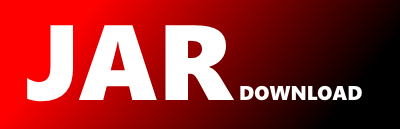
com.amazonaws.services.resourcegroups.AWSResourceGroupsAsync Maven / Gradle / Ivy
Show all versions of aws-java-sdk-resourcegroups Show documentation
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.resourcegroups;
import javax.annotation.Generated;
import com.amazonaws.services.resourcegroups.model.*;
/**
* Interface for accessing Resource Groups asynchronously. Each asynchronous method will return a Java Future object
* representing the asynchronous operation; overloads which accept an {@code AsyncHandler} can be used to receive
* notification when an asynchronous operation completes.
*
* Note: Do not directly implement this interface, new methods are added to it regularly. Extend from
* {@link com.amazonaws.services.resourcegroups.AbstractAWSResourceGroupsAsync} instead.
*
*
*
* Resource Groups lets you organize Amazon Web Services resources such as Amazon Elastic Compute Cloud instances,
* Amazon Relational Database Service databases, and Amazon Simple Storage Service buckets into groups using criteria
* that you define as tags. A resource group is a collection of resources that match the resource types specified in a
* query, and share one or more tags or portions of tags. You can create a group of resources based on their roles in
* your cloud infrastructure, lifecycle stages, regions, application layers, or virtually any criteria. Resource Groups
* enable you to automate management tasks, such as those in Amazon Web Services Systems Manager Automation documents,
* on tag-related resources in Amazon Web Services Systems Manager. Groups of tagged resources also let you quickly view
* a custom console in Amazon Web Services Systems Manager that shows Config compliance and other monitoring data about
* member resources.
*
*
* To create a resource group, build a resource query, and specify tags that identify the criteria that members of the
* group have in common. Tags are key-value pairs.
*
*
* For more information about Resource Groups, see the Resource Groups User Guide.
*
*
* Resource Groups uses a REST-compliant API that you can use to perform the following types of operations.
*
*
* -
*
* Create, Read, Update, and Delete (CRUD) operations on resource groups and resource query entities
*
*
* -
*
* Applying, editing, and removing tags from resource groups
*
*
* -
*
* Resolving resource group member ARNs so they can be returned as search results
*
*
* -
*
* Getting data about resources that are members of a group
*
*
* -
*
* Searching Amazon Web Services resources based on a resource query
*
*
*
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public interface AWSResourceGroupsAsync extends AWSResourceGroups {
/**
*
* Creates a resource group with the specified name and description. You can optionally include either a resource
* query or a service configuration. For more information about constructing a resource query, see Build queries and groups in
* Resource Groups in the Resource Groups User Guide. For more information about service-linked groups
* and service configurations, see Service configurations for Resource
* Groups.
*
*
* Minimum permissions
*
*
* To run this command, you must have the following permissions:
*
*
* -
*
* resource-groups:CreateGroup
*
*
*
*
* @param createGroupRequest
* @return A Java Future containing the result of the CreateGroup operation returned by the service.
* @sample AWSResourceGroupsAsync.CreateGroup
* @see AWS
* API Documentation
*/
java.util.concurrent.Future createGroupAsync(CreateGroupRequest createGroupRequest);
/**
*
* Creates a resource group with the specified name and description. You can optionally include either a resource
* query or a service configuration. For more information about constructing a resource query, see Build queries and groups in
* Resource Groups in the Resource Groups User Guide. For more information about service-linked groups
* and service configurations, see Service configurations for Resource
* Groups.
*
*
* Minimum permissions
*
*
* To run this command, you must have the following permissions:
*
*
* -
*
* resource-groups:CreateGroup
*
*
*
*
* @param createGroupRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateGroup operation returned by the service.
* @sample AWSResourceGroupsAsyncHandler.CreateGroup
* @see AWS
* API Documentation
*/
java.util.concurrent.Future createGroupAsync(CreateGroupRequest createGroupRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deletes the specified resource group. Deleting a resource group does not delete any resources that are members of
* the group; it only deletes the group structure.
*
*
* Minimum permissions
*
*
* To run this command, you must have the following permissions:
*
*
* -
*
* resource-groups:DeleteGroup
*
*
*
*
* @param deleteGroupRequest
* @return A Java Future containing the result of the DeleteGroup operation returned by the service.
* @sample AWSResourceGroupsAsync.DeleteGroup
* @see AWS
* API Documentation
*/
java.util.concurrent.Future deleteGroupAsync(DeleteGroupRequest deleteGroupRequest);
/**
*
* Deletes the specified resource group. Deleting a resource group does not delete any resources that are members of
* the group; it only deletes the group structure.
*
*
* Minimum permissions
*
*
* To run this command, you must have the following permissions:
*
*
* -
*
* resource-groups:DeleteGroup
*
*
*
*
* @param deleteGroupRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteGroup operation returned by the service.
* @sample AWSResourceGroupsAsyncHandler.DeleteGroup
* @see AWS
* API Documentation
*/
java.util.concurrent.Future deleteGroupAsync(DeleteGroupRequest deleteGroupRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Retrieves the current status of optional features in Resource Groups.
*
*
* @param getAccountSettingsRequest
* @return A Java Future containing the result of the GetAccountSettings operation returned by the service.
* @sample AWSResourceGroupsAsync.GetAccountSettings
* @see AWS API Documentation
*/
java.util.concurrent.Future getAccountSettingsAsync(GetAccountSettingsRequest getAccountSettingsRequest);
/**
*
* Retrieves the current status of optional features in Resource Groups.
*
*
* @param getAccountSettingsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetAccountSettings operation returned by the service.
* @sample AWSResourceGroupsAsyncHandler.GetAccountSettings
* @see AWS API Documentation
*/
java.util.concurrent.Future getAccountSettingsAsync(GetAccountSettingsRequest getAccountSettingsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns information about a specified resource group.
*
*
* Minimum permissions
*
*
* To run this command, you must have the following permissions:
*
*
* -
*
* resource-groups:GetGroup
*
*
*
*
* @param getGroupRequest
* @return A Java Future containing the result of the GetGroup operation returned by the service.
* @sample AWSResourceGroupsAsync.GetGroup
* @see AWS API
* Documentation
*/
java.util.concurrent.Future getGroupAsync(GetGroupRequest getGroupRequest);
/**
*
* Returns information about a specified resource group.
*
*
* Minimum permissions
*
*
* To run this command, you must have the following permissions:
*
*
* -
*
* resource-groups:GetGroup
*
*
*
*
* @param getGroupRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetGroup operation returned by the service.
* @sample AWSResourceGroupsAsyncHandler.GetGroup
* @see AWS API
* Documentation
*/
java.util.concurrent.Future getGroupAsync(GetGroupRequest getGroupRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Retrieves the service configuration associated with the specified resource group. For details about the service
* configuration syntax, see Service
* configurations for Resource Groups.
*
*
* Minimum permissions
*
*
* To run this command, you must have the following permissions:
*
*
* -
*
* resource-groups:GetGroupConfiguration
*
*
*
*
* @param getGroupConfigurationRequest
* @return A Java Future containing the result of the GetGroupConfiguration operation returned by the service.
* @sample AWSResourceGroupsAsync.GetGroupConfiguration
* @see AWS API Documentation
*/
java.util.concurrent.Future getGroupConfigurationAsync(GetGroupConfigurationRequest getGroupConfigurationRequest);
/**
*
* Retrieves the service configuration associated with the specified resource group. For details about the service
* configuration syntax, see Service
* configurations for Resource Groups.
*
*
* Minimum permissions
*
*
* To run this command, you must have the following permissions:
*
*
* -
*
* resource-groups:GetGroupConfiguration
*
*
*
*
* @param getGroupConfigurationRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetGroupConfiguration operation returned by the service.
* @sample AWSResourceGroupsAsyncHandler.GetGroupConfiguration
* @see AWS API Documentation
*/
java.util.concurrent.Future getGroupConfigurationAsync(GetGroupConfigurationRequest getGroupConfigurationRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Retrieves the resource query associated with the specified resource group. For more information about resource
* queries, see Create a tag-based group in Resource Groups.
*
*
* Minimum permissions
*
*
* To run this command, you must have the following permissions:
*
*
* -
*
* resource-groups:GetGroupQuery
*
*
*
*
* @param getGroupQueryRequest
* @return A Java Future containing the result of the GetGroupQuery operation returned by the service.
* @sample AWSResourceGroupsAsync.GetGroupQuery
* @see AWS
* API Documentation
*/
java.util.concurrent.Future getGroupQueryAsync(GetGroupQueryRequest getGroupQueryRequest);
/**
*
* Retrieves the resource query associated with the specified resource group. For more information about resource
* queries, see Create a tag-based group in Resource Groups.
*
*
* Minimum permissions
*
*
* To run this command, you must have the following permissions:
*
*
* -
*
* resource-groups:GetGroupQuery
*
*
*
*
* @param getGroupQueryRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetGroupQuery operation returned by the service.
* @sample AWSResourceGroupsAsyncHandler.GetGroupQuery
* @see AWS
* API Documentation
*/
java.util.concurrent.Future getGroupQueryAsync(GetGroupQueryRequest getGroupQueryRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns a list of tags that are associated with a resource group, specified by an ARN.
*
*
* Minimum permissions
*
*
* To run this command, you must have the following permissions:
*
*
* -
*
* resource-groups:GetTags
*
*
*
*
* @param getTagsRequest
* @return A Java Future containing the result of the GetTags operation returned by the service.
* @sample AWSResourceGroupsAsync.GetTags
* @see AWS API
* Documentation
*/
java.util.concurrent.Future getTagsAsync(GetTagsRequest getTagsRequest);
/**
*
* Returns a list of tags that are associated with a resource group, specified by an ARN.
*
*
* Minimum permissions
*
*
* To run this command, you must have the following permissions:
*
*
* -
*
* resource-groups:GetTags
*
*
*
*
* @param getTagsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetTags operation returned by the service.
* @sample AWSResourceGroupsAsyncHandler.GetTags
* @see AWS API
* Documentation
*/
java.util.concurrent.Future getTagsAsync(GetTagsRequest getTagsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Adds the specified resources to the specified group.
*
*
*
* You can use this operation with only resource groups that are configured with the following types:
*
*
* -
*
* AWS::EC2::HostManagement
*
*
* -
*
* AWS::EC2::CapacityReservationPool
*
*
*
*
* Other resource group type and resource types aren't currently supported by this operation.
*
*
*
* Minimum permissions
*
*
* To run this command, you must have the following permissions:
*
*
* -
*
* resource-groups:GroupResources
*
*
*
*
* @param groupResourcesRequest
* @return A Java Future containing the result of the GroupResources operation returned by the service.
* @sample AWSResourceGroupsAsync.GroupResources
* @see AWS
* API Documentation
*/
java.util.concurrent.Future groupResourcesAsync(GroupResourcesRequest groupResourcesRequest);
/**
*
* Adds the specified resources to the specified group.
*
*
*
* You can use this operation with only resource groups that are configured with the following types:
*
*
* -
*
* AWS::EC2::HostManagement
*
*
* -
*
* AWS::EC2::CapacityReservationPool
*
*
*
*
* Other resource group type and resource types aren't currently supported by this operation.
*
*
*
* Minimum permissions
*
*
* To run this command, you must have the following permissions:
*
*
* -
*
* resource-groups:GroupResources
*
*
*
*
* @param groupResourcesRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GroupResources operation returned by the service.
* @sample AWSResourceGroupsAsyncHandler.GroupResources
* @see AWS
* API Documentation
*/
java.util.concurrent.Future groupResourcesAsync(GroupResourcesRequest groupResourcesRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns a list of ARNs of the resources that are members of a specified resource group.
*
*
* Minimum permissions
*
*
* To run this command, you must have the following permissions:
*
*
* -
*
* resource-groups:ListGroupResources
*
*
* -
*
* cloudformation:DescribeStacks
*
*
* -
*
* cloudformation:ListStackResources
*
*
* -
*
* tag:GetResources
*
*
*
*
* @param listGroupResourcesRequest
* @return A Java Future containing the result of the ListGroupResources operation returned by the service.
* @sample AWSResourceGroupsAsync.ListGroupResources
* @see AWS API Documentation
*/
java.util.concurrent.Future listGroupResourcesAsync(ListGroupResourcesRequest listGroupResourcesRequest);
/**
*
* Returns a list of ARNs of the resources that are members of a specified resource group.
*
*
* Minimum permissions
*
*
* To run this command, you must have the following permissions:
*
*
* -
*
* resource-groups:ListGroupResources
*
*
* -
*
* cloudformation:DescribeStacks
*
*
* -
*
* cloudformation:ListStackResources
*
*
* -
*
* tag:GetResources
*
*
*
*
* @param listGroupResourcesRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListGroupResources operation returned by the service.
* @sample AWSResourceGroupsAsyncHandler.ListGroupResources
* @see AWS API Documentation
*/
java.util.concurrent.Future listGroupResourcesAsync(ListGroupResourcesRequest listGroupResourcesRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns a list of existing Resource Groups in your account.
*
*
* Minimum permissions
*
*
* To run this command, you must have the following permissions:
*
*
* -
*
* resource-groups:ListGroups
*
*
*
*
* @param listGroupsRequest
* @return A Java Future containing the result of the ListGroups operation returned by the service.
* @sample AWSResourceGroupsAsync.ListGroups
* @see AWS API
* Documentation
*/
java.util.concurrent.Future listGroupsAsync(ListGroupsRequest listGroupsRequest);
/**
*
* Returns a list of existing Resource Groups in your account.
*
*
* Minimum permissions
*
*
* To run this command, you must have the following permissions:
*
*
* -
*
* resource-groups:ListGroups
*
*
*
*
* @param listGroupsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListGroups operation returned by the service.
* @sample AWSResourceGroupsAsyncHandler.ListGroups
* @see AWS API
* Documentation
*/
java.util.concurrent.Future listGroupsAsync(ListGroupsRequest listGroupsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Attaches a service configuration to the specified group. This occurs asynchronously, and can take time to
* complete. You can use GetGroupConfiguration to check the status of the update.
*
*
* Minimum permissions
*
*
* To run this command, you must have the following permissions:
*
*
* -
*
* resource-groups:PutGroupConfiguration
*
*
*
*
* @param putGroupConfigurationRequest
* @return A Java Future containing the result of the PutGroupConfiguration operation returned by the service.
* @sample AWSResourceGroupsAsync.PutGroupConfiguration
* @see AWS API Documentation
*/
java.util.concurrent.Future putGroupConfigurationAsync(PutGroupConfigurationRequest putGroupConfigurationRequest);
/**
*
* Attaches a service configuration to the specified group. This occurs asynchronously, and can take time to
* complete. You can use GetGroupConfiguration to check the status of the update.
*
*
* Minimum permissions
*
*
* To run this command, you must have the following permissions:
*
*
* -
*
* resource-groups:PutGroupConfiguration
*
*
*
*
* @param putGroupConfigurationRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the PutGroupConfiguration operation returned by the service.
* @sample AWSResourceGroupsAsyncHandler.PutGroupConfiguration
* @see AWS API Documentation
*/
java.util.concurrent.Future putGroupConfigurationAsync(PutGroupConfigurationRequest putGroupConfigurationRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns a list of Amazon Web Services resource identifiers that matches the specified query. The query uses the
* same format as a resource query in a CreateGroup or UpdateGroupQuery operation.
*
*
* Minimum permissions
*
*
* To run this command, you must have the following permissions:
*
*
* -
*
* resource-groups:SearchResources
*
*
* -
*
* cloudformation:DescribeStacks
*
*
* -
*
* cloudformation:ListStackResources
*
*
* -
*
* tag:GetResources
*
*
*
*
* @param searchResourcesRequest
* @return A Java Future containing the result of the SearchResources operation returned by the service.
* @sample AWSResourceGroupsAsync.SearchResources
* @see AWS API Documentation
*/
java.util.concurrent.Future searchResourcesAsync(SearchResourcesRequest searchResourcesRequest);
/**
*
* Returns a list of Amazon Web Services resource identifiers that matches the specified query. The query uses the
* same format as a resource query in a CreateGroup or UpdateGroupQuery operation.
*
*
* Minimum permissions
*
*
* To run this command, you must have the following permissions:
*
*
* -
*
* resource-groups:SearchResources
*
*
* -
*
* cloudformation:DescribeStacks
*
*
* -
*
* cloudformation:ListStackResources
*
*
* -
*
* tag:GetResources
*
*
*
*
* @param searchResourcesRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the SearchResources operation returned by the service.
* @sample AWSResourceGroupsAsyncHandler.SearchResources
* @see AWS API Documentation
*/
java.util.concurrent.Future searchResourcesAsync(SearchResourcesRequest searchResourcesRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Adds tags to a resource group with the specified ARN. Existing tags on a resource group are not changed if they
* are not specified in the request parameters.
*
*
*
* Do not store personally identifiable information (PII) or other confidential or sensitive information in tags. We
* use tags to provide you with billing and administration services. Tags are not intended to be used for private or
* sensitive data.
*
*
*
* Minimum permissions
*
*
* To run this command, you must have the following permissions:
*
*
* -
*
* resource-groups:Tag
*
*
*
*
* @param tagRequest
* @return A Java Future containing the result of the Tag operation returned by the service.
* @sample AWSResourceGroupsAsync.Tag
* @see AWS API
* Documentation
*/
java.util.concurrent.Future tagAsync(TagRequest tagRequest);
/**
*
* Adds tags to a resource group with the specified ARN. Existing tags on a resource group are not changed if they
* are not specified in the request parameters.
*
*
*
* Do not store personally identifiable information (PII) or other confidential or sensitive information in tags. We
* use tags to provide you with billing and administration services. Tags are not intended to be used for private or
* sensitive data.
*
*
*
* Minimum permissions
*
*
* To run this command, you must have the following permissions:
*
*
* -
*
* resource-groups:Tag
*
*
*
*
* @param tagRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the Tag operation returned by the service.
* @sample AWSResourceGroupsAsyncHandler.Tag
* @see AWS API
* Documentation
*/
java.util.concurrent.Future tagAsync(TagRequest tagRequest, com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Removes the specified resources from the specified group. This operation works only with static groups that you
* populated using the GroupResources operation. It doesn't work with any resource groups that are
* automatically populated by tag-based or CloudFormation stack-based queries.
*
*
* Minimum permissions
*
*
* To run this command, you must have the following permissions:
*
*
* -
*
* resource-groups:UngroupResources
*
*
*
*
* @param ungroupResourcesRequest
* @return A Java Future containing the result of the UngroupResources operation returned by the service.
* @sample AWSResourceGroupsAsync.UngroupResources
* @see AWS API Documentation
*/
java.util.concurrent.Future ungroupResourcesAsync(UngroupResourcesRequest ungroupResourcesRequest);
/**
*
* Removes the specified resources from the specified group. This operation works only with static groups that you
* populated using the GroupResources operation. It doesn't work with any resource groups that are
* automatically populated by tag-based or CloudFormation stack-based queries.
*
*
* Minimum permissions
*
*
* To run this command, you must have the following permissions:
*
*
* -
*
* resource-groups:UngroupResources
*
*
*
*
* @param ungroupResourcesRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the UngroupResources operation returned by the service.
* @sample AWSResourceGroupsAsyncHandler.UngroupResources
* @see AWS API Documentation
*/
java.util.concurrent.Future ungroupResourcesAsync(UngroupResourcesRequest ungroupResourcesRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deletes tags from a specified resource group.
*
*
* Minimum permissions
*
*
* To run this command, you must have the following permissions:
*
*
* -
*
* resource-groups:Untag
*
*
*
*
* @param untagRequest
* @return A Java Future containing the result of the Untag operation returned by the service.
* @sample AWSResourceGroupsAsync.Untag
* @see AWS API
* Documentation
*/
java.util.concurrent.Future untagAsync(UntagRequest untagRequest);
/**
*
* Deletes tags from a specified resource group.
*
*
* Minimum permissions
*
*
* To run this command, you must have the following permissions:
*
*
* -
*
* resource-groups:Untag
*
*
*
*
* @param untagRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the Untag operation returned by the service.
* @sample AWSResourceGroupsAsyncHandler.Untag
* @see AWS API
* Documentation
*/
java.util.concurrent.Future untagAsync(UntagRequest untagRequest, com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Turns on or turns off optional features in Resource Groups.
*
*
* The preceding example shows that the request to turn on group lifecycle events is IN_PROGRESS
. You
* can call the GetAccountSettings operation to check for completion by looking for
* GroupLifecycleEventsStatus
to change to ACTIVE
.
*
*
* @param updateAccountSettingsRequest
* @return A Java Future containing the result of the UpdateAccountSettings operation returned by the service.
* @sample AWSResourceGroupsAsync.UpdateAccountSettings
* @see AWS API Documentation
*/
java.util.concurrent.Future updateAccountSettingsAsync(UpdateAccountSettingsRequest updateAccountSettingsRequest);
/**
*
* Turns on or turns off optional features in Resource Groups.
*
*
* The preceding example shows that the request to turn on group lifecycle events is IN_PROGRESS
. You
* can call the GetAccountSettings operation to check for completion by looking for
* GroupLifecycleEventsStatus
to change to ACTIVE
.
*
*
* @param updateAccountSettingsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the UpdateAccountSettings operation returned by the service.
* @sample AWSResourceGroupsAsyncHandler.UpdateAccountSettings
* @see AWS API Documentation
*/
java.util.concurrent.Future updateAccountSettingsAsync(UpdateAccountSettingsRequest updateAccountSettingsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Updates the description for an existing group. You cannot update the name of a resource group.
*
*
* Minimum permissions
*
*
* To run this command, you must have the following permissions:
*
*
* -
*
* resource-groups:UpdateGroup
*
*
*
*
* @param updateGroupRequest
* @return A Java Future containing the result of the UpdateGroup operation returned by the service.
* @sample AWSResourceGroupsAsync.UpdateGroup
* @see AWS
* API Documentation
*/
java.util.concurrent.Future updateGroupAsync(UpdateGroupRequest updateGroupRequest);
/**
*
* Updates the description for an existing group. You cannot update the name of a resource group.
*
*
* Minimum permissions
*
*
* To run this command, you must have the following permissions:
*
*
* -
*
* resource-groups:UpdateGroup
*
*
*
*
* @param updateGroupRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the UpdateGroup operation returned by the service.
* @sample AWSResourceGroupsAsyncHandler.UpdateGroup
* @see AWS
* API Documentation
*/
java.util.concurrent.Future updateGroupAsync(UpdateGroupRequest updateGroupRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Updates the resource query of a group. For more information about resource queries, see Create a tag-based group in Resource Groups.
*
*
* Minimum permissions
*
*
* To run this command, you must have the following permissions:
*
*
* -
*
* resource-groups:UpdateGroupQuery
*
*
*
*
* @param updateGroupQueryRequest
* @return A Java Future containing the result of the UpdateGroupQuery operation returned by the service.
* @sample AWSResourceGroupsAsync.UpdateGroupQuery
* @see AWS API Documentation
*/
java.util.concurrent.Future updateGroupQueryAsync(UpdateGroupQueryRequest updateGroupQueryRequest);
/**
*
* Updates the resource query of a group. For more information about resource queries, see Create a tag-based group in Resource Groups.
*
*
* Minimum permissions
*
*
* To run this command, you must have the following permissions:
*
*
* -
*
* resource-groups:UpdateGroupQuery
*
*
*
*
* @param updateGroupQueryRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the UpdateGroupQuery operation returned by the service.
* @sample AWSResourceGroupsAsyncHandler.UpdateGroupQuery
* @see AWS API Documentation
*/
java.util.concurrent.Future updateGroupQueryAsync(UpdateGroupQueryRequest updateGroupQueryRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
}