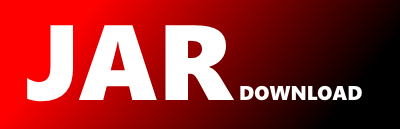
com.amazonaws.services.resourcegroups.model.ResourceQuery Maven / Gradle / Ivy
Show all versions of aws-java-sdk-resourcegroups Show documentation
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.resourcegroups.model;
import java.io.Serializable;
import javax.annotation.Generated;
import com.amazonaws.protocol.StructuredPojo;
import com.amazonaws.protocol.ProtocolMarshaller;
/**
*
* The query you can use to define a resource group or a search for resources. A ResourceQuery
specifies
* both a query Type
and a Query
string as JSON string objects. See the examples section for
* example JSON strings. For more information about creating a resource group with a resource query, see Build queries and groups in
* Resource Groups in the Resource Groups User Guide
*
*
* When you combine all of the elements together into a single string, any double quotes that are embedded inside
* another double quote pair must be escaped by preceding the embedded double quote with a backslash character (\). For
* example, a complete ResourceQuery
parameter must be formatted like the following CLI parameter example:
*
*
* --resource-query '{"Type":"TAG_FILTERS_1_0","Query":"{\"ResourceTypeFilters\":[\"AWS::AllSupported\"],\"TagFilters\":[{\"Key\":\"Stage\",\"Values\":[\"Test\"]}]}"}'
*
*
* In the preceding example, all of the double quote characters in the value part of the Query
element must
* be escaped because the value itself is surrounded by double quotes. For more information, see Quoting strings
* in the Command Line Interface User Guide.
*
*
* For the complete list of resource types that you can use in the array value for ResourceTypeFilters
, see
* Resources you can use with
* Resource Groups and Tag Editor in the Resource Groups User Guide. For example:
*
*
* "ResourceTypeFilters":["AWS::S3::Bucket", "AWS::EC2::Instance"]
*
*
* @see AWS API
* Documentation
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class ResourceQuery implements Serializable, Cloneable, StructuredPojo {
/**
*
* The type of the query to perform. This can have one of two values:
*
*
* -
*
* CLOUDFORMATION_STACK_1_0:
Specifies that you want the group to contain the members of an
* CloudFormation stack. The Query
contains a StackIdentifier
element with an ARN for a
* CloudFormation stack.
*
*
* -
*
* TAG_FILTERS_1_0:
Specifies that you want the group to include resource that have tags that
* match the query.
*
*
*
*/
private String type;
/**
*
* The query that defines a group or a search. The contents depends on the value of the Type
element.
*
*
* -
*
* ResourceTypeFilters
– Applies to all ResourceQuery
objects of either Type
.
* This element contains one of the following two items:
*
*
* -
*
* The value AWS::AllSupported
. This causes the ResourceQuery to match resources of any resource type
* that also match the query.
*
*
* -
*
* A list (a JSON array) of resource type identifiers that limit the query to only resources of the specified types.
* For the complete list of resource types that you can use in the array value for ResourceTypeFilters
,
* see Resources you can use
* with Resource Groups and Tag Editor in the Resource Groups User Guide.
*
*
*
*
* Example: "ResourceTypeFilters": ["AWS::AllSupported"]
or
* "ResourceTypeFilters": ["AWS::EC2::Instance", "AWS::S3::Bucket"]
*
*
* -
*
* TagFilters
– applicable only if Type
= TAG_FILTERS_1_0
. The
* Query
contains a JSON string that represents a collection of simple tag filters. The JSON string
* uses a syntax similar to the
* GetResources
* operation, but uses only the
* ResourceTypeFilters
* and
* TagFilters
* fields. If you specify more than one tag key, only resources that match all tag keys, and at least one value of
* each specified tag key, are returned in your query. If you specify more than one value for a tag key, a resource
* matches the filter if it has a tag key value that matches any of the specified values.
*
*
* For example, consider the following sample query for resources that have two tags, Stage
and
* Version
, with two values each:
*
*
* [{"Stage":["Test","Deploy"]},{"Version":["1","2"]}]
*
*
* The results of this resource query could include the following.
*
*
* -
*
* An Amazon EC2 instance that has the following two tags: {"Stage":"Deploy"}
, and
* {"Version":"2"}
*
*
* -
*
* An S3 bucket that has the following two tags: {"Stage":"Test"}
, and {"Version":"1"}
*
*
*
*
* The resource query results would not include the following items in the results, however.
*
*
* -
*
* An Amazon EC2 instance that has only the following tag: {"Stage":"Deploy"}
.
*
*
* The instance does not have all of the tag keys specified in the filter, so it is excluded from the
* results.
*
*
* -
*
* An RDS database that has the following two tags: {"Stage":"Archived"}
and
* {"Version":"4"}
*
*
* The database has all of the tag keys, but none of those keys has an associated value that matches at least one of
* the specified values in the filter.
*
*
*
*
* Example: "TagFilters": [ { "Key": "Stage", "Values": [ "Gamma", "Beta" ] }
*
*
* -
*
* StackIdentifier
– applicable only if Type
= CLOUDFORMATION_STACK_1_0
. The
* value of this parameter is the Amazon Resource Name (ARN) of the CloudFormation stack whose resources you want
* included in the group.
*
*
*
*/
private String query;
/**
*
* The type of the query to perform. This can have one of two values:
*
*
* -
*
* CLOUDFORMATION_STACK_1_0:
Specifies that you want the group to contain the members of an
* CloudFormation stack. The Query
contains a StackIdentifier
element with an ARN for a
* CloudFormation stack.
*
*
* -
*
* TAG_FILTERS_1_0:
Specifies that you want the group to include resource that have tags that
* match the query.
*
*
*
*
* @param type
* The type of the query to perform. This can have one of two values:
*
* -
*
* CLOUDFORMATION_STACK_1_0:
Specifies that you want the group to contain the members
* of an CloudFormation stack. The Query
contains a StackIdentifier
element with an
* ARN for a CloudFormation stack.
*
*
* -
*
* TAG_FILTERS_1_0:
Specifies that you want the group to include resource that have
* tags that match the query.
*
*
* @see QueryType
*/
public void setType(String type) {
this.type = type;
}
/**
*
* The type of the query to perform. This can have one of two values:
*
*
* -
*
* CLOUDFORMATION_STACK_1_0:
Specifies that you want the group to contain the members of an
* CloudFormation stack. The Query
contains a StackIdentifier
element with an ARN for a
* CloudFormation stack.
*
*
* -
*
* TAG_FILTERS_1_0:
Specifies that you want the group to include resource that have tags that
* match the query.
*
*
*
*
* @return The type of the query to perform. This can have one of two values:
*
* -
*
* CLOUDFORMATION_STACK_1_0:
Specifies that you want the group to contain the members
* of an CloudFormation stack. The Query
contains a StackIdentifier
element with
* an ARN for a CloudFormation stack.
*
*
* -
*
* TAG_FILTERS_1_0:
Specifies that you want the group to include resource that have
* tags that match the query.
*
*
* @see QueryType
*/
public String getType() {
return this.type;
}
/**
*
* The type of the query to perform. This can have one of two values:
*
*
* -
*
* CLOUDFORMATION_STACK_1_0:
Specifies that you want the group to contain the members of an
* CloudFormation stack. The Query
contains a StackIdentifier
element with an ARN for a
* CloudFormation stack.
*
*
* -
*
* TAG_FILTERS_1_0:
Specifies that you want the group to include resource that have tags that
* match the query.
*
*
*
*
* @param type
* The type of the query to perform. This can have one of two values:
*
* -
*
* CLOUDFORMATION_STACK_1_0:
Specifies that you want the group to contain the members
* of an CloudFormation stack. The Query
contains a StackIdentifier
element with an
* ARN for a CloudFormation stack.
*
*
* -
*
* TAG_FILTERS_1_0:
Specifies that you want the group to include resource that have
* tags that match the query.
*
*
* @return Returns a reference to this object so that method calls can be chained together.
* @see QueryType
*/
public ResourceQuery withType(String type) {
setType(type);
return this;
}
/**
*
* The type of the query to perform. This can have one of two values:
*
*
* -
*
* CLOUDFORMATION_STACK_1_0:
Specifies that you want the group to contain the members of an
* CloudFormation stack. The Query
contains a StackIdentifier
element with an ARN for a
* CloudFormation stack.
*
*
* -
*
* TAG_FILTERS_1_0:
Specifies that you want the group to include resource that have tags that
* match the query.
*
*
*
*
* @param type
* The type of the query to perform. This can have one of two values:
*
* -
*
* CLOUDFORMATION_STACK_1_0:
Specifies that you want the group to contain the members
* of an CloudFormation stack. The Query
contains a StackIdentifier
element with an
* ARN for a CloudFormation stack.
*
*
* -
*
* TAG_FILTERS_1_0:
Specifies that you want the group to include resource that have
* tags that match the query.
*
*
* @return Returns a reference to this object so that method calls can be chained together.
* @see QueryType
*/
public ResourceQuery withType(QueryType type) {
this.type = type.toString();
return this;
}
/**
*
* The query that defines a group or a search. The contents depends on the value of the Type
element.
*
*
* -
*
* ResourceTypeFilters
– Applies to all ResourceQuery
objects of either Type
.
* This element contains one of the following two items:
*
*
* -
*
* The value AWS::AllSupported
. This causes the ResourceQuery to match resources of any resource type
* that also match the query.
*
*
* -
*
* A list (a JSON array) of resource type identifiers that limit the query to only resources of the specified types.
* For the complete list of resource types that you can use in the array value for ResourceTypeFilters
,
* see Resources you can use
* with Resource Groups and Tag Editor in the Resource Groups User Guide.
*
*
*
*
* Example: "ResourceTypeFilters": ["AWS::AllSupported"]
or
* "ResourceTypeFilters": ["AWS::EC2::Instance", "AWS::S3::Bucket"]
*
*
* -
*
* TagFilters
– applicable only if Type
= TAG_FILTERS_1_0
. The
* Query
contains a JSON string that represents a collection of simple tag filters. The JSON string
* uses a syntax similar to the
* GetResources
* operation, but uses only the
* ResourceTypeFilters
* and
* TagFilters
* fields. If you specify more than one tag key, only resources that match all tag keys, and at least one value of
* each specified tag key, are returned in your query. If you specify more than one value for a tag key, a resource
* matches the filter if it has a tag key value that matches any of the specified values.
*
*
* For example, consider the following sample query for resources that have two tags, Stage
and
* Version
, with two values each:
*
*
* [{"Stage":["Test","Deploy"]},{"Version":["1","2"]}]
*
*
* The results of this resource query could include the following.
*
*
* -
*
* An Amazon EC2 instance that has the following two tags: {"Stage":"Deploy"}
, and
* {"Version":"2"}
*
*
* -
*
* An S3 bucket that has the following two tags: {"Stage":"Test"}
, and {"Version":"1"}
*
*
*
*
* The resource query results would not include the following items in the results, however.
*
*
* -
*
* An Amazon EC2 instance that has only the following tag: {"Stage":"Deploy"}
.
*
*
* The instance does not have all of the tag keys specified in the filter, so it is excluded from the
* results.
*
*
* -
*
* An RDS database that has the following two tags: {"Stage":"Archived"}
and
* {"Version":"4"}
*
*
* The database has all of the tag keys, but none of those keys has an associated value that matches at least one of
* the specified values in the filter.
*
*
*
*
* Example: "TagFilters": [ { "Key": "Stage", "Values": [ "Gamma", "Beta" ] }
*
*
* -
*
* StackIdentifier
– applicable only if Type
= CLOUDFORMATION_STACK_1_0
. The
* value of this parameter is the Amazon Resource Name (ARN) of the CloudFormation stack whose resources you want
* included in the group.
*
*
*
*
* @param query
* The query that defines a group or a search. The contents depends on the value of the Type
* element.
*
* -
*
* ResourceTypeFilters
– Applies to all ResourceQuery
objects of either
* Type
. This element contains one of the following two items:
*
*
* -
*
* The value AWS::AllSupported
. This causes the ResourceQuery to match resources of any resource
* type that also match the query.
*
*
* -
*
* A list (a JSON array) of resource type identifiers that limit the query to only resources of the specified
* types. For the complete list of resource types that you can use in the array value for
* ResourceTypeFilters
, see Resources you can use
* with Resource Groups and Tag Editor in the Resource Groups User Guide.
*
*
*
*
* Example: "ResourceTypeFilters": ["AWS::AllSupported"]
or
* "ResourceTypeFilters": ["AWS::EC2::Instance", "AWS::S3::Bucket"]
*
*
* -
*
* TagFilters
– applicable only if Type
= TAG_FILTERS_1_0
. The
* Query
contains a JSON string that represents a collection of simple tag filters. The JSON
* string uses a syntax similar to the
* GetResources
* operation, but uses only the
* ResourceTypeFilters
* and
* TagFilters
* fields. If you specify more than one tag key, only resources that match all tag keys, and at least one
* value of each specified tag key, are returned in your query. If you specify more than one value for a tag
* key, a resource matches the filter if it has a tag key value that matches any of the specified
* values.
*
*
* For example, consider the following sample query for resources that have two tags, Stage
and
* Version
, with two values each:
*
*
* [{"Stage":["Test","Deploy"]},{"Version":["1","2"]}]
*
*
* The results of this resource query could include the following.
*
*
* -
*
* An Amazon EC2 instance that has the following two tags: {"Stage":"Deploy"}
, and
* {"Version":"2"}
*
*
* -
*
* An S3 bucket that has the following two tags: {"Stage":"Test"}
, and
* {"Version":"1"}
*
*
*
*
* The resource query results would not include the following items in the results, however.
*
*
* -
*
* An Amazon EC2 instance that has only the following tag: {"Stage":"Deploy"}
.
*
*
* The instance does not have all of the tag keys specified in the filter, so it is excluded from the
* results.
*
*
* -
*
* An RDS database that has the following two tags: {"Stage":"Archived"}
and
* {"Version":"4"}
*
*
* The database has all of the tag keys, but none of those keys has an associated value that matches at least
* one of the specified values in the filter.
*
*
*
*
* Example: "TagFilters": [ { "Key": "Stage", "Values": [ "Gamma", "Beta" ] }
*
*
* -
*
* StackIdentifier
– applicable only if Type
=
* CLOUDFORMATION_STACK_1_0
. The value of this parameter is the Amazon Resource Name (ARN) of
* the CloudFormation stack whose resources you want included in the group.
*
*
*/
public void setQuery(String query) {
this.query = query;
}
/**
*
* The query that defines a group or a search. The contents depends on the value of the Type
element.
*
*
* -
*
* ResourceTypeFilters
– Applies to all ResourceQuery
objects of either Type
.
* This element contains one of the following two items:
*
*
* -
*
* The value AWS::AllSupported
. This causes the ResourceQuery to match resources of any resource type
* that also match the query.
*
*
* -
*
* A list (a JSON array) of resource type identifiers that limit the query to only resources of the specified types.
* For the complete list of resource types that you can use in the array value for ResourceTypeFilters
,
* see Resources you can use
* with Resource Groups and Tag Editor in the Resource Groups User Guide.
*
*
*
*
* Example: "ResourceTypeFilters": ["AWS::AllSupported"]
or
* "ResourceTypeFilters": ["AWS::EC2::Instance", "AWS::S3::Bucket"]
*
*
* -
*
* TagFilters
– applicable only if Type
= TAG_FILTERS_1_0
. The
* Query
contains a JSON string that represents a collection of simple tag filters. The JSON string
* uses a syntax similar to the
* GetResources
* operation, but uses only the
* ResourceTypeFilters
* and
* TagFilters
* fields. If you specify more than one tag key, only resources that match all tag keys, and at least one value of
* each specified tag key, are returned in your query. If you specify more than one value for a tag key, a resource
* matches the filter if it has a tag key value that matches any of the specified values.
*
*
* For example, consider the following sample query for resources that have two tags, Stage
and
* Version
, with two values each:
*
*
* [{"Stage":["Test","Deploy"]},{"Version":["1","2"]}]
*
*
* The results of this resource query could include the following.
*
*
* -
*
* An Amazon EC2 instance that has the following two tags: {"Stage":"Deploy"}
, and
* {"Version":"2"}
*
*
* -
*
* An S3 bucket that has the following two tags: {"Stage":"Test"}
, and {"Version":"1"}
*
*
*
*
* The resource query results would not include the following items in the results, however.
*
*
* -
*
* An Amazon EC2 instance that has only the following tag: {"Stage":"Deploy"}
.
*
*
* The instance does not have all of the tag keys specified in the filter, so it is excluded from the
* results.
*
*
* -
*
* An RDS database that has the following two tags: {"Stage":"Archived"}
and
* {"Version":"4"}
*
*
* The database has all of the tag keys, but none of those keys has an associated value that matches at least one of
* the specified values in the filter.
*
*
*
*
* Example: "TagFilters": [ { "Key": "Stage", "Values": [ "Gamma", "Beta" ] }
*
*
* -
*
* StackIdentifier
– applicable only if Type
= CLOUDFORMATION_STACK_1_0
. The
* value of this parameter is the Amazon Resource Name (ARN) of the CloudFormation stack whose resources you want
* included in the group.
*
*
*
*
* @return The query that defines a group or a search. The contents depends on the value of the Type
* element.
*
* -
*
* ResourceTypeFilters
– Applies to all ResourceQuery
objects of either
* Type
. This element contains one of the following two items:
*
*
* -
*
* The value AWS::AllSupported
. This causes the ResourceQuery to match resources of any
* resource type that also match the query.
*
*
* -
*
* A list (a JSON array) of resource type identifiers that limit the query to only resources of the
* specified types. For the complete list of resource types that you can use in the array value for
* ResourceTypeFilters
, see Resources you can use
* with Resource Groups and Tag Editor in the Resource Groups User Guide.
*
*
*
*
* Example: "ResourceTypeFilters": ["AWS::AllSupported"]
or
* "ResourceTypeFilters": ["AWS::EC2::Instance", "AWS::S3::Bucket"]
*
*
* -
*
* TagFilters
– applicable only if Type
= TAG_FILTERS_1_0
. The
* Query
contains a JSON string that represents a collection of simple tag filters. The JSON
* string uses a syntax similar to the
* GetResources
* operation, but uses only the
* ResourceTypeFilters
* and
* TagFilters
* fields. If you specify more than one tag key, only resources that match all tag keys, and at least one
* value of each specified tag key, are returned in your query. If you specify more than one value for a tag
* key, a resource matches the filter if it has a tag key value that matches any of the specified
* values.
*
*
* For example, consider the following sample query for resources that have two tags, Stage
and
* Version
, with two values each:
*
*
* [{"Stage":["Test","Deploy"]},{"Version":["1","2"]}]
*
*
* The results of this resource query could include the following.
*
*
* -
*
* An Amazon EC2 instance that has the following two tags: {"Stage":"Deploy"}
, and
* {"Version":"2"}
*
*
* -
*
* An S3 bucket that has the following two tags: {"Stage":"Test"}
, and
* {"Version":"1"}
*
*
*
*
* The resource query results would not include the following items in the results, however.
*
*
* -
*
* An Amazon EC2 instance that has only the following tag: {"Stage":"Deploy"}
.
*
*
* The instance does not have all of the tag keys specified in the filter, so it is excluded from the
* results.
*
*
* -
*
* An RDS database that has the following two tags: {"Stage":"Archived"}
and
* {"Version":"4"}
*
*
* The database has all of the tag keys, but none of those keys has an associated value that matches at
* least one of the specified values in the filter.
*
*
*
*
* Example: "TagFilters": [ { "Key": "Stage", "Values": [ "Gamma", "Beta" ] }
*
*
* -
*
* StackIdentifier
– applicable only if Type
=
* CLOUDFORMATION_STACK_1_0
. The value of this parameter is the Amazon Resource Name (ARN) of
* the CloudFormation stack whose resources you want included in the group.
*
*
*/
public String getQuery() {
return this.query;
}
/**
*
* The query that defines a group or a search. The contents depends on the value of the Type
element.
*
*
* -
*
* ResourceTypeFilters
– Applies to all ResourceQuery
objects of either Type
.
* This element contains one of the following two items:
*
*
* -
*
* The value AWS::AllSupported
. This causes the ResourceQuery to match resources of any resource type
* that also match the query.
*
*
* -
*
* A list (a JSON array) of resource type identifiers that limit the query to only resources of the specified types.
* For the complete list of resource types that you can use in the array value for ResourceTypeFilters
,
* see Resources you can use
* with Resource Groups and Tag Editor in the Resource Groups User Guide.
*
*
*
*
* Example: "ResourceTypeFilters": ["AWS::AllSupported"]
or
* "ResourceTypeFilters": ["AWS::EC2::Instance", "AWS::S3::Bucket"]
*
*
* -
*
* TagFilters
– applicable only if Type
= TAG_FILTERS_1_0
. The
* Query
contains a JSON string that represents a collection of simple tag filters. The JSON string
* uses a syntax similar to the
* GetResources
* operation, but uses only the
* ResourceTypeFilters
* and
* TagFilters
* fields. If you specify more than one tag key, only resources that match all tag keys, and at least one value of
* each specified tag key, are returned in your query. If you specify more than one value for a tag key, a resource
* matches the filter if it has a tag key value that matches any of the specified values.
*
*
* For example, consider the following sample query for resources that have two tags, Stage
and
* Version
, with two values each:
*
*
* [{"Stage":["Test","Deploy"]},{"Version":["1","2"]}]
*
*
* The results of this resource query could include the following.
*
*
* -
*
* An Amazon EC2 instance that has the following two tags: {"Stage":"Deploy"}
, and
* {"Version":"2"}
*
*
* -
*
* An S3 bucket that has the following two tags: {"Stage":"Test"}
, and {"Version":"1"}
*
*
*
*
* The resource query results would not include the following items in the results, however.
*
*
* -
*
* An Amazon EC2 instance that has only the following tag: {"Stage":"Deploy"}
.
*
*
* The instance does not have all of the tag keys specified in the filter, so it is excluded from the
* results.
*
*
* -
*
* An RDS database that has the following two tags: {"Stage":"Archived"}
and
* {"Version":"4"}
*
*
* The database has all of the tag keys, but none of those keys has an associated value that matches at least one of
* the specified values in the filter.
*
*
*
*
* Example: "TagFilters": [ { "Key": "Stage", "Values": [ "Gamma", "Beta" ] }
*
*
* -
*
* StackIdentifier
– applicable only if Type
= CLOUDFORMATION_STACK_1_0
. The
* value of this parameter is the Amazon Resource Name (ARN) of the CloudFormation stack whose resources you want
* included in the group.
*
*
*
*
* @param query
* The query that defines a group or a search. The contents depends on the value of the Type
* element.
*
* -
*
* ResourceTypeFilters
– Applies to all ResourceQuery
objects of either
* Type
. This element contains one of the following two items:
*
*
* -
*
* The value AWS::AllSupported
. This causes the ResourceQuery to match resources of any resource
* type that also match the query.
*
*
* -
*
* A list (a JSON array) of resource type identifiers that limit the query to only resources of the specified
* types. For the complete list of resource types that you can use in the array value for
* ResourceTypeFilters
, see Resources you can use
* with Resource Groups and Tag Editor in the Resource Groups User Guide.
*
*
*
*
* Example: "ResourceTypeFilters": ["AWS::AllSupported"]
or
* "ResourceTypeFilters": ["AWS::EC2::Instance", "AWS::S3::Bucket"]
*
*
* -
*
* TagFilters
– applicable only if Type
= TAG_FILTERS_1_0
. The
* Query
contains a JSON string that represents a collection of simple tag filters. The JSON
* string uses a syntax similar to the
* GetResources
* operation, but uses only the
* ResourceTypeFilters
* and
* TagFilters
* fields. If you specify more than one tag key, only resources that match all tag keys, and at least one
* value of each specified tag key, are returned in your query. If you specify more than one value for a tag
* key, a resource matches the filter if it has a tag key value that matches any of the specified
* values.
*
*
* For example, consider the following sample query for resources that have two tags, Stage
and
* Version
, with two values each:
*
*
* [{"Stage":["Test","Deploy"]},{"Version":["1","2"]}]
*
*
* The results of this resource query could include the following.
*
*
* -
*
* An Amazon EC2 instance that has the following two tags: {"Stage":"Deploy"}
, and
* {"Version":"2"}
*
*
* -
*
* An S3 bucket that has the following two tags: {"Stage":"Test"}
, and
* {"Version":"1"}
*
*
*
*
* The resource query results would not include the following items in the results, however.
*
*
* -
*
* An Amazon EC2 instance that has only the following tag: {"Stage":"Deploy"}
.
*
*
* The instance does not have all of the tag keys specified in the filter, so it is excluded from the
* results.
*
*
* -
*
* An RDS database that has the following two tags: {"Stage":"Archived"}
and
* {"Version":"4"}
*
*
* The database has all of the tag keys, but none of those keys has an associated value that matches at least
* one of the specified values in the filter.
*
*
*
*
* Example: "TagFilters": [ { "Key": "Stage", "Values": [ "Gamma", "Beta" ] }
*
*
* -
*
* StackIdentifier
– applicable only if Type
=
* CLOUDFORMATION_STACK_1_0
. The value of this parameter is the Amazon Resource Name (ARN) of
* the CloudFormation stack whose resources you want included in the group.
*
*
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ResourceQuery withQuery(String query) {
setQuery(query);
return this;
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getType() != null)
sb.append("Type: ").append(getType()).append(",");
if (getQuery() != null)
sb.append("Query: ").append(getQuery());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof ResourceQuery == false)
return false;
ResourceQuery other = (ResourceQuery) obj;
if (other.getType() == null ^ this.getType() == null)
return false;
if (other.getType() != null && other.getType().equals(this.getType()) == false)
return false;
if (other.getQuery() == null ^ this.getQuery() == null)
return false;
if (other.getQuery() != null && other.getQuery().equals(this.getQuery()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getType() == null) ? 0 : getType().hashCode());
hashCode = prime * hashCode + ((getQuery() == null) ? 0 : getQuery().hashCode());
return hashCode;
}
@Override
public ResourceQuery clone() {
try {
return (ResourceQuery) super.clone();
} catch (CloneNotSupportedException e) {
throw new IllegalStateException("Got a CloneNotSupportedException from Object.clone() " + "even though we're Cloneable!", e);
}
}
@com.amazonaws.annotation.SdkInternalApi
@Override
public void marshall(ProtocolMarshaller protocolMarshaller) {
com.amazonaws.services.resourcegroups.model.transform.ResourceQueryMarshaller.getInstance().marshall(this, protocolMarshaller);
}
}