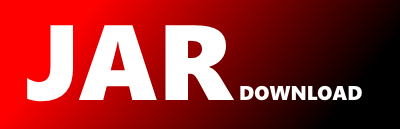
com.amazonaws.services.resiliencehub.AWSResilienceHubAsync Maven / Gradle / Ivy
Show all versions of aws-java-sdk-resiliencehub Show documentation
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.resiliencehub;
import javax.annotation.Generated;
import com.amazonaws.services.resiliencehub.model.*;
/**
* Interface for accessing AWS Resilience Hub asynchronously. Each asynchronous method will return a Java Future object
* representing the asynchronous operation; overloads which accept an {@code AsyncHandler} can be used to receive
* notification when an asynchronous operation completes.
*
* Note: Do not directly implement this interface, new methods are added to it regularly. Extend from
* {@link com.amazonaws.services.resiliencehub.AbstractAWSResilienceHubAsync} instead.
*
*
*
* Resilience Hub helps you proactively prepare and protect your Amazon Web Services applications from disruptions. It
* offers continual resiliency assessment and validation that integrates into your software development lifecycle. This
* enables you to uncover resiliency weaknesses, ensure recovery time objective (RTO) and recovery point objective (RPO)
* targets for your applications are met, and resolve issues before they are released into production.
*
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public interface AWSResilienceHubAsync extends AWSResilienceHub {
/**
*
* Adds the source of resource-maps to the draft version of an application. During assessment, Resilience Hub will
* use these resource-maps to resolve the latest physical ID for each resource in the application template. For more
* information about different types of resources suported by Resilience Hub and how to add them in your
* application, see Step
* 2: How is your application managed? in the Resilience Hub User Guide.
*
*
* @param addDraftAppVersionResourceMappingsRequest
* @return A Java Future containing the result of the AddDraftAppVersionResourceMappings operation returned by the
* service.
* @sample AWSResilienceHubAsync.AddDraftAppVersionResourceMappings
* @see AWS API Documentation
*/
java.util.concurrent.Future addDraftAppVersionResourceMappingsAsync(
AddDraftAppVersionResourceMappingsRequest addDraftAppVersionResourceMappingsRequest);
/**
*
* Adds the source of resource-maps to the draft version of an application. During assessment, Resilience Hub will
* use these resource-maps to resolve the latest physical ID for each resource in the application template. For more
* information about different types of resources suported by Resilience Hub and how to add them in your
* application, see Step
* 2: How is your application managed? in the Resilience Hub User Guide.
*
*
* @param addDraftAppVersionResourceMappingsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the AddDraftAppVersionResourceMappings operation returned by the
* service.
* @sample AWSResilienceHubAsyncHandler.AddDraftAppVersionResourceMappings
* @see AWS API Documentation
*/
java.util.concurrent.Future addDraftAppVersionResourceMappingsAsync(
AddDraftAppVersionResourceMappingsRequest addDraftAppVersionResourceMappingsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Enables you to include or exclude one or more operational recommendations.
*
*
* @param batchUpdateRecommendationStatusRequest
* @return A Java Future containing the result of the BatchUpdateRecommendationStatus operation returned by the
* service.
* @sample AWSResilienceHubAsync.BatchUpdateRecommendationStatus
* @see AWS API Documentation
*/
java.util.concurrent.Future batchUpdateRecommendationStatusAsync(
BatchUpdateRecommendationStatusRequest batchUpdateRecommendationStatusRequest);
/**
*
* Enables you to include or exclude one or more operational recommendations.
*
*
* @param batchUpdateRecommendationStatusRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the BatchUpdateRecommendationStatus operation returned by the
* service.
* @sample AWSResilienceHubAsyncHandler.BatchUpdateRecommendationStatus
* @see AWS API Documentation
*/
java.util.concurrent.Future batchUpdateRecommendationStatusAsync(
BatchUpdateRecommendationStatusRequest batchUpdateRecommendationStatusRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates an Resilience Hub application. An Resilience Hub application is a collection of Amazon Web Services
* resources structured to prevent and recover Amazon Web Services application disruptions. To describe a Resilience
* Hub application, you provide an application name, resources from one or more CloudFormation stacks, Resource
* Groups, Terraform state files, AppRegistry applications, and an appropriate resiliency policy. In addition, you
* can also add resources that are located on Amazon Elastic Kubernetes Service (Amazon EKS) clusters as optional
* resources. For more information about the number of resources supported per application, see Service quotas.
*
*
* After you create an Resilience Hub application, you publish it so that you can run a resiliency assessment on it.
* You can then use recommendations from the assessment to improve resiliency by running another assessment,
* comparing results, and then iterating the process until you achieve your goals for recovery time objective (RTO)
* and recovery point objective (RPO).
*
*
* @param createAppRequest
* @return A Java Future containing the result of the CreateApp operation returned by the service.
* @sample AWSResilienceHubAsync.CreateApp
* @see AWS API
* Documentation
*/
java.util.concurrent.Future createAppAsync(CreateAppRequest createAppRequest);
/**
*
* Creates an Resilience Hub application. An Resilience Hub application is a collection of Amazon Web Services
* resources structured to prevent and recover Amazon Web Services application disruptions. To describe a Resilience
* Hub application, you provide an application name, resources from one or more CloudFormation stacks, Resource
* Groups, Terraform state files, AppRegistry applications, and an appropriate resiliency policy. In addition, you
* can also add resources that are located on Amazon Elastic Kubernetes Service (Amazon EKS) clusters as optional
* resources. For more information about the number of resources supported per application, see Service quotas.
*
*
* After you create an Resilience Hub application, you publish it so that you can run a resiliency assessment on it.
* You can then use recommendations from the assessment to improve resiliency by running another assessment,
* comparing results, and then iterating the process until you achieve your goals for recovery time objective (RTO)
* and recovery point objective (RPO).
*
*
* @param createAppRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateApp operation returned by the service.
* @sample AWSResilienceHubAsyncHandler.CreateApp
* @see AWS API
* Documentation
*/
java.util.concurrent.Future createAppAsync(CreateAppRequest createAppRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates a new Application Component in the Resilience Hub application.
*
*
*
* This API updates the Resilience Hub application draft version. To use this Application Component for running
* assessments, you must publish the Resilience Hub application using the PublishAppVersion
API.
*
*
*
* @param createAppVersionAppComponentRequest
* @return A Java Future containing the result of the CreateAppVersionAppComponent operation returned by the
* service.
* @sample AWSResilienceHubAsync.CreateAppVersionAppComponent
* @see AWS API Documentation
*/
java.util.concurrent.Future createAppVersionAppComponentAsync(
CreateAppVersionAppComponentRequest createAppVersionAppComponentRequest);
/**
*
* Creates a new Application Component in the Resilience Hub application.
*
*
*
* This API updates the Resilience Hub application draft version. To use this Application Component for running
* assessments, you must publish the Resilience Hub application using the PublishAppVersion
API.
*
*
*
* @param createAppVersionAppComponentRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateAppVersionAppComponent operation returned by the
* service.
* @sample AWSResilienceHubAsyncHandler.CreateAppVersionAppComponent
* @see AWS API Documentation
*/
java.util.concurrent.Future createAppVersionAppComponentAsync(
CreateAppVersionAppComponentRequest createAppVersionAppComponentRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Adds a resource to the Resilience Hub application and assigns it to the specified Application Components. If you
* specify a new Application Component, Resilience Hub will automatically create the Application Component.
*
*
*
* -
*
* This action has no effect outside Resilience Hub.
*
*
* -
*
* This API updates the Resilience Hub application draft version. To use this resource for running resiliency
* assessments, you must publish the Resilience Hub application using the PublishAppVersion
API.
*
*
* -
*
* To update application version with new physicalResourceID
, you must call
* ResolveAppVersionResources
API.
*
*
*
*
*
* @param createAppVersionResourceRequest
* @return A Java Future containing the result of the CreateAppVersionResource operation returned by the service.
* @sample AWSResilienceHubAsync.CreateAppVersionResource
* @see AWS API Documentation
*/
java.util.concurrent.Future createAppVersionResourceAsync(CreateAppVersionResourceRequest createAppVersionResourceRequest);
/**
*
* Adds a resource to the Resilience Hub application and assigns it to the specified Application Components. If you
* specify a new Application Component, Resilience Hub will automatically create the Application Component.
*
*
*
* -
*
* This action has no effect outside Resilience Hub.
*
*
* -
*
* This API updates the Resilience Hub application draft version. To use this resource for running resiliency
* assessments, you must publish the Resilience Hub application using the PublishAppVersion
API.
*
*
* -
*
* To update application version with new physicalResourceID
, you must call
* ResolveAppVersionResources
API.
*
*
*
*
*
* @param createAppVersionResourceRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateAppVersionResource operation returned by the service.
* @sample AWSResilienceHubAsyncHandler.CreateAppVersionResource
* @see AWS API Documentation
*/
java.util.concurrent.Future createAppVersionResourceAsync(CreateAppVersionResourceRequest createAppVersionResourceRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates a new recommendation template for the Resilience Hub application.
*
*
* @param createRecommendationTemplateRequest
* @return A Java Future containing the result of the CreateRecommendationTemplate operation returned by the
* service.
* @sample AWSResilienceHubAsync.CreateRecommendationTemplate
* @see AWS API Documentation
*/
java.util.concurrent.Future createRecommendationTemplateAsync(
CreateRecommendationTemplateRequest createRecommendationTemplateRequest);
/**
*
* Creates a new recommendation template for the Resilience Hub application.
*
*
* @param createRecommendationTemplateRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateRecommendationTemplate operation returned by the
* service.
* @sample AWSResilienceHubAsyncHandler.CreateRecommendationTemplate
* @see AWS API Documentation
*/
java.util.concurrent.Future createRecommendationTemplateAsync(
CreateRecommendationTemplateRequest createRecommendationTemplateRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates a resiliency policy for an application.
*
*
*
* Resilience Hub allows you to provide a value of zero for rtoInSecs
and rpoInSecs
of
* your resiliency policy. But, while assessing your application, the lowest possible assessment result is near
* zero. Hence, if you provide value zero for rtoInSecs
and rpoInSecs
, the estimated
* workload RTO and estimated workload RPO result will be near zero and the Compliance status for your
* application will be set to Policy breached.
*
*
*
* @param createResiliencyPolicyRequest
* @return A Java Future containing the result of the CreateResiliencyPolicy operation returned by the service.
* @sample AWSResilienceHubAsync.CreateResiliencyPolicy
* @see AWS API Documentation
*/
java.util.concurrent.Future createResiliencyPolicyAsync(CreateResiliencyPolicyRequest createResiliencyPolicyRequest);
/**
*
* Creates a resiliency policy for an application.
*
*
*
* Resilience Hub allows you to provide a value of zero for rtoInSecs
and rpoInSecs
of
* your resiliency policy. But, while assessing your application, the lowest possible assessment result is near
* zero. Hence, if you provide value zero for rtoInSecs
and rpoInSecs
, the estimated
* workload RTO and estimated workload RPO result will be near zero and the Compliance status for your
* application will be set to Policy breached.
*
*
*
* @param createResiliencyPolicyRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateResiliencyPolicy operation returned by the service.
* @sample AWSResilienceHubAsyncHandler.CreateResiliencyPolicy
* @see AWS API Documentation
*/
java.util.concurrent.Future createResiliencyPolicyAsync(CreateResiliencyPolicyRequest createResiliencyPolicyRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deletes an Resilience Hub application. This is a destructive action that can't be undone.
*
*
* @param deleteAppRequest
* @return A Java Future containing the result of the DeleteApp operation returned by the service.
* @sample AWSResilienceHubAsync.DeleteApp
* @see AWS API
* Documentation
*/
java.util.concurrent.Future deleteAppAsync(DeleteAppRequest deleteAppRequest);
/**
*
* Deletes an Resilience Hub application. This is a destructive action that can't be undone.
*
*
* @param deleteAppRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteApp operation returned by the service.
* @sample AWSResilienceHubAsyncHandler.DeleteApp
* @see AWS API
* Documentation
*/
java.util.concurrent.Future deleteAppAsync(DeleteAppRequest deleteAppRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deletes an Resilience Hub application assessment. This is a destructive action that can't be undone.
*
*
* @param deleteAppAssessmentRequest
* @return A Java Future containing the result of the DeleteAppAssessment operation returned by the service.
* @sample AWSResilienceHubAsync.DeleteAppAssessment
* @see AWS API Documentation
*/
java.util.concurrent.Future deleteAppAssessmentAsync(DeleteAppAssessmentRequest deleteAppAssessmentRequest);
/**
*
* Deletes an Resilience Hub application assessment. This is a destructive action that can't be undone.
*
*
* @param deleteAppAssessmentRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteAppAssessment operation returned by the service.
* @sample AWSResilienceHubAsyncHandler.DeleteAppAssessment
* @see AWS API Documentation
*/
java.util.concurrent.Future deleteAppAssessmentAsync(DeleteAppAssessmentRequest deleteAppAssessmentRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deletes the input source and all of its imported resources from the Resilience Hub application.
*
*
* @param deleteAppInputSourceRequest
* @return A Java Future containing the result of the DeleteAppInputSource operation returned by the service.
* @sample AWSResilienceHubAsync.DeleteAppInputSource
* @see AWS API Documentation
*/
java.util.concurrent.Future deleteAppInputSourceAsync(DeleteAppInputSourceRequest deleteAppInputSourceRequest);
/**
*
* Deletes the input source and all of its imported resources from the Resilience Hub application.
*
*
* @param deleteAppInputSourceRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteAppInputSource operation returned by the service.
* @sample AWSResilienceHubAsyncHandler.DeleteAppInputSource
* @see AWS API Documentation
*/
java.util.concurrent.Future deleteAppInputSourceAsync(DeleteAppInputSourceRequest deleteAppInputSourceRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deletes an Application Component from the Resilience Hub application.
*
*
*
* -
*
* This API updates the Resilience Hub application draft version. To use this Application Component for running
* assessments, you must publish the Resilience Hub application using the PublishAppVersion
API.
*
*
* -
*
* You will not be able to delete an Application Component if it has resources associated with it.
*
*
*
*
*
* @param deleteAppVersionAppComponentRequest
* @return A Java Future containing the result of the DeleteAppVersionAppComponent operation returned by the
* service.
* @sample AWSResilienceHubAsync.DeleteAppVersionAppComponent
* @see AWS API Documentation
*/
java.util.concurrent.Future deleteAppVersionAppComponentAsync(
DeleteAppVersionAppComponentRequest deleteAppVersionAppComponentRequest);
/**
*
* Deletes an Application Component from the Resilience Hub application.
*
*
*
* -
*
* This API updates the Resilience Hub application draft version. To use this Application Component for running
* assessments, you must publish the Resilience Hub application using the PublishAppVersion
API.
*
*
* -
*
* You will not be able to delete an Application Component if it has resources associated with it.
*
*
*
*
*
* @param deleteAppVersionAppComponentRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteAppVersionAppComponent operation returned by the
* service.
* @sample AWSResilienceHubAsyncHandler.DeleteAppVersionAppComponent
* @see AWS API Documentation
*/
java.util.concurrent.Future deleteAppVersionAppComponentAsync(
DeleteAppVersionAppComponentRequest deleteAppVersionAppComponentRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deletes a resource from the Resilience Hub application.
*
*
*
* -
*
* You can only delete a manually added resource. To exclude non-manually added resources, use the
* UpdateAppVersionResource
API.
*
*
* -
*
* This action has no effect outside Resilience Hub.
*
*
* -
*
* This API updates the Resilience Hub application draft version. To use this resource for running resiliency
* assessments, you must publish the Resilience Hub application using the PublishAppVersion
API.
*
*
*
*
*
* @param deleteAppVersionResourceRequest
* @return A Java Future containing the result of the DeleteAppVersionResource operation returned by the service.
* @sample AWSResilienceHubAsync.DeleteAppVersionResource
* @see AWS API Documentation
*/
java.util.concurrent.Future deleteAppVersionResourceAsync(DeleteAppVersionResourceRequest deleteAppVersionResourceRequest);
/**
*
* Deletes a resource from the Resilience Hub application.
*
*
*
* -
*
* You can only delete a manually added resource. To exclude non-manually added resources, use the
* UpdateAppVersionResource
API.
*
*
* -
*
* This action has no effect outside Resilience Hub.
*
*
* -
*
* This API updates the Resilience Hub application draft version. To use this resource for running resiliency
* assessments, you must publish the Resilience Hub application using the PublishAppVersion
API.
*
*
*
*
*
* @param deleteAppVersionResourceRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteAppVersionResource operation returned by the service.
* @sample AWSResilienceHubAsyncHandler.DeleteAppVersionResource
* @see AWS API Documentation
*/
java.util.concurrent.Future deleteAppVersionResourceAsync(DeleteAppVersionResourceRequest deleteAppVersionResourceRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deletes a recommendation template. This is a destructive action that can't be undone.
*
*
* @param deleteRecommendationTemplateRequest
* @return A Java Future containing the result of the DeleteRecommendationTemplate operation returned by the
* service.
* @sample AWSResilienceHubAsync.DeleteRecommendationTemplate
* @see AWS API Documentation
*/
java.util.concurrent.Future deleteRecommendationTemplateAsync(
DeleteRecommendationTemplateRequest deleteRecommendationTemplateRequest);
/**
*
* Deletes a recommendation template. This is a destructive action that can't be undone.
*
*
* @param deleteRecommendationTemplateRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteRecommendationTemplate operation returned by the
* service.
* @sample AWSResilienceHubAsyncHandler.DeleteRecommendationTemplate
* @see AWS API Documentation
*/
java.util.concurrent.Future deleteRecommendationTemplateAsync(
DeleteRecommendationTemplateRequest deleteRecommendationTemplateRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deletes a resiliency policy. This is a destructive action that can't be undone.
*
*
* @param deleteResiliencyPolicyRequest
* @return A Java Future containing the result of the DeleteResiliencyPolicy operation returned by the service.
* @sample AWSResilienceHubAsync.DeleteResiliencyPolicy
* @see AWS API Documentation
*/
java.util.concurrent.Future deleteResiliencyPolicyAsync(DeleteResiliencyPolicyRequest deleteResiliencyPolicyRequest);
/**
*
* Deletes a resiliency policy. This is a destructive action that can't be undone.
*
*
* @param deleteResiliencyPolicyRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteResiliencyPolicy operation returned by the service.
* @sample AWSResilienceHubAsyncHandler.DeleteResiliencyPolicy
* @see AWS API Documentation
*/
java.util.concurrent.Future deleteResiliencyPolicyAsync(DeleteResiliencyPolicyRequest deleteResiliencyPolicyRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Describes an Resilience Hub application.
*
*
* @param describeAppRequest
* @return A Java Future containing the result of the DescribeApp operation returned by the service.
* @sample AWSResilienceHubAsync.DescribeApp
* @see AWS API
* Documentation
*/
java.util.concurrent.Future describeAppAsync(DescribeAppRequest describeAppRequest);
/**
*
* Describes an Resilience Hub application.
*
*
* @param describeAppRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeApp operation returned by the service.
* @sample AWSResilienceHubAsyncHandler.DescribeApp
* @see AWS API
* Documentation
*/
java.util.concurrent.Future describeAppAsync(DescribeAppRequest describeAppRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Describes an assessment for an Resilience Hub application.
*
*
* @param describeAppAssessmentRequest
* @return A Java Future containing the result of the DescribeAppAssessment operation returned by the service.
* @sample AWSResilienceHubAsync.DescribeAppAssessment
* @see AWS API Documentation
*/
java.util.concurrent.Future describeAppAssessmentAsync(DescribeAppAssessmentRequest describeAppAssessmentRequest);
/**
*
* Describes an assessment for an Resilience Hub application.
*
*
* @param describeAppAssessmentRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeAppAssessment operation returned by the service.
* @sample AWSResilienceHubAsyncHandler.DescribeAppAssessment
* @see AWS API Documentation
*/
java.util.concurrent.Future describeAppAssessmentAsync(DescribeAppAssessmentRequest describeAppAssessmentRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Describes the Resilience Hub application version.
*
*
* @param describeAppVersionRequest
* @return A Java Future containing the result of the DescribeAppVersion operation returned by the service.
* @sample AWSResilienceHubAsync.DescribeAppVersion
* @see AWS API Documentation
*/
java.util.concurrent.Future describeAppVersionAsync(DescribeAppVersionRequest describeAppVersionRequest);
/**
*
* Describes the Resilience Hub application version.
*
*
* @param describeAppVersionRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeAppVersion operation returned by the service.
* @sample AWSResilienceHubAsyncHandler.DescribeAppVersion
* @see AWS API Documentation
*/
java.util.concurrent.Future describeAppVersionAsync(DescribeAppVersionRequest describeAppVersionRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Describes an Application Component in the Resilience Hub application.
*
*
* @param describeAppVersionAppComponentRequest
* @return A Java Future containing the result of the DescribeAppVersionAppComponent operation returned by the
* service.
* @sample AWSResilienceHubAsync.DescribeAppVersionAppComponent
* @see AWS API Documentation
*/
java.util.concurrent.Future describeAppVersionAppComponentAsync(
DescribeAppVersionAppComponentRequest describeAppVersionAppComponentRequest);
/**
*
* Describes an Application Component in the Resilience Hub application.
*
*
* @param describeAppVersionAppComponentRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeAppVersionAppComponent operation returned by the
* service.
* @sample AWSResilienceHubAsyncHandler.DescribeAppVersionAppComponent
* @see AWS API Documentation
*/
java.util.concurrent.Future describeAppVersionAppComponentAsync(
DescribeAppVersionAppComponentRequest describeAppVersionAppComponentRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Describes a resource of the Resilience Hub application.
*
*
*
* This API accepts only one of the following parameters to descibe the resource:
*
*
* -
*
* resourceName
*
*
* -
*
* logicalResourceId
*
*
* -
*
* physicalResourceId
(Along with physicalResourceId
, you can also provide
* awsAccountId
, and awsRegion
)
*
*
*
*
*
* @param describeAppVersionResourceRequest
* @return A Java Future containing the result of the DescribeAppVersionResource operation returned by the service.
* @sample AWSResilienceHubAsync.DescribeAppVersionResource
* @see AWS API Documentation
*/
java.util.concurrent.Future describeAppVersionResourceAsync(
DescribeAppVersionResourceRequest describeAppVersionResourceRequest);
/**
*
* Describes a resource of the Resilience Hub application.
*
*
*
* This API accepts only one of the following parameters to descibe the resource:
*
*
* -
*
* resourceName
*
*
* -
*
* logicalResourceId
*
*
* -
*
* physicalResourceId
(Along with physicalResourceId
, you can also provide
* awsAccountId
, and awsRegion
)
*
*
*
*
*
* @param describeAppVersionResourceRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeAppVersionResource operation returned by the service.
* @sample AWSResilienceHubAsyncHandler.DescribeAppVersionResource
* @see AWS API Documentation
*/
java.util.concurrent.Future describeAppVersionResourceAsync(
DescribeAppVersionResourceRequest describeAppVersionResourceRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns the resolution status for the specified resolution identifier for an application version. If
* resolutionId
is not specified, the current resolution status is returned.
*
*
* @param describeAppVersionResourcesResolutionStatusRequest
* @return A Java Future containing the result of the DescribeAppVersionResourcesResolutionStatus operation returned
* by the service.
* @sample AWSResilienceHubAsync.DescribeAppVersionResourcesResolutionStatus
* @see AWS API Documentation
*/
java.util.concurrent.Future describeAppVersionResourcesResolutionStatusAsync(
DescribeAppVersionResourcesResolutionStatusRequest describeAppVersionResourcesResolutionStatusRequest);
/**
*
* Returns the resolution status for the specified resolution identifier for an application version. If
* resolutionId
is not specified, the current resolution status is returned.
*
*
* @param describeAppVersionResourcesResolutionStatusRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeAppVersionResourcesResolutionStatus operation returned
* by the service.
* @sample AWSResilienceHubAsyncHandler.DescribeAppVersionResourcesResolutionStatus
* @see AWS API Documentation
*/
java.util.concurrent.Future describeAppVersionResourcesResolutionStatusAsync(
DescribeAppVersionResourcesResolutionStatusRequest describeAppVersionResourcesResolutionStatusRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Describes details about an Resilience Hub application.
*
*
* @param describeAppVersionTemplateRequest
* @return A Java Future containing the result of the DescribeAppVersionTemplate operation returned by the service.
* @sample AWSResilienceHubAsync.DescribeAppVersionTemplate
* @see AWS API Documentation
*/
java.util.concurrent.Future describeAppVersionTemplateAsync(
DescribeAppVersionTemplateRequest describeAppVersionTemplateRequest);
/**
*
* Describes details about an Resilience Hub application.
*
*
* @param describeAppVersionTemplateRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeAppVersionTemplate operation returned by the service.
* @sample AWSResilienceHubAsyncHandler.DescribeAppVersionTemplate
* @see AWS API Documentation
*/
java.util.concurrent.Future describeAppVersionTemplateAsync(
DescribeAppVersionTemplateRequest describeAppVersionTemplateRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Describes the status of importing resources to an application version.
*
*
*
* If you get a 404 error with ResourceImportStatusNotFoundAppMetadataException
, you must call
* importResourcesToDraftAppVersion
after creating the application and before calling
* describeDraftAppVersionResourcesImportStatus
to obtain the status.
*
*
*
* @param describeDraftAppVersionResourcesImportStatusRequest
* @return A Java Future containing the result of the DescribeDraftAppVersionResourcesImportStatus operation
* returned by the service.
* @sample AWSResilienceHubAsync.DescribeDraftAppVersionResourcesImportStatus
* @see AWS API Documentation
*/
java.util.concurrent.Future describeDraftAppVersionResourcesImportStatusAsync(
DescribeDraftAppVersionResourcesImportStatusRequest describeDraftAppVersionResourcesImportStatusRequest);
/**
*
* Describes the status of importing resources to an application version.
*
*
*
* If you get a 404 error with ResourceImportStatusNotFoundAppMetadataException
, you must call
* importResourcesToDraftAppVersion
after creating the application and before calling
* describeDraftAppVersionResourcesImportStatus
to obtain the status.
*
*
*
* @param describeDraftAppVersionResourcesImportStatusRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeDraftAppVersionResourcesImportStatus operation
* returned by the service.
* @sample AWSResilienceHubAsyncHandler.DescribeDraftAppVersionResourcesImportStatus
* @see AWS API Documentation
*/
java.util.concurrent.Future describeDraftAppVersionResourcesImportStatusAsync(
DescribeDraftAppVersionResourcesImportStatusRequest describeDraftAppVersionResourcesImportStatusRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Describes a specified resiliency policy for an Resilience Hub application. The returned policy object includes
* creation time, data location constraints, the Amazon Resource Name (ARN) for the policy, tags, tier, and more.
*
*
* @param describeResiliencyPolicyRequest
* @return A Java Future containing the result of the DescribeResiliencyPolicy operation returned by the service.
* @sample AWSResilienceHubAsync.DescribeResiliencyPolicy
* @see AWS API Documentation
*/
java.util.concurrent.Future describeResiliencyPolicyAsync(DescribeResiliencyPolicyRequest describeResiliencyPolicyRequest);
/**
*
* Describes a specified resiliency policy for an Resilience Hub application. The returned policy object includes
* creation time, data location constraints, the Amazon Resource Name (ARN) for the policy, tags, tier, and more.
*
*
* @param describeResiliencyPolicyRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeResiliencyPolicy operation returned by the service.
* @sample AWSResilienceHubAsyncHandler.DescribeResiliencyPolicy
* @see AWS API Documentation
*/
java.util.concurrent.Future describeResiliencyPolicyAsync(DescribeResiliencyPolicyRequest describeResiliencyPolicyRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Imports resources to Resilience Hub application draft version from different input sources. For more information
* about the input sources supported by Resilience Hub, see Discover the structure
* and describe your Resilience Hub application.
*
*
* @param importResourcesToDraftAppVersionRequest
* @return A Java Future containing the result of the ImportResourcesToDraftAppVersion operation returned by the
* service.
* @sample AWSResilienceHubAsync.ImportResourcesToDraftAppVersion
* @see AWS API Documentation
*/
java.util.concurrent.Future importResourcesToDraftAppVersionAsync(
ImportResourcesToDraftAppVersionRequest importResourcesToDraftAppVersionRequest);
/**
*
* Imports resources to Resilience Hub application draft version from different input sources. For more information
* about the input sources supported by Resilience Hub, see Discover the structure
* and describe your Resilience Hub application.
*
*
* @param importResourcesToDraftAppVersionRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ImportResourcesToDraftAppVersion operation returned by the
* service.
* @sample AWSResilienceHubAsyncHandler.ImportResourcesToDraftAppVersion
* @see AWS API Documentation
*/
java.util.concurrent.Future importResourcesToDraftAppVersionAsync(
ImportResourcesToDraftAppVersionRequest importResourcesToDraftAppVersionRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Lists the alarm recommendations for an Resilience Hub application.
*
*
* @param listAlarmRecommendationsRequest
* @return A Java Future containing the result of the ListAlarmRecommendations operation returned by the service.
* @sample AWSResilienceHubAsync.ListAlarmRecommendations
* @see AWS API Documentation
*/
java.util.concurrent.Future listAlarmRecommendationsAsync(ListAlarmRecommendationsRequest listAlarmRecommendationsRequest);
/**
*
* Lists the alarm recommendations for an Resilience Hub application.
*
*
* @param listAlarmRecommendationsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListAlarmRecommendations operation returned by the service.
* @sample AWSResilienceHubAsyncHandler.ListAlarmRecommendations
* @see AWS API Documentation
*/
java.util.concurrent.Future listAlarmRecommendationsAsync(ListAlarmRecommendationsRequest listAlarmRecommendationsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* List of compliance drifts that were detected while running an assessment.
*
*
* @param listAppAssessmentComplianceDriftsRequest
* @return A Java Future containing the result of the ListAppAssessmentComplianceDrifts operation returned by the
* service.
* @sample AWSResilienceHubAsync.ListAppAssessmentComplianceDrifts
* @see AWS API Documentation
*/
java.util.concurrent.Future listAppAssessmentComplianceDriftsAsync(
ListAppAssessmentComplianceDriftsRequest listAppAssessmentComplianceDriftsRequest);
/**
*
* List of compliance drifts that were detected while running an assessment.
*
*
* @param listAppAssessmentComplianceDriftsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListAppAssessmentComplianceDrifts operation returned by the
* service.
* @sample AWSResilienceHubAsyncHandler.ListAppAssessmentComplianceDrifts
* @see AWS API Documentation
*/
java.util.concurrent.Future listAppAssessmentComplianceDriftsAsync(
ListAppAssessmentComplianceDriftsRequest listAppAssessmentComplianceDriftsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Indicates the list of resource drifts that were detected while running an assessment.
*
*
* @param listAppAssessmentResourceDriftsRequest
* @return A Java Future containing the result of the ListAppAssessmentResourceDrifts operation returned by the
* service.
* @sample AWSResilienceHubAsync.ListAppAssessmentResourceDrifts
* @see AWS API Documentation
*/
java.util.concurrent.Future listAppAssessmentResourceDriftsAsync(
ListAppAssessmentResourceDriftsRequest listAppAssessmentResourceDriftsRequest);
/**
*
* Indicates the list of resource drifts that were detected while running an assessment.
*
*
* @param listAppAssessmentResourceDriftsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListAppAssessmentResourceDrifts operation returned by the
* service.
* @sample AWSResilienceHubAsyncHandler.ListAppAssessmentResourceDrifts
* @see AWS API Documentation
*/
java.util.concurrent.Future listAppAssessmentResourceDriftsAsync(
ListAppAssessmentResourceDriftsRequest listAppAssessmentResourceDriftsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Lists the assessments for an Resilience Hub application. You can use request parameters to refine the results for
* the response object.
*
*
* @param listAppAssessmentsRequest
* @return A Java Future containing the result of the ListAppAssessments operation returned by the service.
* @sample AWSResilienceHubAsync.ListAppAssessments
* @see AWS API Documentation
*/
java.util.concurrent.Future listAppAssessmentsAsync(ListAppAssessmentsRequest listAppAssessmentsRequest);
/**
*
* Lists the assessments for an Resilience Hub application. You can use request parameters to refine the results for
* the response object.
*
*
* @param listAppAssessmentsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListAppAssessments operation returned by the service.
* @sample AWSResilienceHubAsyncHandler.ListAppAssessments
* @see AWS API Documentation
*/
java.util.concurrent.Future listAppAssessmentsAsync(ListAppAssessmentsRequest listAppAssessmentsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Lists the compliances for an Resilience Hub Application Component.
*
*
* @param listAppComponentCompliancesRequest
* @return A Java Future containing the result of the ListAppComponentCompliances operation returned by the service.
* @sample AWSResilienceHubAsync.ListAppComponentCompliances
* @see AWS API Documentation
*/
java.util.concurrent.Future listAppComponentCompliancesAsync(
ListAppComponentCompliancesRequest listAppComponentCompliancesRequest);
/**
*
* Lists the compliances for an Resilience Hub Application Component.
*
*
* @param listAppComponentCompliancesRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListAppComponentCompliances operation returned by the service.
* @sample AWSResilienceHubAsyncHandler.ListAppComponentCompliances
* @see AWS API Documentation
*/
java.util.concurrent.Future listAppComponentCompliancesAsync(
ListAppComponentCompliancesRequest listAppComponentCompliancesRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Lists the recommendations for an Resilience Hub Application Component.
*
*
* @param listAppComponentRecommendationsRequest
* @return A Java Future containing the result of the ListAppComponentRecommendations operation returned by the
* service.
* @sample AWSResilienceHubAsync.ListAppComponentRecommendations
* @see AWS API Documentation
*/
java.util.concurrent.Future listAppComponentRecommendationsAsync(
ListAppComponentRecommendationsRequest listAppComponentRecommendationsRequest);
/**
*
* Lists the recommendations for an Resilience Hub Application Component.
*
*
* @param listAppComponentRecommendationsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListAppComponentRecommendations operation returned by the
* service.
* @sample AWSResilienceHubAsyncHandler.ListAppComponentRecommendations
* @see AWS API Documentation
*/
java.util.concurrent.Future listAppComponentRecommendationsAsync(
ListAppComponentRecommendationsRequest listAppComponentRecommendationsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Lists all the input sources of the Resilience Hub application. For more information about the input sources
* supported by Resilience Hub, see Discover the structure
* and describe your Resilience Hub application.
*
*
* @param listAppInputSourcesRequest
* @return A Java Future containing the result of the ListAppInputSources operation returned by the service.
* @sample AWSResilienceHubAsync.ListAppInputSources
* @see AWS API Documentation
*/
java.util.concurrent.Future listAppInputSourcesAsync(ListAppInputSourcesRequest listAppInputSourcesRequest);
/**
*
* Lists all the input sources of the Resilience Hub application. For more information about the input sources
* supported by Resilience Hub, see Discover the structure
* and describe your Resilience Hub application.
*
*
* @param listAppInputSourcesRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListAppInputSources operation returned by the service.
* @sample AWSResilienceHubAsyncHandler.ListAppInputSources
* @see AWS API Documentation
*/
java.util.concurrent.Future listAppInputSourcesAsync(ListAppInputSourcesRequest listAppInputSourcesRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Lists all the Application Components in the Resilience Hub application.
*
*
* @param listAppVersionAppComponentsRequest
* @return A Java Future containing the result of the ListAppVersionAppComponents operation returned by the service.
* @sample AWSResilienceHubAsync.ListAppVersionAppComponents
* @see AWS API Documentation
*/
java.util.concurrent.Future listAppVersionAppComponentsAsync(
ListAppVersionAppComponentsRequest listAppVersionAppComponentsRequest);
/**
*
* Lists all the Application Components in the Resilience Hub application.
*
*
* @param listAppVersionAppComponentsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListAppVersionAppComponents operation returned by the service.
* @sample AWSResilienceHubAsyncHandler.ListAppVersionAppComponents
* @see AWS API Documentation
*/
java.util.concurrent.Future listAppVersionAppComponentsAsync(
ListAppVersionAppComponentsRequest listAppVersionAppComponentsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Lists how the resources in an application version are mapped/sourced from. Mappings can be physical resource
* identifiers, CloudFormation stacks, resource-groups, or an application registry app.
*
*
* @param listAppVersionResourceMappingsRequest
* @return A Java Future containing the result of the ListAppVersionResourceMappings operation returned by the
* service.
* @sample AWSResilienceHubAsync.ListAppVersionResourceMappings
* @see AWS API Documentation
*/
java.util.concurrent.Future listAppVersionResourceMappingsAsync(
ListAppVersionResourceMappingsRequest listAppVersionResourceMappingsRequest);
/**
*
* Lists how the resources in an application version are mapped/sourced from. Mappings can be physical resource
* identifiers, CloudFormation stacks, resource-groups, or an application registry app.
*
*
* @param listAppVersionResourceMappingsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListAppVersionResourceMappings operation returned by the
* service.
* @sample AWSResilienceHubAsyncHandler.ListAppVersionResourceMappings
* @see AWS API Documentation
*/
java.util.concurrent.Future listAppVersionResourceMappingsAsync(
ListAppVersionResourceMappingsRequest listAppVersionResourceMappingsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Lists all the resources in an Resilience Hub application.
*
*
* @param listAppVersionResourcesRequest
* @return A Java Future containing the result of the ListAppVersionResources operation returned by the service.
* @sample AWSResilienceHubAsync.ListAppVersionResources
* @see AWS API Documentation
*/
java.util.concurrent.Future listAppVersionResourcesAsync(ListAppVersionResourcesRequest listAppVersionResourcesRequest);
/**
*
* Lists all the resources in an Resilience Hub application.
*
*
* @param listAppVersionResourcesRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListAppVersionResources operation returned by the service.
* @sample AWSResilienceHubAsyncHandler.ListAppVersionResources
* @see AWS API Documentation
*/
java.util.concurrent.Future listAppVersionResourcesAsync(ListAppVersionResourcesRequest listAppVersionResourcesRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Lists the different versions for the Resilience Hub applications.
*
*
* @param listAppVersionsRequest
* @return A Java Future containing the result of the ListAppVersions operation returned by the service.
* @sample AWSResilienceHubAsync.ListAppVersions
* @see AWS
* API Documentation
*/
java.util.concurrent.Future listAppVersionsAsync(ListAppVersionsRequest listAppVersionsRequest);
/**
*
* Lists the different versions for the Resilience Hub applications.
*
*
* @param listAppVersionsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListAppVersions operation returned by the service.
* @sample AWSResilienceHubAsyncHandler.ListAppVersions
* @see AWS
* API Documentation
*/
java.util.concurrent.Future listAppVersionsAsync(ListAppVersionsRequest listAppVersionsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Lists your Resilience Hub applications.
*
*
*
* You can filter applications using only one filter at a time or without using any filter. If you try to filter
* applications using multiple filters, you will get the following error:
*
*
* An error occurred (ValidationException) when calling the ListApps operation: Only one filter is supported for this operation.
*
*
*
* @param listAppsRequest
* @return A Java Future containing the result of the ListApps operation returned by the service.
* @sample AWSResilienceHubAsync.ListApps
* @see AWS API
* Documentation
*/
java.util.concurrent.Future listAppsAsync(ListAppsRequest listAppsRequest);
/**
*
* Lists your Resilience Hub applications.
*
*
*
* You can filter applications using only one filter at a time or without using any filter. If you try to filter
* applications using multiple filters, you will get the following error:
*
*
* An error occurred (ValidationException) when calling the ListApps operation: Only one filter is supported for this operation.
*
*
*
* @param listAppsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListApps operation returned by the service.
* @sample AWSResilienceHubAsyncHandler.ListApps
* @see AWS API
* Documentation
*/
java.util.concurrent.Future listAppsAsync(ListAppsRequest listAppsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Lists the recommendation templates for the Resilience Hub applications.
*
*
* @param listRecommendationTemplatesRequest
* @return A Java Future containing the result of the ListRecommendationTemplates operation returned by the service.
* @sample AWSResilienceHubAsync.ListRecommendationTemplates
* @see AWS API Documentation
*/
java.util.concurrent.Future listRecommendationTemplatesAsync(
ListRecommendationTemplatesRequest listRecommendationTemplatesRequest);
/**
*
* Lists the recommendation templates for the Resilience Hub applications.
*
*
* @param listRecommendationTemplatesRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListRecommendationTemplates operation returned by the service.
* @sample AWSResilienceHubAsyncHandler.ListRecommendationTemplates
* @see AWS API Documentation
*/
java.util.concurrent.Future listRecommendationTemplatesAsync(
ListRecommendationTemplatesRequest listRecommendationTemplatesRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Lists the resiliency policies for the Resilience Hub applications.
*
*
* @param listResiliencyPoliciesRequest
* @return A Java Future containing the result of the ListResiliencyPolicies operation returned by the service.
* @sample AWSResilienceHubAsync.ListResiliencyPolicies
* @see AWS API Documentation
*/
java.util.concurrent.Future listResiliencyPoliciesAsync(ListResiliencyPoliciesRequest listResiliencyPoliciesRequest);
/**
*
* Lists the resiliency policies for the Resilience Hub applications.
*
*
* @param listResiliencyPoliciesRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListResiliencyPolicies operation returned by the service.
* @sample AWSResilienceHubAsyncHandler.ListResiliencyPolicies
* @see AWS API Documentation
*/
java.util.concurrent.Future listResiliencyPoliciesAsync(ListResiliencyPoliciesRequest listResiliencyPoliciesRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Lists the standard operating procedure (SOP) recommendations for the Resilience Hub applications.
*
*
* @param listSopRecommendationsRequest
* @return A Java Future containing the result of the ListSopRecommendations operation returned by the service.
* @sample AWSResilienceHubAsync.ListSopRecommendations
* @see AWS API Documentation
*/
java.util.concurrent.Future listSopRecommendationsAsync(ListSopRecommendationsRequest listSopRecommendationsRequest);
/**
*
* Lists the standard operating procedure (SOP) recommendations for the Resilience Hub applications.
*
*
* @param listSopRecommendationsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListSopRecommendations operation returned by the service.
* @sample AWSResilienceHubAsyncHandler.ListSopRecommendations
* @see AWS API Documentation
*/
java.util.concurrent.Future listSopRecommendationsAsync(ListSopRecommendationsRequest listSopRecommendationsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Lists the suggested resiliency policies for the Resilience Hub applications.
*
*
* @param listSuggestedResiliencyPoliciesRequest
* @return A Java Future containing the result of the ListSuggestedResiliencyPolicies operation returned by the
* service.
* @sample AWSResilienceHubAsync.ListSuggestedResiliencyPolicies
* @see AWS API Documentation
*/
java.util.concurrent.Future listSuggestedResiliencyPoliciesAsync(
ListSuggestedResiliencyPoliciesRequest listSuggestedResiliencyPoliciesRequest);
/**
*
* Lists the suggested resiliency policies for the Resilience Hub applications.
*
*
* @param listSuggestedResiliencyPoliciesRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListSuggestedResiliencyPolicies operation returned by the
* service.
* @sample AWSResilienceHubAsyncHandler.ListSuggestedResiliencyPolicies
* @see AWS API Documentation
*/
java.util.concurrent.Future listSuggestedResiliencyPoliciesAsync(
ListSuggestedResiliencyPoliciesRequest listSuggestedResiliencyPoliciesRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Lists the tags for your resources in your Resilience Hub applications.
*
*
* @param listTagsForResourceRequest
* @return A Java Future containing the result of the ListTagsForResource operation returned by the service.
* @sample AWSResilienceHubAsync.ListTagsForResource
* @see AWS API Documentation
*/
java.util.concurrent.Future listTagsForResourceAsync(ListTagsForResourceRequest listTagsForResourceRequest);
/**
*
* Lists the tags for your resources in your Resilience Hub applications.
*
*
* @param listTagsForResourceRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListTagsForResource operation returned by the service.
* @sample AWSResilienceHubAsyncHandler.ListTagsForResource
* @see AWS API Documentation
*/
java.util.concurrent.Future listTagsForResourceAsync(ListTagsForResourceRequest listTagsForResourceRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Lists the test recommendations for the Resilience Hub application.
*
*
* @param listTestRecommendationsRequest
* @return A Java Future containing the result of the ListTestRecommendations operation returned by the service.
* @sample AWSResilienceHubAsync.ListTestRecommendations
* @see AWS API Documentation
*/
java.util.concurrent.Future listTestRecommendationsAsync(ListTestRecommendationsRequest listTestRecommendationsRequest);
/**
*
* Lists the test recommendations for the Resilience Hub application.
*
*
* @param listTestRecommendationsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListTestRecommendations operation returned by the service.
* @sample AWSResilienceHubAsyncHandler.ListTestRecommendations
* @see AWS API Documentation
*/
java.util.concurrent.Future listTestRecommendationsAsync(ListTestRecommendationsRequest listTestRecommendationsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Lists the resources that are not currently supported in Resilience Hub. An unsupported resource is a resource
* that exists in the object that was used to create an app, but is not supported by Resilience Hub.
*
*
* @param listUnsupportedAppVersionResourcesRequest
* @return A Java Future containing the result of the ListUnsupportedAppVersionResources operation returned by the
* service.
* @sample AWSResilienceHubAsync.ListUnsupportedAppVersionResources
* @see AWS API Documentation
*/
java.util.concurrent.Future listUnsupportedAppVersionResourcesAsync(
ListUnsupportedAppVersionResourcesRequest listUnsupportedAppVersionResourcesRequest);
/**
*
* Lists the resources that are not currently supported in Resilience Hub. An unsupported resource is a resource
* that exists in the object that was used to create an app, but is not supported by Resilience Hub.
*
*
* @param listUnsupportedAppVersionResourcesRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListUnsupportedAppVersionResources operation returned by the
* service.
* @sample AWSResilienceHubAsyncHandler.ListUnsupportedAppVersionResources
* @see AWS API Documentation
*/
java.util.concurrent.Future listUnsupportedAppVersionResourcesAsync(
ListUnsupportedAppVersionResourcesRequest listUnsupportedAppVersionResourcesRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Publishes a new version of a specific Resilience Hub application.
*
*
* @param publishAppVersionRequest
* @return A Java Future containing the result of the PublishAppVersion operation returned by the service.
* @sample AWSResilienceHubAsync.PublishAppVersion
* @see AWS API Documentation
*/
java.util.concurrent.Future publishAppVersionAsync(PublishAppVersionRequest publishAppVersionRequest);
/**
*
* Publishes a new version of a specific Resilience Hub application.
*
*
* @param publishAppVersionRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the PublishAppVersion operation returned by the service.
* @sample AWSResilienceHubAsyncHandler.PublishAppVersion
* @see AWS API Documentation
*/
java.util.concurrent.Future publishAppVersionAsync(PublishAppVersionRequest publishAppVersionRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Adds or updates the app template for an Resilience Hub application draft version.
*
*
* @param putDraftAppVersionTemplateRequest
* @return A Java Future containing the result of the PutDraftAppVersionTemplate operation returned by the service.
* @sample AWSResilienceHubAsync.PutDraftAppVersionTemplate
* @see AWS API Documentation
*/
java.util.concurrent.Future putDraftAppVersionTemplateAsync(
PutDraftAppVersionTemplateRequest putDraftAppVersionTemplateRequest);
/**
*
* Adds or updates the app template for an Resilience Hub application draft version.
*
*
* @param putDraftAppVersionTemplateRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the PutDraftAppVersionTemplate operation returned by the service.
* @sample AWSResilienceHubAsyncHandler.PutDraftAppVersionTemplate
* @see AWS API Documentation
*/
java.util.concurrent.Future putDraftAppVersionTemplateAsync(
PutDraftAppVersionTemplateRequest putDraftAppVersionTemplateRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Removes resource mappings from a draft application version.
*
*
* @param removeDraftAppVersionResourceMappingsRequest
* @return A Java Future containing the result of the RemoveDraftAppVersionResourceMappings operation returned by
* the service.
* @sample AWSResilienceHubAsync.RemoveDraftAppVersionResourceMappings
* @see AWS API Documentation
*/
java.util.concurrent.Future removeDraftAppVersionResourceMappingsAsync(
RemoveDraftAppVersionResourceMappingsRequest removeDraftAppVersionResourceMappingsRequest);
/**
*
* Removes resource mappings from a draft application version.
*
*
* @param removeDraftAppVersionResourceMappingsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the RemoveDraftAppVersionResourceMappings operation returned by
* the service.
* @sample AWSResilienceHubAsyncHandler.RemoveDraftAppVersionResourceMappings
* @see AWS API Documentation
*/
java.util.concurrent.Future removeDraftAppVersionResourceMappingsAsync(
RemoveDraftAppVersionResourceMappingsRequest removeDraftAppVersionResourceMappingsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Resolves the resources for an application version.
*
*
* @param resolveAppVersionResourcesRequest
* @return A Java Future containing the result of the ResolveAppVersionResources operation returned by the service.
* @sample AWSResilienceHubAsync.ResolveAppVersionResources
* @see AWS API Documentation
*/
java.util.concurrent.Future resolveAppVersionResourcesAsync(
ResolveAppVersionResourcesRequest resolveAppVersionResourcesRequest);
/**
*
* Resolves the resources for an application version.
*
*
* @param resolveAppVersionResourcesRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ResolveAppVersionResources operation returned by the service.
* @sample AWSResilienceHubAsyncHandler.ResolveAppVersionResources
* @see AWS API Documentation
*/
java.util.concurrent.Future resolveAppVersionResourcesAsync(
ResolveAppVersionResourcesRequest resolveAppVersionResourcesRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates a new application assessment for an application.
*
*
* @param startAppAssessmentRequest
* @return A Java Future containing the result of the StartAppAssessment operation returned by the service.
* @sample AWSResilienceHubAsync.StartAppAssessment
* @see AWS API Documentation
*/
java.util.concurrent.Future startAppAssessmentAsync(StartAppAssessmentRequest startAppAssessmentRequest);
/**
*
* Creates a new application assessment for an application.
*
*
* @param startAppAssessmentRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the StartAppAssessment operation returned by the service.
* @sample AWSResilienceHubAsyncHandler.StartAppAssessment
* @see AWS API Documentation
*/
java.util.concurrent.Future startAppAssessmentAsync(StartAppAssessmentRequest startAppAssessmentRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Applies one or more tags to a resource.
*
*
* @param tagResourceRequest
* @return A Java Future containing the result of the TagResource operation returned by the service.
* @sample AWSResilienceHubAsync.TagResource
* @see AWS API
* Documentation
*/
java.util.concurrent.Future tagResourceAsync(TagResourceRequest tagResourceRequest);
/**
*
* Applies one or more tags to a resource.
*
*
* @param tagResourceRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the TagResource operation returned by the service.
* @sample AWSResilienceHubAsyncHandler.TagResource
* @see AWS API
* Documentation
*/
java.util.concurrent.Future tagResourceAsync(TagResourceRequest tagResourceRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Removes one or more tags from a resource.
*
*
* @param untagResourceRequest
* @return A Java Future containing the result of the UntagResource operation returned by the service.
* @sample AWSResilienceHubAsync.UntagResource
* @see AWS
* API Documentation
*/
java.util.concurrent.Future untagResourceAsync(UntagResourceRequest untagResourceRequest);
/**
*
* Removes one or more tags from a resource.
*
*
* @param untagResourceRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the UntagResource operation returned by the service.
* @sample AWSResilienceHubAsyncHandler.UntagResource
* @see AWS
* API Documentation
*/
java.util.concurrent.Future untagResourceAsync(UntagResourceRequest untagResourceRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Updates an application.
*
*
* @param updateAppRequest
* @return A Java Future containing the result of the UpdateApp operation returned by the service.
* @sample AWSResilienceHubAsync.UpdateApp
* @see AWS API
* Documentation
*/
java.util.concurrent.Future updateAppAsync(UpdateAppRequest updateAppRequest);
/**
*
* Updates an application.
*
*
* @param updateAppRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the UpdateApp operation returned by the service.
* @sample AWSResilienceHubAsyncHandler.UpdateApp
* @see AWS API
* Documentation
*/
java.util.concurrent.Future updateAppAsync(UpdateAppRequest updateAppRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Updates the Resilience Hub application version.
*
*
*
* This API updates the Resilience Hub application draft version. To use this information for running resiliency
* assessments, you must publish the Resilience Hub application using the PublishAppVersion
API.
*
*
*
* @param updateAppVersionRequest
* @return A Java Future containing the result of the UpdateAppVersion operation returned by the service.
* @sample AWSResilienceHubAsync.UpdateAppVersion
* @see AWS
* API Documentation
*/
java.util.concurrent.Future updateAppVersionAsync(UpdateAppVersionRequest updateAppVersionRequest);
/**
*
* Updates the Resilience Hub application version.
*
*
*
* This API updates the Resilience Hub application draft version. To use this information for running resiliency
* assessments, you must publish the Resilience Hub application using the PublishAppVersion
API.
*
*
*
* @param updateAppVersionRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the UpdateAppVersion operation returned by the service.
* @sample AWSResilienceHubAsyncHandler.UpdateAppVersion
* @see AWS
* API Documentation
*/
java.util.concurrent.Future updateAppVersionAsync(UpdateAppVersionRequest updateAppVersionRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Updates an existing Application Component in the Resilience Hub application.
*
*
*
* This API updates the Resilience Hub application draft version. To use this Application Component for running
* assessments, you must publish the Resilience Hub application using the PublishAppVersion
API.
*
*
*
* @param updateAppVersionAppComponentRequest
* @return A Java Future containing the result of the UpdateAppVersionAppComponent operation returned by the
* service.
* @sample AWSResilienceHubAsync.UpdateAppVersionAppComponent
* @see AWS API Documentation
*/
java.util.concurrent.Future updateAppVersionAppComponentAsync(
UpdateAppVersionAppComponentRequest updateAppVersionAppComponentRequest);
/**
*
* Updates an existing Application Component in the Resilience Hub application.
*
*
*
* This API updates the Resilience Hub application draft version. To use this Application Component for running
* assessments, you must publish the Resilience Hub application using the PublishAppVersion
API.
*
*
*
* @param updateAppVersionAppComponentRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the UpdateAppVersionAppComponent operation returned by the
* service.
* @sample AWSResilienceHubAsyncHandler.UpdateAppVersionAppComponent
* @see AWS API Documentation
*/
java.util.concurrent.Future updateAppVersionAppComponentAsync(
UpdateAppVersionAppComponentRequest updateAppVersionAppComponentRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Updates the resource details in the Resilience Hub application.
*
*
*
* -
*
* This action has no effect outside Resilience Hub.
*
*
* -
*
* This API updates the Resilience Hub application draft version. To use this resource for running resiliency
* assessments, you must publish the Resilience Hub application using the PublishAppVersion
API.
*
*
* -
*
* To update application version with new physicalResourceID
, you must call
* ResolveAppVersionResources
API.
*
*
*
*
*
* @param updateAppVersionResourceRequest
* @return A Java Future containing the result of the UpdateAppVersionResource operation returned by the service.
* @sample AWSResilienceHubAsync.UpdateAppVersionResource
* @see AWS API Documentation
*/
java.util.concurrent.Future updateAppVersionResourceAsync(UpdateAppVersionResourceRequest updateAppVersionResourceRequest);
/**
*
* Updates the resource details in the Resilience Hub application.
*
*
*
* -
*
* This action has no effect outside Resilience Hub.
*
*
* -
*
* This API updates the Resilience Hub application draft version. To use this resource for running resiliency
* assessments, you must publish the Resilience Hub application using the PublishAppVersion
API.
*
*
* -
*
* To update application version with new physicalResourceID
, you must call
* ResolveAppVersionResources
API.
*
*
*
*
*
* @param updateAppVersionResourceRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the UpdateAppVersionResource operation returned by the service.
* @sample AWSResilienceHubAsyncHandler.UpdateAppVersionResource
* @see AWS API Documentation
*/
java.util.concurrent.Future updateAppVersionResourceAsync(UpdateAppVersionResourceRequest updateAppVersionResourceRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Updates a resiliency policy.
*
*
*
* Resilience Hub allows you to provide a value of zero for rtoInSecs
and rpoInSecs
of
* your resiliency policy. But, while assessing your application, the lowest possible assessment result is near
* zero. Hence, if you provide value zero for rtoInSecs
and rpoInSecs
, the estimated
* workload RTO and estimated workload RPO result will be near zero and the Compliance status for your
* application will be set to Policy breached.
*
*
*
* @param updateResiliencyPolicyRequest
* @return A Java Future containing the result of the UpdateResiliencyPolicy operation returned by the service.
* @sample AWSResilienceHubAsync.UpdateResiliencyPolicy
* @see AWS API Documentation
*/
java.util.concurrent.Future updateResiliencyPolicyAsync(UpdateResiliencyPolicyRequest updateResiliencyPolicyRequest);
/**
*
* Updates a resiliency policy.
*
*
*
* Resilience Hub allows you to provide a value of zero for rtoInSecs
and rpoInSecs
of
* your resiliency policy. But, while assessing your application, the lowest possible assessment result is near
* zero. Hence, if you provide value zero for rtoInSecs
and rpoInSecs
, the estimated
* workload RTO and estimated workload RPO result will be near zero and the Compliance status for your
* application will be set to Policy breached.
*
*
*
* @param updateResiliencyPolicyRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the UpdateResiliencyPolicy operation returned by the service.
* @sample AWSResilienceHubAsyncHandler.UpdateResiliencyPolicy
* @see AWS API Documentation
*/
java.util.concurrent.Future updateResiliencyPolicyAsync(UpdateResiliencyPolicyRequest updateResiliencyPolicyRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
}