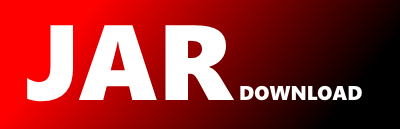
com.amazonaws.services.route53.model.ListHostedZonesRequest Maven / Gradle / Ivy
Show all versions of aws-java-sdk-route53 Show documentation
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.route53.model;
import java.io.Serializable;
import javax.annotation.Generated;
import com.amazonaws.AmazonWebServiceRequest;
/**
*
* A request to retrieve a list of the public and private hosted zones that are associated with the current Amazon Web
* Services account.
*
*
* @see AWS API
* Documentation
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class ListHostedZonesRequest extends com.amazonaws.AmazonWebServiceRequest implements Serializable, Cloneable {
/**
*
* If the value of IsTruncated
in the previous response was true
, you have more hosted
* zones. To get more hosted zones, submit another ListHostedZones
request.
*
*
* For the value of marker
, specify the value of NextMarker
from the previous response,
* which is the ID of the first hosted zone that Amazon Route 53 will return if you submit another request.
*
*
* If the value of IsTruncated
in the previous response was false
, there are no more
* hosted zones to get.
*
*/
private String marker;
/**
*
* (Optional) The maximum number of hosted zones that you want Amazon Route 53 to return. If you have more than
* maxitems
hosted zones, the value of IsTruncated
in the response is true
,
* and the value of NextMarker
is the hosted zone ID of the first hosted zone that Route 53 will return
* if you submit another request.
*
*/
private String maxItems;
/**
*
* If you're using reusable delegation sets and you want to list all of the hosted zones that are associated with a
* reusable delegation set, specify the ID of that reusable delegation set.
*
*/
private String delegationSetId;
/**
*
* (Optional) Specifies if the hosted zone is private.
*
*/
private String hostedZoneType;
/**
*
* If the value of IsTruncated
in the previous response was true
, you have more hosted
* zones. To get more hosted zones, submit another ListHostedZones
request.
*
*
* For the value of marker
, specify the value of NextMarker
from the previous response,
* which is the ID of the first hosted zone that Amazon Route 53 will return if you submit another request.
*
*
* If the value of IsTruncated
in the previous response was false
, there are no more
* hosted zones to get.
*
*
* @param marker
* If the value of IsTruncated
in the previous response was true
, you have more
* hosted zones. To get more hosted zones, submit another ListHostedZones
request.
*
* For the value of marker
, specify the value of NextMarker
from the previous
* response, which is the ID of the first hosted zone that Amazon Route 53 will return if you submit another
* request.
*
*
* If the value of IsTruncated
in the previous response was false
, there are no
* more hosted zones to get.
*/
public void setMarker(String marker) {
this.marker = marker;
}
/**
*
* If the value of IsTruncated
in the previous response was true
, you have more hosted
* zones. To get more hosted zones, submit another ListHostedZones
request.
*
*
* For the value of marker
, specify the value of NextMarker
from the previous response,
* which is the ID of the first hosted zone that Amazon Route 53 will return if you submit another request.
*
*
* If the value of IsTruncated
in the previous response was false
, there are no more
* hosted zones to get.
*
*
* @return If the value of IsTruncated
in the previous response was true
, you have more
* hosted zones. To get more hosted zones, submit another ListHostedZones
request.
*
* For the value of marker
, specify the value of NextMarker
from the previous
* response, which is the ID of the first hosted zone that Amazon Route 53 will return if you submit another
* request.
*
*
* If the value of IsTruncated
in the previous response was false
, there are no
* more hosted zones to get.
*/
public String getMarker() {
return this.marker;
}
/**
*
* If the value of IsTruncated
in the previous response was true
, you have more hosted
* zones. To get more hosted zones, submit another ListHostedZones
request.
*
*
* For the value of marker
, specify the value of NextMarker
from the previous response,
* which is the ID of the first hosted zone that Amazon Route 53 will return if you submit another request.
*
*
* If the value of IsTruncated
in the previous response was false
, there are no more
* hosted zones to get.
*
*
* @param marker
* If the value of IsTruncated
in the previous response was true
, you have more
* hosted zones. To get more hosted zones, submit another ListHostedZones
request.
*
* For the value of marker
, specify the value of NextMarker
from the previous
* response, which is the ID of the first hosted zone that Amazon Route 53 will return if you submit another
* request.
*
*
* If the value of IsTruncated
in the previous response was false
, there are no
* more hosted zones to get.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ListHostedZonesRequest withMarker(String marker) {
setMarker(marker);
return this;
}
/**
*
* (Optional) The maximum number of hosted zones that you want Amazon Route 53 to return. If you have more than
* maxitems
hosted zones, the value of IsTruncated
in the response is true
,
* and the value of NextMarker
is the hosted zone ID of the first hosted zone that Route 53 will return
* if you submit another request.
*
*
* @param maxItems
* (Optional) The maximum number of hosted zones that you want Amazon Route 53 to return. If you have more
* than maxitems
hosted zones, the value of IsTruncated
in the response is
* true
, and the value of NextMarker
is the hosted zone ID of the first hosted zone
* that Route 53 will return if you submit another request.
*/
public void setMaxItems(String maxItems) {
this.maxItems = maxItems;
}
/**
*
* (Optional) The maximum number of hosted zones that you want Amazon Route 53 to return. If you have more than
* maxitems
hosted zones, the value of IsTruncated
in the response is true
,
* and the value of NextMarker
is the hosted zone ID of the first hosted zone that Route 53 will return
* if you submit another request.
*
*
* @return (Optional) The maximum number of hosted zones that you want Amazon Route 53 to return. If you have more
* than maxitems
hosted zones, the value of IsTruncated
in the response is
* true
, and the value of NextMarker
is the hosted zone ID of the first hosted
* zone that Route 53 will return if you submit another request.
*/
public String getMaxItems() {
return this.maxItems;
}
/**
*
* (Optional) The maximum number of hosted zones that you want Amazon Route 53 to return. If you have more than
* maxitems
hosted zones, the value of IsTruncated
in the response is true
,
* and the value of NextMarker
is the hosted zone ID of the first hosted zone that Route 53 will return
* if you submit another request.
*
*
* @param maxItems
* (Optional) The maximum number of hosted zones that you want Amazon Route 53 to return. If you have more
* than maxitems
hosted zones, the value of IsTruncated
in the response is
* true
, and the value of NextMarker
is the hosted zone ID of the first hosted zone
* that Route 53 will return if you submit another request.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ListHostedZonesRequest withMaxItems(String maxItems) {
setMaxItems(maxItems);
return this;
}
/**
*
* If you're using reusable delegation sets and you want to list all of the hosted zones that are associated with a
* reusable delegation set, specify the ID of that reusable delegation set.
*
*
* @param delegationSetId
* If you're using reusable delegation sets and you want to list all of the hosted zones that are associated
* with a reusable delegation set, specify the ID of that reusable delegation set.
*/
public void setDelegationSetId(String delegationSetId) {
this.delegationSetId = delegationSetId;
}
/**
*
* If you're using reusable delegation sets and you want to list all of the hosted zones that are associated with a
* reusable delegation set, specify the ID of that reusable delegation set.
*
*
* @return If you're using reusable delegation sets and you want to list all of the hosted zones that are associated
* with a reusable delegation set, specify the ID of that reusable delegation set.
*/
public String getDelegationSetId() {
return this.delegationSetId;
}
/**
*
* If you're using reusable delegation sets and you want to list all of the hosted zones that are associated with a
* reusable delegation set, specify the ID of that reusable delegation set.
*
*
* @param delegationSetId
* If you're using reusable delegation sets and you want to list all of the hosted zones that are associated
* with a reusable delegation set, specify the ID of that reusable delegation set.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ListHostedZonesRequest withDelegationSetId(String delegationSetId) {
setDelegationSetId(delegationSetId);
return this;
}
/**
*
* (Optional) Specifies if the hosted zone is private.
*
*
* @param hostedZoneType
* (Optional) Specifies if the hosted zone is private.
* @see HostedZoneType
*/
public void setHostedZoneType(String hostedZoneType) {
this.hostedZoneType = hostedZoneType;
}
/**
*
* (Optional) Specifies if the hosted zone is private.
*
*
* @return (Optional) Specifies if the hosted zone is private.
* @see HostedZoneType
*/
public String getHostedZoneType() {
return this.hostedZoneType;
}
/**
*
* (Optional) Specifies if the hosted zone is private.
*
*
* @param hostedZoneType
* (Optional) Specifies if the hosted zone is private.
* @return Returns a reference to this object so that method calls can be chained together.
* @see HostedZoneType
*/
public ListHostedZonesRequest withHostedZoneType(String hostedZoneType) {
setHostedZoneType(hostedZoneType);
return this;
}
/**
*
* (Optional) Specifies if the hosted zone is private.
*
*
* @param hostedZoneType
* (Optional) Specifies if the hosted zone is private.
* @return Returns a reference to this object so that method calls can be chained together.
* @see HostedZoneType
*/
public ListHostedZonesRequest withHostedZoneType(HostedZoneType hostedZoneType) {
this.hostedZoneType = hostedZoneType.toString();
return this;
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getMarker() != null)
sb.append("Marker: ").append(getMarker()).append(",");
if (getMaxItems() != null)
sb.append("MaxItems: ").append(getMaxItems()).append(",");
if (getDelegationSetId() != null)
sb.append("DelegationSetId: ").append(getDelegationSetId()).append(",");
if (getHostedZoneType() != null)
sb.append("HostedZoneType: ").append(getHostedZoneType());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof ListHostedZonesRequest == false)
return false;
ListHostedZonesRequest other = (ListHostedZonesRequest) obj;
if (other.getMarker() == null ^ this.getMarker() == null)
return false;
if (other.getMarker() != null && other.getMarker().equals(this.getMarker()) == false)
return false;
if (other.getMaxItems() == null ^ this.getMaxItems() == null)
return false;
if (other.getMaxItems() != null && other.getMaxItems().equals(this.getMaxItems()) == false)
return false;
if (other.getDelegationSetId() == null ^ this.getDelegationSetId() == null)
return false;
if (other.getDelegationSetId() != null && other.getDelegationSetId().equals(this.getDelegationSetId()) == false)
return false;
if (other.getHostedZoneType() == null ^ this.getHostedZoneType() == null)
return false;
if (other.getHostedZoneType() != null && other.getHostedZoneType().equals(this.getHostedZoneType()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getMarker() == null) ? 0 : getMarker().hashCode());
hashCode = prime * hashCode + ((getMaxItems() == null) ? 0 : getMaxItems().hashCode());
hashCode = prime * hashCode + ((getDelegationSetId() == null) ? 0 : getDelegationSetId().hashCode());
hashCode = prime * hashCode + ((getHostedZoneType() == null) ? 0 : getHostedZoneType().hashCode());
return hashCode;
}
@Override
public ListHostedZonesRequest clone() {
return (ListHostedZonesRequest) super.clone();
}
}