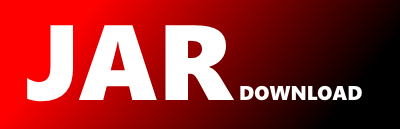
com.amazonaws.services.route53domains.model.UpdateDomainContactRequest Maven / Gradle / Ivy
Show all versions of aws-java-sdk-route53 Show documentation
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.route53domains.model;
import java.io.Serializable;
import javax.annotation.Generated;
import com.amazonaws.AmazonWebServiceRequest;
/**
*
* The UpdateDomainContact request includes the following elements.
*
*
* @see AWS
* API Documentation
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class UpdateDomainContactRequest extends com.amazonaws.AmazonWebServiceRequest implements Serializable, Cloneable {
/**
*
* The name of the domain that you want to update contact information for.
*
*/
private String domainName;
/**
*
* Provides detailed contact information.
*
*/
private ContactDetail adminContact;
/**
*
* Provides detailed contact information.
*
*/
private ContactDetail registrantContact;
/**
*
* Provides detailed contact information.
*
*/
private ContactDetail techContact;
/**
*
* Customer's consent for the owner change request. Required if the domain is not free (consent price is more than
* $0.00).
*
*/
private Consent consent;
/**
*
* Provides detailed contact information.
*
*/
private ContactDetail billingContact;
/**
*
* The name of the domain that you want to update contact information for.
*
*
* @param domainName
* The name of the domain that you want to update contact information for.
*/
public void setDomainName(String domainName) {
this.domainName = domainName;
}
/**
*
* The name of the domain that you want to update contact information for.
*
*
* @return The name of the domain that you want to update contact information for.
*/
public String getDomainName() {
return this.domainName;
}
/**
*
* The name of the domain that you want to update contact information for.
*
*
* @param domainName
* The name of the domain that you want to update contact information for.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public UpdateDomainContactRequest withDomainName(String domainName) {
setDomainName(domainName);
return this;
}
/**
*
* Provides detailed contact information.
*
*
* @param adminContact
* Provides detailed contact information.
*/
public void setAdminContact(ContactDetail adminContact) {
this.adminContact = adminContact;
}
/**
*
* Provides detailed contact information.
*
*
* @return Provides detailed contact information.
*/
public ContactDetail getAdminContact() {
return this.adminContact;
}
/**
*
* Provides detailed contact information.
*
*
* @param adminContact
* Provides detailed contact information.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public UpdateDomainContactRequest withAdminContact(ContactDetail adminContact) {
setAdminContact(adminContact);
return this;
}
/**
*
* Provides detailed contact information.
*
*
* @param registrantContact
* Provides detailed contact information.
*/
public void setRegistrantContact(ContactDetail registrantContact) {
this.registrantContact = registrantContact;
}
/**
*
* Provides detailed contact information.
*
*
* @return Provides detailed contact information.
*/
public ContactDetail getRegistrantContact() {
return this.registrantContact;
}
/**
*
* Provides detailed contact information.
*
*
* @param registrantContact
* Provides detailed contact information.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public UpdateDomainContactRequest withRegistrantContact(ContactDetail registrantContact) {
setRegistrantContact(registrantContact);
return this;
}
/**
*
* Provides detailed contact information.
*
*
* @param techContact
* Provides detailed contact information.
*/
public void setTechContact(ContactDetail techContact) {
this.techContact = techContact;
}
/**
*
* Provides detailed contact information.
*
*
* @return Provides detailed contact information.
*/
public ContactDetail getTechContact() {
return this.techContact;
}
/**
*
* Provides detailed contact information.
*
*
* @param techContact
* Provides detailed contact information.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public UpdateDomainContactRequest withTechContact(ContactDetail techContact) {
setTechContact(techContact);
return this;
}
/**
*
* Customer's consent for the owner change request. Required if the domain is not free (consent price is more than
* $0.00).
*
*
* @param consent
* Customer's consent for the owner change request. Required if the domain is not free (consent price is more
* than $0.00).
*/
public void setConsent(Consent consent) {
this.consent = consent;
}
/**
*
* Customer's consent for the owner change request. Required if the domain is not free (consent price is more than
* $0.00).
*
*
* @return Customer's consent for the owner change request. Required if the domain is not free (consent price is
* more than $0.00).
*/
public Consent getConsent() {
return this.consent;
}
/**
*
* Customer's consent for the owner change request. Required if the domain is not free (consent price is more than
* $0.00).
*
*
* @param consent
* Customer's consent for the owner change request. Required if the domain is not free (consent price is more
* than $0.00).
* @return Returns a reference to this object so that method calls can be chained together.
*/
public UpdateDomainContactRequest withConsent(Consent consent) {
setConsent(consent);
return this;
}
/**
*
* Provides detailed contact information.
*
*
* @param billingContact
* Provides detailed contact information.
*/
public void setBillingContact(ContactDetail billingContact) {
this.billingContact = billingContact;
}
/**
*
* Provides detailed contact information.
*
*
* @return Provides detailed contact information.
*/
public ContactDetail getBillingContact() {
return this.billingContact;
}
/**
*
* Provides detailed contact information.
*
*
* @param billingContact
* Provides detailed contact information.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public UpdateDomainContactRequest withBillingContact(ContactDetail billingContact) {
setBillingContact(billingContact);
return this;
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getDomainName() != null)
sb.append("DomainName: ").append(getDomainName()).append(",");
if (getAdminContact() != null)
sb.append("AdminContact: ").append("***Sensitive Data Redacted***").append(",");
if (getRegistrantContact() != null)
sb.append("RegistrantContact: ").append("***Sensitive Data Redacted***").append(",");
if (getTechContact() != null)
sb.append("TechContact: ").append("***Sensitive Data Redacted***").append(",");
if (getConsent() != null)
sb.append("Consent: ").append(getConsent()).append(",");
if (getBillingContact() != null)
sb.append("BillingContact: ").append("***Sensitive Data Redacted***");
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof UpdateDomainContactRequest == false)
return false;
UpdateDomainContactRequest other = (UpdateDomainContactRequest) obj;
if (other.getDomainName() == null ^ this.getDomainName() == null)
return false;
if (other.getDomainName() != null && other.getDomainName().equals(this.getDomainName()) == false)
return false;
if (other.getAdminContact() == null ^ this.getAdminContact() == null)
return false;
if (other.getAdminContact() != null && other.getAdminContact().equals(this.getAdminContact()) == false)
return false;
if (other.getRegistrantContact() == null ^ this.getRegistrantContact() == null)
return false;
if (other.getRegistrantContact() != null && other.getRegistrantContact().equals(this.getRegistrantContact()) == false)
return false;
if (other.getTechContact() == null ^ this.getTechContact() == null)
return false;
if (other.getTechContact() != null && other.getTechContact().equals(this.getTechContact()) == false)
return false;
if (other.getConsent() == null ^ this.getConsent() == null)
return false;
if (other.getConsent() != null && other.getConsent().equals(this.getConsent()) == false)
return false;
if (other.getBillingContact() == null ^ this.getBillingContact() == null)
return false;
if (other.getBillingContact() != null && other.getBillingContact().equals(this.getBillingContact()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getDomainName() == null) ? 0 : getDomainName().hashCode());
hashCode = prime * hashCode + ((getAdminContact() == null) ? 0 : getAdminContact().hashCode());
hashCode = prime * hashCode + ((getRegistrantContact() == null) ? 0 : getRegistrantContact().hashCode());
hashCode = prime * hashCode + ((getTechContact() == null) ? 0 : getTechContact().hashCode());
hashCode = prime * hashCode + ((getConsent() == null) ? 0 : getConsent().hashCode());
hashCode = prime * hashCode + ((getBillingContact() == null) ? 0 : getBillingContact().hashCode());
return hashCode;
}
@Override
public UpdateDomainContactRequest clone() {
return (UpdateDomainContactRequest) super.clone();
}
}