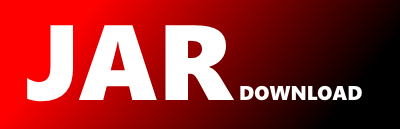
com.amazonaws.services.route53domains.model.GetDomainDetailResult Maven / Gradle / Ivy
Show all versions of aws-java-sdk-route53 Show documentation
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.route53domains.model;
import java.io.Serializable;
import javax.annotation.Generated;
/**
*
* The GetDomainDetail response includes the following elements.
*
*
* @see AWS API
* Documentation
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class GetDomainDetailResult extends com.amazonaws.AmazonWebServiceResult implements Serializable, Cloneable {
/**
*
* The name of a domain.
*
*/
private String domainName;
/**
*
* The name servers of the domain.
*
*/
private com.amazonaws.internal.SdkInternalList nameservers;
/**
*
* Specifies whether the domain registration is set to renew automatically.
*
*/
private Boolean autoRenew;
/**
*
* Provides details about the domain administrative contact.
*
*/
private ContactDetail adminContact;
/**
*
* Provides details about the domain registrant.
*
*/
private ContactDetail registrantContact;
/**
*
* Provides details about the domain technical contact.
*
*/
private ContactDetail techContact;
/**
*
* Specifies whether contact information is concealed from WHOIS queries. If the value is true
, WHOIS
* ("who is") queries return contact information either for Amazon Registrar or for our registrar associate, Gandi.
* If the value is false
, WHOIS queries return the information that you entered for the admin contact.
*
*/
private Boolean adminPrivacy;
/**
*
* Specifies whether contact information is concealed from WHOIS queries. If the value is true
, WHOIS
* ("who is") queries return contact information either for Amazon Registrar or for our registrar associate, Gandi.
* If the value is false
, WHOIS queries return the information that you entered for the registrant
* contact (domain owner).
*
*/
private Boolean registrantPrivacy;
/**
*
* Specifies whether contact information is concealed from WHOIS queries. If the value is true
, WHOIS
* ("who is") queries return contact information either for Amazon Registrar or for our registrar associate, Gandi.
* If the value is false
, WHOIS queries return the information that you entered for the technical
* contact.
*
*/
private Boolean techPrivacy;
/**
*
* Name of the registrar of the domain as identified in the registry.
*
*/
private String registrarName;
/**
*
* The fully qualified name of the WHOIS server that can answer the WHOIS query for the domain.
*
*/
private String whoIsServer;
/**
*
* Web address of the registrar.
*
*/
private String registrarUrl;
/**
*
* Email address to contact to report incorrect contact information for a domain, to report that the domain is being
* used to send spam, to report that someone is cybersquatting on a domain name, or report some other type of abuse.
*
*/
private String abuseContactEmail;
/**
*
* Phone number for reporting abuse.
*
*/
private String abuseContactPhone;
/**
*
* Reserved for future use.
*
*/
private String registryDomainId;
/**
*
* The date when the domain was created as found in the response to a WHOIS query. The date and time is in Unix time
* format and Coordinated Universal time (UTC).
*
*/
private java.util.Date creationDate;
/**
*
* The last updated date of the domain as found in the response to a WHOIS query. The date and time is in Unix time
* format and Coordinated Universal time (UTC).
*
*/
private java.util.Date updatedDate;
/**
*
* The date when the registration for the domain is set to expire. The date and time is in Unix time format and
* Coordinated Universal time (UTC).
*
*/
private java.util.Date expirationDate;
/**
*
* Reseller of the domain. Domains registered or transferred using Route 53 domains will have "Amazon"
* as the reseller.
*
*/
private String reseller;
/**
*
* Deprecated.
*
*/
private String dnsSec;
/**
*
* An array of domain name status codes, also known as Extensible Provisioning Protocol (EPP) status codes.
*
*
* ICANN, the organization that maintains a central database of domain names, has developed a set of domain name
* status codes that tell you the status of a variety of operations on a domain name, for example, registering a
* domain name, transferring a domain name to another registrar, renewing the registration for a domain name, and so
* on. All registrars use this same set of status codes.
*
*
* For a current list of domain name status codes and an explanation of what each code means, go to the ICANN website and search for epp status codes
. (Search on the
* ICANN website; web searches sometimes return an old version of the document.)
*
*/
private com.amazonaws.internal.SdkInternalList statusList;
/**
*
* A complex type that contains information about the DNSSEC configuration.
*
*/
private com.amazonaws.internal.SdkInternalList dnssecKeys;
/**
*
* Provides details about the domain billing contact.
*
*/
private ContactDetail billingContact;
/**
*
* Specifies whether contact information is concealed from WHOIS queries. If the value is true
, WHOIS
* ("who is") queries return contact information either for Amazon Registrar or for our registrar associate, Gandi.
* If the value is false
, WHOIS queries return the information that you entered for the billing
* contact.
*
*/
private Boolean billingPrivacy;
/**
*
* The name of a domain.
*
*
* @param domainName
* The name of a domain.
*/
public void setDomainName(String domainName) {
this.domainName = domainName;
}
/**
*
* The name of a domain.
*
*
* @return The name of a domain.
*/
public String getDomainName() {
return this.domainName;
}
/**
*
* The name of a domain.
*
*
* @param domainName
* The name of a domain.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public GetDomainDetailResult withDomainName(String domainName) {
setDomainName(domainName);
return this;
}
/**
*
* The name servers of the domain.
*
*
* @return The name servers of the domain.
*/
public java.util.List getNameservers() {
if (nameservers == null) {
nameservers = new com.amazonaws.internal.SdkInternalList();
}
return nameservers;
}
/**
*
* The name servers of the domain.
*
*
* @param nameservers
* The name servers of the domain.
*/
public void setNameservers(java.util.Collection nameservers) {
if (nameservers == null) {
this.nameservers = null;
return;
}
this.nameservers = new com.amazonaws.internal.SdkInternalList(nameservers);
}
/**
*
* The name servers of the domain.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setNameservers(java.util.Collection)} or {@link #withNameservers(java.util.Collection)} if you want to
* override the existing values.
*
*
* @param nameservers
* The name servers of the domain.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public GetDomainDetailResult withNameservers(Nameserver... nameservers) {
if (this.nameservers == null) {
setNameservers(new com.amazonaws.internal.SdkInternalList(nameservers.length));
}
for (Nameserver ele : nameservers) {
this.nameservers.add(ele);
}
return this;
}
/**
*
* The name servers of the domain.
*
*
* @param nameservers
* The name servers of the domain.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public GetDomainDetailResult withNameservers(java.util.Collection nameservers) {
setNameservers(nameservers);
return this;
}
/**
*
* Specifies whether the domain registration is set to renew automatically.
*
*
* @param autoRenew
* Specifies whether the domain registration is set to renew automatically.
*/
public void setAutoRenew(Boolean autoRenew) {
this.autoRenew = autoRenew;
}
/**
*
* Specifies whether the domain registration is set to renew automatically.
*
*
* @return Specifies whether the domain registration is set to renew automatically.
*/
public Boolean getAutoRenew() {
return this.autoRenew;
}
/**
*
* Specifies whether the domain registration is set to renew automatically.
*
*
* @param autoRenew
* Specifies whether the domain registration is set to renew automatically.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public GetDomainDetailResult withAutoRenew(Boolean autoRenew) {
setAutoRenew(autoRenew);
return this;
}
/**
*
* Specifies whether the domain registration is set to renew automatically.
*
*
* @return Specifies whether the domain registration is set to renew automatically.
*/
public Boolean isAutoRenew() {
return this.autoRenew;
}
/**
*
* Provides details about the domain administrative contact.
*
*
* @param adminContact
* Provides details about the domain administrative contact.
*/
public void setAdminContact(ContactDetail adminContact) {
this.adminContact = adminContact;
}
/**
*
* Provides details about the domain administrative contact.
*
*
* @return Provides details about the domain administrative contact.
*/
public ContactDetail getAdminContact() {
return this.adminContact;
}
/**
*
* Provides details about the domain administrative contact.
*
*
* @param adminContact
* Provides details about the domain administrative contact.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public GetDomainDetailResult withAdminContact(ContactDetail adminContact) {
setAdminContact(adminContact);
return this;
}
/**
*
* Provides details about the domain registrant.
*
*
* @param registrantContact
* Provides details about the domain registrant.
*/
public void setRegistrantContact(ContactDetail registrantContact) {
this.registrantContact = registrantContact;
}
/**
*
* Provides details about the domain registrant.
*
*
* @return Provides details about the domain registrant.
*/
public ContactDetail getRegistrantContact() {
return this.registrantContact;
}
/**
*
* Provides details about the domain registrant.
*
*
* @param registrantContact
* Provides details about the domain registrant.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public GetDomainDetailResult withRegistrantContact(ContactDetail registrantContact) {
setRegistrantContact(registrantContact);
return this;
}
/**
*
* Provides details about the domain technical contact.
*
*
* @param techContact
* Provides details about the domain technical contact.
*/
public void setTechContact(ContactDetail techContact) {
this.techContact = techContact;
}
/**
*
* Provides details about the domain technical contact.
*
*
* @return Provides details about the domain technical contact.
*/
public ContactDetail getTechContact() {
return this.techContact;
}
/**
*
* Provides details about the domain technical contact.
*
*
* @param techContact
* Provides details about the domain technical contact.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public GetDomainDetailResult withTechContact(ContactDetail techContact) {
setTechContact(techContact);
return this;
}
/**
*
* Specifies whether contact information is concealed from WHOIS queries. If the value is true
, WHOIS
* ("who is") queries return contact information either for Amazon Registrar or for our registrar associate, Gandi.
* If the value is false
, WHOIS queries return the information that you entered for the admin contact.
*
*
* @param adminPrivacy
* Specifies whether contact information is concealed from WHOIS queries. If the value is true
,
* WHOIS ("who is") queries return contact information either for Amazon Registrar or for our registrar
* associate, Gandi. If the value is false
, WHOIS queries return the information that you
* entered for the admin contact.
*/
public void setAdminPrivacy(Boolean adminPrivacy) {
this.adminPrivacy = adminPrivacy;
}
/**
*
* Specifies whether contact information is concealed from WHOIS queries. If the value is true
, WHOIS
* ("who is") queries return contact information either for Amazon Registrar or for our registrar associate, Gandi.
* If the value is false
, WHOIS queries return the information that you entered for the admin contact.
*
*
* @return Specifies whether contact information is concealed from WHOIS queries. If the value is true
,
* WHOIS ("who is") queries return contact information either for Amazon Registrar or for our registrar
* associate, Gandi. If the value is false
, WHOIS queries return the information that you
* entered for the admin contact.
*/
public Boolean getAdminPrivacy() {
return this.adminPrivacy;
}
/**
*
* Specifies whether contact information is concealed from WHOIS queries. If the value is true
, WHOIS
* ("who is") queries return contact information either for Amazon Registrar or for our registrar associate, Gandi.
* If the value is false
, WHOIS queries return the information that you entered for the admin contact.
*
*
* @param adminPrivacy
* Specifies whether contact information is concealed from WHOIS queries. If the value is true
,
* WHOIS ("who is") queries return contact information either for Amazon Registrar or for our registrar
* associate, Gandi. If the value is false
, WHOIS queries return the information that you
* entered for the admin contact.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public GetDomainDetailResult withAdminPrivacy(Boolean adminPrivacy) {
setAdminPrivacy(adminPrivacy);
return this;
}
/**
*
* Specifies whether contact information is concealed from WHOIS queries. If the value is true
, WHOIS
* ("who is") queries return contact information either for Amazon Registrar or for our registrar associate, Gandi.
* If the value is false
, WHOIS queries return the information that you entered for the admin contact.
*
*
* @return Specifies whether contact information is concealed from WHOIS queries. If the value is true
,
* WHOIS ("who is") queries return contact information either for Amazon Registrar or for our registrar
* associate, Gandi. If the value is false
, WHOIS queries return the information that you
* entered for the admin contact.
*/
public Boolean isAdminPrivacy() {
return this.adminPrivacy;
}
/**
*
* Specifies whether contact information is concealed from WHOIS queries. If the value is true
, WHOIS
* ("who is") queries return contact information either for Amazon Registrar or for our registrar associate, Gandi.
* If the value is false
, WHOIS queries return the information that you entered for the registrant
* contact (domain owner).
*
*
* @param registrantPrivacy
* Specifies whether contact information is concealed from WHOIS queries. If the value is true
,
* WHOIS ("who is") queries return contact information either for Amazon Registrar or for our registrar
* associate, Gandi. If the value is false
, WHOIS queries return the information that you
* entered for the registrant contact (domain owner).
*/
public void setRegistrantPrivacy(Boolean registrantPrivacy) {
this.registrantPrivacy = registrantPrivacy;
}
/**
*
* Specifies whether contact information is concealed from WHOIS queries. If the value is true
, WHOIS
* ("who is") queries return contact information either for Amazon Registrar or for our registrar associate, Gandi.
* If the value is false
, WHOIS queries return the information that you entered for the registrant
* contact (domain owner).
*
*
* @return Specifies whether contact information is concealed from WHOIS queries. If the value is true
,
* WHOIS ("who is") queries return contact information either for Amazon Registrar or for our registrar
* associate, Gandi. If the value is false
, WHOIS queries return the information that you
* entered for the registrant contact (domain owner).
*/
public Boolean getRegistrantPrivacy() {
return this.registrantPrivacy;
}
/**
*
* Specifies whether contact information is concealed from WHOIS queries. If the value is true
, WHOIS
* ("who is") queries return contact information either for Amazon Registrar or for our registrar associate, Gandi.
* If the value is false
, WHOIS queries return the information that you entered for the registrant
* contact (domain owner).
*
*
* @param registrantPrivacy
* Specifies whether contact information is concealed from WHOIS queries. If the value is true
,
* WHOIS ("who is") queries return contact information either for Amazon Registrar or for our registrar
* associate, Gandi. If the value is false
, WHOIS queries return the information that you
* entered for the registrant contact (domain owner).
* @return Returns a reference to this object so that method calls can be chained together.
*/
public GetDomainDetailResult withRegistrantPrivacy(Boolean registrantPrivacy) {
setRegistrantPrivacy(registrantPrivacy);
return this;
}
/**
*
* Specifies whether contact information is concealed from WHOIS queries. If the value is true
, WHOIS
* ("who is") queries return contact information either for Amazon Registrar or for our registrar associate, Gandi.
* If the value is false
, WHOIS queries return the information that you entered for the registrant
* contact (domain owner).
*
*
* @return Specifies whether contact information is concealed from WHOIS queries. If the value is true
,
* WHOIS ("who is") queries return contact information either for Amazon Registrar or for our registrar
* associate, Gandi. If the value is false
, WHOIS queries return the information that you
* entered for the registrant contact (domain owner).
*/
public Boolean isRegistrantPrivacy() {
return this.registrantPrivacy;
}
/**
*
* Specifies whether contact information is concealed from WHOIS queries. If the value is true
, WHOIS
* ("who is") queries return contact information either for Amazon Registrar or for our registrar associate, Gandi.
* If the value is false
, WHOIS queries return the information that you entered for the technical
* contact.
*
*
* @param techPrivacy
* Specifies whether contact information is concealed from WHOIS queries. If the value is true
,
* WHOIS ("who is") queries return contact information either for Amazon Registrar or for our registrar
* associate, Gandi. If the value is false
, WHOIS queries return the information that you
* entered for the technical contact.
*/
public void setTechPrivacy(Boolean techPrivacy) {
this.techPrivacy = techPrivacy;
}
/**
*
* Specifies whether contact information is concealed from WHOIS queries. If the value is true
, WHOIS
* ("who is") queries return contact information either for Amazon Registrar or for our registrar associate, Gandi.
* If the value is false
, WHOIS queries return the information that you entered for the technical
* contact.
*
*
* @return Specifies whether contact information is concealed from WHOIS queries. If the value is true
,
* WHOIS ("who is") queries return contact information either for Amazon Registrar or for our registrar
* associate, Gandi. If the value is false
, WHOIS queries return the information that you
* entered for the technical contact.
*/
public Boolean getTechPrivacy() {
return this.techPrivacy;
}
/**
*
* Specifies whether contact information is concealed from WHOIS queries. If the value is true
, WHOIS
* ("who is") queries return contact information either for Amazon Registrar or for our registrar associate, Gandi.
* If the value is false
, WHOIS queries return the information that you entered for the technical
* contact.
*
*
* @param techPrivacy
* Specifies whether contact information is concealed from WHOIS queries. If the value is true
,
* WHOIS ("who is") queries return contact information either for Amazon Registrar or for our registrar
* associate, Gandi. If the value is false
, WHOIS queries return the information that you
* entered for the technical contact.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public GetDomainDetailResult withTechPrivacy(Boolean techPrivacy) {
setTechPrivacy(techPrivacy);
return this;
}
/**
*
* Specifies whether contact information is concealed from WHOIS queries. If the value is true
, WHOIS
* ("who is") queries return contact information either for Amazon Registrar or for our registrar associate, Gandi.
* If the value is false
, WHOIS queries return the information that you entered for the technical
* contact.
*
*
* @return Specifies whether contact information is concealed from WHOIS queries. If the value is true
,
* WHOIS ("who is") queries return contact information either for Amazon Registrar or for our registrar
* associate, Gandi. If the value is false
, WHOIS queries return the information that you
* entered for the technical contact.
*/
public Boolean isTechPrivacy() {
return this.techPrivacy;
}
/**
*
* Name of the registrar of the domain as identified in the registry.
*
*
* @param registrarName
* Name of the registrar of the domain as identified in the registry.
*/
public void setRegistrarName(String registrarName) {
this.registrarName = registrarName;
}
/**
*
* Name of the registrar of the domain as identified in the registry.
*
*
* @return Name of the registrar of the domain as identified in the registry.
*/
public String getRegistrarName() {
return this.registrarName;
}
/**
*
* Name of the registrar of the domain as identified in the registry.
*
*
* @param registrarName
* Name of the registrar of the domain as identified in the registry.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public GetDomainDetailResult withRegistrarName(String registrarName) {
setRegistrarName(registrarName);
return this;
}
/**
*
* The fully qualified name of the WHOIS server that can answer the WHOIS query for the domain.
*
*
* @param whoIsServer
* The fully qualified name of the WHOIS server that can answer the WHOIS query for the domain.
*/
public void setWhoIsServer(String whoIsServer) {
this.whoIsServer = whoIsServer;
}
/**
*
* The fully qualified name of the WHOIS server that can answer the WHOIS query for the domain.
*
*
* @return The fully qualified name of the WHOIS server that can answer the WHOIS query for the domain.
*/
public String getWhoIsServer() {
return this.whoIsServer;
}
/**
*
* The fully qualified name of the WHOIS server that can answer the WHOIS query for the domain.
*
*
* @param whoIsServer
* The fully qualified name of the WHOIS server that can answer the WHOIS query for the domain.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public GetDomainDetailResult withWhoIsServer(String whoIsServer) {
setWhoIsServer(whoIsServer);
return this;
}
/**
*
* Web address of the registrar.
*
*
* @param registrarUrl
* Web address of the registrar.
*/
public void setRegistrarUrl(String registrarUrl) {
this.registrarUrl = registrarUrl;
}
/**
*
* Web address of the registrar.
*
*
* @return Web address of the registrar.
*/
public String getRegistrarUrl() {
return this.registrarUrl;
}
/**
*
* Web address of the registrar.
*
*
* @param registrarUrl
* Web address of the registrar.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public GetDomainDetailResult withRegistrarUrl(String registrarUrl) {
setRegistrarUrl(registrarUrl);
return this;
}
/**
*
* Email address to contact to report incorrect contact information for a domain, to report that the domain is being
* used to send spam, to report that someone is cybersquatting on a domain name, or report some other type of abuse.
*
*
* @param abuseContactEmail
* Email address to contact to report incorrect contact information for a domain, to report that the domain
* is being used to send spam, to report that someone is cybersquatting on a domain name, or report some
* other type of abuse.
*/
public void setAbuseContactEmail(String abuseContactEmail) {
this.abuseContactEmail = abuseContactEmail;
}
/**
*
* Email address to contact to report incorrect contact information for a domain, to report that the domain is being
* used to send spam, to report that someone is cybersquatting on a domain name, or report some other type of abuse.
*
*
* @return Email address to contact to report incorrect contact information for a domain, to report that the domain
* is being used to send spam, to report that someone is cybersquatting on a domain name, or report some
* other type of abuse.
*/
public String getAbuseContactEmail() {
return this.abuseContactEmail;
}
/**
*
* Email address to contact to report incorrect contact information for a domain, to report that the domain is being
* used to send spam, to report that someone is cybersquatting on a domain name, or report some other type of abuse.
*
*
* @param abuseContactEmail
* Email address to contact to report incorrect contact information for a domain, to report that the domain
* is being used to send spam, to report that someone is cybersquatting on a domain name, or report some
* other type of abuse.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public GetDomainDetailResult withAbuseContactEmail(String abuseContactEmail) {
setAbuseContactEmail(abuseContactEmail);
return this;
}
/**
*
* Phone number for reporting abuse.
*
*
* @param abuseContactPhone
* Phone number for reporting abuse.
*/
public void setAbuseContactPhone(String abuseContactPhone) {
this.abuseContactPhone = abuseContactPhone;
}
/**
*
* Phone number for reporting abuse.
*
*
* @return Phone number for reporting abuse.
*/
public String getAbuseContactPhone() {
return this.abuseContactPhone;
}
/**
*
* Phone number for reporting abuse.
*
*
* @param abuseContactPhone
* Phone number for reporting abuse.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public GetDomainDetailResult withAbuseContactPhone(String abuseContactPhone) {
setAbuseContactPhone(abuseContactPhone);
return this;
}
/**
*
* Reserved for future use.
*
*
* @param registryDomainId
* Reserved for future use.
*/
public void setRegistryDomainId(String registryDomainId) {
this.registryDomainId = registryDomainId;
}
/**
*
* Reserved for future use.
*
*
* @return Reserved for future use.
*/
public String getRegistryDomainId() {
return this.registryDomainId;
}
/**
*
* Reserved for future use.
*
*
* @param registryDomainId
* Reserved for future use.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public GetDomainDetailResult withRegistryDomainId(String registryDomainId) {
setRegistryDomainId(registryDomainId);
return this;
}
/**
*
* The date when the domain was created as found in the response to a WHOIS query. The date and time is in Unix time
* format and Coordinated Universal time (UTC).
*
*
* @param creationDate
* The date when the domain was created as found in the response to a WHOIS query. The date and time is in
* Unix time format and Coordinated Universal time (UTC).
*/
public void setCreationDate(java.util.Date creationDate) {
this.creationDate = creationDate;
}
/**
*
* The date when the domain was created as found in the response to a WHOIS query. The date and time is in Unix time
* format and Coordinated Universal time (UTC).
*
*
* @return The date when the domain was created as found in the response to a WHOIS query. The date and time is in
* Unix time format and Coordinated Universal time (UTC).
*/
public java.util.Date getCreationDate() {
return this.creationDate;
}
/**
*
* The date when the domain was created as found in the response to a WHOIS query. The date and time is in Unix time
* format and Coordinated Universal time (UTC).
*
*
* @param creationDate
* The date when the domain was created as found in the response to a WHOIS query. The date and time is in
* Unix time format and Coordinated Universal time (UTC).
* @return Returns a reference to this object so that method calls can be chained together.
*/
public GetDomainDetailResult withCreationDate(java.util.Date creationDate) {
setCreationDate(creationDate);
return this;
}
/**
*
* The last updated date of the domain as found in the response to a WHOIS query. The date and time is in Unix time
* format and Coordinated Universal time (UTC).
*
*
* @param updatedDate
* The last updated date of the domain as found in the response to a WHOIS query. The date and time is in
* Unix time format and Coordinated Universal time (UTC).
*/
public void setUpdatedDate(java.util.Date updatedDate) {
this.updatedDate = updatedDate;
}
/**
*
* The last updated date of the domain as found in the response to a WHOIS query. The date and time is in Unix time
* format and Coordinated Universal time (UTC).
*
*
* @return The last updated date of the domain as found in the response to a WHOIS query. The date and time is in
* Unix time format and Coordinated Universal time (UTC).
*/
public java.util.Date getUpdatedDate() {
return this.updatedDate;
}
/**
*
* The last updated date of the domain as found in the response to a WHOIS query. The date and time is in Unix time
* format and Coordinated Universal time (UTC).
*
*
* @param updatedDate
* The last updated date of the domain as found in the response to a WHOIS query. The date and time is in
* Unix time format and Coordinated Universal time (UTC).
* @return Returns a reference to this object so that method calls can be chained together.
*/
public GetDomainDetailResult withUpdatedDate(java.util.Date updatedDate) {
setUpdatedDate(updatedDate);
return this;
}
/**
*
* The date when the registration for the domain is set to expire. The date and time is in Unix time format and
* Coordinated Universal time (UTC).
*
*
* @param expirationDate
* The date when the registration for the domain is set to expire. The date and time is in Unix time format
* and Coordinated Universal time (UTC).
*/
public void setExpirationDate(java.util.Date expirationDate) {
this.expirationDate = expirationDate;
}
/**
*
* The date when the registration for the domain is set to expire. The date and time is in Unix time format and
* Coordinated Universal time (UTC).
*
*
* @return The date when the registration for the domain is set to expire. The date and time is in Unix time format
* and Coordinated Universal time (UTC).
*/
public java.util.Date getExpirationDate() {
return this.expirationDate;
}
/**
*
* The date when the registration for the domain is set to expire. The date and time is in Unix time format and
* Coordinated Universal time (UTC).
*
*
* @param expirationDate
* The date when the registration for the domain is set to expire. The date and time is in Unix time format
* and Coordinated Universal time (UTC).
* @return Returns a reference to this object so that method calls can be chained together.
*/
public GetDomainDetailResult withExpirationDate(java.util.Date expirationDate) {
setExpirationDate(expirationDate);
return this;
}
/**
*
* Reseller of the domain. Domains registered or transferred using Route 53 domains will have "Amazon"
* as the reseller.
*
*
* @param reseller
* Reseller of the domain. Domains registered or transferred using Route 53 domains will have
* "Amazon"
as the reseller.
*/
public void setReseller(String reseller) {
this.reseller = reseller;
}
/**
*
* Reseller of the domain. Domains registered or transferred using Route 53 domains will have "Amazon"
* as the reseller.
*
*
* @return Reseller of the domain. Domains registered or transferred using Route 53 domains will have
* "Amazon"
as the reseller.
*/
public String getReseller() {
return this.reseller;
}
/**
*
* Reseller of the domain. Domains registered or transferred using Route 53 domains will have "Amazon"
* as the reseller.
*
*
* @param reseller
* Reseller of the domain. Domains registered or transferred using Route 53 domains will have
* "Amazon"
as the reseller.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public GetDomainDetailResult withReseller(String reseller) {
setReseller(reseller);
return this;
}
/**
*
* Deprecated.
*
*
* @param dnsSec
* Deprecated.
*/
public void setDnsSec(String dnsSec) {
this.dnsSec = dnsSec;
}
/**
*
* Deprecated.
*
*
* @return Deprecated.
*/
public String getDnsSec() {
return this.dnsSec;
}
/**
*
* Deprecated.
*
*
* @param dnsSec
* Deprecated.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public GetDomainDetailResult withDnsSec(String dnsSec) {
setDnsSec(dnsSec);
return this;
}
/**
*
* An array of domain name status codes, also known as Extensible Provisioning Protocol (EPP) status codes.
*
*
* ICANN, the organization that maintains a central database of domain names, has developed a set of domain name
* status codes that tell you the status of a variety of operations on a domain name, for example, registering a
* domain name, transferring a domain name to another registrar, renewing the registration for a domain name, and so
* on. All registrars use this same set of status codes.
*
*
* For a current list of domain name status codes and an explanation of what each code means, go to the ICANN website and search for epp status codes
. (Search on the
* ICANN website; web searches sometimes return an old version of the document.)
*
*
* @return An array of domain name status codes, also known as Extensible Provisioning Protocol (EPP) status
* codes.
*
* ICANN, the organization that maintains a central database of domain names, has developed a set of domain
* name status codes that tell you the status of a variety of operations on a domain name, for example,
* registering a domain name, transferring a domain name to another registrar, renewing the registration for
* a domain name, and so on. All registrars use this same set of status codes.
*
*
* For a current list of domain name status codes and an explanation of what each code means, go to the ICANN website and search for epp status codes
. (Search on
* the ICANN website; web searches sometimes return an old version of the document.)
*/
public java.util.List getStatusList() {
if (statusList == null) {
statusList = new com.amazonaws.internal.SdkInternalList();
}
return statusList;
}
/**
*
* An array of domain name status codes, also known as Extensible Provisioning Protocol (EPP) status codes.
*
*
* ICANN, the organization that maintains a central database of domain names, has developed a set of domain name
* status codes that tell you the status of a variety of operations on a domain name, for example, registering a
* domain name, transferring a domain name to another registrar, renewing the registration for a domain name, and so
* on. All registrars use this same set of status codes.
*
*
* For a current list of domain name status codes and an explanation of what each code means, go to the ICANN website and search for epp status codes
. (Search on the
* ICANN website; web searches sometimes return an old version of the document.)
*
*
* @param statusList
* An array of domain name status codes, also known as Extensible Provisioning Protocol (EPP) status
* codes.
*
* ICANN, the organization that maintains a central database of domain names, has developed a set of domain
* name status codes that tell you the status of a variety of operations on a domain name, for example,
* registering a domain name, transferring a domain name to another registrar, renewing the registration for
* a domain name, and so on. All registrars use this same set of status codes.
*
*
* For a current list of domain name status codes and an explanation of what each code means, go to the ICANN website and search for epp status codes
. (Search on
* the ICANN website; web searches sometimes return an old version of the document.)
*/
public void setStatusList(java.util.Collection statusList) {
if (statusList == null) {
this.statusList = null;
return;
}
this.statusList = new com.amazonaws.internal.SdkInternalList(statusList);
}
/**
*
* An array of domain name status codes, also known as Extensible Provisioning Protocol (EPP) status codes.
*
*
* ICANN, the organization that maintains a central database of domain names, has developed a set of domain name
* status codes that tell you the status of a variety of operations on a domain name, for example, registering a
* domain name, transferring a domain name to another registrar, renewing the registration for a domain name, and so
* on. All registrars use this same set of status codes.
*
*
* For a current list of domain name status codes and an explanation of what each code means, go to the ICANN website and search for epp status codes
. (Search on the
* ICANN website; web searches sometimes return an old version of the document.)
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setStatusList(java.util.Collection)} or {@link #withStatusList(java.util.Collection)} if you want to
* override the existing values.
*
*
* @param statusList
* An array of domain name status codes, also known as Extensible Provisioning Protocol (EPP) status
* codes.
*
* ICANN, the organization that maintains a central database of domain names, has developed a set of domain
* name status codes that tell you the status of a variety of operations on a domain name, for example,
* registering a domain name, transferring a domain name to another registrar, renewing the registration for
* a domain name, and so on. All registrars use this same set of status codes.
*
*
* For a current list of domain name status codes and an explanation of what each code means, go to the ICANN website and search for epp status codes
. (Search on
* the ICANN website; web searches sometimes return an old version of the document.)
* @return Returns a reference to this object so that method calls can be chained together.
*/
public GetDomainDetailResult withStatusList(String... statusList) {
if (this.statusList == null) {
setStatusList(new com.amazonaws.internal.SdkInternalList(statusList.length));
}
for (String ele : statusList) {
this.statusList.add(ele);
}
return this;
}
/**
*
* An array of domain name status codes, also known as Extensible Provisioning Protocol (EPP) status codes.
*
*
* ICANN, the organization that maintains a central database of domain names, has developed a set of domain name
* status codes that tell you the status of a variety of operations on a domain name, for example, registering a
* domain name, transferring a domain name to another registrar, renewing the registration for a domain name, and so
* on. All registrars use this same set of status codes.
*
*
* For a current list of domain name status codes and an explanation of what each code means, go to the ICANN website and search for epp status codes
. (Search on the
* ICANN website; web searches sometimes return an old version of the document.)
*
*
* @param statusList
* An array of domain name status codes, also known as Extensible Provisioning Protocol (EPP) status
* codes.
*
* ICANN, the organization that maintains a central database of domain names, has developed a set of domain
* name status codes that tell you the status of a variety of operations on a domain name, for example,
* registering a domain name, transferring a domain name to another registrar, renewing the registration for
* a domain name, and so on. All registrars use this same set of status codes.
*
*
* For a current list of domain name status codes and an explanation of what each code means, go to the ICANN website and search for epp status codes
. (Search on
* the ICANN website; web searches sometimes return an old version of the document.)
* @return Returns a reference to this object so that method calls can be chained together.
*/
public GetDomainDetailResult withStatusList(java.util.Collection statusList) {
setStatusList(statusList);
return this;
}
/**
*
* A complex type that contains information about the DNSSEC configuration.
*
*
* @return A complex type that contains information about the DNSSEC configuration.
*/
public java.util.List getDnssecKeys() {
if (dnssecKeys == null) {
dnssecKeys = new com.amazonaws.internal.SdkInternalList();
}
return dnssecKeys;
}
/**
*
* A complex type that contains information about the DNSSEC configuration.
*
*
* @param dnssecKeys
* A complex type that contains information about the DNSSEC configuration.
*/
public void setDnssecKeys(java.util.Collection dnssecKeys) {
if (dnssecKeys == null) {
this.dnssecKeys = null;
return;
}
this.dnssecKeys = new com.amazonaws.internal.SdkInternalList(dnssecKeys);
}
/**
*
* A complex type that contains information about the DNSSEC configuration.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setDnssecKeys(java.util.Collection)} or {@link #withDnssecKeys(java.util.Collection)} if you want to
* override the existing values.
*
*
* @param dnssecKeys
* A complex type that contains information about the DNSSEC configuration.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public GetDomainDetailResult withDnssecKeys(DnssecKey... dnssecKeys) {
if (this.dnssecKeys == null) {
setDnssecKeys(new com.amazonaws.internal.SdkInternalList(dnssecKeys.length));
}
for (DnssecKey ele : dnssecKeys) {
this.dnssecKeys.add(ele);
}
return this;
}
/**
*
* A complex type that contains information about the DNSSEC configuration.
*
*
* @param dnssecKeys
* A complex type that contains information about the DNSSEC configuration.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public GetDomainDetailResult withDnssecKeys(java.util.Collection dnssecKeys) {
setDnssecKeys(dnssecKeys);
return this;
}
/**
*
* Provides details about the domain billing contact.
*
*
* @param billingContact
* Provides details about the domain billing contact.
*/
public void setBillingContact(ContactDetail billingContact) {
this.billingContact = billingContact;
}
/**
*
* Provides details about the domain billing contact.
*
*
* @return Provides details about the domain billing contact.
*/
public ContactDetail getBillingContact() {
return this.billingContact;
}
/**
*
* Provides details about the domain billing contact.
*
*
* @param billingContact
* Provides details about the domain billing contact.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public GetDomainDetailResult withBillingContact(ContactDetail billingContact) {
setBillingContact(billingContact);
return this;
}
/**
*
* Specifies whether contact information is concealed from WHOIS queries. If the value is true
, WHOIS
* ("who is") queries return contact information either for Amazon Registrar or for our registrar associate, Gandi.
* If the value is false
, WHOIS queries return the information that you entered for the billing
* contact.
*
*
* @param billingPrivacy
* Specifies whether contact information is concealed from WHOIS queries. If the value is true
,
* WHOIS ("who is") queries return contact information either for Amazon Registrar or for our registrar
* associate, Gandi. If the value is false
, WHOIS queries return the information that you
* entered for the billing contact.
*/
public void setBillingPrivacy(Boolean billingPrivacy) {
this.billingPrivacy = billingPrivacy;
}
/**
*
* Specifies whether contact information is concealed from WHOIS queries. If the value is true
, WHOIS
* ("who is") queries return contact information either for Amazon Registrar or for our registrar associate, Gandi.
* If the value is false
, WHOIS queries return the information that you entered for the billing
* contact.
*
*
* @return Specifies whether contact information is concealed from WHOIS queries. If the value is true
,
* WHOIS ("who is") queries return contact information either for Amazon Registrar or for our registrar
* associate, Gandi. If the value is false
, WHOIS queries return the information that you
* entered for the billing contact.
*/
public Boolean getBillingPrivacy() {
return this.billingPrivacy;
}
/**
*
* Specifies whether contact information is concealed from WHOIS queries. If the value is true
, WHOIS
* ("who is") queries return contact information either for Amazon Registrar or for our registrar associate, Gandi.
* If the value is false
, WHOIS queries return the information that you entered for the billing
* contact.
*
*
* @param billingPrivacy
* Specifies whether contact information is concealed from WHOIS queries. If the value is true
,
* WHOIS ("who is") queries return contact information either for Amazon Registrar or for our registrar
* associate, Gandi. If the value is false
, WHOIS queries return the information that you
* entered for the billing contact.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public GetDomainDetailResult withBillingPrivacy(Boolean billingPrivacy) {
setBillingPrivacy(billingPrivacy);
return this;
}
/**
*
* Specifies whether contact information is concealed from WHOIS queries. If the value is true
, WHOIS
* ("who is") queries return contact information either for Amazon Registrar or for our registrar associate, Gandi.
* If the value is false
, WHOIS queries return the information that you entered for the billing
* contact.
*
*
* @return Specifies whether contact information is concealed from WHOIS queries. If the value is true
,
* WHOIS ("who is") queries return contact information either for Amazon Registrar or for our registrar
* associate, Gandi. If the value is false
, WHOIS queries return the information that you
* entered for the billing contact.
*/
public Boolean isBillingPrivacy() {
return this.billingPrivacy;
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getDomainName() != null)
sb.append("DomainName: ").append(getDomainName()).append(",");
if (getNameservers() != null)
sb.append("Nameservers: ").append(getNameservers()).append(",");
if (getAutoRenew() != null)
sb.append("AutoRenew: ").append(getAutoRenew()).append(",");
if (getAdminContact() != null)
sb.append("AdminContact: ").append("***Sensitive Data Redacted***").append(",");
if (getRegistrantContact() != null)
sb.append("RegistrantContact: ").append("***Sensitive Data Redacted***").append(",");
if (getTechContact() != null)
sb.append("TechContact: ").append("***Sensitive Data Redacted***").append(",");
if (getAdminPrivacy() != null)
sb.append("AdminPrivacy: ").append(getAdminPrivacy()).append(",");
if (getRegistrantPrivacy() != null)
sb.append("RegistrantPrivacy: ").append(getRegistrantPrivacy()).append(",");
if (getTechPrivacy() != null)
sb.append("TechPrivacy: ").append(getTechPrivacy()).append(",");
if (getRegistrarName() != null)
sb.append("RegistrarName: ").append(getRegistrarName()).append(",");
if (getWhoIsServer() != null)
sb.append("WhoIsServer: ").append(getWhoIsServer()).append(",");
if (getRegistrarUrl() != null)
sb.append("RegistrarUrl: ").append(getRegistrarUrl()).append(",");
if (getAbuseContactEmail() != null)
sb.append("AbuseContactEmail: ").append("***Sensitive Data Redacted***").append(",");
if (getAbuseContactPhone() != null)
sb.append("AbuseContactPhone: ").append("***Sensitive Data Redacted***").append(",");
if (getRegistryDomainId() != null)
sb.append("RegistryDomainId: ").append(getRegistryDomainId()).append(",");
if (getCreationDate() != null)
sb.append("CreationDate: ").append(getCreationDate()).append(",");
if (getUpdatedDate() != null)
sb.append("UpdatedDate: ").append(getUpdatedDate()).append(",");
if (getExpirationDate() != null)
sb.append("ExpirationDate: ").append(getExpirationDate()).append(",");
if (getReseller() != null)
sb.append("Reseller: ").append(getReseller()).append(",");
if (getDnsSec() != null)
sb.append("DnsSec: ").append(getDnsSec()).append(",");
if (getStatusList() != null)
sb.append("StatusList: ").append(getStatusList()).append(",");
if (getDnssecKeys() != null)
sb.append("DnssecKeys: ").append(getDnssecKeys()).append(",");
if (getBillingContact() != null)
sb.append("BillingContact: ").append("***Sensitive Data Redacted***").append(",");
if (getBillingPrivacy() != null)
sb.append("BillingPrivacy: ").append(getBillingPrivacy());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof GetDomainDetailResult == false)
return false;
GetDomainDetailResult other = (GetDomainDetailResult) obj;
if (other.getDomainName() == null ^ this.getDomainName() == null)
return false;
if (other.getDomainName() != null && other.getDomainName().equals(this.getDomainName()) == false)
return false;
if (other.getNameservers() == null ^ this.getNameservers() == null)
return false;
if (other.getNameservers() != null && other.getNameservers().equals(this.getNameservers()) == false)
return false;
if (other.getAutoRenew() == null ^ this.getAutoRenew() == null)
return false;
if (other.getAutoRenew() != null && other.getAutoRenew().equals(this.getAutoRenew()) == false)
return false;
if (other.getAdminContact() == null ^ this.getAdminContact() == null)
return false;
if (other.getAdminContact() != null && other.getAdminContact().equals(this.getAdminContact()) == false)
return false;
if (other.getRegistrantContact() == null ^ this.getRegistrantContact() == null)
return false;
if (other.getRegistrantContact() != null && other.getRegistrantContact().equals(this.getRegistrantContact()) == false)
return false;
if (other.getTechContact() == null ^ this.getTechContact() == null)
return false;
if (other.getTechContact() != null && other.getTechContact().equals(this.getTechContact()) == false)
return false;
if (other.getAdminPrivacy() == null ^ this.getAdminPrivacy() == null)
return false;
if (other.getAdminPrivacy() != null && other.getAdminPrivacy().equals(this.getAdminPrivacy()) == false)
return false;
if (other.getRegistrantPrivacy() == null ^ this.getRegistrantPrivacy() == null)
return false;
if (other.getRegistrantPrivacy() != null && other.getRegistrantPrivacy().equals(this.getRegistrantPrivacy()) == false)
return false;
if (other.getTechPrivacy() == null ^ this.getTechPrivacy() == null)
return false;
if (other.getTechPrivacy() != null && other.getTechPrivacy().equals(this.getTechPrivacy()) == false)
return false;
if (other.getRegistrarName() == null ^ this.getRegistrarName() == null)
return false;
if (other.getRegistrarName() != null && other.getRegistrarName().equals(this.getRegistrarName()) == false)
return false;
if (other.getWhoIsServer() == null ^ this.getWhoIsServer() == null)
return false;
if (other.getWhoIsServer() != null && other.getWhoIsServer().equals(this.getWhoIsServer()) == false)
return false;
if (other.getRegistrarUrl() == null ^ this.getRegistrarUrl() == null)
return false;
if (other.getRegistrarUrl() != null && other.getRegistrarUrl().equals(this.getRegistrarUrl()) == false)
return false;
if (other.getAbuseContactEmail() == null ^ this.getAbuseContactEmail() == null)
return false;
if (other.getAbuseContactEmail() != null && other.getAbuseContactEmail().equals(this.getAbuseContactEmail()) == false)
return false;
if (other.getAbuseContactPhone() == null ^ this.getAbuseContactPhone() == null)
return false;
if (other.getAbuseContactPhone() != null && other.getAbuseContactPhone().equals(this.getAbuseContactPhone()) == false)
return false;
if (other.getRegistryDomainId() == null ^ this.getRegistryDomainId() == null)
return false;
if (other.getRegistryDomainId() != null && other.getRegistryDomainId().equals(this.getRegistryDomainId()) == false)
return false;
if (other.getCreationDate() == null ^ this.getCreationDate() == null)
return false;
if (other.getCreationDate() != null && other.getCreationDate().equals(this.getCreationDate()) == false)
return false;
if (other.getUpdatedDate() == null ^ this.getUpdatedDate() == null)
return false;
if (other.getUpdatedDate() != null && other.getUpdatedDate().equals(this.getUpdatedDate()) == false)
return false;
if (other.getExpirationDate() == null ^ this.getExpirationDate() == null)
return false;
if (other.getExpirationDate() != null && other.getExpirationDate().equals(this.getExpirationDate()) == false)
return false;
if (other.getReseller() == null ^ this.getReseller() == null)
return false;
if (other.getReseller() != null && other.getReseller().equals(this.getReseller()) == false)
return false;
if (other.getDnsSec() == null ^ this.getDnsSec() == null)
return false;
if (other.getDnsSec() != null && other.getDnsSec().equals(this.getDnsSec()) == false)
return false;
if (other.getStatusList() == null ^ this.getStatusList() == null)
return false;
if (other.getStatusList() != null && other.getStatusList().equals(this.getStatusList()) == false)
return false;
if (other.getDnssecKeys() == null ^ this.getDnssecKeys() == null)
return false;
if (other.getDnssecKeys() != null && other.getDnssecKeys().equals(this.getDnssecKeys()) == false)
return false;
if (other.getBillingContact() == null ^ this.getBillingContact() == null)
return false;
if (other.getBillingContact() != null && other.getBillingContact().equals(this.getBillingContact()) == false)
return false;
if (other.getBillingPrivacy() == null ^ this.getBillingPrivacy() == null)
return false;
if (other.getBillingPrivacy() != null && other.getBillingPrivacy().equals(this.getBillingPrivacy()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getDomainName() == null) ? 0 : getDomainName().hashCode());
hashCode = prime * hashCode + ((getNameservers() == null) ? 0 : getNameservers().hashCode());
hashCode = prime * hashCode + ((getAutoRenew() == null) ? 0 : getAutoRenew().hashCode());
hashCode = prime * hashCode + ((getAdminContact() == null) ? 0 : getAdminContact().hashCode());
hashCode = prime * hashCode + ((getRegistrantContact() == null) ? 0 : getRegistrantContact().hashCode());
hashCode = prime * hashCode + ((getTechContact() == null) ? 0 : getTechContact().hashCode());
hashCode = prime * hashCode + ((getAdminPrivacy() == null) ? 0 : getAdminPrivacy().hashCode());
hashCode = prime * hashCode + ((getRegistrantPrivacy() == null) ? 0 : getRegistrantPrivacy().hashCode());
hashCode = prime * hashCode + ((getTechPrivacy() == null) ? 0 : getTechPrivacy().hashCode());
hashCode = prime * hashCode + ((getRegistrarName() == null) ? 0 : getRegistrarName().hashCode());
hashCode = prime * hashCode + ((getWhoIsServer() == null) ? 0 : getWhoIsServer().hashCode());
hashCode = prime * hashCode + ((getRegistrarUrl() == null) ? 0 : getRegistrarUrl().hashCode());
hashCode = prime * hashCode + ((getAbuseContactEmail() == null) ? 0 : getAbuseContactEmail().hashCode());
hashCode = prime * hashCode + ((getAbuseContactPhone() == null) ? 0 : getAbuseContactPhone().hashCode());
hashCode = prime * hashCode + ((getRegistryDomainId() == null) ? 0 : getRegistryDomainId().hashCode());
hashCode = prime * hashCode + ((getCreationDate() == null) ? 0 : getCreationDate().hashCode());
hashCode = prime * hashCode + ((getUpdatedDate() == null) ? 0 : getUpdatedDate().hashCode());
hashCode = prime * hashCode + ((getExpirationDate() == null) ? 0 : getExpirationDate().hashCode());
hashCode = prime * hashCode + ((getReseller() == null) ? 0 : getReseller().hashCode());
hashCode = prime * hashCode + ((getDnsSec() == null) ? 0 : getDnsSec().hashCode());
hashCode = prime * hashCode + ((getStatusList() == null) ? 0 : getStatusList().hashCode());
hashCode = prime * hashCode + ((getDnssecKeys() == null) ? 0 : getDnssecKeys().hashCode());
hashCode = prime * hashCode + ((getBillingContact() == null) ? 0 : getBillingContact().hashCode());
hashCode = prime * hashCode + ((getBillingPrivacy() == null) ? 0 : getBillingPrivacy().hashCode());
return hashCode;
}
@Override
public GetDomainDetailResult clone() {
try {
return (GetDomainDetailResult) super.clone();
} catch (CloneNotSupportedException e) {
throw new IllegalStateException("Got a CloneNotSupportedException from Object.clone() " + "even though we're Cloneable!", e);
}
}
}