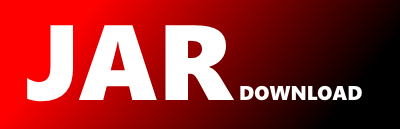
com.amazonaws.services.route53recoveryreadiness.AWSRoute53RecoveryReadinessClient Maven / Gradle / Ivy
Show all versions of aws-java-sdk-route53recoveryreadiness Show documentation
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.route53recoveryreadiness;
import org.w3c.dom.*;
import java.net.*;
import java.util.*;
import javax.annotation.Generated;
import org.apache.commons.logging.*;
import com.amazonaws.*;
import com.amazonaws.annotation.SdkInternalApi;
import com.amazonaws.auth.*;
import com.amazonaws.handlers.*;
import com.amazonaws.http.*;
import com.amazonaws.internal.*;
import com.amazonaws.internal.auth.*;
import com.amazonaws.metrics.*;
import com.amazonaws.regions.*;
import com.amazonaws.transform.*;
import com.amazonaws.util.*;
import com.amazonaws.protocol.json.*;
import com.amazonaws.util.AWSRequestMetrics.Field;
import com.amazonaws.annotation.ThreadSafe;
import com.amazonaws.client.AwsSyncClientParams;
import com.amazonaws.client.builder.AdvancedConfig;
import com.amazonaws.services.route53recoveryreadiness.AWSRoute53RecoveryReadinessClientBuilder;
import com.amazonaws.AmazonServiceException;
import com.amazonaws.services.route53recoveryreadiness.model.*;
import com.amazonaws.services.route53recoveryreadiness.model.transform.*;
/**
* Client for accessing AWS Route53 Recovery Readiness. All service calls made using this client are blocking, and will
* not return until the service call completes.
*
*
* Recovery readiness
*
*/
@ThreadSafe
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class AWSRoute53RecoveryReadinessClient extends AmazonWebServiceClient implements AWSRoute53RecoveryReadiness {
/** Provider for AWS credentials. */
private final AWSCredentialsProvider awsCredentialsProvider;
private static final Log log = LogFactory.getLog(AWSRoute53RecoveryReadiness.class);
/** Default signing name for the service. */
private static final String DEFAULT_SIGNING_NAME = "route53-recovery-readiness";
/** Client configuration factory providing ClientConfigurations tailored to this client */
protected static final ClientConfigurationFactory configFactory = new ClientConfigurationFactory();
private final AdvancedConfig advancedConfig;
private static final com.amazonaws.protocol.json.SdkJsonProtocolFactory protocolFactory = new com.amazonaws.protocol.json.SdkJsonProtocolFactory(
new JsonClientMetadata()
.withProtocolVersion("1.1")
.withSupportsCbor(false)
.withSupportsIon(false)
.withContentTypeOverride("application/json")
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("AccessDeniedException").withExceptionUnmarshaller(
com.amazonaws.services.route53recoveryreadiness.model.transform.AccessDeniedExceptionUnmarshaller.getInstance()))
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("ConflictException").withExceptionUnmarshaller(
com.amazonaws.services.route53recoveryreadiness.model.transform.ConflictExceptionUnmarshaller.getInstance()))
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("ResourceNotFoundException").withExceptionUnmarshaller(
com.amazonaws.services.route53recoveryreadiness.model.transform.ResourceNotFoundExceptionUnmarshaller.getInstance()))
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("ThrottlingException").withExceptionUnmarshaller(
com.amazonaws.services.route53recoveryreadiness.model.transform.ThrottlingExceptionUnmarshaller.getInstance()))
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("ValidationException").withExceptionUnmarshaller(
com.amazonaws.services.route53recoveryreadiness.model.transform.ValidationExceptionUnmarshaller.getInstance()))
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("InternalServerException").withExceptionUnmarshaller(
com.amazonaws.services.route53recoveryreadiness.model.transform.InternalServerExceptionUnmarshaller.getInstance()))
.withBaseServiceExceptionClass(com.amazonaws.services.route53recoveryreadiness.model.AWSRoute53RecoveryReadinessException.class));
public static AWSRoute53RecoveryReadinessClientBuilder builder() {
return AWSRoute53RecoveryReadinessClientBuilder.standard();
}
/**
* Constructs a new client to invoke service methods on AWS Route53 Recovery Readiness using the specified
* parameters.
*
*
* All service calls made using this new client object are blocking, and will not return until the service call
* completes.
*
* @param clientParams
* Object providing client parameters.
*/
AWSRoute53RecoveryReadinessClient(AwsSyncClientParams clientParams) {
this(clientParams, false);
}
/**
* Constructs a new client to invoke service methods on AWS Route53 Recovery Readiness using the specified
* parameters.
*
*
* All service calls made using this new client object are blocking, and will not return until the service call
* completes.
*
* @param clientParams
* Object providing client parameters.
*/
AWSRoute53RecoveryReadinessClient(AwsSyncClientParams clientParams, boolean endpointDiscoveryEnabled) {
super(clientParams);
this.awsCredentialsProvider = clientParams.getCredentialsProvider();
this.advancedConfig = clientParams.getAdvancedConfig();
init();
}
private void init() {
setServiceNameIntern(DEFAULT_SIGNING_NAME);
setEndpointPrefix(ENDPOINT_PREFIX);
// calling this.setEndPoint(...) will also modify the signer accordingly
setEndpoint("route53-recovery-readiness.us-east-1.amazonaws.com");
HandlerChainFactory chainFactory = new HandlerChainFactory();
requestHandler2s.addAll(chainFactory.newRequestHandlerChain("/com/amazonaws/services/route53recoveryreadiness/request.handlers"));
requestHandler2s.addAll(chainFactory.newRequestHandler2Chain("/com/amazonaws/services/route53recoveryreadiness/request.handler2s"));
requestHandler2s.addAll(chainFactory.getGlobalHandlers());
}
/**
*
* Creates a cell in an account.
*
*
* @param createCellRequest
* @return Result of the CreateCell operation returned by the service.
* @throws ThrottlingException
* 429 response - Limit exceeded exception or too many requests exception.
* @throws ValidationException
* 400 response - Multiple causes. For example, you might have a malformed query string, an input parameter
* might be out of range, or you used parameters together incorrectly.
* @throws InternalServerException
* 500 response - Internal service error or temporary service error. Retry the request.
* @throws ConflictException
* 409 response - Conflict exception. You might be using a predefined variable.
* @throws AccessDeniedException
* 403 response - Access denied exception. You do not have sufficient access to perform this action.
* @sample AWSRoute53RecoveryReadiness.CreateCell
* @see AWS API Documentation
*/
@Override
public CreateCellResult createCell(CreateCellRequest request) {
request = beforeClientExecution(request);
return executeCreateCell(request);
}
@SdkInternalApi
final CreateCellResult executeCreateCell(CreateCellRequest createCellRequest) {
ExecutionContext executionContext = createExecutionContext(createCellRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new CreateCellRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(createCellRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Route53 Recovery Readiness");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "CreateCell");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(new JsonOperationMetadata()
.withPayloadJson(true).withHasStreamingSuccessResponse(false), new CreateCellResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Creates a cross-account readiness authorization. This lets you authorize another account to work with Route 53
* Application Recovery Controller, for example, to check the readiness status of resources in a separate account.
*
*
* @param createCrossAccountAuthorizationRequest
* @return Result of the CreateCrossAccountAuthorization operation returned by the service.
* @throws ThrottlingException
* 429 response - Limit exceeded exception or too many requests exception.
* @throws ValidationException
* 400 response - Multiple causes. For example, you might have a malformed query string, an input parameter
* might be out of range, or you used parameters together incorrectly.
* @throws InternalServerException
* 500 response - Internal service error or temporary service error. Retry the request.
* @throws ConflictException
* 409 response - Conflict exception. You might be using a predefined variable.
* @throws AccessDeniedException
* 403 response - Access denied exception. You do not have sufficient access to perform this action.
* @sample AWSRoute53RecoveryReadiness.CreateCrossAccountAuthorization
* @see AWS API Documentation
*/
@Override
public CreateCrossAccountAuthorizationResult createCrossAccountAuthorization(CreateCrossAccountAuthorizationRequest request) {
request = beforeClientExecution(request);
return executeCreateCrossAccountAuthorization(request);
}
@SdkInternalApi
final CreateCrossAccountAuthorizationResult executeCreateCrossAccountAuthorization(
CreateCrossAccountAuthorizationRequest createCrossAccountAuthorizationRequest) {
ExecutionContext executionContext = createExecutionContext(createCrossAccountAuthorizationRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new CreateCrossAccountAuthorizationRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(createCrossAccountAuthorizationRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Route53 Recovery Readiness");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "CreateCrossAccountAuthorization");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new CreateCrossAccountAuthorizationResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Creates a readiness check in an account. A readiness check monitors a resource set in your application, such as a
* set of Amazon Aurora instances, that Application Recovery Controller is auditing recovery readiness for. The
* audits run once every minute on every resource that's associated with a readiness check.
*
*
* @param createReadinessCheckRequest
* @return Result of the CreateReadinessCheck operation returned by the service.
* @throws ThrottlingException
* 429 response - Limit exceeded exception or too many requests exception.
* @throws ValidationException
* 400 response - Multiple causes. For example, you might have a malformed query string, an input parameter
* might be out of range, or you used parameters together incorrectly.
* @throws InternalServerException
* 500 response - Internal service error or temporary service error. Retry the request.
* @throws ConflictException
* 409 response - Conflict exception. You might be using a predefined variable.
* @throws AccessDeniedException
* 403 response - Access denied exception. You do not have sufficient access to perform this action.
* @sample AWSRoute53RecoveryReadiness.CreateReadinessCheck
* @see AWS API Documentation
*/
@Override
public CreateReadinessCheckResult createReadinessCheck(CreateReadinessCheckRequest request) {
request = beforeClientExecution(request);
return executeCreateReadinessCheck(request);
}
@SdkInternalApi
final CreateReadinessCheckResult executeCreateReadinessCheck(CreateReadinessCheckRequest createReadinessCheckRequest) {
ExecutionContext executionContext = createExecutionContext(createReadinessCheckRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new CreateReadinessCheckRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(createReadinessCheckRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Route53 Recovery Readiness");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "CreateReadinessCheck");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new CreateReadinessCheckResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Creates a recovery group in an account. A recovery group corresponds to an application and includes a list of the
* cells that make up the application.
*
*
* @param createRecoveryGroupRequest
* @return Result of the CreateRecoveryGroup operation returned by the service.
* @throws ThrottlingException
* 429 response - Limit exceeded exception or too many requests exception.
* @throws ValidationException
* 400 response - Multiple causes. For example, you might have a malformed query string, an input parameter
* might be out of range, or you used parameters together incorrectly.
* @throws InternalServerException
* 500 response - Internal service error or temporary service error. Retry the request.
* @throws ConflictException
* 409 response - Conflict exception. You might be using a predefined variable.
* @throws AccessDeniedException
* 403 response - Access denied exception. You do not have sufficient access to perform this action.
* @sample AWSRoute53RecoveryReadiness.CreateRecoveryGroup
* @see AWS API Documentation
*/
@Override
public CreateRecoveryGroupResult createRecoveryGroup(CreateRecoveryGroupRequest request) {
request = beforeClientExecution(request);
return executeCreateRecoveryGroup(request);
}
@SdkInternalApi
final CreateRecoveryGroupResult executeCreateRecoveryGroup(CreateRecoveryGroupRequest createRecoveryGroupRequest) {
ExecutionContext executionContext = createExecutionContext(createRecoveryGroupRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new CreateRecoveryGroupRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(createRecoveryGroupRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Route53 Recovery Readiness");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "CreateRecoveryGroup");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new CreateRecoveryGroupResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Creates a resource set. A resource set is a set of resources of one type that span multiple cells. You can
* associate a resource set with a readiness check to monitor the resources for failover readiness.
*
*
* @param createResourceSetRequest
* @return Result of the CreateResourceSet operation returned by the service.
* @throws ThrottlingException
* 429 response - Limit exceeded exception or too many requests exception.
* @throws ValidationException
* 400 response - Multiple causes. For example, you might have a malformed query string, an input parameter
* might be out of range, or you used parameters together incorrectly.
* @throws InternalServerException
* 500 response - Internal service error or temporary service error. Retry the request.
* @throws ConflictException
* 409 response - Conflict exception. You might be using a predefined variable.
* @throws AccessDeniedException
* 403 response - Access denied exception. You do not have sufficient access to perform this action.
* @sample AWSRoute53RecoveryReadiness.CreateResourceSet
* @see AWS API Documentation
*/
@Override
public CreateResourceSetResult createResourceSet(CreateResourceSetRequest request) {
request = beforeClientExecution(request);
return executeCreateResourceSet(request);
}
@SdkInternalApi
final CreateResourceSetResult executeCreateResourceSet(CreateResourceSetRequest createResourceSetRequest) {
ExecutionContext executionContext = createExecutionContext(createResourceSetRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new CreateResourceSetRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(createResourceSetRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Route53 Recovery Readiness");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "CreateResourceSet");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new CreateResourceSetResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Delete a cell. When successful, the response code is 204, with no response body.
*
*
* @param deleteCellRequest
* @return Result of the DeleteCell operation returned by the service.
* @throws ResourceNotFoundException
* 404 response - Malformed query string. The query string contains a syntax error or resource not found.
* @throws ThrottlingException
* 429 response - Limit exceeded exception or too many requests exception.
* @throws ValidationException
* 400 response - Multiple causes. For example, you might have a malformed query string, an input parameter
* might be out of range, or you used parameters together incorrectly.
* @throws InternalServerException
* 500 response - Internal service error or temporary service error. Retry the request.
* @throws AccessDeniedException
* 403 response - Access denied exception. You do not have sufficient access to perform this action.
* @sample AWSRoute53RecoveryReadiness.DeleteCell
* @see AWS API Documentation
*/
@Override
public DeleteCellResult deleteCell(DeleteCellRequest request) {
request = beforeClientExecution(request);
return executeDeleteCell(request);
}
@SdkInternalApi
final DeleteCellResult executeDeleteCell(DeleteCellRequest deleteCellRequest) {
ExecutionContext executionContext = createExecutionContext(deleteCellRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DeleteCellRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(deleteCellRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Route53 Recovery Readiness");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DeleteCell");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(new JsonOperationMetadata()
.withPayloadJson(true).withHasStreamingSuccessResponse(false), new DeleteCellResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Deletes cross account readiness authorization.
*
*
* @param deleteCrossAccountAuthorizationRequest
* @return Result of the DeleteCrossAccountAuthorization operation returned by the service.
* @throws ThrottlingException
* 429 response - Limit exceeded exception or too many requests exception.
* @throws ValidationException
* 400 response - Multiple causes. For example, you might have a malformed query string, an input parameter
* might be out of range, or you used parameters together incorrectly.
* @throws InternalServerException
* 500 response - Internal service error or temporary service error. Retry the request.
* @throws AccessDeniedException
* 403 response - Access denied exception. You do not have sufficient access to perform this action.
* @sample AWSRoute53RecoveryReadiness.DeleteCrossAccountAuthorization
* @see AWS API Documentation
*/
@Override
public DeleteCrossAccountAuthorizationResult deleteCrossAccountAuthorization(DeleteCrossAccountAuthorizationRequest request) {
request = beforeClientExecution(request);
return executeDeleteCrossAccountAuthorization(request);
}
@SdkInternalApi
final DeleteCrossAccountAuthorizationResult executeDeleteCrossAccountAuthorization(
DeleteCrossAccountAuthorizationRequest deleteCrossAccountAuthorizationRequest) {
ExecutionContext executionContext = createExecutionContext(deleteCrossAccountAuthorizationRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DeleteCrossAccountAuthorizationRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(deleteCrossAccountAuthorizationRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Route53 Recovery Readiness");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DeleteCrossAccountAuthorization");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new DeleteCrossAccountAuthorizationResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Deletes a readiness check.
*
*
* @param deleteReadinessCheckRequest
* @return Result of the DeleteReadinessCheck operation returned by the service.
* @throws ResourceNotFoundException
* 404 response - Malformed query string. The query string contains a syntax error or resource not found.
* @throws ThrottlingException
* 429 response - Limit exceeded exception or too many requests exception.
* @throws ValidationException
* 400 response - Multiple causes. For example, you might have a malformed query string, an input parameter
* might be out of range, or you used parameters together incorrectly.
* @throws InternalServerException
* 500 response - Internal service error or temporary service error. Retry the request.
* @throws AccessDeniedException
* 403 response - Access denied exception. You do not have sufficient access to perform this action.
* @sample AWSRoute53RecoveryReadiness.DeleteReadinessCheck
* @see AWS API Documentation
*/
@Override
public DeleteReadinessCheckResult deleteReadinessCheck(DeleteReadinessCheckRequest request) {
request = beforeClientExecution(request);
return executeDeleteReadinessCheck(request);
}
@SdkInternalApi
final DeleteReadinessCheckResult executeDeleteReadinessCheck(DeleteReadinessCheckRequest deleteReadinessCheckRequest) {
ExecutionContext executionContext = createExecutionContext(deleteReadinessCheckRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DeleteReadinessCheckRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(deleteReadinessCheckRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Route53 Recovery Readiness");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DeleteReadinessCheck");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new DeleteReadinessCheckResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Deletes a recovery group.
*
*
* @param deleteRecoveryGroupRequest
* @return Result of the DeleteRecoveryGroup operation returned by the service.
* @throws ResourceNotFoundException
* 404 response - Malformed query string. The query string contains a syntax error or resource not found.
* @throws ThrottlingException
* 429 response - Limit exceeded exception or too many requests exception.
* @throws ValidationException
* 400 response - Multiple causes. For example, you might have a malformed query string, an input parameter
* might be out of range, or you used parameters together incorrectly.
* @throws InternalServerException
* 500 response - Internal service error or temporary service error. Retry the request.
* @throws AccessDeniedException
* 403 response - Access denied exception. You do not have sufficient access to perform this action.
* @sample AWSRoute53RecoveryReadiness.DeleteRecoveryGroup
* @see AWS API Documentation
*/
@Override
public DeleteRecoveryGroupResult deleteRecoveryGroup(DeleteRecoveryGroupRequest request) {
request = beforeClientExecution(request);
return executeDeleteRecoveryGroup(request);
}
@SdkInternalApi
final DeleteRecoveryGroupResult executeDeleteRecoveryGroup(DeleteRecoveryGroupRequest deleteRecoveryGroupRequest) {
ExecutionContext executionContext = createExecutionContext(deleteRecoveryGroupRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DeleteRecoveryGroupRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(deleteRecoveryGroupRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Route53 Recovery Readiness");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DeleteRecoveryGroup");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new DeleteRecoveryGroupResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Deletes a resource set.
*
*
* @param deleteResourceSetRequest
* @return Result of the DeleteResourceSet operation returned by the service.
* @throws ResourceNotFoundException
* 404 response - Malformed query string. The query string contains a syntax error or resource not found.
* @throws ThrottlingException
* 429 response - Limit exceeded exception or too many requests exception.
* @throws ValidationException
* 400 response - Multiple causes. For example, you might have a malformed query string, an input parameter
* might be out of range, or you used parameters together incorrectly.
* @throws InternalServerException
* 500 response - Internal service error or temporary service error. Retry the request.
* @throws AccessDeniedException
* 403 response - Access denied exception. You do not have sufficient access to perform this action.
* @sample AWSRoute53RecoveryReadiness.DeleteResourceSet
* @see AWS API Documentation
*/
@Override
public DeleteResourceSetResult deleteResourceSet(DeleteResourceSetRequest request) {
request = beforeClientExecution(request);
return executeDeleteResourceSet(request);
}
@SdkInternalApi
final DeleteResourceSetResult executeDeleteResourceSet(DeleteResourceSetRequest deleteResourceSetRequest) {
ExecutionContext executionContext = createExecutionContext(deleteResourceSetRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DeleteResourceSetRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(deleteResourceSetRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Route53 Recovery Readiness");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DeleteResourceSet");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new DeleteResourceSetResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Gets recommendations about architecture designs for improving resiliency for an application, based on a recovery
* group.
*
*
* @param getArchitectureRecommendationsRequest
* @return Result of the GetArchitectureRecommendations operation returned by the service.
* @throws ResourceNotFoundException
* 404 response - Malformed query string. The query string contains a syntax error or resource not found.
* @throws ThrottlingException
* 429 response - Limit exceeded exception or too many requests exception.
* @throws ValidationException
* 400 response - Multiple causes. For example, you might have a malformed query string, an input parameter
* might be out of range, or you used parameters together incorrectly.
* @throws InternalServerException
* 500 response - Internal service error or temporary service error. Retry the request.
* @throws AccessDeniedException
* 403 response - Access denied exception. You do not have sufficient access to perform this action.
* @sample AWSRoute53RecoveryReadiness.GetArchitectureRecommendations
* @see AWS API Documentation
*/
@Override
public GetArchitectureRecommendationsResult getArchitectureRecommendations(GetArchitectureRecommendationsRequest request) {
request = beforeClientExecution(request);
return executeGetArchitectureRecommendations(request);
}
@SdkInternalApi
final GetArchitectureRecommendationsResult executeGetArchitectureRecommendations(GetArchitectureRecommendationsRequest getArchitectureRecommendationsRequest) {
ExecutionContext executionContext = createExecutionContext(getArchitectureRecommendationsRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new GetArchitectureRecommendationsRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(getArchitectureRecommendationsRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Route53 Recovery Readiness");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "GetArchitectureRecommendations");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new GetArchitectureRecommendationsResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Gets information about a cell including cell name, cell Amazon Resource Name (ARN), ARNs of nested cells for this
* cell, and a list of those cell ARNs with their associated recovery group ARNs.
*
*
* @param getCellRequest
* @return Result of the GetCell operation returned by the service.
* @throws ResourceNotFoundException
* 404 response - Malformed query string. The query string contains a syntax error or resource not found.
* @throws ThrottlingException
* 429 response - Limit exceeded exception or too many requests exception.
* @throws ValidationException
* 400 response - Multiple causes. For example, you might have a malformed query string, an input parameter
* might be out of range, or you used parameters together incorrectly.
* @throws InternalServerException
* 500 response - Internal service error or temporary service error. Retry the request.
* @throws AccessDeniedException
* 403 response - Access denied exception. You do not have sufficient access to perform this action.
* @sample AWSRoute53RecoveryReadiness.GetCell
* @see AWS API Documentation
*/
@Override
public GetCellResult getCell(GetCellRequest request) {
request = beforeClientExecution(request);
return executeGetCell(request);
}
@SdkInternalApi
final GetCellResult executeGetCell(GetCellRequest getCellRequest) {
ExecutionContext executionContext = createExecutionContext(getCellRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new GetCellRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(getCellRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Route53 Recovery Readiness");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "GetCell");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(new JsonOperationMetadata()
.withPayloadJson(true).withHasStreamingSuccessResponse(false), new GetCellResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Gets readiness for a cell. Aggregates the readiness of all the resources that are associated with the cell into a
* single value.
*
*
* @param getCellReadinessSummaryRequest
* @return Result of the GetCellReadinessSummary operation returned by the service.
* @throws ResourceNotFoundException
* 404 response - Malformed query string. The query string contains a syntax error or resource not found.
* @throws ThrottlingException
* 429 response - Limit exceeded exception or too many requests exception.
* @throws ValidationException
* 400 response - Multiple causes. For example, you might have a malformed query string, an input parameter
* might be out of range, or you used parameters together incorrectly.
* @throws InternalServerException
* 500 response - Internal service error or temporary service error. Retry the request.
* @throws AccessDeniedException
* 403 response - Access denied exception. You do not have sufficient access to perform this action.
* @sample AWSRoute53RecoveryReadiness.GetCellReadinessSummary
* @see AWS API Documentation
*/
@Override
public GetCellReadinessSummaryResult getCellReadinessSummary(GetCellReadinessSummaryRequest request) {
request = beforeClientExecution(request);
return executeGetCellReadinessSummary(request);
}
@SdkInternalApi
final GetCellReadinessSummaryResult executeGetCellReadinessSummary(GetCellReadinessSummaryRequest getCellReadinessSummaryRequest) {
ExecutionContext executionContext = createExecutionContext(getCellReadinessSummaryRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new GetCellReadinessSummaryRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(getCellReadinessSummaryRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Route53 Recovery Readiness");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "GetCellReadinessSummary");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new GetCellReadinessSummaryResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Gets details about a readiness check.
*
*
* @param getReadinessCheckRequest
* @return Result of the GetReadinessCheck operation returned by the service.
* @throws ResourceNotFoundException
* 404 response - Malformed query string. The query string contains a syntax error or resource not found.
* @throws ThrottlingException
* 429 response - Limit exceeded exception or too many requests exception.
* @throws ValidationException
* 400 response - Multiple causes. For example, you might have a malformed query string, an input parameter
* might be out of range, or you used parameters together incorrectly.
* @throws InternalServerException
* 500 response - Internal service error or temporary service error. Retry the request.
* @throws AccessDeniedException
* 403 response - Access denied exception. You do not have sufficient access to perform this action.
* @sample AWSRoute53RecoveryReadiness.GetReadinessCheck
* @see AWS API Documentation
*/
@Override
public GetReadinessCheckResult getReadinessCheck(GetReadinessCheckRequest request) {
request = beforeClientExecution(request);
return executeGetReadinessCheck(request);
}
@SdkInternalApi
final GetReadinessCheckResult executeGetReadinessCheck(GetReadinessCheckRequest getReadinessCheckRequest) {
ExecutionContext executionContext = createExecutionContext(getReadinessCheckRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new GetReadinessCheckRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(getReadinessCheckRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Route53 Recovery Readiness");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "GetReadinessCheck");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new GetReadinessCheckResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Gets individual readiness status for a readiness check. To see the overall readiness status for a recovery group,
* that considers the readiness status for all the readiness checks in the recovery group, use
* GetRecoveryGroupReadinessSummary.
*
*
* @param getReadinessCheckResourceStatusRequest
* @return Result of the GetReadinessCheckResourceStatus operation returned by the service.
* @throws ResourceNotFoundException
* 404 response - Malformed query string. The query string contains a syntax error or resource not found.
* @throws ThrottlingException
* 429 response - Limit exceeded exception or too many requests exception.
* @throws ValidationException
* 400 response - Multiple causes. For example, you might have a malformed query string, an input parameter
* might be out of range, or you used parameters together incorrectly.
* @throws InternalServerException
* 500 response - Internal service error or temporary service error. Retry the request.
* @throws AccessDeniedException
* 403 response - Access denied exception. You do not have sufficient access to perform this action.
* @sample AWSRoute53RecoveryReadiness.GetReadinessCheckResourceStatus
* @see AWS API Documentation
*/
@Override
public GetReadinessCheckResourceStatusResult getReadinessCheckResourceStatus(GetReadinessCheckResourceStatusRequest request) {
request = beforeClientExecution(request);
return executeGetReadinessCheckResourceStatus(request);
}
@SdkInternalApi
final GetReadinessCheckResourceStatusResult executeGetReadinessCheckResourceStatus(
GetReadinessCheckResourceStatusRequest getReadinessCheckResourceStatusRequest) {
ExecutionContext executionContext = createExecutionContext(getReadinessCheckResourceStatusRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new GetReadinessCheckResourceStatusRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(getReadinessCheckResourceStatusRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Route53 Recovery Readiness");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "GetReadinessCheckResourceStatus");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new GetReadinessCheckResourceStatusResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Gets the readiness status for an individual readiness check. To see the overall readiness status for a recovery
* group, that considers the readiness status for all the readiness checks in a recovery group, use
* GetRecoveryGroupReadinessSummary.
*
*
* @param getReadinessCheckStatusRequest
* @return Result of the GetReadinessCheckStatus operation returned by the service.
* @throws ResourceNotFoundException
* 404 response - Malformed query string. The query string contains a syntax error or resource not found.
* @throws ThrottlingException
* 429 response - Limit exceeded exception or too many requests exception.
* @throws ValidationException
* 400 response - Multiple causes. For example, you might have a malformed query string, an input parameter
* might be out of range, or you used parameters together incorrectly.
* @throws InternalServerException
* 500 response - Internal service error or temporary service error. Retry the request.
* @throws AccessDeniedException
* 403 response - Access denied exception. You do not have sufficient access to perform this action.
* @sample AWSRoute53RecoveryReadiness.GetReadinessCheckStatus
* @see AWS API Documentation
*/
@Override
public GetReadinessCheckStatusResult getReadinessCheckStatus(GetReadinessCheckStatusRequest request) {
request = beforeClientExecution(request);
return executeGetReadinessCheckStatus(request);
}
@SdkInternalApi
final GetReadinessCheckStatusResult executeGetReadinessCheckStatus(GetReadinessCheckStatusRequest getReadinessCheckStatusRequest) {
ExecutionContext executionContext = createExecutionContext(getReadinessCheckStatusRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new GetReadinessCheckStatusRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(getReadinessCheckStatusRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Route53 Recovery Readiness");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "GetReadinessCheckStatus");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new GetReadinessCheckStatusResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Gets details about a recovery group, including a list of the cells that are included in it.
*
*
* @param getRecoveryGroupRequest
* @return Result of the GetRecoveryGroup operation returned by the service.
* @throws ResourceNotFoundException
* 404 response - Malformed query string. The query string contains a syntax error or resource not found.
* @throws ThrottlingException
* 429 response - Limit exceeded exception or too many requests exception.
* @throws ValidationException
* 400 response - Multiple causes. For example, you might have a malformed query string, an input parameter
* might be out of range, or you used parameters together incorrectly.
* @throws InternalServerException
* 500 response - Internal service error or temporary service error. Retry the request.
* @throws AccessDeniedException
* 403 response - Access denied exception. You do not have sufficient access to perform this action.
* @sample AWSRoute53RecoveryReadiness.GetRecoveryGroup
* @see AWS API Documentation
*/
@Override
public GetRecoveryGroupResult getRecoveryGroup(GetRecoveryGroupRequest request) {
request = beforeClientExecution(request);
return executeGetRecoveryGroup(request);
}
@SdkInternalApi
final GetRecoveryGroupResult executeGetRecoveryGroup(GetRecoveryGroupRequest getRecoveryGroupRequest) {
ExecutionContext executionContext = createExecutionContext(getRecoveryGroupRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new GetRecoveryGroupRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(getRecoveryGroupRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Route53 Recovery Readiness");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "GetRecoveryGroup");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new GetRecoveryGroupResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Displays a summary of information about a recovery group's readiness status. Includes the readiness checks for
* resources in the recovery group and the readiness status of each one.
*
*
* @param getRecoveryGroupReadinessSummaryRequest
* @return Result of the GetRecoveryGroupReadinessSummary operation returned by the service.
* @throws ResourceNotFoundException
* 404 response - Malformed query string. The query string contains a syntax error or resource not found.
* @throws ThrottlingException
* 429 response - Limit exceeded exception or too many requests exception.
* @throws ValidationException
* 400 response - Multiple causes. For example, you might have a malformed query string, an input parameter
* might be out of range, or you used parameters together incorrectly.
* @throws InternalServerException
* 500 response - Internal service error or temporary service error. Retry the request.
* @throws AccessDeniedException
* 403 response - Access denied exception. You do not have sufficient access to perform this action.
* @sample AWSRoute53RecoveryReadiness.GetRecoveryGroupReadinessSummary
* @see AWS API Documentation
*/
@Override
public GetRecoveryGroupReadinessSummaryResult getRecoveryGroupReadinessSummary(GetRecoveryGroupReadinessSummaryRequest request) {
request = beforeClientExecution(request);
return executeGetRecoveryGroupReadinessSummary(request);
}
@SdkInternalApi
final GetRecoveryGroupReadinessSummaryResult executeGetRecoveryGroupReadinessSummary(
GetRecoveryGroupReadinessSummaryRequest getRecoveryGroupReadinessSummaryRequest) {
ExecutionContext executionContext = createExecutionContext(getRecoveryGroupReadinessSummaryRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new GetRecoveryGroupReadinessSummaryRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(getRecoveryGroupReadinessSummaryRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Route53 Recovery Readiness");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "GetRecoveryGroupReadinessSummary");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new GetRecoveryGroupReadinessSummaryResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Displays the details about a resource set, including a list of the resources in the set.
*
*
* @param getResourceSetRequest
* @return Result of the GetResourceSet operation returned by the service.
* @throws ResourceNotFoundException
* 404 response - Malformed query string. The query string contains a syntax error or resource not found.
* @throws ThrottlingException
* 429 response - Limit exceeded exception or too many requests exception.
* @throws ValidationException
* 400 response - Multiple causes. For example, you might have a malformed query string, an input parameter
* might be out of range, or you used parameters together incorrectly.
* @throws InternalServerException
* 500 response - Internal service error or temporary service error. Retry the request.
* @throws AccessDeniedException
* 403 response - Access denied exception. You do not have sufficient access to perform this action.
* @sample AWSRoute53RecoveryReadiness.GetResourceSet
* @see AWS API Documentation
*/
@Override
public GetResourceSetResult getResourceSet(GetResourceSetRequest request) {
request = beforeClientExecution(request);
return executeGetResourceSet(request);
}
@SdkInternalApi
final GetResourceSetResult executeGetResourceSet(GetResourceSetRequest getResourceSetRequest) {
ExecutionContext executionContext = createExecutionContext(getResourceSetRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new GetResourceSetRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(getResourceSetRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Route53 Recovery Readiness");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "GetResourceSet");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new GetResourceSetResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Lists the cells for an account.
*
*
* @param listCellsRequest
* @return Result of the ListCells operation returned by the service.
* @throws ThrottlingException
* 429 response - Limit exceeded exception or too many requests exception.
* @throws ValidationException
* 400 response - Multiple causes. For example, you might have a malformed query string, an input parameter
* might be out of range, or you used parameters together incorrectly.
* @throws InternalServerException
* 500 response - Internal service error or temporary service error. Retry the request.
* @throws AccessDeniedException
* 403 response - Access denied exception. You do not have sufficient access to perform this action.
* @sample AWSRoute53RecoveryReadiness.ListCells
* @see AWS API Documentation
*/
@Override
public ListCellsResult listCells(ListCellsRequest request) {
request = beforeClientExecution(request);
return executeListCells(request);
}
@SdkInternalApi
final ListCellsResult executeListCells(ListCellsRequest listCellsRequest) {
ExecutionContext executionContext = createExecutionContext(listCellsRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new ListCellsRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(listCellsRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Route53 Recovery Readiness");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "ListCells");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(new JsonOperationMetadata()
.withPayloadJson(true).withHasStreamingSuccessResponse(false), new ListCellsResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Lists the cross-account readiness authorizations that are in place for an account.
*
*
* @param listCrossAccountAuthorizationsRequest
* @return Result of the ListCrossAccountAuthorizations operation returned by the service.
* @throws ThrottlingException
* 429 response - Limit exceeded exception or too many requests exception.
* @throws ValidationException
* 400 response - Multiple causes. For example, you might have a malformed query string, an input parameter
* might be out of range, or you used parameters together incorrectly.
* @throws InternalServerException
* 500 response - Internal service error or temporary service error. Retry the request.
* @throws AccessDeniedException
* 403 response - Access denied exception. You do not have sufficient access to perform this action.
* @sample AWSRoute53RecoveryReadiness.ListCrossAccountAuthorizations
* @see AWS API Documentation
*/
@Override
public ListCrossAccountAuthorizationsResult listCrossAccountAuthorizations(ListCrossAccountAuthorizationsRequest request) {
request = beforeClientExecution(request);
return executeListCrossAccountAuthorizations(request);
}
@SdkInternalApi
final ListCrossAccountAuthorizationsResult executeListCrossAccountAuthorizations(ListCrossAccountAuthorizationsRequest listCrossAccountAuthorizationsRequest) {
ExecutionContext executionContext = createExecutionContext(listCrossAccountAuthorizationsRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new ListCrossAccountAuthorizationsRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(listCrossAccountAuthorizationsRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Route53 Recovery Readiness");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "ListCrossAccountAuthorizations");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new ListCrossAccountAuthorizationsResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Lists the readiness checks for an account.
*
*
* @param listReadinessChecksRequest
* @return Result of the ListReadinessChecks operation returned by the service.
* @throws ThrottlingException
* 429 response - Limit exceeded exception or too many requests exception.
* @throws ValidationException
* 400 response - Multiple causes. For example, you might have a malformed query string, an input parameter
* might be out of range, or you used parameters together incorrectly.
* @throws InternalServerException
* 500 response - Internal service error or temporary service error. Retry the request.
* @throws AccessDeniedException
* 403 response - Access denied exception. You do not have sufficient access to perform this action.
* @sample AWSRoute53RecoveryReadiness.ListReadinessChecks
* @see AWS API Documentation
*/
@Override
public ListReadinessChecksResult listReadinessChecks(ListReadinessChecksRequest request) {
request = beforeClientExecution(request);
return executeListReadinessChecks(request);
}
@SdkInternalApi
final ListReadinessChecksResult executeListReadinessChecks(ListReadinessChecksRequest listReadinessChecksRequest) {
ExecutionContext executionContext = createExecutionContext(listReadinessChecksRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new ListReadinessChecksRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(listReadinessChecksRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Route53 Recovery Readiness");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "ListReadinessChecks");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new ListReadinessChecksResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Lists the recovery groups in an account.
*
*
* @param listRecoveryGroupsRequest
* @return Result of the ListRecoveryGroups operation returned by the service.
* @throws ThrottlingException
* 429 response - Limit exceeded exception or too many requests exception.
* @throws ValidationException
* 400 response - Multiple causes. For example, you might have a malformed query string, an input parameter
* might be out of range, or you used parameters together incorrectly.
* @throws InternalServerException
* 500 response - Internal service error or temporary service error. Retry the request.
* @throws AccessDeniedException
* 403 response - Access denied exception. You do not have sufficient access to perform this action.
* @sample AWSRoute53RecoveryReadiness.ListRecoveryGroups
* @see AWS API Documentation
*/
@Override
public ListRecoveryGroupsResult listRecoveryGroups(ListRecoveryGroupsRequest request) {
request = beforeClientExecution(request);
return executeListRecoveryGroups(request);
}
@SdkInternalApi
final ListRecoveryGroupsResult executeListRecoveryGroups(ListRecoveryGroupsRequest listRecoveryGroupsRequest) {
ExecutionContext executionContext = createExecutionContext(listRecoveryGroupsRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new ListRecoveryGroupsRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(listRecoveryGroupsRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Route53 Recovery Readiness");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "ListRecoveryGroups");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new ListRecoveryGroupsResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Lists the resource sets in an account.
*
*
* @param listResourceSetsRequest
* @return Result of the ListResourceSets operation returned by the service.
* @throws ThrottlingException
* 429 response - Limit exceeded exception or too many requests exception.
* @throws ValidationException
* 400 response - Multiple causes. For example, you might have a malformed query string, an input parameter
* might be out of range, or you used parameters together incorrectly.
* @throws InternalServerException
* 500 response - Internal service error or temporary service error. Retry the request.
* @throws AccessDeniedException
* 403 response - Access denied exception. You do not have sufficient access to perform this action.
* @sample AWSRoute53RecoveryReadiness.ListResourceSets
* @see AWS API Documentation
*/
@Override
public ListResourceSetsResult listResourceSets(ListResourceSetsRequest request) {
request = beforeClientExecution(request);
return executeListResourceSets(request);
}
@SdkInternalApi
final ListResourceSetsResult executeListResourceSets(ListResourceSetsRequest listResourceSetsRequest) {
ExecutionContext executionContext = createExecutionContext(listResourceSetsRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new ListResourceSetsRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(listResourceSetsRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Route53 Recovery Readiness");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "ListResourceSets");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new ListResourceSetsResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Lists all readiness rules, or lists the readiness rules for a specific resource type.
*
*
* @param listRulesRequest
* @return Result of the ListRules operation returned by the service.
* @throws ThrottlingException
* 429 response - Limit exceeded exception or too many requests exception.
* @throws ValidationException
* 400 response - Multiple causes. For example, you might have a malformed query string, an input parameter
* might be out of range, or you used parameters together incorrectly.
* @throws InternalServerException
* 500 response - Internal service error or temporary service error. Retry the request.
* @throws AccessDeniedException
* 403 response - Access denied exception. You do not have sufficient access to perform this action.
* @sample AWSRoute53RecoveryReadiness.ListRules
* @see AWS API Documentation
*/
@Override
public ListRulesResult listRules(ListRulesRequest request) {
request = beforeClientExecution(request);
return executeListRules(request);
}
@SdkInternalApi
final ListRulesResult executeListRules(ListRulesRequest listRulesRequest) {
ExecutionContext executionContext = createExecutionContext(listRulesRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new ListRulesRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(listRulesRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Route53 Recovery Readiness");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "ListRules");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(new JsonOperationMetadata()
.withPayloadJson(true).withHasStreamingSuccessResponse(false), new ListRulesResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Lists the tags for a resource.
*
*
* @param listTagsForResourcesRequest
* @return Result of the ListTagsForResources operation returned by the service.
* @throws ResourceNotFoundException
* 404 response - Malformed query string. The query string contains a syntax error or resource not found.
* @throws ValidationException
* 400 response - Multiple causes. For example, you might have a malformed query string, an input parameter
* might be out of range, or you used parameters together incorrectly.
* @throws InternalServerException
* 500 response - Internal service error or temporary service error. Retry the request.
* @sample AWSRoute53RecoveryReadiness.ListTagsForResources
* @see AWS API Documentation
*/
@Override
public ListTagsForResourcesResult listTagsForResources(ListTagsForResourcesRequest request) {
request = beforeClientExecution(request);
return executeListTagsForResources(request);
}
@SdkInternalApi
final ListTagsForResourcesResult executeListTagsForResources(ListTagsForResourcesRequest listTagsForResourcesRequest) {
ExecutionContext executionContext = createExecutionContext(listTagsForResourcesRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new ListTagsForResourcesRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(listTagsForResourcesRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Route53 Recovery Readiness");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "ListTagsForResources");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new ListTagsForResourcesResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Adds a tag to a resource.
*
*
* @param tagResourceRequest
* @return Result of the TagResource operation returned by the service.
* @throws ResourceNotFoundException
* 404 response - Malformed query string. The query string contains a syntax error or resource not found.
* @throws ValidationException
* 400 response - Multiple causes. For example, you might have a malformed query string, an input parameter
* might be out of range, or you used parameters together incorrectly.
* @throws InternalServerException
* 500 response - Internal service error or temporary service error. Retry the request.
* @sample AWSRoute53RecoveryReadiness.TagResource
* @see AWS API Documentation
*/
@Override
public TagResourceResult tagResource(TagResourceRequest request) {
request = beforeClientExecution(request);
return executeTagResource(request);
}
@SdkInternalApi
final TagResourceResult executeTagResource(TagResourceRequest tagResourceRequest) {
ExecutionContext executionContext = createExecutionContext(tagResourceRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new TagResourceRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(tagResourceRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Route53 Recovery Readiness");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "TagResource");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new TagResourceResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Removes a tag from a resource.
*
*
* @param untagResourceRequest
* @return Result of the UntagResource operation returned by the service.
* @throws ResourceNotFoundException
* 404 response - Malformed query string. The query string contains a syntax error or resource not found.
* @throws ValidationException
* 400 response - Multiple causes. For example, you might have a malformed query string, an input parameter
* might be out of range, or you used parameters together incorrectly.
* @throws InternalServerException
* 500 response - Internal service error or temporary service error. Retry the request.
* @sample AWSRoute53RecoveryReadiness.UntagResource
* @see AWS API Documentation
*/
@Override
public UntagResourceResult untagResource(UntagResourceRequest request) {
request = beforeClientExecution(request);
return executeUntagResource(request);
}
@SdkInternalApi
final UntagResourceResult executeUntagResource(UntagResourceRequest untagResourceRequest) {
ExecutionContext executionContext = createExecutionContext(untagResourceRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new UntagResourceRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(untagResourceRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Route53 Recovery Readiness");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "UntagResource");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new UntagResourceResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Updates a cell to replace the list of nested cells with a new list of nested cells.
*
*
* @param updateCellRequest
* @return Result of the UpdateCell operation returned by the service.
* @throws ResourceNotFoundException
* 404 response - Malformed query string. The query string contains a syntax error or resource not found.
* @throws ThrottlingException
* 429 response - Limit exceeded exception or too many requests exception.
* @throws ValidationException
* 400 response - Multiple causes. For example, you might have a malformed query string, an input parameter
* might be out of range, or you used parameters together incorrectly.
* @throws InternalServerException
* 500 response - Internal service error or temporary service error. Retry the request.
* @throws AccessDeniedException
* 403 response - Access denied exception. You do not have sufficient access to perform this action.
* @sample AWSRoute53RecoveryReadiness.UpdateCell
* @see AWS API Documentation
*/
@Override
public UpdateCellResult updateCell(UpdateCellRequest request) {
request = beforeClientExecution(request);
return executeUpdateCell(request);
}
@SdkInternalApi
final UpdateCellResult executeUpdateCell(UpdateCellRequest updateCellRequest) {
ExecutionContext executionContext = createExecutionContext(updateCellRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new UpdateCellRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(updateCellRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Route53 Recovery Readiness");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "UpdateCell");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(new JsonOperationMetadata()
.withPayloadJson(true).withHasStreamingSuccessResponse(false), new UpdateCellResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Updates a readiness check.
*
*
* @param updateReadinessCheckRequest
* Name of a readiness check to describe.
* @return Result of the UpdateReadinessCheck operation returned by the service.
* @throws ResourceNotFoundException
* 404 response - Malformed query string. The query string contains a syntax error or resource not found.
* @throws ThrottlingException
* 429 response - Limit exceeded exception or too many requests exception.
* @throws ValidationException
* 400 response - Multiple causes. For example, you might have a malformed query string, an input parameter
* might be out of range, or you used parameters together incorrectly.
* @throws InternalServerException
* 500 response - Internal service error or temporary service error. Retry the request.
* @throws AccessDeniedException
* 403 response - Access denied exception. You do not have sufficient access to perform this action.
* @sample AWSRoute53RecoveryReadiness.UpdateReadinessCheck
* @see AWS API Documentation
*/
@Override
public UpdateReadinessCheckResult updateReadinessCheck(UpdateReadinessCheckRequest request) {
request = beforeClientExecution(request);
return executeUpdateReadinessCheck(request);
}
@SdkInternalApi
final UpdateReadinessCheckResult executeUpdateReadinessCheck(UpdateReadinessCheckRequest updateReadinessCheckRequest) {
ExecutionContext executionContext = createExecutionContext(updateReadinessCheckRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new UpdateReadinessCheckRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(updateReadinessCheckRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Route53 Recovery Readiness");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "UpdateReadinessCheck");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new UpdateReadinessCheckResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Updates a recovery group.
*
*
* @param updateRecoveryGroupRequest
* Name of a recovery group.
* @return Result of the UpdateRecoveryGroup operation returned by the service.
* @throws ResourceNotFoundException
* 404 response - Malformed query string. The query string contains a syntax error or resource not found.
* @throws ThrottlingException
* 429 response - Limit exceeded exception or too many requests exception.
* @throws ValidationException
* 400 response - Multiple causes. For example, you might have a malformed query string, an input parameter
* might be out of range, or you used parameters together incorrectly.
* @throws InternalServerException
* 500 response - Internal service error or temporary service error. Retry the request.
* @throws AccessDeniedException
* 403 response - Access denied exception. You do not have sufficient access to perform this action.
* @sample AWSRoute53RecoveryReadiness.UpdateRecoveryGroup
* @see AWS API Documentation
*/
@Override
public UpdateRecoveryGroupResult updateRecoveryGroup(UpdateRecoveryGroupRequest request) {
request = beforeClientExecution(request);
return executeUpdateRecoveryGroup(request);
}
@SdkInternalApi
final UpdateRecoveryGroupResult executeUpdateRecoveryGroup(UpdateRecoveryGroupRequest updateRecoveryGroupRequest) {
ExecutionContext executionContext = createExecutionContext(updateRecoveryGroupRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new UpdateRecoveryGroupRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(updateRecoveryGroupRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Route53 Recovery Readiness");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "UpdateRecoveryGroup");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new UpdateRecoveryGroupResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Updates a resource set.
*
*
* @param updateResourceSetRequest
* Name of a resource set.
* @return Result of the UpdateResourceSet operation returned by the service.
* @throws ResourceNotFoundException
* 404 response - Malformed query string. The query string contains a syntax error or resource not found.
* @throws ThrottlingException
* 429 response - Limit exceeded exception or too many requests exception.
* @throws ValidationException
* 400 response - Multiple causes. For example, you might have a malformed query string, an input parameter
* might be out of range, or you used parameters together incorrectly.
* @throws InternalServerException
* 500 response - Internal service error or temporary service error. Retry the request.
* @throws AccessDeniedException
* 403 response - Access denied exception. You do not have sufficient access to perform this action.
* @sample AWSRoute53RecoveryReadiness.UpdateResourceSet
* @see AWS API Documentation
*/
@Override
public UpdateResourceSetResult updateResourceSet(UpdateResourceSetRequest request) {
request = beforeClientExecution(request);
return executeUpdateResourceSet(request);
}
@SdkInternalApi
final UpdateResourceSetResult executeUpdateResourceSet(UpdateResourceSetRequest updateResourceSetRequest) {
ExecutionContext executionContext = createExecutionContext(updateResourceSetRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new UpdateResourceSetRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(updateResourceSetRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Route53 Recovery Readiness");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "UpdateResourceSet");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new UpdateResourceSetResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
* Returns additional metadata for a previously executed successful, request, typically used for debugging issues
* where a service isn't acting as expected. This data isn't considered part of the result data returned by an
* operation, so it's available through this separate, diagnostic interface.
*
* Response metadata is only cached for a limited period of time, so if you need to access this extra diagnostic
* information for an executed request, you should use this method to retrieve it as soon as possible after
* executing the request.
*
* @param request
* The originally executed request
*
* @return The response metadata for the specified request, or null if none is available.
*/
public ResponseMetadata getCachedResponseMetadata(AmazonWebServiceRequest request) {
return client.getResponseMetadataForRequest(request);
}
/**
* Normal invoke with authentication. Credentials are required and may be overriden at the request level.
**/
private Response invoke(Request request, HttpResponseHandler> responseHandler,
ExecutionContext executionContext) {
return invoke(request, responseHandler, executionContext, null, null);
}
/**
* Normal invoke with authentication. Credentials are required and may be overriden at the request level.
**/
private Response invoke(Request request, HttpResponseHandler> responseHandler,
ExecutionContext executionContext, URI cachedEndpoint, URI uriFromEndpointTrait) {
executionContext.setCredentialsProvider(CredentialUtils.getCredentialsProvider(request.getOriginalRequest(), awsCredentialsProvider));
return doInvoke(request, responseHandler, executionContext, cachedEndpoint, uriFromEndpointTrait);
}
/**
* Invoke with no authentication. Credentials are not required and any credentials set on the client or request will
* be ignored for this operation.
**/
private Response anonymousInvoke(Request request,
HttpResponseHandler> responseHandler, ExecutionContext executionContext) {
return doInvoke(request, responseHandler, executionContext, null, null);
}
/**
* Invoke the request using the http client. Assumes credentials (or lack thereof) have been configured in the
* ExecutionContext beforehand.
**/
private Response doInvoke(Request request, HttpResponseHandler> responseHandler,
ExecutionContext executionContext, URI discoveredEndpoint, URI uriFromEndpointTrait) {
if (discoveredEndpoint != null) {
request.setEndpoint(discoveredEndpoint);
request.getOriginalRequest().getRequestClientOptions().appendUserAgent("endpoint-discovery");
} else if (uriFromEndpointTrait != null) {
request.setEndpoint(uriFromEndpointTrait);
} else {
request.setEndpoint(endpoint);
}
request.setTimeOffset(timeOffset);
HttpResponseHandler errorResponseHandler = protocolFactory.createErrorResponseHandler(new JsonErrorResponseMetadata());
return client.execute(request, responseHandler, errorResponseHandler, executionContext);
}
@com.amazonaws.annotation.SdkInternalApi
static com.amazonaws.protocol.json.SdkJsonProtocolFactory getProtocolFactory() {
return protocolFactory;
}
@Override
public void shutdown() {
super.shutdown();
}
}