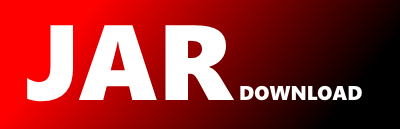
com.amazonaws.services.s3control.AWSS3ControlClient Maven / Gradle / Ivy
Show all versions of aws-java-sdk-s3control Show documentation
/*
* Copyright 2017-2022 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.s3control;
import org.w3c.dom.*;
import java.net.*;
import java.util.*;
import javax.annotation.Generated;
import org.apache.commons.logging.*;
import com.amazonaws.*;
import com.amazonaws.annotation.SdkInternalApi;
import com.amazonaws.auth.*;
import com.amazonaws.handlers.*;
import com.amazonaws.http.*;
import com.amazonaws.internal.*;
import com.amazonaws.internal.auth.*;
import com.amazonaws.metrics.*;
import com.amazonaws.regions.*;
import com.amazonaws.transform.*;
import com.amazonaws.util.*;
import com.amazonaws.protocol.json.*;
import com.amazonaws.util.AWSRequestMetrics.Field;
import com.amazonaws.annotation.ThreadSafe;
import com.amazonaws.client.AwsSyncClientParams;
import com.amazonaws.client.builder.AdvancedConfig;
import com.amazonaws.services.s3control.AWSS3ControlClientBuilder;
import com.amazonaws.AmazonServiceException;
import com.amazonaws.services.s3control.model.*;
import com.amazonaws.services.s3control.model.transform.*;
import com.amazonaws.arn.Arn;
import com.amazonaws.arn.AwsResource;
import static com.amazonaws.services.s3control.S3ControlHandlerContextKey.S3_ARNABLE_FIELD;
/**
* Client for accessing AWS S3 Control. All service calls made using this client are blocking, and will not return until
* the service call completes.
*
*
* Amazon Web Services S3 Control provides access to Amazon S3 control plane actions.
*
*/
@ThreadSafe
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class AWSS3ControlClient extends AmazonWebServiceClient implements AWSS3Control {
/** Provider for AWS credentials. */
private final AWSCredentialsProvider awsCredentialsProvider;
private static final Log log = LogFactory.getLog(AWSS3Control.class);
/** Default signing name for the service. */
private static final String DEFAULT_SIGNING_NAME = "s3";
/** Client configuration factory providing ClientConfigurations tailored to this client */
protected static final ClientConfigurationFactory configFactory = new ClientConfigurationFactory();
private final AdvancedConfig advancedConfig;
/**
* Map of exception unmarshallers for all modeled exceptions
*/
private final Map> exceptionUnmarshallersMap = new HashMap>();
/**
* List of exception unmarshallers for all modeled exceptions Even though this exceptionUnmarshallers is not used in
* Clients, this is not removed since this was directly used by Client extended classes. Using this list can cause
* performance impact.
*/
protected final List> exceptionUnmarshallers = new ArrayList>();
protected Unmarshaller defaultUnmarshaller;
public static AWSS3ControlClientBuilder builder() {
return AWSS3ControlClientBuilder.standard();
}
/**
* Constructs a new client to invoke service methods on AWS S3 Control using the specified parameters.
*
*
* All service calls made using this new client object are blocking, and will not return until the service call
* completes.
*
* @param clientParams
* Object providing client parameters.
*/
AWSS3ControlClient(AwsSyncClientParams clientParams) {
this(clientParams, false);
}
/**
* Constructs a new client to invoke service methods on AWS S3 Control using the specified parameters.
*
*
* All service calls made using this new client object are blocking, and will not return until the service call
* completes.
*
* @param clientParams
* Object providing client parameters.
*/
AWSS3ControlClient(AwsSyncClientParams clientParams, boolean endpointDiscoveryEnabled) {
super(clientParams);
this.awsCredentialsProvider = clientParams.getCredentialsProvider();
this.advancedConfig = clientParams.getAdvancedConfig();
init();
}
private void init() {
if (exceptionUnmarshallersMap.get("InvalidRequestException") == null) {
exceptionUnmarshallersMap.put("InvalidRequestException", new InvalidRequestExceptionUnmarshaller());
}
exceptionUnmarshallers.add(new InvalidRequestExceptionUnmarshaller());
if (exceptionUnmarshallersMap.get("InvalidNextTokenException") == null) {
exceptionUnmarshallersMap.put("InvalidNextTokenException", new InvalidNextTokenExceptionUnmarshaller());
}
exceptionUnmarshallers.add(new InvalidNextTokenExceptionUnmarshaller());
if (exceptionUnmarshallersMap.get("JobStatusException") == null) {
exceptionUnmarshallersMap.put("JobStatusException", new JobStatusExceptionUnmarshaller());
}
exceptionUnmarshallers.add(new JobStatusExceptionUnmarshaller());
if (exceptionUnmarshallersMap.get("NoSuchPublicAccessBlockConfiguration") == null) {
exceptionUnmarshallersMap.put("NoSuchPublicAccessBlockConfiguration", new NoSuchPublicAccessBlockConfigurationExceptionUnmarshaller());
}
exceptionUnmarshallers.add(new NoSuchPublicAccessBlockConfigurationExceptionUnmarshaller());
if (exceptionUnmarshallersMap.get("InternalServiceException") == null) {
exceptionUnmarshallersMap.put("InternalServiceException", new InternalServiceExceptionUnmarshaller());
}
exceptionUnmarshallers.add(new InternalServiceExceptionUnmarshaller());
if (exceptionUnmarshallersMap.get("BucketAlreadyExists") == null) {
exceptionUnmarshallersMap.put("BucketAlreadyExists", new BucketAlreadyExistsExceptionUnmarshaller());
}
exceptionUnmarshallers.add(new BucketAlreadyExistsExceptionUnmarshaller());
if (exceptionUnmarshallersMap.get("NotFoundException") == null) {
exceptionUnmarshallersMap.put("NotFoundException", new NotFoundExceptionUnmarshaller());
}
exceptionUnmarshallers.add(new NotFoundExceptionUnmarshaller());
if (exceptionUnmarshallersMap.get("TooManyTagsException") == null) {
exceptionUnmarshallersMap.put("TooManyTagsException", new TooManyTagsExceptionUnmarshaller());
}
exceptionUnmarshallers.add(new TooManyTagsExceptionUnmarshaller());
if (exceptionUnmarshallersMap.get("IdempotencyException") == null) {
exceptionUnmarshallersMap.put("IdempotencyException", new IdempotencyExceptionUnmarshaller());
}
exceptionUnmarshallers.add(new IdempotencyExceptionUnmarshaller());
if (exceptionUnmarshallersMap.get("BucketAlreadyOwnedByYou") == null) {
exceptionUnmarshallersMap.put("BucketAlreadyOwnedByYou", new BucketAlreadyOwnedByYouExceptionUnmarshaller());
}
exceptionUnmarshallers.add(new BucketAlreadyOwnedByYouExceptionUnmarshaller());
if (exceptionUnmarshallersMap.get("TooManyRequestsException") == null) {
exceptionUnmarshallersMap.put("TooManyRequestsException", new TooManyRequestsExceptionUnmarshaller());
}
exceptionUnmarshallers.add(new TooManyRequestsExceptionUnmarshaller());
if (exceptionUnmarshallersMap.get("BadRequestException") == null) {
exceptionUnmarshallersMap.put("BadRequestException", new BadRequestExceptionUnmarshaller());
}
exceptionUnmarshallers.add(new BadRequestExceptionUnmarshaller());
defaultUnmarshaller = new StandardErrorUnmarshaller(com.amazonaws.services.s3control.model.AWSS3ControlException.class);
exceptionUnmarshallers.add(new StandardErrorUnmarshaller(com.amazonaws.services.s3control.model.AWSS3ControlException.class));
setServiceNameIntern(DEFAULT_SIGNING_NAME);
setEndpointPrefix(ENDPOINT_PREFIX);
// calling this.setEndPoint(...) will also modify the signer accordingly
this.setEndpoint("s3-control.us-east-1.amazonaws.com");
HandlerChainFactory chainFactory = new HandlerChainFactory();
requestHandler2s.addAll(chainFactory.newRequestHandlerChain("/com/amazonaws/services/s3control/request.handlers"));
requestHandler2s.addAll(chainFactory.newRequestHandler2Chain("/com/amazonaws/services/s3control/request.handler2s"));
requestHandler2s.addAll(chainFactory.getGlobalHandlers());
}
/**
*
* Creates an access point and associates it with the specified bucket. For more information, see Managing Data Access with Amazon
* S3 Access Points in the Amazon S3 User Guide.
*
*
*
*
* S3 on Outposts only supports VPC-style access points.
*
*
* For more information, see
* Accessing Amazon S3 on Outposts using virtual private cloud (VPC) only access points in the Amazon S3 User
* Guide.
*
*
*
* All Amazon S3 on Outposts REST API requests for this action require an additional parameter of
* x-amz-outpost-id
to be passed with the request and an S3 on Outposts endpoint hostname prefix
* instead of s3-control
. For an example of the request syntax for Amazon S3 on Outposts that uses the
* S3 on Outposts endpoint hostname prefix and the x-amz-outpost-id
derived using the access point ARN,
* see the Examples section.
*
*
*
* The following actions are related to CreateAccessPoint
:
*
*
* -
*
* GetAccessPoint
*
*
* -
*
*
* -
*
* ListAccessPoints
*
*
*
*
* @param createAccessPointRequest
* @return Result of the CreateAccessPoint operation returned by the service.
* @sample AWSS3Control.CreateAccessPoint
* @see AWS
* API Documentation
*/
@Override
public CreateAccessPointResult createAccessPoint(CreateAccessPointRequest request) {
request = beforeClientExecution(request);
return executeCreateAccessPoint(request);
}
@SdkInternalApi
final CreateAccessPointResult executeCreateAccessPoint(CreateAccessPointRequest createAccessPointRequest) {
ExecutionContext executionContext = createExecutionContext(createAccessPointRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
String bucket = createAccessPointRequest.getBucket();
Arn arn = null;
if (bucket != null && bucket.startsWith("arn:")) {
arn = Arn.fromString(bucket);
AwsResource awsResource = com.amazonaws.services.s3control.internal.S3ControlArnConverter.getInstance().convertArn(arn);
if (!(awsResource instanceof com.amazonaws.services.s3control.internal.S3ControlBucketResource)) {
throw new IllegalArgumentException("Unsupported ARN type: " + awsResource.getClass()
+ ". Expected com.amazonaws.services.s3control.internal.S3ControlBucketResource.");
}
com.amazonaws.services.s3control.internal.S3ControlBucketResource resource = (com.amazonaws.services.s3control.internal.S3ControlBucketResource) awsResource;
createAccessPointRequest = createAccessPointRequest.clone();
createAccessPointRequest.setBucket(resource.getBucketName());
String accountId = createAccessPointRequest.getAccountId();
String accountIdInArn = resource.getAccountId();
if (accountId != null && !accountId.equals(accountIdInArn)) {
throw new IllegalArgumentException(String.format("%s field provided from the request (%s) is different from the one in the ARN (%s)",
"accountId", accountId, accountIdInArn));
}
createAccessPointRequest.setAccountId(accountIdInArn);
}
request = new CreateAccessPointRequestMarshaller().marshall(super.beforeMarshalling(createAccessPointRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "S3 Control");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "CreateAccessPoint");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
request.addHandlerContext(S3ControlHandlerContextKey.S3_ARNABLE_FIELD, new S3ArnableField().withArn(arn));
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
URI endpointTraitHost = null;
if (!clientConfiguration.isDisableHostPrefixInjection()) {
ValidationUtils.assertStringNotEmpty(createAccessPointRequest.getAccountId(), "AccountId");
HostnameValidator.validateHostnameCompliant(createAccessPointRequest.getAccountId(), "AccountId", "createAccessPointRequest");
String hostPrefix = "{AccountId}.";
String resolvedHostPrefix = String.format("%s.", createAccessPointRequest.getAccountId());
endpointTraitHost = UriResourcePathUtils.updateUriHost(endpoint, resolvedHostPrefix);
}
StaxResponseHandler responseHandler = new com.amazonaws.services.s3control.internal.S3ControlStaxResponseHandler(
new CreateAccessPointResultStaxUnmarshaller());
response = invoke(request, responseHandler, executionContext, null, endpointTraitHost);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Creates an Object Lambda Access Point. For more information, see Transforming objects with
* Object Lambda Access Points in the Amazon S3 User Guide.
*
*
* The following actions are related to CreateAccessPointForObjectLambda
:
*
*
* -
*
*
* -
*
*
* -
*
*
*
*
* @param createAccessPointForObjectLambdaRequest
* @return Result of the CreateAccessPointForObjectLambda operation returned by the service.
* @sample AWSS3Control.CreateAccessPointForObjectLambda
* @see AWS API Documentation
*/
@Override
public CreateAccessPointForObjectLambdaResult createAccessPointForObjectLambda(CreateAccessPointForObjectLambdaRequest request) {
request = beforeClientExecution(request);
return executeCreateAccessPointForObjectLambda(request);
}
@SdkInternalApi
final CreateAccessPointForObjectLambdaResult executeCreateAccessPointForObjectLambda(
CreateAccessPointForObjectLambdaRequest createAccessPointForObjectLambdaRequest) {
ExecutionContext executionContext = createExecutionContext(createAccessPointForObjectLambdaRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new CreateAccessPointForObjectLambdaRequestMarshaller().marshall(super.beforeMarshalling(createAccessPointForObjectLambdaRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "S3 Control");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "CreateAccessPointForObjectLambda");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
URI endpointTraitHost = null;
if (!clientConfiguration.isDisableHostPrefixInjection()) {
ValidationUtils.assertStringNotEmpty(createAccessPointForObjectLambdaRequest.getAccountId(), "AccountId");
HostnameValidator.validateHostnameCompliant(createAccessPointForObjectLambdaRequest.getAccountId(), "AccountId",
"createAccessPointForObjectLambdaRequest");
String hostPrefix = "{AccountId}.";
String resolvedHostPrefix = String.format("%s.", createAccessPointForObjectLambdaRequest.getAccountId());
endpointTraitHost = UriResourcePathUtils.updateUriHost(endpoint, resolvedHostPrefix);
}
StaxResponseHandler responseHandler = new com.amazonaws.services.s3control.internal.S3ControlStaxResponseHandler(
new CreateAccessPointForObjectLambdaResultStaxUnmarshaller());
response = invoke(request, responseHandler, executionContext, null, endpointTraitHost);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
*
* This action creates an Amazon S3 on Outposts bucket. To create an S3 bucket, see Create Bucket in the Amazon
* S3 API Reference.
*
*
*
* Creates a new Outposts bucket. By creating the bucket, you become the bucket owner. To create an Outposts bucket,
* you must have S3 on Outposts. For more information, see Using Amazon S3 on Outposts in
* Amazon S3 User Guide.
*
*
* Not every string is an acceptable bucket name. For information on bucket naming restrictions, see Working
* with Amazon S3 Buckets.
*
*
* S3 on Outposts buckets support:
*
*
* -
*
* Tags
*
*
* -
*
* LifecycleConfigurations for deleting expired objects
*
*
*
*
* For a complete list of restrictions and Amazon S3 feature limitations on S3 on Outposts, see Amazon S3
* on Outposts Restrictions and Limitations.
*
*
* For an example of the request syntax for Amazon S3 on Outposts that uses the S3 on Outposts endpoint hostname
* prefix and x-amz-outpost-id
in your API request, see the Examples section.
*
*
* The following actions are related to CreateBucket
for Amazon S3 on Outposts:
*
*
* -
*
* PutObject
*
*
* -
*
* GetBucket
*
*
* -
*
* DeleteBucket
*
*
* -
*
*
* -
*
*
*
*
* @param createBucketRequest
* @return Result of the CreateBucket operation returned by the service.
* @throws BucketAlreadyExistsException
* The requested Outposts bucket name is not available. The bucket namespace is shared by all users of the
* Outposts in this Region. Select a different name and try again.
* @throws BucketAlreadyOwnedByYouException
* The Outposts bucket you tried to create already exists, and you own it.
* @sample AWSS3Control.CreateBucket
* @see AWS API
* Documentation
*/
@Override
public CreateBucketResult createBucket(CreateBucketRequest request) {
request = beforeClientExecution(request);
return executeCreateBucket(request);
}
@SdkInternalApi
final CreateBucketResult executeCreateBucket(CreateBucketRequest createBucketRequest) {
ExecutionContext executionContext = createExecutionContext(createBucketRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new CreateBucketRequestMarshaller().marshall(super.beforeMarshalling(createBucketRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "S3 Control");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "CreateBucket");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
StaxResponseHandler responseHandler = new com.amazonaws.services.s3control.internal.S3ControlStaxResponseHandler(
new CreateBucketResultStaxUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* You can use S3 Batch Operations to perform large-scale batch actions on Amazon S3 objects. Batch Operations can
* run a single action on lists of Amazon S3 objects that you specify. For more information, see S3 Batch Operations in the
* Amazon S3 User Guide.
*
*
* This action creates a S3 Batch Operations job.
*
*
*
* Related actions include:
*
*
* -
*
* DescribeJob
*
*
* -
*
* ListJobs
*
*
* -
*
*
* -
*
* UpdateJobStatus
*
*
* -
*
* JobOperation
*
*
*
*
* @param createJobRequest
* @return Result of the CreateJob operation returned by the service.
* @throws TooManyRequestsException
* @throws BadRequestException
* @throws IdempotencyException
* @throws InternalServiceException
* @sample AWSS3Control.CreateJob
* @see AWS API
* Documentation
*/
@Override
public CreateJobResult createJob(CreateJobRequest request) {
request = beforeClientExecution(request);
return executeCreateJob(request);
}
@SdkInternalApi
final CreateJobResult executeCreateJob(CreateJobRequest createJobRequest) {
ExecutionContext executionContext = createExecutionContext(createJobRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new CreateJobRequestMarshaller().marshall(super.beforeMarshalling(createJobRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "S3 Control");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "CreateJob");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
URI endpointTraitHost = null;
if (!clientConfiguration.isDisableHostPrefixInjection()) {
ValidationUtils.assertStringNotEmpty(createJobRequest.getAccountId(), "AccountId");
HostnameValidator.validateHostnameCompliant(createJobRequest.getAccountId(), "AccountId", "createJobRequest");
String hostPrefix = "{AccountId}.";
String resolvedHostPrefix = String.format("%s.", createJobRequest.getAccountId());
endpointTraitHost = UriResourcePathUtils.updateUriHost(endpoint, resolvedHostPrefix);
}
StaxResponseHandler responseHandler = new com.amazonaws.services.s3control.internal.S3ControlStaxResponseHandler(
new CreateJobResultStaxUnmarshaller());
response = invoke(request, responseHandler, executionContext, null, endpointTraitHost);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Creates a Multi-Region Access Point and associates it with the specified buckets. For more information about
* creating Multi-Region Access Points, see Creating
* Multi-Region Access Points in the Amazon S3 User Guide.
*
*
* This action will always be routed to the US West (Oregon) Region. For more information about the restrictions
* around managing Multi-Region Access Points, see Managing
* Multi-Region Access Points in the Amazon S3 User Guide.
*
*
* This request is asynchronous, meaning that you might receive a response before the command has completed. When
* this request provides a response, it provides a token that you can use to monitor the status of the request with
* DescribeMultiRegionAccessPointOperation
.
*
*
* The following actions are related to CreateMultiRegionAccessPoint
:
*
*
* -
*
*
* -
*
*
* -
*
*
* -
*
*
*
*
* @param createMultiRegionAccessPointRequest
* @return Result of the CreateMultiRegionAccessPoint operation returned by the service.
* @sample AWSS3Control.CreateMultiRegionAccessPoint
* @see AWS API Documentation
*/
@Override
public CreateMultiRegionAccessPointResult createMultiRegionAccessPoint(CreateMultiRegionAccessPointRequest request) {
request = beforeClientExecution(request);
return executeCreateMultiRegionAccessPoint(request);
}
@SdkInternalApi
final CreateMultiRegionAccessPointResult executeCreateMultiRegionAccessPoint(CreateMultiRegionAccessPointRequest createMultiRegionAccessPointRequest) {
ExecutionContext executionContext = createExecutionContext(createMultiRegionAccessPointRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new CreateMultiRegionAccessPointRequestMarshaller().marshall(super.beforeMarshalling(createMultiRegionAccessPointRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "S3 Control");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "CreateMultiRegionAccessPoint");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
URI endpointTraitHost = null;
if (!clientConfiguration.isDisableHostPrefixInjection()) {
ValidationUtils.assertStringNotEmpty(createMultiRegionAccessPointRequest.getAccountId(), "AccountId");
HostnameValidator.validateHostnameCompliant(createMultiRegionAccessPointRequest.getAccountId(), "AccountId",
"createMultiRegionAccessPointRequest");
String hostPrefix = "{AccountId}.";
String resolvedHostPrefix = String.format("%s.", createMultiRegionAccessPointRequest.getAccountId());
endpointTraitHost = UriResourcePathUtils.updateUriHost(endpoint, resolvedHostPrefix);
}
StaxResponseHandler responseHandler = new com.amazonaws.services.s3control.internal.S3ControlStaxResponseHandler(
new CreateMultiRegionAccessPointResultStaxUnmarshaller());
response = invoke(request, responseHandler, executionContext, null, endpointTraitHost);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Deletes the specified access point.
*
*
* All Amazon S3 on Outposts REST API requests for this action require an additional parameter of
* x-amz-outpost-id
to be passed with the request and an S3 on Outposts endpoint hostname prefix
* instead of s3-control
. For an example of the request syntax for Amazon S3 on Outposts that uses the
* S3 on Outposts endpoint hostname prefix and the x-amz-outpost-id
derived using the access point ARN,
* see the Examples section.
*
*
* The following actions are related to DeleteAccessPoint
:
*
*
* -
*
*
* -
*
* GetAccessPoint
*
*
* -
*
* ListAccessPoints
*
*
*
*
* @param deleteAccessPointRequest
* @return Result of the DeleteAccessPoint operation returned by the service.
* @sample AWSS3Control.DeleteAccessPoint
* @see AWS
* API Documentation
*/
@Override
public DeleteAccessPointResult deleteAccessPoint(DeleteAccessPointRequest request) {
request = beforeClientExecution(request);
return executeDeleteAccessPoint(request);
}
@SdkInternalApi
final DeleteAccessPointResult executeDeleteAccessPoint(DeleteAccessPointRequest deleteAccessPointRequest) {
ExecutionContext executionContext = createExecutionContext(deleteAccessPointRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
String name = deleteAccessPointRequest.getName();
Arn arn = null;
if (name != null && name.startsWith("arn:")) {
arn = Arn.fromString(name);
AwsResource awsResource = com.amazonaws.services.s3control.internal.S3ControlArnConverter.getInstance().convertArn(arn);
if (!(awsResource instanceof com.amazonaws.services.s3.S3AccessPointResource)) {
throw new IllegalArgumentException("Unsupported ARN type: " + awsResource.getClass()
+ ". Expected com.amazonaws.services.s3.S3AccessPointResource.");
}
com.amazonaws.services.s3.S3AccessPointResource resource = (com.amazonaws.services.s3.S3AccessPointResource) awsResource;
deleteAccessPointRequest = deleteAccessPointRequest.clone();
deleteAccessPointRequest.setName(resource.getAccessPointName());
String accountId = deleteAccessPointRequest.getAccountId();
String accountIdInArn = resource.getAccountId();
if (accountId != null && !accountId.equals(accountIdInArn)) {
throw new IllegalArgumentException(String.format("%s field provided from the request (%s) is different from the one in the ARN (%s)",
"accountId", accountId, accountIdInArn));
}
deleteAccessPointRequest.setAccountId(accountIdInArn);
}
request = new DeleteAccessPointRequestMarshaller().marshall(super.beforeMarshalling(deleteAccessPointRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "S3 Control");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DeleteAccessPoint");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
request.addHandlerContext(S3ControlHandlerContextKey.S3_ARNABLE_FIELD, new S3ArnableField().withArn(arn));
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
URI endpointTraitHost = null;
if (!clientConfiguration.isDisableHostPrefixInjection()) {
ValidationUtils.assertStringNotEmpty(deleteAccessPointRequest.getAccountId(), "AccountId");
HostnameValidator.validateHostnameCompliant(deleteAccessPointRequest.getAccountId(), "AccountId", "deleteAccessPointRequest");
String hostPrefix = "{AccountId}.";
String resolvedHostPrefix = String.format("%s.", deleteAccessPointRequest.getAccountId());
endpointTraitHost = UriResourcePathUtils.updateUriHost(endpoint, resolvedHostPrefix);
}
StaxResponseHandler responseHandler = new com.amazonaws.services.s3control.internal.S3ControlStaxResponseHandler(
new DeleteAccessPointResultStaxUnmarshaller());
response = invoke(request, responseHandler, executionContext, null, endpointTraitHost);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Deletes the specified Object Lambda Access Point.
*
*
* The following actions are related to DeleteAccessPointForObjectLambda
:
*
*
* -
*
*
* -
*
*
* -
*
*
*
*
* @param deleteAccessPointForObjectLambdaRequest
* @return Result of the DeleteAccessPointForObjectLambda operation returned by the service.
* @sample AWSS3Control.DeleteAccessPointForObjectLambda
* @see AWS API Documentation
*/
@Override
public DeleteAccessPointForObjectLambdaResult deleteAccessPointForObjectLambda(DeleteAccessPointForObjectLambdaRequest request) {
request = beforeClientExecution(request);
return executeDeleteAccessPointForObjectLambda(request);
}
@SdkInternalApi
final DeleteAccessPointForObjectLambdaResult executeDeleteAccessPointForObjectLambda(
DeleteAccessPointForObjectLambdaRequest deleteAccessPointForObjectLambdaRequest) {
ExecutionContext executionContext = createExecutionContext(deleteAccessPointForObjectLambdaRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DeleteAccessPointForObjectLambdaRequestMarshaller().marshall(super.beforeMarshalling(deleteAccessPointForObjectLambdaRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "S3 Control");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DeleteAccessPointForObjectLambda");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
URI endpointTraitHost = null;
if (!clientConfiguration.isDisableHostPrefixInjection()) {
ValidationUtils.assertStringNotEmpty(deleteAccessPointForObjectLambdaRequest.getAccountId(), "AccountId");
HostnameValidator.validateHostnameCompliant(deleteAccessPointForObjectLambdaRequest.getAccountId(), "AccountId",
"deleteAccessPointForObjectLambdaRequest");
String hostPrefix = "{AccountId}.";
String resolvedHostPrefix = String.format("%s.", deleteAccessPointForObjectLambdaRequest.getAccountId());
endpointTraitHost = UriResourcePathUtils.updateUriHost(endpoint, resolvedHostPrefix);
}
StaxResponseHandler responseHandler = new com.amazonaws.services.s3control.internal.S3ControlStaxResponseHandler(
new DeleteAccessPointForObjectLambdaResultStaxUnmarshaller());
response = invoke(request, responseHandler, executionContext, null, endpointTraitHost);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Deletes the access point policy for the specified access point.
*
*
*
* All Amazon S3 on Outposts REST API requests for this action require an additional parameter of
* x-amz-outpost-id
to be passed with the request and an S3 on Outposts endpoint hostname prefix
* instead of s3-control
. For an example of the request syntax for Amazon S3 on Outposts that uses the
* S3 on Outposts endpoint hostname prefix and the x-amz-outpost-id
derived using the access point ARN,
* see the Examples section.
*
*
* The following actions are related to DeleteAccessPointPolicy
:
*
*
* -
*
*
* -
*
*
*
*
* @param deleteAccessPointPolicyRequest
* @return Result of the DeleteAccessPointPolicy operation returned by the service.
* @sample AWSS3Control.DeleteAccessPointPolicy
* @see AWS API Documentation
*/
@Override
public DeleteAccessPointPolicyResult deleteAccessPointPolicy(DeleteAccessPointPolicyRequest request) {
request = beforeClientExecution(request);
return executeDeleteAccessPointPolicy(request);
}
@SdkInternalApi
final DeleteAccessPointPolicyResult executeDeleteAccessPointPolicy(DeleteAccessPointPolicyRequest deleteAccessPointPolicyRequest) {
ExecutionContext executionContext = createExecutionContext(deleteAccessPointPolicyRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
String name = deleteAccessPointPolicyRequest.getName();
Arn arn = null;
if (name != null && name.startsWith("arn:")) {
arn = Arn.fromString(name);
AwsResource awsResource = com.amazonaws.services.s3control.internal.S3ControlArnConverter.getInstance().convertArn(arn);
if (!(awsResource instanceof com.amazonaws.services.s3.S3AccessPointResource)) {
throw new IllegalArgumentException("Unsupported ARN type: " + awsResource.getClass()
+ ". Expected com.amazonaws.services.s3.S3AccessPointResource.");
}
com.amazonaws.services.s3.S3AccessPointResource resource = (com.amazonaws.services.s3.S3AccessPointResource) awsResource;
deleteAccessPointPolicyRequest = deleteAccessPointPolicyRequest.clone();
deleteAccessPointPolicyRequest.setName(resource.getAccessPointName());
String accountId = deleteAccessPointPolicyRequest.getAccountId();
String accountIdInArn = resource.getAccountId();
if (accountId != null && !accountId.equals(accountIdInArn)) {
throw new IllegalArgumentException(String.format("%s field provided from the request (%s) is different from the one in the ARN (%s)",
"accountId", accountId, accountIdInArn));
}
deleteAccessPointPolicyRequest.setAccountId(accountIdInArn);
}
request = new DeleteAccessPointPolicyRequestMarshaller().marshall(super.beforeMarshalling(deleteAccessPointPolicyRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "S3 Control");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DeleteAccessPointPolicy");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
request.addHandlerContext(S3ControlHandlerContextKey.S3_ARNABLE_FIELD, new S3ArnableField().withArn(arn));
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
URI endpointTraitHost = null;
if (!clientConfiguration.isDisableHostPrefixInjection()) {
ValidationUtils.assertStringNotEmpty(deleteAccessPointPolicyRequest.getAccountId(), "AccountId");
HostnameValidator.validateHostnameCompliant(deleteAccessPointPolicyRequest.getAccountId(), "AccountId", "deleteAccessPointPolicyRequest");
String hostPrefix = "{AccountId}.";
String resolvedHostPrefix = String.format("%s.", deleteAccessPointPolicyRequest.getAccountId());
endpointTraitHost = UriResourcePathUtils.updateUriHost(endpoint, resolvedHostPrefix);
}
StaxResponseHandler responseHandler = new com.amazonaws.services.s3control.internal.S3ControlStaxResponseHandler(
new DeleteAccessPointPolicyResultStaxUnmarshaller());
response = invoke(request, responseHandler, executionContext, null, endpointTraitHost);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Removes the resource policy for an Object Lambda Access Point.
*
*
* The following actions are related to DeleteAccessPointPolicyForObjectLambda
:
*
*
* -
*
*
* -
*
*
*
*
* @param deleteAccessPointPolicyForObjectLambdaRequest
* @return Result of the DeleteAccessPointPolicyForObjectLambda operation returned by the service.
* @sample AWSS3Control.DeleteAccessPointPolicyForObjectLambda
* @see AWS API Documentation
*/
@Override
public DeleteAccessPointPolicyForObjectLambdaResult deleteAccessPointPolicyForObjectLambda(DeleteAccessPointPolicyForObjectLambdaRequest request) {
request = beforeClientExecution(request);
return executeDeleteAccessPointPolicyForObjectLambda(request);
}
@SdkInternalApi
final DeleteAccessPointPolicyForObjectLambdaResult executeDeleteAccessPointPolicyForObjectLambda(
DeleteAccessPointPolicyForObjectLambdaRequest deleteAccessPointPolicyForObjectLambdaRequest) {
ExecutionContext executionContext = createExecutionContext(deleteAccessPointPolicyForObjectLambdaRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DeleteAccessPointPolicyForObjectLambdaRequestMarshaller().marshall(super
.beforeMarshalling(deleteAccessPointPolicyForObjectLambdaRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "S3 Control");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DeleteAccessPointPolicyForObjectLambda");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
URI endpointTraitHost = null;
if (!clientConfiguration.isDisableHostPrefixInjection()) {
ValidationUtils.assertStringNotEmpty(deleteAccessPointPolicyForObjectLambdaRequest.getAccountId(), "AccountId");
HostnameValidator.validateHostnameCompliant(deleteAccessPointPolicyForObjectLambdaRequest.getAccountId(), "AccountId",
"deleteAccessPointPolicyForObjectLambdaRequest");
String hostPrefix = "{AccountId}.";
String resolvedHostPrefix = String.format("%s.", deleteAccessPointPolicyForObjectLambdaRequest.getAccountId());
endpointTraitHost = UriResourcePathUtils.updateUriHost(endpoint, resolvedHostPrefix);
}
StaxResponseHandler responseHandler = new com.amazonaws.services.s3control.internal.S3ControlStaxResponseHandler(
new DeleteAccessPointPolicyForObjectLambdaResultStaxUnmarshaller());
response = invoke(request, responseHandler, executionContext, null, endpointTraitHost);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
*
* This action deletes an Amazon S3 on Outposts bucket. To delete an S3 bucket, see DeleteBucket in the Amazon S3
* API Reference.
*
*
*
* Deletes the Amazon S3 on Outposts bucket. All objects (including all object versions and delete markers) in the
* bucket must be deleted before the bucket itself can be deleted. For more information, see Using Amazon S3 on Outposts in
* Amazon S3 User Guide.
*
*
* All Amazon S3 on Outposts REST API requests for this action require an additional parameter of
* x-amz-outpost-id
to be passed with the request and an S3 on Outposts endpoint hostname prefix
* instead of s3-control
. For an example of the request syntax for Amazon S3 on Outposts that uses the
* S3 on Outposts endpoint hostname prefix and the x-amz-outpost-id
derived using the access point ARN,
* see the Examples section.
*
*
* Related Resources
*
*
* -
*
* CreateBucket
*
*
* -
*
* GetBucket
*
*
* -
*
* DeleteObject
*
*
*
*
* @param deleteBucketRequest
* @return Result of the DeleteBucket operation returned by the service.
* @sample AWSS3Control.DeleteBucket
* @see AWS API
* Documentation
*/
@Override
public DeleteBucketResult deleteBucket(DeleteBucketRequest request) {
request = beforeClientExecution(request);
return executeDeleteBucket(request);
}
@SdkInternalApi
final DeleteBucketResult executeDeleteBucket(DeleteBucketRequest deleteBucketRequest) {
ExecutionContext executionContext = createExecutionContext(deleteBucketRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
String bucket = deleteBucketRequest.getBucket();
Arn arn = null;
if (bucket != null && bucket.startsWith("arn:")) {
arn = Arn.fromString(bucket);
AwsResource awsResource = com.amazonaws.services.s3control.internal.S3ControlArnConverter.getInstance().convertArn(arn);
if (!(awsResource instanceof com.amazonaws.services.s3control.internal.S3ControlBucketResource)) {
throw new IllegalArgumentException("Unsupported ARN type: " + awsResource.getClass()
+ ". Expected com.amazonaws.services.s3control.internal.S3ControlBucketResource.");
}
com.amazonaws.services.s3control.internal.S3ControlBucketResource resource = (com.amazonaws.services.s3control.internal.S3ControlBucketResource) awsResource;
deleteBucketRequest = deleteBucketRequest.clone();
deleteBucketRequest.setBucket(resource.getBucketName());
String accountId = deleteBucketRequest.getAccountId();
String accountIdInArn = resource.getAccountId();
if (accountId != null && !accountId.equals(accountIdInArn)) {
throw new IllegalArgumentException(String.format("%s field provided from the request (%s) is different from the one in the ARN (%s)",
"accountId", accountId, accountIdInArn));
}
deleteBucketRequest.setAccountId(accountIdInArn);
}
request = new DeleteBucketRequestMarshaller().marshall(super.beforeMarshalling(deleteBucketRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "S3 Control");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DeleteBucket");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
request.addHandlerContext(S3ControlHandlerContextKey.S3_ARNABLE_FIELD, new S3ArnableField().withArn(arn));
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
URI endpointTraitHost = null;
if (!clientConfiguration.isDisableHostPrefixInjection()) {
ValidationUtils.assertStringNotEmpty(deleteBucketRequest.getAccountId(), "AccountId");
HostnameValidator.validateHostnameCompliant(deleteBucketRequest.getAccountId(), "AccountId", "deleteBucketRequest");
String hostPrefix = "{AccountId}.";
String resolvedHostPrefix = String.format("%s.", deleteBucketRequest.getAccountId());
endpointTraitHost = UriResourcePathUtils.updateUriHost(endpoint, resolvedHostPrefix);
}
StaxResponseHandler responseHandler = new com.amazonaws.services.s3control.internal.S3ControlStaxResponseHandler(
new DeleteBucketResultStaxUnmarshaller());
response = invoke(request, responseHandler, executionContext, null, endpointTraitHost);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
*
* This action deletes an Amazon S3 on Outposts bucket's lifecycle configuration. To delete an S3 bucket's lifecycle
* configuration, see DeleteBucketLifecycle
* in the Amazon S3 API Reference.
*
*
*
* Deletes the lifecycle configuration from the specified Outposts bucket. Amazon S3 on Outposts removes all the
* lifecycle configuration rules in the lifecycle subresource associated with the bucket. Your objects never expire,
* and Amazon S3 on Outposts no longer automatically deletes any objects on the basis of rules contained in the
* deleted lifecycle configuration. For more information, see Using Amazon S3 on Outposts in
* Amazon S3 User Guide.
*
*
* To use this action, you must have permission to perform the s3-outposts:DeleteLifecycleConfiguration
* action. By default, the bucket owner has this permission and the Outposts bucket owner can grant this permission
* to others.
*
*
* All Amazon S3 on Outposts REST API requests for this action require an additional parameter of
* x-amz-outpost-id
to be passed with the request and an S3 on Outposts endpoint hostname prefix
* instead of s3-control
. For an example of the request syntax for Amazon S3 on Outposts that uses the
* S3 on Outposts endpoint hostname prefix and the x-amz-outpost-id
derived using the access point ARN,
* see the Examples section.
*
*
* For more information about object expiration, see Elements to Describe Lifecycle Actions.
*
*
* Related actions include:
*
*
* -
*
*
* -
*
*
*
*
* @param deleteBucketLifecycleConfigurationRequest
* @return Result of the DeleteBucketLifecycleConfiguration operation returned by the service.
* @sample AWSS3Control.DeleteBucketLifecycleConfiguration
* @see AWS API Documentation
*/
@Override
public DeleteBucketLifecycleConfigurationResult deleteBucketLifecycleConfiguration(DeleteBucketLifecycleConfigurationRequest request) {
request = beforeClientExecution(request);
return executeDeleteBucketLifecycleConfiguration(request);
}
@SdkInternalApi
final DeleteBucketLifecycleConfigurationResult executeDeleteBucketLifecycleConfiguration(
DeleteBucketLifecycleConfigurationRequest deleteBucketLifecycleConfigurationRequest) {
ExecutionContext executionContext = createExecutionContext(deleteBucketLifecycleConfigurationRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
String bucket = deleteBucketLifecycleConfigurationRequest.getBucket();
Arn arn = null;
if (bucket != null && bucket.startsWith("arn:")) {
arn = Arn.fromString(bucket);
AwsResource awsResource = com.amazonaws.services.s3control.internal.S3ControlArnConverter.getInstance().convertArn(arn);
if (!(awsResource instanceof com.amazonaws.services.s3control.internal.S3ControlBucketResource)) {
throw new IllegalArgumentException("Unsupported ARN type: " + awsResource.getClass()
+ ". Expected com.amazonaws.services.s3control.internal.S3ControlBucketResource.");
}
com.amazonaws.services.s3control.internal.S3ControlBucketResource resource = (com.amazonaws.services.s3control.internal.S3ControlBucketResource) awsResource;
deleteBucketLifecycleConfigurationRequest = deleteBucketLifecycleConfigurationRequest.clone();
deleteBucketLifecycleConfigurationRequest.setBucket(resource.getBucketName());
String accountId = deleteBucketLifecycleConfigurationRequest.getAccountId();
String accountIdInArn = resource.getAccountId();
if (accountId != null && !accountId.equals(accountIdInArn)) {
throw new IllegalArgumentException(String.format("%s field provided from the request (%s) is different from the one in the ARN (%s)",
"accountId", accountId, accountIdInArn));
}
deleteBucketLifecycleConfigurationRequest.setAccountId(accountIdInArn);
}
request = new DeleteBucketLifecycleConfigurationRequestMarshaller()
.marshall(super.beforeMarshalling(deleteBucketLifecycleConfigurationRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "S3 Control");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DeleteBucketLifecycleConfiguration");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
request.addHandlerContext(S3ControlHandlerContextKey.S3_ARNABLE_FIELD, new S3ArnableField().withArn(arn));
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
URI endpointTraitHost = null;
if (!clientConfiguration.isDisableHostPrefixInjection()) {
ValidationUtils.assertStringNotEmpty(deleteBucketLifecycleConfigurationRequest.getAccountId(), "AccountId");
HostnameValidator.validateHostnameCompliant(deleteBucketLifecycleConfigurationRequest.getAccountId(), "AccountId",
"deleteBucketLifecycleConfigurationRequest");
String hostPrefix = "{AccountId}.";
String resolvedHostPrefix = String.format("%s.", deleteBucketLifecycleConfigurationRequest.getAccountId());
endpointTraitHost = UriResourcePathUtils.updateUriHost(endpoint, resolvedHostPrefix);
}
StaxResponseHandler responseHandler = new com.amazonaws.services.s3control.internal.S3ControlStaxResponseHandler(
new DeleteBucketLifecycleConfigurationResultStaxUnmarshaller());
response = invoke(request, responseHandler, executionContext, null, endpointTraitHost);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
*
* This action deletes an Amazon S3 on Outposts bucket policy. To delete an S3 bucket policy, see DeleteBucketPolicy in the
* Amazon S3 API Reference.
*
*
*
* This implementation of the DELETE action uses the policy subresource to delete the policy of a specified Amazon
* S3 on Outposts bucket. If you are using an identity other than the root user of the Amazon Web Services account
* that owns the bucket, the calling identity must have the s3-outposts:DeleteBucketPolicy
permissions
* on the specified Outposts bucket and belong to the bucket owner's account to use this action. For more
* information, see Using Amazon
* S3 on Outposts in Amazon S3 User Guide.
*
*
* If you don't have DeleteBucketPolicy
permissions, Amazon S3 returns a 403 Access Denied
* error. If you have the correct permissions, but you're not using an identity that belongs to the bucket owner's
* account, Amazon S3 returns a 405 Method Not Allowed
error.
*
*
*
* As a security precaution, the root user of the Amazon Web Services account that owns a bucket can always use this
* action, even if the policy explicitly denies the root user the ability to perform this action.
*
*
*
* For more information about bucket policies, see Using Bucket Policies and User
* Policies.
*
*
* All Amazon S3 on Outposts REST API requests for this action require an additional parameter of
* x-amz-outpost-id
to be passed with the request and an S3 on Outposts endpoint hostname prefix
* instead of s3-control
. For an example of the request syntax for Amazon S3 on Outposts that uses the
* S3 on Outposts endpoint hostname prefix and the x-amz-outpost-id
derived using the access point ARN,
* see the Examples section.
*
*
* The following actions are related to DeleteBucketPolicy
:
*
*
* -
*
* GetBucketPolicy
*
*
* -
*
* PutBucketPolicy
*
*
*
*
* @param deleteBucketPolicyRequest
* @return Result of the DeleteBucketPolicy operation returned by the service.
* @sample AWSS3Control.DeleteBucketPolicy
* @see AWS
* API Documentation
*/
@Override
public DeleteBucketPolicyResult deleteBucketPolicy(DeleteBucketPolicyRequest request) {
request = beforeClientExecution(request);
return executeDeleteBucketPolicy(request);
}
@SdkInternalApi
final DeleteBucketPolicyResult executeDeleteBucketPolicy(DeleteBucketPolicyRequest deleteBucketPolicyRequest) {
ExecutionContext executionContext = createExecutionContext(deleteBucketPolicyRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
String bucket = deleteBucketPolicyRequest.getBucket();
Arn arn = null;
if (bucket != null && bucket.startsWith("arn:")) {
arn = Arn.fromString(bucket);
AwsResource awsResource = com.amazonaws.services.s3control.internal.S3ControlArnConverter.getInstance().convertArn(arn);
if (!(awsResource instanceof com.amazonaws.services.s3control.internal.S3ControlBucketResource)) {
throw new IllegalArgumentException("Unsupported ARN type: " + awsResource.getClass()
+ ". Expected com.amazonaws.services.s3control.internal.S3ControlBucketResource.");
}
com.amazonaws.services.s3control.internal.S3ControlBucketResource resource = (com.amazonaws.services.s3control.internal.S3ControlBucketResource) awsResource;
deleteBucketPolicyRequest = deleteBucketPolicyRequest.clone();
deleteBucketPolicyRequest.setBucket(resource.getBucketName());
String accountId = deleteBucketPolicyRequest.getAccountId();
String accountIdInArn = resource.getAccountId();
if (accountId != null && !accountId.equals(accountIdInArn)) {
throw new IllegalArgumentException(String.format("%s field provided from the request (%s) is different from the one in the ARN (%s)",
"accountId", accountId, accountIdInArn));
}
deleteBucketPolicyRequest.setAccountId(accountIdInArn);
}
request = new DeleteBucketPolicyRequestMarshaller().marshall(super.beforeMarshalling(deleteBucketPolicyRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "S3 Control");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DeleteBucketPolicy");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
request.addHandlerContext(S3ControlHandlerContextKey.S3_ARNABLE_FIELD, new S3ArnableField().withArn(arn));
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
URI endpointTraitHost = null;
if (!clientConfiguration.isDisableHostPrefixInjection()) {
ValidationUtils.assertStringNotEmpty(deleteBucketPolicyRequest.getAccountId(), "AccountId");
HostnameValidator.validateHostnameCompliant(deleteBucketPolicyRequest.getAccountId(), "AccountId", "deleteBucketPolicyRequest");
String hostPrefix = "{AccountId}.";
String resolvedHostPrefix = String.format("%s.", deleteBucketPolicyRequest.getAccountId());
endpointTraitHost = UriResourcePathUtils.updateUriHost(endpoint, resolvedHostPrefix);
}
StaxResponseHandler responseHandler = new com.amazonaws.services.s3control.internal.S3ControlStaxResponseHandler(
new DeleteBucketPolicyResultStaxUnmarshaller());
response = invoke(request, responseHandler, executionContext, null, endpointTraitHost);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
*
* This action deletes an Amazon S3 on Outposts bucket's tags. To delete an S3 bucket tags, see DeleteBucketTagging in
* the Amazon S3 API Reference.
*
*
*
* Deletes the tags from the Outposts bucket. For more information, see Using Amazon S3 on Outposts in
* Amazon S3 User Guide.
*
*
* To use this action, you must have permission to perform the PutBucketTagging
action. By default, the
* bucket owner has this permission and can grant this permission to others.
*
*
* All Amazon S3 on Outposts REST API requests for this action require an additional parameter of
* x-amz-outpost-id
to be passed with the request and an S3 on Outposts endpoint hostname prefix
* instead of s3-control
. For an example of the request syntax for Amazon S3 on Outposts that uses the
* S3 on Outposts endpoint hostname prefix and the x-amz-outpost-id
derived using the access point ARN,
* see the Examples section.
*
*
* The following actions are related to DeleteBucketTagging
:
*
*
* -
*
* GetBucketTagging
*
*
* -
*
* PutBucketTagging
*
*
*
*
* @param deleteBucketTaggingRequest
* @return Result of the DeleteBucketTagging operation returned by the service.
* @sample AWSS3Control.DeleteBucketTagging
* @see AWS
* API Documentation
*/
@Override
public DeleteBucketTaggingResult deleteBucketTagging(DeleteBucketTaggingRequest request) {
request = beforeClientExecution(request);
return executeDeleteBucketTagging(request);
}
@SdkInternalApi
final DeleteBucketTaggingResult executeDeleteBucketTagging(DeleteBucketTaggingRequest deleteBucketTaggingRequest) {
ExecutionContext executionContext = createExecutionContext(deleteBucketTaggingRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
String bucket = deleteBucketTaggingRequest.getBucket();
Arn arn = null;
if (bucket != null && bucket.startsWith("arn:")) {
arn = Arn.fromString(bucket);
AwsResource awsResource = com.amazonaws.services.s3control.internal.S3ControlArnConverter.getInstance().convertArn(arn);
if (!(awsResource instanceof com.amazonaws.services.s3control.internal.S3ControlBucketResource)) {
throw new IllegalArgumentException("Unsupported ARN type: " + awsResource.getClass()
+ ". Expected com.amazonaws.services.s3control.internal.S3ControlBucketResource.");
}
com.amazonaws.services.s3control.internal.S3ControlBucketResource resource = (com.amazonaws.services.s3control.internal.S3ControlBucketResource) awsResource;
deleteBucketTaggingRequest = deleteBucketTaggingRequest.clone();
deleteBucketTaggingRequest.setBucket(resource.getBucketName());
String accountId = deleteBucketTaggingRequest.getAccountId();
String accountIdInArn = resource.getAccountId();
if (accountId != null && !accountId.equals(accountIdInArn)) {
throw new IllegalArgumentException(String.format("%s field provided from the request (%s) is different from the one in the ARN (%s)",
"accountId", accountId, accountIdInArn));
}
deleteBucketTaggingRequest.setAccountId(accountIdInArn);
}
request = new DeleteBucketTaggingRequestMarshaller().marshall(super.beforeMarshalling(deleteBucketTaggingRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "S3 Control");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DeleteBucketTagging");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
request.addHandlerContext(S3ControlHandlerContextKey.S3_ARNABLE_FIELD, new S3ArnableField().withArn(arn));
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
URI endpointTraitHost = null;
if (!clientConfiguration.isDisableHostPrefixInjection()) {
ValidationUtils.assertStringNotEmpty(deleteBucketTaggingRequest.getAccountId(), "AccountId");
HostnameValidator.validateHostnameCompliant(deleteBucketTaggingRequest.getAccountId(), "AccountId", "deleteBucketTaggingRequest");
String hostPrefix = "{AccountId}.";
String resolvedHostPrefix = String.format("%s.", deleteBucketTaggingRequest.getAccountId());
endpointTraitHost = UriResourcePathUtils.updateUriHost(endpoint, resolvedHostPrefix);
}
StaxResponseHandler responseHandler = new com.amazonaws.services.s3control.internal.S3ControlStaxResponseHandler(
new DeleteBucketTaggingResultStaxUnmarshaller());
response = invoke(request, responseHandler, executionContext, null, endpointTraitHost);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Removes the entire tag set from the specified S3 Batch Operations job. To use this operation, you must have
* permission to perform the s3:DeleteJobTagging
action. For more information, see Controlling access and labeling jobs using tags in the Amazon S3 User Guide.
*
*
*
* Related actions include:
*
*
* -
*
* CreateJob
*
*
* -
*
* GetJobTagging
*
*
* -
*
* PutJobTagging
*
*
*
*
* @param deleteJobTaggingRequest
* @return Result of the DeleteJobTagging operation returned by the service.
* @throws InternalServiceException
* @throws TooManyRequestsException
* @throws NotFoundException
* @sample AWSS3Control.DeleteJobTagging
* @see AWS API
* Documentation
*/
@Override
public DeleteJobTaggingResult deleteJobTagging(DeleteJobTaggingRequest request) {
request = beforeClientExecution(request);
return executeDeleteJobTagging(request);
}
@SdkInternalApi
final DeleteJobTaggingResult executeDeleteJobTagging(DeleteJobTaggingRequest deleteJobTaggingRequest) {
ExecutionContext executionContext = createExecutionContext(deleteJobTaggingRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DeleteJobTaggingRequestMarshaller().marshall(super.beforeMarshalling(deleteJobTaggingRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "S3 Control");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DeleteJobTagging");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
URI endpointTraitHost = null;
if (!clientConfiguration.isDisableHostPrefixInjection()) {
ValidationUtils.assertStringNotEmpty(deleteJobTaggingRequest.getAccountId(), "AccountId");
HostnameValidator.validateHostnameCompliant(deleteJobTaggingRequest.getAccountId(), "AccountId", "deleteJobTaggingRequest");
String hostPrefix = "{AccountId}.";
String resolvedHostPrefix = String.format("%s.", deleteJobTaggingRequest.getAccountId());
endpointTraitHost = UriResourcePathUtils.updateUriHost(endpoint, resolvedHostPrefix);
}
StaxResponseHandler responseHandler = new com.amazonaws.services.s3control.internal.S3ControlStaxResponseHandler(
new DeleteJobTaggingResultStaxUnmarshaller());
response = invoke(request, responseHandler, executionContext, null, endpointTraitHost);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Deletes a Multi-Region Access Point. This action does not delete the buckets associated with the Multi-Region
* Access Point, only the Multi-Region Access Point itself.
*
*
* This action will always be routed to the US West (Oregon) Region. For more information about the restrictions
* around managing Multi-Region Access Points, see Managing
* Multi-Region Access Points in the Amazon S3 User Guide.
*
*
* This request is asynchronous, meaning that you might receive a response before the command has completed. When
* this request provides a response, it provides a token that you can use to monitor the status of the request with
* DescribeMultiRegionAccessPointOperation
.
*
*
* The following actions are related to DeleteMultiRegionAccessPoint
:
*
*
* -
*
*
* -
*
*
* -
*
*
* -
*
*
*
*
* @param deleteMultiRegionAccessPointRequest
* @return Result of the DeleteMultiRegionAccessPoint operation returned by the service.
* @sample AWSS3Control.DeleteMultiRegionAccessPoint
* @see AWS API Documentation
*/
@Override
public DeleteMultiRegionAccessPointResult deleteMultiRegionAccessPoint(DeleteMultiRegionAccessPointRequest request) {
request = beforeClientExecution(request);
return executeDeleteMultiRegionAccessPoint(request);
}
@SdkInternalApi
final DeleteMultiRegionAccessPointResult executeDeleteMultiRegionAccessPoint(DeleteMultiRegionAccessPointRequest deleteMultiRegionAccessPointRequest) {
ExecutionContext executionContext = createExecutionContext(deleteMultiRegionAccessPointRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DeleteMultiRegionAccessPointRequestMarshaller().marshall(super.beforeMarshalling(deleteMultiRegionAccessPointRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "S3 Control");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DeleteMultiRegionAccessPoint");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
URI endpointTraitHost = null;
if (!clientConfiguration.isDisableHostPrefixInjection()) {
ValidationUtils.assertStringNotEmpty(deleteMultiRegionAccessPointRequest.getAccountId(), "AccountId");
HostnameValidator.validateHostnameCompliant(deleteMultiRegionAccessPointRequest.getAccountId(), "AccountId",
"deleteMultiRegionAccessPointRequest");
String hostPrefix = "{AccountId}.";
String resolvedHostPrefix = String.format("%s.", deleteMultiRegionAccessPointRequest.getAccountId());
endpointTraitHost = UriResourcePathUtils.updateUriHost(endpoint, resolvedHostPrefix);
}
StaxResponseHandler responseHandler = new com.amazonaws.services.s3control.internal.S3ControlStaxResponseHandler(
new DeleteMultiRegionAccessPointResultStaxUnmarshaller());
response = invoke(request, responseHandler, executionContext, null, endpointTraitHost);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Removes the PublicAccessBlock
configuration for an Amazon Web Services account. For more
* information, see Using Amazon S3
* block public access.
*
*
* Related actions include:
*
*
* -
*
*
* -
*
*
*
*
* @param deletePublicAccessBlockRequest
* @return Result of the DeletePublicAccessBlock operation returned by the service.
* @sample AWSS3Control.DeletePublicAccessBlock
* @see AWS API Documentation
*/
@Override
public DeletePublicAccessBlockResult deletePublicAccessBlock(DeletePublicAccessBlockRequest request) {
request = beforeClientExecution(request);
return executeDeletePublicAccessBlock(request);
}
@SdkInternalApi
final DeletePublicAccessBlockResult executeDeletePublicAccessBlock(DeletePublicAccessBlockRequest deletePublicAccessBlockRequest) {
ExecutionContext executionContext = createExecutionContext(deletePublicAccessBlockRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DeletePublicAccessBlockRequestMarshaller().marshall(super.beforeMarshalling(deletePublicAccessBlockRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "S3 Control");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DeletePublicAccessBlock");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
URI endpointTraitHost = null;
if (!clientConfiguration.isDisableHostPrefixInjection()) {
ValidationUtils.assertStringNotEmpty(deletePublicAccessBlockRequest.getAccountId(), "AccountId");
HostnameValidator.validateHostnameCompliant(deletePublicAccessBlockRequest.getAccountId(), "AccountId", "deletePublicAccessBlockRequest");
String hostPrefix = "{AccountId}.";
String resolvedHostPrefix = String.format("%s.", deletePublicAccessBlockRequest.getAccountId());
endpointTraitHost = UriResourcePathUtils.updateUriHost(endpoint, resolvedHostPrefix);
}
StaxResponseHandler responseHandler = new com.amazonaws.services.s3control.internal.S3ControlStaxResponseHandler(
new DeletePublicAccessBlockResultStaxUnmarshaller());
response = invoke(request, responseHandler, executionContext, null, endpointTraitHost);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Deletes the Amazon S3 Storage Lens configuration. For more information about S3 Storage Lens, see Assessing your storage activity and
* usage with Amazon S3 Storage Lens in the Amazon S3 User Guide.
*
*
*
* To use this action, you must have permission to perform the s3:DeleteStorageLensConfiguration
* action. For more information, see Setting permissions to
* use Amazon S3 Storage Lens in the Amazon S3 User Guide.
*
*
*
* @param deleteStorageLensConfigurationRequest
* @return Result of the DeleteStorageLensConfiguration operation returned by the service.
* @sample AWSS3Control.DeleteStorageLensConfiguration
* @see AWS API Documentation
*/
@Override
public DeleteStorageLensConfigurationResult deleteStorageLensConfiguration(DeleteStorageLensConfigurationRequest request) {
request = beforeClientExecution(request);
return executeDeleteStorageLensConfiguration(request);
}
@SdkInternalApi
final DeleteStorageLensConfigurationResult executeDeleteStorageLensConfiguration(DeleteStorageLensConfigurationRequest deleteStorageLensConfigurationRequest) {
ExecutionContext executionContext = createExecutionContext(deleteStorageLensConfigurationRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DeleteStorageLensConfigurationRequestMarshaller().marshall(super.beforeMarshalling(deleteStorageLensConfigurationRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "S3 Control");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DeleteStorageLensConfiguration");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
URI endpointTraitHost = null;
if (!clientConfiguration.isDisableHostPrefixInjection()) {
ValidationUtils.assertStringNotEmpty(deleteStorageLensConfigurationRequest.getAccountId(), "AccountId");
HostnameValidator.validateHostnameCompliant(deleteStorageLensConfigurationRequest.getAccountId(), "AccountId",
"deleteStorageLensConfigurationRequest");
String hostPrefix = "{AccountId}.";
String resolvedHostPrefix = String.format("%s.", deleteStorageLensConfigurationRequest.getAccountId());
endpointTraitHost = UriResourcePathUtils.updateUriHost(endpoint, resolvedHostPrefix);
}
StaxResponseHandler responseHandler = new com.amazonaws.services.s3control.internal.S3ControlStaxResponseHandler(
new DeleteStorageLensConfigurationResultStaxUnmarshaller());
response = invoke(request, responseHandler, executionContext, null, endpointTraitHost);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Deletes the Amazon S3 Storage Lens configuration tags. For more information about S3 Storage Lens, see Assessing your storage activity and
* usage with Amazon S3 Storage Lens in the Amazon S3 User Guide.
*
*
*
* To use this action, you must have permission to perform the s3:DeleteStorageLensConfigurationTagging
* action. For more information, see Setting permissions to
* use Amazon S3 Storage Lens in the Amazon S3 User Guide.
*
*
*
* @param deleteStorageLensConfigurationTaggingRequest
* @return Result of the DeleteStorageLensConfigurationTagging operation returned by the service.
* @sample AWSS3Control.DeleteStorageLensConfigurationTagging
* @see AWS API Documentation
*/
@Override
public DeleteStorageLensConfigurationTaggingResult deleteStorageLensConfigurationTagging(DeleteStorageLensConfigurationTaggingRequest request) {
request = beforeClientExecution(request);
return executeDeleteStorageLensConfigurationTagging(request);
}
@SdkInternalApi
final DeleteStorageLensConfigurationTaggingResult executeDeleteStorageLensConfigurationTagging(
DeleteStorageLensConfigurationTaggingRequest deleteStorageLensConfigurationTaggingRequest) {
ExecutionContext executionContext = createExecutionContext(deleteStorageLensConfigurationTaggingRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DeleteStorageLensConfigurationTaggingRequestMarshaller().marshall(super
.beforeMarshalling(deleteStorageLensConfigurationTaggingRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "S3 Control");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DeleteStorageLensConfigurationTagging");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
URI endpointTraitHost = null;
if (!clientConfiguration.isDisableHostPrefixInjection()) {
ValidationUtils.assertStringNotEmpty(deleteStorageLensConfigurationTaggingRequest.getAccountId(), "AccountId");
HostnameValidator.validateHostnameCompliant(deleteStorageLensConfigurationTaggingRequest.getAccountId(), "AccountId",
"deleteStorageLensConfigurationTaggingRequest");
String hostPrefix = "{AccountId}.";
String resolvedHostPrefix = String.format("%s.", deleteStorageLensConfigurationTaggingRequest.getAccountId());
endpointTraitHost = UriResourcePathUtils.updateUriHost(endpoint, resolvedHostPrefix);
}
StaxResponseHandler responseHandler = new com.amazonaws.services.s3control.internal.S3ControlStaxResponseHandler(
new DeleteStorageLensConfigurationTaggingResultStaxUnmarshaller());
response = invoke(request, responseHandler, executionContext, null, endpointTraitHost);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Retrieves the configuration parameters and status for a Batch Operations job. For more information, see S3 Batch Operations in the
* Amazon S3 User Guide.
*
*
*
* Related actions include:
*
*
* -
*
* CreateJob
*
*
* -
*
* ListJobs
*
*
* -
*
*
* -
*
* UpdateJobStatus
*
*
*
*
* @param describeJobRequest
* @return Result of the DescribeJob operation returned by the service.
* @throws BadRequestException
* @throws TooManyRequestsException
* @throws NotFoundException
* @throws InternalServiceException
* @sample AWSS3Control.DescribeJob
* @see AWS API
* Documentation
*/
@Override
public DescribeJobResult describeJob(DescribeJobRequest request) {
request = beforeClientExecution(request);
return executeDescribeJob(request);
}
@SdkInternalApi
final DescribeJobResult executeDescribeJob(DescribeJobRequest describeJobRequest) {
ExecutionContext executionContext = createExecutionContext(describeJobRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DescribeJobRequestMarshaller().marshall(super.beforeMarshalling(describeJobRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "S3 Control");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DescribeJob");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
URI endpointTraitHost = null;
if (!clientConfiguration.isDisableHostPrefixInjection()) {
ValidationUtils.assertStringNotEmpty(describeJobRequest.getAccountId(), "AccountId");
HostnameValidator.validateHostnameCompliant(describeJobRequest.getAccountId(), "AccountId", "describeJobRequest");
String hostPrefix = "{AccountId}.";
String resolvedHostPrefix = String.format("%s.", describeJobRequest.getAccountId());
endpointTraitHost = UriResourcePathUtils.updateUriHost(endpoint, resolvedHostPrefix);
}
StaxResponseHandler responseHandler = new com.amazonaws.services.s3control.internal.S3ControlStaxResponseHandler(
new DescribeJobResultStaxUnmarshaller());
response = invoke(request, responseHandler, executionContext, null, endpointTraitHost);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Retrieves the status of an asynchronous request to manage a Multi-Region Access Point. For more information about
* managing Multi-Region Access Points and how asynchronous requests work, see Managing
* Multi-Region Access Points in the Amazon S3 User Guide.
*
*
* The following actions are related to GetMultiRegionAccessPoint
:
*
*
* -
*
*
* -
*
*
* -
*
*
* -
*
*
*
*
* @param describeMultiRegionAccessPointOperationRequest
* @return Result of the DescribeMultiRegionAccessPointOperation operation returned by the service.
* @sample AWSS3Control.DescribeMultiRegionAccessPointOperation
* @see AWS API Documentation
*/
@Override
public DescribeMultiRegionAccessPointOperationResult describeMultiRegionAccessPointOperation(DescribeMultiRegionAccessPointOperationRequest request) {
request = beforeClientExecution(request);
return executeDescribeMultiRegionAccessPointOperation(request);
}
@SdkInternalApi
final DescribeMultiRegionAccessPointOperationResult executeDescribeMultiRegionAccessPointOperation(
DescribeMultiRegionAccessPointOperationRequest describeMultiRegionAccessPointOperationRequest) {
ExecutionContext executionContext = createExecutionContext(describeMultiRegionAccessPointOperationRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DescribeMultiRegionAccessPointOperationRequestMarshaller().marshall(super
.beforeMarshalling(describeMultiRegionAccessPointOperationRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "S3 Control");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DescribeMultiRegionAccessPointOperation");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
URI endpointTraitHost = null;
if (!clientConfiguration.isDisableHostPrefixInjection()) {
ValidationUtils.assertStringNotEmpty(describeMultiRegionAccessPointOperationRequest.getAccountId(), "AccountId");
HostnameValidator.validateHostnameCompliant(describeMultiRegionAccessPointOperationRequest.getAccountId(), "AccountId",
"describeMultiRegionAccessPointOperationRequest");
String hostPrefix = "{AccountId}.";
String resolvedHostPrefix = String.format("%s.", describeMultiRegionAccessPointOperationRequest.getAccountId());
endpointTraitHost = UriResourcePathUtils.updateUriHost(endpoint, resolvedHostPrefix);
}
StaxResponseHandler responseHandler = new com.amazonaws.services.s3control.internal.S3ControlStaxResponseHandler(
new DescribeMultiRegionAccessPointOperationResultStaxUnmarshaller());
response = invoke(request, responseHandler, executionContext, null, endpointTraitHost);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Returns configuration information about the specified access point.
*
*
*
* All Amazon S3 on Outposts REST API requests for this action require an additional parameter of
* x-amz-outpost-id
to be passed with the request and an S3 on Outposts endpoint hostname prefix
* instead of s3-control
. For an example of the request syntax for Amazon S3 on Outposts that uses the
* S3 on Outposts endpoint hostname prefix and the x-amz-outpost-id
derived using the access point ARN,
* see the Examples section.
*
*
* The following actions are related to GetAccessPoint
:
*
*
* -
*
*
* -
*
*
* -
*
* ListAccessPoints
*
*
*
*
* @param getAccessPointRequest
* @return Result of the GetAccessPoint operation returned by the service.
* @sample AWSS3Control.GetAccessPoint
* @see AWS API
* Documentation
*/
@Override
public GetAccessPointResult getAccessPoint(GetAccessPointRequest request) {
request = beforeClientExecution(request);
return executeGetAccessPoint(request);
}
@SdkInternalApi
final GetAccessPointResult executeGetAccessPoint(GetAccessPointRequest getAccessPointRequest) {
ExecutionContext executionContext = createExecutionContext(getAccessPointRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
String name = getAccessPointRequest.getName();
Arn arn = null;
if (name != null && name.startsWith("arn:")) {
arn = Arn.fromString(name);
AwsResource awsResource = com.amazonaws.services.s3control.internal.S3ControlArnConverter.getInstance().convertArn(arn);
if (!(awsResource instanceof com.amazonaws.services.s3.S3AccessPointResource)) {
throw new IllegalArgumentException("Unsupported ARN type: " + awsResource.getClass()
+ ". Expected com.amazonaws.services.s3.S3AccessPointResource.");
}
com.amazonaws.services.s3.S3AccessPointResource resource = (com.amazonaws.services.s3.S3AccessPointResource) awsResource;
getAccessPointRequest = getAccessPointRequest.clone();
getAccessPointRequest.setName(resource.getAccessPointName());
String accountId = getAccessPointRequest.getAccountId();
String accountIdInArn = resource.getAccountId();
if (accountId != null && !accountId.equals(accountIdInArn)) {
throw new IllegalArgumentException(String.format("%s field provided from the request (%s) is different from the one in the ARN (%s)",
"accountId", accountId, accountIdInArn));
}
getAccessPointRequest.setAccountId(accountIdInArn);
}
request = new GetAccessPointRequestMarshaller().marshall(super.beforeMarshalling(getAccessPointRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "S3 Control");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "GetAccessPoint");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
request.addHandlerContext(S3ControlHandlerContextKey.S3_ARNABLE_FIELD, new S3ArnableField().withArn(arn));
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
URI endpointTraitHost = null;
if (!clientConfiguration.isDisableHostPrefixInjection()) {
ValidationUtils.assertStringNotEmpty(getAccessPointRequest.getAccountId(), "AccountId");
HostnameValidator.validateHostnameCompliant(getAccessPointRequest.getAccountId(), "AccountId", "getAccessPointRequest");
String hostPrefix = "{AccountId}.";
String resolvedHostPrefix = String.format("%s.", getAccessPointRequest.getAccountId());
endpointTraitHost = UriResourcePathUtils.updateUriHost(endpoint, resolvedHostPrefix);
}
StaxResponseHandler responseHandler = new com.amazonaws.services.s3control.internal.S3ControlStaxResponseHandler(
new GetAccessPointResultStaxUnmarshaller());
response = invoke(request, responseHandler, executionContext, null, endpointTraitHost);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Returns configuration for an Object Lambda Access Point.
*
*
* The following actions are related to GetAccessPointConfigurationForObjectLambda
:
*
*
* -
*
*
*
*
* @param getAccessPointConfigurationForObjectLambdaRequest
* @return Result of the GetAccessPointConfigurationForObjectLambda operation returned by the service.
* @sample AWSS3Control.GetAccessPointConfigurationForObjectLambda
* @see AWS API Documentation
*/
@Override
public GetAccessPointConfigurationForObjectLambdaResult getAccessPointConfigurationForObjectLambda(GetAccessPointConfigurationForObjectLambdaRequest request) {
request = beforeClientExecution(request);
return executeGetAccessPointConfigurationForObjectLambda(request);
}
@SdkInternalApi
final GetAccessPointConfigurationForObjectLambdaResult executeGetAccessPointConfigurationForObjectLambda(
GetAccessPointConfigurationForObjectLambdaRequest getAccessPointConfigurationForObjectLambdaRequest) {
ExecutionContext executionContext = createExecutionContext(getAccessPointConfigurationForObjectLambdaRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new GetAccessPointConfigurationForObjectLambdaRequestMarshaller().marshall(super
.beforeMarshalling(getAccessPointConfigurationForObjectLambdaRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "S3 Control");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "GetAccessPointConfigurationForObjectLambda");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
URI endpointTraitHost = null;
if (!clientConfiguration.isDisableHostPrefixInjection()) {
ValidationUtils.assertStringNotEmpty(getAccessPointConfigurationForObjectLambdaRequest.getAccountId(), "AccountId");
HostnameValidator.validateHostnameCompliant(getAccessPointConfigurationForObjectLambdaRequest.getAccountId(), "AccountId",
"getAccessPointConfigurationForObjectLambdaRequest");
String hostPrefix = "{AccountId}.";
String resolvedHostPrefix = String.format("%s.", getAccessPointConfigurationForObjectLambdaRequest.getAccountId());
endpointTraitHost = UriResourcePathUtils.updateUriHost(endpoint, resolvedHostPrefix);
}
StaxResponseHandler responseHandler = new com.amazonaws.services.s3control.internal.S3ControlStaxResponseHandler(
new GetAccessPointConfigurationForObjectLambdaResultStaxUnmarshaller());
response = invoke(request, responseHandler, executionContext, null, endpointTraitHost);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Returns configuration information about the specified Object Lambda Access Point
*
*
* The following actions are related to GetAccessPointForObjectLambda
:
*
*
* -
*
*
* -
*
*
* -
*
*
*
*
* @param getAccessPointForObjectLambdaRequest
* @return Result of the GetAccessPointForObjectLambda operation returned by the service.
* @sample AWSS3Control.GetAccessPointForObjectLambda
* @see AWS API Documentation
*/
@Override
public GetAccessPointForObjectLambdaResult getAccessPointForObjectLambda(GetAccessPointForObjectLambdaRequest request) {
request = beforeClientExecution(request);
return executeGetAccessPointForObjectLambda(request);
}
@SdkInternalApi
final GetAccessPointForObjectLambdaResult executeGetAccessPointForObjectLambda(GetAccessPointForObjectLambdaRequest getAccessPointForObjectLambdaRequest) {
ExecutionContext executionContext = createExecutionContext(getAccessPointForObjectLambdaRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new GetAccessPointForObjectLambdaRequestMarshaller().marshall(super.beforeMarshalling(getAccessPointForObjectLambdaRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "S3 Control");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "GetAccessPointForObjectLambda");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
URI endpointTraitHost = null;
if (!clientConfiguration.isDisableHostPrefixInjection()) {
ValidationUtils.assertStringNotEmpty(getAccessPointForObjectLambdaRequest.getAccountId(), "AccountId");
HostnameValidator.validateHostnameCompliant(getAccessPointForObjectLambdaRequest.getAccountId(), "AccountId",
"getAccessPointForObjectLambdaRequest");
String hostPrefix = "{AccountId}.";
String resolvedHostPrefix = String.format("%s.", getAccessPointForObjectLambdaRequest.getAccountId());
endpointTraitHost = UriResourcePathUtils.updateUriHost(endpoint, resolvedHostPrefix);
}
StaxResponseHandler responseHandler = new com.amazonaws.services.s3control.internal.S3ControlStaxResponseHandler(
new GetAccessPointForObjectLambdaResultStaxUnmarshaller());
response = invoke(request, responseHandler, executionContext, null, endpointTraitHost);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Returns the access point policy associated with the specified access point.
*
*
* The following actions are related to GetAccessPointPolicy
:
*
*
* -
*
*
* -
*
*
*
*
* @param getAccessPointPolicyRequest
* @return Result of the GetAccessPointPolicy operation returned by the service.
* @sample AWSS3Control.GetAccessPointPolicy
* @see AWS
* API Documentation
*/
@Override
public GetAccessPointPolicyResult getAccessPointPolicy(GetAccessPointPolicyRequest request) {
request = beforeClientExecution(request);
return executeGetAccessPointPolicy(request);
}
@SdkInternalApi
final GetAccessPointPolicyResult executeGetAccessPointPolicy(GetAccessPointPolicyRequest getAccessPointPolicyRequest) {
ExecutionContext executionContext = createExecutionContext(getAccessPointPolicyRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
String name = getAccessPointPolicyRequest.getName();
Arn arn = null;
if (name != null && name.startsWith("arn:")) {
arn = Arn.fromString(name);
AwsResource awsResource = com.amazonaws.services.s3control.internal.S3ControlArnConverter.getInstance().convertArn(arn);
if (!(awsResource instanceof com.amazonaws.services.s3.S3AccessPointResource)) {
throw new IllegalArgumentException("Unsupported ARN type: " + awsResource.getClass()
+ ". Expected com.amazonaws.services.s3.S3AccessPointResource.");
}
com.amazonaws.services.s3.S3AccessPointResource resource = (com.amazonaws.services.s3.S3AccessPointResource) awsResource;
getAccessPointPolicyRequest = getAccessPointPolicyRequest.clone();
getAccessPointPolicyRequest.setName(resource.getAccessPointName());
String accountId = getAccessPointPolicyRequest.getAccountId();
String accountIdInArn = resource.getAccountId();
if (accountId != null && !accountId.equals(accountIdInArn)) {
throw new IllegalArgumentException(String.format("%s field provided from the request (%s) is different from the one in the ARN (%s)",
"accountId", accountId, accountIdInArn));
}
getAccessPointPolicyRequest.setAccountId(accountIdInArn);
}
request = new GetAccessPointPolicyRequestMarshaller().marshall(super.beforeMarshalling(getAccessPointPolicyRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "S3 Control");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "GetAccessPointPolicy");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
request.addHandlerContext(S3ControlHandlerContextKey.S3_ARNABLE_FIELD, new S3ArnableField().withArn(arn));
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
URI endpointTraitHost = null;
if (!clientConfiguration.isDisableHostPrefixInjection()) {
ValidationUtils.assertStringNotEmpty(getAccessPointPolicyRequest.getAccountId(), "AccountId");
HostnameValidator.validateHostnameCompliant(getAccessPointPolicyRequest.getAccountId(), "AccountId", "getAccessPointPolicyRequest");
String hostPrefix = "{AccountId}.";
String resolvedHostPrefix = String.format("%s.", getAccessPointPolicyRequest.getAccountId());
endpointTraitHost = UriResourcePathUtils.updateUriHost(endpoint, resolvedHostPrefix);
}
StaxResponseHandler responseHandler = new com.amazonaws.services.s3control.internal.S3ControlStaxResponseHandler(
new GetAccessPointPolicyResultStaxUnmarshaller());
response = invoke(request, responseHandler, executionContext, null, endpointTraitHost);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Returns the resource policy for an Object Lambda Access Point.
*
*
* The following actions are related to GetAccessPointPolicyForObjectLambda
:
*
*
* -
*
*
* -
*
*
*
*
* @param getAccessPointPolicyForObjectLambdaRequest
* @return Result of the GetAccessPointPolicyForObjectLambda operation returned by the service.
* @sample AWSS3Control.GetAccessPointPolicyForObjectLambda
* @see AWS API Documentation
*/
@Override
public GetAccessPointPolicyForObjectLambdaResult getAccessPointPolicyForObjectLambda(GetAccessPointPolicyForObjectLambdaRequest request) {
request = beforeClientExecution(request);
return executeGetAccessPointPolicyForObjectLambda(request);
}
@SdkInternalApi
final GetAccessPointPolicyForObjectLambdaResult executeGetAccessPointPolicyForObjectLambda(
GetAccessPointPolicyForObjectLambdaRequest getAccessPointPolicyForObjectLambdaRequest) {
ExecutionContext executionContext = createExecutionContext(getAccessPointPolicyForObjectLambdaRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new GetAccessPointPolicyForObjectLambdaRequestMarshaller().marshall(super
.beforeMarshalling(getAccessPointPolicyForObjectLambdaRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "S3 Control");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "GetAccessPointPolicyForObjectLambda");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
URI endpointTraitHost = null;
if (!clientConfiguration.isDisableHostPrefixInjection()) {
ValidationUtils.assertStringNotEmpty(getAccessPointPolicyForObjectLambdaRequest.getAccountId(), "AccountId");
HostnameValidator.validateHostnameCompliant(getAccessPointPolicyForObjectLambdaRequest.getAccountId(), "AccountId",
"getAccessPointPolicyForObjectLambdaRequest");
String hostPrefix = "{AccountId}.";
String resolvedHostPrefix = String.format("%s.", getAccessPointPolicyForObjectLambdaRequest.getAccountId());
endpointTraitHost = UriResourcePathUtils.updateUriHost(endpoint, resolvedHostPrefix);
}
StaxResponseHandler responseHandler = new com.amazonaws.services.s3control.internal.S3ControlStaxResponseHandler(
new GetAccessPointPolicyForObjectLambdaResultStaxUnmarshaller());
response = invoke(request, responseHandler, executionContext, null, endpointTraitHost);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Indicates whether the specified access point currently has a policy that allows public access. For more
* information about public access through access points, see Managing Data Access with Amazon
* S3 access points in the Amazon S3 User Guide.
*
*
* @param getAccessPointPolicyStatusRequest
* @return Result of the GetAccessPointPolicyStatus operation returned by the service.
* @sample AWSS3Control.GetAccessPointPolicyStatus
* @see AWS API Documentation
*/
@Override
public GetAccessPointPolicyStatusResult getAccessPointPolicyStatus(GetAccessPointPolicyStatusRequest request) {
request = beforeClientExecution(request);
return executeGetAccessPointPolicyStatus(request);
}
@SdkInternalApi
final GetAccessPointPolicyStatusResult executeGetAccessPointPolicyStatus(GetAccessPointPolicyStatusRequest getAccessPointPolicyStatusRequest) {
ExecutionContext executionContext = createExecutionContext(getAccessPointPolicyStatusRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new GetAccessPointPolicyStatusRequestMarshaller().marshall(super.beforeMarshalling(getAccessPointPolicyStatusRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "S3 Control");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "GetAccessPointPolicyStatus");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
URI endpointTraitHost = null;
if (!clientConfiguration.isDisableHostPrefixInjection()) {
ValidationUtils.assertStringNotEmpty(getAccessPointPolicyStatusRequest.getAccountId(), "AccountId");
HostnameValidator.validateHostnameCompliant(getAccessPointPolicyStatusRequest.getAccountId(), "AccountId", "getAccessPointPolicyStatusRequest");
String hostPrefix = "{AccountId}.";
String resolvedHostPrefix = String.format("%s.", getAccessPointPolicyStatusRequest.getAccountId());
endpointTraitHost = UriResourcePathUtils.updateUriHost(endpoint, resolvedHostPrefix);
}
StaxResponseHandler responseHandler = new com.amazonaws.services.s3control.internal.S3ControlStaxResponseHandler(
new GetAccessPointPolicyStatusResultStaxUnmarshaller());
response = invoke(request, responseHandler, executionContext, null, endpointTraitHost);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Returns the status of the resource policy associated with an Object Lambda Access Point.
*
*
* @param getAccessPointPolicyStatusForObjectLambdaRequest
* @return Result of the GetAccessPointPolicyStatusForObjectLambda operation returned by the service.
* @sample AWSS3Control.GetAccessPointPolicyStatusForObjectLambda
* @see AWS API Documentation
*/
@Override
public GetAccessPointPolicyStatusForObjectLambdaResult getAccessPointPolicyStatusForObjectLambda(GetAccessPointPolicyStatusForObjectLambdaRequest request) {
request = beforeClientExecution(request);
return executeGetAccessPointPolicyStatusForObjectLambda(request);
}
@SdkInternalApi
final GetAccessPointPolicyStatusForObjectLambdaResult executeGetAccessPointPolicyStatusForObjectLambda(
GetAccessPointPolicyStatusForObjectLambdaRequest getAccessPointPolicyStatusForObjectLambdaRequest) {
ExecutionContext executionContext = createExecutionContext(getAccessPointPolicyStatusForObjectLambdaRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new GetAccessPointPolicyStatusForObjectLambdaRequestMarshaller().marshall(super
.beforeMarshalling(getAccessPointPolicyStatusForObjectLambdaRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "S3 Control");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "GetAccessPointPolicyStatusForObjectLambda");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
URI endpointTraitHost = null;
if (!clientConfiguration.isDisableHostPrefixInjection()) {
ValidationUtils.assertStringNotEmpty(getAccessPointPolicyStatusForObjectLambdaRequest.getAccountId(), "AccountId");
HostnameValidator.validateHostnameCompliant(getAccessPointPolicyStatusForObjectLambdaRequest.getAccountId(), "AccountId",
"getAccessPointPolicyStatusForObjectLambdaRequest");
String hostPrefix = "{AccountId}.";
String resolvedHostPrefix = String.format("%s.", getAccessPointPolicyStatusForObjectLambdaRequest.getAccountId());
endpointTraitHost = UriResourcePathUtils.updateUriHost(endpoint, resolvedHostPrefix);
}
StaxResponseHandler responseHandler = new com.amazonaws.services.s3control.internal.S3ControlStaxResponseHandler(
new GetAccessPointPolicyStatusForObjectLambdaResultStaxUnmarshaller());
response = invoke(request, responseHandler, executionContext, null, endpointTraitHost);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Gets an Amazon S3 on Outposts bucket. For more information, see Using Amazon S3 on Outposts
* in the Amazon S3 User Guide.
*
*
* If you are using an identity other than the root user of the Amazon Web Services account that owns the Outposts
* bucket, the calling identity must have the s3-outposts:GetBucket
permissions on the specified
* Outposts bucket and belong to the Outposts bucket owner's account in order to use this action. Only users from
* Outposts bucket owner account with the right permissions can perform actions on an Outposts bucket.
*
*
* If you don't have s3-outposts:GetBucket
permissions or you're not using an identity that belongs to
* the bucket owner's account, Amazon S3 returns a 403 Access Denied
error.
*
*
* The following actions are related to GetBucket
for Amazon S3 on Outposts:
*
*
* All Amazon S3 on Outposts REST API requests for this action require an additional parameter of
* x-amz-outpost-id
to be passed with the request and an S3 on Outposts endpoint hostname prefix
* instead of s3-control
. For an example of the request syntax for Amazon S3 on Outposts that uses the
* S3 on Outposts endpoint hostname prefix and the x-amz-outpost-id
derived using the access point ARN,
* see the Examples section.
*
*
* -
*
* PutObject
*
*
* -
*
* CreateBucket
*
*
* -
*
* DeleteBucket
*
*
*
*
* @param getBucketRequest
* @return Result of the GetBucket operation returned by the service.
* @sample AWSS3Control.GetBucket
* @see AWS API
* Documentation
*/
@Override
public GetBucketResult getBucket(GetBucketRequest request) {
request = beforeClientExecution(request);
return executeGetBucket(request);
}
@SdkInternalApi
final GetBucketResult executeGetBucket(GetBucketRequest getBucketRequest) {
ExecutionContext executionContext = createExecutionContext(getBucketRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
String bucket = getBucketRequest.getBucket();
Arn arn = null;
if (bucket != null && bucket.startsWith("arn:")) {
arn = Arn.fromString(bucket);
AwsResource awsResource = com.amazonaws.services.s3control.internal.S3ControlArnConverter.getInstance().convertArn(arn);
if (!(awsResource instanceof com.amazonaws.services.s3control.internal.S3ControlBucketResource)) {
throw new IllegalArgumentException("Unsupported ARN type: " + awsResource.getClass()
+ ". Expected com.amazonaws.services.s3control.internal.S3ControlBucketResource.");
}
com.amazonaws.services.s3control.internal.S3ControlBucketResource resource = (com.amazonaws.services.s3control.internal.S3ControlBucketResource) awsResource;
getBucketRequest = getBucketRequest.clone();
getBucketRequest.setBucket(resource.getBucketName());
String accountId = getBucketRequest.getAccountId();
String accountIdInArn = resource.getAccountId();
if (accountId != null && !accountId.equals(accountIdInArn)) {
throw new IllegalArgumentException(String.format("%s field provided from the request (%s) is different from the one in the ARN (%s)",
"accountId", accountId, accountIdInArn));
}
getBucketRequest.setAccountId(accountIdInArn);
}
request = new GetBucketRequestMarshaller().marshall(super.beforeMarshalling(getBucketRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "S3 Control");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "GetBucket");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
request.addHandlerContext(S3ControlHandlerContextKey.S3_ARNABLE_FIELD, new S3ArnableField().withArn(arn));
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
URI endpointTraitHost = null;
if (!clientConfiguration.isDisableHostPrefixInjection()) {
ValidationUtils.assertStringNotEmpty(getBucketRequest.getAccountId(), "AccountId");
HostnameValidator.validateHostnameCompliant(getBucketRequest.getAccountId(), "AccountId", "getBucketRequest");
String hostPrefix = "{AccountId}.";
String resolvedHostPrefix = String.format("%s.", getBucketRequest.getAccountId());
endpointTraitHost = UriResourcePathUtils.updateUriHost(endpoint, resolvedHostPrefix);
}
StaxResponseHandler responseHandler = new com.amazonaws.services.s3control.internal.S3ControlStaxResponseHandler(
new GetBucketResultStaxUnmarshaller());
response = invoke(request, responseHandler, executionContext, null, endpointTraitHost);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
*
* This action gets an Amazon S3 on Outposts bucket's lifecycle configuration. To get an S3 bucket's lifecycle
* configuration, see GetBucketLifecycleConfiguration in the Amazon S3 API Reference.
*
*
*
* Returns the lifecycle configuration information set on the Outposts bucket. For more information, see Using Amazon S3 on Outposts
* and for information about lifecycle configuration, see Object Lifecycle
* Management in Amazon S3 User Guide.
*
*
* To use this action, you must have permission to perform the s3-outposts:GetLifecycleConfiguration
* action. The Outposts bucket owner has this permission, by default. The bucket owner can grant this permission to
* others. For more information about permissions, see Permissions Related to Bucket Subresource Operations and Managing Access Permissions
* to Your Amazon S3 Resources.
*
*
* All Amazon S3 on Outposts REST API requests for this action require an additional parameter of
* x-amz-outpost-id
to be passed with the request and an S3 on Outposts endpoint hostname prefix
* instead of s3-control
. For an example of the request syntax for Amazon S3 on Outposts that uses the
* S3 on Outposts endpoint hostname prefix and the x-amz-outpost-id
derived using the access point ARN,
* see the Examples section.
*
*
* GetBucketLifecycleConfiguration
has the following special error:
*
*
* -
*
* Error code: NoSuchLifecycleConfiguration
*
*
* -
*
* Description: The lifecycle configuration does not exist.
*
*
* -
*
* HTTP Status Code: 404 Not Found
*
*
* -
*
* SOAP Fault Code Prefix: Client
*
*
*
*
*
*
* The following actions are related to GetBucketLifecycleConfiguration
:
*
*
* -
*
*
* -
*
*
*
*
* @param getBucketLifecycleConfigurationRequest
* @return Result of the GetBucketLifecycleConfiguration operation returned by the service.
* @sample AWSS3Control.GetBucketLifecycleConfiguration
* @see AWS API Documentation
*/
@Override
public GetBucketLifecycleConfigurationResult getBucketLifecycleConfiguration(GetBucketLifecycleConfigurationRequest request) {
request = beforeClientExecution(request);
return executeGetBucketLifecycleConfiguration(request);
}
@SdkInternalApi
final GetBucketLifecycleConfigurationResult executeGetBucketLifecycleConfiguration(
GetBucketLifecycleConfigurationRequest getBucketLifecycleConfigurationRequest) {
ExecutionContext executionContext = createExecutionContext(getBucketLifecycleConfigurationRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
String bucket = getBucketLifecycleConfigurationRequest.getBucket();
Arn arn = null;
if (bucket != null && bucket.startsWith("arn:")) {
arn = Arn.fromString(bucket);
AwsResource awsResource = com.amazonaws.services.s3control.internal.S3ControlArnConverter.getInstance().convertArn(arn);
if (!(awsResource instanceof com.amazonaws.services.s3control.internal.S3ControlBucketResource)) {
throw new IllegalArgumentException("Unsupported ARN type: " + awsResource.getClass()
+ ". Expected com.amazonaws.services.s3control.internal.S3ControlBucketResource.");
}
com.amazonaws.services.s3control.internal.S3ControlBucketResource resource = (com.amazonaws.services.s3control.internal.S3ControlBucketResource) awsResource;
getBucketLifecycleConfigurationRequest = getBucketLifecycleConfigurationRequest.clone();
getBucketLifecycleConfigurationRequest.setBucket(resource.getBucketName());
String accountId = getBucketLifecycleConfigurationRequest.getAccountId();
String accountIdInArn = resource.getAccountId();
if (accountId != null && !accountId.equals(accountIdInArn)) {
throw new IllegalArgumentException(String.format("%s field provided from the request (%s) is different from the one in the ARN (%s)",
"accountId", accountId, accountIdInArn));
}
getBucketLifecycleConfigurationRequest.setAccountId(accountIdInArn);
}
request = new GetBucketLifecycleConfigurationRequestMarshaller().marshall(super.beforeMarshalling(getBucketLifecycleConfigurationRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "S3 Control");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "GetBucketLifecycleConfiguration");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
request.addHandlerContext(S3ControlHandlerContextKey.S3_ARNABLE_FIELD, new S3ArnableField().withArn(arn));
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
URI endpointTraitHost = null;
if (!clientConfiguration.isDisableHostPrefixInjection()) {
ValidationUtils.assertStringNotEmpty(getBucketLifecycleConfigurationRequest.getAccountId(), "AccountId");
HostnameValidator.validateHostnameCompliant(getBucketLifecycleConfigurationRequest.getAccountId(), "AccountId",
"getBucketLifecycleConfigurationRequest");
String hostPrefix = "{AccountId}.";
String resolvedHostPrefix = String.format("%s.", getBucketLifecycleConfigurationRequest.getAccountId());
endpointTraitHost = UriResourcePathUtils.updateUriHost(endpoint, resolvedHostPrefix);
}
StaxResponseHandler responseHandler = new com.amazonaws.services.s3control.internal.S3ControlStaxResponseHandler(
new GetBucketLifecycleConfigurationResultStaxUnmarshaller());
response = invoke(request, responseHandler, executionContext, null, endpointTraitHost);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
*
* This action gets a bucket policy for an Amazon S3 on Outposts bucket. To get a policy for an S3 bucket, see GetBucketPolicy in the
* Amazon S3 API Reference.
*
*
*
* Returns the policy of a specified Outposts bucket. For more information, see Using Amazon S3 on Outposts in
* the Amazon S3 User Guide.
*
*
* If you are using an identity other than the root user of the Amazon Web Services account that owns the bucket,
* the calling identity must have the GetBucketPolicy
permissions on the specified bucket and belong to
* the bucket owner's account in order to use this action.
*
*
* Only users from Outposts bucket owner account with the right permissions can perform actions on an Outposts
* bucket. If you don't have s3-outposts:GetBucketPolicy
permissions or you're not using an identity
* that belongs to the bucket owner's account, Amazon S3 returns a 403 Access Denied
error.
*
*
*
* As a security precaution, the root user of the Amazon Web Services account that owns a bucket can always use this
* action, even if the policy explicitly denies the root user the ability to perform this action.
*
*
*
* For more information about bucket policies, see Using Bucket Policies and User
* Policies.
*
*
* All Amazon S3 on Outposts REST API requests for this action require an additional parameter of
* x-amz-outpost-id
to be passed with the request and an S3 on Outposts endpoint hostname prefix
* instead of s3-control
. For an example of the request syntax for Amazon S3 on Outposts that uses the
* S3 on Outposts endpoint hostname prefix and the x-amz-outpost-id
derived using the access point ARN,
* see the Examples section.
*
*
* The following actions are related to GetBucketPolicy
:
*
*
* -
*
* GetObject
*
*
* -
*
* PutBucketPolicy
*
*
* -
*
*
*
*
* @param getBucketPolicyRequest
* @return Result of the GetBucketPolicy operation returned by the service.
* @sample AWSS3Control.GetBucketPolicy
* @see AWS API
* Documentation
*/
@Override
public GetBucketPolicyResult getBucketPolicy(GetBucketPolicyRequest request) {
request = beforeClientExecution(request);
return executeGetBucketPolicy(request);
}
@SdkInternalApi
final GetBucketPolicyResult executeGetBucketPolicy(GetBucketPolicyRequest getBucketPolicyRequest) {
ExecutionContext executionContext = createExecutionContext(getBucketPolicyRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
String bucket = getBucketPolicyRequest.getBucket();
Arn arn = null;
if (bucket != null && bucket.startsWith("arn:")) {
arn = Arn.fromString(bucket);
AwsResource awsResource = com.amazonaws.services.s3control.internal.S3ControlArnConverter.getInstance().convertArn(arn);
if (!(awsResource instanceof com.amazonaws.services.s3control.internal.S3ControlBucketResource)) {
throw new IllegalArgumentException("Unsupported ARN type: " + awsResource.getClass()
+ ". Expected com.amazonaws.services.s3control.internal.S3ControlBucketResource.");
}
com.amazonaws.services.s3control.internal.S3ControlBucketResource resource = (com.amazonaws.services.s3control.internal.S3ControlBucketResource) awsResource;
getBucketPolicyRequest = getBucketPolicyRequest.clone();
getBucketPolicyRequest.setBucket(resource.getBucketName());
String accountId = getBucketPolicyRequest.getAccountId();
String accountIdInArn = resource.getAccountId();
if (accountId != null && !accountId.equals(accountIdInArn)) {
throw new IllegalArgumentException(String.format("%s field provided from the request (%s) is different from the one in the ARN (%s)",
"accountId", accountId, accountIdInArn));
}
getBucketPolicyRequest.setAccountId(accountIdInArn);
}
request = new GetBucketPolicyRequestMarshaller().marshall(super.beforeMarshalling(getBucketPolicyRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "S3 Control");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "GetBucketPolicy");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
request.addHandlerContext(S3ControlHandlerContextKey.S3_ARNABLE_FIELD, new S3ArnableField().withArn(arn));
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
URI endpointTraitHost = null;
if (!clientConfiguration.isDisableHostPrefixInjection()) {
ValidationUtils.assertStringNotEmpty(getBucketPolicyRequest.getAccountId(), "AccountId");
HostnameValidator.validateHostnameCompliant(getBucketPolicyRequest.getAccountId(), "AccountId", "getBucketPolicyRequest");
String hostPrefix = "{AccountId}.";
String resolvedHostPrefix = String.format("%s.", getBucketPolicyRequest.getAccountId());
endpointTraitHost = UriResourcePathUtils.updateUriHost(endpoint, resolvedHostPrefix);
}
StaxResponseHandler responseHandler = new com.amazonaws.services.s3control.internal.S3ControlStaxResponseHandler(
new GetBucketPolicyResultStaxUnmarshaller());
response = invoke(request, responseHandler, executionContext, null, endpointTraitHost);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
*
* This action gets an Amazon S3 on Outposts bucket's tags. To get an S3 bucket tags, see GetBucketTagging in the
* Amazon S3 API Reference.
*
*
*
* Returns the tag set associated with the Outposts bucket. For more information, see Using Amazon S3 on Outposts in
* the Amazon S3 User Guide.
*
*
* To use this action, you must have permission to perform the GetBucketTagging
action. By default, the
* bucket owner has this permission and can grant this permission to others.
*
*
* GetBucketTagging
has the following special error:
*
*
* -
*
* Error code: NoSuchTagSetError
*
*
* -
*
* Description: There is no tag set associated with the bucket.
*
*
*
*
*
*
* All Amazon S3 on Outposts REST API requests for this action require an additional parameter of
* x-amz-outpost-id
to be passed with the request and an S3 on Outposts endpoint hostname prefix
* instead of s3-control
. For an example of the request syntax for Amazon S3 on Outposts that uses the
* S3 on Outposts endpoint hostname prefix and the x-amz-outpost-id
derived using the access point ARN,
* see the Examples section.
*
*
* The following actions are related to GetBucketTagging
:
*
*
* -
*
* PutBucketTagging
*
*
* -
*
*
*
*
* @param getBucketTaggingRequest
* @return Result of the GetBucketTagging operation returned by the service.
* @sample AWSS3Control.GetBucketTagging
* @see AWS API
* Documentation
*/
@Override
public GetBucketTaggingResult getBucketTagging(GetBucketTaggingRequest request) {
request = beforeClientExecution(request);
return executeGetBucketTagging(request);
}
@SdkInternalApi
final GetBucketTaggingResult executeGetBucketTagging(GetBucketTaggingRequest getBucketTaggingRequest) {
ExecutionContext executionContext = createExecutionContext(getBucketTaggingRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
String bucket = getBucketTaggingRequest.getBucket();
Arn arn = null;
if (bucket != null && bucket.startsWith("arn:")) {
arn = Arn.fromString(bucket);
AwsResource awsResource = com.amazonaws.services.s3control.internal.S3ControlArnConverter.getInstance().convertArn(arn);
if (!(awsResource instanceof com.amazonaws.services.s3control.internal.S3ControlBucketResource)) {
throw new IllegalArgumentException("Unsupported ARN type: " + awsResource.getClass()
+ ". Expected com.amazonaws.services.s3control.internal.S3ControlBucketResource.");
}
com.amazonaws.services.s3control.internal.S3ControlBucketResource resource = (com.amazonaws.services.s3control.internal.S3ControlBucketResource) awsResource;
getBucketTaggingRequest = getBucketTaggingRequest.clone();
getBucketTaggingRequest.setBucket(resource.getBucketName());
String accountId = getBucketTaggingRequest.getAccountId();
String accountIdInArn = resource.getAccountId();
if (accountId != null && !accountId.equals(accountIdInArn)) {
throw new IllegalArgumentException(String.format("%s field provided from the request (%s) is different from the one in the ARN (%s)",
"accountId", accountId, accountIdInArn));
}
getBucketTaggingRequest.setAccountId(accountIdInArn);
}
request = new GetBucketTaggingRequestMarshaller().marshall(super.beforeMarshalling(getBucketTaggingRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "S3 Control");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "GetBucketTagging");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
request.addHandlerContext(S3ControlHandlerContextKey.S3_ARNABLE_FIELD, new S3ArnableField().withArn(arn));
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
URI endpointTraitHost = null;
if (!clientConfiguration.isDisableHostPrefixInjection()) {
ValidationUtils.assertStringNotEmpty(getBucketTaggingRequest.getAccountId(), "AccountId");
HostnameValidator.validateHostnameCompliant(getBucketTaggingRequest.getAccountId(), "AccountId", "getBucketTaggingRequest");
String hostPrefix = "{AccountId}.";
String resolvedHostPrefix = String.format("%s.", getBucketTaggingRequest.getAccountId());
endpointTraitHost = UriResourcePathUtils.updateUriHost(endpoint, resolvedHostPrefix);
}
StaxResponseHandler responseHandler = new com.amazonaws.services.s3control.internal.S3ControlStaxResponseHandler(
new GetBucketTaggingResultStaxUnmarshaller());
response = invoke(request, responseHandler, executionContext, null, endpointTraitHost);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Returns the tags on an S3 Batch Operations job. To use this operation, you must have permission to perform the
* s3:GetJobTagging
action. For more information, see Controlling access and labeling jobs using tags in the Amazon S3 User Guide.
*
*
*
* Related actions include:
*
*
* -
*
* CreateJob
*
*
* -
*
* PutJobTagging
*
*
* -
*
* DeleteJobTagging
*
*
*
*
* @param getJobTaggingRequest
* @return Result of the GetJobTagging operation returned by the service.
* @throws InternalServiceException
* @throws TooManyRequestsException
* @throws NotFoundException
* @sample AWSS3Control.GetJobTagging
* @see AWS API
* Documentation
*/
@Override
public GetJobTaggingResult getJobTagging(GetJobTaggingRequest request) {
request = beforeClientExecution(request);
return executeGetJobTagging(request);
}
@SdkInternalApi
final GetJobTaggingResult executeGetJobTagging(GetJobTaggingRequest getJobTaggingRequest) {
ExecutionContext executionContext = createExecutionContext(getJobTaggingRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new GetJobTaggingRequestMarshaller().marshall(super.beforeMarshalling(getJobTaggingRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "S3 Control");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "GetJobTagging");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
URI endpointTraitHost = null;
if (!clientConfiguration.isDisableHostPrefixInjection()) {
ValidationUtils.assertStringNotEmpty(getJobTaggingRequest.getAccountId(), "AccountId");
HostnameValidator.validateHostnameCompliant(getJobTaggingRequest.getAccountId(), "AccountId", "getJobTaggingRequest");
String hostPrefix = "{AccountId}.";
String resolvedHostPrefix = String.format("%s.", getJobTaggingRequest.getAccountId());
endpointTraitHost = UriResourcePathUtils.updateUriHost(endpoint, resolvedHostPrefix);
}
StaxResponseHandler responseHandler = new com.amazonaws.services.s3control.internal.S3ControlStaxResponseHandler(
new GetJobTaggingResultStaxUnmarshaller());
response = invoke(request, responseHandler, executionContext, null, endpointTraitHost);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Returns configuration information about the specified Multi-Region Access Point.
*
*
* This action will always be routed to the US West (Oregon) Region. For more information about the restrictions
* around managing Multi-Region Access Points, see Managing
* Multi-Region Access Points in the Amazon S3 User Guide.
*
*
* The following actions are related to GetMultiRegionAccessPoint
:
*
*
* -
*
*
* -
*
*
* -
*
*
* -
*
*
*
*
* @param getMultiRegionAccessPointRequest
* @return Result of the GetMultiRegionAccessPoint operation returned by the service.
* @sample AWSS3Control.GetMultiRegionAccessPoint
* @see AWS API Documentation
*/
@Override
public GetMultiRegionAccessPointResult getMultiRegionAccessPoint(GetMultiRegionAccessPointRequest request) {
request = beforeClientExecution(request);
return executeGetMultiRegionAccessPoint(request);
}
@SdkInternalApi
final GetMultiRegionAccessPointResult executeGetMultiRegionAccessPoint(GetMultiRegionAccessPointRequest getMultiRegionAccessPointRequest) {
ExecutionContext executionContext = createExecutionContext(getMultiRegionAccessPointRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new GetMultiRegionAccessPointRequestMarshaller().marshall(super.beforeMarshalling(getMultiRegionAccessPointRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "S3 Control");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "GetMultiRegionAccessPoint");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
URI endpointTraitHost = null;
if (!clientConfiguration.isDisableHostPrefixInjection()) {
ValidationUtils.assertStringNotEmpty(getMultiRegionAccessPointRequest.getAccountId(), "AccountId");
HostnameValidator.validateHostnameCompliant(getMultiRegionAccessPointRequest.getAccountId(), "AccountId", "getMultiRegionAccessPointRequest");
String hostPrefix = "{AccountId}.";
String resolvedHostPrefix = String.format("%s.", getMultiRegionAccessPointRequest.getAccountId());
endpointTraitHost = UriResourcePathUtils.updateUriHost(endpoint, resolvedHostPrefix);
}
StaxResponseHandler responseHandler = new com.amazonaws.services.s3control.internal.S3ControlStaxResponseHandler(
new GetMultiRegionAccessPointResultStaxUnmarshaller());
response = invoke(request, responseHandler, executionContext, null, endpointTraitHost);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Returns the access control policy of the specified Multi-Region Access Point.
*
*
* This action will always be routed to the US West (Oregon) Region. For more information about the restrictions
* around managing Multi-Region Access Points, see Managing
* Multi-Region Access Points in the Amazon S3 User Guide.
*
*
* The following actions are related to GetMultiRegionAccessPointPolicy
:
*
*
* -
*
*
* -
*
*
*
*
* @param getMultiRegionAccessPointPolicyRequest
* @return Result of the GetMultiRegionAccessPointPolicy operation returned by the service.
* @sample AWSS3Control.GetMultiRegionAccessPointPolicy
* @see AWS API Documentation
*/
@Override
public GetMultiRegionAccessPointPolicyResult getMultiRegionAccessPointPolicy(GetMultiRegionAccessPointPolicyRequest request) {
request = beforeClientExecution(request);
return executeGetMultiRegionAccessPointPolicy(request);
}
@SdkInternalApi
final GetMultiRegionAccessPointPolicyResult executeGetMultiRegionAccessPointPolicy(
GetMultiRegionAccessPointPolicyRequest getMultiRegionAccessPointPolicyRequest) {
ExecutionContext executionContext = createExecutionContext(getMultiRegionAccessPointPolicyRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new GetMultiRegionAccessPointPolicyRequestMarshaller().marshall(super.beforeMarshalling(getMultiRegionAccessPointPolicyRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "S3 Control");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "GetMultiRegionAccessPointPolicy");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
URI endpointTraitHost = null;
if (!clientConfiguration.isDisableHostPrefixInjection()) {
ValidationUtils.assertStringNotEmpty(getMultiRegionAccessPointPolicyRequest.getAccountId(), "AccountId");
HostnameValidator.validateHostnameCompliant(getMultiRegionAccessPointPolicyRequest.getAccountId(), "AccountId",
"getMultiRegionAccessPointPolicyRequest");
String hostPrefix = "{AccountId}.";
String resolvedHostPrefix = String.format("%s.", getMultiRegionAccessPointPolicyRequest.getAccountId());
endpointTraitHost = UriResourcePathUtils.updateUriHost(endpoint, resolvedHostPrefix);
}
StaxResponseHandler responseHandler = new com.amazonaws.services.s3control.internal.S3ControlStaxResponseHandler(
new GetMultiRegionAccessPointPolicyResultStaxUnmarshaller());
response = invoke(request, responseHandler, executionContext, null, endpointTraitHost);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Indicates whether the specified Multi-Region Access Point has an access control policy that allows public access.
*
*
* This action will always be routed to the US West (Oregon) Region. For more information about the restrictions
* around managing Multi-Region Access Points, see Managing
* Multi-Region Access Points in the Amazon S3 User Guide.
*
*
* The following actions are related to GetMultiRegionAccessPointPolicyStatus
:
*
*
* -
*
*
* -
*
*
*
*
* @param getMultiRegionAccessPointPolicyStatusRequest
* @return Result of the GetMultiRegionAccessPointPolicyStatus operation returned by the service.
* @sample AWSS3Control.GetMultiRegionAccessPointPolicyStatus
* @see AWS API Documentation
*/
@Override
public GetMultiRegionAccessPointPolicyStatusResult getMultiRegionAccessPointPolicyStatus(GetMultiRegionAccessPointPolicyStatusRequest request) {
request = beforeClientExecution(request);
return executeGetMultiRegionAccessPointPolicyStatus(request);
}
@SdkInternalApi
final GetMultiRegionAccessPointPolicyStatusResult executeGetMultiRegionAccessPointPolicyStatus(
GetMultiRegionAccessPointPolicyStatusRequest getMultiRegionAccessPointPolicyStatusRequest) {
ExecutionContext executionContext = createExecutionContext(getMultiRegionAccessPointPolicyStatusRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new GetMultiRegionAccessPointPolicyStatusRequestMarshaller().marshall(super
.beforeMarshalling(getMultiRegionAccessPointPolicyStatusRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "S3 Control");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "GetMultiRegionAccessPointPolicyStatus");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
URI endpointTraitHost = null;
if (!clientConfiguration.isDisableHostPrefixInjection()) {
ValidationUtils.assertStringNotEmpty(getMultiRegionAccessPointPolicyStatusRequest.getAccountId(), "AccountId");
HostnameValidator.validateHostnameCompliant(getMultiRegionAccessPointPolicyStatusRequest.getAccountId(), "AccountId",
"getMultiRegionAccessPointPolicyStatusRequest");
String hostPrefix = "{AccountId}.";
String resolvedHostPrefix = String.format("%s.", getMultiRegionAccessPointPolicyStatusRequest.getAccountId());
endpointTraitHost = UriResourcePathUtils.updateUriHost(endpoint, resolvedHostPrefix);
}
StaxResponseHandler responseHandler = new com.amazonaws.services.s3control.internal.S3ControlStaxResponseHandler(
new GetMultiRegionAccessPointPolicyStatusResultStaxUnmarshaller());
response = invoke(request, responseHandler, executionContext, null, endpointTraitHost);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Retrieves the PublicAccessBlock
configuration for an Amazon Web Services account. For more
* information, see Using Amazon S3
* block public access.
*
*
* Related actions include:
*
*
* -
*
*
* -
*
*
*
*
* @param getPublicAccessBlockRequest
* @return Result of the GetPublicAccessBlock operation returned by the service.
* @throws NoSuchPublicAccessBlockConfigurationException
* Amazon S3 throws this exception if you make a GetPublicAccessBlock
request against an
* account that doesn't have a PublicAccessBlockConfiguration
set.
* @sample AWSS3Control.GetPublicAccessBlock
* @see AWS
* API Documentation
*/
@Override
public GetPublicAccessBlockResult getPublicAccessBlock(GetPublicAccessBlockRequest request) {
request = beforeClientExecution(request);
return executeGetPublicAccessBlock(request);
}
@SdkInternalApi
final GetPublicAccessBlockResult executeGetPublicAccessBlock(GetPublicAccessBlockRequest getPublicAccessBlockRequest) {
ExecutionContext executionContext = createExecutionContext(getPublicAccessBlockRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new GetPublicAccessBlockRequestMarshaller().marshall(super.beforeMarshalling(getPublicAccessBlockRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "S3 Control");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "GetPublicAccessBlock");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
URI endpointTraitHost = null;
if (!clientConfiguration.isDisableHostPrefixInjection()) {
ValidationUtils.assertStringNotEmpty(getPublicAccessBlockRequest.getAccountId(), "AccountId");
HostnameValidator.validateHostnameCompliant(getPublicAccessBlockRequest.getAccountId(), "AccountId", "getPublicAccessBlockRequest");
String hostPrefix = "{AccountId}.";
String resolvedHostPrefix = String.format("%s.", getPublicAccessBlockRequest.getAccountId());
endpointTraitHost = UriResourcePathUtils.updateUriHost(endpoint, resolvedHostPrefix);
}
StaxResponseHandler responseHandler = new com.amazonaws.services.s3control.internal.S3ControlStaxResponseHandler(
new GetPublicAccessBlockResultStaxUnmarshaller());
response = invoke(request, responseHandler, executionContext, null, endpointTraitHost);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Gets the Amazon S3 Storage Lens configuration. For more information, see Assessing your storage activity and
* usage with Amazon S3 Storage Lens in the Amazon S3 User Guide.
*
*
*
* To use this action, you must have permission to perform the s3:GetStorageLensConfiguration
action.
* For more information, see Setting permissions to
* use Amazon S3 Storage Lens in the Amazon S3 User Guide.
*
*
*
* @param getStorageLensConfigurationRequest
* @return Result of the GetStorageLensConfiguration operation returned by the service.
* @sample AWSS3Control.GetStorageLensConfiguration
* @see AWS API Documentation
*/
@Override
public GetStorageLensConfigurationResult getStorageLensConfiguration(GetStorageLensConfigurationRequest request) {
request = beforeClientExecution(request);
return executeGetStorageLensConfiguration(request);
}
@SdkInternalApi
final GetStorageLensConfigurationResult executeGetStorageLensConfiguration(GetStorageLensConfigurationRequest getStorageLensConfigurationRequest) {
ExecutionContext executionContext = createExecutionContext(getStorageLensConfigurationRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new GetStorageLensConfigurationRequestMarshaller().marshall(super.beforeMarshalling(getStorageLensConfigurationRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "S3 Control");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "GetStorageLensConfiguration");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
URI endpointTraitHost = null;
if (!clientConfiguration.isDisableHostPrefixInjection()) {
ValidationUtils.assertStringNotEmpty(getStorageLensConfigurationRequest.getAccountId(), "AccountId");
HostnameValidator.validateHostnameCompliant(getStorageLensConfigurationRequest.getAccountId(), "AccountId",
"getStorageLensConfigurationRequest");
String hostPrefix = "{AccountId}.";
String resolvedHostPrefix = String.format("%s.", getStorageLensConfigurationRequest.getAccountId());
endpointTraitHost = UriResourcePathUtils.updateUriHost(endpoint, resolvedHostPrefix);
}
StaxResponseHandler responseHandler = new com.amazonaws.services.s3control.internal.S3ControlStaxResponseHandler(
new GetStorageLensConfigurationResultStaxUnmarshaller());
response = invoke(request, responseHandler, executionContext, null, endpointTraitHost);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Gets the tags of Amazon S3 Storage Lens configuration. For more information about S3 Storage Lens, see Assessing your storage activity and
* usage with Amazon S3 Storage Lens in the Amazon S3 User Guide.
*
*
*
* To use this action, you must have permission to perform the s3:GetStorageLensConfigurationTagging
* action. For more information, see Setting permissions to
* use Amazon S3 Storage Lens in the Amazon S3 User Guide.
*
*
*
* @param getStorageLensConfigurationTaggingRequest
* @return Result of the GetStorageLensConfigurationTagging operation returned by the service.
* @sample AWSS3Control.GetStorageLensConfigurationTagging
* @see AWS API Documentation
*/
@Override
public GetStorageLensConfigurationTaggingResult getStorageLensConfigurationTagging(GetStorageLensConfigurationTaggingRequest request) {
request = beforeClientExecution(request);
return executeGetStorageLensConfigurationTagging(request);
}
@SdkInternalApi
final GetStorageLensConfigurationTaggingResult executeGetStorageLensConfigurationTagging(
GetStorageLensConfigurationTaggingRequest getStorageLensConfigurationTaggingRequest) {
ExecutionContext executionContext = createExecutionContext(getStorageLensConfigurationTaggingRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new GetStorageLensConfigurationTaggingRequestMarshaller()
.marshall(super.beforeMarshalling(getStorageLensConfigurationTaggingRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "S3 Control");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "GetStorageLensConfigurationTagging");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
URI endpointTraitHost = null;
if (!clientConfiguration.isDisableHostPrefixInjection()) {
ValidationUtils.assertStringNotEmpty(getStorageLensConfigurationTaggingRequest.getAccountId(), "AccountId");
HostnameValidator.validateHostnameCompliant(getStorageLensConfigurationTaggingRequest.getAccountId(), "AccountId",
"getStorageLensConfigurationTaggingRequest");
String hostPrefix = "{AccountId}.";
String resolvedHostPrefix = String.format("%s.", getStorageLensConfigurationTaggingRequest.getAccountId());
endpointTraitHost = UriResourcePathUtils.updateUriHost(endpoint, resolvedHostPrefix);
}
StaxResponseHandler responseHandler = new com.amazonaws.services.s3control.internal.S3ControlStaxResponseHandler(
new GetStorageLensConfigurationTaggingResultStaxUnmarshaller());
response = invoke(request, responseHandler, executionContext, null, endpointTraitHost);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Returns a list of the access points currently associated with the specified bucket. You can retrieve up to 1000
* access points per call. If the specified bucket has more than 1,000 access points (or the number specified in
* maxResults
, whichever is less), the response will include a continuation token that you can use to
* list the additional access points.
*
*
*
* All Amazon S3 on Outposts REST API requests for this action require an additional parameter of
* x-amz-outpost-id
to be passed with the request and an S3 on Outposts endpoint hostname prefix
* instead of s3-control
. For an example of the request syntax for Amazon S3 on Outposts that uses the
* S3 on Outposts endpoint hostname prefix and the x-amz-outpost-id
derived using the access point ARN,
* see the Examples section.
*
*
* The following actions are related to ListAccessPoints
:
*
*
* -
*
*
* -
*
*
* -
*
* GetAccessPoint
*
*
*
*
* @param listAccessPointsRequest
* @return Result of the ListAccessPoints operation returned by the service.
* @sample AWSS3Control.ListAccessPoints
* @see AWS API
* Documentation
*/
@Override
public ListAccessPointsResult listAccessPoints(ListAccessPointsRequest request) {
request = beforeClientExecution(request);
return executeListAccessPoints(request);
}
@SdkInternalApi
final ListAccessPointsResult executeListAccessPoints(ListAccessPointsRequest listAccessPointsRequest) {
ExecutionContext executionContext = createExecutionContext(listAccessPointsRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
String bucket = listAccessPointsRequest.getBucket();
Arn arn = null;
if (bucket != null && bucket.startsWith("arn:")) {
arn = Arn.fromString(bucket);
AwsResource awsResource = com.amazonaws.services.s3control.internal.S3ControlArnConverter.getInstance().convertArn(arn);
if (!(awsResource instanceof com.amazonaws.services.s3control.internal.S3ControlBucketResource)) {
throw new IllegalArgumentException("Unsupported ARN type: " + awsResource.getClass()
+ ". Expected com.amazonaws.services.s3control.internal.S3ControlBucketResource.");
}
com.amazonaws.services.s3control.internal.S3ControlBucketResource resource = (com.amazonaws.services.s3control.internal.S3ControlBucketResource) awsResource;
listAccessPointsRequest = listAccessPointsRequest.clone();
listAccessPointsRequest.setBucket(resource.getBucketName());
String accountId = listAccessPointsRequest.getAccountId();
String accountIdInArn = resource.getAccountId();
if (accountId != null && !accountId.equals(accountIdInArn)) {
throw new IllegalArgumentException(String.format("%s field provided from the request (%s) is different from the one in the ARN (%s)",
"accountId", accountId, accountIdInArn));
}
listAccessPointsRequest.setAccountId(accountIdInArn);
}
request = new ListAccessPointsRequestMarshaller().marshall(super.beforeMarshalling(listAccessPointsRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "S3 Control");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "ListAccessPoints");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
request.addHandlerContext(S3ControlHandlerContextKey.S3_ARNABLE_FIELD, new S3ArnableField().withArn(arn));
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
URI endpointTraitHost = null;
if (!clientConfiguration.isDisableHostPrefixInjection()) {
ValidationUtils.assertStringNotEmpty(listAccessPointsRequest.getAccountId(), "AccountId");
HostnameValidator.validateHostnameCompliant(listAccessPointsRequest.getAccountId(), "AccountId", "listAccessPointsRequest");
String hostPrefix = "{AccountId}.";
String resolvedHostPrefix = String.format("%s.", listAccessPointsRequest.getAccountId());
endpointTraitHost = UriResourcePathUtils.updateUriHost(endpoint, resolvedHostPrefix);
}
StaxResponseHandler responseHandler = new com.amazonaws.services.s3control.internal.S3ControlStaxResponseHandler(
new ListAccessPointsResultStaxUnmarshaller());
response = invoke(request, responseHandler, executionContext, null, endpointTraitHost);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Returns some or all (up to 1,000) access points associated with the Object Lambda Access Point per call. If there
* are more access points than what can be returned in one call, the response will include a continuation token that
* you can use to list the additional access points.
*
*
* The following actions are related to ListAccessPointsForObjectLambda
:
*
*
* -
*
*
* -
*
*
* -
*
*
*
*
* @param listAccessPointsForObjectLambdaRequest
* @return Result of the ListAccessPointsForObjectLambda operation returned by the service.
* @sample AWSS3Control.ListAccessPointsForObjectLambda
* @see AWS API Documentation
*/
@Override
public ListAccessPointsForObjectLambdaResult listAccessPointsForObjectLambda(ListAccessPointsForObjectLambdaRequest request) {
request = beforeClientExecution(request);
return executeListAccessPointsForObjectLambda(request);
}
@SdkInternalApi
final ListAccessPointsForObjectLambdaResult executeListAccessPointsForObjectLambda(
ListAccessPointsForObjectLambdaRequest listAccessPointsForObjectLambdaRequest) {
ExecutionContext executionContext = createExecutionContext(listAccessPointsForObjectLambdaRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new ListAccessPointsForObjectLambdaRequestMarshaller().marshall(super.beforeMarshalling(listAccessPointsForObjectLambdaRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "S3 Control");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "ListAccessPointsForObjectLambda");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
URI endpointTraitHost = null;
if (!clientConfiguration.isDisableHostPrefixInjection()) {
ValidationUtils.assertStringNotEmpty(listAccessPointsForObjectLambdaRequest.getAccountId(), "AccountId");
HostnameValidator.validateHostnameCompliant(listAccessPointsForObjectLambdaRequest.getAccountId(), "AccountId",
"listAccessPointsForObjectLambdaRequest");
String hostPrefix = "{AccountId}.";
String resolvedHostPrefix = String.format("%s.", listAccessPointsForObjectLambdaRequest.getAccountId());
endpointTraitHost = UriResourcePathUtils.updateUriHost(endpoint, resolvedHostPrefix);
}
StaxResponseHandler responseHandler = new com.amazonaws.services.s3control.internal.S3ControlStaxResponseHandler(
new ListAccessPointsForObjectLambdaResultStaxUnmarshaller());
response = invoke(request, responseHandler, executionContext, null, endpointTraitHost);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Lists current S3 Batch Operations jobs and jobs that have ended within the last 30 days for the Amazon Web
* Services account making the request. For more information, see S3 Batch Operations in the
* Amazon S3 User Guide.
*
*
* Related actions include:
*
*
*
* -
*
* CreateJob
*
*
* -
*
* DescribeJob
*
*
* -
*
*
* -
*
* UpdateJobStatus
*
*
*
*
* @param listJobsRequest
* @return Result of the ListJobs operation returned by the service.
* @throws InvalidRequestException
* @throws InternalServiceException
* @throws InvalidNextTokenException
* @sample AWSS3Control.ListJobs
* @see AWS API
* Documentation
*/
@Override
public ListJobsResult listJobs(ListJobsRequest request) {
request = beforeClientExecution(request);
return executeListJobs(request);
}
@SdkInternalApi
final ListJobsResult executeListJobs(ListJobsRequest listJobsRequest) {
ExecutionContext executionContext = createExecutionContext(listJobsRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new ListJobsRequestMarshaller().marshall(super.beforeMarshalling(listJobsRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "S3 Control");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "ListJobs");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
URI endpointTraitHost = null;
if (!clientConfiguration.isDisableHostPrefixInjection()) {
ValidationUtils.assertStringNotEmpty(listJobsRequest.getAccountId(), "AccountId");
HostnameValidator.validateHostnameCompliant(listJobsRequest.getAccountId(), "AccountId", "listJobsRequest");
String hostPrefix = "{AccountId}.";
String resolvedHostPrefix = String.format("%s.", listJobsRequest.getAccountId());
endpointTraitHost = UriResourcePathUtils.updateUriHost(endpoint, resolvedHostPrefix);
}
StaxResponseHandler responseHandler = new com.amazonaws.services.s3control.internal.S3ControlStaxResponseHandler(
new ListJobsResultStaxUnmarshaller());
response = invoke(request, responseHandler, executionContext, null, endpointTraitHost);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Returns a list of the Multi-Region Access Points currently associated with the specified Amazon Web Services
* account. Each call can return up to 100 Multi-Region Access Points, the maximum number of Multi-Region Access
* Points that can be associated with a single account.
*
*
* This action will always be routed to the US West (Oregon) Region. For more information about the restrictions
* around managing Multi-Region Access Points, see Managing
* Multi-Region Access Points in the Amazon S3 User Guide.
*
*
* The following actions are related to ListMultiRegionAccessPoint
:
*
*
* -
*
*
* -
*
*
* -
*
*
* -
*
*
*
*
* @param listMultiRegionAccessPointsRequest
* @return Result of the ListMultiRegionAccessPoints operation returned by the service.
* @sample AWSS3Control.ListMultiRegionAccessPoints
* @see AWS API Documentation
*/
@Override
public ListMultiRegionAccessPointsResult listMultiRegionAccessPoints(ListMultiRegionAccessPointsRequest request) {
request = beforeClientExecution(request);
return executeListMultiRegionAccessPoints(request);
}
@SdkInternalApi
final ListMultiRegionAccessPointsResult executeListMultiRegionAccessPoints(ListMultiRegionAccessPointsRequest listMultiRegionAccessPointsRequest) {
ExecutionContext executionContext = createExecutionContext(listMultiRegionAccessPointsRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new ListMultiRegionAccessPointsRequestMarshaller().marshall(super.beforeMarshalling(listMultiRegionAccessPointsRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "S3 Control");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "ListMultiRegionAccessPoints");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
URI endpointTraitHost = null;
if (!clientConfiguration.isDisableHostPrefixInjection()) {
ValidationUtils.assertStringNotEmpty(listMultiRegionAccessPointsRequest.getAccountId(), "AccountId");
HostnameValidator.validateHostnameCompliant(listMultiRegionAccessPointsRequest.getAccountId(), "AccountId",
"listMultiRegionAccessPointsRequest");
String hostPrefix = "{AccountId}.";
String resolvedHostPrefix = String.format("%s.", listMultiRegionAccessPointsRequest.getAccountId());
endpointTraitHost = UriResourcePathUtils.updateUriHost(endpoint, resolvedHostPrefix);
}
StaxResponseHandler responseHandler = new com.amazonaws.services.s3control.internal.S3ControlStaxResponseHandler(
new ListMultiRegionAccessPointsResultStaxUnmarshaller());
response = invoke(request, responseHandler, executionContext, null, endpointTraitHost);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Returns a list of all Outposts buckets in an Outpost that are owned by the authenticated sender of the request.
* For more information, see Using
* Amazon S3 on Outposts in the Amazon S3 User Guide.
*
*
* For an example of the request syntax for Amazon S3 on Outposts that uses the S3 on Outposts endpoint hostname
* prefix and x-amz-outpost-id
in your request, see the Examples section.
*
*
* @param listRegionalBucketsRequest
* @return Result of the ListRegionalBuckets operation returned by the service.
* @sample AWSS3Control.ListRegionalBuckets
* @see AWS
* API Documentation
*/
@Override
public ListRegionalBucketsResult listRegionalBuckets(ListRegionalBucketsRequest request) {
request = beforeClientExecution(request);
return executeListRegionalBuckets(request);
}
@SdkInternalApi
final ListRegionalBucketsResult executeListRegionalBuckets(ListRegionalBucketsRequest listRegionalBucketsRequest) {
ExecutionContext executionContext = createExecutionContext(listRegionalBucketsRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new ListRegionalBucketsRequestMarshaller().marshall(super.beforeMarshalling(listRegionalBucketsRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "S3 Control");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "ListRegionalBuckets");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
URI endpointTraitHost = null;
if (!clientConfiguration.isDisableHostPrefixInjection()) {
ValidationUtils.assertStringNotEmpty(listRegionalBucketsRequest.getAccountId(), "AccountId");
HostnameValidator.validateHostnameCompliant(listRegionalBucketsRequest.getAccountId(), "AccountId", "listRegionalBucketsRequest");
String hostPrefix = "{AccountId}.";
String resolvedHostPrefix = String.format("%s.", listRegionalBucketsRequest.getAccountId());
endpointTraitHost = UriResourcePathUtils.updateUriHost(endpoint, resolvedHostPrefix);
}
StaxResponseHandler responseHandler = new com.amazonaws.services.s3control.internal.S3ControlStaxResponseHandler(
new ListRegionalBucketsResultStaxUnmarshaller());
response = invoke(request, responseHandler, executionContext, null, endpointTraitHost);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Gets a list of Amazon S3 Storage Lens configurations. For more information about S3 Storage Lens, see Assessing your storage activity and
* usage with Amazon S3 Storage Lens in the Amazon S3 User Guide.
*
*
*
* To use this action, you must have permission to perform the s3:ListStorageLensConfigurations
action.
* For more information, see Setting permissions to
* use Amazon S3 Storage Lens in the Amazon S3 User Guide.
*
*
*
* @param listStorageLensConfigurationsRequest
* @return Result of the ListStorageLensConfigurations operation returned by the service.
* @sample AWSS3Control.ListStorageLensConfigurations
* @see AWS API Documentation
*/
@Override
public ListStorageLensConfigurationsResult listStorageLensConfigurations(ListStorageLensConfigurationsRequest request) {
request = beforeClientExecution(request);
return executeListStorageLensConfigurations(request);
}
@SdkInternalApi
final ListStorageLensConfigurationsResult executeListStorageLensConfigurations(ListStorageLensConfigurationsRequest listStorageLensConfigurationsRequest) {
ExecutionContext executionContext = createExecutionContext(listStorageLensConfigurationsRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new ListStorageLensConfigurationsRequestMarshaller().marshall(super.beforeMarshalling(listStorageLensConfigurationsRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "S3 Control");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "ListStorageLensConfigurations");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
URI endpointTraitHost = null;
if (!clientConfiguration.isDisableHostPrefixInjection()) {
ValidationUtils.assertStringNotEmpty(listStorageLensConfigurationsRequest.getAccountId(), "AccountId");
HostnameValidator.validateHostnameCompliant(listStorageLensConfigurationsRequest.getAccountId(), "AccountId",
"listStorageLensConfigurationsRequest");
String hostPrefix = "{AccountId}.";
String resolvedHostPrefix = String.format("%s.", listStorageLensConfigurationsRequest.getAccountId());
endpointTraitHost = UriResourcePathUtils.updateUriHost(endpoint, resolvedHostPrefix);
}
StaxResponseHandler responseHandler = new com.amazonaws.services.s3control.internal.S3ControlStaxResponseHandler(
new ListStorageLensConfigurationsResultStaxUnmarshaller());
response = invoke(request, responseHandler, executionContext, null, endpointTraitHost);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Replaces configuration for an Object Lambda Access Point.
*
*
* The following actions are related to PutAccessPointConfigurationForObjectLambda
:
*
*
* -
*
*
*
*
* @param putAccessPointConfigurationForObjectLambdaRequest
* @return Result of the PutAccessPointConfigurationForObjectLambda operation returned by the service.
* @sample AWSS3Control.PutAccessPointConfigurationForObjectLambda
* @see AWS API Documentation
*/
@Override
public PutAccessPointConfigurationForObjectLambdaResult putAccessPointConfigurationForObjectLambda(PutAccessPointConfigurationForObjectLambdaRequest request) {
request = beforeClientExecution(request);
return executePutAccessPointConfigurationForObjectLambda(request);
}
@SdkInternalApi
final PutAccessPointConfigurationForObjectLambdaResult executePutAccessPointConfigurationForObjectLambda(
PutAccessPointConfigurationForObjectLambdaRequest putAccessPointConfigurationForObjectLambdaRequest) {
ExecutionContext executionContext = createExecutionContext(putAccessPointConfigurationForObjectLambdaRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new PutAccessPointConfigurationForObjectLambdaRequestMarshaller().marshall(super
.beforeMarshalling(putAccessPointConfigurationForObjectLambdaRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "S3 Control");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "PutAccessPointConfigurationForObjectLambda");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
URI endpointTraitHost = null;
if (!clientConfiguration.isDisableHostPrefixInjection()) {
ValidationUtils.assertStringNotEmpty(putAccessPointConfigurationForObjectLambdaRequest.getAccountId(), "AccountId");
HostnameValidator.validateHostnameCompliant(putAccessPointConfigurationForObjectLambdaRequest.getAccountId(), "AccountId",
"putAccessPointConfigurationForObjectLambdaRequest");
String hostPrefix = "{AccountId}.";
String resolvedHostPrefix = String.format("%s.", putAccessPointConfigurationForObjectLambdaRequest.getAccountId());
endpointTraitHost = UriResourcePathUtils.updateUriHost(endpoint, resolvedHostPrefix);
}
StaxResponseHandler responseHandler = new com.amazonaws.services.s3control.internal.S3ControlStaxResponseHandler(
new PutAccessPointConfigurationForObjectLambdaResultStaxUnmarshaller());
response = invoke(request, responseHandler, executionContext, null, endpointTraitHost);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Associates an access policy with the specified access point. Each access point can have only one policy, so a
* request made to this API replaces any existing policy associated with the specified access point.
*
*
*
* All Amazon S3 on Outposts REST API requests for this action require an additional parameter of
* x-amz-outpost-id
to be passed with the request and an S3 on Outposts endpoint hostname prefix
* instead of s3-control
. For an example of the request syntax for Amazon S3 on Outposts that uses the
* S3 on Outposts endpoint hostname prefix and the x-amz-outpost-id
derived using the access point ARN,
* see the Examples section.
*
*
* The following actions are related to PutAccessPointPolicy
:
*
*
* -
*
*
* -
*
*
*
*
* @param putAccessPointPolicyRequest
* @return Result of the PutAccessPointPolicy operation returned by the service.
* @sample AWSS3Control.PutAccessPointPolicy
* @see AWS
* API Documentation
*/
@Override
public PutAccessPointPolicyResult putAccessPointPolicy(PutAccessPointPolicyRequest request) {
request = beforeClientExecution(request);
return executePutAccessPointPolicy(request);
}
@SdkInternalApi
final PutAccessPointPolicyResult executePutAccessPointPolicy(PutAccessPointPolicyRequest putAccessPointPolicyRequest) {
ExecutionContext executionContext = createExecutionContext(putAccessPointPolicyRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
String name = putAccessPointPolicyRequest.getName();
Arn arn = null;
if (name != null && name.startsWith("arn:")) {
arn = Arn.fromString(name);
AwsResource awsResource = com.amazonaws.services.s3control.internal.S3ControlArnConverter.getInstance().convertArn(arn);
if (!(awsResource instanceof com.amazonaws.services.s3.S3AccessPointResource)) {
throw new IllegalArgumentException("Unsupported ARN type: " + awsResource.getClass()
+ ". Expected com.amazonaws.services.s3.S3AccessPointResource.");
}
com.amazonaws.services.s3.S3AccessPointResource resource = (com.amazonaws.services.s3.S3AccessPointResource) awsResource;
putAccessPointPolicyRequest = putAccessPointPolicyRequest.clone();
putAccessPointPolicyRequest.setName(resource.getAccessPointName());
String accountId = putAccessPointPolicyRequest.getAccountId();
String accountIdInArn = resource.getAccountId();
if (accountId != null && !accountId.equals(accountIdInArn)) {
throw new IllegalArgumentException(String.format("%s field provided from the request (%s) is different from the one in the ARN (%s)",
"accountId", accountId, accountIdInArn));
}
putAccessPointPolicyRequest.setAccountId(accountIdInArn);
}
request = new PutAccessPointPolicyRequestMarshaller().marshall(super.beforeMarshalling(putAccessPointPolicyRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "S3 Control");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "PutAccessPointPolicy");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
request.addHandlerContext(S3ControlHandlerContextKey.S3_ARNABLE_FIELD, new S3ArnableField().withArn(arn));
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
URI endpointTraitHost = null;
if (!clientConfiguration.isDisableHostPrefixInjection()) {
ValidationUtils.assertStringNotEmpty(putAccessPointPolicyRequest.getAccountId(), "AccountId");
HostnameValidator.validateHostnameCompliant(putAccessPointPolicyRequest.getAccountId(), "AccountId", "putAccessPointPolicyRequest");
String hostPrefix = "{AccountId}.";
String resolvedHostPrefix = String.format("%s.", putAccessPointPolicyRequest.getAccountId());
endpointTraitHost = UriResourcePathUtils.updateUriHost(endpoint, resolvedHostPrefix);
}
StaxResponseHandler responseHandler = new com.amazonaws.services.s3control.internal.S3ControlStaxResponseHandler(
new PutAccessPointPolicyResultStaxUnmarshaller());
response = invoke(request, responseHandler, executionContext, null, endpointTraitHost);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Creates or replaces resource policy for an Object Lambda Access Point. For an example policy, see Creating Object
* Lambda Access Points in the Amazon S3 User Guide.
*
*
* The following actions are related to PutAccessPointPolicyForObjectLambda
:
*
*
* -
*
*
* -
*
*
*
*
* @param putAccessPointPolicyForObjectLambdaRequest
* @return Result of the PutAccessPointPolicyForObjectLambda operation returned by the service.
* @sample AWSS3Control.PutAccessPointPolicyForObjectLambda
* @see AWS API Documentation
*/
@Override
public PutAccessPointPolicyForObjectLambdaResult putAccessPointPolicyForObjectLambda(PutAccessPointPolicyForObjectLambdaRequest request) {
request = beforeClientExecution(request);
return executePutAccessPointPolicyForObjectLambda(request);
}
@SdkInternalApi
final PutAccessPointPolicyForObjectLambdaResult executePutAccessPointPolicyForObjectLambda(
PutAccessPointPolicyForObjectLambdaRequest putAccessPointPolicyForObjectLambdaRequest) {
ExecutionContext executionContext = createExecutionContext(putAccessPointPolicyForObjectLambdaRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new PutAccessPointPolicyForObjectLambdaRequestMarshaller().marshall(super
.beforeMarshalling(putAccessPointPolicyForObjectLambdaRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "S3 Control");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "PutAccessPointPolicyForObjectLambda");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
URI endpointTraitHost = null;
if (!clientConfiguration.isDisableHostPrefixInjection()) {
ValidationUtils.assertStringNotEmpty(putAccessPointPolicyForObjectLambdaRequest.getAccountId(), "AccountId");
HostnameValidator.validateHostnameCompliant(putAccessPointPolicyForObjectLambdaRequest.getAccountId(), "AccountId",
"putAccessPointPolicyForObjectLambdaRequest");
String hostPrefix = "{AccountId}.";
String resolvedHostPrefix = String.format("%s.", putAccessPointPolicyForObjectLambdaRequest.getAccountId());
endpointTraitHost = UriResourcePathUtils.updateUriHost(endpoint, resolvedHostPrefix);
}
StaxResponseHandler responseHandler = new com.amazonaws.services.s3control.internal.S3ControlStaxResponseHandler(
new PutAccessPointPolicyForObjectLambdaResultStaxUnmarshaller());
response = invoke(request, responseHandler, executionContext, null, endpointTraitHost);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
*
* This action puts a lifecycle configuration to an Amazon S3 on Outposts bucket. To put a lifecycle configuration
* to an S3 bucket, see PutBucketLifecycleConfiguration in the Amazon S3 API Reference.
*
*
*
* Creates a new lifecycle configuration for the S3 on Outposts bucket or replaces an existing lifecycle
* configuration. Outposts buckets only support lifecycle configurations that delete/expire objects after a certain
* period of time and abort incomplete multipart uploads.
*
*
*
* All Amazon S3 on Outposts REST API requests for this action require an additional parameter of
* x-amz-outpost-id
to be passed with the request and an S3 on Outposts endpoint hostname prefix
* instead of s3-control
. For an example of the request syntax for Amazon S3 on Outposts that uses the
* S3 on Outposts endpoint hostname prefix and the x-amz-outpost-id
derived using the access point ARN,
* see the Examples section.
*
*
* The following actions are related to PutBucketLifecycleConfiguration
:
*
*
* -
*
*
* -
*
*
*
*
* @param putBucketLifecycleConfigurationRequest
* @return Result of the PutBucketLifecycleConfiguration operation returned by the service.
* @sample AWSS3Control.PutBucketLifecycleConfiguration
* @see AWS API Documentation
*/
@Override
public PutBucketLifecycleConfigurationResult putBucketLifecycleConfiguration(PutBucketLifecycleConfigurationRequest request) {
request = beforeClientExecution(request);
return executePutBucketLifecycleConfiguration(request);
}
@SdkInternalApi
final PutBucketLifecycleConfigurationResult executePutBucketLifecycleConfiguration(
PutBucketLifecycleConfigurationRequest putBucketLifecycleConfigurationRequest) {
ExecutionContext executionContext = createExecutionContext(putBucketLifecycleConfigurationRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
String bucket = putBucketLifecycleConfigurationRequest.getBucket();
Arn arn = null;
if (bucket != null && bucket.startsWith("arn:")) {
arn = Arn.fromString(bucket);
AwsResource awsResource = com.amazonaws.services.s3control.internal.S3ControlArnConverter.getInstance().convertArn(arn);
if (!(awsResource instanceof com.amazonaws.services.s3control.internal.S3ControlBucketResource)) {
throw new IllegalArgumentException("Unsupported ARN type: " + awsResource.getClass()
+ ". Expected com.amazonaws.services.s3control.internal.S3ControlBucketResource.");
}
com.amazonaws.services.s3control.internal.S3ControlBucketResource resource = (com.amazonaws.services.s3control.internal.S3ControlBucketResource) awsResource;
putBucketLifecycleConfigurationRequest = putBucketLifecycleConfigurationRequest.clone();
putBucketLifecycleConfigurationRequest.setBucket(resource.getBucketName());
String accountId = putBucketLifecycleConfigurationRequest.getAccountId();
String accountIdInArn = resource.getAccountId();
if (accountId != null && !accountId.equals(accountIdInArn)) {
throw new IllegalArgumentException(String.format("%s field provided from the request (%s) is different from the one in the ARN (%s)",
"accountId", accountId, accountIdInArn));
}
putBucketLifecycleConfigurationRequest.setAccountId(accountIdInArn);
}
request = new PutBucketLifecycleConfigurationRequestMarshaller().marshall(super.beforeMarshalling(putBucketLifecycleConfigurationRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "S3 Control");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "PutBucketLifecycleConfiguration");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
request.addHandlerContext(S3ControlHandlerContextKey.S3_ARNABLE_FIELD, new S3ArnableField().withArn(arn));
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
URI endpointTraitHost = null;
if (!clientConfiguration.isDisableHostPrefixInjection()) {
ValidationUtils.assertStringNotEmpty(putBucketLifecycleConfigurationRequest.getAccountId(), "AccountId");
HostnameValidator.validateHostnameCompliant(putBucketLifecycleConfigurationRequest.getAccountId(), "AccountId",
"putBucketLifecycleConfigurationRequest");
String hostPrefix = "{AccountId}.";
String resolvedHostPrefix = String.format("%s.", putBucketLifecycleConfigurationRequest.getAccountId());
endpointTraitHost = UriResourcePathUtils.updateUriHost(endpoint, resolvedHostPrefix);
}
StaxResponseHandler responseHandler = new com.amazonaws.services.s3control.internal.S3ControlStaxResponseHandler(
new PutBucketLifecycleConfigurationResultStaxUnmarshaller());
response = invoke(request, responseHandler, executionContext, null, endpointTraitHost);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
*
* This action puts a bucket policy to an Amazon S3 on Outposts bucket. To put a policy on an S3 bucket, see PutBucketPolicy in the
* Amazon S3 API Reference.
*
*
*
* Applies an Amazon S3 bucket policy to an Outposts bucket. For more information, see Using Amazon S3 on Outposts in
* the Amazon S3 User Guide.
*
*
* If you are using an identity other than the root user of the Amazon Web Services account that owns the Outposts
* bucket, the calling identity must have the PutBucketPolicy
permissions on the specified Outposts
* bucket and belong to the bucket owner's account in order to use this action.
*
*
* If you don't have PutBucketPolicy
permissions, Amazon S3 returns a 403 Access Denied
* error. If you have the correct permissions, but you're not using an identity that belongs to the bucket owner's
* account, Amazon S3 returns a 405 Method Not Allowed
error.
*
*
*
* As a security precaution, the root user of the Amazon Web Services account that owns a bucket can always use this
* action, even if the policy explicitly denies the root user the ability to perform this action.
*
*
*
* For more information about bucket policies, see Using Bucket Policies and User
* Policies.
*
*
* All Amazon S3 on Outposts REST API requests for this action require an additional parameter of
* x-amz-outpost-id
to be passed with the request and an S3 on Outposts endpoint hostname prefix
* instead of s3-control
. For an example of the request syntax for Amazon S3 on Outposts that uses the
* S3 on Outposts endpoint hostname prefix and the x-amz-outpost-id
derived using the access point ARN,
* see the Examples section.
*
*
* The following actions are related to PutBucketPolicy
:
*
*
* -
*
* GetBucketPolicy
*
*
* -
*
*
*
*
* @param putBucketPolicyRequest
* @return Result of the PutBucketPolicy operation returned by the service.
* @sample AWSS3Control.PutBucketPolicy
* @see AWS API
* Documentation
*/
@Override
public PutBucketPolicyResult putBucketPolicy(PutBucketPolicyRequest request) {
request = beforeClientExecution(request);
return executePutBucketPolicy(request);
}
@SdkInternalApi
final PutBucketPolicyResult executePutBucketPolicy(PutBucketPolicyRequest putBucketPolicyRequest) {
ExecutionContext executionContext = createExecutionContext(putBucketPolicyRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
String bucket = putBucketPolicyRequest.getBucket();
Arn arn = null;
if (bucket != null && bucket.startsWith("arn:")) {
arn = Arn.fromString(bucket);
AwsResource awsResource = com.amazonaws.services.s3control.internal.S3ControlArnConverter.getInstance().convertArn(arn);
if (!(awsResource instanceof com.amazonaws.services.s3control.internal.S3ControlBucketResource)) {
throw new IllegalArgumentException("Unsupported ARN type: " + awsResource.getClass()
+ ". Expected com.amazonaws.services.s3control.internal.S3ControlBucketResource.");
}
com.amazonaws.services.s3control.internal.S3ControlBucketResource resource = (com.amazonaws.services.s3control.internal.S3ControlBucketResource) awsResource;
putBucketPolicyRequest = putBucketPolicyRequest.clone();
putBucketPolicyRequest.setBucket(resource.getBucketName());
String accountId = putBucketPolicyRequest.getAccountId();
String accountIdInArn = resource.getAccountId();
if (accountId != null && !accountId.equals(accountIdInArn)) {
throw new IllegalArgumentException(String.format("%s field provided from the request (%s) is different from the one in the ARN (%s)",
"accountId", accountId, accountIdInArn));
}
putBucketPolicyRequest.setAccountId(accountIdInArn);
}
request = new PutBucketPolicyRequestMarshaller().marshall(super.beforeMarshalling(putBucketPolicyRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "S3 Control");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "PutBucketPolicy");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
request.addHandlerContext(S3ControlHandlerContextKey.S3_ARNABLE_FIELD, new S3ArnableField().withArn(arn));
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
URI endpointTraitHost = null;
if (!clientConfiguration.isDisableHostPrefixInjection()) {
ValidationUtils.assertStringNotEmpty(putBucketPolicyRequest.getAccountId(), "AccountId");
HostnameValidator.validateHostnameCompliant(putBucketPolicyRequest.getAccountId(), "AccountId", "putBucketPolicyRequest");
String hostPrefix = "{AccountId}.";
String resolvedHostPrefix = String.format("%s.", putBucketPolicyRequest.getAccountId());
endpointTraitHost = UriResourcePathUtils.updateUriHost(endpoint, resolvedHostPrefix);
}
StaxResponseHandler responseHandler = new com.amazonaws.services.s3control.internal.S3ControlStaxResponseHandler(
new PutBucketPolicyResultStaxUnmarshaller());
response = invoke(request, responseHandler, executionContext, null, endpointTraitHost);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
*
* This action puts tags on an Amazon S3 on Outposts bucket. To put tags on an S3 bucket, see PutBucketTagging in the
* Amazon S3 API Reference.
*
*
*
* Sets the tags for an S3 on Outposts bucket. For more information, see Using Amazon S3 on Outposts in
* the Amazon S3 User Guide.
*
*
* Use tags to organize your Amazon Web Services bill to reflect your own cost structure. To do this, sign up to get
* your Amazon Web Services account bill with tag key values included. Then, to see the cost of combined resources,
* organize your billing information according to resources with the same tag key values. For example, you can tag
* several resources with a specific application name, and then organize your billing information to see the total
* cost of that application across several services. For more information, see Cost allocation and
* tagging.
*
*
*
* Within a bucket, if you add a tag that has the same key as an existing tag, the new value overwrites the old
* value. For more information, see Using cost allocation in
* Amazon S3 bucket tags.
*
*
*
* To use this action, you must have permissions to perform the s3-outposts:PutBucketTagging
action.
* The Outposts bucket owner has this permission by default and can grant this permission to others. For more
* information about permissions, see Permissions Related to Bucket Subresource Operations and Managing access permissions
* to your Amazon S3 resources.
*
*
* PutBucketTagging
has the following special errors:
*
*
* -
*
* Error code: InvalidTagError
*
*
* -
*
* Description: The tag provided was not a valid tag. This error can occur if the tag did not pass input validation.
* For information about tag restrictions, see
* User-Defined Tag Restrictions and Amazon Web
* Services-Generated Cost Allocation Tag Restrictions.
*
*
*
*
* -
*
* Error code: MalformedXMLError
*
*
* -
*
* Description: The XML provided does not match the schema.
*
*
*
*
* -
*
* Error code: OperationAbortedError
*
*
* -
*
* Description: A conflicting conditional action is currently in progress against this resource. Try again.
*
*
*
*
* -
*
* Error code: InternalError
*
*
* -
*
* Description: The service was unable to apply the provided tag to the bucket.
*
*
*
*
*
*
* All Amazon S3 on Outposts REST API requests for this action require an additional parameter of
* x-amz-outpost-id
to be passed with the request and an S3 on Outposts endpoint hostname prefix
* instead of s3-control
. For an example of the request syntax for Amazon S3 on Outposts that uses the
* S3 on Outposts endpoint hostname prefix and the x-amz-outpost-id
derived using the access point ARN,
* see the Examples section.
*
*
* The following actions are related to PutBucketTagging
:
*
*
* -
*
* GetBucketTagging
*
*
* -
*
*
*
*
* @param putBucketTaggingRequest
* @return Result of the PutBucketTagging operation returned by the service.
* @sample AWSS3Control.PutBucketTagging
* @see AWS API
* Documentation
*/
@Override
public PutBucketTaggingResult putBucketTagging(PutBucketTaggingRequest request) {
request = beforeClientExecution(request);
return executePutBucketTagging(request);
}
@SdkInternalApi
final PutBucketTaggingResult executePutBucketTagging(PutBucketTaggingRequest putBucketTaggingRequest) {
ExecutionContext executionContext = createExecutionContext(putBucketTaggingRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
String bucket = putBucketTaggingRequest.getBucket();
Arn arn = null;
if (bucket != null && bucket.startsWith("arn:")) {
arn = Arn.fromString(bucket);
AwsResource awsResource = com.amazonaws.services.s3control.internal.S3ControlArnConverter.getInstance().convertArn(arn);
if (!(awsResource instanceof com.amazonaws.services.s3control.internal.S3ControlBucketResource)) {
throw new IllegalArgumentException("Unsupported ARN type: " + awsResource.getClass()
+ ". Expected com.amazonaws.services.s3control.internal.S3ControlBucketResource.");
}
com.amazonaws.services.s3control.internal.S3ControlBucketResource resource = (com.amazonaws.services.s3control.internal.S3ControlBucketResource) awsResource;
putBucketTaggingRequest = putBucketTaggingRequest.clone();
putBucketTaggingRequest.setBucket(resource.getBucketName());
String accountId = putBucketTaggingRequest.getAccountId();
String accountIdInArn = resource.getAccountId();
if (accountId != null && !accountId.equals(accountIdInArn)) {
throw new IllegalArgumentException(String.format("%s field provided from the request (%s) is different from the one in the ARN (%s)",
"accountId", accountId, accountIdInArn));
}
putBucketTaggingRequest.setAccountId(accountIdInArn);
}
request = new PutBucketTaggingRequestMarshaller().marshall(super.beforeMarshalling(putBucketTaggingRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "S3 Control");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "PutBucketTagging");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
request.addHandlerContext(S3ControlHandlerContextKey.S3_ARNABLE_FIELD, new S3ArnableField().withArn(arn));
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
URI endpointTraitHost = null;
if (!clientConfiguration.isDisableHostPrefixInjection()) {
ValidationUtils.assertStringNotEmpty(putBucketTaggingRequest.getAccountId(), "AccountId");
HostnameValidator.validateHostnameCompliant(putBucketTaggingRequest.getAccountId(), "AccountId", "putBucketTaggingRequest");
String hostPrefix = "{AccountId}.";
String resolvedHostPrefix = String.format("%s.", putBucketTaggingRequest.getAccountId());
endpointTraitHost = UriResourcePathUtils.updateUriHost(endpoint, resolvedHostPrefix);
}
StaxResponseHandler responseHandler = new com.amazonaws.services.s3control.internal.S3ControlStaxResponseHandler(
new PutBucketTaggingResultStaxUnmarshaller());
response = invoke(request, responseHandler, executionContext, null, endpointTraitHost);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Sets the supplied tag-set on an S3 Batch Operations job.
*
*
* A tag is a key-value pair. You can associate S3 Batch Operations tags with any job by sending a PUT request
* against the tagging subresource that is associated with the job. To modify the existing tag set, you can either
* replace the existing tag set entirely, or make changes within the existing tag set by retrieving the existing tag
* set using GetJobTagging, modify
* that tag set, and use this action to replace the tag set with the one you modified. For more information, see Controlling
* access and labeling jobs using tags in the Amazon S3 User Guide.
*
*
*
*
* -
*
* If you send this request with an empty tag set, Amazon S3 deletes the existing tag set on the Batch Operations
* job. If you use this method, you are charged for a Tier 1 Request (PUT). For more information, see Amazon S3 pricing.
*
*
* -
*
* For deleting existing tags for your Batch Operations job, a DeleteJobTagging
* request is preferred because it achieves the same result without incurring charges.
*
*
* -
*
* A few things to consider about using tags:
*
*
* -
*
* Amazon S3 limits the maximum number of tags to 50 tags per job.
*
*
* -
*
* You can associate up to 50 tags with a job as long as they have unique tag keys.
*
*
* -
*
* A tag key can be up to 128 Unicode characters in length, and tag values can be up to 256 Unicode characters in
* length.
*
*
* -
*
* The key and values are case sensitive.
*
*
* -
*
* For tagging-related restrictions related to characters and encodings, see User-Defined
* Tag Restrictions in the Billing and Cost Management User Guide.
*
*
*
*
*
*
*
*
* To use this action, you must have permission to perform the s3:PutJobTagging
action.
*
*
* Related actions include:
*
*
* -
*
* CreateJob
*
*
* -
*
* GetJobTagging
*
*
* -
*
* DeleteJobTagging
*
*
*
*
* @param putJobTaggingRequest
* @return Result of the PutJobTagging operation returned by the service.
* @throws InternalServiceException
* @throws TooManyRequestsException
* @throws NotFoundException
* @throws TooManyTagsException
* Amazon S3 throws this exception if you have too many tags in your tag set.
* @sample AWSS3Control.PutJobTagging
* @see AWS API
* Documentation
*/
@Override
public PutJobTaggingResult putJobTagging(PutJobTaggingRequest request) {
request = beforeClientExecution(request);
return executePutJobTagging(request);
}
@SdkInternalApi
final PutJobTaggingResult executePutJobTagging(PutJobTaggingRequest putJobTaggingRequest) {
ExecutionContext executionContext = createExecutionContext(putJobTaggingRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new PutJobTaggingRequestMarshaller().marshall(super.beforeMarshalling(putJobTaggingRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "S3 Control");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "PutJobTagging");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
URI endpointTraitHost = null;
if (!clientConfiguration.isDisableHostPrefixInjection()) {
ValidationUtils.assertStringNotEmpty(putJobTaggingRequest.getAccountId(), "AccountId");
HostnameValidator.validateHostnameCompliant(putJobTaggingRequest.getAccountId(), "AccountId", "putJobTaggingRequest");
String hostPrefix = "{AccountId}.";
String resolvedHostPrefix = String.format("%s.", putJobTaggingRequest.getAccountId());
endpointTraitHost = UriResourcePathUtils.updateUriHost(endpoint, resolvedHostPrefix);
}
StaxResponseHandler responseHandler = new com.amazonaws.services.s3control.internal.S3ControlStaxResponseHandler(
new PutJobTaggingResultStaxUnmarshaller());
response = invoke(request, responseHandler, executionContext, null, endpointTraitHost);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Associates an access control policy with the specified Multi-Region Access Point. Each Multi-Region Access Point
* can have only one policy, so a request made to this action replaces any existing policy that is associated with
* the specified Multi-Region Access Point.
*
*
* This action will always be routed to the US West (Oregon) Region. For more information about the restrictions
* around managing Multi-Region Access Points, see Managing
* Multi-Region Access Points in the Amazon S3 User Guide.
*
*
* The following actions are related to PutMultiRegionAccessPointPolicy
:
*
*
* -
*
*
* -
*
*
*
*
* @param putMultiRegionAccessPointPolicyRequest
* @return Result of the PutMultiRegionAccessPointPolicy operation returned by the service.
* @sample AWSS3Control.PutMultiRegionAccessPointPolicy
* @see AWS API Documentation
*/
@Override
public PutMultiRegionAccessPointPolicyResult putMultiRegionAccessPointPolicy(PutMultiRegionAccessPointPolicyRequest request) {
request = beforeClientExecution(request);
return executePutMultiRegionAccessPointPolicy(request);
}
@SdkInternalApi
final PutMultiRegionAccessPointPolicyResult executePutMultiRegionAccessPointPolicy(
PutMultiRegionAccessPointPolicyRequest putMultiRegionAccessPointPolicyRequest) {
ExecutionContext executionContext = createExecutionContext(putMultiRegionAccessPointPolicyRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new PutMultiRegionAccessPointPolicyRequestMarshaller().marshall(super.beforeMarshalling(putMultiRegionAccessPointPolicyRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "S3 Control");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "PutMultiRegionAccessPointPolicy");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
URI endpointTraitHost = null;
if (!clientConfiguration.isDisableHostPrefixInjection()) {
ValidationUtils.assertStringNotEmpty(putMultiRegionAccessPointPolicyRequest.getAccountId(), "AccountId");
HostnameValidator.validateHostnameCompliant(putMultiRegionAccessPointPolicyRequest.getAccountId(), "AccountId",
"putMultiRegionAccessPointPolicyRequest");
String hostPrefix = "{AccountId}.";
String resolvedHostPrefix = String.format("%s.", putMultiRegionAccessPointPolicyRequest.getAccountId());
endpointTraitHost = UriResourcePathUtils.updateUriHost(endpoint, resolvedHostPrefix);
}
StaxResponseHandler responseHandler = new com.amazonaws.services.s3control.internal.S3ControlStaxResponseHandler(
new PutMultiRegionAccessPointPolicyResultStaxUnmarshaller());
response = invoke(request, responseHandler, executionContext, null, endpointTraitHost);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Creates or modifies the PublicAccessBlock
configuration for an Amazon Web Services account. For this
* operation, users must have the s3:PutBucketPublicAccessBlock
permission. For more information, see
* Using Amazon
* S3 block public access.
*
*
* Related actions include:
*
*
* -
*
*
* -
*
*
*
*
* @param putPublicAccessBlockRequest
* @return Result of the PutPublicAccessBlock operation returned by the service.
* @sample AWSS3Control.PutPublicAccessBlock
* @see AWS
* API Documentation
*/
@Override
public PutPublicAccessBlockResult putPublicAccessBlock(PutPublicAccessBlockRequest request) {
request = beforeClientExecution(request);
return executePutPublicAccessBlock(request);
}
@SdkInternalApi
final PutPublicAccessBlockResult executePutPublicAccessBlock(PutPublicAccessBlockRequest putPublicAccessBlockRequest) {
ExecutionContext executionContext = createExecutionContext(putPublicAccessBlockRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new PutPublicAccessBlockRequestMarshaller().marshall(super.beforeMarshalling(putPublicAccessBlockRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "S3 Control");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "PutPublicAccessBlock");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
URI endpointTraitHost = null;
if (!clientConfiguration.isDisableHostPrefixInjection()) {
ValidationUtils.assertStringNotEmpty(putPublicAccessBlockRequest.getAccountId(), "AccountId");
HostnameValidator.validateHostnameCompliant(putPublicAccessBlockRequest.getAccountId(), "AccountId", "putPublicAccessBlockRequest");
String hostPrefix = "{AccountId}.";
String resolvedHostPrefix = String.format("%s.", putPublicAccessBlockRequest.getAccountId());
endpointTraitHost = UriResourcePathUtils.updateUriHost(endpoint, resolvedHostPrefix);
}
StaxResponseHandler responseHandler = new com.amazonaws.services.s3control.internal.S3ControlStaxResponseHandler(
new PutPublicAccessBlockResultStaxUnmarshaller());
response = invoke(request, responseHandler, executionContext, null, endpointTraitHost);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Puts an Amazon S3 Storage Lens configuration. For more information about S3 Storage Lens, see Working with Amazon S3 Storage Lens
* in the Amazon S3 User Guide.
*
*
*
* To use this action, you must have permission to perform the s3:PutStorageLensConfiguration
action.
* For more information, see Setting permissions to
* use Amazon S3 Storage Lens in the Amazon S3 User Guide.
*
*
*
* @param putStorageLensConfigurationRequest
* @return Result of the PutStorageLensConfiguration operation returned by the service.
* @sample AWSS3Control.PutStorageLensConfiguration
* @see AWS API Documentation
*/
@Override
public PutStorageLensConfigurationResult putStorageLensConfiguration(PutStorageLensConfigurationRequest request) {
request = beforeClientExecution(request);
return executePutStorageLensConfiguration(request);
}
@SdkInternalApi
final PutStorageLensConfigurationResult executePutStorageLensConfiguration(PutStorageLensConfigurationRequest putStorageLensConfigurationRequest) {
ExecutionContext executionContext = createExecutionContext(putStorageLensConfigurationRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new PutStorageLensConfigurationRequestMarshaller().marshall(super.beforeMarshalling(putStorageLensConfigurationRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "S3 Control");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "PutStorageLensConfiguration");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
URI endpointTraitHost = null;
if (!clientConfiguration.isDisableHostPrefixInjection()) {
ValidationUtils.assertStringNotEmpty(putStorageLensConfigurationRequest.getAccountId(), "AccountId");
HostnameValidator.validateHostnameCompliant(putStorageLensConfigurationRequest.getAccountId(), "AccountId",
"putStorageLensConfigurationRequest");
String hostPrefix = "{AccountId}.";
String resolvedHostPrefix = String.format("%s.", putStorageLensConfigurationRequest.getAccountId());
endpointTraitHost = UriResourcePathUtils.updateUriHost(endpoint, resolvedHostPrefix);
}
StaxResponseHandler responseHandler = new com.amazonaws.services.s3control.internal.S3ControlStaxResponseHandler(
new PutStorageLensConfigurationResultStaxUnmarshaller());
response = invoke(request, responseHandler, executionContext, null, endpointTraitHost);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Put or replace tags on an existing Amazon S3 Storage Lens configuration. For more information about S3 Storage
* Lens, see Assessing your storage
* activity and usage with Amazon S3 Storage Lens in the Amazon S3 User Guide.
*
*
*
* To use this action, you must have permission to perform the s3:PutStorageLensConfigurationTagging
* action. For more information, see Setting permissions to
* use Amazon S3 Storage Lens in the Amazon S3 User Guide.
*
*
*
* @param putStorageLensConfigurationTaggingRequest
* @return Result of the PutStorageLensConfigurationTagging operation returned by the service.
* @sample AWSS3Control.PutStorageLensConfigurationTagging
* @see AWS API Documentation
*/
@Override
public PutStorageLensConfigurationTaggingResult putStorageLensConfigurationTagging(PutStorageLensConfigurationTaggingRequest request) {
request = beforeClientExecution(request);
return executePutStorageLensConfigurationTagging(request);
}
@SdkInternalApi
final PutStorageLensConfigurationTaggingResult executePutStorageLensConfigurationTagging(
PutStorageLensConfigurationTaggingRequest putStorageLensConfigurationTaggingRequest) {
ExecutionContext executionContext = createExecutionContext(putStorageLensConfigurationTaggingRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new PutStorageLensConfigurationTaggingRequestMarshaller()
.marshall(super.beforeMarshalling(putStorageLensConfigurationTaggingRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "S3 Control");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "PutStorageLensConfigurationTagging");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
URI endpointTraitHost = null;
if (!clientConfiguration.isDisableHostPrefixInjection()) {
ValidationUtils.assertStringNotEmpty(putStorageLensConfigurationTaggingRequest.getAccountId(), "AccountId");
HostnameValidator.validateHostnameCompliant(putStorageLensConfigurationTaggingRequest.getAccountId(), "AccountId",
"putStorageLensConfigurationTaggingRequest");
String hostPrefix = "{AccountId}.";
String resolvedHostPrefix = String.format("%s.", putStorageLensConfigurationTaggingRequest.getAccountId());
endpointTraitHost = UriResourcePathUtils.updateUriHost(endpoint, resolvedHostPrefix);
}
StaxResponseHandler responseHandler = new com.amazonaws.services.s3control.internal.S3ControlStaxResponseHandler(
new PutStorageLensConfigurationTaggingResultStaxUnmarshaller());
response = invoke(request, responseHandler, executionContext, null, endpointTraitHost);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Updates an existing S3 Batch Operations job's priority. For more information, see S3 Batch Operations in the
* Amazon S3 User Guide.
*
*
*
* Related actions include:
*
*
* -
*
* CreateJob
*
*
* -
*
* ListJobs
*
*
* -
*
* DescribeJob
*
*
* -
*
* UpdateJobStatus
*
*
*
*
* @param updateJobPriorityRequest
* @return Result of the UpdateJobPriority operation returned by the service.
* @throws BadRequestException
* @throws TooManyRequestsException
* @throws NotFoundException
* @throws InternalServiceException
* @sample AWSS3Control.UpdateJobPriority
* @see AWS
* API Documentation
*/
@Override
public UpdateJobPriorityResult updateJobPriority(UpdateJobPriorityRequest request) {
request = beforeClientExecution(request);
return executeUpdateJobPriority(request);
}
@SdkInternalApi
final UpdateJobPriorityResult executeUpdateJobPriority(UpdateJobPriorityRequest updateJobPriorityRequest) {
ExecutionContext executionContext = createExecutionContext(updateJobPriorityRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new UpdateJobPriorityRequestMarshaller().marshall(super.beforeMarshalling(updateJobPriorityRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "S3 Control");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "UpdateJobPriority");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
URI endpointTraitHost = null;
if (!clientConfiguration.isDisableHostPrefixInjection()) {
ValidationUtils.assertStringNotEmpty(updateJobPriorityRequest.getAccountId(), "AccountId");
HostnameValidator.validateHostnameCompliant(updateJobPriorityRequest.getAccountId(), "AccountId", "updateJobPriorityRequest");
String hostPrefix = "{AccountId}.";
String resolvedHostPrefix = String.format("%s.", updateJobPriorityRequest.getAccountId());
endpointTraitHost = UriResourcePathUtils.updateUriHost(endpoint, resolvedHostPrefix);
}
StaxResponseHandler responseHandler = new com.amazonaws.services.s3control.internal.S3ControlStaxResponseHandler(
new UpdateJobPriorityResultStaxUnmarshaller());
response = invoke(request, responseHandler, executionContext, null, endpointTraitHost);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Updates the status for the specified job. Use this action to confirm that you want to run a job or to cancel an
* existing job. For more information, see S3 Batch Operations in the
* Amazon S3 User Guide.
*
*
*
* Related actions include:
*
*
* -
*
* CreateJob
*
*
* -
*
* ListJobs
*
*
* -
*
* DescribeJob
*
*
* -
*
* UpdateJobStatus
*
*
*
*
* @param updateJobStatusRequest
* @return Result of the UpdateJobStatus operation returned by the service.
* @throws BadRequestException
* @throws TooManyRequestsException
* @throws NotFoundException
* @throws JobStatusException
* @throws InternalServiceException
* @sample AWSS3Control.UpdateJobStatus
* @see AWS API
* Documentation
*/
@Override
public UpdateJobStatusResult updateJobStatus(UpdateJobStatusRequest request) {
request = beforeClientExecution(request);
return executeUpdateJobStatus(request);
}
@SdkInternalApi
final UpdateJobStatusResult executeUpdateJobStatus(UpdateJobStatusRequest updateJobStatusRequest) {
ExecutionContext executionContext = createExecutionContext(updateJobStatusRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new UpdateJobStatusRequestMarshaller().marshall(super.beforeMarshalling(updateJobStatusRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "S3 Control");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "UpdateJobStatus");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
URI endpointTraitHost = null;
if (!clientConfiguration.isDisableHostPrefixInjection()) {
ValidationUtils.assertStringNotEmpty(updateJobStatusRequest.getAccountId(), "AccountId");
HostnameValidator.validateHostnameCompliant(updateJobStatusRequest.getAccountId(), "AccountId", "updateJobStatusRequest");
String hostPrefix = "{AccountId}.";
String resolvedHostPrefix = String.format("%s.", updateJobStatusRequest.getAccountId());
endpointTraitHost = UriResourcePathUtils.updateUriHost(endpoint, resolvedHostPrefix);
}
StaxResponseHandler responseHandler = new com.amazonaws.services.s3control.internal.S3ControlStaxResponseHandler(
new UpdateJobStatusResultStaxUnmarshaller());
response = invoke(request, responseHandler, executionContext, null, endpointTraitHost);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
* Returns additional metadata for a previously executed successful, request, typically used for debugging issues
* where a service isn't acting as expected. This data isn't considered part of the result data returned by an
* operation, so it's available through this separate, diagnostic interface.
*
* Response metadata is only cached for a limited period of time, so if you need to access this extra diagnostic
* information for an executed request, you should use this method to retrieve it as soon as possible after
* executing the request.
*
* @param request
* The originally executed request
*
* @return The response metadata for the specified request, or null if none is available.
*/
public com.amazonaws.services.s3control.S3ControlResponseMetadata getCachedResponseMetadata(AmazonWebServiceRequest request) {
ResponseMetadata metadata = client.getResponseMetadataForRequest(request);
if (metadata != null)
return new com.amazonaws.services.s3control.S3ControlResponseMetadata(metadata);
else
return null;
}
/**
* Normal invoke with authentication. Credentials are required and may be overriden at the request level.
**/
private Response invoke(Request request, HttpResponseHandler> responseHandler,
ExecutionContext executionContext) {
return invoke(request, responseHandler, executionContext, null, null);
}
/**
* Normal invoke with authentication. Credentials are required and may be overriden at the request level.
**/
private Response invoke(Request request, HttpResponseHandler> responseHandler,
ExecutionContext executionContext, URI cachedEndpoint, URI uriFromEndpointTrait) {
executionContext.setCredentialsProvider(CredentialUtils.getCredentialsProvider(request.getOriginalRequest(), awsCredentialsProvider));
return doInvoke(request, responseHandler, executionContext, cachedEndpoint, uriFromEndpointTrait);
}
/**
* Invoke with no authentication. Credentials are not required and any credentials set on the client or request will
* be ignored for this operation.
**/
private Response anonymousInvoke(Request request,
HttpResponseHandler> responseHandler, ExecutionContext executionContext) {
return doInvoke(request, responseHandler, executionContext, null, null);
}
/**
* Invoke the request using the http client. Assumes credentials (or lack thereof) have been configured in the
* ExecutionContext beforehand.
**/
private Response doInvoke(Request request, HttpResponseHandler> responseHandler,
ExecutionContext executionContext, URI discoveredEndpoint, URI uriFromEndpointTrait) {
if (discoveredEndpoint != null) {
request.setEndpoint(discoveredEndpoint);
request.getOriginalRequest().getRequestClientOptions().appendUserAgent("endpoint-discovery");
} else if (uriFromEndpointTrait != null) {
request.setEndpoint(uriFromEndpointTrait);
} else {
request.setEndpoint(endpoint);
}
request.setTimeOffset(timeOffset);
DefaultErrorResponseHandler errorResponseHandler = new DefaultErrorResponseHandler(exceptionUnmarshallersMap, defaultUnmarshaller);
return client.execute(request, responseHandler, errorResponseHandler, executionContext);
}
@Override
public void shutdown() {
super.shutdown();
}
}