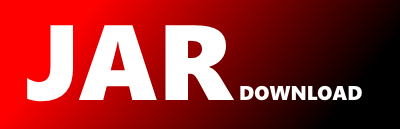
com.amazonaws.services.route53recoveryreadiness.AWSRoute53RecoveryReadiness Maven / Gradle / Ivy
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.route53recoveryreadiness;
import javax.annotation.Generated;
import com.amazonaws.*;
import com.amazonaws.regions.*;
import com.amazonaws.services.route53recoveryreadiness.model.*;
/**
* Interface for accessing AWS Route53 Recovery Readiness.
*
* Note: Do not directly implement this interface, new methods are added to it regularly. Extend from
* {@link com.amazonaws.services.route53recoveryreadiness.AbstractAWSRoute53RecoveryReadiness} instead.
*
*
*
* Recovery readiness
*
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public interface AWSRoute53RecoveryReadiness {
/**
* The region metadata service name for computing region endpoints. You can use this value to retrieve metadata
* (such as supported regions) of the service.
*
* @see RegionUtils#getRegionsForService(String)
*/
String ENDPOINT_PREFIX = "route53-recovery-readiness";
/**
*
* Creates a cell in an account.
*
*
* @param createCellRequest
* @return Result of the CreateCell operation returned by the service.
* @throws ThrottlingException
* 429 response - Limit exceeded exception or too many requests exception.
* @throws ValidationException
* 400 response - Multiple causes. For example, you might have a malformed query string, an input parameter
* might be out of range, or you used parameters together incorrectly.
* @throws InternalServerException
* 500 response - Internal service error or temporary service error. Retry the request.
* @throws ConflictException
* 409 response - Conflict exception. You might be using a predefined variable.
* @throws AccessDeniedException
* 403 response - Access denied exception. You do not have sufficient access to perform this action.
* @sample AWSRoute53RecoveryReadiness.CreateCell
* @see AWS API Documentation
*/
CreateCellResult createCell(CreateCellRequest createCellRequest);
/**
*
* Creates a cross-account readiness authorization. This lets you authorize another account to work with Route 53
* Application Recovery Controller, for example, to check the readiness status of resources in a separate account.
*
*
* @param createCrossAccountAuthorizationRequest
* @return Result of the CreateCrossAccountAuthorization operation returned by the service.
* @throws ThrottlingException
* 429 response - Limit exceeded exception or too many requests exception.
* @throws ValidationException
* 400 response - Multiple causes. For example, you might have a malformed query string, an input parameter
* might be out of range, or you used parameters together incorrectly.
* @throws InternalServerException
* 500 response - Internal service error or temporary service error. Retry the request.
* @throws ConflictException
* 409 response - Conflict exception. You might be using a predefined variable.
* @throws AccessDeniedException
* 403 response - Access denied exception. You do not have sufficient access to perform this action.
* @sample AWSRoute53RecoveryReadiness.CreateCrossAccountAuthorization
* @see AWS API Documentation
*/
CreateCrossAccountAuthorizationResult createCrossAccountAuthorization(CreateCrossAccountAuthorizationRequest createCrossAccountAuthorizationRequest);
/**
*
* Creates a readiness check in an account. A readiness check monitors a resource set in your application, such as a
* set of Amazon Aurora instances, that Application Recovery Controller is auditing recovery readiness for. The
* audits run once every minute on every resource that's associated with a readiness check.
*
*
* @param createReadinessCheckRequest
* @return Result of the CreateReadinessCheck operation returned by the service.
* @throws ThrottlingException
* 429 response - Limit exceeded exception or too many requests exception.
* @throws ValidationException
* 400 response - Multiple causes. For example, you might have a malformed query string, an input parameter
* might be out of range, or you used parameters together incorrectly.
* @throws InternalServerException
* 500 response - Internal service error or temporary service error. Retry the request.
* @throws ConflictException
* 409 response - Conflict exception. You might be using a predefined variable.
* @throws AccessDeniedException
* 403 response - Access denied exception. You do not have sufficient access to perform this action.
* @sample AWSRoute53RecoveryReadiness.CreateReadinessCheck
* @see AWS API Documentation
*/
CreateReadinessCheckResult createReadinessCheck(CreateReadinessCheckRequest createReadinessCheckRequest);
/**
*
* Creates a recovery group in an account. A recovery group corresponds to an application and includes a list of the
* cells that make up the application.
*
*
* @param createRecoveryGroupRequest
* @return Result of the CreateRecoveryGroup operation returned by the service.
* @throws ThrottlingException
* 429 response - Limit exceeded exception or too many requests exception.
* @throws ValidationException
* 400 response - Multiple causes. For example, you might have a malformed query string, an input parameter
* might be out of range, or you used parameters together incorrectly.
* @throws InternalServerException
* 500 response - Internal service error or temporary service error. Retry the request.
* @throws ConflictException
* 409 response - Conflict exception. You might be using a predefined variable.
* @throws AccessDeniedException
* 403 response - Access denied exception. You do not have sufficient access to perform this action.
* @sample AWSRoute53RecoveryReadiness.CreateRecoveryGroup
* @see AWS API Documentation
*/
CreateRecoveryGroupResult createRecoveryGroup(CreateRecoveryGroupRequest createRecoveryGroupRequest);
/**
*
* Creates a resource set. A resource set is a set of resources of one type that span multiple cells. You can
* associate a resource set with a readiness check to monitor the resources for failover readiness.
*
*
* @param createResourceSetRequest
* @return Result of the CreateResourceSet operation returned by the service.
* @throws ThrottlingException
* 429 response - Limit exceeded exception or too many requests exception.
* @throws ValidationException
* 400 response - Multiple causes. For example, you might have a malformed query string, an input parameter
* might be out of range, or you used parameters together incorrectly.
* @throws InternalServerException
* 500 response - Internal service error or temporary service error. Retry the request.
* @throws ConflictException
* 409 response - Conflict exception. You might be using a predefined variable.
* @throws AccessDeniedException
* 403 response - Access denied exception. You do not have sufficient access to perform this action.
* @sample AWSRoute53RecoveryReadiness.CreateResourceSet
* @see AWS API Documentation
*/
CreateResourceSetResult createResourceSet(CreateResourceSetRequest createResourceSetRequest);
/**
*
* Delete a cell. When successful, the response code is 204, with no response body.
*
*
* @param deleteCellRequest
* @return Result of the DeleteCell operation returned by the service.
* @throws ResourceNotFoundException
* 404 response - Malformed query string. The query string contains a syntax error or resource not found.
* @throws ThrottlingException
* 429 response - Limit exceeded exception or too many requests exception.
* @throws ValidationException
* 400 response - Multiple causes. For example, you might have a malformed query string, an input parameter
* might be out of range, or you used parameters together incorrectly.
* @throws InternalServerException
* 500 response - Internal service error or temporary service error. Retry the request.
* @throws AccessDeniedException
* 403 response - Access denied exception. You do not have sufficient access to perform this action.
* @sample AWSRoute53RecoveryReadiness.DeleteCell
* @see AWS API Documentation
*/
DeleteCellResult deleteCell(DeleteCellRequest deleteCellRequest);
/**
*
* Deletes cross account readiness authorization.
*
*
* @param deleteCrossAccountAuthorizationRequest
* @return Result of the DeleteCrossAccountAuthorization operation returned by the service.
* @throws ThrottlingException
* 429 response - Limit exceeded exception or too many requests exception.
* @throws ValidationException
* 400 response - Multiple causes. For example, you might have a malformed query string, an input parameter
* might be out of range, or you used parameters together incorrectly.
* @throws InternalServerException
* 500 response - Internal service error or temporary service error. Retry the request.
* @throws AccessDeniedException
* 403 response - Access denied exception. You do not have sufficient access to perform this action.
* @sample AWSRoute53RecoveryReadiness.DeleteCrossAccountAuthorization
* @see AWS API Documentation
*/
DeleteCrossAccountAuthorizationResult deleteCrossAccountAuthorization(DeleteCrossAccountAuthorizationRequest deleteCrossAccountAuthorizationRequest);
/**
*
* Deletes a readiness check.
*
*
* @param deleteReadinessCheckRequest
* @return Result of the DeleteReadinessCheck operation returned by the service.
* @throws ResourceNotFoundException
* 404 response - Malformed query string. The query string contains a syntax error or resource not found.
* @throws ThrottlingException
* 429 response - Limit exceeded exception or too many requests exception.
* @throws ValidationException
* 400 response - Multiple causes. For example, you might have a malformed query string, an input parameter
* might be out of range, or you used parameters together incorrectly.
* @throws InternalServerException
* 500 response - Internal service error or temporary service error. Retry the request.
* @throws AccessDeniedException
* 403 response - Access denied exception. You do not have sufficient access to perform this action.
* @sample AWSRoute53RecoveryReadiness.DeleteReadinessCheck
* @see AWS API Documentation
*/
DeleteReadinessCheckResult deleteReadinessCheck(DeleteReadinessCheckRequest deleteReadinessCheckRequest);
/**
*
* Deletes a recovery group.
*
*
* @param deleteRecoveryGroupRequest
* @return Result of the DeleteRecoveryGroup operation returned by the service.
* @throws ResourceNotFoundException
* 404 response - Malformed query string. The query string contains a syntax error or resource not found.
* @throws ThrottlingException
* 429 response - Limit exceeded exception or too many requests exception.
* @throws ValidationException
* 400 response - Multiple causes. For example, you might have a malformed query string, an input parameter
* might be out of range, or you used parameters together incorrectly.
* @throws InternalServerException
* 500 response - Internal service error or temporary service error. Retry the request.
* @throws AccessDeniedException
* 403 response - Access denied exception. You do not have sufficient access to perform this action.
* @sample AWSRoute53RecoveryReadiness.DeleteRecoveryGroup
* @see AWS API Documentation
*/
DeleteRecoveryGroupResult deleteRecoveryGroup(DeleteRecoveryGroupRequest deleteRecoveryGroupRequest);
/**
*
* Deletes a resource set.
*
*
* @param deleteResourceSetRequest
* @return Result of the DeleteResourceSet operation returned by the service.
* @throws ResourceNotFoundException
* 404 response - Malformed query string. The query string contains a syntax error or resource not found.
* @throws ThrottlingException
* 429 response - Limit exceeded exception or too many requests exception.
* @throws ValidationException
* 400 response - Multiple causes. For example, you might have a malformed query string, an input parameter
* might be out of range, or you used parameters together incorrectly.
* @throws InternalServerException
* 500 response - Internal service error or temporary service error. Retry the request.
* @throws AccessDeniedException
* 403 response - Access denied exception. You do not have sufficient access to perform this action.
* @sample AWSRoute53RecoveryReadiness.DeleteResourceSet
* @see AWS API Documentation
*/
DeleteResourceSetResult deleteResourceSet(DeleteResourceSetRequest deleteResourceSetRequest);
/**
*
* Gets recommendations about architecture designs for improving resiliency for an application, based on a recovery
* group.
*
*
* @param getArchitectureRecommendationsRequest
* @return Result of the GetArchitectureRecommendations operation returned by the service.
* @throws ResourceNotFoundException
* 404 response - Malformed query string. The query string contains a syntax error or resource not found.
* @throws ThrottlingException
* 429 response - Limit exceeded exception or too many requests exception.
* @throws ValidationException
* 400 response - Multiple causes. For example, you might have a malformed query string, an input parameter
* might be out of range, or you used parameters together incorrectly.
* @throws InternalServerException
* 500 response - Internal service error or temporary service error. Retry the request.
* @throws AccessDeniedException
* 403 response - Access denied exception. You do not have sufficient access to perform this action.
* @sample AWSRoute53RecoveryReadiness.GetArchitectureRecommendations
* @see AWS API Documentation
*/
GetArchitectureRecommendationsResult getArchitectureRecommendations(GetArchitectureRecommendationsRequest getArchitectureRecommendationsRequest);
/**
*
* Gets information about a cell including cell name, cell Amazon Resource Name (ARN), ARNs of nested cells for this
* cell, and a list of those cell ARNs with their associated recovery group ARNs.
*
*
* @param getCellRequest
* @return Result of the GetCell operation returned by the service.
* @throws ResourceNotFoundException
* 404 response - Malformed query string. The query string contains a syntax error or resource not found.
* @throws ThrottlingException
* 429 response - Limit exceeded exception or too many requests exception.
* @throws ValidationException
* 400 response - Multiple causes. For example, you might have a malformed query string, an input parameter
* might be out of range, or you used parameters together incorrectly.
* @throws InternalServerException
* 500 response - Internal service error or temporary service error. Retry the request.
* @throws AccessDeniedException
* 403 response - Access denied exception. You do not have sufficient access to perform this action.
* @sample AWSRoute53RecoveryReadiness.GetCell
* @see AWS API Documentation
*/
GetCellResult getCell(GetCellRequest getCellRequest);
/**
*
* Gets readiness for a cell. Aggregates the readiness of all the resources that are associated with the cell into a
* single value.
*
*
* @param getCellReadinessSummaryRequest
* @return Result of the GetCellReadinessSummary operation returned by the service.
* @throws ResourceNotFoundException
* 404 response - Malformed query string. The query string contains a syntax error or resource not found.
* @throws ThrottlingException
* 429 response - Limit exceeded exception or too many requests exception.
* @throws ValidationException
* 400 response - Multiple causes. For example, you might have a malformed query string, an input parameter
* might be out of range, or you used parameters together incorrectly.
* @throws InternalServerException
* 500 response - Internal service error or temporary service error. Retry the request.
* @throws AccessDeniedException
* 403 response - Access denied exception. You do not have sufficient access to perform this action.
* @sample AWSRoute53RecoveryReadiness.GetCellReadinessSummary
* @see AWS API Documentation
*/
GetCellReadinessSummaryResult getCellReadinessSummary(GetCellReadinessSummaryRequest getCellReadinessSummaryRequest);
/**
*
* Gets details about a readiness check.
*
*
* @param getReadinessCheckRequest
* @return Result of the GetReadinessCheck operation returned by the service.
* @throws ResourceNotFoundException
* 404 response - Malformed query string. The query string contains a syntax error or resource not found.
* @throws ThrottlingException
* 429 response - Limit exceeded exception or too many requests exception.
* @throws ValidationException
* 400 response - Multiple causes. For example, you might have a malformed query string, an input parameter
* might be out of range, or you used parameters together incorrectly.
* @throws InternalServerException
* 500 response - Internal service error or temporary service error. Retry the request.
* @throws AccessDeniedException
* 403 response - Access denied exception. You do not have sufficient access to perform this action.
* @sample AWSRoute53RecoveryReadiness.GetReadinessCheck
* @see AWS API Documentation
*/
GetReadinessCheckResult getReadinessCheck(GetReadinessCheckRequest getReadinessCheckRequest);
/**
*
* Gets individual readiness status for a readiness check. To see the overall readiness status for a recovery group,
* that considers the readiness status for all the readiness checks in the recovery group, use
* GetRecoveryGroupReadinessSummary.
*
*
* @param getReadinessCheckResourceStatusRequest
* @return Result of the GetReadinessCheckResourceStatus operation returned by the service.
* @throws ResourceNotFoundException
* 404 response - Malformed query string. The query string contains a syntax error or resource not found.
* @throws ThrottlingException
* 429 response - Limit exceeded exception or too many requests exception.
* @throws ValidationException
* 400 response - Multiple causes. For example, you might have a malformed query string, an input parameter
* might be out of range, or you used parameters together incorrectly.
* @throws InternalServerException
* 500 response - Internal service error or temporary service error. Retry the request.
* @throws AccessDeniedException
* 403 response - Access denied exception. You do not have sufficient access to perform this action.
* @sample AWSRoute53RecoveryReadiness.GetReadinessCheckResourceStatus
* @see AWS API Documentation
*/
GetReadinessCheckResourceStatusResult getReadinessCheckResourceStatus(GetReadinessCheckResourceStatusRequest getReadinessCheckResourceStatusRequest);
/**
*
* Gets the readiness status for an individual readiness check. To see the overall readiness status for a recovery
* group, that considers the readiness status for all the readiness checks in a recovery group, use
* GetRecoveryGroupReadinessSummary.
*
*
* @param getReadinessCheckStatusRequest
* @return Result of the GetReadinessCheckStatus operation returned by the service.
* @throws ResourceNotFoundException
* 404 response - Malformed query string. The query string contains a syntax error or resource not found.
* @throws ThrottlingException
* 429 response - Limit exceeded exception or too many requests exception.
* @throws ValidationException
* 400 response - Multiple causes. For example, you might have a malformed query string, an input parameter
* might be out of range, or you used parameters together incorrectly.
* @throws InternalServerException
* 500 response - Internal service error or temporary service error. Retry the request.
* @throws AccessDeniedException
* 403 response - Access denied exception. You do not have sufficient access to perform this action.
* @sample AWSRoute53RecoveryReadiness.GetReadinessCheckStatus
* @see AWS API Documentation
*/
GetReadinessCheckStatusResult getReadinessCheckStatus(GetReadinessCheckStatusRequest getReadinessCheckStatusRequest);
/**
*
* Gets details about a recovery group, including a list of the cells that are included in it.
*
*
* @param getRecoveryGroupRequest
* @return Result of the GetRecoveryGroup operation returned by the service.
* @throws ResourceNotFoundException
* 404 response - Malformed query string. The query string contains a syntax error or resource not found.
* @throws ThrottlingException
* 429 response - Limit exceeded exception or too many requests exception.
* @throws ValidationException
* 400 response - Multiple causes. For example, you might have a malformed query string, an input parameter
* might be out of range, or you used parameters together incorrectly.
* @throws InternalServerException
* 500 response - Internal service error or temporary service error. Retry the request.
* @throws AccessDeniedException
* 403 response - Access denied exception. You do not have sufficient access to perform this action.
* @sample AWSRoute53RecoveryReadiness.GetRecoveryGroup
* @see AWS API Documentation
*/
GetRecoveryGroupResult getRecoveryGroup(GetRecoveryGroupRequest getRecoveryGroupRequest);
/**
*
* Displays a summary of information about a recovery group's readiness status. Includes the readiness checks for
* resources in the recovery group and the readiness status of each one.
*
*
* @param getRecoveryGroupReadinessSummaryRequest
* @return Result of the GetRecoveryGroupReadinessSummary operation returned by the service.
* @throws ResourceNotFoundException
* 404 response - Malformed query string. The query string contains a syntax error or resource not found.
* @throws ThrottlingException
* 429 response - Limit exceeded exception or too many requests exception.
* @throws ValidationException
* 400 response - Multiple causes. For example, you might have a malformed query string, an input parameter
* might be out of range, or you used parameters together incorrectly.
* @throws InternalServerException
* 500 response - Internal service error or temporary service error. Retry the request.
* @throws AccessDeniedException
* 403 response - Access denied exception. You do not have sufficient access to perform this action.
* @sample AWSRoute53RecoveryReadiness.GetRecoveryGroupReadinessSummary
* @see AWS API Documentation
*/
GetRecoveryGroupReadinessSummaryResult getRecoveryGroupReadinessSummary(GetRecoveryGroupReadinessSummaryRequest getRecoveryGroupReadinessSummaryRequest);
/**
*
* Displays the details about a resource set, including a list of the resources in the set.
*
*
* @param getResourceSetRequest
* @return Result of the GetResourceSet operation returned by the service.
* @throws ResourceNotFoundException
* 404 response - Malformed query string. The query string contains a syntax error or resource not found.
* @throws ThrottlingException
* 429 response - Limit exceeded exception or too many requests exception.
* @throws ValidationException
* 400 response - Multiple causes. For example, you might have a malformed query string, an input parameter
* might be out of range, or you used parameters together incorrectly.
* @throws InternalServerException
* 500 response - Internal service error or temporary service error. Retry the request.
* @throws AccessDeniedException
* 403 response - Access denied exception. You do not have sufficient access to perform this action.
* @sample AWSRoute53RecoveryReadiness.GetResourceSet
* @see AWS API Documentation
*/
GetResourceSetResult getResourceSet(GetResourceSetRequest getResourceSetRequest);
/**
*
* Lists the cells for an account.
*
*
* @param listCellsRequest
* @return Result of the ListCells operation returned by the service.
* @throws ThrottlingException
* 429 response - Limit exceeded exception or too many requests exception.
* @throws ValidationException
* 400 response - Multiple causes. For example, you might have a malformed query string, an input parameter
* might be out of range, or you used parameters together incorrectly.
* @throws InternalServerException
* 500 response - Internal service error or temporary service error. Retry the request.
* @throws AccessDeniedException
* 403 response - Access denied exception. You do not have sufficient access to perform this action.
* @sample AWSRoute53RecoveryReadiness.ListCells
* @see AWS API Documentation
*/
ListCellsResult listCells(ListCellsRequest listCellsRequest);
/**
*
* Lists the cross-account readiness authorizations that are in place for an account.
*
*
* @param listCrossAccountAuthorizationsRequest
* @return Result of the ListCrossAccountAuthorizations operation returned by the service.
* @throws ThrottlingException
* 429 response - Limit exceeded exception or too many requests exception.
* @throws ValidationException
* 400 response - Multiple causes. For example, you might have a malformed query string, an input parameter
* might be out of range, or you used parameters together incorrectly.
* @throws InternalServerException
* 500 response - Internal service error or temporary service error. Retry the request.
* @throws AccessDeniedException
* 403 response - Access denied exception. You do not have sufficient access to perform this action.
* @sample AWSRoute53RecoveryReadiness.ListCrossAccountAuthorizations
* @see AWS API Documentation
*/
ListCrossAccountAuthorizationsResult listCrossAccountAuthorizations(ListCrossAccountAuthorizationsRequest listCrossAccountAuthorizationsRequest);
/**
*
* Lists the readiness checks for an account.
*
*
* @param listReadinessChecksRequest
* @return Result of the ListReadinessChecks operation returned by the service.
* @throws ThrottlingException
* 429 response - Limit exceeded exception or too many requests exception.
* @throws ValidationException
* 400 response - Multiple causes. For example, you might have a malformed query string, an input parameter
* might be out of range, or you used parameters together incorrectly.
* @throws InternalServerException
* 500 response - Internal service error or temporary service error. Retry the request.
* @throws AccessDeniedException
* 403 response - Access denied exception. You do not have sufficient access to perform this action.
* @sample AWSRoute53RecoveryReadiness.ListReadinessChecks
* @see AWS API Documentation
*/
ListReadinessChecksResult listReadinessChecks(ListReadinessChecksRequest listReadinessChecksRequest);
/**
*
* Lists the recovery groups in an account.
*
*
* @param listRecoveryGroupsRequest
* @return Result of the ListRecoveryGroups operation returned by the service.
* @throws ThrottlingException
* 429 response - Limit exceeded exception or too many requests exception.
* @throws ValidationException
* 400 response - Multiple causes. For example, you might have a malformed query string, an input parameter
* might be out of range, or you used parameters together incorrectly.
* @throws InternalServerException
* 500 response - Internal service error or temporary service error. Retry the request.
* @throws AccessDeniedException
* 403 response - Access denied exception. You do not have sufficient access to perform this action.
* @sample AWSRoute53RecoveryReadiness.ListRecoveryGroups
* @see AWS API Documentation
*/
ListRecoveryGroupsResult listRecoveryGroups(ListRecoveryGroupsRequest listRecoveryGroupsRequest);
/**
*
* Lists the resource sets in an account.
*
*
* @param listResourceSetsRequest
* @return Result of the ListResourceSets operation returned by the service.
* @throws ThrottlingException
* 429 response - Limit exceeded exception or too many requests exception.
* @throws ValidationException
* 400 response - Multiple causes. For example, you might have a malformed query string, an input parameter
* might be out of range, or you used parameters together incorrectly.
* @throws InternalServerException
* 500 response - Internal service error or temporary service error. Retry the request.
* @throws AccessDeniedException
* 403 response - Access denied exception. You do not have sufficient access to perform this action.
* @sample AWSRoute53RecoveryReadiness.ListResourceSets
* @see AWS API Documentation
*/
ListResourceSetsResult listResourceSets(ListResourceSetsRequest listResourceSetsRequest);
/**
*
* Lists all readiness rules, or lists the readiness rules for a specific resource type.
*
*
* @param listRulesRequest
* @return Result of the ListRules operation returned by the service.
* @throws ThrottlingException
* 429 response - Limit exceeded exception or too many requests exception.
* @throws ValidationException
* 400 response - Multiple causes. For example, you might have a malformed query string, an input parameter
* might be out of range, or you used parameters together incorrectly.
* @throws InternalServerException
* 500 response - Internal service error or temporary service error. Retry the request.
* @throws AccessDeniedException
* 403 response - Access denied exception. You do not have sufficient access to perform this action.
* @sample AWSRoute53RecoveryReadiness.ListRules
* @see AWS API Documentation
*/
ListRulesResult listRules(ListRulesRequest listRulesRequest);
/**
*
* Lists the tags for a resource.
*
*
* @param listTagsForResourcesRequest
* @return Result of the ListTagsForResources operation returned by the service.
* @throws ResourceNotFoundException
* 404 response - Malformed query string. The query string contains a syntax error or resource not found.
* @throws ValidationException
* 400 response - Multiple causes. For example, you might have a malformed query string, an input parameter
* might be out of range, or you used parameters together incorrectly.
* @throws InternalServerException
* 500 response - Internal service error or temporary service error. Retry the request.
* @sample AWSRoute53RecoveryReadiness.ListTagsForResources
* @see AWS API Documentation
*/
ListTagsForResourcesResult listTagsForResources(ListTagsForResourcesRequest listTagsForResourcesRequest);
/**
*
* Adds a tag to a resource.
*
*
* @param tagResourceRequest
* @return Result of the TagResource operation returned by the service.
* @throws ResourceNotFoundException
* 404 response - Malformed query string. The query string contains a syntax error or resource not found.
* @throws ValidationException
* 400 response - Multiple causes. For example, you might have a malformed query string, an input parameter
* might be out of range, or you used parameters together incorrectly.
* @throws InternalServerException
* 500 response - Internal service error or temporary service error. Retry the request.
* @sample AWSRoute53RecoveryReadiness.TagResource
* @see AWS API Documentation
*/
TagResourceResult tagResource(TagResourceRequest tagResourceRequest);
/**
*
* Removes a tag from a resource.
*
*
* @param untagResourceRequest
* @return Result of the UntagResource operation returned by the service.
* @throws ResourceNotFoundException
* 404 response - Malformed query string. The query string contains a syntax error or resource not found.
* @throws ValidationException
* 400 response - Multiple causes. For example, you might have a malformed query string, an input parameter
* might be out of range, or you used parameters together incorrectly.
* @throws InternalServerException
* 500 response - Internal service error or temporary service error. Retry the request.
* @sample AWSRoute53RecoveryReadiness.UntagResource
* @see AWS API Documentation
*/
UntagResourceResult untagResource(UntagResourceRequest untagResourceRequest);
/**
*
* Updates a cell to replace the list of nested cells with a new list of nested cells.
*
*
* @param updateCellRequest
* @return Result of the UpdateCell operation returned by the service.
* @throws ResourceNotFoundException
* 404 response - Malformed query string. The query string contains a syntax error or resource not found.
* @throws ThrottlingException
* 429 response - Limit exceeded exception or too many requests exception.
* @throws ValidationException
* 400 response - Multiple causes. For example, you might have a malformed query string, an input parameter
* might be out of range, or you used parameters together incorrectly.
* @throws InternalServerException
* 500 response - Internal service error or temporary service error. Retry the request.
* @throws AccessDeniedException
* 403 response - Access denied exception. You do not have sufficient access to perform this action.
* @sample AWSRoute53RecoveryReadiness.UpdateCell
* @see AWS API Documentation
*/
UpdateCellResult updateCell(UpdateCellRequest updateCellRequest);
/**
*
* Updates a readiness check.
*
*
* @param updateReadinessCheckRequest
* Name of a readiness check to describe.
* @return Result of the UpdateReadinessCheck operation returned by the service.
* @throws ResourceNotFoundException
* 404 response - Malformed query string. The query string contains a syntax error or resource not found.
* @throws ThrottlingException
* 429 response - Limit exceeded exception or too many requests exception.
* @throws ValidationException
* 400 response - Multiple causes. For example, you might have a malformed query string, an input parameter
* might be out of range, or you used parameters together incorrectly.
* @throws InternalServerException
* 500 response - Internal service error or temporary service error. Retry the request.
* @throws AccessDeniedException
* 403 response - Access denied exception. You do not have sufficient access to perform this action.
* @sample AWSRoute53RecoveryReadiness.UpdateReadinessCheck
* @see AWS API Documentation
*/
UpdateReadinessCheckResult updateReadinessCheck(UpdateReadinessCheckRequest updateReadinessCheckRequest);
/**
*
* Updates a recovery group.
*
*
* @param updateRecoveryGroupRequest
* Name of a recovery group.
* @return Result of the UpdateRecoveryGroup operation returned by the service.
* @throws ResourceNotFoundException
* 404 response - Malformed query string. The query string contains a syntax error or resource not found.
* @throws ThrottlingException
* 429 response - Limit exceeded exception or too many requests exception.
* @throws ValidationException
* 400 response - Multiple causes. For example, you might have a malformed query string, an input parameter
* might be out of range, or you used parameters together incorrectly.
* @throws InternalServerException
* 500 response - Internal service error or temporary service error. Retry the request.
* @throws AccessDeniedException
* 403 response - Access denied exception. You do not have sufficient access to perform this action.
* @sample AWSRoute53RecoveryReadiness.UpdateRecoveryGroup
* @see AWS API Documentation
*/
UpdateRecoveryGroupResult updateRecoveryGroup(UpdateRecoveryGroupRequest updateRecoveryGroupRequest);
/**
*
* Updates a resource set.
*
*
* @param updateResourceSetRequest
* Name of a resource set.
* @return Result of the UpdateResourceSet operation returned by the service.
* @throws ResourceNotFoundException
* 404 response - Malformed query string. The query string contains a syntax error or resource not found.
* @throws ThrottlingException
* 429 response - Limit exceeded exception or too many requests exception.
* @throws ValidationException
* 400 response - Multiple causes. For example, you might have a malformed query string, an input parameter
* might be out of range, or you used parameters together incorrectly.
* @throws InternalServerException
* 500 response - Internal service error or temporary service error. Retry the request.
* @throws AccessDeniedException
* 403 response - Access denied exception. You do not have sufficient access to perform this action.
* @sample AWSRoute53RecoveryReadiness.UpdateResourceSet
* @see AWS API Documentation
*/
UpdateResourceSetResult updateResourceSet(UpdateResourceSetRequest updateResourceSetRequest);
/**
* Shuts down this client object, releasing any resources that might be held open. This is an optional method, and
* callers are not expected to call it, but can if they want to explicitly release any open resources. Once a client
* has been shutdown, it should not be used to make any more requests.
*/
void shutdown();
/**
* Returns additional metadata for a previously executed successful request, typically used for debugging issues
* where a service isn't acting as expected. This data isn't considered part of the result data returned by an
* operation, so it's available through this separate, diagnostic interface.
*
* Response metadata is only cached for a limited period of time, so if you need to access this extra diagnostic
* information for an executed request, you should use this method to retrieve it as soon as possible after
* executing a request.
*
* @param request
* The originally executed request.
*
* @return The response metadata for the specified request, or null if none is available.
*/
ResponseMetadata getCachedResponseMetadata(AmazonWebServiceRequest request);
}