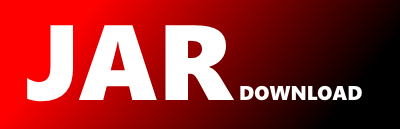
com.amazonaws.services.robomaker.model.CreateSimulationJobRequest Maven / Gradle / Ivy
Show all versions of aws-java-sdk-robomaker Show documentation
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.robomaker.model;
import java.io.Serializable;
import javax.annotation.Generated;
import com.amazonaws.AmazonWebServiceRequest;
/**
*
* @see AWS API
* Documentation
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class CreateSimulationJobRequest extends com.amazonaws.AmazonWebServiceRequest implements Serializable, Cloneable {
/**
*
* Unique, case-sensitive identifier that you provide to ensure the idempotency of the request.
*
*/
private String clientRequestToken;
/**
*
* Location for output files generated by the simulation job.
*
*/
private OutputLocation outputLocation;
/**
*
* The logging configuration.
*
*/
private LoggingConfig loggingConfig;
/**
*
* The maximum simulation job duration in seconds (up to 14 days or 1,209,600 seconds. When
* maxJobDurationInSeconds
is reached, the simulation job will status will transition to
* Completed
.
*
*/
private Long maxJobDurationInSeconds;
/**
*
* The IAM role name that allows the simulation instance to call the AWS APIs that are specified in its associated
* policies on your behalf. This is how credentials are passed in to your simulation job.
*
*/
private String iamRole;
/**
*
* The failure behavior the simulation job.
*
*
* - Continue
* -
*
* Leaves the instance running for its maximum timeout duration after a 4XX
error code.
*
*
* - Fail
* -
*
* Stop the simulation job and terminate the instance.
*
*
*
*/
private String failureBehavior;
/**
*
* The robot application to use in the simulation job.
*
*/
private java.util.List robotApplications;
/**
*
* The simulation application to use in the simulation job.
*
*/
private java.util.List simulationApplications;
/**
*
* Specify data sources to mount read-only files from S3 into your simulation. These files are available under
* /opt/robomaker/datasources/data_source_name
.
*
*
*
* There is a limit of 100 files and a combined size of 25GB for all DataSourceConfig
objects.
*
*
*/
private java.util.List dataSources;
/**
*
* A map that contains tag keys and tag values that are attached to the simulation job.
*
*/
private java.util.Map tags;
/**
*
* If your simulation job accesses resources in a VPC, you provide this parameter identifying the list of security
* group IDs and subnet IDs. These must belong to the same VPC. You must provide at least one security group and one
* subnet ID.
*
*/
private VPCConfig vpcConfig;
/**
*
* Compute information for the simulation job.
*
*/
private Compute compute;
/**
*
* Unique, case-sensitive identifier that you provide to ensure the idempotency of the request.
*
*
* @param clientRequestToken
* Unique, case-sensitive identifier that you provide to ensure the idempotency of the request.
*/
public void setClientRequestToken(String clientRequestToken) {
this.clientRequestToken = clientRequestToken;
}
/**
*
* Unique, case-sensitive identifier that you provide to ensure the idempotency of the request.
*
*
* @return Unique, case-sensitive identifier that you provide to ensure the idempotency of the request.
*/
public String getClientRequestToken() {
return this.clientRequestToken;
}
/**
*
* Unique, case-sensitive identifier that you provide to ensure the idempotency of the request.
*
*
* @param clientRequestToken
* Unique, case-sensitive identifier that you provide to ensure the idempotency of the request.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateSimulationJobRequest withClientRequestToken(String clientRequestToken) {
setClientRequestToken(clientRequestToken);
return this;
}
/**
*
* Location for output files generated by the simulation job.
*
*
* @param outputLocation
* Location for output files generated by the simulation job.
*/
public void setOutputLocation(OutputLocation outputLocation) {
this.outputLocation = outputLocation;
}
/**
*
* Location for output files generated by the simulation job.
*
*
* @return Location for output files generated by the simulation job.
*/
public OutputLocation getOutputLocation() {
return this.outputLocation;
}
/**
*
* Location for output files generated by the simulation job.
*
*
* @param outputLocation
* Location for output files generated by the simulation job.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateSimulationJobRequest withOutputLocation(OutputLocation outputLocation) {
setOutputLocation(outputLocation);
return this;
}
/**
*
* The logging configuration.
*
*
* @param loggingConfig
* The logging configuration.
*/
public void setLoggingConfig(LoggingConfig loggingConfig) {
this.loggingConfig = loggingConfig;
}
/**
*
* The logging configuration.
*
*
* @return The logging configuration.
*/
public LoggingConfig getLoggingConfig() {
return this.loggingConfig;
}
/**
*
* The logging configuration.
*
*
* @param loggingConfig
* The logging configuration.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateSimulationJobRequest withLoggingConfig(LoggingConfig loggingConfig) {
setLoggingConfig(loggingConfig);
return this;
}
/**
*
* The maximum simulation job duration in seconds (up to 14 days or 1,209,600 seconds. When
* maxJobDurationInSeconds
is reached, the simulation job will status will transition to
* Completed
.
*
*
* @param maxJobDurationInSeconds
* The maximum simulation job duration in seconds (up to 14 days or 1,209,600 seconds. When
* maxJobDurationInSeconds
is reached, the simulation job will status will transition to
* Completed
.
*/
public void setMaxJobDurationInSeconds(Long maxJobDurationInSeconds) {
this.maxJobDurationInSeconds = maxJobDurationInSeconds;
}
/**
*
* The maximum simulation job duration in seconds (up to 14 days or 1,209,600 seconds. When
* maxJobDurationInSeconds
is reached, the simulation job will status will transition to
* Completed
.
*
*
* @return The maximum simulation job duration in seconds (up to 14 days or 1,209,600 seconds. When
* maxJobDurationInSeconds
is reached, the simulation job will status will transition to
* Completed
.
*/
public Long getMaxJobDurationInSeconds() {
return this.maxJobDurationInSeconds;
}
/**
*
* The maximum simulation job duration in seconds (up to 14 days or 1,209,600 seconds. When
* maxJobDurationInSeconds
is reached, the simulation job will status will transition to
* Completed
.
*
*
* @param maxJobDurationInSeconds
* The maximum simulation job duration in seconds (up to 14 days or 1,209,600 seconds. When
* maxJobDurationInSeconds
is reached, the simulation job will status will transition to
* Completed
.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateSimulationJobRequest withMaxJobDurationInSeconds(Long maxJobDurationInSeconds) {
setMaxJobDurationInSeconds(maxJobDurationInSeconds);
return this;
}
/**
*
* The IAM role name that allows the simulation instance to call the AWS APIs that are specified in its associated
* policies on your behalf. This is how credentials are passed in to your simulation job.
*
*
* @param iamRole
* The IAM role name that allows the simulation instance to call the AWS APIs that are specified in its
* associated policies on your behalf. This is how credentials are passed in to your simulation job.
*/
public void setIamRole(String iamRole) {
this.iamRole = iamRole;
}
/**
*
* The IAM role name that allows the simulation instance to call the AWS APIs that are specified in its associated
* policies on your behalf. This is how credentials are passed in to your simulation job.
*
*
* @return The IAM role name that allows the simulation instance to call the AWS APIs that are specified in its
* associated policies on your behalf. This is how credentials are passed in to your simulation job.
*/
public String getIamRole() {
return this.iamRole;
}
/**
*
* The IAM role name that allows the simulation instance to call the AWS APIs that are specified in its associated
* policies on your behalf. This is how credentials are passed in to your simulation job.
*
*
* @param iamRole
* The IAM role name that allows the simulation instance to call the AWS APIs that are specified in its
* associated policies on your behalf. This is how credentials are passed in to your simulation job.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateSimulationJobRequest withIamRole(String iamRole) {
setIamRole(iamRole);
return this;
}
/**
*
* The failure behavior the simulation job.
*
*
* - Continue
* -
*
* Leaves the instance running for its maximum timeout duration after a 4XX
error code.
*
*
* - Fail
* -
*
* Stop the simulation job and terminate the instance.
*
*
*
*
* @param failureBehavior
* The failure behavior the simulation job.
*
* - Continue
* -
*
* Leaves the instance running for its maximum timeout duration after a 4XX
error code.
*
*
* - Fail
* -
*
* Stop the simulation job and terminate the instance.
*
*
* @see FailureBehavior
*/
public void setFailureBehavior(String failureBehavior) {
this.failureBehavior = failureBehavior;
}
/**
*
* The failure behavior the simulation job.
*
*
* - Continue
* -
*
* Leaves the instance running for its maximum timeout duration after a 4XX
error code.
*
*
* - Fail
* -
*
* Stop the simulation job and terminate the instance.
*
*
*
*
* @return The failure behavior the simulation job.
*
* - Continue
* -
*
* Leaves the instance running for its maximum timeout duration after a 4XX
error code.
*
*
* - Fail
* -
*
* Stop the simulation job and terminate the instance.
*
*
* @see FailureBehavior
*/
public String getFailureBehavior() {
return this.failureBehavior;
}
/**
*
* The failure behavior the simulation job.
*
*
* - Continue
* -
*
* Leaves the instance running for its maximum timeout duration after a 4XX
error code.
*
*
* - Fail
* -
*
* Stop the simulation job and terminate the instance.
*
*
*
*
* @param failureBehavior
* The failure behavior the simulation job.
*
* - Continue
* -
*
* Leaves the instance running for its maximum timeout duration after a 4XX
error code.
*
*
* - Fail
* -
*
* Stop the simulation job and terminate the instance.
*
*
* @return Returns a reference to this object so that method calls can be chained together.
* @see FailureBehavior
*/
public CreateSimulationJobRequest withFailureBehavior(String failureBehavior) {
setFailureBehavior(failureBehavior);
return this;
}
/**
*
* The failure behavior the simulation job.
*
*
* - Continue
* -
*
* Leaves the instance running for its maximum timeout duration after a 4XX
error code.
*
*
* - Fail
* -
*
* Stop the simulation job and terminate the instance.
*
*
*
*
* @param failureBehavior
* The failure behavior the simulation job.
*
* - Continue
* -
*
* Leaves the instance running for its maximum timeout duration after a 4XX
error code.
*
*
* - Fail
* -
*
* Stop the simulation job and terminate the instance.
*
*
* @return Returns a reference to this object so that method calls can be chained together.
* @see FailureBehavior
*/
public CreateSimulationJobRequest withFailureBehavior(FailureBehavior failureBehavior) {
this.failureBehavior = failureBehavior.toString();
return this;
}
/**
*
* The robot application to use in the simulation job.
*
*
* @return The robot application to use in the simulation job.
*/
public java.util.List getRobotApplications() {
return robotApplications;
}
/**
*
* The robot application to use in the simulation job.
*
*
* @param robotApplications
* The robot application to use in the simulation job.
*/
public void setRobotApplications(java.util.Collection robotApplications) {
if (robotApplications == null) {
this.robotApplications = null;
return;
}
this.robotApplications = new java.util.ArrayList(robotApplications);
}
/**
*
* The robot application to use in the simulation job.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setRobotApplications(java.util.Collection)} or {@link #withRobotApplications(java.util.Collection)} if
* you want to override the existing values.
*
*
* @param robotApplications
* The robot application to use in the simulation job.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateSimulationJobRequest withRobotApplications(RobotApplicationConfig... robotApplications) {
if (this.robotApplications == null) {
setRobotApplications(new java.util.ArrayList(robotApplications.length));
}
for (RobotApplicationConfig ele : robotApplications) {
this.robotApplications.add(ele);
}
return this;
}
/**
*
* The robot application to use in the simulation job.
*
*
* @param robotApplications
* The robot application to use in the simulation job.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateSimulationJobRequest withRobotApplications(java.util.Collection robotApplications) {
setRobotApplications(robotApplications);
return this;
}
/**
*
* The simulation application to use in the simulation job.
*
*
* @return The simulation application to use in the simulation job.
*/
public java.util.List getSimulationApplications() {
return simulationApplications;
}
/**
*
* The simulation application to use in the simulation job.
*
*
* @param simulationApplications
* The simulation application to use in the simulation job.
*/
public void setSimulationApplications(java.util.Collection simulationApplications) {
if (simulationApplications == null) {
this.simulationApplications = null;
return;
}
this.simulationApplications = new java.util.ArrayList(simulationApplications);
}
/**
*
* The simulation application to use in the simulation job.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setSimulationApplications(java.util.Collection)} or
* {@link #withSimulationApplications(java.util.Collection)} if you want to override the existing values.
*
*
* @param simulationApplications
* The simulation application to use in the simulation job.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateSimulationJobRequest withSimulationApplications(SimulationApplicationConfig... simulationApplications) {
if (this.simulationApplications == null) {
setSimulationApplications(new java.util.ArrayList(simulationApplications.length));
}
for (SimulationApplicationConfig ele : simulationApplications) {
this.simulationApplications.add(ele);
}
return this;
}
/**
*
* The simulation application to use in the simulation job.
*
*
* @param simulationApplications
* The simulation application to use in the simulation job.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateSimulationJobRequest withSimulationApplications(java.util.Collection simulationApplications) {
setSimulationApplications(simulationApplications);
return this;
}
/**
*
* Specify data sources to mount read-only files from S3 into your simulation. These files are available under
* /opt/robomaker/datasources/data_source_name
.
*
*
*
* There is a limit of 100 files and a combined size of 25GB for all DataSourceConfig
objects.
*
*
*
* @return Specify data sources to mount read-only files from S3 into your simulation. These files are available
* under /opt/robomaker/datasources/data_source_name
.
*
* There is a limit of 100 files and a combined size of 25GB for all DataSourceConfig
objects.
*
*/
public java.util.List getDataSources() {
return dataSources;
}
/**
*
* Specify data sources to mount read-only files from S3 into your simulation. These files are available under
* /opt/robomaker/datasources/data_source_name
.
*
*
*
* There is a limit of 100 files and a combined size of 25GB for all DataSourceConfig
objects.
*
*
*
* @param dataSources
* Specify data sources to mount read-only files from S3 into your simulation. These files are available
* under /opt/robomaker/datasources/data_source_name
.
*
* There is a limit of 100 files and a combined size of 25GB for all DataSourceConfig
objects.
*
*/
public void setDataSources(java.util.Collection dataSources) {
if (dataSources == null) {
this.dataSources = null;
return;
}
this.dataSources = new java.util.ArrayList(dataSources);
}
/**
*
* Specify data sources to mount read-only files from S3 into your simulation. These files are available under
* /opt/robomaker/datasources/data_source_name
.
*
*
*
* There is a limit of 100 files and a combined size of 25GB for all DataSourceConfig
objects.
*
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setDataSources(java.util.Collection)} or {@link #withDataSources(java.util.Collection)} if you want to
* override the existing values.
*
*
* @param dataSources
* Specify data sources to mount read-only files from S3 into your simulation. These files are available
* under /opt/robomaker/datasources/data_source_name
.
*
* There is a limit of 100 files and a combined size of 25GB for all DataSourceConfig
objects.
*
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateSimulationJobRequest withDataSources(DataSourceConfig... dataSources) {
if (this.dataSources == null) {
setDataSources(new java.util.ArrayList(dataSources.length));
}
for (DataSourceConfig ele : dataSources) {
this.dataSources.add(ele);
}
return this;
}
/**
*
* Specify data sources to mount read-only files from S3 into your simulation. These files are available under
* /opt/robomaker/datasources/data_source_name
.
*
*
*
* There is a limit of 100 files and a combined size of 25GB for all DataSourceConfig
objects.
*
*
*
* @param dataSources
* Specify data sources to mount read-only files from S3 into your simulation. These files are available
* under /opt/robomaker/datasources/data_source_name
.
*
* There is a limit of 100 files and a combined size of 25GB for all DataSourceConfig
objects.
*
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateSimulationJobRequest withDataSources(java.util.Collection dataSources) {
setDataSources(dataSources);
return this;
}
/**
*
* A map that contains tag keys and tag values that are attached to the simulation job.
*
*
* @return A map that contains tag keys and tag values that are attached to the simulation job.
*/
public java.util.Map getTags() {
return tags;
}
/**
*
* A map that contains tag keys and tag values that are attached to the simulation job.
*
*
* @param tags
* A map that contains tag keys and tag values that are attached to the simulation job.
*/
public void setTags(java.util.Map tags) {
this.tags = tags;
}
/**
*
* A map that contains tag keys and tag values that are attached to the simulation job.
*
*
* @param tags
* A map that contains tag keys and tag values that are attached to the simulation job.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateSimulationJobRequest withTags(java.util.Map tags) {
setTags(tags);
return this;
}
/**
* Add a single Tags entry
*
* @see CreateSimulationJobRequest#withTags
* @returns a reference to this object so that method calls can be chained together.
*/
public CreateSimulationJobRequest addTagsEntry(String key, String value) {
if (null == this.tags) {
this.tags = new java.util.HashMap();
}
if (this.tags.containsKey(key))
throw new IllegalArgumentException("Duplicated keys (" + key.toString() + ") are provided.");
this.tags.put(key, value);
return this;
}
/**
* Removes all the entries added into Tags.
*
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateSimulationJobRequest clearTagsEntries() {
this.tags = null;
return this;
}
/**
*
* If your simulation job accesses resources in a VPC, you provide this parameter identifying the list of security
* group IDs and subnet IDs. These must belong to the same VPC. You must provide at least one security group and one
* subnet ID.
*
*
* @param vpcConfig
* If your simulation job accesses resources in a VPC, you provide this parameter identifying the list of
* security group IDs and subnet IDs. These must belong to the same VPC. You must provide at least one
* security group and one subnet ID.
*/
public void setVpcConfig(VPCConfig vpcConfig) {
this.vpcConfig = vpcConfig;
}
/**
*
* If your simulation job accesses resources in a VPC, you provide this parameter identifying the list of security
* group IDs and subnet IDs. These must belong to the same VPC. You must provide at least one security group and one
* subnet ID.
*
*
* @return If your simulation job accesses resources in a VPC, you provide this parameter identifying the list of
* security group IDs and subnet IDs. These must belong to the same VPC. You must provide at least one
* security group and one subnet ID.
*/
public VPCConfig getVpcConfig() {
return this.vpcConfig;
}
/**
*
* If your simulation job accesses resources in a VPC, you provide this parameter identifying the list of security
* group IDs and subnet IDs. These must belong to the same VPC. You must provide at least one security group and one
* subnet ID.
*
*
* @param vpcConfig
* If your simulation job accesses resources in a VPC, you provide this parameter identifying the list of
* security group IDs and subnet IDs. These must belong to the same VPC. You must provide at least one
* security group and one subnet ID.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateSimulationJobRequest withVpcConfig(VPCConfig vpcConfig) {
setVpcConfig(vpcConfig);
return this;
}
/**
*
* Compute information for the simulation job.
*
*
* @param compute
* Compute information for the simulation job.
*/
public void setCompute(Compute compute) {
this.compute = compute;
}
/**
*
* Compute information for the simulation job.
*
*
* @return Compute information for the simulation job.
*/
public Compute getCompute() {
return this.compute;
}
/**
*
* Compute information for the simulation job.
*
*
* @param compute
* Compute information for the simulation job.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateSimulationJobRequest withCompute(Compute compute) {
setCompute(compute);
return this;
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getClientRequestToken() != null)
sb.append("ClientRequestToken: ").append(getClientRequestToken()).append(",");
if (getOutputLocation() != null)
sb.append("OutputLocation: ").append(getOutputLocation()).append(",");
if (getLoggingConfig() != null)
sb.append("LoggingConfig: ").append(getLoggingConfig()).append(",");
if (getMaxJobDurationInSeconds() != null)
sb.append("MaxJobDurationInSeconds: ").append(getMaxJobDurationInSeconds()).append(",");
if (getIamRole() != null)
sb.append("IamRole: ").append(getIamRole()).append(",");
if (getFailureBehavior() != null)
sb.append("FailureBehavior: ").append(getFailureBehavior()).append(",");
if (getRobotApplications() != null)
sb.append("RobotApplications: ").append(getRobotApplications()).append(",");
if (getSimulationApplications() != null)
sb.append("SimulationApplications: ").append(getSimulationApplications()).append(",");
if (getDataSources() != null)
sb.append("DataSources: ").append(getDataSources()).append(",");
if (getTags() != null)
sb.append("Tags: ").append(getTags()).append(",");
if (getVpcConfig() != null)
sb.append("VpcConfig: ").append(getVpcConfig()).append(",");
if (getCompute() != null)
sb.append("Compute: ").append(getCompute());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof CreateSimulationJobRequest == false)
return false;
CreateSimulationJobRequest other = (CreateSimulationJobRequest) obj;
if (other.getClientRequestToken() == null ^ this.getClientRequestToken() == null)
return false;
if (other.getClientRequestToken() != null && other.getClientRequestToken().equals(this.getClientRequestToken()) == false)
return false;
if (other.getOutputLocation() == null ^ this.getOutputLocation() == null)
return false;
if (other.getOutputLocation() != null && other.getOutputLocation().equals(this.getOutputLocation()) == false)
return false;
if (other.getLoggingConfig() == null ^ this.getLoggingConfig() == null)
return false;
if (other.getLoggingConfig() != null && other.getLoggingConfig().equals(this.getLoggingConfig()) == false)
return false;
if (other.getMaxJobDurationInSeconds() == null ^ this.getMaxJobDurationInSeconds() == null)
return false;
if (other.getMaxJobDurationInSeconds() != null && other.getMaxJobDurationInSeconds().equals(this.getMaxJobDurationInSeconds()) == false)
return false;
if (other.getIamRole() == null ^ this.getIamRole() == null)
return false;
if (other.getIamRole() != null && other.getIamRole().equals(this.getIamRole()) == false)
return false;
if (other.getFailureBehavior() == null ^ this.getFailureBehavior() == null)
return false;
if (other.getFailureBehavior() != null && other.getFailureBehavior().equals(this.getFailureBehavior()) == false)
return false;
if (other.getRobotApplications() == null ^ this.getRobotApplications() == null)
return false;
if (other.getRobotApplications() != null && other.getRobotApplications().equals(this.getRobotApplications()) == false)
return false;
if (other.getSimulationApplications() == null ^ this.getSimulationApplications() == null)
return false;
if (other.getSimulationApplications() != null && other.getSimulationApplications().equals(this.getSimulationApplications()) == false)
return false;
if (other.getDataSources() == null ^ this.getDataSources() == null)
return false;
if (other.getDataSources() != null && other.getDataSources().equals(this.getDataSources()) == false)
return false;
if (other.getTags() == null ^ this.getTags() == null)
return false;
if (other.getTags() != null && other.getTags().equals(this.getTags()) == false)
return false;
if (other.getVpcConfig() == null ^ this.getVpcConfig() == null)
return false;
if (other.getVpcConfig() != null && other.getVpcConfig().equals(this.getVpcConfig()) == false)
return false;
if (other.getCompute() == null ^ this.getCompute() == null)
return false;
if (other.getCompute() != null && other.getCompute().equals(this.getCompute()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getClientRequestToken() == null) ? 0 : getClientRequestToken().hashCode());
hashCode = prime * hashCode + ((getOutputLocation() == null) ? 0 : getOutputLocation().hashCode());
hashCode = prime * hashCode + ((getLoggingConfig() == null) ? 0 : getLoggingConfig().hashCode());
hashCode = prime * hashCode + ((getMaxJobDurationInSeconds() == null) ? 0 : getMaxJobDurationInSeconds().hashCode());
hashCode = prime * hashCode + ((getIamRole() == null) ? 0 : getIamRole().hashCode());
hashCode = prime * hashCode + ((getFailureBehavior() == null) ? 0 : getFailureBehavior().hashCode());
hashCode = prime * hashCode + ((getRobotApplications() == null) ? 0 : getRobotApplications().hashCode());
hashCode = prime * hashCode + ((getSimulationApplications() == null) ? 0 : getSimulationApplications().hashCode());
hashCode = prime * hashCode + ((getDataSources() == null) ? 0 : getDataSources().hashCode());
hashCode = prime * hashCode + ((getTags() == null) ? 0 : getTags().hashCode());
hashCode = prime * hashCode + ((getVpcConfig() == null) ? 0 : getVpcConfig().hashCode());
hashCode = prime * hashCode + ((getCompute() == null) ? 0 : getCompute().hashCode());
return hashCode;
}
@Override
public CreateSimulationJobRequest clone() {
return (CreateSimulationJobRequest) super.clone();
}
}