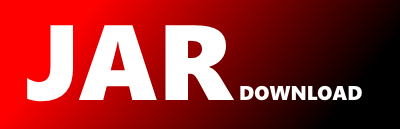
com.amazonaws.services.resiliencehub.model.ResourceMapping Maven / Gradle / Ivy
Show all versions of aws-java-sdk-resiliencehub Show documentation
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.resiliencehub.model;
import java.io.Serializable;
import javax.annotation.Generated;
import com.amazonaws.protocol.StructuredPojo;
import com.amazonaws.protocol.ProtocolMarshaller;
/**
*
* Defines a resource mapping.
*
*
* @see AWS API
* Documentation
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class ResourceMapping implements Serializable, Cloneable, StructuredPojo {
/**
*
* Name of the application this resource is mapped to when the mappingType
is
* AppRegistryApp
.
*
*/
private String appRegistryAppName;
/**
*
* Name of the Amazon Elastic Kubernetes Service cluster and namespace that this resource is mapped to when the
* mappingType
is EKS
.
*
*
*
* This parameter accepts values in "eks-cluster/namespace" format.
*
*
*/
private String eksSourceName;
/**
*
* Name of the CloudFormation stack this resource is mapped to when the mappingType
is
* CfnStack
.
*
*/
private String logicalStackName;
/**
*
* Specifies the type of resource mapping.
*
*/
private String mappingType;
/**
*
* Identifier of the physical resource.
*
*/
private PhysicalResourceId physicalResourceId;
/**
*
* Name of the Resource Groups that this resource is mapped to when the mappingType
is
* ResourceGroup
.
*
*/
private String resourceGroupName;
/**
*
* Name of the resource that this resource is mapped to when the mappingType
is Resource
.
*
*/
private String resourceName;
/**
*
* Name of the Terraform source that this resource is mapped to when the mappingType
is
* Terraform
.
*
*/
private String terraformSourceName;
/**
*
* Name of the application this resource is mapped to when the mappingType
is
* AppRegistryApp
.
*
*
* @param appRegistryAppName
* Name of the application this resource is mapped to when the mappingType
is
* AppRegistryApp
.
*/
public void setAppRegistryAppName(String appRegistryAppName) {
this.appRegistryAppName = appRegistryAppName;
}
/**
*
* Name of the application this resource is mapped to when the mappingType
is
* AppRegistryApp
.
*
*
* @return Name of the application this resource is mapped to when the mappingType
is
* AppRegistryApp
.
*/
public String getAppRegistryAppName() {
return this.appRegistryAppName;
}
/**
*
* Name of the application this resource is mapped to when the mappingType
is
* AppRegistryApp
.
*
*
* @param appRegistryAppName
* Name of the application this resource is mapped to when the mappingType
is
* AppRegistryApp
.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ResourceMapping withAppRegistryAppName(String appRegistryAppName) {
setAppRegistryAppName(appRegistryAppName);
return this;
}
/**
*
* Name of the Amazon Elastic Kubernetes Service cluster and namespace that this resource is mapped to when the
* mappingType
is EKS
.
*
*
*
* This parameter accepts values in "eks-cluster/namespace" format.
*
*
*
* @param eksSourceName
* Name of the Amazon Elastic Kubernetes Service cluster and namespace that this resource is mapped to when
* the mappingType
is EKS
.
*
* This parameter accepts values in "eks-cluster/namespace" format.
*
*/
public void setEksSourceName(String eksSourceName) {
this.eksSourceName = eksSourceName;
}
/**
*
* Name of the Amazon Elastic Kubernetes Service cluster and namespace that this resource is mapped to when the
* mappingType
is EKS
.
*
*
*
* This parameter accepts values in "eks-cluster/namespace" format.
*
*
*
* @return Name of the Amazon Elastic Kubernetes Service cluster and namespace that this resource is mapped to when
* the mappingType
is EKS
.
*
* This parameter accepts values in "eks-cluster/namespace" format.
*
*/
public String getEksSourceName() {
return this.eksSourceName;
}
/**
*
* Name of the Amazon Elastic Kubernetes Service cluster and namespace that this resource is mapped to when the
* mappingType
is EKS
.
*
*
*
* This parameter accepts values in "eks-cluster/namespace" format.
*
*
*
* @param eksSourceName
* Name of the Amazon Elastic Kubernetes Service cluster and namespace that this resource is mapped to when
* the mappingType
is EKS
.
*
* This parameter accepts values in "eks-cluster/namespace" format.
*
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ResourceMapping withEksSourceName(String eksSourceName) {
setEksSourceName(eksSourceName);
return this;
}
/**
*
* Name of the CloudFormation stack this resource is mapped to when the mappingType
is
* CfnStack
.
*
*
* @param logicalStackName
* Name of the CloudFormation stack this resource is mapped to when the mappingType
is
* CfnStack
.
*/
public void setLogicalStackName(String logicalStackName) {
this.logicalStackName = logicalStackName;
}
/**
*
* Name of the CloudFormation stack this resource is mapped to when the mappingType
is
* CfnStack
.
*
*
* @return Name of the CloudFormation stack this resource is mapped to when the mappingType
is
* CfnStack
.
*/
public String getLogicalStackName() {
return this.logicalStackName;
}
/**
*
* Name of the CloudFormation stack this resource is mapped to when the mappingType
is
* CfnStack
.
*
*
* @param logicalStackName
* Name of the CloudFormation stack this resource is mapped to when the mappingType
is
* CfnStack
.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ResourceMapping withLogicalStackName(String logicalStackName) {
setLogicalStackName(logicalStackName);
return this;
}
/**
*
* Specifies the type of resource mapping.
*
*
* @param mappingType
* Specifies the type of resource mapping.
* @see ResourceMappingType
*/
public void setMappingType(String mappingType) {
this.mappingType = mappingType;
}
/**
*
* Specifies the type of resource mapping.
*
*
* @return Specifies the type of resource mapping.
* @see ResourceMappingType
*/
public String getMappingType() {
return this.mappingType;
}
/**
*
* Specifies the type of resource mapping.
*
*
* @param mappingType
* Specifies the type of resource mapping.
* @return Returns a reference to this object so that method calls can be chained together.
* @see ResourceMappingType
*/
public ResourceMapping withMappingType(String mappingType) {
setMappingType(mappingType);
return this;
}
/**
*
* Specifies the type of resource mapping.
*
*
* @param mappingType
* Specifies the type of resource mapping.
* @return Returns a reference to this object so that method calls can be chained together.
* @see ResourceMappingType
*/
public ResourceMapping withMappingType(ResourceMappingType mappingType) {
this.mappingType = mappingType.toString();
return this;
}
/**
*
* Identifier of the physical resource.
*
*
* @param physicalResourceId
* Identifier of the physical resource.
*/
public void setPhysicalResourceId(PhysicalResourceId physicalResourceId) {
this.physicalResourceId = physicalResourceId;
}
/**
*
* Identifier of the physical resource.
*
*
* @return Identifier of the physical resource.
*/
public PhysicalResourceId getPhysicalResourceId() {
return this.physicalResourceId;
}
/**
*
* Identifier of the physical resource.
*
*
* @param physicalResourceId
* Identifier of the physical resource.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ResourceMapping withPhysicalResourceId(PhysicalResourceId physicalResourceId) {
setPhysicalResourceId(physicalResourceId);
return this;
}
/**
*
* Name of the Resource Groups that this resource is mapped to when the mappingType
is
* ResourceGroup
.
*
*
* @param resourceGroupName
* Name of the Resource Groups that this resource is mapped to when the mappingType
is
* ResourceGroup
.
*/
public void setResourceGroupName(String resourceGroupName) {
this.resourceGroupName = resourceGroupName;
}
/**
*
* Name of the Resource Groups that this resource is mapped to when the mappingType
is
* ResourceGroup
.
*
*
* @return Name of the Resource Groups that this resource is mapped to when the mappingType
is
* ResourceGroup
.
*/
public String getResourceGroupName() {
return this.resourceGroupName;
}
/**
*
* Name of the Resource Groups that this resource is mapped to when the mappingType
is
* ResourceGroup
.
*
*
* @param resourceGroupName
* Name of the Resource Groups that this resource is mapped to when the mappingType
is
* ResourceGroup
.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ResourceMapping withResourceGroupName(String resourceGroupName) {
setResourceGroupName(resourceGroupName);
return this;
}
/**
*
* Name of the resource that this resource is mapped to when the mappingType
is Resource
.
*
*
* @param resourceName
* Name of the resource that this resource is mapped to when the mappingType
is
* Resource
.
*/
public void setResourceName(String resourceName) {
this.resourceName = resourceName;
}
/**
*
* Name of the resource that this resource is mapped to when the mappingType
is Resource
.
*
*
* @return Name of the resource that this resource is mapped to when the mappingType
is
* Resource
.
*/
public String getResourceName() {
return this.resourceName;
}
/**
*
* Name of the resource that this resource is mapped to when the mappingType
is Resource
.
*
*
* @param resourceName
* Name of the resource that this resource is mapped to when the mappingType
is
* Resource
.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ResourceMapping withResourceName(String resourceName) {
setResourceName(resourceName);
return this;
}
/**
*
* Name of the Terraform source that this resource is mapped to when the mappingType
is
* Terraform
.
*
*
* @param terraformSourceName
* Name of the Terraform source that this resource is mapped to when the mappingType
is
* Terraform
.
*/
public void setTerraformSourceName(String terraformSourceName) {
this.terraformSourceName = terraformSourceName;
}
/**
*
* Name of the Terraform source that this resource is mapped to when the mappingType
is
* Terraform
.
*
*
* @return Name of the Terraform source that this resource is mapped to when the mappingType
is
* Terraform
.
*/
public String getTerraformSourceName() {
return this.terraformSourceName;
}
/**
*
* Name of the Terraform source that this resource is mapped to when the mappingType
is
* Terraform
.
*
*
* @param terraformSourceName
* Name of the Terraform source that this resource is mapped to when the mappingType
is
* Terraform
.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ResourceMapping withTerraformSourceName(String terraformSourceName) {
setTerraformSourceName(terraformSourceName);
return this;
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getAppRegistryAppName() != null)
sb.append("AppRegistryAppName: ").append(getAppRegistryAppName()).append(",");
if (getEksSourceName() != null)
sb.append("EksSourceName: ").append(getEksSourceName()).append(",");
if (getLogicalStackName() != null)
sb.append("LogicalStackName: ").append(getLogicalStackName()).append(",");
if (getMappingType() != null)
sb.append("MappingType: ").append(getMappingType()).append(",");
if (getPhysicalResourceId() != null)
sb.append("PhysicalResourceId: ").append(getPhysicalResourceId()).append(",");
if (getResourceGroupName() != null)
sb.append("ResourceGroupName: ").append(getResourceGroupName()).append(",");
if (getResourceName() != null)
sb.append("ResourceName: ").append(getResourceName()).append(",");
if (getTerraformSourceName() != null)
sb.append("TerraformSourceName: ").append(getTerraformSourceName());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof ResourceMapping == false)
return false;
ResourceMapping other = (ResourceMapping) obj;
if (other.getAppRegistryAppName() == null ^ this.getAppRegistryAppName() == null)
return false;
if (other.getAppRegistryAppName() != null && other.getAppRegistryAppName().equals(this.getAppRegistryAppName()) == false)
return false;
if (other.getEksSourceName() == null ^ this.getEksSourceName() == null)
return false;
if (other.getEksSourceName() != null && other.getEksSourceName().equals(this.getEksSourceName()) == false)
return false;
if (other.getLogicalStackName() == null ^ this.getLogicalStackName() == null)
return false;
if (other.getLogicalStackName() != null && other.getLogicalStackName().equals(this.getLogicalStackName()) == false)
return false;
if (other.getMappingType() == null ^ this.getMappingType() == null)
return false;
if (other.getMappingType() != null && other.getMappingType().equals(this.getMappingType()) == false)
return false;
if (other.getPhysicalResourceId() == null ^ this.getPhysicalResourceId() == null)
return false;
if (other.getPhysicalResourceId() != null && other.getPhysicalResourceId().equals(this.getPhysicalResourceId()) == false)
return false;
if (other.getResourceGroupName() == null ^ this.getResourceGroupName() == null)
return false;
if (other.getResourceGroupName() != null && other.getResourceGroupName().equals(this.getResourceGroupName()) == false)
return false;
if (other.getResourceName() == null ^ this.getResourceName() == null)
return false;
if (other.getResourceName() != null && other.getResourceName().equals(this.getResourceName()) == false)
return false;
if (other.getTerraformSourceName() == null ^ this.getTerraformSourceName() == null)
return false;
if (other.getTerraformSourceName() != null && other.getTerraformSourceName().equals(this.getTerraformSourceName()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getAppRegistryAppName() == null) ? 0 : getAppRegistryAppName().hashCode());
hashCode = prime * hashCode + ((getEksSourceName() == null) ? 0 : getEksSourceName().hashCode());
hashCode = prime * hashCode + ((getLogicalStackName() == null) ? 0 : getLogicalStackName().hashCode());
hashCode = prime * hashCode + ((getMappingType() == null) ? 0 : getMappingType().hashCode());
hashCode = prime * hashCode + ((getPhysicalResourceId() == null) ? 0 : getPhysicalResourceId().hashCode());
hashCode = prime * hashCode + ((getResourceGroupName() == null) ? 0 : getResourceGroupName().hashCode());
hashCode = prime * hashCode + ((getResourceName() == null) ? 0 : getResourceName().hashCode());
hashCode = prime * hashCode + ((getTerraformSourceName() == null) ? 0 : getTerraformSourceName().hashCode());
return hashCode;
}
@Override
public ResourceMapping clone() {
try {
return (ResourceMapping) super.clone();
} catch (CloneNotSupportedException e) {
throw new IllegalStateException("Got a CloneNotSupportedException from Object.clone() " + "even though we're Cloneable!", e);
}
}
@com.amazonaws.annotation.SdkInternalApi
@Override
public void marshall(ProtocolMarshaller protocolMarshaller) {
com.amazonaws.services.resiliencehub.model.transform.ResourceMappingMarshaller.getInstance().marshall(this, protocolMarshaller);
}
}