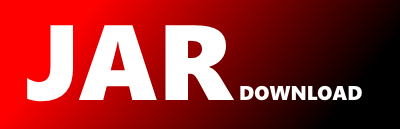
com.amazonaws.services.robomaker.AWSRoboMakerAsync Maven / Gradle / Ivy
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.robomaker;
import javax.annotation.Generated;
import com.amazonaws.services.robomaker.model.*;
/**
* Interface for accessing RoboMaker asynchronously. Each asynchronous method will return a Java Future object
* representing the asynchronous operation; overloads which accept an {@code AsyncHandler} can be used to receive
* notification when an asynchronous operation completes.
*
* Note: Do not directly implement this interface, new methods are added to it regularly. Extend from
* {@link com.amazonaws.services.robomaker.AbstractAWSRoboMakerAsync} instead.
*
*
*
* This section provides documentation for the AWS RoboMaker API operations.
*
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public interface AWSRoboMakerAsync extends AWSRoboMaker {
/**
*
* Deletes one or more worlds in a batch operation.
*
*
* @param batchDeleteWorldsRequest
* @return A Java Future containing the result of the BatchDeleteWorlds operation returned by the service.
* @sample AWSRoboMakerAsync.BatchDeleteWorlds
* @see AWS
* API Documentation
*/
java.util.concurrent.Future batchDeleteWorldsAsync(BatchDeleteWorldsRequest batchDeleteWorldsRequest);
/**
*
* Deletes one or more worlds in a batch operation.
*
*
* @param batchDeleteWorldsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the BatchDeleteWorlds operation returned by the service.
* @sample AWSRoboMakerAsyncHandler.BatchDeleteWorlds
* @see AWS
* API Documentation
*/
java.util.concurrent.Future batchDeleteWorldsAsync(BatchDeleteWorldsRequest batchDeleteWorldsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Describes one or more simulation jobs.
*
*
* @param batchDescribeSimulationJobRequest
* @return A Java Future containing the result of the BatchDescribeSimulationJob operation returned by the service.
* @sample AWSRoboMakerAsync.BatchDescribeSimulationJob
* @see AWS API Documentation
*/
java.util.concurrent.Future batchDescribeSimulationJobAsync(
BatchDescribeSimulationJobRequest batchDescribeSimulationJobRequest);
/**
*
* Describes one or more simulation jobs.
*
*
* @param batchDescribeSimulationJobRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the BatchDescribeSimulationJob operation returned by the service.
* @sample AWSRoboMakerAsyncHandler.BatchDescribeSimulationJob
* @see AWS API Documentation
*/
java.util.concurrent.Future batchDescribeSimulationJobAsync(
BatchDescribeSimulationJobRequest batchDescribeSimulationJobRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Cancels the specified deployment job.
*
*
*
* This API will no longer be supported as of May 2, 2022. Use it to remove resources that were created for
* Deployment Service.
*
*
*
* @param cancelDeploymentJobRequest
* @return A Java Future containing the result of the CancelDeploymentJob operation returned by the service.
* @sample AWSRoboMakerAsync.CancelDeploymentJob
* @see AWS
* API Documentation
*/
@Deprecated
java.util.concurrent.Future cancelDeploymentJobAsync(CancelDeploymentJobRequest cancelDeploymentJobRequest);
/**
*
* Cancels the specified deployment job.
*
*
*
* This API will no longer be supported as of May 2, 2022. Use it to remove resources that were created for
* Deployment Service.
*
*
*
* @param cancelDeploymentJobRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CancelDeploymentJob operation returned by the service.
* @sample AWSRoboMakerAsyncHandler.CancelDeploymentJob
* @see AWS
* API Documentation
*/
@Deprecated
java.util.concurrent.Future cancelDeploymentJobAsync(CancelDeploymentJobRequest cancelDeploymentJobRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Cancels the specified simulation job.
*
*
* @param cancelSimulationJobRequest
* @return A Java Future containing the result of the CancelSimulationJob operation returned by the service.
* @sample AWSRoboMakerAsync.CancelSimulationJob
* @see AWS
* API Documentation
*/
java.util.concurrent.Future cancelSimulationJobAsync(CancelSimulationJobRequest cancelSimulationJobRequest);
/**
*
* Cancels the specified simulation job.
*
*
* @param cancelSimulationJobRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CancelSimulationJob operation returned by the service.
* @sample AWSRoboMakerAsyncHandler.CancelSimulationJob
* @see AWS
* API Documentation
*/
java.util.concurrent.Future cancelSimulationJobAsync(CancelSimulationJobRequest cancelSimulationJobRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Cancels a simulation job batch. When you cancel a simulation job batch, you are also cancelling all of the active
* simulation jobs created as part of the batch.
*
*
* @param cancelSimulationJobBatchRequest
* @return A Java Future containing the result of the CancelSimulationJobBatch operation returned by the service.
* @sample AWSRoboMakerAsync.CancelSimulationJobBatch
* @see AWS API Documentation
*/
java.util.concurrent.Future cancelSimulationJobBatchAsync(CancelSimulationJobBatchRequest cancelSimulationJobBatchRequest);
/**
*
* Cancels a simulation job batch. When you cancel a simulation job batch, you are also cancelling all of the active
* simulation jobs created as part of the batch.
*
*
* @param cancelSimulationJobBatchRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CancelSimulationJobBatch operation returned by the service.
* @sample AWSRoboMakerAsyncHandler.CancelSimulationJobBatch
* @see AWS API Documentation
*/
java.util.concurrent.Future cancelSimulationJobBatchAsync(CancelSimulationJobBatchRequest cancelSimulationJobBatchRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Cancels the specified export job.
*
*
* @param cancelWorldExportJobRequest
* @return A Java Future containing the result of the CancelWorldExportJob operation returned by the service.
* @sample AWSRoboMakerAsync.CancelWorldExportJob
* @see AWS
* API Documentation
*/
java.util.concurrent.Future cancelWorldExportJobAsync(CancelWorldExportJobRequest cancelWorldExportJobRequest);
/**
*
* Cancels the specified export job.
*
*
* @param cancelWorldExportJobRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CancelWorldExportJob operation returned by the service.
* @sample AWSRoboMakerAsyncHandler.CancelWorldExportJob
* @see AWS
* API Documentation
*/
java.util.concurrent.Future cancelWorldExportJobAsync(CancelWorldExportJobRequest cancelWorldExportJobRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Cancels the specified world generator job.
*
*
* @param cancelWorldGenerationJobRequest
* @return A Java Future containing the result of the CancelWorldGenerationJob operation returned by the service.
* @sample AWSRoboMakerAsync.CancelWorldGenerationJob
* @see AWS API Documentation
*/
java.util.concurrent.Future cancelWorldGenerationJobAsync(CancelWorldGenerationJobRequest cancelWorldGenerationJobRequest);
/**
*
* Cancels the specified world generator job.
*
*
* @param cancelWorldGenerationJobRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CancelWorldGenerationJob operation returned by the service.
* @sample AWSRoboMakerAsyncHandler.CancelWorldGenerationJob
* @see AWS API Documentation
*/
java.util.concurrent.Future cancelWorldGenerationJobAsync(CancelWorldGenerationJobRequest cancelWorldGenerationJobRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deploys a specific version of a robot application to robots in a fleet.
*
*
*
* This API is no longer supported and will throw an error if used.
*
*
*
* The robot application must have a numbered applicationVersion
for consistency reasons. To create a
* new version, use CreateRobotApplicationVersion
or see Creating a Robot
* Application Version.
*
*
*
* After 90 days, deployment jobs expire and will be deleted. They will no longer be accessible.
*
*
*
* @param createDeploymentJobRequest
* @return A Java Future containing the result of the CreateDeploymentJob operation returned by the service.
* @sample AWSRoboMakerAsync.CreateDeploymentJob
* @see AWS
* API Documentation
*/
@Deprecated
java.util.concurrent.Future createDeploymentJobAsync(CreateDeploymentJobRequest createDeploymentJobRequest);
/**
*
* Deploys a specific version of a robot application to robots in a fleet.
*
*
*
* This API is no longer supported and will throw an error if used.
*
*
*
* The robot application must have a numbered applicationVersion
for consistency reasons. To create a
* new version, use CreateRobotApplicationVersion
or see Creating a Robot
* Application Version.
*
*
*
* After 90 days, deployment jobs expire and will be deleted. They will no longer be accessible.
*
*
*
* @param createDeploymentJobRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateDeploymentJob operation returned by the service.
* @sample AWSRoboMakerAsyncHandler.CreateDeploymentJob
* @see AWS
* API Documentation
*/
@Deprecated
java.util.concurrent.Future createDeploymentJobAsync(CreateDeploymentJobRequest createDeploymentJobRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates a fleet, a logical group of robots running the same robot application.
*
*
*
* This API is no longer supported and will throw an error if used.
*
*
*
* @param createFleetRequest
* @return A Java Future containing the result of the CreateFleet operation returned by the service.
* @sample AWSRoboMakerAsync.CreateFleet
* @see AWS API
* Documentation
*/
@Deprecated
java.util.concurrent.Future createFleetAsync(CreateFleetRequest createFleetRequest);
/**
*
* Creates a fleet, a logical group of robots running the same robot application.
*
*
*
* This API is no longer supported and will throw an error if used.
*
*
*
* @param createFleetRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateFleet operation returned by the service.
* @sample AWSRoboMakerAsyncHandler.CreateFleet
* @see AWS API
* Documentation
*/
@Deprecated
java.util.concurrent.Future createFleetAsync(CreateFleetRequest createFleetRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates a robot.
*
*
*
* This API is no longer supported and will throw an error if used.
*
*
*
* @param createRobotRequest
* @return A Java Future containing the result of the CreateRobot operation returned by the service.
* @sample AWSRoboMakerAsync.CreateRobot
* @see AWS API
* Documentation
*/
@Deprecated
java.util.concurrent.Future createRobotAsync(CreateRobotRequest createRobotRequest);
/**
*
* Creates a robot.
*
*
*
* This API is no longer supported and will throw an error if used.
*
*
*
* @param createRobotRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateRobot operation returned by the service.
* @sample AWSRoboMakerAsyncHandler.CreateRobot
* @see AWS API
* Documentation
*/
@Deprecated
java.util.concurrent.Future createRobotAsync(CreateRobotRequest createRobotRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates a robot application.
*
*
* @param createRobotApplicationRequest
* @return A Java Future containing the result of the CreateRobotApplication operation returned by the service.
* @sample AWSRoboMakerAsync.CreateRobotApplication
* @see AWS API Documentation
*/
java.util.concurrent.Future createRobotApplicationAsync(CreateRobotApplicationRequest createRobotApplicationRequest);
/**
*
* Creates a robot application.
*
*
* @param createRobotApplicationRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateRobotApplication operation returned by the service.
* @sample AWSRoboMakerAsyncHandler.CreateRobotApplication
* @see AWS API Documentation
*/
java.util.concurrent.Future createRobotApplicationAsync(CreateRobotApplicationRequest createRobotApplicationRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates a version of a robot application.
*
*
* @param createRobotApplicationVersionRequest
* @return A Java Future containing the result of the CreateRobotApplicationVersion operation returned by the
* service.
* @sample AWSRoboMakerAsync.CreateRobotApplicationVersion
* @see AWS API Documentation
*/
java.util.concurrent.Future createRobotApplicationVersionAsync(
CreateRobotApplicationVersionRequest createRobotApplicationVersionRequest);
/**
*
* Creates a version of a robot application.
*
*
* @param createRobotApplicationVersionRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateRobotApplicationVersion operation returned by the
* service.
* @sample AWSRoboMakerAsyncHandler.CreateRobotApplicationVersion
* @see AWS API Documentation
*/
java.util.concurrent.Future createRobotApplicationVersionAsync(
CreateRobotApplicationVersionRequest createRobotApplicationVersionRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates a simulation application.
*
*
* @param createSimulationApplicationRequest
* @return A Java Future containing the result of the CreateSimulationApplication operation returned by the service.
* @sample AWSRoboMakerAsync.CreateSimulationApplication
* @see AWS API Documentation
*/
java.util.concurrent.Future createSimulationApplicationAsync(
CreateSimulationApplicationRequest createSimulationApplicationRequest);
/**
*
* Creates a simulation application.
*
*
* @param createSimulationApplicationRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateSimulationApplication operation returned by the service.
* @sample AWSRoboMakerAsyncHandler.CreateSimulationApplication
* @see AWS API Documentation
*/
java.util.concurrent.Future createSimulationApplicationAsync(
CreateSimulationApplicationRequest createSimulationApplicationRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates a simulation application with a specific revision id.
*
*
* @param createSimulationApplicationVersionRequest
* @return A Java Future containing the result of the CreateSimulationApplicationVersion operation returned by the
* service.
* @sample AWSRoboMakerAsync.CreateSimulationApplicationVersion
* @see AWS API Documentation
*/
java.util.concurrent.Future createSimulationApplicationVersionAsync(
CreateSimulationApplicationVersionRequest createSimulationApplicationVersionRequest);
/**
*
* Creates a simulation application with a specific revision id.
*
*
* @param createSimulationApplicationVersionRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateSimulationApplicationVersion operation returned by the
* service.
* @sample AWSRoboMakerAsyncHandler.CreateSimulationApplicationVersion
* @see AWS API Documentation
*/
java.util.concurrent.Future createSimulationApplicationVersionAsync(
CreateSimulationApplicationVersionRequest createSimulationApplicationVersionRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates a simulation job.
*
*
*
* After 90 days, simulation jobs expire and will be deleted. They will no longer be accessible.
*
*
*
* @param createSimulationJobRequest
* @return A Java Future containing the result of the CreateSimulationJob operation returned by the service.
* @sample AWSRoboMakerAsync.CreateSimulationJob
* @see AWS
* API Documentation
*/
java.util.concurrent.Future createSimulationJobAsync(CreateSimulationJobRequest createSimulationJobRequest);
/**
*
* Creates a simulation job.
*
*
*
* After 90 days, simulation jobs expire and will be deleted. They will no longer be accessible.
*
*
*
* @param createSimulationJobRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateSimulationJob operation returned by the service.
* @sample AWSRoboMakerAsyncHandler.CreateSimulationJob
* @see AWS
* API Documentation
*/
java.util.concurrent.Future createSimulationJobAsync(CreateSimulationJobRequest createSimulationJobRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates a world export job.
*
*
* @param createWorldExportJobRequest
* @return A Java Future containing the result of the CreateWorldExportJob operation returned by the service.
* @sample AWSRoboMakerAsync.CreateWorldExportJob
* @see AWS
* API Documentation
*/
java.util.concurrent.Future createWorldExportJobAsync(CreateWorldExportJobRequest createWorldExportJobRequest);
/**
*
* Creates a world export job.
*
*
* @param createWorldExportJobRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateWorldExportJob operation returned by the service.
* @sample AWSRoboMakerAsyncHandler.CreateWorldExportJob
* @see AWS
* API Documentation
*/
java.util.concurrent.Future createWorldExportJobAsync(CreateWorldExportJobRequest createWorldExportJobRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates worlds using the specified template.
*
*
* @param createWorldGenerationJobRequest
* @return A Java Future containing the result of the CreateWorldGenerationJob operation returned by the service.
* @sample AWSRoboMakerAsync.CreateWorldGenerationJob
* @see AWS API Documentation
*/
java.util.concurrent.Future createWorldGenerationJobAsync(CreateWorldGenerationJobRequest createWorldGenerationJobRequest);
/**
*
* Creates worlds using the specified template.
*
*
* @param createWorldGenerationJobRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateWorldGenerationJob operation returned by the service.
* @sample AWSRoboMakerAsyncHandler.CreateWorldGenerationJob
* @see AWS API Documentation
*/
java.util.concurrent.Future createWorldGenerationJobAsync(CreateWorldGenerationJobRequest createWorldGenerationJobRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates a world template.
*
*
* @param createWorldTemplateRequest
* @return A Java Future containing the result of the CreateWorldTemplate operation returned by the service.
* @sample AWSRoboMakerAsync.CreateWorldTemplate
* @see AWS
* API Documentation
*/
java.util.concurrent.Future createWorldTemplateAsync(CreateWorldTemplateRequest createWorldTemplateRequest);
/**
*
* Creates a world template.
*
*
* @param createWorldTemplateRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateWorldTemplate operation returned by the service.
* @sample AWSRoboMakerAsyncHandler.CreateWorldTemplate
* @see AWS
* API Documentation
*/
java.util.concurrent.Future createWorldTemplateAsync(CreateWorldTemplateRequest createWorldTemplateRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deletes a fleet.
*
*
*
* This API will no longer be supported as of May 2, 2022. Use it to remove resources that were created for
* Deployment Service.
*
*
*
* @param deleteFleetRequest
* @return A Java Future containing the result of the DeleteFleet operation returned by the service.
* @sample AWSRoboMakerAsync.DeleteFleet
* @see AWS API
* Documentation
*/
@Deprecated
java.util.concurrent.Future deleteFleetAsync(DeleteFleetRequest deleteFleetRequest);
/**
*
* Deletes a fleet.
*
*
*
* This API will no longer be supported as of May 2, 2022. Use it to remove resources that were created for
* Deployment Service.
*
*
*
* @param deleteFleetRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteFleet operation returned by the service.
* @sample AWSRoboMakerAsyncHandler.DeleteFleet
* @see AWS API
* Documentation
*/
@Deprecated
java.util.concurrent.Future deleteFleetAsync(DeleteFleetRequest deleteFleetRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deletes a robot.
*
*
*
* This API will no longer be supported as of May 2, 2022. Use it to remove resources that were created for
* Deployment Service.
*
*
*
* @param deleteRobotRequest
* @return A Java Future containing the result of the DeleteRobot operation returned by the service.
* @sample AWSRoboMakerAsync.DeleteRobot
* @see AWS API
* Documentation
*/
@Deprecated
java.util.concurrent.Future deleteRobotAsync(DeleteRobotRequest deleteRobotRequest);
/**
*
* Deletes a robot.
*
*
*
* This API will no longer be supported as of May 2, 2022. Use it to remove resources that were created for
* Deployment Service.
*
*
*
* @param deleteRobotRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteRobot operation returned by the service.
* @sample AWSRoboMakerAsyncHandler.DeleteRobot
* @see AWS API
* Documentation
*/
@Deprecated
java.util.concurrent.Future deleteRobotAsync(DeleteRobotRequest deleteRobotRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deletes a robot application.
*
*
* @param deleteRobotApplicationRequest
* @return A Java Future containing the result of the DeleteRobotApplication operation returned by the service.
* @sample AWSRoboMakerAsync.DeleteRobotApplication
* @see AWS API Documentation
*/
java.util.concurrent.Future deleteRobotApplicationAsync(DeleteRobotApplicationRequest deleteRobotApplicationRequest);
/**
*
* Deletes a robot application.
*
*
* @param deleteRobotApplicationRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteRobotApplication operation returned by the service.
* @sample AWSRoboMakerAsyncHandler.DeleteRobotApplication
* @see AWS API Documentation
*/
java.util.concurrent.Future deleteRobotApplicationAsync(DeleteRobotApplicationRequest deleteRobotApplicationRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deletes a simulation application.
*
*
* @param deleteSimulationApplicationRequest
* @return A Java Future containing the result of the DeleteSimulationApplication operation returned by the service.
* @sample AWSRoboMakerAsync.DeleteSimulationApplication
* @see AWS API Documentation
*/
java.util.concurrent.Future deleteSimulationApplicationAsync(
DeleteSimulationApplicationRequest deleteSimulationApplicationRequest);
/**
*
* Deletes a simulation application.
*
*
* @param deleteSimulationApplicationRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteSimulationApplication operation returned by the service.
* @sample AWSRoboMakerAsyncHandler.DeleteSimulationApplication
* @see AWS API Documentation
*/
java.util.concurrent.Future deleteSimulationApplicationAsync(
DeleteSimulationApplicationRequest deleteSimulationApplicationRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deletes a world template.
*
*
* @param deleteWorldTemplateRequest
* @return A Java Future containing the result of the DeleteWorldTemplate operation returned by the service.
* @sample AWSRoboMakerAsync.DeleteWorldTemplate
* @see AWS
* API Documentation
*/
java.util.concurrent.Future deleteWorldTemplateAsync(DeleteWorldTemplateRequest deleteWorldTemplateRequest);
/**
*
* Deletes a world template.
*
*
* @param deleteWorldTemplateRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteWorldTemplate operation returned by the service.
* @sample AWSRoboMakerAsyncHandler.DeleteWorldTemplate
* @see AWS
* API Documentation
*/
java.util.concurrent.Future deleteWorldTemplateAsync(DeleteWorldTemplateRequest deleteWorldTemplateRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deregisters a robot.
*
*
*
* This API will no longer be supported as of May 2, 2022. Use it to remove resources that were created for
* Deployment Service.
*
*
*
* @param deregisterRobotRequest
* @return A Java Future containing the result of the DeregisterRobot operation returned by the service.
* @sample AWSRoboMakerAsync.DeregisterRobot
* @see AWS API
* Documentation
*/
@Deprecated
java.util.concurrent.Future deregisterRobotAsync(DeregisterRobotRequest deregisterRobotRequest);
/**
*
* Deregisters a robot.
*
*
*
* This API will no longer be supported as of May 2, 2022. Use it to remove resources that were created for
* Deployment Service.
*
*
*
* @param deregisterRobotRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeregisterRobot operation returned by the service.
* @sample AWSRoboMakerAsyncHandler.DeregisterRobot
* @see AWS API
* Documentation
*/
@Deprecated
java.util.concurrent.Future deregisterRobotAsync(DeregisterRobotRequest deregisterRobotRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Describes a deployment job.
*
*
*
* This API will no longer be supported as of May 2, 2022. Use it to remove resources that were created for
* Deployment Service.
*
*
*
* @param describeDeploymentJobRequest
* @return A Java Future containing the result of the DescribeDeploymentJob operation returned by the service.
* @sample AWSRoboMakerAsync.DescribeDeploymentJob
* @see AWS API Documentation
*/
@Deprecated
java.util.concurrent.Future describeDeploymentJobAsync(DescribeDeploymentJobRequest describeDeploymentJobRequest);
/**
*
* Describes a deployment job.
*
*
*
* This API will no longer be supported as of May 2, 2022. Use it to remove resources that were created for
* Deployment Service.
*
*
*
* @param describeDeploymentJobRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeDeploymentJob operation returned by the service.
* @sample AWSRoboMakerAsyncHandler.DescribeDeploymentJob
* @see AWS API Documentation
*/
@Deprecated
java.util.concurrent.Future describeDeploymentJobAsync(DescribeDeploymentJobRequest describeDeploymentJobRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Describes a fleet.
*
*
*
* This API will no longer be supported as of May 2, 2022. Use it to remove resources that were created for
* Deployment Service.
*
*
*
* @param describeFleetRequest
* @return A Java Future containing the result of the DescribeFleet operation returned by the service.
* @sample AWSRoboMakerAsync.DescribeFleet
* @see AWS API
* Documentation
*/
@Deprecated
java.util.concurrent.Future describeFleetAsync(DescribeFleetRequest describeFleetRequest);
/**
*
* Describes a fleet.
*
*
*
* This API will no longer be supported as of May 2, 2022. Use it to remove resources that were created for
* Deployment Service.
*
*
*
* @param describeFleetRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeFleet operation returned by the service.
* @sample AWSRoboMakerAsyncHandler.DescribeFleet
* @see AWS API
* Documentation
*/
@Deprecated
java.util.concurrent.Future describeFleetAsync(DescribeFleetRequest describeFleetRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Describes a robot.
*
*
*
* This API will no longer be supported as of May 2, 2022. Use it to remove resources that were created for
* Deployment Service.
*
*
*
* @param describeRobotRequest
* @return A Java Future containing the result of the DescribeRobot operation returned by the service.
* @sample AWSRoboMakerAsync.DescribeRobot
* @see AWS API
* Documentation
*/
@Deprecated
java.util.concurrent.Future describeRobotAsync(DescribeRobotRequest describeRobotRequest);
/**
*
* Describes a robot.
*
*
*
* This API will no longer be supported as of May 2, 2022. Use it to remove resources that were created for
* Deployment Service.
*
*
*
* @param describeRobotRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeRobot operation returned by the service.
* @sample AWSRoboMakerAsyncHandler.DescribeRobot
* @see AWS API
* Documentation
*/
@Deprecated
java.util.concurrent.Future describeRobotAsync(DescribeRobotRequest describeRobotRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Describes a robot application.
*
*
* @param describeRobotApplicationRequest
* @return A Java Future containing the result of the DescribeRobotApplication operation returned by the service.
* @sample AWSRoboMakerAsync.DescribeRobotApplication
* @see AWS API Documentation
*/
java.util.concurrent.Future describeRobotApplicationAsync(DescribeRobotApplicationRequest describeRobotApplicationRequest);
/**
*
* Describes a robot application.
*
*
* @param describeRobotApplicationRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeRobotApplication operation returned by the service.
* @sample AWSRoboMakerAsyncHandler.DescribeRobotApplication
* @see AWS API Documentation
*/
java.util.concurrent.Future describeRobotApplicationAsync(DescribeRobotApplicationRequest describeRobotApplicationRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Describes a simulation application.
*
*
* @param describeSimulationApplicationRequest
* @return A Java Future containing the result of the DescribeSimulationApplication operation returned by the
* service.
* @sample AWSRoboMakerAsync.DescribeSimulationApplication
* @see AWS API Documentation
*/
java.util.concurrent.Future describeSimulationApplicationAsync(
DescribeSimulationApplicationRequest describeSimulationApplicationRequest);
/**
*
* Describes a simulation application.
*
*
* @param describeSimulationApplicationRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeSimulationApplication operation returned by the
* service.
* @sample AWSRoboMakerAsyncHandler.DescribeSimulationApplication
* @see AWS API Documentation
*/
java.util.concurrent.Future describeSimulationApplicationAsync(
DescribeSimulationApplicationRequest describeSimulationApplicationRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Describes a simulation job.
*
*
* @param describeSimulationJobRequest
* @return A Java Future containing the result of the DescribeSimulationJob operation returned by the service.
* @sample AWSRoboMakerAsync.DescribeSimulationJob
* @see AWS API Documentation
*/
java.util.concurrent.Future describeSimulationJobAsync(DescribeSimulationJobRequest describeSimulationJobRequest);
/**
*
* Describes a simulation job.
*
*
* @param describeSimulationJobRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeSimulationJob operation returned by the service.
* @sample AWSRoboMakerAsyncHandler.DescribeSimulationJob
* @see AWS API Documentation
*/
java.util.concurrent.Future describeSimulationJobAsync(DescribeSimulationJobRequest describeSimulationJobRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Describes a simulation job batch.
*
*
* @param describeSimulationJobBatchRequest
* @return A Java Future containing the result of the DescribeSimulationJobBatch operation returned by the service.
* @sample AWSRoboMakerAsync.DescribeSimulationJobBatch
* @see AWS API Documentation
*/
java.util.concurrent.Future describeSimulationJobBatchAsync(
DescribeSimulationJobBatchRequest describeSimulationJobBatchRequest);
/**
*
* Describes a simulation job batch.
*
*
* @param describeSimulationJobBatchRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeSimulationJobBatch operation returned by the service.
* @sample AWSRoboMakerAsyncHandler.DescribeSimulationJobBatch
* @see AWS API Documentation
*/
java.util.concurrent.Future describeSimulationJobBatchAsync(
DescribeSimulationJobBatchRequest describeSimulationJobBatchRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Describes a world.
*
*
* @param describeWorldRequest
* @return A Java Future containing the result of the DescribeWorld operation returned by the service.
* @sample AWSRoboMakerAsync.DescribeWorld
* @see AWS API
* Documentation
*/
java.util.concurrent.Future describeWorldAsync(DescribeWorldRequest describeWorldRequest);
/**
*
* Describes a world.
*
*
* @param describeWorldRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeWorld operation returned by the service.
* @sample AWSRoboMakerAsyncHandler.DescribeWorld
* @see AWS API
* Documentation
*/
java.util.concurrent.Future describeWorldAsync(DescribeWorldRequest describeWorldRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Describes a world export job.
*
*
* @param describeWorldExportJobRequest
* @return A Java Future containing the result of the DescribeWorldExportJob operation returned by the service.
* @sample AWSRoboMakerAsync.DescribeWorldExportJob
* @see AWS API Documentation
*/
java.util.concurrent.Future describeWorldExportJobAsync(DescribeWorldExportJobRequest describeWorldExportJobRequest);
/**
*
* Describes a world export job.
*
*
* @param describeWorldExportJobRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeWorldExportJob operation returned by the service.
* @sample AWSRoboMakerAsyncHandler.DescribeWorldExportJob
* @see AWS API Documentation
*/
java.util.concurrent.Future describeWorldExportJobAsync(DescribeWorldExportJobRequest describeWorldExportJobRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Describes a world generation job.
*
*
* @param describeWorldGenerationJobRequest
* @return A Java Future containing the result of the DescribeWorldGenerationJob operation returned by the service.
* @sample AWSRoboMakerAsync.DescribeWorldGenerationJob
* @see AWS API Documentation
*/
java.util.concurrent.Future describeWorldGenerationJobAsync(
DescribeWorldGenerationJobRequest describeWorldGenerationJobRequest);
/**
*
* Describes a world generation job.
*
*
* @param describeWorldGenerationJobRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeWorldGenerationJob operation returned by the service.
* @sample AWSRoboMakerAsyncHandler.DescribeWorldGenerationJob
* @see AWS API Documentation
*/
java.util.concurrent.Future describeWorldGenerationJobAsync(
DescribeWorldGenerationJobRequest describeWorldGenerationJobRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Describes a world template.
*
*
* @param describeWorldTemplateRequest
* @return A Java Future containing the result of the DescribeWorldTemplate operation returned by the service.
* @sample AWSRoboMakerAsync.DescribeWorldTemplate
* @see AWS API Documentation
*/
java.util.concurrent.Future describeWorldTemplateAsync(DescribeWorldTemplateRequest describeWorldTemplateRequest);
/**
*
* Describes a world template.
*
*
* @param describeWorldTemplateRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeWorldTemplate operation returned by the service.
* @sample AWSRoboMakerAsyncHandler.DescribeWorldTemplate
* @see AWS API Documentation
*/
java.util.concurrent.Future describeWorldTemplateAsync(DescribeWorldTemplateRequest describeWorldTemplateRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Gets the world template body.
*
*
* @param getWorldTemplateBodyRequest
* @return A Java Future containing the result of the GetWorldTemplateBody operation returned by the service.
* @sample AWSRoboMakerAsync.GetWorldTemplateBody
* @see AWS
* API Documentation
*/
java.util.concurrent.Future getWorldTemplateBodyAsync(GetWorldTemplateBodyRequest getWorldTemplateBodyRequest);
/**
*
* Gets the world template body.
*
*
* @param getWorldTemplateBodyRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetWorldTemplateBody operation returned by the service.
* @sample AWSRoboMakerAsyncHandler.GetWorldTemplateBody
* @see AWS
* API Documentation
*/
java.util.concurrent.Future getWorldTemplateBodyAsync(GetWorldTemplateBodyRequest getWorldTemplateBodyRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns a list of deployment jobs for a fleet. You can optionally provide filters to retrieve specific deployment
* jobs.
*
*
*
* This API will no longer be supported as of May 2, 2022. Use it to remove resources that were created for
* Deployment Service.
*
*
*
* @param listDeploymentJobsRequest
* @return A Java Future containing the result of the ListDeploymentJobs operation returned by the service.
* @sample AWSRoboMakerAsync.ListDeploymentJobs
* @see AWS
* API Documentation
*/
@Deprecated
java.util.concurrent.Future listDeploymentJobsAsync(ListDeploymentJobsRequest listDeploymentJobsRequest);
/**
*
* Returns a list of deployment jobs for a fleet. You can optionally provide filters to retrieve specific deployment
* jobs.
*
*
*
* This API will no longer be supported as of May 2, 2022. Use it to remove resources that were created for
* Deployment Service.
*
*
*
* @param listDeploymentJobsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListDeploymentJobs operation returned by the service.
* @sample AWSRoboMakerAsyncHandler.ListDeploymentJobs
* @see AWS
* API Documentation
*/
@Deprecated
java.util.concurrent.Future listDeploymentJobsAsync(ListDeploymentJobsRequest listDeploymentJobsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns a list of fleets. You can optionally provide filters to retrieve specific fleets.
*
*
*
* This API will no longer be supported as of May 2, 2022. Use it to remove resources that were created for
* Deployment Service.
*
*
*
* @param listFleetsRequest
* @return A Java Future containing the result of the ListFleets operation returned by the service.
* @sample AWSRoboMakerAsync.ListFleets
* @see AWS API
* Documentation
*/
@Deprecated
java.util.concurrent.Future listFleetsAsync(ListFleetsRequest listFleetsRequest);
/**
*
* Returns a list of fleets. You can optionally provide filters to retrieve specific fleets.
*
*
*
* This API will no longer be supported as of May 2, 2022. Use it to remove resources that were created for
* Deployment Service.
*
*
*
* @param listFleetsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListFleets operation returned by the service.
* @sample AWSRoboMakerAsyncHandler.ListFleets
* @see AWS API
* Documentation
*/
@Deprecated
java.util.concurrent.Future listFleetsAsync(ListFleetsRequest listFleetsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns a list of robot application. You can optionally provide filters to retrieve specific robot applications.
*
*
* @param listRobotApplicationsRequest
* @return A Java Future containing the result of the ListRobotApplications operation returned by the service.
* @sample AWSRoboMakerAsync.ListRobotApplications
* @see AWS API Documentation
*/
java.util.concurrent.Future listRobotApplicationsAsync(ListRobotApplicationsRequest listRobotApplicationsRequest);
/**
*
* Returns a list of robot application. You can optionally provide filters to retrieve specific robot applications.
*
*
* @param listRobotApplicationsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListRobotApplications operation returned by the service.
* @sample AWSRoboMakerAsyncHandler.ListRobotApplications
* @see AWS API Documentation
*/
java.util.concurrent.Future listRobotApplicationsAsync(ListRobotApplicationsRequest listRobotApplicationsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns a list of robots. You can optionally provide filters to retrieve specific robots.
*
*
*
* This API will no longer be supported as of May 2, 2022. Use it to remove resources that were created for
* Deployment Service.
*
*
*
* @param listRobotsRequest
* @return A Java Future containing the result of the ListRobots operation returned by the service.
* @sample AWSRoboMakerAsync.ListRobots
* @see AWS API
* Documentation
*/
@Deprecated
java.util.concurrent.Future listRobotsAsync(ListRobotsRequest listRobotsRequest);
/**
*
* Returns a list of robots. You can optionally provide filters to retrieve specific robots.
*
*
*
* This API will no longer be supported as of May 2, 2022. Use it to remove resources that were created for
* Deployment Service.
*
*
*
* @param listRobotsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListRobots operation returned by the service.
* @sample AWSRoboMakerAsyncHandler.ListRobots
* @see AWS API
* Documentation
*/
@Deprecated
java.util.concurrent.Future listRobotsAsync(ListRobotsRequest listRobotsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns a list of simulation applications. You can optionally provide filters to retrieve specific simulation
* applications.
*
*
* @param listSimulationApplicationsRequest
* @return A Java Future containing the result of the ListSimulationApplications operation returned by the service.
* @sample AWSRoboMakerAsync.ListSimulationApplications
* @see AWS API Documentation
*/
java.util.concurrent.Future listSimulationApplicationsAsync(
ListSimulationApplicationsRequest listSimulationApplicationsRequest);
/**
*
* Returns a list of simulation applications. You can optionally provide filters to retrieve specific simulation
* applications.
*
*
* @param listSimulationApplicationsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListSimulationApplications operation returned by the service.
* @sample AWSRoboMakerAsyncHandler.ListSimulationApplications
* @see AWS API Documentation
*/
java.util.concurrent.Future listSimulationApplicationsAsync(
ListSimulationApplicationsRequest listSimulationApplicationsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns a list simulation job batches. You can optionally provide filters to retrieve specific simulation batch
* jobs.
*
*
* @param listSimulationJobBatchesRequest
* @return A Java Future containing the result of the ListSimulationJobBatches operation returned by the service.
* @sample AWSRoboMakerAsync.ListSimulationJobBatches
* @see AWS API Documentation
*/
java.util.concurrent.Future listSimulationJobBatchesAsync(ListSimulationJobBatchesRequest listSimulationJobBatchesRequest);
/**
*
* Returns a list simulation job batches. You can optionally provide filters to retrieve specific simulation batch
* jobs.
*
*
* @param listSimulationJobBatchesRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListSimulationJobBatches operation returned by the service.
* @sample AWSRoboMakerAsyncHandler.ListSimulationJobBatches
* @see AWS API Documentation
*/
java.util.concurrent.Future listSimulationJobBatchesAsync(ListSimulationJobBatchesRequest listSimulationJobBatchesRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns a list of simulation jobs. You can optionally provide filters to retrieve specific simulation jobs.
*
*
* @param listSimulationJobsRequest
* @return A Java Future containing the result of the ListSimulationJobs operation returned by the service.
* @sample AWSRoboMakerAsync.ListSimulationJobs
* @see AWS
* API Documentation
*/
java.util.concurrent.Future listSimulationJobsAsync(ListSimulationJobsRequest listSimulationJobsRequest);
/**
*
* Returns a list of simulation jobs. You can optionally provide filters to retrieve specific simulation jobs.
*
*
* @param listSimulationJobsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListSimulationJobs operation returned by the service.
* @sample AWSRoboMakerAsyncHandler.ListSimulationJobs
* @see AWS
* API Documentation
*/
java.util.concurrent.Future listSimulationJobsAsync(ListSimulationJobsRequest listSimulationJobsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Lists all tags on a AWS RoboMaker resource.
*
*
* @param listTagsForResourceRequest
* @return A Java Future containing the result of the ListTagsForResource operation returned by the service.
* @sample AWSRoboMakerAsync.ListTagsForResource
* @see AWS
* API Documentation
*/
java.util.concurrent.Future listTagsForResourceAsync(ListTagsForResourceRequest listTagsForResourceRequest);
/**
*
* Lists all tags on a AWS RoboMaker resource.
*
*
* @param listTagsForResourceRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListTagsForResource operation returned by the service.
* @sample AWSRoboMakerAsyncHandler.ListTagsForResource
* @see AWS
* API Documentation
*/
java.util.concurrent.Future listTagsForResourceAsync(ListTagsForResourceRequest listTagsForResourceRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Lists world export jobs.
*
*
* @param listWorldExportJobsRequest
* @return A Java Future containing the result of the ListWorldExportJobs operation returned by the service.
* @sample AWSRoboMakerAsync.ListWorldExportJobs
* @see AWS
* API Documentation
*/
java.util.concurrent.Future listWorldExportJobsAsync(ListWorldExportJobsRequest listWorldExportJobsRequest);
/**
*
* Lists world export jobs.
*
*
* @param listWorldExportJobsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListWorldExportJobs operation returned by the service.
* @sample AWSRoboMakerAsyncHandler.ListWorldExportJobs
* @see AWS
* API Documentation
*/
java.util.concurrent.Future listWorldExportJobsAsync(ListWorldExportJobsRequest listWorldExportJobsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Lists world generator jobs.
*
*
* @param listWorldGenerationJobsRequest
* @return A Java Future containing the result of the ListWorldGenerationJobs operation returned by the service.
* @sample AWSRoboMakerAsync.ListWorldGenerationJobs
* @see AWS API Documentation
*/
java.util.concurrent.Future listWorldGenerationJobsAsync(ListWorldGenerationJobsRequest listWorldGenerationJobsRequest);
/**
*
* Lists world generator jobs.
*
*
* @param listWorldGenerationJobsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListWorldGenerationJobs operation returned by the service.
* @sample AWSRoboMakerAsyncHandler.ListWorldGenerationJobs
* @see AWS API Documentation
*/
java.util.concurrent.Future listWorldGenerationJobsAsync(ListWorldGenerationJobsRequest listWorldGenerationJobsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Lists world templates.
*
*
* @param listWorldTemplatesRequest
* @return A Java Future containing the result of the ListWorldTemplates operation returned by the service.
* @sample AWSRoboMakerAsync.ListWorldTemplates
* @see AWS
* API Documentation
*/
java.util.concurrent.Future listWorldTemplatesAsync(ListWorldTemplatesRequest listWorldTemplatesRequest);
/**
*
* Lists world templates.
*
*
* @param listWorldTemplatesRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListWorldTemplates operation returned by the service.
* @sample AWSRoboMakerAsyncHandler.ListWorldTemplates
* @see AWS
* API Documentation
*/
java.util.concurrent.Future listWorldTemplatesAsync(ListWorldTemplatesRequest listWorldTemplatesRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Lists worlds.
*
*
* @param listWorldsRequest
* @return A Java Future containing the result of the ListWorlds operation returned by the service.
* @sample AWSRoboMakerAsync.ListWorlds
* @see AWS API
* Documentation
*/
java.util.concurrent.Future listWorldsAsync(ListWorldsRequest listWorldsRequest);
/**
*
* Lists worlds.
*
*
* @param listWorldsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListWorlds operation returned by the service.
* @sample AWSRoboMakerAsyncHandler.ListWorlds
* @see AWS API
* Documentation
*/
java.util.concurrent.Future listWorldsAsync(ListWorldsRequest listWorldsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Registers a robot with a fleet.
*
*
*
* This API is no longer supported and will throw an error if used.
*
*
*
* @param registerRobotRequest
* @return A Java Future containing the result of the RegisterRobot operation returned by the service.
* @sample AWSRoboMakerAsync.RegisterRobot
* @see AWS API
* Documentation
*/
@Deprecated
java.util.concurrent.Future registerRobotAsync(RegisterRobotRequest registerRobotRequest);
/**
*
* Registers a robot with a fleet.
*
*
*
* This API is no longer supported and will throw an error if used.
*
*
*
* @param registerRobotRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the RegisterRobot operation returned by the service.
* @sample AWSRoboMakerAsyncHandler.RegisterRobot
* @see AWS API
* Documentation
*/
@Deprecated
java.util.concurrent.Future registerRobotAsync(RegisterRobotRequest registerRobotRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Restarts a running simulation job.
*
*
* @param restartSimulationJobRequest
* @return A Java Future containing the result of the RestartSimulationJob operation returned by the service.
* @sample AWSRoboMakerAsync.RestartSimulationJob
* @see AWS
* API Documentation
*/
java.util.concurrent.Future restartSimulationJobAsync(RestartSimulationJobRequest restartSimulationJobRequest);
/**
*
* Restarts a running simulation job.
*
*
* @param restartSimulationJobRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the RestartSimulationJob operation returned by the service.
* @sample AWSRoboMakerAsyncHandler.RestartSimulationJob
* @see AWS
* API Documentation
*/
java.util.concurrent.Future restartSimulationJobAsync(RestartSimulationJobRequest restartSimulationJobRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Starts a new simulation job batch. The batch is defined using one or more SimulationJobRequest
* objects.
*
*
* @param startSimulationJobBatchRequest
* @return A Java Future containing the result of the StartSimulationJobBatch operation returned by the service.
* @sample AWSRoboMakerAsync.StartSimulationJobBatch
* @see AWS API Documentation
*/
java.util.concurrent.Future startSimulationJobBatchAsync(StartSimulationJobBatchRequest startSimulationJobBatchRequest);
/**
*
* Starts a new simulation job batch. The batch is defined using one or more SimulationJobRequest
* objects.
*
*
* @param startSimulationJobBatchRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the StartSimulationJobBatch operation returned by the service.
* @sample AWSRoboMakerAsyncHandler.StartSimulationJobBatch
* @see AWS API Documentation
*/
java.util.concurrent.Future startSimulationJobBatchAsync(StartSimulationJobBatchRequest startSimulationJobBatchRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Syncrhonizes robots in a fleet to the latest deployment. This is helpful if robots were added after a deployment.
*
*
*
* This API will no longer be supported as of May 2, 2022. Use it to remove resources that were created for
* Deployment Service.
*
*
*
* @param syncDeploymentJobRequest
* @return A Java Future containing the result of the SyncDeploymentJob operation returned by the service.
* @sample AWSRoboMakerAsync.SyncDeploymentJob
* @see AWS
* API Documentation
*/
@Deprecated
java.util.concurrent.Future syncDeploymentJobAsync(SyncDeploymentJobRequest syncDeploymentJobRequest);
/**
*
* Syncrhonizes robots in a fleet to the latest deployment. This is helpful if robots were added after a deployment.
*
*
*
* This API will no longer be supported as of May 2, 2022. Use it to remove resources that were created for
* Deployment Service.
*
*
*
* @param syncDeploymentJobRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the SyncDeploymentJob operation returned by the service.
* @sample AWSRoboMakerAsyncHandler.SyncDeploymentJob
* @see AWS
* API Documentation
*/
@Deprecated
java.util.concurrent.Future syncDeploymentJobAsync(SyncDeploymentJobRequest syncDeploymentJobRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Adds or edits tags for a AWS RoboMaker resource.
*
*
* Each tag consists of a tag key and a tag value. Tag keys and tag values are both required, but tag values can be
* empty strings.
*
*
* For information about the rules that apply to tag keys and tag values, see User-Defined
* Tag Restrictions in the AWS Billing and Cost Management User Guide.
*
*
* @param tagResourceRequest
* @return A Java Future containing the result of the TagResource operation returned by the service.
* @sample AWSRoboMakerAsync.TagResource
* @see AWS API
* Documentation
*/
java.util.concurrent.Future tagResourceAsync(TagResourceRequest tagResourceRequest);
/**
*
* Adds or edits tags for a AWS RoboMaker resource.
*
*
* Each tag consists of a tag key and a tag value. Tag keys and tag values are both required, but tag values can be
* empty strings.
*
*
* For information about the rules that apply to tag keys and tag values, see User-Defined
* Tag Restrictions in the AWS Billing and Cost Management User Guide.
*
*
* @param tagResourceRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the TagResource operation returned by the service.
* @sample AWSRoboMakerAsyncHandler.TagResource
* @see AWS API
* Documentation
*/
java.util.concurrent.Future tagResourceAsync(TagResourceRequest tagResourceRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Removes the specified tags from the specified AWS RoboMaker resource.
*
*
* To remove a tag, specify the tag key. To change the tag value of an existing tag key, use TagResource
.
*
*
* @param untagResourceRequest
* @return A Java Future containing the result of the UntagResource operation returned by the service.
* @sample AWSRoboMakerAsync.UntagResource
* @see AWS API
* Documentation
*/
java.util.concurrent.Future untagResourceAsync(UntagResourceRequest untagResourceRequest);
/**
*
* Removes the specified tags from the specified AWS RoboMaker resource.
*
*
* To remove a tag, specify the tag key. To change the tag value of an existing tag key, use TagResource
.
*
*
* @param untagResourceRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the UntagResource operation returned by the service.
* @sample AWSRoboMakerAsyncHandler.UntagResource
* @see AWS API
* Documentation
*/
java.util.concurrent.Future untagResourceAsync(UntagResourceRequest untagResourceRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Updates a robot application.
*
*
* @param updateRobotApplicationRequest
* @return A Java Future containing the result of the UpdateRobotApplication operation returned by the service.
* @sample AWSRoboMakerAsync.UpdateRobotApplication
* @see AWS API Documentation
*/
java.util.concurrent.Future updateRobotApplicationAsync(UpdateRobotApplicationRequest updateRobotApplicationRequest);
/**
*
* Updates a robot application.
*
*
* @param updateRobotApplicationRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the UpdateRobotApplication operation returned by the service.
* @sample AWSRoboMakerAsyncHandler.UpdateRobotApplication
* @see AWS API Documentation
*/
java.util.concurrent.Future updateRobotApplicationAsync(UpdateRobotApplicationRequest updateRobotApplicationRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Updates a simulation application.
*
*
* @param updateSimulationApplicationRequest
* @return A Java Future containing the result of the UpdateSimulationApplication operation returned by the service.
* @sample AWSRoboMakerAsync.UpdateSimulationApplication
* @see AWS API Documentation
*/
java.util.concurrent.Future updateSimulationApplicationAsync(
UpdateSimulationApplicationRequest updateSimulationApplicationRequest);
/**
*
* Updates a simulation application.
*
*
* @param updateSimulationApplicationRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the UpdateSimulationApplication operation returned by the service.
* @sample AWSRoboMakerAsyncHandler.UpdateSimulationApplication
* @see AWS API Documentation
*/
java.util.concurrent.Future updateSimulationApplicationAsync(
UpdateSimulationApplicationRequest updateSimulationApplicationRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Updates a world template.
*
*
* @param updateWorldTemplateRequest
* @return A Java Future containing the result of the UpdateWorldTemplate operation returned by the service.
* @sample AWSRoboMakerAsync.UpdateWorldTemplate
* @see AWS
* API Documentation
*/
java.util.concurrent.Future updateWorldTemplateAsync(UpdateWorldTemplateRequest updateWorldTemplateRequest);
/**
*
* Updates a world template.
*
*
* @param updateWorldTemplateRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the UpdateWorldTemplate operation returned by the service.
* @sample AWSRoboMakerAsyncHandler.UpdateWorldTemplate
* @see AWS
* API Documentation
*/
java.util.concurrent.Future updateWorldTemplateAsync(UpdateWorldTemplateRequest updateWorldTemplateRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
}