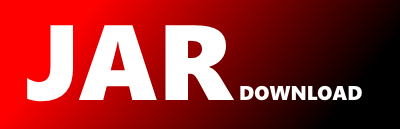
com.amazonaws.services.robomaker.model.Compute Maven / Gradle / Ivy
Show all versions of aws-java-sdk-robomaker Show documentation
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.robomaker.model;
import java.io.Serializable;
import javax.annotation.Generated;
import com.amazonaws.protocol.StructuredPojo;
import com.amazonaws.protocol.ProtocolMarshaller;
/**
*
* Compute information for the simulation job.
*
*
* @see AWS API
* Documentation
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class Compute implements Serializable, Cloneable, StructuredPojo {
/**
*
* The simulation unit limit. Your simulation is allocated CPU and memory proportional to the supplied simulation
* unit limit. A simulation unit is 1 vcpu and 2GB of memory. You are only billed for the SU utilization you consume
* up to the maximum value provided. The default is 15.
*
*/
private Integer simulationUnitLimit;
/**
*
* Compute type information for the simulation job.
*
*/
private String computeType;
/**
*
* Compute GPU unit limit for the simulation job. It is the same as the number of GPUs allocated to the
* SimulationJob.
*
*/
private Integer gpuUnitLimit;
/**
*
* The simulation unit limit. Your simulation is allocated CPU and memory proportional to the supplied simulation
* unit limit. A simulation unit is 1 vcpu and 2GB of memory. You are only billed for the SU utilization you consume
* up to the maximum value provided. The default is 15.
*
*
* @param simulationUnitLimit
* The simulation unit limit. Your simulation is allocated CPU and memory proportional to the supplied
* simulation unit limit. A simulation unit is 1 vcpu and 2GB of memory. You are only billed for the SU
* utilization you consume up to the maximum value provided. The default is 15.
*/
public void setSimulationUnitLimit(Integer simulationUnitLimit) {
this.simulationUnitLimit = simulationUnitLimit;
}
/**
*
* The simulation unit limit. Your simulation is allocated CPU and memory proportional to the supplied simulation
* unit limit. A simulation unit is 1 vcpu and 2GB of memory. You are only billed for the SU utilization you consume
* up to the maximum value provided. The default is 15.
*
*
* @return The simulation unit limit. Your simulation is allocated CPU and memory proportional to the supplied
* simulation unit limit. A simulation unit is 1 vcpu and 2GB of memory. You are only billed for the SU
* utilization you consume up to the maximum value provided. The default is 15.
*/
public Integer getSimulationUnitLimit() {
return this.simulationUnitLimit;
}
/**
*
* The simulation unit limit. Your simulation is allocated CPU and memory proportional to the supplied simulation
* unit limit. A simulation unit is 1 vcpu and 2GB of memory. You are only billed for the SU utilization you consume
* up to the maximum value provided. The default is 15.
*
*
* @param simulationUnitLimit
* The simulation unit limit. Your simulation is allocated CPU and memory proportional to the supplied
* simulation unit limit. A simulation unit is 1 vcpu and 2GB of memory. You are only billed for the SU
* utilization you consume up to the maximum value provided. The default is 15.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Compute withSimulationUnitLimit(Integer simulationUnitLimit) {
setSimulationUnitLimit(simulationUnitLimit);
return this;
}
/**
*
* Compute type information for the simulation job.
*
*
* @param computeType
* Compute type information for the simulation job.
* @see ComputeType
*/
public void setComputeType(String computeType) {
this.computeType = computeType;
}
/**
*
* Compute type information for the simulation job.
*
*
* @return Compute type information for the simulation job.
* @see ComputeType
*/
public String getComputeType() {
return this.computeType;
}
/**
*
* Compute type information for the simulation job.
*
*
* @param computeType
* Compute type information for the simulation job.
* @return Returns a reference to this object so that method calls can be chained together.
* @see ComputeType
*/
public Compute withComputeType(String computeType) {
setComputeType(computeType);
return this;
}
/**
*
* Compute type information for the simulation job.
*
*
* @param computeType
* Compute type information for the simulation job.
* @return Returns a reference to this object so that method calls can be chained together.
* @see ComputeType
*/
public Compute withComputeType(ComputeType computeType) {
this.computeType = computeType.toString();
return this;
}
/**
*
* Compute GPU unit limit for the simulation job. It is the same as the number of GPUs allocated to the
* SimulationJob.
*
*
* @param gpuUnitLimit
* Compute GPU unit limit for the simulation job. It is the same as the number of GPUs allocated to the
* SimulationJob.
*/
public void setGpuUnitLimit(Integer gpuUnitLimit) {
this.gpuUnitLimit = gpuUnitLimit;
}
/**
*
* Compute GPU unit limit for the simulation job. It is the same as the number of GPUs allocated to the
* SimulationJob.
*
*
* @return Compute GPU unit limit for the simulation job. It is the same as the number of GPUs allocated to the
* SimulationJob.
*/
public Integer getGpuUnitLimit() {
return this.gpuUnitLimit;
}
/**
*
* Compute GPU unit limit for the simulation job. It is the same as the number of GPUs allocated to the
* SimulationJob.
*
*
* @param gpuUnitLimit
* Compute GPU unit limit for the simulation job. It is the same as the number of GPUs allocated to the
* SimulationJob.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Compute withGpuUnitLimit(Integer gpuUnitLimit) {
setGpuUnitLimit(gpuUnitLimit);
return this;
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getSimulationUnitLimit() != null)
sb.append("SimulationUnitLimit: ").append(getSimulationUnitLimit()).append(",");
if (getComputeType() != null)
sb.append("ComputeType: ").append(getComputeType()).append(",");
if (getGpuUnitLimit() != null)
sb.append("GpuUnitLimit: ").append(getGpuUnitLimit());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof Compute == false)
return false;
Compute other = (Compute) obj;
if (other.getSimulationUnitLimit() == null ^ this.getSimulationUnitLimit() == null)
return false;
if (other.getSimulationUnitLimit() != null && other.getSimulationUnitLimit().equals(this.getSimulationUnitLimit()) == false)
return false;
if (other.getComputeType() == null ^ this.getComputeType() == null)
return false;
if (other.getComputeType() != null && other.getComputeType().equals(this.getComputeType()) == false)
return false;
if (other.getGpuUnitLimit() == null ^ this.getGpuUnitLimit() == null)
return false;
if (other.getGpuUnitLimit() != null && other.getGpuUnitLimit().equals(this.getGpuUnitLimit()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getSimulationUnitLimit() == null) ? 0 : getSimulationUnitLimit().hashCode());
hashCode = prime * hashCode + ((getComputeType() == null) ? 0 : getComputeType().hashCode());
hashCode = prime * hashCode + ((getGpuUnitLimit() == null) ? 0 : getGpuUnitLimit().hashCode());
return hashCode;
}
@Override
public Compute clone() {
try {
return (Compute) super.clone();
} catch (CloneNotSupportedException e) {
throw new IllegalStateException("Got a CloneNotSupportedException from Object.clone() " + "even though we're Cloneable!", e);
}
}
@com.amazonaws.annotation.SdkInternalApi
@Override
public void marshall(ProtocolMarshaller protocolMarshaller) {
com.amazonaws.services.robomaker.model.transform.ComputeMarshaller.getInstance().marshall(this, protocolMarshaller);
}
}