the service.
* @throws InternalServerException
* The request processing has failed because of an unknown error, exception or failure.
* @throws ResourceNotFoundException
* The resource could not be found.
* @throws ConflictException
* The submitted action has conflicts.
* @throws ValidationException
* The input failed to satisfy the constraints specified by an AWS service.
* @sample AWSRedshiftServerless.UpdateNamespace
* @see AWS API Documentation
*/
UpdateNamespaceResult updateNamespace(UpdateNamespaceRequest updateNamespaceRequest);
/**
*
* Updates a scheduled action.
*
*
* @param updateScheduledActionRequest
* @return Result of the UpdateScheduledAction operation returned by the service.
* @throws InternalServerException
* The request processing has failed because of an unknown error, exception or failure.
* @throws ResourceNotFoundException
* The resource could not be found.
* @throws ConflictException
* The submitted action has conflicts.
* @throws ValidationException
* The input failed to satisfy the constraints specified by an AWS service.
* @sample AWSRedshiftServerless.UpdateScheduledAction
* @see AWS API Documentation
*/
UpdateScheduledActionResult updateScheduledAction(UpdateScheduledActionRequest updateScheduledActionRequest);
/**
*
* Updates a snapshot.
*
*
* @param updateSnapshotRequest
* @return Result of the UpdateSnapshot operation returned by the service.
* @throws InternalServerException
* The request processing has failed because of an unknown error, exception or failure.
* @throws ResourceNotFoundException
* The resource could not be found.
* @throws ConflictException
* The submitted action has conflicts.
* @throws ValidationException
* The input failed to satisfy the constraints specified by an AWS service.
* @sample AWSRedshiftServerless.UpdateSnapshot
* @see AWS API Documentation
*/
UpdateSnapshotResult updateSnapshot(UpdateSnapshotRequest updateSnapshotRequest);
/**
*
* Updates a snapshot copy configuration.
*
*
* @param updateSnapshotCopyConfigurationRequest
* @return Result of the UpdateSnapshotCopyConfiguration operation returned by the service.
* @throws InternalServerException
* The request processing has failed because of an unknown error, exception or failure.
* @throws ResourceNotFoundException
* The resource could not be found.
* @throws ConflictException
* The submitted action has conflicts.
* @throws ValidationException
* The input failed to satisfy the constraints specified by an AWS service.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @sample AWSRedshiftServerless.UpdateSnapshotCopyConfiguration
* @see AWS API Documentation
*/
UpdateSnapshotCopyConfigurationResult updateSnapshotCopyConfiguration(UpdateSnapshotCopyConfigurationRequest updateSnapshotCopyConfigurationRequest);
/**
*
* Update a usage limit in Amazon Redshift Serverless. You can't update the usage type or period of a usage limit.
*
*
* @param updateUsageLimitRequest
* @return Result of the UpdateUsageLimit operation returned by the service.
* @throws InternalServerException
* The request processing has failed because of an unknown error, exception or failure.
* @throws ResourceNotFoundException
* The resource could not be found.
* @throws ConflictException
* The submitted action has conflicts.
* @throws ValidationException
* The input failed to satisfy the constraints specified by an AWS service.
* @sample AWSRedshiftServerless.UpdateUsageLimit
* @see AWS API Documentation
*/
UpdateUsageLimitResult updateUsageLimit(UpdateUsageLimitRequest updateUsageLimitRequest);
/**
*
* Updates a workgroup with the specified configuration settings. You can't update multiple parameters in one
* request. For example, you can update baseCapacity
or port
in a single request, but you
* can't update both in the same request.
*
*
* @param updateWorkgroupRequest
* @return Result of the UpdateWorkgroup operation returned by the service.
* @throws InternalServerException
* The request processing has failed because of an unknown error, exception or failure.
* @throws InsufficientCapacityException
* There is an insufficient capacity to perform the action.
* @throws ResourceNotFoundException
* The resource could not be found.
* @throws ConflictException
* The submitted action has conflicts.
* @throws ValidationException
* The input failed to satisfy the constraints specified by an AWS service.
* @throws Ipv6CidrBlockNotFoundException
* There are no subnets in your VPC with associated IPv6 CIDR blocks. To use dual-stack mode, associate an
* IPv6 CIDR block with each subnet in your VPC.
* @sample AWSRedshiftServerless.UpdateWorkgroup
* @see AWS API Documentation
*/
UpdateWorkgroupResult updateWorkgroup(UpdateWorkgroupRequest updateWorkgroupRequest);
/**
* Shuts down this client object, releasing any resources that might be held open. This is an optional method, and
* callers are not expected to call it, but can if they want to explicitly release any open resources. Once a client
* has been shutdown, it should not be used to make any more requests.
*/
void shutdown();
/**
* Returns additional metadata for a previously executed successful request, typically used for debugging issues
* where a service isn't acting as expected. This data isn't considered part of the result data returned by an
* operation, so it's available through this separate, diagnostic interface.
*
* Response metadata is only cached for a limited period of time, so if you need to access this extra diagnostic
* information for an executed request, you should use this method to retrieve it as soon as possible after
* executing a request.
*
* @param request
* The originally executed request.
*
* @return The response metadata for the specified request, or null if none is available.
*/
ResponseMetadata getCachedResponseMetadata(AmazonWebServiceRequest request);
}