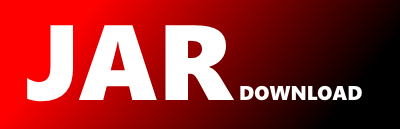
com.amazonaws.services.resiliencehub.AWSResilienceHubClient Maven / Gradle / Ivy
Show all versions of aws-java-sdk-resiliencehub Show documentation
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.resiliencehub;
import org.w3c.dom.*;
import java.net.*;
import java.util.*;
import javax.annotation.Generated;
import org.apache.commons.logging.*;
import com.amazonaws.*;
import com.amazonaws.annotation.SdkInternalApi;
import com.amazonaws.auth.*;
import com.amazonaws.handlers.*;
import com.amazonaws.http.*;
import com.amazonaws.internal.*;
import com.amazonaws.internal.auth.*;
import com.amazonaws.metrics.*;
import com.amazonaws.regions.*;
import com.amazonaws.transform.*;
import com.amazonaws.util.*;
import com.amazonaws.protocol.json.*;
import com.amazonaws.util.AWSRequestMetrics.Field;
import com.amazonaws.annotation.ThreadSafe;
import com.amazonaws.client.AwsSyncClientParams;
import com.amazonaws.client.builder.AdvancedConfig;
import com.amazonaws.services.resiliencehub.AWSResilienceHubClientBuilder;
import com.amazonaws.AmazonServiceException;
import com.amazonaws.services.resiliencehub.model.*;
import com.amazonaws.services.resiliencehub.model.transform.*;
/**
* Client for accessing AWS Resilience Hub. All service calls made using this client are blocking, and will not return
* until the service call completes.
*
*
* Resilience Hub helps you proactively prepare and protect your Amazon Web Services applications from disruptions. It
* offers continual resiliency assessment and validation that integrates into your software development lifecycle. This
* enables you to uncover resiliency weaknesses, ensure recovery time objective (RTO) and recovery point objective (RPO)
* targets for your applications are met, and resolve issues before they are released into production.
*
*/
@ThreadSafe
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class AWSResilienceHubClient extends AmazonWebServiceClient implements AWSResilienceHub {
/** Provider for AWS credentials. */
private final AWSCredentialsProvider awsCredentialsProvider;
private static final Log log = LogFactory.getLog(AWSResilienceHub.class);
/** Default signing name for the service. */
private static final String DEFAULT_SIGNING_NAME = "resiliencehub";
/** Client configuration factory providing ClientConfigurations tailored to this client */
protected static final ClientConfigurationFactory configFactory = new ClientConfigurationFactory();
private final AdvancedConfig advancedConfig;
private static final com.amazonaws.protocol.json.SdkJsonProtocolFactory protocolFactory = new com.amazonaws.protocol.json.SdkJsonProtocolFactory(
new JsonClientMetadata()
.withProtocolVersion("1.1")
.withSupportsCbor(false)
.withSupportsIon(false)
.withContentTypeOverride("application/json")
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("ServiceQuotaExceededException").withExceptionUnmarshaller(
com.amazonaws.services.resiliencehub.model.transform.ServiceQuotaExceededExceptionUnmarshaller.getInstance()))
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("InternalServerException").withExceptionUnmarshaller(
com.amazonaws.services.resiliencehub.model.transform.InternalServerExceptionUnmarshaller.getInstance()))
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("ResourceNotFoundException").withExceptionUnmarshaller(
com.amazonaws.services.resiliencehub.model.transform.ResourceNotFoundExceptionUnmarshaller.getInstance()))
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("ValidationException").withExceptionUnmarshaller(
com.amazonaws.services.resiliencehub.model.transform.ValidationExceptionUnmarshaller.getInstance()))
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("ThrottlingException").withExceptionUnmarshaller(
com.amazonaws.services.resiliencehub.model.transform.ThrottlingExceptionUnmarshaller.getInstance()))
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("AccessDeniedException").withExceptionUnmarshaller(
com.amazonaws.services.resiliencehub.model.transform.AccessDeniedExceptionUnmarshaller.getInstance()))
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("ConflictException").withExceptionUnmarshaller(
com.amazonaws.services.resiliencehub.model.transform.ConflictExceptionUnmarshaller.getInstance()))
.withBaseServiceExceptionClass(com.amazonaws.services.resiliencehub.model.AWSResilienceHubException.class));
public static AWSResilienceHubClientBuilder builder() {
return AWSResilienceHubClientBuilder.standard();
}
/**
* Constructs a new client to invoke service methods on AWS Resilience Hub using the specified parameters.
*
*
* All service calls made using this new client object are blocking, and will not return until the service call
* completes.
*
* @param clientParams
* Object providing client parameters.
*/
AWSResilienceHubClient(AwsSyncClientParams clientParams) {
this(clientParams, false);
}
/**
* Constructs a new client to invoke service methods on AWS Resilience Hub using the specified parameters.
*
*
* All service calls made using this new client object are blocking, and will not return until the service call
* completes.
*
* @param clientParams
* Object providing client parameters.
*/
AWSResilienceHubClient(AwsSyncClientParams clientParams, boolean endpointDiscoveryEnabled) {
super(clientParams);
this.awsCredentialsProvider = clientParams.getCredentialsProvider();
this.advancedConfig = clientParams.getAdvancedConfig();
init();
}
private void init() {
setServiceNameIntern(DEFAULT_SIGNING_NAME);
setEndpointPrefix(ENDPOINT_PREFIX);
// calling this.setEndPoint(...) will also modify the signer accordingly
setEndpoint("resiliencehub.us-east-1.amazonaws.com");
HandlerChainFactory chainFactory = new HandlerChainFactory();
requestHandler2s.addAll(chainFactory.newRequestHandlerChain("/com/amazonaws/services/resiliencehub/request.handlers"));
requestHandler2s.addAll(chainFactory.newRequestHandler2Chain("/com/amazonaws/services/resiliencehub/request.handler2s"));
requestHandler2s.addAll(chainFactory.getGlobalHandlers());
}
/**
*
* Adds the source of resource-maps to the draft version of an application. During assessment, Resilience Hub will
* use these resource-maps to resolve the latest physical ID for each resource in the application template. For more
* information about different types of resources suported by Resilience Hub and how to add them in your
* application, see Step
* 2: How is your application managed? in the Resilience Hub User Guide.
*
*
* @param addDraftAppVersionResourceMappingsRequest
* @return Result of the AddDraftAppVersionResourceMappings operation returned by the service.
* @throws InternalServerException
* This exception occurs when there is an internal failure in the Resilience Hub service.
* @throws ResourceNotFoundException
* This exception occurs when the specified resource could not be found.
* @throws ConflictException
* This exception occurs when a conflict with a previous successful write is detected. This generally occurs
* when the previous write did not have time to propagate to the host serving the current request. A retry
* (with appropriate backoff logic) is the recommended response to this exception.
* @throws ThrottlingException
* This exception occurs when you have exceeded the limit on the number of requests per second.
* @throws ValidationException
* This exception occurs when a request is not valid.
* @throws AccessDeniedException
* You don't have permissions to perform the requested operation. The user or role that is making the
* request must have at least one IAM permissions policy attached that grants the required permissions.
* @sample AWSResilienceHub.AddDraftAppVersionResourceMappings
* @see AWS API Documentation
*/
@Override
public AddDraftAppVersionResourceMappingsResult addDraftAppVersionResourceMappings(AddDraftAppVersionResourceMappingsRequest request) {
request = beforeClientExecution(request);
return executeAddDraftAppVersionResourceMappings(request);
}
@SdkInternalApi
final AddDraftAppVersionResourceMappingsResult executeAddDraftAppVersionResourceMappings(
AddDraftAppVersionResourceMappingsRequest addDraftAppVersionResourceMappingsRequest) {
ExecutionContext executionContext = createExecutionContext(addDraftAppVersionResourceMappingsRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new AddDraftAppVersionResourceMappingsRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(addDraftAppVersionResourceMappingsRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "resiliencehub");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "AddDraftAppVersionResourceMappings");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new AddDraftAppVersionResourceMappingsResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Enables you to include or exclude one or more operational recommendations.
*
*
* @param batchUpdateRecommendationStatusRequest
* @return Result of the BatchUpdateRecommendationStatus operation returned by the service.
* @throws InternalServerException
* This exception occurs when there is an internal failure in the Resilience Hub service.
* @throws ResourceNotFoundException
* This exception occurs when the specified resource could not be found.
* @throws ThrottlingException
* This exception occurs when you have exceeded the limit on the number of requests per second.
* @throws ValidationException
* This exception occurs when a request is not valid.
* @throws AccessDeniedException
* You don't have permissions to perform the requested operation. The user or role that is making the
* request must have at least one IAM permissions policy attached that grants the required permissions.
* @sample AWSResilienceHub.BatchUpdateRecommendationStatus
* @see AWS API Documentation
*/
@Override
public BatchUpdateRecommendationStatusResult batchUpdateRecommendationStatus(BatchUpdateRecommendationStatusRequest request) {
request = beforeClientExecution(request);
return executeBatchUpdateRecommendationStatus(request);
}
@SdkInternalApi
final BatchUpdateRecommendationStatusResult executeBatchUpdateRecommendationStatus(
BatchUpdateRecommendationStatusRequest batchUpdateRecommendationStatusRequest) {
ExecutionContext executionContext = createExecutionContext(batchUpdateRecommendationStatusRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new BatchUpdateRecommendationStatusRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(batchUpdateRecommendationStatusRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "resiliencehub");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "BatchUpdateRecommendationStatus");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new BatchUpdateRecommendationStatusResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Creates an Resilience Hub application. An Resilience Hub application is a collection of Amazon Web Services
* resources structured to prevent and recover Amazon Web Services application disruptions. To describe a Resilience
* Hub application, you provide an application name, resources from one or more CloudFormation stacks, Resource
* Groups, Terraform state files, AppRegistry applications, and an appropriate resiliency policy. In addition, you
* can also add resources that are located on Amazon Elastic Kubernetes Service (Amazon EKS) clusters as optional
* resources. For more information about the number of resources supported per application, see Service quotas.
*
*
* After you create an Resilience Hub application, you publish it so that you can run a resiliency assessment on it.
* You can then use recommendations from the assessment to improve resiliency by running another assessment,
* comparing results, and then iterating the process until you achieve your goals for recovery time objective (RTO)
* and recovery point objective (RPO).
*
*
* @param createAppRequest
* @return Result of the CreateApp operation returned by the service.
* @throws InternalServerException
* This exception occurs when there is an internal failure in the Resilience Hub service.
* @throws ResourceNotFoundException
* This exception occurs when the specified resource could not be found.
* @throws ConflictException
* This exception occurs when a conflict with a previous successful write is detected. This generally occurs
* when the previous write did not have time to propagate to the host serving the current request. A retry
* (with appropriate backoff logic) is the recommended response to this exception.
* @throws ServiceQuotaExceededException
* This exception occurs when you have exceeded your service quota. To perform the requested action, remove
* some of the relevant resources, or use Service Quotas to request a service quota increase.
* @throws ThrottlingException
* This exception occurs when you have exceeded the limit on the number of requests per second.
* @throws ValidationException
* This exception occurs when a request is not valid.
* @throws AccessDeniedException
* You don't have permissions to perform the requested operation. The user or role that is making the
* request must have at least one IAM permissions policy attached that grants the required permissions.
* @sample AWSResilienceHub.CreateApp
* @see AWS API
* Documentation
*/
@Override
public CreateAppResult createApp(CreateAppRequest request) {
request = beforeClientExecution(request);
return executeCreateApp(request);
}
@SdkInternalApi
final CreateAppResult executeCreateApp(CreateAppRequest createAppRequest) {
ExecutionContext executionContext = createExecutionContext(createAppRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new CreateAppRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(createAppRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "resiliencehub");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "CreateApp");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(new JsonOperationMetadata()
.withPayloadJson(true).withHasStreamingSuccessResponse(false), new CreateAppResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Creates a new Application Component in the Resilience Hub application.
*
*
*
* This API updates the Resilience Hub application draft version. To use this Application Component for running
* assessments, you must publish the Resilience Hub application using the PublishAppVersion
API.
*
*
*
* @param createAppVersionAppComponentRequest
* @return Result of the CreateAppVersionAppComponent operation returned by the service.
* @throws InternalServerException
* This exception occurs when there is an internal failure in the Resilience Hub service.
* @throws ResourceNotFoundException
* This exception occurs when the specified resource could not be found.
* @throws ConflictException
* This exception occurs when a conflict with a previous successful write is detected. This generally occurs
* when the previous write did not have time to propagate to the host serving the current request. A retry
* (with appropriate backoff logic) is the recommended response to this exception.
* @throws ServiceQuotaExceededException
* This exception occurs when you have exceeded your service quota. To perform the requested action, remove
* some of the relevant resources, or use Service Quotas to request a service quota increase.
* @throws ThrottlingException
* This exception occurs when you have exceeded the limit on the number of requests per second.
* @throws ValidationException
* This exception occurs when a request is not valid.
* @throws AccessDeniedException
* You don't have permissions to perform the requested operation. The user or role that is making the
* request must have at least one IAM permissions policy attached that grants the required permissions.
* @sample AWSResilienceHub.CreateAppVersionAppComponent
* @see AWS API Documentation
*/
@Override
public CreateAppVersionAppComponentResult createAppVersionAppComponent(CreateAppVersionAppComponentRequest request) {
request = beforeClientExecution(request);
return executeCreateAppVersionAppComponent(request);
}
@SdkInternalApi
final CreateAppVersionAppComponentResult executeCreateAppVersionAppComponent(CreateAppVersionAppComponentRequest createAppVersionAppComponentRequest) {
ExecutionContext executionContext = createExecutionContext(createAppVersionAppComponentRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new CreateAppVersionAppComponentRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(createAppVersionAppComponentRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "resiliencehub");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "CreateAppVersionAppComponent");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new CreateAppVersionAppComponentResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Adds a resource to the Resilience Hub application and assigns it to the specified Application Components. If you
* specify a new Application Component, Resilience Hub will automatically create the Application Component.
*
*
*
* -
*
* This action has no effect outside Resilience Hub.
*
*
* -
*
* This API updates the Resilience Hub application draft version. To use this resource for running resiliency
* assessments, you must publish the Resilience Hub application using the PublishAppVersion
API.
*
*
* -
*
* To update application version with new physicalResourceID
, you must call
* ResolveAppVersionResources
API.
*
*
*
*
*
* @param createAppVersionResourceRequest
* @return Result of the CreateAppVersionResource operation returned by the service.
* @throws InternalServerException
* This exception occurs when there is an internal failure in the Resilience Hub service.
* @throws ResourceNotFoundException
* This exception occurs when the specified resource could not be found.
* @throws ConflictException
* This exception occurs when a conflict with a previous successful write is detected. This generally occurs
* when the previous write did not have time to propagate to the host serving the current request. A retry
* (with appropriate backoff logic) is the recommended response to this exception.
* @throws ServiceQuotaExceededException
* This exception occurs when you have exceeded your service quota. To perform the requested action, remove
* some of the relevant resources, or use Service Quotas to request a service quota increase.
* @throws ThrottlingException
* This exception occurs when you have exceeded the limit on the number of requests per second.
* @throws ValidationException
* This exception occurs when a request is not valid.
* @throws AccessDeniedException
* You don't have permissions to perform the requested operation. The user or role that is making the
* request must have at least one IAM permissions policy attached that grants the required permissions.
* @sample AWSResilienceHub.CreateAppVersionResource
* @see AWS API Documentation
*/
@Override
public CreateAppVersionResourceResult createAppVersionResource(CreateAppVersionResourceRequest request) {
request = beforeClientExecution(request);
return executeCreateAppVersionResource(request);
}
@SdkInternalApi
final CreateAppVersionResourceResult executeCreateAppVersionResource(CreateAppVersionResourceRequest createAppVersionResourceRequest) {
ExecutionContext executionContext = createExecutionContext(createAppVersionResourceRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new CreateAppVersionResourceRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(createAppVersionResourceRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "resiliencehub");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "CreateAppVersionResource");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new CreateAppVersionResourceResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Creates a new recommendation template for the Resilience Hub application.
*
*
* @param createRecommendationTemplateRequest
* @return Result of the CreateRecommendationTemplate operation returned by the service.
* @throws InternalServerException
* This exception occurs when there is an internal failure in the Resilience Hub service.
* @throws ResourceNotFoundException
* This exception occurs when the specified resource could not be found.
* @throws ConflictException
* This exception occurs when a conflict with a previous successful write is detected. This generally occurs
* when the previous write did not have time to propagate to the host serving the current request. A retry
* (with appropriate backoff logic) is the recommended response to this exception.
* @throws ServiceQuotaExceededException
* This exception occurs when you have exceeded your service quota. To perform the requested action, remove
* some of the relevant resources, or use Service Quotas to request a service quota increase.
* @throws ThrottlingException
* This exception occurs when you have exceeded the limit on the number of requests per second.
* @throws ValidationException
* This exception occurs when a request is not valid.
* @throws AccessDeniedException
* You don't have permissions to perform the requested operation. The user or role that is making the
* request must have at least one IAM permissions policy attached that grants the required permissions.
* @sample AWSResilienceHub.CreateRecommendationTemplate
* @see AWS API Documentation
*/
@Override
public CreateRecommendationTemplateResult createRecommendationTemplate(CreateRecommendationTemplateRequest request) {
request = beforeClientExecution(request);
return executeCreateRecommendationTemplate(request);
}
@SdkInternalApi
final CreateRecommendationTemplateResult executeCreateRecommendationTemplate(CreateRecommendationTemplateRequest createRecommendationTemplateRequest) {
ExecutionContext executionContext = createExecutionContext(createRecommendationTemplateRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new CreateRecommendationTemplateRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(createRecommendationTemplateRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "resiliencehub");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "CreateRecommendationTemplate");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new CreateRecommendationTemplateResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Creates a resiliency policy for an application.
*
*
*
* Resilience Hub allows you to provide a value of zero for rtoInSecs
and rpoInSecs
of
* your resiliency policy. But, while assessing your application, the lowest possible assessment result is near
* zero. Hence, if you provide value zero for rtoInSecs
and rpoInSecs
, the estimated
* workload RTO and estimated workload RPO result will be near zero and the Compliance status for your
* application will be set to Policy breached.
*
*
*
* @param createResiliencyPolicyRequest
* @return Result of the CreateResiliencyPolicy operation returned by the service.
* @throws InternalServerException
* This exception occurs when there is an internal failure in the Resilience Hub service.
* @throws ConflictException
* This exception occurs when a conflict with a previous successful write is detected. This generally occurs
* when the previous write did not have time to propagate to the host serving the current request. A retry
* (with appropriate backoff logic) is the recommended response to this exception.
* @throws ServiceQuotaExceededException
* This exception occurs when you have exceeded your service quota. To perform the requested action, remove
* some of the relevant resources, or use Service Quotas to request a service quota increase.
* @throws ThrottlingException
* This exception occurs when you have exceeded the limit on the number of requests per second.
* @throws ValidationException
* This exception occurs when a request is not valid.
* @throws AccessDeniedException
* You don't have permissions to perform the requested operation. The user or role that is making the
* request must have at least one IAM permissions policy attached that grants the required permissions.
* @sample AWSResilienceHub.CreateResiliencyPolicy
* @see AWS API Documentation
*/
@Override
public CreateResiliencyPolicyResult createResiliencyPolicy(CreateResiliencyPolicyRequest request) {
request = beforeClientExecution(request);
return executeCreateResiliencyPolicy(request);
}
@SdkInternalApi
final CreateResiliencyPolicyResult executeCreateResiliencyPolicy(CreateResiliencyPolicyRequest createResiliencyPolicyRequest) {
ExecutionContext executionContext = createExecutionContext(createResiliencyPolicyRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new CreateResiliencyPolicyRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(createResiliencyPolicyRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "resiliencehub");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "CreateResiliencyPolicy");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new CreateResiliencyPolicyResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Deletes an Resilience Hub application. This is a destructive action that can't be undone.
*
*
* @param deleteAppRequest
* @return Result of the DeleteApp operation returned by the service.
* @throws InternalServerException
* This exception occurs when there is an internal failure in the Resilience Hub service.
* @throws ResourceNotFoundException
* This exception occurs when the specified resource could not be found.
* @throws ConflictException
* This exception occurs when a conflict with a previous successful write is detected. This generally occurs
* when the previous write did not have time to propagate to the host serving the current request. A retry
* (with appropriate backoff logic) is the recommended response to this exception.
* @throws ThrottlingException
* This exception occurs when you have exceeded the limit on the number of requests per second.
* @throws ValidationException
* This exception occurs when a request is not valid.
* @sample AWSResilienceHub.DeleteApp
* @see AWS API
* Documentation
*/
@Override
public DeleteAppResult deleteApp(DeleteAppRequest request) {
request = beforeClientExecution(request);
return executeDeleteApp(request);
}
@SdkInternalApi
final DeleteAppResult executeDeleteApp(DeleteAppRequest deleteAppRequest) {
ExecutionContext executionContext = createExecutionContext(deleteAppRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DeleteAppRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(deleteAppRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "resiliencehub");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DeleteApp");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(new JsonOperationMetadata()
.withPayloadJson(true).withHasStreamingSuccessResponse(false), new DeleteAppResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Deletes an Resilience Hub application assessment. This is a destructive action that can't be undone.
*
*
* @param deleteAppAssessmentRequest
* @return Result of the DeleteAppAssessment operation returned by the service.
* @throws InternalServerException
* This exception occurs when there is an internal failure in the Resilience Hub service.
* @throws ResourceNotFoundException
* This exception occurs when the specified resource could not be found.
* @throws ConflictException
* This exception occurs when a conflict with a previous successful write is detected. This generally occurs
* when the previous write did not have time to propagate to the host serving the current request. A retry
* (with appropriate backoff logic) is the recommended response to this exception.
* @throws ThrottlingException
* This exception occurs when you have exceeded the limit on the number of requests per second.
* @throws ValidationException
* This exception occurs when a request is not valid.
* @throws AccessDeniedException
* You don't have permissions to perform the requested operation. The user or role that is making the
* request must have at least one IAM permissions policy attached that grants the required permissions.
* @sample AWSResilienceHub.DeleteAppAssessment
* @see AWS API Documentation
*/
@Override
public DeleteAppAssessmentResult deleteAppAssessment(DeleteAppAssessmentRequest request) {
request = beforeClientExecution(request);
return executeDeleteAppAssessment(request);
}
@SdkInternalApi
final DeleteAppAssessmentResult executeDeleteAppAssessment(DeleteAppAssessmentRequest deleteAppAssessmentRequest) {
ExecutionContext executionContext = createExecutionContext(deleteAppAssessmentRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DeleteAppAssessmentRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(deleteAppAssessmentRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "resiliencehub");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DeleteAppAssessment");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new DeleteAppAssessmentResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Deletes the input source and all of its imported resources from the Resilience Hub application.
*
*
* @param deleteAppInputSourceRequest
* @return Result of the DeleteAppInputSource operation returned by the service.
* @throws InternalServerException
* This exception occurs when there is an internal failure in the Resilience Hub service.
* @throws ResourceNotFoundException
* This exception occurs when the specified resource could not be found.
* @throws ConflictException
* This exception occurs when a conflict with a previous successful write is detected. This generally occurs
* when the previous write did not have time to propagate to the host serving the current request. A retry
* (with appropriate backoff logic) is the recommended response to this exception.
* @throws ThrottlingException
* This exception occurs when you have exceeded the limit on the number of requests per second.
* @throws ValidationException
* This exception occurs when a request is not valid.
* @throws AccessDeniedException
* You don't have permissions to perform the requested operation. The user or role that is making the
* request must have at least one IAM permissions policy attached that grants the required permissions.
* @sample AWSResilienceHub.DeleteAppInputSource
* @see AWS API Documentation
*/
@Override
public DeleteAppInputSourceResult deleteAppInputSource(DeleteAppInputSourceRequest request) {
request = beforeClientExecution(request);
return executeDeleteAppInputSource(request);
}
@SdkInternalApi
final DeleteAppInputSourceResult executeDeleteAppInputSource(DeleteAppInputSourceRequest deleteAppInputSourceRequest) {
ExecutionContext executionContext = createExecutionContext(deleteAppInputSourceRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DeleteAppInputSourceRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(deleteAppInputSourceRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "resiliencehub");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DeleteAppInputSource");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new DeleteAppInputSourceResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Deletes an Application Component from the Resilience Hub application.
*
*
*
* -
*
* This API updates the Resilience Hub application draft version. To use this Application Component for running
* assessments, you must publish the Resilience Hub application using the PublishAppVersion
API.
*
*
* -
*
* You will not be able to delete an Application Component if it has resources associated with it.
*
*
*
*
*
* @param deleteAppVersionAppComponentRequest
* @return Result of the DeleteAppVersionAppComponent operation returned by the service.
* @throws InternalServerException
* This exception occurs when there is an internal failure in the Resilience Hub service.
* @throws ResourceNotFoundException
* This exception occurs when the specified resource could not be found.
* @throws ConflictException
* This exception occurs when a conflict with a previous successful write is detected. This generally occurs
* when the previous write did not have time to propagate to the host serving the current request. A retry
* (with appropriate backoff logic) is the recommended response to this exception.
* @throws ThrottlingException
* This exception occurs when you have exceeded the limit on the number of requests per second.
* @throws ValidationException
* This exception occurs when a request is not valid.
* @throws AccessDeniedException
* You don't have permissions to perform the requested operation. The user or role that is making the
* request must have at least one IAM permissions policy attached that grants the required permissions.
* @sample AWSResilienceHub.DeleteAppVersionAppComponent
* @see AWS API Documentation
*/
@Override
public DeleteAppVersionAppComponentResult deleteAppVersionAppComponent(DeleteAppVersionAppComponentRequest request) {
request = beforeClientExecution(request);
return executeDeleteAppVersionAppComponent(request);
}
@SdkInternalApi
final DeleteAppVersionAppComponentResult executeDeleteAppVersionAppComponent(DeleteAppVersionAppComponentRequest deleteAppVersionAppComponentRequest) {
ExecutionContext executionContext = createExecutionContext(deleteAppVersionAppComponentRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DeleteAppVersionAppComponentRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(deleteAppVersionAppComponentRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "resiliencehub");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DeleteAppVersionAppComponent");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new DeleteAppVersionAppComponentResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Deletes a resource from the Resilience Hub application.
*
*
*
* -
*
* You can only delete a manually added resource. To exclude non-manually added resources, use the
* UpdateAppVersionResource
API.
*
*
* -
*
* This action has no effect outside Resilience Hub.
*
*
* -
*
* This API updates the Resilience Hub application draft version. To use this resource for running resiliency
* assessments, you must publish the Resilience Hub application using the PublishAppVersion
API.
*
*
*
*
*
* @param deleteAppVersionResourceRequest
* @return Result of the DeleteAppVersionResource operation returned by the service.
* @throws InternalServerException
* This exception occurs when there is an internal failure in the Resilience Hub service.
* @throws ResourceNotFoundException
* This exception occurs when the specified resource could not be found.
* @throws ConflictException
* This exception occurs when a conflict with a previous successful write is detected. This generally occurs
* when the previous write did not have time to propagate to the host serving the current request. A retry
* (with appropriate backoff logic) is the recommended response to this exception.
* @throws ThrottlingException
* This exception occurs when you have exceeded the limit on the number of requests per second.
* @throws ValidationException
* This exception occurs when a request is not valid.
* @throws AccessDeniedException
* You don't have permissions to perform the requested operation. The user or role that is making the
* request must have at least one IAM permissions policy attached that grants the required permissions.
* @sample AWSResilienceHub.DeleteAppVersionResource
* @see AWS API Documentation
*/
@Override
public DeleteAppVersionResourceResult deleteAppVersionResource(DeleteAppVersionResourceRequest request) {
request = beforeClientExecution(request);
return executeDeleteAppVersionResource(request);
}
@SdkInternalApi
final DeleteAppVersionResourceResult executeDeleteAppVersionResource(DeleteAppVersionResourceRequest deleteAppVersionResourceRequest) {
ExecutionContext executionContext = createExecutionContext(deleteAppVersionResourceRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DeleteAppVersionResourceRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(deleteAppVersionResourceRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "resiliencehub");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DeleteAppVersionResource");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new DeleteAppVersionResourceResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Deletes a recommendation template. This is a destructive action that can't be undone.
*
*
* @param deleteRecommendationTemplateRequest
* @return Result of the DeleteRecommendationTemplate operation returned by the service.
* @throws InternalServerException
* This exception occurs when there is an internal failure in the Resilience Hub service.
* @throws ResourceNotFoundException
* This exception occurs when the specified resource could not be found.
* @throws ThrottlingException
* This exception occurs when you have exceeded the limit on the number of requests per second.
* @throws ValidationException
* This exception occurs when a request is not valid.
* @throws AccessDeniedException
* You don't have permissions to perform the requested operation. The user or role that is making the
* request must have at least one IAM permissions policy attached that grants the required permissions.
* @sample AWSResilienceHub.DeleteRecommendationTemplate
* @see AWS API Documentation
*/
@Override
public DeleteRecommendationTemplateResult deleteRecommendationTemplate(DeleteRecommendationTemplateRequest request) {
request = beforeClientExecution(request);
return executeDeleteRecommendationTemplate(request);
}
@SdkInternalApi
final DeleteRecommendationTemplateResult executeDeleteRecommendationTemplate(DeleteRecommendationTemplateRequest deleteRecommendationTemplateRequest) {
ExecutionContext executionContext = createExecutionContext(deleteRecommendationTemplateRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DeleteRecommendationTemplateRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(deleteRecommendationTemplateRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "resiliencehub");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DeleteRecommendationTemplate");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new DeleteRecommendationTemplateResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Deletes a resiliency policy. This is a destructive action that can't be undone.
*
*
* @param deleteResiliencyPolicyRequest
* @return Result of the DeleteResiliencyPolicy operation returned by the service.
* @throws InternalServerException
* This exception occurs when there is an internal failure in the Resilience Hub service.
* @throws ResourceNotFoundException
* This exception occurs when the specified resource could not be found.
* @throws ConflictException
* This exception occurs when a conflict with a previous successful write is detected. This generally occurs
* when the previous write did not have time to propagate to the host serving the current request. A retry
* (with appropriate backoff logic) is the recommended response to this exception.
* @throws ThrottlingException
* This exception occurs when you have exceeded the limit on the number of requests per second.
* @throws ValidationException
* This exception occurs when a request is not valid.
* @throws AccessDeniedException
* You don't have permissions to perform the requested operation. The user or role that is making the
* request must have at least one IAM permissions policy attached that grants the required permissions.
* @sample AWSResilienceHub.DeleteResiliencyPolicy
* @see AWS API Documentation
*/
@Override
public DeleteResiliencyPolicyResult deleteResiliencyPolicy(DeleteResiliencyPolicyRequest request) {
request = beforeClientExecution(request);
return executeDeleteResiliencyPolicy(request);
}
@SdkInternalApi
final DeleteResiliencyPolicyResult executeDeleteResiliencyPolicy(DeleteResiliencyPolicyRequest deleteResiliencyPolicyRequest) {
ExecutionContext executionContext = createExecutionContext(deleteResiliencyPolicyRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DeleteResiliencyPolicyRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(deleteResiliencyPolicyRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "resiliencehub");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DeleteResiliencyPolicy");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new DeleteResiliencyPolicyResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Describes an Resilience Hub application.
*
*
* @param describeAppRequest
* @return Result of the DescribeApp operation returned by the service.
* @throws InternalServerException
* This exception occurs when there is an internal failure in the Resilience Hub service.
* @throws ResourceNotFoundException
* This exception occurs when the specified resource could not be found.
* @throws ThrottlingException
* This exception occurs when you have exceeded the limit on the number of requests per second.
* @throws ValidationException
* This exception occurs when a request is not valid.
* @throws AccessDeniedException
* You don't have permissions to perform the requested operation. The user or role that is making the
* request must have at least one IAM permissions policy attached that grants the required permissions.
* @sample AWSResilienceHub.DescribeApp
* @see AWS API
* Documentation
*/
@Override
public DescribeAppResult describeApp(DescribeAppRequest request) {
request = beforeClientExecution(request);
return executeDescribeApp(request);
}
@SdkInternalApi
final DescribeAppResult executeDescribeApp(DescribeAppRequest describeAppRequest) {
ExecutionContext executionContext = createExecutionContext(describeAppRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DescribeAppRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(describeAppRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "resiliencehub");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DescribeApp");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new DescribeAppResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Describes an assessment for an Resilience Hub application.
*
*
* @param describeAppAssessmentRequest
* @return Result of the DescribeAppAssessment operation returned by the service.
* @throws InternalServerException
* This exception occurs when there is an internal failure in the Resilience Hub service.
* @throws ResourceNotFoundException
* This exception occurs when the specified resource could not be found.
* @throws ThrottlingException
* This exception occurs when you have exceeded the limit on the number of requests per second.
* @throws ValidationException
* This exception occurs when a request is not valid.
* @throws AccessDeniedException
* You don't have permissions to perform the requested operation. The user or role that is making the
* request must have at least one IAM permissions policy attached that grants the required permissions.
* @sample AWSResilienceHub.DescribeAppAssessment
* @see AWS API Documentation
*/
@Override
public DescribeAppAssessmentResult describeAppAssessment(DescribeAppAssessmentRequest request) {
request = beforeClientExecution(request);
return executeDescribeAppAssessment(request);
}
@SdkInternalApi
final DescribeAppAssessmentResult executeDescribeAppAssessment(DescribeAppAssessmentRequest describeAppAssessmentRequest) {
ExecutionContext executionContext = createExecutionContext(describeAppAssessmentRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DescribeAppAssessmentRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(describeAppAssessmentRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "resiliencehub");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DescribeAppAssessment");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory
.createResponseHandler(new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new DescribeAppAssessmentResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Describes the Resilience Hub application version.
*
*
* @param describeAppVersionRequest
* @return Result of the DescribeAppVersion operation returned by the service.
* @throws InternalServerException
* This exception occurs when there is an internal failure in the Resilience Hub service.
* @throws ResourceNotFoundException
* This exception occurs when the specified resource could not be found.
* @throws ThrottlingException
* This exception occurs when you have exceeded the limit on the number of requests per second.
* @throws ValidationException
* This exception occurs when a request is not valid.
* @throws AccessDeniedException
* You don't have permissions to perform the requested operation. The user or role that is making the
* request must have at least one IAM permissions policy attached that grants the required permissions.
* @sample AWSResilienceHub.DescribeAppVersion
* @see AWS API Documentation
*/
@Override
public DescribeAppVersionResult describeAppVersion(DescribeAppVersionRequest request) {
request = beforeClientExecution(request);
return executeDescribeAppVersion(request);
}
@SdkInternalApi
final DescribeAppVersionResult executeDescribeAppVersion(DescribeAppVersionRequest describeAppVersionRequest) {
ExecutionContext executionContext = createExecutionContext(describeAppVersionRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DescribeAppVersionRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(describeAppVersionRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "resiliencehub");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DescribeAppVersion");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new DescribeAppVersionResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Describes an Application Component in the Resilience Hub application.
*
*
* @param describeAppVersionAppComponentRequest
* @return Result of the DescribeAppVersionAppComponent operation returned by the service.
* @throws InternalServerException
* This exception occurs when there is an internal failure in the Resilience Hub service.
* @throws ResourceNotFoundException
* This exception occurs when the specified resource could not be found.
* @throws ConflictException
* This exception occurs when a conflict with a previous successful write is detected. This generally occurs
* when the previous write did not have time to propagate to the host serving the current request. A retry
* (with appropriate backoff logic) is the recommended response to this exception.
* @throws ThrottlingException
* This exception occurs when you have exceeded the limit on the number of requests per second.
* @throws ValidationException
* This exception occurs when a request is not valid.
* @throws AccessDeniedException
* You don't have permissions to perform the requested operation. The user or role that is making the
* request must have at least one IAM permissions policy attached that grants the required permissions.
* @sample AWSResilienceHub.DescribeAppVersionAppComponent
* @see AWS API Documentation
*/
@Override
public DescribeAppVersionAppComponentResult describeAppVersionAppComponent(DescribeAppVersionAppComponentRequest request) {
request = beforeClientExecution(request);
return executeDescribeAppVersionAppComponent(request);
}
@SdkInternalApi
final DescribeAppVersionAppComponentResult executeDescribeAppVersionAppComponent(DescribeAppVersionAppComponentRequest describeAppVersionAppComponentRequest) {
ExecutionContext executionContext = createExecutionContext(describeAppVersionAppComponentRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DescribeAppVersionAppComponentRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(describeAppVersionAppComponentRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "resiliencehub");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DescribeAppVersionAppComponent");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new DescribeAppVersionAppComponentResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Describes a resource of the Resilience Hub application.
*
*
*
* This API accepts only one of the following parameters to descibe the resource:
*
*
* -
*
* resourceName
*
*
* -
*
* logicalResourceId
*
*
* -
*
* physicalResourceId
(Along with physicalResourceId
, you can also provide
* awsAccountId
, and awsRegion
)
*
*
*
*
*
* @param describeAppVersionResourceRequest
* @return Result of the DescribeAppVersionResource operation returned by the service.
* @throws InternalServerException
* This exception occurs when there is an internal failure in the Resilience Hub service.
* @throws ResourceNotFoundException
* This exception occurs when the specified resource could not be found.
* @throws ConflictException
* This exception occurs when a conflict with a previous successful write is detected. This generally occurs
* when the previous write did not have time to propagate to the host serving the current request. A retry
* (with appropriate backoff logic) is the recommended response to this exception.
* @throws ThrottlingException
* This exception occurs when you have exceeded the limit on the number of requests per second.
* @throws ValidationException
* This exception occurs when a request is not valid.
* @throws AccessDeniedException
* You don't have permissions to perform the requested operation. The user or role that is making the
* request must have at least one IAM permissions policy attached that grants the required permissions.
* @sample AWSResilienceHub.DescribeAppVersionResource
* @see AWS API Documentation
*/
@Override
public DescribeAppVersionResourceResult describeAppVersionResource(DescribeAppVersionResourceRequest request) {
request = beforeClientExecution(request);
return executeDescribeAppVersionResource(request);
}
@SdkInternalApi
final DescribeAppVersionResourceResult executeDescribeAppVersionResource(DescribeAppVersionResourceRequest describeAppVersionResourceRequest) {
ExecutionContext executionContext = createExecutionContext(describeAppVersionResourceRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DescribeAppVersionResourceRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(describeAppVersionResourceRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "resiliencehub");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DescribeAppVersionResource");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new DescribeAppVersionResourceResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Returns the resolution status for the specified resolution identifier for an application version. If
* resolutionId
is not specified, the current resolution status is returned.
*
*
* @param describeAppVersionResourcesResolutionStatusRequest
* @return Result of the DescribeAppVersionResourcesResolutionStatus operation returned by the service.
* @throws InternalServerException
* This exception occurs when there is an internal failure in the Resilience Hub service.
* @throws ResourceNotFoundException
* This exception occurs when the specified resource could not be found.
* @throws ThrottlingException
* This exception occurs when you have exceeded the limit on the number of requests per second.
* @throws ValidationException
* This exception occurs when a request is not valid.
* @throws AccessDeniedException
* You don't have permissions to perform the requested operation. The user or role that is making the
* request must have at least one IAM permissions policy attached that grants the required permissions.
* @sample AWSResilienceHub.DescribeAppVersionResourcesResolutionStatus
* @see AWS API Documentation
*/
@Override
public DescribeAppVersionResourcesResolutionStatusResult describeAppVersionResourcesResolutionStatus(
DescribeAppVersionResourcesResolutionStatusRequest request) {
request = beforeClientExecution(request);
return executeDescribeAppVersionResourcesResolutionStatus(request);
}
@SdkInternalApi
final DescribeAppVersionResourcesResolutionStatusResult executeDescribeAppVersionResourcesResolutionStatus(
DescribeAppVersionResourcesResolutionStatusRequest describeAppVersionResourcesResolutionStatusRequest) {
ExecutionContext executionContext = createExecutionContext(describeAppVersionResourcesResolutionStatusRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DescribeAppVersionResourcesResolutionStatusRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(describeAppVersionResourcesResolutionStatusRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "resiliencehub");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DescribeAppVersionResourcesResolutionStatus");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory
.createResponseHandler(new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new DescribeAppVersionResourcesResolutionStatusResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Describes details about an Resilience Hub application.
*
*
* @param describeAppVersionTemplateRequest
* @return Result of the DescribeAppVersionTemplate operation returned by the service.
* @throws InternalServerException
* This exception occurs when there is an internal failure in the Resilience Hub service.
* @throws ResourceNotFoundException
* This exception occurs when the specified resource could not be found.
* @throws ThrottlingException
* This exception occurs when you have exceeded the limit on the number of requests per second.
* @throws ValidationException
* This exception occurs when a request is not valid.
* @throws AccessDeniedException
* You don't have permissions to perform the requested operation. The user or role that is making the
* request must have at least one IAM permissions policy attached that grants the required permissions.
* @sample AWSResilienceHub.DescribeAppVersionTemplate
* @see AWS API Documentation
*/
@Override
public DescribeAppVersionTemplateResult describeAppVersionTemplate(DescribeAppVersionTemplateRequest request) {
request = beforeClientExecution(request);
return executeDescribeAppVersionTemplate(request);
}
@SdkInternalApi
final DescribeAppVersionTemplateResult executeDescribeAppVersionTemplate(DescribeAppVersionTemplateRequest describeAppVersionTemplateRequest) {
ExecutionContext executionContext = createExecutionContext(describeAppVersionTemplateRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DescribeAppVersionTemplateRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(describeAppVersionTemplateRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "resiliencehub");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DescribeAppVersionTemplate");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new DescribeAppVersionTemplateResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Describes the status of importing resources to an application version.
*
*
*
* If you get a 404 error with ResourceImportStatusNotFoundAppMetadataException
, you must call
* importResourcesToDraftAppVersion
after creating the application and before calling
* describeDraftAppVersionResourcesImportStatus
to obtain the status.
*
*
*
* @param describeDraftAppVersionResourcesImportStatusRequest
* @return Result of the DescribeDraftAppVersionResourcesImportStatus operation returned by the service.
* @throws InternalServerException
* This exception occurs when there is an internal failure in the Resilience Hub service.
* @throws ResourceNotFoundException
* This exception occurs when the specified resource could not be found.
* @throws ThrottlingException
* This exception occurs when you have exceeded the limit on the number of requests per second.
* @throws ValidationException
* This exception occurs when a request is not valid.
* @throws AccessDeniedException
* You don't have permissions to perform the requested operation. The user or role that is making the
* request must have at least one IAM permissions policy attached that grants the required permissions.
* @sample AWSResilienceHub.DescribeDraftAppVersionResourcesImportStatus
* @see AWS API Documentation
*/
@Override
public DescribeDraftAppVersionResourcesImportStatusResult describeDraftAppVersionResourcesImportStatus(
DescribeDraftAppVersionResourcesImportStatusRequest request) {
request = beforeClientExecution(request);
return executeDescribeDraftAppVersionResourcesImportStatus(request);
}
@SdkInternalApi
final DescribeDraftAppVersionResourcesImportStatusResult executeDescribeDraftAppVersionResourcesImportStatus(
DescribeDraftAppVersionResourcesImportStatusRequest describeDraftAppVersionResourcesImportStatusRequest) {
ExecutionContext executionContext = createExecutionContext(describeDraftAppVersionResourcesImportStatusRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DescribeDraftAppVersionResourcesImportStatusRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(describeDraftAppVersionResourcesImportStatusRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "resiliencehub");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DescribeDraftAppVersionResourcesImportStatus");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory
.createResponseHandler(new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new DescribeDraftAppVersionResourcesImportStatusResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Describes a specified resiliency policy for an Resilience Hub application. The returned policy object includes
* creation time, data location constraints, the Amazon Resource Name (ARN) for the policy, tags, tier, and more.
*
*
* @param describeResiliencyPolicyRequest
* @return Result of the DescribeResiliencyPolicy operation returned by the service.
* @throws InternalServerException
* This exception occurs when there is an internal failure in the Resilience Hub service.
* @throws ResourceNotFoundException
* This exception occurs when the specified resource could not be found.
* @throws ThrottlingException
* This exception occurs when you have exceeded the limit on the number of requests per second.
* @throws ValidationException
* This exception occurs when a request is not valid.
* @throws AccessDeniedException
* You don't have permissions to perform the requested operation. The user or role that is making the
* request must have at least one IAM permissions policy attached that grants the required permissions.
* @sample AWSResilienceHub.DescribeResiliencyPolicy
* @see AWS API Documentation
*/
@Override
public DescribeResiliencyPolicyResult describeResiliencyPolicy(DescribeResiliencyPolicyRequest request) {
request = beforeClientExecution(request);
return executeDescribeResiliencyPolicy(request);
}
@SdkInternalApi
final DescribeResiliencyPolicyResult executeDescribeResiliencyPolicy(DescribeResiliencyPolicyRequest describeResiliencyPolicyRequest) {
ExecutionContext executionContext = createExecutionContext(describeResiliencyPolicyRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DescribeResiliencyPolicyRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(describeResiliencyPolicyRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "resiliencehub");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DescribeResiliencyPolicy");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new DescribeResiliencyPolicyResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Imports resources to Resilience Hub application draft version from different input sources. For more information
* about the input sources supported by Resilience Hub, see Discover the structure
* and describe your Resilience Hub application.
*
*
* @param importResourcesToDraftAppVersionRequest
* @return Result of the ImportResourcesToDraftAppVersion operation returned by the service.
* @throws InternalServerException
* This exception occurs when there is an internal failure in the Resilience Hub service.
* @throws ResourceNotFoundException
* This exception occurs when the specified resource could not be found.
* @throws ConflictException
* This exception occurs when a conflict with a previous successful write is detected. This generally occurs
* when the previous write did not have time to propagate to the host serving the current request. A retry
* (with appropriate backoff logic) is the recommended response to this exception.
* @throws ServiceQuotaExceededException
* This exception occurs when you have exceeded your service quota. To perform the requested action, remove
* some of the relevant resources, or use Service Quotas to request a service quota increase.
* @throws ThrottlingException
* This exception occurs when you have exceeded the limit on the number of requests per second.
* @throws ValidationException
* This exception occurs when a request is not valid.
* @throws AccessDeniedException
* You don't have permissions to perform the requested operation. The user or role that is making the
* request must have at least one IAM permissions policy attached that grants the required permissions.
* @sample AWSResilienceHub.ImportResourcesToDraftAppVersion
* @see AWS API Documentation
*/
@Override
public ImportResourcesToDraftAppVersionResult importResourcesToDraftAppVersion(ImportResourcesToDraftAppVersionRequest request) {
request = beforeClientExecution(request);
return executeImportResourcesToDraftAppVersion(request);
}
@SdkInternalApi
final ImportResourcesToDraftAppVersionResult executeImportResourcesToDraftAppVersion(
ImportResourcesToDraftAppVersionRequest importResourcesToDraftAppVersionRequest) {
ExecutionContext executionContext = createExecutionContext(importResourcesToDraftAppVersionRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new ImportResourcesToDraftAppVersionRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(importResourcesToDraftAppVersionRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "resiliencehub");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "ImportResourcesToDraftAppVersion");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new ImportResourcesToDraftAppVersionResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Lists the alarm recommendations for an Resilience Hub application.
*
*
* @param listAlarmRecommendationsRequest
* @return Result of the ListAlarmRecommendations operation returned by the service.
* @throws InternalServerException
* This exception occurs when there is an internal failure in the Resilience Hub service.
* @throws ResourceNotFoundException
* This exception occurs when the specified resource could not be found.
* @throws ThrottlingException
* This exception occurs when you have exceeded the limit on the number of requests per second.
* @throws ValidationException
* This exception occurs when a request is not valid.
* @throws AccessDeniedException
* You don't have permissions to perform the requested operation. The user or role that is making the
* request must have at least one IAM permissions policy attached that grants the required permissions.
* @sample AWSResilienceHub.ListAlarmRecommendations
* @see AWS API Documentation
*/
@Override
public ListAlarmRecommendationsResult listAlarmRecommendations(ListAlarmRecommendationsRequest request) {
request = beforeClientExecution(request);
return executeListAlarmRecommendations(request);
}
@SdkInternalApi
final ListAlarmRecommendationsResult executeListAlarmRecommendations(ListAlarmRecommendationsRequest listAlarmRecommendationsRequest) {
ExecutionContext executionContext = createExecutionContext(listAlarmRecommendationsRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new ListAlarmRecommendationsRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(listAlarmRecommendationsRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "resiliencehub");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "ListAlarmRecommendations");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new ListAlarmRecommendationsResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* List of compliance drifts that were detected while running an assessment.
*
*
* @param listAppAssessmentComplianceDriftsRequest
* @return Result of the ListAppAssessmentComplianceDrifts operation returned by the service.
* @throws InternalServerException
* This exception occurs when there is an internal failure in the Resilience Hub service.
* @throws ThrottlingException
* This exception occurs when you have exceeded the limit on the number of requests per second.
* @throws ValidationException
* This exception occurs when a request is not valid.
* @throws AccessDeniedException
* You don't have permissions to perform the requested operation. The user or role that is making the
* request must have at least one IAM permissions policy attached that grants the required permissions.
* @sample AWSResilienceHub.ListAppAssessmentComplianceDrifts
* @see AWS API Documentation
*/
@Override
public ListAppAssessmentComplianceDriftsResult listAppAssessmentComplianceDrifts(ListAppAssessmentComplianceDriftsRequest request) {
request = beforeClientExecution(request);
return executeListAppAssessmentComplianceDrifts(request);
}
@SdkInternalApi
final ListAppAssessmentComplianceDriftsResult executeListAppAssessmentComplianceDrifts(
ListAppAssessmentComplianceDriftsRequest listAppAssessmentComplianceDriftsRequest) {
ExecutionContext executionContext = createExecutionContext(listAppAssessmentComplianceDriftsRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new ListAppAssessmentComplianceDriftsRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(listAppAssessmentComplianceDriftsRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "resiliencehub");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "ListAppAssessmentComplianceDrifts");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new ListAppAssessmentComplianceDriftsResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Indicates the list of resource drifts that were detected while running an assessment.
*
*
* @param listAppAssessmentResourceDriftsRequest
* @return Result of the ListAppAssessmentResourceDrifts operation returned by the service.
* @throws InternalServerException
* This exception occurs when there is an internal failure in the Resilience Hub service.
* @throws ThrottlingException
* This exception occurs when you have exceeded the limit on the number of requests per second.
* @throws ValidationException
* This exception occurs when a request is not valid.
* @throws AccessDeniedException
* You don't have permissions to perform the requested operation. The user or role that is making the
* request must have at least one IAM permissions policy attached that grants the required permissions.
* @sample AWSResilienceHub.ListAppAssessmentResourceDrifts
* @see AWS API Documentation
*/
@Override
public ListAppAssessmentResourceDriftsResult listAppAssessmentResourceDrifts(ListAppAssessmentResourceDriftsRequest request) {
request = beforeClientExecution(request);
return executeListAppAssessmentResourceDrifts(request);
}
@SdkInternalApi
final ListAppAssessmentResourceDriftsResult executeListAppAssessmentResourceDrifts(
ListAppAssessmentResourceDriftsRequest listAppAssessmentResourceDriftsRequest) {
ExecutionContext executionContext = createExecutionContext(listAppAssessmentResourceDriftsRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new ListAppAssessmentResourceDriftsRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(listAppAssessmentResourceDriftsRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "resiliencehub");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "ListAppAssessmentResourceDrifts");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new ListAppAssessmentResourceDriftsResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Lists the assessments for an Resilience Hub application. You can use request parameters to refine the results for
* the response object.
*
*
* @param listAppAssessmentsRequest
* @return Result of the ListAppAssessments operation returned by the service.
* @throws InternalServerException
* This exception occurs when there is an internal failure in the Resilience Hub service.
* @throws ResourceNotFoundException
* This exception occurs when the specified resource could not be found.
* @throws ThrottlingException
* This exception occurs when you have exceeded the limit on the number of requests per second.
* @throws ValidationException
* This exception occurs when a request is not valid.
* @throws AccessDeniedException
* You don't have permissions to perform the requested operation. The user or role that is making the
* request must have at least one IAM permissions policy attached that grants the required permissions.
* @sample AWSResilienceHub.ListAppAssessments
* @see AWS API Documentation
*/
@Override
public ListAppAssessmentsResult listAppAssessments(ListAppAssessmentsRequest request) {
request = beforeClientExecution(request);
return executeListAppAssessments(request);
}
@SdkInternalApi
final ListAppAssessmentsResult executeListAppAssessments(ListAppAssessmentsRequest listAppAssessmentsRequest) {
ExecutionContext executionContext = createExecutionContext(listAppAssessmentsRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new ListAppAssessmentsRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(listAppAssessmentsRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "resiliencehub");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "ListAppAssessments");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new ListAppAssessmentsResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Lists the compliances for an Resilience Hub Application Component.
*
*
* @param listAppComponentCompliancesRequest
* @return Result of the ListAppComponentCompliances operation returned by the service.
* @throws InternalServerException
* This exception occurs when there is an internal failure in the Resilience Hub service.
* @throws ResourceNotFoundException
* This exception occurs when the specified resource could not be found.
* @throws ThrottlingException
* This exception occurs when you have exceeded the limit on the number of requests per second.
* @throws ValidationException
* This exception occurs when a request is not valid.
* @throws AccessDeniedException
* You don't have permissions to perform the requested operation. The user or role that is making the
* request must have at least one IAM permissions policy attached that grants the required permissions.
* @sample AWSResilienceHub.ListAppComponentCompliances
* @see AWS API Documentation
*/
@Override
public ListAppComponentCompliancesResult listAppComponentCompliances(ListAppComponentCompliancesRequest request) {
request = beforeClientExecution(request);
return executeListAppComponentCompliances(request);
}
@SdkInternalApi
final ListAppComponentCompliancesResult executeListAppComponentCompliances(ListAppComponentCompliancesRequest listAppComponentCompliancesRequest) {
ExecutionContext executionContext = createExecutionContext(listAppComponentCompliancesRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new ListAppComponentCompliancesRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(listAppComponentCompliancesRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "resiliencehub");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "ListAppComponentCompliances");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new ListAppComponentCompliancesResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Lists the recommendations for an Resilience Hub Application Component.
*
*
* @param listAppComponentRecommendationsRequest
* @return Result of the ListAppComponentRecommendations operation returned by the service.
* @throws InternalServerException
* This exception occurs when there is an internal failure in the Resilience Hub service.
* @throws ResourceNotFoundException
* This exception occurs when the specified resource could not be found.
* @throws ThrottlingException
* This exception occurs when you have exceeded the limit on the number of requests per second.
* @throws ValidationException
* This exception occurs when a request is not valid.
* @throws AccessDeniedException
* You don't have permissions to perform the requested operation. The user or role that is making the
* request must have at least one IAM permissions policy attached that grants the required permissions.
* @sample AWSResilienceHub.ListAppComponentRecommendations
* @see AWS API Documentation
*/
@Override
public ListAppComponentRecommendationsResult listAppComponentRecommendations(ListAppComponentRecommendationsRequest request) {
request = beforeClientExecution(request);
return executeListAppComponentRecommendations(request);
}
@SdkInternalApi
final ListAppComponentRecommendationsResult executeListAppComponentRecommendations(
ListAppComponentRecommendationsRequest listAppComponentRecommendationsRequest) {
ExecutionContext executionContext = createExecutionContext(listAppComponentRecommendationsRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new ListAppComponentRecommendationsRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(listAppComponentRecommendationsRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "resiliencehub");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "ListAppComponentRecommendations");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new ListAppComponentRecommendationsResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Lists all the input sources of the Resilience Hub application. For more information about the input sources
* supported by Resilience Hub, see Discover the structure
* and describe your Resilience Hub application.
*
*
* @param listAppInputSourcesRequest
* @return Result of the ListAppInputSources operation returned by the service.
* @throws InternalServerException
* This exception occurs when there is an internal failure in the Resilience Hub service.
* @throws ResourceNotFoundException
* This exception occurs when the specified resource could not be found.
* @throws ThrottlingException
* This exception occurs when you have exceeded the limit on the number of requests per second.
* @throws ValidationException
* This exception occurs when a request is not valid.
* @throws AccessDeniedException
* You don't have permissions to perform the requested operation. The user or role that is making the
* request must have at least one IAM permissions policy attached that grants the required permissions.
* @sample AWSResilienceHub.ListAppInputSources
* @see AWS API Documentation
*/
@Override
public ListAppInputSourcesResult listAppInputSources(ListAppInputSourcesRequest request) {
request = beforeClientExecution(request);
return executeListAppInputSources(request);
}
@SdkInternalApi
final ListAppInputSourcesResult executeListAppInputSources(ListAppInputSourcesRequest listAppInputSourcesRequest) {
ExecutionContext executionContext = createExecutionContext(listAppInputSourcesRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new ListAppInputSourcesRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(listAppInputSourcesRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "resiliencehub");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "ListAppInputSources");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new ListAppInputSourcesResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Lists all the Application Components in the Resilience Hub application.
*
*
* @param listAppVersionAppComponentsRequest
* @return Result of the ListAppVersionAppComponents operation returned by the service.
* @throws InternalServerException
* This exception occurs when there is an internal failure in the Resilience Hub service.
* @throws ResourceNotFoundException
* This exception occurs when the specified resource could not be found.
* @throws ConflictException
* This exception occurs when a conflict with a previous successful write is detected. This generally occurs
* when the previous write did not have time to propagate to the host serving the current request. A retry
* (with appropriate backoff logic) is the recommended response to this exception.
* @throws ThrottlingException
* This exception occurs when you have exceeded the limit on the number of requests per second.
* @throws ValidationException
* This exception occurs when a request is not valid.
* @throws AccessDeniedException
* You don't have permissions to perform the requested operation. The user or role that is making the
* request must have at least one IAM permissions policy attached that grants the required permissions.
* @sample AWSResilienceHub.ListAppVersionAppComponents
* @see AWS API Documentation
*/
@Override
public ListAppVersionAppComponentsResult listAppVersionAppComponents(ListAppVersionAppComponentsRequest request) {
request = beforeClientExecution(request);
return executeListAppVersionAppComponents(request);
}
@SdkInternalApi
final ListAppVersionAppComponentsResult executeListAppVersionAppComponents(ListAppVersionAppComponentsRequest listAppVersionAppComponentsRequest) {
ExecutionContext executionContext = createExecutionContext(listAppVersionAppComponentsRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new ListAppVersionAppComponentsRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(listAppVersionAppComponentsRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "resiliencehub");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "ListAppVersionAppComponents");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new ListAppVersionAppComponentsResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Lists how the resources in an application version are mapped/sourced from. Mappings can be physical resource
* identifiers, CloudFormation stacks, resource-groups, or an application registry app.
*
*
* @param listAppVersionResourceMappingsRequest
* @return Result of the ListAppVersionResourceMappings operation returned by the service.
* @throws InternalServerException
* This exception occurs when there is an internal failure in the Resilience Hub service.
* @throws ResourceNotFoundException
* This exception occurs when the specified resource could not be found.
* @throws ThrottlingException
* This exception occurs when you have exceeded the limit on the number of requests per second.
* @throws ValidationException
* This exception occurs when a request is not valid.
* @throws AccessDeniedException
* You don't have permissions to perform the requested operation. The user or role that is making the
* request must have at least one IAM permissions policy attached that grants the required permissions.
* @sample AWSResilienceHub.ListAppVersionResourceMappings
* @see AWS API Documentation
*/
@Override
public ListAppVersionResourceMappingsResult listAppVersionResourceMappings(ListAppVersionResourceMappingsRequest request) {
request = beforeClientExecution(request);
return executeListAppVersionResourceMappings(request);
}
@SdkInternalApi
final ListAppVersionResourceMappingsResult executeListAppVersionResourceMappings(ListAppVersionResourceMappingsRequest listAppVersionResourceMappingsRequest) {
ExecutionContext executionContext = createExecutionContext(listAppVersionResourceMappingsRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new ListAppVersionResourceMappingsRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(listAppVersionResourceMappingsRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "resiliencehub");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "ListAppVersionResourceMappings");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new ListAppVersionResourceMappingsResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Lists all the resources in an Resilience Hub application.
*
*
* @param listAppVersionResourcesRequest
* @return Result of the ListAppVersionResources operation returned by the service.
* @throws InternalServerException
* This exception occurs when there is an internal failure in the Resilience Hub service.
* @throws ResourceNotFoundException
* This exception occurs when the specified resource could not be found.
* @throws ConflictException
* This exception occurs when a conflict with a previous successful write is detected. This generally occurs
* when the previous write did not have time to propagate to the host serving the current request. A retry
* (with appropriate backoff logic) is the recommended response to this exception.
* @throws ThrottlingException
* This exception occurs when you have exceeded the limit on the number of requests per second.
* @throws ValidationException
* This exception occurs when a request is not valid.
* @throws AccessDeniedException
* You don't have permissions to perform the requested operation. The user or role that is making the
* request must have at least one IAM permissions policy attached that grants the required permissions.
* @sample AWSResilienceHub.ListAppVersionResources
* @see AWS API Documentation
*/
@Override
public ListAppVersionResourcesResult listAppVersionResources(ListAppVersionResourcesRequest request) {
request = beforeClientExecution(request);
return executeListAppVersionResources(request);
}
@SdkInternalApi
final ListAppVersionResourcesResult executeListAppVersionResources(ListAppVersionResourcesRequest listAppVersionResourcesRequest) {
ExecutionContext executionContext = createExecutionContext(listAppVersionResourcesRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new ListAppVersionResourcesRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(listAppVersionResourcesRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "resiliencehub");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "ListAppVersionResources");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new ListAppVersionResourcesResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Lists the different versions for the Resilience Hub applications.
*
*
* @param listAppVersionsRequest
* @return Result of the ListAppVersions operation returned by the service.
* @throws InternalServerException
* This exception occurs when there is an internal failure in the Resilience Hub service.
* @throws ResourceNotFoundException
* This exception occurs when the specified resource could not be found.
* @throws ValidationException
* This exception occurs when a request is not valid.
* @throws AccessDeniedException
* You don't have permissions to perform the requested operation. The user or role that is making the
* request must have at least one IAM permissions policy attached that grants the required permissions.
* @sample AWSResilienceHub.ListAppVersions
* @see AWS
* API Documentation
*/
@Override
public ListAppVersionsResult listAppVersions(ListAppVersionsRequest request) {
request = beforeClientExecution(request);
return executeListAppVersions(request);
}
@SdkInternalApi
final ListAppVersionsResult executeListAppVersions(ListAppVersionsRequest listAppVersionsRequest) {
ExecutionContext executionContext = createExecutionContext(listAppVersionsRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new ListAppVersionsRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(listAppVersionsRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "resiliencehub");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "ListAppVersions");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new ListAppVersionsResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Lists your Resilience Hub applications.
*
*
*
* You can filter applications using only one filter at a time or without using any filter. If you try to filter
* applications using multiple filters, you will get the following error:
*
*
* An error occurred (ValidationException) when calling the ListApps operation: Only one filter is supported for this operation.
*
*
*
* @param listAppsRequest
* @return Result of the ListApps operation returned by the service.
* @throws InternalServerException
* This exception occurs when there is an internal failure in the Resilience Hub service.
* @throws ThrottlingException
* This exception occurs when you have exceeded the limit on the number of requests per second.
* @throws ValidationException
* This exception occurs when a request is not valid.
* @throws AccessDeniedException
* You don't have permissions to perform the requested operation. The user or role that is making the
* request must have at least one IAM permissions policy attached that grants the required permissions.
* @sample AWSResilienceHub.ListApps
* @see AWS API
* Documentation
*/
@Override
public ListAppsResult listApps(ListAppsRequest request) {
request = beforeClientExecution(request);
return executeListApps(request);
}
@SdkInternalApi
final ListAppsResult executeListApps(ListAppsRequest listAppsRequest) {
ExecutionContext executionContext = createExecutionContext(listAppsRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new ListAppsRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(listAppsRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "resiliencehub");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "ListApps");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(new JsonOperationMetadata()
.withPayloadJson(true).withHasStreamingSuccessResponse(false), new ListAppsResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Lists the recommendation templates for the Resilience Hub applications.
*
*
* @param listRecommendationTemplatesRequest
* @return Result of the ListRecommendationTemplates operation returned by the service.
* @throws InternalServerException
* This exception occurs when there is an internal failure in the Resilience Hub service.
* @throws ThrottlingException
* This exception occurs when you have exceeded the limit on the number of requests per second.
* @throws ValidationException
* This exception occurs when a request is not valid.
* @throws AccessDeniedException
* You don't have permissions to perform the requested operation. The user or role that is making the
* request must have at least one IAM permissions policy attached that grants the required permissions.
* @sample AWSResilienceHub.ListRecommendationTemplates
* @see AWS API Documentation
*/
@Override
public ListRecommendationTemplatesResult listRecommendationTemplates(ListRecommendationTemplatesRequest request) {
request = beforeClientExecution(request);
return executeListRecommendationTemplates(request);
}
@SdkInternalApi
final ListRecommendationTemplatesResult executeListRecommendationTemplates(ListRecommendationTemplatesRequest listRecommendationTemplatesRequest) {
ExecutionContext executionContext = createExecutionContext(listRecommendationTemplatesRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new ListRecommendationTemplatesRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(listRecommendationTemplatesRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "resiliencehub");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "ListRecommendationTemplates");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new ListRecommendationTemplatesResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Lists the resiliency policies for the Resilience Hub applications.
*
*
* @param listResiliencyPoliciesRequest
* @return Result of the ListResiliencyPolicies operation returned by the service.
* @throws InternalServerException
* This exception occurs when there is an internal failure in the Resilience Hub service.
* @throws ResourceNotFoundException
* This exception occurs when the specified resource could not be found.
* @throws ThrottlingException
* This exception occurs when you have exceeded the limit on the number of requests per second.
* @throws ValidationException
* This exception occurs when a request is not valid.
* @throws AccessDeniedException
* You don't have permissions to perform the requested operation. The user or role that is making the
* request must have at least one IAM permissions policy attached that grants the required permissions.
* @sample AWSResilienceHub.ListResiliencyPolicies
* @see AWS API Documentation
*/
@Override
public ListResiliencyPoliciesResult listResiliencyPolicies(ListResiliencyPoliciesRequest request) {
request = beforeClientExecution(request);
return executeListResiliencyPolicies(request);
}
@SdkInternalApi
final ListResiliencyPoliciesResult executeListResiliencyPolicies(ListResiliencyPoliciesRequest listResiliencyPoliciesRequest) {
ExecutionContext executionContext = createExecutionContext(listResiliencyPoliciesRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new ListResiliencyPoliciesRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(listResiliencyPoliciesRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "resiliencehub");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "ListResiliencyPolicies");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new ListResiliencyPoliciesResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Lists the standard operating procedure (SOP) recommendations for the Resilience Hub applications.
*
*
* @param listSopRecommendationsRequest
* @return Result of the ListSopRecommendations operation returned by the service.
* @throws InternalServerException
* This exception occurs when there is an internal failure in the Resilience Hub service.
* @throws ResourceNotFoundException
* This exception occurs when the specified resource could not be found.
* @throws ConflictException
* This exception occurs when a conflict with a previous successful write is detected. This generally occurs
* when the previous write did not have time to propagate to the host serving the current request. A retry
* (with appropriate backoff logic) is the recommended response to this exception.
* @throws ThrottlingException
* This exception occurs when you have exceeded the limit on the number of requests per second.
* @throws ValidationException
* This exception occurs when a request is not valid.
* @throws AccessDeniedException
* You don't have permissions to perform the requested operation. The user or role that is making the
* request must have at least one IAM permissions policy attached that grants the required permissions.
* @sample AWSResilienceHub.ListSopRecommendations
* @see AWS API Documentation
*/
@Override
public ListSopRecommendationsResult listSopRecommendations(ListSopRecommendationsRequest request) {
request = beforeClientExecution(request);
return executeListSopRecommendations(request);
}
@SdkInternalApi
final ListSopRecommendationsResult executeListSopRecommendations(ListSopRecommendationsRequest listSopRecommendationsRequest) {
ExecutionContext executionContext = createExecutionContext(listSopRecommendationsRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new ListSopRecommendationsRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(listSopRecommendationsRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "resiliencehub");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "ListSopRecommendations");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new ListSopRecommendationsResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Lists the suggested resiliency policies for the Resilience Hub applications.
*
*
* @param listSuggestedResiliencyPoliciesRequest
* @return Result of the ListSuggestedResiliencyPolicies operation returned by the service.
* @throws InternalServerException
* This exception occurs when there is an internal failure in the Resilience Hub service.
* @throws ResourceNotFoundException
* This exception occurs when the specified resource could not be found.
* @throws ThrottlingException
* This exception occurs when you have exceeded the limit on the number of requests per second.
* @throws ValidationException
* This exception occurs when a request is not valid.
* @throws AccessDeniedException
* You don't have permissions to perform the requested operation. The user or role that is making the
* request must have at least one IAM permissions policy attached that grants the required permissions.
* @sample AWSResilienceHub.ListSuggestedResiliencyPolicies
* @see AWS API Documentation
*/
@Override
public ListSuggestedResiliencyPoliciesResult listSuggestedResiliencyPolicies(ListSuggestedResiliencyPoliciesRequest request) {
request = beforeClientExecution(request);
return executeListSuggestedResiliencyPolicies(request);
}
@SdkInternalApi
final ListSuggestedResiliencyPoliciesResult executeListSuggestedResiliencyPolicies(
ListSuggestedResiliencyPoliciesRequest listSuggestedResiliencyPoliciesRequest) {
ExecutionContext executionContext = createExecutionContext(listSuggestedResiliencyPoliciesRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new ListSuggestedResiliencyPoliciesRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(listSuggestedResiliencyPoliciesRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "resiliencehub");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "ListSuggestedResiliencyPolicies");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new ListSuggestedResiliencyPoliciesResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Lists the tags for your resources in your Resilience Hub applications.
*
*
* @param listTagsForResourceRequest
* @return Result of the ListTagsForResource operation returned by the service.
* @throws InternalServerException
* This exception occurs when there is an internal failure in the Resilience Hub service.
* @throws ResourceNotFoundException
* This exception occurs when the specified resource could not be found.
* @throws ThrottlingException
* This exception occurs when you have exceeded the limit on the number of requests per second.
* @throws ValidationException
* This exception occurs when a request is not valid.
* @throws AccessDeniedException
* You don't have permissions to perform the requested operation. The user or role that is making the
* request must have at least one IAM permissions policy attached that grants the required permissions.
* @sample AWSResilienceHub.ListTagsForResource
* @see AWS API Documentation
*/
@Override
public ListTagsForResourceResult listTagsForResource(ListTagsForResourceRequest request) {
request = beforeClientExecution(request);
return executeListTagsForResource(request);
}
@SdkInternalApi
final ListTagsForResourceResult executeListTagsForResource(ListTagsForResourceRequest listTagsForResourceRequest) {
ExecutionContext executionContext = createExecutionContext(listTagsForResourceRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new ListTagsForResourceRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(listTagsForResourceRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "resiliencehub");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "ListTagsForResource");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new ListTagsForResourceResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Lists the test recommendations for the Resilience Hub application.
*
*
* @param listTestRecommendationsRequest
* @return Result of the ListTestRecommendations operation returned by the service.
* @throws InternalServerException
* This exception occurs when there is an internal failure in the Resilience Hub service.
* @throws ResourceNotFoundException
* This exception occurs when the specified resource could not be found.
* @throws ConflictException
* This exception occurs when a conflict with a previous successful write is detected. This generally occurs
* when the previous write did not have time to propagate to the host serving the current request. A retry
* (with appropriate backoff logic) is the recommended response to this exception.
* @throws ThrottlingException
* This exception occurs when you have exceeded the limit on the number of requests per second.
* @throws ValidationException
* This exception occurs when a request is not valid.
* @throws AccessDeniedException
* You don't have permissions to perform the requested operation. The user or role that is making the
* request must have at least one IAM permissions policy attached that grants the required permissions.
* @sample AWSResilienceHub.ListTestRecommendations
* @see AWS API Documentation
*/
@Override
public ListTestRecommendationsResult listTestRecommendations(ListTestRecommendationsRequest request) {
request = beforeClientExecution(request);
return executeListTestRecommendations(request);
}
@SdkInternalApi
final ListTestRecommendationsResult executeListTestRecommendations(ListTestRecommendationsRequest listTestRecommendationsRequest) {
ExecutionContext executionContext = createExecutionContext(listTestRecommendationsRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new ListTestRecommendationsRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(listTestRecommendationsRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "resiliencehub");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "ListTestRecommendations");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new ListTestRecommendationsResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Lists the resources that are not currently supported in Resilience Hub. An unsupported resource is a resource
* that exists in the object that was used to create an app, but is not supported by Resilience Hub.
*
*
* @param listUnsupportedAppVersionResourcesRequest
* @return Result of the ListUnsupportedAppVersionResources operation returned by the service.
* @throws InternalServerException
* This exception occurs when there is an internal failure in the Resilience Hub service.
* @throws ResourceNotFoundException
* This exception occurs when the specified resource could not be found.
* @throws ConflictException
* This exception occurs when a conflict with a previous successful write is detected. This generally occurs
* when the previous write did not have time to propagate to the host serving the current request. A retry
* (with appropriate backoff logic) is the recommended response to this exception.
* @throws ThrottlingException
* This exception occurs when you have exceeded the limit on the number of requests per second.
* @throws ValidationException
* This exception occurs when a request is not valid.
* @throws AccessDeniedException
* You don't have permissions to perform the requested operation. The user or role that is making the
* request must have at least one IAM permissions policy attached that grants the required permissions.
* @sample AWSResilienceHub.ListUnsupportedAppVersionResources
* @see AWS API Documentation
*/
@Override
public ListUnsupportedAppVersionResourcesResult listUnsupportedAppVersionResources(ListUnsupportedAppVersionResourcesRequest request) {
request = beforeClientExecution(request);
return executeListUnsupportedAppVersionResources(request);
}
@SdkInternalApi
final ListUnsupportedAppVersionResourcesResult executeListUnsupportedAppVersionResources(
ListUnsupportedAppVersionResourcesRequest listUnsupportedAppVersionResourcesRequest) {
ExecutionContext executionContext = createExecutionContext(listUnsupportedAppVersionResourcesRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new ListUnsupportedAppVersionResourcesRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(listUnsupportedAppVersionResourcesRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "resiliencehub");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "ListUnsupportedAppVersionResources");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new ListUnsupportedAppVersionResourcesResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Publishes a new version of a specific Resilience Hub application.
*
*
* @param publishAppVersionRequest
* @return Result of the PublishAppVersion operation returned by the service.
* @throws InternalServerException
* This exception occurs when there is an internal failure in the Resilience Hub service.
* @throws ResourceNotFoundException
* This exception occurs when the specified resource could not be found.
* @throws ConflictException
* This exception occurs when a conflict with a previous successful write is detected. This generally occurs
* when the previous write did not have time to propagate to the host serving the current request. A retry
* (with appropriate backoff logic) is the recommended response to this exception.
* @throws ThrottlingException
* This exception occurs when you have exceeded the limit on the number of requests per second.
* @throws ValidationException
* This exception occurs when a request is not valid.
* @throws AccessDeniedException
* You don't have permissions to perform the requested operation. The user or role that is making the
* request must have at least one IAM permissions policy attached that grants the required permissions.
* @sample AWSResilienceHub.PublishAppVersion
* @see AWS API Documentation
*/
@Override
public PublishAppVersionResult publishAppVersion(PublishAppVersionRequest request) {
request = beforeClientExecution(request);
return executePublishAppVersion(request);
}
@SdkInternalApi
final PublishAppVersionResult executePublishAppVersion(PublishAppVersionRequest publishAppVersionRequest) {
ExecutionContext executionContext = createExecutionContext(publishAppVersionRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new PublishAppVersionRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(publishAppVersionRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "resiliencehub");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "PublishAppVersion");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new PublishAppVersionResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Adds or updates the app template for an Resilience Hub application draft version.
*
*
* @param putDraftAppVersionTemplateRequest
* @return Result of the PutDraftAppVersionTemplate operation returned by the service.
* @throws InternalServerException
* This exception occurs when there is an internal failure in the Resilience Hub service.
* @throws ResourceNotFoundException
* This exception occurs when the specified resource could not be found.
* @throws ConflictException
* This exception occurs when a conflict with a previous successful write is detected. This generally occurs
* when the previous write did not have time to propagate to the host serving the current request. A retry
* (with appropriate backoff logic) is the recommended response to this exception.
* @throws ThrottlingException
* This exception occurs when you have exceeded the limit on the number of requests per second.
* @throws ValidationException
* This exception occurs when a request is not valid.
* @throws AccessDeniedException
* You don't have permissions to perform the requested operation. The user or role that is making the
* request must have at least one IAM permissions policy attached that grants the required permissions.
* @sample AWSResilienceHub.PutDraftAppVersionTemplate
* @see AWS API Documentation
*/
@Override
public PutDraftAppVersionTemplateResult putDraftAppVersionTemplate(PutDraftAppVersionTemplateRequest request) {
request = beforeClientExecution(request);
return executePutDraftAppVersionTemplate(request);
}
@SdkInternalApi
final PutDraftAppVersionTemplateResult executePutDraftAppVersionTemplate(PutDraftAppVersionTemplateRequest putDraftAppVersionTemplateRequest) {
ExecutionContext executionContext = createExecutionContext(putDraftAppVersionTemplateRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new PutDraftAppVersionTemplateRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(putDraftAppVersionTemplateRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "resiliencehub");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "PutDraftAppVersionTemplate");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new PutDraftAppVersionTemplateResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Removes resource mappings from a draft application version.
*
*
* @param removeDraftAppVersionResourceMappingsRequest
* @return Result of the RemoveDraftAppVersionResourceMappings operation returned by the service.
* @throws InternalServerException
* This exception occurs when there is an internal failure in the Resilience Hub service.
* @throws ResourceNotFoundException
* This exception occurs when the specified resource could not be found.
* @throws ConflictException
* This exception occurs when a conflict with a previous successful write is detected. This generally occurs
* when the previous write did not have time to propagate to the host serving the current request. A retry
* (with appropriate backoff logic) is the recommended response to this exception.
* @throws ThrottlingException
* This exception occurs when you have exceeded the limit on the number of requests per second.
* @throws ValidationException
* This exception occurs when a request is not valid.
* @throws AccessDeniedException
* You don't have permissions to perform the requested operation. The user or role that is making the
* request must have at least one IAM permissions policy attached that grants the required permissions.
* @sample AWSResilienceHub.RemoveDraftAppVersionResourceMappings
* @see AWS API Documentation
*/
@Override
public RemoveDraftAppVersionResourceMappingsResult removeDraftAppVersionResourceMappings(RemoveDraftAppVersionResourceMappingsRequest request) {
request = beforeClientExecution(request);
return executeRemoveDraftAppVersionResourceMappings(request);
}
@SdkInternalApi
final RemoveDraftAppVersionResourceMappingsResult executeRemoveDraftAppVersionResourceMappings(
RemoveDraftAppVersionResourceMappingsRequest removeDraftAppVersionResourceMappingsRequest) {
ExecutionContext executionContext = createExecutionContext(removeDraftAppVersionResourceMappingsRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new RemoveDraftAppVersionResourceMappingsRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(removeDraftAppVersionResourceMappingsRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "resiliencehub");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "RemoveDraftAppVersionResourceMappings");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new RemoveDraftAppVersionResourceMappingsResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Resolves the resources for an application version.
*
*
* @param resolveAppVersionResourcesRequest
* @return Result of the ResolveAppVersionResources operation returned by the service.
* @throws InternalServerException
* This exception occurs when there is an internal failure in the Resilience Hub service.
* @throws ResourceNotFoundException
* This exception occurs when the specified resource could not be found.
* @throws ConflictException
* This exception occurs when a conflict with a previous successful write is detected. This generally occurs
* when the previous write did not have time to propagate to the host serving the current request. A retry
* (with appropriate backoff logic) is the recommended response to this exception.
* @throws ThrottlingException
* This exception occurs when you have exceeded the limit on the number of requests per second.
* @throws ValidationException
* This exception occurs when a request is not valid.
* @throws AccessDeniedException
* You don't have permissions to perform the requested operation. The user or role that is making the
* request must have at least one IAM permissions policy attached that grants the required permissions.
* @sample AWSResilienceHub.ResolveAppVersionResources
* @see AWS API Documentation
*/
@Override
public ResolveAppVersionResourcesResult resolveAppVersionResources(ResolveAppVersionResourcesRequest request) {
request = beforeClientExecution(request);
return executeResolveAppVersionResources(request);
}
@SdkInternalApi
final ResolveAppVersionResourcesResult executeResolveAppVersionResources(ResolveAppVersionResourcesRequest resolveAppVersionResourcesRequest) {
ExecutionContext executionContext = createExecutionContext(resolveAppVersionResourcesRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new ResolveAppVersionResourcesRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(resolveAppVersionResourcesRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "resiliencehub");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "ResolveAppVersionResources");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new ResolveAppVersionResourcesResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Creates a new application assessment for an application.
*
*
* @param startAppAssessmentRequest
* @return Result of the StartAppAssessment operation returned by the service.
* @throws InternalServerException
* This exception occurs when there is an internal failure in the Resilience Hub service.
* @throws ResourceNotFoundException
* This exception occurs when the specified resource could not be found.
* @throws ConflictException
* This exception occurs when a conflict with a previous successful write is detected. This generally occurs
* when the previous write did not have time to propagate to the host serving the current request. A retry
* (with appropriate backoff logic) is the recommended response to this exception.
* @throws ServiceQuotaExceededException
* This exception occurs when you have exceeded your service quota. To perform the requested action, remove
* some of the relevant resources, or use Service Quotas to request a service quota increase.
* @throws ThrottlingException
* This exception occurs when you have exceeded the limit on the number of requests per second.
* @throws ValidationException
* This exception occurs when a request is not valid.
* @throws AccessDeniedException
* You don't have permissions to perform the requested operation. The user or role that is making the
* request must have at least one IAM permissions policy attached that grants the required permissions.
* @sample AWSResilienceHub.StartAppAssessment
* @see AWS API Documentation
*/
@Override
public StartAppAssessmentResult startAppAssessment(StartAppAssessmentRequest request) {
request = beforeClientExecution(request);
return executeStartAppAssessment(request);
}
@SdkInternalApi
final StartAppAssessmentResult executeStartAppAssessment(StartAppAssessmentRequest startAppAssessmentRequest) {
ExecutionContext executionContext = createExecutionContext(startAppAssessmentRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new StartAppAssessmentRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(startAppAssessmentRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "resiliencehub");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "StartAppAssessment");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new StartAppAssessmentResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Applies one or more tags to a resource.
*
*
* @param tagResourceRequest
* @return Result of the TagResource operation returned by the service.
* @throws InternalServerException
* This exception occurs when there is an internal failure in the Resilience Hub service.
* @throws ResourceNotFoundException
* This exception occurs when the specified resource could not be found.
* @throws ThrottlingException
* This exception occurs when you have exceeded the limit on the number of requests per second.
* @throws ValidationException
* This exception occurs when a request is not valid.
* @throws AccessDeniedException
* You don't have permissions to perform the requested operation. The user or role that is making the
* request must have at least one IAM permissions policy attached that grants the required permissions.
* @sample AWSResilienceHub.TagResource
* @see AWS API
* Documentation
*/
@Override
public TagResourceResult tagResource(TagResourceRequest request) {
request = beforeClientExecution(request);
return executeTagResource(request);
}
@SdkInternalApi
final TagResourceResult executeTagResource(TagResourceRequest tagResourceRequest) {
ExecutionContext executionContext = createExecutionContext(tagResourceRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new TagResourceRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(tagResourceRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "resiliencehub");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "TagResource");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new TagResourceResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Removes one or more tags from a resource.
*
*
* @param untagResourceRequest
* @return Result of the UntagResource operation returned by the service.
* @throws InternalServerException
* This exception occurs when there is an internal failure in the Resilience Hub service.
* @throws ResourceNotFoundException
* This exception occurs when the specified resource could not be found.
* @throws ThrottlingException
* This exception occurs when you have exceeded the limit on the number of requests per second.
* @throws ValidationException
* This exception occurs when a request is not valid.
* @throws AccessDeniedException
* You don't have permissions to perform the requested operation. The user or role that is making the
* request must have at least one IAM permissions policy attached that grants the required permissions.
* @sample AWSResilienceHub.UntagResource
* @see AWS
* API Documentation
*/
@Override
public UntagResourceResult untagResource(UntagResourceRequest request) {
request = beforeClientExecution(request);
return executeUntagResource(request);
}
@SdkInternalApi
final UntagResourceResult executeUntagResource(UntagResourceRequest untagResourceRequest) {
ExecutionContext executionContext = createExecutionContext(untagResourceRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new UntagResourceRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(untagResourceRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "resiliencehub");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "UntagResource");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new UntagResourceResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Updates an application.
*
*
* @param updateAppRequest
* @return Result of the UpdateApp operation returned by the service.
* @throws InternalServerException
* This exception occurs when there is an internal failure in the Resilience Hub service.
* @throws ResourceNotFoundException
* This exception occurs when the specified resource could not be found.
* @throws ConflictException
* This exception occurs when a conflict with a previous successful write is detected. This generally occurs
* when the previous write did not have time to propagate to the host serving the current request. A retry
* (with appropriate backoff logic) is the recommended response to this exception.
* @throws ThrottlingException
* This exception occurs when you have exceeded the limit on the number of requests per second.
* @throws ValidationException
* This exception occurs when a request is not valid.
* @throws AccessDeniedException
* You don't have permissions to perform the requested operation. The user or role that is making the
* request must have at least one IAM permissions policy attached that grants the required permissions.
* @sample AWSResilienceHub.UpdateApp
* @see AWS API
* Documentation
*/
@Override
public UpdateAppResult updateApp(UpdateAppRequest request) {
request = beforeClientExecution(request);
return executeUpdateApp(request);
}
@SdkInternalApi
final UpdateAppResult executeUpdateApp(UpdateAppRequest updateAppRequest) {
ExecutionContext executionContext = createExecutionContext(updateAppRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new UpdateAppRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(updateAppRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "resiliencehub");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "UpdateApp");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(new JsonOperationMetadata()
.withPayloadJson(true).withHasStreamingSuccessResponse(false), new UpdateAppResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Updates the Resilience Hub application version.
*
*
*
* This API updates the Resilience Hub application draft version. To use this information for running resiliency
* assessments, you must publish the Resilience Hub application using the PublishAppVersion
API.
*
*
*
* @param updateAppVersionRequest
* @return Result of the UpdateAppVersion operation returned by the service.
* @throws InternalServerException
* This exception occurs when there is an internal failure in the Resilience Hub service.
* @throws ResourceNotFoundException
* This exception occurs when the specified resource could not be found.
* @throws ConflictException
* This exception occurs when a conflict with a previous successful write is detected. This generally occurs
* when the previous write did not have time to propagate to the host serving the current request. A retry
* (with appropriate backoff logic) is the recommended response to this exception.
* @throws ThrottlingException
* This exception occurs when you have exceeded the limit on the number of requests per second.
* @throws ValidationException
* This exception occurs when a request is not valid.
* @throws AccessDeniedException
* You don't have permissions to perform the requested operation. The user or role that is making the
* request must have at least one IAM permissions policy attached that grants the required permissions.
* @sample AWSResilienceHub.UpdateAppVersion
* @see AWS
* API Documentation
*/
@Override
public UpdateAppVersionResult updateAppVersion(UpdateAppVersionRequest request) {
request = beforeClientExecution(request);
return executeUpdateAppVersion(request);
}
@SdkInternalApi
final UpdateAppVersionResult executeUpdateAppVersion(UpdateAppVersionRequest updateAppVersionRequest) {
ExecutionContext executionContext = createExecutionContext(updateAppVersionRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new UpdateAppVersionRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(updateAppVersionRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "resiliencehub");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "UpdateAppVersion");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new UpdateAppVersionResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Updates an existing Application Component in the Resilience Hub application.
*
*
*
* This API updates the Resilience Hub application draft version. To use this Application Component for running
* assessments, you must publish the Resilience Hub application using the PublishAppVersion
API.
*
*
*
* @param updateAppVersionAppComponentRequest
* @return Result of the UpdateAppVersionAppComponent operation returned by the service.
* @throws InternalServerException
* This exception occurs when there is an internal failure in the Resilience Hub service.
* @throws ResourceNotFoundException
* This exception occurs when the specified resource could not be found.
* @throws ConflictException
* This exception occurs when a conflict with a previous successful write is detected. This generally occurs
* when the previous write did not have time to propagate to the host serving the current request. A retry
* (with appropriate backoff logic) is the recommended response to this exception.
* @throws ThrottlingException
* This exception occurs when you have exceeded the limit on the number of requests per second.
* @throws ValidationException
* This exception occurs when a request is not valid.
* @throws AccessDeniedException
* You don't have permissions to perform the requested operation. The user or role that is making the
* request must have at least one IAM permissions policy attached that grants the required permissions.
* @sample AWSResilienceHub.UpdateAppVersionAppComponent
* @see AWS API Documentation
*/
@Override
public UpdateAppVersionAppComponentResult updateAppVersionAppComponent(UpdateAppVersionAppComponentRequest request) {
request = beforeClientExecution(request);
return executeUpdateAppVersionAppComponent(request);
}
@SdkInternalApi
final UpdateAppVersionAppComponentResult executeUpdateAppVersionAppComponent(UpdateAppVersionAppComponentRequest updateAppVersionAppComponentRequest) {
ExecutionContext executionContext = createExecutionContext(updateAppVersionAppComponentRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new UpdateAppVersionAppComponentRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(updateAppVersionAppComponentRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "resiliencehub");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "UpdateAppVersionAppComponent");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new UpdateAppVersionAppComponentResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Updates the resource details in the Resilience Hub application.
*
*
*
* -
*
* This action has no effect outside Resilience Hub.
*
*
* -
*
* This API updates the Resilience Hub application draft version. To use this resource for running resiliency
* assessments, you must publish the Resilience Hub application using the PublishAppVersion
API.
*
*
* -
*
* To update application version with new physicalResourceID
, you must call
* ResolveAppVersionResources
API.
*
*
*
*
*
* @param updateAppVersionResourceRequest
* @return Result of the UpdateAppVersionResource operation returned by the service.
* @throws InternalServerException
* This exception occurs when there is an internal failure in the Resilience Hub service.
* @throws ResourceNotFoundException
* This exception occurs when the specified resource could not be found.
* @throws ConflictException
* This exception occurs when a conflict with a previous successful write is detected. This generally occurs
* when the previous write did not have time to propagate to the host serving the current request. A retry
* (with appropriate backoff logic) is the recommended response to this exception.
* @throws ServiceQuotaExceededException
* This exception occurs when you have exceeded your service quota. To perform the requested action, remove
* some of the relevant resources, or use Service Quotas to request a service quota increase.
* @throws ThrottlingException
* This exception occurs when you have exceeded the limit on the number of requests per second.
* @throws ValidationException
* This exception occurs when a request is not valid.
* @throws AccessDeniedException
* You don't have permissions to perform the requested operation. The user or role that is making the
* request must have at least one IAM permissions policy attached that grants the required permissions.
* @sample AWSResilienceHub.UpdateAppVersionResource
* @see AWS API Documentation
*/
@Override
public UpdateAppVersionResourceResult updateAppVersionResource(UpdateAppVersionResourceRequest request) {
request = beforeClientExecution(request);
return executeUpdateAppVersionResource(request);
}
@SdkInternalApi
final UpdateAppVersionResourceResult executeUpdateAppVersionResource(UpdateAppVersionResourceRequest updateAppVersionResourceRequest) {
ExecutionContext executionContext = createExecutionContext(updateAppVersionResourceRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new UpdateAppVersionResourceRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(updateAppVersionResourceRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "resiliencehub");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "UpdateAppVersionResource");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new UpdateAppVersionResourceResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Updates a resiliency policy.
*
*
*
* Resilience Hub allows you to provide a value of zero for rtoInSecs
and rpoInSecs
of
* your resiliency policy. But, while assessing your application, the lowest possible assessment result is near
* zero. Hence, if you provide value zero for rtoInSecs
and rpoInSecs
, the estimated
* workload RTO and estimated workload RPO result will be near zero and the Compliance status for your
* application will be set to Policy breached.
*
*
*
* @param updateResiliencyPolicyRequest
* @return Result of the UpdateResiliencyPolicy operation returned by the service.
* @throws InternalServerException
* This exception occurs when there is an internal failure in the Resilience Hub service.
* @throws ResourceNotFoundException
* This exception occurs when the specified resource could not be found.
* @throws ConflictException
* This exception occurs when a conflict with a previous successful write is detected. This generally occurs
* when the previous write did not have time to propagate to the host serving the current request. A retry
* (with appropriate backoff logic) is the recommended response to this exception.
* @throws ThrottlingException
* This exception occurs when you have exceeded the limit on the number of requests per second.
* @throws ValidationException
* This exception occurs when a request is not valid.
* @throws AccessDeniedException
* You don't have permissions to perform the requested operation. The user or role that is making the
* request must have at least one IAM permissions policy attached that grants the required permissions.
* @sample AWSResilienceHub.UpdateResiliencyPolicy
* @see AWS API Documentation
*/
@Override
public UpdateResiliencyPolicyResult updateResiliencyPolicy(UpdateResiliencyPolicyRequest request) {
request = beforeClientExecution(request);
return executeUpdateResiliencyPolicy(request);
}
@SdkInternalApi
final UpdateResiliencyPolicyResult executeUpdateResiliencyPolicy(UpdateResiliencyPolicyRequest updateResiliencyPolicyRequest) {
ExecutionContext executionContext = createExecutionContext(updateResiliencyPolicyRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new UpdateResiliencyPolicyRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(updateResiliencyPolicyRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "resiliencehub");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "UpdateResiliencyPolicy");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new UpdateResiliencyPolicyResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
* Returns additional metadata for a previously executed successful, request, typically used for debugging issues
* where a service isn't acting as expected. This data isn't considered part of the result data returned by an
* operation, so it's available through this separate, diagnostic interface.
*
* Response metadata is only cached for a limited period of time, so if you need to access this extra diagnostic
* information for an executed request, you should use this method to retrieve it as soon as possible after
* executing the request.
*
* @param request
* The originally executed request
*
* @return The response metadata for the specified request, or null if none is available.
*/
public ResponseMetadata getCachedResponseMetadata(AmazonWebServiceRequest request) {
return client.getResponseMetadataForRequest(request);
}
/**
* Normal invoke with authentication. Credentials are required and may be overriden at the request level.
**/
private Response invoke(Request request, HttpResponseHandler> responseHandler,
ExecutionContext executionContext) {
return invoke(request, responseHandler, executionContext, null, null);
}
/**
* Normal invoke with authentication. Credentials are required and may be overriden at the request level.
**/
private Response invoke(Request request, HttpResponseHandler> responseHandler,
ExecutionContext executionContext, URI cachedEndpoint, URI uriFromEndpointTrait) {
executionContext.setCredentialsProvider(CredentialUtils.getCredentialsProvider(request.getOriginalRequest(), awsCredentialsProvider));
return doInvoke(request, responseHandler, executionContext, cachedEndpoint, uriFromEndpointTrait);
}
/**
* Invoke with no authentication. Credentials are not required and any credentials set on the client or request will
* be ignored for this operation.
**/
private Response anonymousInvoke(Request request,
HttpResponseHandler> responseHandler, ExecutionContext executionContext) {
return doInvoke(request, responseHandler, executionContext, null, null);
}
/**
* Invoke the request using the http client. Assumes credentials (or lack thereof) have been configured in the
* ExecutionContext beforehand.
**/
private Response doInvoke(Request request, HttpResponseHandler> responseHandler,
ExecutionContext executionContext, URI discoveredEndpoint, URI uriFromEndpointTrait) {
if (discoveredEndpoint != null) {
request.setEndpoint(discoveredEndpoint);
request.getOriginalRequest().getRequestClientOptions().appendUserAgent("endpoint-discovery");
} else if (uriFromEndpointTrait != null) {
request.setEndpoint(uriFromEndpointTrait);
} else {
request.setEndpoint(endpoint);
}
request.setTimeOffset(timeOffset);
HttpResponseHandler errorResponseHandler = protocolFactory.createErrorResponseHandler(new JsonErrorResponseMetadata());
return client.execute(request, responseHandler, errorResponseHandler, executionContext);
}
@com.amazonaws.annotation.SdkInternalApi
static com.amazonaws.protocol.json.SdkJsonProtocolFactory getProtocolFactory() {
return protocolFactory;
}
@Override
public void shutdown() {
super.shutdown();
}
}