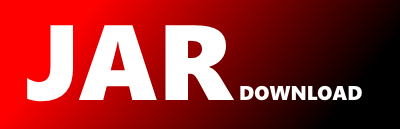
com.amazonaws.services.resiliencehub.model.ComplianceDrift Maven / Gradle / Ivy
Show all versions of aws-java-sdk-resiliencehub Show documentation
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.resiliencehub.model;
import java.io.Serializable;
import javax.annotation.Generated;
import com.amazonaws.protocol.StructuredPojo;
import com.amazonaws.protocol.ProtocolMarshaller;
/**
*
* Indicates the compliance drifts (recovery time objective (RTO) and recovery point objective (RPO)) that were detected
* for an assessed entity.
*
*
* @see AWS API
* Documentation
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class ComplianceDrift implements Serializable, Cloneable, StructuredPojo {
/**
*
* Assessment identifier that is associated with this drift item.
*
*/
private String actualReferenceId;
/**
*
* Actual compliance value of the entity.
*
*/
private java.util.Map actualValue;
/**
*
* Identifier of your application.
*
*/
private String appId;
/**
*
* Published version of your application on which drift was detected.
*
*/
private String appVersion;
/**
*
* Difference type between actual and expected recovery point objective (RPO) and recovery time objective (RTO)
* values. Currently, Resilience Hub supports only NotEqual difference type.
*
*/
private String diffType;
/**
*
* The type of drift detected. Currently, Resilience Hub supports only ApplicationCompliance drift type.
*
*/
private String driftType;
/**
*
* Identifier of an entity in which drift was detected. For compliance drift, the entity ID can be either
* application ID or the AppComponent ID.
*
*/
private String entityId;
/**
*
* The type of entity in which drift was detected. For compliance drifts, Resilience Hub supports
* AWS::ResilienceHub::AppComponent
and AWS::ResilienceHub::Application
.
*
*/
private String entityType;
/**
*
* Assessment identifier of a previous assessment of the same application version. Resilience Hub uses the previous
* assessment (associated with the reference identifier) to compare the compliance with the current assessment to
* identify drifts.
*
*/
private String expectedReferenceId;
/**
*
* The expected compliance value of an entity.
*
*/
private java.util.Map expectedValue;
/**
*
* Assessment identifier that is associated with this drift item.
*
*
* @param actualReferenceId
* Assessment identifier that is associated with this drift item.
*/
public void setActualReferenceId(String actualReferenceId) {
this.actualReferenceId = actualReferenceId;
}
/**
*
* Assessment identifier that is associated with this drift item.
*
*
* @return Assessment identifier that is associated with this drift item.
*/
public String getActualReferenceId() {
return this.actualReferenceId;
}
/**
*
* Assessment identifier that is associated with this drift item.
*
*
* @param actualReferenceId
* Assessment identifier that is associated with this drift item.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ComplianceDrift withActualReferenceId(String actualReferenceId) {
setActualReferenceId(actualReferenceId);
return this;
}
/**
*
* Actual compliance value of the entity.
*
*
* @return Actual compliance value of the entity.
*/
public java.util.Map getActualValue() {
return actualValue;
}
/**
*
* Actual compliance value of the entity.
*
*
* @param actualValue
* Actual compliance value of the entity.
*/
public void setActualValue(java.util.Map actualValue) {
this.actualValue = actualValue;
}
/**
*
* Actual compliance value of the entity.
*
*
* @param actualValue
* Actual compliance value of the entity.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ComplianceDrift withActualValue(java.util.Map actualValue) {
setActualValue(actualValue);
return this;
}
/**
* Add a single ActualValue entry
*
* @see ComplianceDrift#withActualValue
* @returns a reference to this object so that method calls can be chained together.
*/
public ComplianceDrift addActualValueEntry(String key, DisruptionCompliance value) {
if (null == this.actualValue) {
this.actualValue = new java.util.HashMap();
}
if (this.actualValue.containsKey(key))
throw new IllegalArgumentException("Duplicated keys (" + key.toString() + ") are provided.");
this.actualValue.put(key, value);
return this;
}
/**
* Removes all the entries added into ActualValue.
*
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ComplianceDrift clearActualValueEntries() {
this.actualValue = null;
return this;
}
/**
*
* Identifier of your application.
*
*
* @param appId
* Identifier of your application.
*/
public void setAppId(String appId) {
this.appId = appId;
}
/**
*
* Identifier of your application.
*
*
* @return Identifier of your application.
*/
public String getAppId() {
return this.appId;
}
/**
*
* Identifier of your application.
*
*
* @param appId
* Identifier of your application.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ComplianceDrift withAppId(String appId) {
setAppId(appId);
return this;
}
/**
*
* Published version of your application on which drift was detected.
*
*
* @param appVersion
* Published version of your application on which drift was detected.
*/
public void setAppVersion(String appVersion) {
this.appVersion = appVersion;
}
/**
*
* Published version of your application on which drift was detected.
*
*
* @return Published version of your application on which drift was detected.
*/
public String getAppVersion() {
return this.appVersion;
}
/**
*
* Published version of your application on which drift was detected.
*
*
* @param appVersion
* Published version of your application on which drift was detected.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ComplianceDrift withAppVersion(String appVersion) {
setAppVersion(appVersion);
return this;
}
/**
*
* Difference type between actual and expected recovery point objective (RPO) and recovery time objective (RTO)
* values. Currently, Resilience Hub supports only NotEqual difference type.
*
*
* @param diffType
* Difference type between actual and expected recovery point objective (RPO) and recovery time objective
* (RTO) values. Currently, Resilience Hub supports only NotEqual difference type.
* @see DifferenceType
*/
public void setDiffType(String diffType) {
this.diffType = diffType;
}
/**
*
* Difference type between actual and expected recovery point objective (RPO) and recovery time objective (RTO)
* values. Currently, Resilience Hub supports only NotEqual difference type.
*
*
* @return Difference type between actual and expected recovery point objective (RPO) and recovery time objective
* (RTO) values. Currently, Resilience Hub supports only NotEqual difference type.
* @see DifferenceType
*/
public String getDiffType() {
return this.diffType;
}
/**
*
* Difference type between actual and expected recovery point objective (RPO) and recovery time objective (RTO)
* values. Currently, Resilience Hub supports only NotEqual difference type.
*
*
* @param diffType
* Difference type between actual and expected recovery point objective (RPO) and recovery time objective
* (RTO) values. Currently, Resilience Hub supports only NotEqual difference type.
* @return Returns a reference to this object so that method calls can be chained together.
* @see DifferenceType
*/
public ComplianceDrift withDiffType(String diffType) {
setDiffType(diffType);
return this;
}
/**
*
* Difference type between actual and expected recovery point objective (RPO) and recovery time objective (RTO)
* values. Currently, Resilience Hub supports only NotEqual difference type.
*
*
* @param diffType
* Difference type between actual and expected recovery point objective (RPO) and recovery time objective
* (RTO) values. Currently, Resilience Hub supports only NotEqual difference type.
* @return Returns a reference to this object so that method calls can be chained together.
* @see DifferenceType
*/
public ComplianceDrift withDiffType(DifferenceType diffType) {
this.diffType = diffType.toString();
return this;
}
/**
*
* The type of drift detected. Currently, Resilience Hub supports only ApplicationCompliance drift type.
*
*
* @param driftType
* The type of drift detected. Currently, Resilience Hub supports only ApplicationCompliance drift
* type.
* @see DriftType
*/
public void setDriftType(String driftType) {
this.driftType = driftType;
}
/**
*
* The type of drift detected. Currently, Resilience Hub supports only ApplicationCompliance drift type.
*
*
* @return The type of drift detected. Currently, Resilience Hub supports only ApplicationCompliance drift
* type.
* @see DriftType
*/
public String getDriftType() {
return this.driftType;
}
/**
*
* The type of drift detected. Currently, Resilience Hub supports only ApplicationCompliance drift type.
*
*
* @param driftType
* The type of drift detected. Currently, Resilience Hub supports only ApplicationCompliance drift
* type.
* @return Returns a reference to this object so that method calls can be chained together.
* @see DriftType
*/
public ComplianceDrift withDriftType(String driftType) {
setDriftType(driftType);
return this;
}
/**
*
* The type of drift detected. Currently, Resilience Hub supports only ApplicationCompliance drift type.
*
*
* @param driftType
* The type of drift detected. Currently, Resilience Hub supports only ApplicationCompliance drift
* type.
* @return Returns a reference to this object so that method calls can be chained together.
* @see DriftType
*/
public ComplianceDrift withDriftType(DriftType driftType) {
this.driftType = driftType.toString();
return this;
}
/**
*
* Identifier of an entity in which drift was detected. For compliance drift, the entity ID can be either
* application ID or the AppComponent ID.
*
*
* @param entityId
* Identifier of an entity in which drift was detected. For compliance drift, the entity ID can be either
* application ID or the AppComponent ID.
*/
public void setEntityId(String entityId) {
this.entityId = entityId;
}
/**
*
* Identifier of an entity in which drift was detected. For compliance drift, the entity ID can be either
* application ID or the AppComponent ID.
*
*
* @return Identifier of an entity in which drift was detected. For compliance drift, the entity ID can be either
* application ID or the AppComponent ID.
*/
public String getEntityId() {
return this.entityId;
}
/**
*
* Identifier of an entity in which drift was detected. For compliance drift, the entity ID can be either
* application ID or the AppComponent ID.
*
*
* @param entityId
* Identifier of an entity in which drift was detected. For compliance drift, the entity ID can be either
* application ID or the AppComponent ID.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ComplianceDrift withEntityId(String entityId) {
setEntityId(entityId);
return this;
}
/**
*
* The type of entity in which drift was detected. For compliance drifts, Resilience Hub supports
* AWS::ResilienceHub::AppComponent
and AWS::ResilienceHub::Application
.
*
*
* @param entityType
* The type of entity in which drift was detected. For compliance drifts, Resilience Hub supports
* AWS::ResilienceHub::AppComponent
and AWS::ResilienceHub::Application
.
*/
public void setEntityType(String entityType) {
this.entityType = entityType;
}
/**
*
* The type of entity in which drift was detected. For compliance drifts, Resilience Hub supports
* AWS::ResilienceHub::AppComponent
and AWS::ResilienceHub::Application
.
*
*
* @return The type of entity in which drift was detected. For compliance drifts, Resilience Hub supports
* AWS::ResilienceHub::AppComponent
and AWS::ResilienceHub::Application
.
*/
public String getEntityType() {
return this.entityType;
}
/**
*
* The type of entity in which drift was detected. For compliance drifts, Resilience Hub supports
* AWS::ResilienceHub::AppComponent
and AWS::ResilienceHub::Application
.
*
*
* @param entityType
* The type of entity in which drift was detected. For compliance drifts, Resilience Hub supports
* AWS::ResilienceHub::AppComponent
and AWS::ResilienceHub::Application
.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ComplianceDrift withEntityType(String entityType) {
setEntityType(entityType);
return this;
}
/**
*
* Assessment identifier of a previous assessment of the same application version. Resilience Hub uses the previous
* assessment (associated with the reference identifier) to compare the compliance with the current assessment to
* identify drifts.
*
*
* @param expectedReferenceId
* Assessment identifier of a previous assessment of the same application version. Resilience Hub uses the
* previous assessment (associated with the reference identifier) to compare the compliance with the current
* assessment to identify drifts.
*/
public void setExpectedReferenceId(String expectedReferenceId) {
this.expectedReferenceId = expectedReferenceId;
}
/**
*
* Assessment identifier of a previous assessment of the same application version. Resilience Hub uses the previous
* assessment (associated with the reference identifier) to compare the compliance with the current assessment to
* identify drifts.
*
*
* @return Assessment identifier of a previous assessment of the same application version. Resilience Hub uses the
* previous assessment (associated with the reference identifier) to compare the compliance with the current
* assessment to identify drifts.
*/
public String getExpectedReferenceId() {
return this.expectedReferenceId;
}
/**
*
* Assessment identifier of a previous assessment of the same application version. Resilience Hub uses the previous
* assessment (associated with the reference identifier) to compare the compliance with the current assessment to
* identify drifts.
*
*
* @param expectedReferenceId
* Assessment identifier of a previous assessment of the same application version. Resilience Hub uses the
* previous assessment (associated with the reference identifier) to compare the compliance with the current
* assessment to identify drifts.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ComplianceDrift withExpectedReferenceId(String expectedReferenceId) {
setExpectedReferenceId(expectedReferenceId);
return this;
}
/**
*
* The expected compliance value of an entity.
*
*
* @return The expected compliance value of an entity.
*/
public java.util.Map getExpectedValue() {
return expectedValue;
}
/**
*
* The expected compliance value of an entity.
*
*
* @param expectedValue
* The expected compliance value of an entity.
*/
public void setExpectedValue(java.util.Map expectedValue) {
this.expectedValue = expectedValue;
}
/**
*
* The expected compliance value of an entity.
*
*
* @param expectedValue
* The expected compliance value of an entity.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ComplianceDrift withExpectedValue(java.util.Map expectedValue) {
setExpectedValue(expectedValue);
return this;
}
/**
* Add a single ExpectedValue entry
*
* @see ComplianceDrift#withExpectedValue
* @returns a reference to this object so that method calls can be chained together.
*/
public ComplianceDrift addExpectedValueEntry(String key, DisruptionCompliance value) {
if (null == this.expectedValue) {
this.expectedValue = new java.util.HashMap();
}
if (this.expectedValue.containsKey(key))
throw new IllegalArgumentException("Duplicated keys (" + key.toString() + ") are provided.");
this.expectedValue.put(key, value);
return this;
}
/**
* Removes all the entries added into ExpectedValue.
*
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ComplianceDrift clearExpectedValueEntries() {
this.expectedValue = null;
return this;
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getActualReferenceId() != null)
sb.append("ActualReferenceId: ").append(getActualReferenceId()).append(",");
if (getActualValue() != null)
sb.append("ActualValue: ").append(getActualValue()).append(",");
if (getAppId() != null)
sb.append("AppId: ").append(getAppId()).append(",");
if (getAppVersion() != null)
sb.append("AppVersion: ").append(getAppVersion()).append(",");
if (getDiffType() != null)
sb.append("DiffType: ").append(getDiffType()).append(",");
if (getDriftType() != null)
sb.append("DriftType: ").append(getDriftType()).append(",");
if (getEntityId() != null)
sb.append("EntityId: ").append(getEntityId()).append(",");
if (getEntityType() != null)
sb.append("EntityType: ").append(getEntityType()).append(",");
if (getExpectedReferenceId() != null)
sb.append("ExpectedReferenceId: ").append(getExpectedReferenceId()).append(",");
if (getExpectedValue() != null)
sb.append("ExpectedValue: ").append(getExpectedValue());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof ComplianceDrift == false)
return false;
ComplianceDrift other = (ComplianceDrift) obj;
if (other.getActualReferenceId() == null ^ this.getActualReferenceId() == null)
return false;
if (other.getActualReferenceId() != null && other.getActualReferenceId().equals(this.getActualReferenceId()) == false)
return false;
if (other.getActualValue() == null ^ this.getActualValue() == null)
return false;
if (other.getActualValue() != null && other.getActualValue().equals(this.getActualValue()) == false)
return false;
if (other.getAppId() == null ^ this.getAppId() == null)
return false;
if (other.getAppId() != null && other.getAppId().equals(this.getAppId()) == false)
return false;
if (other.getAppVersion() == null ^ this.getAppVersion() == null)
return false;
if (other.getAppVersion() != null && other.getAppVersion().equals(this.getAppVersion()) == false)
return false;
if (other.getDiffType() == null ^ this.getDiffType() == null)
return false;
if (other.getDiffType() != null && other.getDiffType().equals(this.getDiffType()) == false)
return false;
if (other.getDriftType() == null ^ this.getDriftType() == null)
return false;
if (other.getDriftType() != null && other.getDriftType().equals(this.getDriftType()) == false)
return false;
if (other.getEntityId() == null ^ this.getEntityId() == null)
return false;
if (other.getEntityId() != null && other.getEntityId().equals(this.getEntityId()) == false)
return false;
if (other.getEntityType() == null ^ this.getEntityType() == null)
return false;
if (other.getEntityType() != null && other.getEntityType().equals(this.getEntityType()) == false)
return false;
if (other.getExpectedReferenceId() == null ^ this.getExpectedReferenceId() == null)
return false;
if (other.getExpectedReferenceId() != null && other.getExpectedReferenceId().equals(this.getExpectedReferenceId()) == false)
return false;
if (other.getExpectedValue() == null ^ this.getExpectedValue() == null)
return false;
if (other.getExpectedValue() != null && other.getExpectedValue().equals(this.getExpectedValue()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getActualReferenceId() == null) ? 0 : getActualReferenceId().hashCode());
hashCode = prime * hashCode + ((getActualValue() == null) ? 0 : getActualValue().hashCode());
hashCode = prime * hashCode + ((getAppId() == null) ? 0 : getAppId().hashCode());
hashCode = prime * hashCode + ((getAppVersion() == null) ? 0 : getAppVersion().hashCode());
hashCode = prime * hashCode + ((getDiffType() == null) ? 0 : getDiffType().hashCode());
hashCode = prime * hashCode + ((getDriftType() == null) ? 0 : getDriftType().hashCode());
hashCode = prime * hashCode + ((getEntityId() == null) ? 0 : getEntityId().hashCode());
hashCode = prime * hashCode + ((getEntityType() == null) ? 0 : getEntityType().hashCode());
hashCode = prime * hashCode + ((getExpectedReferenceId() == null) ? 0 : getExpectedReferenceId().hashCode());
hashCode = prime * hashCode + ((getExpectedValue() == null) ? 0 : getExpectedValue().hashCode());
return hashCode;
}
@Override
public ComplianceDrift clone() {
try {
return (ComplianceDrift) super.clone();
} catch (CloneNotSupportedException e) {
throw new IllegalStateException("Got a CloneNotSupportedException from Object.clone() " + "even though we're Cloneable!", e);
}
}
@com.amazonaws.annotation.SdkInternalApi
@Override
public void marshall(ProtocolMarshaller protocolMarshaller) {
com.amazonaws.services.resiliencehub.model.transform.ComplianceDriftMarshaller.getInstance().marshall(this, protocolMarshaller);
}
}