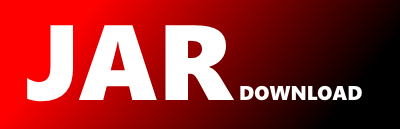
com.amazonaws.services.s3control.model.JobProgressSummary Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of aws-java-sdk-s3control Show documentation
Show all versions of aws-java-sdk-s3control Show documentation
The AWS Java SDK for AWS S3 Control module holds the client classes that are used for communicating with AWS S3 Control Service
/*
* Copyright 2017-2022 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.s3control.model;
import java.io.Serializable;
import javax.annotation.Generated;
/**
*
* Describes the total number of tasks that the specified job has started, the number of tasks that succeeded, and the
* number of tasks that failed.
*
*
* @see AWS API
* Documentation
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class JobProgressSummary implements Serializable, Cloneable {
/** */
private Long totalNumberOfTasks;
/** */
private Long numberOfTasksSucceeded;
/** */
private Long numberOfTasksFailed;
/**
*
* The JobTimers attribute of a job's progress summary.
*
*/
private JobTimers timers;
/**
*
*
* @param totalNumberOfTasks
*/
public void setTotalNumberOfTasks(Long totalNumberOfTasks) {
this.totalNumberOfTasks = totalNumberOfTasks;
}
/**
*
*
* @return
*/
public Long getTotalNumberOfTasks() {
return this.totalNumberOfTasks;
}
/**
*
*
* @param totalNumberOfTasks
* @return Returns a reference to this object so that method calls can be chained together.
*/
public JobProgressSummary withTotalNumberOfTasks(Long totalNumberOfTasks) {
setTotalNumberOfTasks(totalNumberOfTasks);
return this;
}
/**
*
*
* @param numberOfTasksSucceeded
*/
public void setNumberOfTasksSucceeded(Long numberOfTasksSucceeded) {
this.numberOfTasksSucceeded = numberOfTasksSucceeded;
}
/**
*
*
* @return
*/
public Long getNumberOfTasksSucceeded() {
return this.numberOfTasksSucceeded;
}
/**
*
*
* @param numberOfTasksSucceeded
* @return Returns a reference to this object so that method calls can be chained together.
*/
public JobProgressSummary withNumberOfTasksSucceeded(Long numberOfTasksSucceeded) {
setNumberOfTasksSucceeded(numberOfTasksSucceeded);
return this;
}
/**
*
*
* @param numberOfTasksFailed
*/
public void setNumberOfTasksFailed(Long numberOfTasksFailed) {
this.numberOfTasksFailed = numberOfTasksFailed;
}
/**
*
*
* @return
*/
public Long getNumberOfTasksFailed() {
return this.numberOfTasksFailed;
}
/**
*
*
* @param numberOfTasksFailed
* @return Returns a reference to this object so that method calls can be chained together.
*/
public JobProgressSummary withNumberOfTasksFailed(Long numberOfTasksFailed) {
setNumberOfTasksFailed(numberOfTasksFailed);
return this;
}
/**
*
* The JobTimers attribute of a job's progress summary.
*
*
* @param timers
* The JobTimers attribute of a job's progress summary.
*/
public void setTimers(JobTimers timers) {
this.timers = timers;
}
/**
*
* The JobTimers attribute of a job's progress summary.
*
*
* @return The JobTimers attribute of a job's progress summary.
*/
public JobTimers getTimers() {
return this.timers;
}
/**
*
* The JobTimers attribute of a job's progress summary.
*
*
* @param timers
* The JobTimers attribute of a job's progress summary.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public JobProgressSummary withTimers(JobTimers timers) {
setTimers(timers);
return this;
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getTotalNumberOfTasks() != null)
sb.append("TotalNumberOfTasks: ").append(getTotalNumberOfTasks()).append(",");
if (getNumberOfTasksSucceeded() != null)
sb.append("NumberOfTasksSucceeded: ").append(getNumberOfTasksSucceeded()).append(",");
if (getNumberOfTasksFailed() != null)
sb.append("NumberOfTasksFailed: ").append(getNumberOfTasksFailed()).append(",");
if (getTimers() != null)
sb.append("Timers: ").append(getTimers());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof JobProgressSummary == false)
return false;
JobProgressSummary other = (JobProgressSummary) obj;
if (other.getTotalNumberOfTasks() == null ^ this.getTotalNumberOfTasks() == null)
return false;
if (other.getTotalNumberOfTasks() != null && other.getTotalNumberOfTasks().equals(this.getTotalNumberOfTasks()) == false)
return false;
if (other.getNumberOfTasksSucceeded() == null ^ this.getNumberOfTasksSucceeded() == null)
return false;
if (other.getNumberOfTasksSucceeded() != null && other.getNumberOfTasksSucceeded().equals(this.getNumberOfTasksSucceeded()) == false)
return false;
if (other.getNumberOfTasksFailed() == null ^ this.getNumberOfTasksFailed() == null)
return false;
if (other.getNumberOfTasksFailed() != null && other.getNumberOfTasksFailed().equals(this.getNumberOfTasksFailed()) == false)
return false;
if (other.getTimers() == null ^ this.getTimers() == null)
return false;
if (other.getTimers() != null && other.getTimers().equals(this.getTimers()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getTotalNumberOfTasks() == null) ? 0 : getTotalNumberOfTasks().hashCode());
hashCode = prime * hashCode + ((getNumberOfTasksSucceeded() == null) ? 0 : getNumberOfTasksSucceeded().hashCode());
hashCode = prime * hashCode + ((getNumberOfTasksFailed() == null) ? 0 : getNumberOfTasksFailed().hashCode());
hashCode = prime * hashCode + ((getTimers() == null) ? 0 : getTimers().hashCode());
return hashCode;
}
@Override
public JobProgressSummary clone() {
try {
return (JobProgressSummary) super.clone();
} catch (CloneNotSupportedException e) {
throw new IllegalStateException("Got a CloneNotSupportedException from Object.clone() " + "even though we're Cloneable!", e);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy