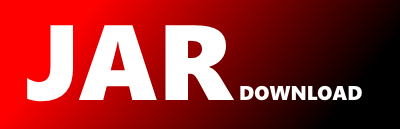
com.amazonaws.services.robomaker.model.LaunchConfig Maven / Gradle / Ivy
Show all versions of aws-java-sdk-robomaker Show documentation
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.robomaker.model;
import java.io.Serializable;
import javax.annotation.Generated;
import com.amazonaws.protocol.StructuredPojo;
import com.amazonaws.protocol.ProtocolMarshaller;
/**
*
* Information about a launch configuration.
*
*
* @see AWS API
* Documentation
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class LaunchConfig implements Serializable, Cloneable, StructuredPojo {
/**
*
* The package name.
*
*/
private String packageName;
/**
*
* The launch file name.
*
*/
private String launchFile;
/**
*
* The environment variables for the application launch.
*
*/
private java.util.Map environmentVariables;
/**
*
* The port forwarding configuration.
*
*/
private PortForwardingConfig portForwardingConfig;
/**
*
* Boolean indicating whether a streaming session will be configured for the application. If True
, AWS
* RoboMaker will configure a connection so you can interact with your application as it is running in the
* simulation. You must configure and launch the component. It must have a graphical user interface.
*
*/
private Boolean streamUI;
/**
*
* If you've specified General
as the value for your RobotSoftwareSuite
, you can use this
* field to specify a list of commands for your container image.
*
*
* If you've specified SimulationRuntime
as the value for your SimulationSoftwareSuite
,
* you can use this field to specify a list of commands for your container image.
*
*/
private java.util.List command;
/**
*
* The package name.
*
*
* @param packageName
* The package name.
*/
public void setPackageName(String packageName) {
this.packageName = packageName;
}
/**
*
* The package name.
*
*
* @return The package name.
*/
public String getPackageName() {
return this.packageName;
}
/**
*
* The package name.
*
*
* @param packageName
* The package name.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public LaunchConfig withPackageName(String packageName) {
setPackageName(packageName);
return this;
}
/**
*
* The launch file name.
*
*
* @param launchFile
* The launch file name.
*/
public void setLaunchFile(String launchFile) {
this.launchFile = launchFile;
}
/**
*
* The launch file name.
*
*
* @return The launch file name.
*/
public String getLaunchFile() {
return this.launchFile;
}
/**
*
* The launch file name.
*
*
* @param launchFile
* The launch file name.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public LaunchConfig withLaunchFile(String launchFile) {
setLaunchFile(launchFile);
return this;
}
/**
*
* The environment variables for the application launch.
*
*
* @return The environment variables for the application launch.
*/
public java.util.Map getEnvironmentVariables() {
return environmentVariables;
}
/**
*
* The environment variables for the application launch.
*
*
* @param environmentVariables
* The environment variables for the application launch.
*/
public void setEnvironmentVariables(java.util.Map environmentVariables) {
this.environmentVariables = environmentVariables;
}
/**
*
* The environment variables for the application launch.
*
*
* @param environmentVariables
* The environment variables for the application launch.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public LaunchConfig withEnvironmentVariables(java.util.Map environmentVariables) {
setEnvironmentVariables(environmentVariables);
return this;
}
/**
* Add a single EnvironmentVariables entry
*
* @see LaunchConfig#withEnvironmentVariables
* @returns a reference to this object so that method calls can be chained together.
*/
public LaunchConfig addEnvironmentVariablesEntry(String key, String value) {
if (null == this.environmentVariables) {
this.environmentVariables = new java.util.HashMap();
}
if (this.environmentVariables.containsKey(key))
throw new IllegalArgumentException("Duplicated keys (" + key.toString() + ") are provided.");
this.environmentVariables.put(key, value);
return this;
}
/**
* Removes all the entries added into EnvironmentVariables.
*
* @return Returns a reference to this object so that method calls can be chained together.
*/
public LaunchConfig clearEnvironmentVariablesEntries() {
this.environmentVariables = null;
return this;
}
/**
*
* The port forwarding configuration.
*
*
* @param portForwardingConfig
* The port forwarding configuration.
*/
public void setPortForwardingConfig(PortForwardingConfig portForwardingConfig) {
this.portForwardingConfig = portForwardingConfig;
}
/**
*
* The port forwarding configuration.
*
*
* @return The port forwarding configuration.
*/
public PortForwardingConfig getPortForwardingConfig() {
return this.portForwardingConfig;
}
/**
*
* The port forwarding configuration.
*
*
* @param portForwardingConfig
* The port forwarding configuration.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public LaunchConfig withPortForwardingConfig(PortForwardingConfig portForwardingConfig) {
setPortForwardingConfig(portForwardingConfig);
return this;
}
/**
*
* Boolean indicating whether a streaming session will be configured for the application. If True
, AWS
* RoboMaker will configure a connection so you can interact with your application as it is running in the
* simulation. You must configure and launch the component. It must have a graphical user interface.
*
*
* @param streamUI
* Boolean indicating whether a streaming session will be configured for the application. If
* True
, AWS RoboMaker will configure a connection so you can interact with your application as
* it is running in the simulation. You must configure and launch the component. It must have a graphical
* user interface.
*/
public void setStreamUI(Boolean streamUI) {
this.streamUI = streamUI;
}
/**
*
* Boolean indicating whether a streaming session will be configured for the application. If True
, AWS
* RoboMaker will configure a connection so you can interact with your application as it is running in the
* simulation. You must configure and launch the component. It must have a graphical user interface.
*
*
* @return Boolean indicating whether a streaming session will be configured for the application. If
* True
, AWS RoboMaker will configure a connection so you can interact with your application as
* it is running in the simulation. You must configure and launch the component. It must have a graphical
* user interface.
*/
public Boolean getStreamUI() {
return this.streamUI;
}
/**
*
* Boolean indicating whether a streaming session will be configured for the application. If True
, AWS
* RoboMaker will configure a connection so you can interact with your application as it is running in the
* simulation. You must configure and launch the component. It must have a graphical user interface.
*
*
* @param streamUI
* Boolean indicating whether a streaming session will be configured for the application. If
* True
, AWS RoboMaker will configure a connection so you can interact with your application as
* it is running in the simulation. You must configure and launch the component. It must have a graphical
* user interface.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public LaunchConfig withStreamUI(Boolean streamUI) {
setStreamUI(streamUI);
return this;
}
/**
*
* Boolean indicating whether a streaming session will be configured for the application. If True
, AWS
* RoboMaker will configure a connection so you can interact with your application as it is running in the
* simulation. You must configure and launch the component. It must have a graphical user interface.
*
*
* @return Boolean indicating whether a streaming session will be configured for the application. If
* True
, AWS RoboMaker will configure a connection so you can interact with your application as
* it is running in the simulation. You must configure and launch the component. It must have a graphical
* user interface.
*/
public Boolean isStreamUI() {
return this.streamUI;
}
/**
*
* If you've specified General
as the value for your RobotSoftwareSuite
, you can use this
* field to specify a list of commands for your container image.
*
*
* If you've specified SimulationRuntime
as the value for your SimulationSoftwareSuite
,
* you can use this field to specify a list of commands for your container image.
*
*
* @return If you've specified General
as the value for your RobotSoftwareSuite
, you can
* use this field to specify a list of commands for your container image.
*
* If you've specified SimulationRuntime
as the value for your
* SimulationSoftwareSuite
, you can use this field to specify a list of commands for your
* container image.
*/
public java.util.List getCommand() {
return command;
}
/**
*
* If you've specified General
as the value for your RobotSoftwareSuite
, you can use this
* field to specify a list of commands for your container image.
*
*
* If you've specified SimulationRuntime
as the value for your SimulationSoftwareSuite
,
* you can use this field to specify a list of commands for your container image.
*
*
* @param command
* If you've specified General
as the value for your RobotSoftwareSuite
, you can
* use this field to specify a list of commands for your container image.
*
* If you've specified SimulationRuntime
as the value for your
* SimulationSoftwareSuite
, you can use this field to specify a list of commands for your
* container image.
*/
public void setCommand(java.util.Collection command) {
if (command == null) {
this.command = null;
return;
}
this.command = new java.util.ArrayList(command);
}
/**
*
* If you've specified General
as the value for your RobotSoftwareSuite
, you can use this
* field to specify a list of commands for your container image.
*
*
* If you've specified SimulationRuntime
as the value for your SimulationSoftwareSuite
,
* you can use this field to specify a list of commands for your container image.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setCommand(java.util.Collection)} or {@link #withCommand(java.util.Collection)} if you want to override
* the existing values.
*
*
* @param command
* If you've specified General
as the value for your RobotSoftwareSuite
, you can
* use this field to specify a list of commands for your container image.
*
* If you've specified SimulationRuntime
as the value for your
* SimulationSoftwareSuite
, you can use this field to specify a list of commands for your
* container image.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public LaunchConfig withCommand(String... command) {
if (this.command == null) {
setCommand(new java.util.ArrayList(command.length));
}
for (String ele : command) {
this.command.add(ele);
}
return this;
}
/**
*
* If you've specified General
as the value for your RobotSoftwareSuite
, you can use this
* field to specify a list of commands for your container image.
*
*
* If you've specified SimulationRuntime
as the value for your SimulationSoftwareSuite
,
* you can use this field to specify a list of commands for your container image.
*
*
* @param command
* If you've specified General
as the value for your RobotSoftwareSuite
, you can
* use this field to specify a list of commands for your container image.
*
* If you've specified SimulationRuntime
as the value for your
* SimulationSoftwareSuite
, you can use this field to specify a list of commands for your
* container image.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public LaunchConfig withCommand(java.util.Collection command) {
setCommand(command);
return this;
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getPackageName() != null)
sb.append("PackageName: ").append(getPackageName()).append(",");
if (getLaunchFile() != null)
sb.append("LaunchFile: ").append(getLaunchFile()).append(",");
if (getEnvironmentVariables() != null)
sb.append("EnvironmentVariables: ").append(getEnvironmentVariables()).append(",");
if (getPortForwardingConfig() != null)
sb.append("PortForwardingConfig: ").append(getPortForwardingConfig()).append(",");
if (getStreamUI() != null)
sb.append("StreamUI: ").append(getStreamUI()).append(",");
if (getCommand() != null)
sb.append("Command: ").append(getCommand());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof LaunchConfig == false)
return false;
LaunchConfig other = (LaunchConfig) obj;
if (other.getPackageName() == null ^ this.getPackageName() == null)
return false;
if (other.getPackageName() != null && other.getPackageName().equals(this.getPackageName()) == false)
return false;
if (other.getLaunchFile() == null ^ this.getLaunchFile() == null)
return false;
if (other.getLaunchFile() != null && other.getLaunchFile().equals(this.getLaunchFile()) == false)
return false;
if (other.getEnvironmentVariables() == null ^ this.getEnvironmentVariables() == null)
return false;
if (other.getEnvironmentVariables() != null && other.getEnvironmentVariables().equals(this.getEnvironmentVariables()) == false)
return false;
if (other.getPortForwardingConfig() == null ^ this.getPortForwardingConfig() == null)
return false;
if (other.getPortForwardingConfig() != null && other.getPortForwardingConfig().equals(this.getPortForwardingConfig()) == false)
return false;
if (other.getStreamUI() == null ^ this.getStreamUI() == null)
return false;
if (other.getStreamUI() != null && other.getStreamUI().equals(this.getStreamUI()) == false)
return false;
if (other.getCommand() == null ^ this.getCommand() == null)
return false;
if (other.getCommand() != null && other.getCommand().equals(this.getCommand()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getPackageName() == null) ? 0 : getPackageName().hashCode());
hashCode = prime * hashCode + ((getLaunchFile() == null) ? 0 : getLaunchFile().hashCode());
hashCode = prime * hashCode + ((getEnvironmentVariables() == null) ? 0 : getEnvironmentVariables().hashCode());
hashCode = prime * hashCode + ((getPortForwardingConfig() == null) ? 0 : getPortForwardingConfig().hashCode());
hashCode = prime * hashCode + ((getStreamUI() == null) ? 0 : getStreamUI().hashCode());
hashCode = prime * hashCode + ((getCommand() == null) ? 0 : getCommand().hashCode());
return hashCode;
}
@Override
public LaunchConfig clone() {
try {
return (LaunchConfig) super.clone();
} catch (CloneNotSupportedException e) {
throw new IllegalStateException("Got a CloneNotSupportedException from Object.clone() " + "even though we're Cloneable!", e);
}
}
@com.amazonaws.annotation.SdkInternalApi
@Override
public void marshall(ProtocolMarshaller protocolMarshaller) {
com.amazonaws.services.robomaker.model.transform.LaunchConfigMarshaller.getInstance().marshall(this, protocolMarshaller);
}
}