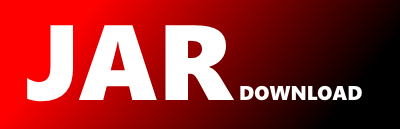
com.amazonaws.services.route53.model.CreateHostedZoneRequest Maven / Gradle / Ivy
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.route53.model;
import java.io.Serializable;
import javax.annotation.Generated;
import com.amazonaws.AmazonWebServiceRequest;
/**
*
* A complex type that contains information about the request to create a public or private hosted zone.
*
*
* @see AWS API
* Documentation
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class CreateHostedZoneRequest extends com.amazonaws.AmazonWebServiceRequest implements Serializable, Cloneable {
/**
*
* The name of the domain. Specify a fully qualified domain name, for example, www.example.com. The trailing
* dot is optional; Amazon Route 53 assumes that the domain name is fully qualified. This means that Route 53 treats
* www.example.com (without a trailing dot) and www.example.com. (with a trailing dot) as identical.
*
*
* If you're creating a public hosted zone, this is the name you have registered with your DNS registrar. If your
* domain name is registered with a registrar other than Route 53, change the name servers for your domain to the
* set of NameServers
that CreateHostedZone
returns in DelegationSet
.
*
*/
private String name;
/**
*
* (Private hosted zones only) A complex type that contains information about the Amazon VPC that you're associating
* with this hosted zone.
*
*
* You can specify only one Amazon VPC when you create a private hosted zone. If you are associating a VPC with a
* hosted zone with this request, the paramaters VPCId
and VPCRegion
are also required.
*
*
* To associate additional Amazon VPCs with the hosted zone, use AssociateVPCWithHostedZone after you create a hosted zone.
*
*/
private VPC vPC;
/**
*
* A unique string that identifies the request and that allows failed CreateHostedZone
requests to be
* retried without the risk of executing the operation twice. You must use a unique CallerReference
* string every time you submit a CreateHostedZone
request. CallerReference
can be any
* unique string, for example, a date/time stamp.
*
*/
private String callerReference;
/**
*
* (Optional) A complex type that contains the following optional values:
*
*
* -
*
* For public and private hosted zones, an optional comment
*
*
* -
*
* For private hosted zones, an optional PrivateZone
element
*
*
*
*
* If you don't specify a comment or the PrivateZone
element, omit HostedZoneConfig
and
* the other elements.
*
*/
private HostedZoneConfig hostedZoneConfig;
/**
*
* If you want to associate a reusable delegation set with this hosted zone, the ID that Amazon Route 53 assigned to
* the reusable delegation set when you created it. For more information about reusable delegation sets, see
* CreateReusableDelegationSet.
*
*
* If you are using a reusable delegation set to create a public hosted zone for a subdomain, make sure that the
* parent hosted zone doesn't use one or more of the same name servers. If you have overlapping nameservers, the
* operation will cause a ConflictingDomainsExist
error.
*
*/
private String delegationSetId;
/**
* Default constructor for CreateHostedZoneRequest object. Callers should use the setter or fluent setter (with...)
* methods to initialize the object after creating it.
*/
public CreateHostedZoneRequest() {
}
/**
* Constructs a new CreateHostedZoneRequest object. Callers should use the setter or fluent setter (with...) methods
* to initialize any additional object members.
*
* @param name
* The name of the domain. Specify a fully qualified domain name, for example, www.example.com. The
* trailing dot is optional; Amazon Route 53 assumes that the domain name is fully qualified. This means that
* Route 53 treats www.example.com (without a trailing dot) and www.example.com. (with a
* trailing dot) as identical.
*
* If you're creating a public hosted zone, this is the name you have registered with your DNS registrar. If
* your domain name is registered with a registrar other than Route 53, change the name servers for your
* domain to the set of NameServers
that CreateHostedZone
returns in
* DelegationSet
.
* @param callerReference
* A unique string that identifies the request and that allows failed CreateHostedZone
requests
* to be retried without the risk of executing the operation twice. You must use a unique
* CallerReference
string every time you submit a CreateHostedZone
request.
* CallerReference
can be any unique string, for example, a date/time stamp.
*/
public CreateHostedZoneRequest(String name, String callerReference) {
setName(name);
setCallerReference(callerReference);
}
/**
*
* The name of the domain. Specify a fully qualified domain name, for example, www.example.com. The trailing
* dot is optional; Amazon Route 53 assumes that the domain name is fully qualified. This means that Route 53 treats
* www.example.com (without a trailing dot) and www.example.com. (with a trailing dot) as identical.
*
*
* If you're creating a public hosted zone, this is the name you have registered with your DNS registrar. If your
* domain name is registered with a registrar other than Route 53, change the name servers for your domain to the
* set of NameServers
that CreateHostedZone
returns in DelegationSet
.
*
*
* @param name
* The name of the domain. Specify a fully qualified domain name, for example, www.example.com. The
* trailing dot is optional; Amazon Route 53 assumes that the domain name is fully qualified. This means that
* Route 53 treats www.example.com (without a trailing dot) and www.example.com. (with a
* trailing dot) as identical.
*
* If you're creating a public hosted zone, this is the name you have registered with your DNS registrar. If
* your domain name is registered with a registrar other than Route 53, change the name servers for your
* domain to the set of NameServers
that CreateHostedZone
returns in
* DelegationSet
.
*/
public void setName(String name) {
this.name = name;
}
/**
*
* The name of the domain. Specify a fully qualified domain name, for example, www.example.com. The trailing
* dot is optional; Amazon Route 53 assumes that the domain name is fully qualified. This means that Route 53 treats
* www.example.com (without a trailing dot) and www.example.com. (with a trailing dot) as identical.
*
*
* If you're creating a public hosted zone, this is the name you have registered with your DNS registrar. If your
* domain name is registered with a registrar other than Route 53, change the name servers for your domain to the
* set of NameServers
that CreateHostedZone
returns in DelegationSet
.
*
*
* @return The name of the domain. Specify a fully qualified domain name, for example, www.example.com. The
* trailing dot is optional; Amazon Route 53 assumes that the domain name is fully qualified. This means
* that Route 53 treats www.example.com (without a trailing dot) and www.example.com. (with a
* trailing dot) as identical.
*
* If you're creating a public hosted zone, this is the name you have registered with your DNS registrar. If
* your domain name is registered with a registrar other than Route 53, change the name servers for your
* domain to the set of NameServers
that CreateHostedZone
returns in
* DelegationSet
.
*/
public String getName() {
return this.name;
}
/**
*
* The name of the domain. Specify a fully qualified domain name, for example, www.example.com. The trailing
* dot is optional; Amazon Route 53 assumes that the domain name is fully qualified. This means that Route 53 treats
* www.example.com (without a trailing dot) and www.example.com. (with a trailing dot) as identical.
*
*
* If you're creating a public hosted zone, this is the name you have registered with your DNS registrar. If your
* domain name is registered with a registrar other than Route 53, change the name servers for your domain to the
* set of NameServers
that CreateHostedZone
returns in DelegationSet
.
*
*
* @param name
* The name of the domain. Specify a fully qualified domain name, for example, www.example.com. The
* trailing dot is optional; Amazon Route 53 assumes that the domain name is fully qualified. This means that
* Route 53 treats www.example.com (without a trailing dot) and www.example.com. (with a
* trailing dot) as identical.
*
* If you're creating a public hosted zone, this is the name you have registered with your DNS registrar. If
* your domain name is registered with a registrar other than Route 53, change the name servers for your
* domain to the set of NameServers
that CreateHostedZone
returns in
* DelegationSet
.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateHostedZoneRequest withName(String name) {
setName(name);
return this;
}
/**
*
* (Private hosted zones only) A complex type that contains information about the Amazon VPC that you're associating
* with this hosted zone.
*
*
* You can specify only one Amazon VPC when you create a private hosted zone. If you are associating a VPC with a
* hosted zone with this request, the paramaters VPCId
and VPCRegion
are also required.
*
*
* To associate additional Amazon VPCs with the hosted zone, use AssociateVPCWithHostedZone after you create a hosted zone.
*
*
* @param vPC
* (Private hosted zones only) A complex type that contains information about the Amazon VPC that you're
* associating with this hosted zone.
*
* You can specify only one Amazon VPC when you create a private hosted zone. If you are associating a VPC
* with a hosted zone with this request, the paramaters VPCId
and VPCRegion
are
* also required.
*
*
* To associate additional Amazon VPCs with the hosted zone, use AssociateVPCWithHostedZone after you create a hosted zone.
*/
public void setVPC(VPC vPC) {
this.vPC = vPC;
}
/**
*
* (Private hosted zones only) A complex type that contains information about the Amazon VPC that you're associating
* with this hosted zone.
*
*
* You can specify only one Amazon VPC when you create a private hosted zone. If you are associating a VPC with a
* hosted zone with this request, the paramaters VPCId
and VPCRegion
are also required.
*
*
* To associate additional Amazon VPCs with the hosted zone, use AssociateVPCWithHostedZone after you create a hosted zone.
*
*
* @return (Private hosted zones only) A complex type that contains information about the Amazon VPC that you're
* associating with this hosted zone.
*
* You can specify only one Amazon VPC when you create a private hosted zone. If you are associating a VPC
* with a hosted zone with this request, the paramaters VPCId
and VPCRegion
are
* also required.
*
*
* To associate additional Amazon VPCs with the hosted zone, use AssociateVPCWithHostedZone after you create a hosted zone.
*/
public VPC getVPC() {
return this.vPC;
}
/**
*
* (Private hosted zones only) A complex type that contains information about the Amazon VPC that you're associating
* with this hosted zone.
*
*
* You can specify only one Amazon VPC when you create a private hosted zone. If you are associating a VPC with a
* hosted zone with this request, the paramaters VPCId
and VPCRegion
are also required.
*
*
* To associate additional Amazon VPCs with the hosted zone, use AssociateVPCWithHostedZone after you create a hosted zone.
*
*
* @param vPC
* (Private hosted zones only) A complex type that contains information about the Amazon VPC that you're
* associating with this hosted zone.
*
* You can specify only one Amazon VPC when you create a private hosted zone. If you are associating a VPC
* with a hosted zone with this request, the paramaters VPCId
and VPCRegion
are
* also required.
*
*
* To associate additional Amazon VPCs with the hosted zone, use AssociateVPCWithHostedZone after you create a hosted zone.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateHostedZoneRequest withVPC(VPC vPC) {
setVPC(vPC);
return this;
}
/**
*
* A unique string that identifies the request and that allows failed CreateHostedZone
requests to be
* retried without the risk of executing the operation twice. You must use a unique CallerReference
* string every time you submit a CreateHostedZone
request. CallerReference
can be any
* unique string, for example, a date/time stamp.
*
*
* @param callerReference
* A unique string that identifies the request and that allows failed CreateHostedZone
requests
* to be retried without the risk of executing the operation twice. You must use a unique
* CallerReference
string every time you submit a CreateHostedZone
request.
* CallerReference
can be any unique string, for example, a date/time stamp.
*/
public void setCallerReference(String callerReference) {
this.callerReference = callerReference;
}
/**
*
* A unique string that identifies the request and that allows failed CreateHostedZone
requests to be
* retried without the risk of executing the operation twice. You must use a unique CallerReference
* string every time you submit a CreateHostedZone
request. CallerReference
can be any
* unique string, for example, a date/time stamp.
*
*
* @return A unique string that identifies the request and that allows failed CreateHostedZone
requests
* to be retried without the risk of executing the operation twice. You must use a unique
* CallerReference
string every time you submit a CreateHostedZone
request.
* CallerReference
can be any unique string, for example, a date/time stamp.
*/
public String getCallerReference() {
return this.callerReference;
}
/**
*
* A unique string that identifies the request and that allows failed CreateHostedZone
requests to be
* retried without the risk of executing the operation twice. You must use a unique CallerReference
* string every time you submit a CreateHostedZone
request. CallerReference
can be any
* unique string, for example, a date/time stamp.
*
*
* @param callerReference
* A unique string that identifies the request and that allows failed CreateHostedZone
requests
* to be retried without the risk of executing the operation twice. You must use a unique
* CallerReference
string every time you submit a CreateHostedZone
request.
* CallerReference
can be any unique string, for example, a date/time stamp.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateHostedZoneRequest withCallerReference(String callerReference) {
setCallerReference(callerReference);
return this;
}
/**
*
* (Optional) A complex type that contains the following optional values:
*
*
* -
*
* For public and private hosted zones, an optional comment
*
*
* -
*
* For private hosted zones, an optional PrivateZone
element
*
*
*
*
* If you don't specify a comment or the PrivateZone
element, omit HostedZoneConfig
and
* the other elements.
*
*
* @param hostedZoneConfig
* (Optional) A complex type that contains the following optional values:
*
* -
*
* For public and private hosted zones, an optional comment
*
*
* -
*
* For private hosted zones, an optional PrivateZone
element
*
*
*
*
* If you don't specify a comment or the PrivateZone
element, omit HostedZoneConfig
* and the other elements.
*/
public void setHostedZoneConfig(HostedZoneConfig hostedZoneConfig) {
this.hostedZoneConfig = hostedZoneConfig;
}
/**
*
* (Optional) A complex type that contains the following optional values:
*
*
* -
*
* For public and private hosted zones, an optional comment
*
*
* -
*
* For private hosted zones, an optional PrivateZone
element
*
*
*
*
* If you don't specify a comment or the PrivateZone
element, omit HostedZoneConfig
and
* the other elements.
*
*
* @return (Optional) A complex type that contains the following optional values:
*
* -
*
* For public and private hosted zones, an optional comment
*
*
* -
*
* For private hosted zones, an optional PrivateZone
element
*
*
*
*
* If you don't specify a comment or the PrivateZone
element, omit
* HostedZoneConfig
and the other elements.
*/
public HostedZoneConfig getHostedZoneConfig() {
return this.hostedZoneConfig;
}
/**
*
* (Optional) A complex type that contains the following optional values:
*
*
* -
*
* For public and private hosted zones, an optional comment
*
*
* -
*
* For private hosted zones, an optional PrivateZone
element
*
*
*
*
* If you don't specify a comment or the PrivateZone
element, omit HostedZoneConfig
and
* the other elements.
*
*
* @param hostedZoneConfig
* (Optional) A complex type that contains the following optional values:
*
* -
*
* For public and private hosted zones, an optional comment
*
*
* -
*
* For private hosted zones, an optional PrivateZone
element
*
*
*
*
* If you don't specify a comment or the PrivateZone
element, omit HostedZoneConfig
* and the other elements.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateHostedZoneRequest withHostedZoneConfig(HostedZoneConfig hostedZoneConfig) {
setHostedZoneConfig(hostedZoneConfig);
return this;
}
/**
*
* If you want to associate a reusable delegation set with this hosted zone, the ID that Amazon Route 53 assigned to
* the reusable delegation set when you created it. For more information about reusable delegation sets, see
* CreateReusableDelegationSet.
*
*
* If you are using a reusable delegation set to create a public hosted zone for a subdomain, make sure that the
* parent hosted zone doesn't use one or more of the same name servers. If you have overlapping nameservers, the
* operation will cause a ConflictingDomainsExist
error.
*
*
* @param delegationSetId
* If you want to associate a reusable delegation set with this hosted zone, the ID that Amazon Route 53
* assigned to the reusable delegation set when you created it. For more information about reusable
* delegation sets, see CreateReusableDelegationSet.
*
* If you are using a reusable delegation set to create a public hosted zone for a subdomain, make sure that
* the parent hosted zone doesn't use one or more of the same name servers. If you have overlapping
* nameservers, the operation will cause a ConflictingDomainsExist
error.
*/
public void setDelegationSetId(String delegationSetId) {
this.delegationSetId = delegationSetId;
}
/**
*
* If you want to associate a reusable delegation set with this hosted zone, the ID that Amazon Route 53 assigned to
* the reusable delegation set when you created it. For more information about reusable delegation sets, see
* CreateReusableDelegationSet.
*
*
* If you are using a reusable delegation set to create a public hosted zone for a subdomain, make sure that the
* parent hosted zone doesn't use one or more of the same name servers. If you have overlapping nameservers, the
* operation will cause a ConflictingDomainsExist
error.
*
*
* @return If you want to associate a reusable delegation set with this hosted zone, the ID that Amazon Route 53
* assigned to the reusable delegation set when you created it. For more information about reusable
* delegation sets, see CreateReusableDelegationSet.
*
* If you are using a reusable delegation set to create a public hosted zone for a subdomain, make sure that
* the parent hosted zone doesn't use one or more of the same name servers. If you have overlapping
* nameservers, the operation will cause a ConflictingDomainsExist
error.
*/
public String getDelegationSetId() {
return this.delegationSetId;
}
/**
*
* If you want to associate a reusable delegation set with this hosted zone, the ID that Amazon Route 53 assigned to
* the reusable delegation set when you created it. For more information about reusable delegation sets, see
* CreateReusableDelegationSet.
*
*
* If you are using a reusable delegation set to create a public hosted zone for a subdomain, make sure that the
* parent hosted zone doesn't use one or more of the same name servers. If you have overlapping nameservers, the
* operation will cause a ConflictingDomainsExist
error.
*
*
* @param delegationSetId
* If you want to associate a reusable delegation set with this hosted zone, the ID that Amazon Route 53
* assigned to the reusable delegation set when you created it. For more information about reusable
* delegation sets, see CreateReusableDelegationSet.
*
* If you are using a reusable delegation set to create a public hosted zone for a subdomain, make sure that
* the parent hosted zone doesn't use one or more of the same name servers. If you have overlapping
* nameservers, the operation will cause a ConflictingDomainsExist
error.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateHostedZoneRequest withDelegationSetId(String delegationSetId) {
setDelegationSetId(delegationSetId);
return this;
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getName() != null)
sb.append("Name: ").append(getName()).append(",");
if (getVPC() != null)
sb.append("VPC: ").append(getVPC()).append(",");
if (getCallerReference() != null)
sb.append("CallerReference: ").append(getCallerReference()).append(",");
if (getHostedZoneConfig() != null)
sb.append("HostedZoneConfig: ").append(getHostedZoneConfig()).append(",");
if (getDelegationSetId() != null)
sb.append("DelegationSetId: ").append(getDelegationSetId());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof CreateHostedZoneRequest == false)
return false;
CreateHostedZoneRequest other = (CreateHostedZoneRequest) obj;
if (other.getName() == null ^ this.getName() == null)
return false;
if (other.getName() != null && other.getName().equals(this.getName()) == false)
return false;
if (other.getVPC() == null ^ this.getVPC() == null)
return false;
if (other.getVPC() != null && other.getVPC().equals(this.getVPC()) == false)
return false;
if (other.getCallerReference() == null ^ this.getCallerReference() == null)
return false;
if (other.getCallerReference() != null && other.getCallerReference().equals(this.getCallerReference()) == false)
return false;
if (other.getHostedZoneConfig() == null ^ this.getHostedZoneConfig() == null)
return false;
if (other.getHostedZoneConfig() != null && other.getHostedZoneConfig().equals(this.getHostedZoneConfig()) == false)
return false;
if (other.getDelegationSetId() == null ^ this.getDelegationSetId() == null)
return false;
if (other.getDelegationSetId() != null && other.getDelegationSetId().equals(this.getDelegationSetId()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getName() == null) ? 0 : getName().hashCode());
hashCode = prime * hashCode + ((getVPC() == null) ? 0 : getVPC().hashCode());
hashCode = prime * hashCode + ((getCallerReference() == null) ? 0 : getCallerReference().hashCode());
hashCode = prime * hashCode + ((getHostedZoneConfig() == null) ? 0 : getHostedZoneConfig().hashCode());
hashCode = prime * hashCode + ((getDelegationSetId() == null) ? 0 : getDelegationSetId().hashCode());
return hashCode;
}
@Override
public CreateHostedZoneRequest clone() {
return (CreateHostedZoneRequest) super.clone();
}
}