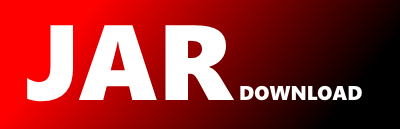
com.amazonaws.services.resourcegroups.AWSResourceGroupsClient Maven / Gradle / Ivy
Show all versions of aws-java-sdk-resourcegroups Show documentation
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.resourcegroups;
import org.w3c.dom.*;
import java.net.*;
import java.util.*;
import javax.annotation.Generated;
import org.apache.commons.logging.*;
import com.amazonaws.*;
import com.amazonaws.annotation.SdkInternalApi;
import com.amazonaws.auth.*;
import com.amazonaws.handlers.*;
import com.amazonaws.http.*;
import com.amazonaws.internal.*;
import com.amazonaws.internal.auth.*;
import com.amazonaws.metrics.*;
import com.amazonaws.regions.*;
import com.amazonaws.transform.*;
import com.amazonaws.util.*;
import com.amazonaws.protocol.json.*;
import com.amazonaws.util.AWSRequestMetrics.Field;
import com.amazonaws.annotation.ThreadSafe;
import com.amazonaws.client.AwsSyncClientParams;
import com.amazonaws.client.builder.AdvancedConfig;
import com.amazonaws.services.resourcegroups.AWSResourceGroupsClientBuilder;
import com.amazonaws.AmazonServiceException;
import com.amazonaws.services.resourcegroups.model.*;
import com.amazonaws.services.resourcegroups.model.transform.*;
/**
* Client for accessing Resource Groups. All service calls made using this client are blocking, and will not return
* until the service call completes.
*
*
* Resource Groups lets you organize Amazon Web Services resources such as Amazon Elastic Compute Cloud instances,
* Amazon Relational Database Service databases, and Amazon Simple Storage Service buckets into groups using criteria
* that you define as tags. A resource group is a collection of resources that match the resource types specified in a
* query, and share one or more tags or portions of tags. You can create a group of resources based on their roles in
* your cloud infrastructure, lifecycle stages, regions, application layers, or virtually any criteria. Resource Groups
* enable you to automate management tasks, such as those in Amazon Web Services Systems Manager Automation documents,
* on tag-related resources in Amazon Web Services Systems Manager. Groups of tagged resources also let you quickly view
* a custom console in Amazon Web Services Systems Manager that shows Config compliance and other monitoring data about
* member resources.
*
*
* To create a resource group, build a resource query, and specify tags that identify the criteria that members of the
* group have in common. Tags are key-value pairs.
*
*
* For more information about Resource Groups, see the Resource Groups User Guide.
*
*
* Resource Groups uses a REST-compliant API that you can use to perform the following types of operations.
*
*
* -
*
* Create, Read, Update, and Delete (CRUD) operations on resource groups and resource query entities
*
*
* -
*
* Applying, editing, and removing tags from resource groups
*
*
* -
*
* Resolving resource group member ARNs so they can be returned as search results
*
*
* -
*
* Getting data about resources that are members of a group
*
*
* -
*
* Searching Amazon Web Services resources based on a resource query
*
*
*
*/
@ThreadSafe
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class AWSResourceGroupsClient extends AmazonWebServiceClient implements AWSResourceGroups {
/** Provider for AWS credentials. */
private final AWSCredentialsProvider awsCredentialsProvider;
private static final Log log = LogFactory.getLog(AWSResourceGroups.class);
/** Default signing name for the service. */
private static final String DEFAULT_SIGNING_NAME = "resource-groups";
/** Client configuration factory providing ClientConfigurations tailored to this client */
protected static final ClientConfigurationFactory configFactory = new ClientConfigurationFactory();
private final AdvancedConfig advancedConfig;
private static final com.amazonaws.protocol.json.SdkJsonProtocolFactory protocolFactory = new com.amazonaws.protocol.json.SdkJsonProtocolFactory(
new JsonClientMetadata()
.withProtocolVersion("1.1")
.withSupportsCbor(false)
.withSupportsIon(false)
.withContentTypeOverride("application/json")
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("NotFoundException").withExceptionUnmarshaller(
com.amazonaws.services.resourcegroups.model.transform.NotFoundExceptionUnmarshaller.getInstance()))
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("UnauthorizedException").withExceptionUnmarshaller(
com.amazonaws.services.resourcegroups.model.transform.UnauthorizedExceptionUnmarshaller.getInstance()))
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("ForbiddenException").withExceptionUnmarshaller(
com.amazonaws.services.resourcegroups.model.transform.ForbiddenExceptionUnmarshaller.getInstance()))
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("MethodNotAllowedException").withExceptionUnmarshaller(
com.amazonaws.services.resourcegroups.model.transform.MethodNotAllowedExceptionUnmarshaller.getInstance()))
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("TooManyRequestsException").withExceptionUnmarshaller(
com.amazonaws.services.resourcegroups.model.transform.TooManyRequestsExceptionUnmarshaller.getInstance()))
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("BadRequestException").withExceptionUnmarshaller(
com.amazonaws.services.resourcegroups.model.transform.BadRequestExceptionUnmarshaller.getInstance()))
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("InternalServerErrorException").withExceptionUnmarshaller(
com.amazonaws.services.resourcegroups.model.transform.InternalServerErrorExceptionUnmarshaller.getInstance()))
.withBaseServiceExceptionClass(com.amazonaws.services.resourcegroups.model.AWSResourceGroupsException.class));
public static AWSResourceGroupsClientBuilder builder() {
return AWSResourceGroupsClientBuilder.standard();
}
/**
* Constructs a new client to invoke service methods on Resource Groups using the specified parameters.
*
*
* All service calls made using this new client object are blocking, and will not return until the service call
* completes.
*
* @param clientParams
* Object providing client parameters.
*/
AWSResourceGroupsClient(AwsSyncClientParams clientParams) {
this(clientParams, false);
}
/**
* Constructs a new client to invoke service methods on Resource Groups using the specified parameters.
*
*
* All service calls made using this new client object are blocking, and will not return until the service call
* completes.
*
* @param clientParams
* Object providing client parameters.
*/
AWSResourceGroupsClient(AwsSyncClientParams clientParams, boolean endpointDiscoveryEnabled) {
super(clientParams);
this.awsCredentialsProvider = clientParams.getCredentialsProvider();
this.advancedConfig = clientParams.getAdvancedConfig();
init();
}
private void init() {
setServiceNameIntern(DEFAULT_SIGNING_NAME);
setEndpointPrefix(ENDPOINT_PREFIX);
// calling this.setEndPoint(...) will also modify the signer accordingly
setEndpoint("resource-groups.us-east-1.amazonaws.com");
HandlerChainFactory chainFactory = new HandlerChainFactory();
requestHandler2s.addAll(chainFactory.newRequestHandlerChain("/com/amazonaws/services/resourcegroups/request.handlers"));
requestHandler2s.addAll(chainFactory.newRequestHandler2Chain("/com/amazonaws/services/resourcegroups/request.handler2s"));
requestHandler2s.addAll(chainFactory.getGlobalHandlers());
}
/**
*
* Creates a resource group with the specified name and description. You can optionally include either a resource
* query or a service configuration. For more information about constructing a resource query, see Build queries and groups in
* Resource Groups in the Resource Groups User Guide. For more information about service-linked groups
* and service configurations, see Service configurations for Resource
* Groups.
*
*
* Minimum permissions
*
*
* To run this command, you must have the following permissions:
*
*
* -
*
* resource-groups:CreateGroup
*
*
*
*
* @param createGroupRequest
* @return Result of the CreateGroup operation returned by the service.
* @throws BadRequestException
* The request includes one or more parameters that violate validation rules.
* @throws ForbiddenException
* The caller isn't authorized to make the request. Check permissions.
* @throws MethodNotAllowedException
* The request uses an HTTP method that isn't allowed for the specified resource.
* @throws TooManyRequestsException
* You've exceeded throttling limits by making too many requests in a period of time.
* @throws InternalServerErrorException
* An internal error occurred while processing the request. Try again later.
* @sample AWSResourceGroups.CreateGroup
* @see AWS
* API Documentation
*/
@Override
public CreateGroupResult createGroup(CreateGroupRequest request) {
request = beforeClientExecution(request);
return executeCreateGroup(request);
}
@SdkInternalApi
final CreateGroupResult executeCreateGroup(CreateGroupRequest createGroupRequest) {
ExecutionContext executionContext = createExecutionContext(createGroupRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new CreateGroupRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(createGroupRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Resource Groups");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "CreateGroup");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new CreateGroupResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Deletes the specified resource group. Deleting a resource group does not delete any resources that are members of
* the group; it only deletes the group structure.
*
*
* Minimum permissions
*
*
* To run this command, you must have the following permissions:
*
*
* -
*
* resource-groups:DeleteGroup
*
*
*
*
* @param deleteGroupRequest
* @return Result of the DeleteGroup operation returned by the service.
* @throws BadRequestException
* The request includes one or more parameters that violate validation rules.
* @throws ForbiddenException
* The caller isn't authorized to make the request. Check permissions.
* @throws NotFoundException
* One or more of the specified resources don't exist.
* @throws MethodNotAllowedException
* The request uses an HTTP method that isn't allowed for the specified resource.
* @throws TooManyRequestsException
* You've exceeded throttling limits by making too many requests in a period of time.
* @throws InternalServerErrorException
* An internal error occurred while processing the request. Try again later.
* @sample AWSResourceGroups.DeleteGroup
* @see AWS
* API Documentation
*/
@Override
public DeleteGroupResult deleteGroup(DeleteGroupRequest request) {
request = beforeClientExecution(request);
return executeDeleteGroup(request);
}
@SdkInternalApi
final DeleteGroupResult executeDeleteGroup(DeleteGroupRequest deleteGroupRequest) {
ExecutionContext executionContext = createExecutionContext(deleteGroupRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DeleteGroupRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(deleteGroupRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Resource Groups");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DeleteGroup");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new DeleteGroupResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Retrieves the current status of optional features in Resource Groups.
*
*
* @param getAccountSettingsRequest
* @return Result of the GetAccountSettings operation returned by the service.
* @throws BadRequestException
* The request includes one or more parameters that violate validation rules.
* @throws ForbiddenException
* The caller isn't authorized to make the request. Check permissions.
* @throws MethodNotAllowedException
* The request uses an HTTP method that isn't allowed for the specified resource.
* @throws TooManyRequestsException
* You've exceeded throttling limits by making too many requests in a period of time.
* @throws InternalServerErrorException
* An internal error occurred while processing the request. Try again later.
* @sample AWSResourceGroups.GetAccountSettings
* @see AWS API Documentation
*/
@Override
public GetAccountSettingsResult getAccountSettings(GetAccountSettingsRequest request) {
request = beforeClientExecution(request);
return executeGetAccountSettings(request);
}
@SdkInternalApi
final GetAccountSettingsResult executeGetAccountSettings(GetAccountSettingsRequest getAccountSettingsRequest) {
ExecutionContext executionContext = createExecutionContext(getAccountSettingsRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new GetAccountSettingsRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(getAccountSettingsRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Resource Groups");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "GetAccountSettings");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new GetAccountSettingsResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Returns information about a specified resource group.
*
*
* Minimum permissions
*
*
* To run this command, you must have the following permissions:
*
*
* -
*
* resource-groups:GetGroup
*
*
*
*
* @param getGroupRequest
* @return Result of the GetGroup operation returned by the service.
* @throws BadRequestException
* The request includes one or more parameters that violate validation rules.
* @throws ForbiddenException
* The caller isn't authorized to make the request. Check permissions.
* @throws NotFoundException
* One or more of the specified resources don't exist.
* @throws MethodNotAllowedException
* The request uses an HTTP method that isn't allowed for the specified resource.
* @throws TooManyRequestsException
* You've exceeded throttling limits by making too many requests in a period of time.
* @throws InternalServerErrorException
* An internal error occurred while processing the request. Try again later.
* @sample AWSResourceGroups.GetGroup
* @see AWS API
* Documentation
*/
@Override
public GetGroupResult getGroup(GetGroupRequest request) {
request = beforeClientExecution(request);
return executeGetGroup(request);
}
@SdkInternalApi
final GetGroupResult executeGetGroup(GetGroupRequest getGroupRequest) {
ExecutionContext executionContext = createExecutionContext(getGroupRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new GetGroupRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(getGroupRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Resource Groups");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "GetGroup");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(new JsonOperationMetadata()
.withPayloadJson(true).withHasStreamingSuccessResponse(false), new GetGroupResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Retrieves the service configuration associated with the specified resource group. For details about the service
* configuration syntax, see Service
* configurations for Resource Groups.
*
*
* Minimum permissions
*
*
* To run this command, you must have the following permissions:
*
*
* -
*
* resource-groups:GetGroupConfiguration
*
*
*
*
* @param getGroupConfigurationRequest
* @return Result of the GetGroupConfiguration operation returned by the service.
* @throws BadRequestException
* The request includes one or more parameters that violate validation rules.
* @throws ForbiddenException
* The caller isn't authorized to make the request. Check permissions.
* @throws NotFoundException
* One or more of the specified resources don't exist.
* @throws MethodNotAllowedException
* The request uses an HTTP method that isn't allowed for the specified resource.
* @throws TooManyRequestsException
* You've exceeded throttling limits by making too many requests in a period of time.
* @throws InternalServerErrorException
* An internal error occurred while processing the request. Try again later.
* @sample AWSResourceGroups.GetGroupConfiguration
* @see AWS API Documentation
*/
@Override
public GetGroupConfigurationResult getGroupConfiguration(GetGroupConfigurationRequest request) {
request = beforeClientExecution(request);
return executeGetGroupConfiguration(request);
}
@SdkInternalApi
final GetGroupConfigurationResult executeGetGroupConfiguration(GetGroupConfigurationRequest getGroupConfigurationRequest) {
ExecutionContext executionContext = createExecutionContext(getGroupConfigurationRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new GetGroupConfigurationRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(getGroupConfigurationRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Resource Groups");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "GetGroupConfiguration");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory
.createResponseHandler(new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new GetGroupConfigurationResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Retrieves the resource query associated with the specified resource group. For more information about resource
* queries, see Create a tag-based group in Resource Groups.
*
*
* Minimum permissions
*
*
* To run this command, you must have the following permissions:
*
*
* -
*
* resource-groups:GetGroupQuery
*
*
*
*
* @param getGroupQueryRequest
* @return Result of the GetGroupQuery operation returned by the service.
* @throws BadRequestException
* The request includes one or more parameters that violate validation rules.
* @throws ForbiddenException
* The caller isn't authorized to make the request. Check permissions.
* @throws NotFoundException
* One or more of the specified resources don't exist.
* @throws MethodNotAllowedException
* The request uses an HTTP method that isn't allowed for the specified resource.
* @throws TooManyRequestsException
* You've exceeded throttling limits by making too many requests in a period of time.
* @throws InternalServerErrorException
* An internal error occurred while processing the request. Try again later.
* @sample AWSResourceGroups.GetGroupQuery
* @see AWS
* API Documentation
*/
@Override
public GetGroupQueryResult getGroupQuery(GetGroupQueryRequest request) {
request = beforeClientExecution(request);
return executeGetGroupQuery(request);
}
@SdkInternalApi
final GetGroupQueryResult executeGetGroupQuery(GetGroupQueryRequest getGroupQueryRequest) {
ExecutionContext executionContext = createExecutionContext(getGroupQueryRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new GetGroupQueryRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(getGroupQueryRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Resource Groups");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "GetGroupQuery");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new GetGroupQueryResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Returns a list of tags that are associated with a resource group, specified by an ARN.
*
*
* Minimum permissions
*
*
* To run this command, you must have the following permissions:
*
*
* -
*
* resource-groups:GetTags
*
*
*
*
* @param getTagsRequest
* @return Result of the GetTags operation returned by the service.
* @throws BadRequestException
* The request includes one or more parameters that violate validation rules.
* @throws ForbiddenException
* The caller isn't authorized to make the request. Check permissions.
* @throws NotFoundException
* One or more of the specified resources don't exist.
* @throws MethodNotAllowedException
* The request uses an HTTP method that isn't allowed for the specified resource.
* @throws TooManyRequestsException
* You've exceeded throttling limits by making too many requests in a period of time.
* @throws InternalServerErrorException
* An internal error occurred while processing the request. Try again later.
* @sample AWSResourceGroups.GetTags
* @see AWS API
* Documentation
*/
@Override
public GetTagsResult getTags(GetTagsRequest request) {
request = beforeClientExecution(request);
return executeGetTags(request);
}
@SdkInternalApi
final GetTagsResult executeGetTags(GetTagsRequest getTagsRequest) {
ExecutionContext executionContext = createExecutionContext(getTagsRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new GetTagsRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(getTagsRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Resource Groups");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "GetTags");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(new JsonOperationMetadata()
.withPayloadJson(true).withHasStreamingSuccessResponse(false), new GetTagsResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Adds the specified resources to the specified group.
*
*
*
* You can use this operation with only resource groups that are configured with the following types:
*
*
* -
*
* AWS::EC2::HostManagement
*
*
* -
*
* AWS::EC2::CapacityReservationPool
*
*
*
*
* Other resource group type and resource types aren't currently supported by this operation.
*
*
*
* Minimum permissions
*
*
* To run this command, you must have the following permissions:
*
*
* -
*
* resource-groups:GroupResources
*
*
*
*
* @param groupResourcesRequest
* @return Result of the GroupResources operation returned by the service.
* @throws BadRequestException
* The request includes one or more parameters that violate validation rules.
* @throws ForbiddenException
* The caller isn't authorized to make the request. Check permissions.
* @throws NotFoundException
* One or more of the specified resources don't exist.
* @throws MethodNotAllowedException
* The request uses an HTTP method that isn't allowed for the specified resource.
* @throws TooManyRequestsException
* You've exceeded throttling limits by making too many requests in a period of time.
* @throws InternalServerErrorException
* An internal error occurred while processing the request. Try again later.
* @sample AWSResourceGroups.GroupResources
* @see AWS
* API Documentation
*/
@Override
public GroupResourcesResult groupResources(GroupResourcesRequest request) {
request = beforeClientExecution(request);
return executeGroupResources(request);
}
@SdkInternalApi
final GroupResourcesResult executeGroupResources(GroupResourcesRequest groupResourcesRequest) {
ExecutionContext executionContext = createExecutionContext(groupResourcesRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new GroupResourcesRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(groupResourcesRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Resource Groups");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "GroupResources");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new GroupResourcesResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Returns a list of ARNs of the resources that are members of a specified resource group.
*
*
* Minimum permissions
*
*
* To run this command, you must have the following permissions:
*
*
* -
*
* resource-groups:ListGroupResources
*
*
* -
*
* cloudformation:DescribeStacks
*
*
* -
*
* cloudformation:ListStackResources
*
*
* -
*
* tag:GetResources
*
*
*
*
* @param listGroupResourcesRequest
* @return Result of the ListGroupResources operation returned by the service.
* @throws UnauthorizedException
* The request was rejected because it doesn't have valid credentials for the target resource.
* @throws BadRequestException
* The request includes one or more parameters that violate validation rules.
* @throws ForbiddenException
* The caller isn't authorized to make the request. Check permissions.
* @throws NotFoundException
* One or more of the specified resources don't exist.
* @throws MethodNotAllowedException
* The request uses an HTTP method that isn't allowed for the specified resource.
* @throws TooManyRequestsException
* You've exceeded throttling limits by making too many requests in a period of time.
* @throws InternalServerErrorException
* An internal error occurred while processing the request. Try again later.
* @sample AWSResourceGroups.ListGroupResources
* @see AWS API Documentation
*/
@Override
public ListGroupResourcesResult listGroupResources(ListGroupResourcesRequest request) {
request = beforeClientExecution(request);
return executeListGroupResources(request);
}
@SdkInternalApi
final ListGroupResourcesResult executeListGroupResources(ListGroupResourcesRequest listGroupResourcesRequest) {
ExecutionContext executionContext = createExecutionContext(listGroupResourcesRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new ListGroupResourcesRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(listGroupResourcesRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Resource Groups");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "ListGroupResources");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new ListGroupResourcesResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Returns a list of existing Resource Groups in your account.
*
*
* Minimum permissions
*
*
* To run this command, you must have the following permissions:
*
*
* -
*
* resource-groups:ListGroups
*
*
*
*
* @param listGroupsRequest
* @return Result of the ListGroups operation returned by the service.
* @throws BadRequestException
* The request includes one or more parameters that violate validation rules.
* @throws ForbiddenException
* The caller isn't authorized to make the request. Check permissions.
* @throws MethodNotAllowedException
* The request uses an HTTP method that isn't allowed for the specified resource.
* @throws TooManyRequestsException
* You've exceeded throttling limits by making too many requests in a period of time.
* @throws InternalServerErrorException
* An internal error occurred while processing the request. Try again later.
* @sample AWSResourceGroups.ListGroups
* @see AWS API
* Documentation
*/
@Override
public ListGroupsResult listGroups(ListGroupsRequest request) {
request = beforeClientExecution(request);
return executeListGroups(request);
}
@SdkInternalApi
final ListGroupsResult executeListGroups(ListGroupsRequest listGroupsRequest) {
ExecutionContext executionContext = createExecutionContext(listGroupsRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new ListGroupsRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(listGroupsRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Resource Groups");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "ListGroups");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(new JsonOperationMetadata()
.withPayloadJson(true).withHasStreamingSuccessResponse(false), new ListGroupsResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Attaches a service configuration to the specified group. This occurs asynchronously, and can take time to
* complete. You can use GetGroupConfiguration to check the status of the update.
*
*
* Minimum permissions
*
*
* To run this command, you must have the following permissions:
*
*
* -
*
* resource-groups:PutGroupConfiguration
*
*
*
*
* @param putGroupConfigurationRequest
* @return Result of the PutGroupConfiguration operation returned by the service.
* @throws BadRequestException
* The request includes one or more parameters that violate validation rules.
* @throws ForbiddenException
* The caller isn't authorized to make the request. Check permissions.
* @throws NotFoundException
* One or more of the specified resources don't exist.
* @throws MethodNotAllowedException
* The request uses an HTTP method that isn't allowed for the specified resource.
* @throws TooManyRequestsException
* You've exceeded throttling limits by making too many requests in a period of time.
* @throws InternalServerErrorException
* An internal error occurred while processing the request. Try again later.
* @sample AWSResourceGroups.PutGroupConfiguration
* @see AWS API Documentation
*/
@Override
public PutGroupConfigurationResult putGroupConfiguration(PutGroupConfigurationRequest request) {
request = beforeClientExecution(request);
return executePutGroupConfiguration(request);
}
@SdkInternalApi
final PutGroupConfigurationResult executePutGroupConfiguration(PutGroupConfigurationRequest putGroupConfigurationRequest) {
ExecutionContext executionContext = createExecutionContext(putGroupConfigurationRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new PutGroupConfigurationRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(putGroupConfigurationRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Resource Groups");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "PutGroupConfiguration");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory
.createResponseHandler(new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new PutGroupConfigurationResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Returns a list of Amazon Web Services resource identifiers that matches the specified query. The query uses the
* same format as a resource query in a CreateGroup or UpdateGroupQuery operation.
*
*
* Minimum permissions
*
*
* To run this command, you must have the following permissions:
*
*
* -
*
* resource-groups:SearchResources
*
*
* -
*
* cloudformation:DescribeStacks
*
*
* -
*
* cloudformation:ListStackResources
*
*
* -
*
* tag:GetResources
*
*
*
*
* @param searchResourcesRequest
* @return Result of the SearchResources operation returned by the service.
* @throws UnauthorizedException
* The request was rejected because it doesn't have valid credentials for the target resource.
* @throws BadRequestException
* The request includes one or more parameters that violate validation rules.
* @throws ForbiddenException
* The caller isn't authorized to make the request. Check permissions.
* @throws MethodNotAllowedException
* The request uses an HTTP method that isn't allowed for the specified resource.
* @throws TooManyRequestsException
* You've exceeded throttling limits by making too many requests in a period of time.
* @throws InternalServerErrorException
* An internal error occurred while processing the request. Try again later.
* @sample AWSResourceGroups.SearchResources
* @see AWS API Documentation
*/
@Override
public SearchResourcesResult searchResources(SearchResourcesRequest request) {
request = beforeClientExecution(request);
return executeSearchResources(request);
}
@SdkInternalApi
final SearchResourcesResult executeSearchResources(SearchResourcesRequest searchResourcesRequest) {
ExecutionContext executionContext = createExecutionContext(searchResourcesRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new SearchResourcesRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(searchResourcesRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Resource Groups");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "SearchResources");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new SearchResourcesResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Adds tags to a resource group with the specified ARN. Existing tags on a resource group are not changed if they
* are not specified in the request parameters.
*
*
*
* Do not store personally identifiable information (PII) or other confidential or sensitive information in tags. We
* use tags to provide you with billing and administration services. Tags are not intended to be used for private or
* sensitive data.
*
*
*
* Minimum permissions
*
*
* To run this command, you must have the following permissions:
*
*
* -
*
* resource-groups:Tag
*
*
*
*
* @param tagRequest
* @return Result of the Tag operation returned by the service.
* @throws BadRequestException
* The request includes one or more parameters that violate validation rules.
* @throws ForbiddenException
* The caller isn't authorized to make the request. Check permissions.
* @throws NotFoundException
* One or more of the specified resources don't exist.
* @throws MethodNotAllowedException
* The request uses an HTTP method that isn't allowed for the specified resource.
* @throws TooManyRequestsException
* You've exceeded throttling limits by making too many requests in a period of time.
* @throws InternalServerErrorException
* An internal error occurred while processing the request. Try again later.
* @sample AWSResourceGroups.Tag
* @see AWS API
* Documentation
*/
@Override
public TagResult tag(TagRequest request) {
request = beforeClientExecution(request);
return executeTag(request);
}
@SdkInternalApi
final TagResult executeTag(TagRequest tagRequest) {
ExecutionContext executionContext = createExecutionContext(tagRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new TagRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(tagRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Resource Groups");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "Tag");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(new JsonOperationMetadata()
.withPayloadJson(true).withHasStreamingSuccessResponse(false), new TagResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Removes the specified resources from the specified group. This operation works only with static groups that you
* populated using the GroupResources operation. It doesn't work with any resource groups that are
* automatically populated by tag-based or CloudFormation stack-based queries.
*
*
* Minimum permissions
*
*
* To run this command, you must have the following permissions:
*
*
* -
*
* resource-groups:UngroupResources
*
*
*
*
* @param ungroupResourcesRequest
* @return Result of the UngroupResources operation returned by the service.
* @throws BadRequestException
* The request includes one or more parameters that violate validation rules.
* @throws ForbiddenException
* The caller isn't authorized to make the request. Check permissions.
* @throws NotFoundException
* One or more of the specified resources don't exist.
* @throws MethodNotAllowedException
* The request uses an HTTP method that isn't allowed for the specified resource.
* @throws TooManyRequestsException
* You've exceeded throttling limits by making too many requests in a period of time.
* @throws InternalServerErrorException
* An internal error occurred while processing the request. Try again later.
* @sample AWSResourceGroups.UngroupResources
* @see AWS API Documentation
*/
@Override
public UngroupResourcesResult ungroupResources(UngroupResourcesRequest request) {
request = beforeClientExecution(request);
return executeUngroupResources(request);
}
@SdkInternalApi
final UngroupResourcesResult executeUngroupResources(UngroupResourcesRequest ungroupResourcesRequest) {
ExecutionContext executionContext = createExecutionContext(ungroupResourcesRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new UngroupResourcesRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(ungroupResourcesRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Resource Groups");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "UngroupResources");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new UngroupResourcesResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Deletes tags from a specified resource group.
*
*
* Minimum permissions
*
*
* To run this command, you must have the following permissions:
*
*
* -
*
* resource-groups:Untag
*
*
*
*
* @param untagRequest
* @return Result of the Untag operation returned by the service.
* @throws BadRequestException
* The request includes one or more parameters that violate validation rules.
* @throws ForbiddenException
* The caller isn't authorized to make the request. Check permissions.
* @throws NotFoundException
* One or more of the specified resources don't exist.
* @throws MethodNotAllowedException
* The request uses an HTTP method that isn't allowed for the specified resource.
* @throws TooManyRequestsException
* You've exceeded throttling limits by making too many requests in a period of time.
* @throws InternalServerErrorException
* An internal error occurred while processing the request. Try again later.
* @sample AWSResourceGroups.Untag
* @see AWS API
* Documentation
*/
@Override
public UntagResult untag(UntagRequest request) {
request = beforeClientExecution(request);
return executeUntag(request);
}
@SdkInternalApi
final UntagResult executeUntag(UntagRequest untagRequest) {
ExecutionContext executionContext = createExecutionContext(untagRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new UntagRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(untagRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Resource Groups");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "Untag");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(new JsonOperationMetadata()
.withPayloadJson(true).withHasStreamingSuccessResponse(false), new UntagResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Turns on or turns off optional features in Resource Groups.
*
*
* The preceding example shows that the request to turn on group lifecycle events is IN_PROGRESS
. You
* can call the GetAccountSettings operation to check for completion by looking for
* GroupLifecycleEventsStatus
to change to ACTIVE
.
*
*
* @param updateAccountSettingsRequest
* @return Result of the UpdateAccountSettings operation returned by the service.
* @throws BadRequestException
* The request includes one or more parameters that violate validation rules.
* @throws ForbiddenException
* The caller isn't authorized to make the request. Check permissions.
* @throws MethodNotAllowedException
* The request uses an HTTP method that isn't allowed for the specified resource.
* @throws TooManyRequestsException
* You've exceeded throttling limits by making too many requests in a period of time.
* @throws InternalServerErrorException
* An internal error occurred while processing the request. Try again later.
* @sample AWSResourceGroups.UpdateAccountSettings
* @see AWS API Documentation
*/
@Override
public UpdateAccountSettingsResult updateAccountSettings(UpdateAccountSettingsRequest request) {
request = beforeClientExecution(request);
return executeUpdateAccountSettings(request);
}
@SdkInternalApi
final UpdateAccountSettingsResult executeUpdateAccountSettings(UpdateAccountSettingsRequest updateAccountSettingsRequest) {
ExecutionContext executionContext = createExecutionContext(updateAccountSettingsRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new UpdateAccountSettingsRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(updateAccountSettingsRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Resource Groups");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "UpdateAccountSettings");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory
.createResponseHandler(new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new UpdateAccountSettingsResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Updates the description for an existing group. You cannot update the name of a resource group.
*
*
* Minimum permissions
*
*
* To run this command, you must have the following permissions:
*
*
* -
*
* resource-groups:UpdateGroup
*
*
*
*
* @param updateGroupRequest
* @return Result of the UpdateGroup operation returned by the service.
* @throws BadRequestException
* The request includes one or more parameters that violate validation rules.
* @throws ForbiddenException
* The caller isn't authorized to make the request. Check permissions.
* @throws NotFoundException
* One or more of the specified resources don't exist.
* @throws MethodNotAllowedException
* The request uses an HTTP method that isn't allowed for the specified resource.
* @throws TooManyRequestsException
* You've exceeded throttling limits by making too many requests in a period of time.
* @throws InternalServerErrorException
* An internal error occurred while processing the request. Try again later.
* @sample AWSResourceGroups.UpdateGroup
* @see AWS
* API Documentation
*/
@Override
public UpdateGroupResult updateGroup(UpdateGroupRequest request) {
request = beforeClientExecution(request);
return executeUpdateGroup(request);
}
@SdkInternalApi
final UpdateGroupResult executeUpdateGroup(UpdateGroupRequest updateGroupRequest) {
ExecutionContext executionContext = createExecutionContext(updateGroupRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new UpdateGroupRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(updateGroupRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Resource Groups");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "UpdateGroup");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new UpdateGroupResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Updates the resource query of a group. For more information about resource queries, see Create a tag-based group in Resource Groups.
*
*
* Minimum permissions
*
*
* To run this command, you must have the following permissions:
*
*
* -
*
* resource-groups:UpdateGroupQuery
*
*
*
*
* @param updateGroupQueryRequest
* @return Result of the UpdateGroupQuery operation returned by the service.
* @throws BadRequestException
* The request includes one or more parameters that violate validation rules.
* @throws ForbiddenException
* The caller isn't authorized to make the request. Check permissions.
* @throws NotFoundException
* One or more of the specified resources don't exist.
* @throws MethodNotAllowedException
* The request uses an HTTP method that isn't allowed for the specified resource.
* @throws TooManyRequestsException
* You've exceeded throttling limits by making too many requests in a period of time.
* @throws InternalServerErrorException
* An internal error occurred while processing the request. Try again later.
* @sample AWSResourceGroups.UpdateGroupQuery
* @see AWS API Documentation
*/
@Override
public UpdateGroupQueryResult updateGroupQuery(UpdateGroupQueryRequest request) {
request = beforeClientExecution(request);
return executeUpdateGroupQuery(request);
}
@SdkInternalApi
final UpdateGroupQueryResult executeUpdateGroupQuery(UpdateGroupQueryRequest updateGroupQueryRequest) {
ExecutionContext executionContext = createExecutionContext(updateGroupQueryRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new UpdateGroupQueryRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(updateGroupQueryRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Resource Groups");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "UpdateGroupQuery");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new UpdateGroupQueryResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
* Returns additional metadata for a previously executed successful, request, typically used for debugging issues
* where a service isn't acting as expected. This data isn't considered part of the result data returned by an
* operation, so it's available through this separate, diagnostic interface.
*
* Response metadata is only cached for a limited period of time, so if you need to access this extra diagnostic
* information for an executed request, you should use this method to retrieve it as soon as possible after
* executing the request.
*
* @param request
* The originally executed request
*
* @return The response metadata for the specified request, or null if none is available.
*/
public ResponseMetadata getCachedResponseMetadata(AmazonWebServiceRequest request) {
return client.getResponseMetadataForRequest(request);
}
/**
* Normal invoke with authentication. Credentials are required and may be overriden at the request level.
**/
private Response invoke(Request request, HttpResponseHandler> responseHandler,
ExecutionContext executionContext) {
return invoke(request, responseHandler, executionContext, null, null);
}
/**
* Normal invoke with authentication. Credentials are required and may be overriden at the request level.
**/
private Response invoke(Request request, HttpResponseHandler> responseHandler,
ExecutionContext executionContext, URI cachedEndpoint, URI uriFromEndpointTrait) {
executionContext.setCredentialsProvider(CredentialUtils.getCredentialsProvider(request.getOriginalRequest(), awsCredentialsProvider));
return doInvoke(request, responseHandler, executionContext, cachedEndpoint, uriFromEndpointTrait);
}
/**
* Invoke with no authentication. Credentials are not required and any credentials set on the client or request will
* be ignored for this operation.
**/
private Response anonymousInvoke(Request request,
HttpResponseHandler> responseHandler, ExecutionContext executionContext) {
return doInvoke(request, responseHandler, executionContext, null, null);
}
/**
* Invoke the request using the http client. Assumes credentials (or lack thereof) have been configured in the
* ExecutionContext beforehand.
**/
private Response doInvoke(Request request, HttpResponseHandler> responseHandler,
ExecutionContext executionContext, URI discoveredEndpoint, URI uriFromEndpointTrait) {
if (discoveredEndpoint != null) {
request.setEndpoint(discoveredEndpoint);
request.getOriginalRequest().getRequestClientOptions().appendUserAgent("endpoint-discovery");
} else if (uriFromEndpointTrait != null) {
request.setEndpoint(uriFromEndpointTrait);
} else {
request.setEndpoint(endpoint);
}
request.setTimeOffset(timeOffset);
HttpResponseHandler errorResponseHandler = protocolFactory.createErrorResponseHandler(new JsonErrorResponseMetadata());
return client.execute(request, responseHandler, errorResponseHandler, executionContext);
}
@com.amazonaws.annotation.SdkInternalApi
static com.amazonaws.protocol.json.SdkJsonProtocolFactory getProtocolFactory() {
return protocolFactory;
}
@Override
public void shutdown() {
super.shutdown();
}
}